Design principles SOLID Design principles circle class Circle
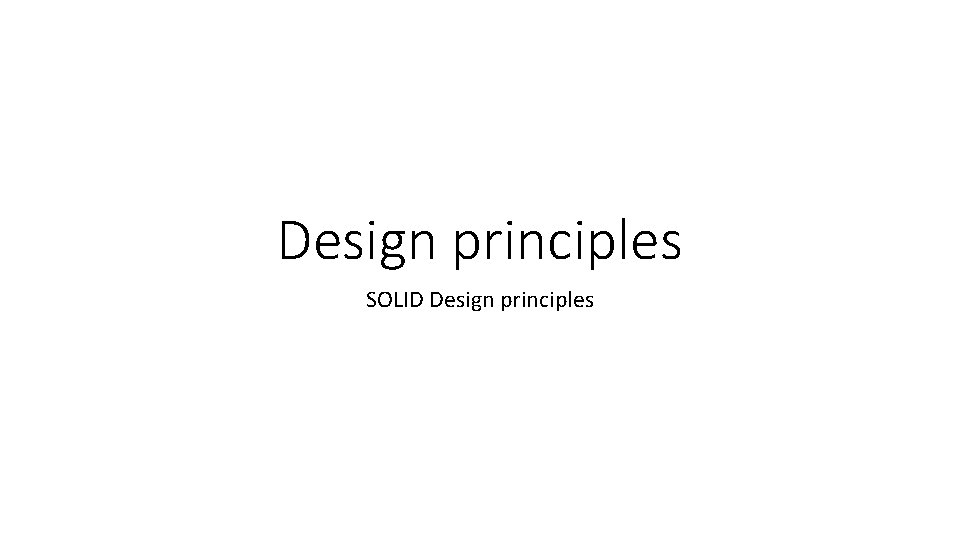
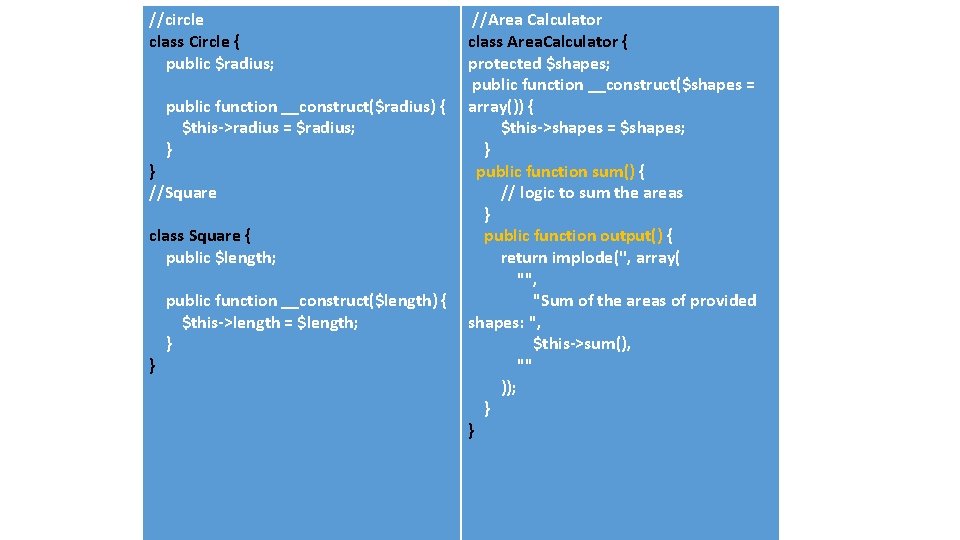
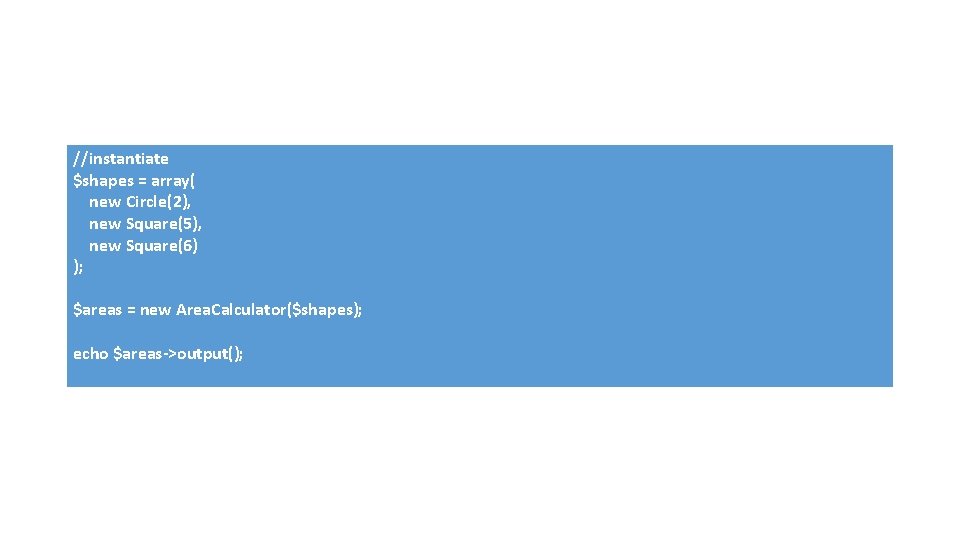
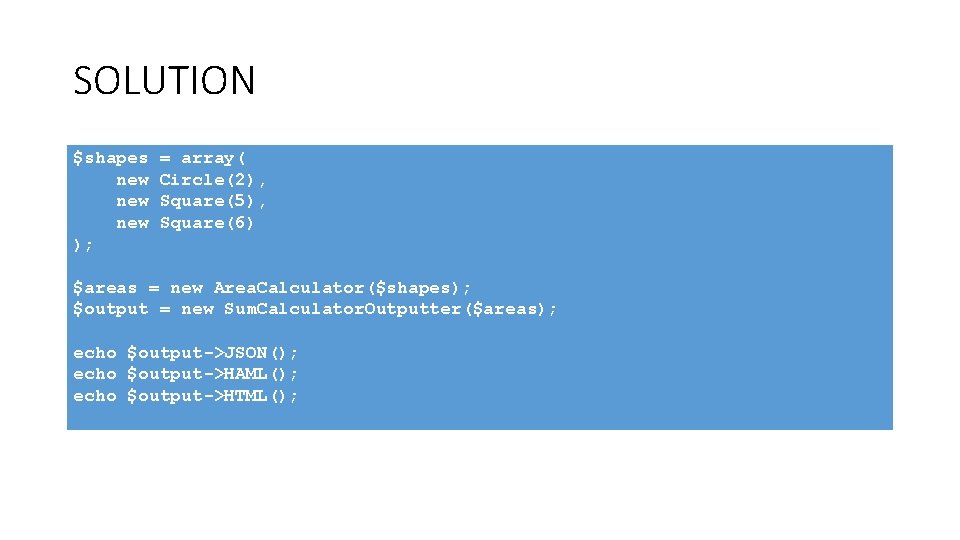
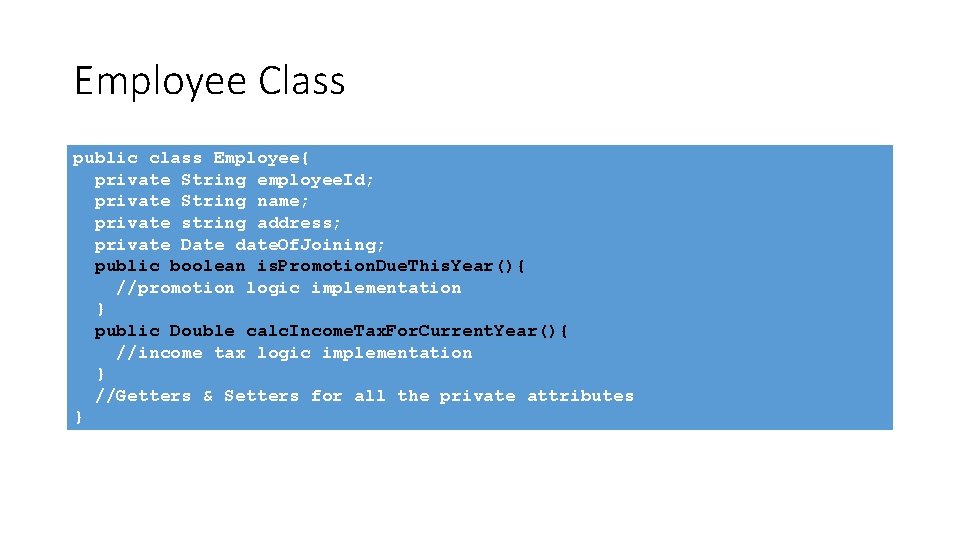
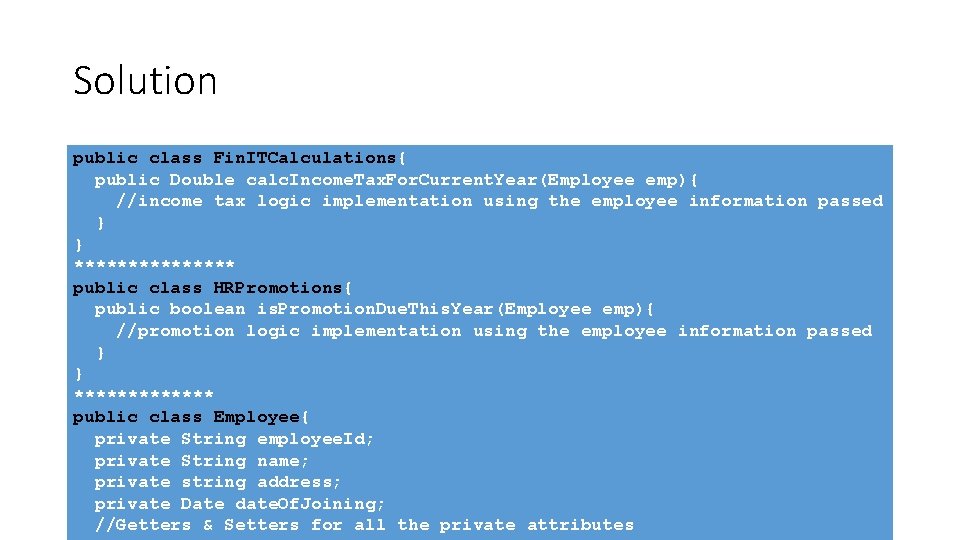
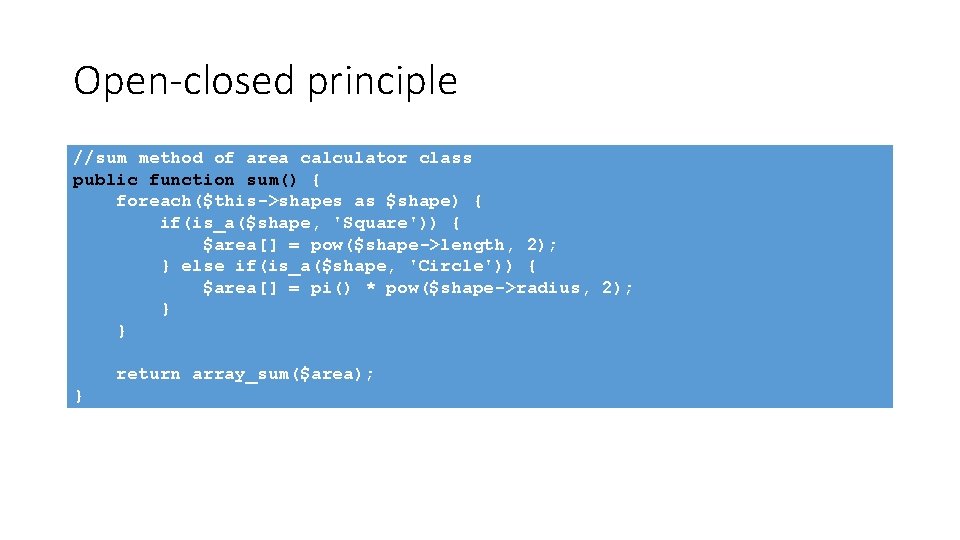
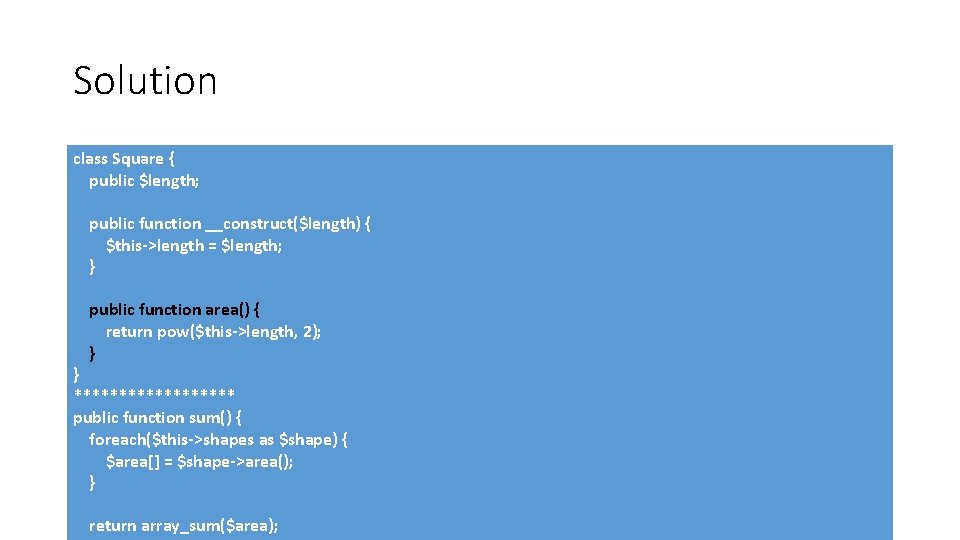
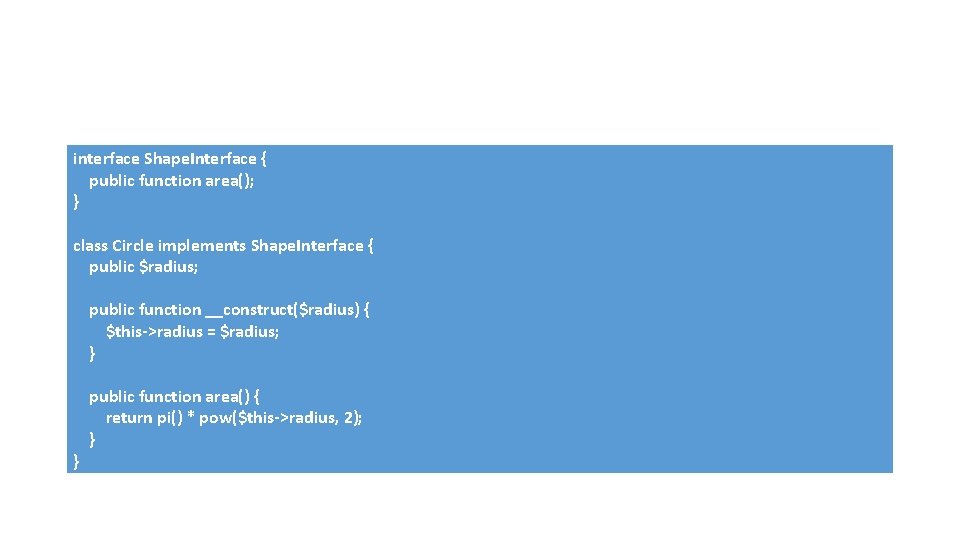
![Solution public function sum() { foreach($this->shapes as $shape) { if(is_a($shape, 'Shape. Interface')) { $area[] Solution public function sum() { foreach($this->shapes as $shape) { if(is_a($shape, 'Shape. Interface')) { $area[]](https://slidetodoc.com/presentation_image_h2/dd079a3fa15f25a5dc0b78bab5df0352/image-10.jpg)
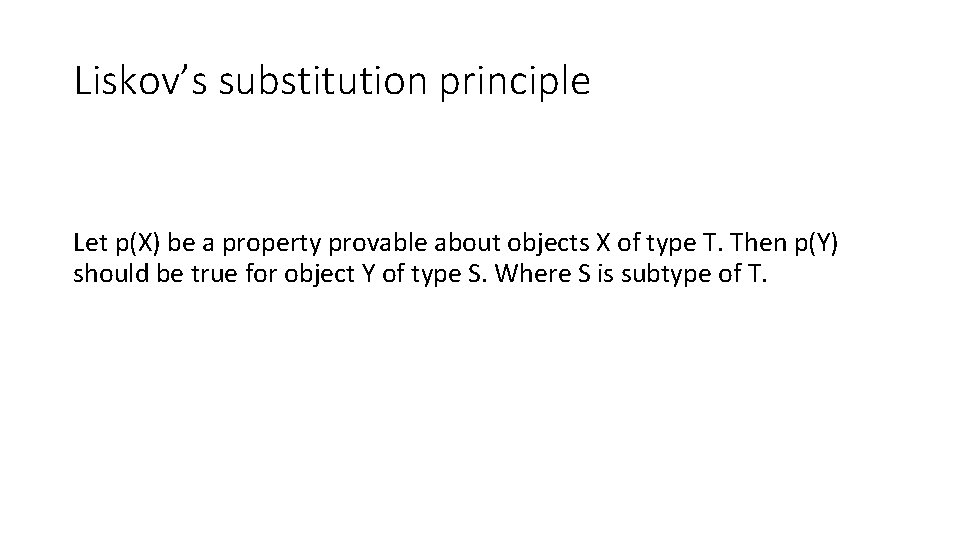
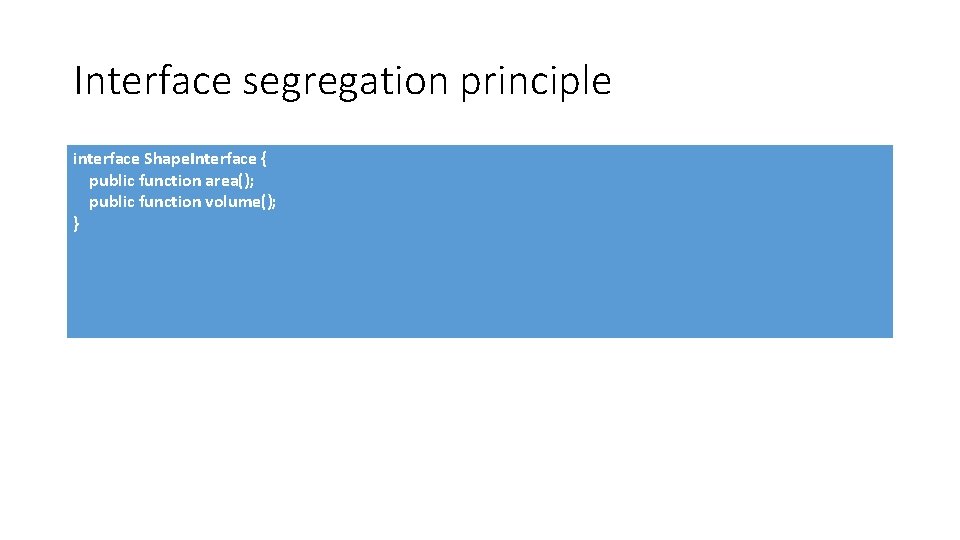
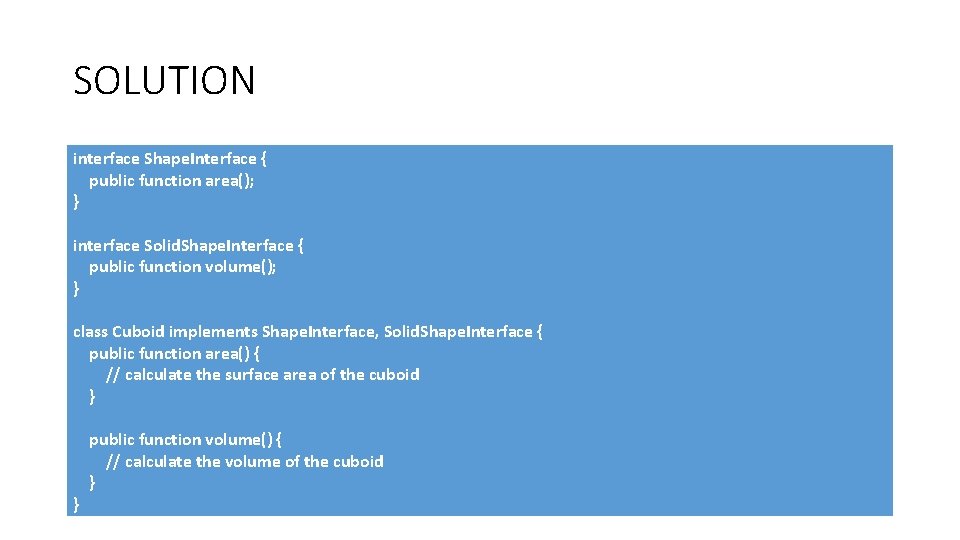
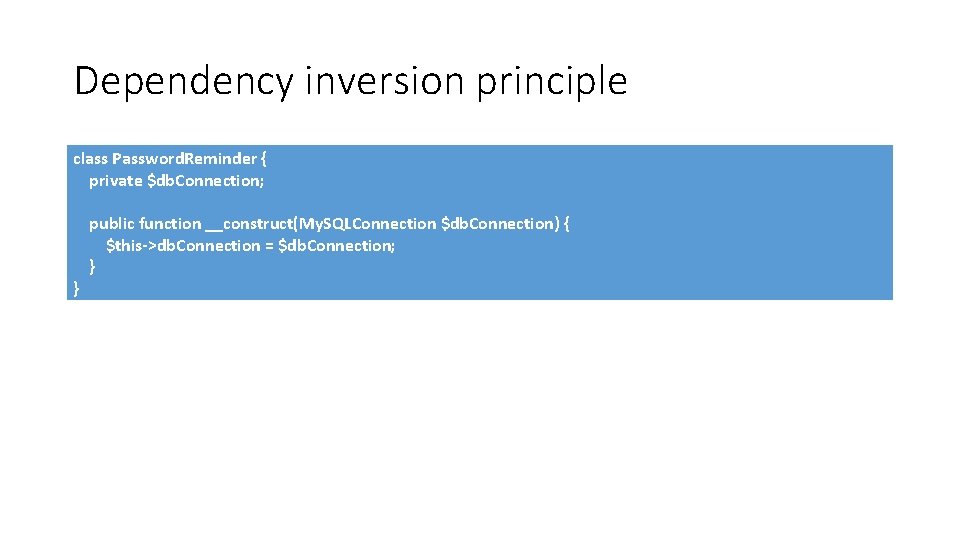
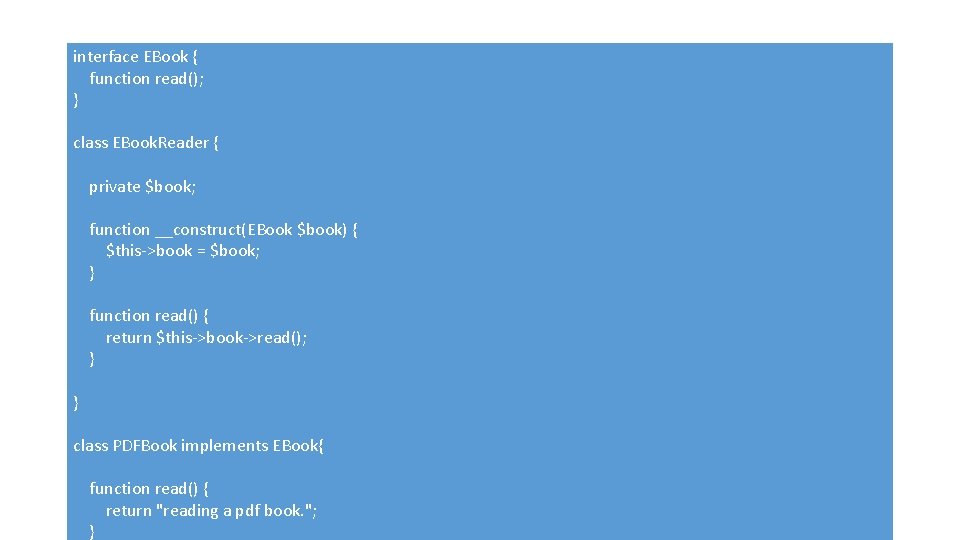
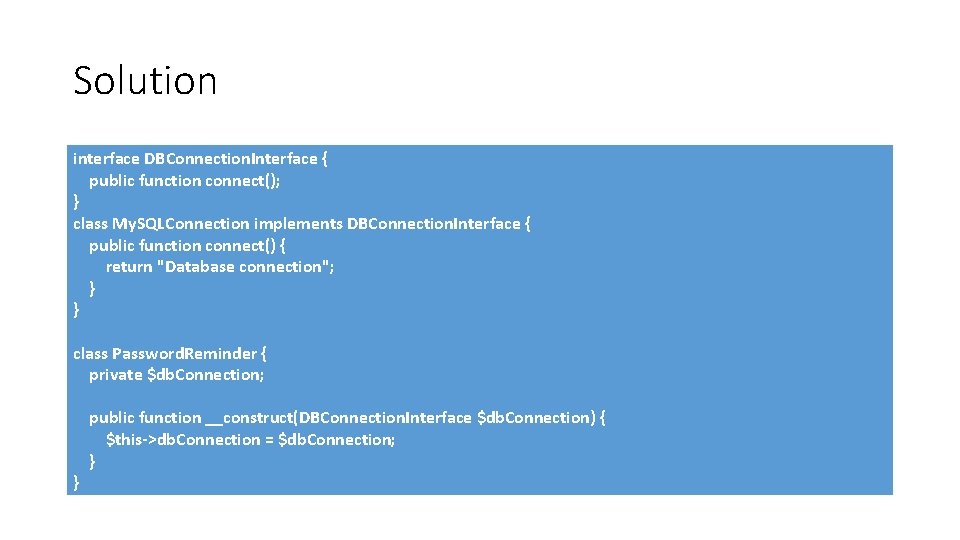
- Slides: 16
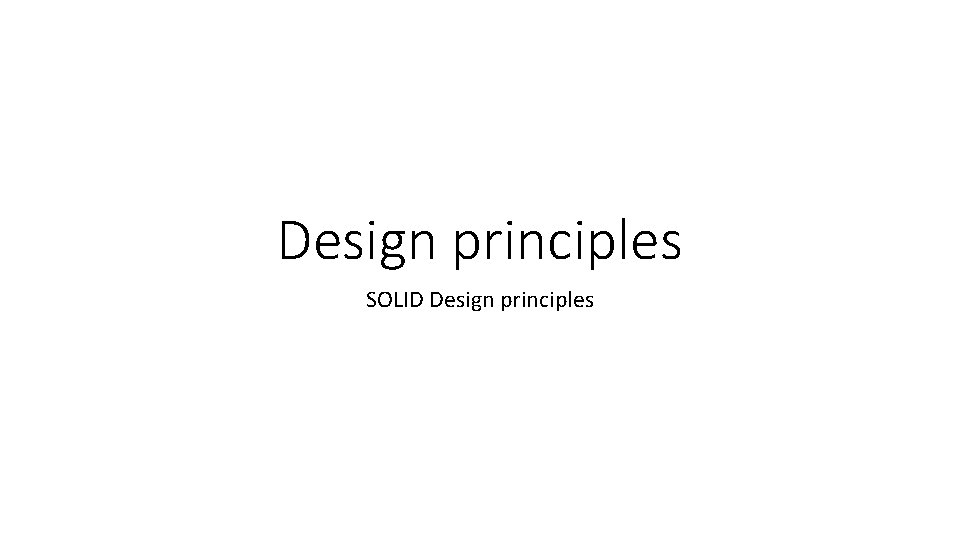
Design principles SOLID Design principles
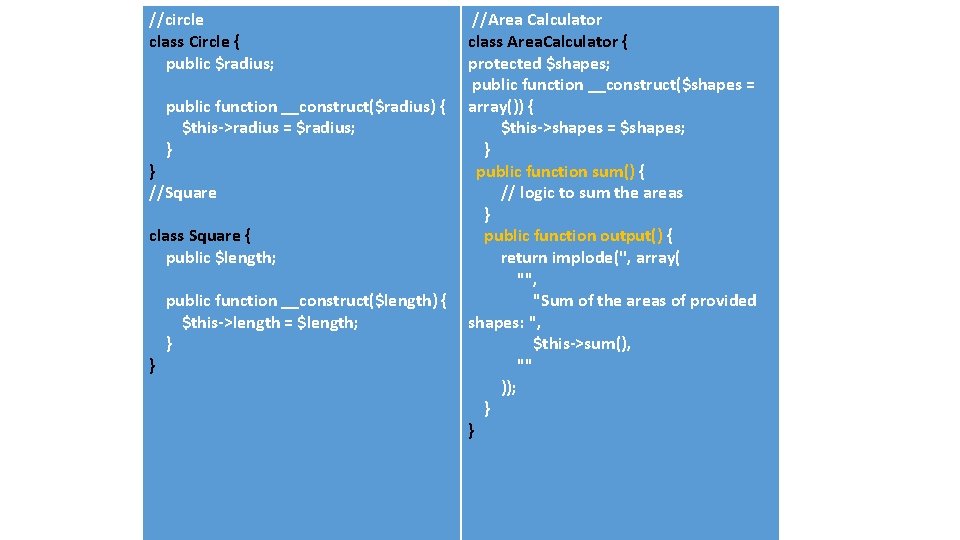
//circle class Circle { public $radius; public function __construct($radius) { $this->radius = $radius; } } //Square class Square { public $length; } public function __construct($length) { $this->length = $length; } //Area Calculator class Area. Calculator { protected $shapes; public function __construct($shapes = array()) { $this->shapes = $shapes; } public function sum() { // logic to sum the areas } public function output() { return implode('', array( "", "Sum of the areas of provided shapes: ", $this->sum(), "" )); } }
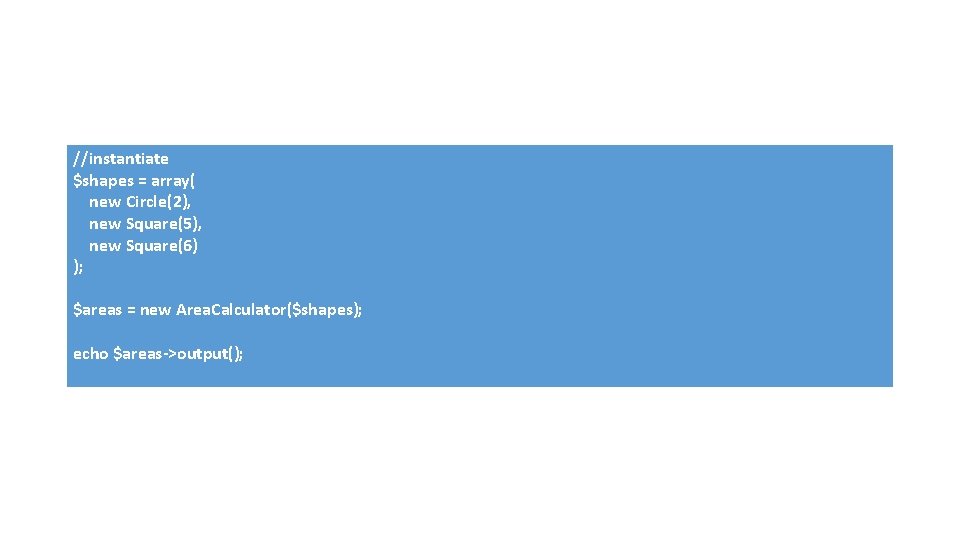
//instantiate $shapes = array( new Circle(2), new Square(5), new Square(6) ); $areas = new Area. Calculator($shapes); echo $areas->output();
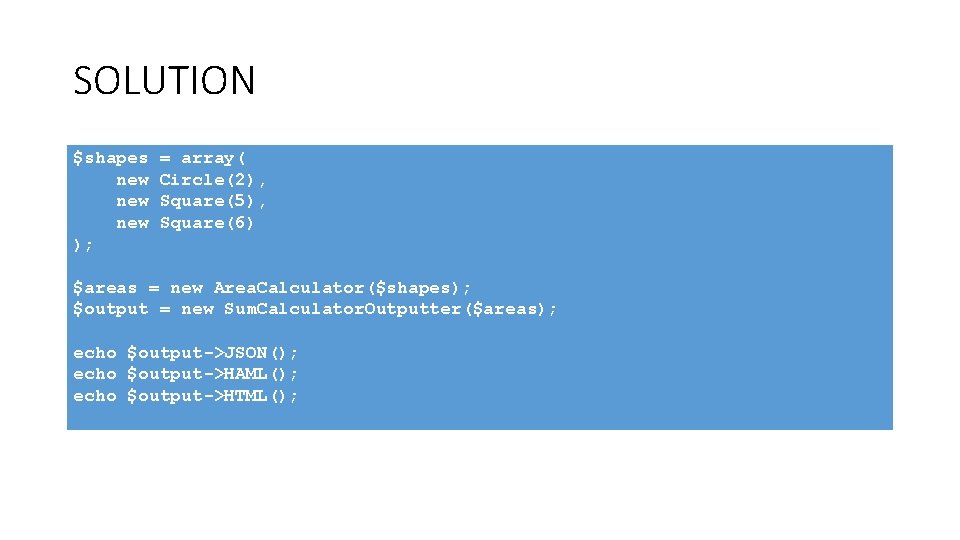
SOLUTION $shapes new new ); = array( Circle(2), Square(5), Square(6) $areas = new Area. Calculator($shapes); $output = new Sum. Calculator. Outputter($areas); echo $output->JSON(); echo $output->HAML(); echo $output->HTML();
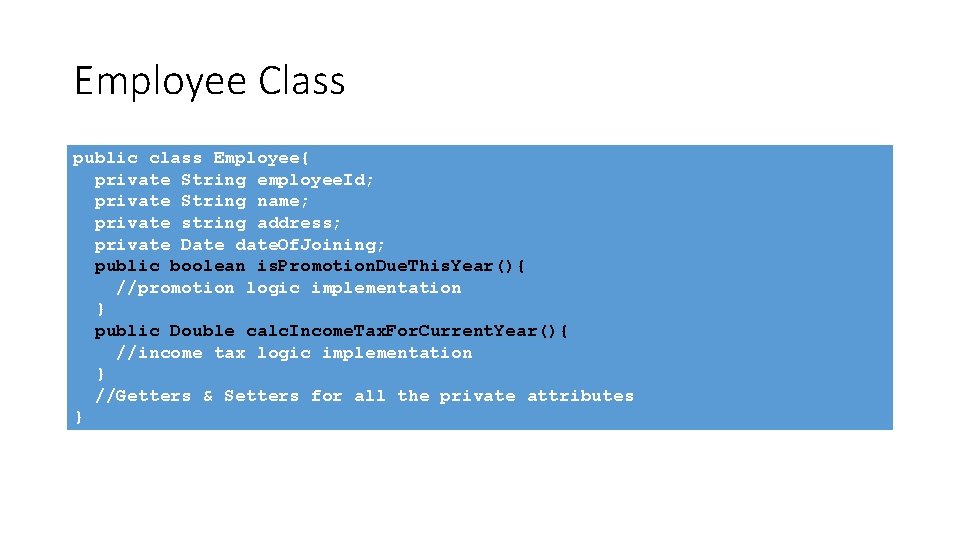
Employee Class public class Employee{ private String employee. Id; private String name; private string address; private Date date. Of. Joining; public boolean is. Promotion. Due. This. Year(){ //promotion logic implementation } public Double calc. Income. Tax. For. Current. Year(){ //income tax logic implementation } //Getters & Setters for all the private attributes }
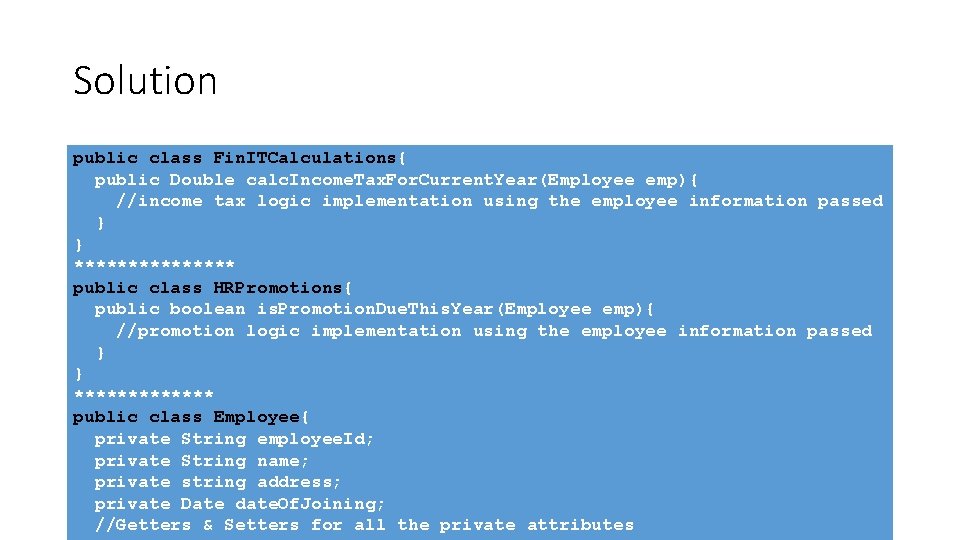
Solution public class Fin. ITCalculations{ public Double calc. Income. Tax. For. Current. Year(Employee emp){ //income tax logic implementation using the employee information passed } } ******** public class HRPromotions{ public boolean is. Promotion. Due. This. Year(Employee emp){ //promotion logic implementation using the employee information passed } } ******* public class Employee{ private String employee. Id; private String name; private string address; private Date date. Of. Joining; //Getters & Setters for all the private attributes
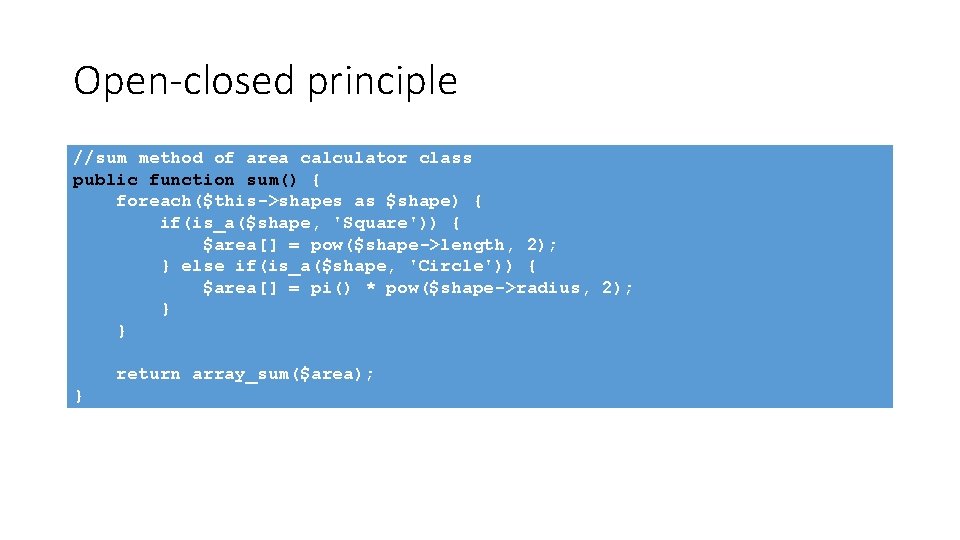
Open-closed principle //sum method of area calculator class public function sum() { foreach($this->shapes as $shape) { if(is_a($shape, 'Square')) { $area[] = pow($shape->length, 2); } else if(is_a($shape, 'Circle')) { $area[] = pi() * pow($shape->radius, 2); } } return array_sum($area); }
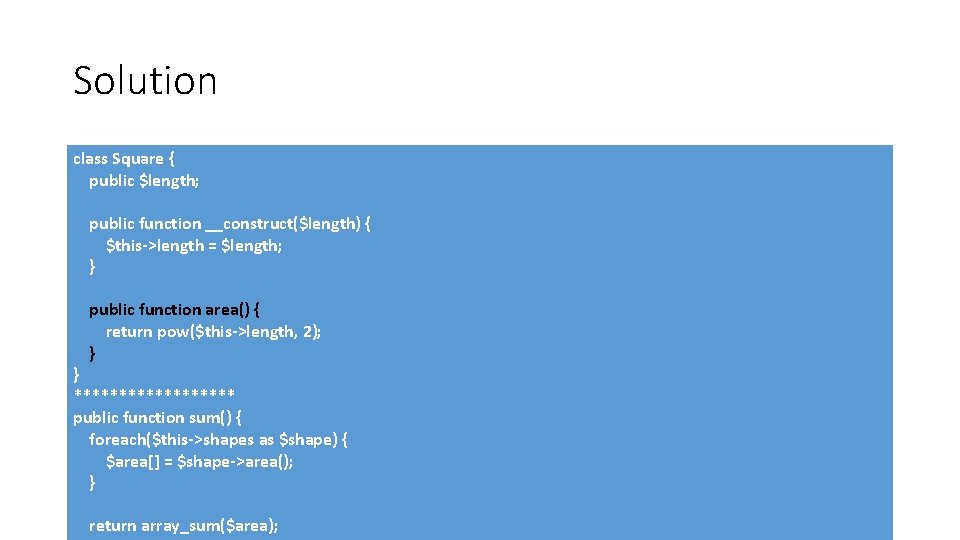
Solution class Square { public $length; public function __construct($length) { $this->length = $length; } public function area() { return pow($this->length, 2); } } ********* public function sum() { foreach($this->shapes as $shape) { $area[] = $shape->area(); } return array_sum($area);
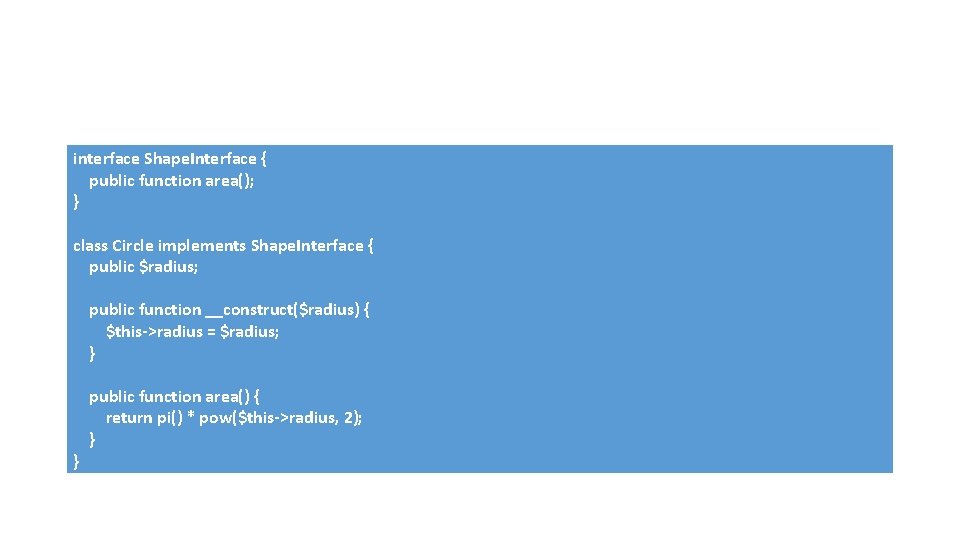
interface Shape. Interface { public function area(); } class Circle implements Shape. Interface { public $radius; public function __construct($radius) { $this->radius = $radius; } } public function area() { return pi() * pow($this->radius, 2); }
![Solution public function sum foreachthisshapes as shape ifisashape Shape Interface area Solution public function sum() { foreach($this->shapes as $shape) { if(is_a($shape, 'Shape. Interface')) { $area[]](https://slidetodoc.com/presentation_image_h2/dd079a3fa15f25a5dc0b78bab5df0352/image-10.jpg)
Solution public function sum() { foreach($this->shapes as $shape) { if(is_a($shape, 'Shape. Interface')) { $area[] = $shape->area(); continue; } } } throw new Area. Calculator. Invalid. Shape. Exception; return array_sum($area);
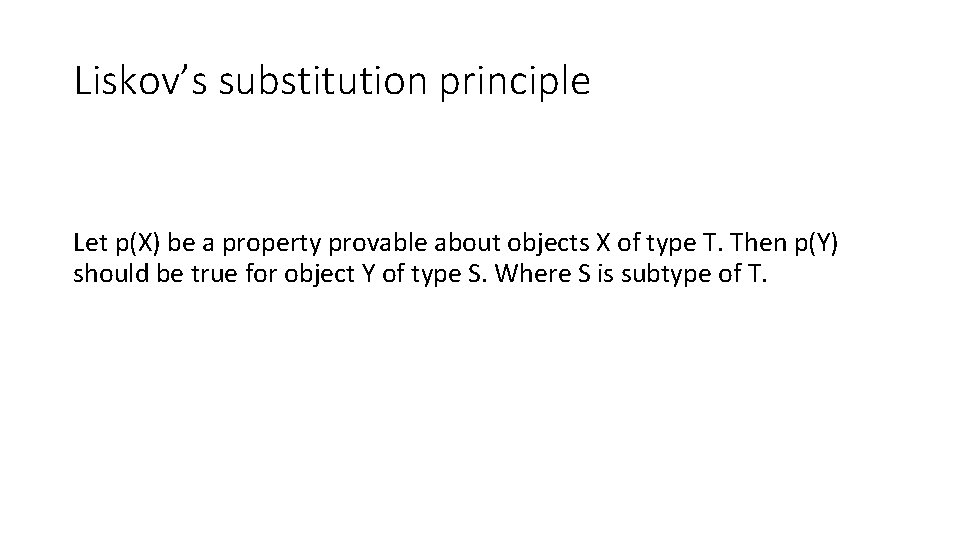
Liskov’s substitution principle Let p(X) be a property provable about objects X of type T. Then p(Y) should be true for object Y of type S. Where S is subtype of T.
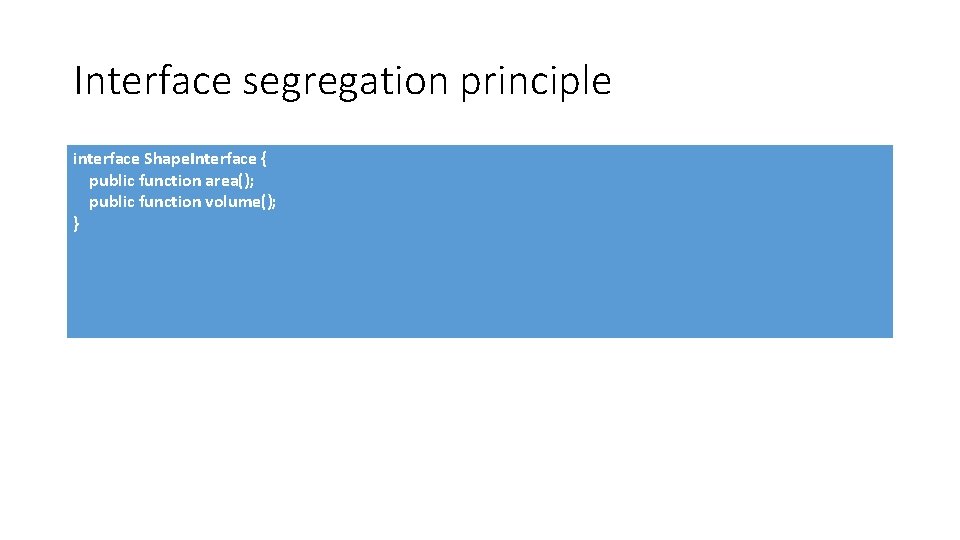
Interface segregation principle interface Shape. Interface { public function area(); public function volume(); }
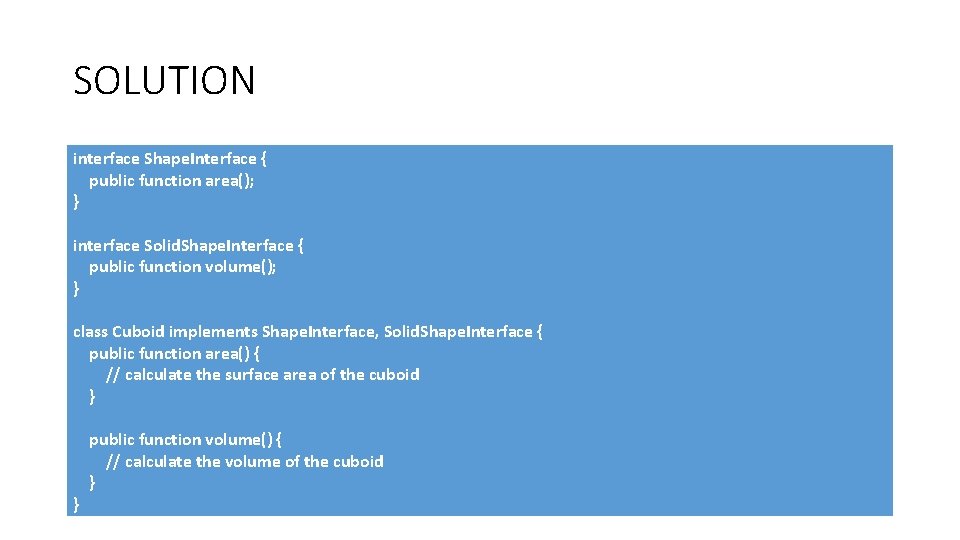
SOLUTION interface Shape. Interface { public function area(); } interface Solid. Shape. Interface { public function volume(); } class Cuboid implements Shape. Interface, Solid. Shape. Interface { public function area() { // calculate the surface area of the cuboid } } public function volume() { // calculate the volume of the cuboid }
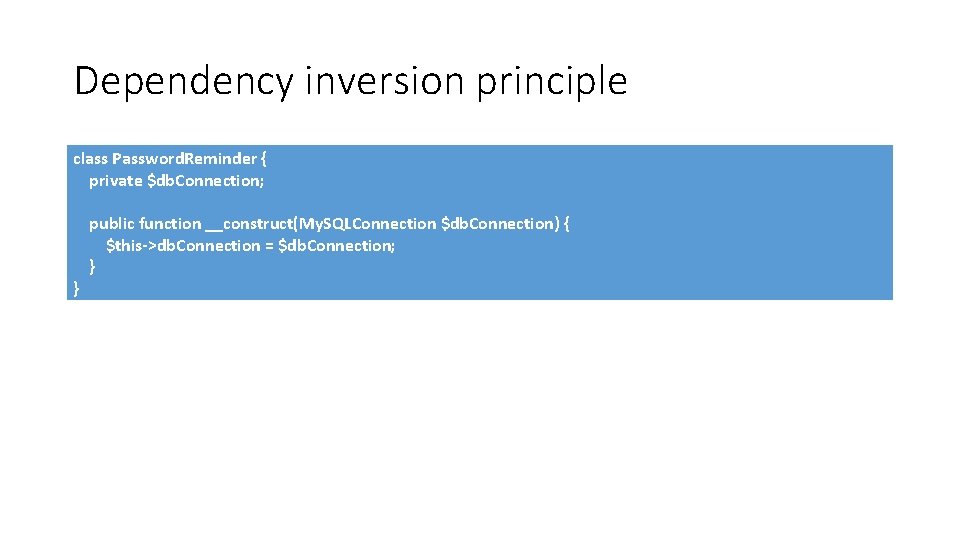
Dependency inversion principle class Password. Reminder { private $db. Connection; } public function __construct(My. SQLConnection $db. Connection) { $this->db. Connection = $db. Connection; }
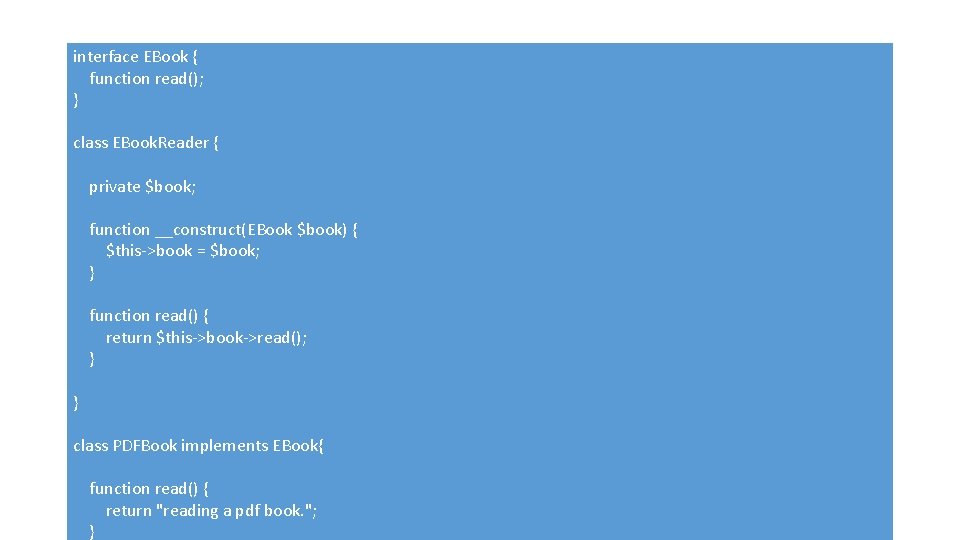
interface EBook { function read(); } class EBook. Reader { private $book; function __construct(EBook $book) { $this->book = $book; } function read() { return $this->book->read(); } } class PDFBook implements EBook{ function read() { return "reading a pdf book. "; }
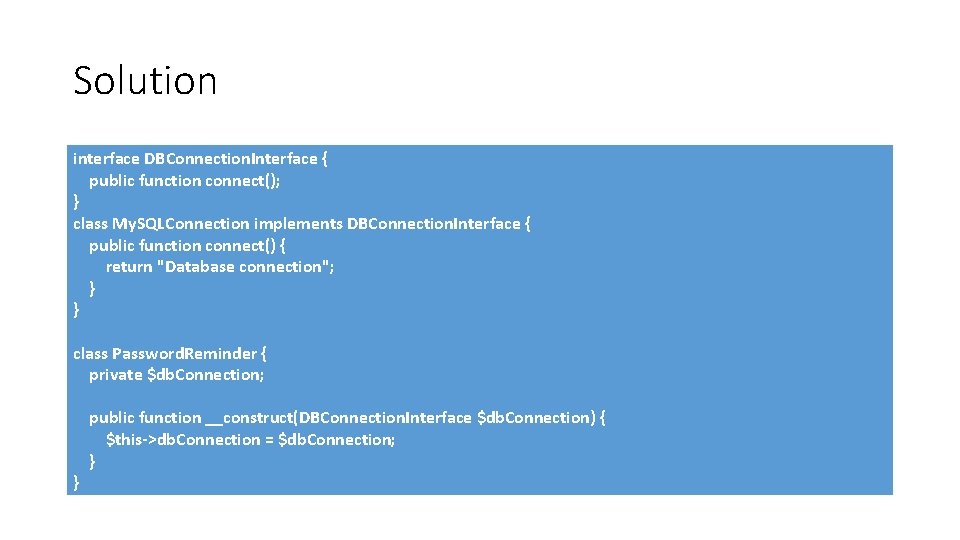
Solution interface DBConnection. Interface { public function connect(); } class My. SQLConnection implements DBConnection. Interface { public function connect() { return "Database connection"; } } class Password. Reminder { private $db. Connection; } public function __construct(DBConnection. Interface $db. Connection) { $this->db. Connection = $db. Connection; }
Solid design principles
Solid design principles
Crystalline vs amorphous
Example of solid solution is
Covalent molecular and covalent network
Crystalline vs non crystalline
Crystal solid and amorphous solid
Crystalline solid and amorphous solid
Crystalline substances
When a solid completely penetrates another solid
Interpenetration of surfaces
Evaporation mixture examples
Solid principles
Loose piece pattern
Vertical and horizontal circle
What is the point of tangency in circle j?
Open and closed circle