Linked Lists struct node int data struct node
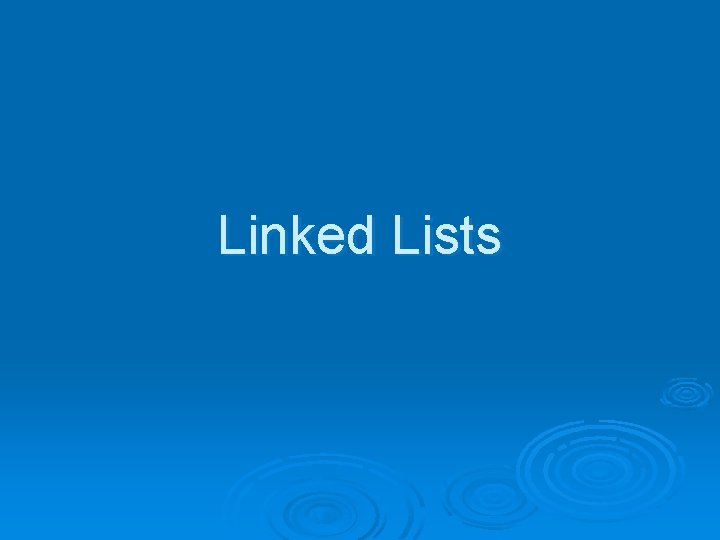
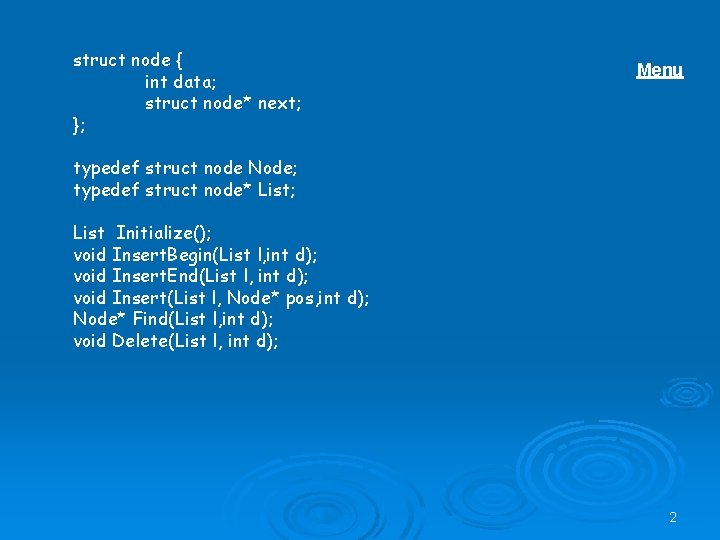
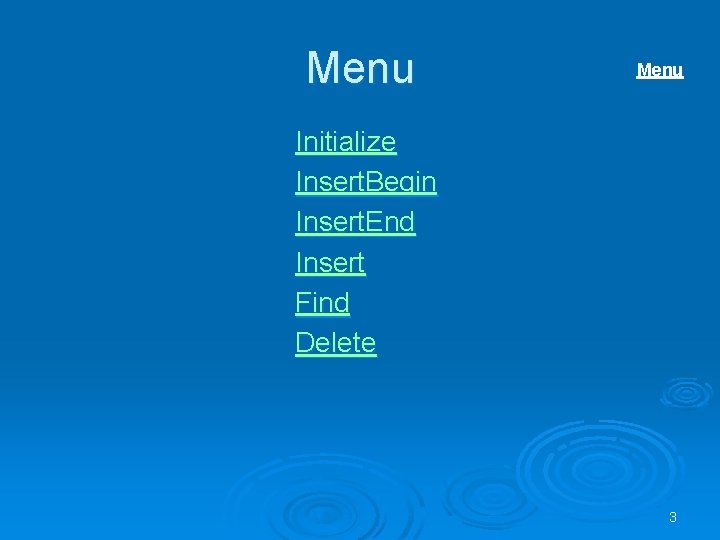
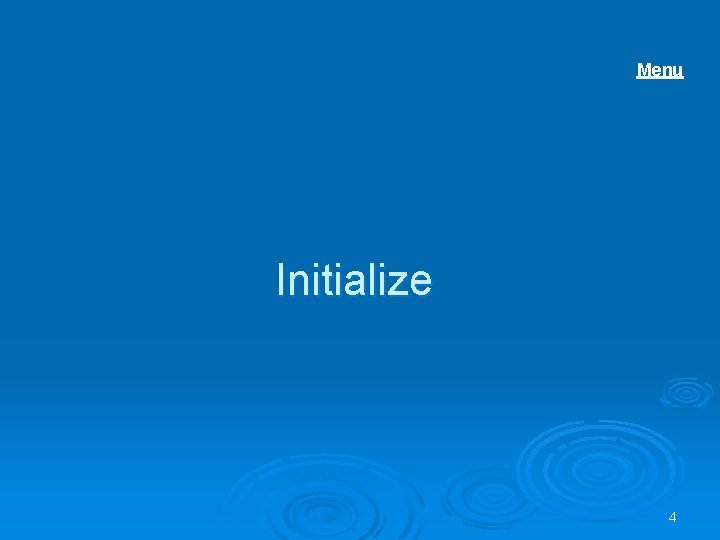
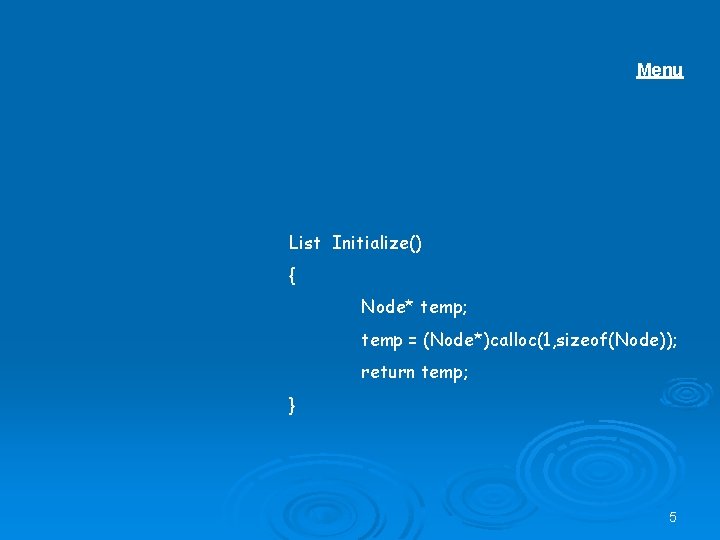
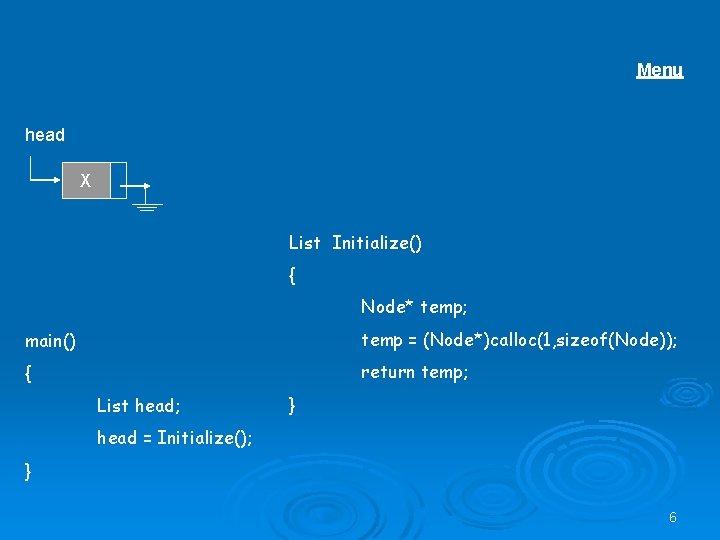
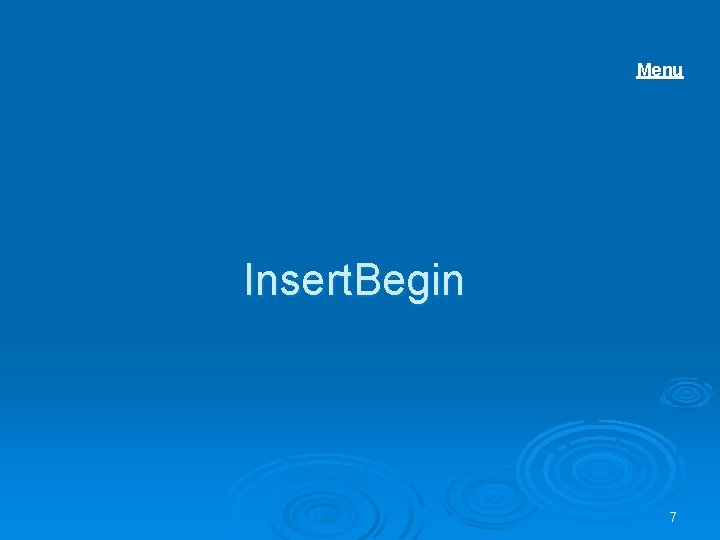
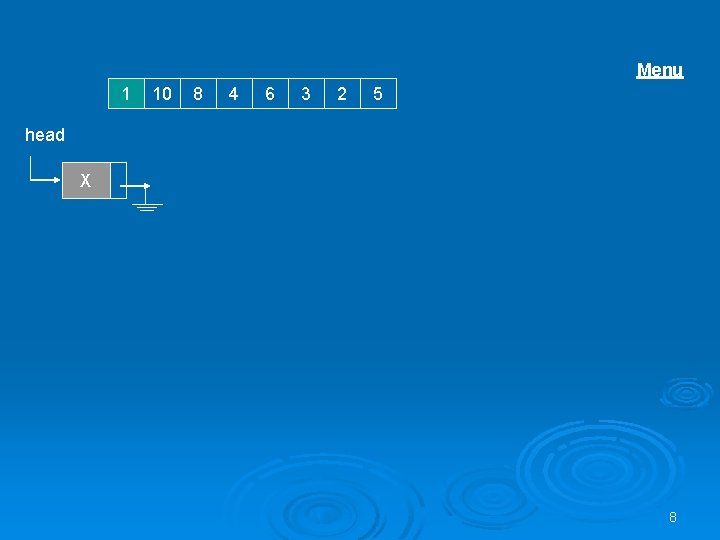
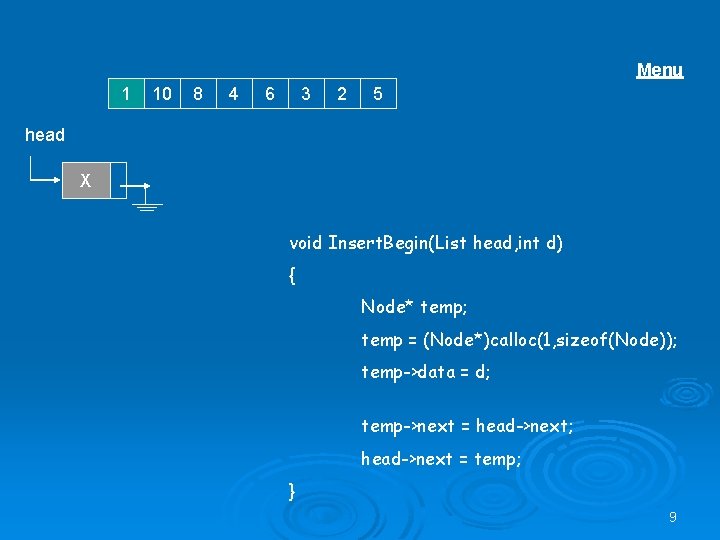
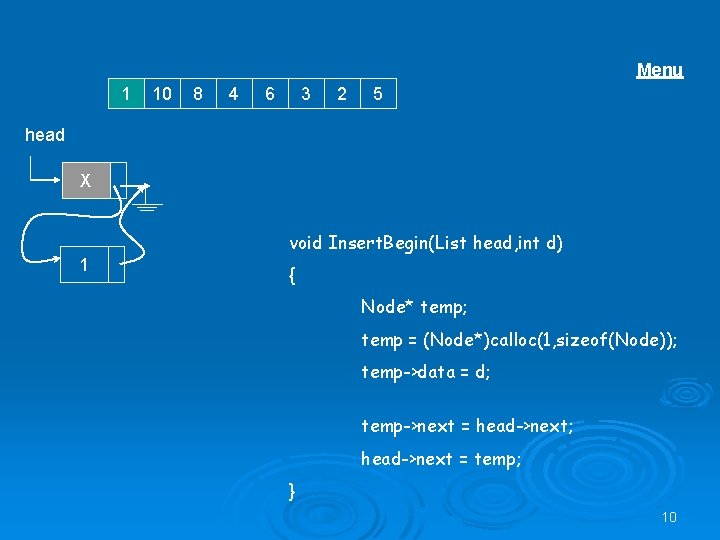
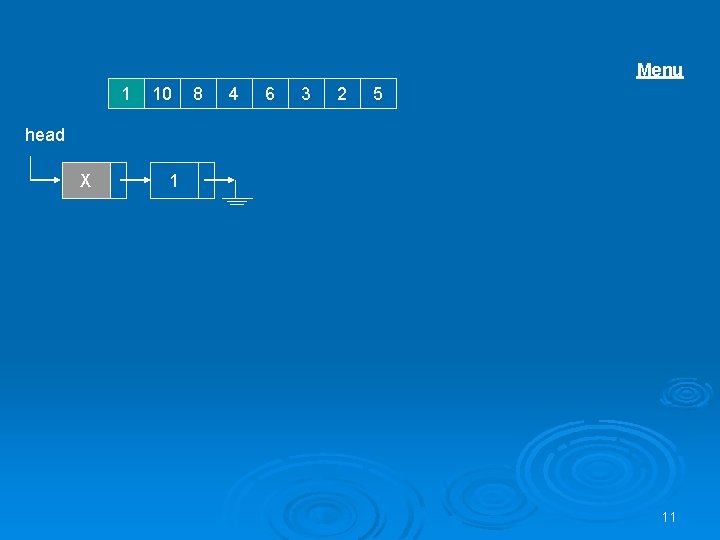
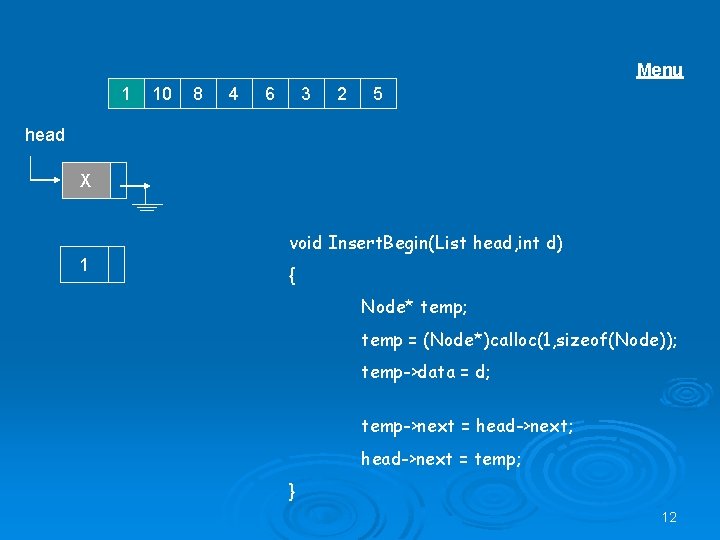
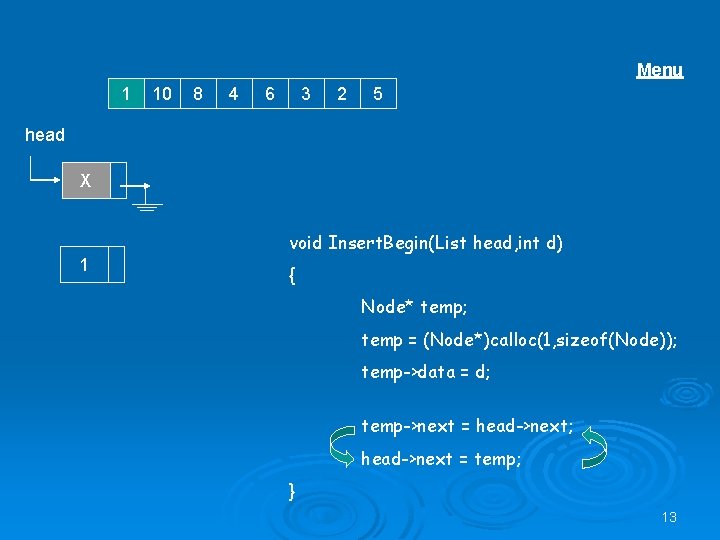
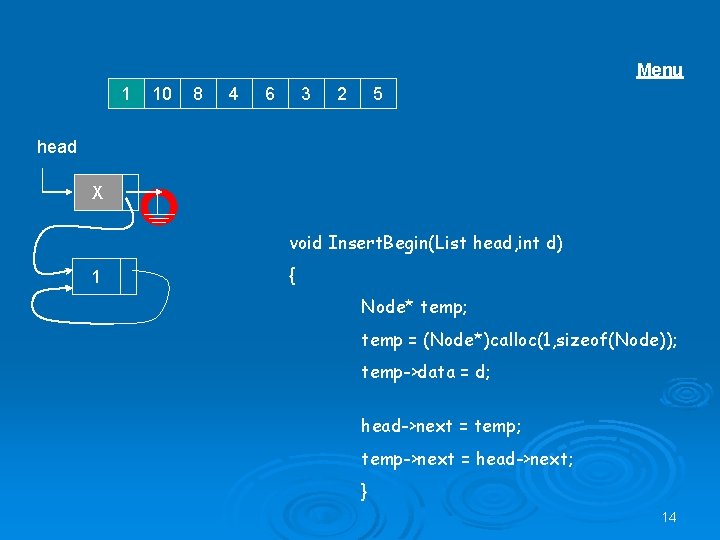
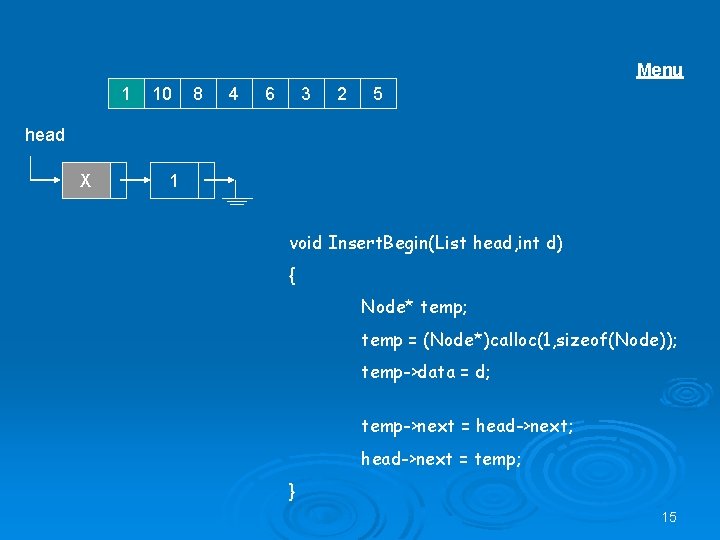
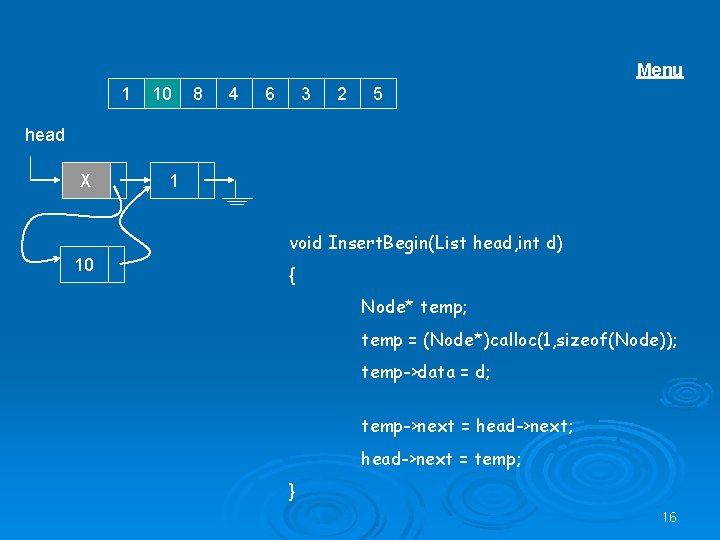
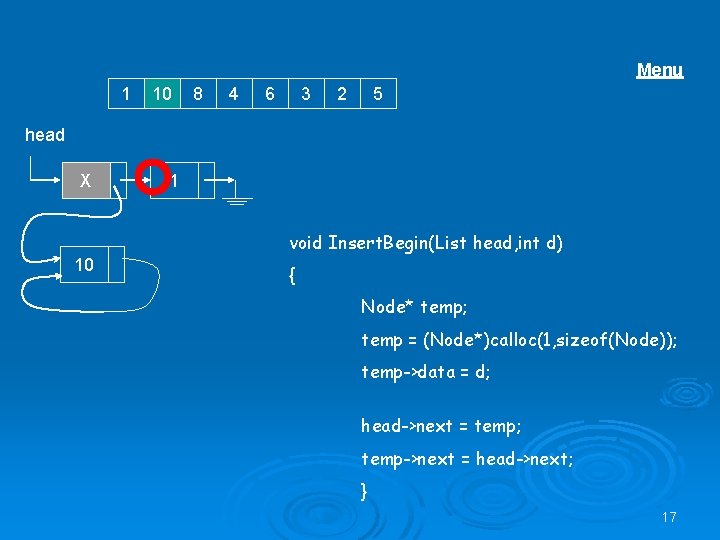
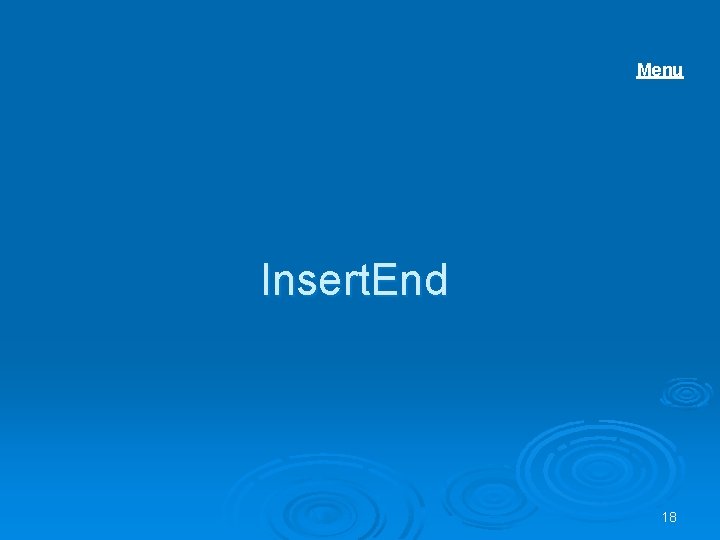
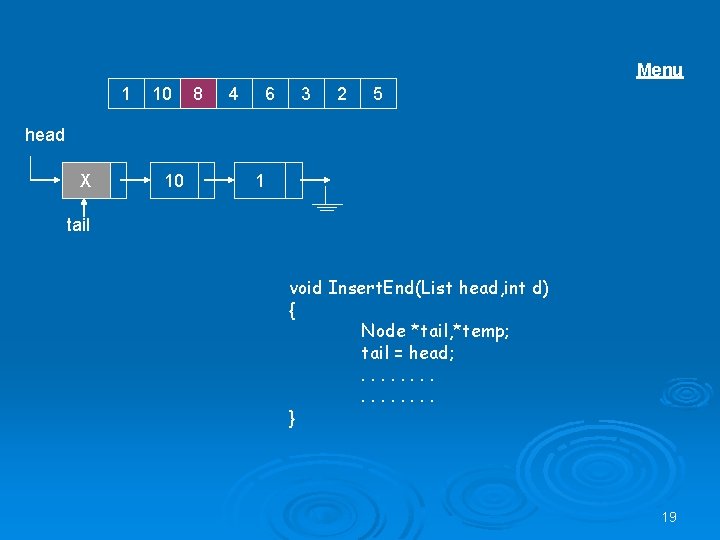
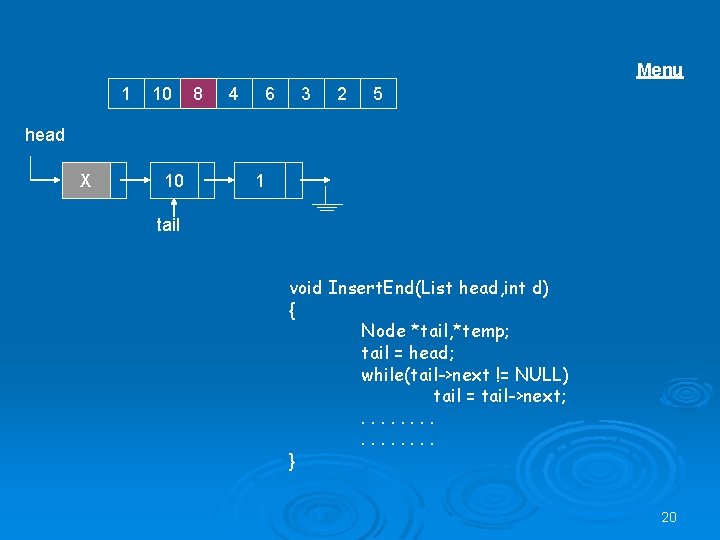
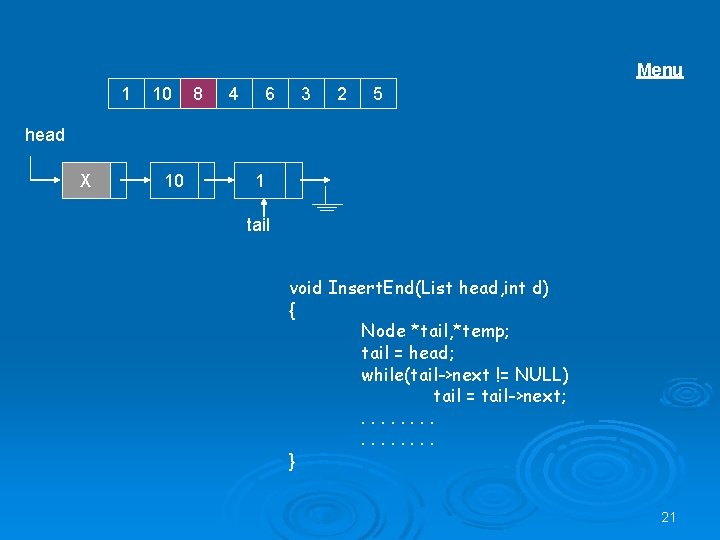
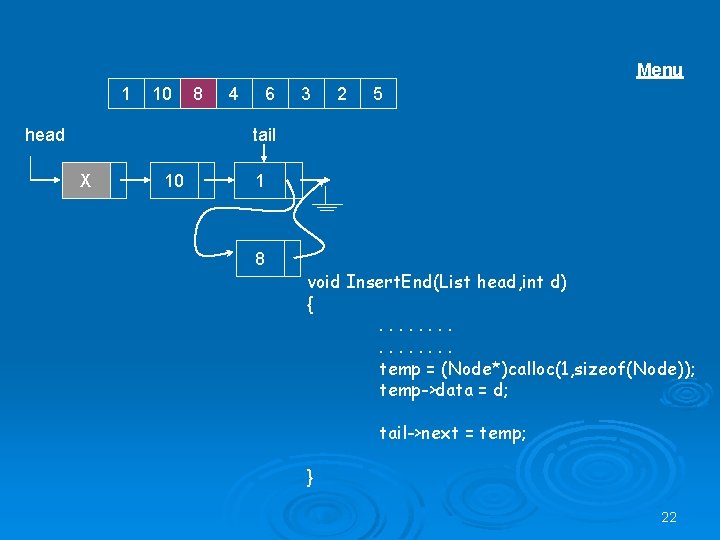
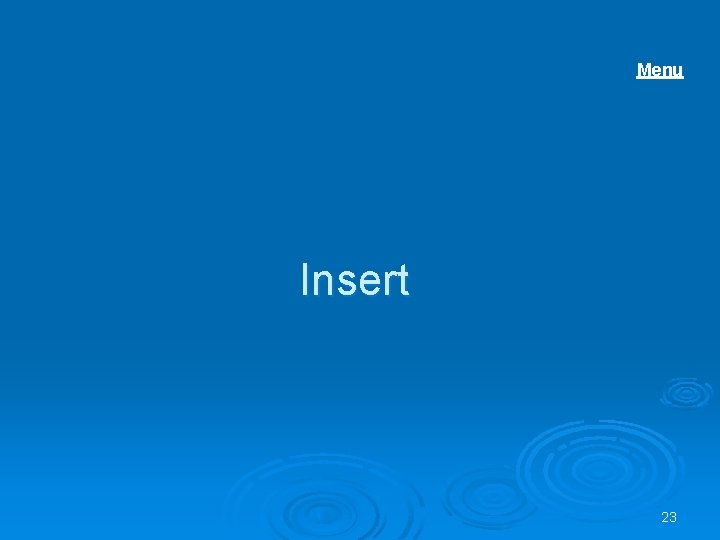
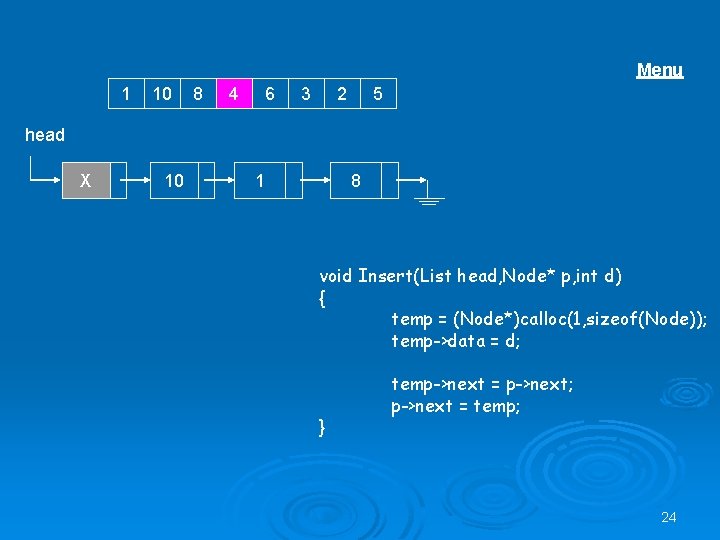
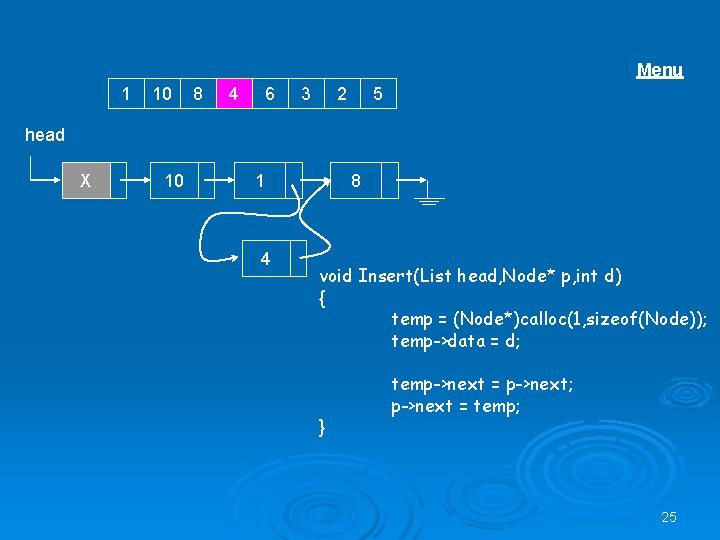
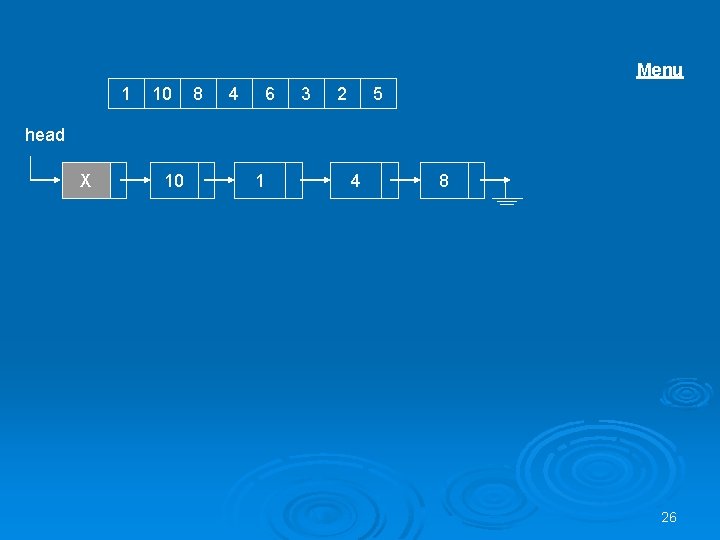
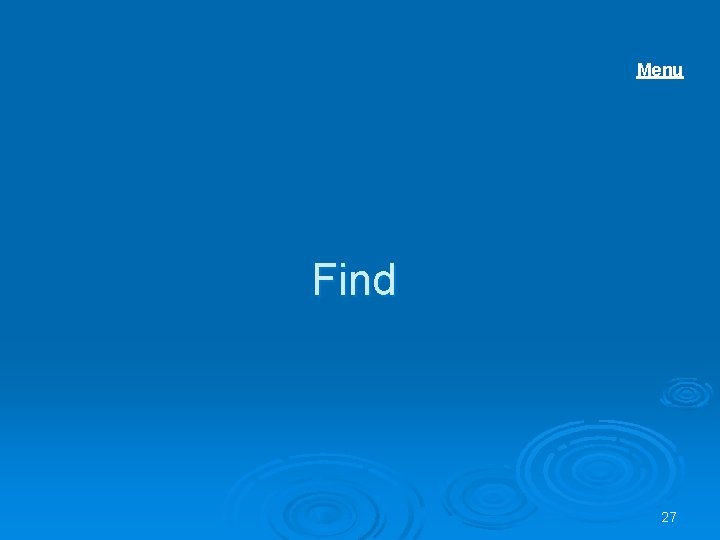
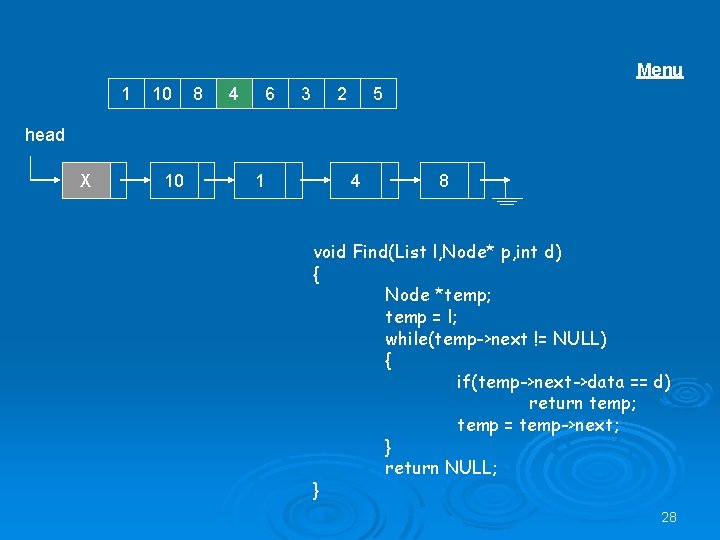
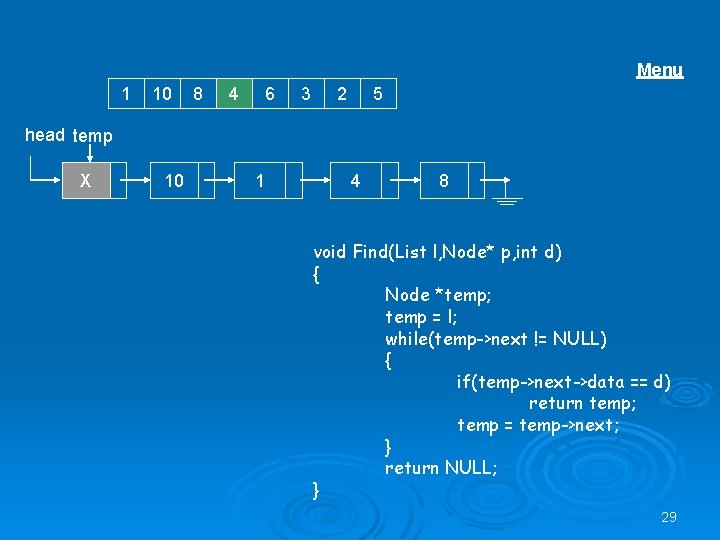
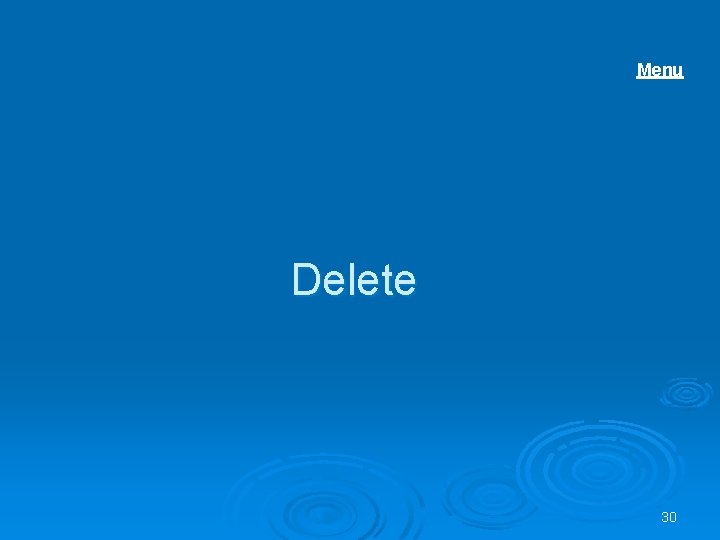
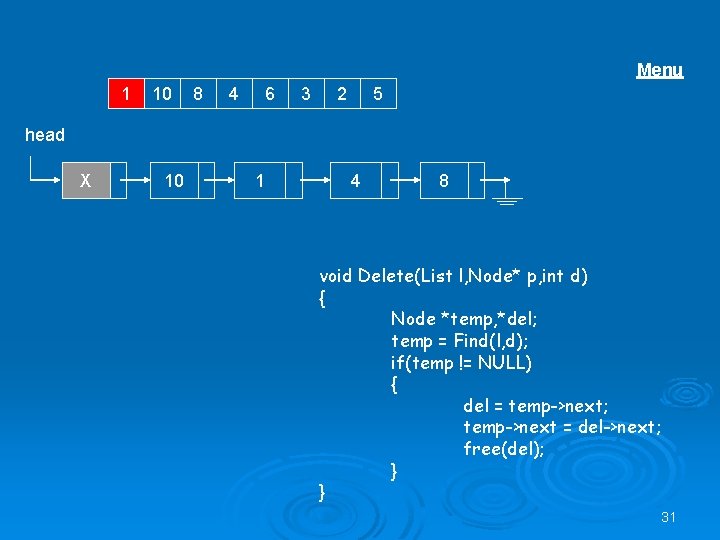
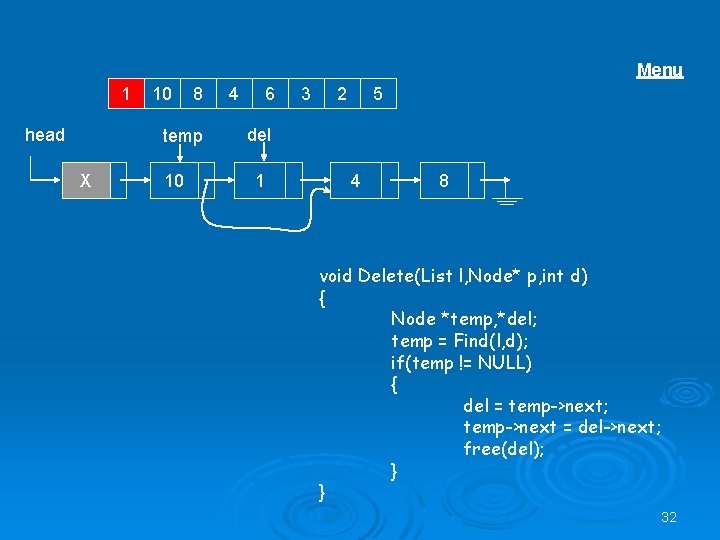
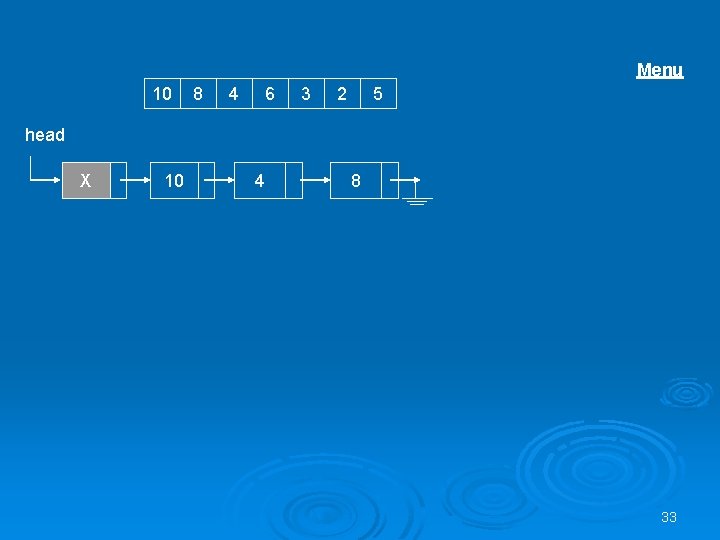
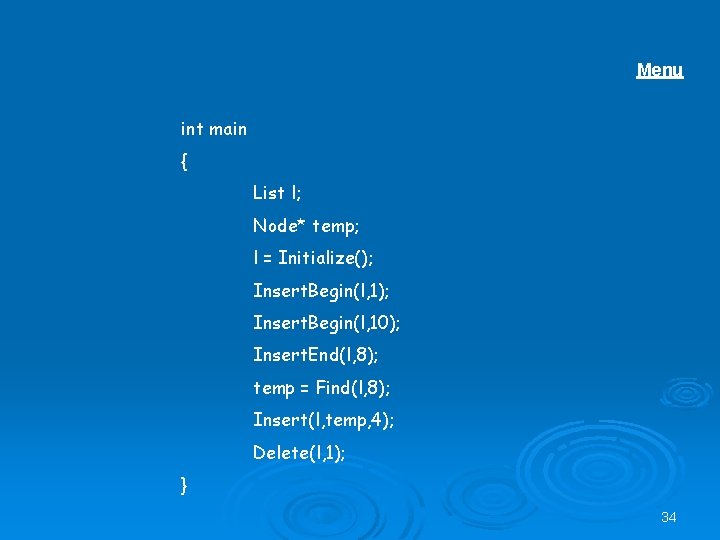
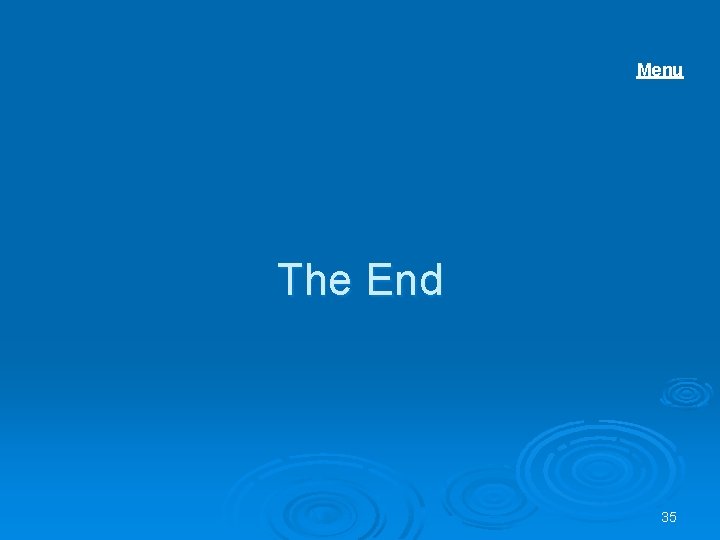
- Slides: 35
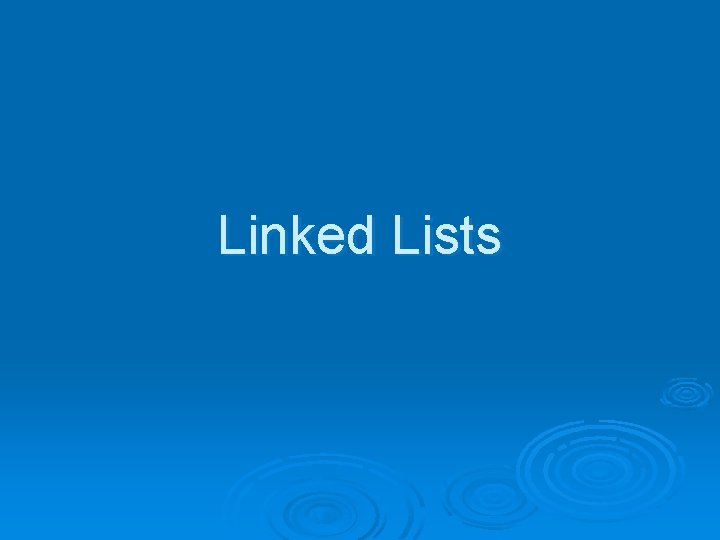
Linked Lists
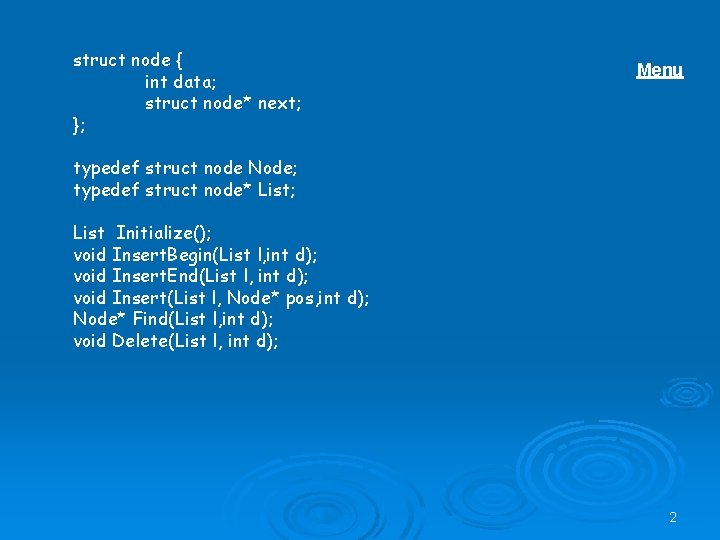
struct node { int data; struct node* next; }; Menu typedef struct node Node; typedef struct node* List; List Initialize(); void Insert. Begin(List l, int d); void Insert. End(List l, int d); void Insert(List l, Node* pos, int d); Node* Find(List l, int d); void Delete(List l, int d); 2
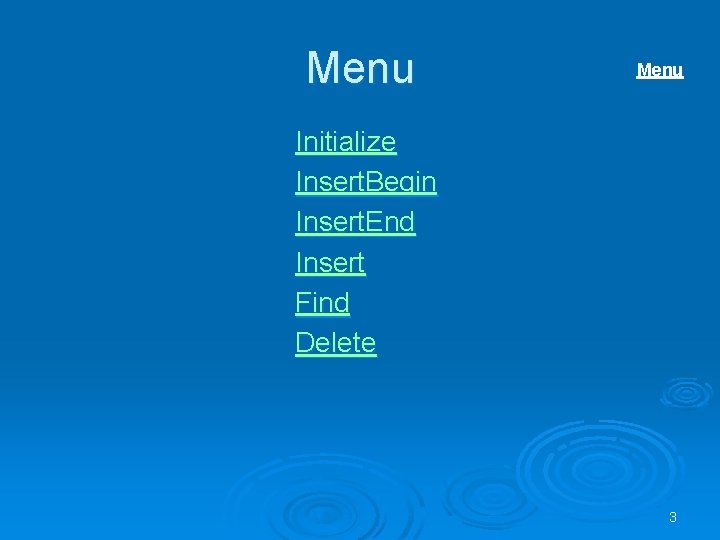
Menu Initialize Insert. Begin Insert. End Insert Find Delete 3
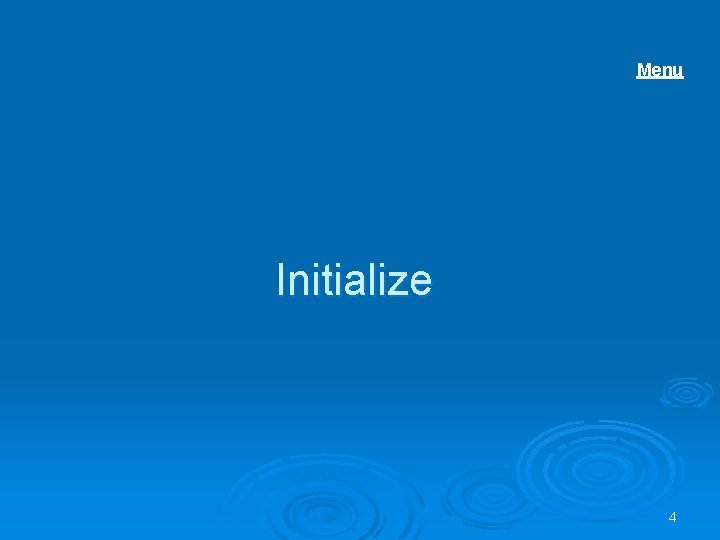
Menu Initialize 4
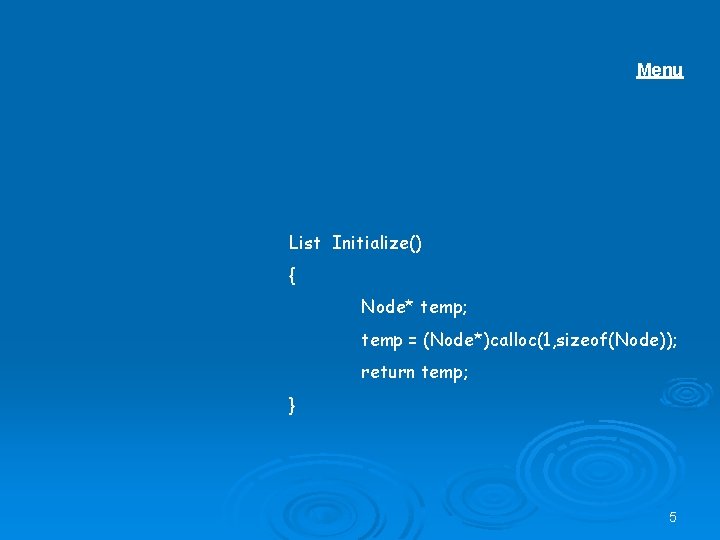
Menu List Initialize() { Node* temp; temp = (Node*)calloc(1, sizeof(Node)); return temp; } 5
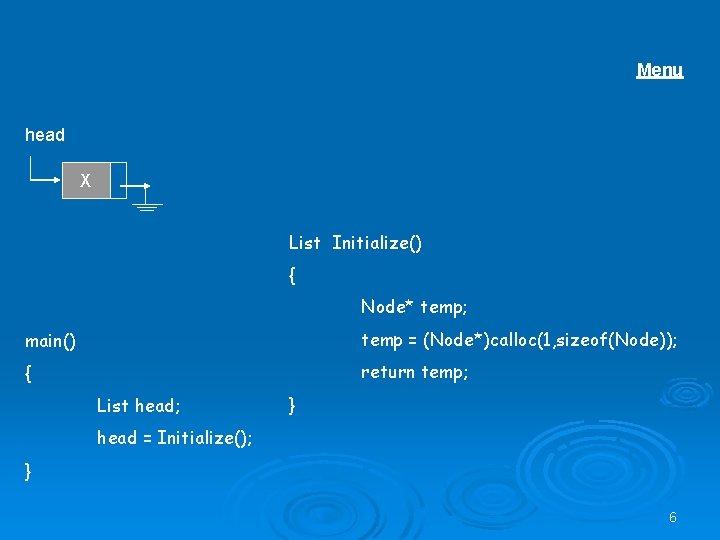
Menu head X List Initialize() { Node* temp; main() temp = (Node*)calloc(1, sizeof(Node)); { return temp; List head; } head = Initialize(); } 6
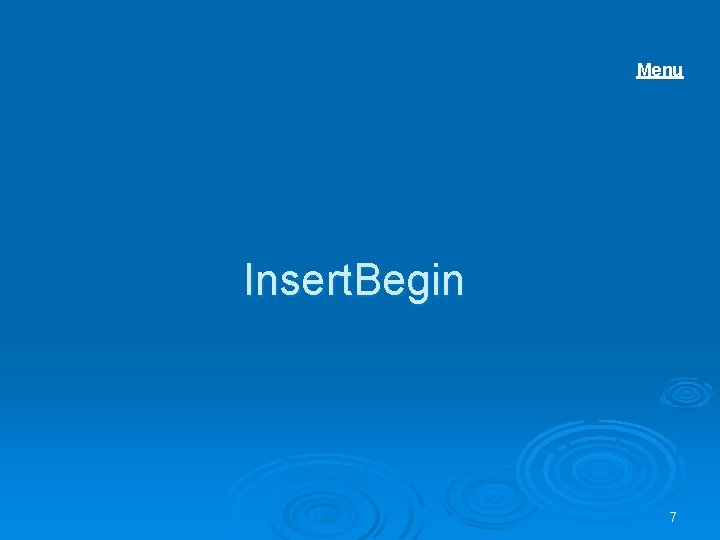
Menu Insert. Begin 7
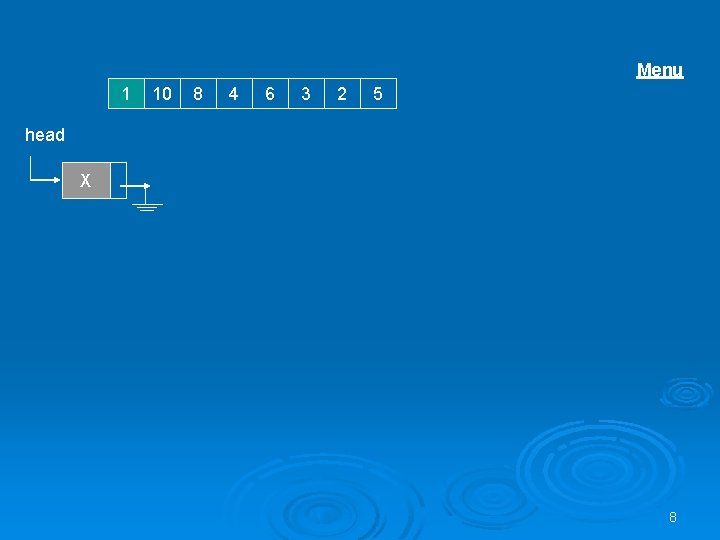
Menu 1 10 8 4 6 3 2 5 head X 8
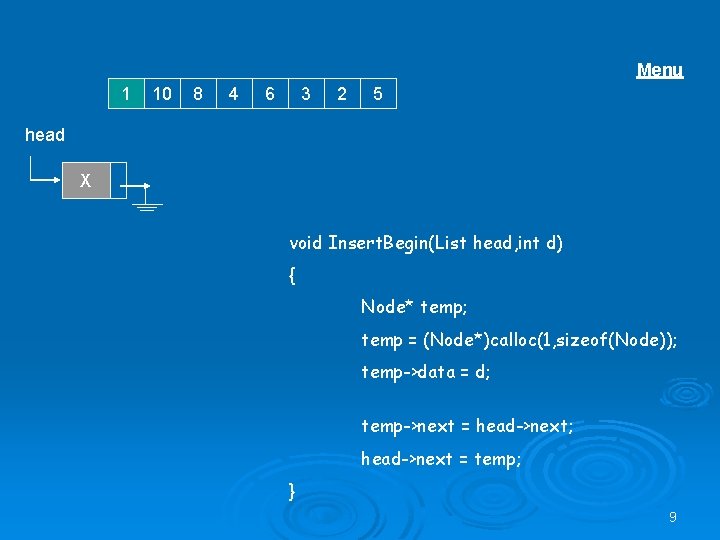
Menu 1 10 8 4 6 3 2 5 head X void Insert. Begin(List head, int d) { Node* temp; temp = (Node*)calloc(1, sizeof(Node)); temp->data = d; temp->next = head->next; head->next = temp; } 9
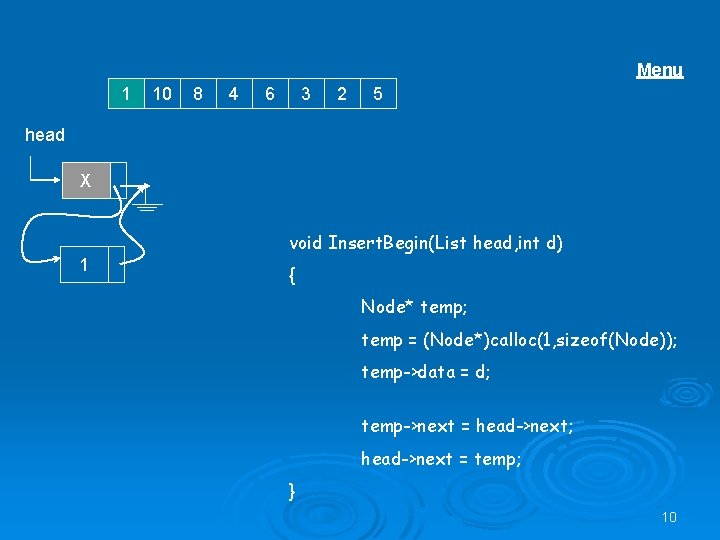
Menu 1 10 8 4 6 3 2 5 head X void Insert. Begin(List head, int d) 1 { Node* temp; temp = (Node*)calloc(1, sizeof(Node)); temp->data = d; temp->next = head->next; head->next = temp; } 10
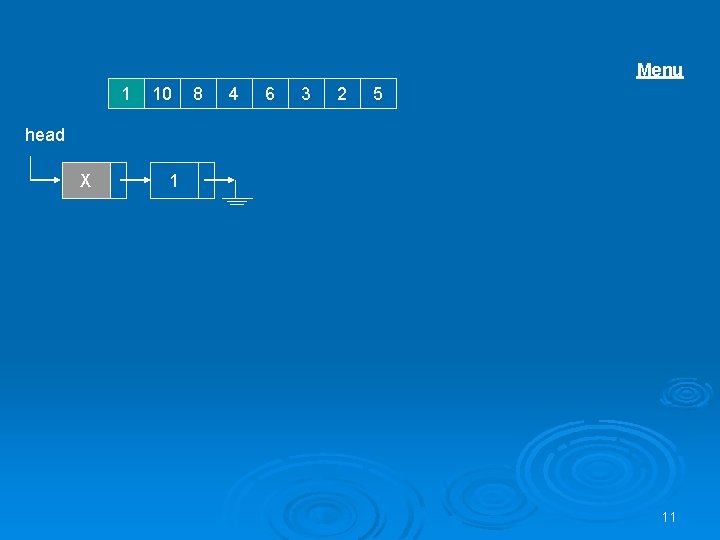
Menu 1 10 8 4 6 3 2 5 head X 1 11
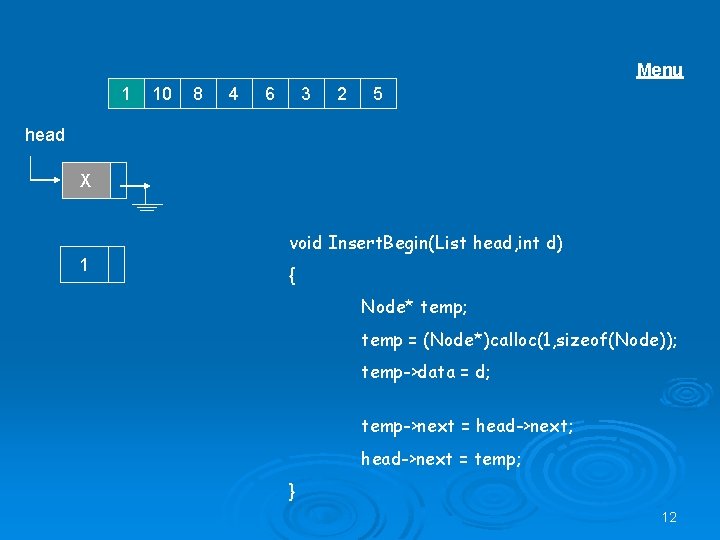
Menu 1 10 8 4 6 3 2 5 head X void Insert. Begin(List head, int d) 1 { Node* temp; temp = (Node*)calloc(1, sizeof(Node)); temp->data = d; temp->next = head->next; head->next = temp; } 12
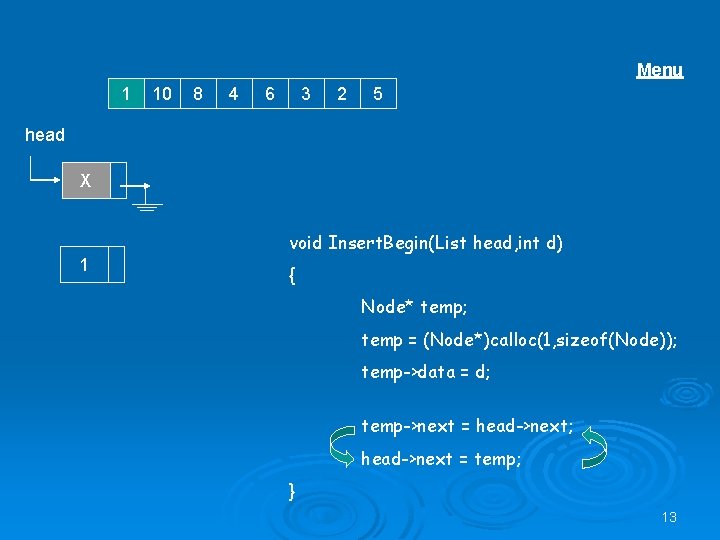
Menu 1 10 8 4 6 3 2 5 head X void Insert. Begin(List head, int d) 1 { Node* temp; temp = (Node*)calloc(1, sizeof(Node)); temp->data = d; temp->next = head->next; head->next = temp; } 13
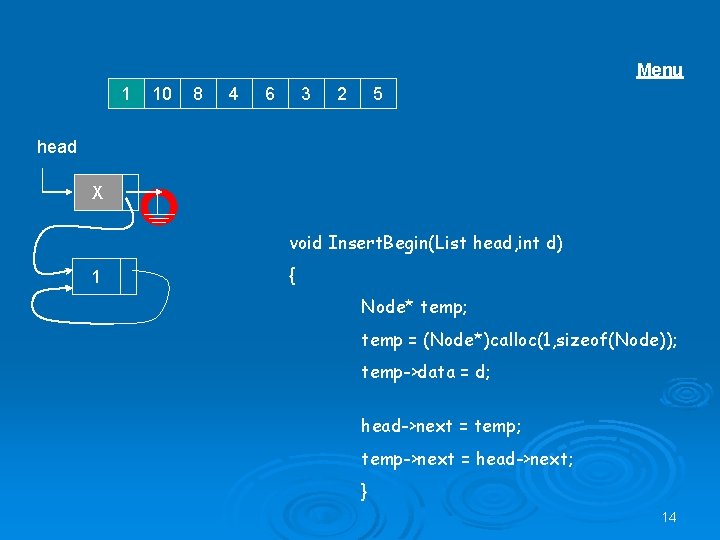
Menu 1 10 8 4 6 3 2 5 head X void Insert. Begin(List head, int d) 1 { Node* temp; temp = (Node*)calloc(1, sizeof(Node)); temp->data = d; head->next = temp; temp->next = head->next; } 14
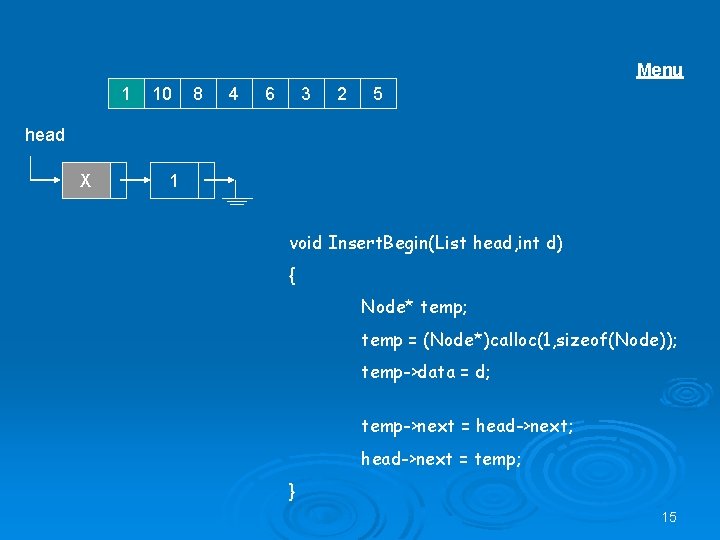
Menu 1 10 8 4 6 3 2 5 head X 1 void Insert. Begin(List head, int d) { Node* temp; temp = (Node*)calloc(1, sizeof(Node)); temp->data = d; temp->next = head->next; head->next = temp; } 15
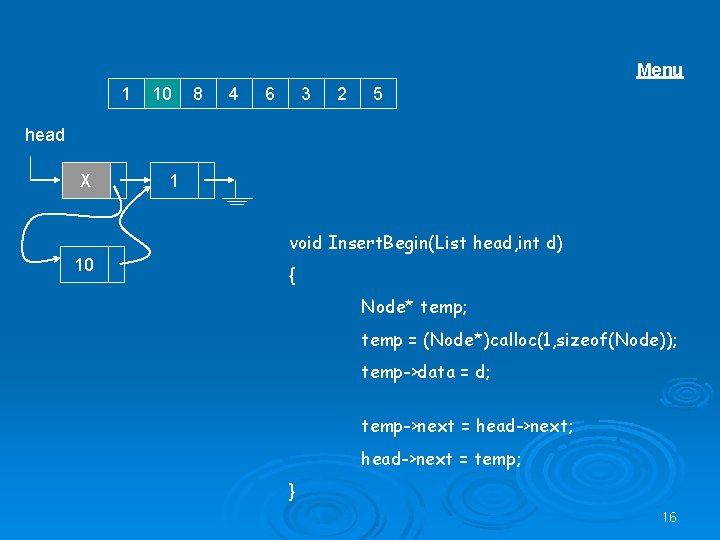
Menu 1 10 8 4 6 3 2 5 head X 1 void Insert. Begin(List head, int d) 10 { Node* temp; temp = (Node*)calloc(1, sizeof(Node)); temp->data = d; temp->next = head->next; head->next = temp; } 16
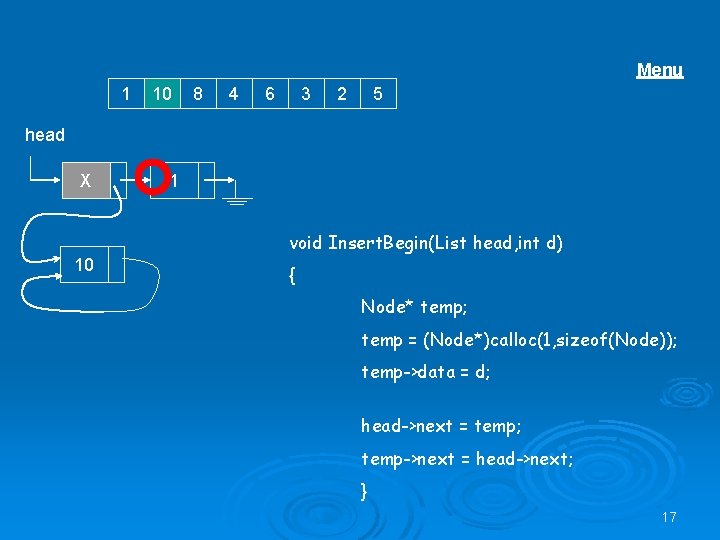
Menu 1 10 8 4 6 3 2 5 head X 1 void Insert. Begin(List head, int d) 10 { Node* temp; temp = (Node*)calloc(1, sizeof(Node)); temp->data = d; head->next = temp; temp->next = head->next; } 17
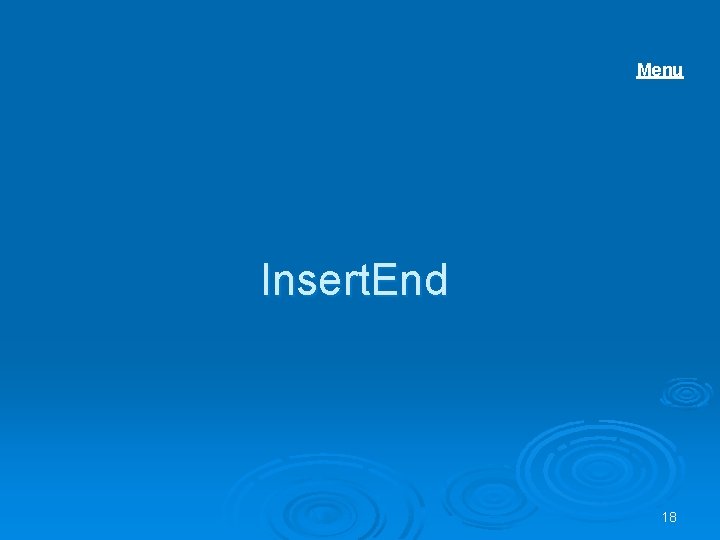
Menu Insert. End 18
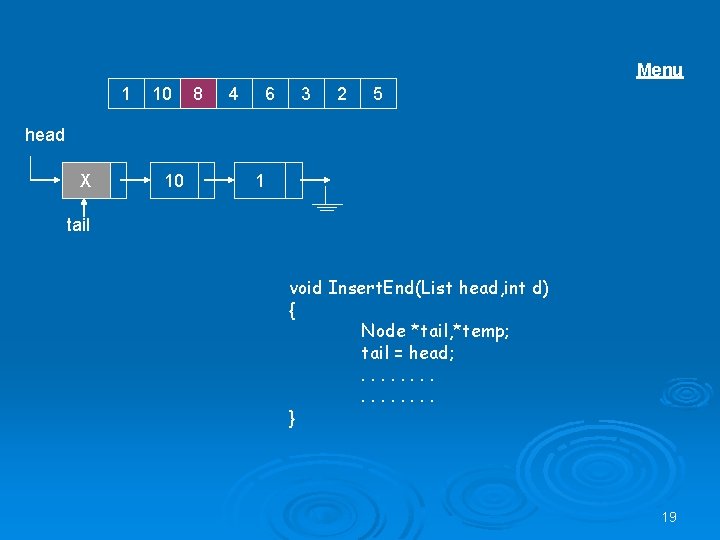
Menu 1 10 8 4 6 3 2 5 head X 10 1 tail void Insert. End(List head, int d) { Node *tail, *temp; tail = head; . . . . } 19
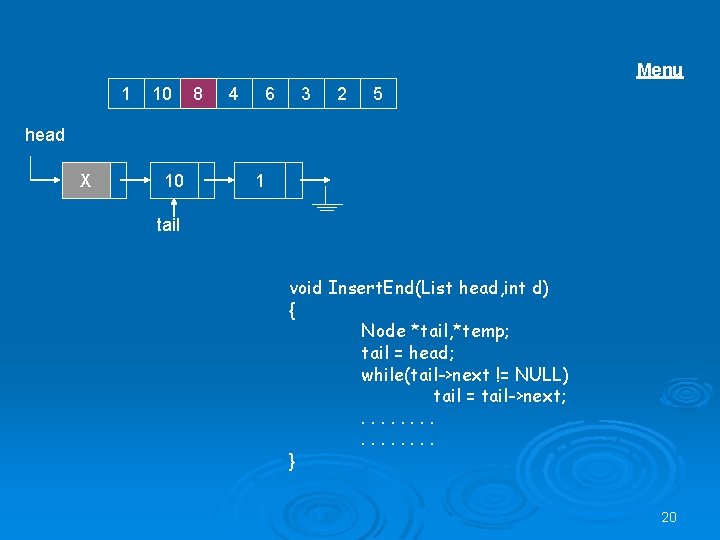
Menu 1 10 8 4 6 3 2 5 head X 10 1 tail void Insert. End(List head, int d) { Node *tail, *temp; tail = head; while(tail->next != NULL) tail = tail->next; . . . . } 20
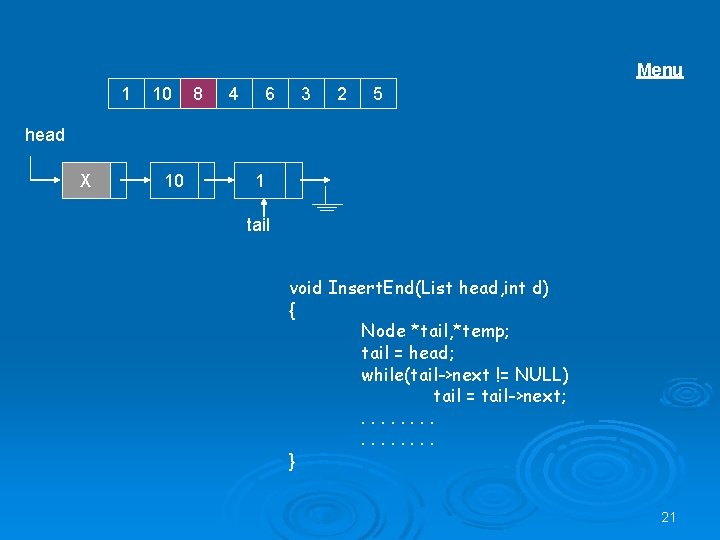
Menu 1 10 8 4 6 3 2 5 head X 10 1 tail void Insert. End(List head, int d) { Node *tail, *temp; tail = head; while(tail->next != NULL) tail = tail->next; . . . . } 21
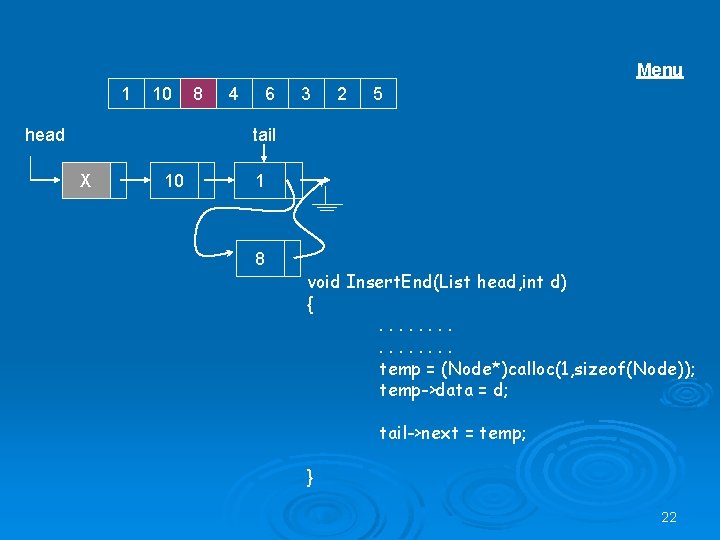
Menu 1 10 head 8 4 6 3 2 5 tail X 10 1 8 void Insert. End(List head, int d) {. . . . temp = (Node*)calloc(1, sizeof(Node)); temp->data = d; tail->next = temp; } 22
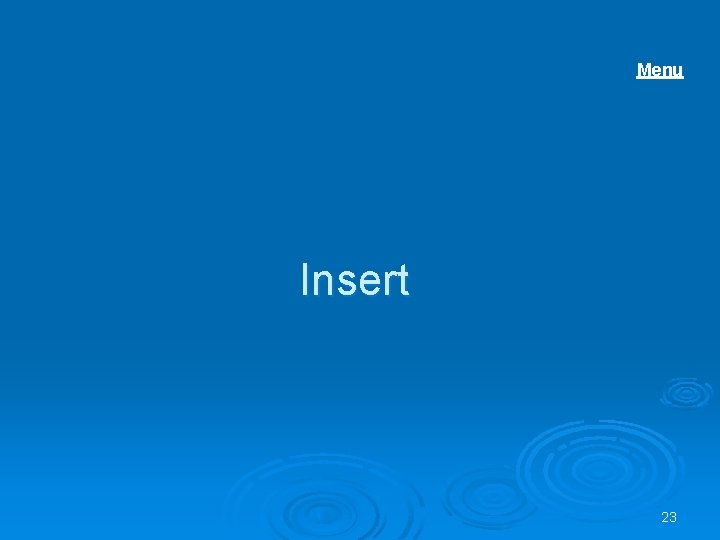
Menu Insert 23
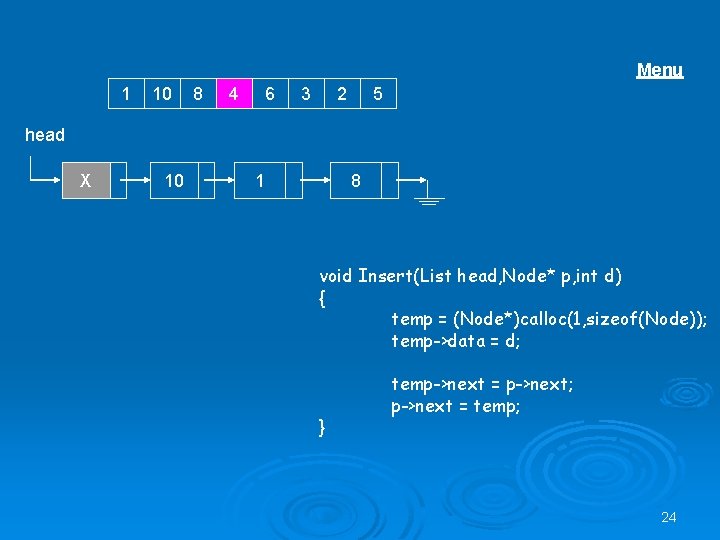
Menu 1 10 8 4 6 3 2 5 head X 10 1 8 void Insert(List head, Node* p, int d) { temp = (Node*)calloc(1, sizeof(Node)); temp->data = d; } temp->next = p->next; p->next = temp; 24
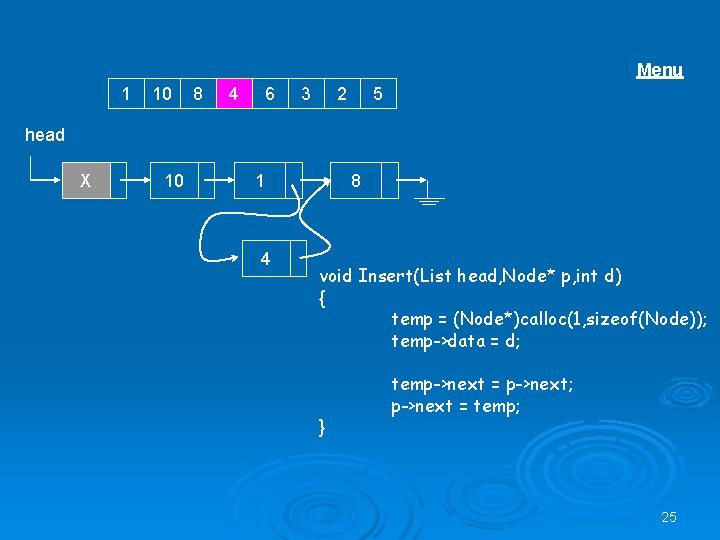
Menu 1 10 8 4 6 3 2 5 head X 10 1 4 8 void Insert(List head, Node* p, int d) { temp = (Node*)calloc(1, sizeof(Node)); temp->data = d; } temp->next = p->next; p->next = temp; 25
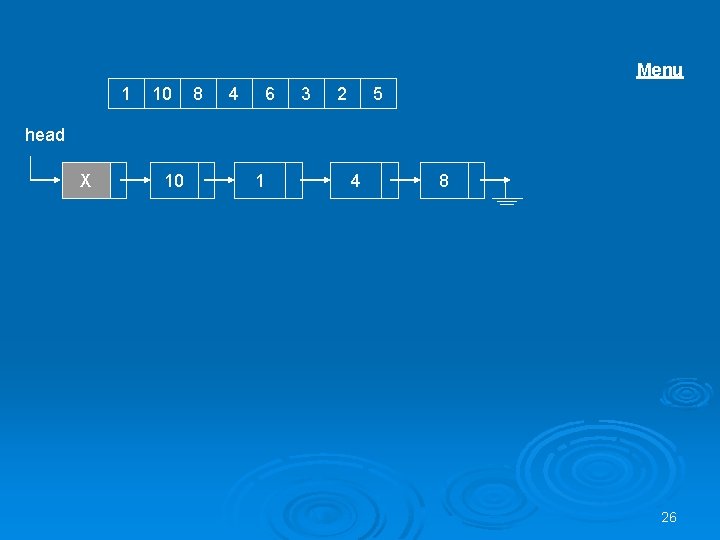
Menu 1 10 8 4 6 3 2 5 head X 10 1 4 8 26
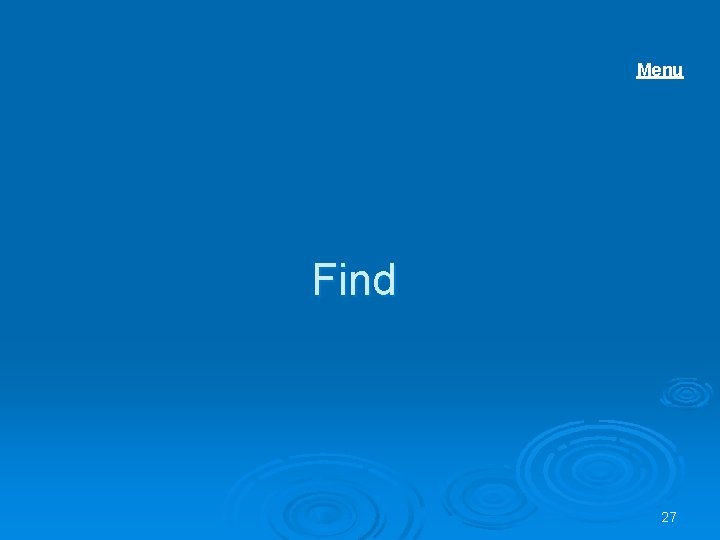
Menu Find 27
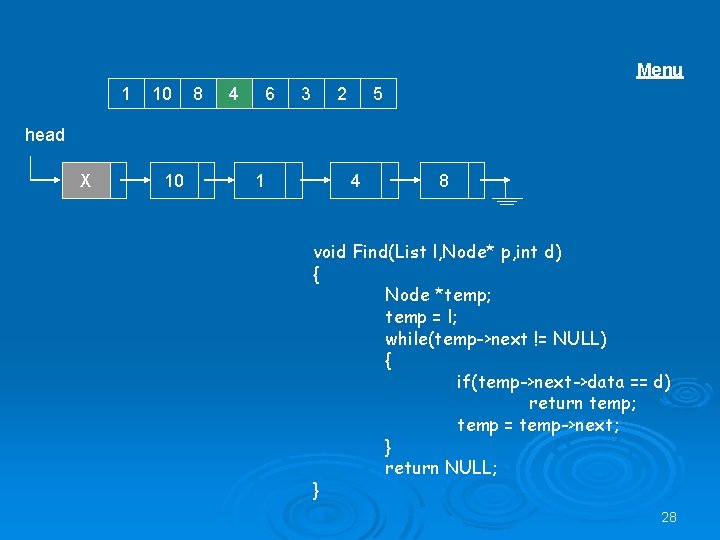
Menu 1 10 8 4 6 3 2 5 head X 10 1 4 8 void Find(List l, Node* p, int d) { Node *temp; temp = l; while(temp->next != NULL) { if(temp->next->data == d) return temp; temp = temp->next; } return NULL; } 28
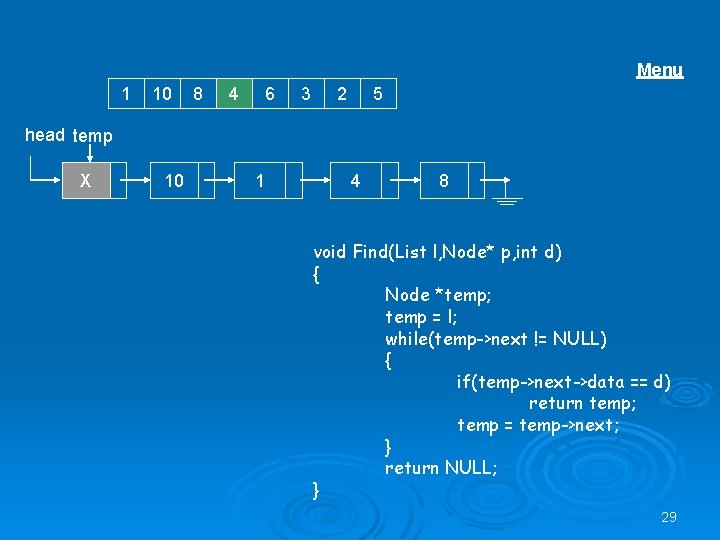
Menu 1 10 8 4 6 3 2 5 head temp X 10 1 4 8 void Find(List l, Node* p, int d) { Node *temp; temp = l; while(temp->next != NULL) { if(temp->next->data == d) return temp; temp = temp->next; } return NULL; } 29
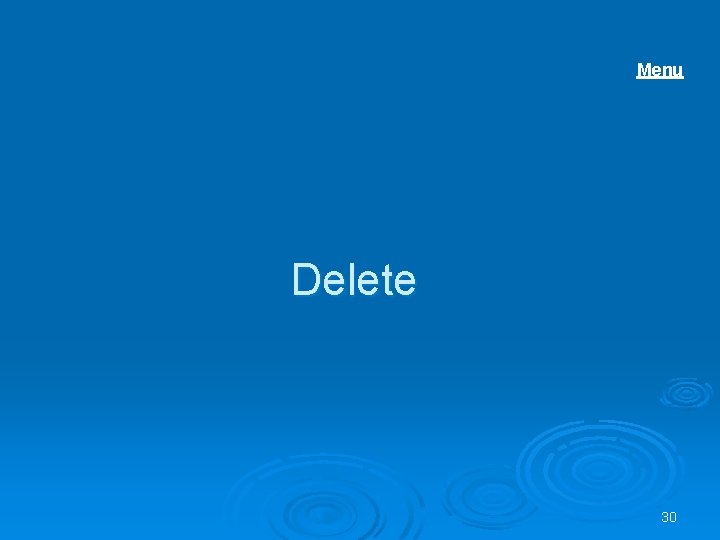
Menu Delete 30
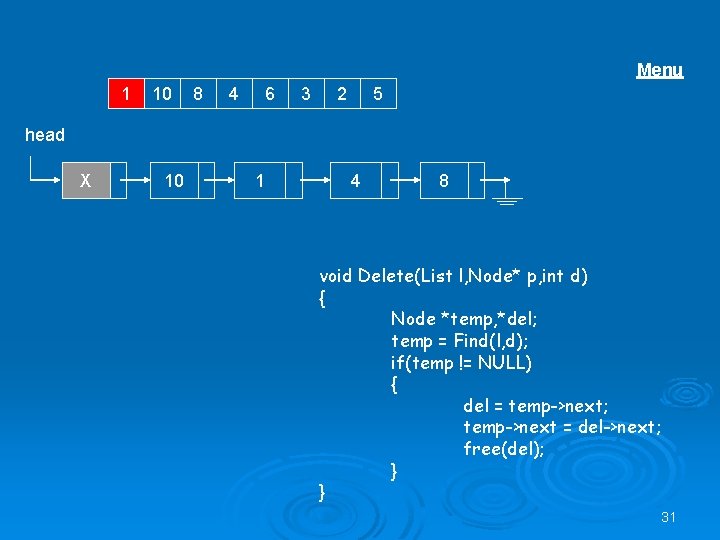
Menu 1 10 8 4 6 3 2 5 head X 10 1 4 8 void Delete(List l, Node* p, int d) { Node *temp, *del; temp = Find(l, d); if(temp != NULL) { del = temp->next; temp->next = del->next; free(del); } } 31
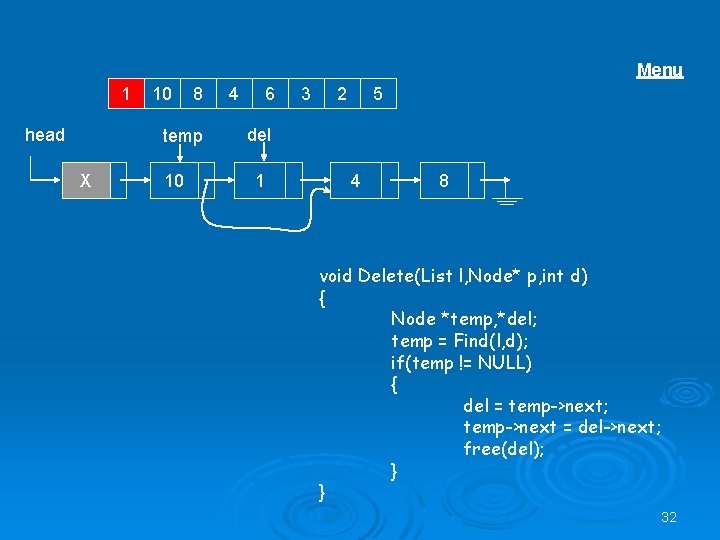
Menu 1 head 10 8 temp X 10 4 6 3 2 5 del 1 4 8 void Delete(List l, Node* p, int d) { Node *temp, *del; temp = Find(l, d); if(temp != NULL) { del = temp->next; temp->next = del->next; free(del); } } 32
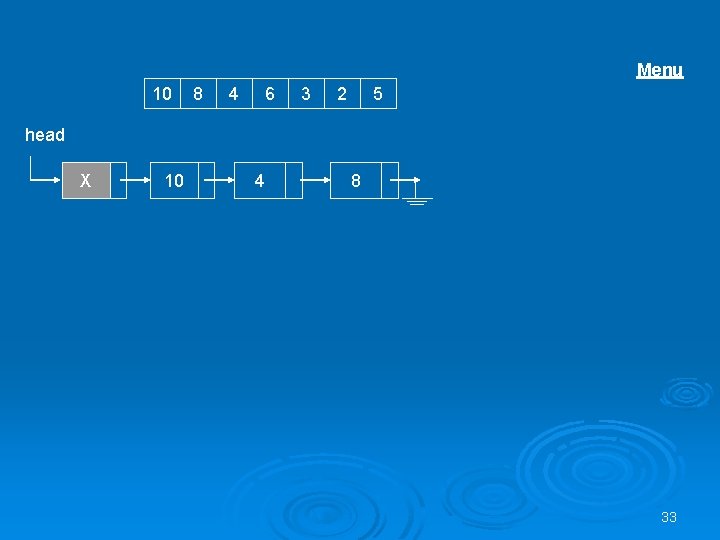
Menu 10 8 4 6 3 2 5 head X 10 4 8 33
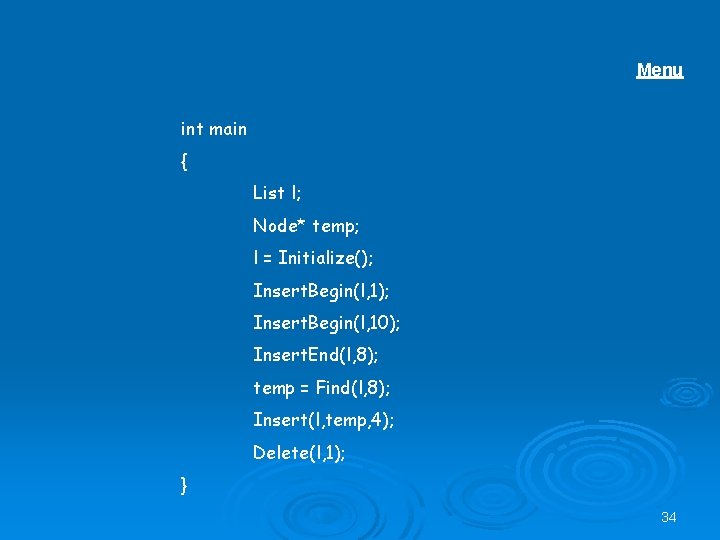
Menu int main { List l; Node* temp; l = Initialize(); Insert. Begin(l, 10); Insert. End(l, 8); temp = Find(l, 8); Insert(l, temp, 4); Delete(l, 1); } 34
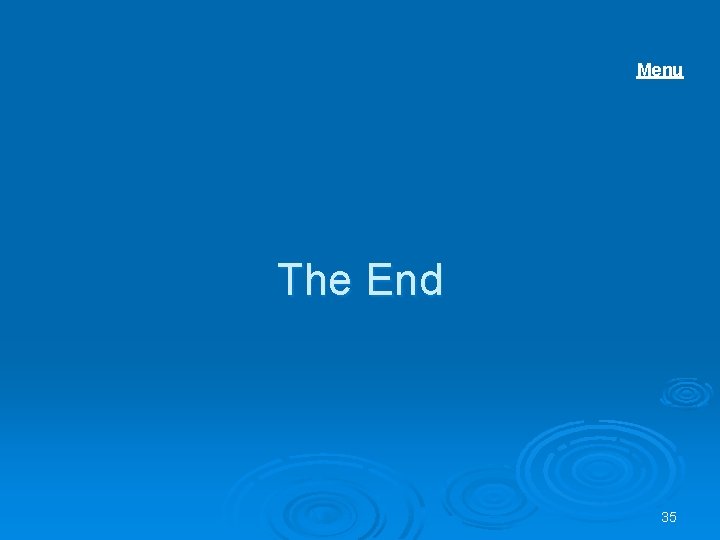
Menu The End 35
Struct node int data struct node* next
Sum0
Struct node int data
Typedef struct tree int info
Int tree
Typedef struct node
Typedef struct in c
Struct node int i float j
What are structure
Nodenext
Int max(int x int y)
Interface calculator public int add(int a int b) class test
Public void drawsquare(int x, int y, int len)
Public int divide(int a int b)
C copy struct
Typedef struct in c linked list
Singly linked list vs doubly linked list
Introduction to linked list
Fungsi linked list
Typedef node
Struct point int x y
Struct point int x y
Struct sample int a=0
Struct node *next
Typedef struct node
Typedef list
Struct node *
Circular header list
Linked list remove first node
Interface myinterface int foo(int x)
Int main int argc char argv
7팩토리얼
Arduino const
Int main() int num=4
Voidswap