CS 2403 Programming Languages StatementLevel Control Structures ChungTa
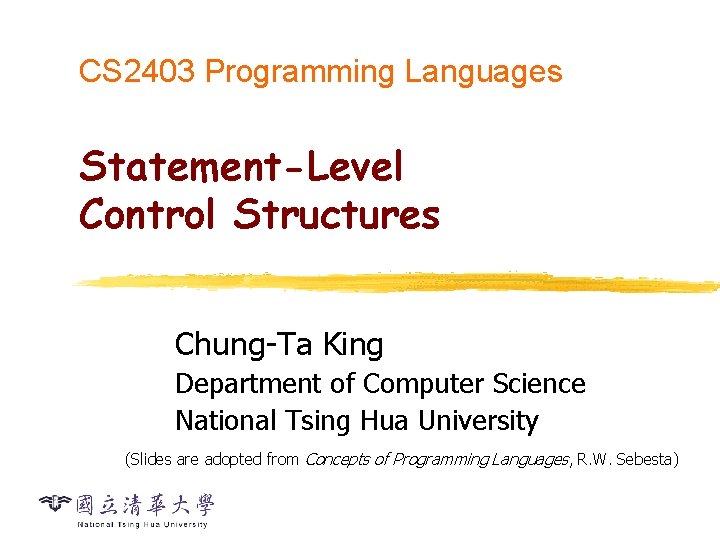
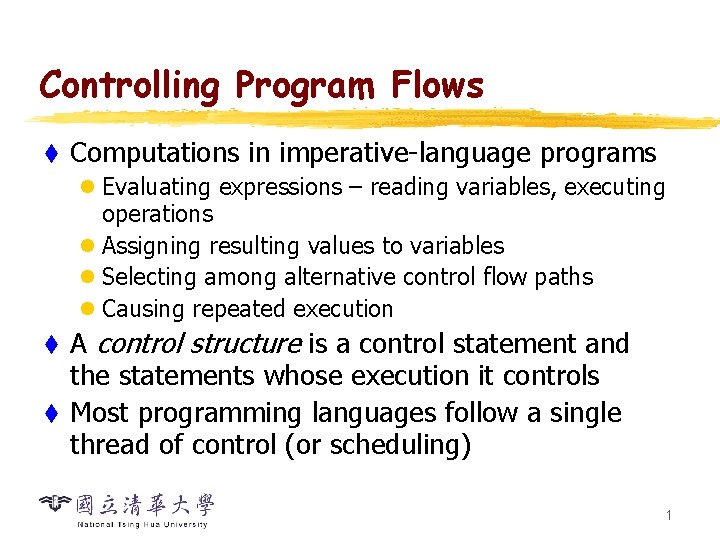
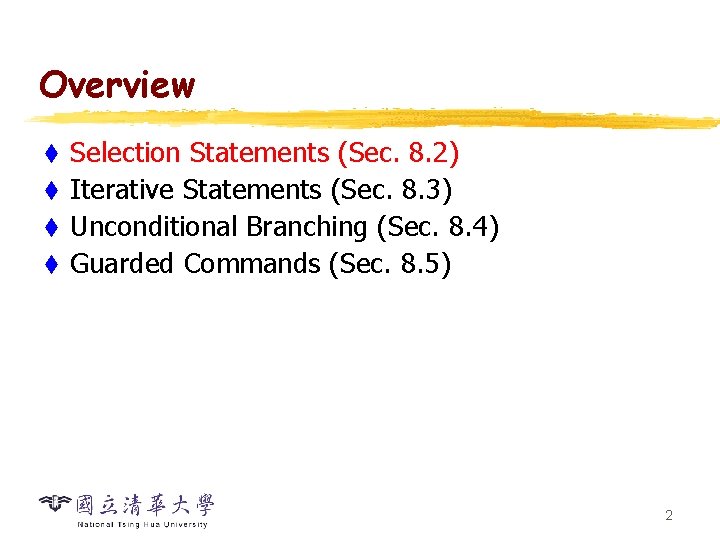
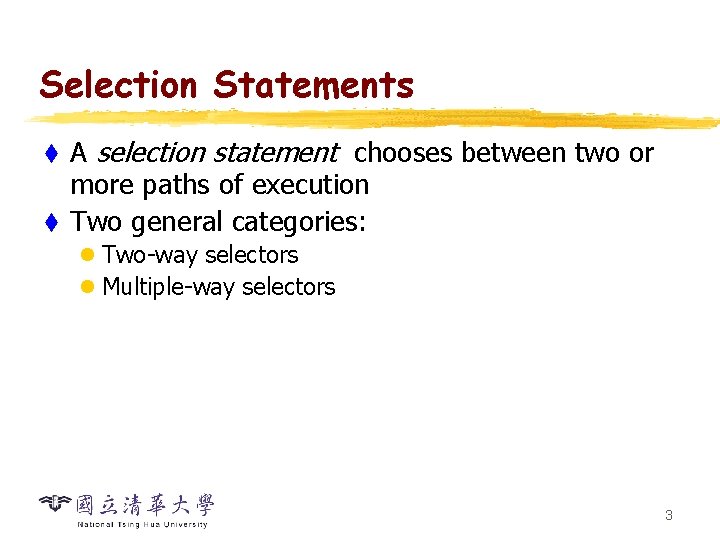
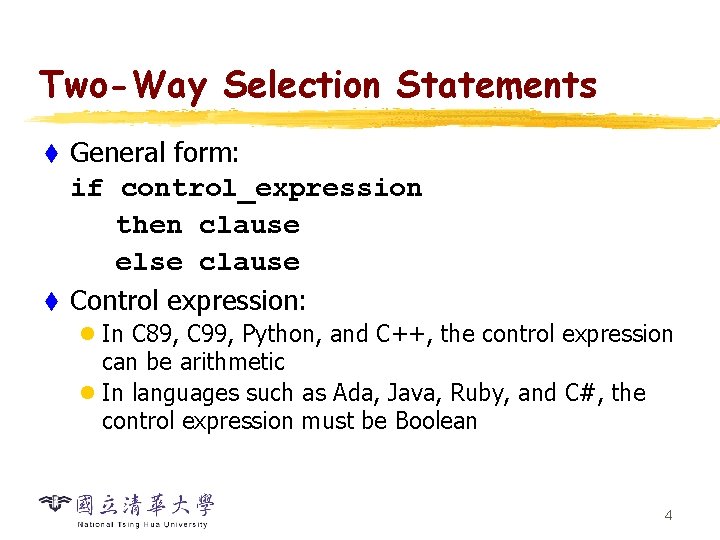
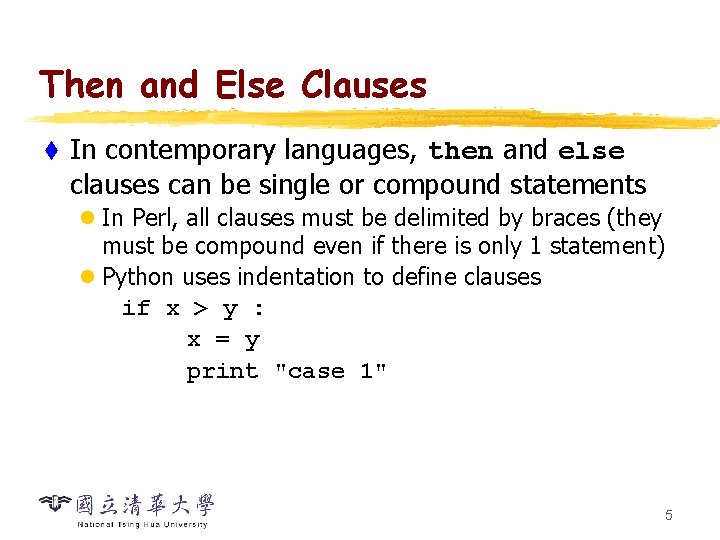
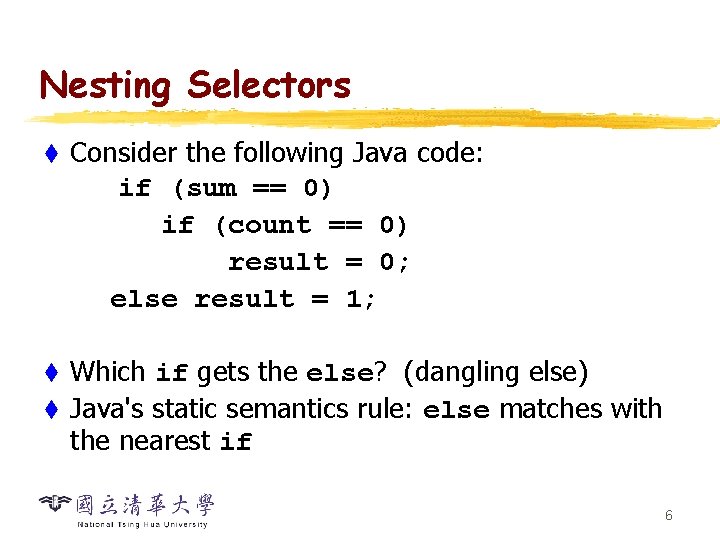
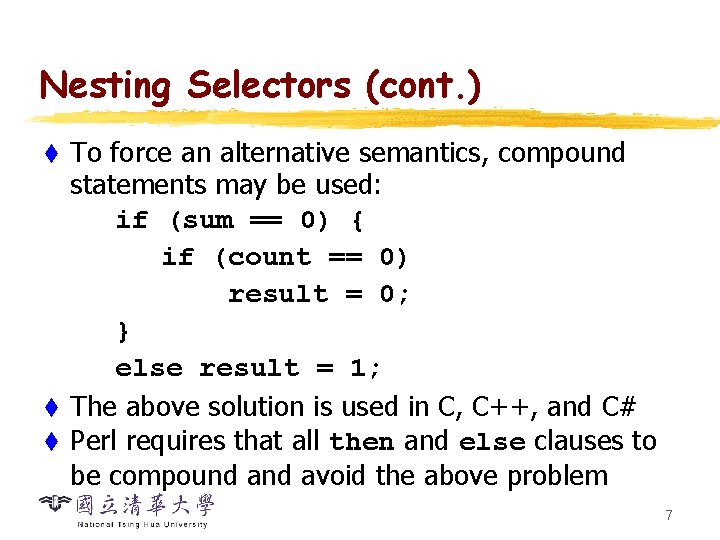
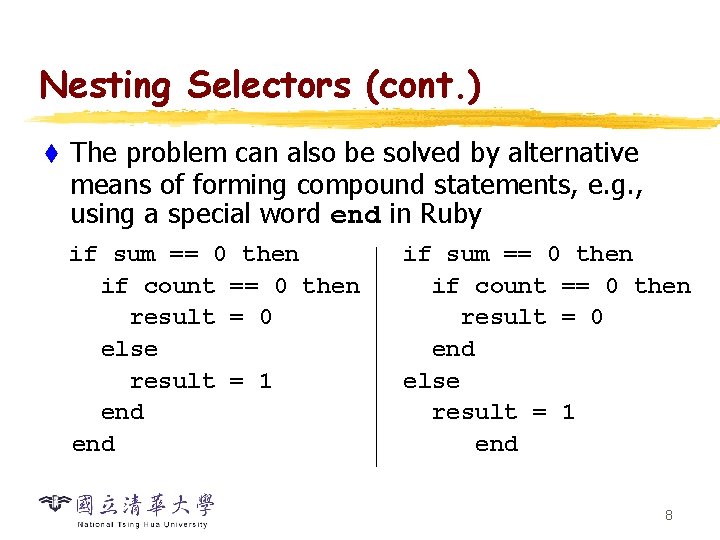
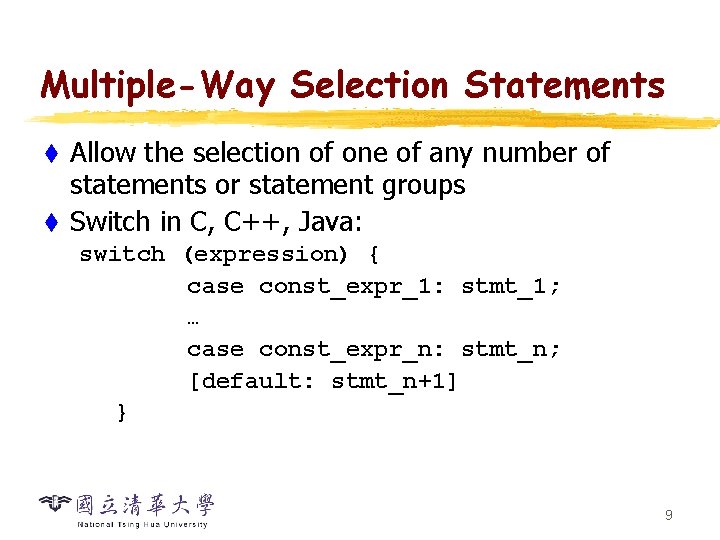
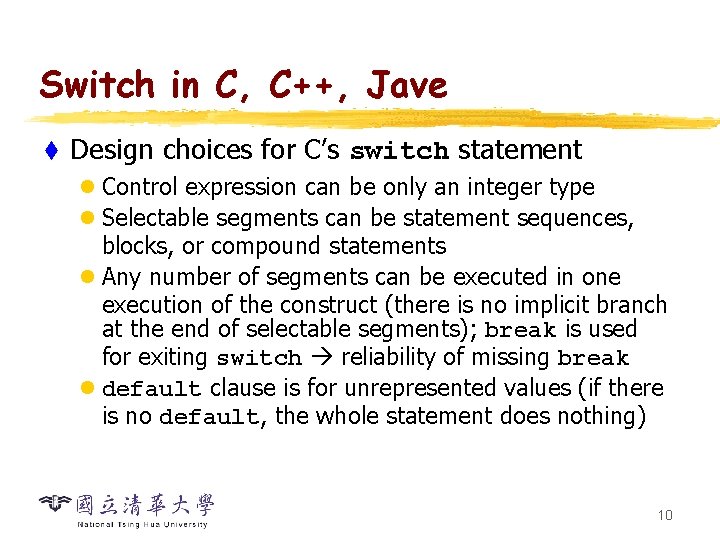
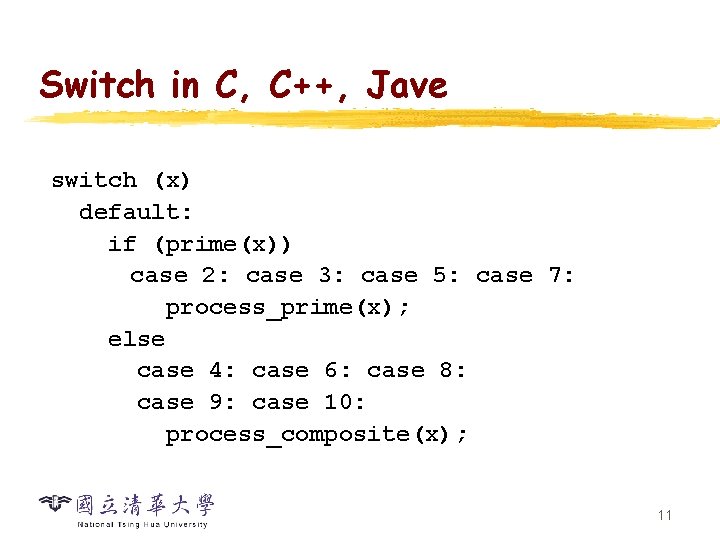
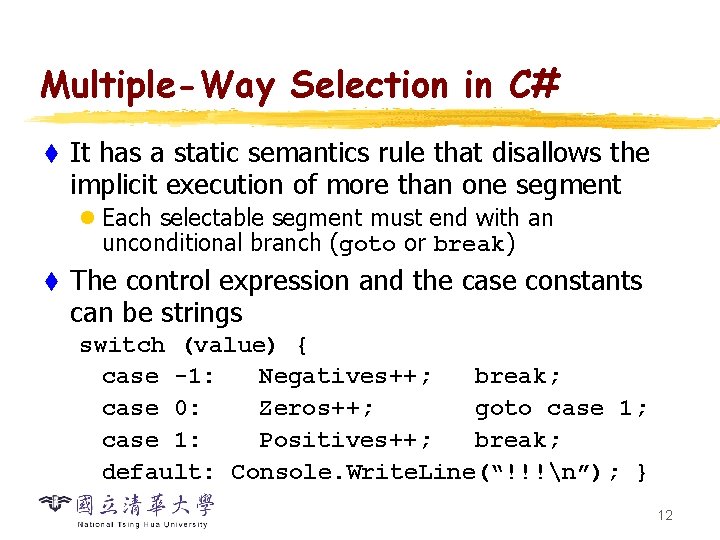
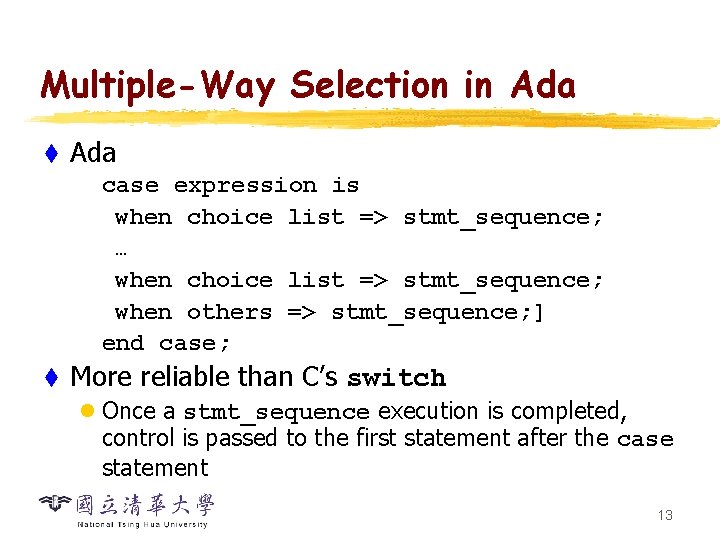
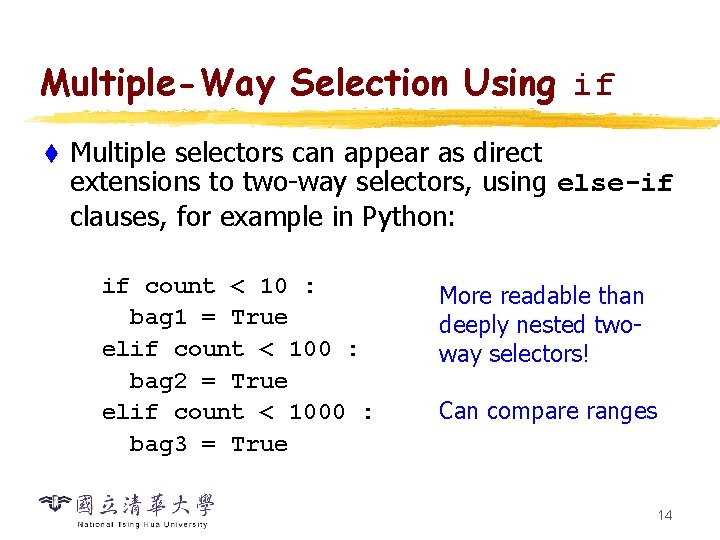
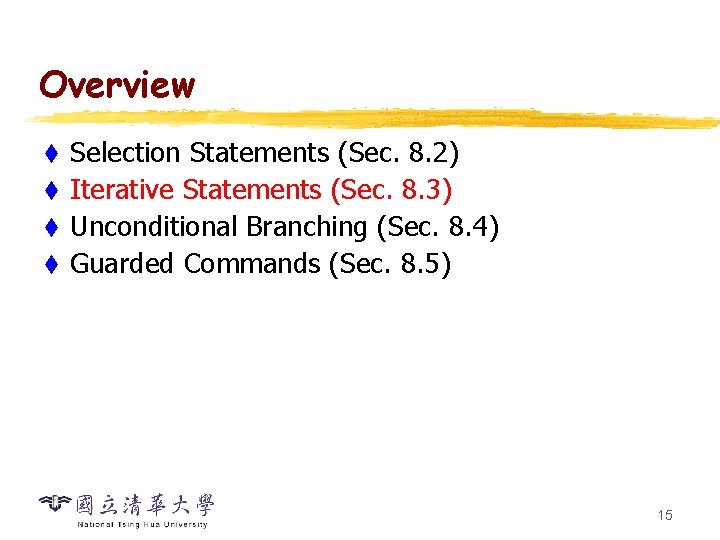
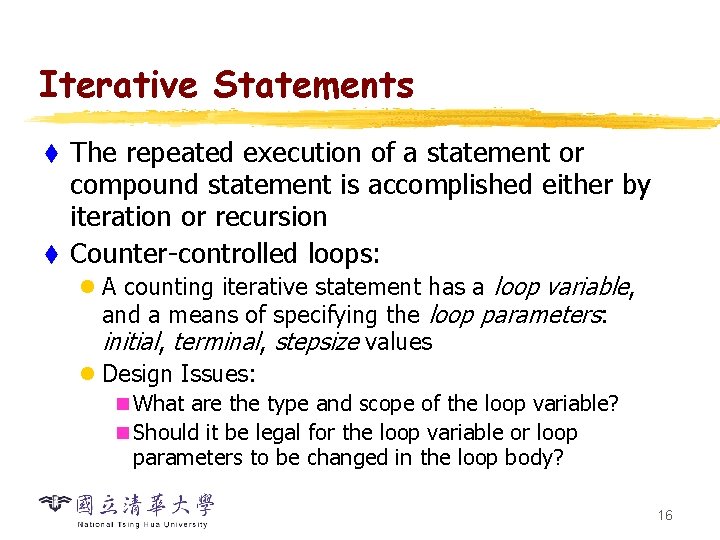
![Iterative Statements: C-based for ([expr_1] ; [expr_2] ; [expr_3]) statement t The expressions can Iterative Statements: C-based for ([expr_1] ; [expr_2] ; [expr_3]) statement t The expressions can](https://slidetodoc.com/presentation_image_h/31ca9655ab0202941c29f9cf09e3af8c/image-18.jpg)
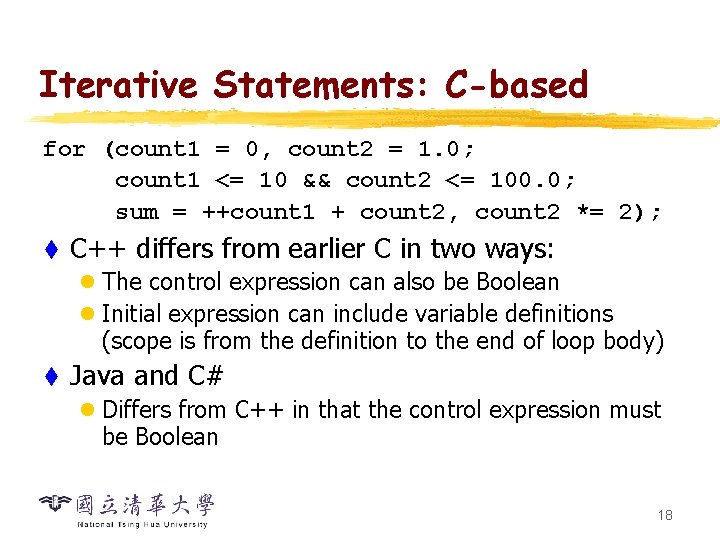
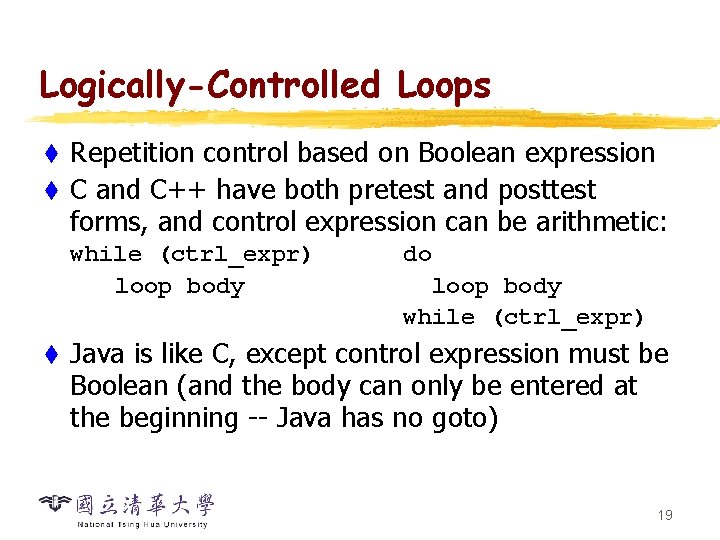
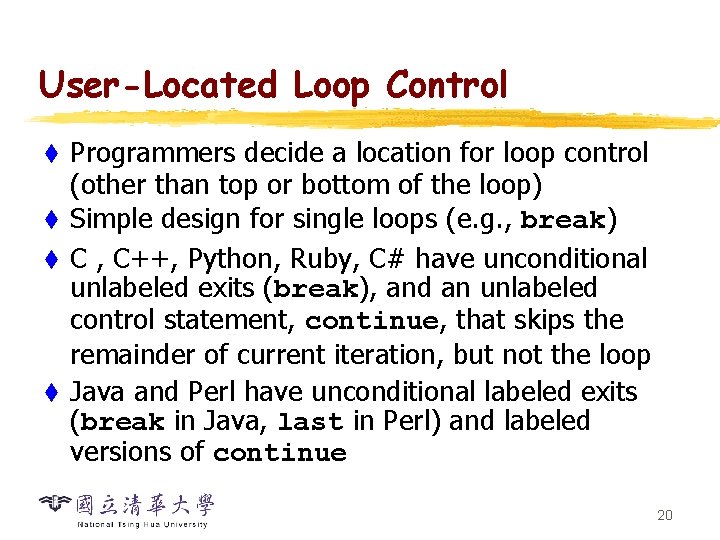
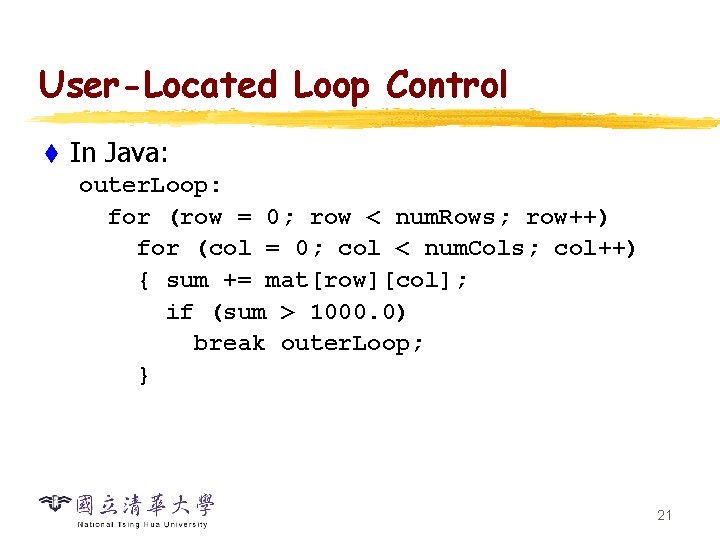
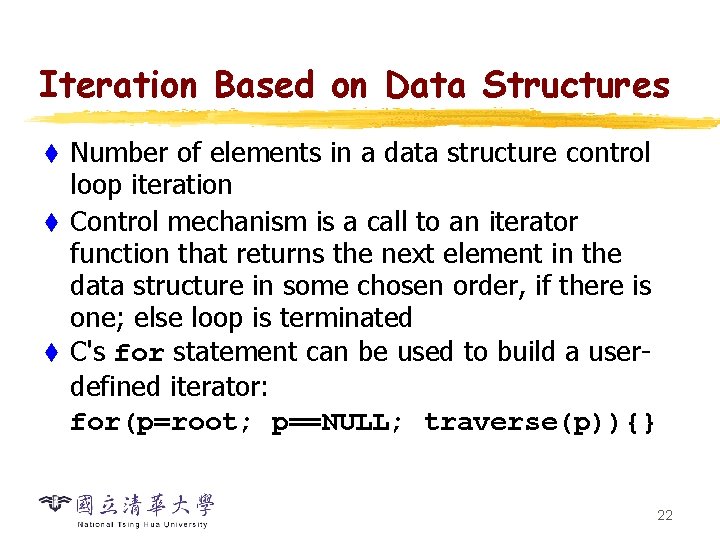
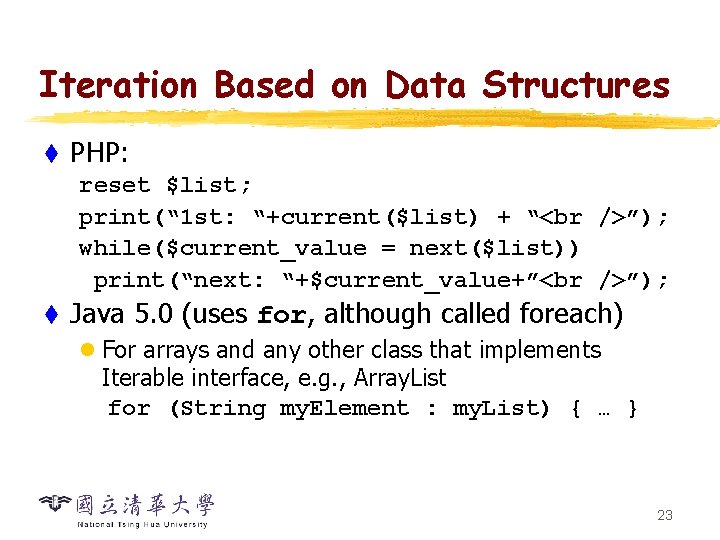
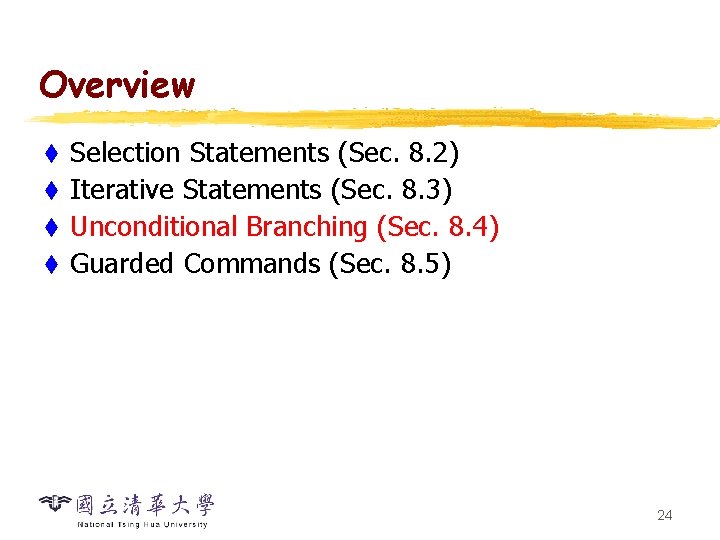
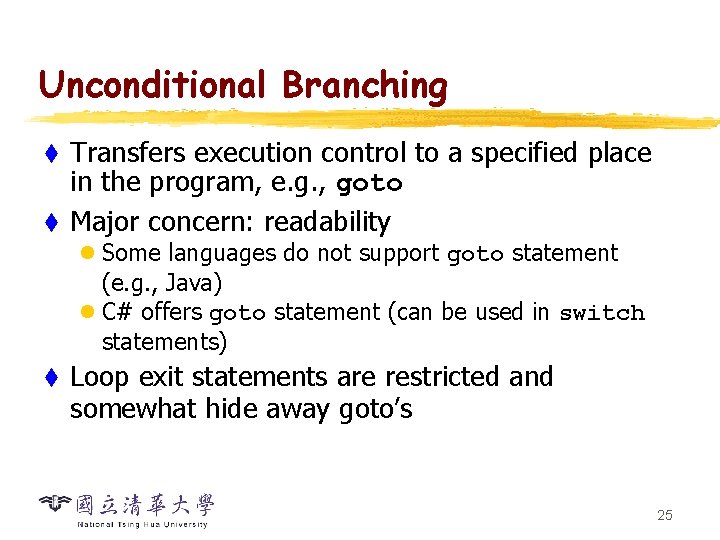
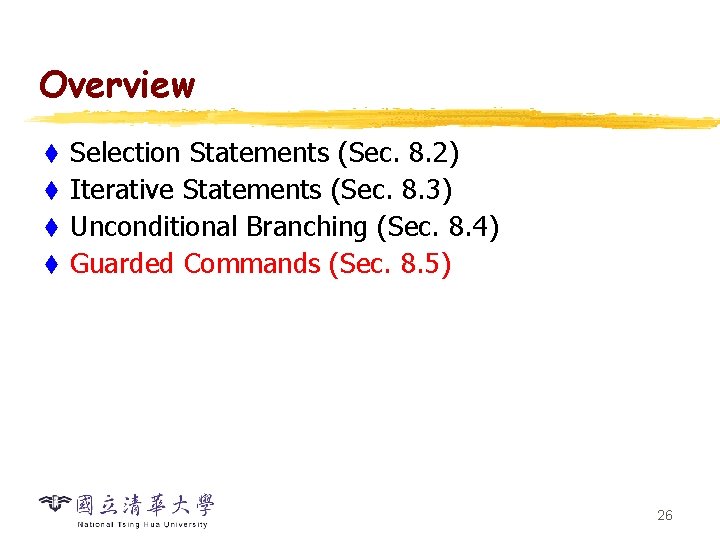
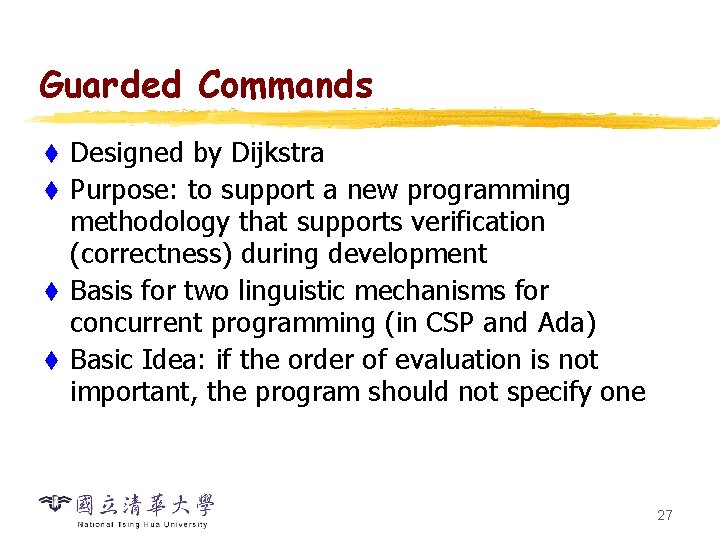
![Selection Guarded Command t Form if <Boolean exp> -> <statement> [] <Boolean exp> -> Selection Guarded Command t Form if <Boolean exp> -> <statement> [] <Boolean exp> ->](https://slidetodoc.com/presentation_image_h/31ca9655ab0202941c29f9cf09e3af8c/image-29.jpg)
![Selection Guarded Command if x >= y -> max : = x [] y Selection Guarded Command if x >= y -> max : = x [] y](https://slidetodoc.com/presentation_image_h/31ca9655ab0202941c29f9cf09e3af8c/image-30.jpg)
![Loop Guarded Command t Form do <Boolean> -> <statement> [] <Boolean> -> <statement>. . Loop Guarded Command t Form do <Boolean> -> <statement> [] <Boolean> -> <statement>. .](https://slidetodoc.com/presentation_image_h/31ca9655ab0202941c29f9cf09e3af8c/image-31.jpg)
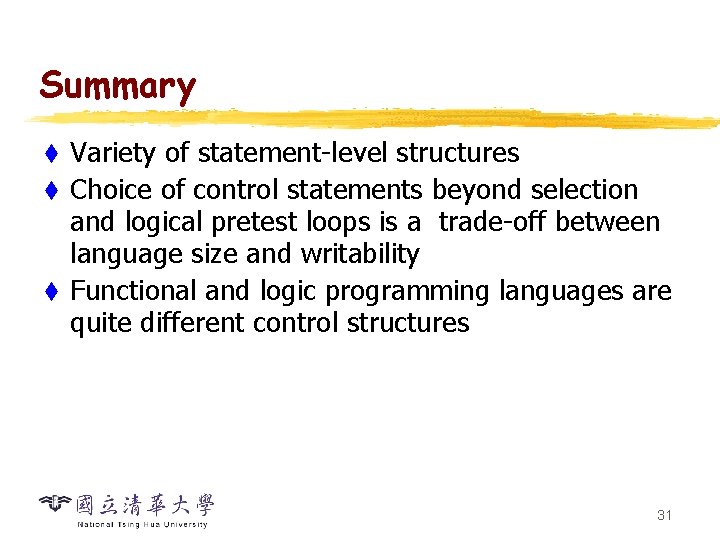
- Slides: 32
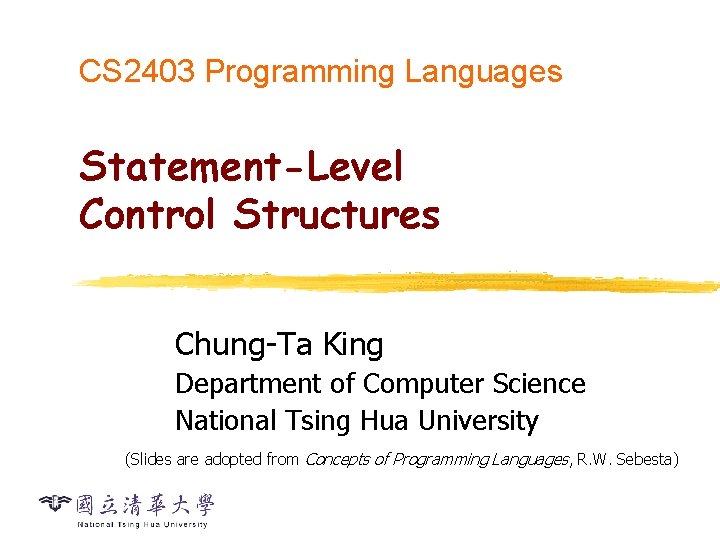
CS 2403 Programming Languages Statement-Level Control Structures Chung-Ta King Department of Computer Science National Tsing Hua University (Slides are adopted from Concepts of Programming Languages, R. W. Sebesta)
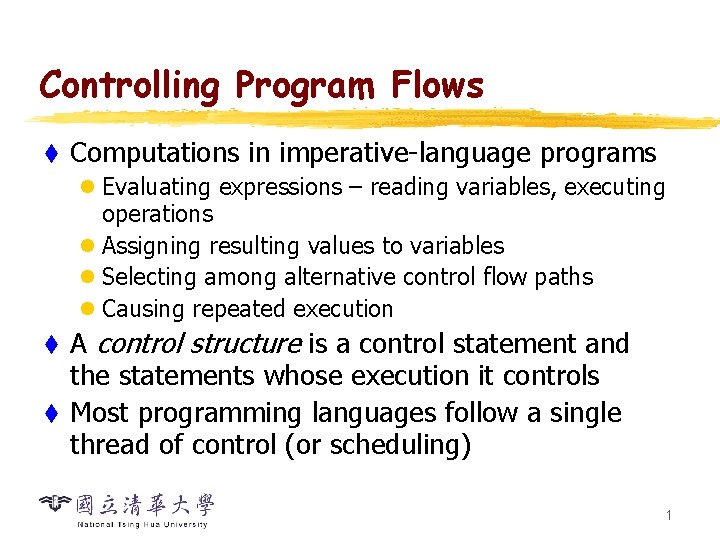
Controlling Program Flows t Computations in imperative-language programs l Evaluating expressions – reading variables, executing operations l Assigning resulting values to variables l Selecting among alternative control flow paths l Causing repeated execution A control structure is a control statement and the statements whose execution it controls t Most programming languages follow a single thread of control (or scheduling) t 1
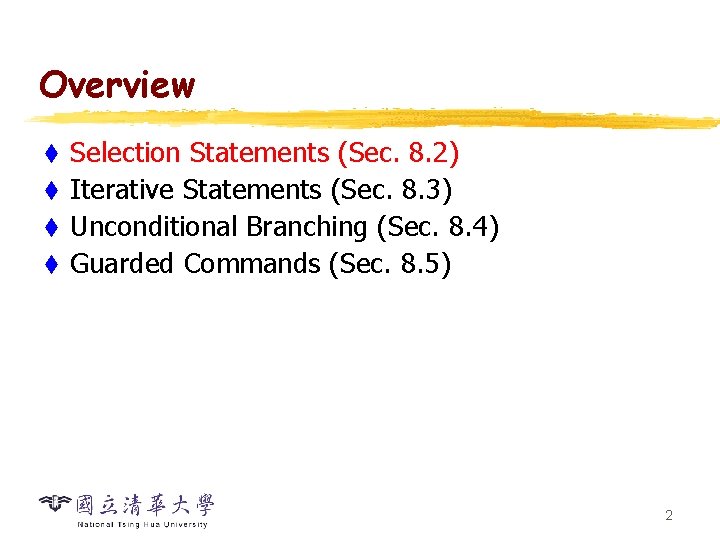
Overview Selection Statements (Sec. 8. 2) t Iterative Statements (Sec. 8. 3) t Unconditional Branching (Sec. 8. 4) t Guarded Commands (Sec. 8. 5) t 2
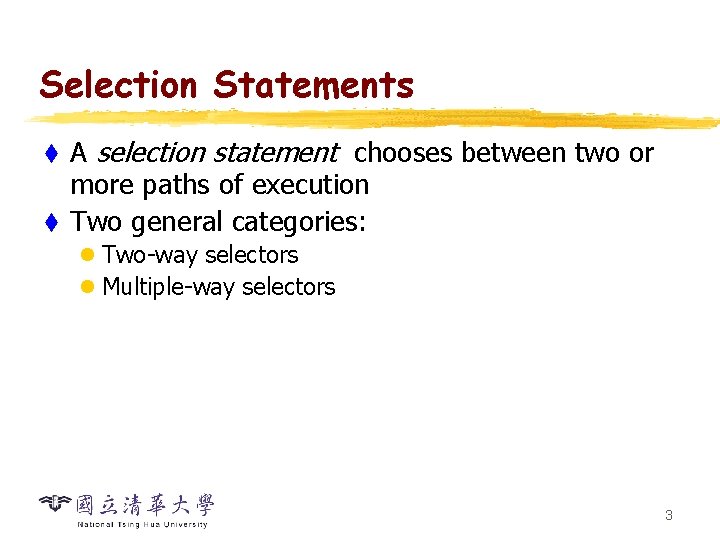
Selection Statements A selection statement chooses between two or more paths of execution t Two general categories: t l Two-way selectors l Multiple-way selectors 3
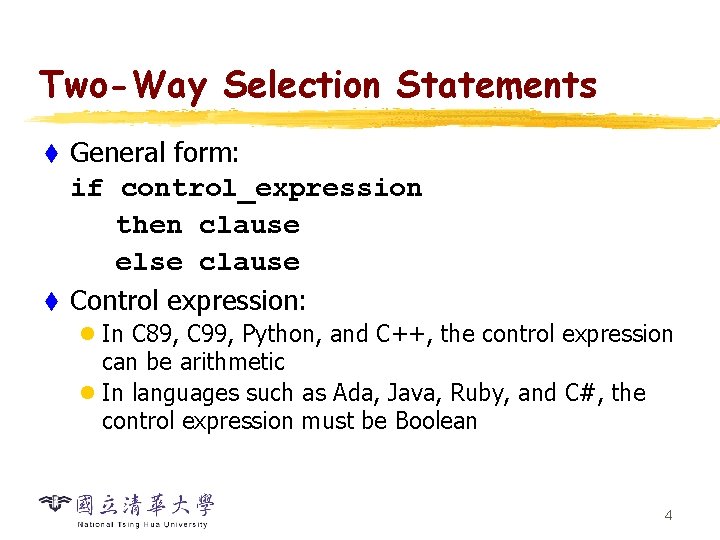
Two-Way Selection Statements General form: if control_expression then clause else clause t Control expression: t l In C 89, C 99, Python, and C++, the control expression can be arithmetic l In languages such as Ada, Java, Ruby, and C#, the control expression must be Boolean 4
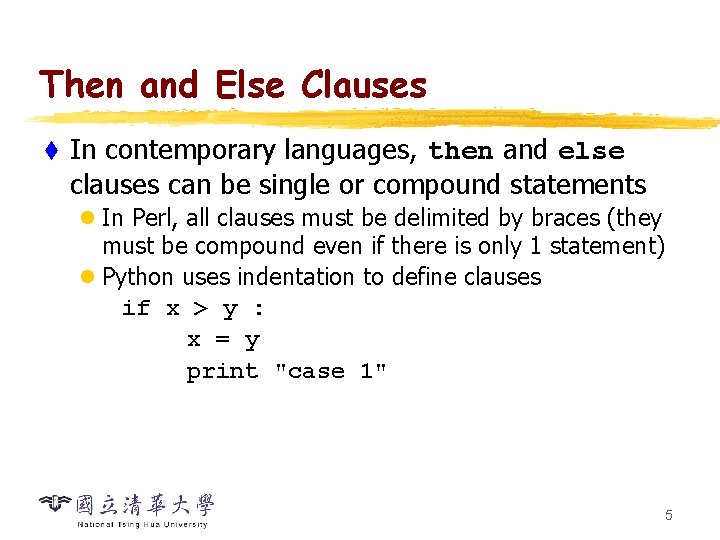
Then and Else Clauses t In contemporary languages, then and else clauses can be single or compound statements l In Perl, all clauses must be delimited by braces (they must be compound even if there is only 1 statement) l Python uses indentation to define clauses if x > y : x = y print "case 1" 5
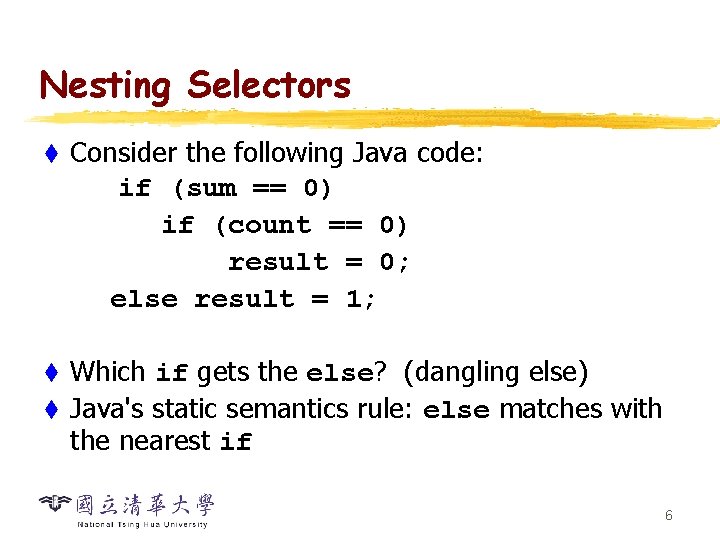
Nesting Selectors t Consider the following Java code: if (sum == 0) if (count == 0) result = 0; else result = 1; Which if gets the else? (dangling else) t Java's static semantics rule: else matches with the nearest if t 6
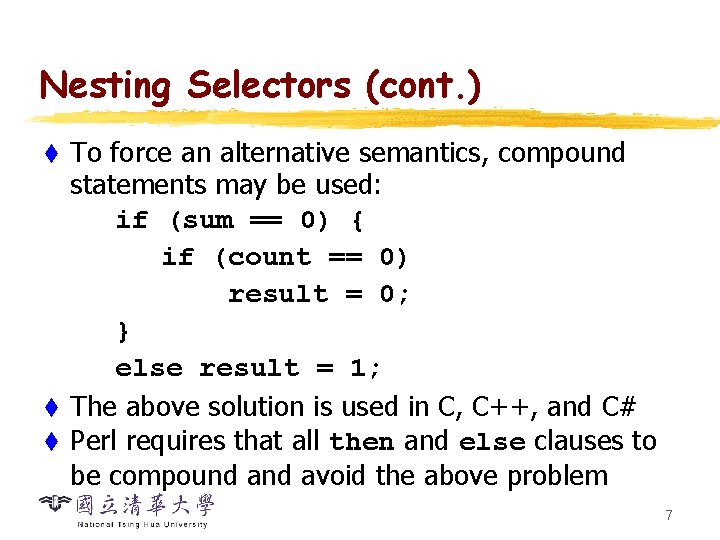
Nesting Selectors (cont. ) To force an alternative semantics, compound statements may be used: if (sum == 0) { if (count == 0) result = 0; } else result = 1; t The above solution is used in C, C++, and C# t Perl requires that all then and else clauses to be compound avoid the above problem t 7
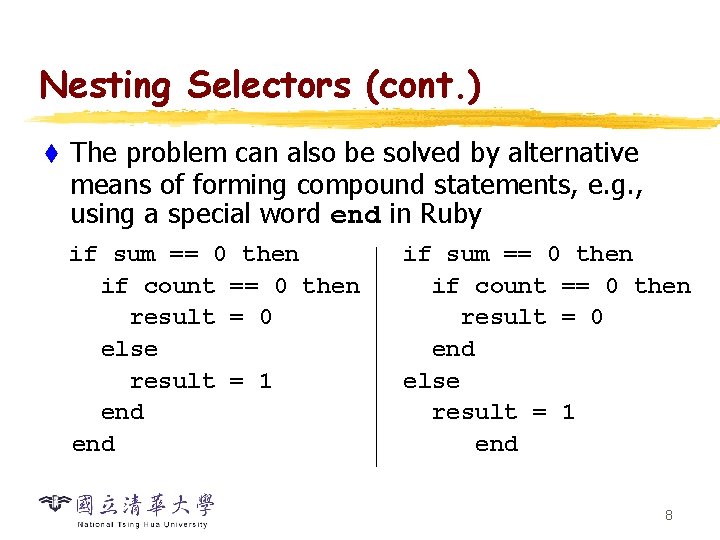
Nesting Selectors (cont. ) t The problem can also be solved by alternative means of forming compound statements, e. g. , using a special word end in Ruby if sum == 0 then if count == 0 then result = 0 else result = 1 end if sum == 0 then if count == 0 then result = 0 end else result = 1 end 8
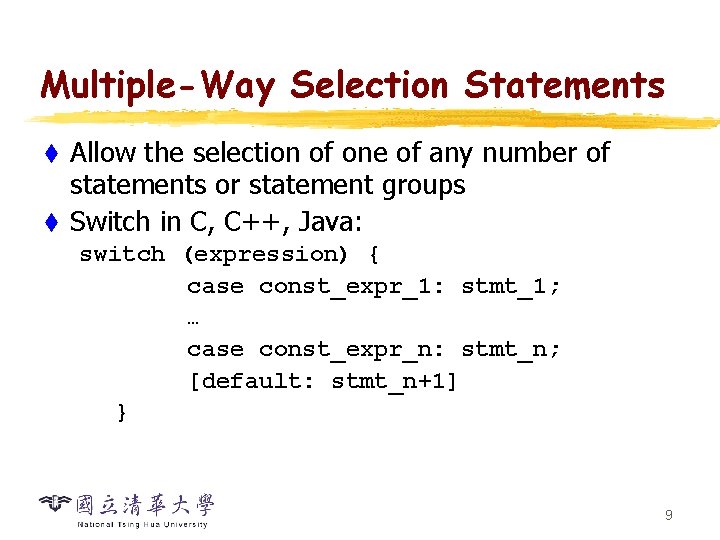
Multiple-Way Selection Statements Allow the selection of one of any number of statements or statement groups t Switch in C, C++, Java: t switch (expression) { case const_expr_1: stmt_1; … case const_expr_n: stmt_n; [default: stmt_n+1] } 9
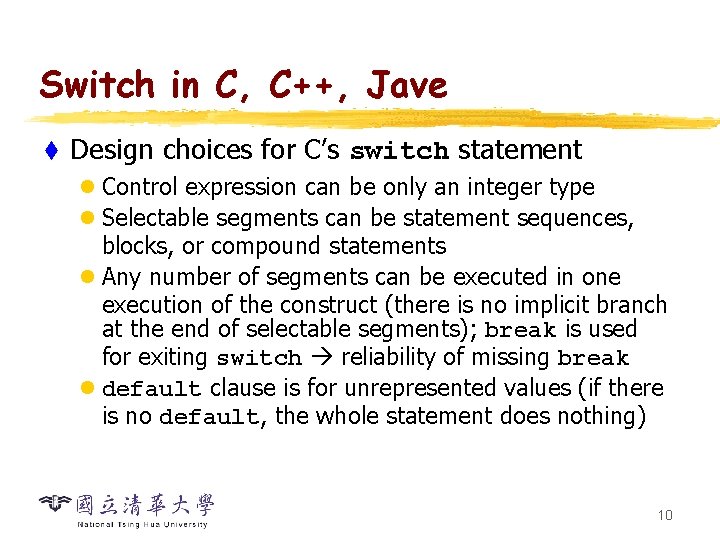
Switch in C, C++, Jave t Design choices for C’s switch statement l Control expression can be only an integer type l Selectable segments can be statement sequences, blocks, or compound statements l Any number of segments can be executed in one execution of the construct (there is no implicit branch at the end of selectable segments); break is used for exiting switch reliability of missing break l default clause is for unrepresented values (if there is no default, the whole statement does nothing) 10
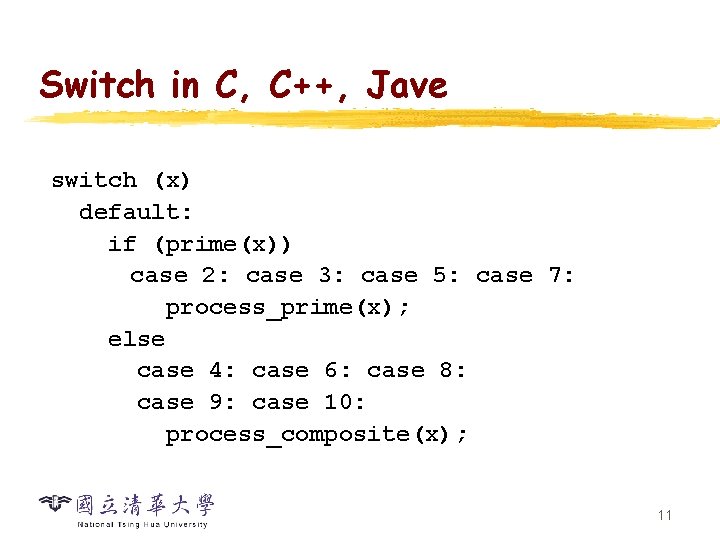
Switch in C, C++, Jave switch (x) default: if (prime(x)) case 2: case 3: case 5: case 7: process_prime(x); else case 4: case 6: case 8: case 9: case 10: process_composite(x); 11
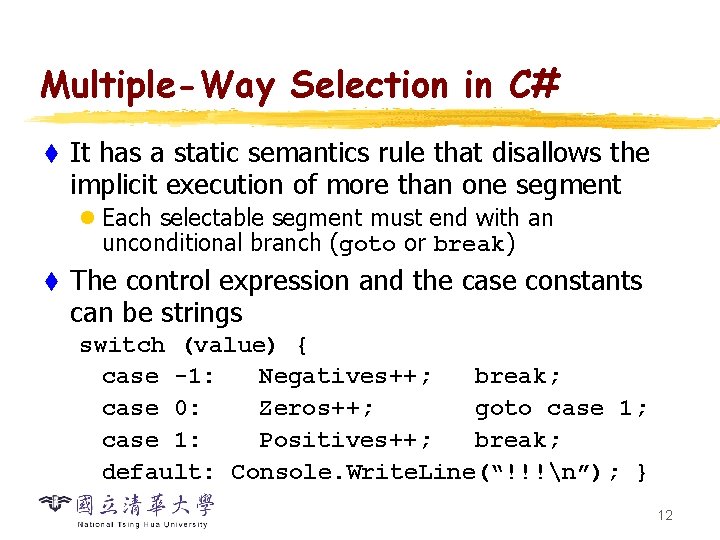
Multiple-Way Selection in C# t It has a static semantics rule that disallows the implicit execution of more than one segment l Each selectable segment must end with an unconditional branch (goto or break) t The control expression and the case constants can be strings switch (value) { case -1: Negatives++; break; case 0: Zeros++; goto case 1; case 1: Positives++; break; default: Console. Write. Line(“!!!n”); } 12
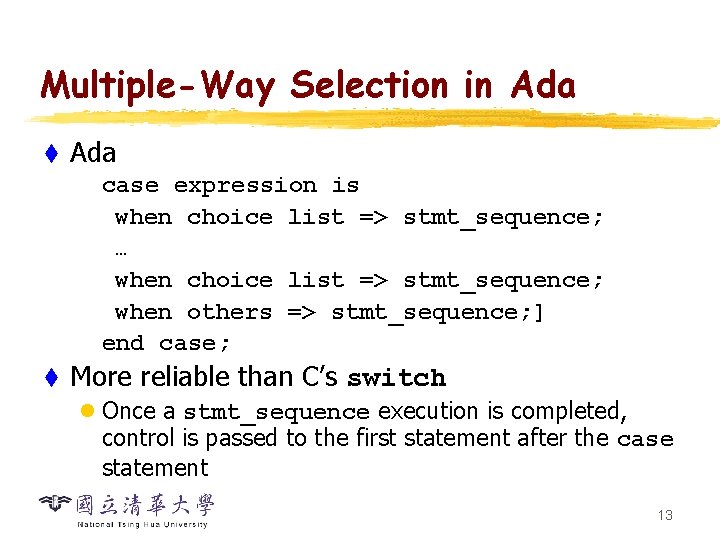
Multiple-Way Selection in Ada t Ada case expression is when choice list => stmt_sequence; … when choice list => stmt_sequence; when others => stmt_sequence; ] end case; t More reliable than C’s switch l Once a stmt_sequence execution is completed, control is passed to the first statement after the case statement 13
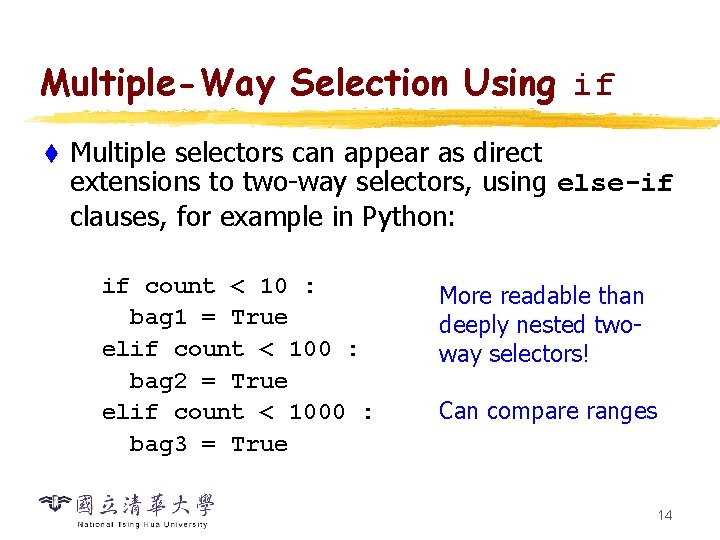
Multiple-Way Selection Using if t Multiple selectors can appear as direct extensions to two-way selectors, using else-if clauses, for example in Python: if count < 10 : bag 1 = True elif count < 100 : bag 2 = True elif count < 1000 : bag 3 = True More readable than deeply nested twoway selectors! Can compare ranges 14
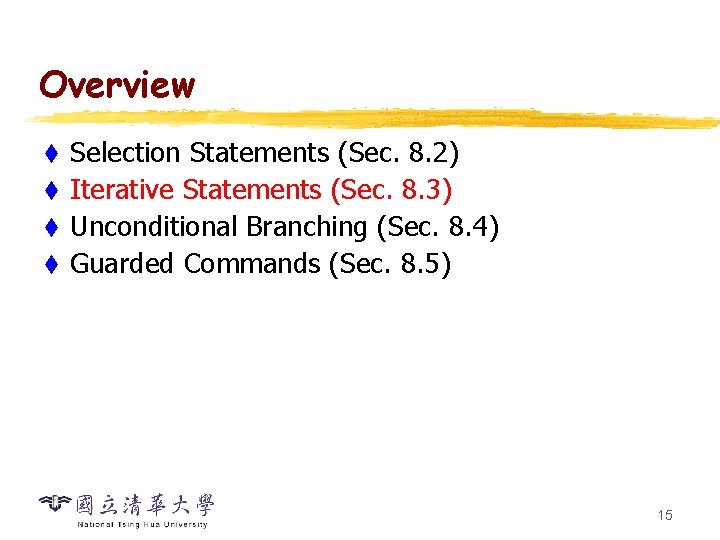
Overview Selection Statements (Sec. 8. 2) t Iterative Statements (Sec. 8. 3) t Unconditional Branching (Sec. 8. 4) t Guarded Commands (Sec. 8. 5) t 15
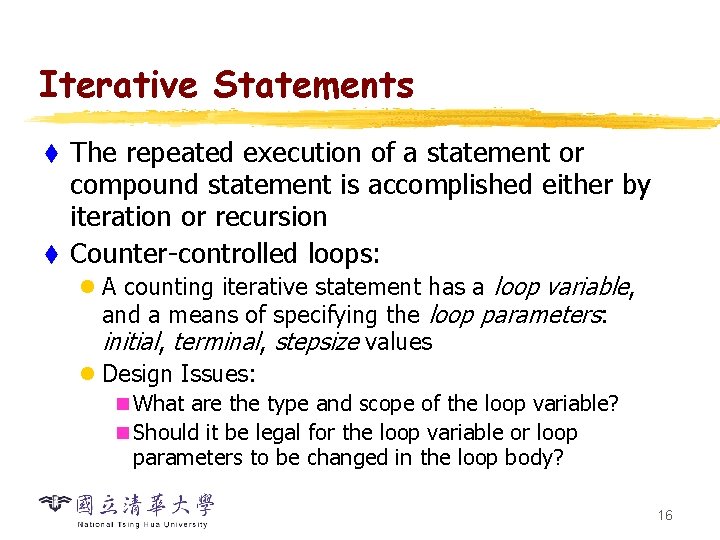
Iterative Statements The repeated execution of a statement or compound statement is accomplished either by iteration or recursion t Counter-controlled loops: t l A counting iterative statement has a loop variable, and a means of specifying the loop parameters: initial, terminal, stepsize values l Design Issues: n What are the type and scope of the loop variable? n Should it be legal for the loop variable or loop parameters to be changed in the loop body? 16
![Iterative Statements Cbased for expr1 expr2 expr3 statement t The expressions can Iterative Statements: C-based for ([expr_1] ; [expr_2] ; [expr_3]) statement t The expressions can](https://slidetodoc.com/presentation_image_h/31ca9655ab0202941c29f9cf09e3af8c/image-18.jpg)
Iterative Statements: C-based for ([expr_1] ; [expr_2] ; [expr_3]) statement t The expressions can be whole statements or statement sequences, separated by commas l The value of a multiple-statement expression is the value of the last statement in the expression l If second expression is absent, it is an infinite loop t Design choices: l No explicit loop variable the loop needs not count l Everything can be changed in the loop l 1 st expr evaluated once, others with each iteration 17
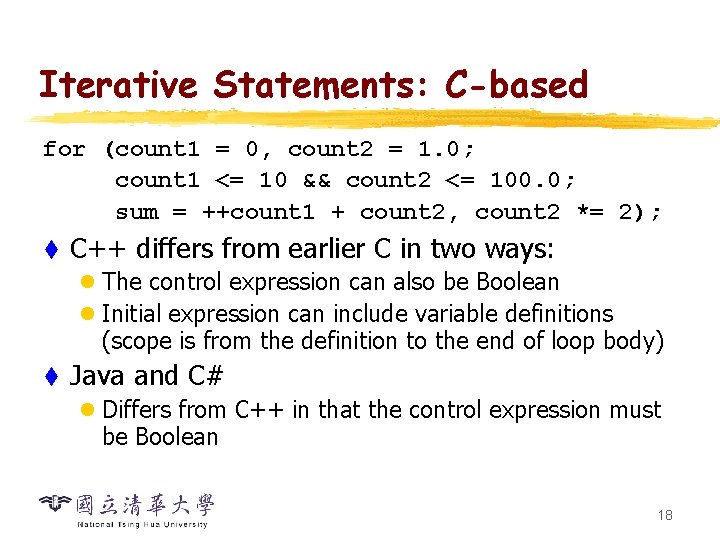
Iterative Statements: C-based for (count 1 = 0, count 2 = 1. 0; count 1 <= 10 && count 2 <= 100. 0; sum = ++count 1 + count 2, count 2 *= 2); t C++ differs from earlier C in two ways: l The control expression can also be Boolean l Initial expression can include variable definitions (scope is from the definition to the end of loop body) t Java and C# l Differs from C++ in that the control expression must be Boolean 18
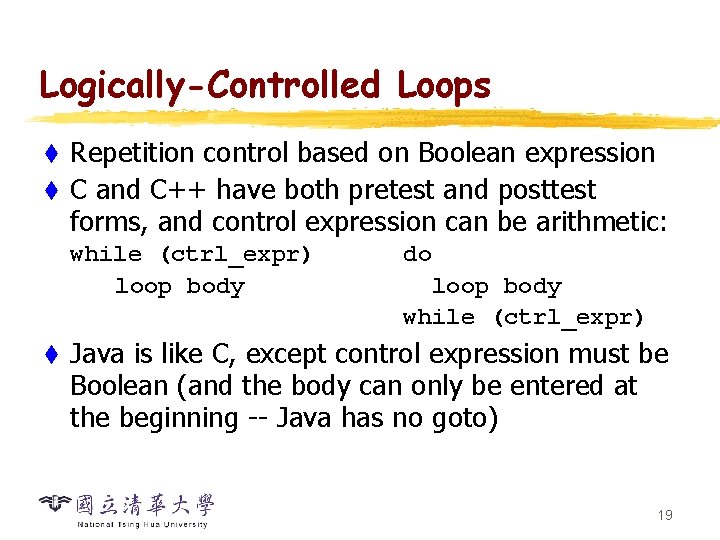
Logically-Controlled Loops Repetition control based on Boolean expression t C and C++ have both pretest and posttest forms, and control expression can be arithmetic: t while (ctrl_expr) loop body t do loop body while (ctrl_expr) Java is like C, except control expression must be Boolean (and the body can only be entered at the beginning -- Java has no goto) 19
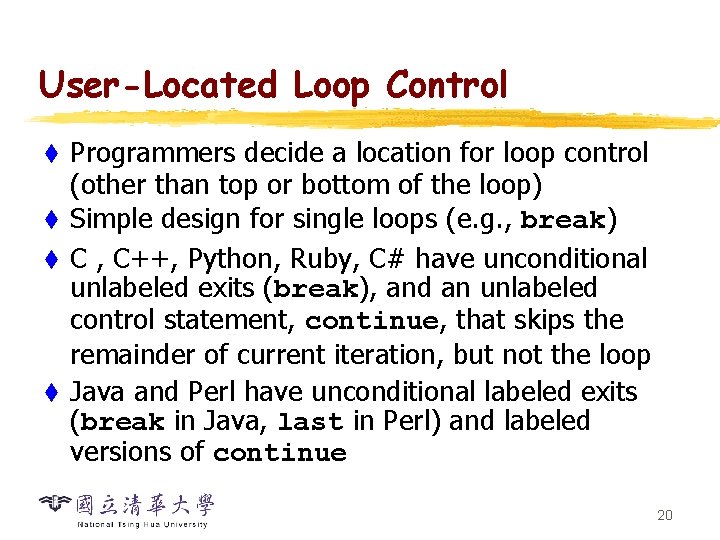
User-Located Loop Control Programmers decide a location for loop control (other than top or bottom of the loop) t Simple design for single loops (e. g. , break) t C , C++, Python, Ruby, C# have unconditional unlabeled exits (break), and an unlabeled control statement, continue, that skips the remainder of current iteration, but not the loop t Java and Perl have unconditional labeled exits (break in Java, last in Perl) and labeled versions of continue t 20
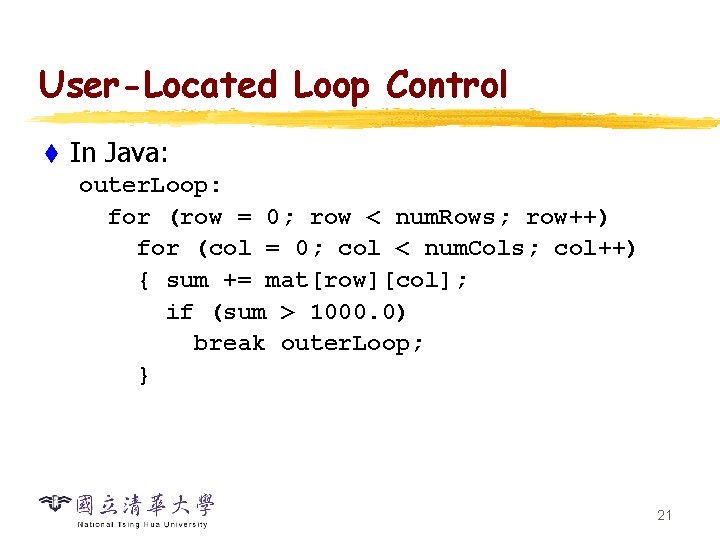
User-Located Loop Control t In Java: outer. Loop: for (row = 0; row < num. Rows; row++) for (col = 0; col < num. Cols; col++) { sum += mat[row][col]; if (sum > 1000. 0) break outer. Loop; } 21
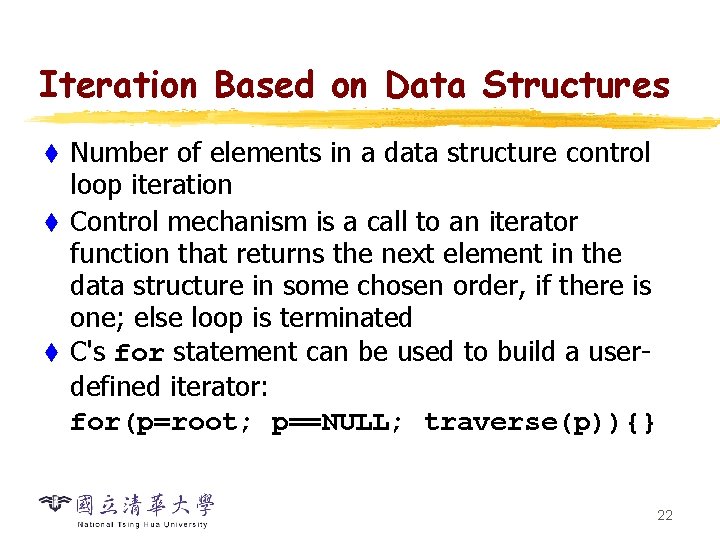
Iteration Based on Data Structures Number of elements in a data structure control loop iteration t Control mechanism is a call to an iterator function that returns the next element in the data structure in some chosen order, if there is one; else loop is terminated t C's for statement can be used to build a userdefined iterator: for(p=root; p==NULL; traverse(p)){} t 22
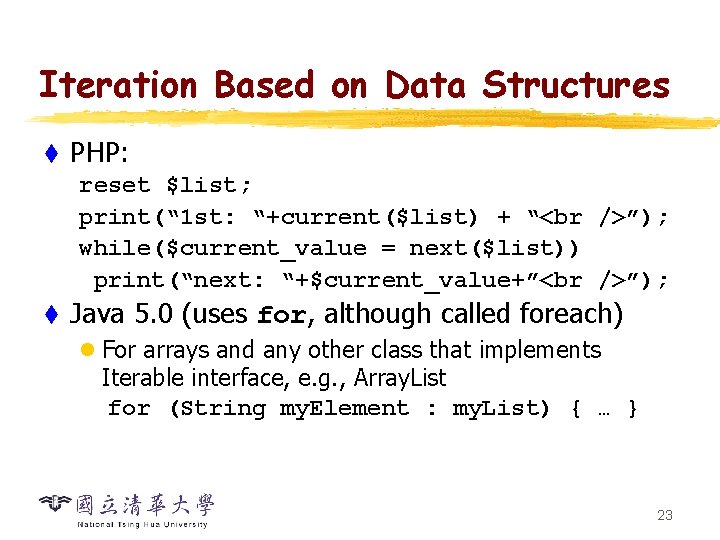
Iteration Based on Data Structures t PHP: reset $list; print(“ 1 st: “+current($list) + “ ”); while($current_value = next($list)) print(“next: “+$current_value+” ”); t Java 5. 0 (uses for, although called foreach) l For arrays and any other class that implements Iterable interface, e. g. , Array. List for (String my. Element : my. List) { … } 23
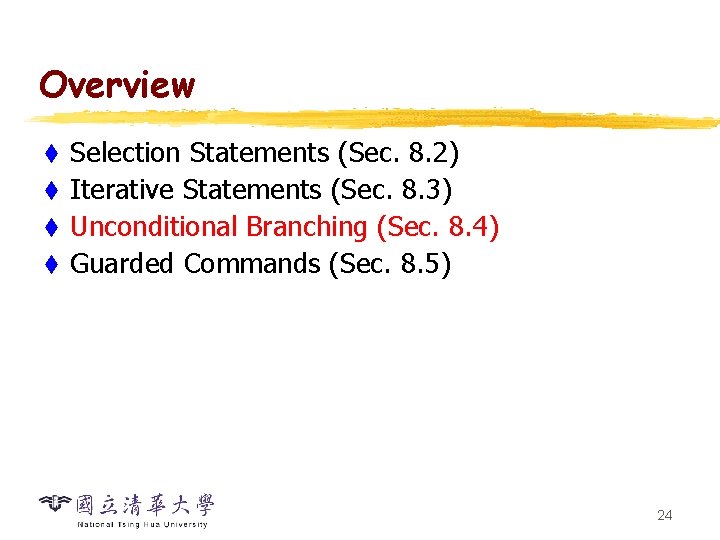
Overview Selection Statements (Sec. 8. 2) t Iterative Statements (Sec. 8. 3) t Unconditional Branching (Sec. 8. 4) t Guarded Commands (Sec. 8. 5) t 24
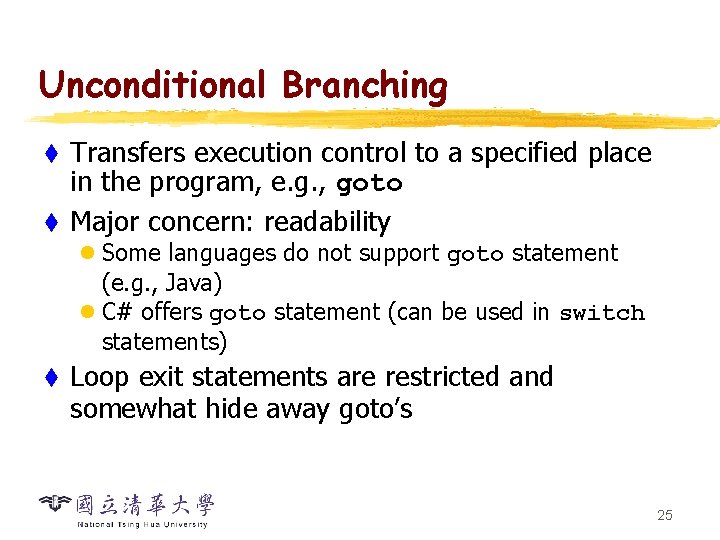
Unconditional Branching Transfers execution control to a specified place in the program, e. g. , goto t Major concern: readability t l Some languages do not support goto statement (e. g. , Java) l C# offers goto statement (can be used in switch statements) t Loop exit statements are restricted and somewhat hide away goto’s 25
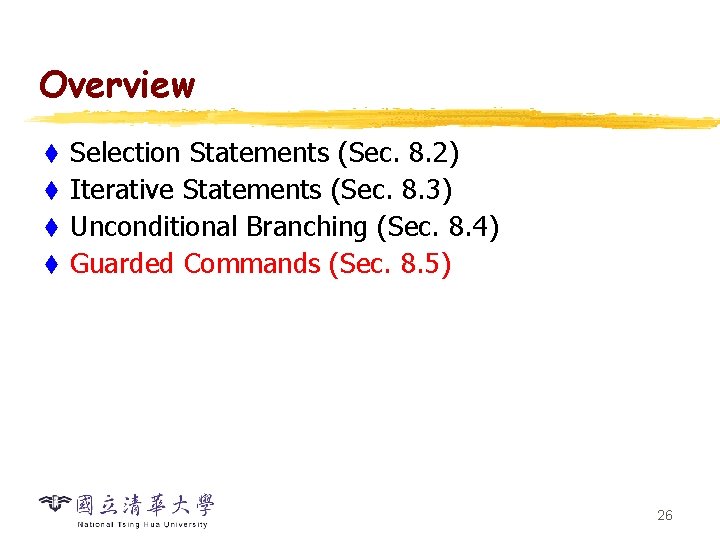
Overview Selection Statements (Sec. 8. 2) t Iterative Statements (Sec. 8. 3) t Unconditional Branching (Sec. 8. 4) t Guarded Commands (Sec. 8. 5) t 26
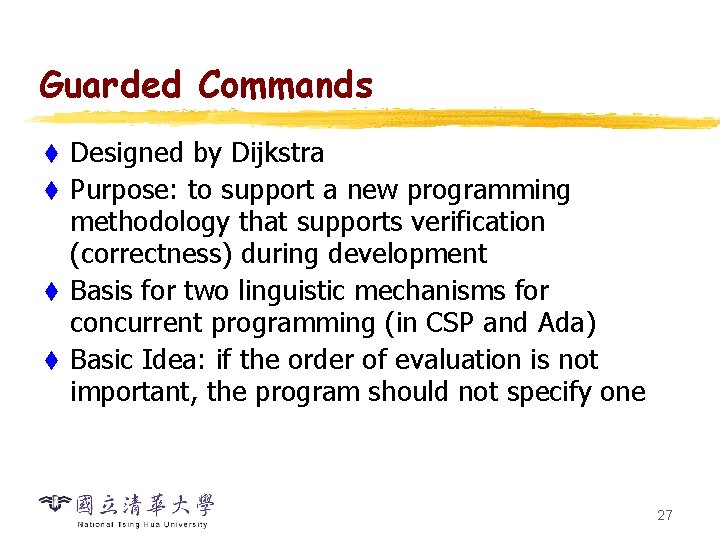
Guarded Commands Designed by Dijkstra t Purpose: to support a new programming methodology that supports verification (correctness) during development t Basis for two linguistic mechanisms for concurrent programming (in CSP and Ada) t Basic Idea: if the order of evaluation is not important, the program should not specify one t 27
![Selection Guarded Command t Form if Boolean exp statement Boolean exp Selection Guarded Command t Form if <Boolean exp> -> <statement> [] <Boolean exp> ->](https://slidetodoc.com/presentation_image_h/31ca9655ab0202941c29f9cf09e3af8c/image-29.jpg)
Selection Guarded Command t Form if <Boolean exp> -> <statement> [] <Boolean exp> -> <statement>. . . [] <Boolean exp> -> <statement> fi t Semantics: l Evaluate all Boolean expressions l If > 1 are true, choose one non-deterministically l If none are true, it is a runtime error l Prog correctness cannot depend on statement chosen 28
![Selection Guarded Command if x y max x y Selection Guarded Command if x >= y -> max : = x [] y](https://slidetodoc.com/presentation_image_h/31ca9655ab0202941c29f9cf09e3af8c/image-30.jpg)
Selection Guarded Command if x >= y -> max : = x [] y >= x => max : = y fi Compare with the following code: if (x >= y) max = x; else max = y; 29
![Loop Guarded Command t Form do Boolean statement Boolean statement Loop Guarded Command t Form do <Boolean> -> <statement> [] <Boolean> -> <statement>. .](https://slidetodoc.com/presentation_image_h/31ca9655ab0202941c29f9cf09e3af8c/image-31.jpg)
Loop Guarded Command t Form do <Boolean> -> <statement> [] <Boolean> -> <statement>. . . [] <Boolean> -> <statement> od t Semantics: for each iteration l Evaluate all Boolean expressions l If more than one are true, choose one non- deterministically; then start loop again l If none are true, exit loop 30
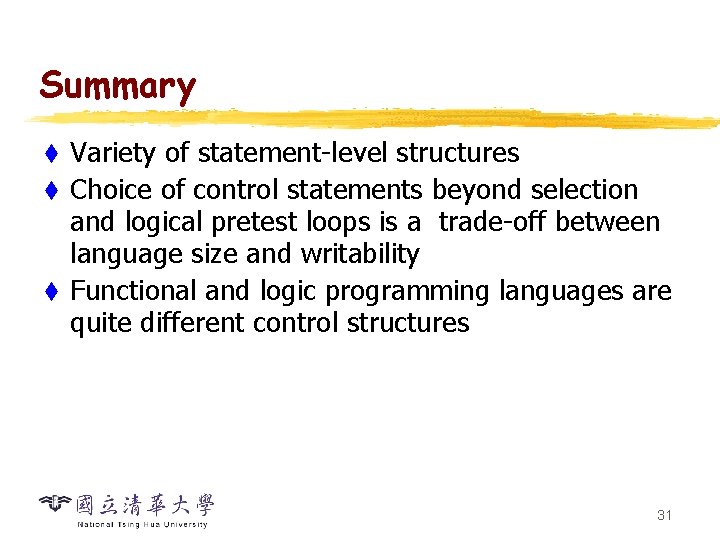
Summary Variety of statement-level structures t Choice of control statements beyond selection and logical pretest loops is a trade-off between language size and writability t Functional and logic programming languages are quite different control structures t 31
Geçici işaretler nelerdir
Mqx rtos
Chungta
Setprecision in c++
Real-time systems and programming languages
Cs 421 programming languages and compilers
Thread dalam java
Programming languages levels
Introduction to programming languages
Plc coding language
Joey paquet
Comparative programming languages
Alternative programming languages
Strongly typed vs weakly typed
Transmission programming languages
Cse 340 principles of programming languages
Int vs short
Xenia programming languages
Advantages of high level language
Mainstream programming languages
Cse 340 principles of programming languages
Programming languages
Programming languages
Programming languages
Programming languages
Attribute grammar in principles of programming languages
Brief history of programming languages
Taxonomy of programming languages
Real-time systems and programming languages
Xkcd programming language
If programming languages were cars
Reasons for studying concepts of programming languages
Cornell programming languages