Defining Macros with Parameters Defining constant macro define
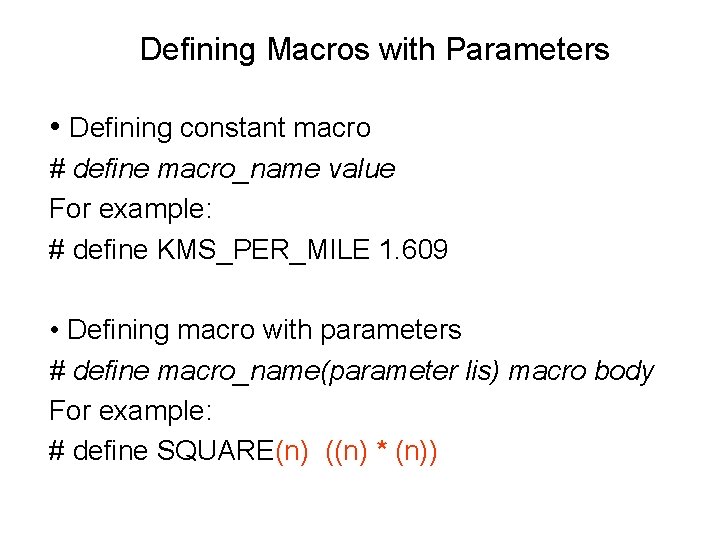
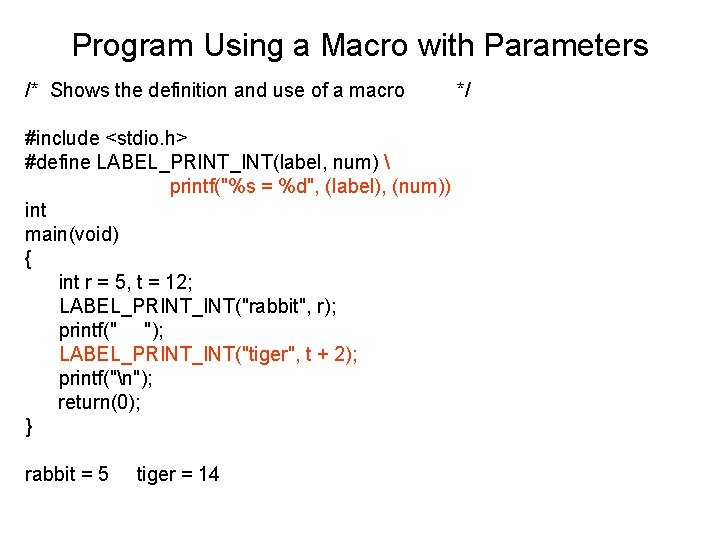
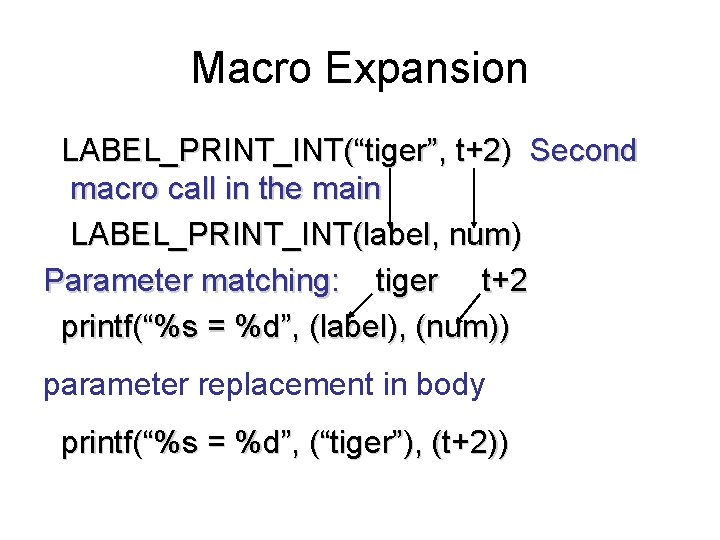
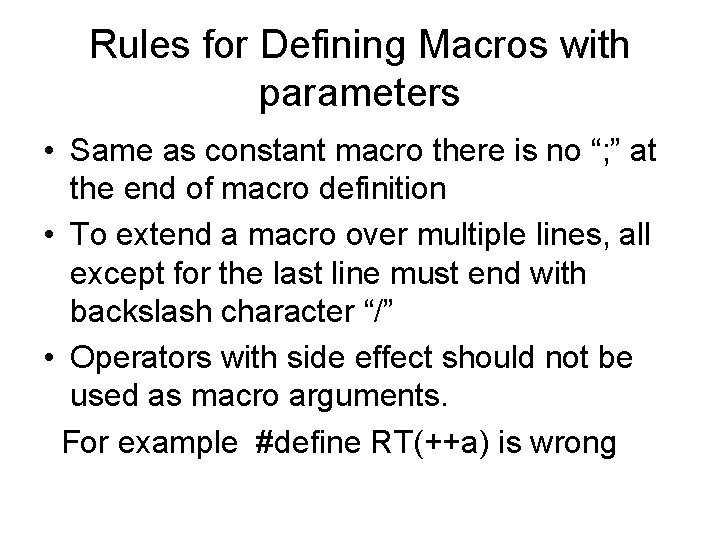
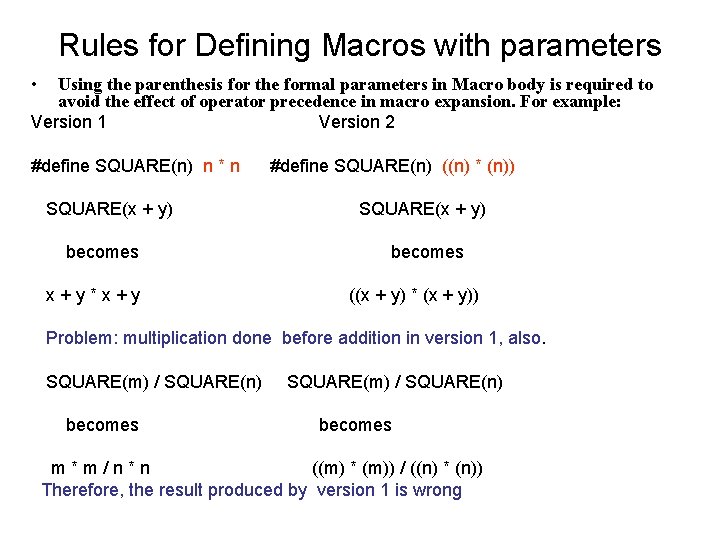
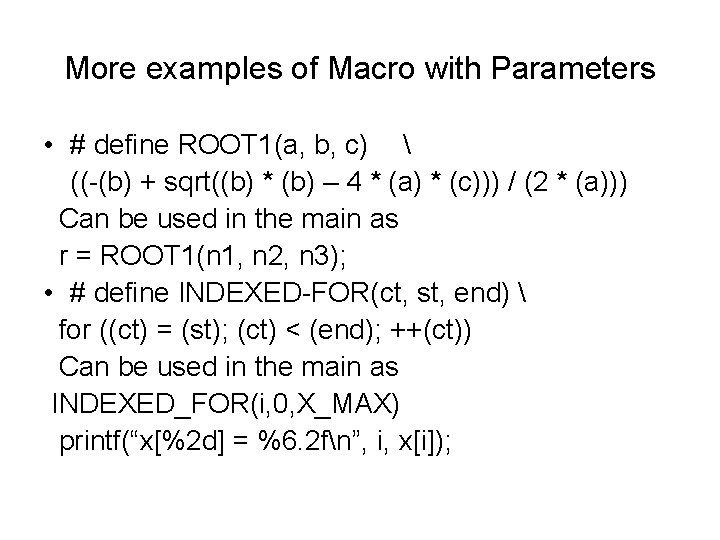
- Slides: 6
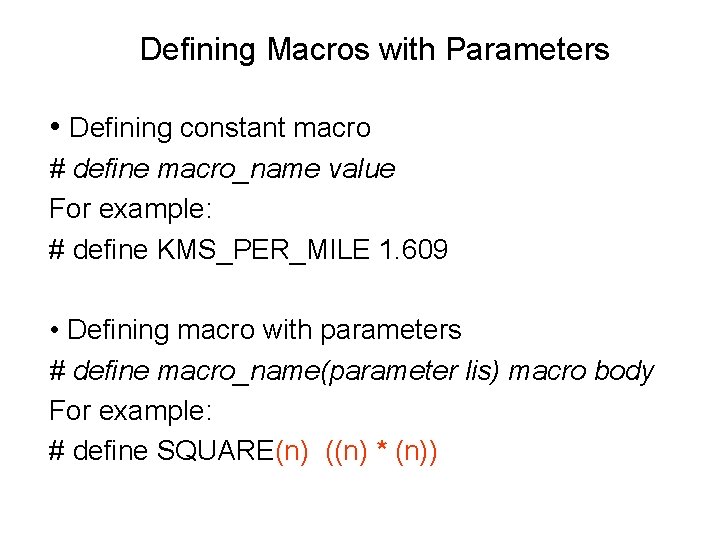
Defining Macros with Parameters • Defining constant macro # define macro_name value For example: # define KMS_PER_MILE 1. 609 • Defining macro with parameters # define macro_name(parameter lis) macro body For example: # define SQUARE(n) ((n) * (n))
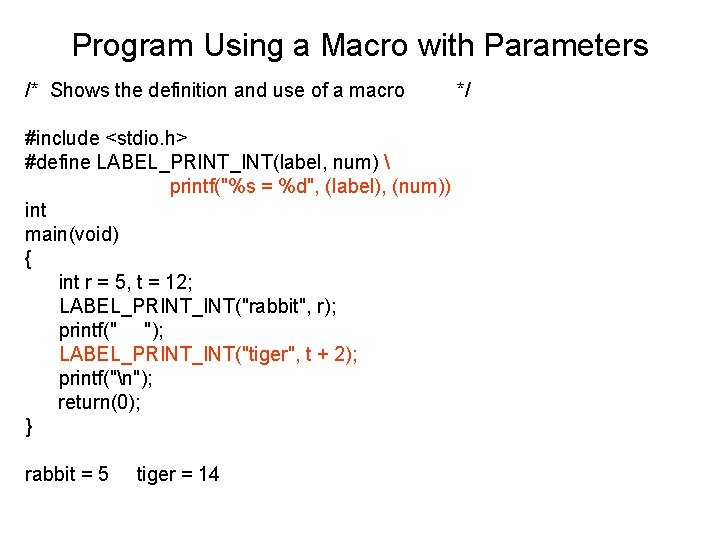
Program Using a Macro with Parameters /* Shows the definition and use of a macro #include <stdio. h> #define LABEL_PRINT_INT(label, num) printf("%s = %d", (label), (num)) int main(void) { int r = 5, t = 12; LABEL_PRINT_INT("rabbit", r); printf(" "); LABEL_PRINT_INT("tiger", t + 2); printf("n"); return(0); } rabbit = 5 tiger = 14 */
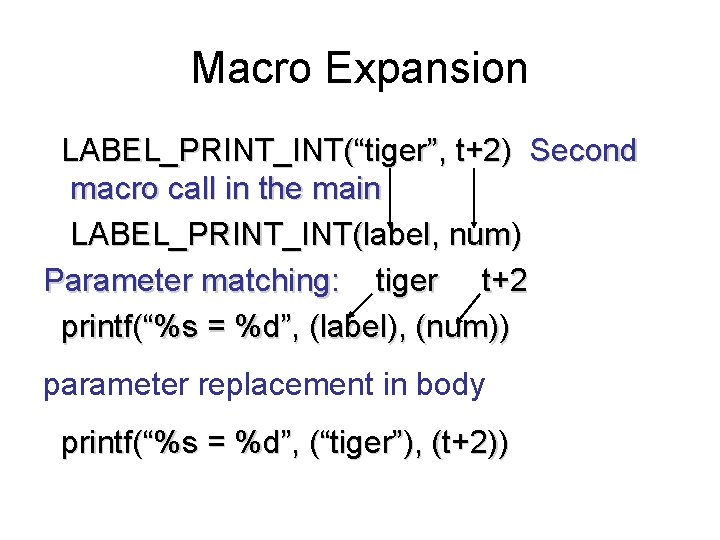
Macro Expansion LABEL_PRINT_INT(“tiger”, t+2) Second macro call in the main LABEL_PRINT_INT(label, num) Parameter matching: tiger t+2 printf(“%s = %d”, (label), (num)) parameter replacement in body printf(“%s = %d”, (“tiger”), (t+2))
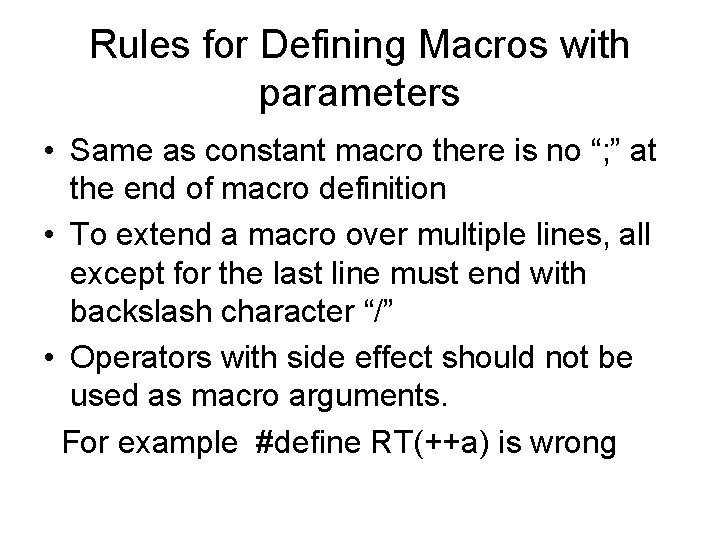
Rules for Defining Macros with parameters • Same as constant macro there is no “; ” at the end of macro definition • To extend a macro over multiple lines, all except for the last line must end with backslash character “/” • Operators with side effect should not be used as macro arguments. For example #define RT(++a) is wrong
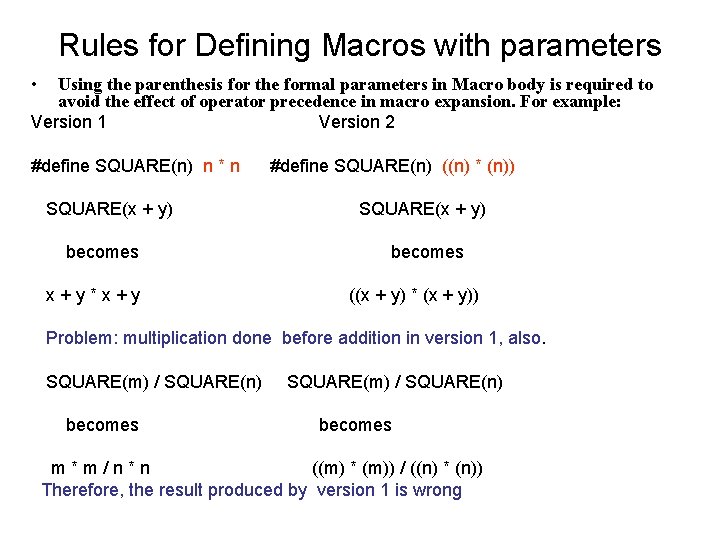
Rules for Defining Macros with parameters • Using the parenthesis for the formal parameters in Macro body is required to avoid the effect of operator precedence in macro expansion. For example: Version 1 Version 2 #define SQUARE(n) n * n SQUARE(x + y) becomes x+y*x+y #define SQUARE(n) ((n) * (n)) SQUARE(x + y) becomes ((x + y) * (x + y)) Problem: multiplication done before addition in version 1, also. SQUARE(m) / SQUARE(n) becomes m*m/n*n ((m) * (m)) / ((n) * (n)) Therefore, the result produced by version 1 is wrong
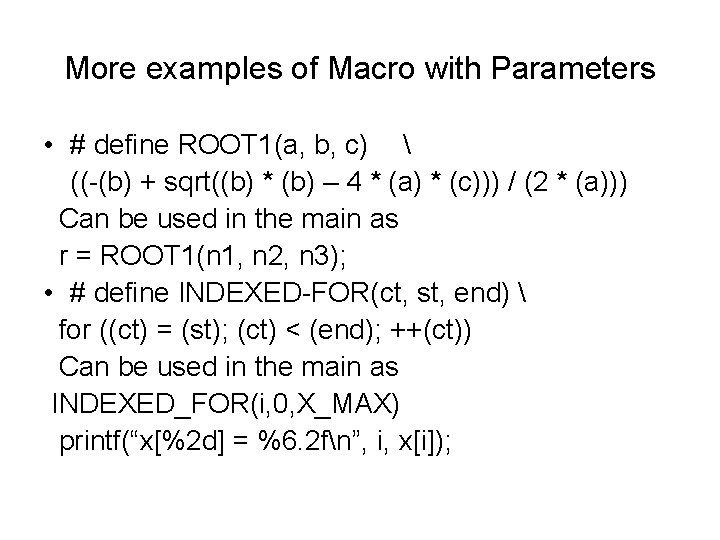
More examples of Macro with Parameters • # define ROOT 1(a, b, c) ((-(b) + sqrt((b) * (b) – 4 * (a) * (c))) / (2 * (a))) Can be used in the main as r = ROOT 1(n 1, n 2, n 3); • # define INDEXED-FOR(ct, st, end) for ((ct) = (st); (ct) < (end); ++(ct)) Can be used in the main as INDEXED_FOR(i, 0, X_MAX) printf(“x[%2 d] = %6. 2 fn”, i, x[i]);