Summary on linked lists Definition struct Node int
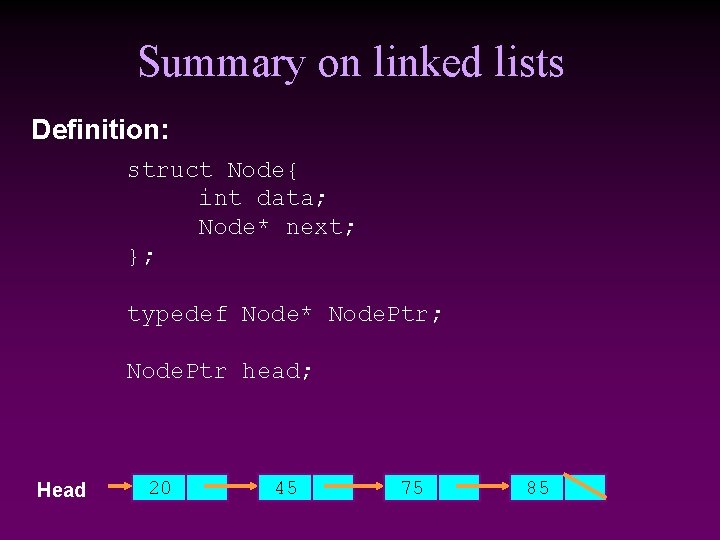
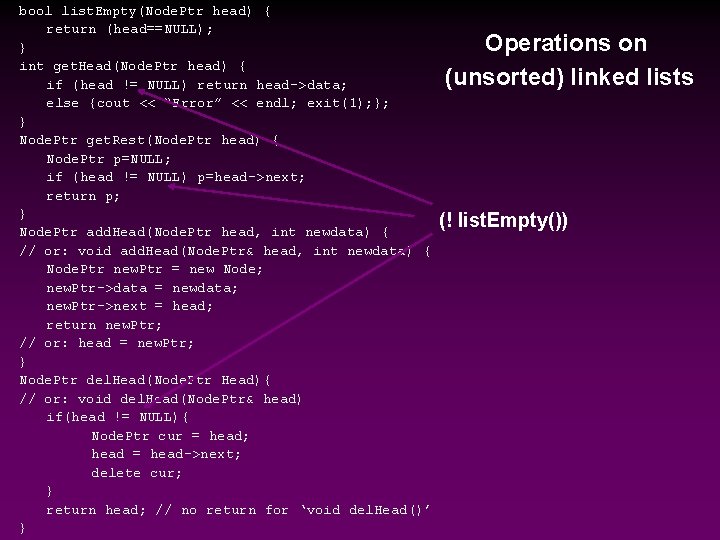
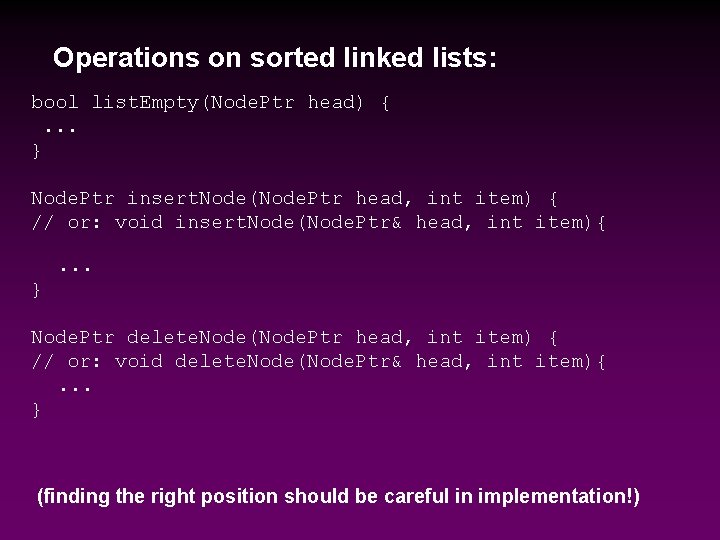
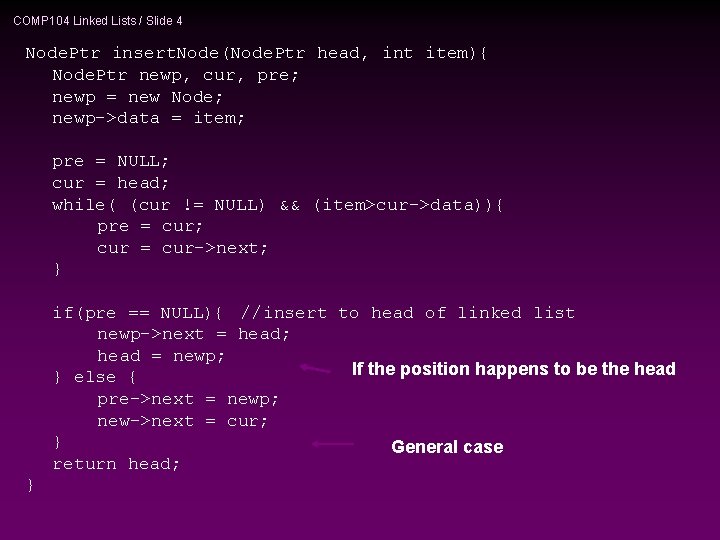
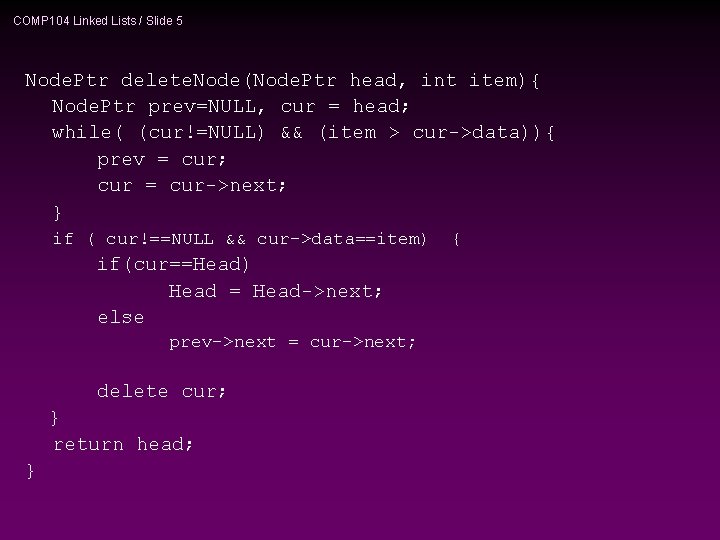
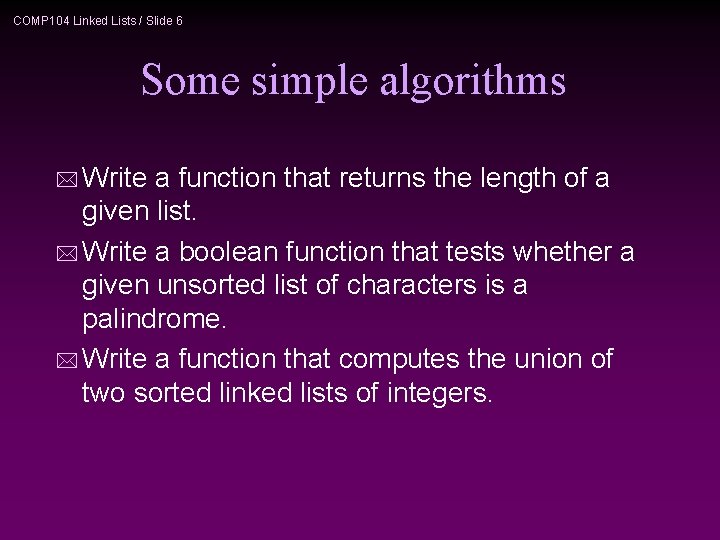
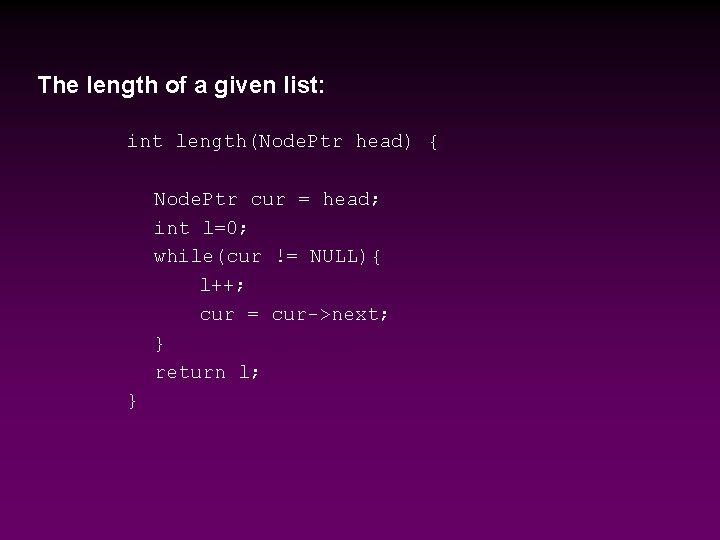
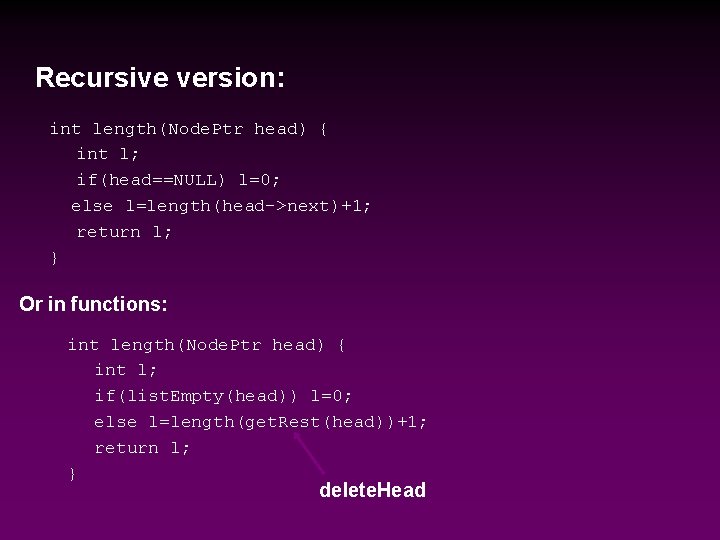
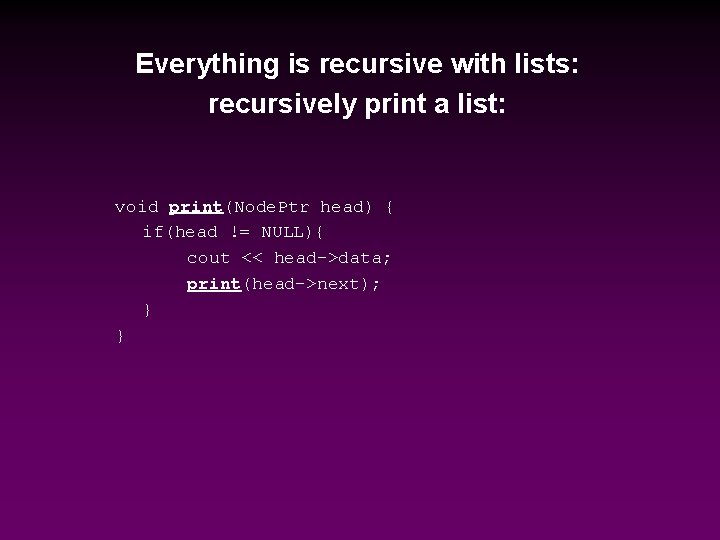
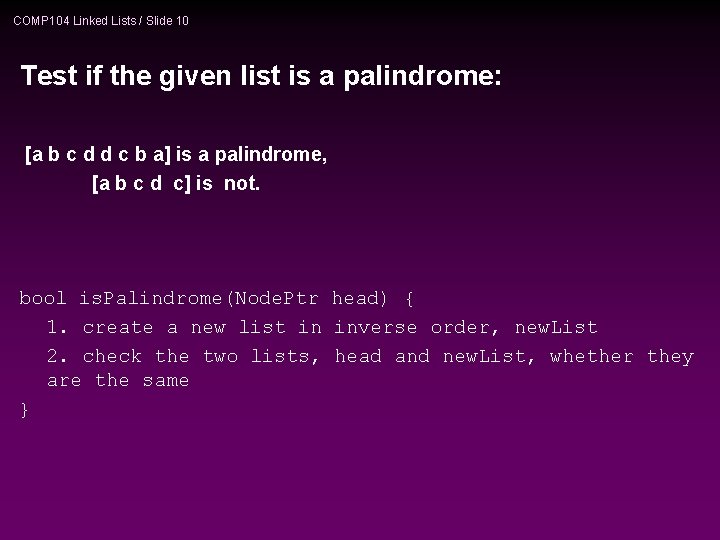
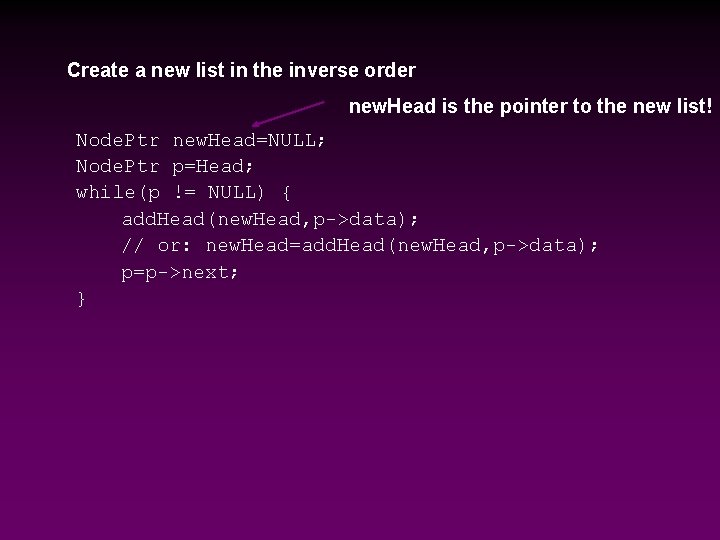
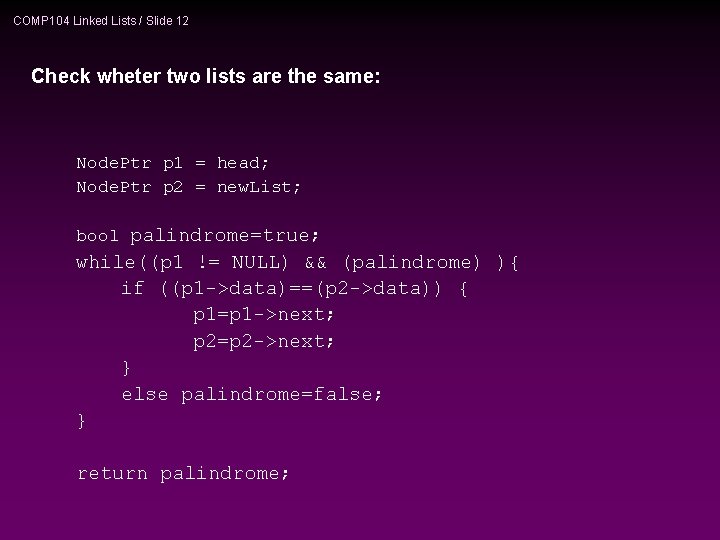
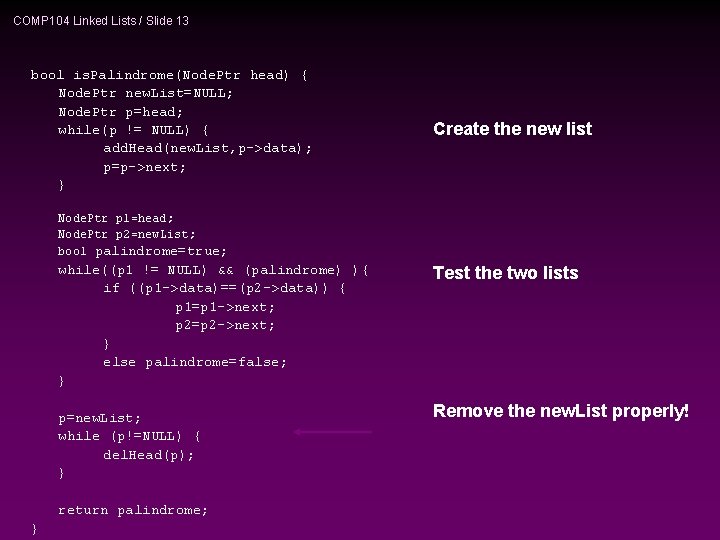
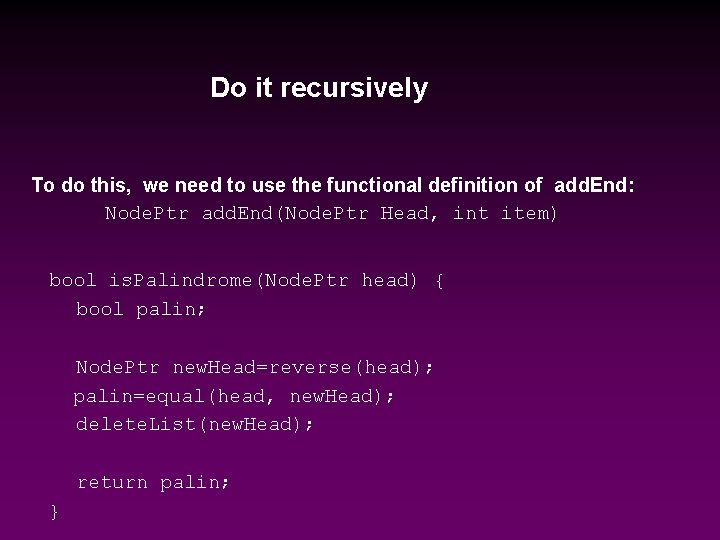
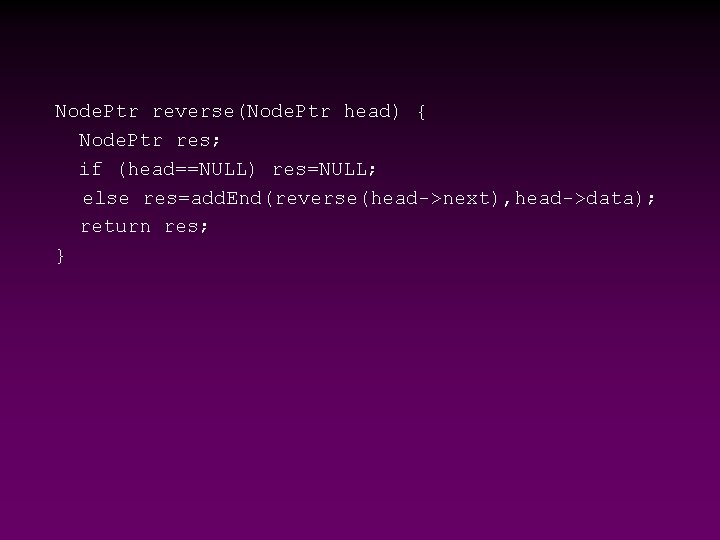
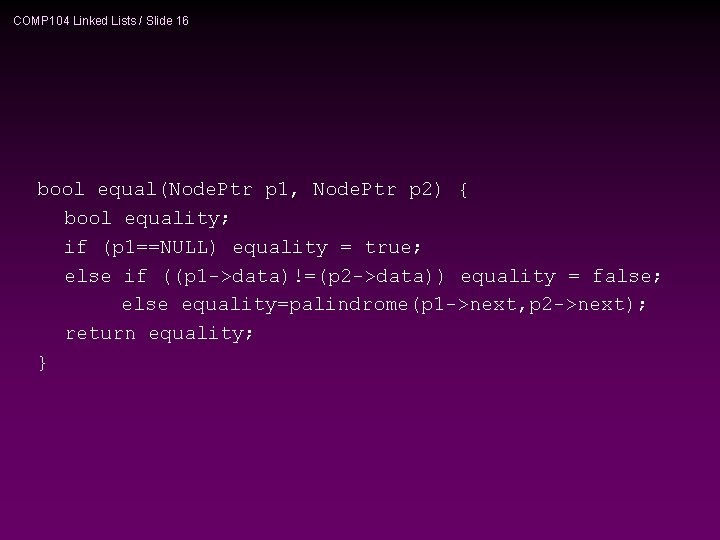
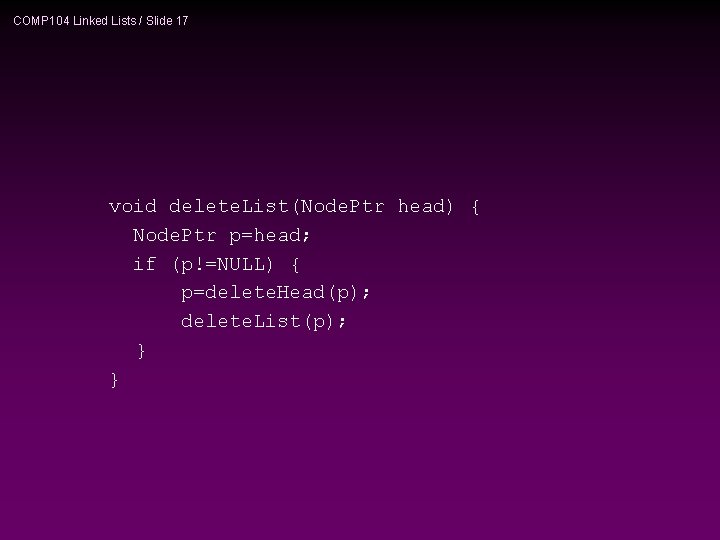
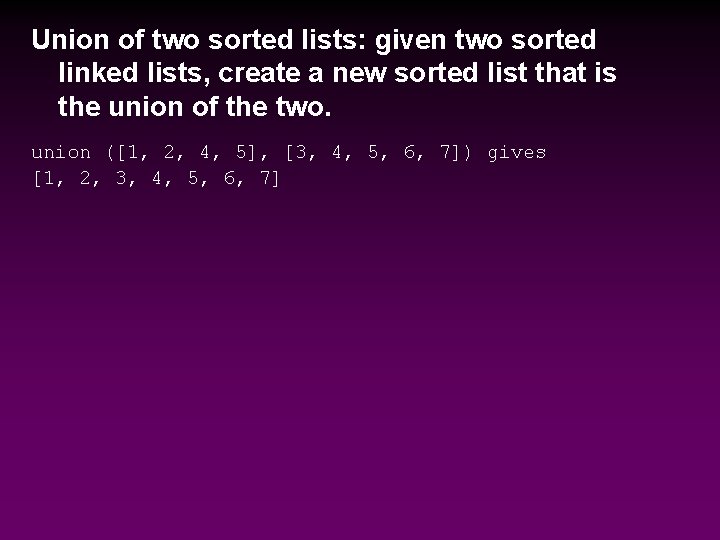
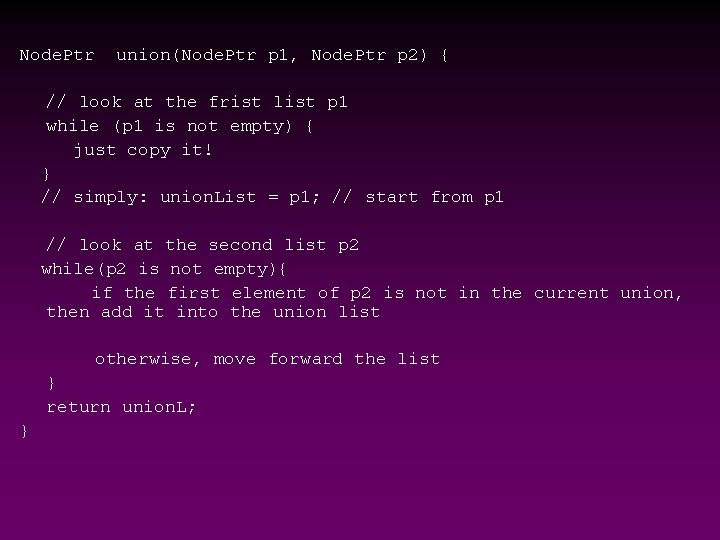
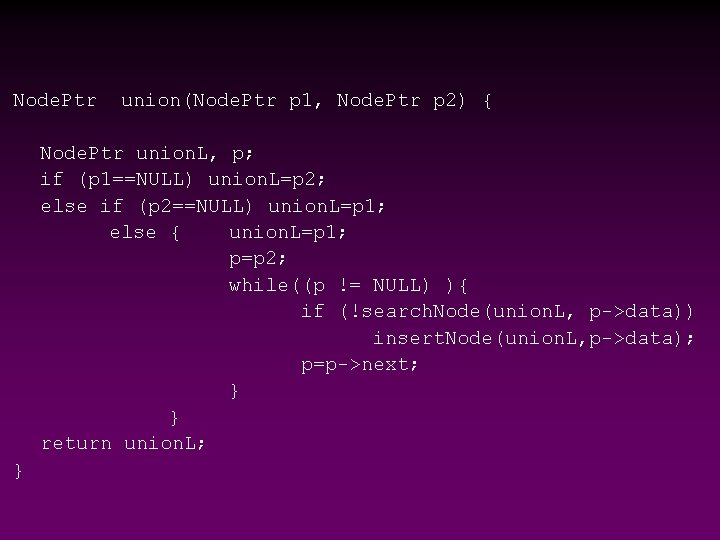
- Slides: 20
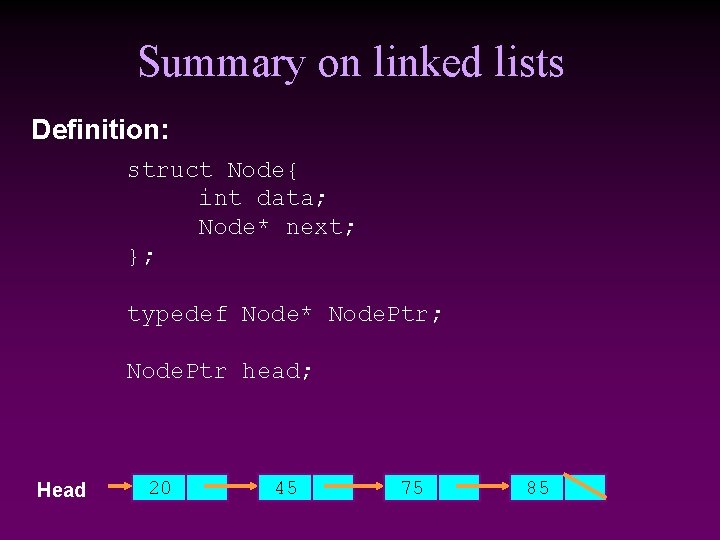
Summary on linked lists Definition: struct Node{ int data; Node* next; }; typedef Node* Node. Ptr; Node. Ptr head; Head 20 45 75 85
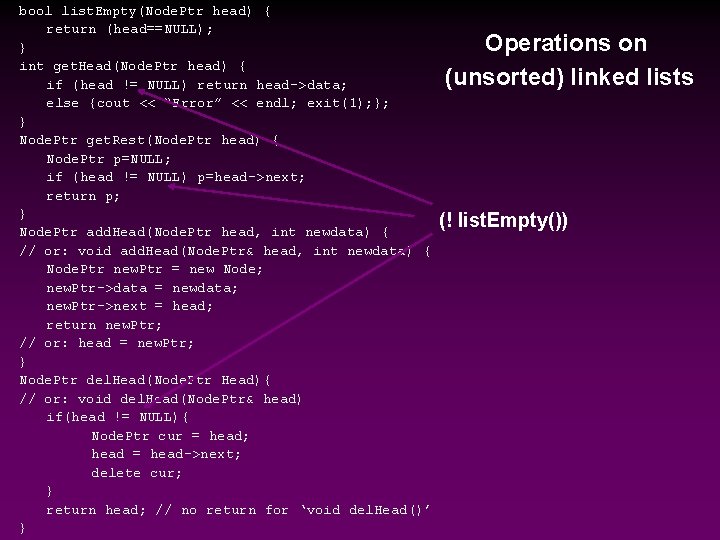
bool list. Empty(Node. Ptr head) { return (head==NULL); } int get. Head(Node. Ptr head) { if (head != NULL) return head->data; else {cout << “Error” << endl; exit(1); }; } Node. Ptr get. Rest(Node. Ptr head) { Node. Ptr p=NULL; if (head != NULL) p=head->next; return p; } Node. Ptr add. Head(Node. Ptr head, int newdata) { // or: void add. Head(Node. Ptr& head, int newdata) { Node. Ptr new. Ptr = new Node; new. Ptr->data = newdata; new. Ptr->next = head; return new. Ptr; // or: head = new. Ptr; } Node. Ptr del. Head(Node. Ptr Head){ // or: void del. Head(Node. Ptr& head) if(head != NULL){ Node. Ptr cur = head; head = head->next; delete cur; } return head; // no return for ‘void del. Head()’ } Operations on (unsorted) linked lists (! list. Empty())
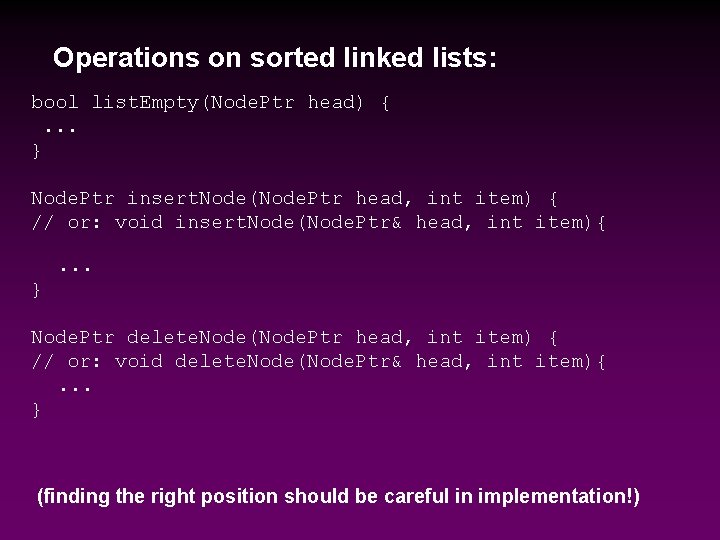
Operations on sorted linked lists: bool list. Empty(Node. Ptr head) {. . . } Node. Ptr insert. Node(Node. Ptr head, int item) { // or: void insert. Node(Node. Ptr& head, int item){. . . } Node. Ptr delete. Node(Node. Ptr head, int item) { // or: void delete. Node(Node. Ptr& head, int item){. . . } (finding the right position should be careful in implementation!)
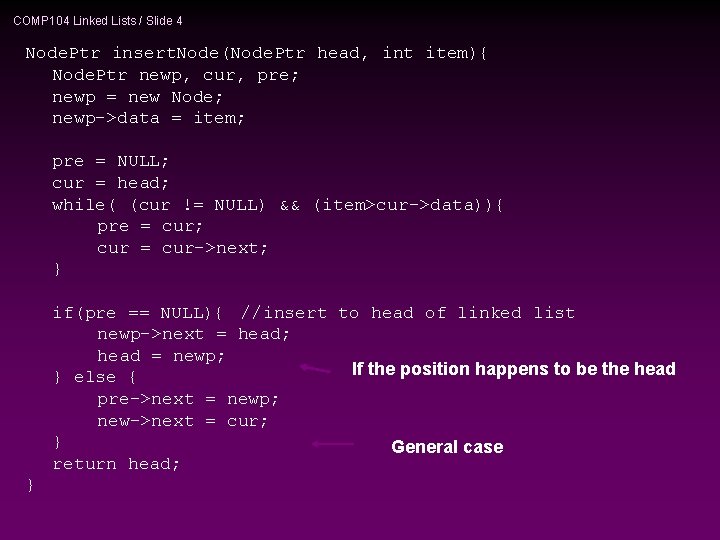
COMP 104 Linked Lists / Slide 4 Node. Ptr insert. Node(Node. Ptr head, int item){ Node. Ptr newp, cur, pre; newp = new Node; newp->data = item; pre = NULL; cur = head; while( (cur != NULL) && (item>cur->data)){ pre = cur; cur = cur->next; } if(pre == NULL){ //insert to head of linked list newp->next = head; head = newp; If the position happens to be the head } else { pre->next = newp; new->next = cur; } General case return head; }
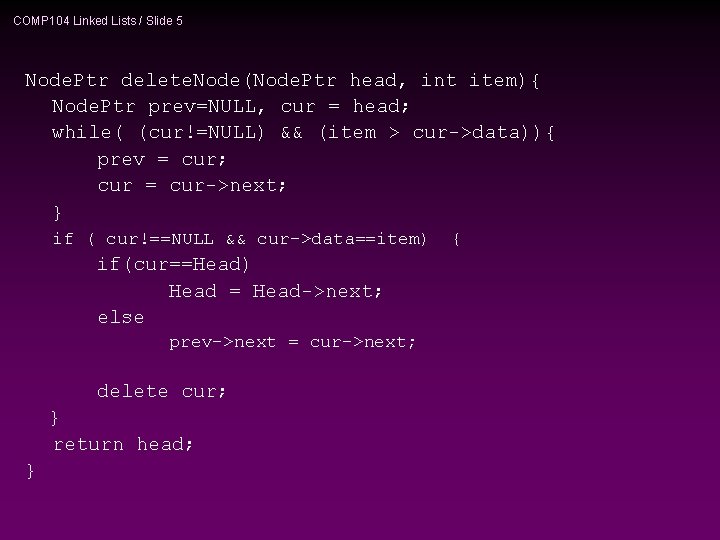
COMP 104 Linked Lists / Slide 5 Node. Ptr delete. Node(Node. Ptr head, int item){ Node. Ptr prev=NULL, cur = head; while( (cur!=NULL) && (item > cur->data)){ prev = cur; cur = cur->next; } if ( cur!==NULL && cur->data==item) if(cur==Head) Head = Head->next; else prev->next = cur->next; delete cur; } return head; } {
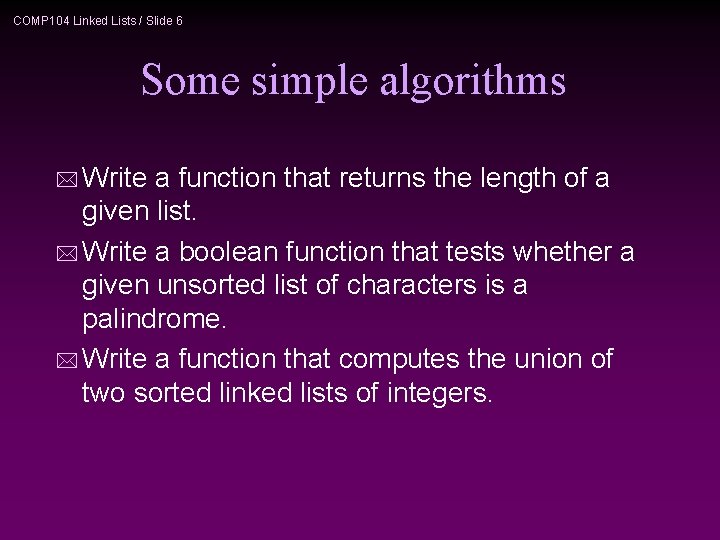
COMP 104 Linked Lists / Slide 6 Some simple algorithms * Write a function that returns the length of a given list. * Write a boolean function that tests whether a given unsorted list of characters is a palindrome. * Write a function that computes the union of two sorted linked lists of integers.
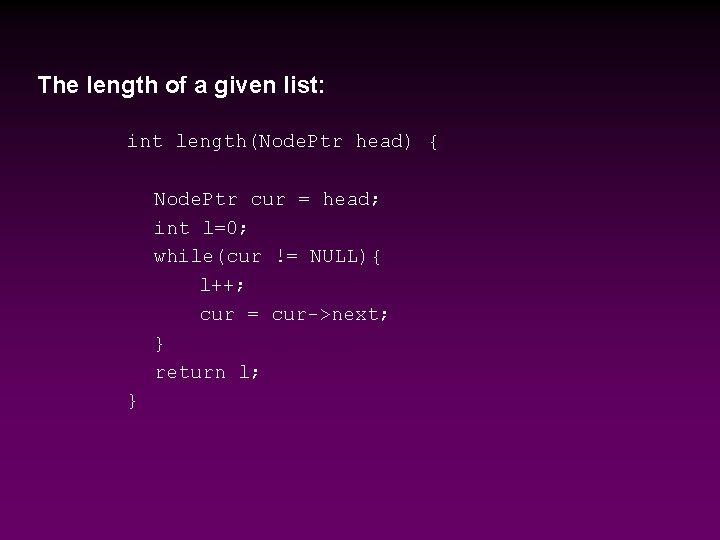
The length of a given list: int length(Node. Ptr head) { Node. Ptr cur = head; int l=0; while(cur != NULL){ l++; cur = cur->next; } return l; }
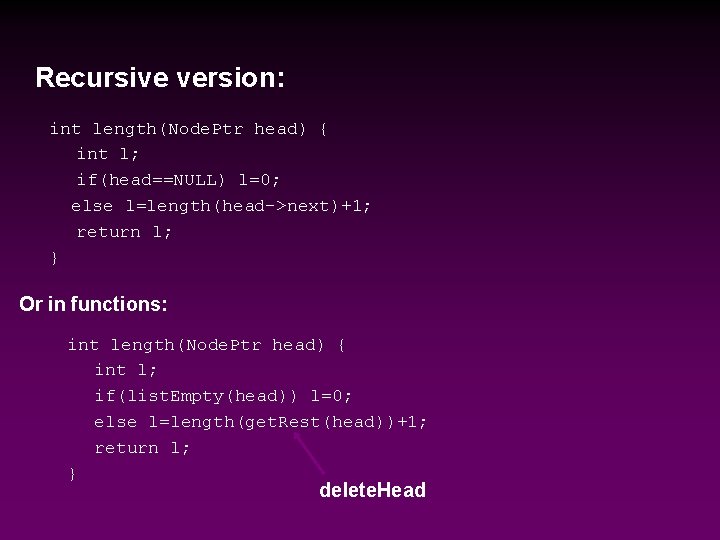
Recursive version: int length(Node. Ptr head) { int l; if(head==NULL) l=0; else l=length(head->next)+1; return l; } Or in functions: int length(Node. Ptr head) { int l; if(list. Empty(head)) l=0; else l=length(get. Rest(head))+1; return l; } delete. Head
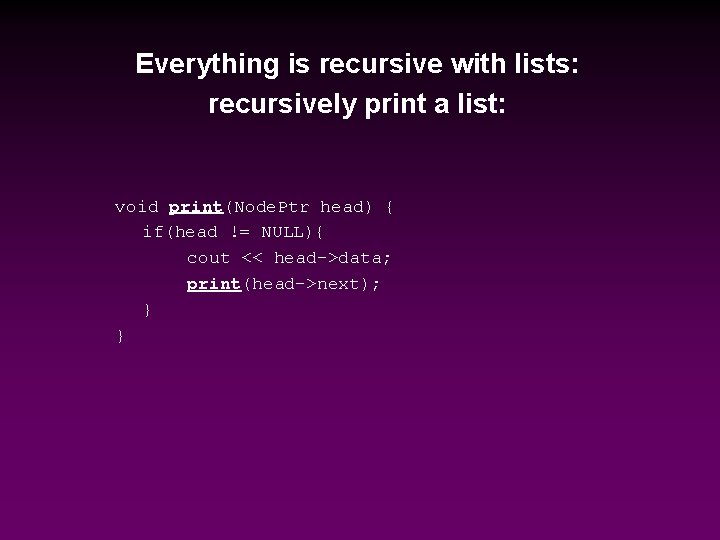
Everything is recursive with lists: recursively print a list: void print(Node. Ptr head) { if(head != NULL){ cout << head->data; print(head->next); } }
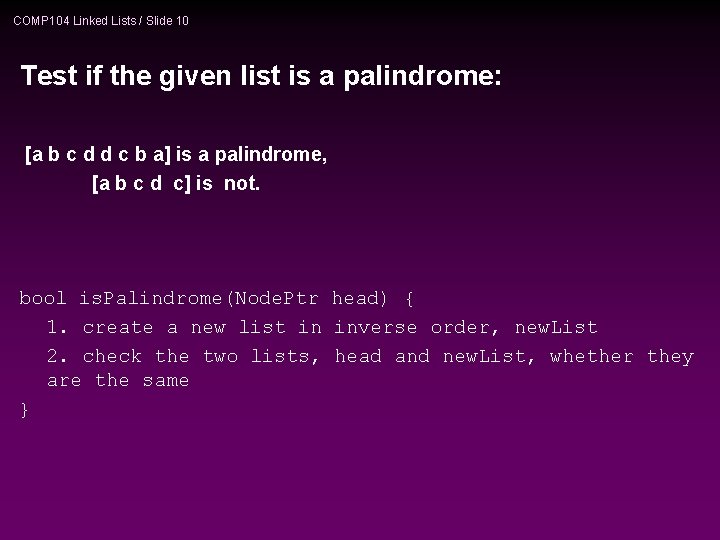
COMP 104 Linked Lists / Slide 10 Test if the given list is a palindrome: [a b c d d c b a] is a palindrome, [a b c d c] is not. bool is. Palindrome(Node. Ptr head) { 1. create a new list in inverse order, new. List 2. check the two lists, head and new. List, whether they are the same }
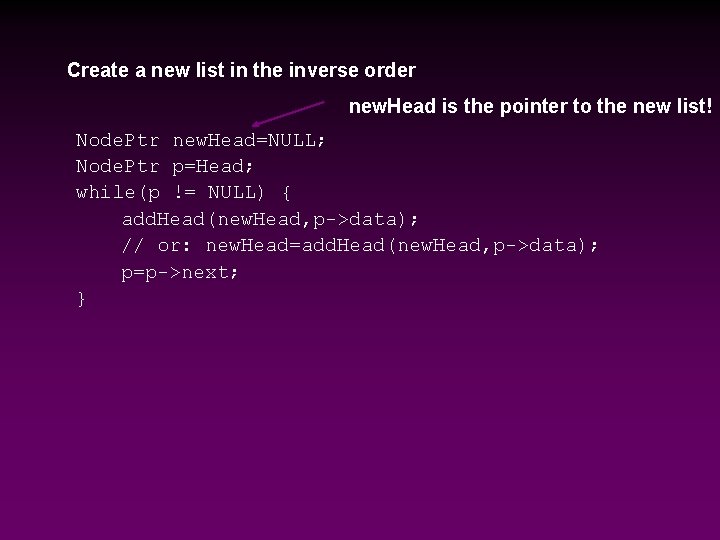
Create a new list in the inverse order new. Head is the pointer to the new list! Node. Ptr new. Head=NULL; Node. Ptr p=Head; while(p != NULL) { add. Head(new. Head, p->data); // or: new. Head=add. Head(new. Head, p->data); p=p->next; }
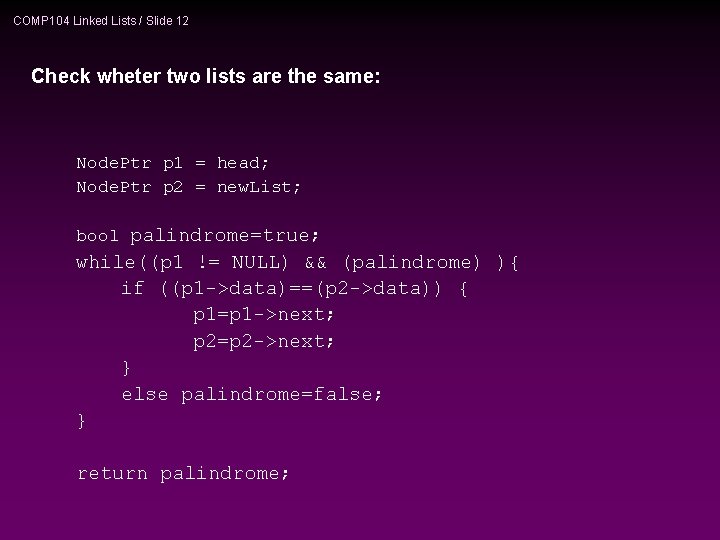
COMP 104 Linked Lists / Slide 12 Check wheter two lists are the same: Node. Ptr p 1 = head; Node. Ptr p 2 = new. List; bool palindrome=true; while((p 1 != NULL) && (palindrome) ){ if ((p 1 ->data)==(p 2 ->data)) { p 1=p 1 ->next; p 2=p 2 ->next; } else palindrome=false; } return palindrome;
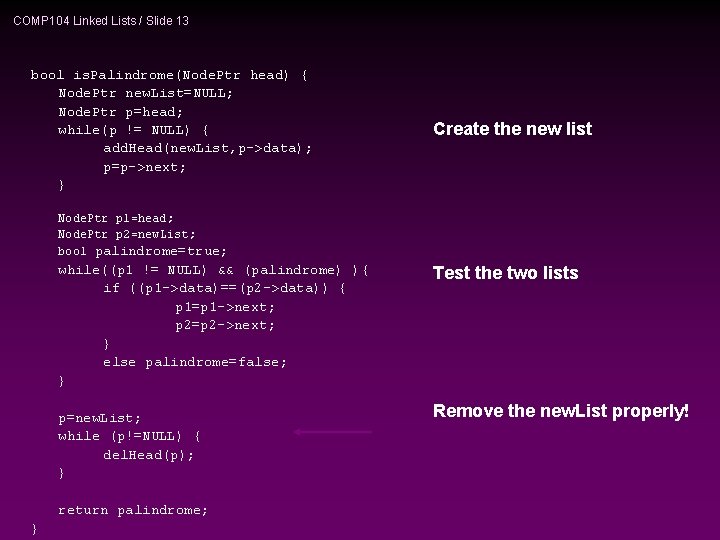
COMP 104 Linked Lists / Slide 13 bool is. Palindrome(Node. Ptr head) { Node. Ptr new. List=NULL; Node. Ptr p=head; while(p != NULL) { add. Head(new. List, p->data); p=p->next; } Create the new list Node. Ptr p 1=head; Node. Ptr p 2=new. List; bool palindrome=true; while((p 1 != NULL) && (palindrome) ){ if ((p 1 ->data)==(p 2 ->data)) { p 1=p 1 ->next; p 2=p 2 ->next; } else palindrome=false; } p=new. List; while (p!=NULL) { del. Head(p); } return palindrome; } Test the two lists Remove the new. List properly!
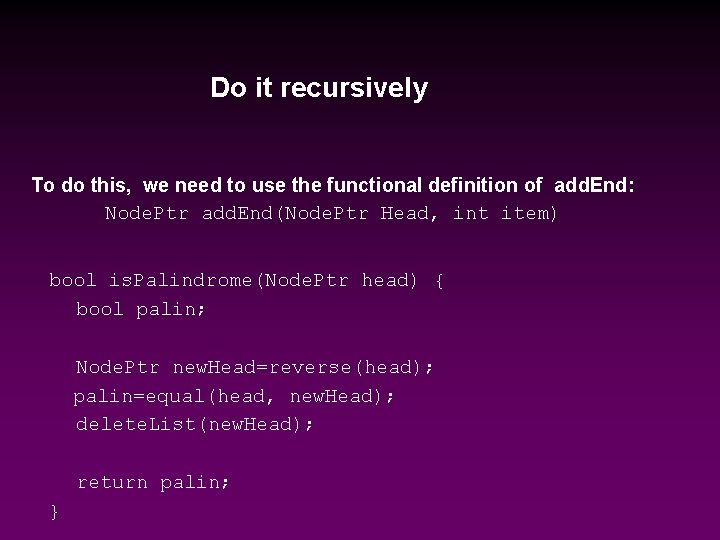
Do it recursively To do this, we need to use the functional definition of add. End: Node. Ptr add. End(Node. Ptr Head, int item) bool is. Palindrome(Node. Ptr head) { bool palin; Node. Ptr new. Head=reverse(head); palin=equal(head, new. Head); delete. List(new. Head); return palin; }
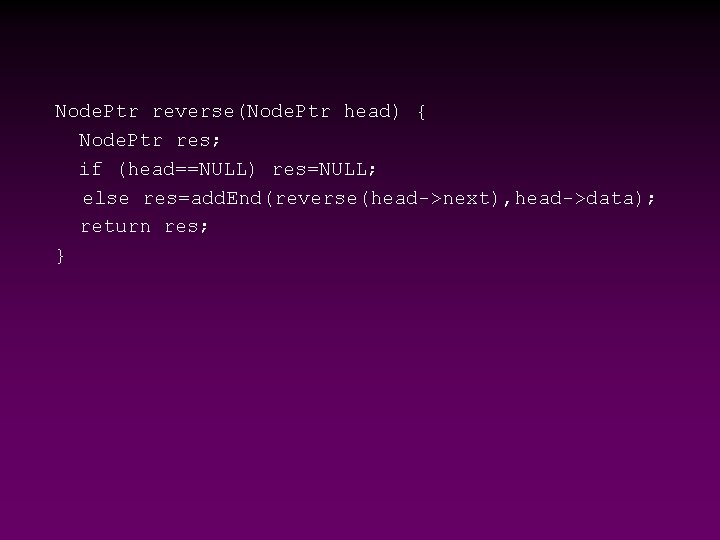
Node. Ptr reverse(Node. Ptr head) { Node. Ptr res; if (head==NULL) res=NULL; else res=add. End(reverse(head->next), head->data); return res; }
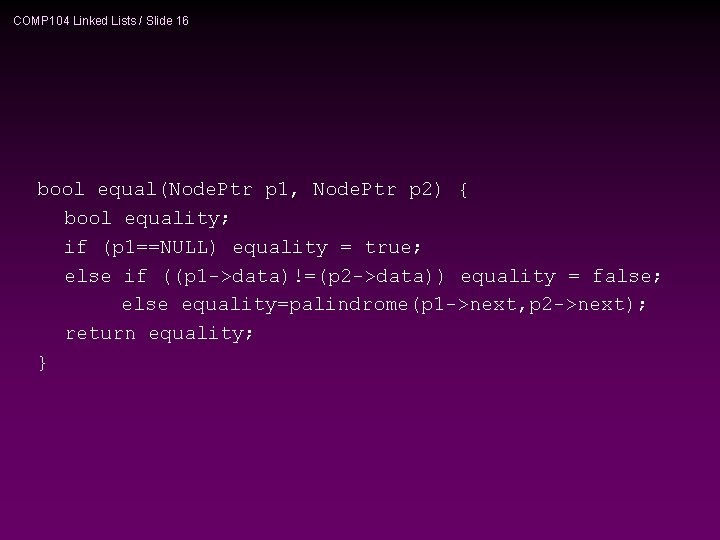
COMP 104 Linked Lists / Slide 16 bool equal(Node. Ptr p 1, Node. Ptr p 2) { bool equality; if (p 1==NULL) equality = true; else if ((p 1 ->data)!=(p 2 ->data)) equality = false; else equality=palindrome(p 1 ->next, p 2 ->next); return equality; }
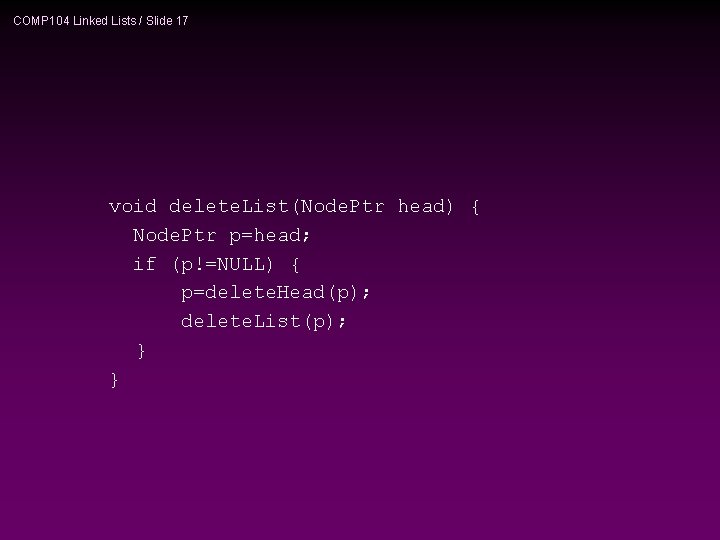
COMP 104 Linked Lists / Slide 17 void delete. List(Node. Ptr head) { Node. Ptr p=head; if (p!=NULL) { p=delete. Head(p); delete. List(p); } }
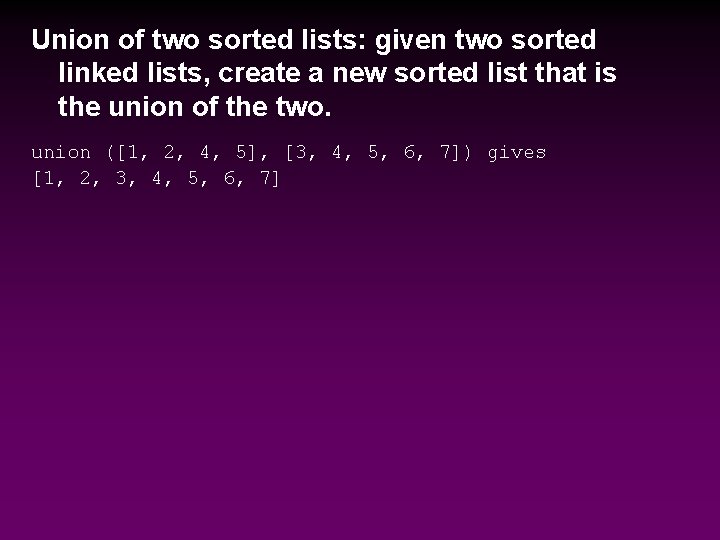
Union of two sorted lists: given two sorted linked lists, create a new sorted list that is the union of the two. union ([1, 2, 4, 5], [3, 4, 5, 6, 7]) gives [1, 2, 3, 4, 5, 6, 7]
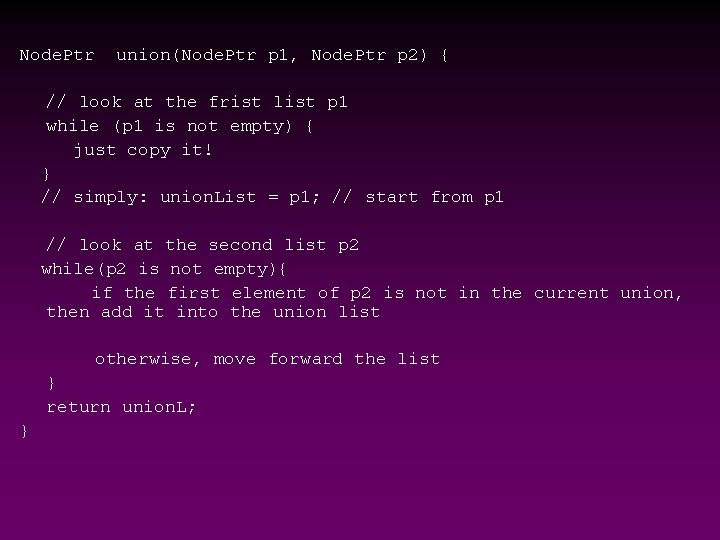
Node. Ptr union(Node. Ptr p 1, Node. Ptr p 2) { // look at the frist list p 1 while (p 1 is not empty) { just copy it! } // simply: union. List = p 1; // start from p 1 // look at the second list p 2 while(p 2 is not empty){ if the first element of p 2 is not in the current union, then add it into the union list otherwise, move forward the list } return union. L; }
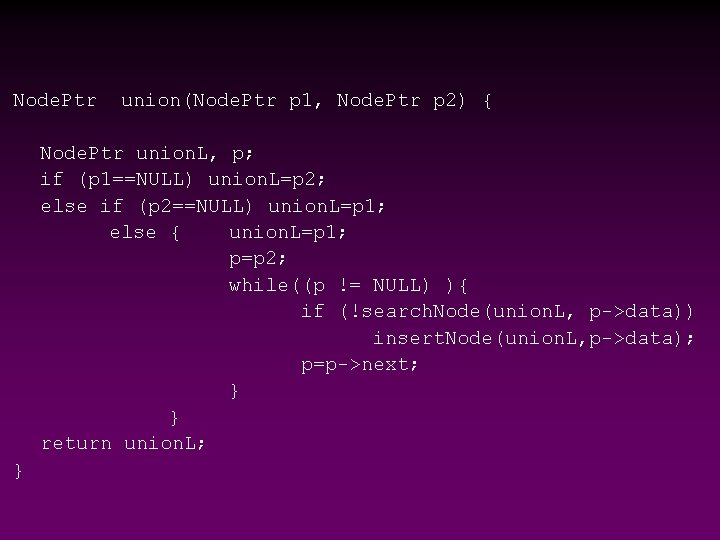
Node. Ptr union(Node. Ptr p 1, Node. Ptr p 2) { Node. Ptr union. L, p; if (p 1==NULL) union. L=p 2; else if (p 2==NULL) union. L=p 1; else { union. L=p 1; p=p 2; while((p != NULL) ){ if (!search. Node(union. L, p->data)) insert. Node(union. L, p->data); p=p->next; } } return union. L; }