Loops and Iteration for Statements while Statements and
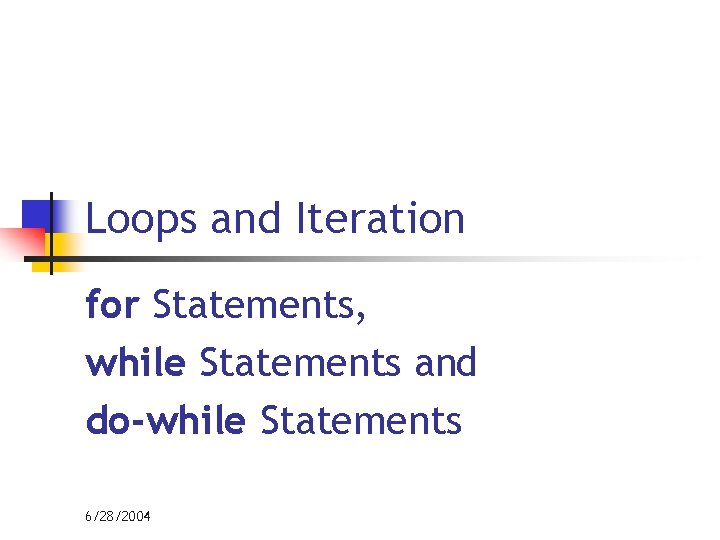
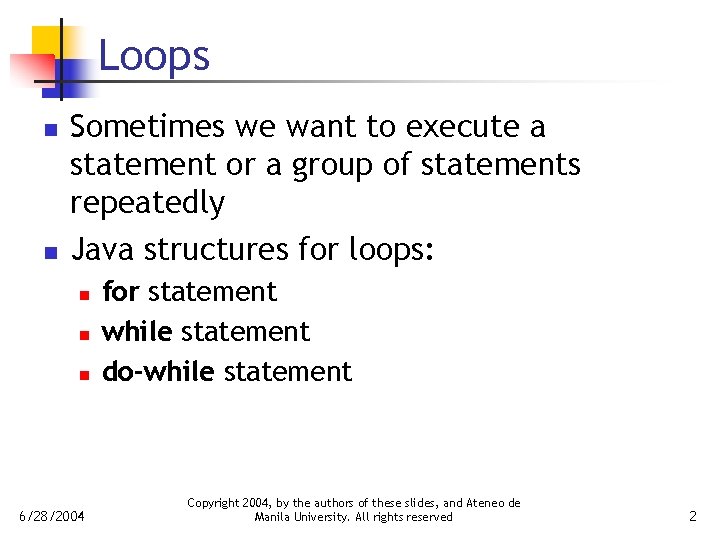
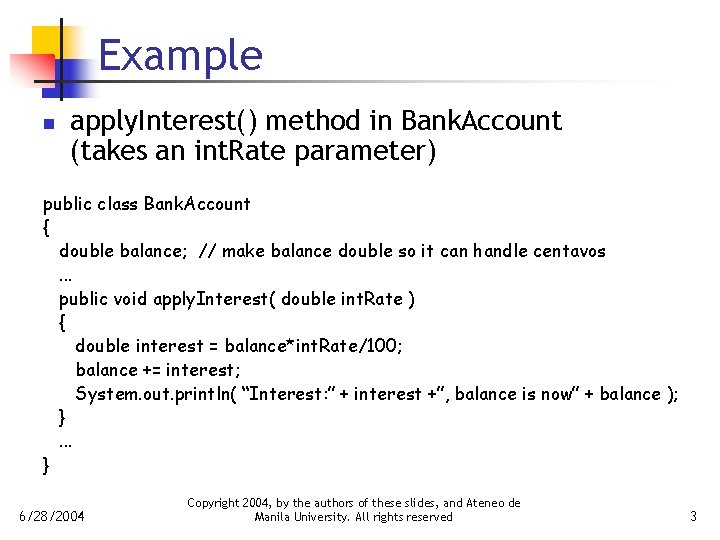
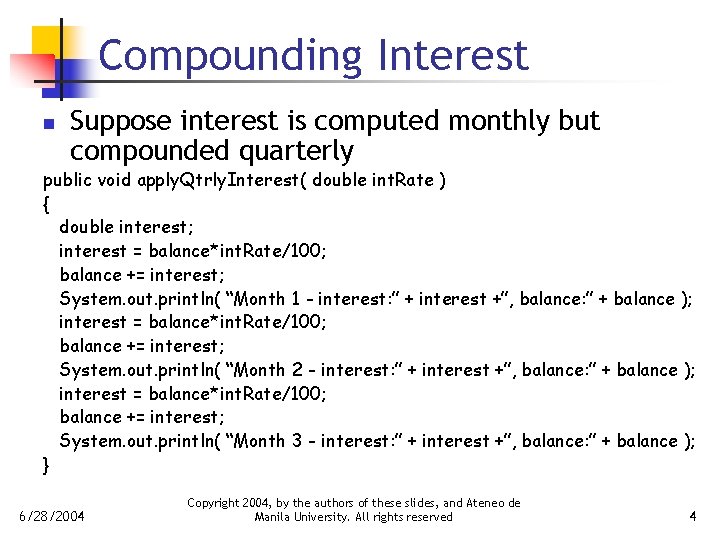
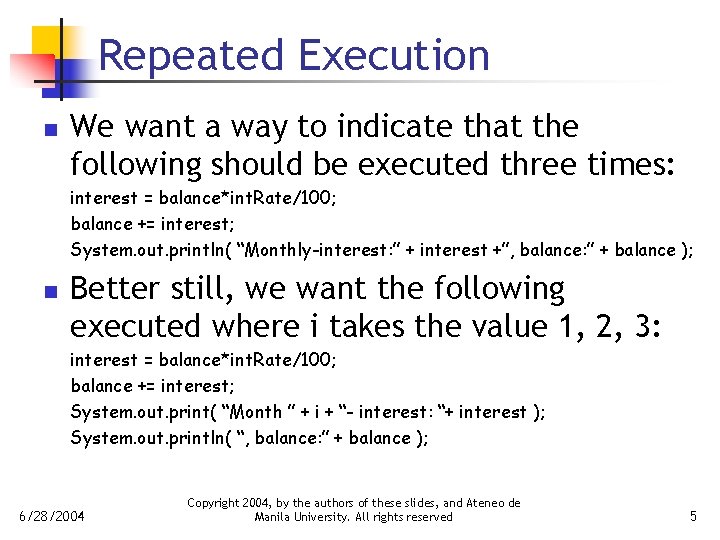
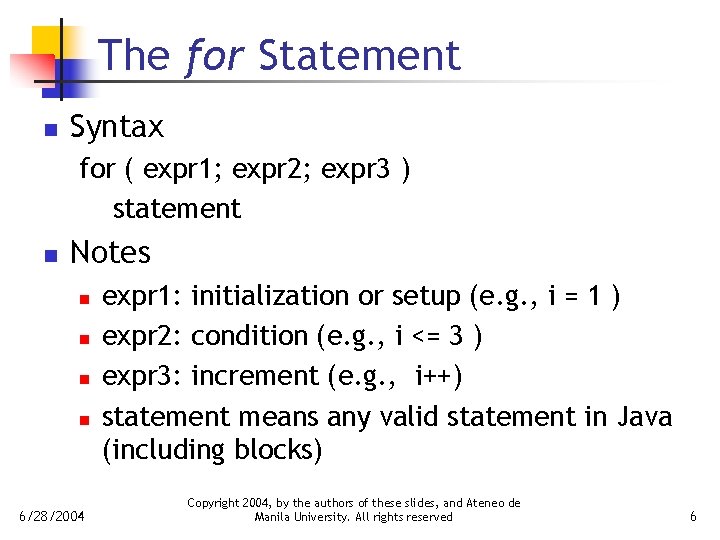
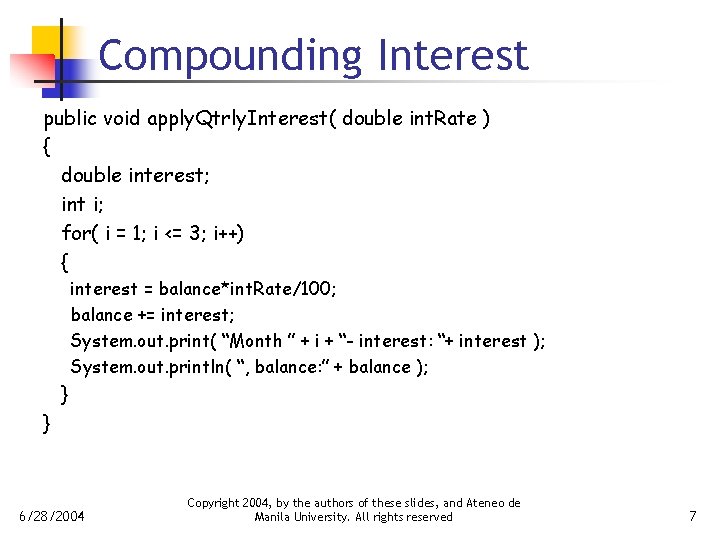
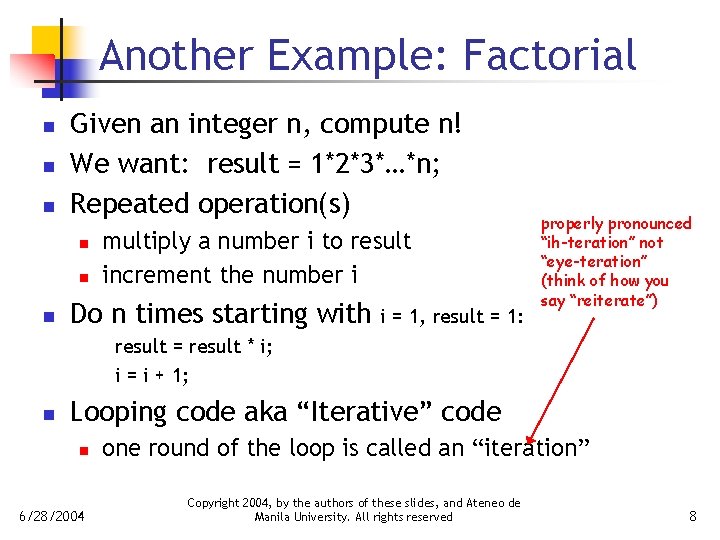
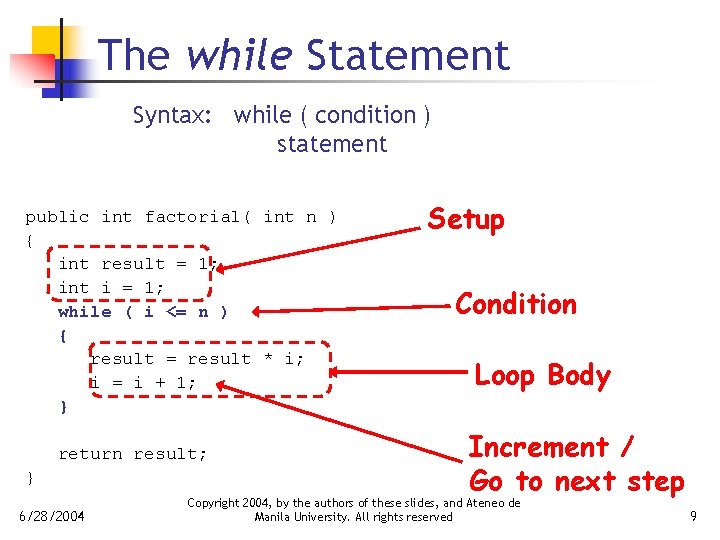
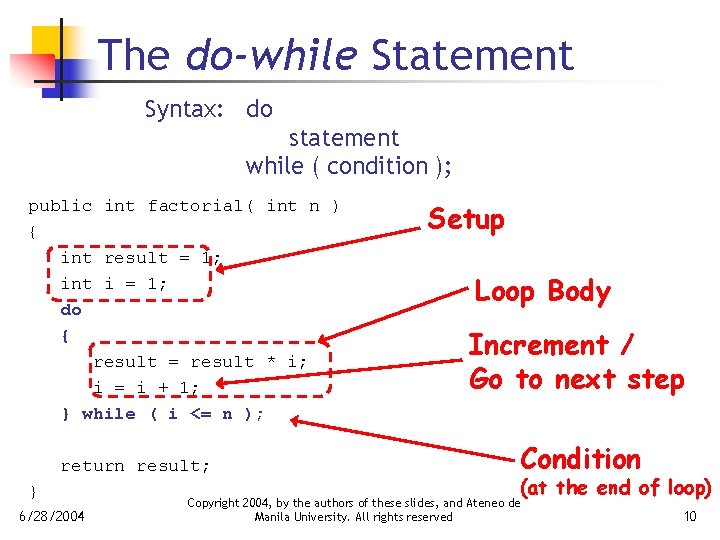
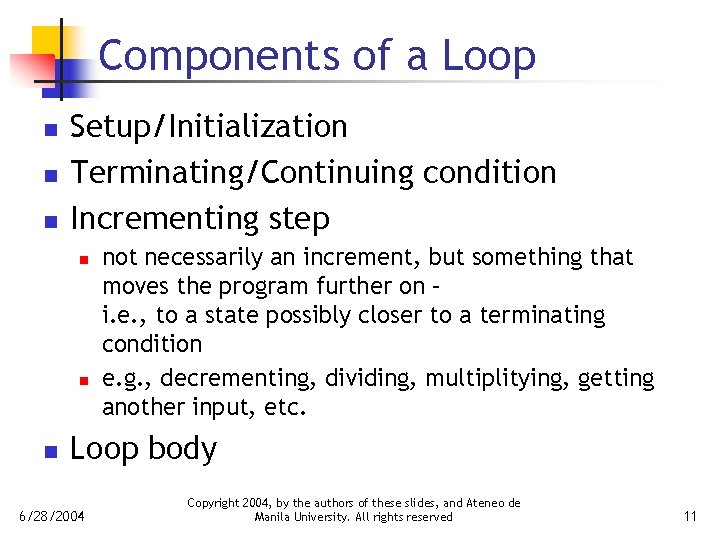
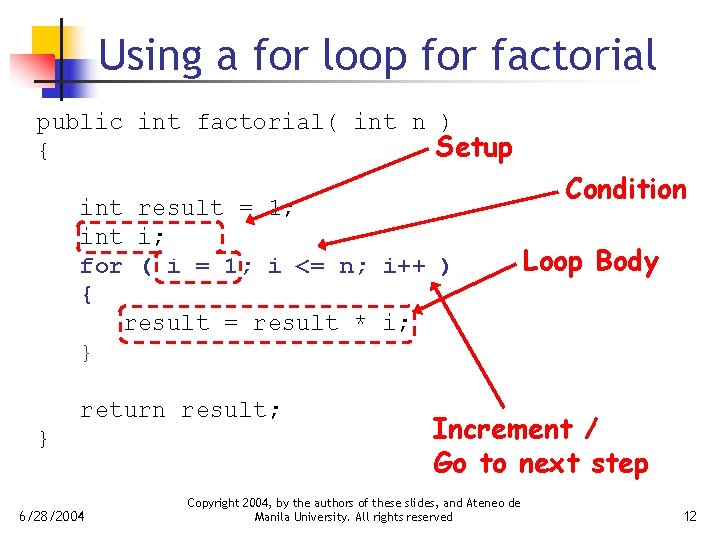
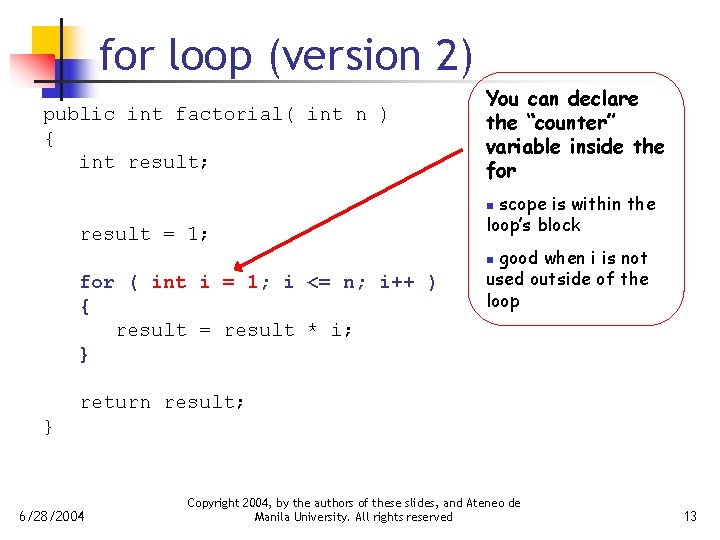
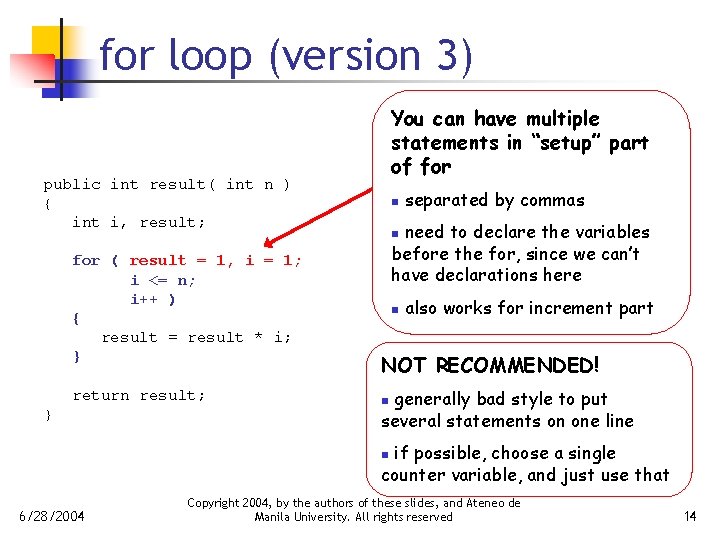
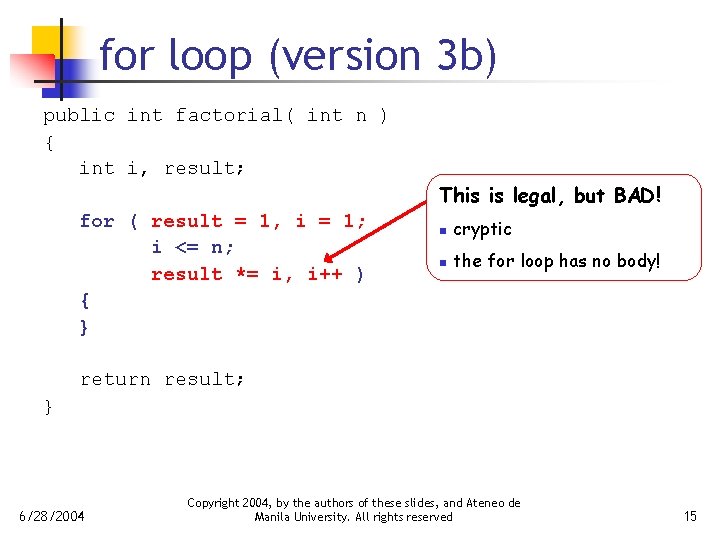
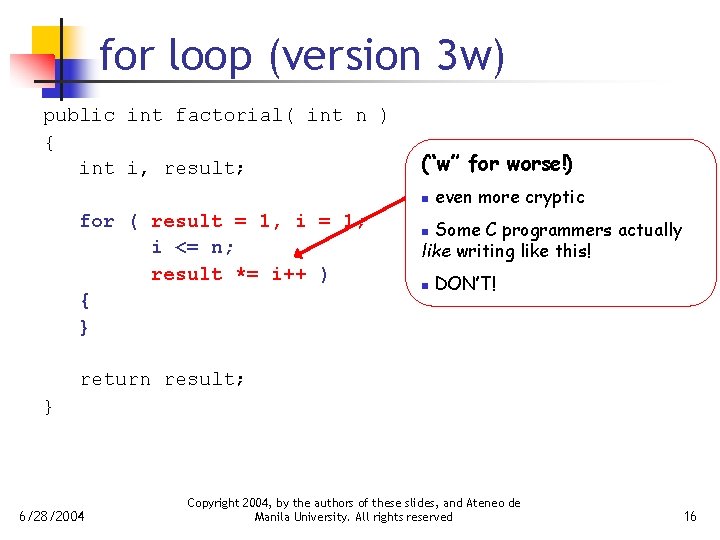
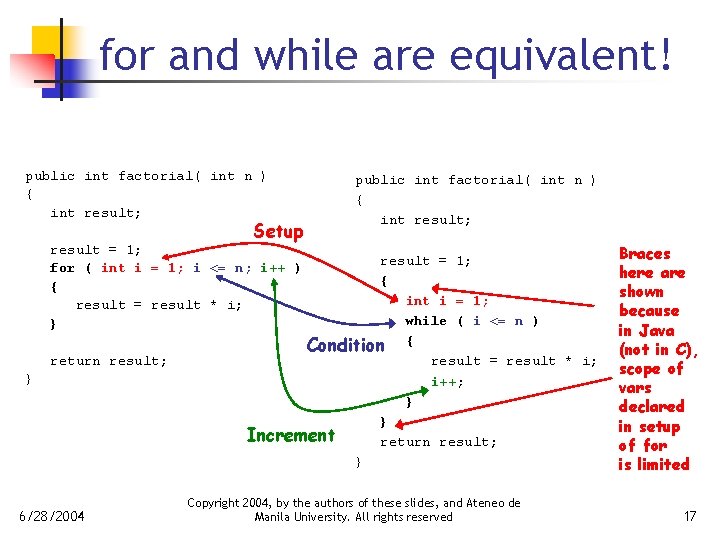
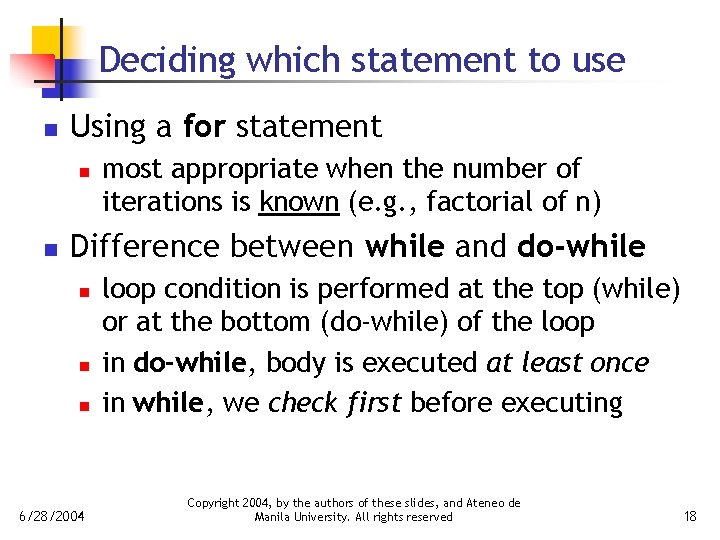
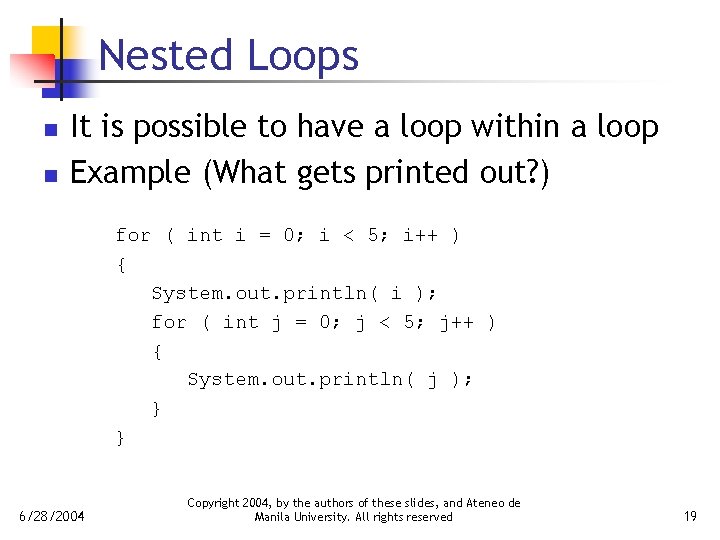
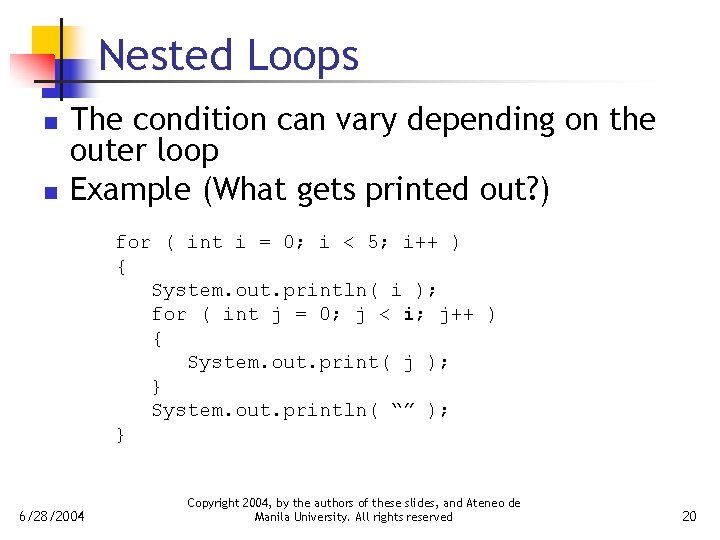
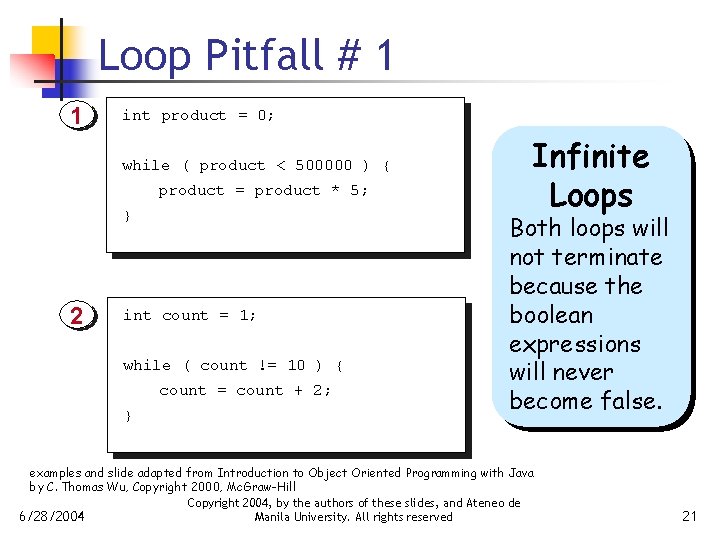
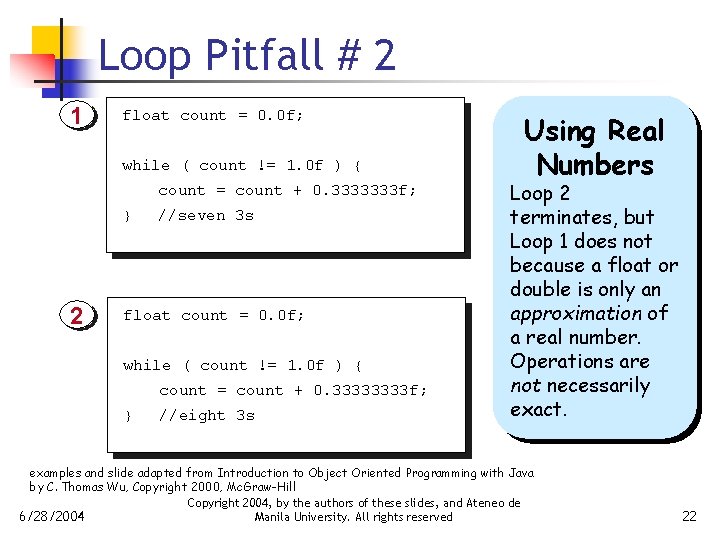
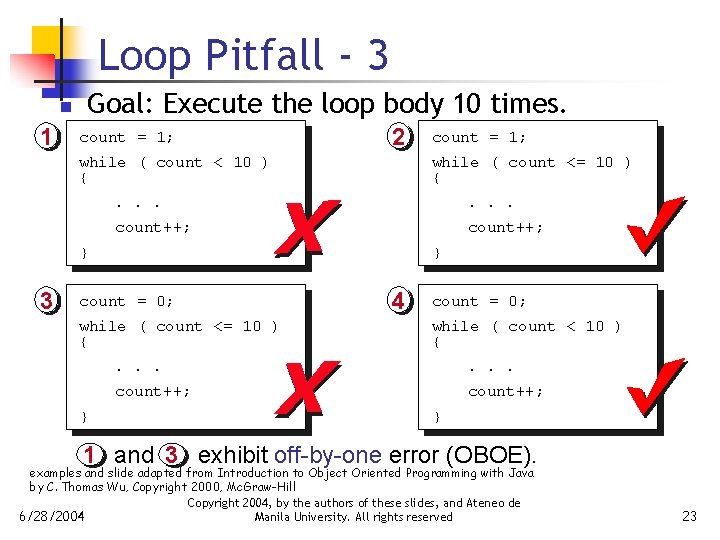
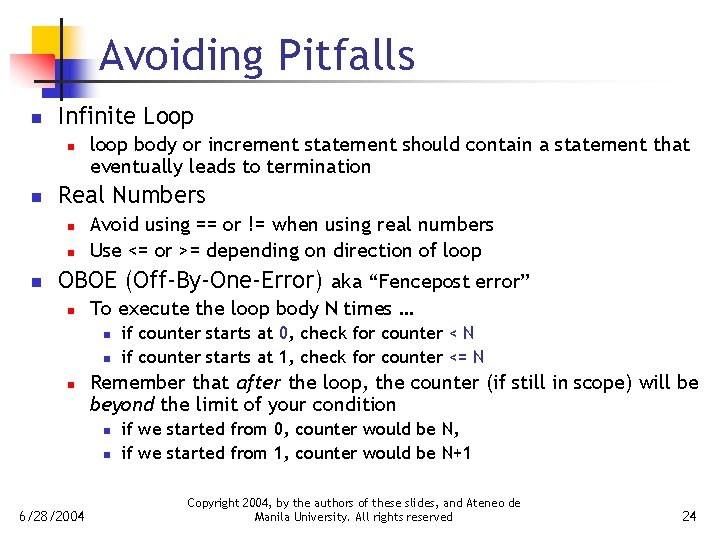
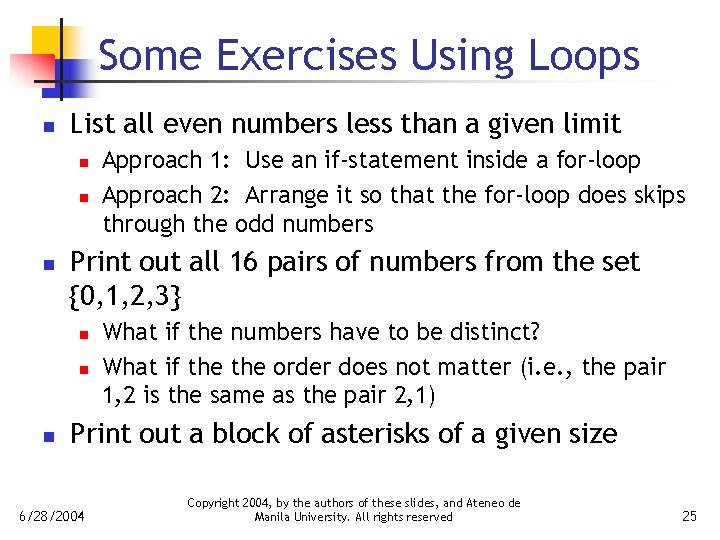
- Slides: 25
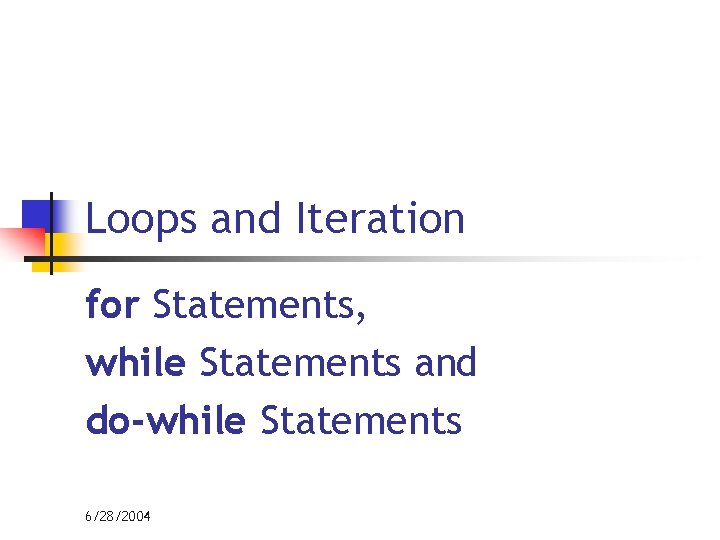
Loops and Iteration for Statements, while Statements and do-while Statements 6/28/2004
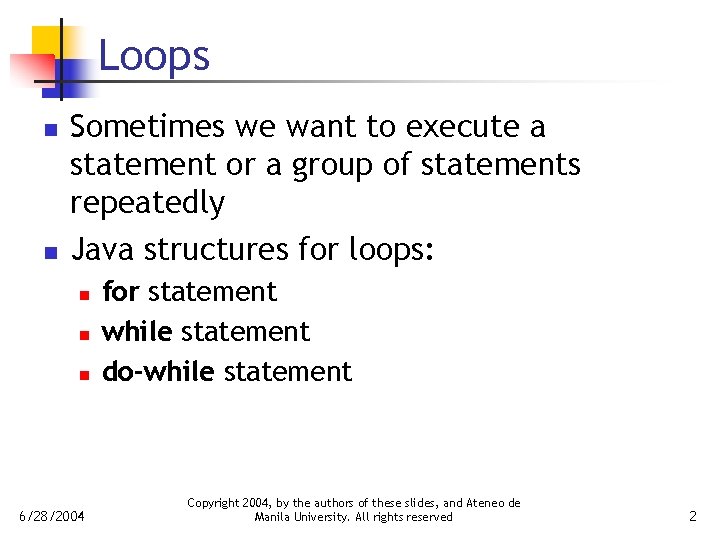
Loops n n Sometimes we want to execute a statement or a group of statements repeatedly Java structures for loops: n n n 6/28/2004 for statement while statement do-while statement Copyright 2004, by the authors of these slides, and Ateneo de Manila University. All rights reserved 2
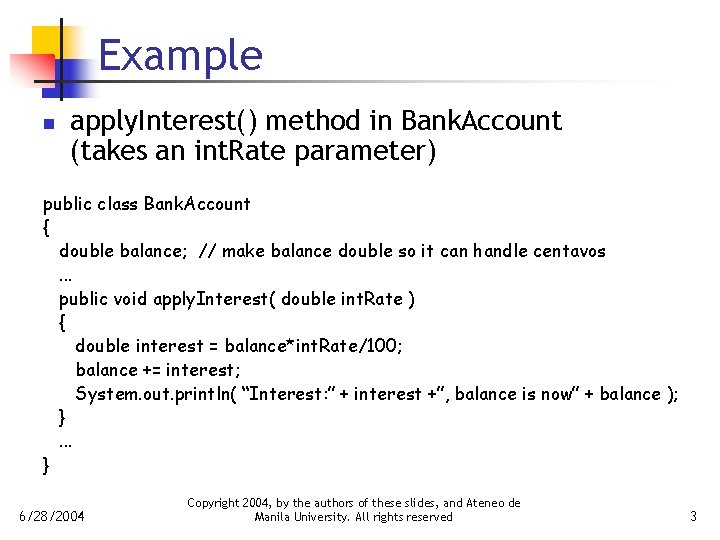
Example n apply. Interest() method in Bank. Account (takes an int. Rate parameter) public class Bank. Account { double balance; // make balance double so it can handle centavos. . . public void apply. Interest( double int. Rate ) { double interest = balance*int. Rate/100; balance += interest; System. out. println( “Interest: ” + interest +”, balance is now” + balance ); }. . . } 6/28/2004 Copyright 2004, by the authors of these slides, and Ateneo de Manila University. All rights reserved 3
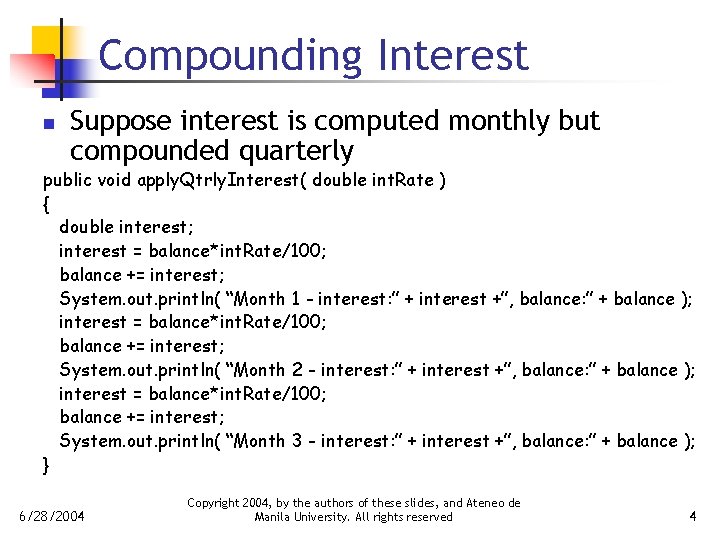
Compounding Interest n Suppose interest is computed monthly but compounded quarterly public void apply. Qtrly. Interest( double int. Rate ) { double interest; interest = balance*int. Rate/100; balance += interest; System. out. println( “Month 1 - interest: ” + interest +”, balance: ” + balance ); interest = balance*int. Rate/100; balance += interest; System. out. println( “Month 2 - interest: ” + interest +”, balance: ” + balance ); interest = balance*int. Rate/100; balance += interest; System. out. println( “Month 3 - interest: ” + interest +”, balance: ” + balance ); } 6/28/2004 Copyright 2004, by the authors of these slides, and Ateneo de Manila University. All rights reserved 4
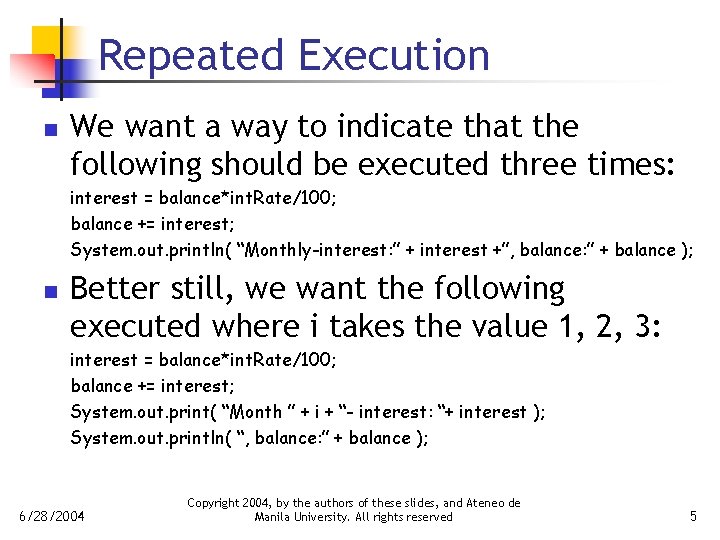
Repeated Execution n We want a way to indicate that the following should be executed three times: interest = balance*int. Rate/100; balance += interest; System. out. println( “Monthly-interest: ” + interest +”, balance: ” + balance ); n Better still, we want the following executed where i takes the value 1, 2, 3: interest = balance*int. Rate/100; balance += interest; System. out. print( “Month ” + i + “- interest: “+ interest ); System. out. println( “, balance: ” + balance ); 6/28/2004 Copyright 2004, by the authors of these slides, and Ateneo de Manila University. All rights reserved 5
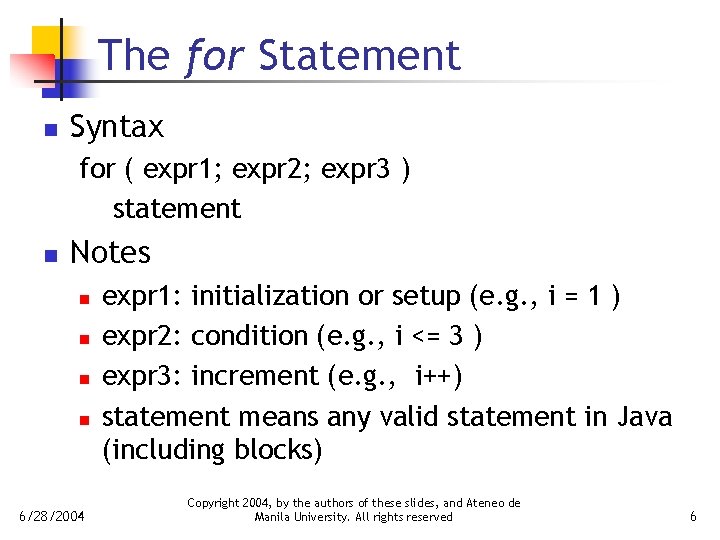
The for Statement n Syntax for ( expr 1; expr 2; expr 3 ) statement n Notes n n 6/28/2004 expr 1: initialization or setup (e. g. , i = 1 ) expr 2: condition (e. g. , i <= 3 ) expr 3: increment (e. g. , i++) statement means any valid statement in Java (including blocks) Copyright 2004, by the authors of these slides, and Ateneo de Manila University. All rights reserved 6
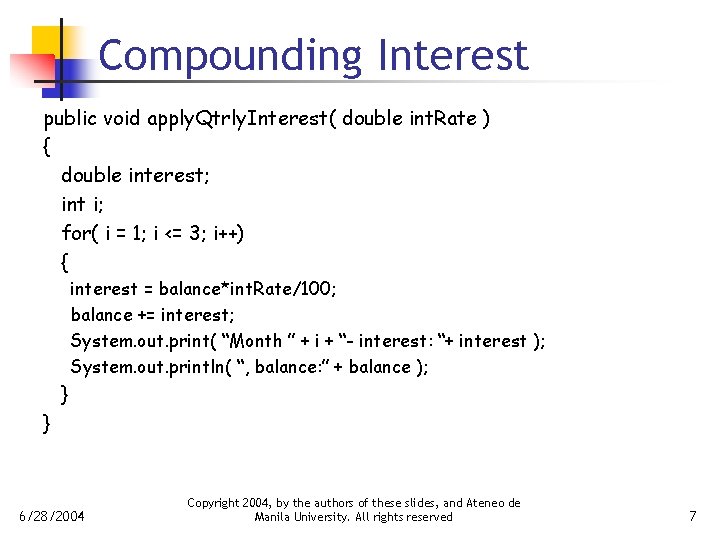
Compounding Interest public void apply. Qtrly. Interest( double int. Rate ) { double interest; int i; for( i = 1; i <= 3; i++) { interest = balance*int. Rate/100; balance += interest; System. out. print( “Month ” + i + “- interest: “+ interest ); System. out. println( “, balance: ” + balance ); } } 6/28/2004 Copyright 2004, by the authors of these slides, and Ateneo de Manila University. All rights reserved 7
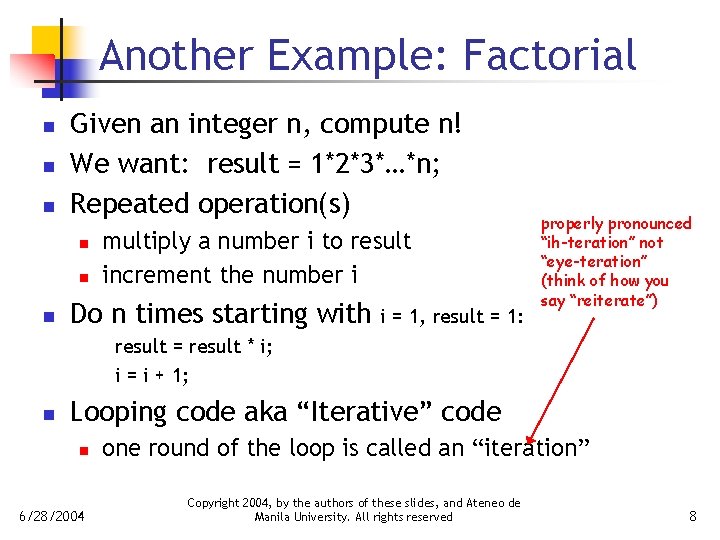
Another Example: Factorial n n n Given an integer n, compute n! We want: result = 1*2*3*…*n; Repeated operation(s) n n n multiply a number i to result increment the number i Do n times starting with i = 1, result = 1: properly pronounced “ih-teration” not “eye-teration” (think of how you say “reiterate”) result = result * i; i = i + 1; n Looping code aka “Iterative” code n 6/28/2004 one round of the loop is called an “iteration” Copyright 2004, by the authors of these slides, and Ateneo de Manila University. All rights reserved 8
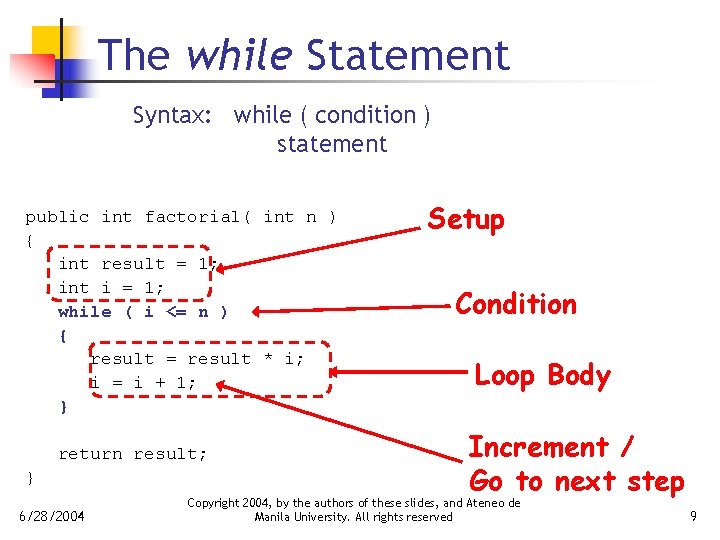
The while Statement Syntax: while ( condition ) statement public int factorial( int n ) { int result = 1; int i = 1; while ( i <= n ) { result = result * i; i = i + 1; } return result; } 6/28/2004 Setup Condition Loop Body Increment / Go to next step Copyright 2004, by the authors of these slides, and Ateneo de Manila University. All rights reserved 9
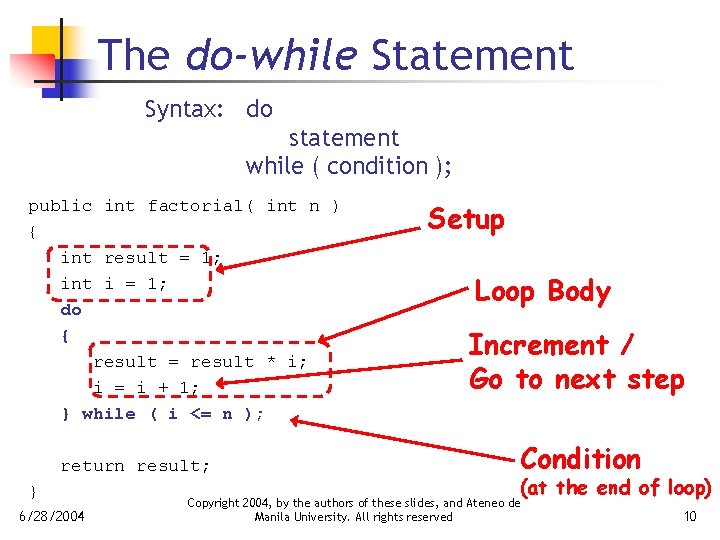
The do-while Statement Syntax: do statement while ( condition ); public int factorial( int n ) { int result = 1; int i = 1; do { result = result * i; i = i + 1; } while ( i <= n ); return result; } 6/28/2004 Setup Loop Body Increment / Go to next step Condition (at the end of loop) Copyright 2004, by the authors of these slides, and Ateneo de Manila University. All rights reserved 10
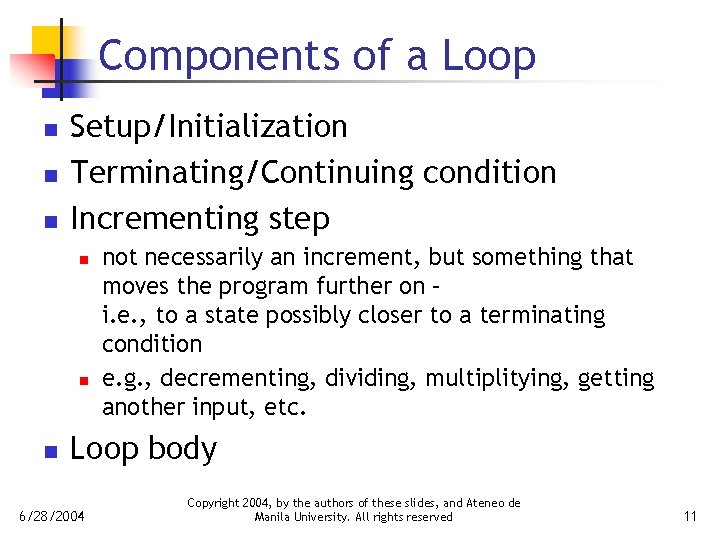
Components of a Loop n n n Setup/Initialization Terminating/Continuing condition Incrementing step n not necessarily an increment, but something that moves the program further on – i. e. , to a state possibly closer to a terminating condition e. g. , decrementing, dividing, multiplitying, getting another input, etc. Loop body 6/28/2004 Copyright 2004, by the authors of these slides, and Ateneo de Manila University. All rights reserved 11
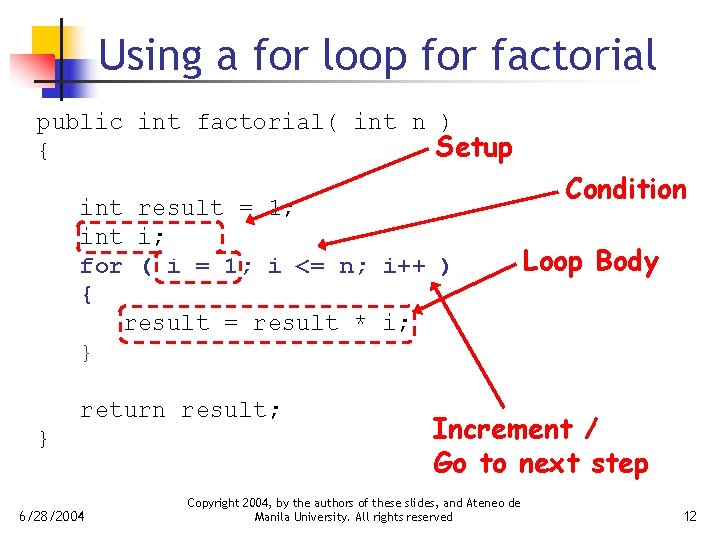
Using a for loop for factorial public int factorial( int n ) Setup { int result = 1; int i; for ( i = 1; i <= n; i++ ) { result = result * i; } return result; } 6/28/2004 Condition Loop Body Increment / Go to next step Copyright 2004, by the authors of these slides, and Ateneo de Manila University. All rights reserved 12
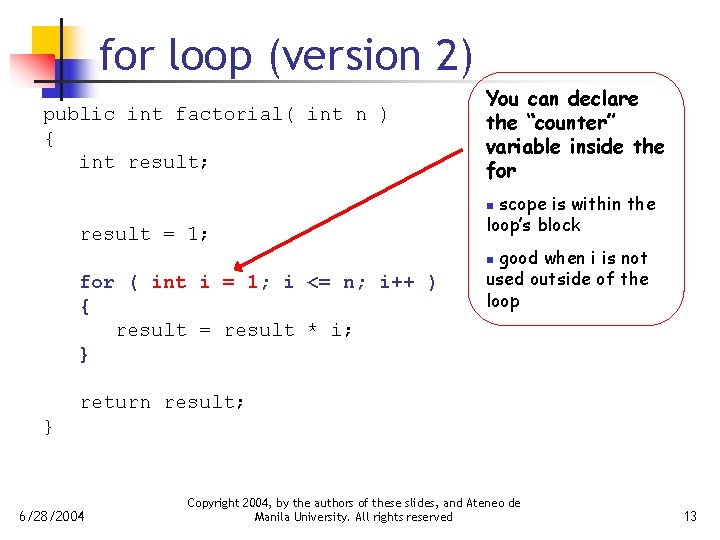
for loop (version 2) public int factorial( int n ) { int result; You can declare the “counter” variable inside the for scope is within the loop’s block n result = 1; good when i is not used outside of the loop n for ( int i = 1; i <= n; i++ ) { result = result * i; } return result; } 6/28/2004 Copyright 2004, by the authors of these slides, and Ateneo de Manila University. All rights reserved 13
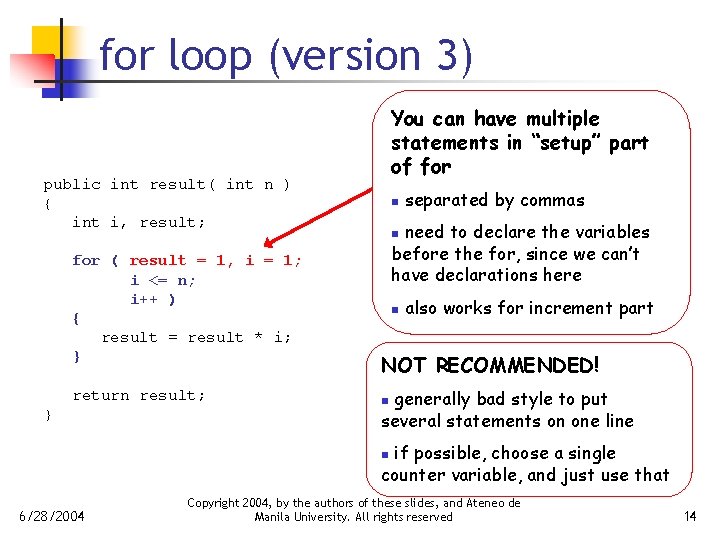
for loop (version 3) You can have multiple statements in “setup” part of for public int result( int n ) { int i, result; for ( result = 1, i = 1; i <= n; i++ ) { result = result * i; } return result; } n separated by commas need to declare the variables before the for, since we can’t have declarations here n n also works for increment part NOT RECOMMENDED! generally bad style to put several statements on one line n if possible, choose a single counter variable, and just use that n 6/28/2004 Copyright 2004, by the authors of these slides, and Ateneo de Manila University. All rights reserved 14
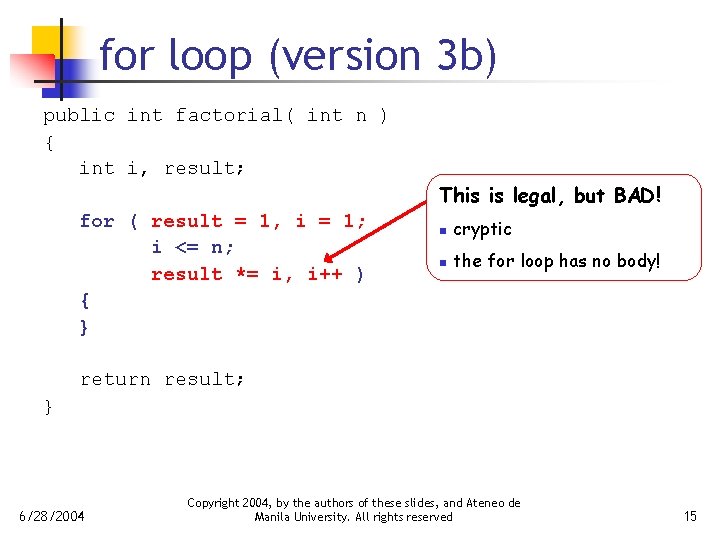
for loop (version 3 b) public int factorial( int n ) { int i, result; for ( result = 1, i = 1; i <= n; result *= i, i++ ) { } This is legal, but BAD! n cryptic n the for loop has no body! return result; } 6/28/2004 Copyright 2004, by the authors of these slides, and Ateneo de Manila University. All rights reserved 15
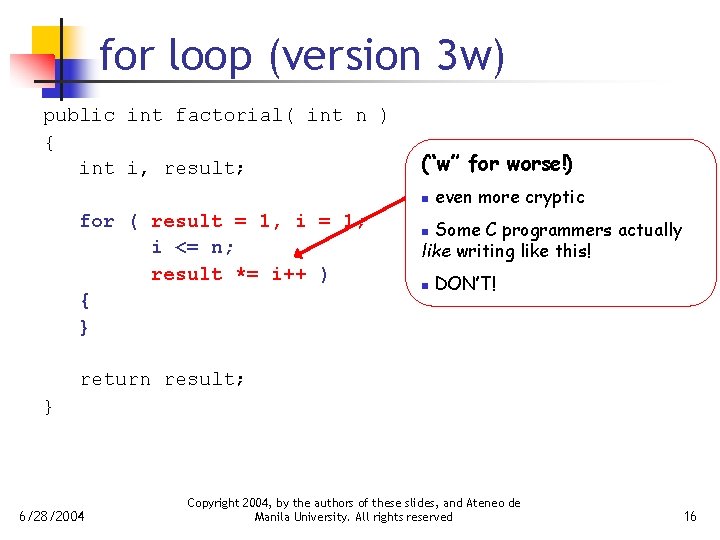
for loop (version 3 w) public int factorial( int n ) { int i, result; (“w” for worse!) n for ( result = 1, i = 1; i <= n; result *= i++ ) { } even more cryptic Some C programmers actually like writing like this! n n DON’T! return result; } 6/28/2004 Copyright 2004, by the authors of these slides, and Ateneo de Manila University. All rights reserved 16
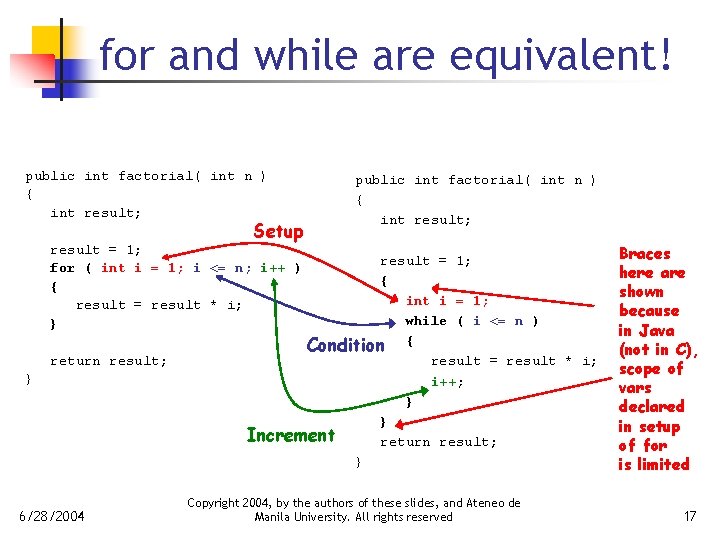
for and while are equivalent! public int factorial( int n ) { int result; Setup public int factorial( int n ) { int result; result = 1; for ( int i = 1; i <= n; i++ ) { result = result * i; } return result; } 6/28/2004 result = 1; { int i = 1; while ( i <= n ) Condition { result = result * i; i++; } } Increment return result; } Copyright 2004, by the authors of these slides, and Ateneo de Manila University. All rights reserved Braces here are shown because in Java (not in C), scope of vars declared in setup of for is limited 17
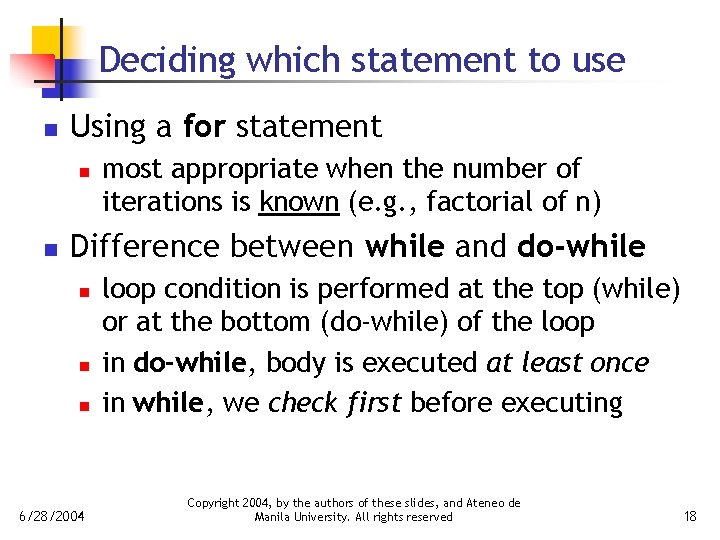
Deciding which statement to use n Using a for statement n n most appropriate when the number of iterations is known (e. g. , factorial of n) Difference between while and do-while n n n 6/28/2004 loop condition is performed at the top (while) or at the bottom (do-while) of the loop in do-while, body is executed at least once in while, we check first before executing Copyright 2004, by the authors of these slides, and Ateneo de Manila University. All rights reserved 18
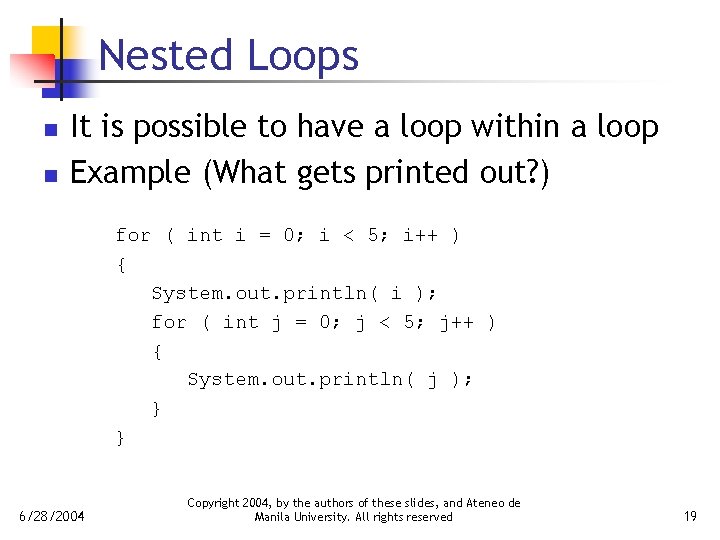
Nested Loops n n It is possible to have a loop within a loop Example (What gets printed out? ) for ( int i = 0; i < 5; i++ ) { System. out. println( i ); for ( int j = 0; j < 5; j++ ) { System. out. println( j ); } } 6/28/2004 Copyright 2004, by the authors of these slides, and Ateneo de Manila University. All rights reserved 19
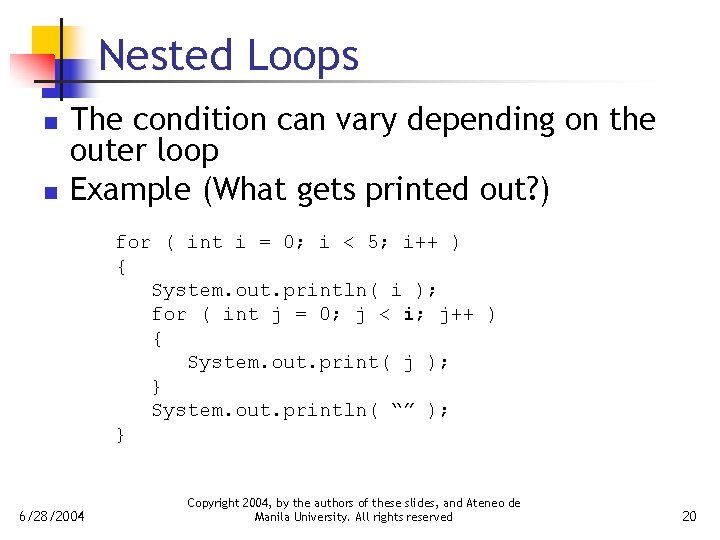
Nested Loops n n The condition can vary depending on the outer loop Example (What gets printed out? ) for ( int i = 0; i < 5; i++ ) { System. out. println( i ); for ( int j = 0; j < i; j++ ) { System. out. print( j ); } System. out. println( “” ); } 6/28/2004 Copyright 2004, by the authors of these slides, and Ateneo de Manila University. All rights reserved 20
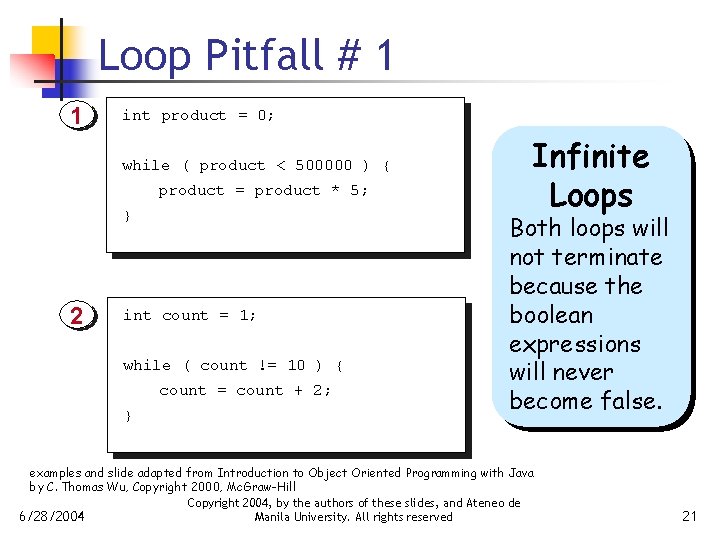
Loop Pitfall # 1 1 int product = 0; while ( product < 500000 ) { product = product * 5; } 2 int count = 1; while ( count != 10 ) { count = count + 2; } Infinite Loops Both loops will not terminate because the boolean expressions will never become false. examples and slide adapted from Introduction to Object Oriented Programming with Java by C. Thomas Wu, Copyright 2000, Mc. Graw-Hill Copyright 2004, by the authors of these slides, and Ateneo de 6/28/2004 Manila University. All rights reserved 21
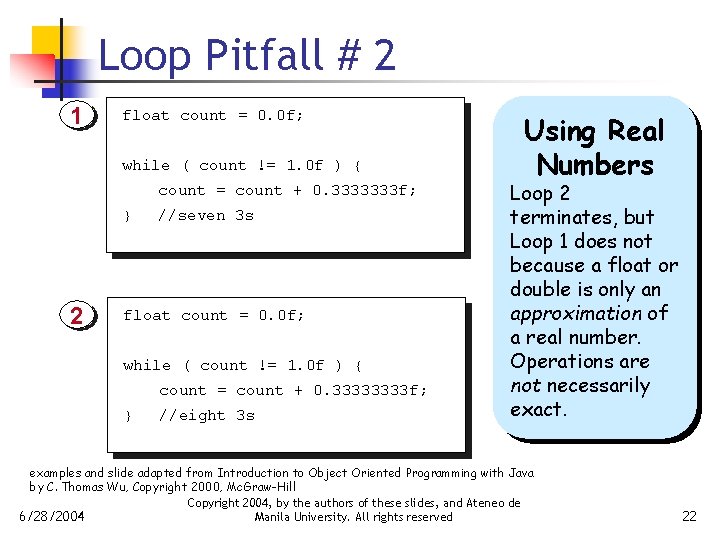
Loop Pitfall # 2 1 float count = 0. 0 f; while ( count != 1. 0 f ) { count = count + 0. 3333333 f; } 2 //seven 3 s float count = 0. 0 f; while ( count != 1. 0 f ) { count = count + 0. 3333 f; } //eight 3 s Using Real Numbers Loop 2 terminates, but Loop 1 does not because a float or double is only an approximation of a real number. Operations are not necessarily exact. examples and slide adapted from Introduction to Object Oriented Programming with Java by C. Thomas Wu, Copyright 2000, Mc. Graw-Hill Copyright 2004, by the authors of these slides, and Ateneo de 6/28/2004 Manila University. All rights reserved 22
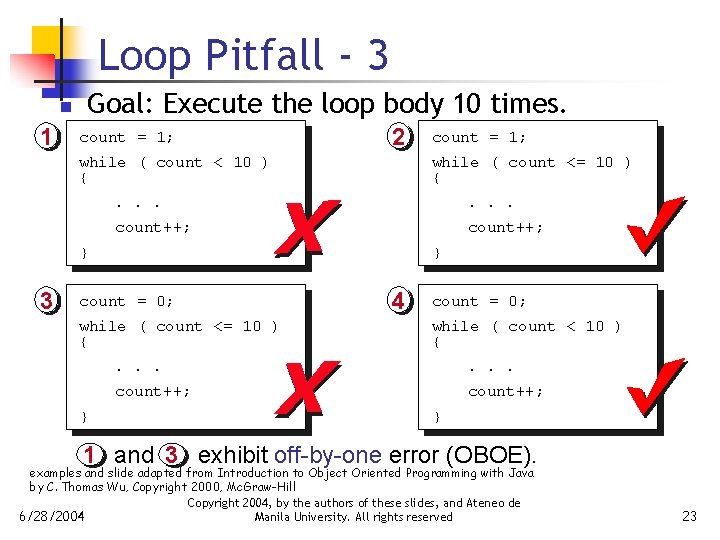
Loop Pitfall - 3 n 1 Goal: Execute the loop body 10 times. count = 1; 2 while ( count < 10 ) { while ( count <= 10 ) { . . . count++; } 3 } count = 0; while ( count <= 10 ) { } count = 1; 4 count = 0; while ( count < 10 ) { . . . count++; } 1 and 3 exhibit off-by-one error (OBOE). examples and slide adapted from Introduction to Object Oriented Programming with Java by C. Thomas Wu, Copyright 2000, Mc. Graw-Hill Copyright 2004, by the authors of these slides, and Ateneo de 6/28/2004 Manila University. All rights reserved 23
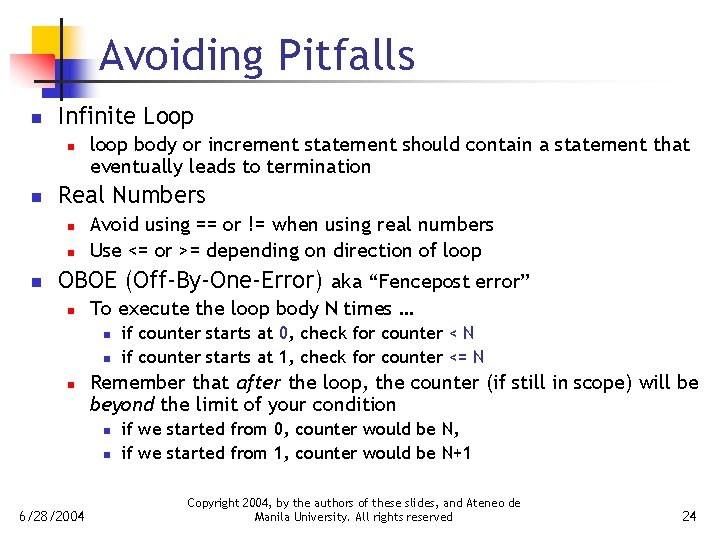
Avoiding Pitfalls n Infinite Loop n n Real Numbers n n n loop body or increment statement should contain a statement that eventually leads to termination Avoid using == or != when using real numbers Use <= or >= depending on direction of loop OBOE (Off-By-One-Error) aka “Fencepost error” n To execute the loop body N times … n n n Remember that after the loop, the counter (if still in scope) will be beyond the limit of your condition n n 6/28/2004 if counter starts at 0, check for counter < N if counter starts at 1, check for counter <= N if we started from 0, counter would be N, if we started from 1, counter would be N+1 Copyright 2004, by the authors of these slides, and Ateneo de Manila University. All rights reserved 24
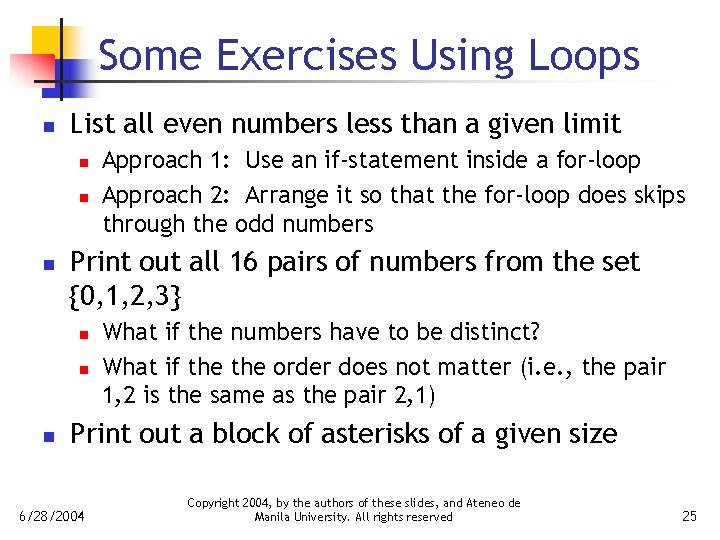
Some Exercises Using Loops n List all even numbers less than a given limit n n n Print out all 16 pairs of numbers from the set {0, 1, 2, 3} n n n Approach 1: Use an if-statement inside a for-loop Approach 2: Arrange it so that the for-loop does skips through the odd numbers What if the numbers have to be distinct? What if the order does not matter (i. e. , the pair 1, 2 is the same as the pair 2, 1) Print out a block of asterisks of a given size 6/28/2004 Copyright 2004, by the authors of these slides, and Ateneo de Manila University. All rights reserved 25
While loops and if-else structures
While loops in matlab
Break matlab
Iteration statements in java
Perbedaan for while do while
Branches electrical circuit
Small basic turtle commands
Flow volume loop obstructive
Reddish loops of gas
Types of loops in matlab
Lesson 13 bee nested loops
Nested loops python
Geothermal trench design
Matlab for loop example
Cakewalk loop construction
Intussusception barium enema claw sign
Adobe audition loops
Nested loops python
Sentinel loops python
Nested if pseudocode
Is nested loops bad
Hirschsprung disease
Reddish loops of gas that link parts of sunspot regions
Dynamic intestinal obstruction
Non touching loops in control system
Control roadmap