Definitions Process An executing program Thread A path
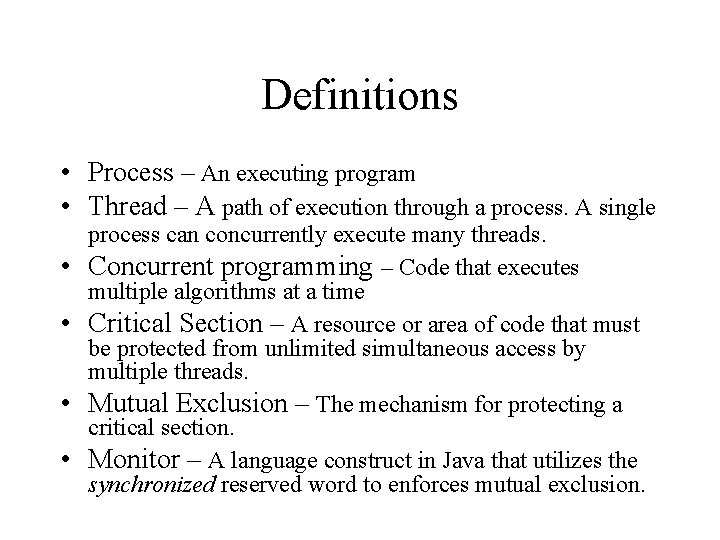
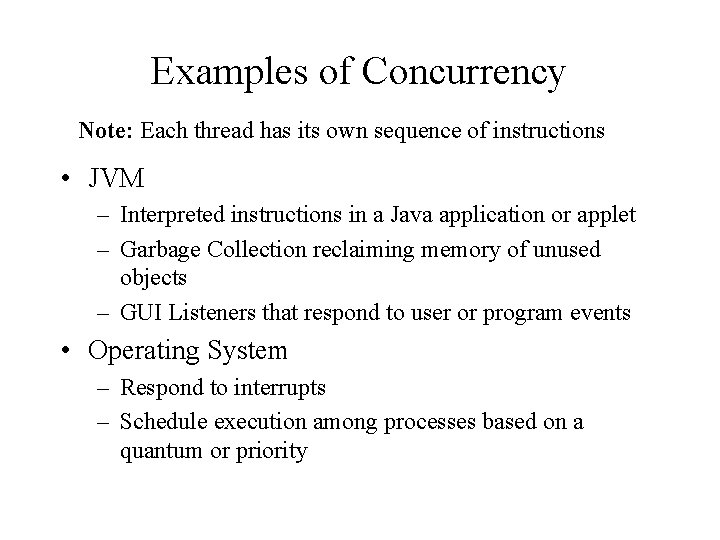
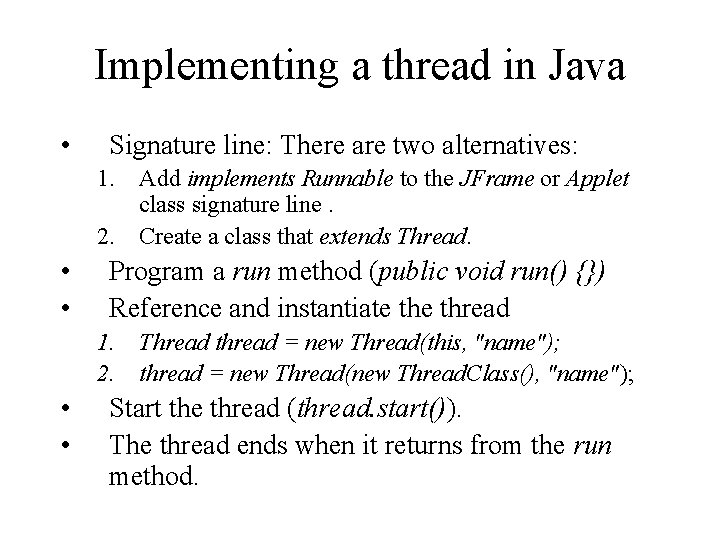
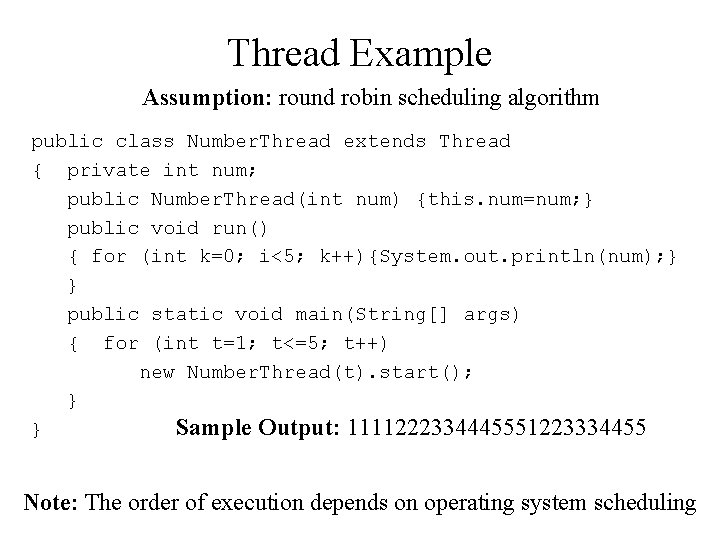
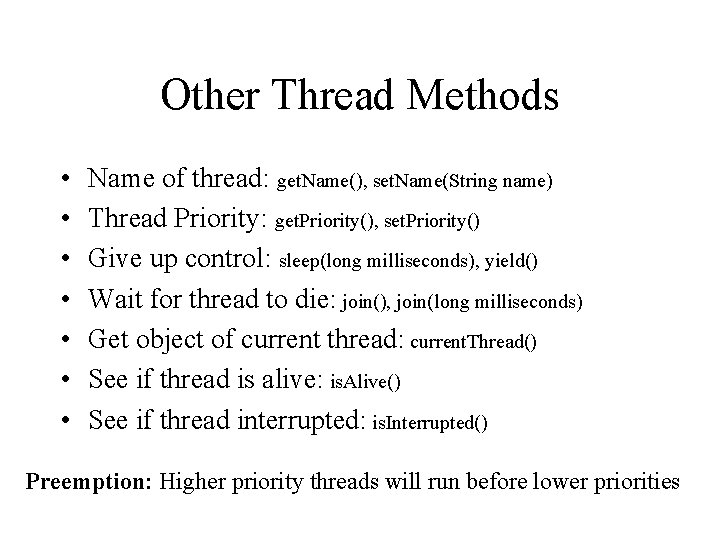
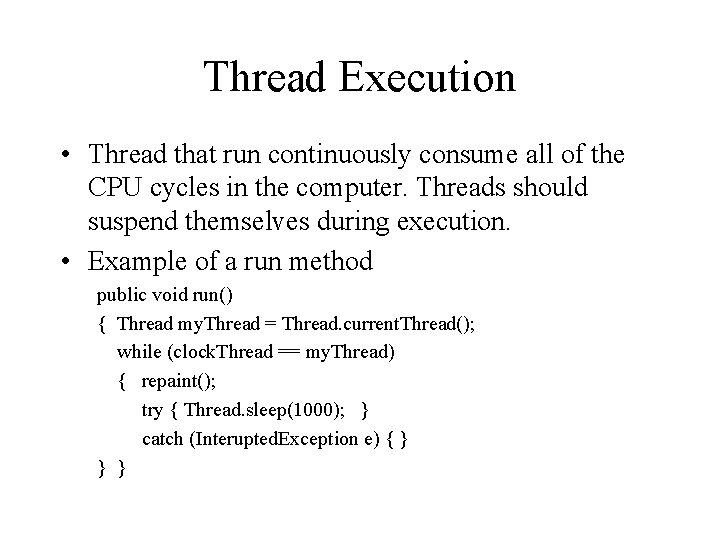
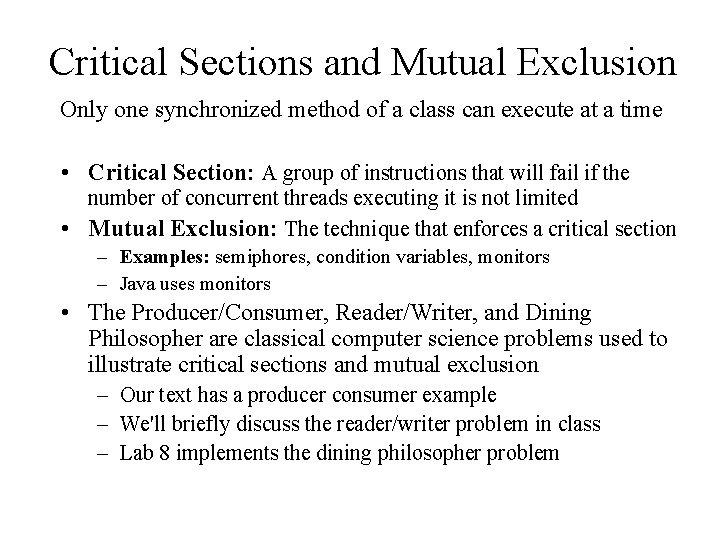
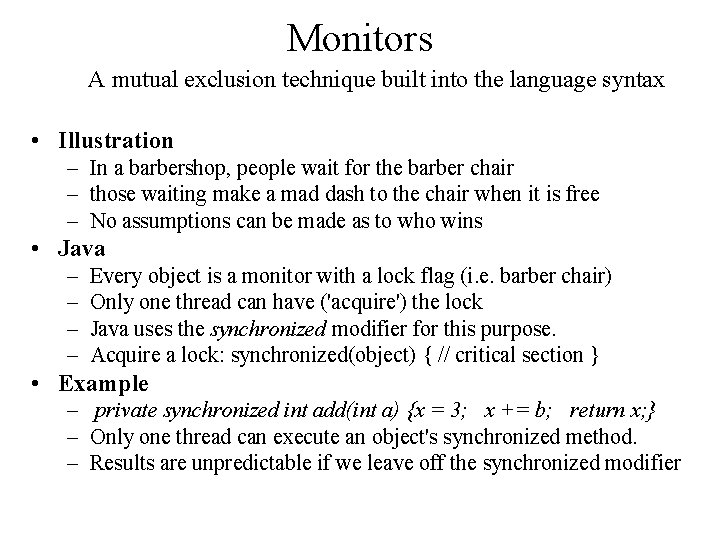
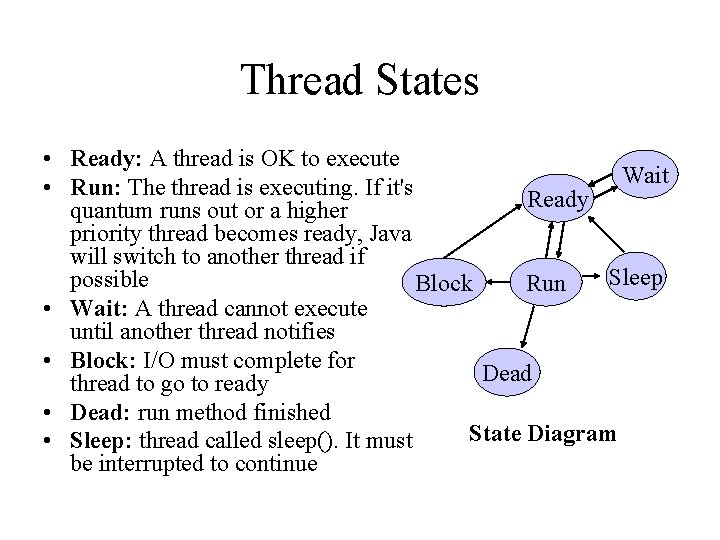
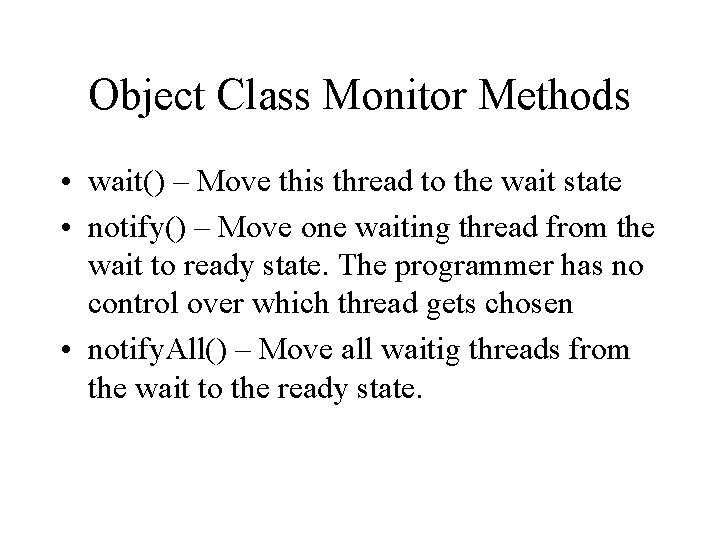
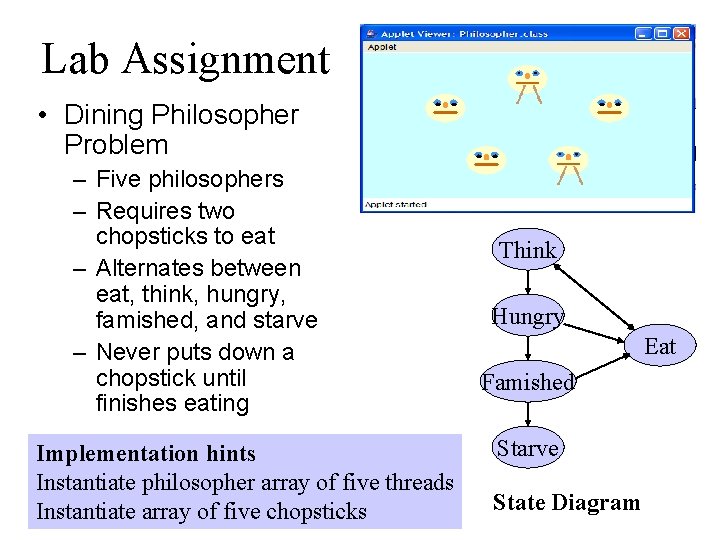
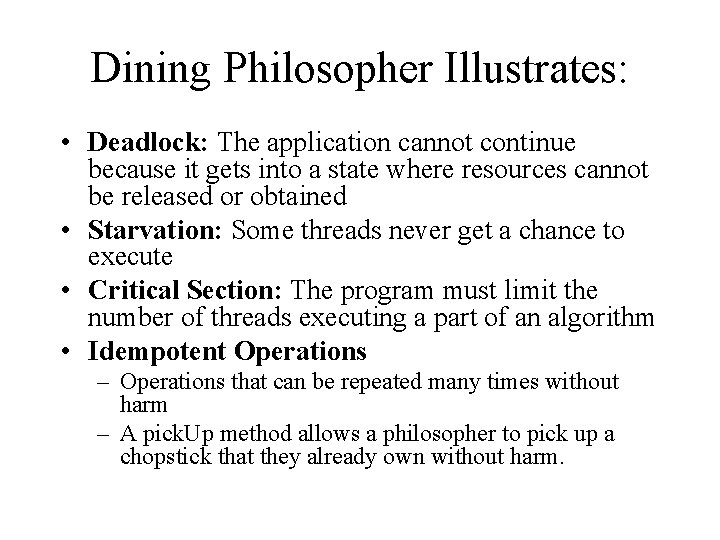
- Slides: 12
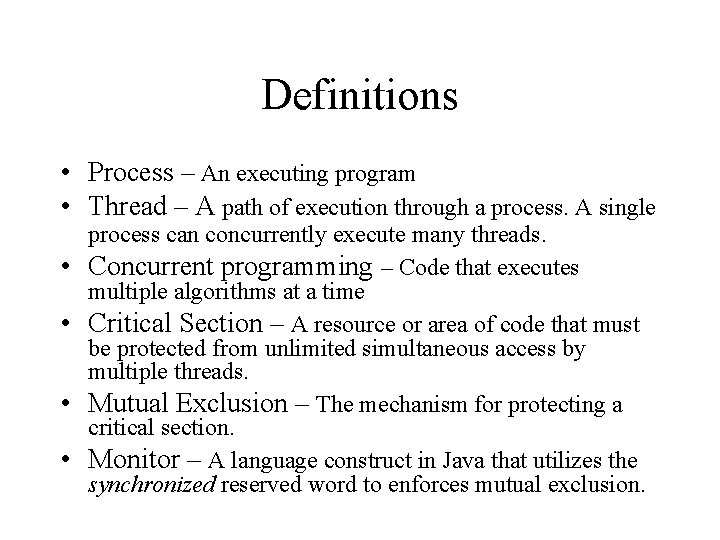
Definitions • Process – An executing program • Thread – A path of execution through a process. A single process can concurrently execute many threads. • Concurrent programming – Code that executes multiple algorithms at a time • Critical Section – A resource or area of code that must be protected from unlimited simultaneous access by multiple threads. • Mutual Exclusion – The mechanism for protecting a critical section. • Monitor – A language construct in Java that utilizes the synchronized reserved word to enforces mutual exclusion.
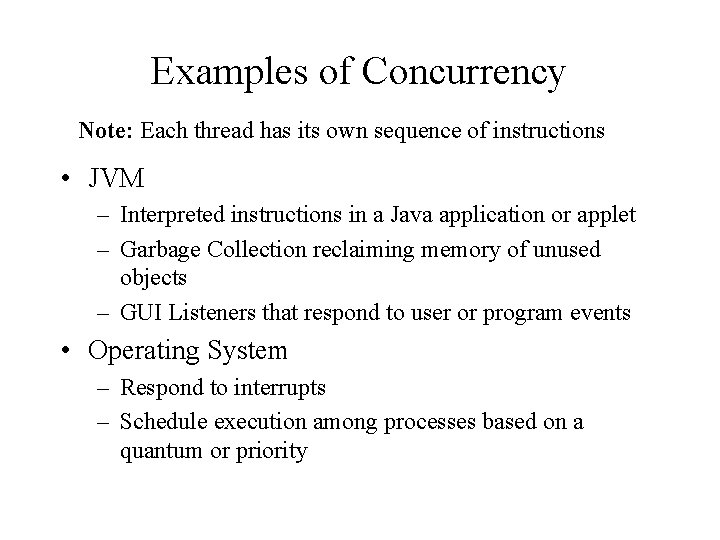
Examples of Concurrency Note: Each thread has its own sequence of instructions • JVM – Interpreted instructions in a Java application or applet – Garbage Collection reclaiming memory of unused objects – GUI Listeners that respond to user or program events • Operating System – Respond to interrupts – Schedule execution among processes based on a quantum or priority
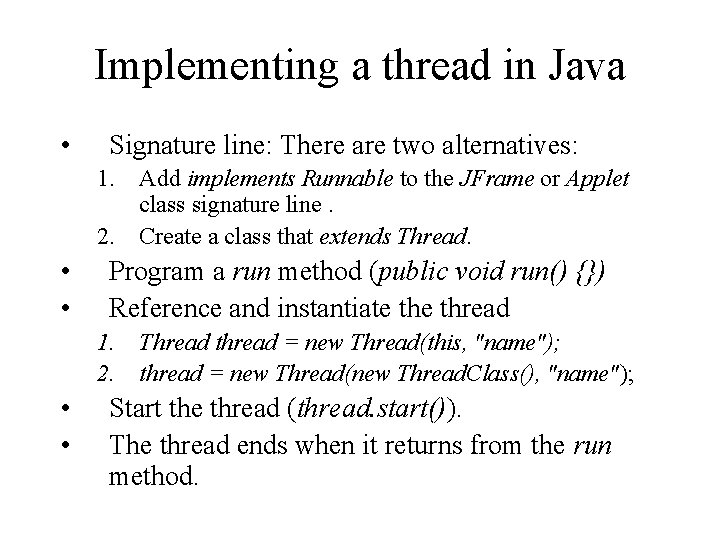
Implementing a thread in Java • Signature line: There are two alternatives: 1. Add implements Runnable to the JFrame or Applet class signature line. 2. Create a class that extends Thread. • • Program a run method (public void run() {}) Reference and instantiate thread 1. Thread thread = new Thread(this, "name"); 2. thread = new Thread(new Thread. Class(), "name"); • • Start the thread (thread. start()). The thread ends when it returns from the run method.
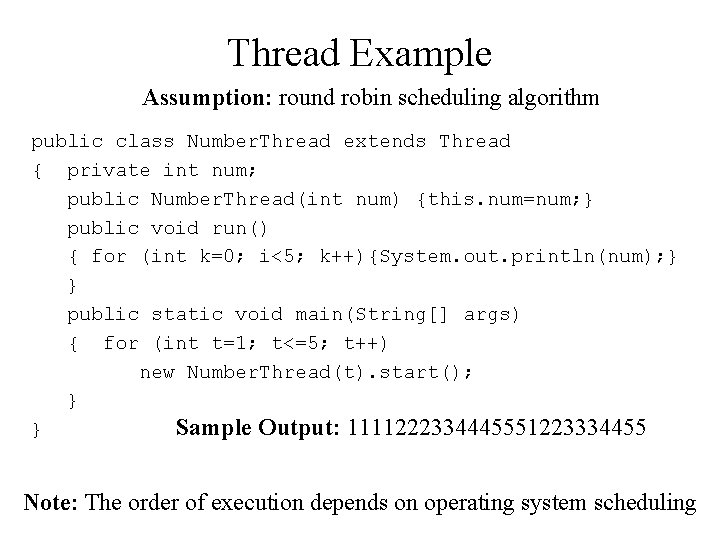
Thread Example Assumption: round robin scheduling algorithm public class Number. Thread extends Thread { private int num; public Number. Thread(int num) {this. num=num; } public void run() { for (int k=0; i<5; k++){System. out. println(num); } } public static void main(String[] args) { for (int t=1; t<=5; t++) new Number. Thread(t). start(); } Sample Output: 1111222334445551223334455 } Note: The order of execution depends on operating system scheduling
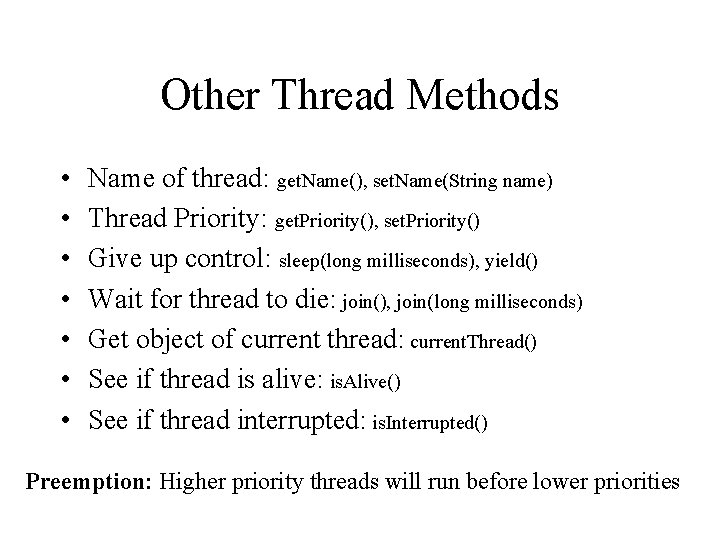
Other Thread Methods • • Name of thread: get. Name(), set. Name(String name) Thread Priority: get. Priority(), set. Priority() Give up control: sleep(long milliseconds), yield() Wait for thread to die: join(), join(long milliseconds) Get object of current thread: current. Thread() See if thread is alive: is. Alive() See if thread interrupted: is. Interrupted() Preemption: Higher priority threads will run before lower priorities
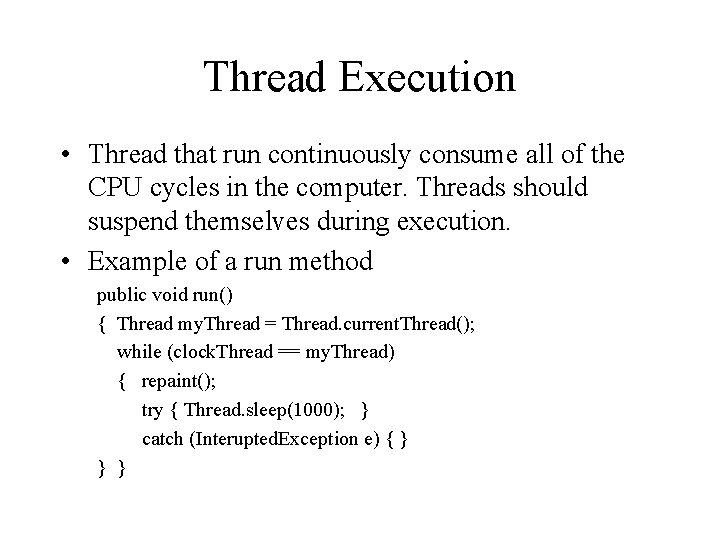
Thread Execution • Thread that run continuously consume all of the CPU cycles in the computer. Threads should suspend themselves during execution. • Example of a run method public void run() { Thread my. Thread = Thread. current. Thread(); while (clock. Thread == my. Thread) { repaint(); try { Thread. sleep(1000); } catch (Interupted. Exception e) { } } }
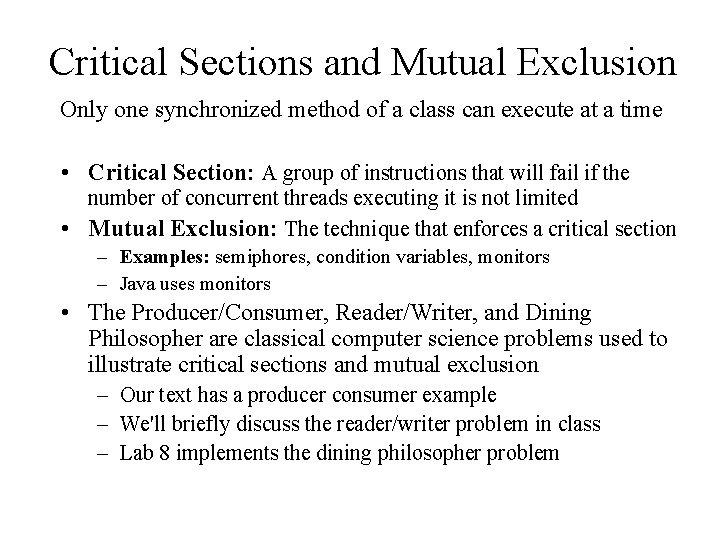
Critical Sections and Mutual Exclusion Only one synchronized method of a class can execute at a time • Critical Section: A group of instructions that will fail if the number of concurrent threads executing it is not limited • Mutual Exclusion: The technique that enforces a critical section – Examples: semiphores, condition variables, monitors – Java uses monitors • The Producer/Consumer, Reader/Writer, and Dining Philosopher are classical computer science problems used to illustrate critical sections and mutual exclusion – Our text has a producer consumer example – We'll briefly discuss the reader/writer problem in class – Lab 8 implements the dining philosopher problem
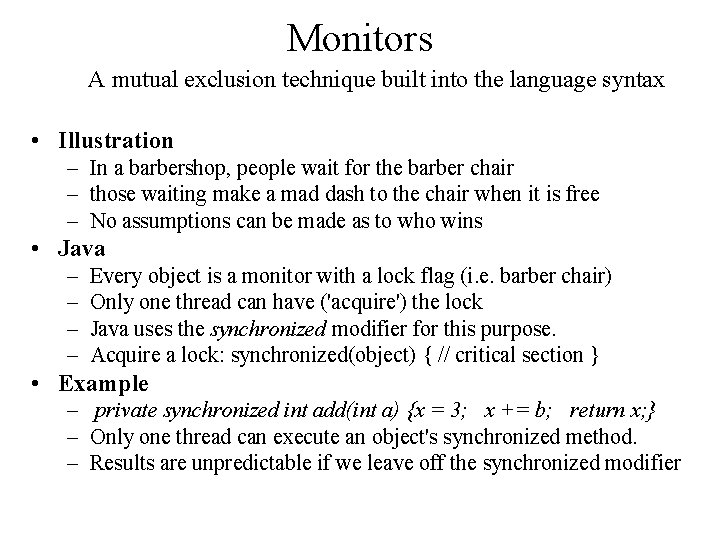
Monitors A mutual exclusion technique built into the language syntax • Illustration – In a barbershop, people wait for the barber chair – those waiting make a mad dash to the chair when it is free – No assumptions can be made as to who wins • Java – – Every object is a monitor with a lock flag (i. e. barber chair) Only one thread can have ('acquire') the lock Java uses the synchronized modifier for this purpose. Acquire a lock: synchronized(object) { // critical section } • Example – private synchronized int add(int a) {x = 3; x += b; return x; } – Only one thread can execute an object's synchronized method. – Results are unpredictable if we leave off the synchronized modifier
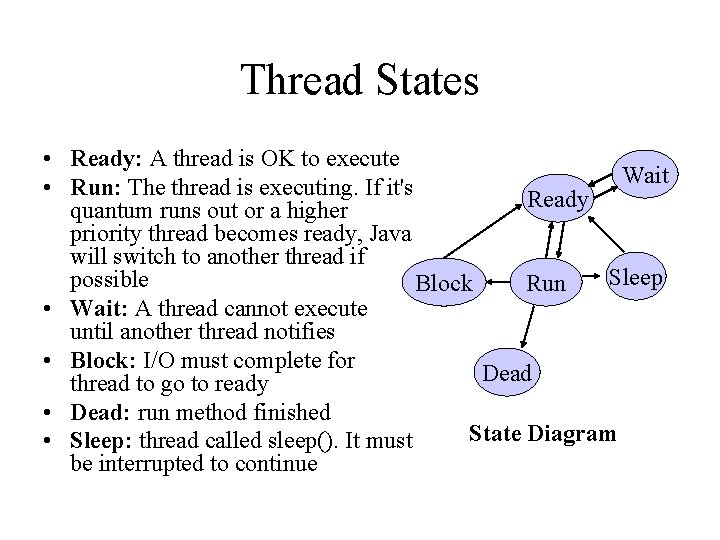
Thread States • Ready: A thread is OK to execute Wait • Run: The thread is executing. If it's Ready quantum runs out or a higher priority thread becomes ready, Java will switch to another thread if Sleep possible Block Run • Wait: A thread cannot execute until another thread notifies • Block: I/O must complete for Dead thread to go to ready • Dead: run method finished State Diagram • Sleep: thread called sleep(). It must be interrupted to continue
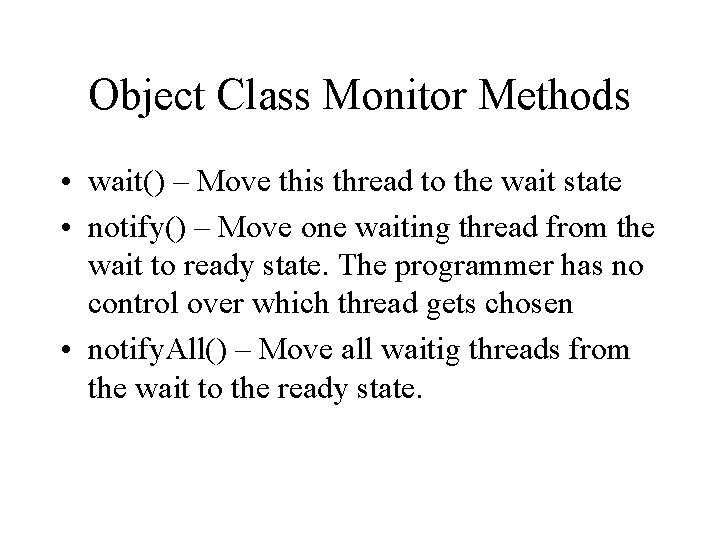
Object Class Monitor Methods • wait() – Move this thread to the wait state • notify() – Move one waiting thread from the wait to ready state. The programmer has no control over which thread gets chosen • notify. All() – Move all waitig threads from the wait to the ready state.
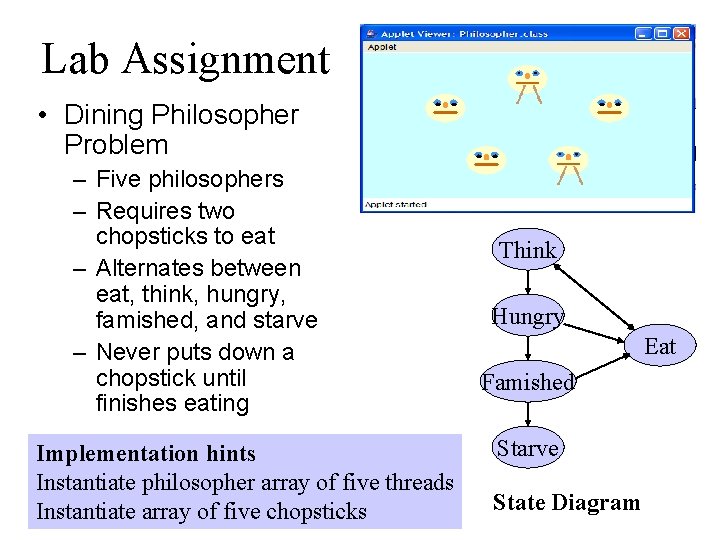
Lab Assignment • Dining Philosopher Problem – Five philosophers – Requires two chopsticks to eat – Alternates between eat, think, hungry, famished, and starve – Never puts down a chopstick until finishes eating Implementation hints Instantiate philosopher array of five threads Instantiate array of five chopsticks Think Hungry Eat Famished Starve State Diagram
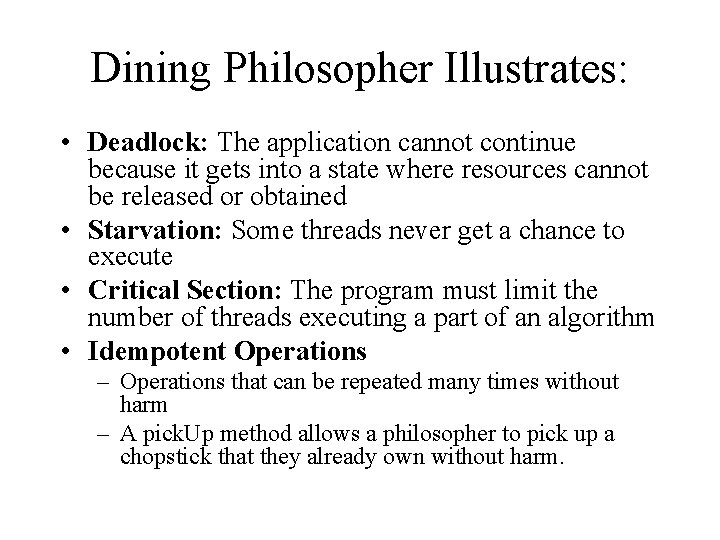
Dining Philosopher Illustrates: • Deadlock: The application cannot continue because it gets into a state where resources cannot be released or obtained • Starvation: Some threads never get a chance to execute • Critical Section: The program must limit the number of threads executing a part of an algorithm • Idempotent Operations – Operations that can be repeated many times without harm – A pick. Up method allows a philosopher to pick up a chopstick that they already own without harm.