CS 152 Programming Language Paradigms April 16 Class
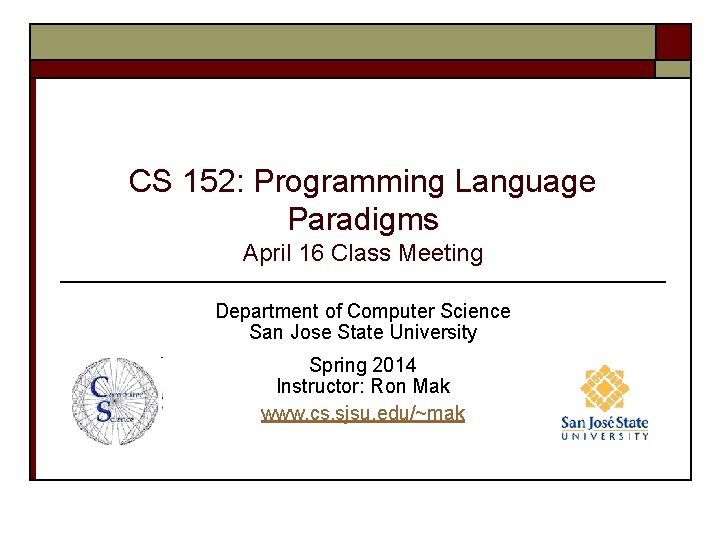
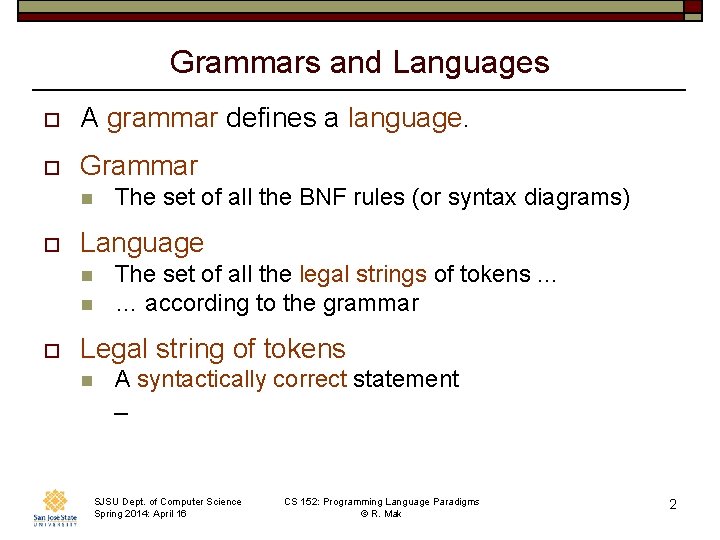
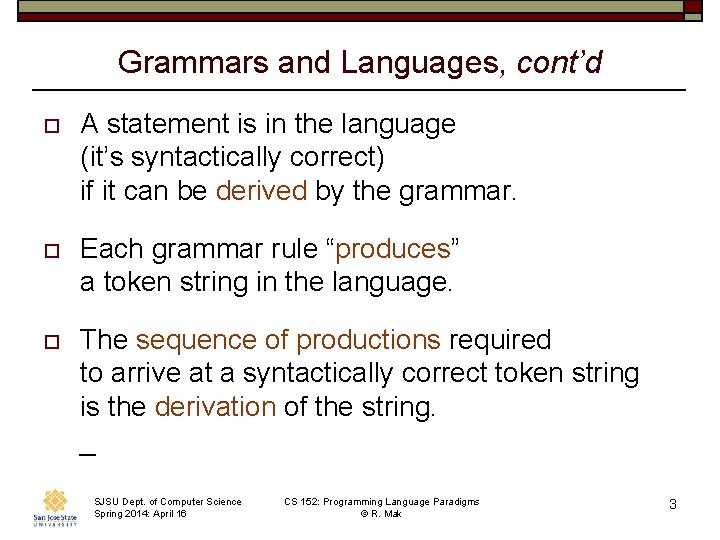
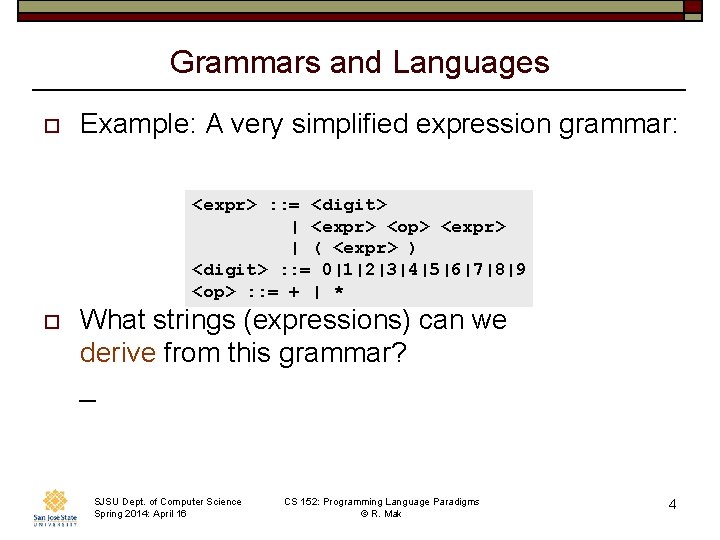
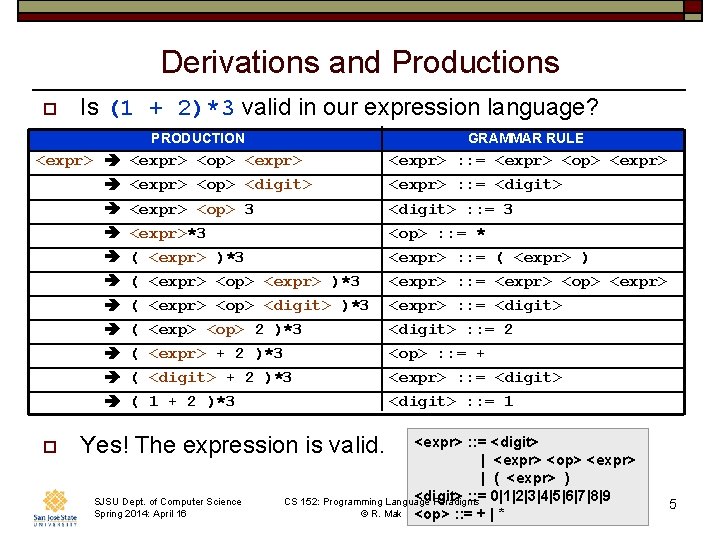
![Extended BNF (EBNF) o Extended BNF (EBNF) adds meta-symbols { } and [ ] Extended BNF (EBNF) o Extended BNF (EBNF) adds meta-symbols { } and [ ]](https://slidetodoc.com/presentation_image_h2/00cd62c62acfc4fb3887cd754bc90fcc/image-6.jpg)
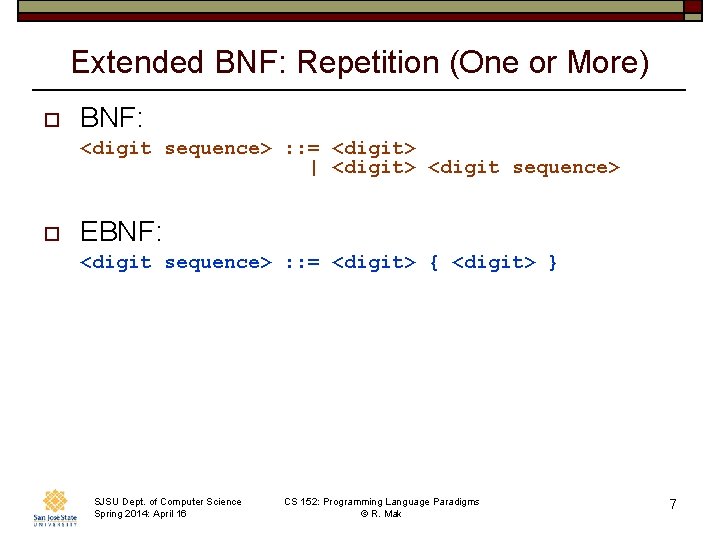
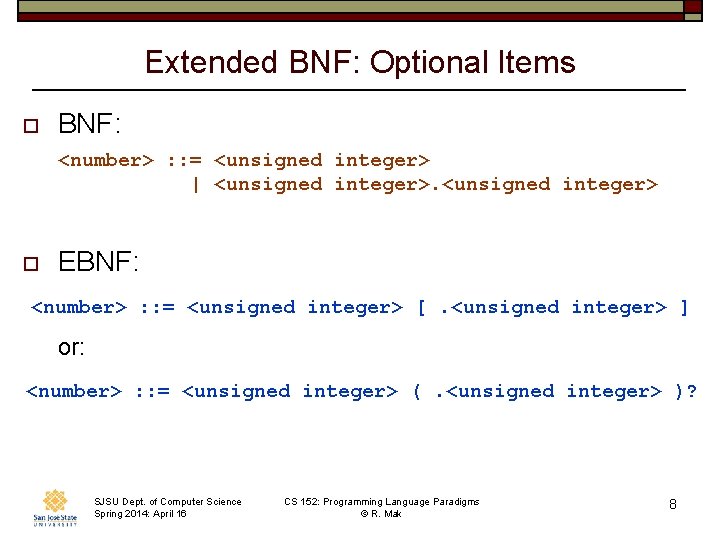
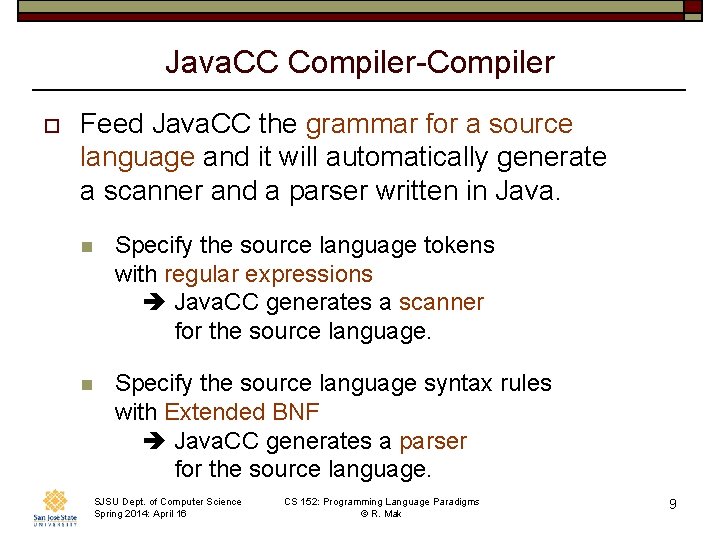
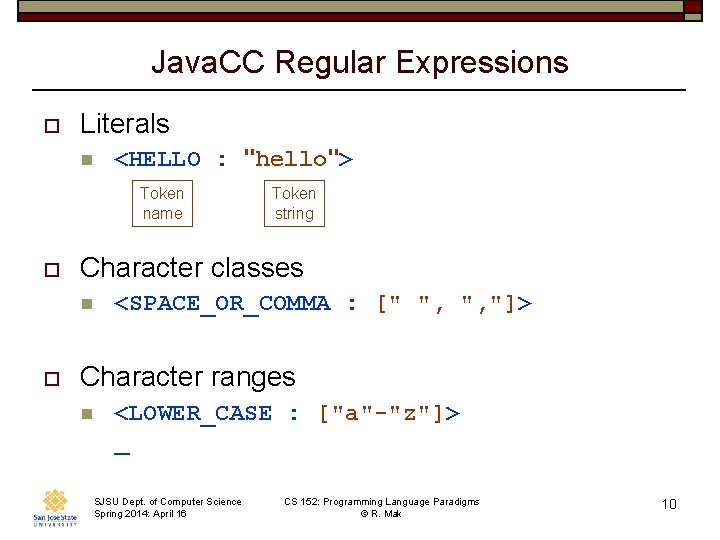
![Java. CC Regular Expressions, cont’d o Alternates <UPPER_OR_LOWER : ["A"-"Z"] | ["a"-"z"]> n o Java. CC Regular Expressions, cont’d o Alternates <UPPER_OR_LOWER : ["A"-"Z"] | ["a"-"z"]> n o](https://slidetodoc.com/presentation_image_h2/00cd62c62acfc4fb3887cd754bc90fcc/image-11.jpg)
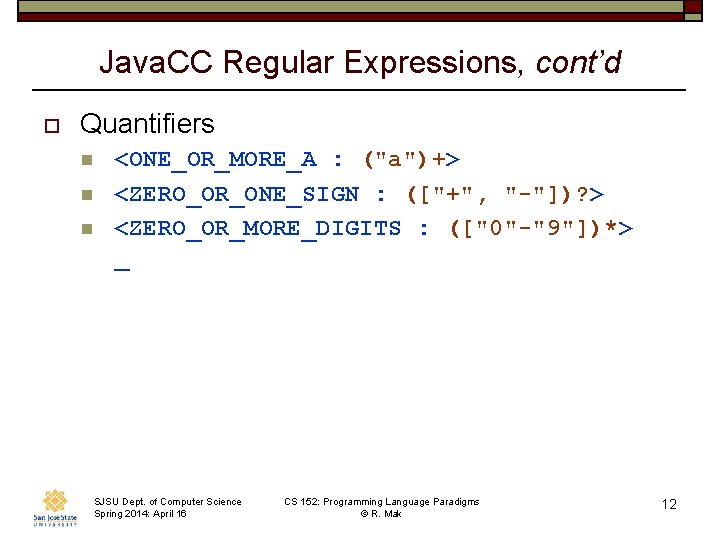
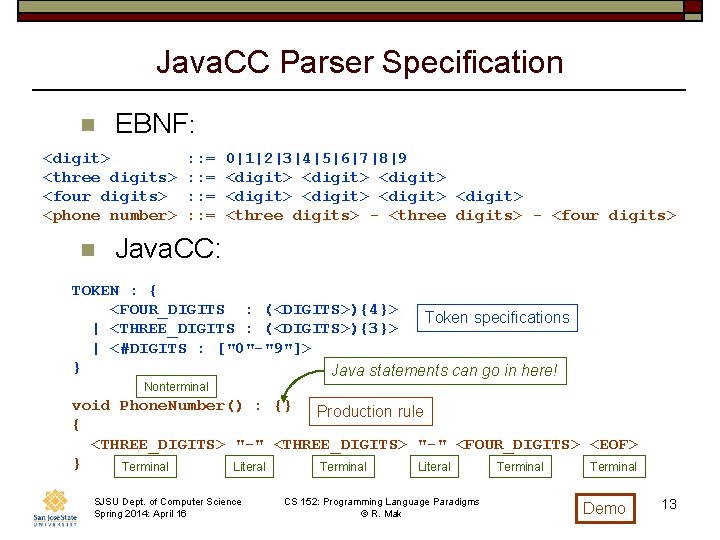
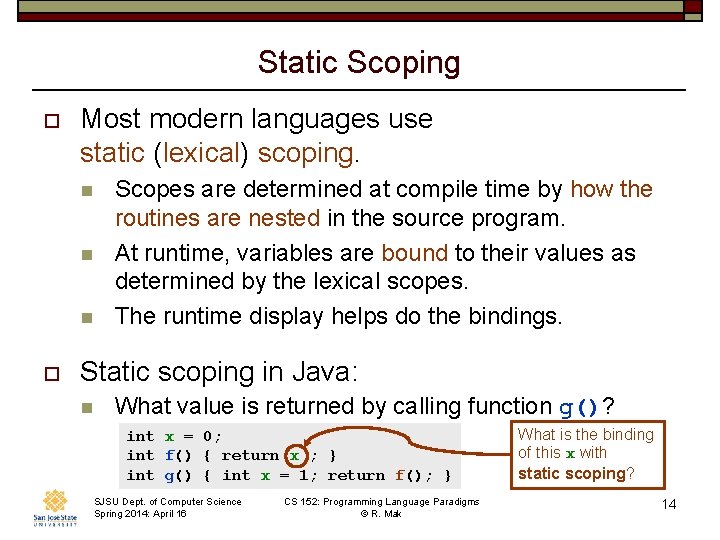
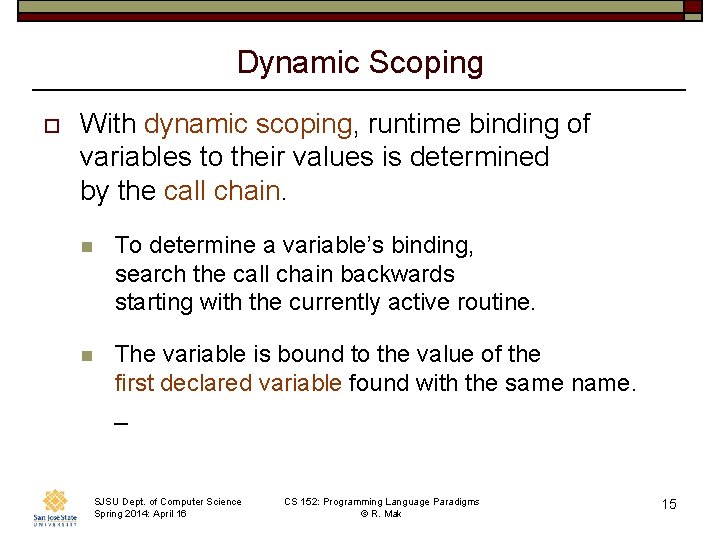
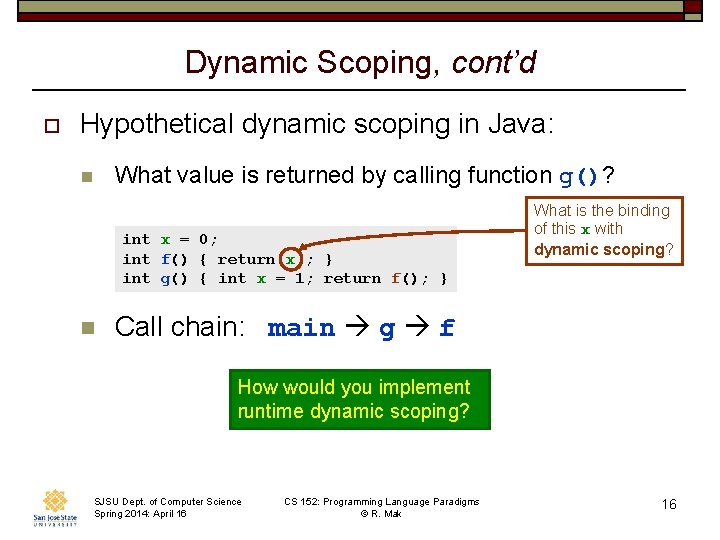
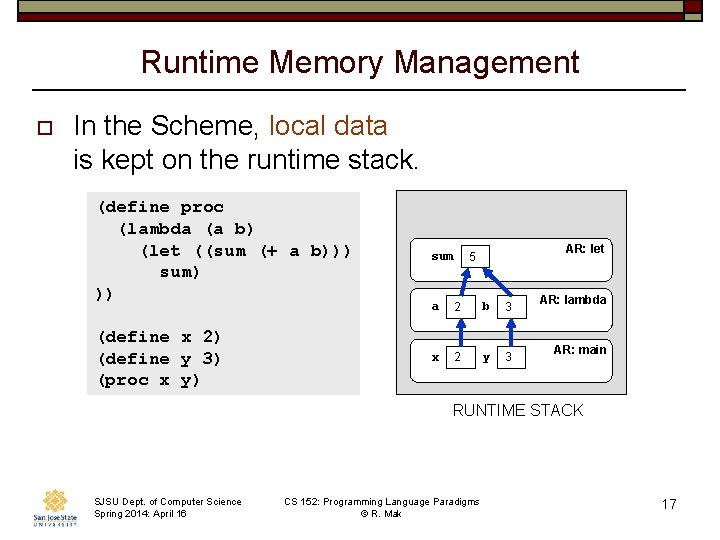
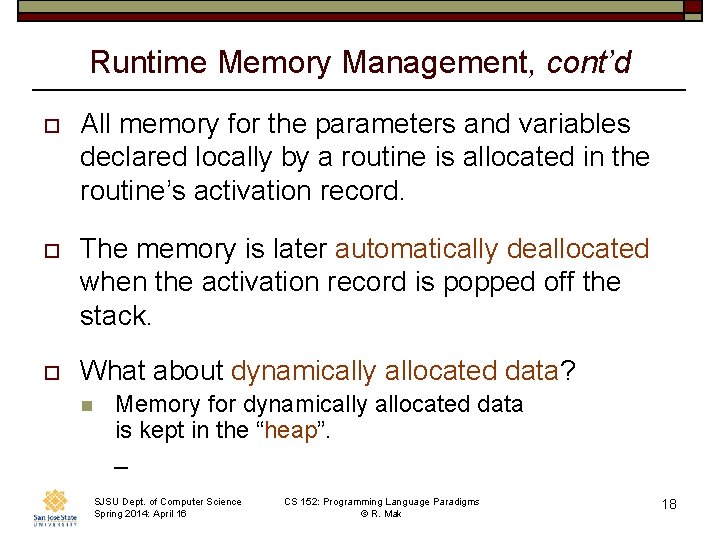
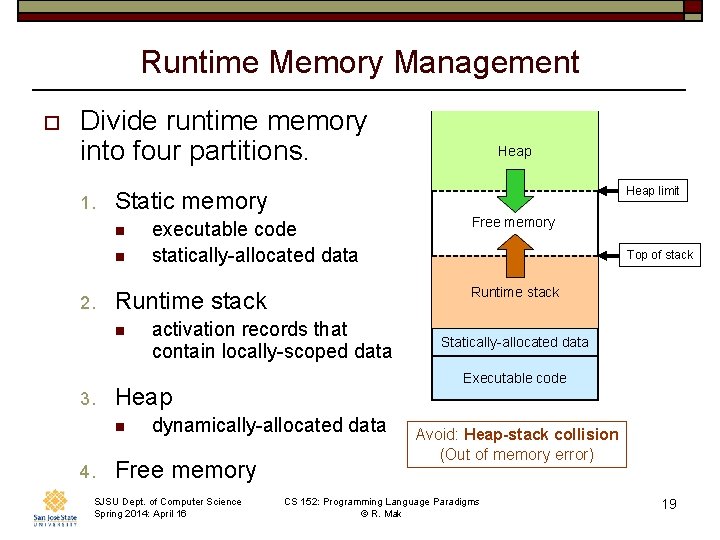
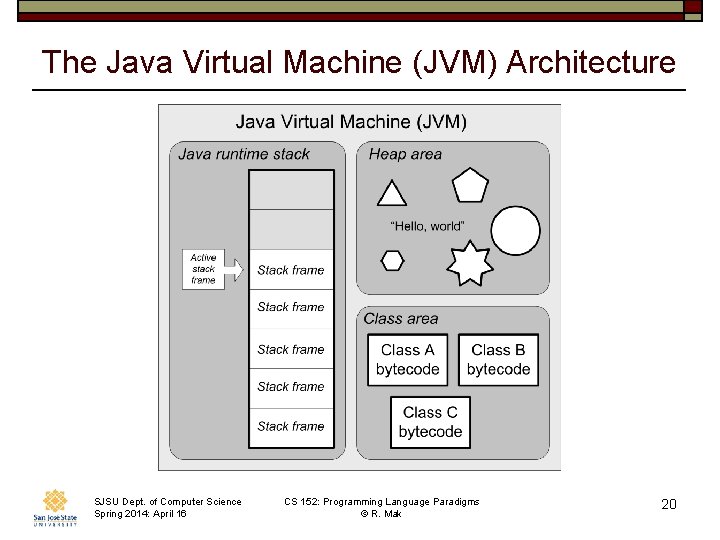
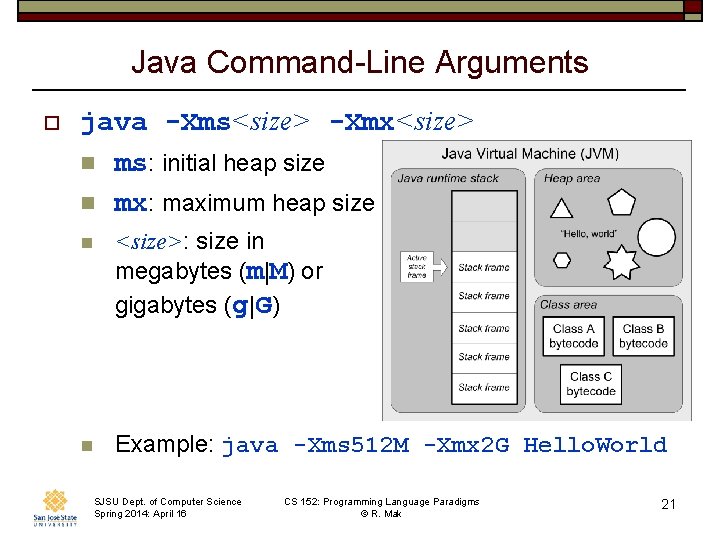
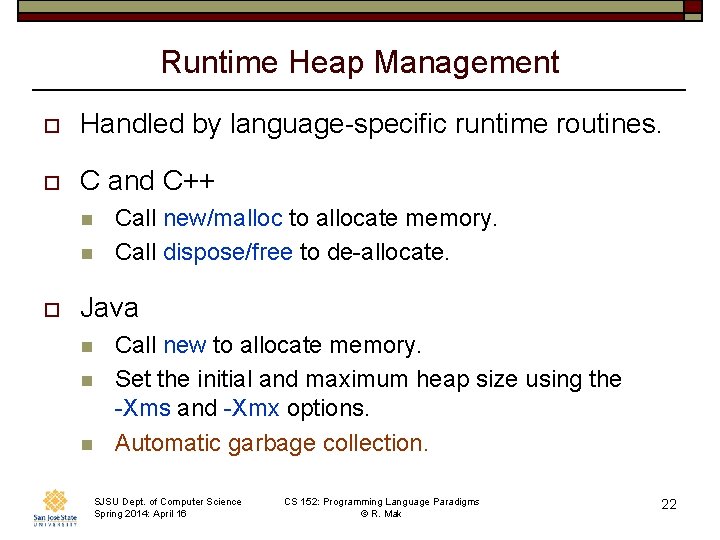
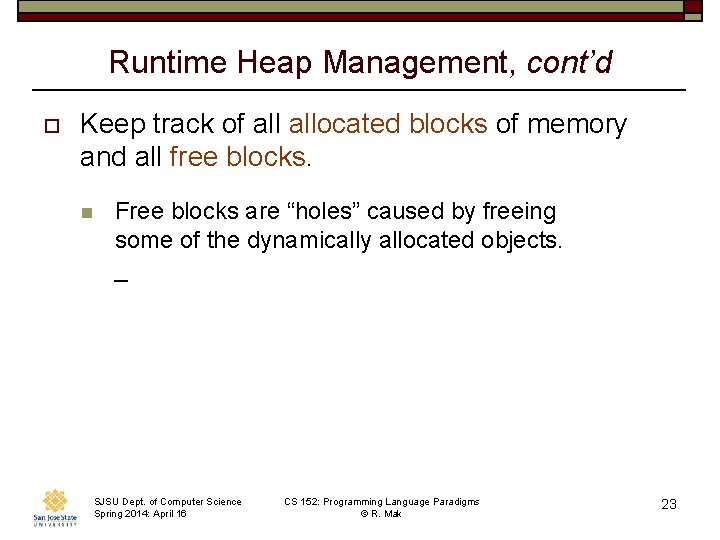
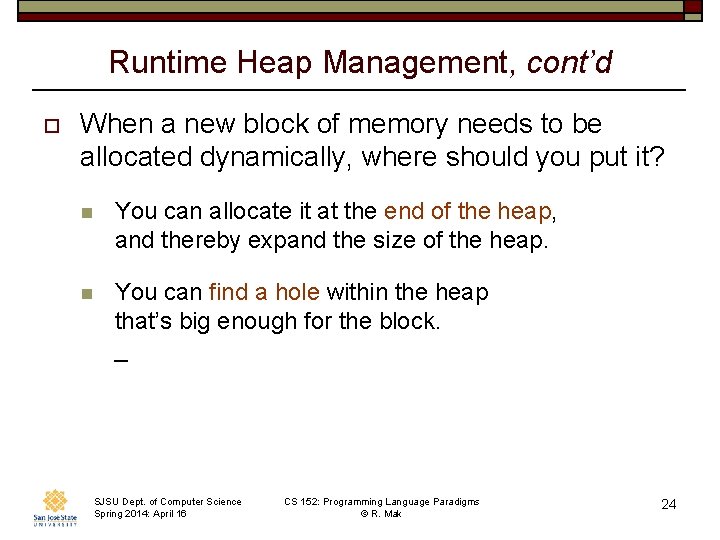
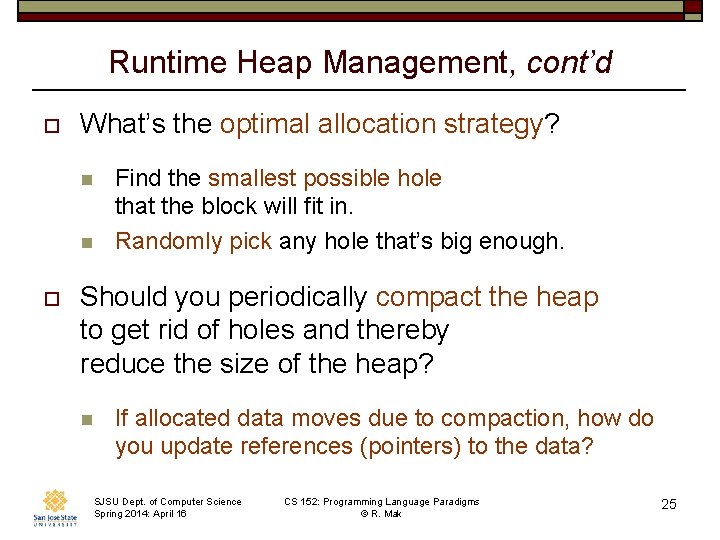
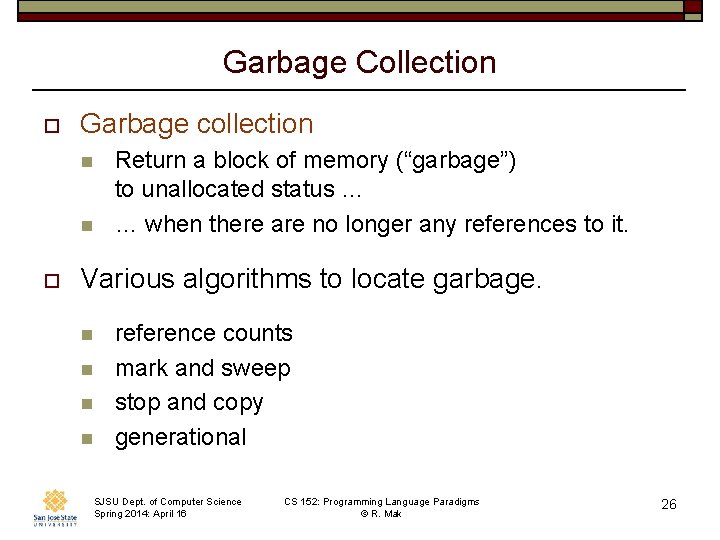
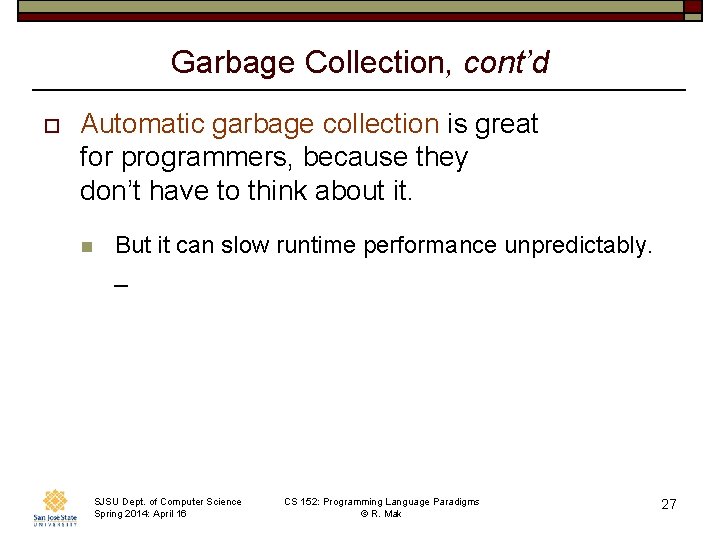
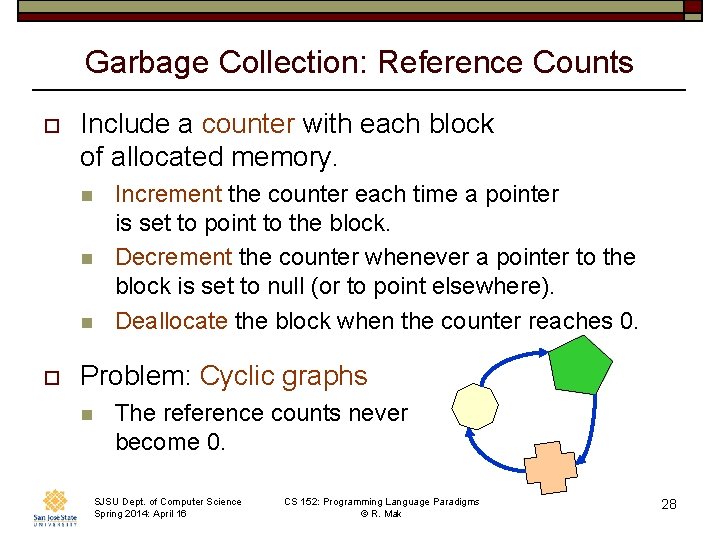
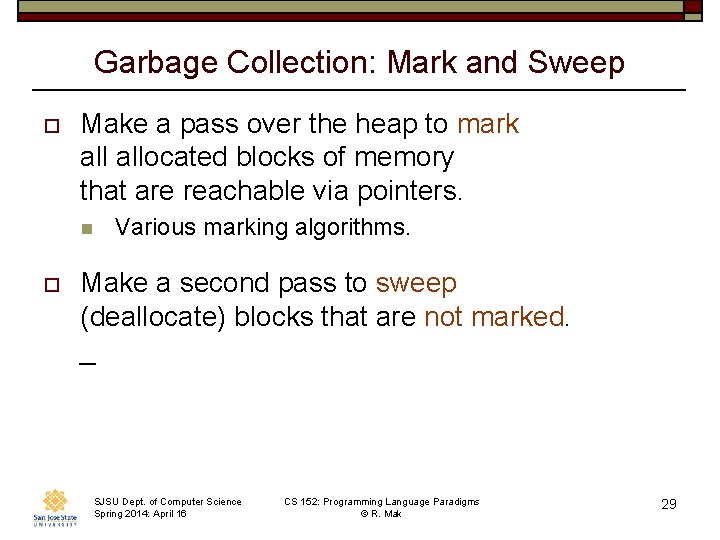
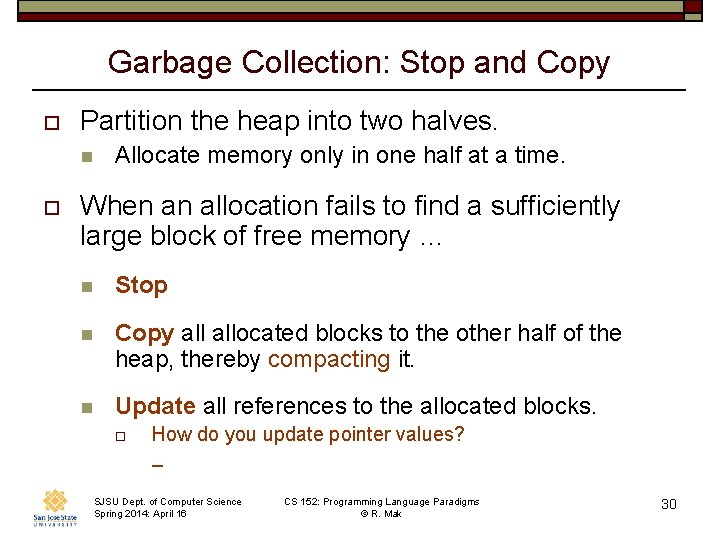
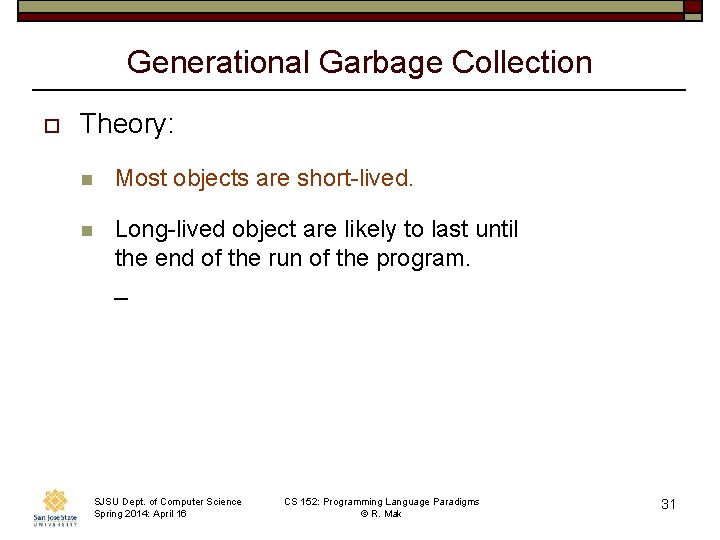
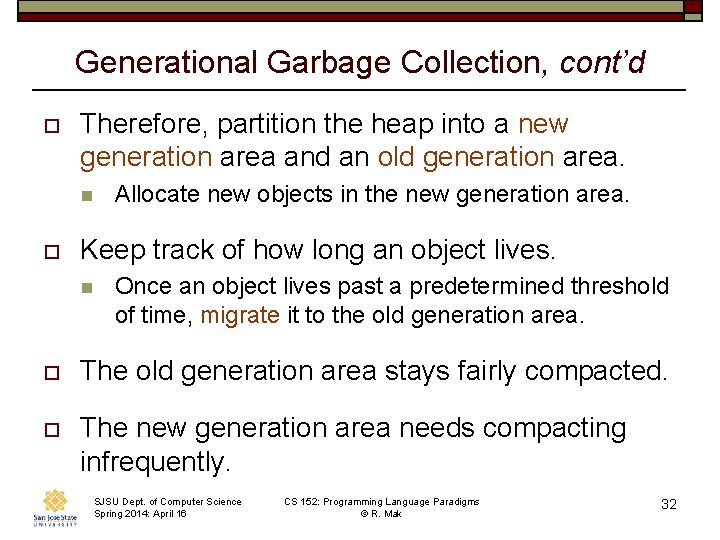
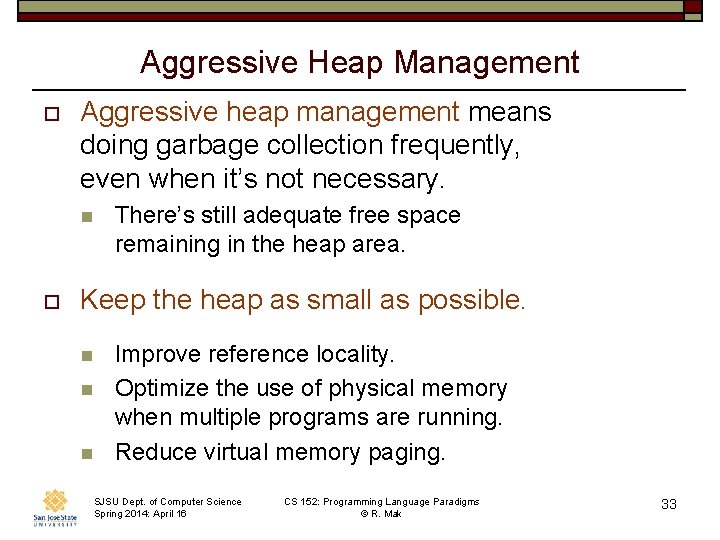
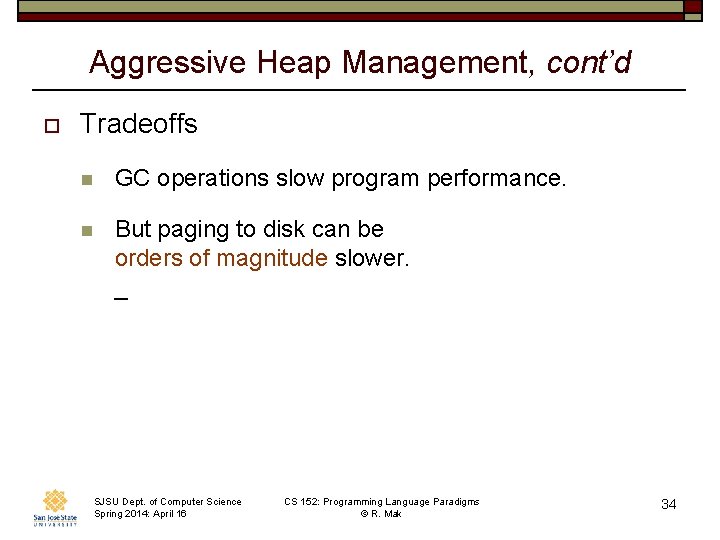
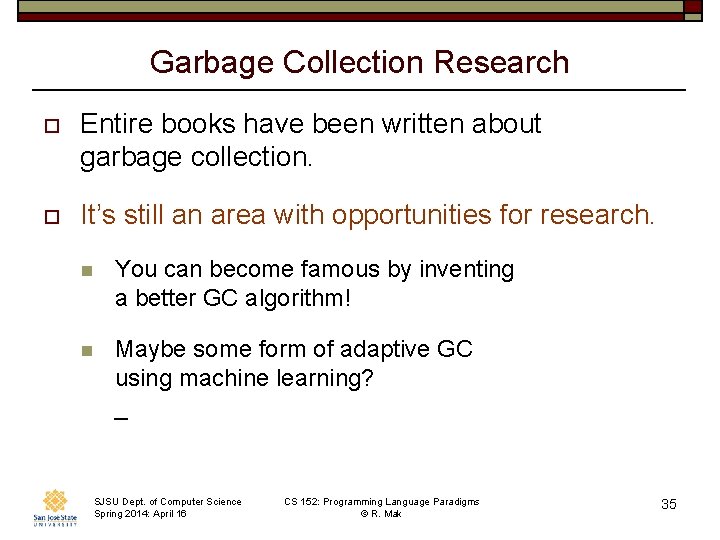
- Slides: 35
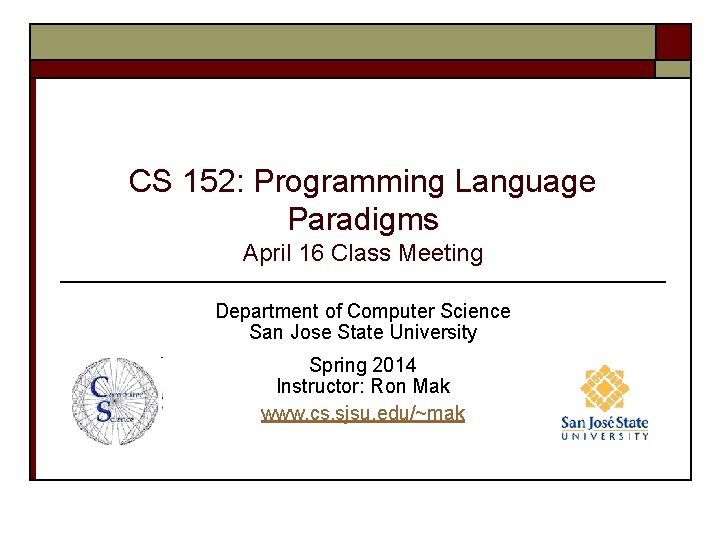
CS 152: Programming Language Paradigms April 16 Class Meeting Department of Computer Science San Jose State University Spring 2014 Instructor: Ron Mak www. cs. sjsu. edu/~mak
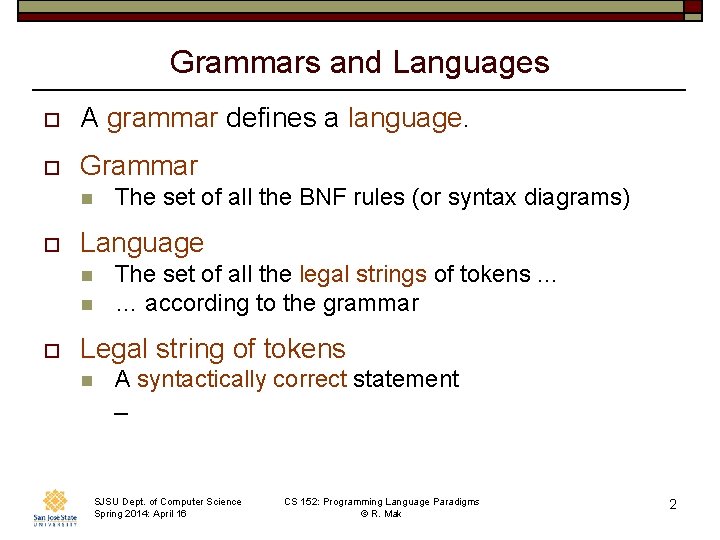
Grammars and Languages o A grammar defines a language. o Grammar n o Language n n o The set of all the BNF rules (or syntax diagrams) The set of all the legal strings of tokens. . . … according to the grammar Legal string of tokens n A syntactically correct statement _ SJSU Dept. of Computer Science Spring 2014: April 16 CS 152: Programming Language Paradigms © R. Mak 2
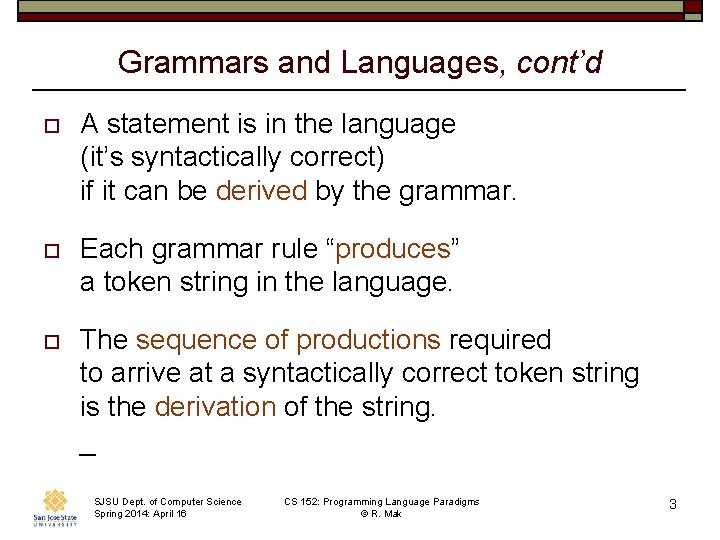
Grammars and Languages, cont’d o A statement is in the language (it’s syntactically correct) if it can be derived by the grammar. o Each grammar rule “produces” a token string in the language. o The sequence of productions required to arrive at a syntactically correct token string is the derivation of the string. _ SJSU Dept. of Computer Science Spring 2014: April 16 CS 152: Programming Language Paradigms © R. Mak 3
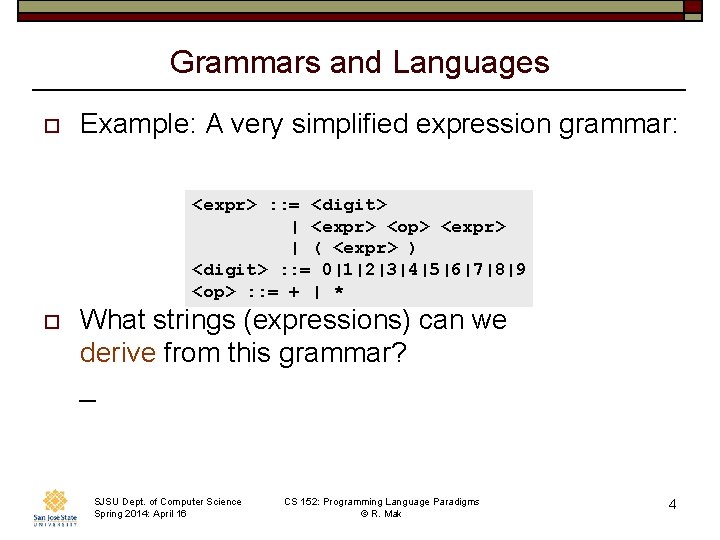
Grammars and Languages o Example: A very simplified expression grammar: <expr> : : = <digit> | <expr> <op> <expr> | ( <expr> ) <digit> : : = 0|1|2|3|4|5|6|7|8|9 <op> : : = + | * o What strings (expressions) can we derive from this grammar? _ SJSU Dept. of Computer Science Spring 2014: April 16 CS 152: Programming Language Paradigms © R. Mak 4
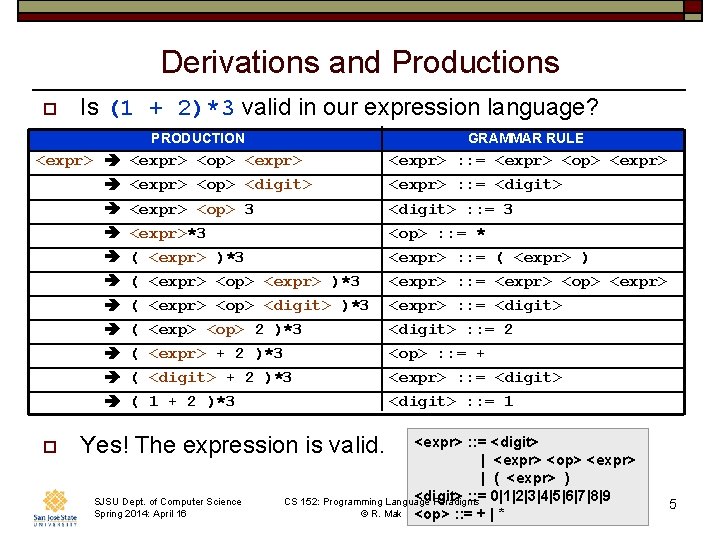
Derivations and Productions o Is (1 + 2)*3 valid in our expression language? PRODUCTION GRAMMAR RULE <expr> <op> <digit> o <expr> : : = <expr> <op> <expr> : : = <digit> <expr> <op> 3 <digit> : : = 3 <expr>*3 <op> : : = * ( <expr> )*3 <expr> : : = ( <expr> ) ( <expr> <op> <expr> )*3 <expr> : : = <expr> <op> <expr> ( <expr> <op> <digit> )*3 <expr> : : = <digit> ( <exp> <op> 2 )*3 ( <expr> + 2 )*3 <digit> : : = 2 <op> : : = + ( <digit> + 2 )*3 <expr> : : = <digit> ( 1 + 2 )*3 <digit> : : = 1 Yes! The expression is valid. SJSU Dept. of Computer Science Spring 2014: April 16 <expr> : : = <digit> | <expr> <op> <expr> | ( <expr> ) <digit> : : = 0|1|2|3|4|5|6|7|8|9 CS 152: Programming Language Paradigms © R. Mak <op> : : = + | * 5
![Extended BNF EBNF o Extended BNF EBNF adds metasymbols and Extended BNF (EBNF) o Extended BNF (EBNF) adds meta-symbols { } and [ ]](https://slidetodoc.com/presentation_image_h2/00cd62c62acfc4fb3887cd754bc90fcc/image-6.jpg)
Extended BNF (EBNF) o Extended BNF (EBNF) adds meta-symbols { } and [ ] { } [ ] ( )? Surround items to be repeated zero or more times. Surround optional items. SJSU Dept. of Computer Science Spring 2014: April 16 CS 152: Programming Language Paradigms © R. Mak 6
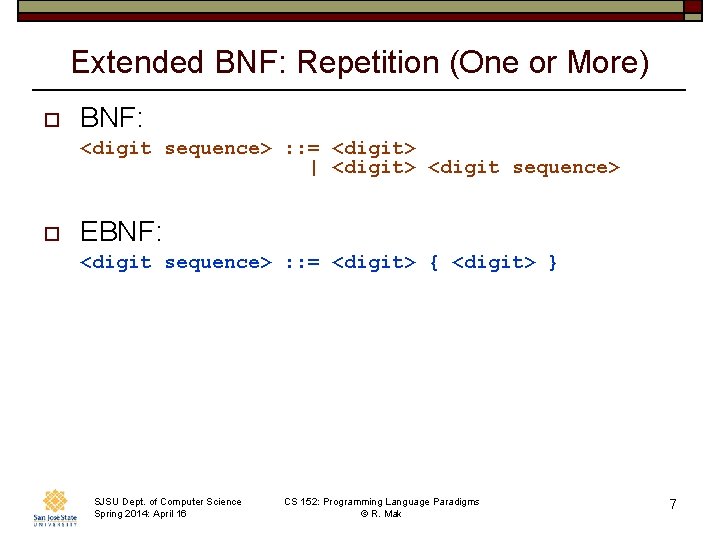
Extended BNF: Repetition (One or More) o BNF: <digit sequence> : : = <digit> | <digit> <digit sequence> o EBNF: <digit sequence> : : = <digit> { <digit> } SJSU Dept. of Computer Science Spring 2014: April 16 CS 152: Programming Language Paradigms © R. Mak 7
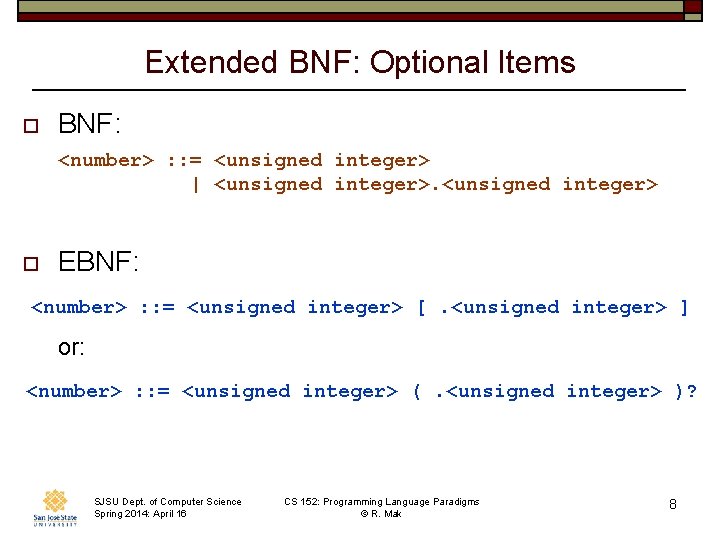
Extended BNF: Optional Items o BNF: <number> : : = <unsigned integer> | <unsigned integer> o EBNF: <number> : : = <unsigned integer> [. <unsigned integer> ] or: <number> : : = <unsigned integer> (. <unsigned integer> )? SJSU Dept. of Computer Science Spring 2014: April 16 CS 152: Programming Language Paradigms © R. Mak 8
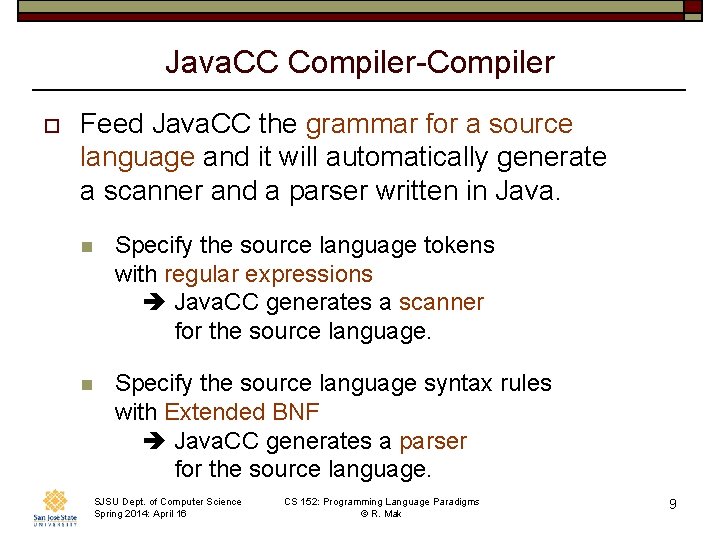
Java. CC Compiler-Compiler o Feed Java. CC the grammar for a source language and it will automatically generate a scanner and a parser written in Java. n Specify the source language tokens with regular expressions Java. CC generates a scanner for the source language. n Specify the source language syntax rules with Extended BNF Java. CC generates a parser for the source language. SJSU Dept. of Computer Science Spring 2014: April 16 CS 152: Programming Language Paradigms © R. Mak 9
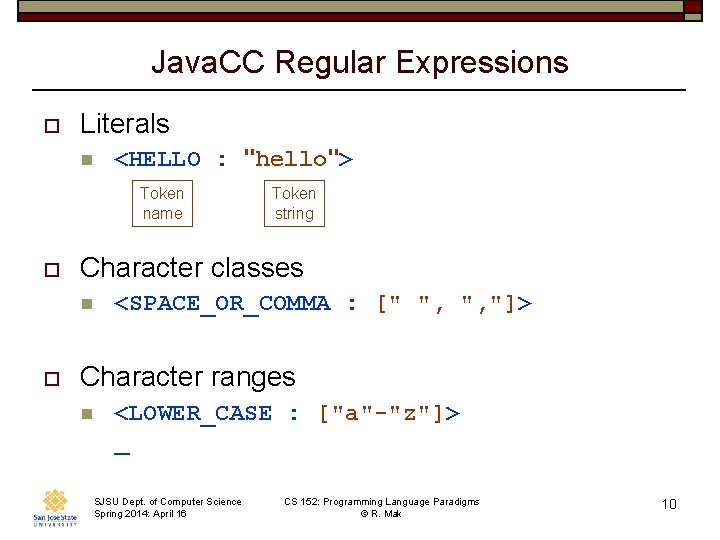
Java. CC Regular Expressions o Literals n <HELLO : "hello"> Token name o Character classes n o Token string <SPACE_OR_COMMA : [" ", ", "]> Character ranges n <LOWER_CASE : ["a"-"z"]> _ SJSU Dept. of Computer Science Spring 2014: April 16 CS 152: Programming Language Paradigms © R. Mak 10
![Java CC Regular Expressions contd o Alternates UPPERORLOWER AZ az n o Java. CC Regular Expressions, cont’d o Alternates <UPPER_OR_LOWER : ["A"-"Z"] | ["a"-"z"]> n o](https://slidetodoc.com/presentation_image_h2/00cd62c62acfc4fb3887cd754bc90fcc/image-11.jpg)
Java. CC Regular Expressions, cont’d o Alternates <UPPER_OR_LOWER : ["A"-"Z"] | ["a"-"z"]> n o Negation n o <NO_DIGITS : ~["0"-"9"]> Repetition n n <THREE_A : ("a"){3}> <TWO_TO_FOUR_A : ("a"){2, 4}> _ SJSU Dept. of Computer Science Spring 2014: April 16 CS 152: Programming Language Paradigms © R. Mak 11
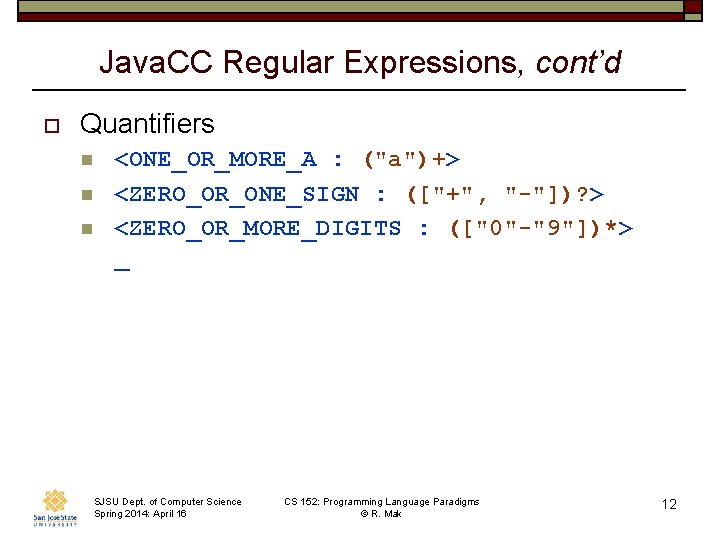
Java. CC Regular Expressions, cont’d o Quantifiers n n n <ONE_OR_MORE_A : ("a")+> <ZERO_OR_ONE_SIGN : (["+", "-"])? > <ZERO_OR_MORE_DIGITS : (["0"-"9"])*> _ SJSU Dept. of Computer Science Spring 2014: April 16 CS 152: Programming Language Paradigms © R. Mak 12
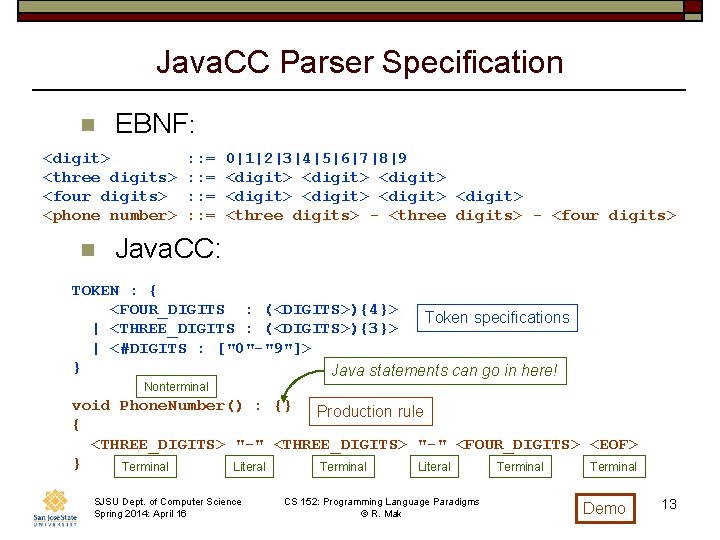
Java. CC Parser Specification n EBNF: <digit> <three digits> <four digits> <phone number> n : : = 0|1|2|3|4|5|6|7|8|9 <digit> <digit> <three digits> - <four digits> Java. CC: TOKEN : { <FOUR_DIGITS : (<DIGITS>){4}> Token specifications | <THREE_DIGITS : (<DIGITS>){3}> | <#DIGITS : ["0"-"9"]> } Java statements can go in here! Nonterminal void Phone. Number() : {} Production rule { <THREE_DIGITS> "-" <FOUR_DIGITS> <EOF> } Terminal Literal Terminal SJSU Dept. of Computer Science Spring 2014: April 16 CS 152: Programming Language Paradigms © R. Mak Demo 13
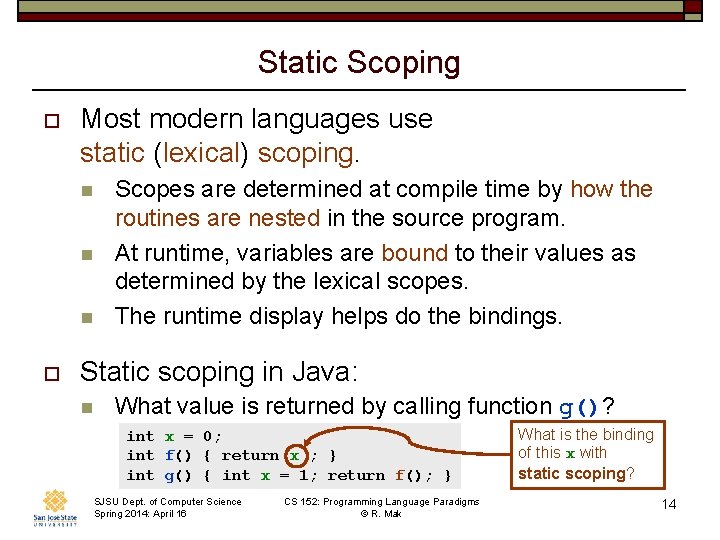
Static Scoping o Most modern languages use static (lexical) scoping. n n n o Scopes are determined at compile time by how the routines are nested in the source program. At runtime, variables are bound to their values as determined by the lexical scopes. The runtime display helps do the bindings. Static scoping in Java: n What value is returned by calling function g()? int x = 0; int f() { return x ; } int g() { int x = 1; return f(); } SJSU Dept. of Computer Science Spring 2014: April 16 CS 152: Programming Language Paradigms © R. Mak What is the binding of this x with static scoping? 14
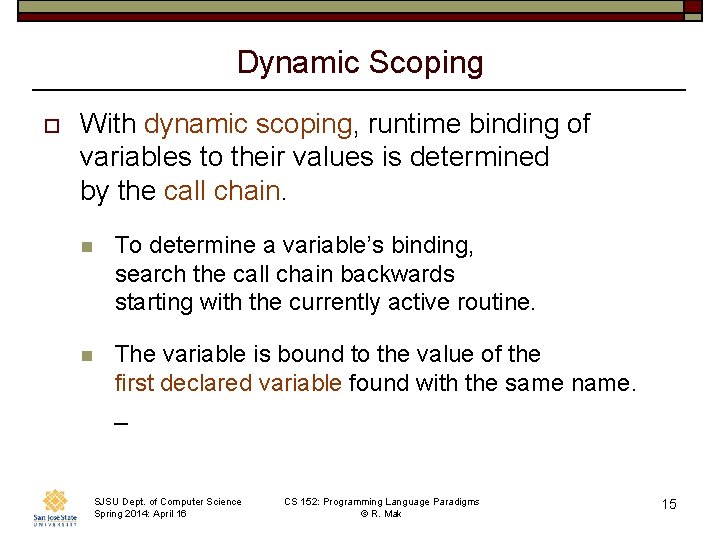
Dynamic Scoping o With dynamic scoping, runtime binding of variables to their values is determined by the call chain. n To determine a variable’s binding, search the call chain backwards starting with the currently active routine. n The variable is bound to the value of the first declared variable found with the same name. _ SJSU Dept. of Computer Science Spring 2014: April 16 CS 152: Programming Language Paradigms © R. Mak 15
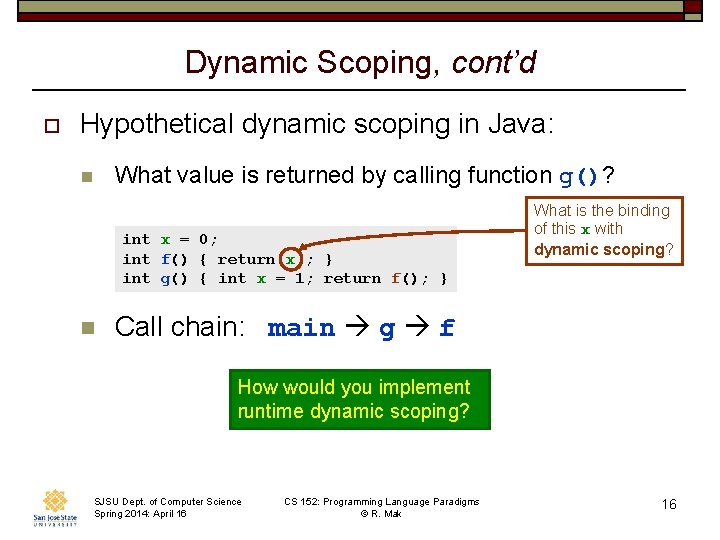
Dynamic Scoping, cont’d o Hypothetical dynamic scoping in Java: n What value is returned by calling function g()? int x = 0; int f() { return x ; } int g() { int x = 1; return f(); } n What is the binding of this x with dynamic scoping? Call chain: main g f How would you implement runtime dynamic scoping? SJSU Dept. of Computer Science Spring 2014: April 16 CS 152: Programming Language Paradigms © R. Mak 16
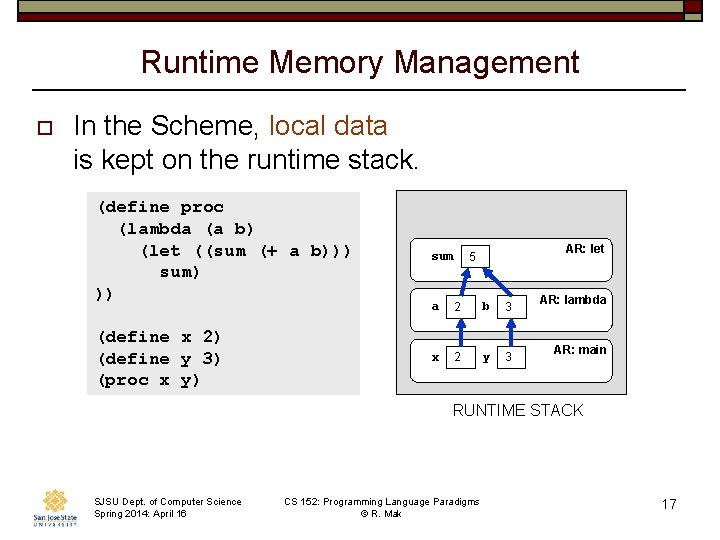
Runtime Memory Management o In the Scheme, local data is kept on the runtime stack. (define proc (lambda (a b) (let ((sum (+ a b))) sum) )) sum a 2 b 3 (define x 2) (define y 3) (proc x y) x 2 y 3 AR: let 5 AR: lambda AR: main RUNTIME STACK SJSU Dept. of Computer Science Spring 2014: April 16 CS 152: Programming Language Paradigms © R. Mak 17
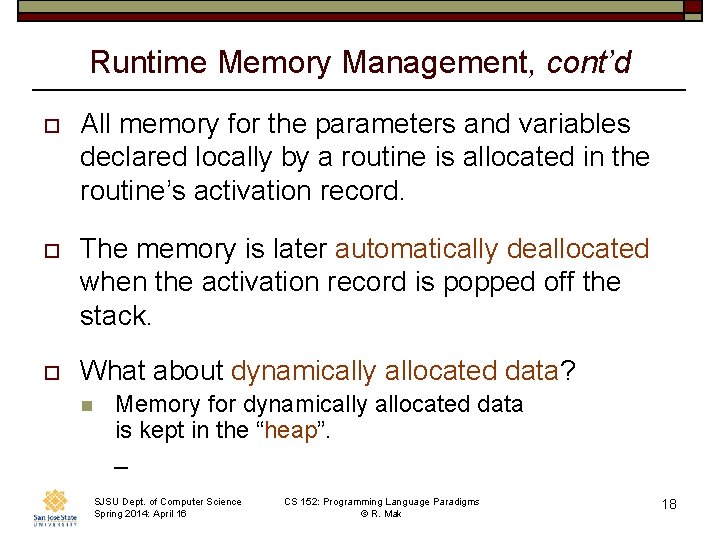
Runtime Memory Management, cont’d o All memory for the parameters and variables declared locally by a routine is allocated in the routine’s activation record. o The memory is later automatically deallocated when the activation record is popped off the stack. o What about dynamically allocated data? n Memory for dynamically allocated data is kept in the “heap”. _ SJSU Dept. of Computer Science Spring 2014: April 16 CS 152: Programming Language Paradigms © R. Mak 18
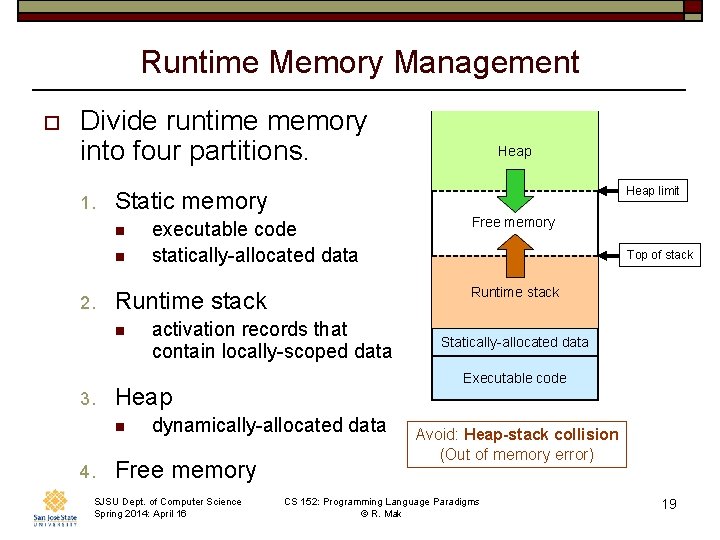
Runtime Memory Management o Divide runtime memory into four partitions. 1. n activation records that contain locally-scoped data SJSU Dept. of Computer Science Spring 2014: April 16 Top of stack Statically-allocated data Executable code dynamically-allocated data Free memory Runtime stack Heap n 4. executable code statically-allocated data Runtime stack n 3. Heap limit Static memory n 2. Heap Avoid: Heap-stack collision (Out of memory error) CS 152: Programming Language Paradigms © R. Mak 19
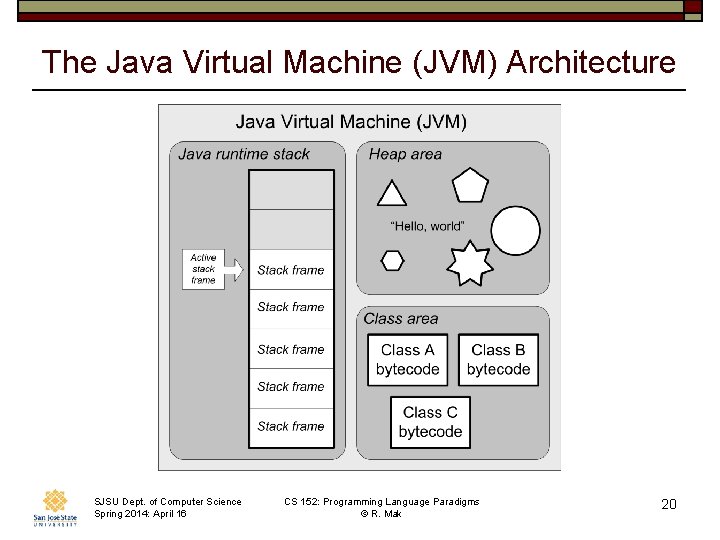
The Java Virtual Machine (JVM) Architecture SJSU Dept. of Computer Science Spring 2014: April 16 CS 152: Programming Language Paradigms © R. Mak 20
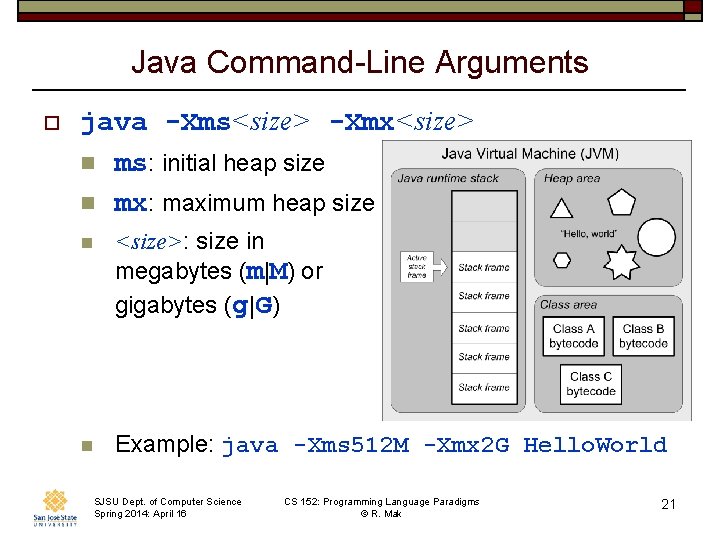
Java Command-Line Arguments o java -Xms<size> -Xmx<size> n ms: initial heap size n mx: maximum heap size n <size>: size in megabytes (m|M) or gigabytes (g|G) n Example: java -Xms 512 M -Xmx 2 G Hello. World SJSU Dept. of Computer Science Spring 2014: April 16 CS 152: Programming Language Paradigms © R. Mak 21
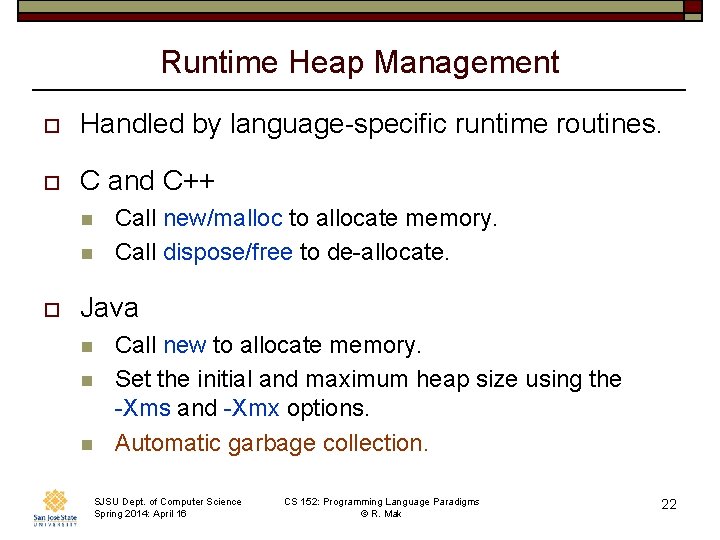
Runtime Heap Management o Handled by language-specific runtime routines. o C and C++ n n o Call new/malloc to allocate memory. Call dispose/free to de-allocate. Java n n n Call new to allocate memory. Set the initial and maximum heap size using the -Xms and -Xmx options. Automatic garbage collection. SJSU Dept. of Computer Science Spring 2014: April 16 CS 152: Programming Language Paradigms © R. Mak 22
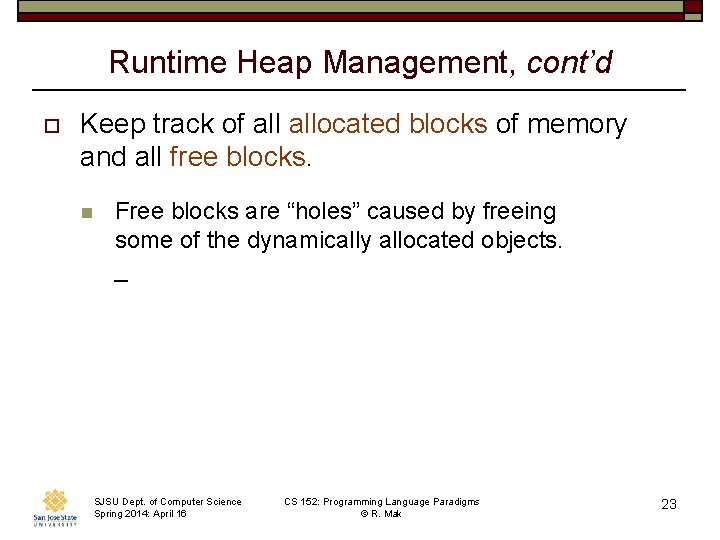
Runtime Heap Management, cont’d o Keep track of allocated blocks of memory and all free blocks. n Free blocks are “holes” caused by freeing some of the dynamically allocated objects. _ SJSU Dept. of Computer Science Spring 2014: April 16 CS 152: Programming Language Paradigms © R. Mak 23
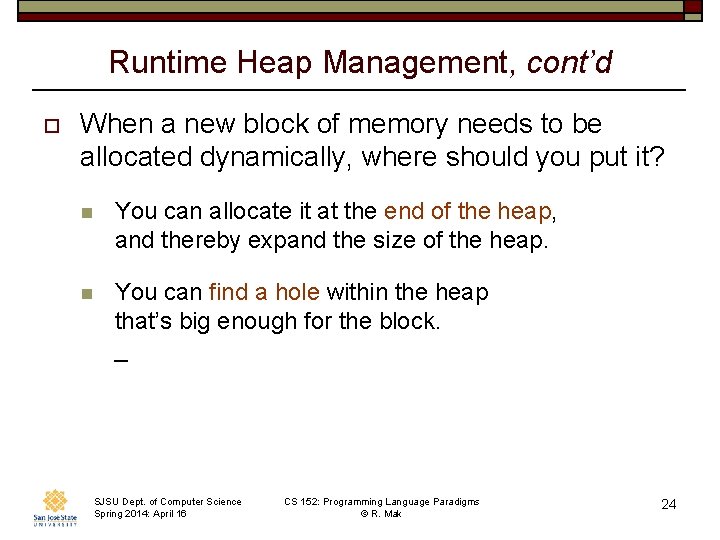
Runtime Heap Management, cont’d o When a new block of memory needs to be allocated dynamically, where should you put it? n You can allocate it at the end of the heap, and thereby expand the size of the heap. n You can find a hole within the heap that’s big enough for the block. _ SJSU Dept. of Computer Science Spring 2014: April 16 CS 152: Programming Language Paradigms © R. Mak 24
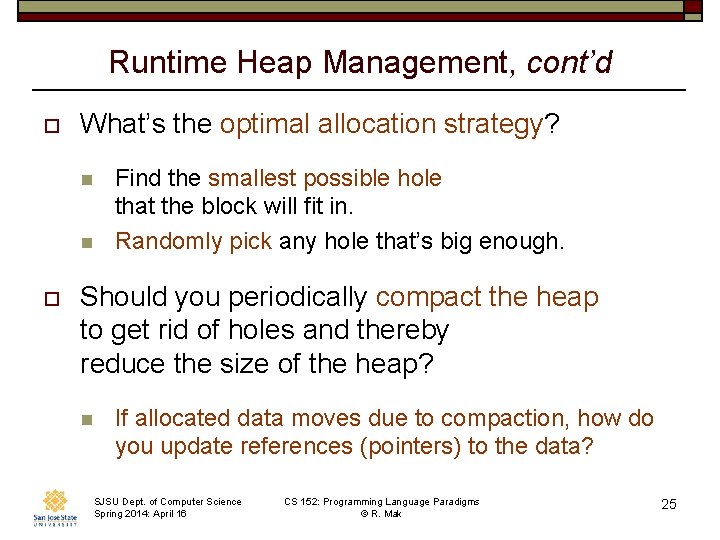
Runtime Heap Management, cont’d o What’s the optimal allocation strategy? n n o Find the smallest possible hole that the block will fit in. Randomly pick any hole that’s big enough. Should you periodically compact the heap to get rid of holes and thereby reduce the size of the heap? n If allocated data moves due to compaction, how do you update references (pointers) to the data? SJSU Dept. of Computer Science Spring 2014: April 16 CS 152: Programming Language Paradigms © R. Mak 25
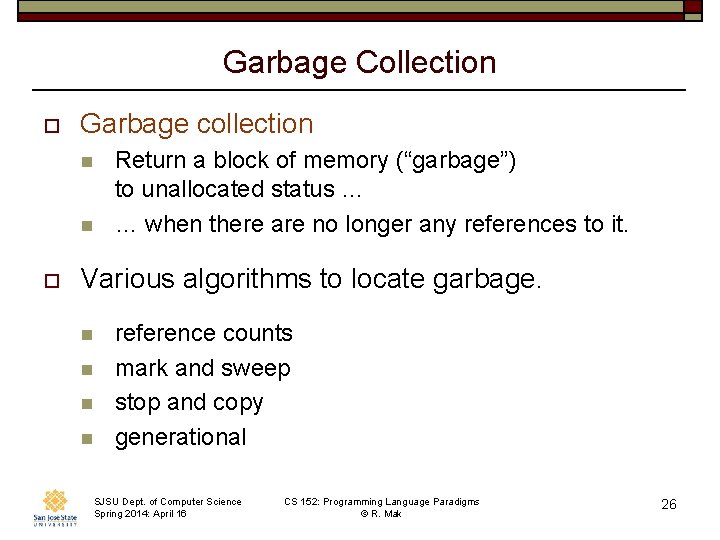
Garbage Collection o Garbage collection n n o Return a block of memory (“garbage”) to unallocated status … … when there are no longer any references to it. Various algorithms to locate garbage. n n reference counts mark and sweep stop and copy generational SJSU Dept. of Computer Science Spring 2014: April 16 CS 152: Programming Language Paradigms © R. Mak 26
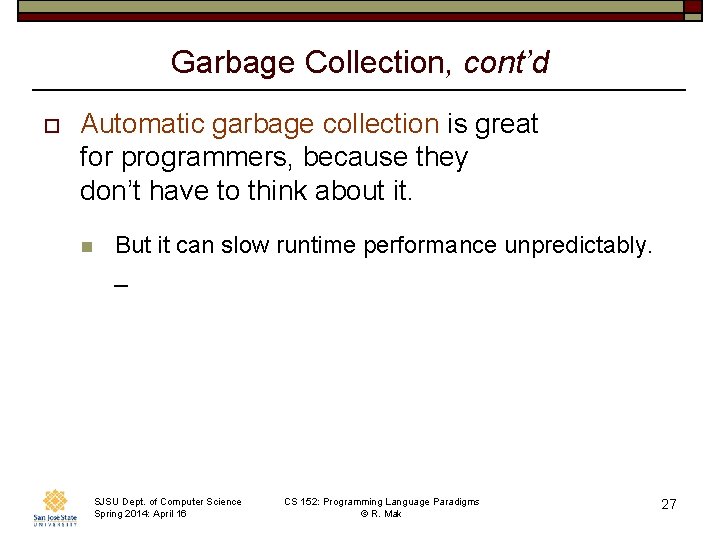
Garbage Collection, cont’d o Automatic garbage collection is great for programmers, because they don’t have to think about it. n But it can slow runtime performance unpredictably. _ SJSU Dept. of Computer Science Spring 2014: April 16 CS 152: Programming Language Paradigms © R. Mak 27
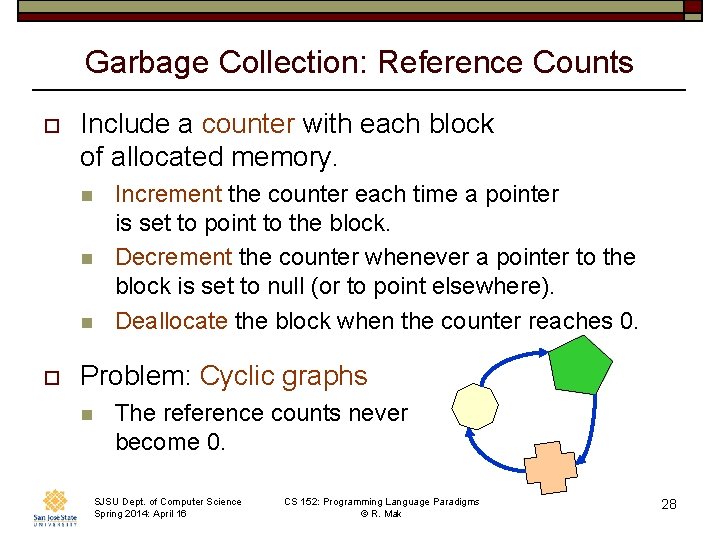
Garbage Collection: Reference Counts o Include a counter with each block of allocated memory. n n n o Increment the counter each time a pointer is set to point to the block. Decrement the counter whenever a pointer to the block is set to null (or to point elsewhere). Deallocate the block when the counter reaches 0. Problem: Cyclic graphs n The reference counts never become 0. SJSU Dept. of Computer Science Spring 2014: April 16 CS 152: Programming Language Paradigms © R. Mak 28
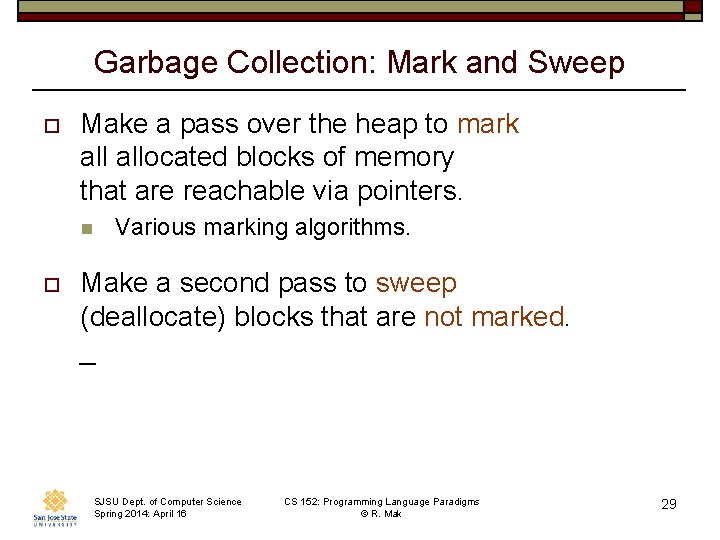
Garbage Collection: Mark and Sweep o Make a pass over the heap to mark allocated blocks of memory that are reachable via pointers. n o Various marking algorithms. Make a second pass to sweep (deallocate) blocks that are not marked. _ SJSU Dept. of Computer Science Spring 2014: April 16 CS 152: Programming Language Paradigms © R. Mak 29
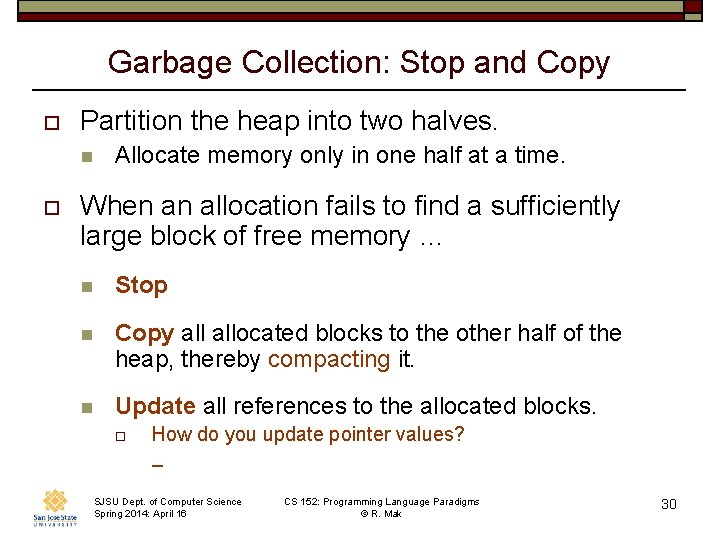
Garbage Collection: Stop and Copy o Partition the heap into two halves. n o Allocate memory only in one half at a time. When an allocation fails to find a sufficiently large block of free memory … n Stop n Copy allocated blocks to the other half of the heap, thereby compacting it. n Update all references to the allocated blocks. o How do you update pointer values? _ SJSU Dept. of Computer Science Spring 2014: April 16 CS 152: Programming Language Paradigms © R. Mak 30
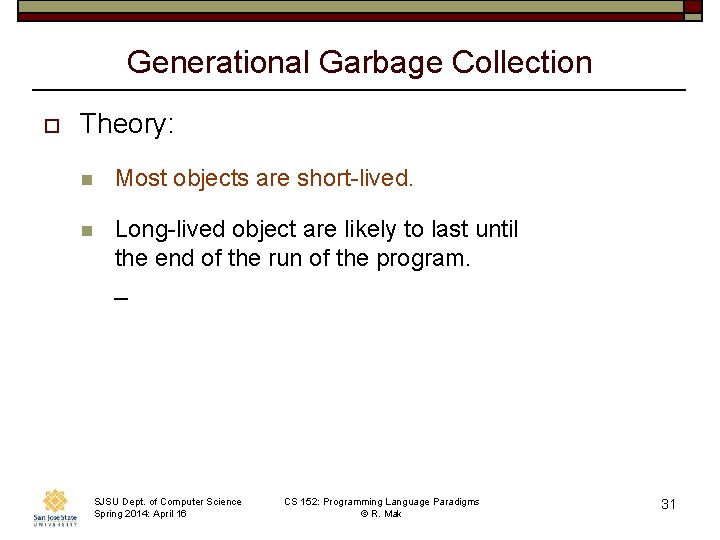
Generational Garbage Collection o Theory: n Most objects are short-lived. n Long-lived object are likely to last until the end of the run of the program. _ SJSU Dept. of Computer Science Spring 2014: April 16 CS 152: Programming Language Paradigms © R. Mak 31
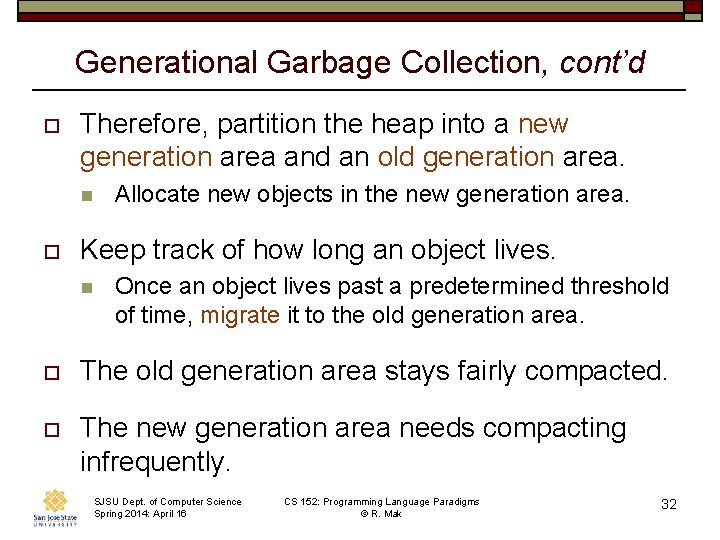
Generational Garbage Collection, cont’d o Therefore, partition the heap into a new generation area and an old generation area. n o Allocate new objects in the new generation area. Keep track of how long an object lives. n Once an object lives past a predetermined threshold of time, migrate it to the old generation area. o The old generation area stays fairly compacted. o The new generation area needs compacting infrequently. SJSU Dept. of Computer Science Spring 2014: April 16 CS 152: Programming Language Paradigms © R. Mak 32
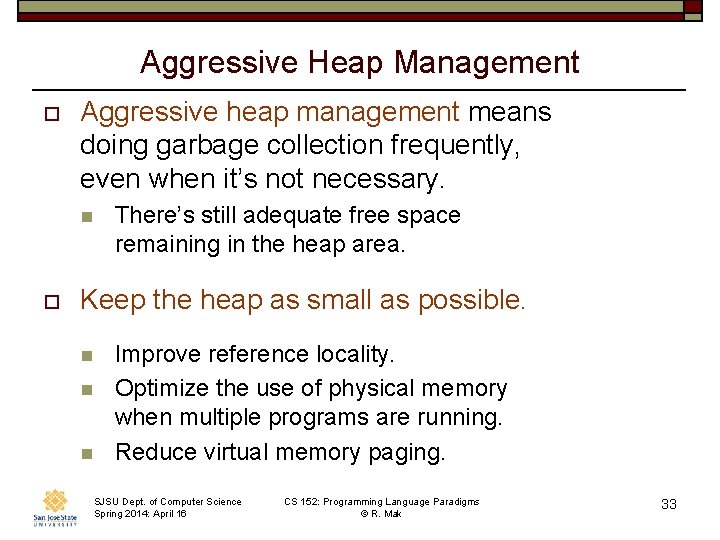
Aggressive Heap Management o Aggressive heap management means doing garbage collection frequently, even when it’s not necessary. n o There’s still adequate free space remaining in the heap area. Keep the heap as small as possible. n n n Improve reference locality. Optimize the use of physical memory when multiple programs are running. Reduce virtual memory paging. SJSU Dept. of Computer Science Spring 2014: April 16 CS 152: Programming Language Paradigms © R. Mak 33
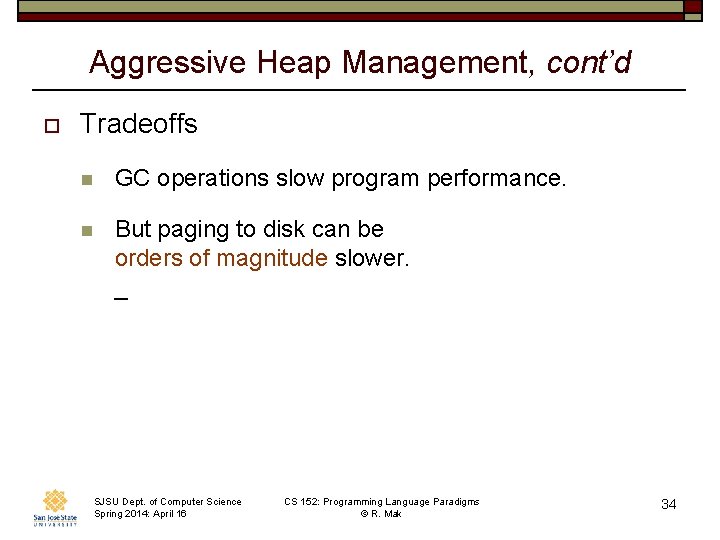
Aggressive Heap Management, cont’d o Tradeoffs n GC operations slow program performance. n But paging to disk can be orders of magnitude slower. _ SJSU Dept. of Computer Science Spring 2014: April 16 CS 152: Programming Language Paradigms © R. Mak 34
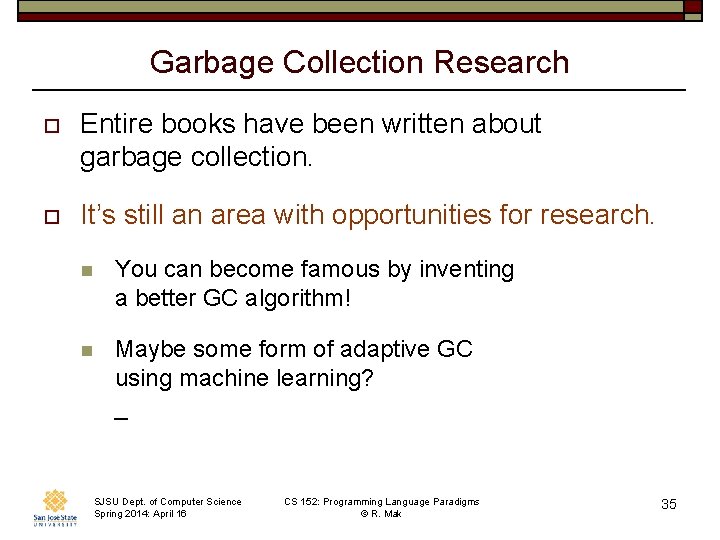
Garbage Collection Research o Entire books have been written about garbage collection. o It’s still an area with opportunities for research. n You can become famous by inventing a better GC algorithm! n Maybe some form of adaptive GC using machine learning? _ SJSU Dept. of Computer Science Spring 2014: April 16 CS 152: Programming Language Paradigms © R. Mak 35
R programming language paradigms
Binding in programming paradigms
Ktu programming paradigms notes
Vhdl hardware description language
Unr152
Ntuser.dat file
Przedszkole 152 łódź
Re liveri 2006 qca 152
Nearest ten thousand
Mae 152
Blending function in computer graphics
Cs 152 stanford
Cs 152 berkeley
Ba 152
Ece 152
Ba 152
Lorne priemaza
Which layer in the osi model covers http, ftp, and rdc?
Ba 152
Macroob
Ba 152
Plasa
Econ 152
Cs152 sjsu
Gfi 152
Peter hall policy paradigms
Careershodh
Paradigms and principles
Paradigmatic analysis example
Paradigms for interaction in hci
Distributed systems andrew s tanenbaum
Message ordering paradigms
Distributed systems principles and paradigms
Object space paradigm
3 paradigms
Interpretivism in consumer behaviour