Java Streams Java Streams q All modern IOs
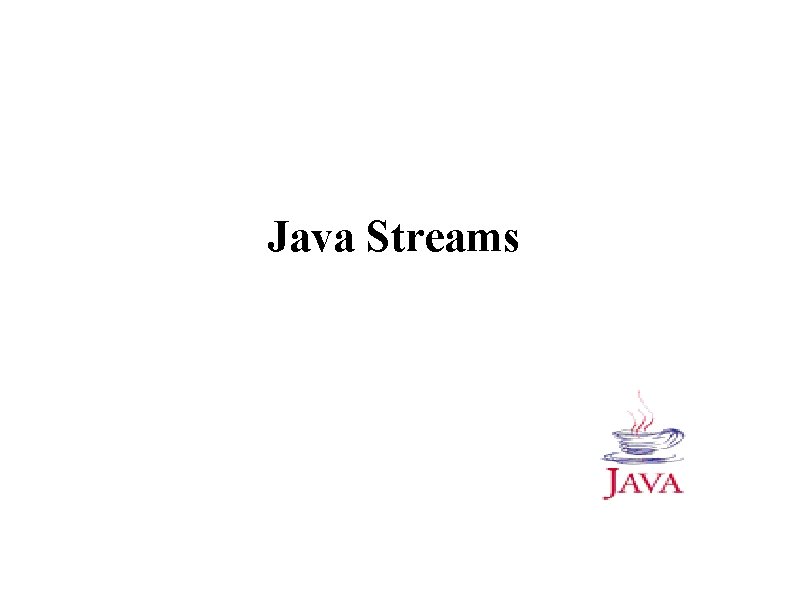
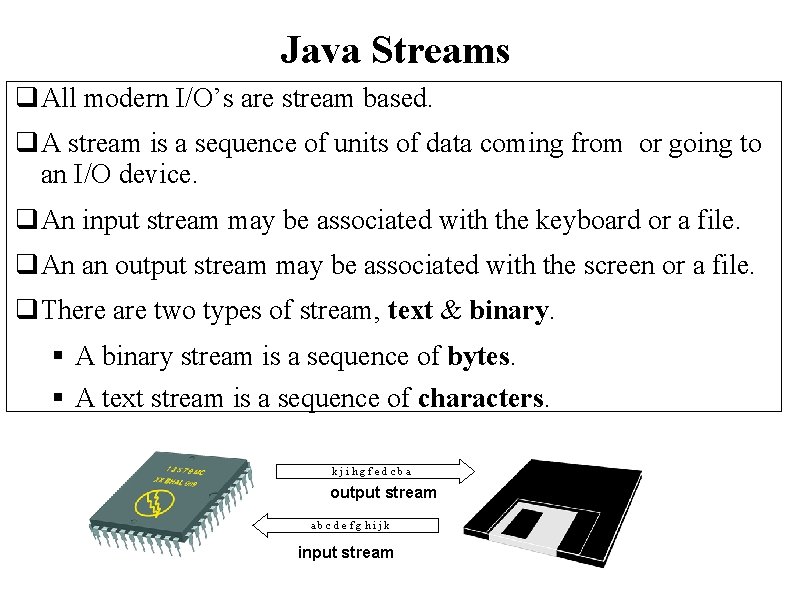
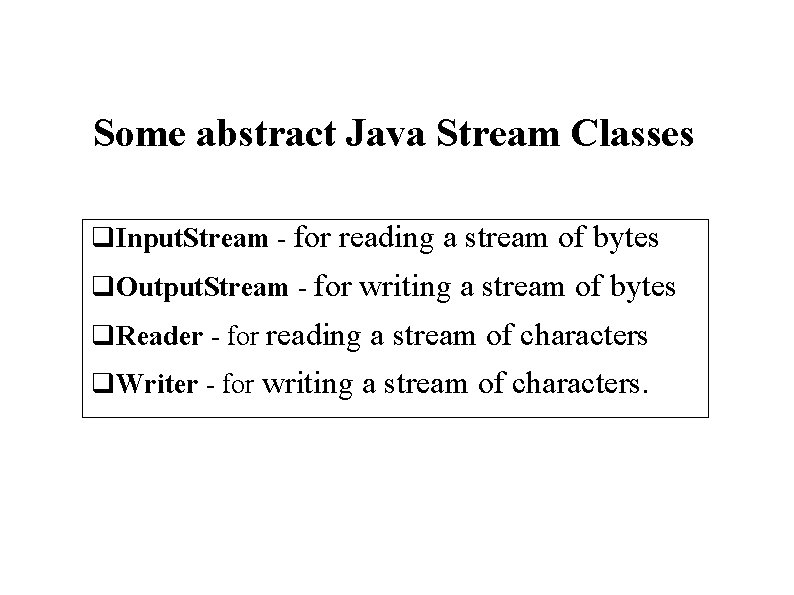
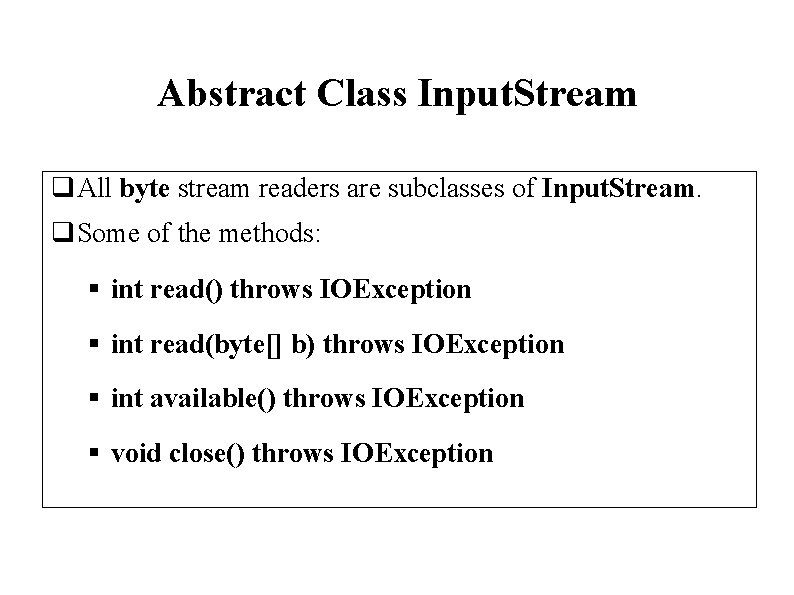
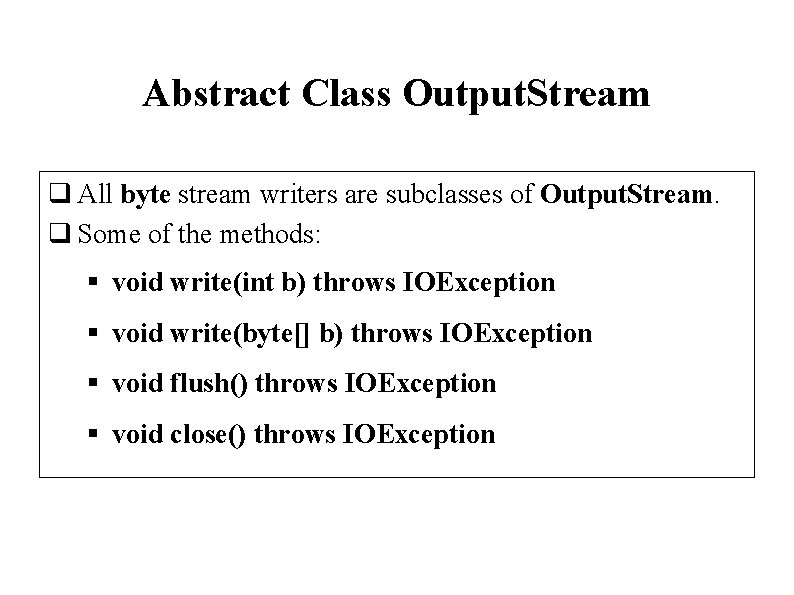
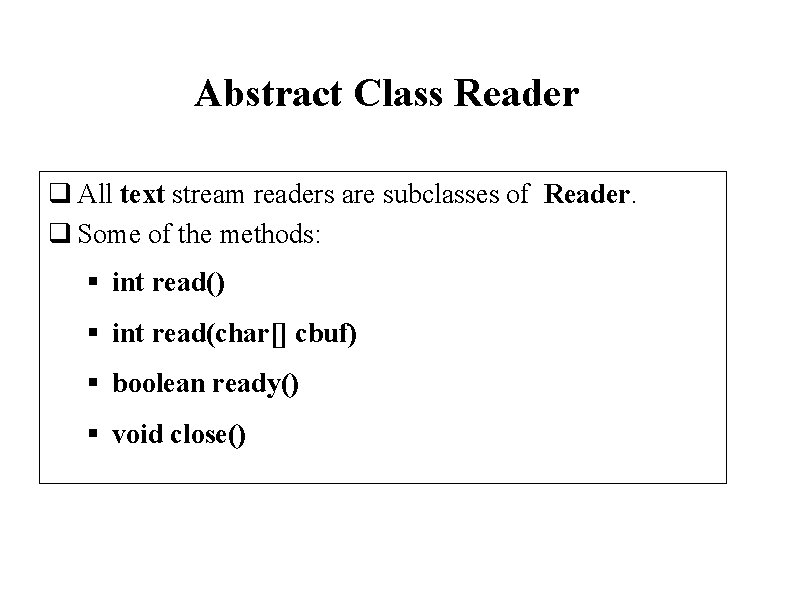
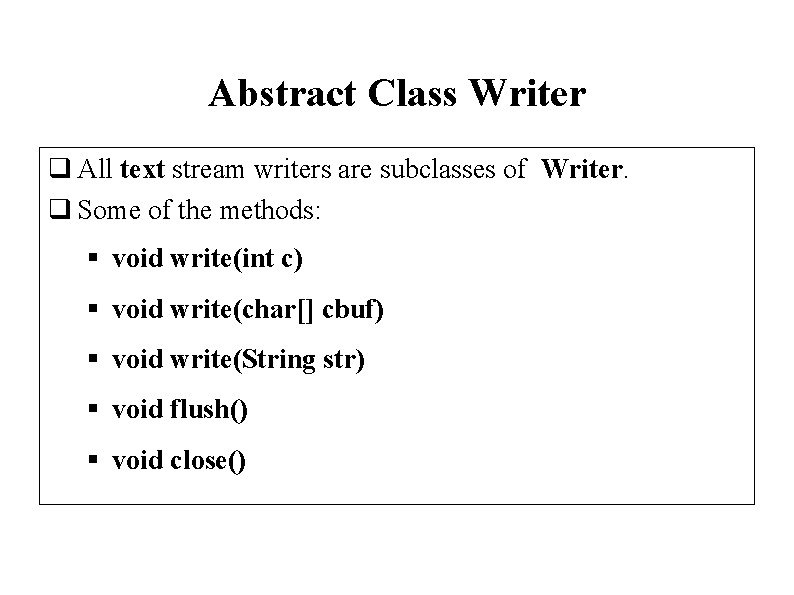
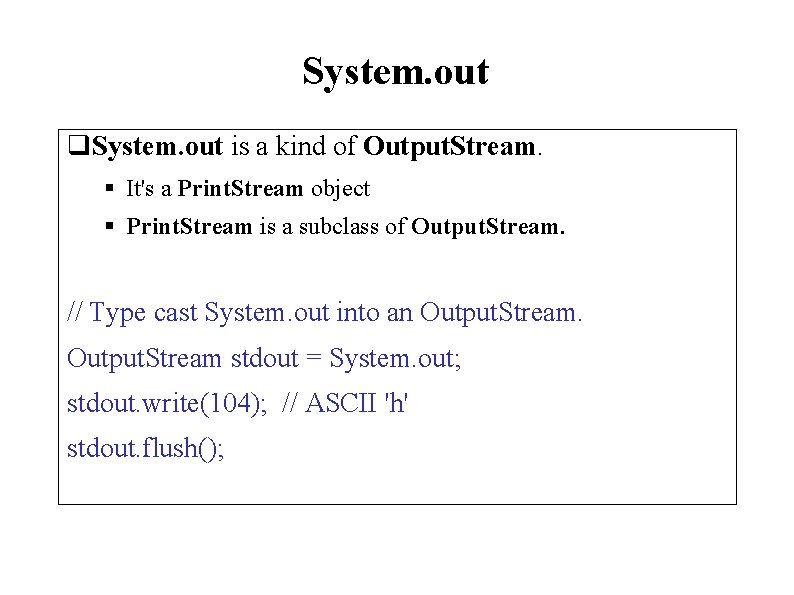
![Handle IOExceptions public static void main(String[] args) { Output. Stream stdout = System. out; Handle IOExceptions public static void main(String[] args) { Output. Stream stdout = System. out;](https://slidetodoc.com/presentation_image_h2/1f91532e981dcdf520cd44e409ec76e0/image-9.jpg)
![Another way public static void main(String[] args) throws IOException { Output. Stream stdout = Another way public static void main(String[] args) throws IOException { Output. Stream stdout =](https://slidetodoc.com/presentation_image_h2/1f91532e981dcdf520cd44e409ec76e0/image-10.jpg)
![System. in q. System. in is a type of Input. Stream. byte[] b = System. in q. System. in is a type of Input. Stream. byte[] b =](https://slidetodoc.com/presentation_image_h2/1f91532e981dcdf520cd44e409ec76e0/image-11.jpg)
![Try available() Input. Stream stdin = System. in; byte[] b = new byte[stdin. available()]; Try available() Input. Stream stdin = System. in; byte[] b = new byte[stdin. available()];](https://slidetodoc.com/presentation_image_h2/1f91532e981dcdf520cd44e409ec76e0/image-12.jpg)
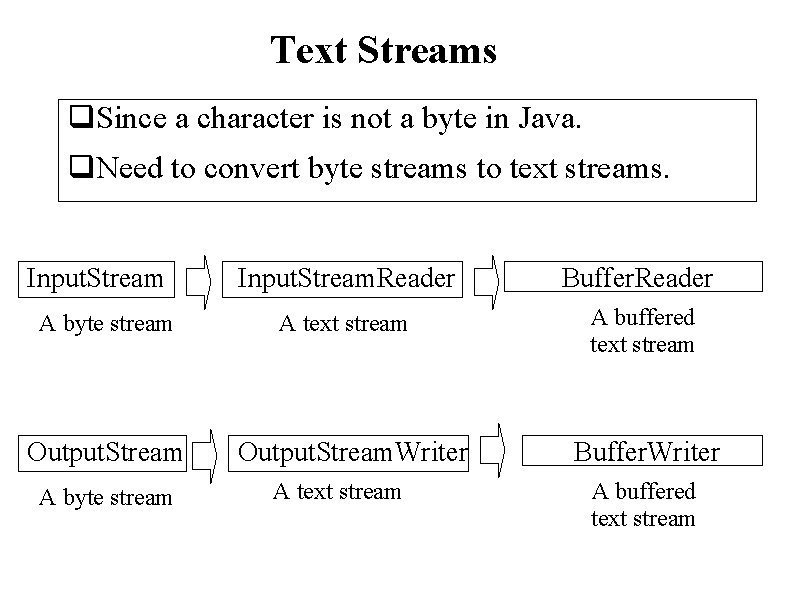
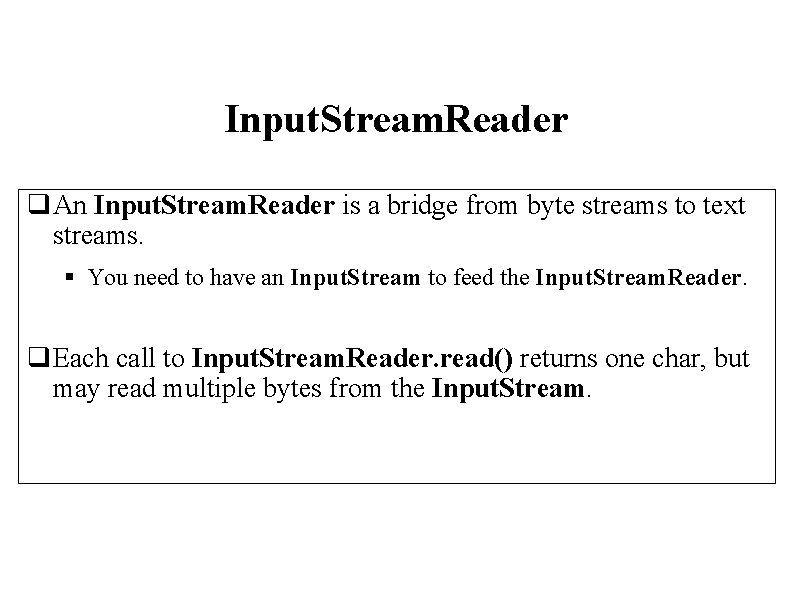
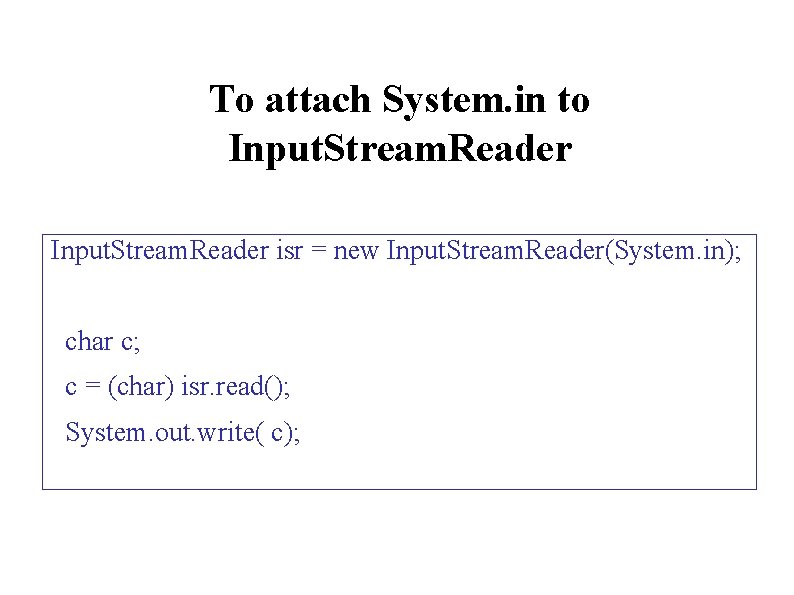
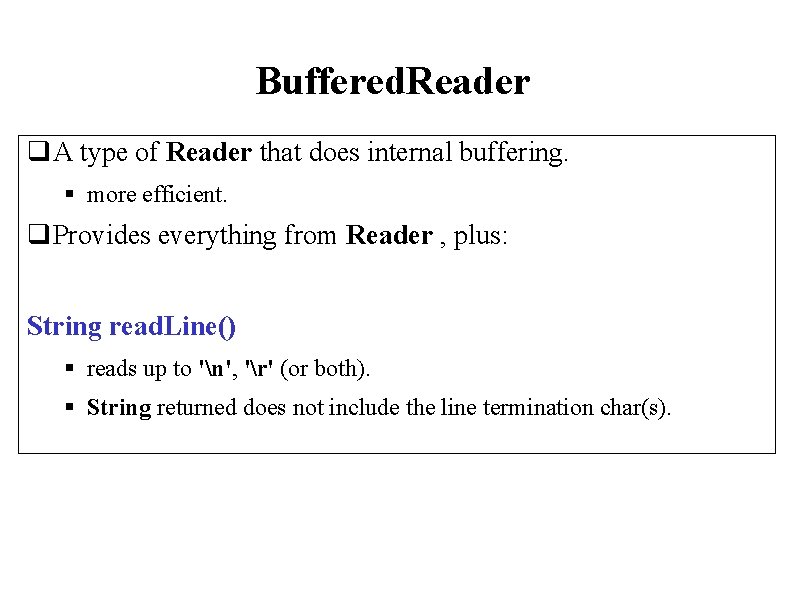
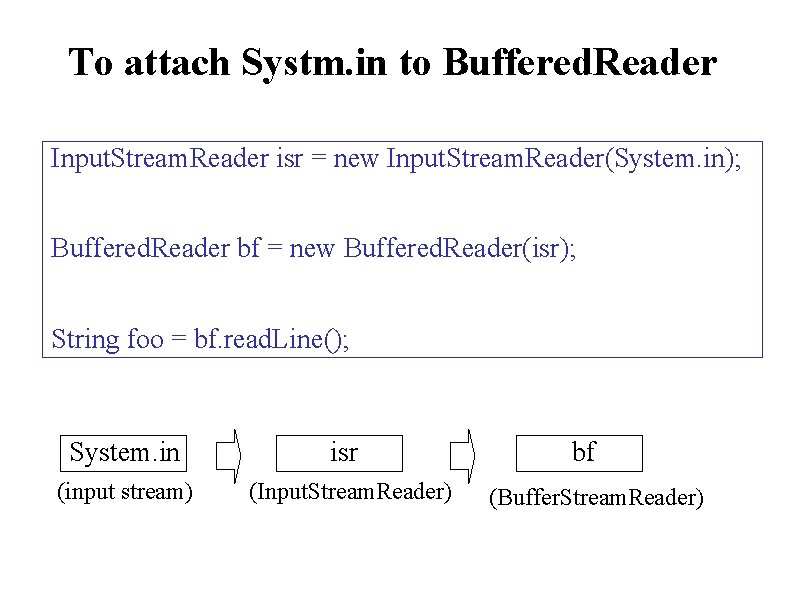
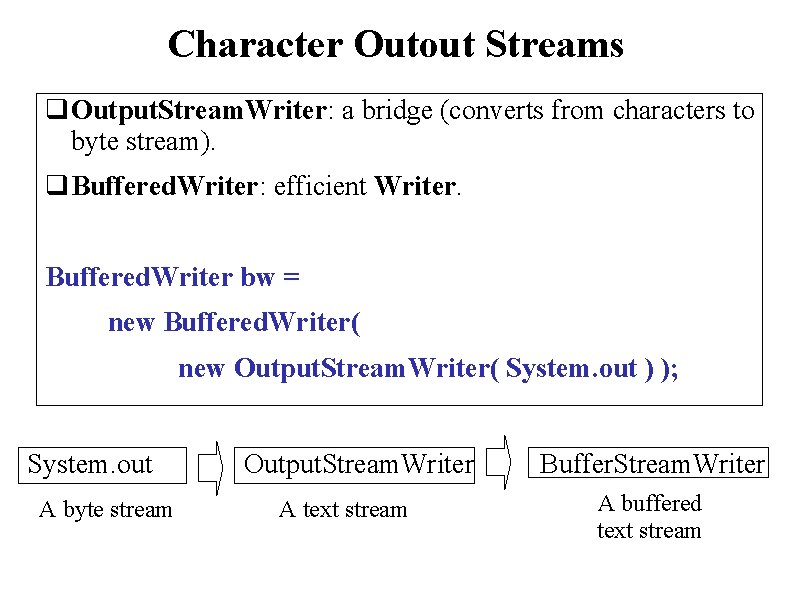
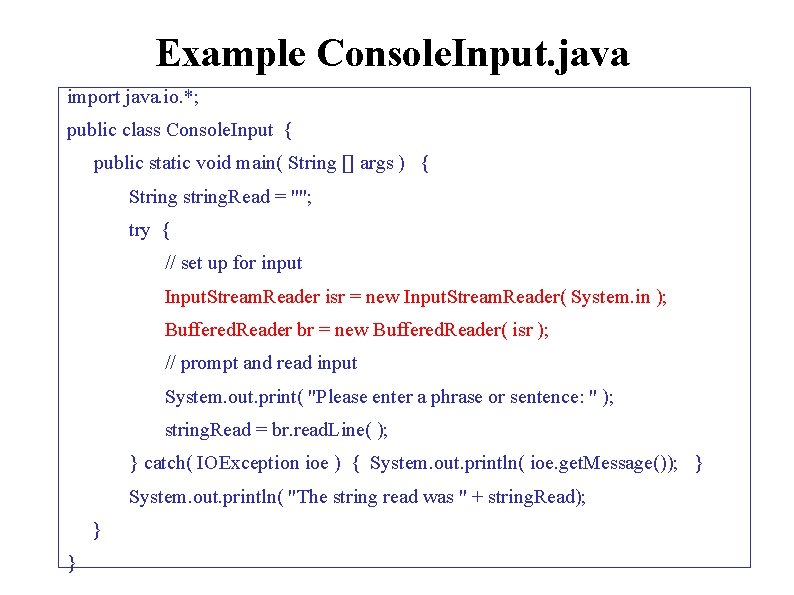
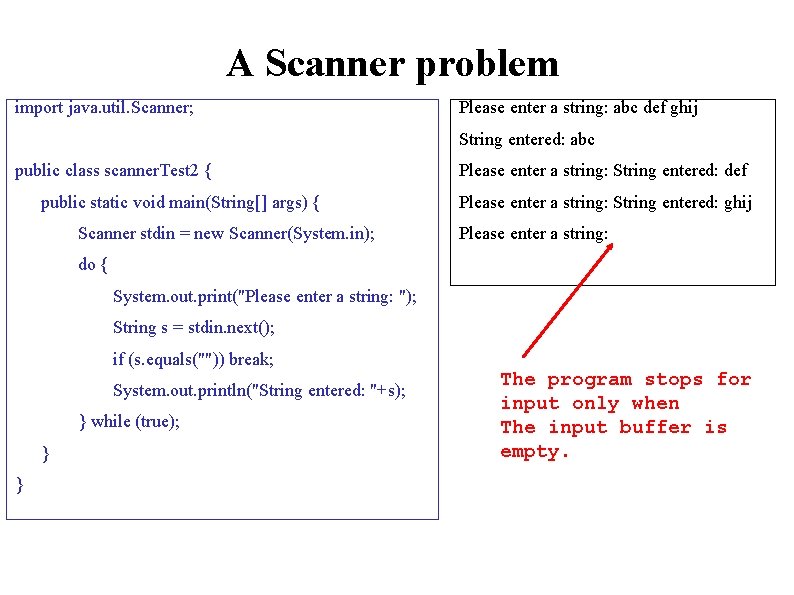
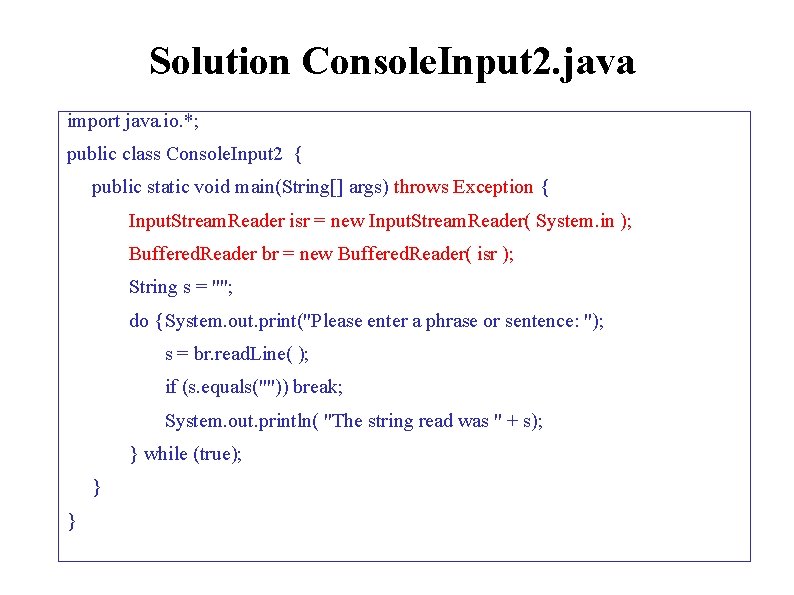
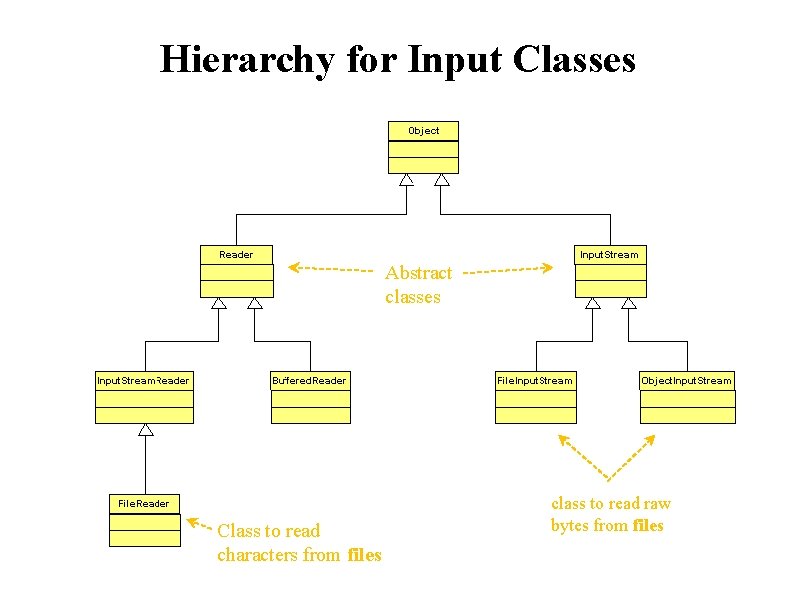
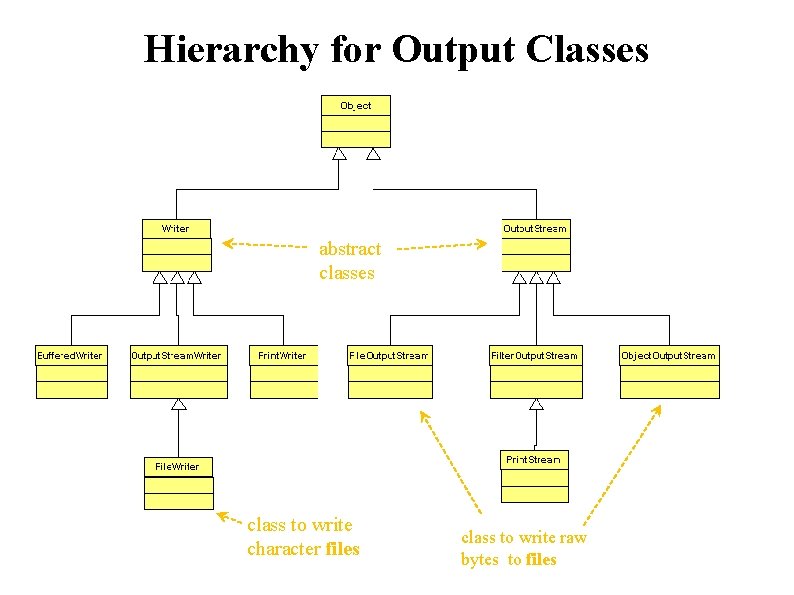
- Slides: 23
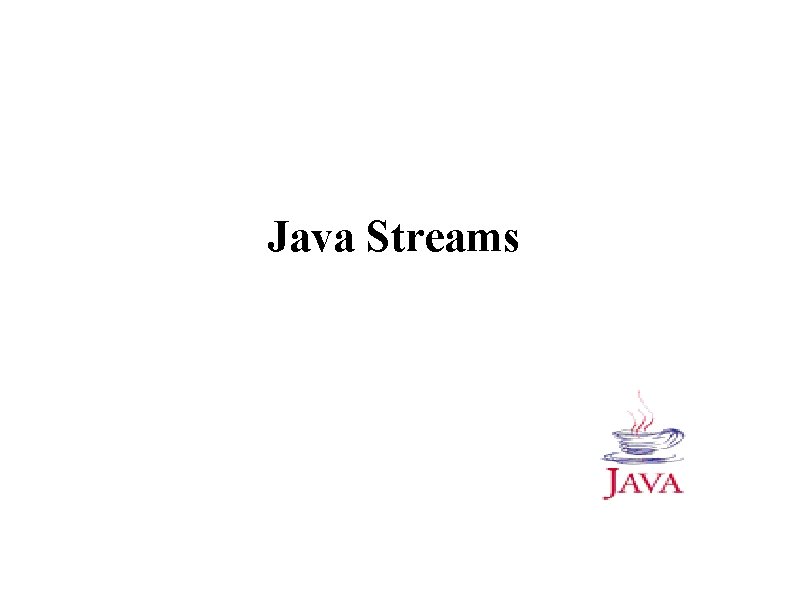
Java Streams
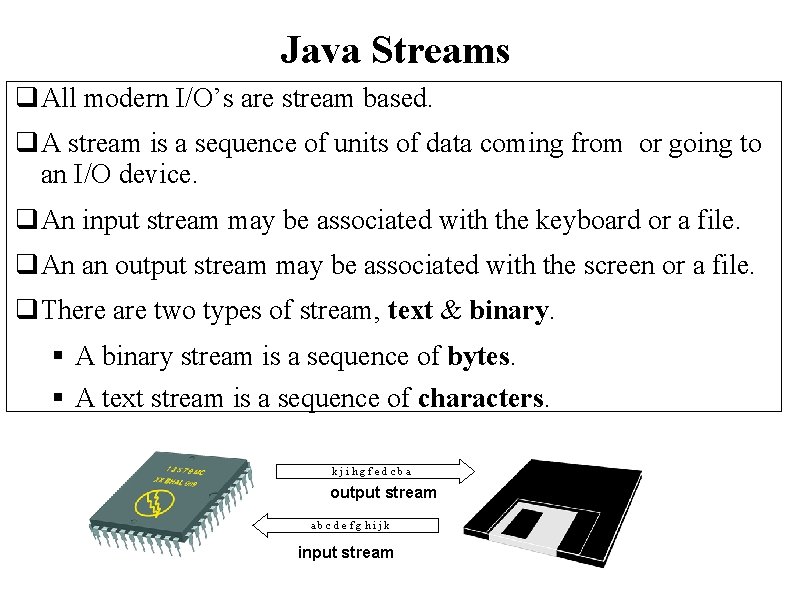
Java Streams q. All modern I/O’s are stream based. q. A stream is a sequence of units of data coming from or going to an I/O device. q. An input stream may be associated with the keyboard or a file. q. An an output stream may be associated with the screen or a file. q. There are two types of stream, text & binary. § A binary stream is a sequence of bytes. § A text stream is a sequence of characters. kjihgfedcba output stream abcdefghijk input stream
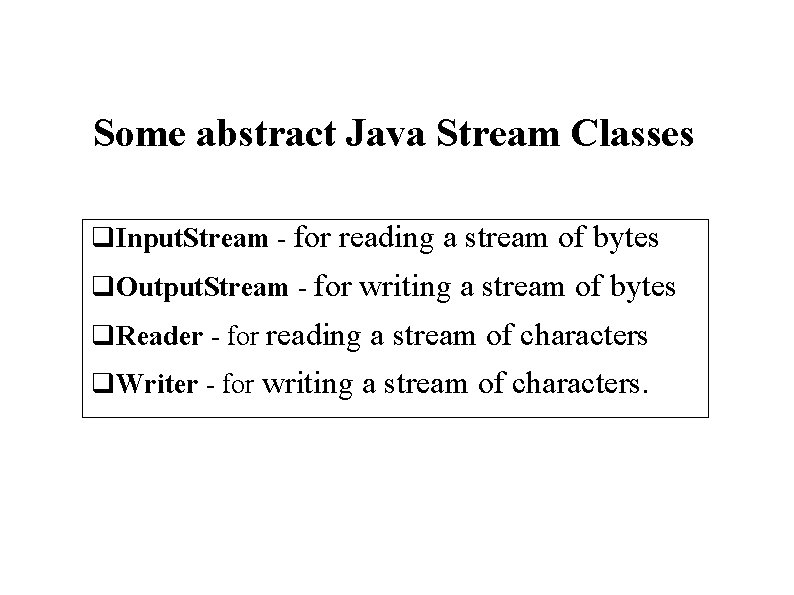
Some abstract Java Stream Classes q. Input. Stream - for reading a stream of bytes q. Output. Stream - for writing a stream of bytes q. Reader - for reading a stream of characters q. Writer - for writing a stream of characters.
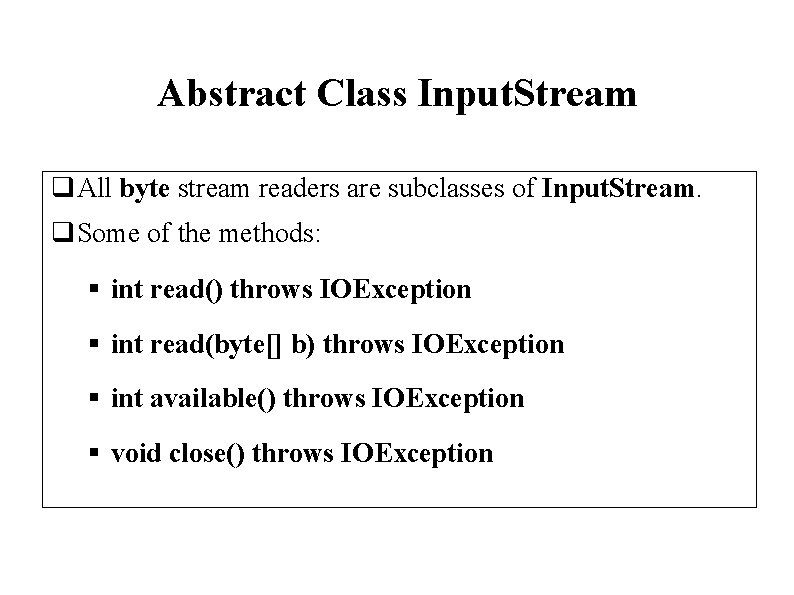
Abstract Class Input. Stream q. All byte stream readers are subclasses of Input. Stream. q. Some of the methods: § int read() throws IOException § int read(byte[] b) throws IOException § int available() throws IOException § void close() throws IOException
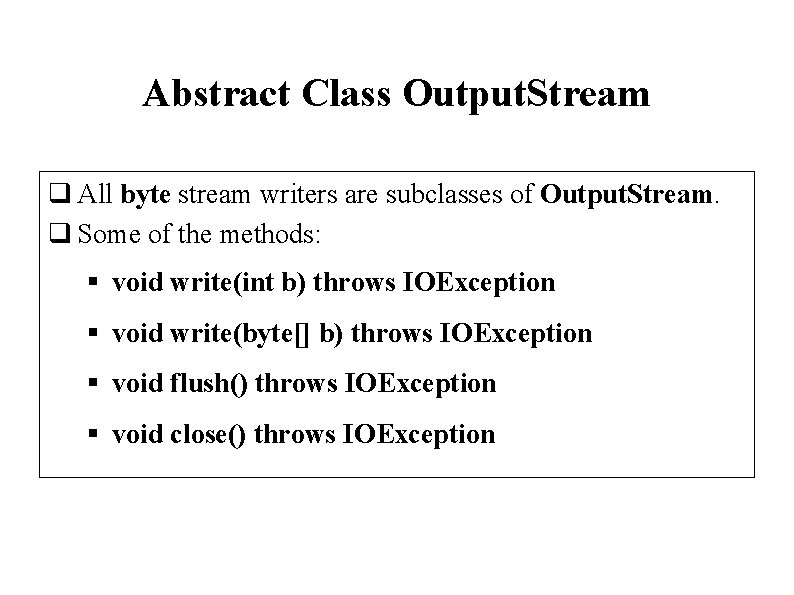
Abstract Class Output. Stream q All byte stream writers are subclasses of Output. Stream. q Some of the methods: § void write(int b) throws IOException § void write(byte[] b) throws IOException § void flush() throws IOException § void close() throws IOException
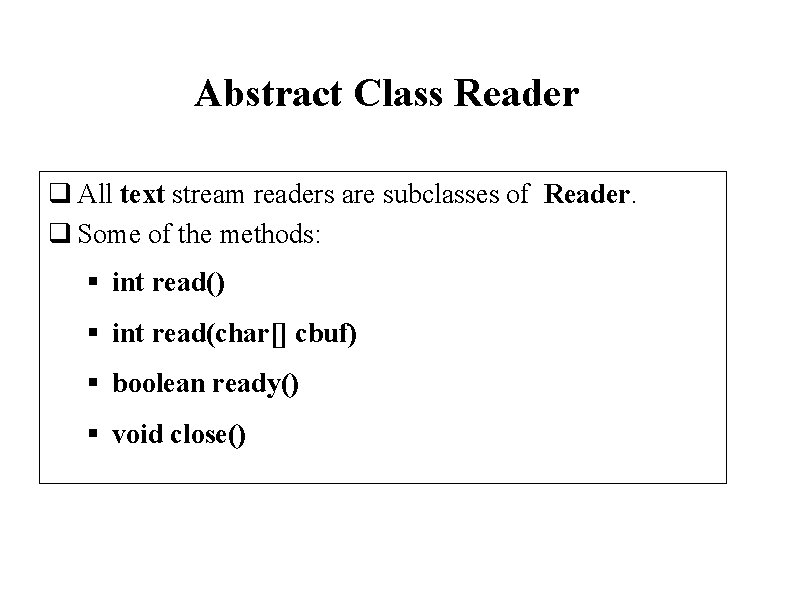
Abstract Class Reader q All text stream readers are subclasses of Reader. q Some of the methods: § int read() § int read(char[] cbuf) § boolean ready() § void close()
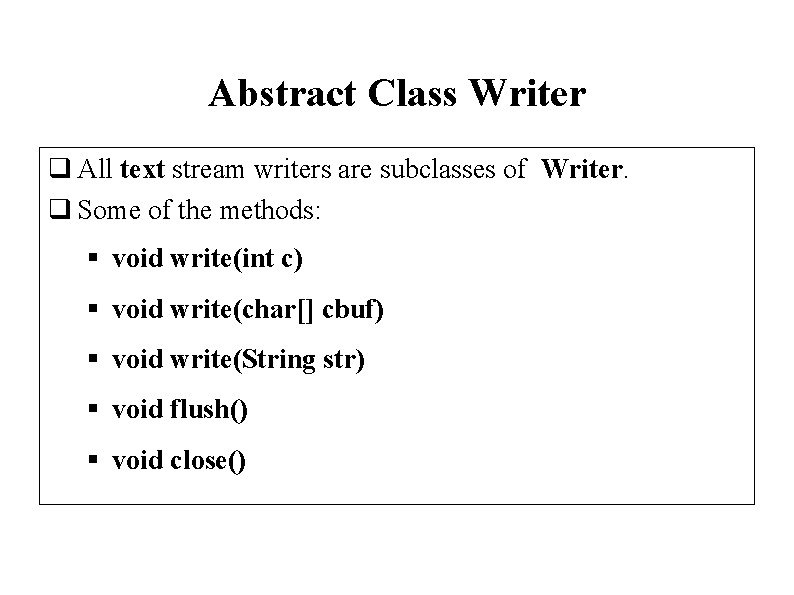
Abstract Class Writer q All text stream writers are subclasses of Writer. q Some of the methods: § void write(int c) § void write(char[] cbuf) § void write(String str) § void flush() § void close()
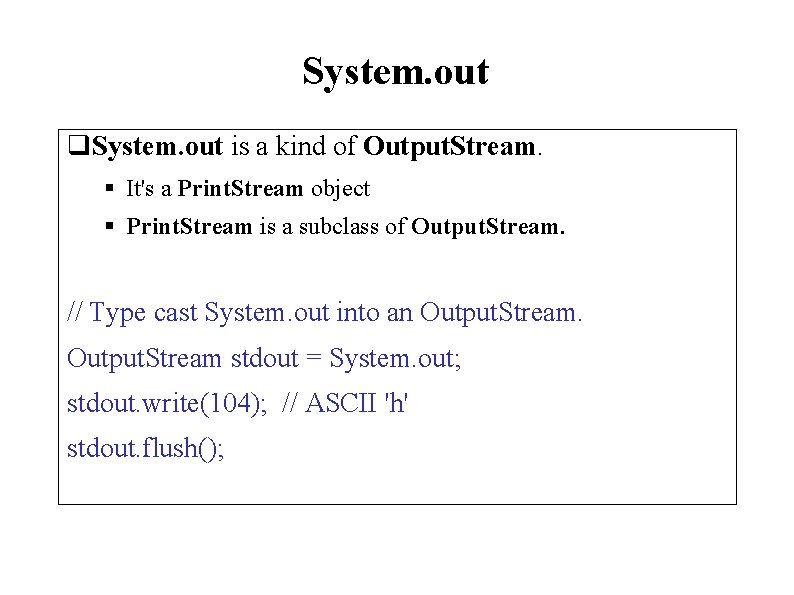
System. out q. System. out is a kind of Output. Stream. § It's a Print. Stream object § Print. Stream is a subclass of Output. Stream. // Type cast System. out into an Output. Stream stdout = System. out; stdout. write(104); // ASCII 'h' stdout. flush();
![Handle IOExceptions public static void mainString args Output Stream stdout System out Handle IOExceptions public static void main(String[] args) { Output. Stream stdout = System. out;](https://slidetodoc.com/presentation_image_h2/1f91532e981dcdf520cd44e409ec76e0/image-9.jpg)
Handle IOExceptions public static void main(String[] args) { Output. Stream stdout = System. out; try { stdout. write(104); // 'h' stdout. write(105); // 'i' stdout. write(10); // 'n' } catch (IOException e) { e. print. Stack. Trace(); } }
![Another way public static void mainString args throws IOException Output Stream stdout Another way public static void main(String[] args) throws IOException { Output. Stream stdout =](https://slidetodoc.com/presentation_image_h2/1f91532e981dcdf520cd44e409ec76e0/image-10.jpg)
Another way public static void main(String[] args) throws IOException { Output. Stream stdout = System. out; stdout. write(104); // 'h' stdout. write(105); // 'i' stdout. write(10); // 'n' }
![System in q System in is a type of Input Stream byte b System. in q. System. in is a type of Input. Stream. byte[] b =](https://slidetodoc.com/presentation_image_h2/1f91532e981dcdf520cd44e409ec76e0/image-11.jpg)
System. in q. System. in is a type of Input. Stream. byte[] b = new byte[10]; Input. Stream stdin = System. in; len = stdin. read(b); for (int i=0; i<len; i++) System. out. write(b[i]);
![Try available Input Stream stdin System in byte b new bytestdin available Try available() Input. Stream stdin = System. in; byte[] b = new byte[stdin. available()];](https://slidetodoc.com/presentation_image_h2/1f91532e981dcdf520cd44e409ec76e0/image-12.jpg)
Try available() Input. Stream stdin = System. in; byte[] b = new byte[stdin. available()]; int len = stdin. read(b); for (int i=0; i<len; i++) System. out. write(b[i]); System. out. flush();
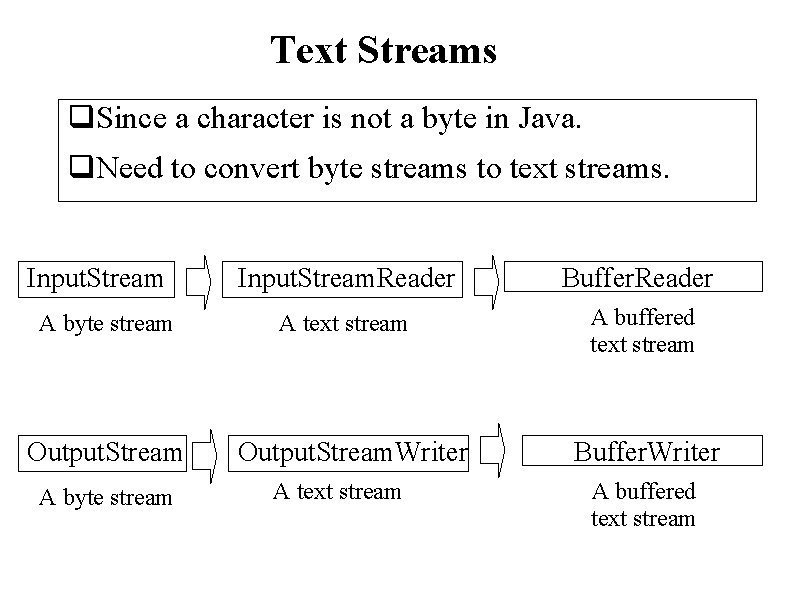
Text Streams q. Since a character is not a byte in Java. q. Need to convert byte streams to text streams. Input. Stream A byte stream Output. Stream A byte stream Input. Stream. Reader Buffer. Reader A text stream A buffered text stream Output. Stream. Writer A text stream Buffer. Writer A buffered text stream
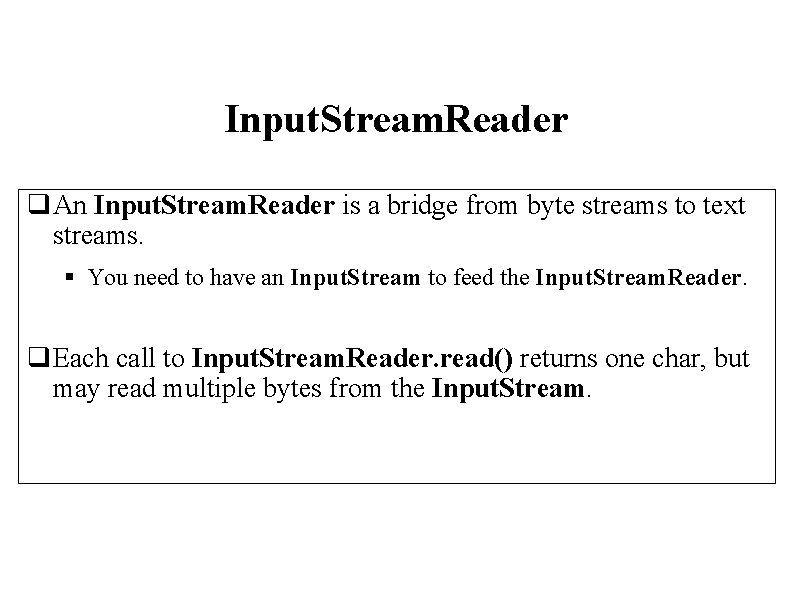
Input. Stream. Reader q. An Input. Stream. Reader is a bridge from byte streams to text streams. § You need to have an Input. Stream to feed the Input. Stream. Reader. q. Each call to Input. Stream. Reader. read() returns one char, but may read multiple bytes from the Input. Stream.
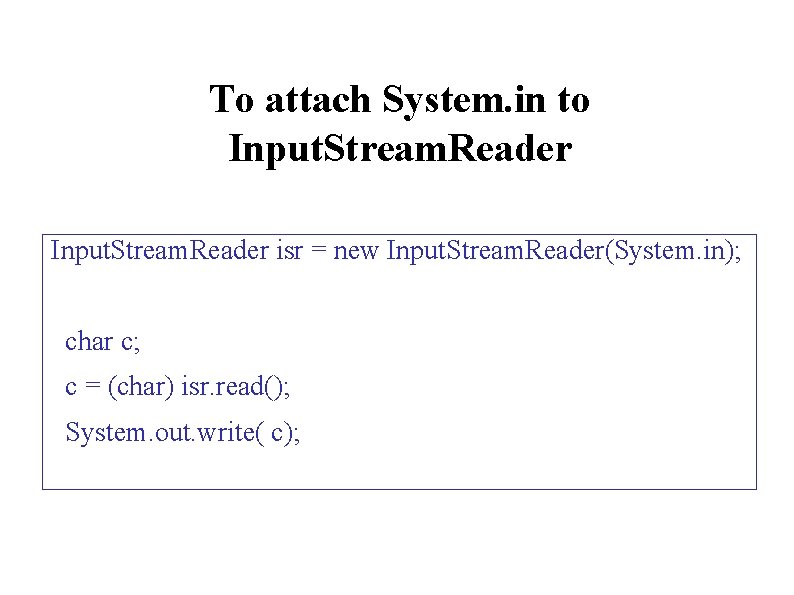
To attach System. in to Input. Stream. Reader isr = new Input. Stream. Reader(System. in); char c; c = (char) isr. read(); System. out. write( c);
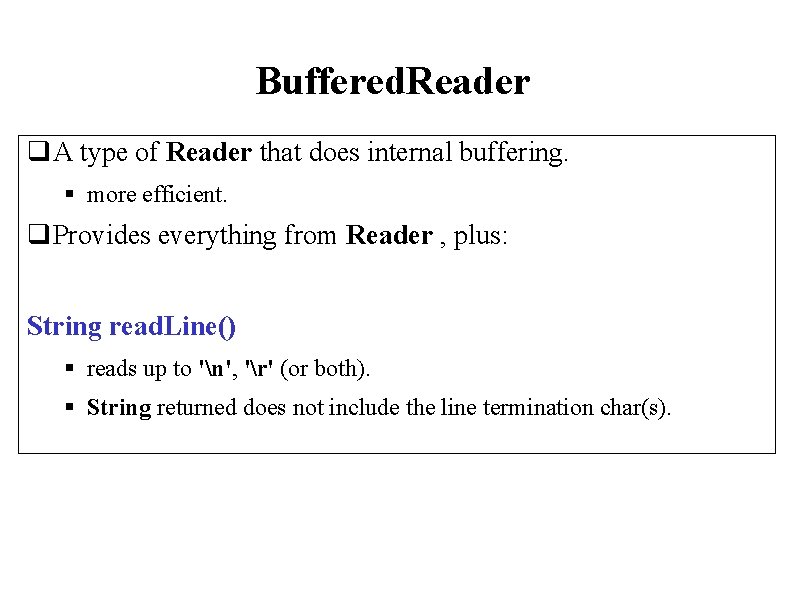
Buffered. Reader q. A type of Reader that does internal buffering. § more efficient. q. Provides everything from Reader , plus: String read. Line() § reads up to 'n', 'r' (or both). § String returned does not include the line termination char(s).
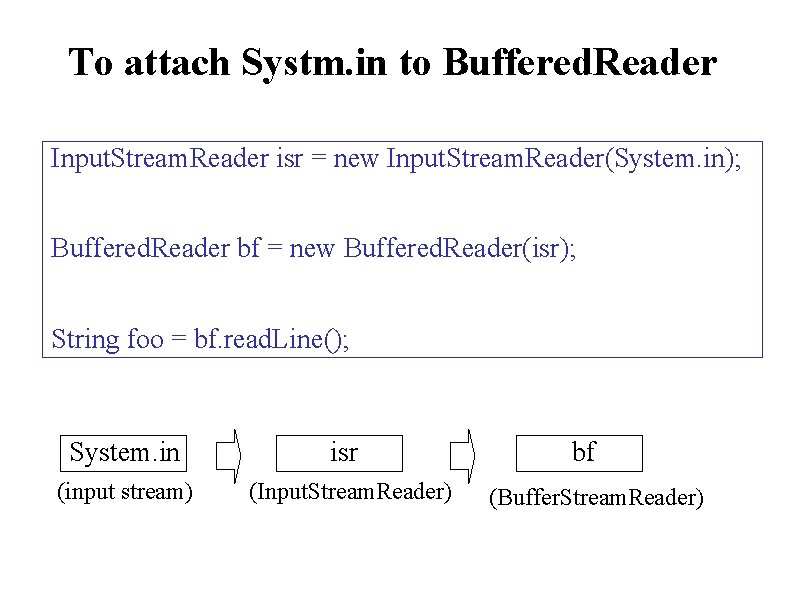
To attach Systm. in to Buffered. Reader Input. Stream. Reader isr = new Input. Stream. Reader(System. in); Buffered. Reader bf = new Buffered. Reader(isr); String foo = bf. read. Line(); System. in isr (input stream) (Input. Stream. Reader) bf (Buffer. Stream. Reader)
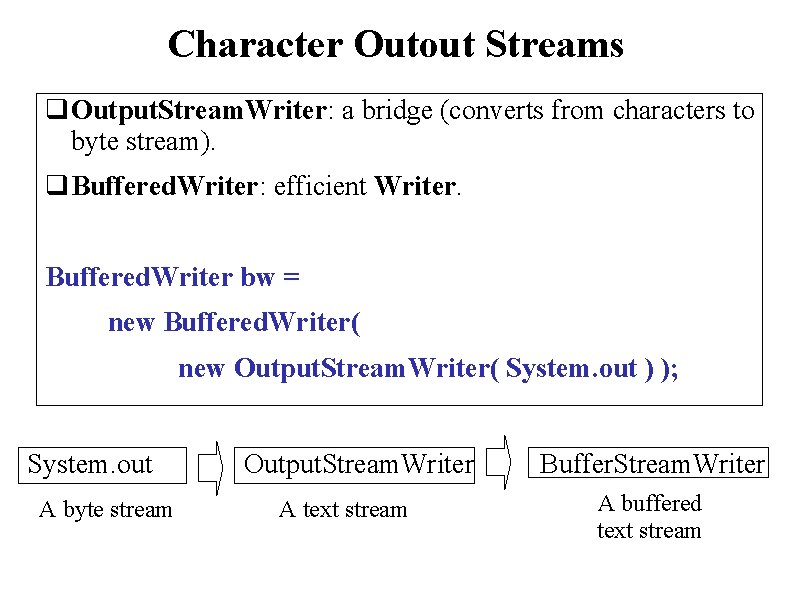
Character Outout Streams q. Output. Stream. Writer: a bridge (converts from characters to byte stream). q. Buffered. Writer: efficient Writer. Buffered. Writer bw = new Buffered. Writer( new Output. Stream. Writer( System. out ) ); System. out A byte stream Output. Stream. Writer A text stream Buffer. Stream. Writer A buffered text stream
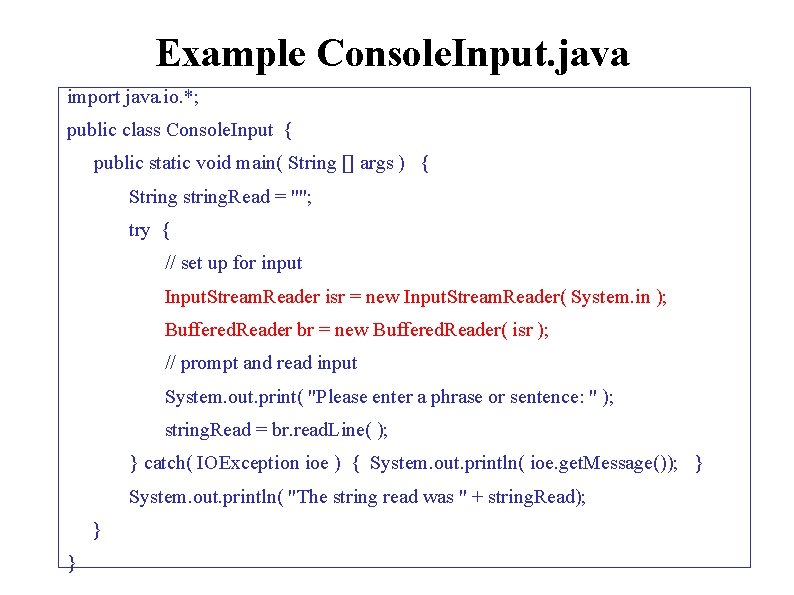
Example Console. Input. java import java. io. *; public class Console. Input { public static void main( String [] args ) { String string. Read = ""; try { // set up for input Input. Stream. Reader isr = new Input. Stream. Reader( System. in ); Buffered. Reader br = new Buffered. Reader( isr ); // prompt and read input System. out. print( "Please enter a phrase or sentence: " ); string. Read = br. read. Line( ); } catch( IOException ioe ) { System. out. println( ioe. get. Message()); } System. out. println( "The string read was " + string. Read); } }
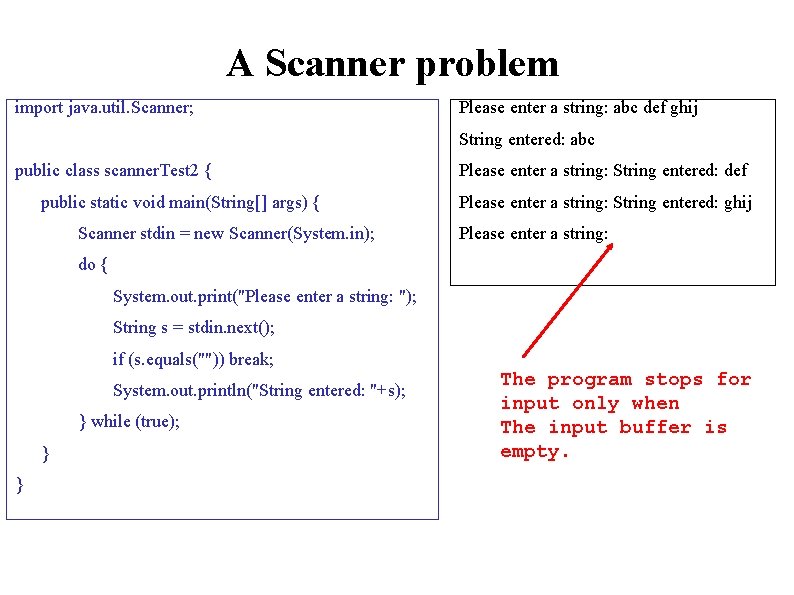
A Scanner problem import java. util. Scanner; Please enter a string: abc def ghij String entered: abc public class scanner. Test 2 { public static void main(String[] args) { Scanner stdin = new Scanner(System. in); Please enter a string: String entered: def Please enter a string: String entered: ghij Please enter a string: do { System. out. print("Please enter a string: "); String s = stdin. next(); if (s. equals("")) break; System. out. println("String entered: "+s); } while (true); } } The program stops for input only when The input buffer is empty.
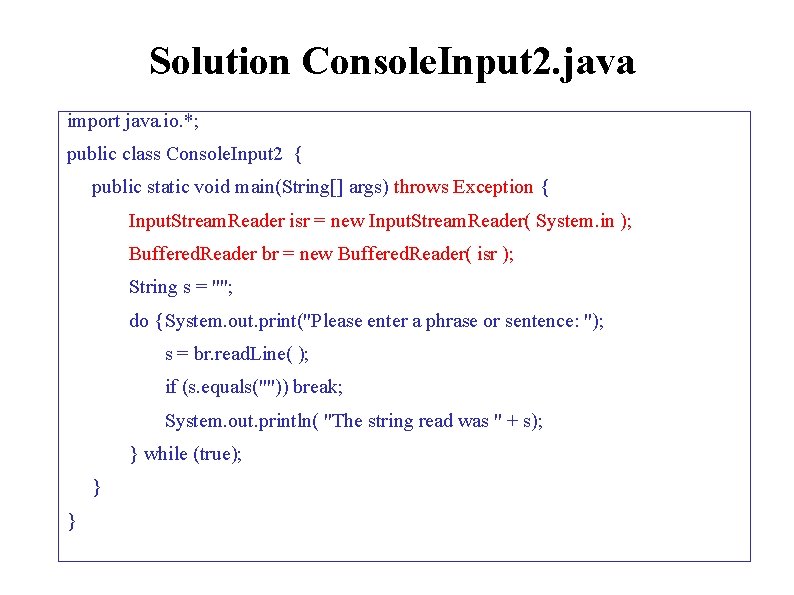
Solution Console. Input 2. java import java. io. *; public class Console. Input 2 { public static void main(String[] args) throws Exception { Input. Stream. Reader isr = new Input. Stream. Reader( System. in ); Buffered. Reader br = new Buffered. Reader( isr ); String s = ""; do {System. out. print("Please enter a phrase or sentence: "); s = br. read. Line( ); if (s. equals("")) break; System. out. println( "The string read was " + s); } while (true); } }
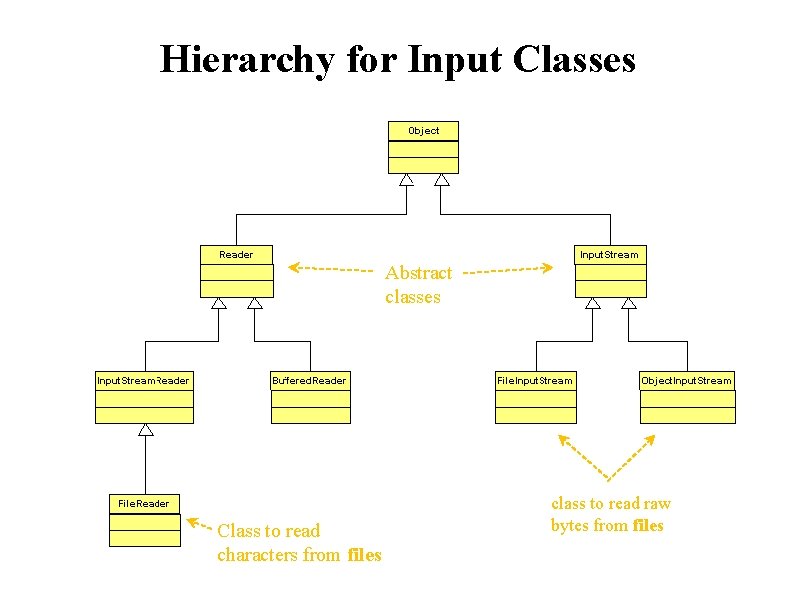
Hierarchy for Input Classes Abstract classes Class to read characters from files class to read raw bytes from files
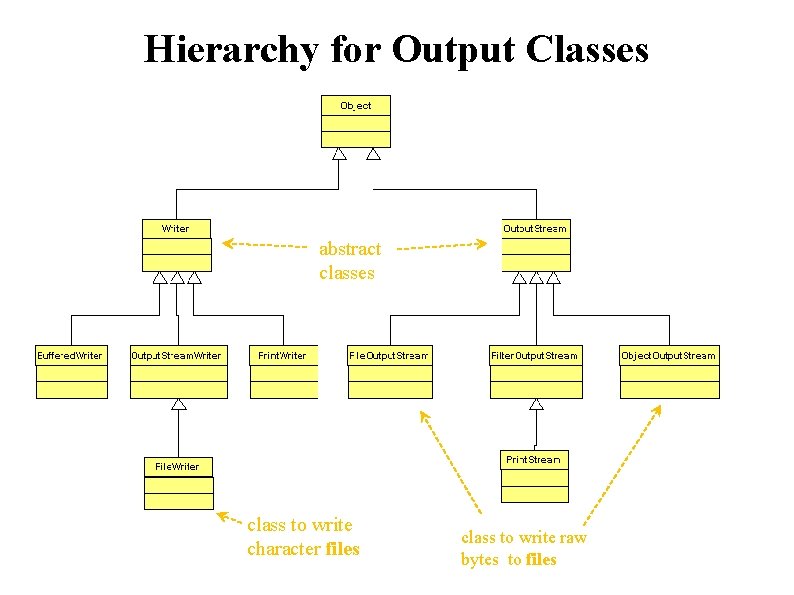
Hierarchy for Output Classes abstract classes class to write character files class to write raw bytes to files
Predefined streams in java
Name 3 points
Japantac
Zero touch provisioning cisco
Ios xr rpl examples
Cisco router technical support
Cisco cbac
Cisco ios naming convention
Ios press release
Cisco router hardware architecture
Grecja ios
Cisco service level agreement
Cisco ios hardening
Setfill
Which component holds the ios a cisco router can use?
Kok-play 아이폰
Ios 110
Ios command line
Ios110
Ios sprachkurse uni due
Cisco ios upgrade planner tool
Cisco ios hardening
Tinc ios
Cisco ios ping indicators