Generate Statement A generate statement provides a mechanism
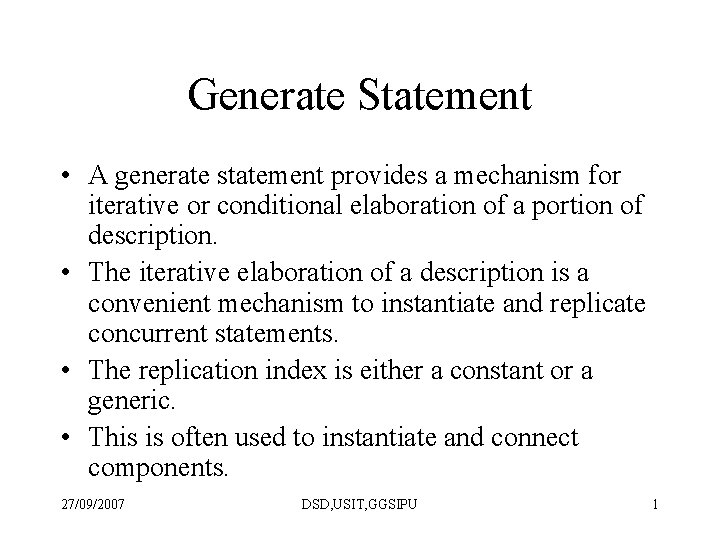
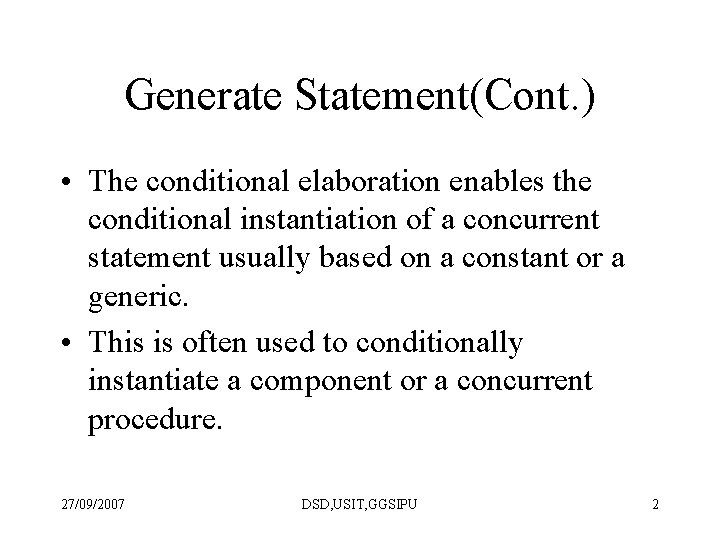
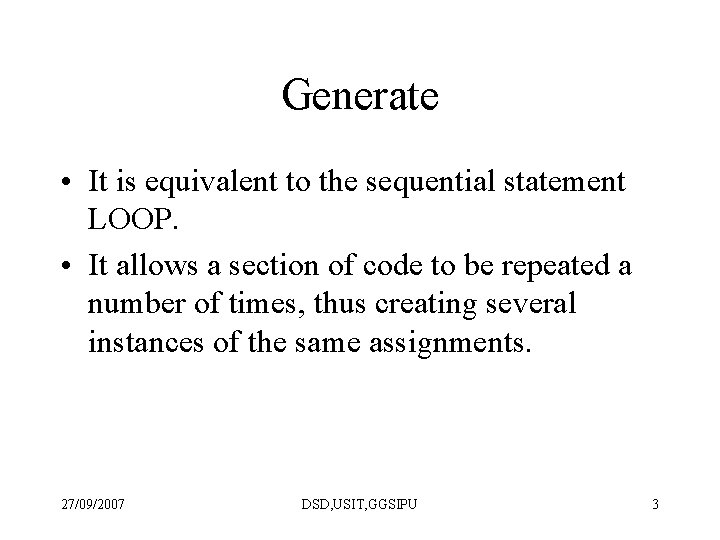
![The syntax Generate_label: generation_scheme generate {concurrent statement} End generate [generate_label]; 27/09/2007 DSD, USIT, GGSIPU The syntax Generate_label: generation_scheme generate {concurrent statement} End generate [generate_label]; 27/09/2007 DSD, USIT, GGSIPU](https://slidetodoc.com/presentation_image_h2/fc76a7a92c6a884a80306fc3627b3093/image-4.jpg)
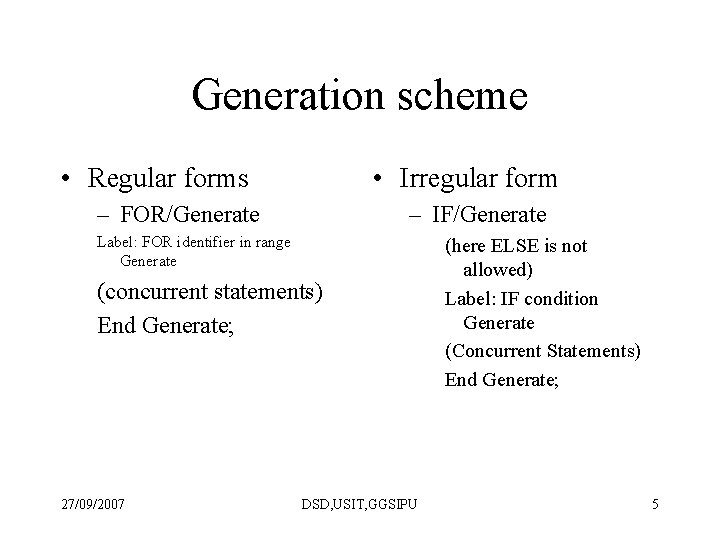
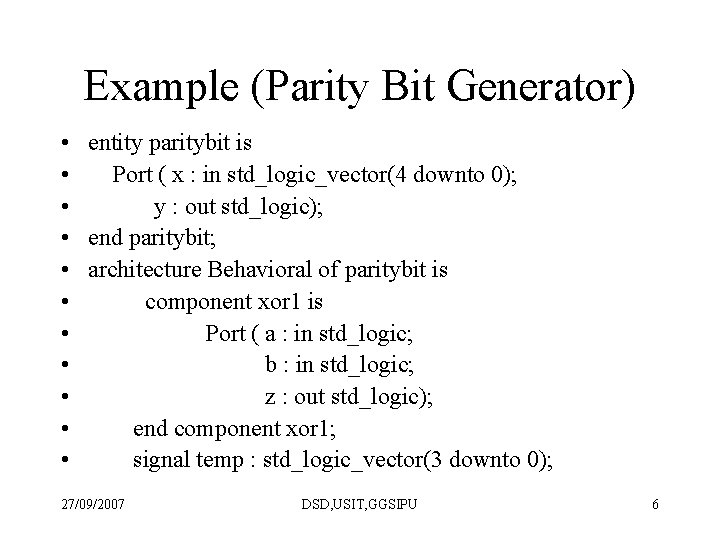
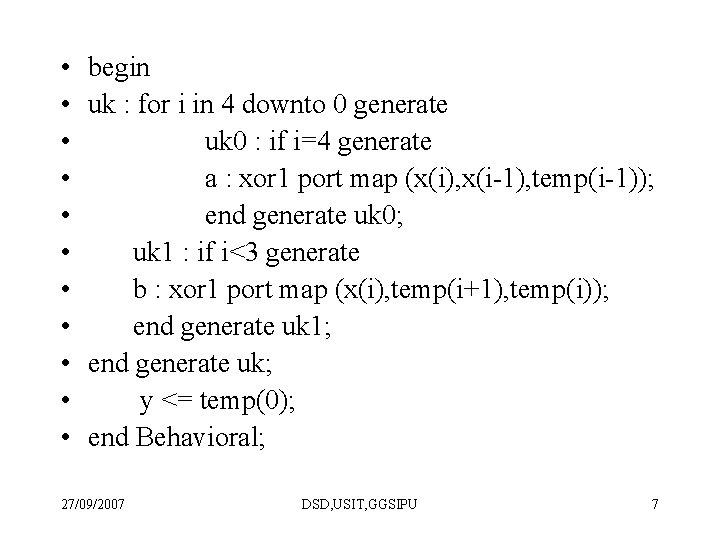
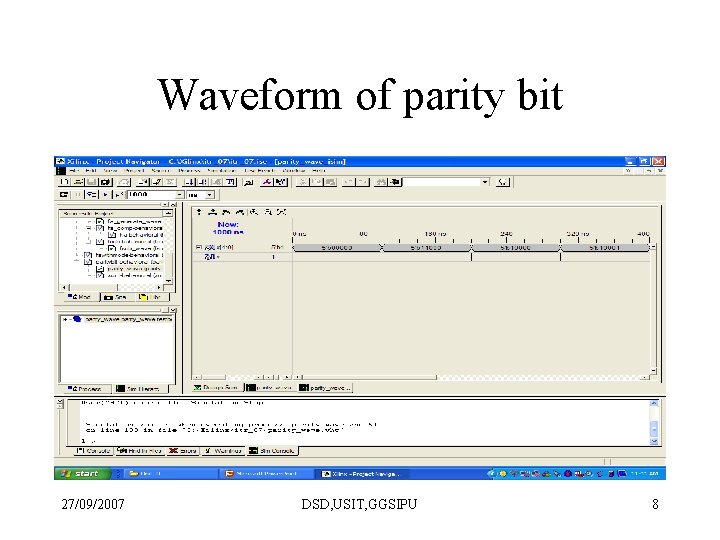
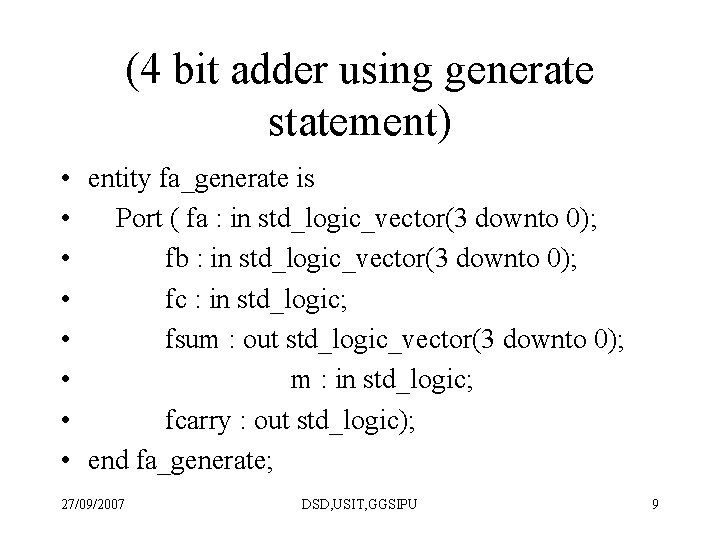
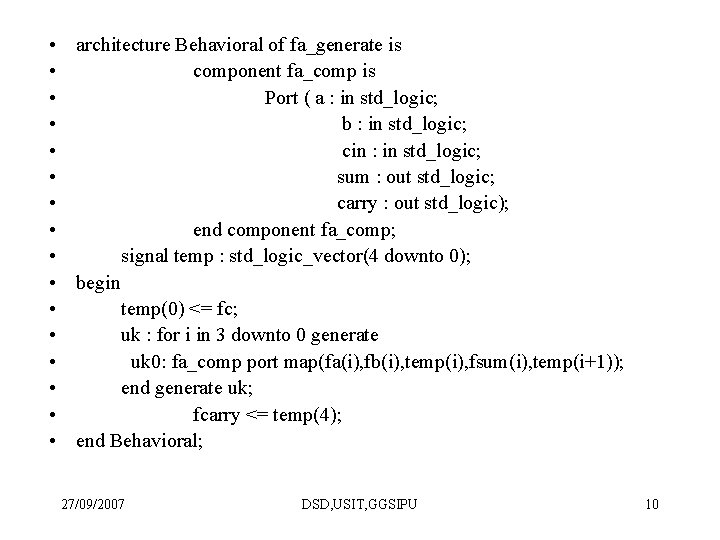
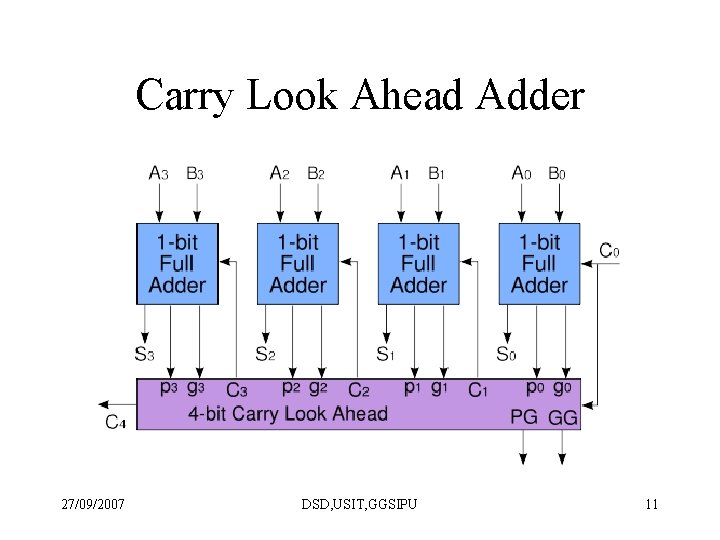
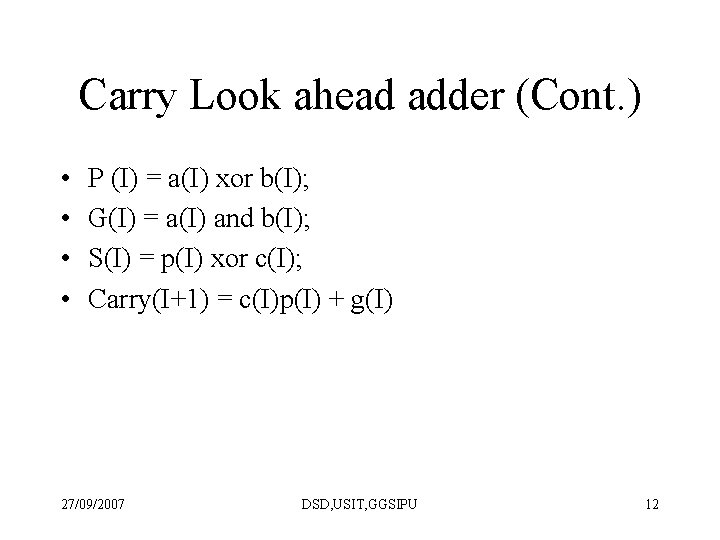
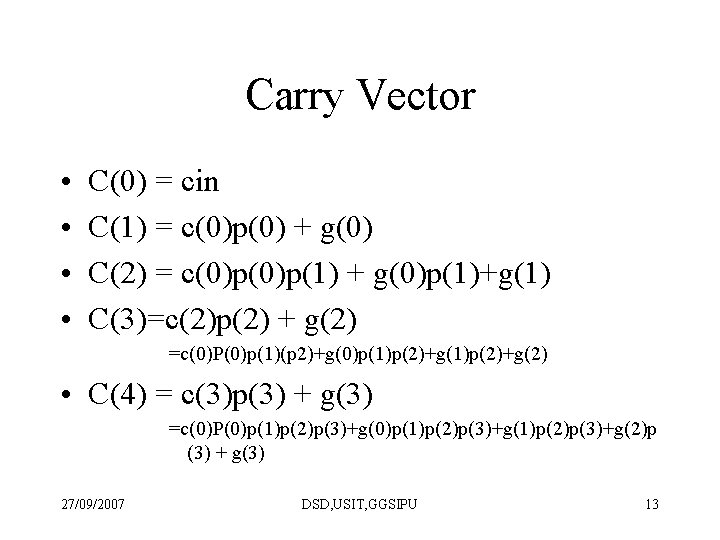
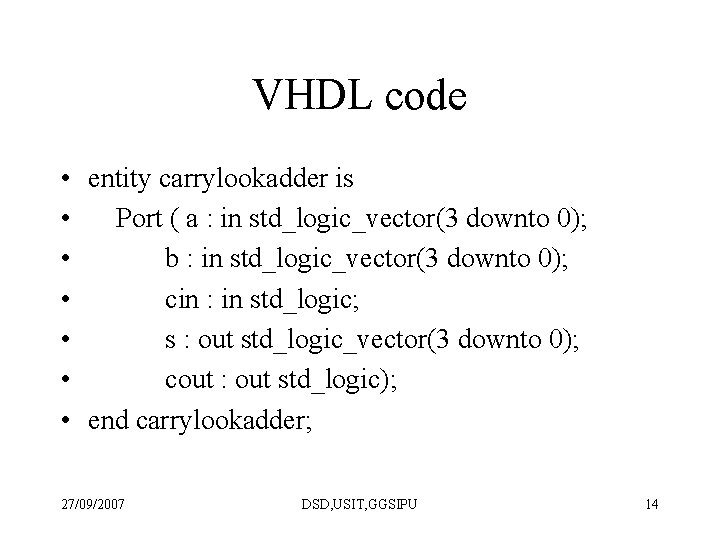
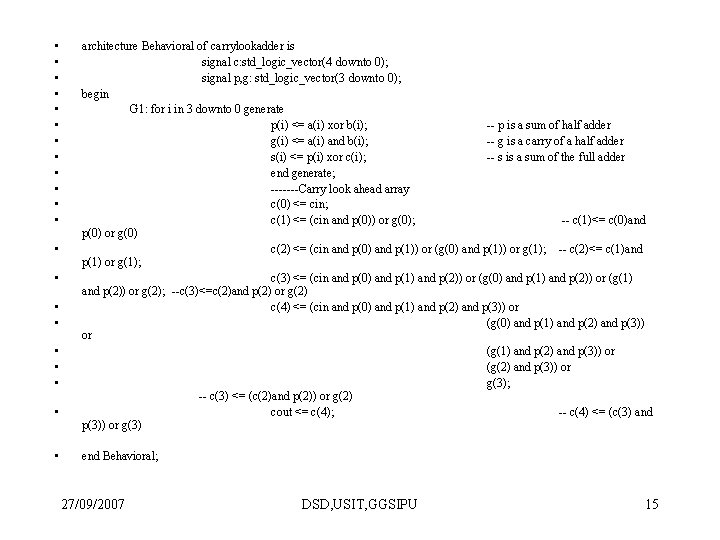
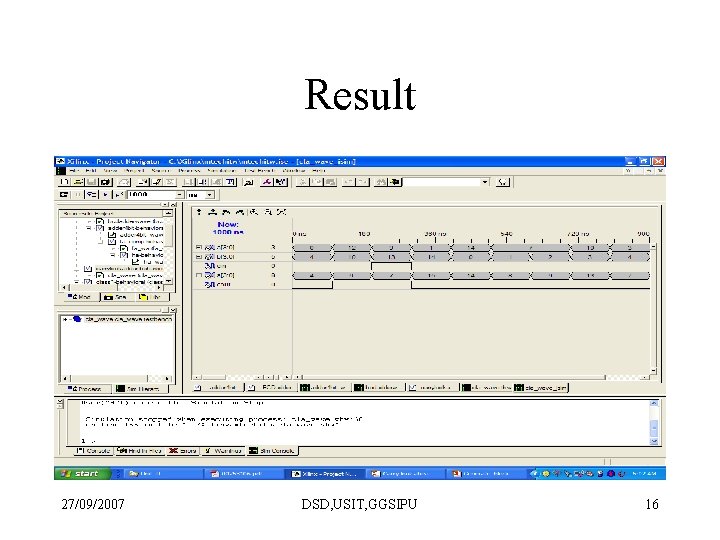
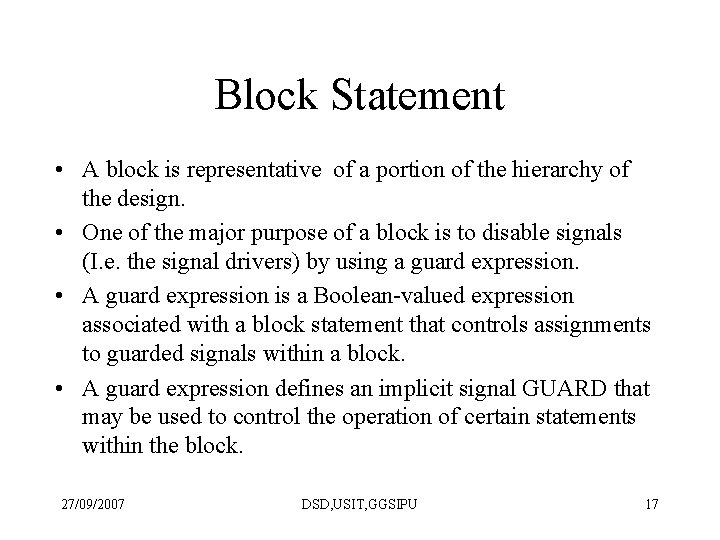
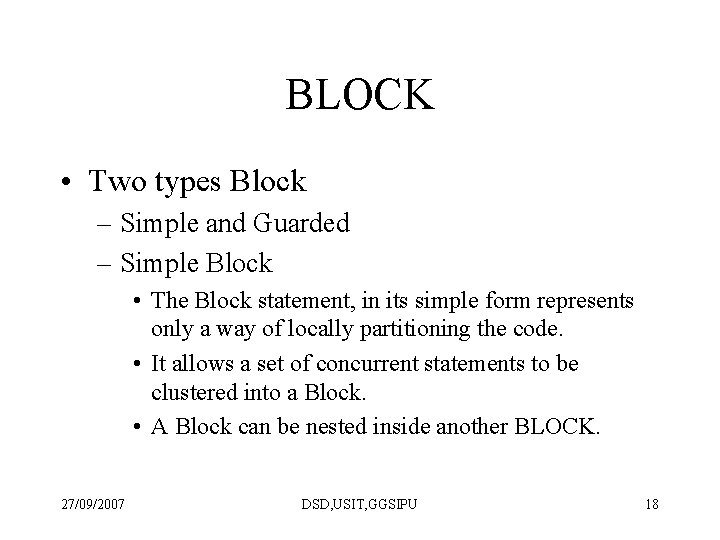
![Block Syntax (Simple) Label : BLOCK [Declartive part] Begin (Concurrent statements) End BLOCK label; Block Syntax (Simple) Label : BLOCK [Declartive part] Begin (Concurrent statements) End BLOCK label;](https://slidetodoc.com/presentation_image_h2/fc76a7a92c6a884a80306fc3627b3093/image-19.jpg)
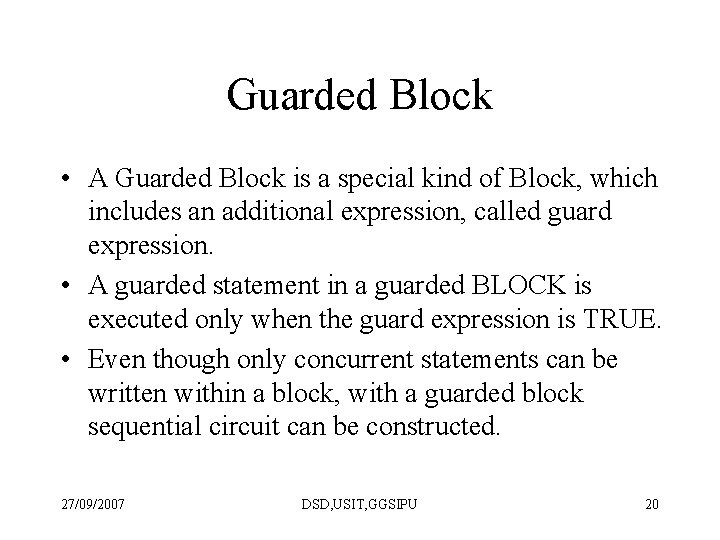
![Guarded Block syntax Label : BLOCK (guard expression) [Declarative part] Begin (Concurrent guarded and Guarded Block syntax Label : BLOCK (guard expression) [Declarative part] Begin (Concurrent guarded and](https://slidetodoc.com/presentation_image_h2/fc76a7a92c6a884a80306fc3627b3093/image-21.jpg)
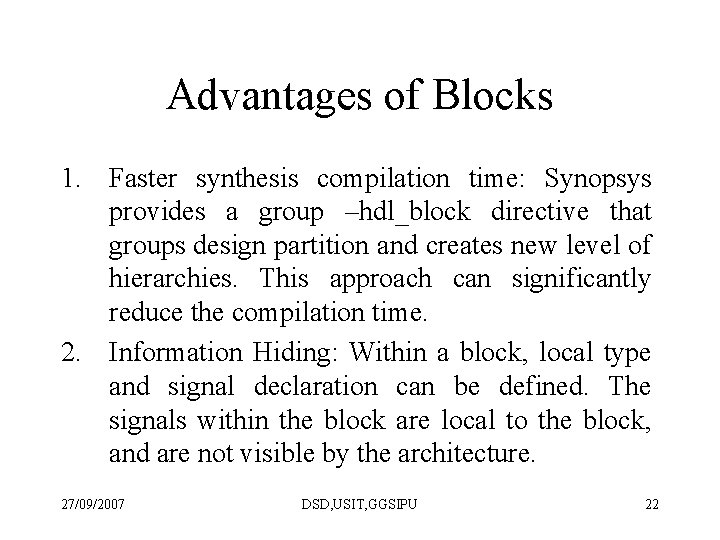
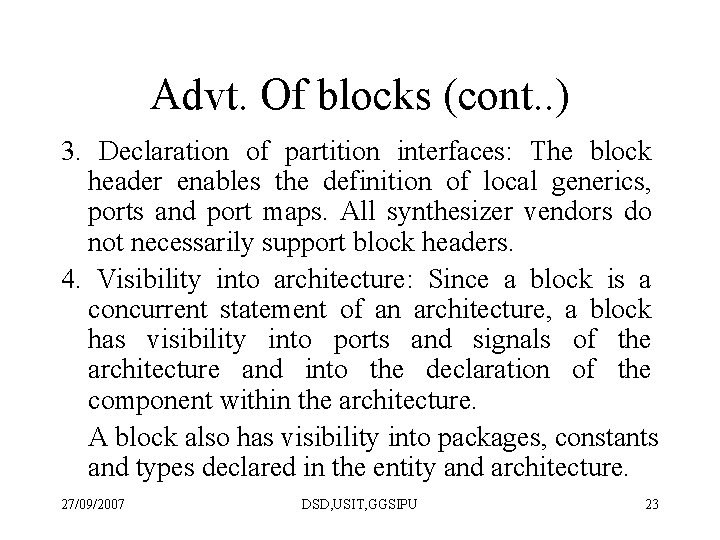
- Slides: 23
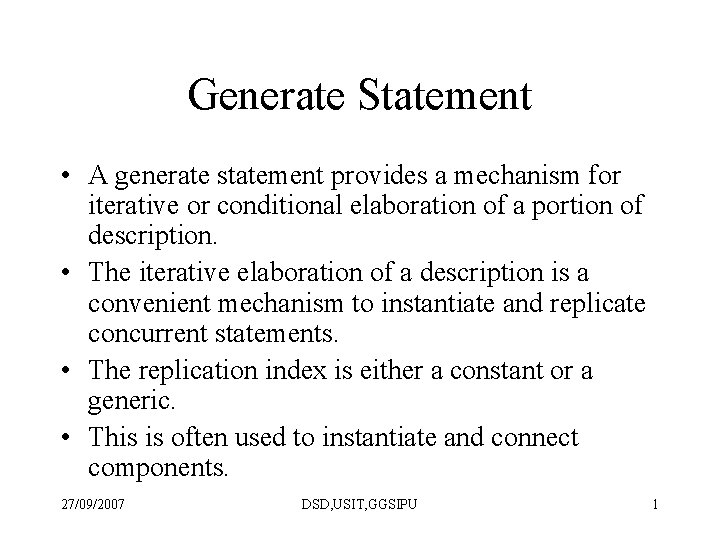
Generate Statement • A generate statement provides a mechanism for iterative or conditional elaboration of a portion of description. • The iterative elaboration of a description is a convenient mechanism to instantiate and replicate concurrent statements. • The replication index is either a constant or a generic. • This is often used to instantiate and connect components. 27/09/2007 DSD, USIT, GGSIPU 1
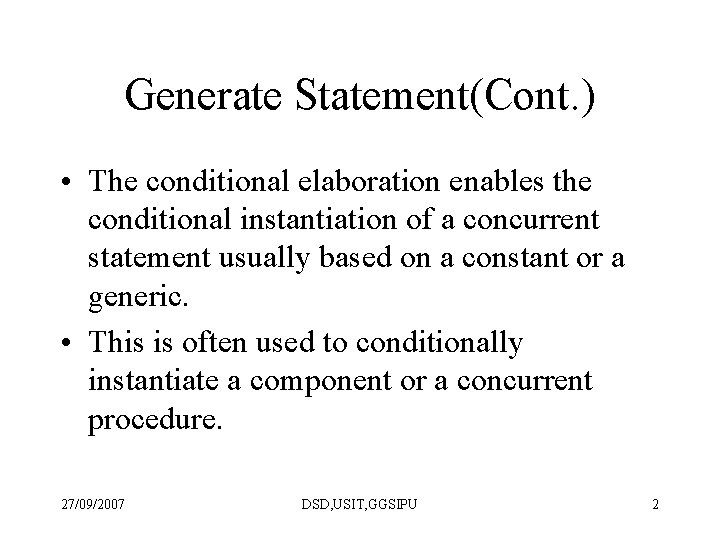
Generate Statement(Cont. ) • The conditional elaboration enables the conditional instantiation of a concurrent statement usually based on a constant or a generic. • This is often used to conditionally instantiate a component or a concurrent procedure. 27/09/2007 DSD, USIT, GGSIPU 2
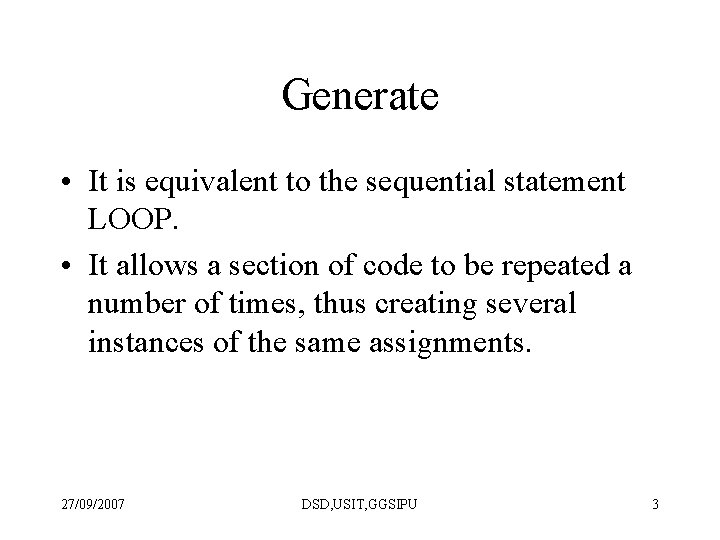
Generate • It is equivalent to the sequential statement LOOP. • It allows a section of code to be repeated a number of times, thus creating several instances of the same assignments. 27/09/2007 DSD, USIT, GGSIPU 3
![The syntax Generatelabel generationscheme generate concurrent statement End generate generatelabel 27092007 DSD USIT GGSIPU The syntax Generate_label: generation_scheme generate {concurrent statement} End generate [generate_label]; 27/09/2007 DSD, USIT, GGSIPU](https://slidetodoc.com/presentation_image_h2/fc76a7a92c6a884a80306fc3627b3093/image-4.jpg)
The syntax Generate_label: generation_scheme generate {concurrent statement} End generate [generate_label]; 27/09/2007 DSD, USIT, GGSIPU 4
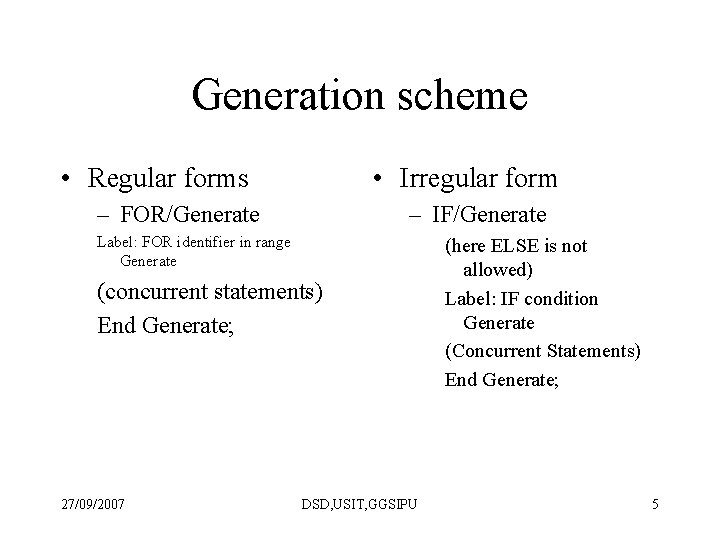
Generation scheme • Regular forms • Irregular form – FOR/Generate – IF/Generate Label: FOR identifier in range Generate (concurrent statements) End Generate; 27/09/2007 DSD, USIT, GGSIPU (here ELSE is not allowed) Label: IF condition Generate (Concurrent Statements) End Generate; 5
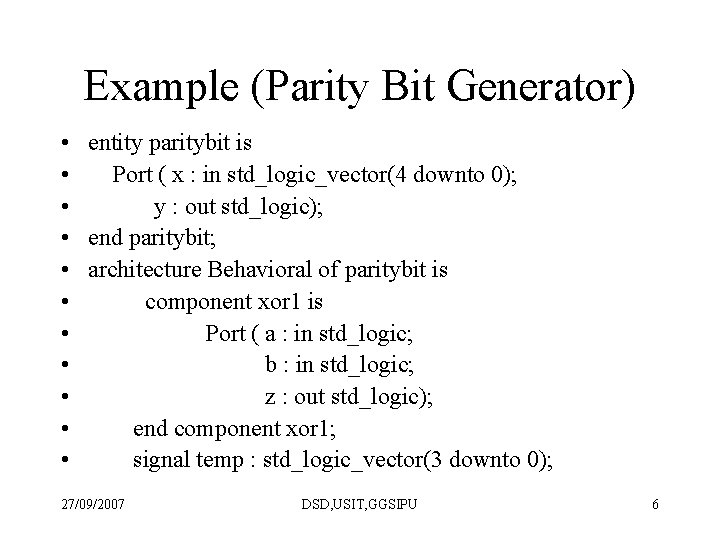
Example (Parity Bit Generator) • entity paritybit is • Port ( x : in std_logic_vector(4 downto 0); • y : out std_logic); • end paritybit; • architecture Behavioral of paritybit is • component xor 1 is • Port ( a : in std_logic; • b : in std_logic; • z : out std_logic); • end component xor 1; • signal temp : std_logic_vector(3 downto 0); 27/09/2007 DSD, USIT, GGSIPU 6
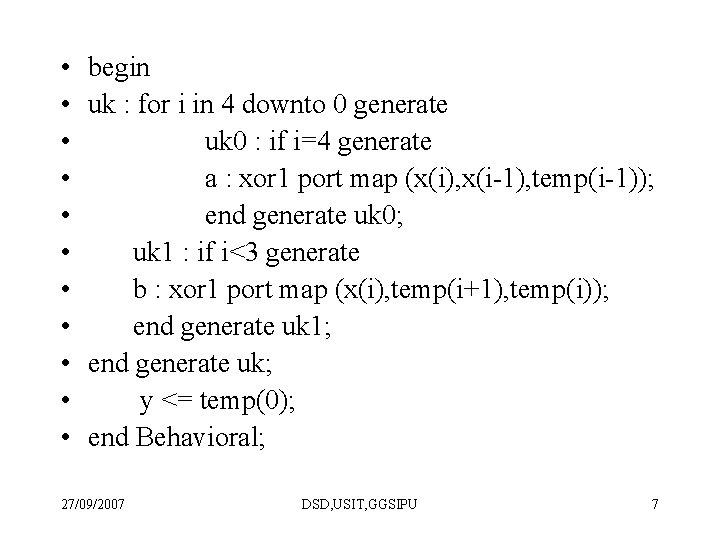
• • • begin uk : for i in 4 downto 0 generate uk 0 : if i=4 generate a : xor 1 port map (x(i), x(i-1), temp(i-1)); end generate uk 0; uk 1 : if i<3 generate b : xor 1 port map (x(i), temp(i+1), temp(i)); end generate uk 1; end generate uk; y <= temp(0); end Behavioral; 27/09/2007 DSD, USIT, GGSIPU 7
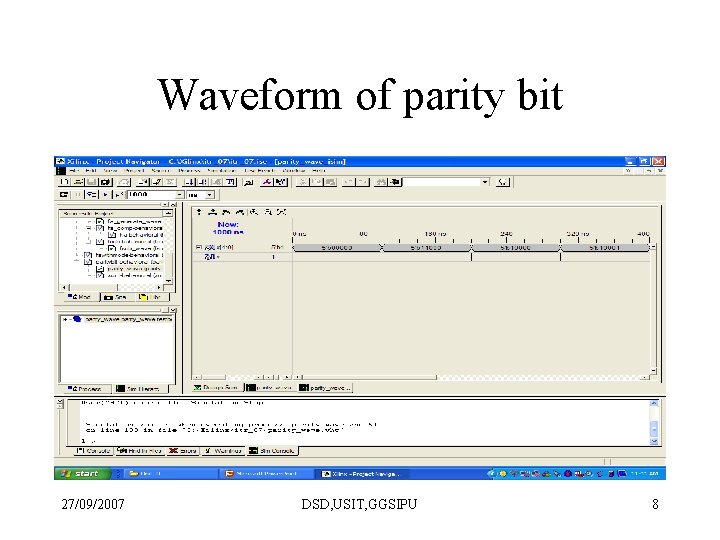
Waveform of parity bit 27/09/2007 DSD, USIT, GGSIPU 8
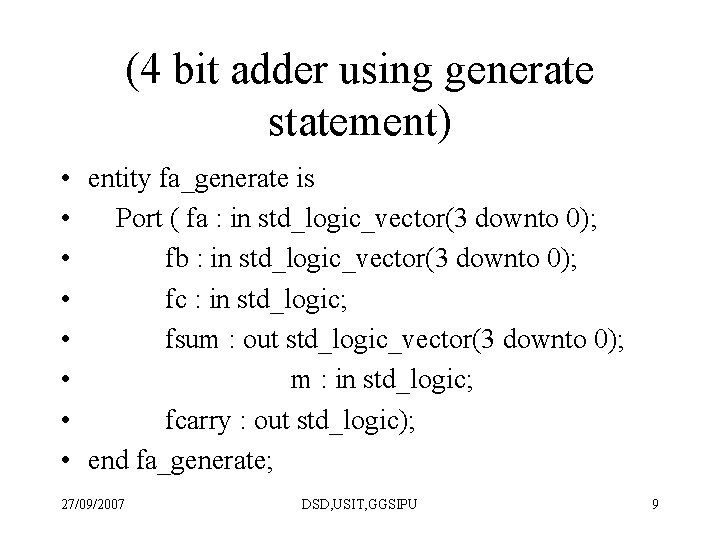
(4 bit adder using generate statement) • entity fa_generate is • Port ( fa : in std_logic_vector(3 downto 0); • fb : in std_logic_vector(3 downto 0); • fc : in std_logic; • fsum : out std_logic_vector(3 downto 0); • m : in std_logic; • fcarry : out std_logic); • end fa_generate; 27/09/2007 DSD, USIT, GGSIPU 9
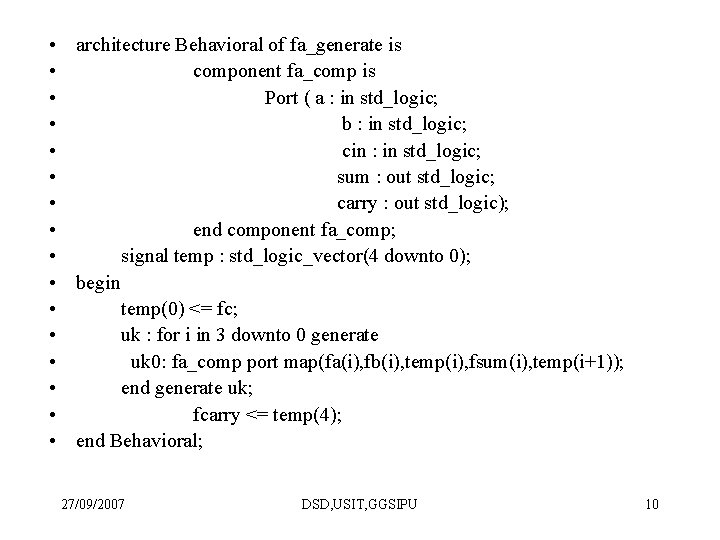
• architecture Behavioral of fa_generate is • component fa_comp is • Port ( a : in std_logic; • b : in std_logic; • cin : in std_logic; • sum : out std_logic; • carry : out std_logic); • end component fa_comp; • signal temp : std_logic_vector(4 downto 0); • begin • temp(0) <= fc; • uk : for i in 3 downto 0 generate • uk 0: fa_comp port map(fa(i), fb(i), temp(i), fsum(i), temp(i+1)); • end generate uk; • fcarry <= temp(4); • end Behavioral; 27/09/2007 DSD, USIT, GGSIPU 10
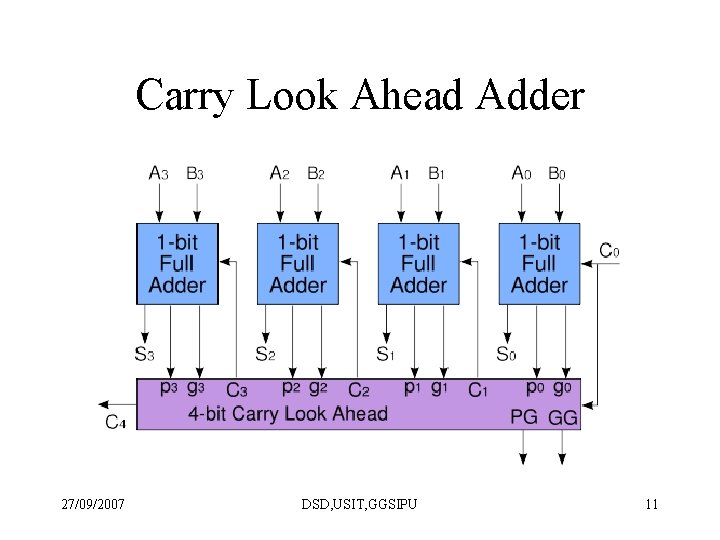
Carry Look Ahead Adder 27/09/2007 DSD, USIT, GGSIPU 11
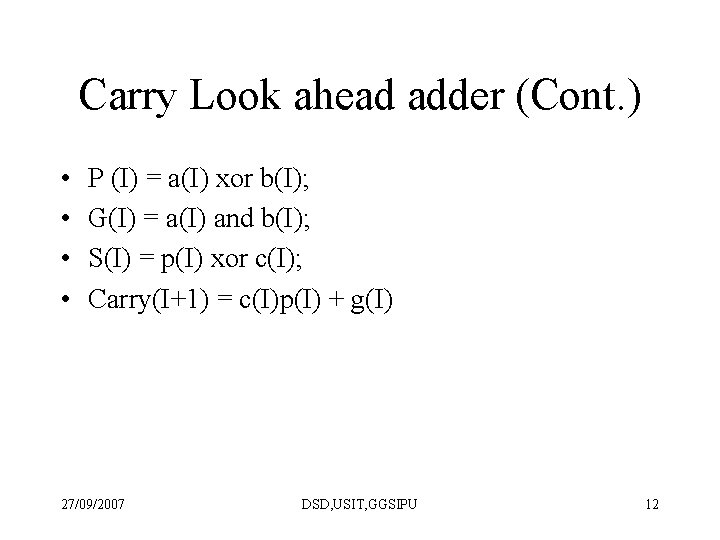
Carry Look ahead adder (Cont. ) • • P (I) = a(I) xor b(I); G(I) = a(I) and b(I); S(I) = p(I) xor c(I); Carry(I+1) = c(I)p(I) + g(I) 27/09/2007 DSD, USIT, GGSIPU 12
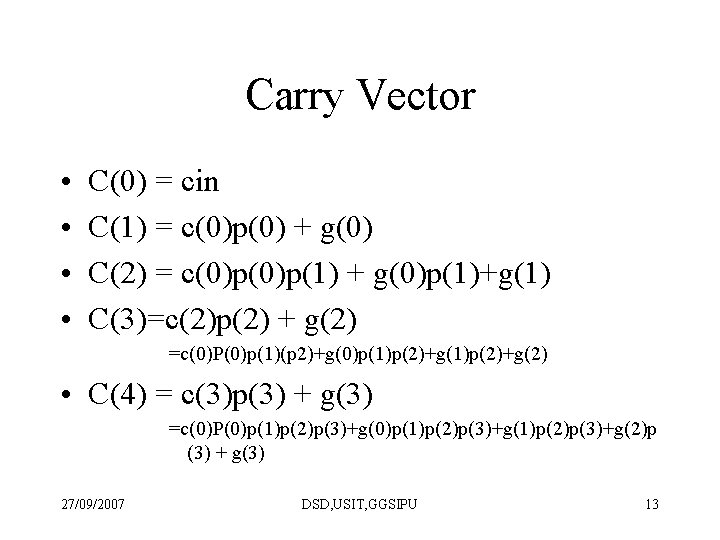
Carry Vector • • C(0) = cin C(1) = c(0)p(0) + g(0) C(2) = c(0)p(1) + g(0)p(1)+g(1) C(3)=c(2)p(2) + g(2) =c(0)P(0)p(1)(p 2)+g(0)p(1)p(2)+g(2) • C(4) = c(3)p(3) + g(3) =c(0)P(0)p(1)p(2)p(3)+g(1)p(2)p(3)+g(2)p (3) + g(3) 27/09/2007 DSD, USIT, GGSIPU 13
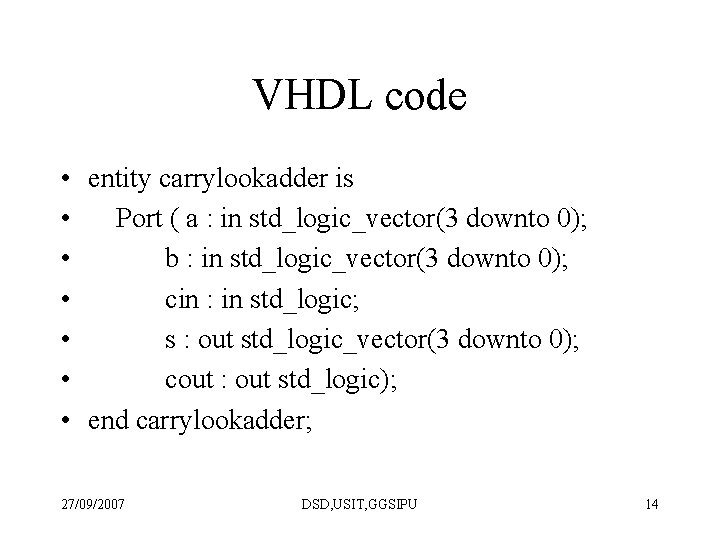
VHDL code • entity carrylookadder is • Port ( a : in std_logic_vector(3 downto 0); • b : in std_logic_vector(3 downto 0); • cin : in std_logic; • s : out std_logic_vector(3 downto 0); • cout : out std_logic); • end carrylookadder; 27/09/2007 DSD, USIT, GGSIPU 14
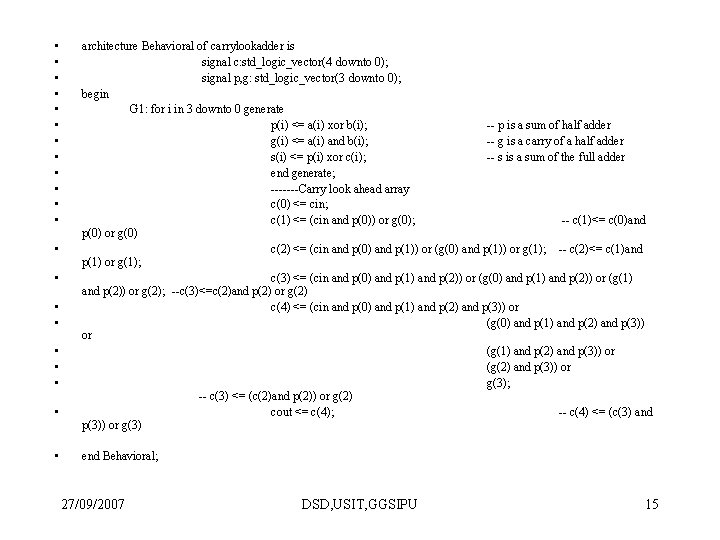
• • • • • • architecture Behavioral of carrylookadder is signal c: std_logic_vector(4 downto 0); signal p, g: std_logic_vector(3 downto 0); begin G 1: for i in 3 downto 0 generate p(i) <= a(i) xor b(i); -- p is a sum of half adder g(i) <= a(i) and b(i); -- g is a carry of a half adder s(i) <= p(i) xor c(i); -- s is a sum of the full adder end generate; -------Carry look ahead array c(0) <= cin; c(1) <= (cin and p(0)) or g(0); -- c(1)<= c(0)and p(0) or g(0) c(2) <= (cin and p(0) and p(1)) or (g(0) and p(1)) or g(1); -- c(2)<= c(1)and p(1) or g(1); c(3) <= (cin and p(0) and p(1) and p(2)) or (g(1) and p(2)) or g(2); --c(3)<=c(2)and p(2) or g(2) c(4) <= (cin and p(0) and p(1) and p(2) and p(3)) or (g(1) and p(2) and p(3)) or (g(2) and p(3)) or g(3); -- c(3) <= (c(2)and p(2)) or g(2) cout <= c(4); -- c(4) <= (c(3) and p(3)) or g(3) end Behavioral; 27/09/2007 DSD, USIT, GGSIPU 15
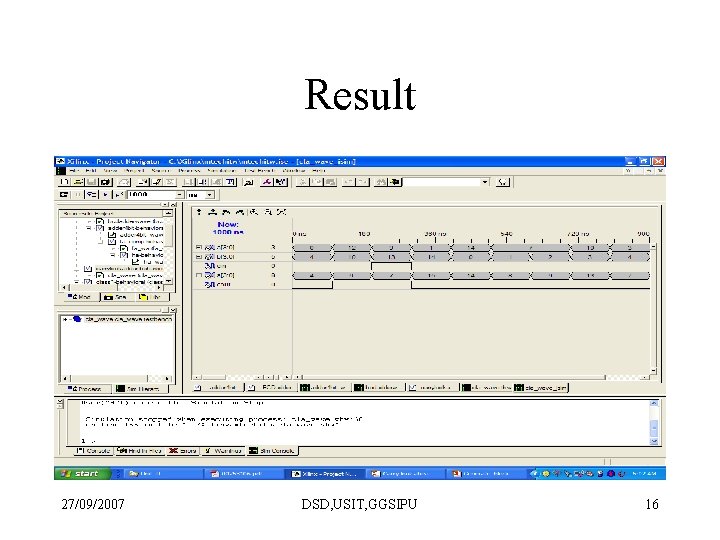
Result 27/09/2007 DSD, USIT, GGSIPU 16
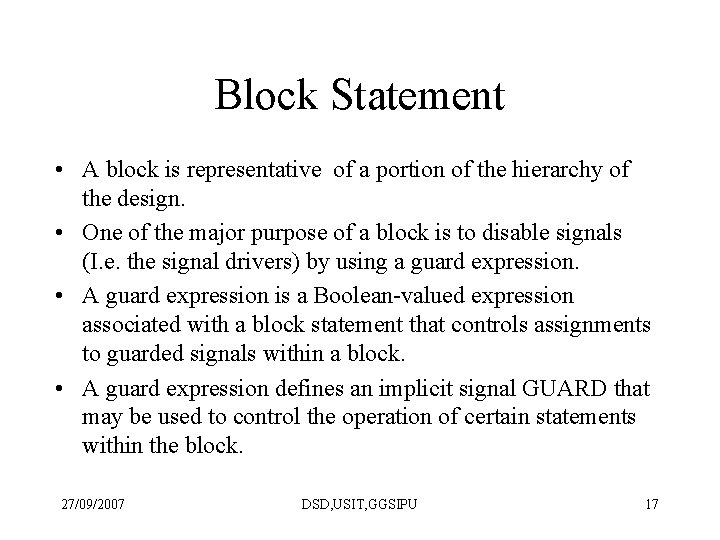
Block Statement • A block is representative of a portion of the hierarchy of the design. • One of the major purpose of a block is to disable signals (I. e. the signal drivers) by using a guard expression. • A guard expression is a Boolean-valued expression associated with a block statement that controls assignments to guarded signals within a block. • A guard expression defines an implicit signal GUARD that may be used to control the operation of certain statements within the block. 27/09/2007 DSD, USIT, GGSIPU 17
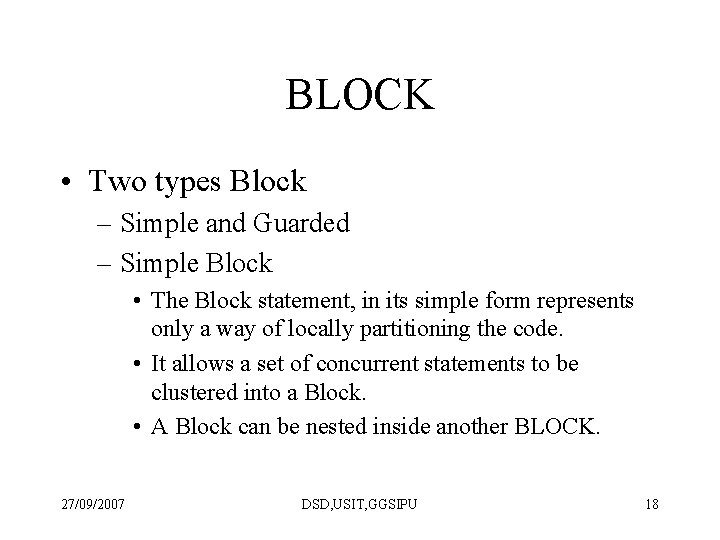
BLOCK • Two types Block – Simple and Guarded – Simple Block • The Block statement, in its simple form represents only a way of locally partitioning the code. • It allows a set of concurrent statements to be clustered into a Block. • A Block can be nested inside another BLOCK. 27/09/2007 DSD, USIT, GGSIPU 18
![Block Syntax Simple Label BLOCK Declartive part Begin Concurrent statements End BLOCK label Block Syntax (Simple) Label : BLOCK [Declartive part] Begin (Concurrent statements) End BLOCK label;](https://slidetodoc.com/presentation_image_h2/fc76a7a92c6a884a80306fc3627b3093/image-19.jpg)
Block Syntax (Simple) Label : BLOCK [Declartive part] Begin (Concurrent statements) End BLOCK label; 27/09/2007 DSD, USIT, GGSIPU 19
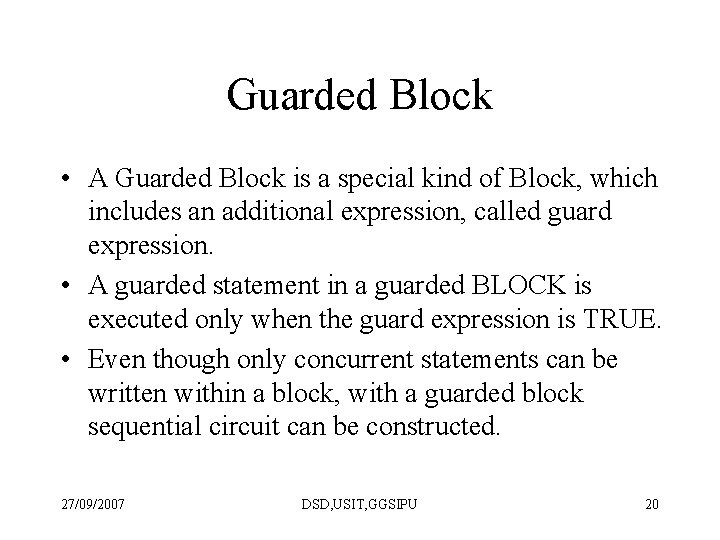
Guarded Block • A Guarded Block is a special kind of Block, which includes an additional expression, called guard expression. • A guarded statement in a guarded BLOCK is executed only when the guard expression is TRUE. • Even though only concurrent statements can be written within a block, with a guarded block sequential circuit can be constructed. 27/09/2007 DSD, USIT, GGSIPU 20
![Guarded Block syntax Label BLOCK guard expression Declarative part Begin Concurrent guarded and Guarded Block syntax Label : BLOCK (guard expression) [Declarative part] Begin (Concurrent guarded and](https://slidetodoc.com/presentation_image_h2/fc76a7a92c6a884a80306fc3627b3093/image-21.jpg)
Guarded Block syntax Label : BLOCK (guard expression) [Declarative part] Begin (Concurrent guarded and unguarded statements) End BLOCK label; 27/09/2007 DSD, USIT, GGSIPU 21
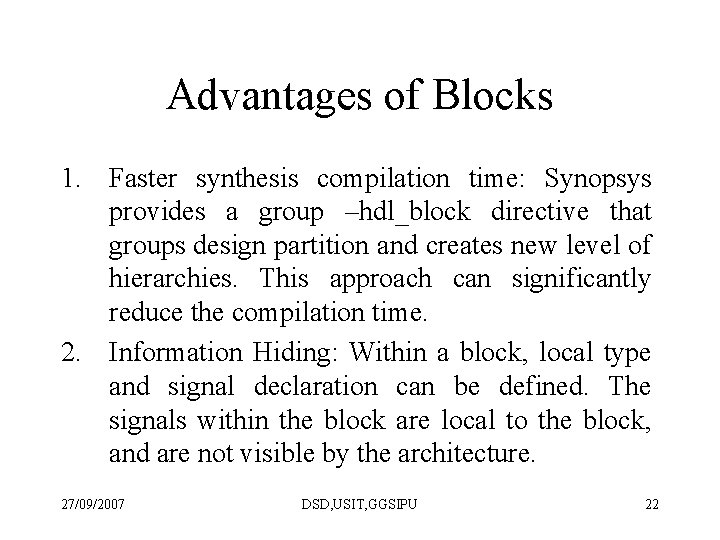
Advantages of Blocks 1. Faster synthesis compilation time: Synopsys provides a group –hdl_block directive that groups design partition and creates new level of hierarchies. This approach can significantly reduce the compilation time. 2. Information Hiding: Within a block, local type and signal declaration can be defined. The signals within the block are local to the block, and are not visible by the architecture. 27/09/2007 DSD, USIT, GGSIPU 22
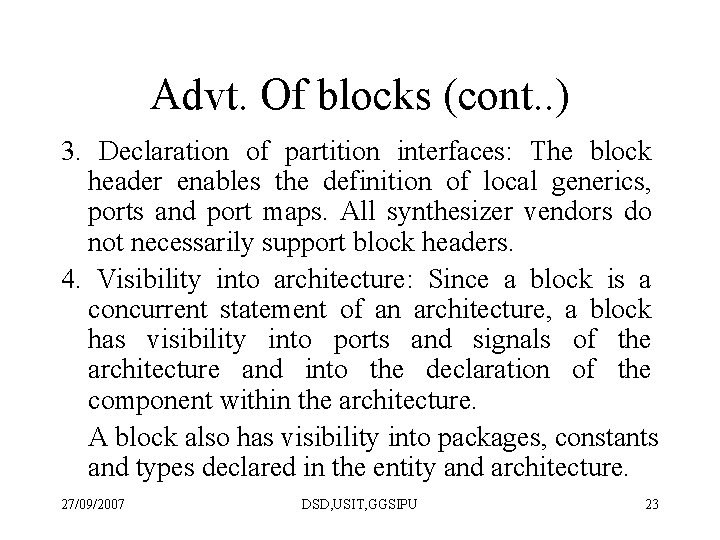
Advt. Of blocks (cont. . ) 3. Declaration of partition interfaces: The block header enables the definition of local generics, ports and port maps. All synthesizer vendors do not necessarily support block headers. 4. Visibility into architecture: Since a block is a concurrent statement of an architecture, a block has visibility into ports and signals of the architecture and into the declaration of the component within the architecture. A block also has visibility into packages, constants and types declared in the entity and architecture. 27/09/2007 DSD, USIT, GGSIPU 23
Generate statement
Generate a thesis statement
Phone number generator club080
Masalah ruang keadaan dan pencarian
How to generate random numbers in spss
Contoh generate and test
Verilog parameters
Pure aloha vs slotted aloha
Axiom of urban economics
Which grammar generates regular language
Purdom power plant
Generate and conceptualize artistic ideas and work
Combination table in product design
Cobol xml
8051
Recent amendments in companies act
How do business processes generate value
Generate and test heuristic search
Generate synonym
Define generate concepts
Synonym of objective
E-dis facility
Generate synonym
Generate ash report for sql_id