CS 152 Programming Language Paradigms April 23 Class
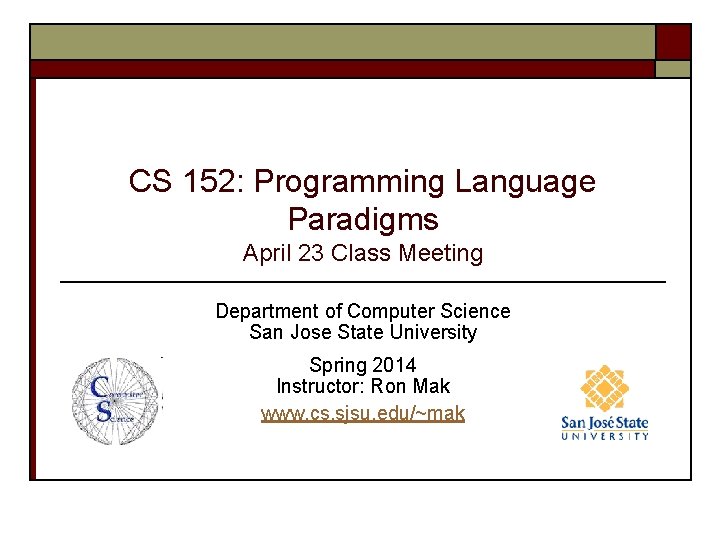
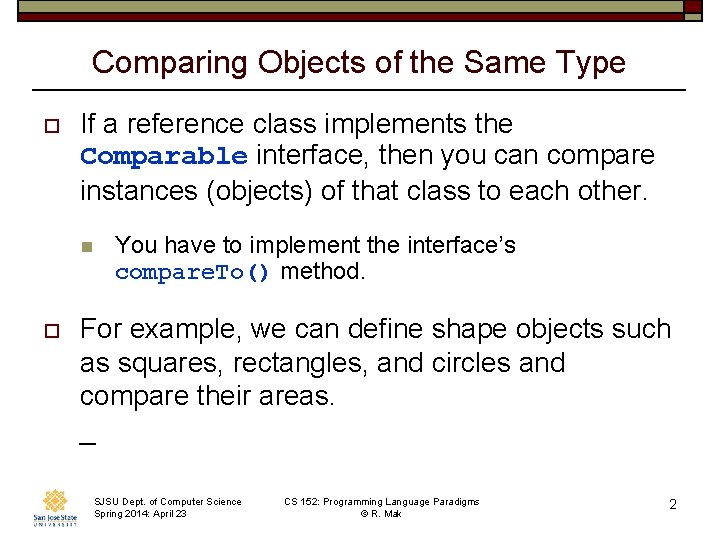
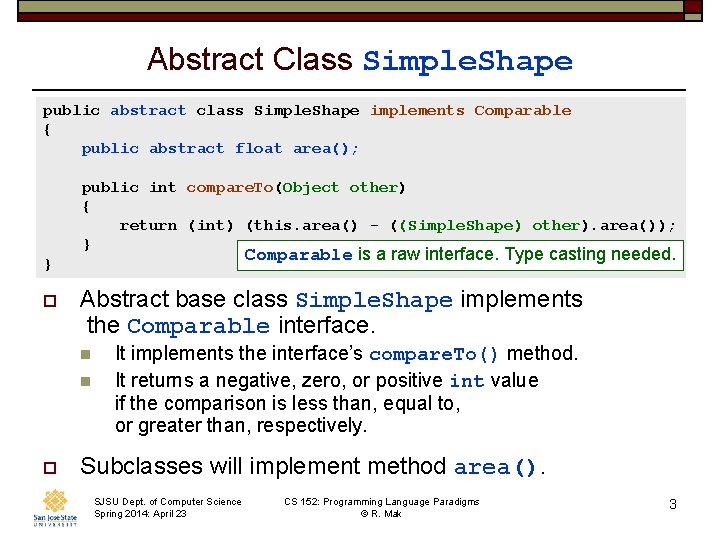
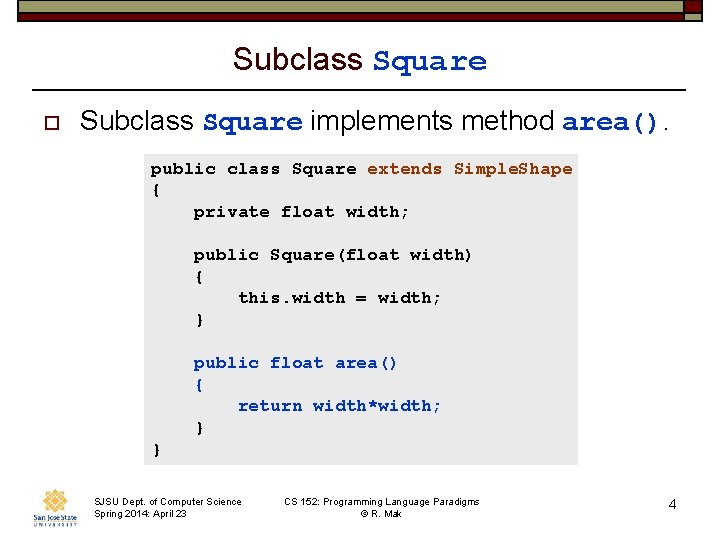
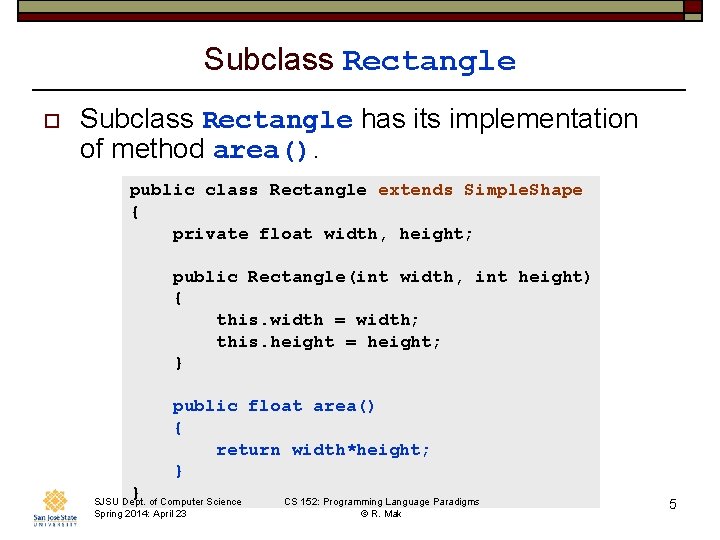
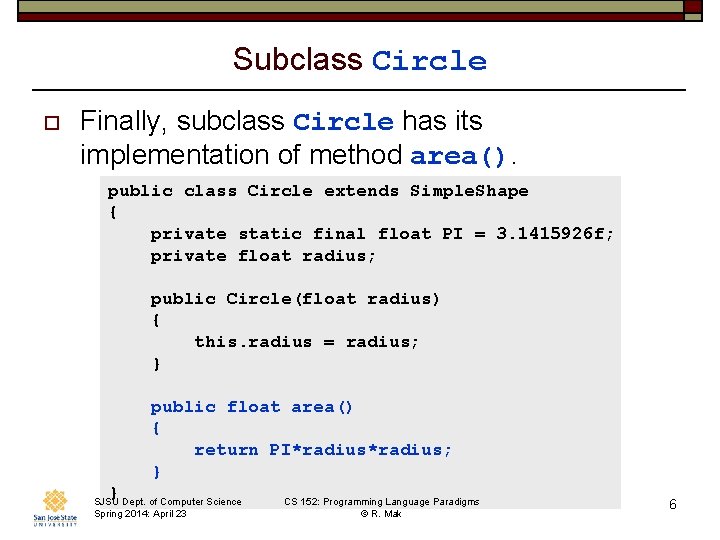
![Array 5: Simple. Shape Objects public static void main(String[] args) { Array. List<Simple. Shape> Array 5: Simple. Shape Objects public static void main(String[] args) { Array. List<Simple. Shape>](https://slidetodoc.com/presentation_image_h/274b00dbf0db0688ebcb176e9f165c63/image-7.jpg)
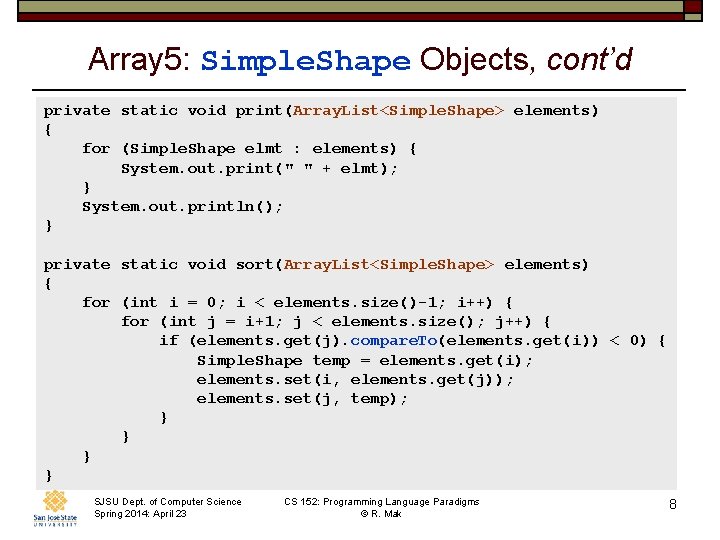
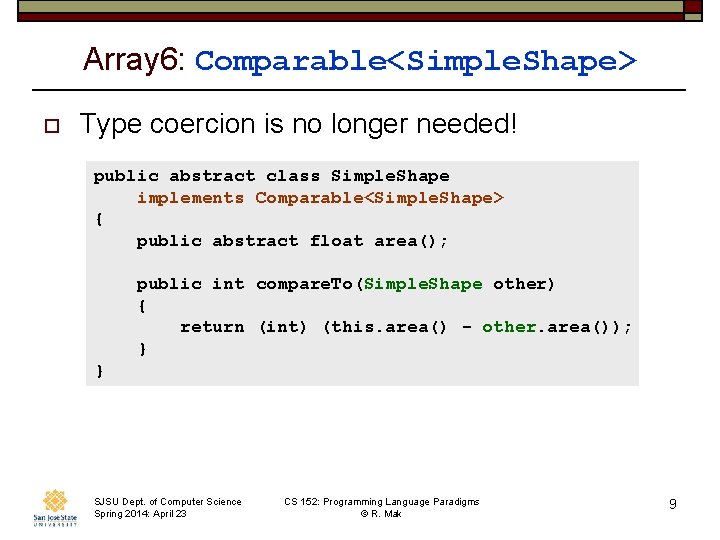
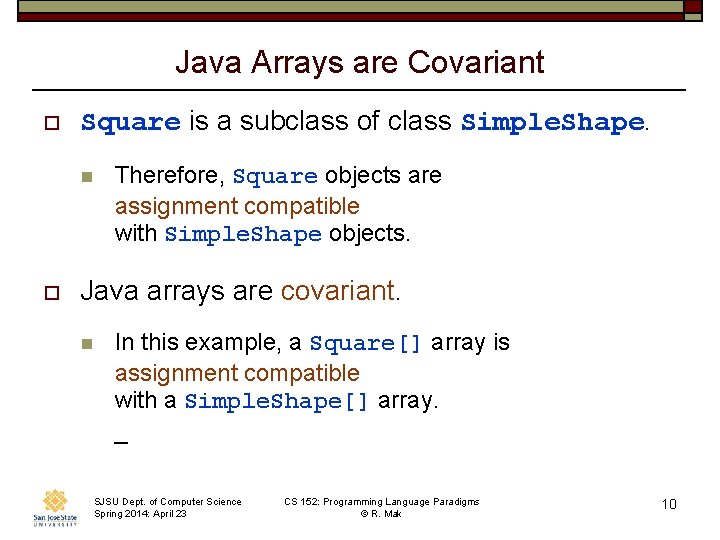
![Java Arrays are Covariant, cont’d private static float total. Area(Simple. Shape elements[]) { The Java Arrays are Covariant, cont’d private static float total. Area(Simple. Shape elements[]) { The](https://slidetodoc.com/presentation_image_h/274b00dbf0db0688ebcb176e9f165c63/image-11.jpg)
![Java Arrays are Covariant, cont’d public static void main(String[] args) { Simple. Shape shapes[] Java Arrays are Covariant, cont’d public static void main(String[] args) { Simple. Shape shapes[]](https://slidetodoc.com/presentation_image_h/274b00dbf0db0688ebcb176e9f165c63/image-12.jpg)
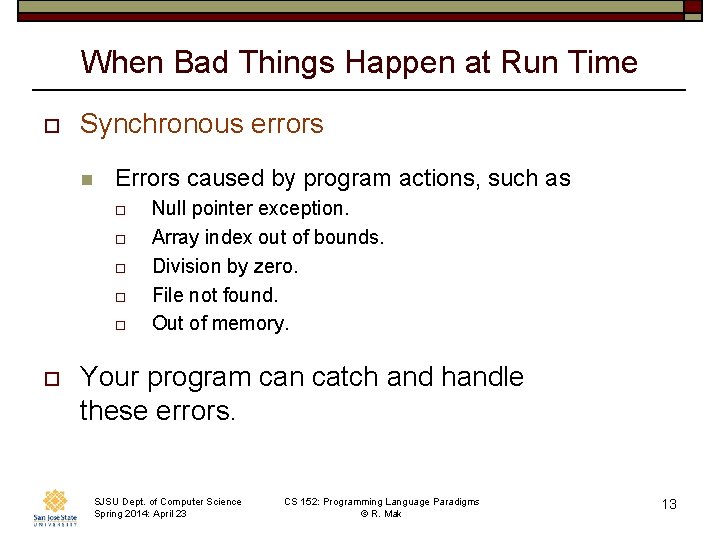
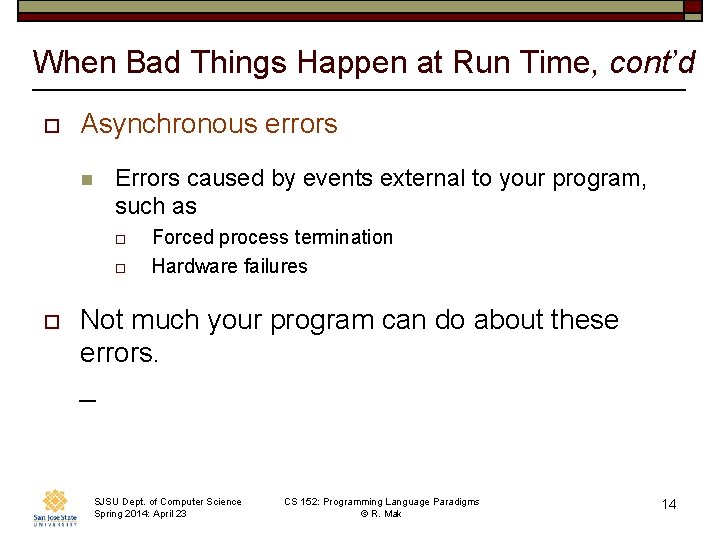
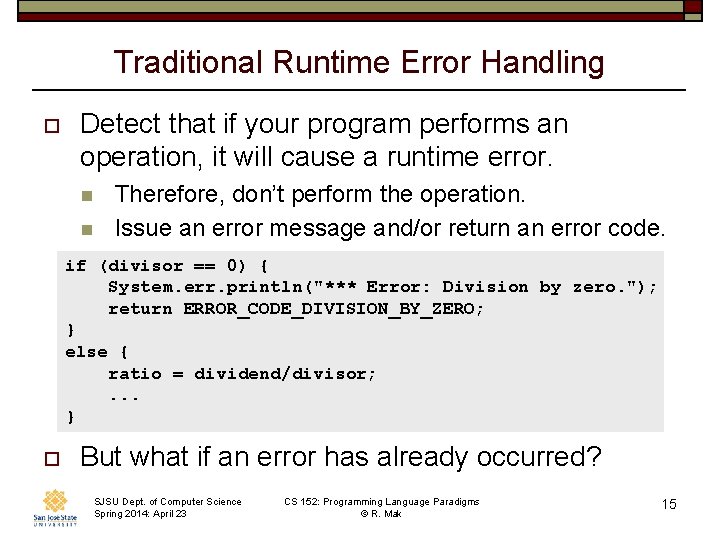
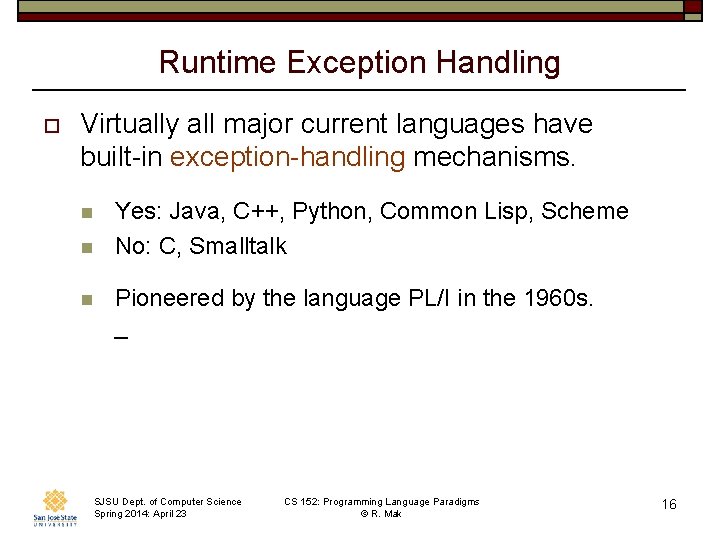
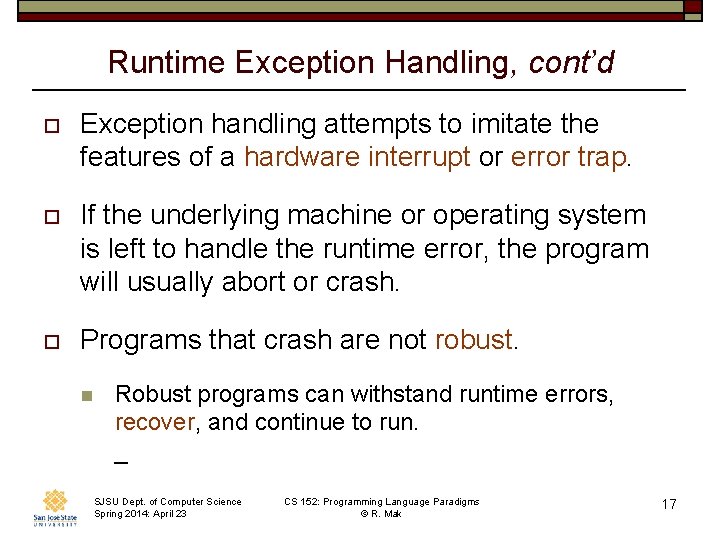
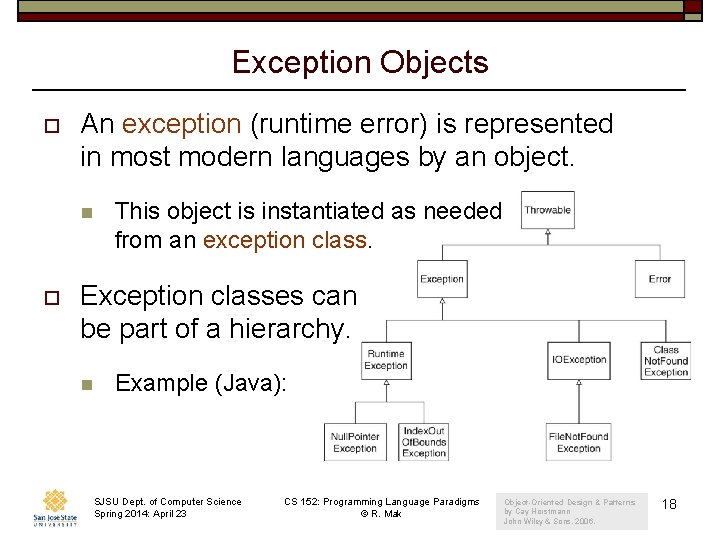
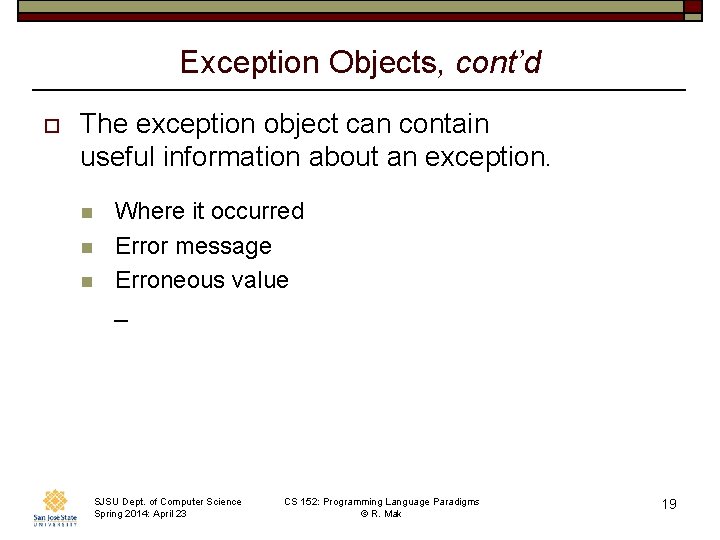
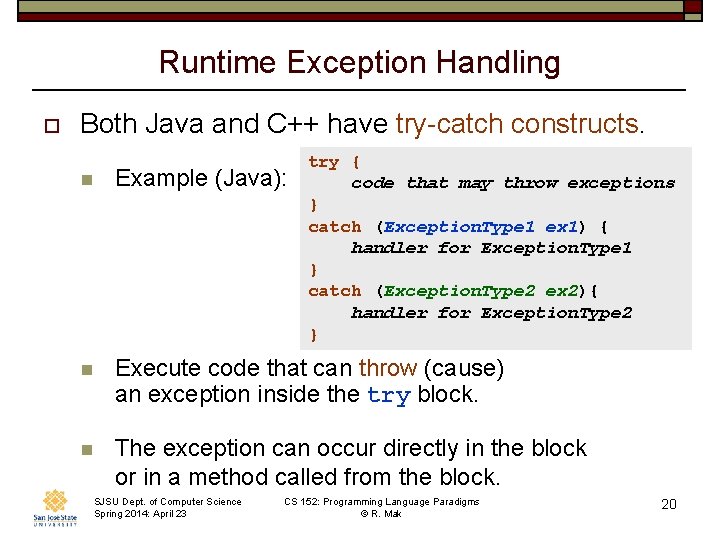
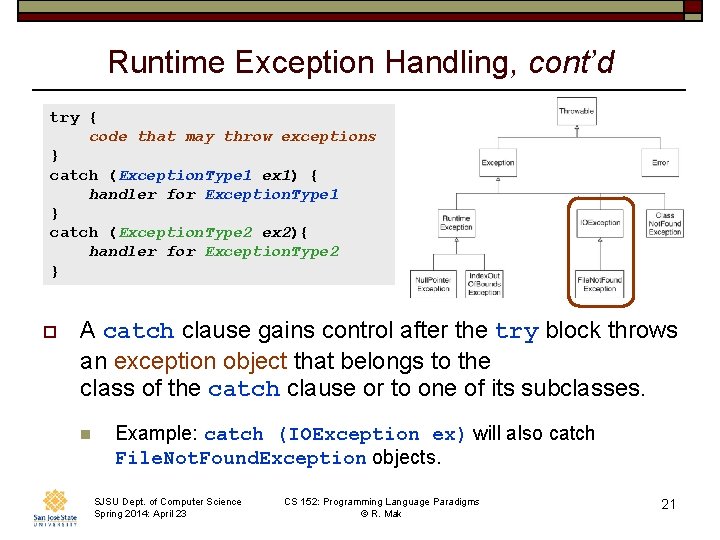
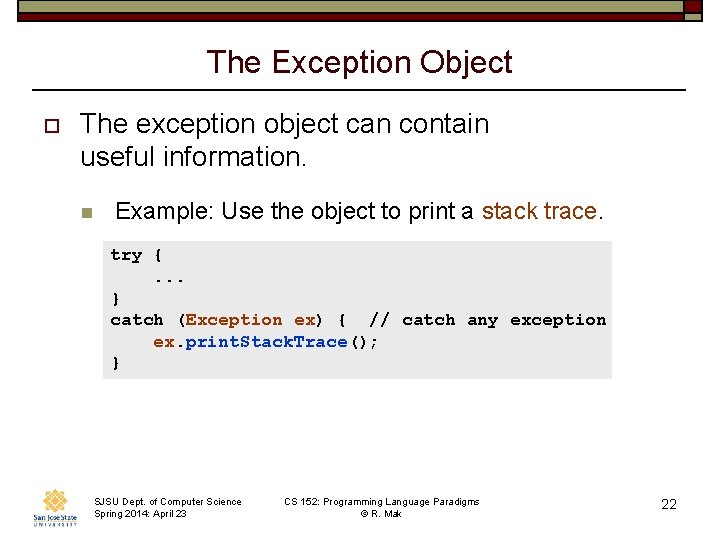
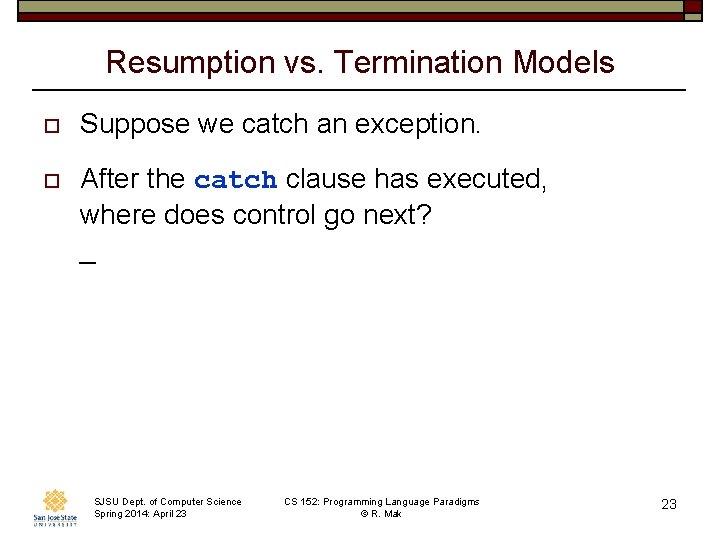
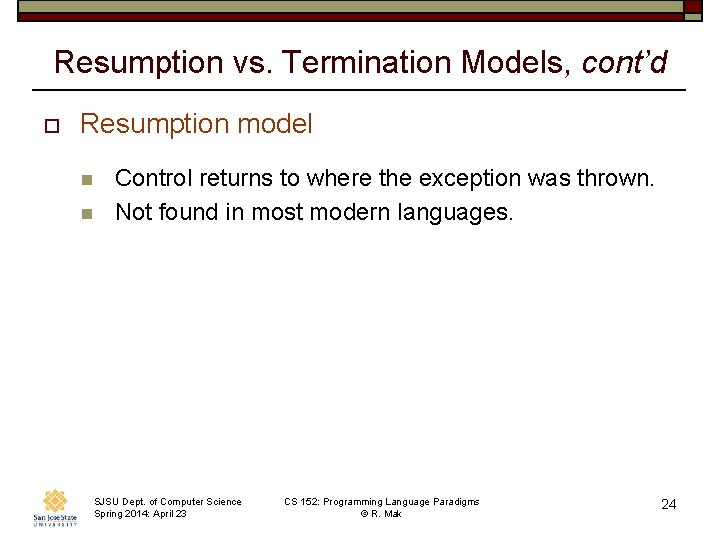
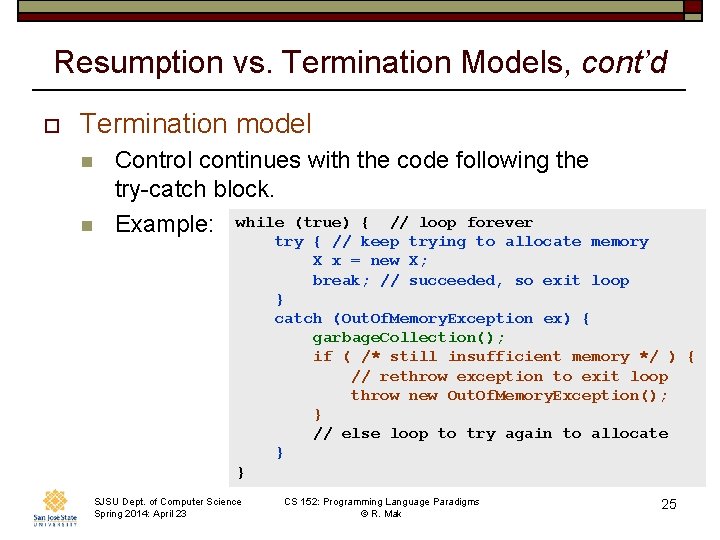
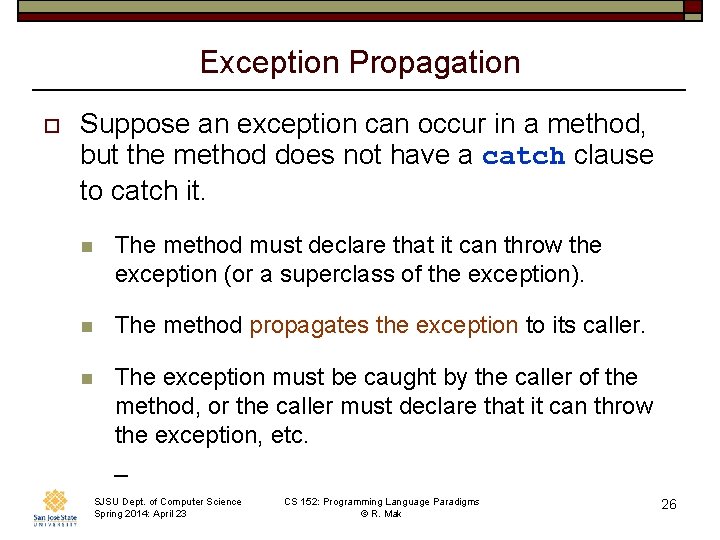
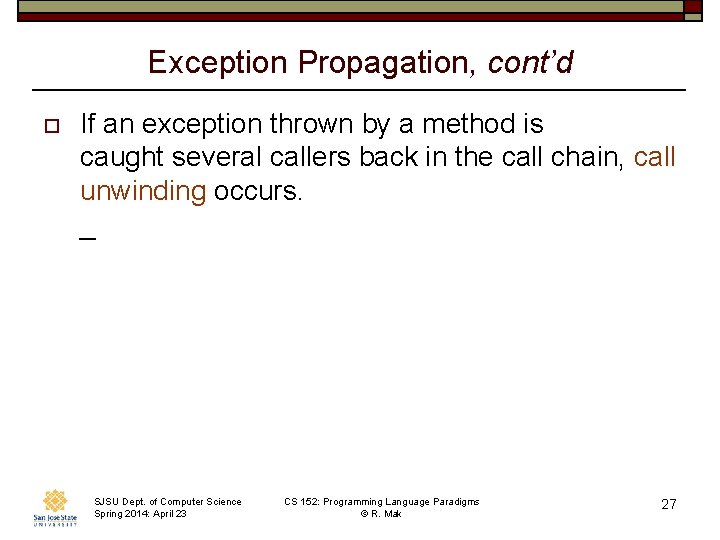
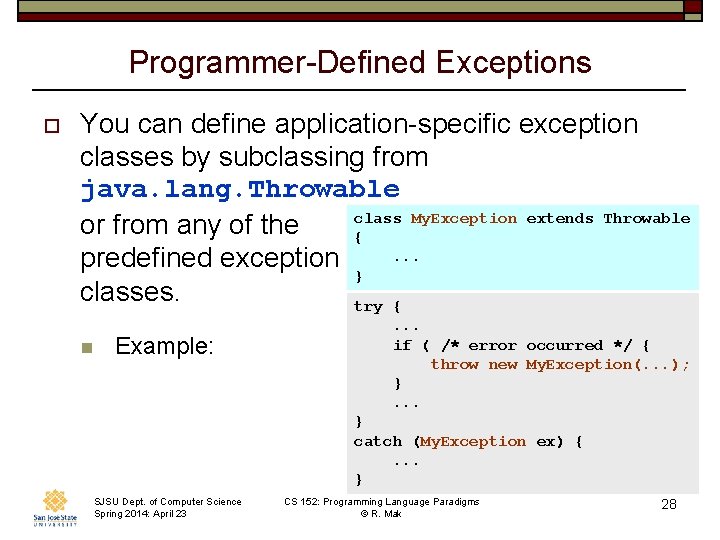
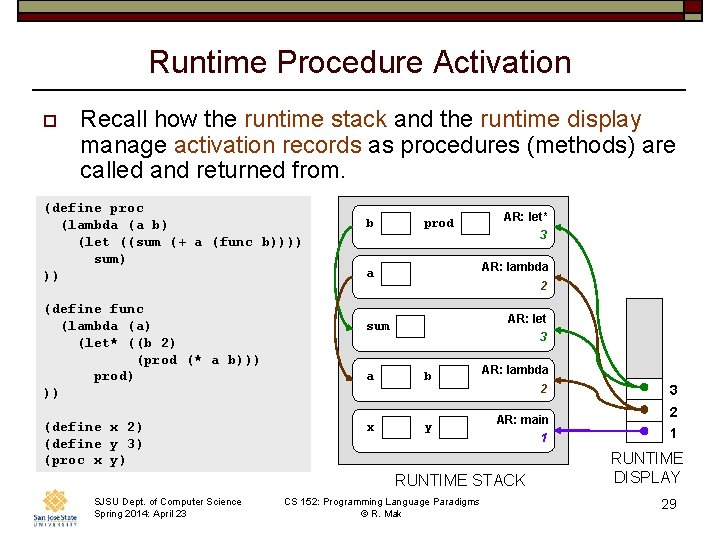
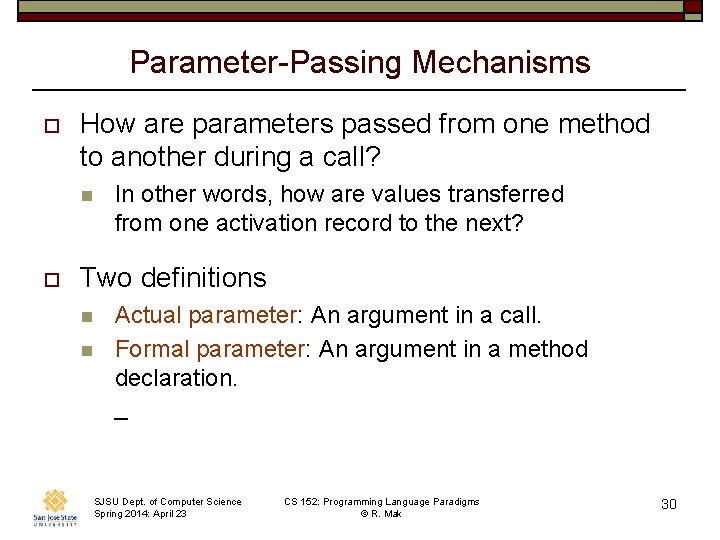
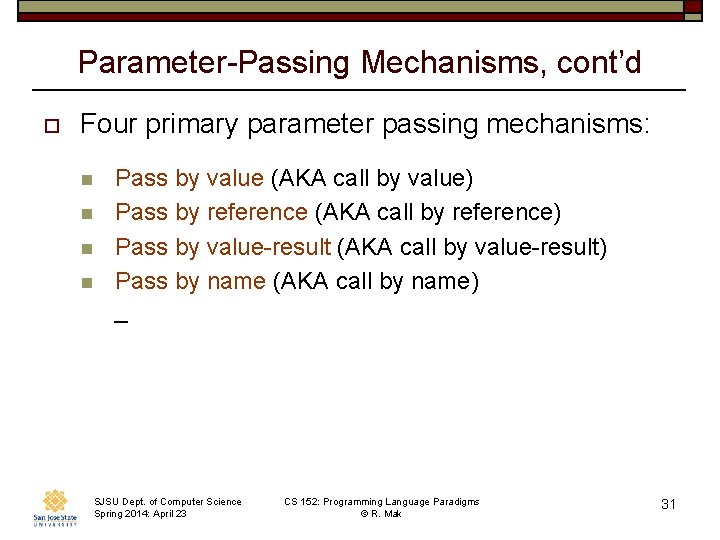
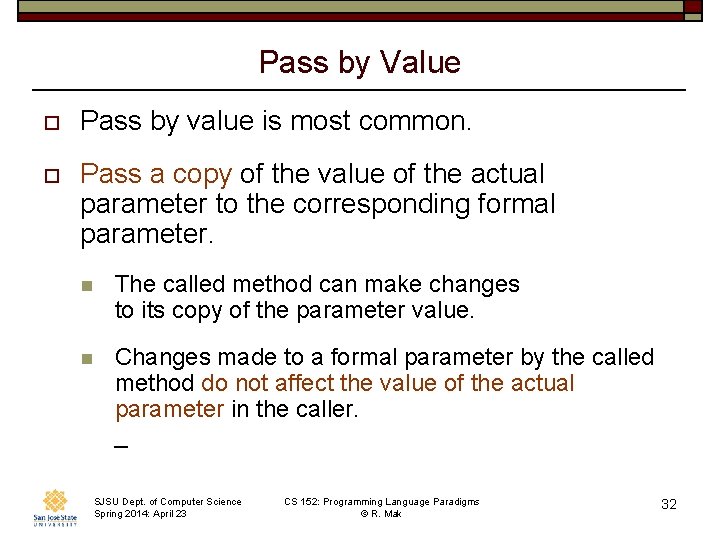
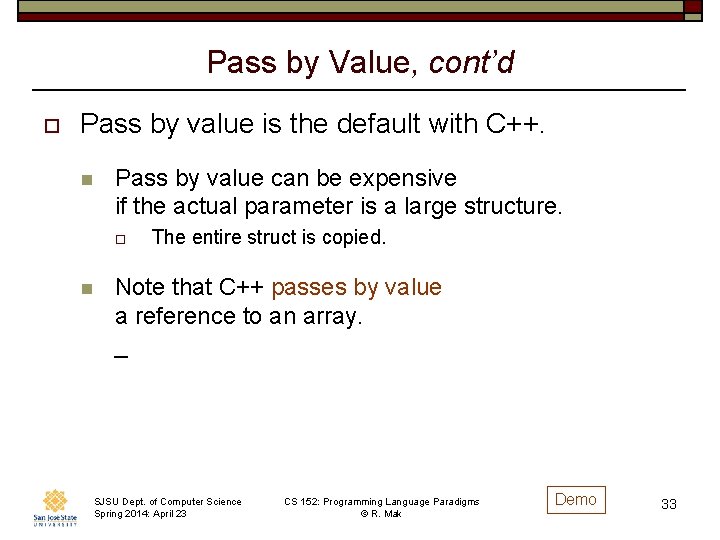
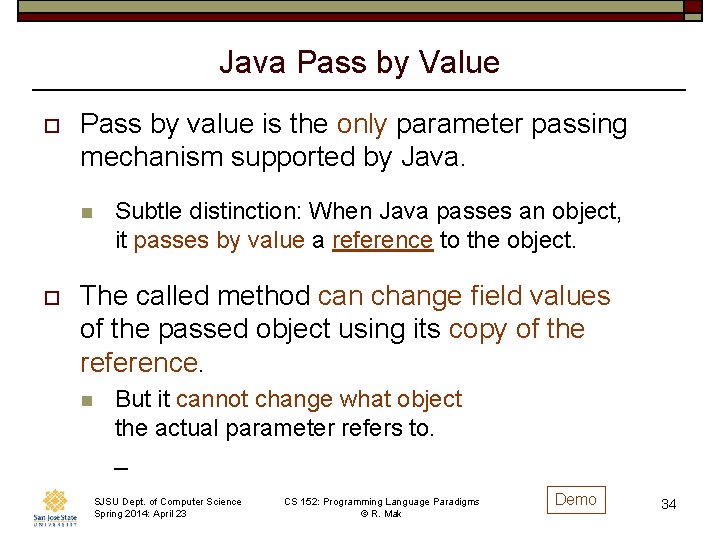
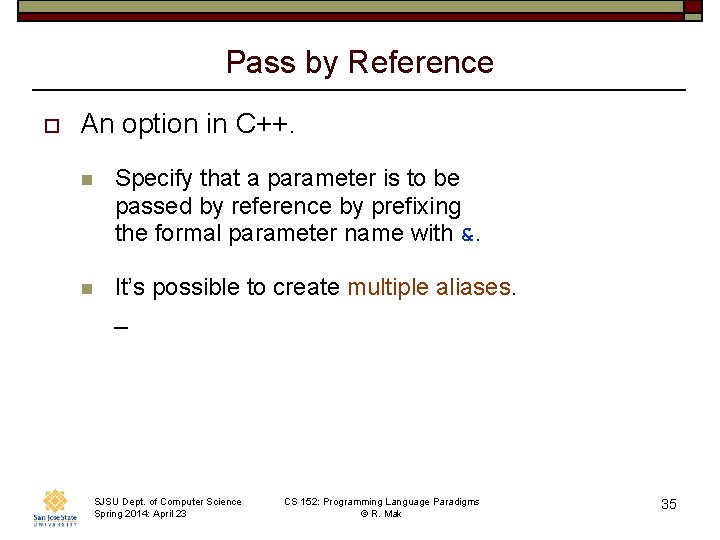
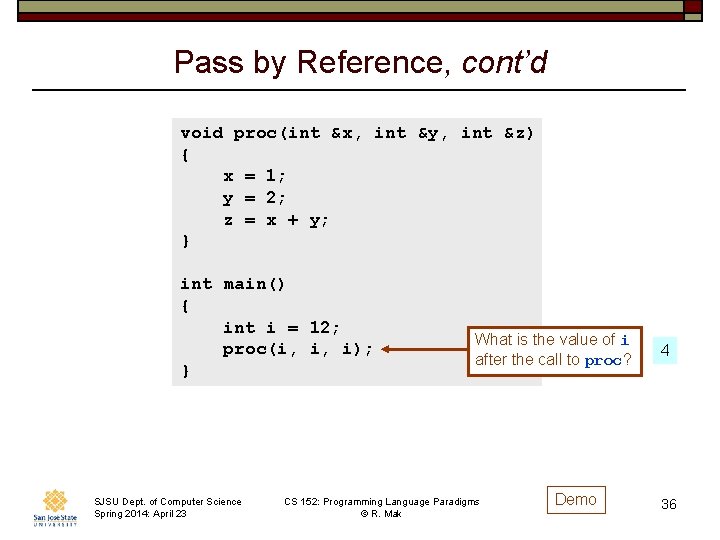
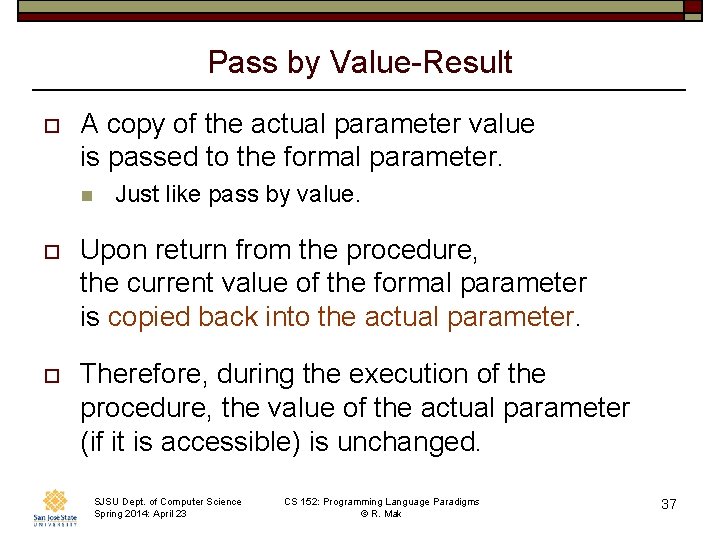
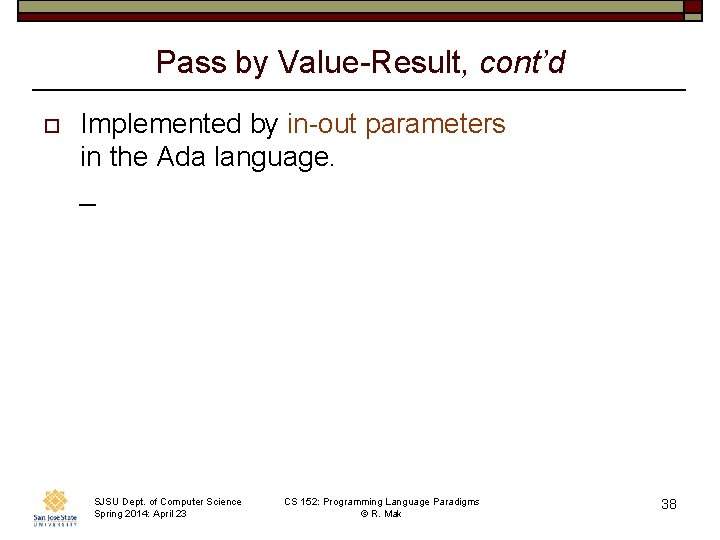
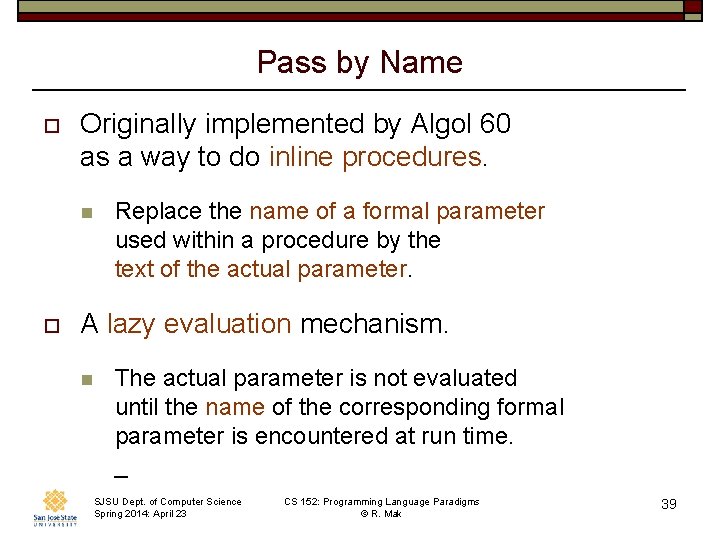
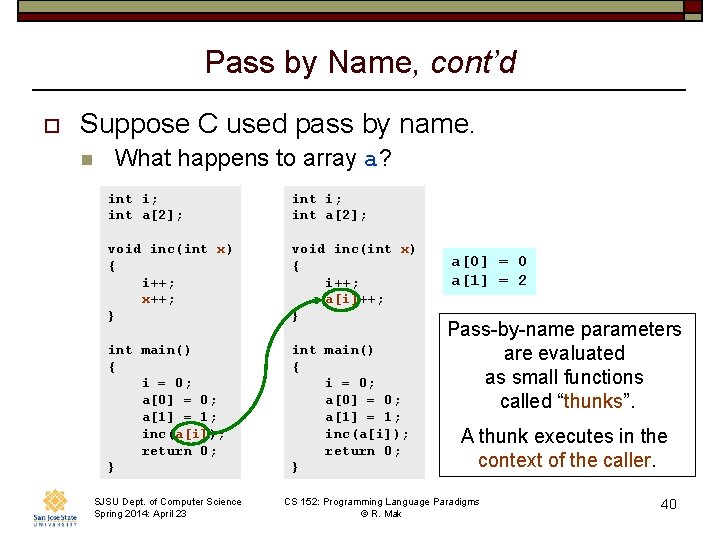
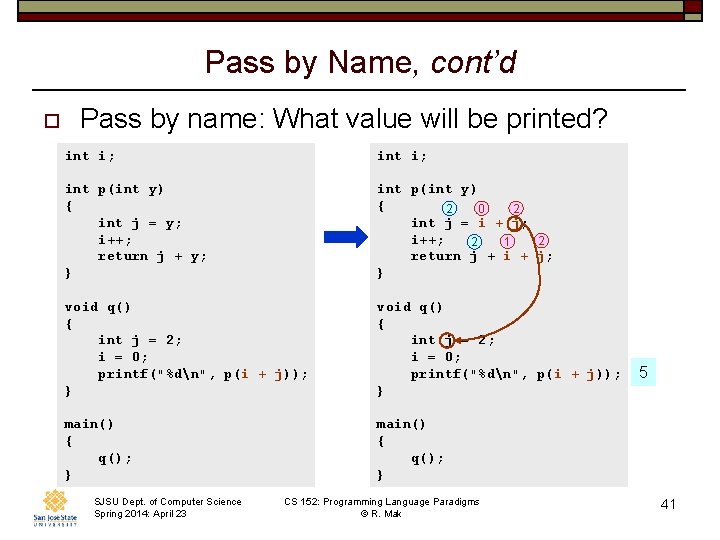
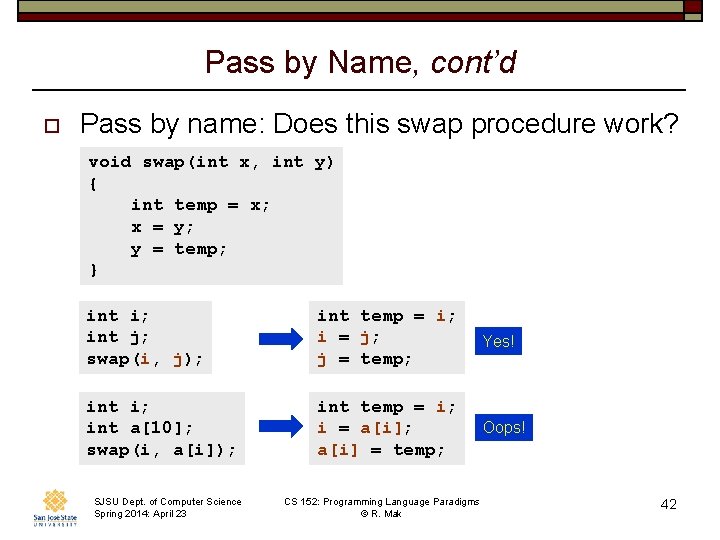
- Slides: 42
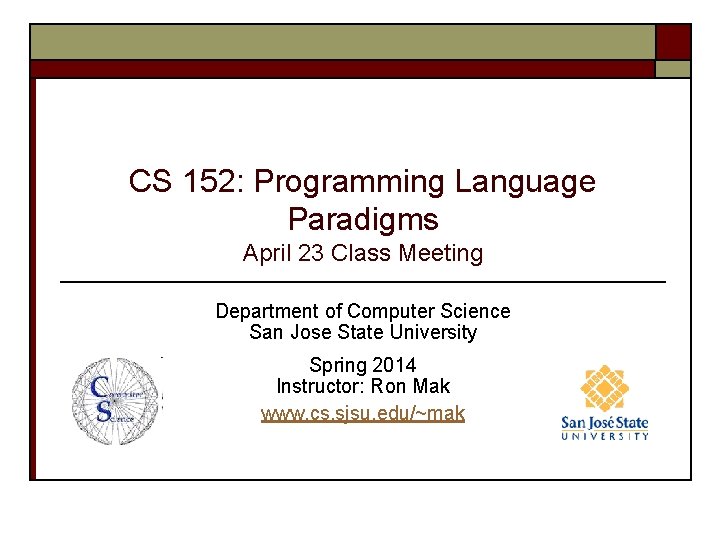
CS 152: Programming Language Paradigms April 23 Class Meeting Department of Computer Science San Jose State University Spring 2014 Instructor: Ron Mak www. cs. sjsu. edu/~mak
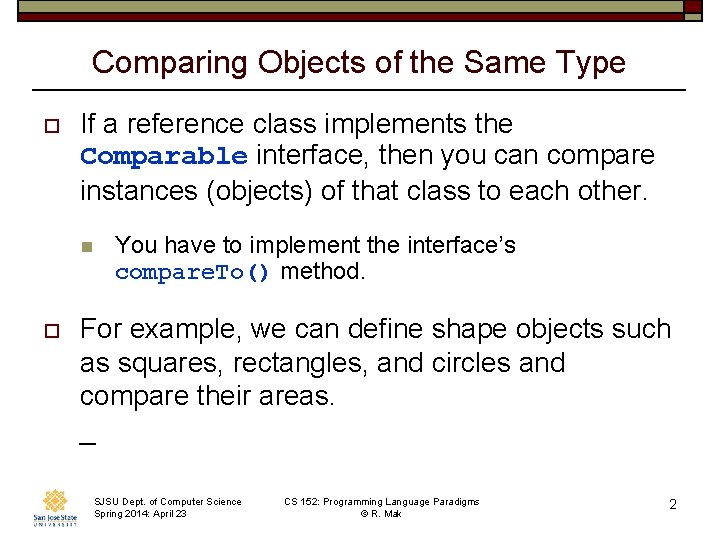
Comparing Objects of the Same Type o If a reference class implements the Comparable interface, then you can compare instances (objects) of that class to each other. n o You have to implement the interface’s compare. To() method. For example, we can define shape objects such as squares, rectangles, and circles and compare their areas. _ SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak 2
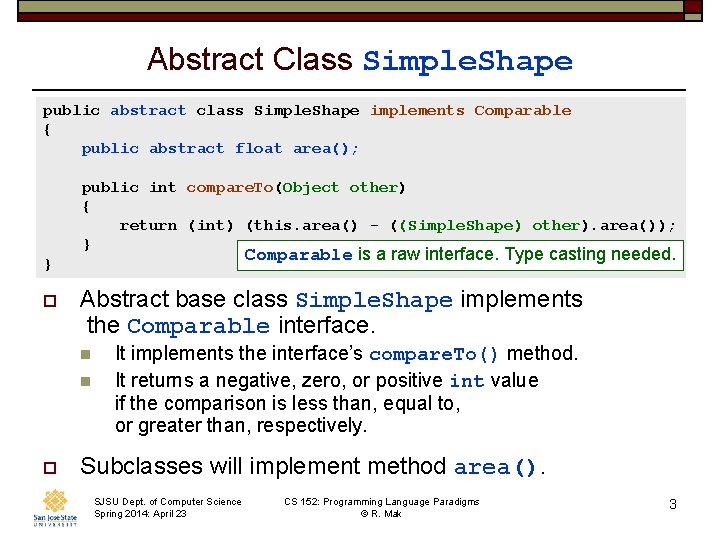
Abstract Class Simple. Shape public abstract class Simple. Shape implements Comparable { public abstract float area(); public int compare. To(Object other) { return (int) (this. area() - ((Simple. Shape) other). area()); } Comparable is a raw interface. Type casting needed. } o Abstract base class Simple. Shape implements the Comparable interface. n n o It implements the interface’s compare. To() method. It returns a negative, zero, or positive int value if the comparison is less than, equal to, or greater than, respectively. Subclasses will implement method area(). SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak 3
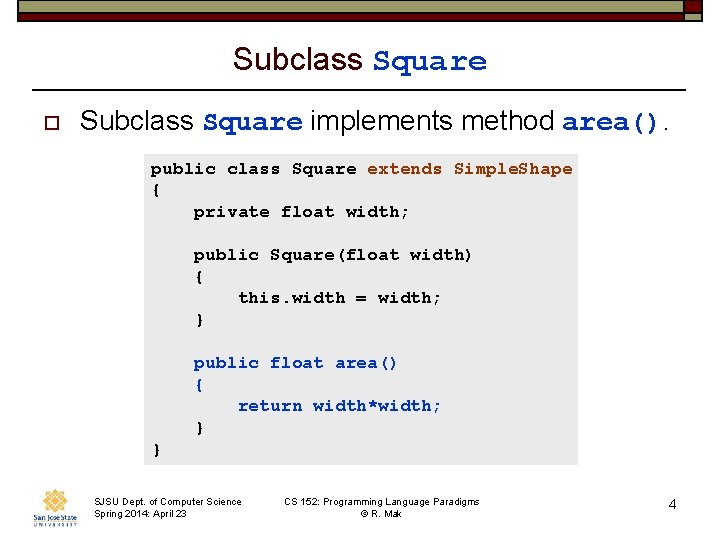
Subclass Square o Subclass Square implements method area(). public class Square extends Simple. Shape { private float width; public Square(float width) { this. width = width; } public float area() { return width*width; } } SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak 4
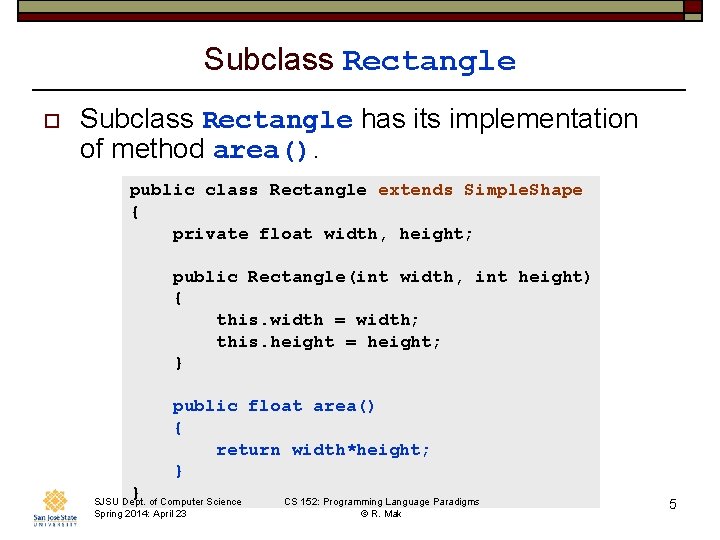
Subclass Rectangle o Subclass Rectangle has its implementation of method area(). public class Rectangle extends Simple. Shape { private float width, height; public Rectangle(int width, int height) { this. width = width; this. height = height; } public float area() { return width*height; } } SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak 5
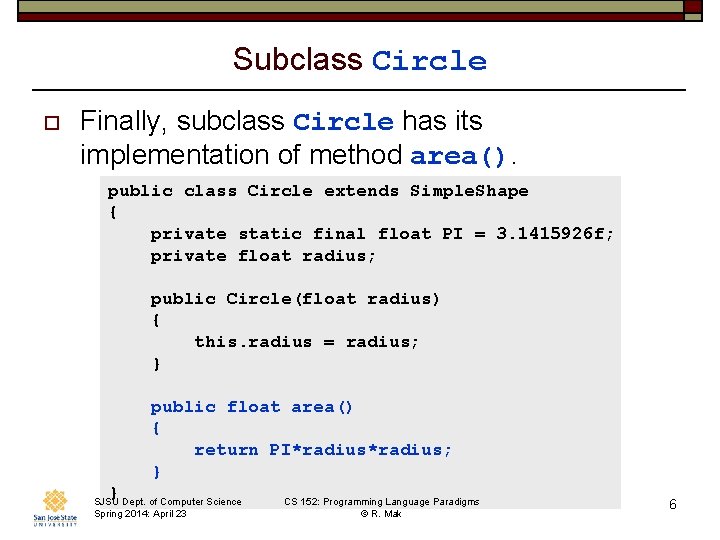
Subclass Circle o Finally, subclass Circle has its implementation of method area(). public class Circle extends Simple. Shape { private static final float PI = 3. 1415926 f; private float radius; public Circle(float radius) { this. radius = radius; } public float area() { return PI*radius; } } SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak 6
![Array 5 Simple Shape Objects public static void mainString args Array ListSimple Shape Array 5: Simple. Shape Objects public static void main(String[] args) { Array. List<Simple. Shape>](https://slidetodoc.com/presentation_image_h/274b00dbf0db0688ebcb176e9f165c63/image-7.jpg)
Array 5: Simple. Shape Objects public static void main(String[] args) { Array. List<Simple. Shape> shapes = new Array. List<>(); shapes. add(new Square(5)); shapes. add(new Rectangle(3, 4)); shapes. add(new Circle(2)); System. out. print("Before sorting: "); print(shapes); sort(shapes); System. out. print(" After sorting: "); print(shapes); } SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak 7
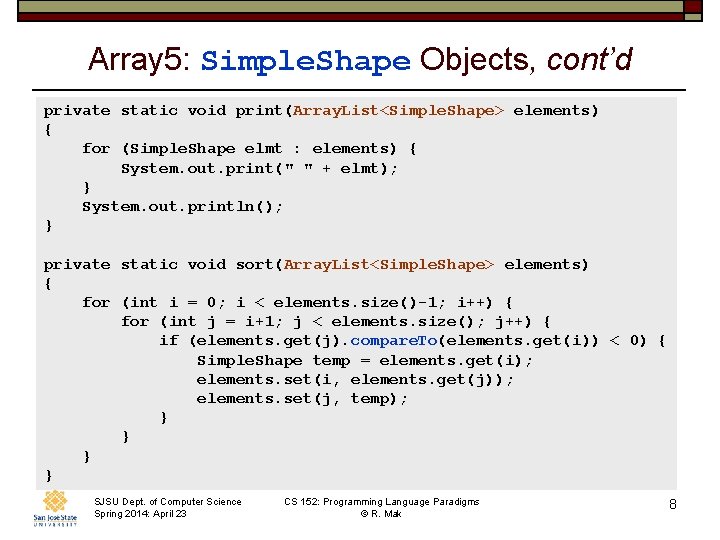
Array 5: Simple. Shape Objects, cont’d private static void print(Array. List<Simple. Shape> elements) { for (Simple. Shape elmt : elements) { System. out. print(" " + elmt); } System. out. println(); } private static void sort(Array. List<Simple. Shape> elements) { for (int i = 0; i < elements. size()-1; i++) { for (int j = i+1; j < elements. size(); j++) { if (elements. get(j). compare. To(elements. get(i)) < 0) { Simple. Shape temp = elements. get(i); elements. set(i, elements. get(j)); elements. set(j, temp); } } SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak 8
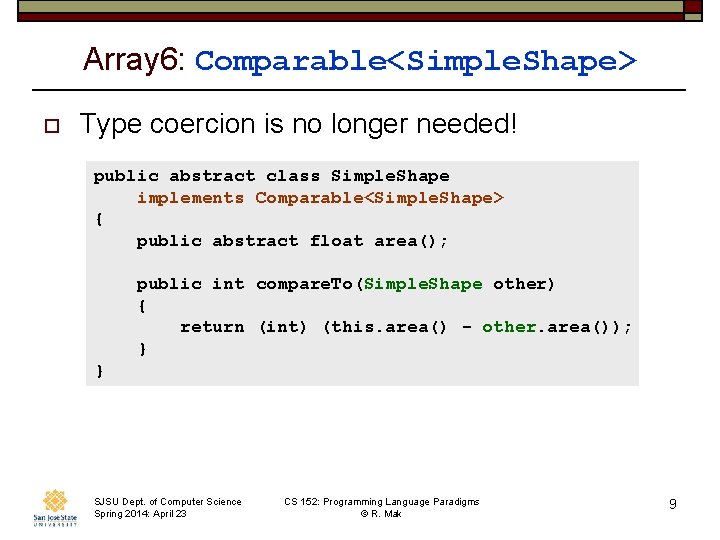
Array 6: Comparable<Simple. Shape> o Type coercion is no longer needed! public abstract class Simple. Shape implements Comparable<Simple. Shape> { public abstract float area(); public int compare. To(Simple. Shape other) { return (int) (this. area() - other. area()); } } SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak 9
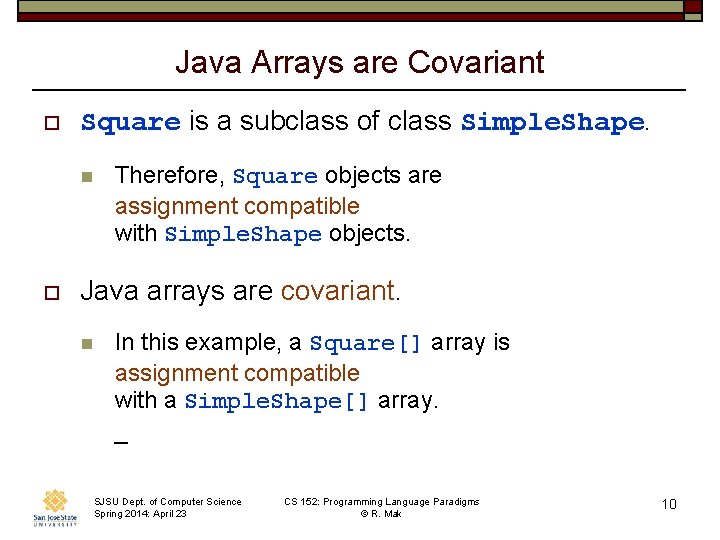
Java Arrays are Covariant o Square is a subclass of class Simple. Shape. n o Therefore, Square objects are assignment compatible with Simple. Shape objects. Java arrays are covariant. n In this example, a Square[] array is assignment compatible with a Simple. Shape[] array. _ SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak 10
![Java Arrays are Covariant contd private static float total AreaSimple Shape elements The Java Arrays are Covariant, cont’d private static float total. Area(Simple. Shape elements[]) { The](https://slidetodoc.com/presentation_image_h/274b00dbf0db0688ebcb176e9f165c63/image-11.jpg)
Java Arrays are Covariant, cont’d private static float total. Area(Simple. Shape elements[]) { The parameter type is Simple. Shape[]. float total = 0; for (Shape elmt : elements) { total += elmt. area(); } return total; } SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak 11
![Java Arrays are Covariant contd public static void mainString args Simple Shape shapes Java Arrays are Covariant, cont’d public static void main(String[] args) { Simple. Shape shapes[]](https://slidetodoc.com/presentation_image_h/274b00dbf0db0688ebcb176e9f165c63/image-12.jpg)
Java Arrays are Covariant, cont’d public static void main(String[] args) { Simple. Shape shapes[] = new Shape[] { new Square(5), new Rectangle(3, 4), new Circle(2) }; System. out. println("Total area: " + total. Area(shapes)); Square squares[] = new Square(5), new Square(3), new Square(2) }; Square[] { Because Square is assignment compatible with Simple. Shape, we can pass an actual Square[] value for a Simple. Shape[] parameter. System. out. println("Total area: " + total. Area(squares)); } SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak Demo 12
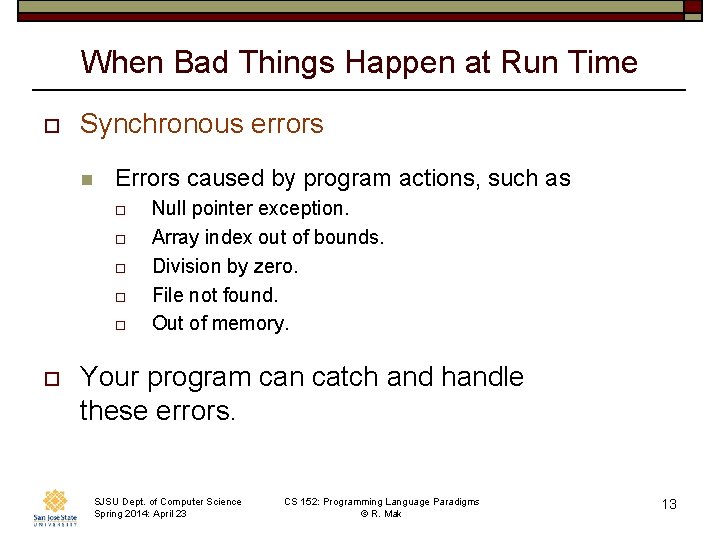
When Bad Things Happen at Run Time o Synchronous errors n Errors caused by program actions, such as o o o Null pointer exception. Array index out of bounds. Division by zero. File not found. Out of memory. Your program can catch and handle these errors. SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak 13
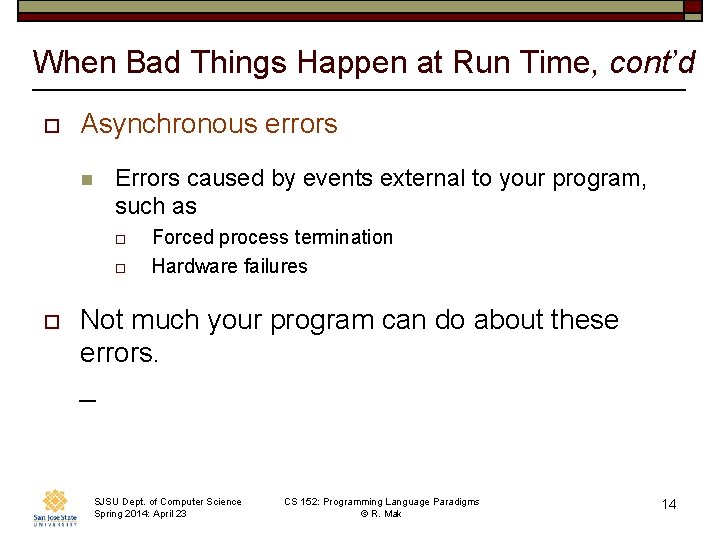
When Bad Things Happen at Run Time, cont’d o Asynchronous errors n Errors caused by events external to your program, such as o o o Forced process termination Hardware failures Not much your program can do about these errors. _ SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak 14
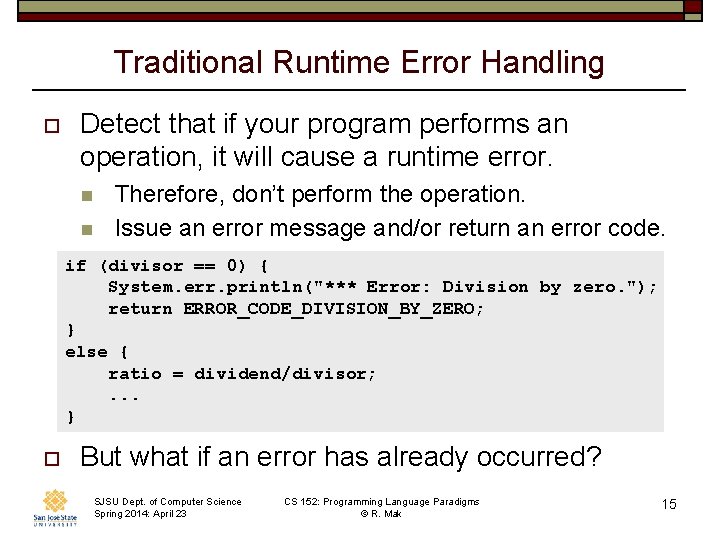
Traditional Runtime Error Handling o Detect that if your program performs an operation, it will cause a runtime error. n n Therefore, don’t perform the operation. Issue an error message and/or return an error code. if (divisor == 0) { System. err. println("*** Error: Division by zero. "); return ERROR_CODE_DIVISION_BY_ZERO; } else { ratio = dividend/divisor; . . . } o But what if an error has already occurred? SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak 15
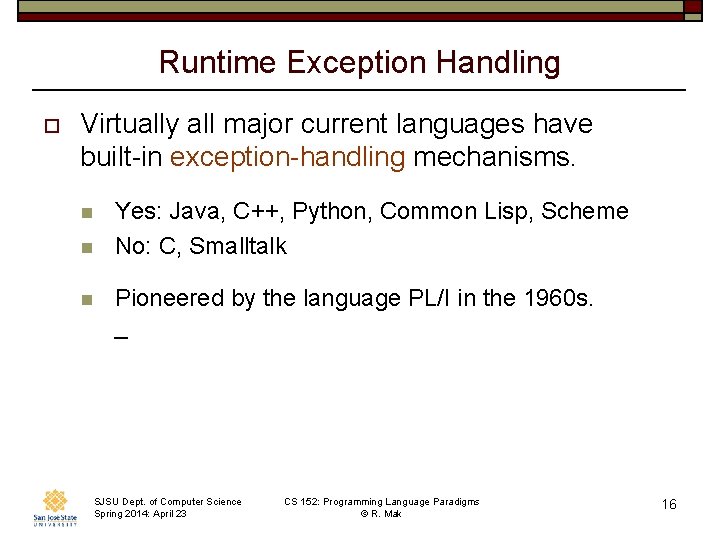
Runtime Exception Handling o Virtually all major current languages have built-in exception-handling mechanisms. n n n Yes: Java, C++, Python, Common Lisp, Scheme No: C, Smalltalk Pioneered by the language PL/I in the 1960 s. _ SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak 16
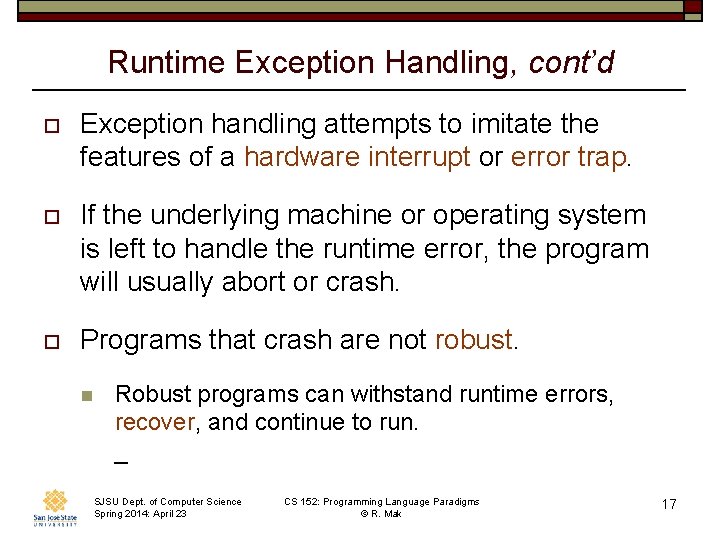
Runtime Exception Handling, cont’d o Exception handling attempts to imitate the features of a hardware interrupt or error trap. o If the underlying machine or operating system is left to handle the runtime error, the program will usually abort or crash. o Programs that crash are not robust. n Robust programs can withstand runtime errors, recover, and continue to run. _ SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak 17
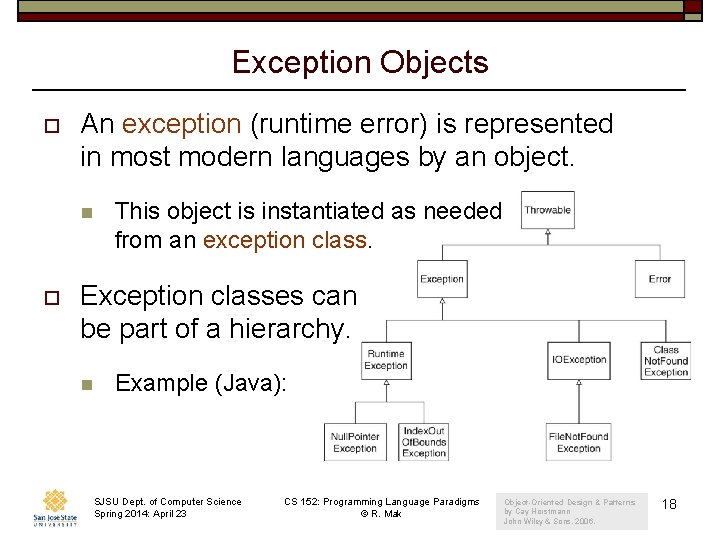
Exception Objects o An exception (runtime error) is represented in most modern languages by an object. n o This object is instantiated as needed from an exception class. Exception classes can be part of a hierarchy. n Example (Java): SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak Object-Oriented Design & Patterns by Cay Horstmann John Wiley & Sons, 2006. 18
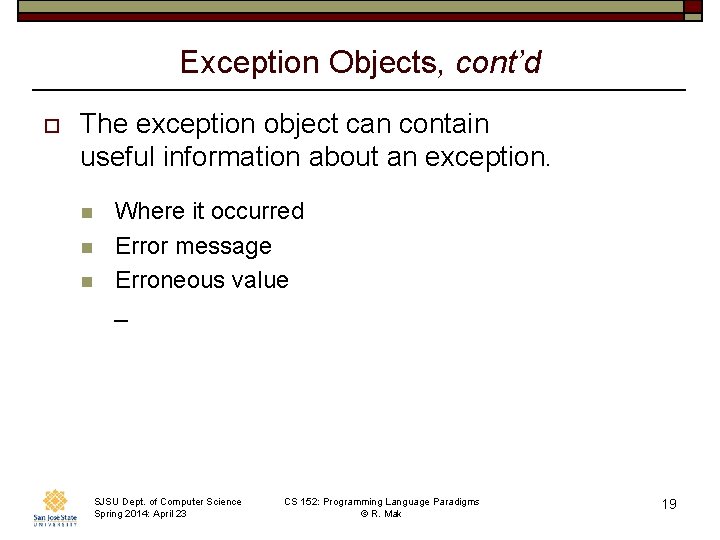
Exception Objects, cont’d o The exception object can contain useful information about an exception. n n n Where it occurred Error message Erroneous value _ SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak 19
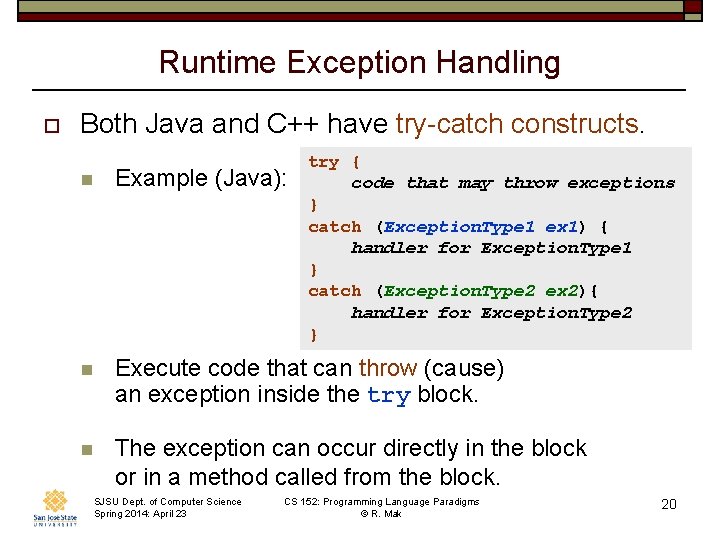
Runtime Exception Handling o Both Java and C++ have try-catch constructs. try { code that may throw exceptions } catch (Exception. Type 1 ex 1) { handler for Exception. Type 1 } catch (Exception. Type 2 ex 2){ handler for Exception. Type 2 } n Example (Java): n Execute code that can throw (cause) an exception inside the try block. n The exception can occur directly in the block or in a method called from the block. SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak 20
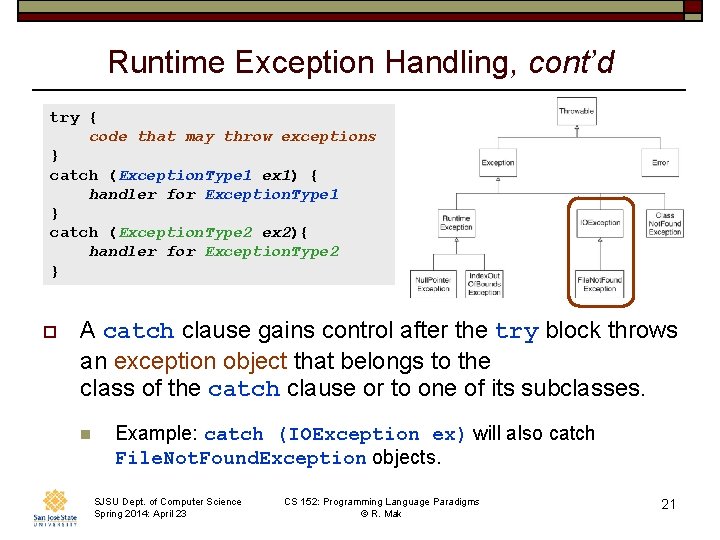
Runtime Exception Handling, cont’d try { code that may throw exceptions } catch (Exception. Type 1 ex 1) { handler for Exception. Type 1 } catch (Exception. Type 2 ex 2){ handler for Exception. Type 2 } o A catch clause gains control after the try block throws an exception object that belongs to the class of the catch clause or to one of its subclasses. n Example: catch (IOException ex) will also catch File. Not. Found. Exception objects. SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak 21
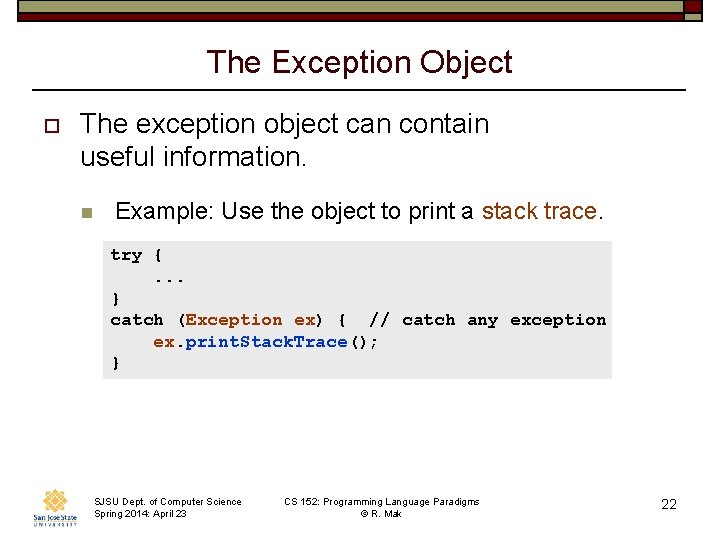
The Exception Object o The exception object can contain useful information. n Example: Use the object to print a stack trace. try {. . . } catch (Exception ex) { // catch any exception ex. print. Stack. Trace(); } SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak 22
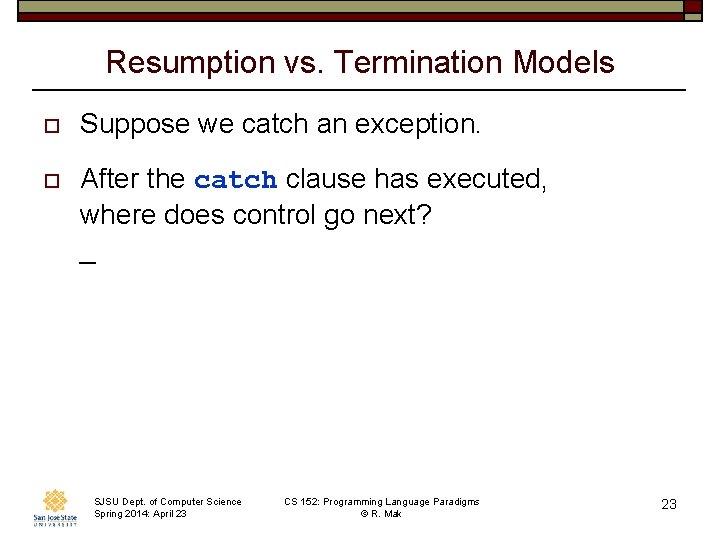
Resumption vs. Termination Models o Suppose we catch an exception. o After the catch clause has executed, where does control go next? _ SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak 23
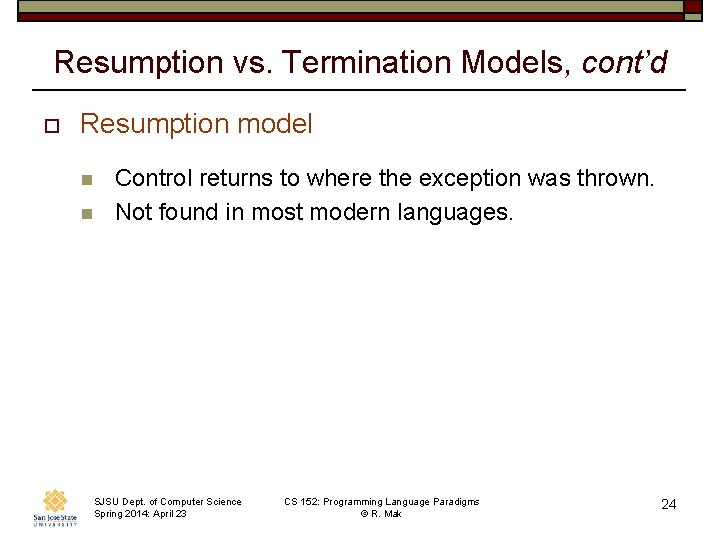
Resumption vs. Termination Models, cont’d o Resumption model n n Control returns to where the exception was thrown. Not found in most modern languages. SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak 24
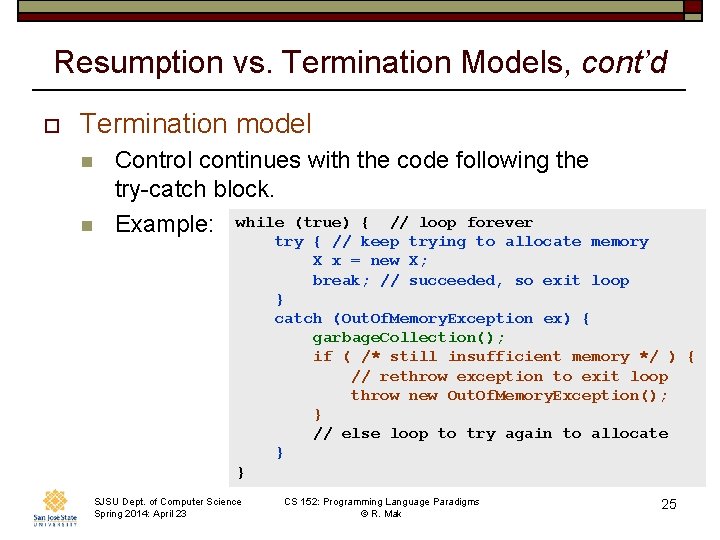
Resumption vs. Termination Models, cont’d o Termination model n n Control continues with the code following the try-catch block. Example: while (true) { // loop forever try { // keep trying to allocate memory X x = new X; break; // succeeded, so exit loop } catch (Out. Of. Memory. Exception ex) { garbage. Collection(); if ( /* still insufficient memory */ ) { // rethrow exception to exit loop throw new Out. Of. Memory. Exception(); } // else loop to try again to allocate } } SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak 25
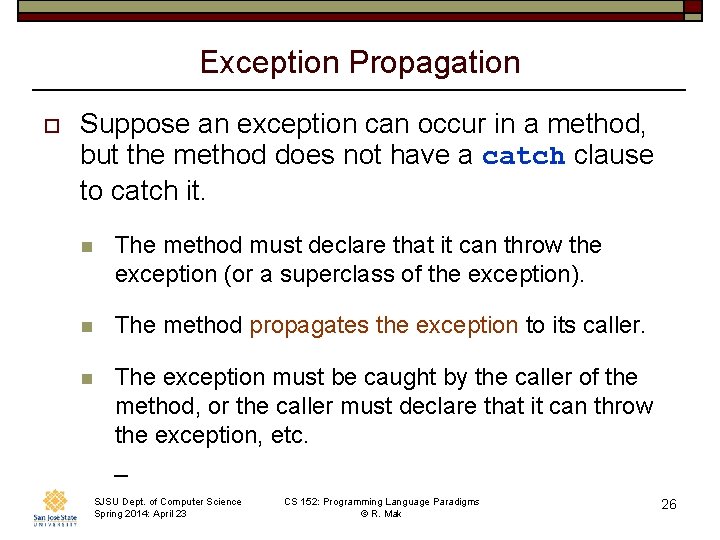
Exception Propagation o Suppose an exception can occur in a method, but the method does not have a catch clause to catch it. n The method must declare that it can throw the exception (or a superclass of the exception). n The method propagates the exception to its caller. n The exception must be caught by the caller of the method, or the caller must declare that it can throw the exception, etc. _ SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak 26
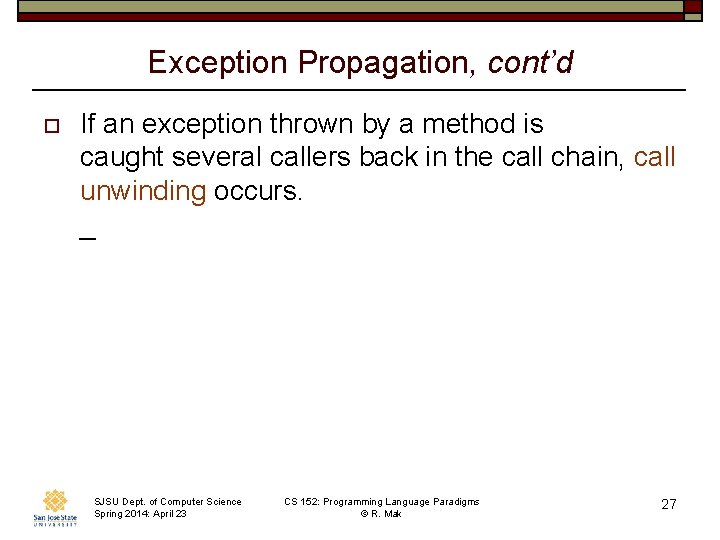
Exception Propagation, cont’d o If an exception thrown by a method is caught several callers back in the call chain, call unwinding occurs. _ SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak 27
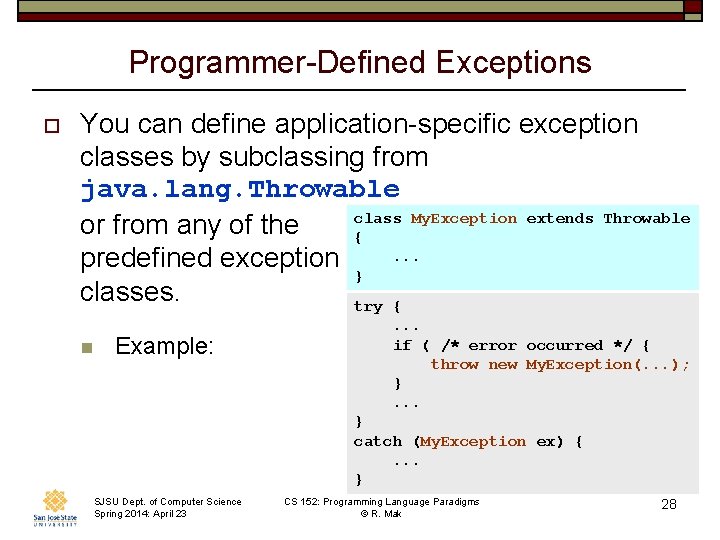
Programmer-Defined Exceptions o You can define application-specific exception classes by subclassing from java. lang. Throwable class My. Exception extends Throwable or from any of the { predefined exception }. . . classes. try { n Example: . . . if ( /* error occurred */ { throw new My. Exception(. . . ); }. . . } catch (My. Exception ex) {. . . } SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak 28
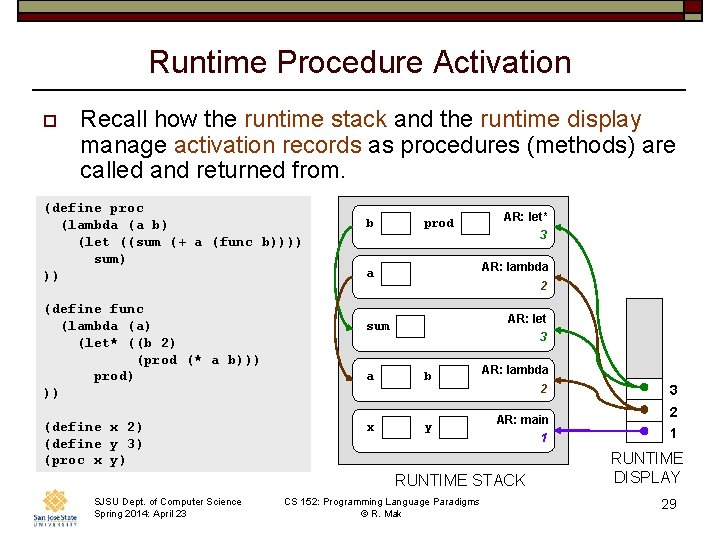
Runtime Procedure Activation o Recall how the runtime stack and the runtime display manage activation records as procedures (methods) are called and returned from. (define proc (lambda (a b) (let ((sum (+ a (func b)))) sum) )) (define func (lambda (a) (let* ((b 2) (prod (* a b))) prod) )) (define x 2) (define y 3) (proc x y) b prod AR: let* 3 AR: lambda a 2 AR: let 3 sum a b x y AR: lambda 2 AR: main 1 RUNTIME STACK SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak 3 2 1 RUNTIME DISPLAY 29
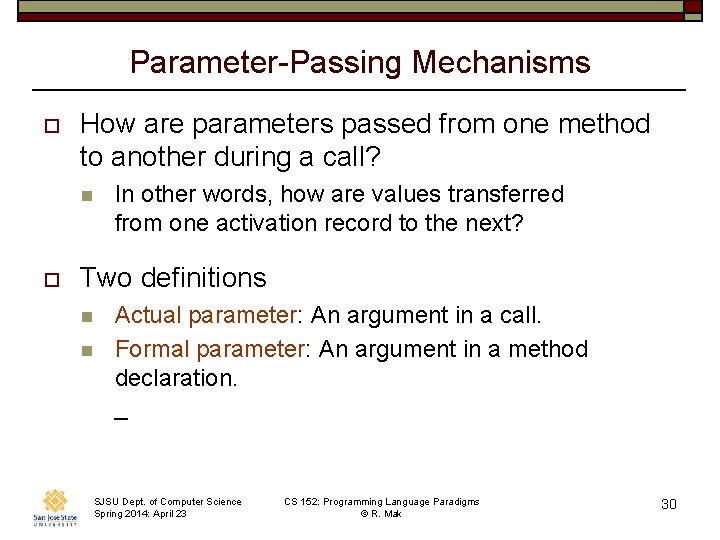
Parameter-Passing Mechanisms o How are parameters passed from one method to another during a call? n o In other words, how are values transferred from one activation record to the next? Two definitions n n Actual parameter: An argument in a call. Formal parameter: An argument in a method declaration. _ SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak 30
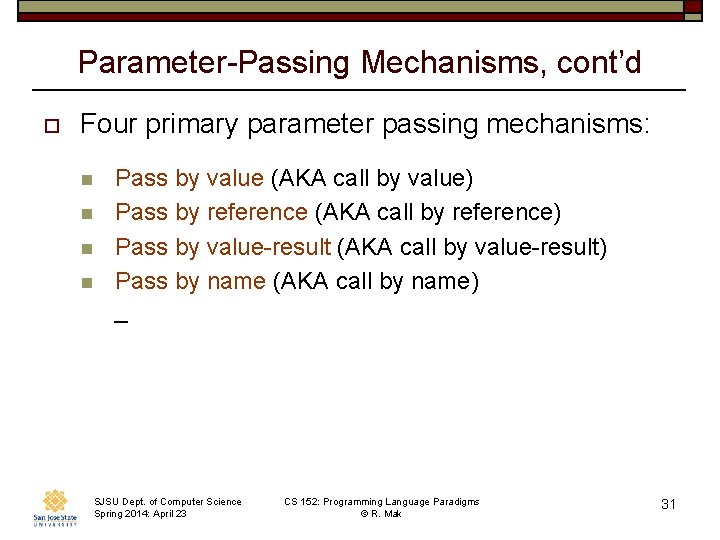
Parameter-Passing Mechanisms, cont’d o Four primary parameter passing mechanisms: n n Pass by value (AKA call by value) Pass by reference (AKA call by reference) Pass by value-result (AKA call by value-result) Pass by name (AKA call by name) _ SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak 31
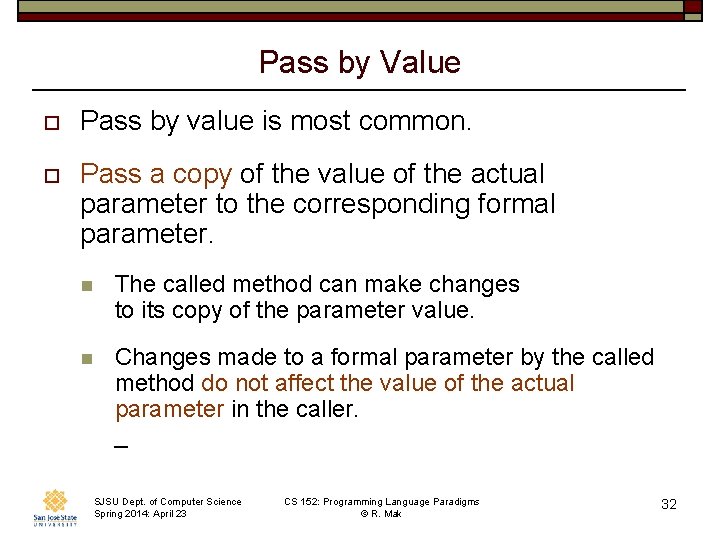
Pass by Value o Pass by value is most common. o Pass a copy of the value of the actual parameter to the corresponding formal parameter. n The called method can make changes to its copy of the parameter value. n Changes made to a formal parameter by the called method do not affect the value of the actual parameter in the caller. _ SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak 32
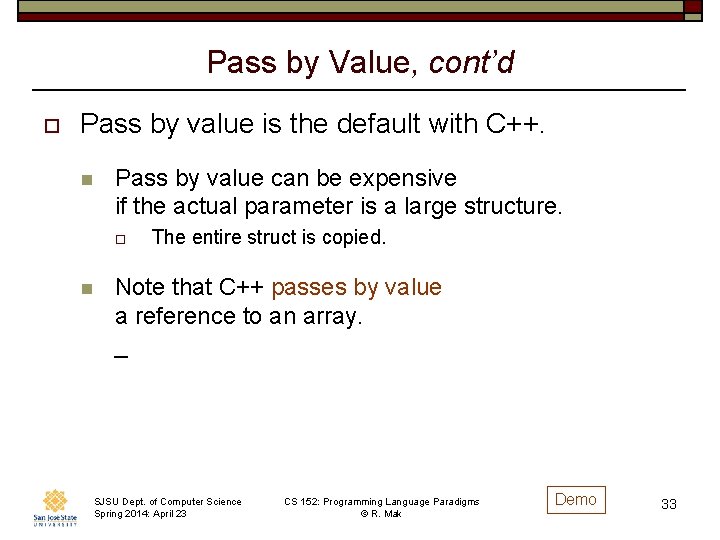
Pass by Value, cont’d o Pass by value is the default with C++. n Pass by value can be expensive if the actual parameter is a large structure. o n The entire struct is copied. Note that C++ passes by value a reference to an array. _ SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak Demo 33
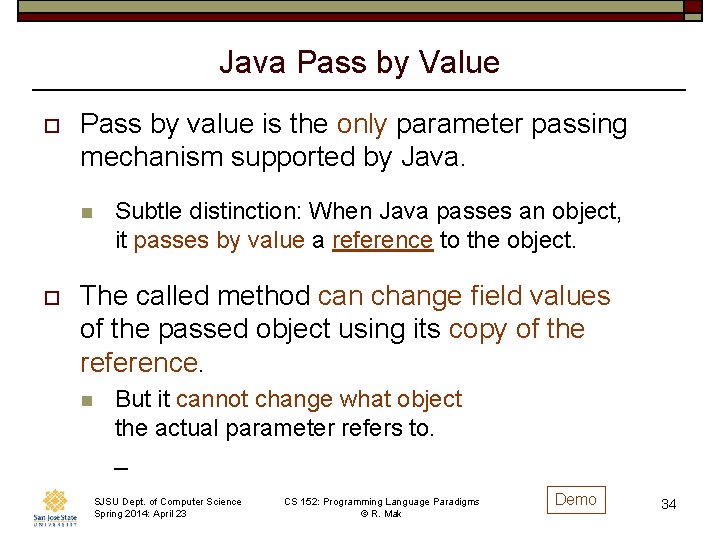
Java Pass by Value o Pass by value is the only parameter passing mechanism supported by Java. n o Subtle distinction: When Java passes an object, it passes by value a reference to the object. The called method can change field values of the passed object using its copy of the reference. n But it cannot change what object the actual parameter refers to. _ SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak Demo 34
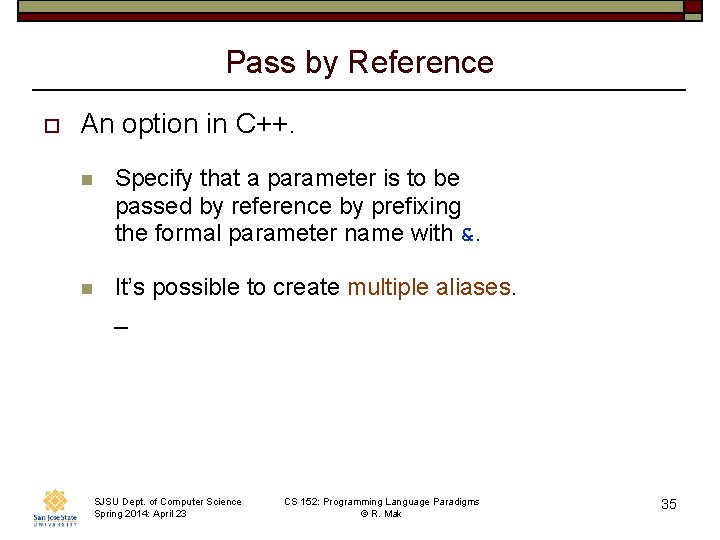
Pass by Reference o An option in C++. n Specify that a parameter is to be passed by reference by prefixing the formal parameter name with &. n It’s possible to create multiple aliases. _ SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak 35
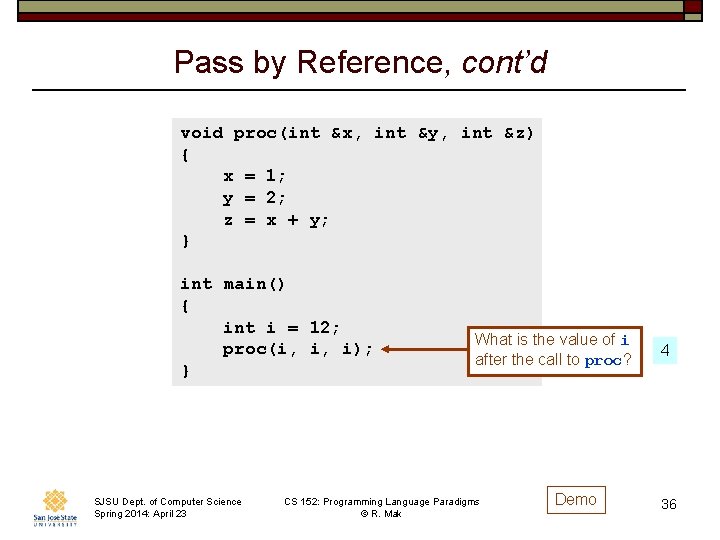
Pass by Reference, cont’d void proc(int &x, int &y, int &z) { x = 1; y = 2; z = x + y; } int main() { int i = 12; proc(i, i, i); } SJSU Dept. of Computer Science Spring 2014: April 23 What is the value of i after the call to proc? CS 152: Programming Language Paradigms © R. Mak Demo 4 36
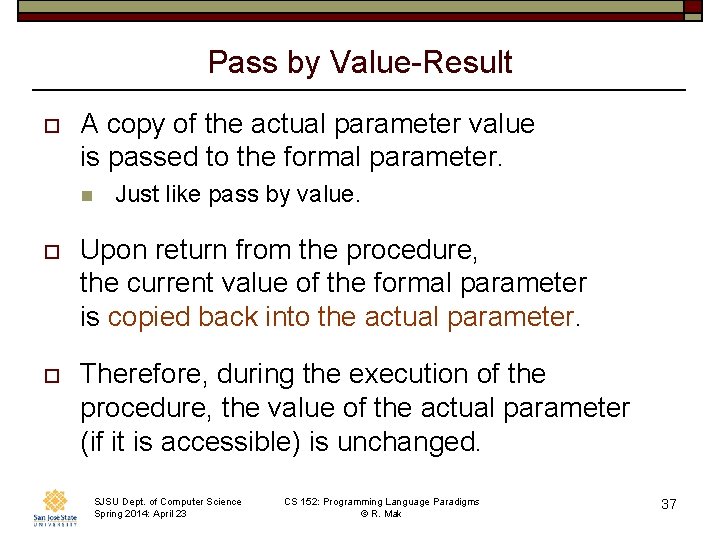
Pass by Value-Result o A copy of the actual parameter value is passed to the formal parameter. n Just like pass by value. o Upon return from the procedure, the current value of the formal parameter is copied back into the actual parameter. o Therefore, during the execution of the procedure, the value of the actual parameter (if it is accessible) is unchanged. SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak 37
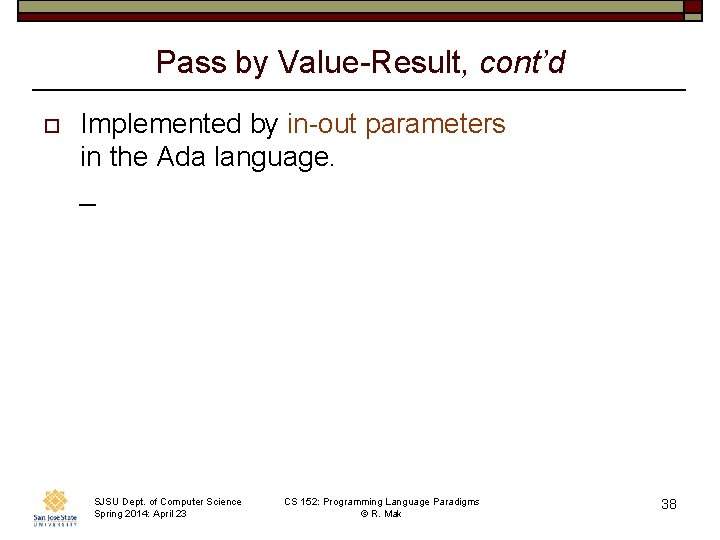
Pass by Value-Result, cont’d o Implemented by in-out parameters in the Ada language. _ SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak 38
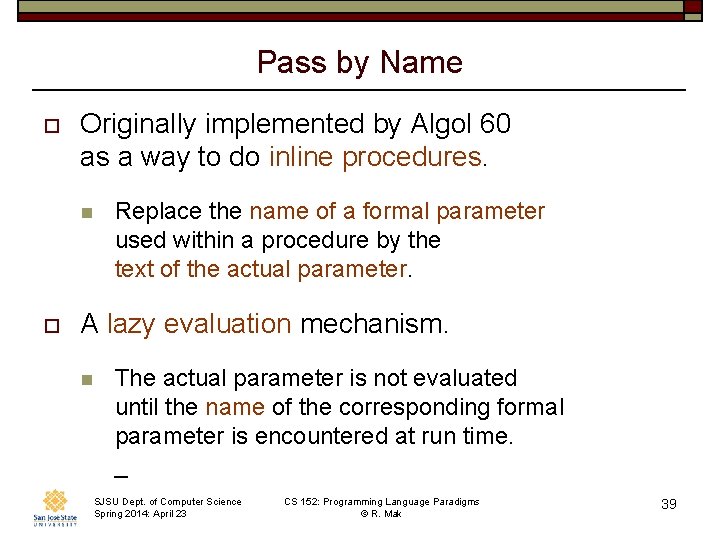
Pass by Name o Originally implemented by Algol 60 as a way to do inline procedures. n o Replace the name of a formal parameter used within a procedure by the text of the actual parameter. A lazy evaluation mechanism. n The actual parameter is not evaluated until the name of the corresponding formal parameter is encountered at run time. _ SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak 39
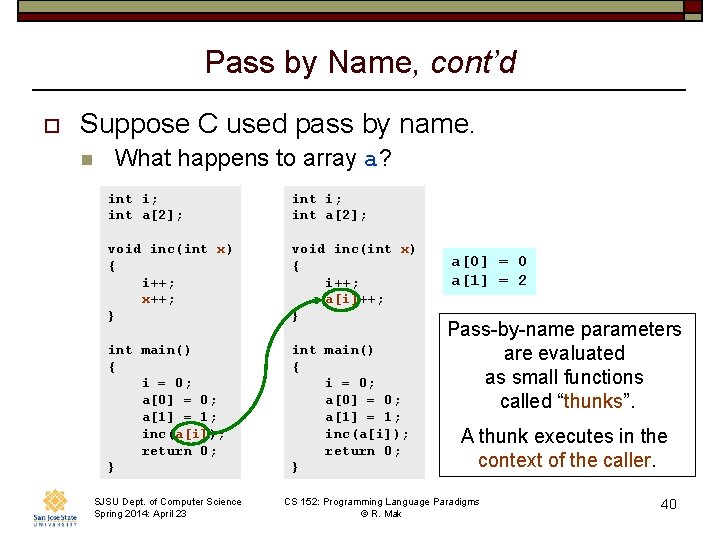
Pass by Name, cont’d o Suppose C used pass by name. n What happens to array a? int i; int a[2]; void inc(int x) { i++; x++; } void inc(int x) { i++; a[i]++; } int main() { i = 0; a[0] = 0; a[1] = 1; inc(a[i]); return 0; } SJSU Dept. of Computer Science Spring 2014: April 23 a[0] = 0 a[1] = 2 Pass-by-name parameters are evaluated as small functions called “thunks”. A thunk executes in the context of the caller. CS 152: Programming Language Paradigms © R. Mak 40
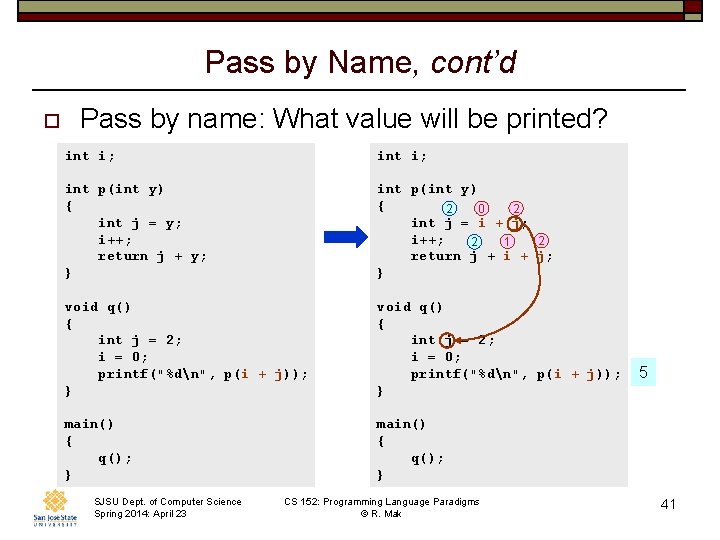
Pass by Name, cont’d o Pass by name: What value will be printed? int i; int p(int y) { int j = y; i++; return j + y; } int p(int y) { 2 0 2 int j = i + j; i++; 2 1 2 return j + i + j; } void q() { int j = 2; i = 0; printf("%dn", p(i + j)); } main() { q(); } SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak 5 41
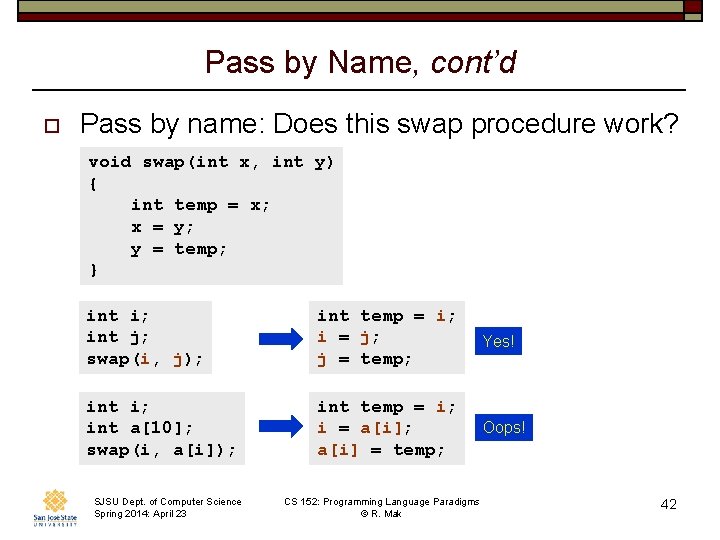
Pass by Name, cont’d o Pass by name: Does this swap procedure work? void swap(int x, int y) { int temp = x; x = y; y = temp; } int i; int j; swap(i, j); int temp = i; i = j; j = temp; Yes! int i; int a[10]; swap(i, a[i]); int temp = i; i = a[i]; a[i] = temp; Oops! SJSU Dept. of Computer Science Spring 2014: April 23 CS 152: Programming Language Paradigms © R. Mak 42