Java Programming Introduction to GUIs Part 2 Phil
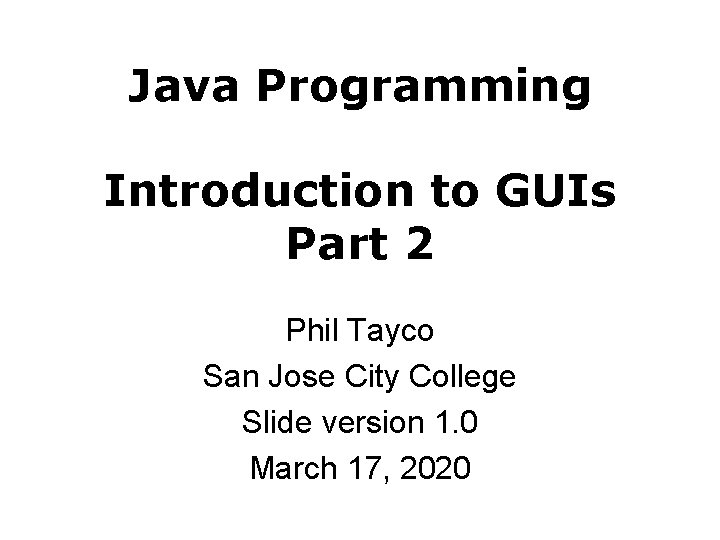
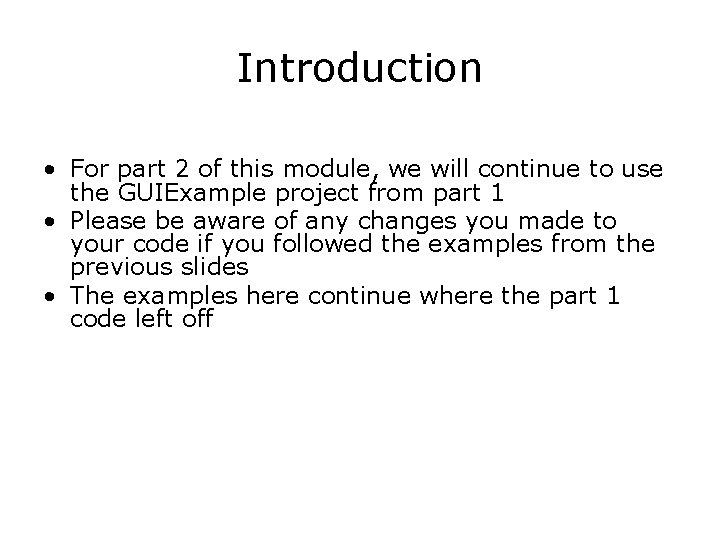
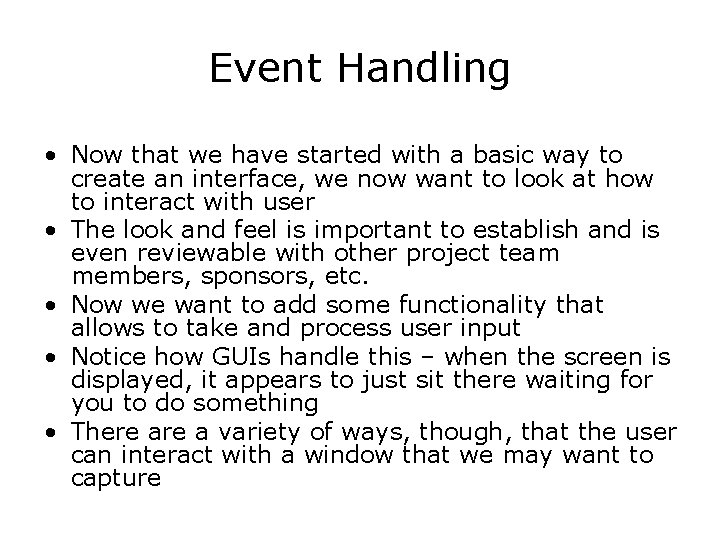
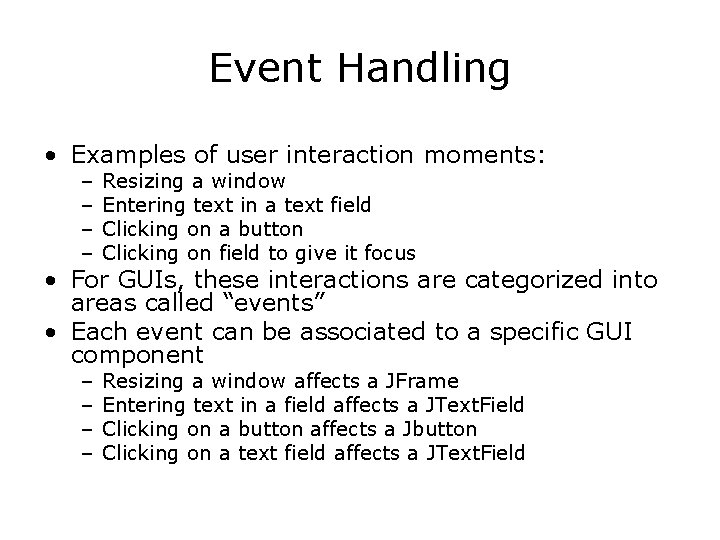
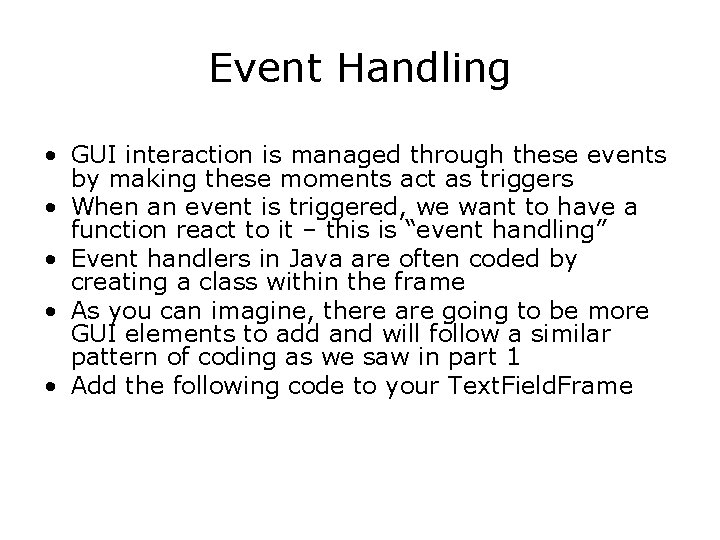
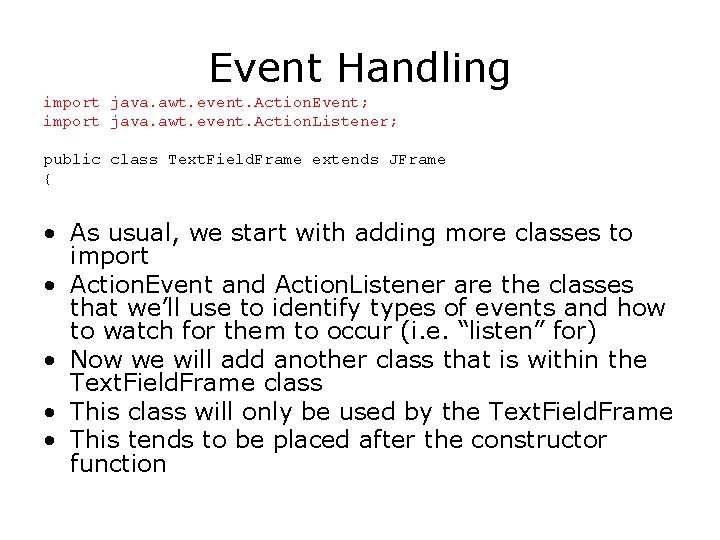
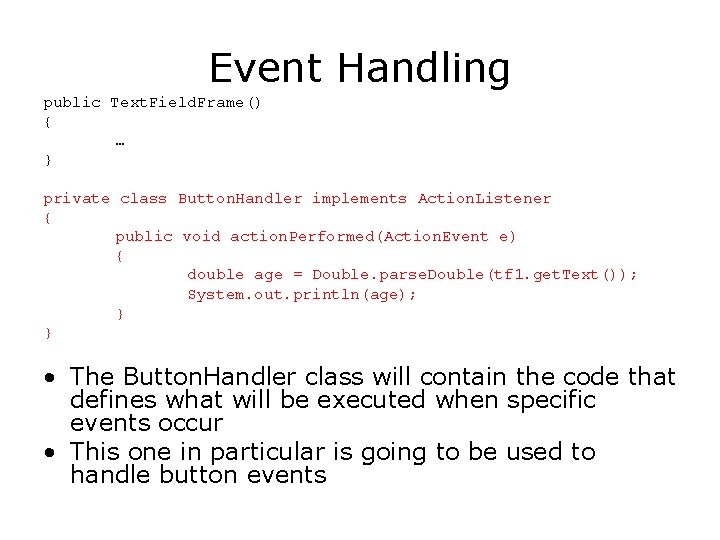
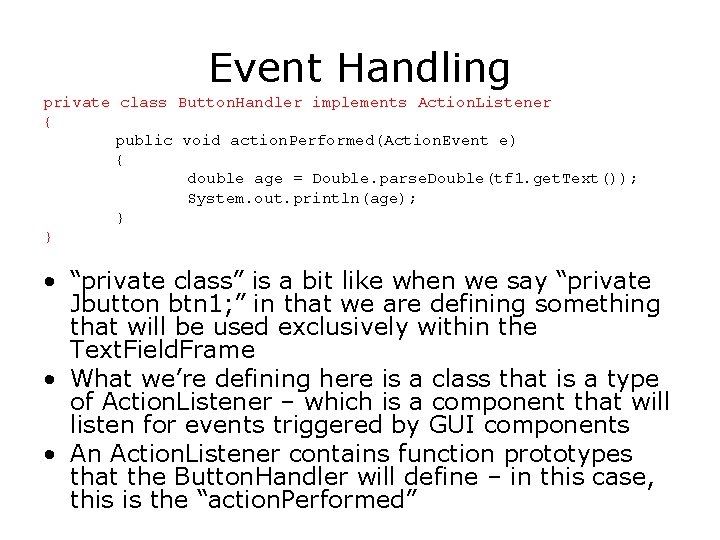
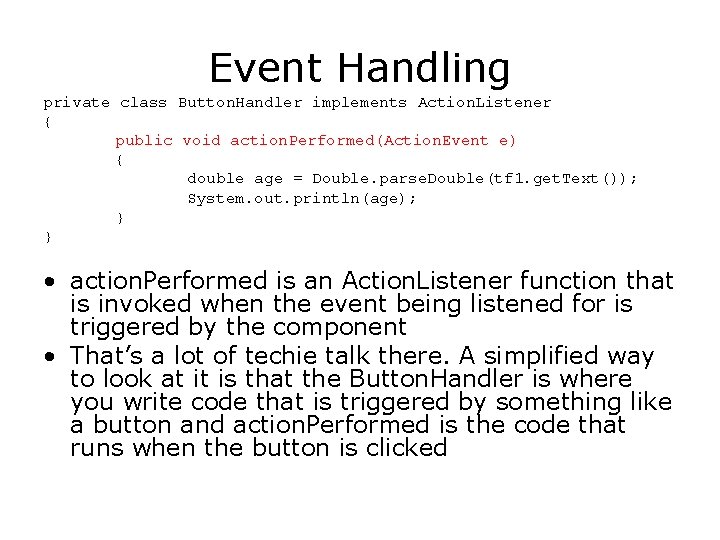
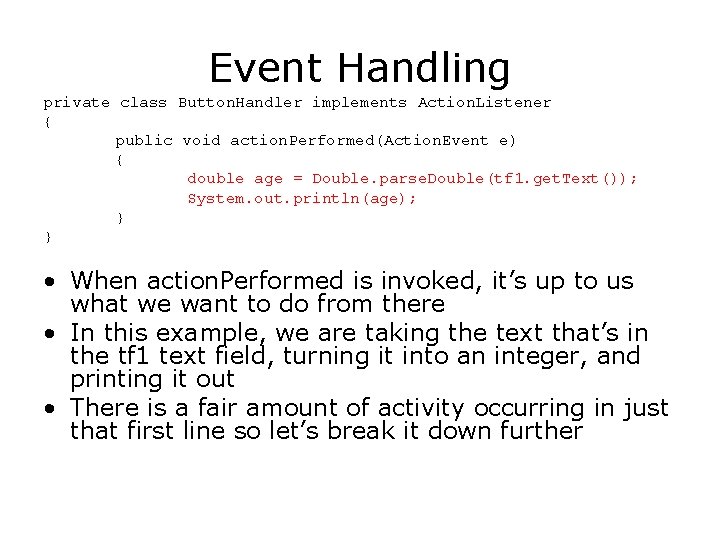
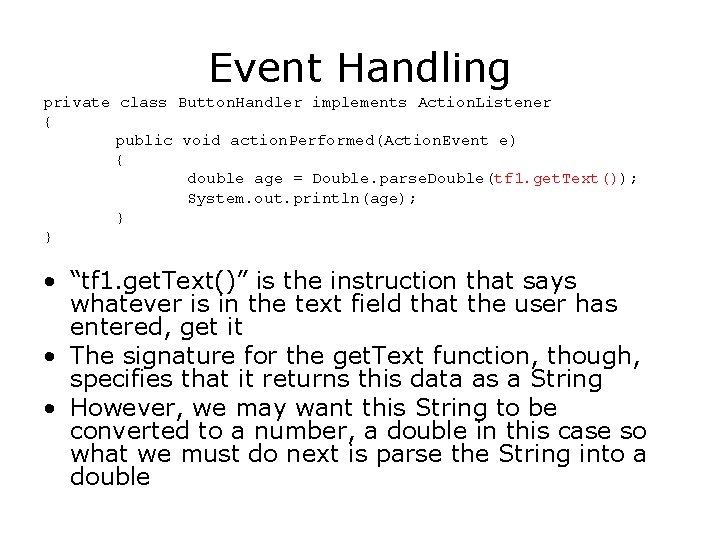
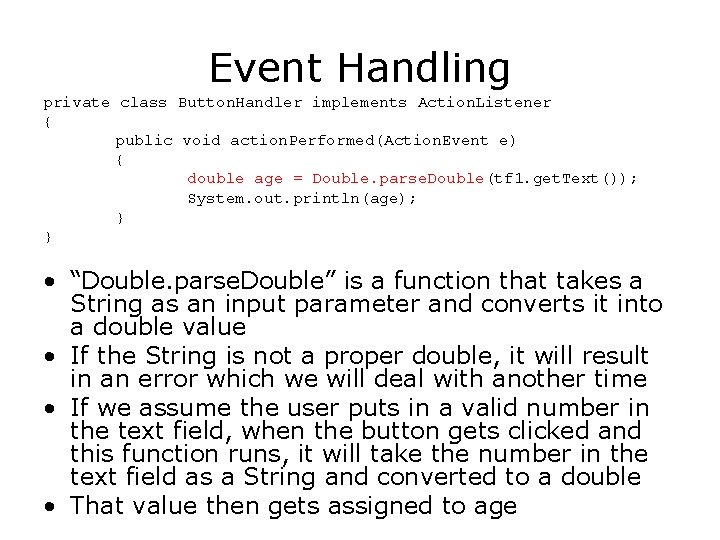
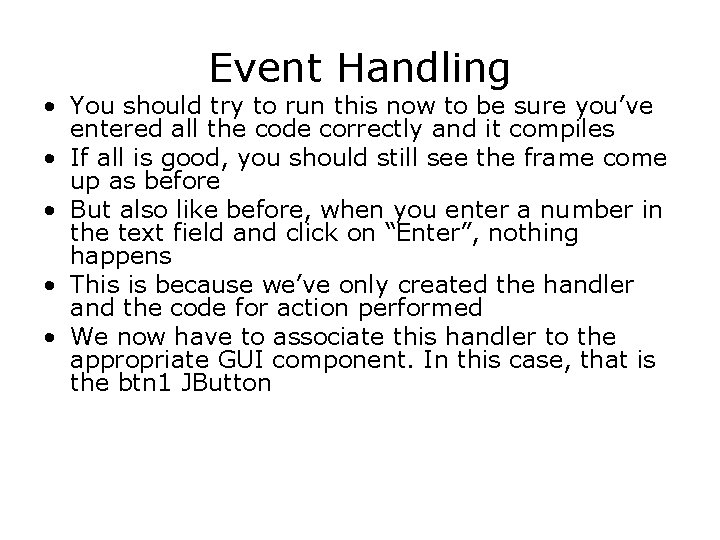
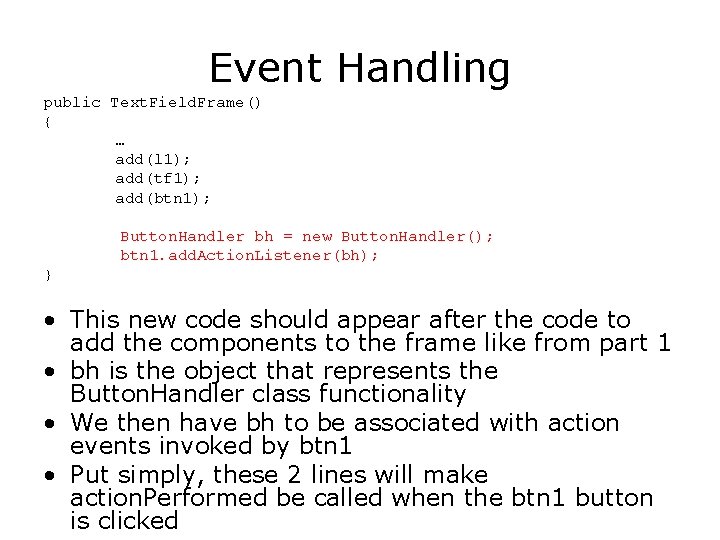
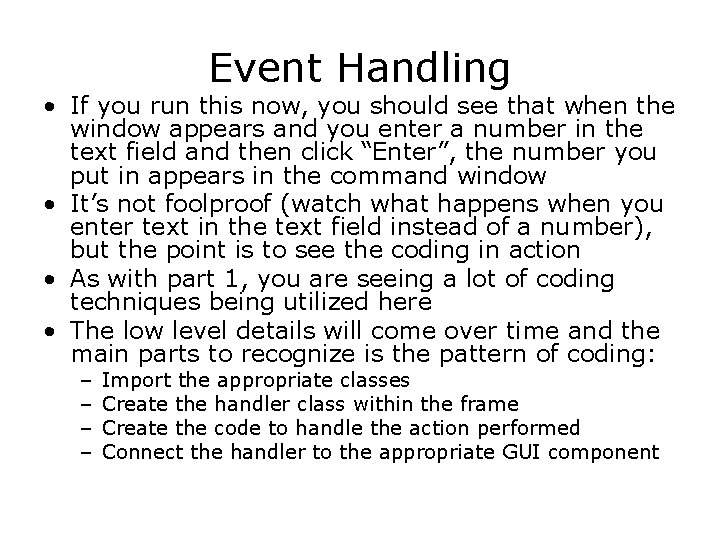
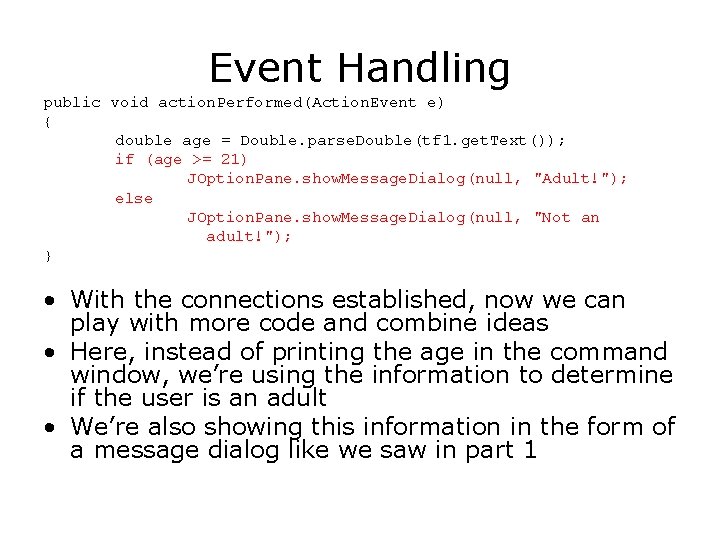
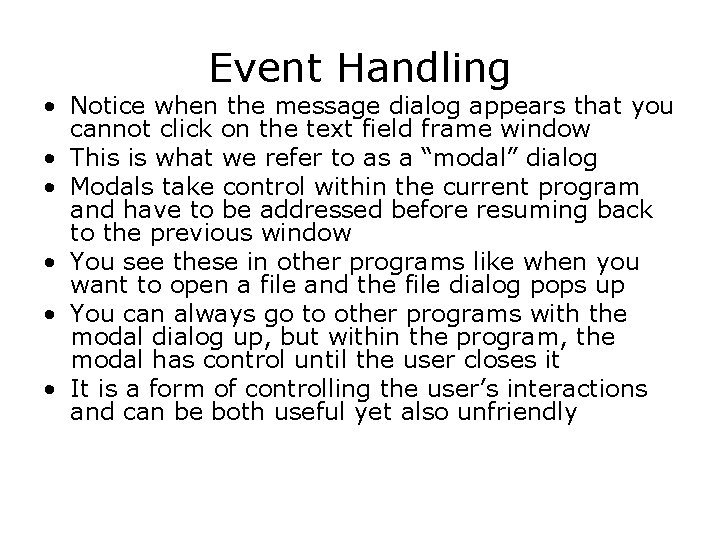
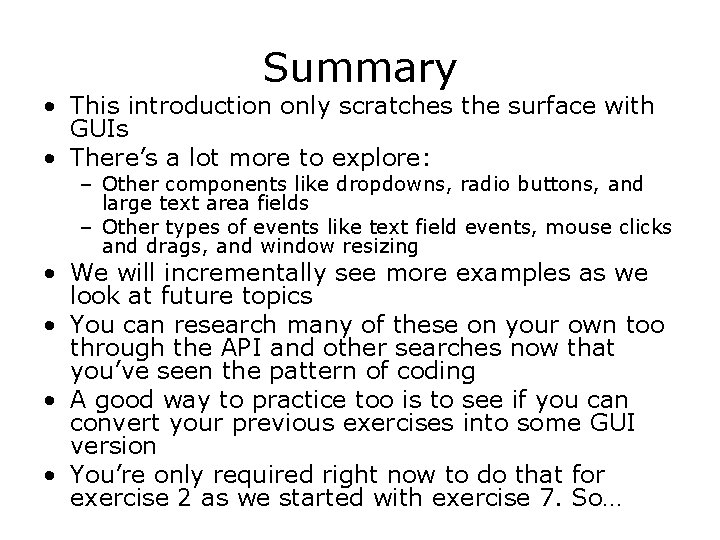
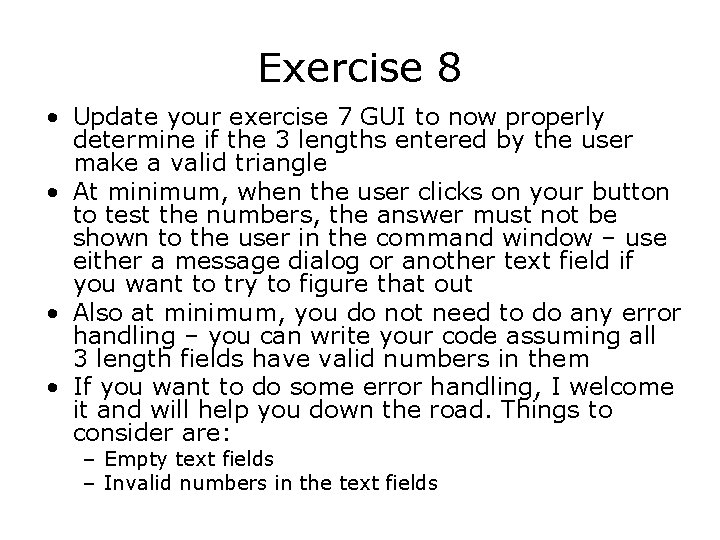
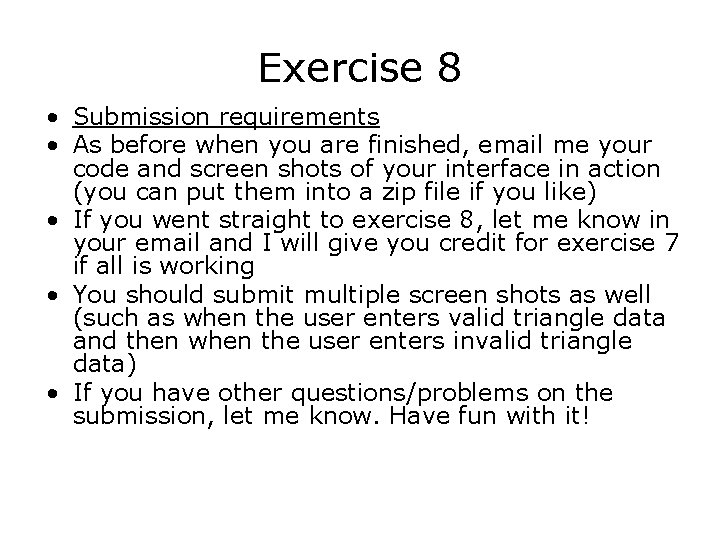
- Slides: 20
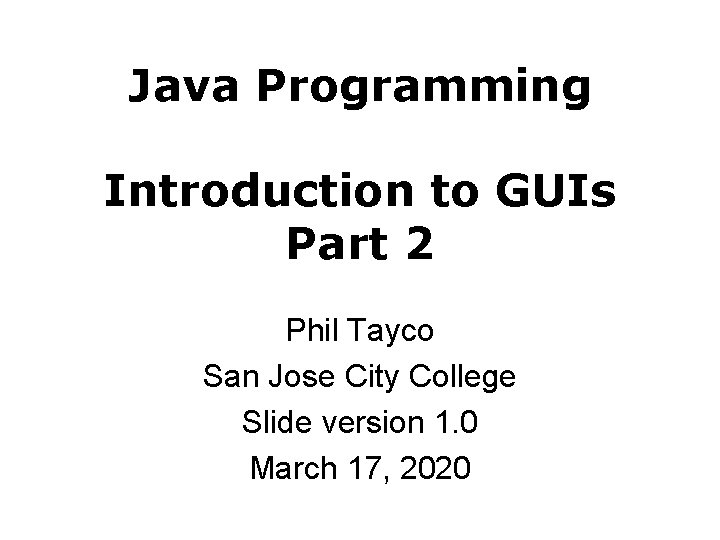
Java Programming Introduction to GUIs Part 2 Phil Tayco San Jose City College Slide version 1. 0 March 17, 2020
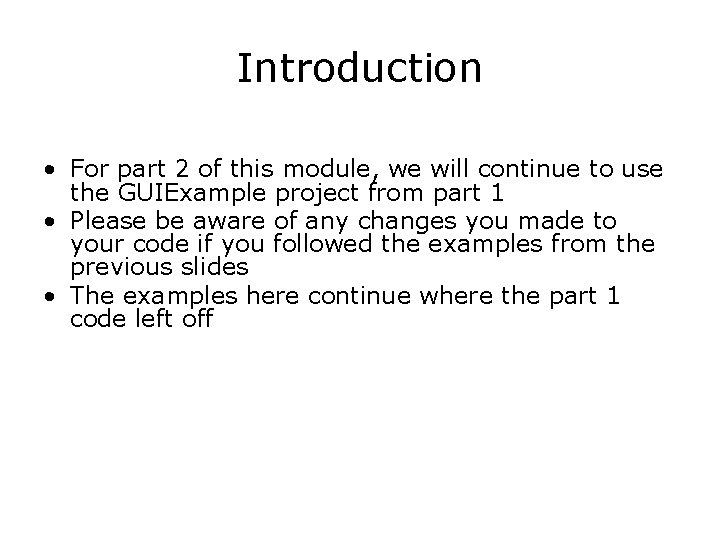
Introduction • For part 2 of this module, we will continue to use the GUIExample project from part 1 • Please be aware of any changes you made to your code if you followed the examples from the previous slides • The examples here continue where the part 1 code left off
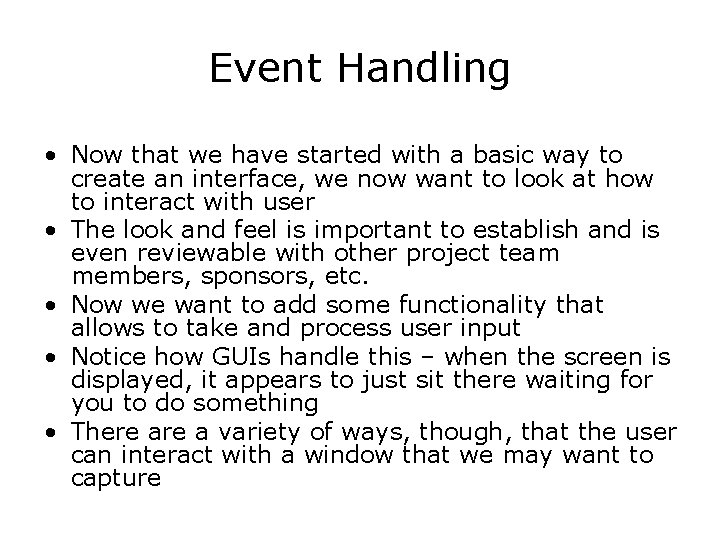
Event Handling • Now that we have started with a basic way to create an interface, we now want to look at how to interact with user • The look and feel is important to establish and is even reviewable with other project team members, sponsors, etc. • Now we want to add some functionality that allows to take and process user input • Notice how GUIs handle this – when the screen is displayed, it appears to just sit there waiting for you to do something • There a variety of ways, though, that the user can interact with a window that we may want to capture
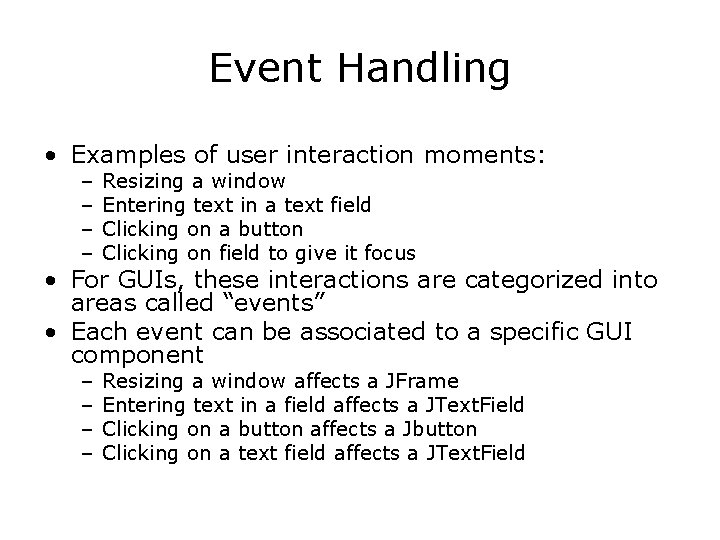
Event Handling • Examples of user interaction moments: – – Resizing a window Entering text in a text field Clicking on a button Clicking on field to give it focus – – Resizing a window affects a JFrame Entering text in a field affects a JText. Field Clicking on a button affects a Jbutton Clicking on a text field affects a JText. Field • For GUIs, these interactions are categorized into areas called “events” • Each event can be associated to a specific GUI component
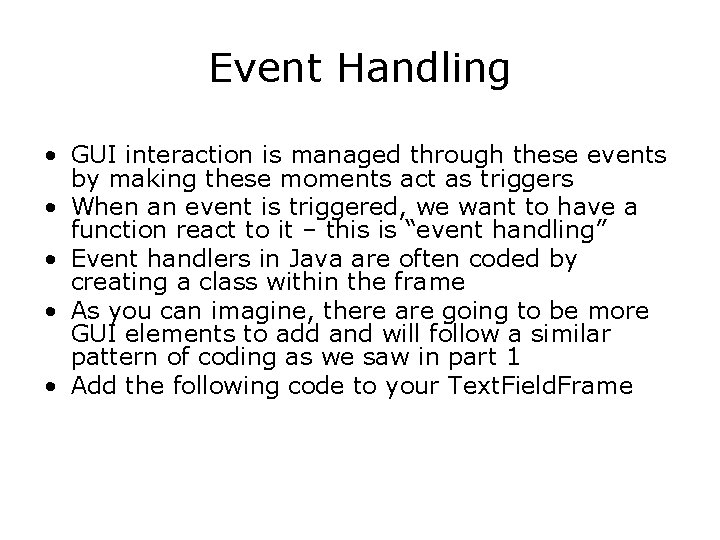
Event Handling • GUI interaction is managed through these events by making these moments act as triggers • When an event is triggered, we want to have a function react to it – this is “event handling” • Event handlers in Java are often coded by creating a class within the frame • As you can imagine, there are going to be more GUI elements to add and will follow a similar pattern of coding as we saw in part 1 • Add the following code to your Text. Field. Frame
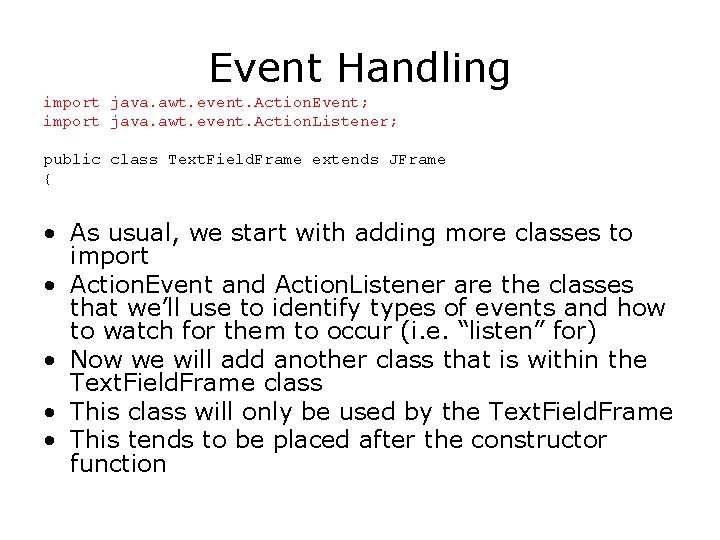
Event Handling import java. awt. event. Action. Event; import java. awt. event. Action. Listener; public class Text. Field. Frame extends JFrame { • As usual, we start with adding more classes to import • Action. Event and Action. Listener are the classes that we’ll use to identify types of events and how to watch for them to occur (i. e. “listen” for) • Now we will add another class that is within the Text. Field. Frame class • This class will only be used by the Text. Field. Frame • This tends to be placed after the constructor function
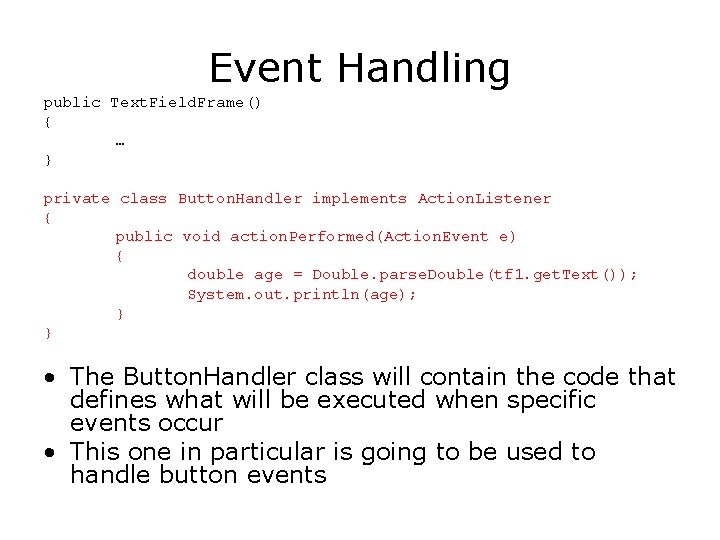
Event Handling public Text. Field. Frame() { … } private class Button. Handler implements Action. Listener { public void action. Performed(Action. Event e) { double age = Double. parse. Double(tf 1. get. Text()); System. out. println(age); } } • The Button. Handler class will contain the code that defines what will be executed when specific events occur • This one in particular is going to be used to handle button events
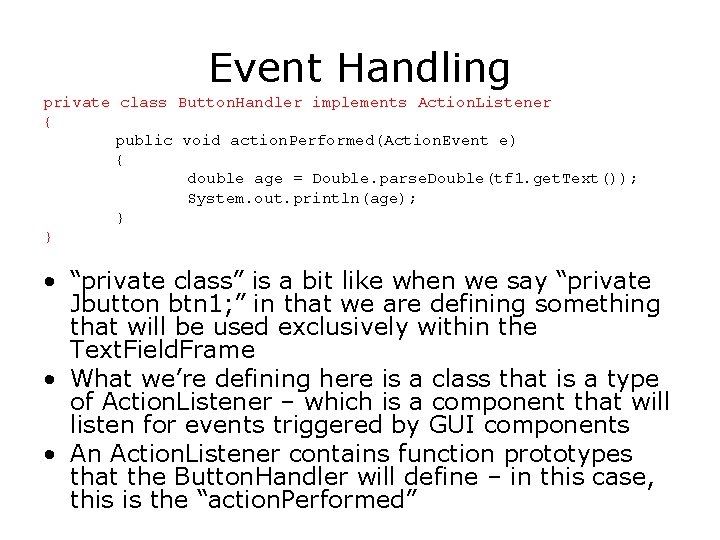
Event Handling private class Button. Handler implements Action. Listener { public void action. Performed(Action. Event e) { double age = Double. parse. Double(tf 1. get. Text()); System. out. println(age); } } • “private class” is a bit like when we say “private Jbutton btn 1; ” in that we are defining something that will be used exclusively within the Text. Field. Frame • What we’re defining here is a class that is a type of Action. Listener – which is a component that will listen for events triggered by GUI components • An Action. Listener contains function prototypes that the Button. Handler will define – in this case, this is the “action. Performed”
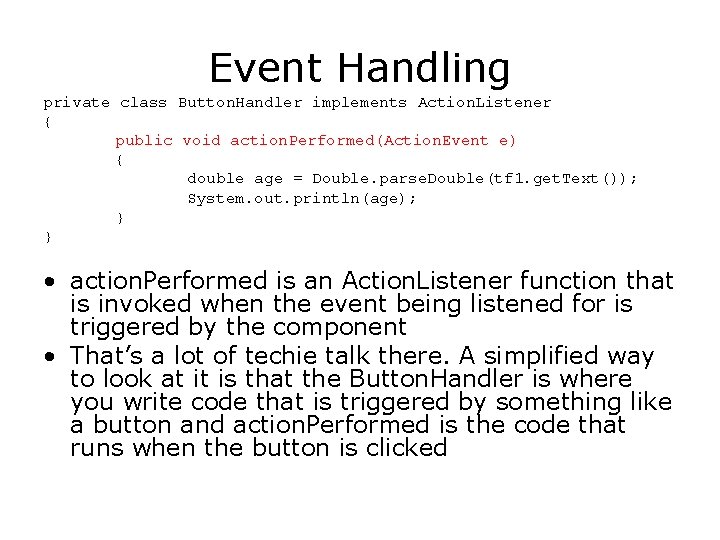
Event Handling private class Button. Handler implements Action. Listener { public void action. Performed(Action. Event e) { double age = Double. parse. Double(tf 1. get. Text()); System. out. println(age); } } • action. Performed is an Action. Listener function that is invoked when the event being listened for is triggered by the component • That’s a lot of techie talk there. A simplified way to look at it is that the Button. Handler is where you write code that is triggered by something like a button and action. Performed is the code that runs when the button is clicked
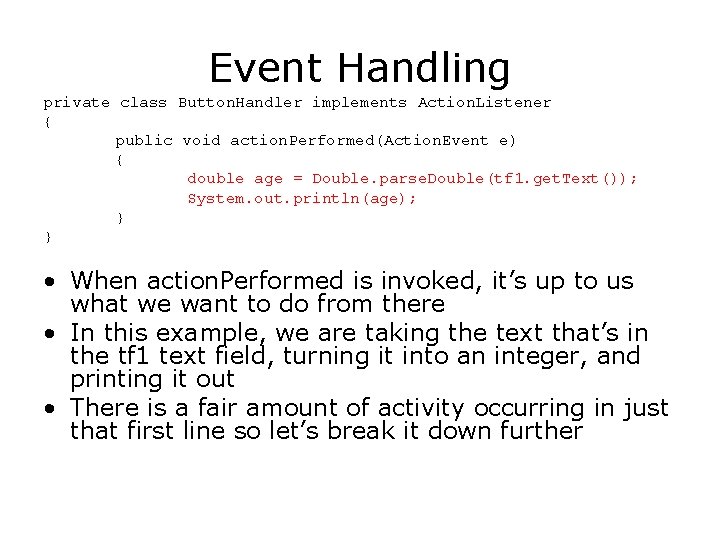
Event Handling private class Button. Handler implements Action. Listener { public void action. Performed(Action. Event e) { double age = Double. parse. Double(tf 1. get. Text()); System. out. println(age); } } • When action. Performed is invoked, it’s up to us what we want to do from there • In this example, we are taking the text that’s in the tf 1 text field, turning it into an integer, and printing it out • There is a fair amount of activity occurring in just that first line so let’s break it down further
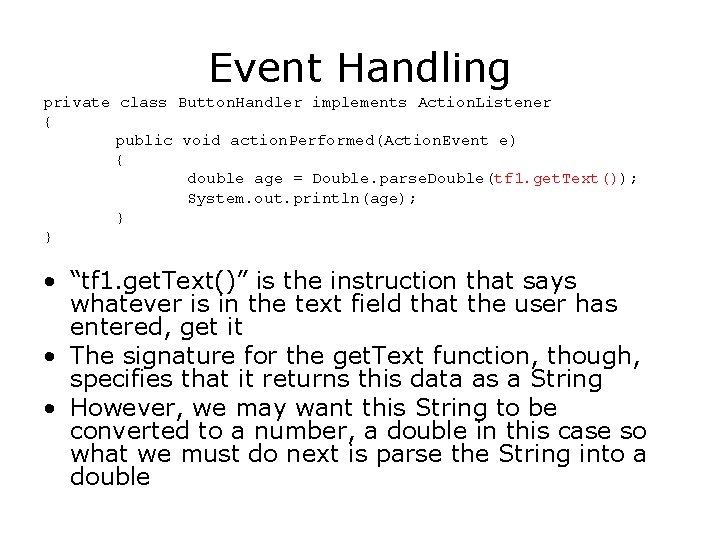
Event Handling private class Button. Handler implements Action. Listener { public void action. Performed(Action. Event e) { double age = Double. parse. Double(tf 1. get. Text()); System. out. println(age); } } • “tf 1. get. Text()” is the instruction that says whatever is in the text field that the user has entered, get it • The signature for the get. Text function, though, specifies that it returns this data as a String • However, we may want this String to be converted to a number, a double in this case so what we must do next is parse the String into a double
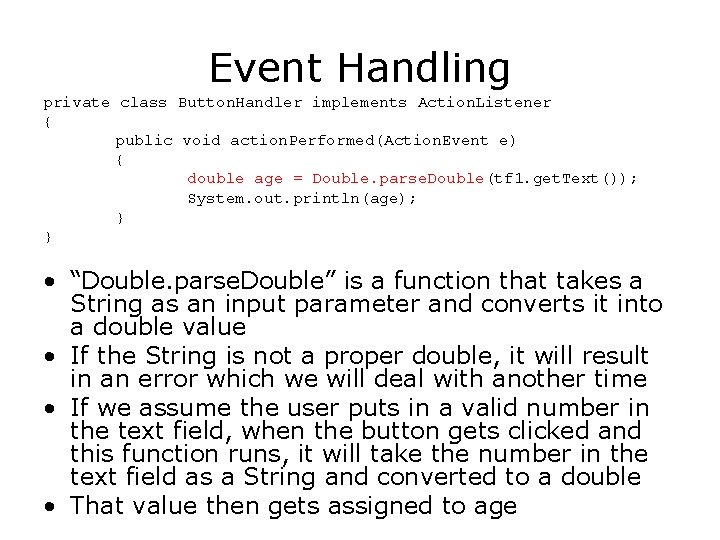
Event Handling private class Button. Handler implements Action. Listener { public void action. Performed(Action. Event e) { double age = Double. parse. Double(tf 1. get. Text()); System. out. println(age); } } • “Double. parse. Double” is a function that takes a String as an input parameter and converts it into a double value • If the String is not a proper double, it will result in an error which we will deal with another time • If we assume the user puts in a valid number in the text field, when the button gets clicked and this function runs, it will take the number in the text field as a String and converted to a double • That value then gets assigned to age
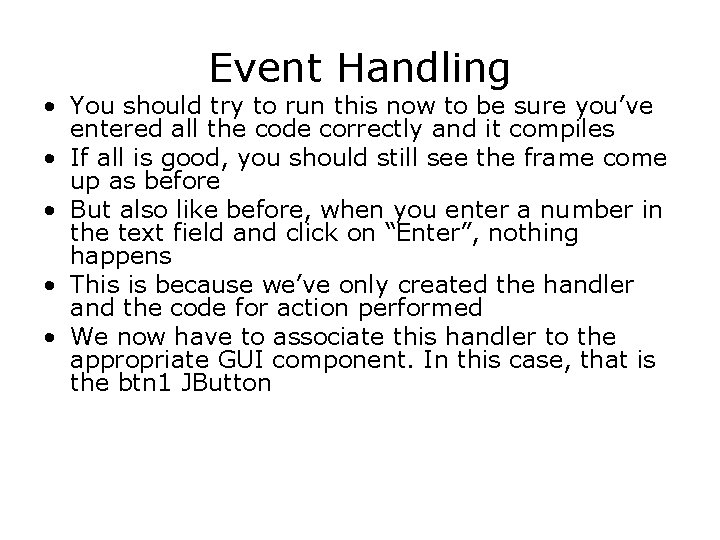
Event Handling • You should try to run this now to be sure you’ve entered all the code correctly and it compiles • If all is good, you should still see the frame come up as before • But also like before, when you enter a number in the text field and click on “Enter”, nothing happens • This is because we’ve only created the handler and the code for action performed • We now have to associate this handler to the appropriate GUI component. In this case, that is the btn 1 JButton
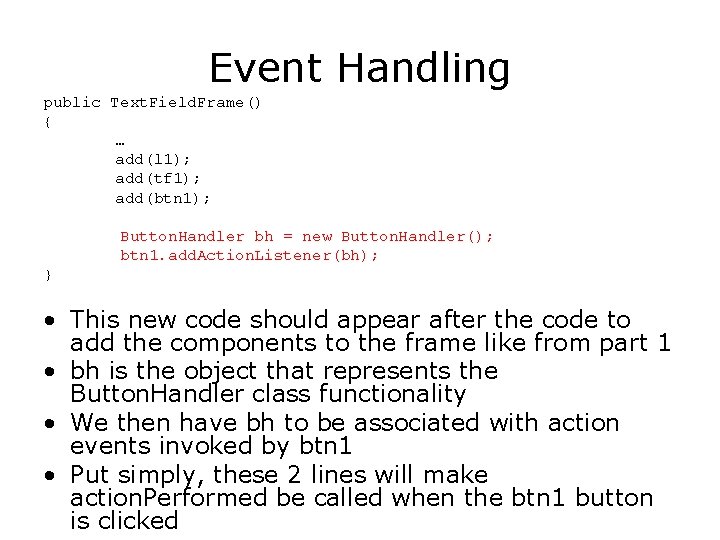
Event Handling public Text. Field. Frame() { … add(l 1); add(tf 1); add(btn 1); Button. Handler bh = new Button. Handler(); btn 1. add. Action. Listener(bh); } • This new code should appear after the code to add the components to the frame like from part 1 • bh is the object that represents the Button. Handler class functionality • We then have bh to be associated with action events invoked by btn 1 • Put simply, these 2 lines will make action. Performed be called when the btn 1 button is clicked
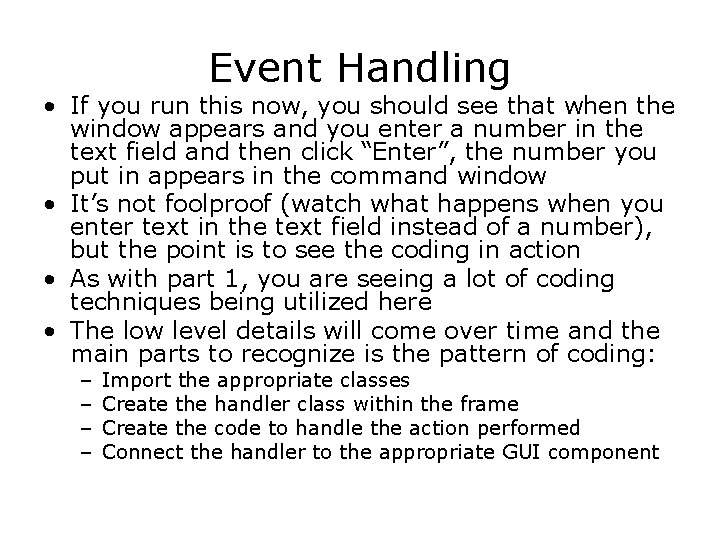
Event Handling • If you run this now, you should see that when the window appears and you enter a number in the text field and then click “Enter”, the number you put in appears in the command window • It’s not foolproof (watch what happens when you enter text in the text field instead of a number), but the point is to see the coding in action • As with part 1, you are seeing a lot of coding techniques being utilized here • The low level details will come over time and the main parts to recognize is the pattern of coding: – – Import the appropriate classes Create the handler class within the frame Create the code to handle the action performed Connect the handler to the appropriate GUI component
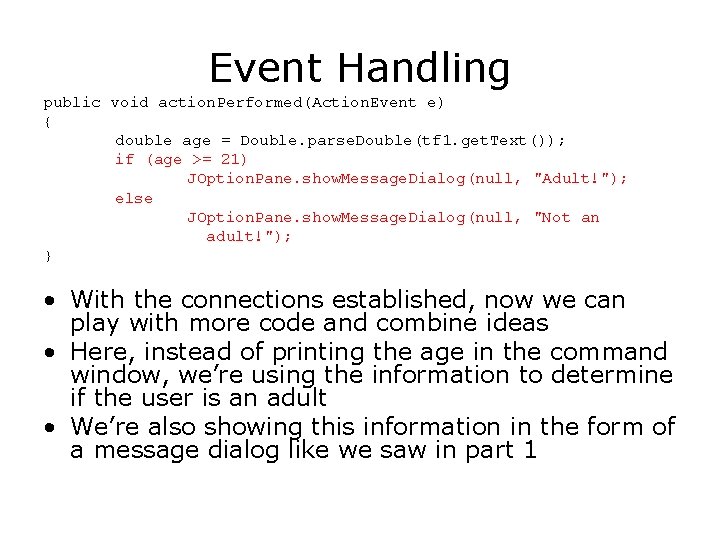
Event Handling public void action. Performed(Action. Event e) { double age = Double. parse. Double(tf 1. get. Text()); if (age >= 21) JOption. Pane. show. Message. Dialog(null, "Adult!"); else JOption. Pane. show. Message. Dialog(null, "Not an adult!"); } • With the connections established, now we can play with more code and combine ideas • Here, instead of printing the age in the command window, we’re using the information to determine if the user is an adult • We’re also showing this information in the form of a message dialog like we saw in part 1
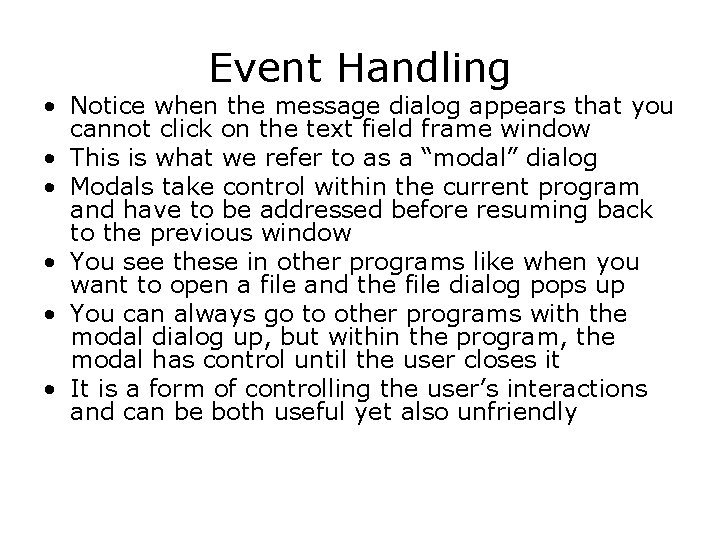
Event Handling • Notice when the message dialog appears that you cannot click on the text field frame window • This is what we refer to as a “modal” dialog • Modals take control within the current program and have to be addressed before resuming back to the previous window • You see these in other programs like when you want to open a file and the file dialog pops up • You can always go to other programs with the modal dialog up, but within the program, the modal has control until the user closes it • It is a form of controlling the user’s interactions and can be both useful yet also unfriendly
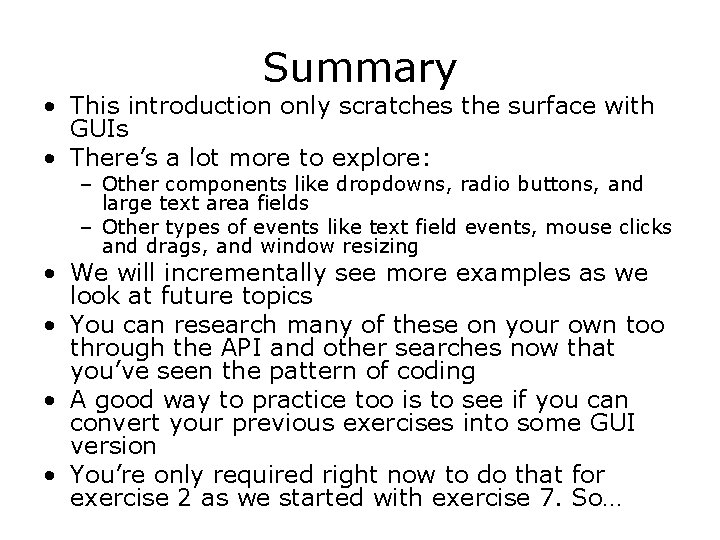
Summary • This introduction only scratches the surface with GUIs • There’s a lot more to explore: – Other components like dropdowns, radio buttons, and large text area fields – Other types of events like text field events, mouse clicks and drags, and window resizing • We will incrementally see more examples as we look at future topics • You can research many of these on your own too through the API and other searches now that you’ve seen the pattern of coding • A good way to practice too is to see if you can convert your previous exercises into some GUI version • You’re only required right now to do that for exercise 2 as we started with exercise 7. So…
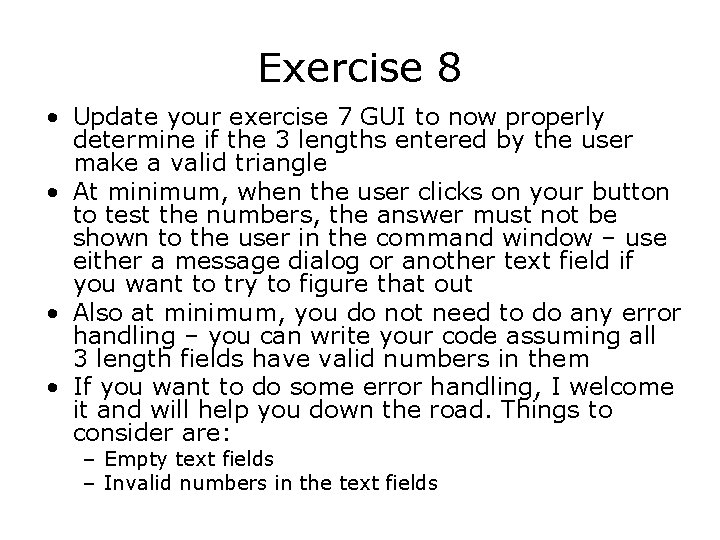
Exercise 8 • Update your exercise 7 GUI to now properly determine if the 3 lengths entered by the user make a valid triangle • At minimum, when the user clicks on your button to test the numbers, the answer must not be shown to the user in the command window – use either a message dialog or another text field if you want to try to figure that out • Also at minimum, you do not need to do any error handling – you can write your code assuming all 3 length fields have valid numbers in them • If you want to do some error handling, I welcome it and will help you down the road. Things to consider are: – Empty text fields – Invalid numbers in the text fields
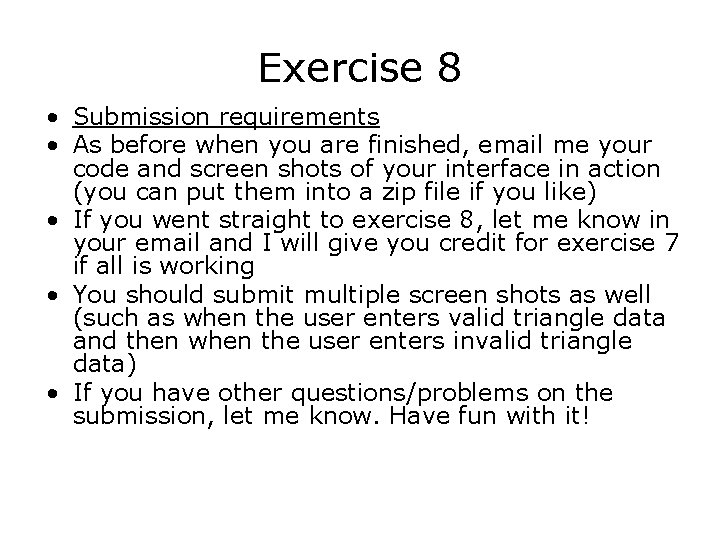
Exercise 8 • Submission requirements • As before when you are finished, email me your code and screen shots of your interface in action (you can put them into a zip file if you like) • If you went straight to exercise 8, let me know in your email and I will give you credit for exercise 7 if all is working • You should submit multiple screen shots as well (such as when the user enters valid triangle data and then when the user enters invalid triangle data) • If you have other questions/problems on the submission, let me know. Have fun with it!