Modular Programming Functions ICS 3 U Theory Purpose
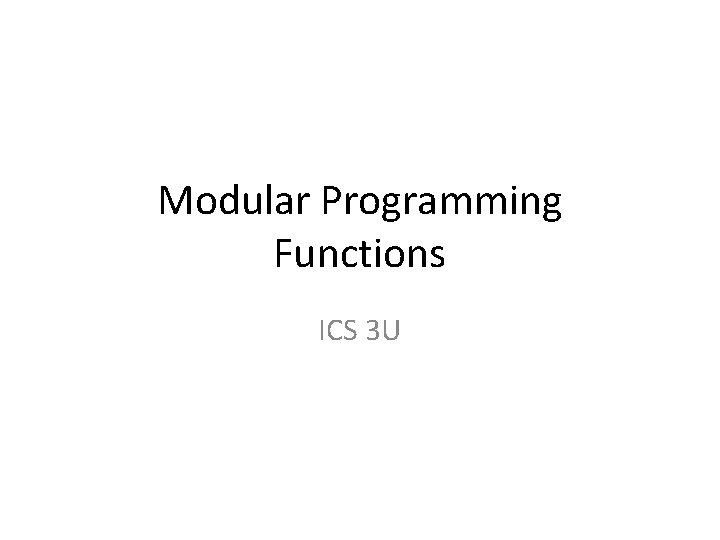
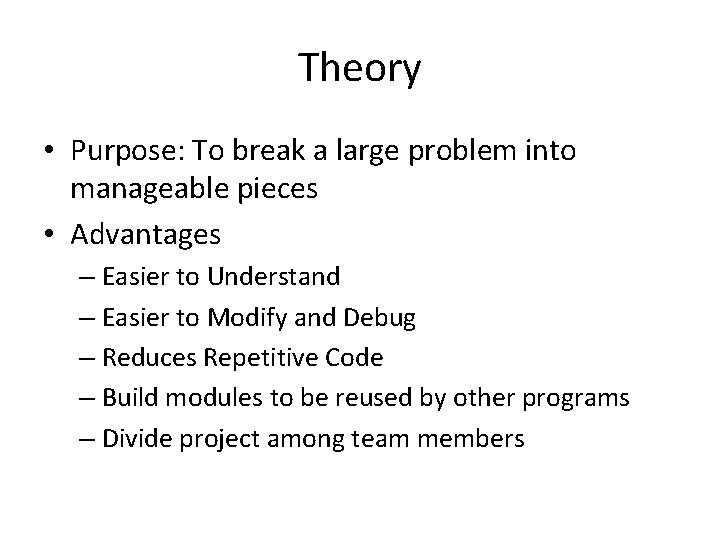
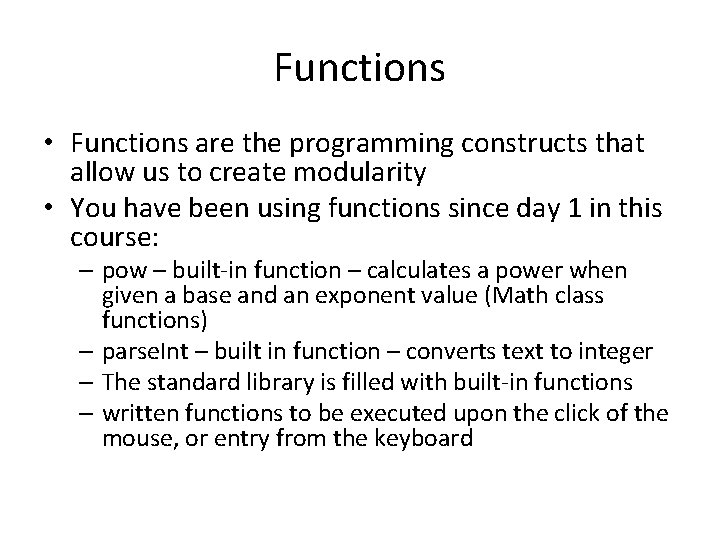
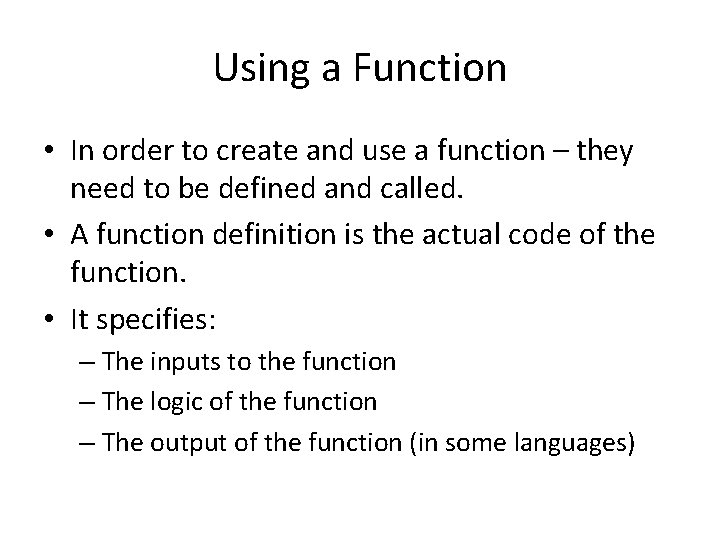
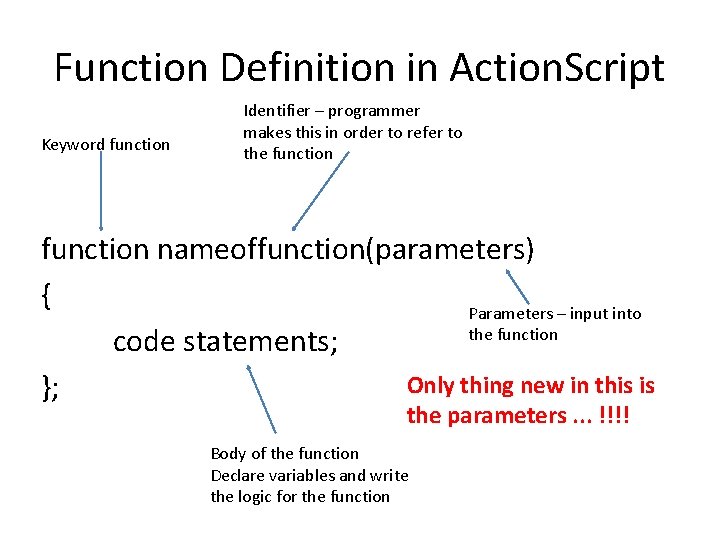
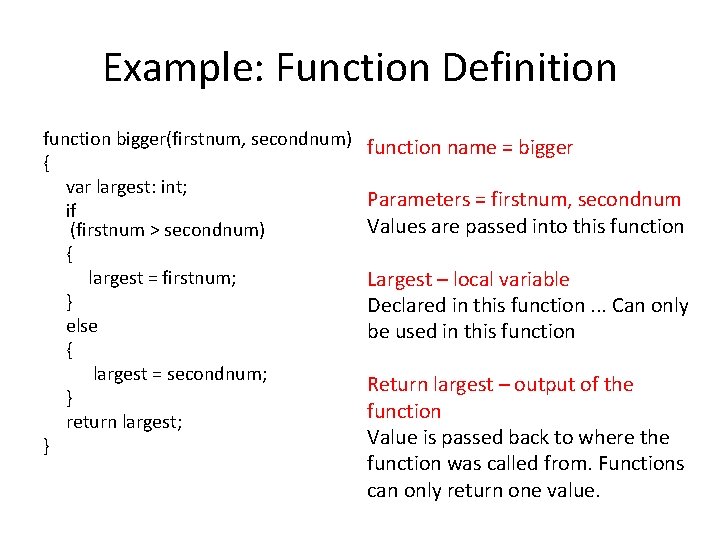
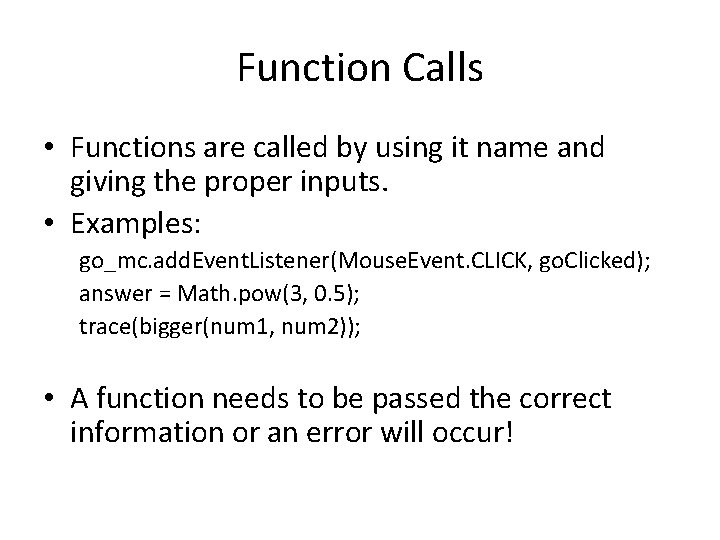
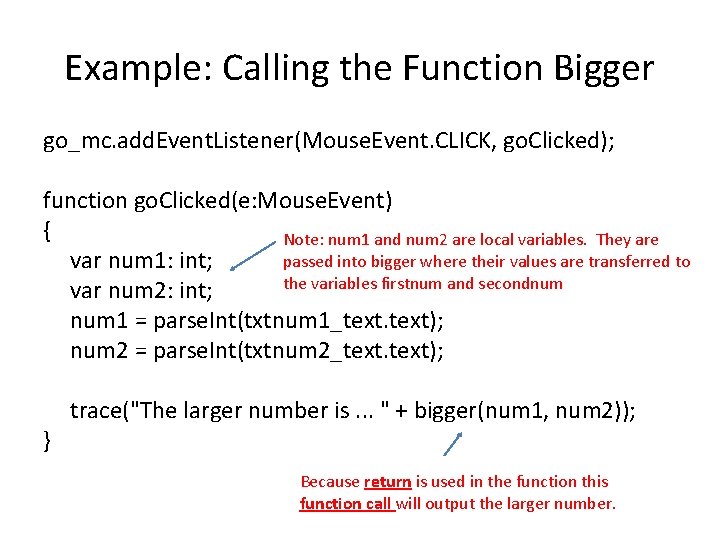
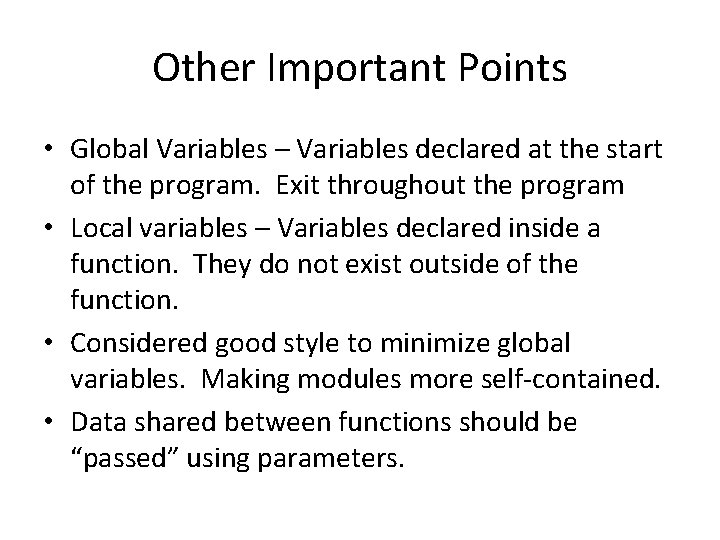
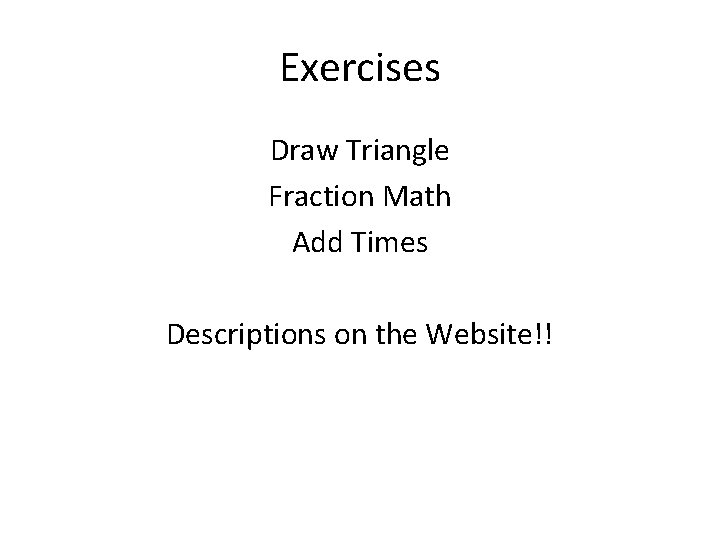
- Slides: 10
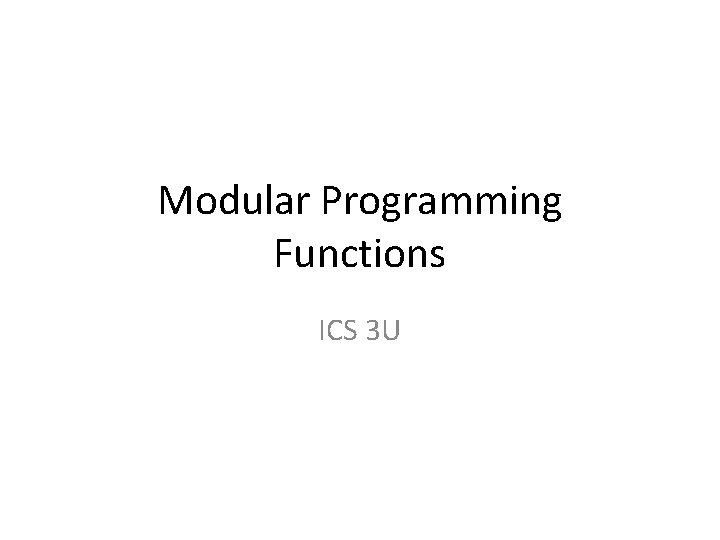
Modular Programming Functions ICS 3 U
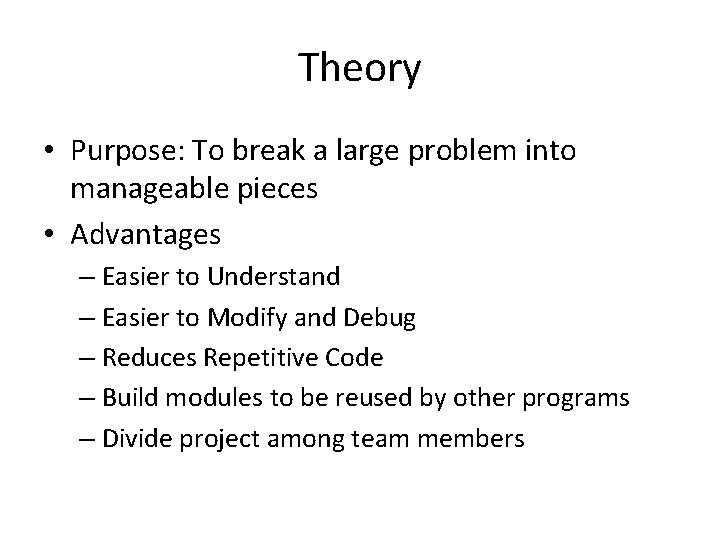
Theory • Purpose: To break a large problem into manageable pieces • Advantages – Easier to Understand – Easier to Modify and Debug – Reduces Repetitive Code – Build modules to be reused by other programs – Divide project among team members
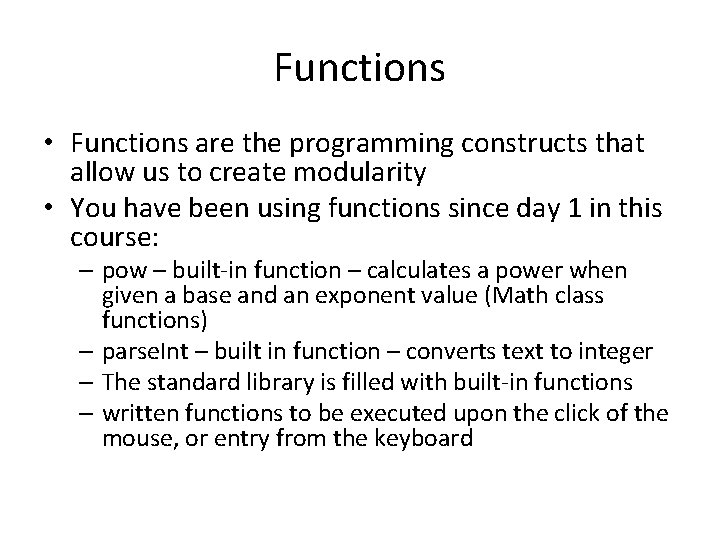
Functions • Functions are the programming constructs that allow us to create modularity • You have been using functions since day 1 in this course: – pow – built-in function – calculates a power when given a base and an exponent value (Math class functions) – parse. Int – built in function – converts text to integer – The standard library is filled with built-in functions – written functions to be executed upon the click of the mouse, or entry from the keyboard
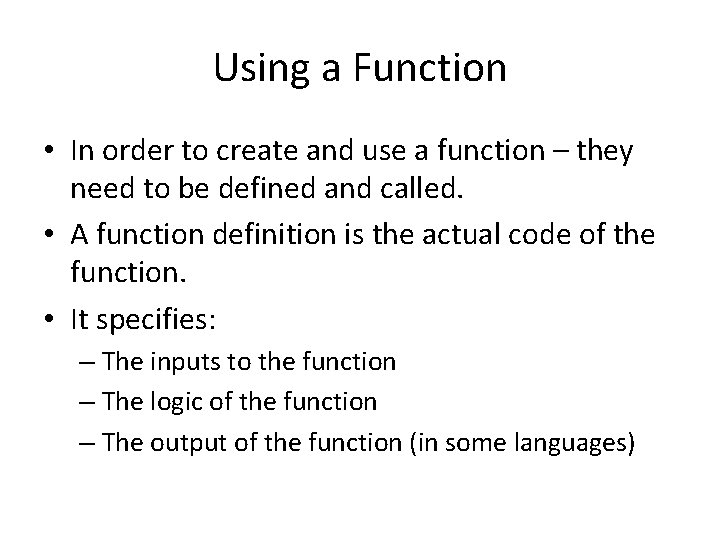
Using a Function • In order to create and use a function – they need to be defined and called. • A function definition is the actual code of the function. • It specifies: – The inputs to the function – The logic of the function – The output of the function (in some languages)
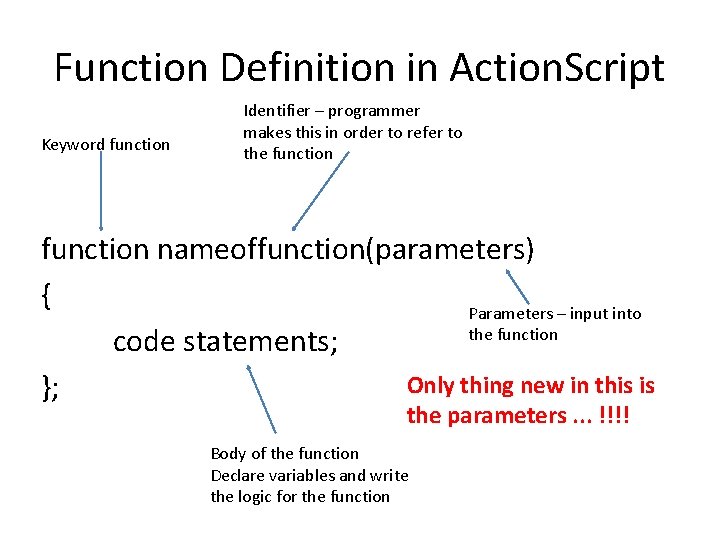
Function Definition in Action. Script Keyword function Identifier – programmer makes this in order to refer to the function nameoffunction(parameters) { Parameters – input into the function code statements; Only thing new in this is }; the parameters. . . !!!! Body of the function Declare variables and write the logic for the function
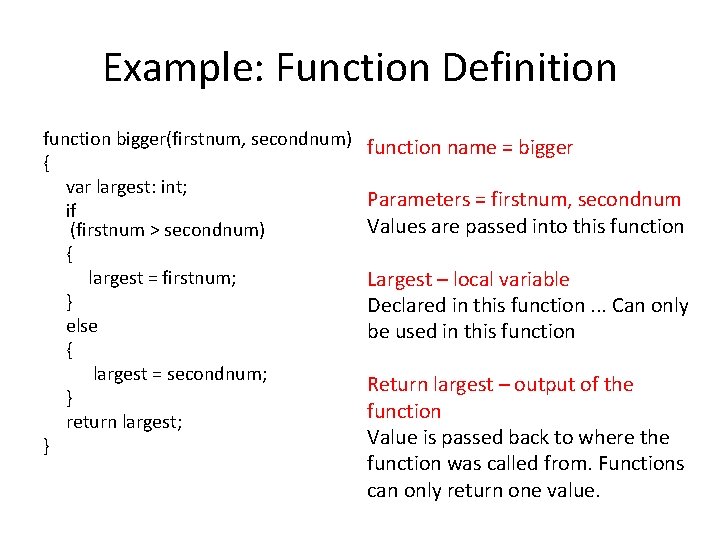
Example: Function Definition function bigger(firstnum, secondnum) { var largest: int; if (firstnum > secondnum) { largest = firstnum; } else { largest = secondnum; } return largest; } function name = bigger Parameters = firstnum, secondnum Values are passed into this function Largest – local variable Declared in this function. . . Can only be used in this function Return largest – output of the function Value is passed back to where the function was called from. Functions can only return one value.
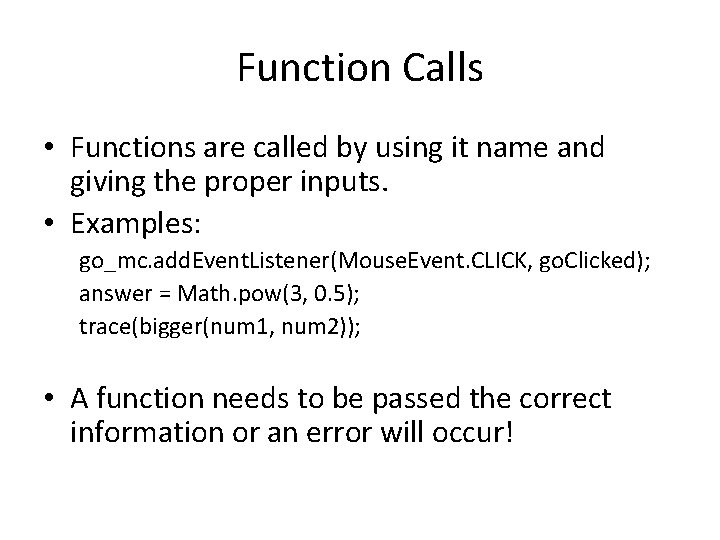
Function Calls • Functions are called by using it name and giving the proper inputs. • Examples: go_mc. add. Event. Listener(Mouse. Event. CLICK, go. Clicked); answer = Math. pow(3, 0. 5); trace(bigger(num 1, num 2)); • A function needs to be passed the correct information or an error will occur!
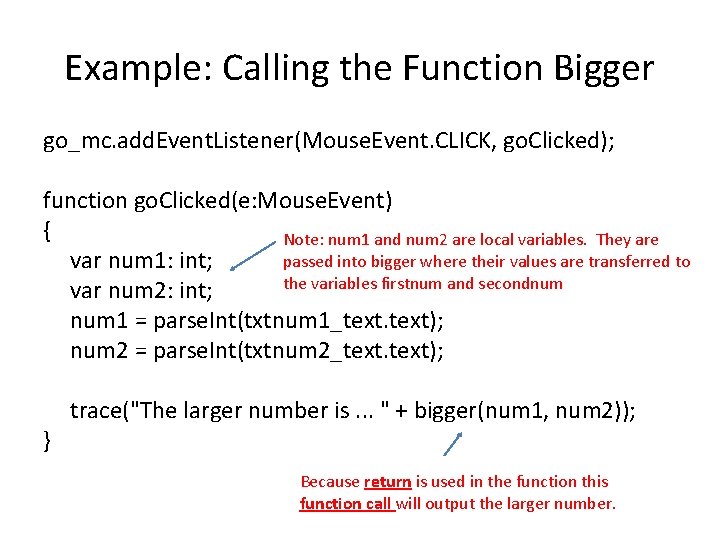
Example: Calling the Function Bigger go_mc. add. Event. Listener(Mouse. Event. CLICK, go. Clicked); function go. Clicked(e: Mouse. Event) { Note: num 1 and num 2 are local variables. They are passed into bigger where their values are transferred to var num 1: int; the variables firstnum and secondnum var num 2: int; num 1 = parse. Int(txtnum 1_text); num 2 = parse. Int(txtnum 2_text); } trace("The larger number is. . . " + bigger(num 1, num 2)); Because return is used in the function this function call will output the larger number.
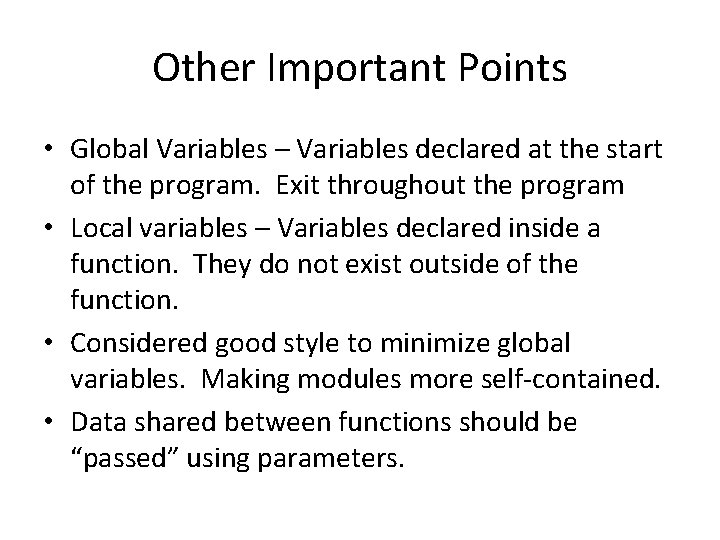
Other Important Points • Global Variables – Variables declared at the start of the program. Exit throughout the program • Local variables – Variables declared inside a function. They do not exist outside of the function. • Considered good style to minimize global variables. Making modules more self-contained. • Data shared between functions should be “passed” using parameters.
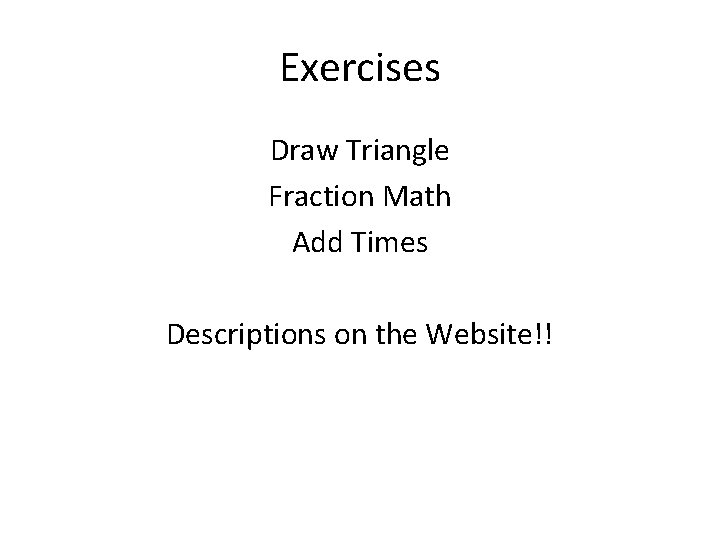
Exercises Draw Triangle Fraction Math Add Times Descriptions on the Website!!
Complex incident
Modular programming
Macros in 8086 microprocessor
Top-down modular design
Objectives of modular software design
Perbedaan linear programming dan integer programming
Greedy vs dynamic
What is system program
Linear vs integer programming
Perbedaan linear programming dan integer programming
Types of functions in programming