Logic Programming OLO G Dr Yasser Nada Fall
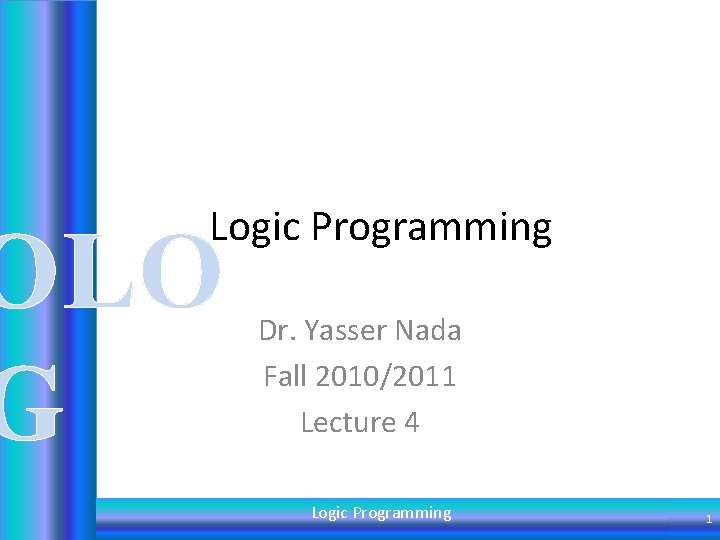
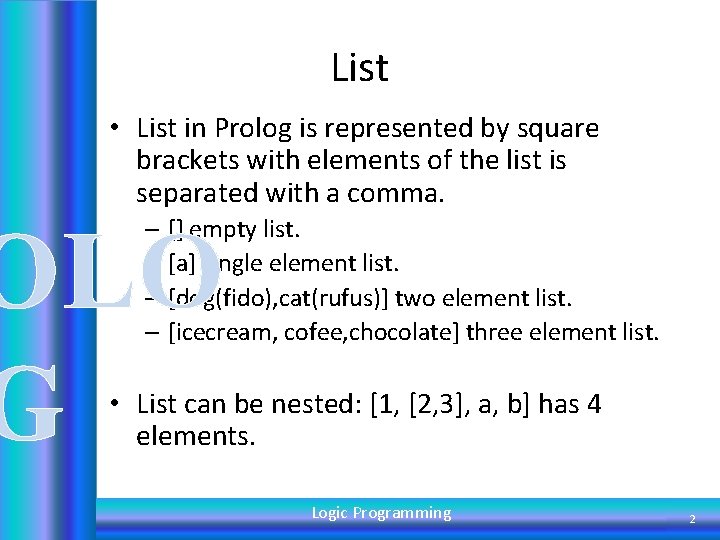
![List • List also is represented as Head and Tail. – [H|T]=[a, b, c] List • List also is represented as Head and Tail. – [H|T]=[a, b, c]](https://slidetodoc.com/presentation_image/c1ea1fda97a0155e7beb2bfbc48146ec/image-3.jpg)
![List Construction • Assume X=[r, e, d]. • To add an element to the List Construction • Assume X=[r, e, d]. • To add an element to the](https://slidetodoc.com/presentation_image/c1ea1fda97a0155e7beb2bfbc48146ec/image-4.jpg)
![List Construction Example • If L = [b, c, d] then – M= [a List Construction Example • If L = [b, c, d] then – M= [a](https://slidetodoc.com/presentation_image/c1ea1fda97a0155e7beb2bfbc48146ec/image-5.jpg)
![List Deletion • Assume X=[r, e, d, c, o, l, o, r]. • To List Deletion • Assume X=[r, e, d, c, o, l, o, r]. • To](https://slidetodoc.com/presentation_image/c1ea1fda97a0155e7beb2bfbc48146ec/image-6.jpg)
![Unification • • [b, a, d] = [d, a, b] [X]=[b, a, d] [X|Y]=[he, Unification • • [b, a, d] = [d, a, b] [X]=[b, a, d] [X|Y]=[he,](https://slidetodoc.com/presentation_image/c1ea1fda97a0155e7beb2bfbc48146ec/image-7.jpg)
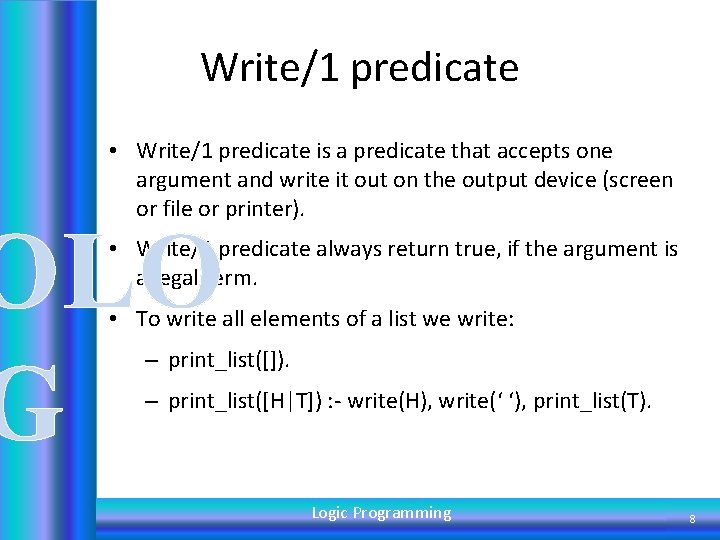
![print_list Example print_list([a, b, c]). H/a, T=[b, c] • print_list([]). write(a), write(‘ ‘), print_list([b, print_list Example print_list([a, b, c]). H/a, T=[b, c] • print_list([]). write(a), write(‘ ‘), print_list([b,](https://slidetodoc.com/presentation_image/c1ea1fda97a0155e7beb2bfbc48146ec/image-9.jpg)
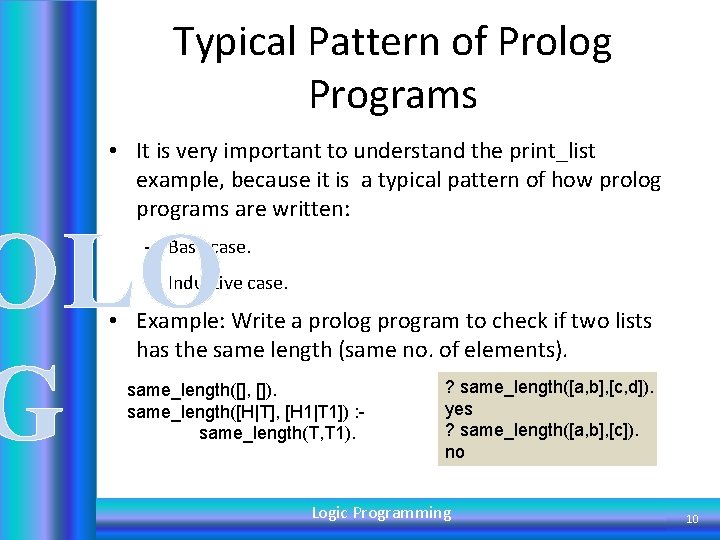
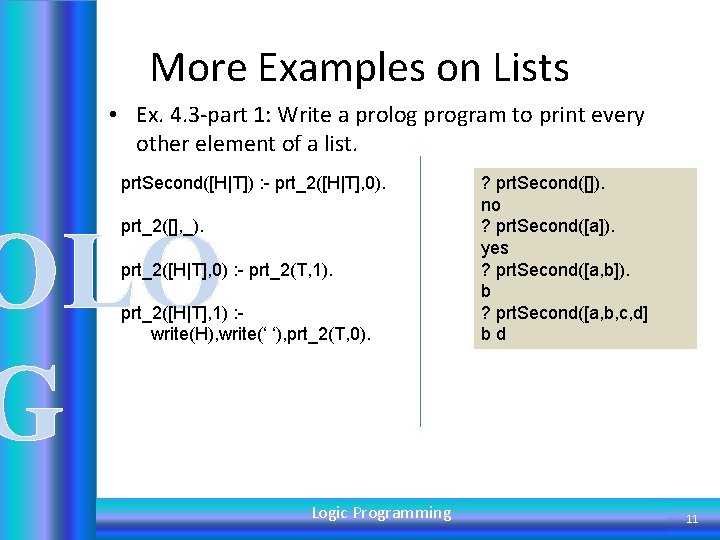
![Member Predicate member(H, [H|T]). member(E, [H|T]) : - memebr(c, [a, b, c, d]). H/c, Member Predicate member(H, [H|T]). member(E, [H|T]) : - memebr(c, [a, b, c, d]). H/c,](https://slidetodoc.com/presentation_image/c1ea1fda97a0155e7beb2bfbc48146ec/image-12.jpg)
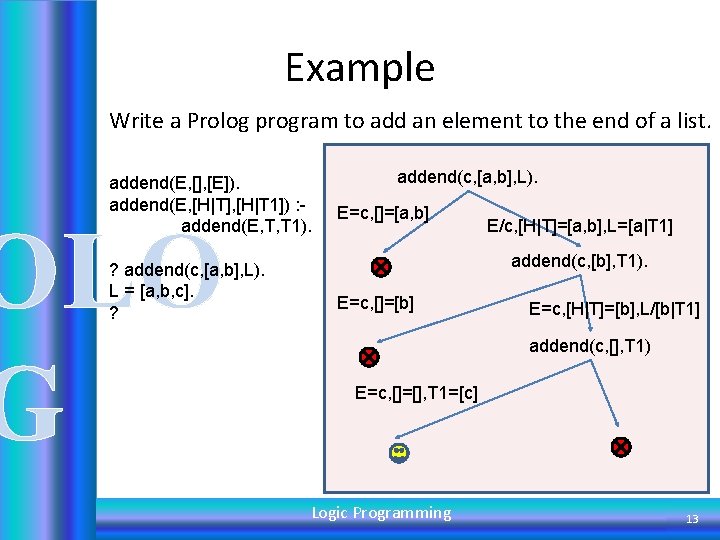
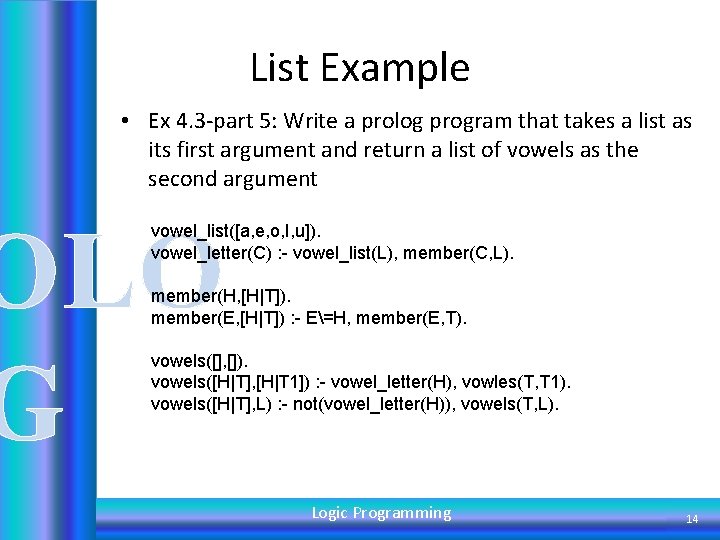
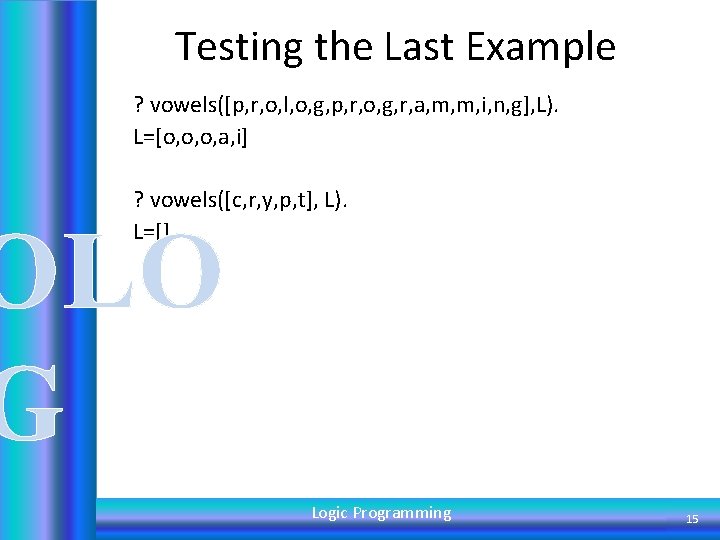
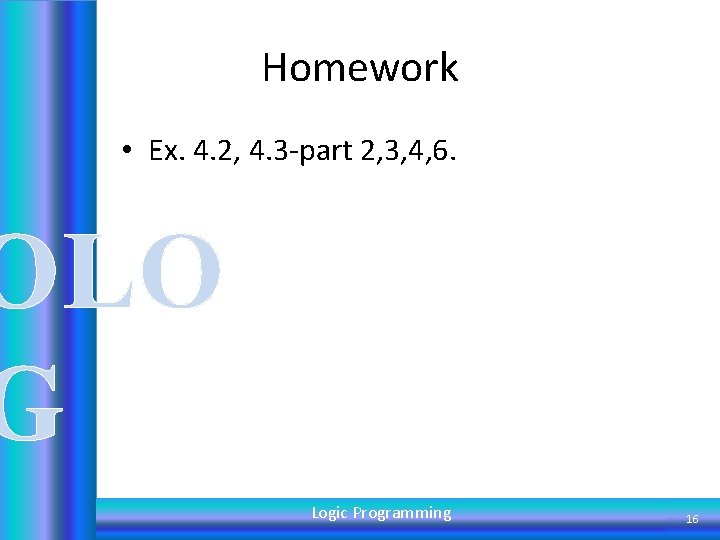
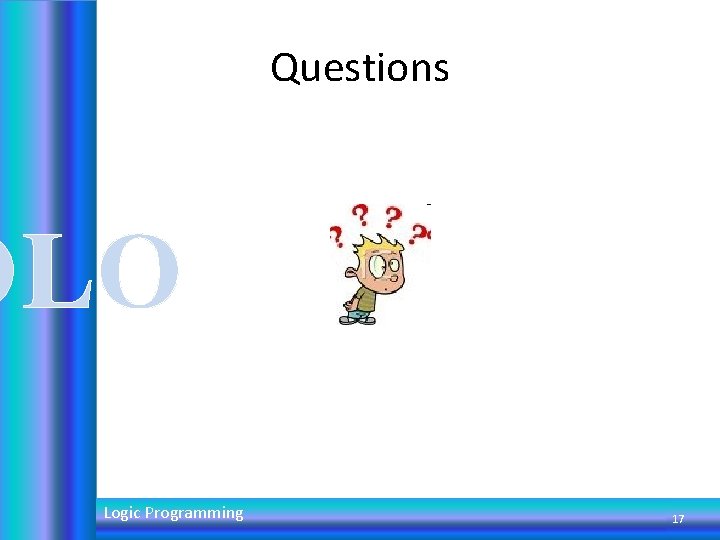
- Slides: 17
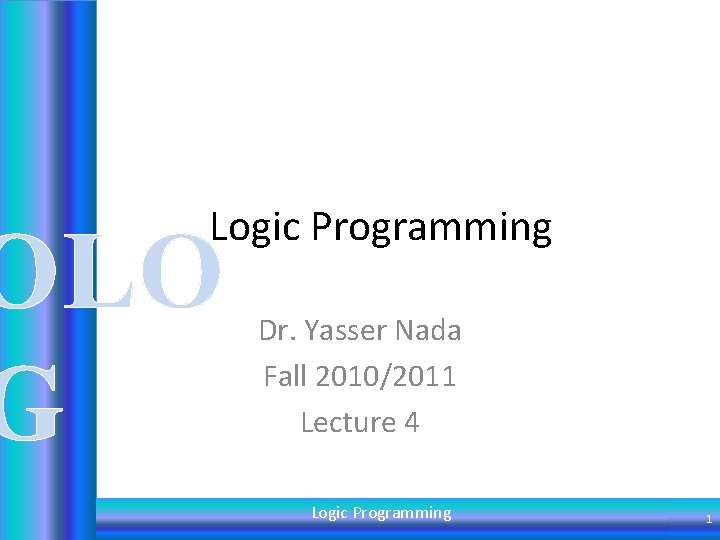
Logic Programming OLO G Dr. Yasser Nada Fall 2010/2011 Lecture 4 Logic Programming 1
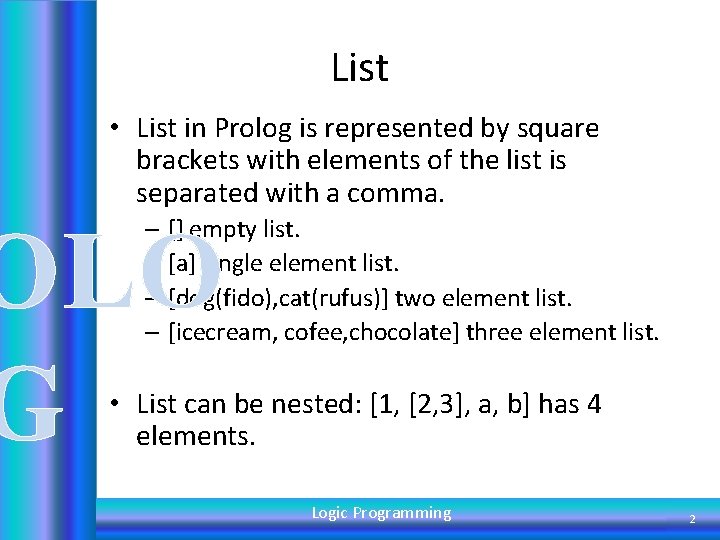
List • List in Prolog is represented by square brackets with elements of the list is separated with a comma. OLO G – [] empty list. – [a] single element list. – [dog(fido), cat(rufus)] two element list. – [icecream, cofee, chocolate] three element list. • List can be nested: [1, [2, 3], a, b] has 4 elements. Logic Programming 2
![List List also is represented as Head and Tail HTa b c List • List also is represented as Head and Tail. – [H|T]=[a, b, c]](https://slidetodoc.com/presentation_image/c1ea1fda97a0155e7beb2bfbc48146ec/image-3.jpg)
List • List also is represented as Head and Tail. – [H|T]=[a, b, c] OLO G • H=a head of list. • T=[b, c] rest of list. • Head is the first element in the list. • Tail is a list of the original list excluding the first element from the list. Logic Programming 3
![List Construction Assume Xr e d To add an element to the List Construction • Assume X=[r, e, d]. • To add an element to the](https://slidetodoc.com/presentation_image/c1ea1fda97a0155e7beb2bfbc48146ec/image-4.jpg)
List Construction • Assume X=[r, e, d]. • To add an element to the head of the list X, we write: OLO G – Y=[b|X]=[b, r, e, d]. • To add three elements to the head of the list, we write: – Y=[a, b, c|X] = [a, b, c, r, e, d]. Logic Programming 4
![List Construction Example If L b c d then M a List Construction Example • If L = [b, c, d] then – M= [a](https://slidetodoc.com/presentation_image/c1ea1fda97a0155e7beb2bfbc48146ec/image-5.jpg)
List Construction Example • If L = [b, c, d] then – M= [a | L] = [a, b, c, d] • If L = [b, c, d] then – N = [[a] | L] = [[a], b, c, d] • If L = [[b], c, d] then – K = [a | L] = [a, [b], c, d] Logic Programming 5
![List Deletion Assume Xr e d c o l o r To List Deletion • Assume X=[r, e, d, c, o, l, o, r]. • To](https://slidetodoc.com/presentation_image/c1ea1fda97a0155e7beb2bfbc48146ec/image-6.jpg)
List Deletion • Assume X=[r, e, d, c, o, l, o, r]. • To delete the first three element from the list X, we write: OLO G – X=[A, B, C|T] A=r, B=e, C=d, T=[c, o, l, o, r]. • If we delete the first element from an empty list, then fail is returned. – If Y=[], then if we write: • Y=[A|T] this returns fail. Logic Programming 6
![Unification b a d d a b Xb a d XYhe Unification • • [b, a, d] = [d, a, b] [X]=[b, a, d] [X|Y]=[he,](https://slidetodoc.com/presentation_image/c1ea1fda97a0155e7beb2bfbc48146ec/image-7.jpg)
Unification • • [b, a, d] = [d, a, b] [X]=[b, a, d] [X|Y]=[he, is, a, cat] [X, Y|Z]=[a, b, c, d] [X|Y]=[[a, [b, c]], d] [X|Y]=[a] OLO G fail to match. fail. X=he Y=[is, a, cat] X=a Y=b Z=[c, d] fail X=[a, [b, c]] Y=[d] X=a Y[] Logic Programming 7
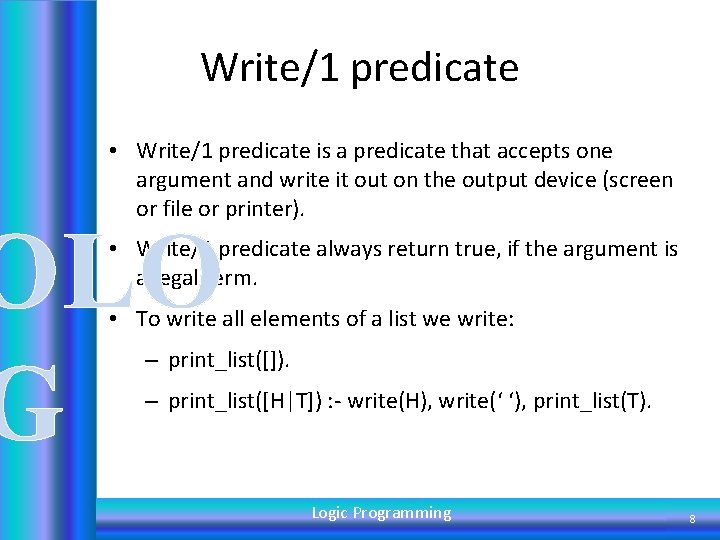
Write/1 predicate • Write/1 predicate is a predicate that accepts one argument and write it out on the output device (screen or file or printer). OLO G • Write/1 predicate always return true, if the argument is a legal term. • To write all elements of a list we write: – print_list([]). – print_list([H|T]) : - write(H), write(‘ ‘), print_list(T). Logic Programming 8
![printlist Example printlista b c Ha Tb c printlist writea write printlistb print_list Example print_list([a, b, c]). H/a, T=[b, c] • print_list([]). write(a), write(‘ ‘), print_list([b,](https://slidetodoc.com/presentation_image/c1ea1fda97a0155e7beb2bfbc48146ec/image-9.jpg)
print_list Example print_list([a, b, c]). H/a, T=[b, c] • print_list([]). write(a), write(‘ ‘), print_list([b, c]). • print_list([H|T]) : write(H), write(‘ ‘), print_list(T). OLO G • ? print_list([a, b, c]). • abc print_list([b, c]) H/b, T=[c] write(b), write(‘ ‘), print_list([c]), H=c, T=[] write(c), write(‘ ‘), print_list([]). [] [H|T] Logic Programming 9
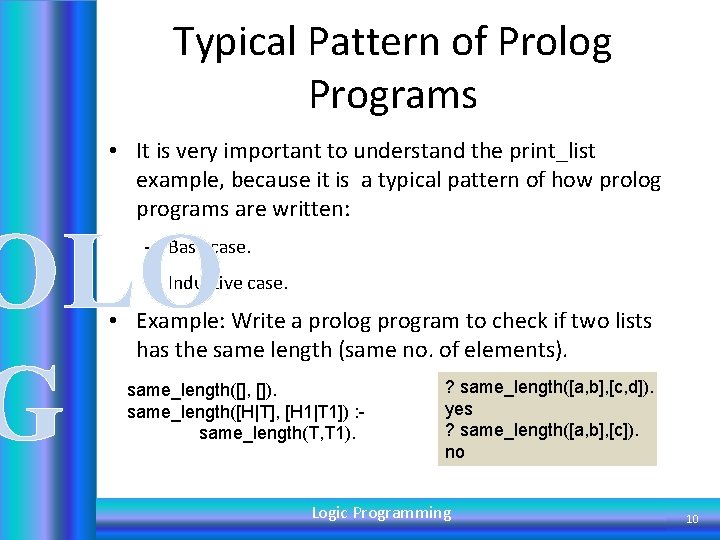
Typical Pattern of Prolog Programs • It is very important to understand the print_list example, because it is a typical pattern of how prolog programs are written: OLO G – Base case. – Inductive case. • Example: Write a prolog program to check if two lists has the same length (same no. of elements). same_length([], []). same_length([H|T], [H 1|T 1]) : same_length(T, T 1). ? same_length([a, b], [c, d]). yes ? same_length([a, b], [c]). no Logic Programming 10
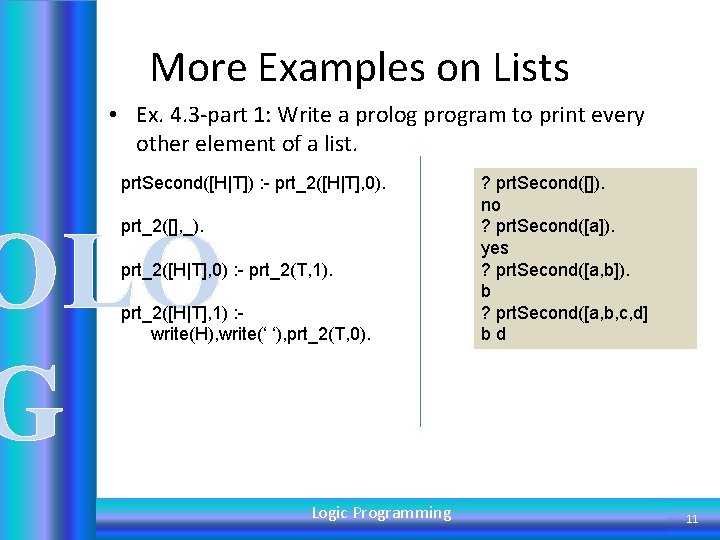
More Examples on Lists • Ex. 4. 3 -part 1: Write a prolog program to print every other element of a list. prt. Second([H|T]) : - prt_2([H|T], 0). OLO G prt_2([], _). prt_2([H|T], 0) : - prt_2(T, 1). prt_2([H|T], 1) : write(H), write(‘ ‘), prt_2(T, 0). Logic Programming ? prt. Second([]). no ? prt. Second([a]). yes ? prt. Second([a, b]). b ? prt. Second([a, b, c, d] bd 11
![Member Predicate memberH HT memberE HT memebrc a b c d Hc Member Predicate member(H, [H|T]). member(E, [H|T]) : - memebr(c, [a, b, c, d]). H/c,](https://slidetodoc.com/presentation_image/c1ea1fda97a0155e7beb2bfbc48146ec/image-12.jpg)
Member Predicate member(H, [H|T]). member(E, [H|T]) : - memebr(c, [a, b, c, d]). H/c, [H|T]=[a, b, c, d] E = H, member(E, T). OLO G E/c, [H|T]=[a, b, c, d] c=a, member(c, [b, c, d]). H=a, [H|T]=[b, c, d] E=c, [H|T]=[b, c, d] c=b, member(c, [c, d]). H=c, [H|T]=[c, b] c=c , member(c, [d]). Logic Programming 12
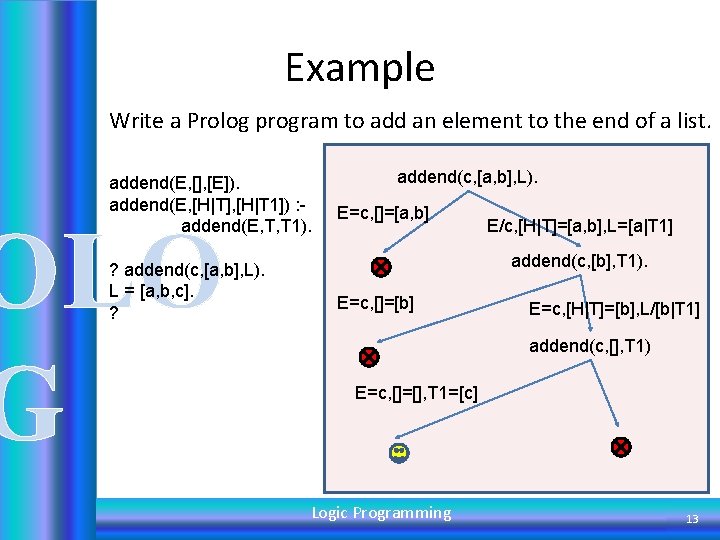
Example Write a Prolog program to add an element to the end of a list. addend(E, [], [E]). addend(E, [H|T], [H|T 1]) : addend(E, T, T 1). OLO G ? addend(c, [a, b], L). L = [a, b, c]. ? addend(c, [a, b], L). E=c, []=[a, b] E/c, [H|T]=[a, b], L=[a|T 1] addend(c, [b], T 1). E=c, []=[b] E=c, [H|T]=[b], L/[b|T 1] addend(c, [], T 1) E=c, []=[], T 1=[c] Logic Programming 13
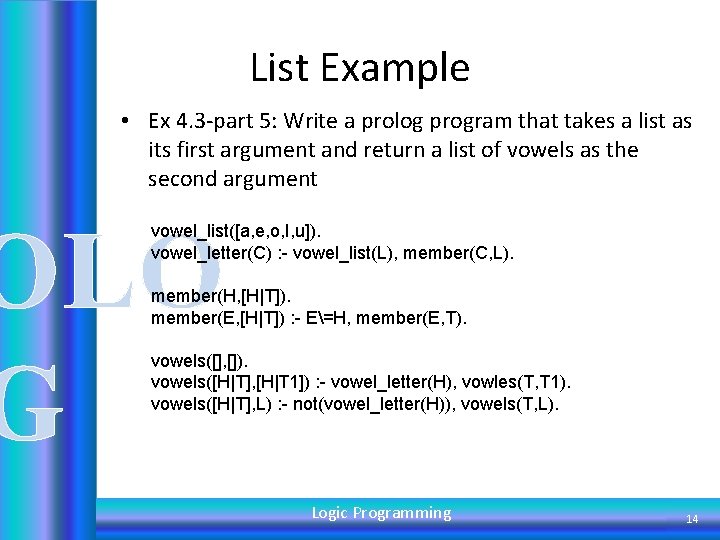
List Example • Ex 4. 3 -part 5: Write a prolog program that takes a list as its first argument and return a list of vowels as the second argument OLO G vowel_list([a, e, o, I, u]). vowel_letter(C) : - vowel_list(L), member(C, L). member(H, [H|T]). member(E, [H|T]) : - E=H, member(E, T). vowels([], []). vowels([H|T], [H|T 1]) : - vowel_letter(H), vowles(T, T 1). vowels([H|T], L) : - not(vowel_letter(H)), vowels(T, L). Logic Programming 14
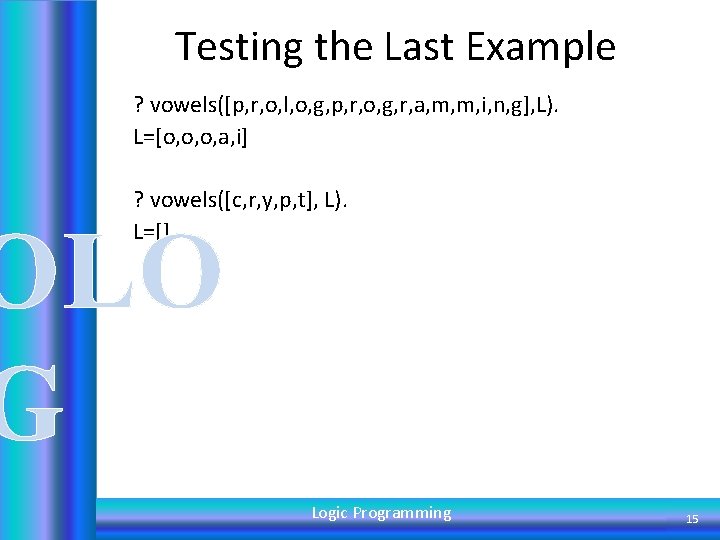
Testing the Last Example ? vowels([p, r, o, l, o, g, p, r, o, g, r, a, m, m, i, n, g], L). L=[o, o, o, a, i] ? vowels([c, r, y, p, t], L). L=[] OLO G Logic Programming 15
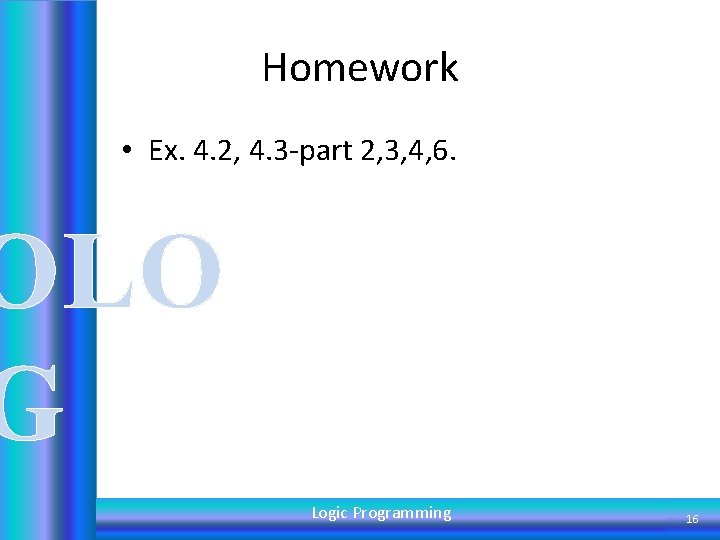
Homework • Ex. 4. 2, 4. 3 -part 2, 3, 4, 6. OLO G Logic Programming 16
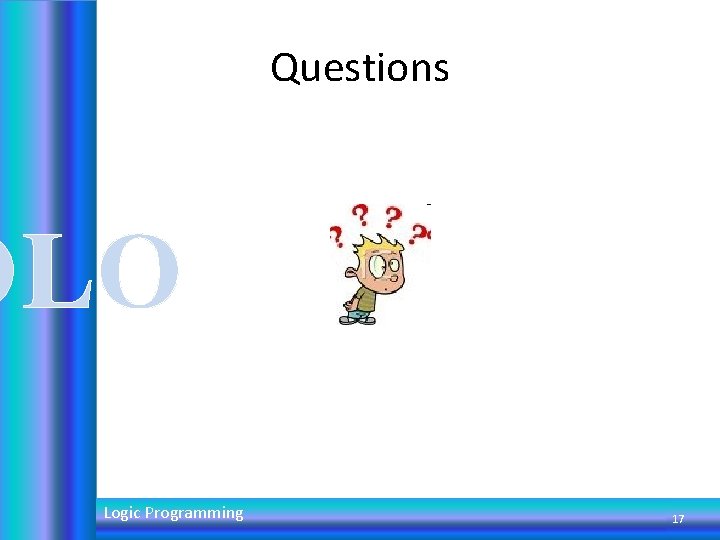
Questions OLO Logic Programming 17
Nada enharmonis dari bb adalah
2322008
Francis yasser
Define olo
Programme olo inscription
Precious blood apostolate olo-nigeria
Olo g
Estamos aqui de passagem nada trouxemos e nada levaremos
Nada te turbe; nada te espante salmo 37
Quien es nada
Nada te turbe santa teresa
Nada te turbe analisis
Salmo 37, 7 nada te turbe
Tudo para o estado nada contra o estado nada fora do estado
Nada des enharmonis dengan nada.
Nada te turbe taize letra
If x = 0 and y = 1, which output line is enabled?
First order logic vs propositional logic