COSE 222 COMP 212 CYDF 210 Computer Architecture
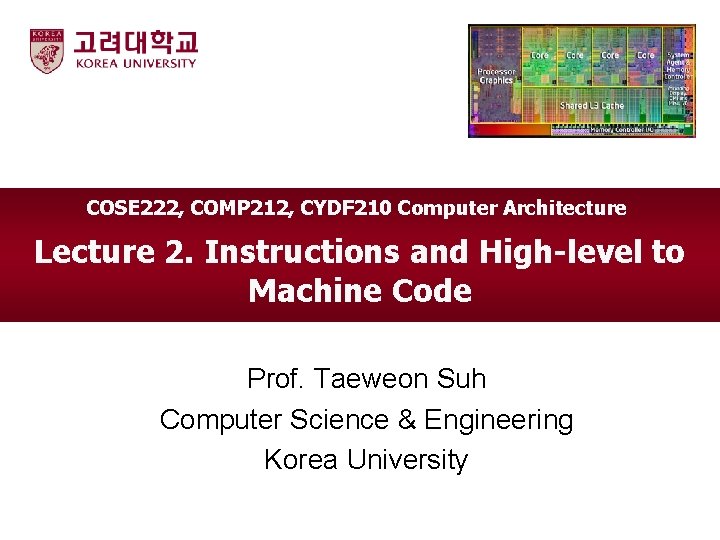
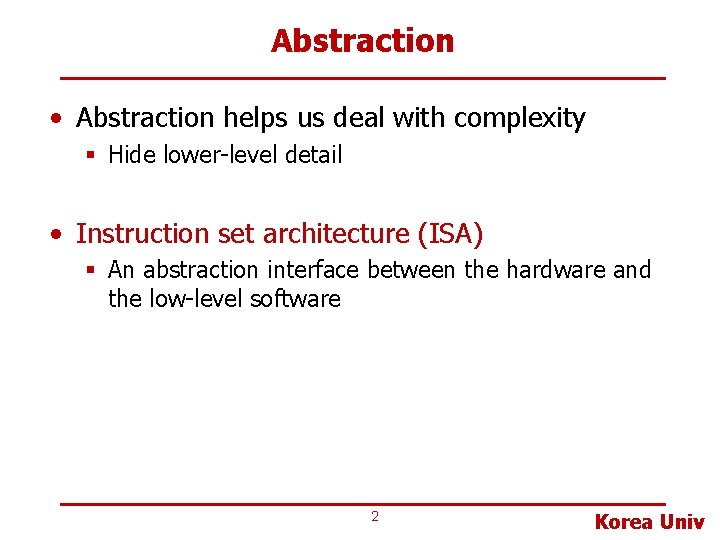
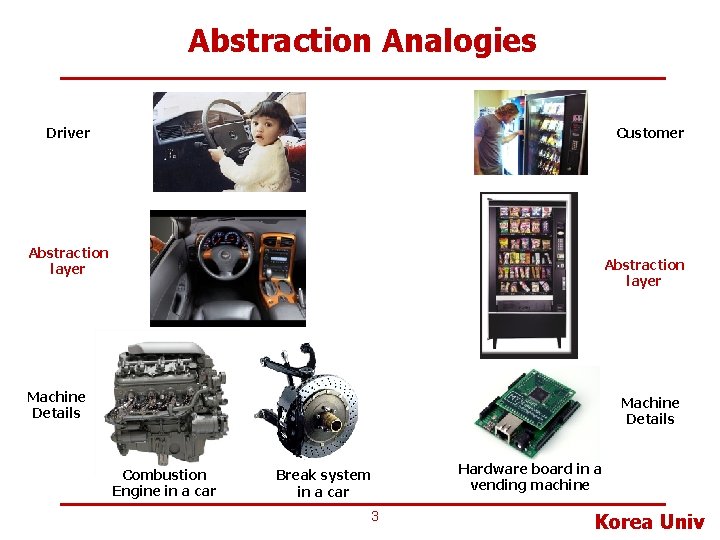
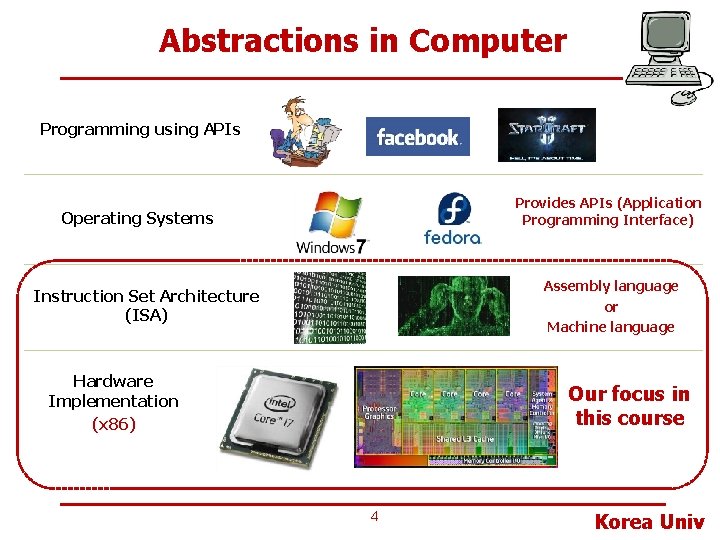
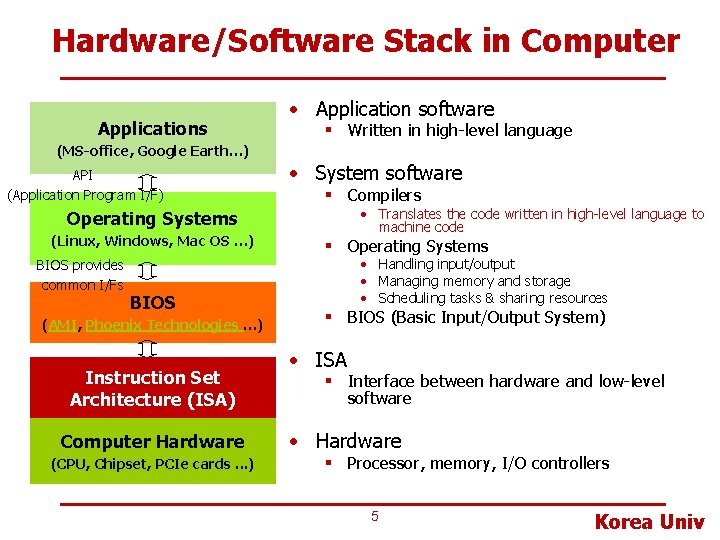
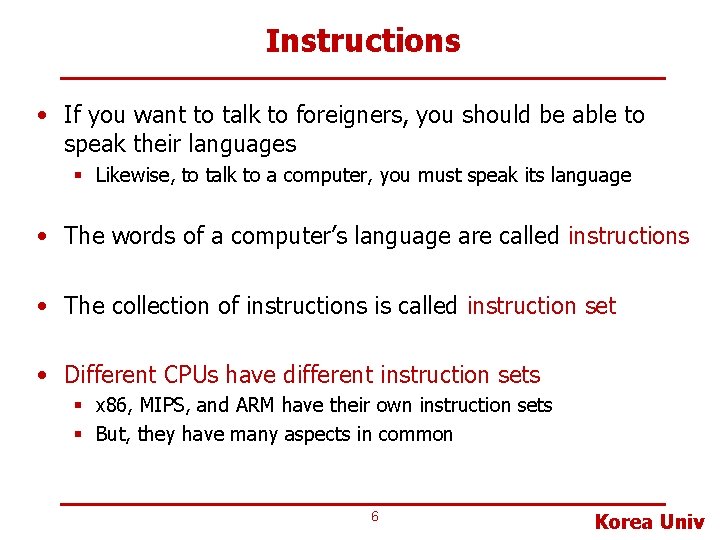
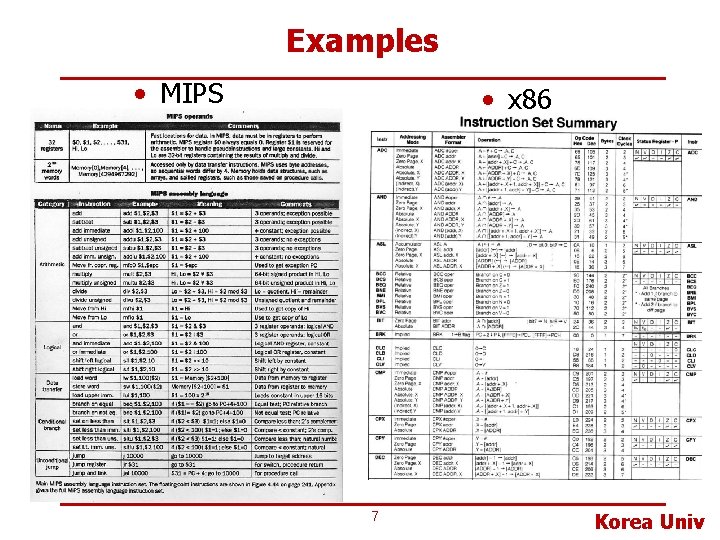
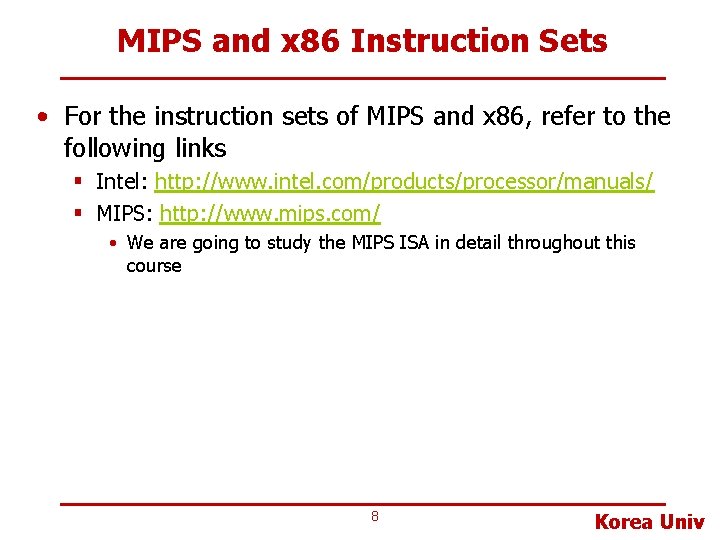
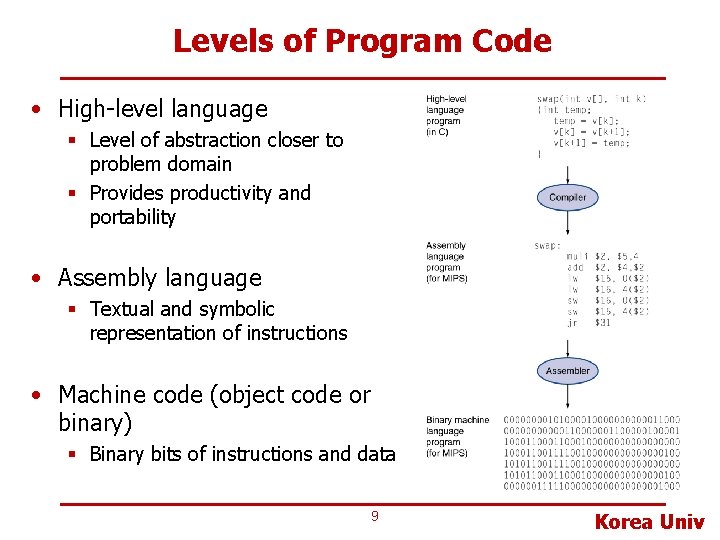
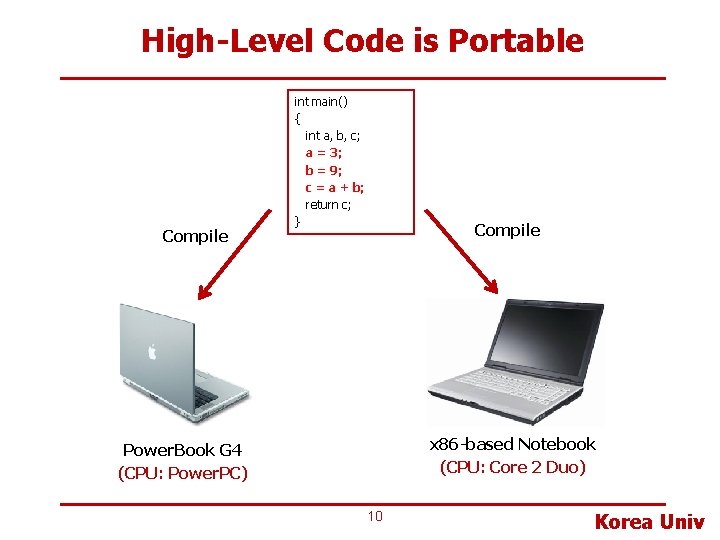
![Levels of Program Code • High-level language program in C swap (int v[], int Levels of Program Code • High-level language program in C swap (int v[], int](https://slidetodoc.com/presentation_image_h2/a843d299a7a09898965d213aa0256165/image-11.jpg)
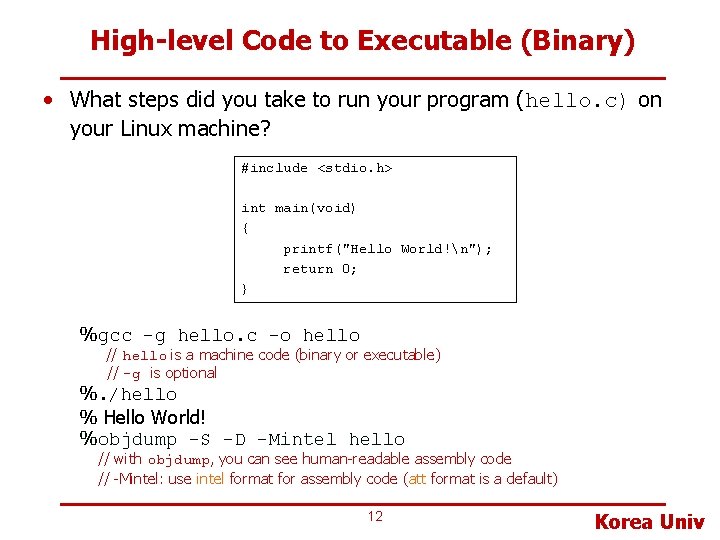
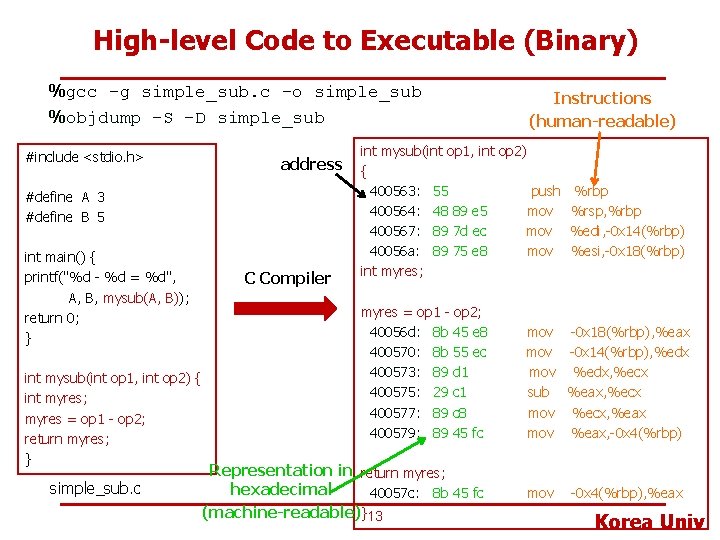
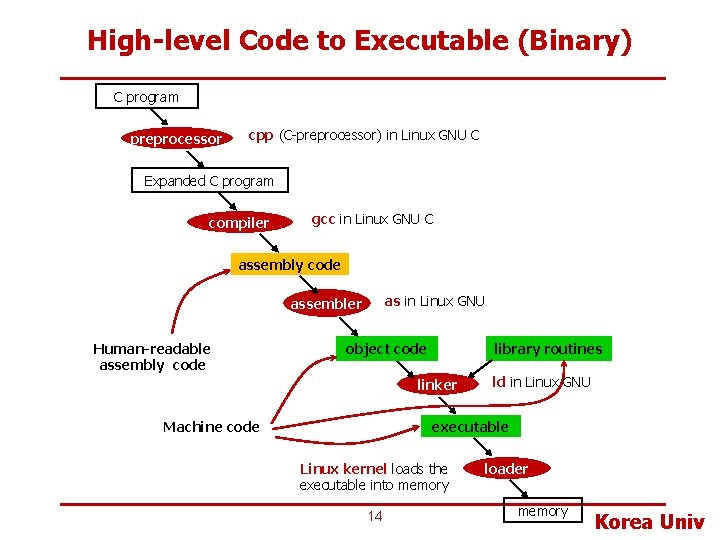
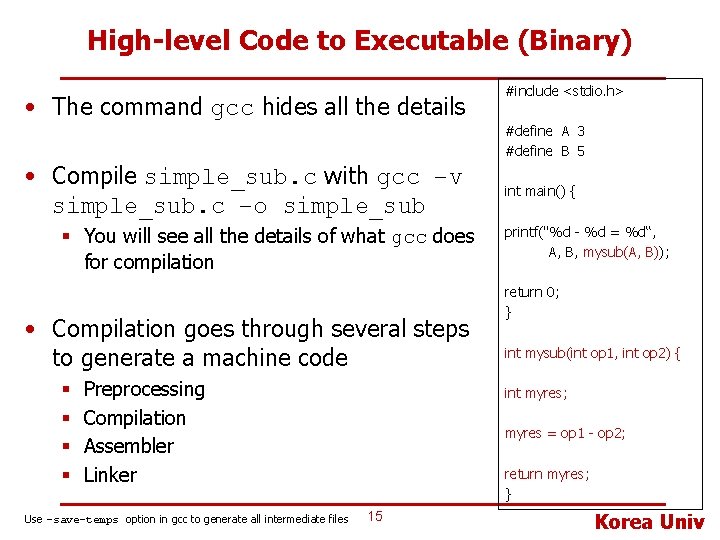
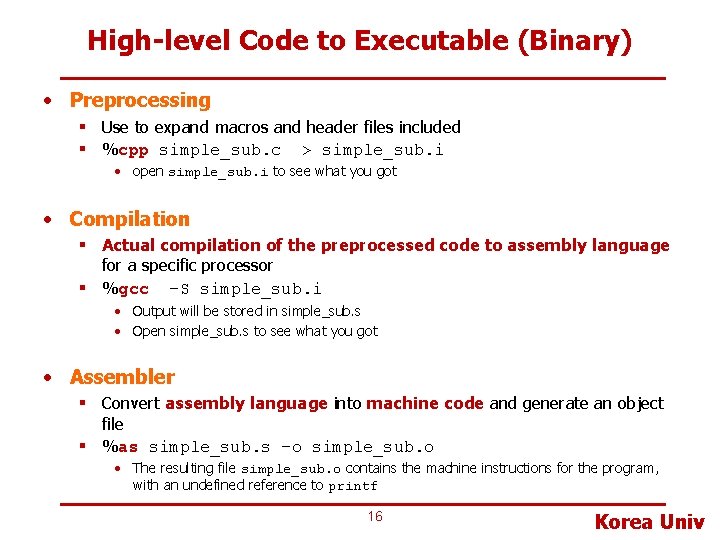
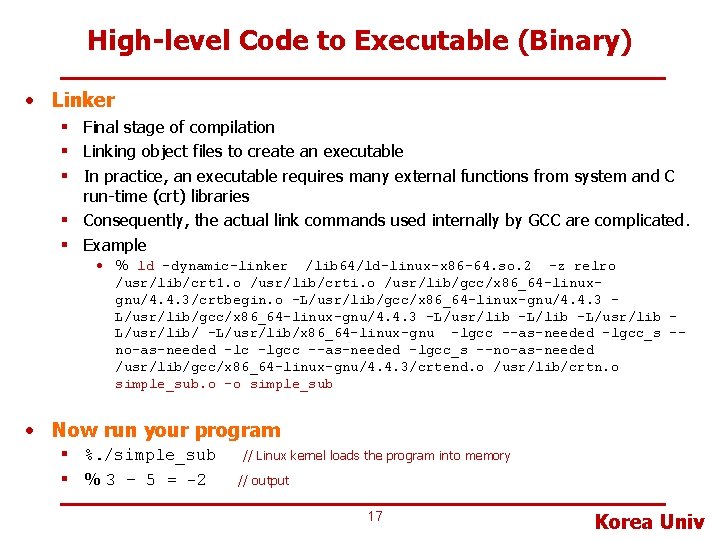
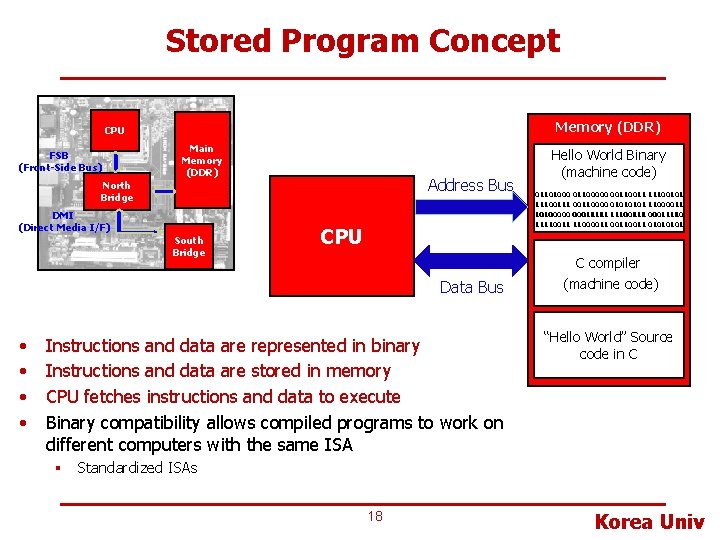
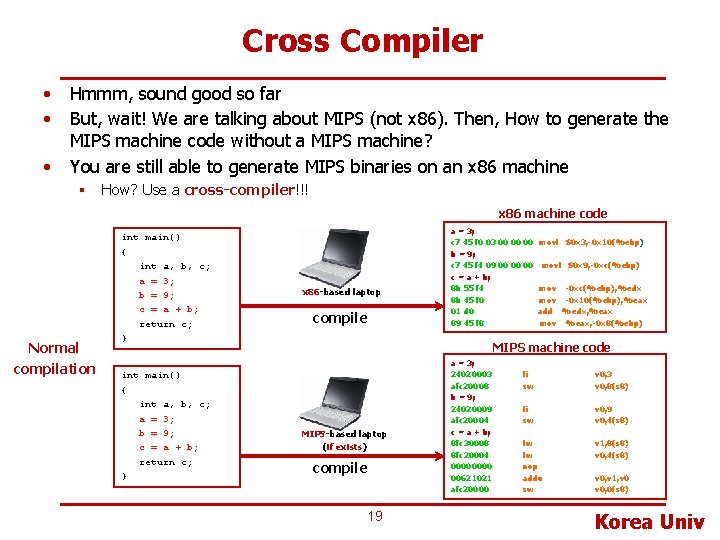
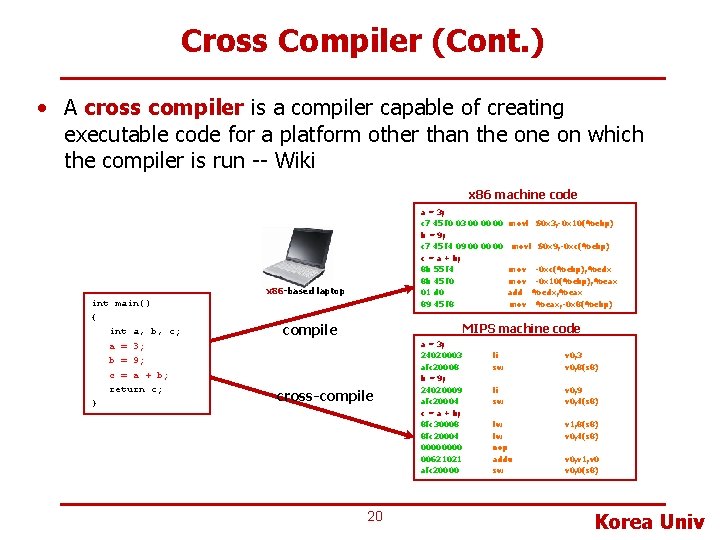
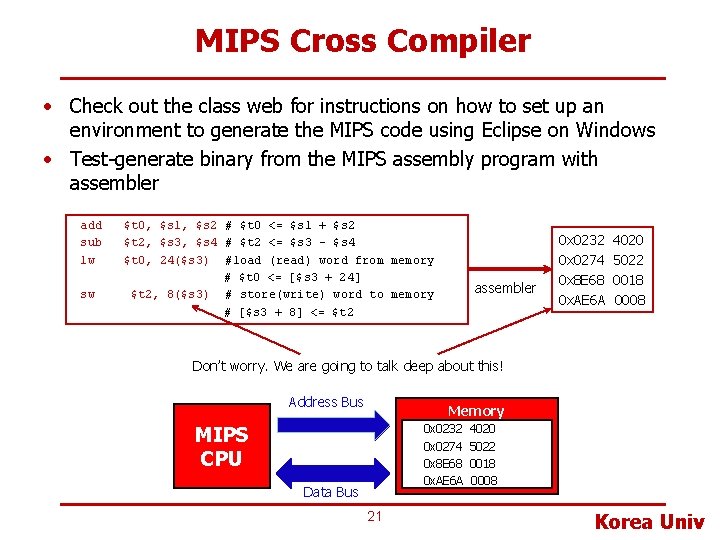
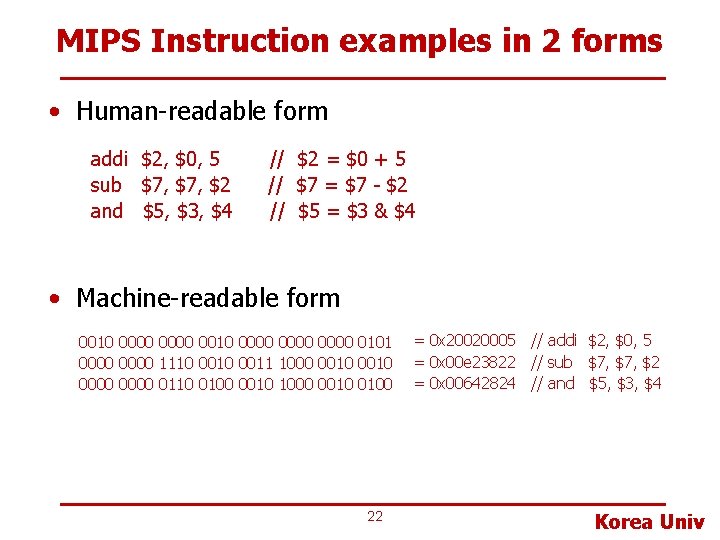
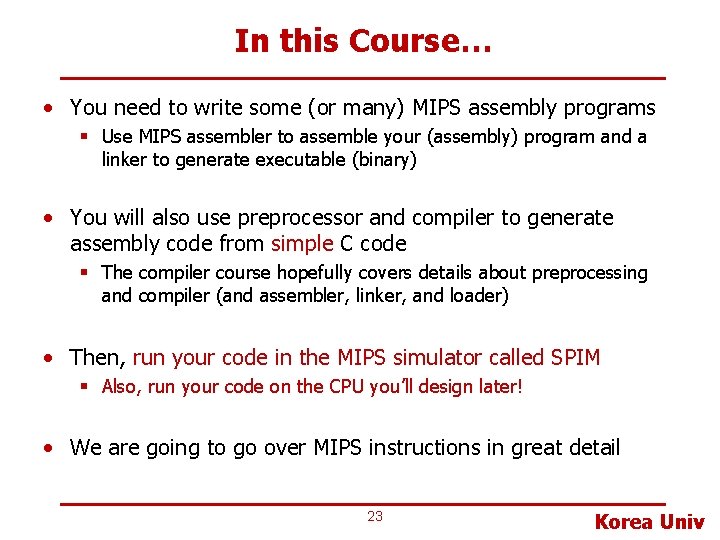
- Slides: 23
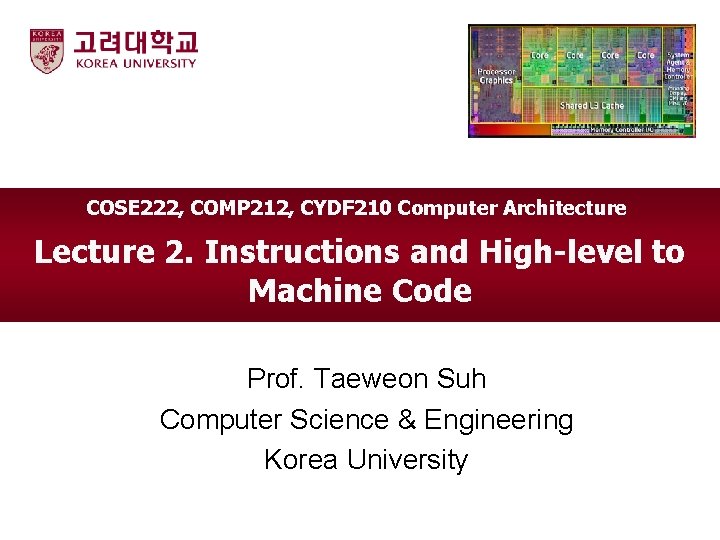
COSE 222, COMP 212, CYDF 210 Computer Architecture Lecture 2. Instructions and High-level to Machine Code Prof. Taeweon Suh Computer Science & Engineering Korea University
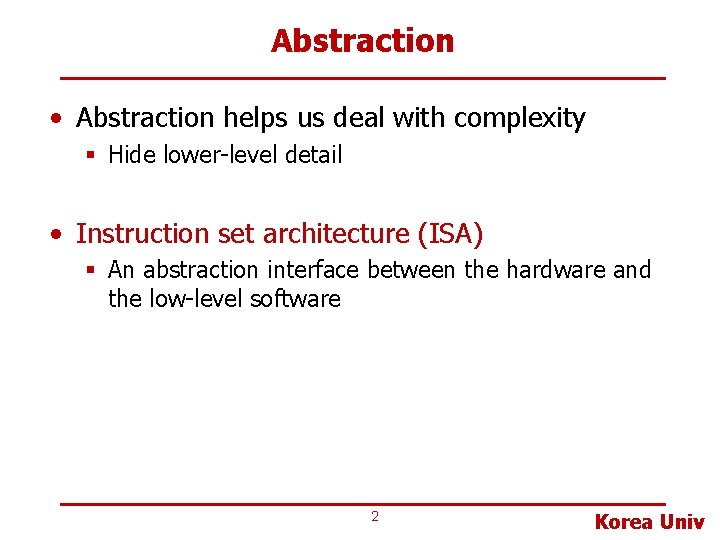
Abstraction • Abstraction helps us deal with complexity § Hide lower-level detail • Instruction set architecture (ISA) § An abstraction interface between the hardware and the low-level software 2 Korea Univ
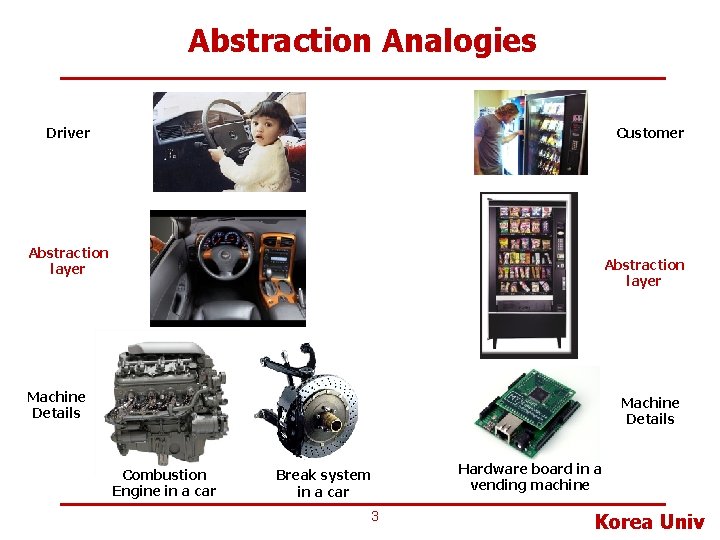
Abstraction Analogies Driver Customer Abstraction layer Machine Details Combustion Engine in a car Break system in a car 3 Hardware board in a vending machine Korea Univ
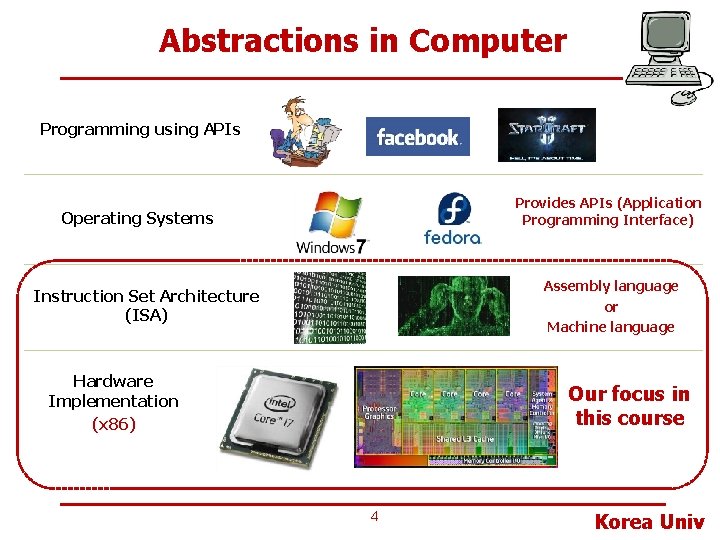
Abstractions in Computer Programming using APIs Provides APIs (Application Programming Interface) Operating Systems Assembly language or Machine language Instruction Set Architecture (ISA) Hardware Implementation (x 86) Our focus in this course 4 Korea Univ
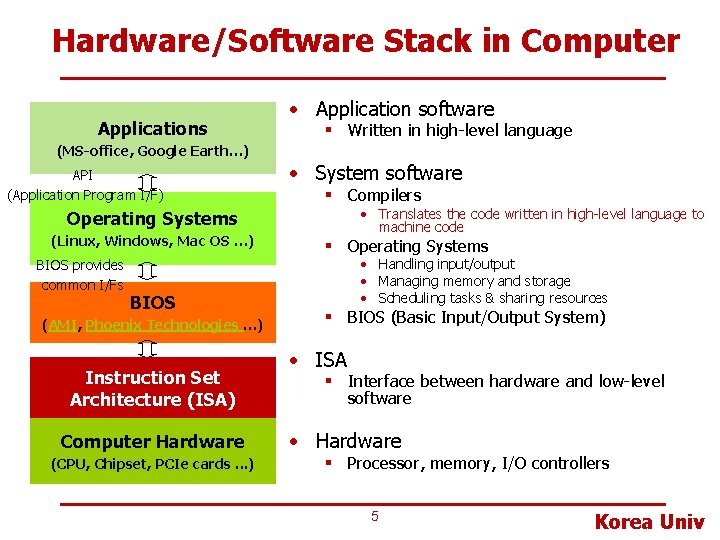
Hardware/Software Stack in Computer Applications • Application software § Written in high-level language (MS-office, Google Earth…) API (Application Program I/F) • System software § Compilers • Translates the code written in high-level language to machine code Operating Systems (Linux, Windows, Mac OS …) BIOS provides common I/Fs BIOS (AMI, Phoenix Technologies …) Instruction Set Architecture (ISA) Computer Hardware (CPU, Chipset, PCIe cards. . . ) § Operating Systems • Handling input/output • Managing memory and storage • Scheduling tasks & sharing resources § BIOS (Basic Input/Output System) • ISA § Interface between hardware and low-level software • Hardware § Processor, memory, I/O controllers 5 Korea Univ
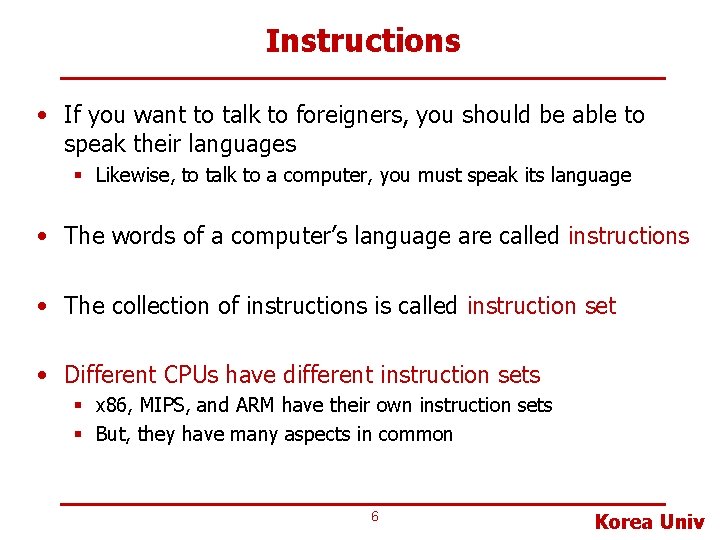
Instructions • If you want to talk to foreigners, you should be able to speak their languages § Likewise, to talk to a computer, you must speak its language • The words of a computer’s language are called instructions • The collection of instructions is called instruction set • Different CPUs have different instruction sets § x 86, MIPS, and ARM have their own instruction sets § But, they have many aspects in common 6 Korea Univ
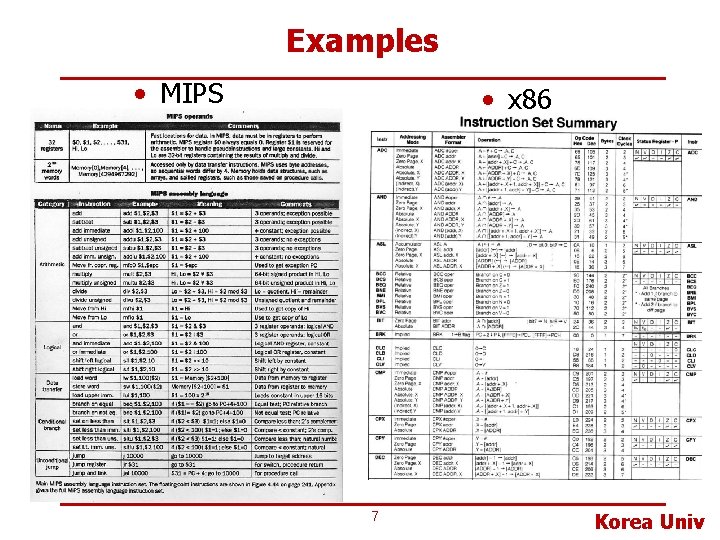
Examples • MIPS • x 86 7 Korea Univ
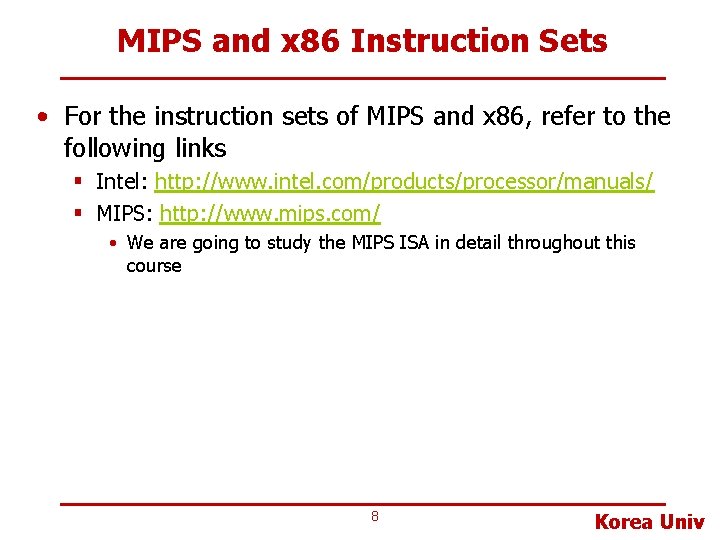
MIPS and x 86 Instruction Sets • For the instruction sets of MIPS and x 86, refer to the following links § Intel: http: //www. intel. com/products/processor/manuals/ § MIPS: http: //www. mips. com/ • We are going to study the MIPS ISA in detail throughout this course 8 Korea Univ
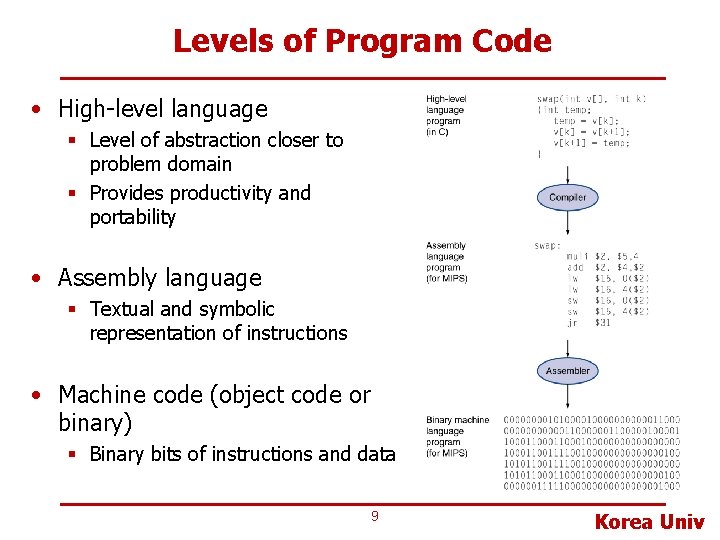
Levels of Program Code • High-level language § Level of abstraction closer to problem domain § Provides productivity and portability • Assembly language § Textual and symbolic representation of instructions • Machine code (object code or binary) § Binary bits of instructions and data 9 Korea Univ
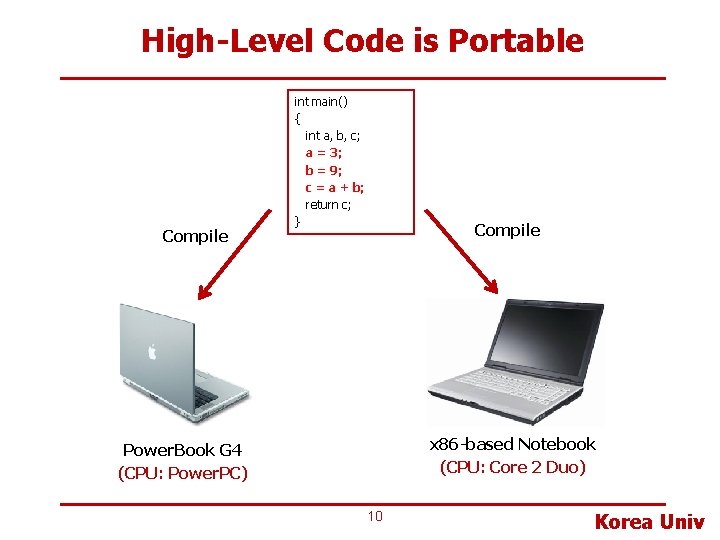
High-Level Code is Portable Compile int main() { int a, b, c; a = 3; b = 9; c = a + b; return c; } Compile x 86 -based Notebook (CPU: Core 2 Duo) Power. Book G 4 (CPU: Power. PC) 10 Korea Univ
![Levels of Program Code Highlevel language program in C swap int v int Levels of Program Code • High-level language program in C swap (int v[], int](https://slidetodoc.com/presentation_image_h2/a843d299a7a09898965d213aa0256165/image-11.jpg)
Levels of Program Code • High-level language program in C swap (int v[], int k) { int temp; temp = v[k]; v[k] = v[k+1]; v[k+1] = temp; } one-to-many • Assembly language program (MIPS) swap: sll add lw lw sw sw jr $2, $5, 2 $2, $4, $2 $15, 0($2) $16, 4($2) $16, 0($2) $15, 4($2) $31 C Compiler one-to-one • Machine (object, binary) code (MIPS) Assembler 000000 00101 00010000000 00100 0001000000100000. . . 11 Korea Univ
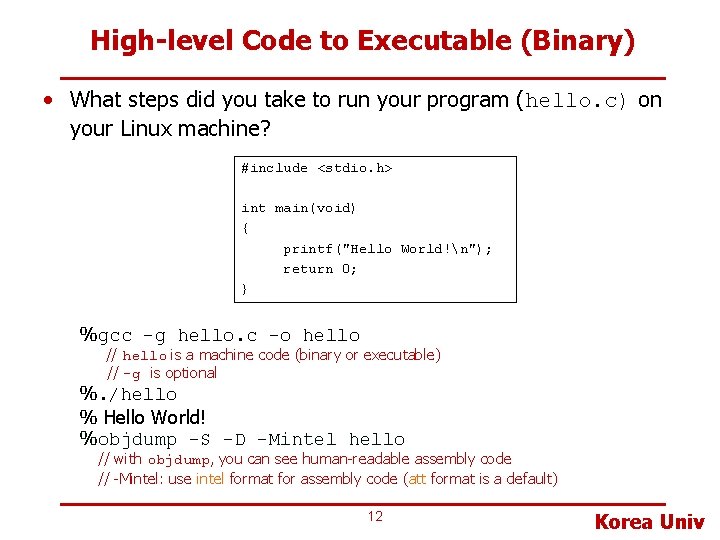
High-level Code to Executable (Binary) • What steps did you take to run your program (hello. c) on your Linux machine? #include <stdio. h> int main(void) { printf("Hello World!n"); return 0; } %gcc –g hello. c -o hello // hello is a machine code (binary or executable) // -g is optional %. /hello % Hello World! %objdump –S –D –Mintel hello // with objdump, you can see human-readable assembly code // -Mintel: use intel format for assembly code (att format is a default) 12 Korea Univ
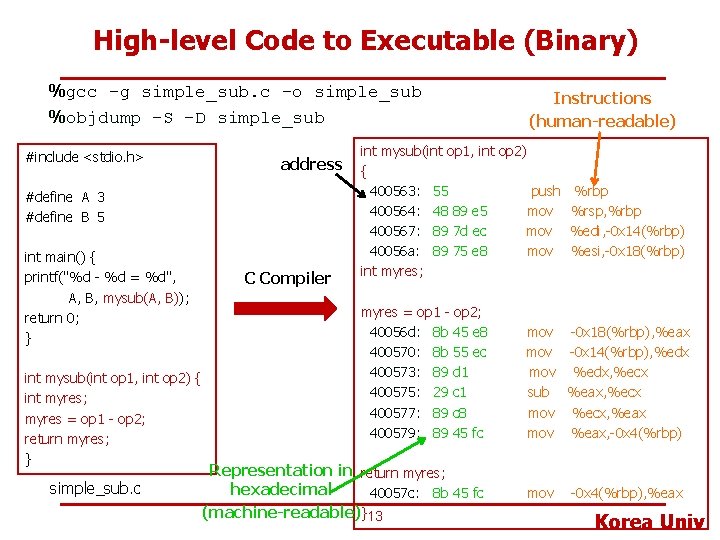
High-level Code to Executable (Binary) %gcc –g simple_sub. c -o simple_sub %objdump –S –D simple_sub #include <stdio. h> address #define A 3 #define B 5 int main() { printf("%d - %d = %d", A, B, mysub(A, B)); return 0; } int mysub(int op 1, int op 2) { int myres; myres = op 1 - op 2; return myres; } simple_sub. c C Compiler Instructions (human-readable) int mysub(int op 1, int op 2) { 400563: 55 push %rbp 400564: 48 89 e 5 mov %rsp, %rbp 400567: 89 7 d ec mov %edi, -0 x 14(%rbp) 40056 a: 89 75 e 8 mov %esi, -0 x 18(%rbp) int myres; myres = op 1 - op 2; 40056 d: 8 b 45 e 8 400570: 8 b 55 ec 400573: 89 d 1 400575: 29 c 1 400577: 89 c 8 400579: 89 45 fc Representation in return myres; hexadecimal 40057 c: 8 b 45 fc (machine-readable)}13 mov -0 x 18(%rbp), %eax mov -0 x 14(%rbp), %edx mov %edx, %ecx sub %eax, %ecx mov %ecx, %eax mov %eax, -0 x 4(%rbp) mov -0 x 4(%rbp), %eax Korea Univ
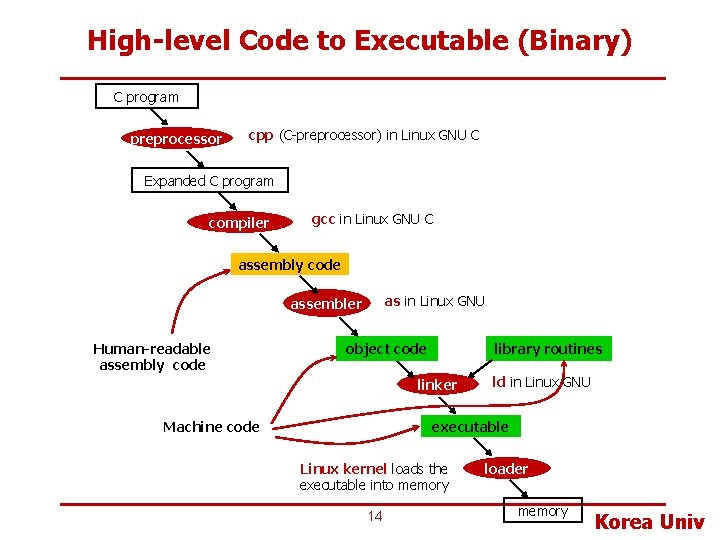
High-level Code to Executable (Binary) C program preprocessor cpp (C-preprocessor) in Linux GNU C Expanded C program compiler gcc in Linux GNU C assembly code as in Linux GNU assembler Human-readable assembly code object code library routines linker ld in Linux GNU executable Machine code Linux kernel loads the executable into memory 14 loader memory Korea Univ
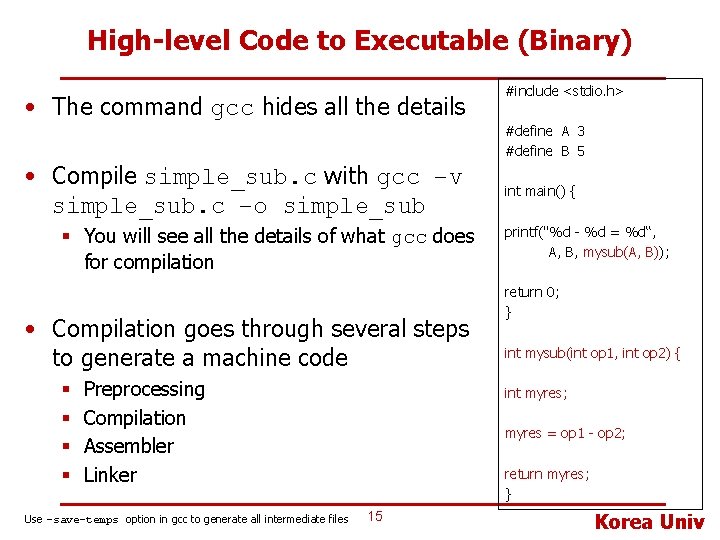
High-level Code to Executable (Binary) • The command gcc hides all the details • Compile simple_sub. c with gcc –v simple_sub. c –o simple_sub § You will see all the details of what gcc does for compilation • Compilation goes through several steps to generate a machine code § § Preprocessing Compilation Assembler Linker Use –save-temps option in gcc to generate all intermediate files #include <stdio. h> #define A 3 #define B 5 int main() { printf("%d - %d = %d“, A, B, mysub(A, B)); return 0; } int mysub(int op 1, int op 2) { int myres; myres = op 1 - op 2; return myres; } 15 Korea Univ
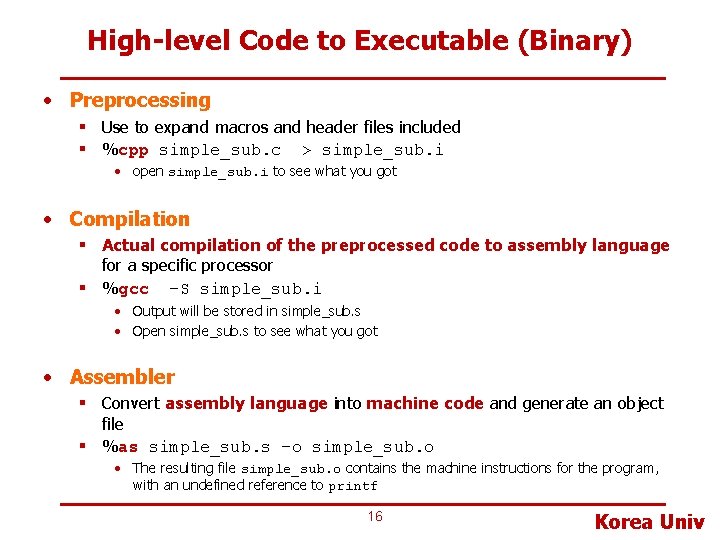
High-level Code to Executable (Binary) • Preprocessing § Use to expand macros and header files included § %cpp simple_sub. c > simple_sub. i • open simple_sub. i to see what you got • Compilation § Actual compilation of the preprocessed code to assembly language for a specific processor § %gcc –S simple_sub. i • Output will be stored in simple_sub. s • Open simple_sub. s to see what you got • Assembler § Convert assembly language into machine code and generate an object file § %as simple_sub. s –o simple_sub. o • The resulting file simple_sub. o contains the machine instructions for the program, with an undefined reference to printf 16 Korea Univ
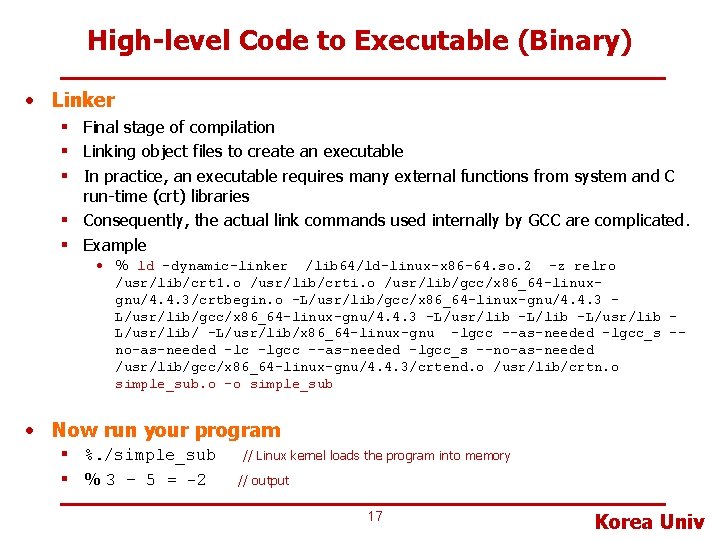
High-level Code to Executable (Binary) • Linker § Final stage of compilation § Linking object files to create an executable § In practice, an executable requires many external functions from system and C run-time (crt) libraries § Consequently, the actual link commands used internally by GCC are complicated. § Example • % ld -dynamic-linker /lib 64/ld-linux-x 86 -64. so. 2 -z relro /usr/lib/crt 1. o /usr/lib/crti. o /usr/lib/gcc/x 86_64 -linuxgnu/4. 4. 3/crtbegin. o -L/usr/lib/gcc/x 86_64 -linux-gnu/4. 4. 3 -L/usr/lib L/usr/lib/ -L/usr/lib/x 86_64 -linux-gnu -lgcc --as-needed -lgcc_s -no-as-needed -lc -lgcc --as-needed -lgcc_s --no-as-needed /usr/lib/gcc/x 86_64 -linux-gnu/4. 4. 3/crtend. o /usr/lib/crtn. o simple_sub. o -o simple_sub • Now run your program § %. /simple_sub § % 3 – 5 = -2 // Linux kernel loads the program into memory // output 17 Korea Univ
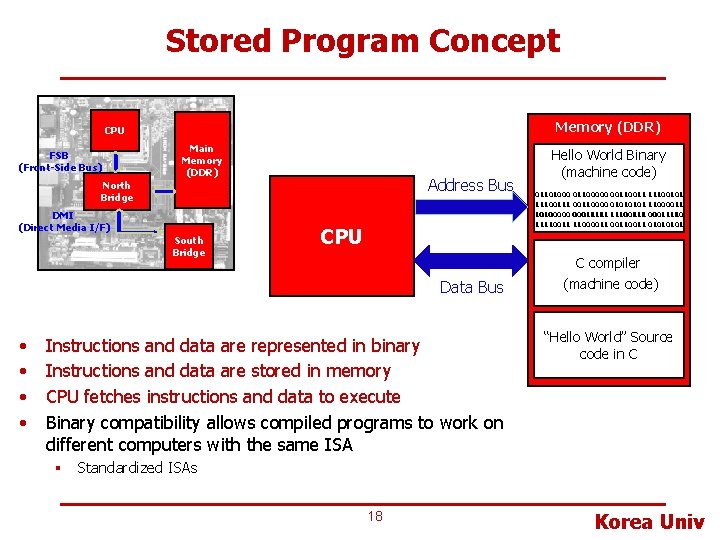
Stored Program Concept Memory (DDR) CPU FSB (Front-Side Bus) Main Memory (DDR) Address Bus North Bridge DMI (Direct Media I/F) South Bridge CPU Data Bus • • Instructions and data are represented in binary Instructions and data are stored in memory CPU fetches instructions and data to execute Binary compatibility allows compiled programs to work on different computers with the same ISA Hello World Binary (machine code) 01101000 01100000 0011 11100101 11100111 00110000 0101 11000011 10100000 00011111 11100111 00011110011 11000011 0101 C compiler (machine code) “Hello World” Source code in C § Standardized ISAs 18 Korea Univ
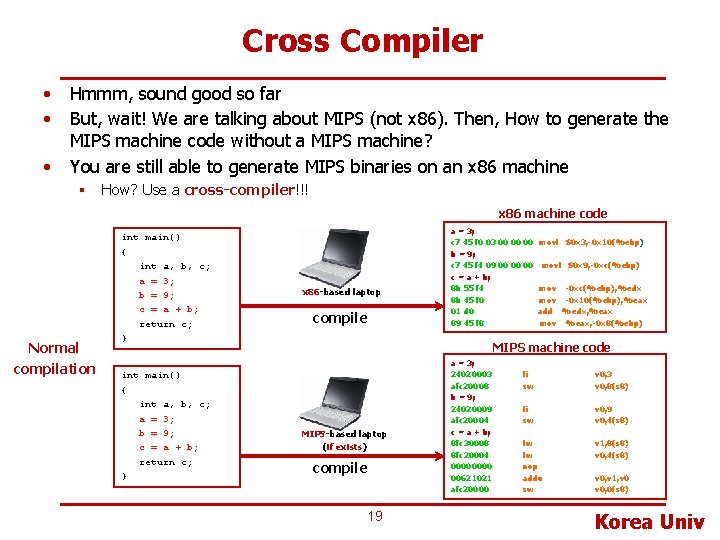
Cross Compiler • • • Hmmm, sound good so far But, wait! We are talking about MIPS (not x 86). Then, How to generate the MIPS machine code without a MIPS machine? You are still able to generate MIPS binaries on an x 86 machine § How? Use a cross-compiler!!! x 86 machine code Normal compilation int main() { int a, b, c; a = 3; b = 9; c = a + b; return c; } x 86 -based laptop compile a = 3; c 7 45 f 0 03 00 00 00 b = 9; c 7 45 f 4 09 00 00 00 c = a + b; 8 b 55 f 4 8 b 45 f 0 01 d 0 89 45 f 8 movl $0 x 3, -0 x 10(%ebp) movl $0 x 9, -0 xc(%ebp) mov -0 xc(%ebp), %edx mov -0 x 10(%ebp), %eax add %edx, %eax mov %eax, -0 x 8(%ebp) MIPS machine code MIPS-based laptop (if exists) compile 19 a = 3; 24020003 afc 20008 b = 9; 24020009 afc 20004 c = a + b; 8 fc 30008 8 fc 20004 0000 00621021 afc 20000 li sw v 0, 3 v 0, 8(s 8) li sw v 0, 9 v 0, 4(s 8) lw lw nop addu sw v 1, 8(s 8) v 0, 4(s 8) v 0, v 1, v 0, 0(s 8) Korea Univ
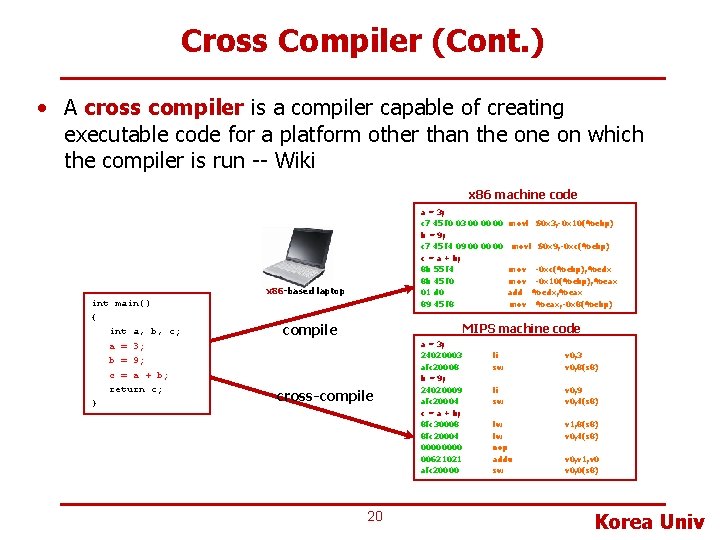
Cross Compiler (Cont. ) • A cross compiler is a compiler capable of creating executable code for a platform other than the on which the compiler is run -- Wiki x 86 machine code a = 3; c 7 45 f 0 03 00 00 00 b = 9; c 7 45 f 4 09 00 00 00 c = a + b; 8 b 55 f 4 8 b 45 f 0 01 d 0 89 45 f 8 x 86 -based laptop int main() { int a, b, c; a = 3; b = 9; c = a + b; return c; } compile movl $0 x 3, -0 x 10(%ebp) movl $0 x 9, -0 xc(%ebp) mov -0 xc(%ebp), %edx mov -0 x 10(%ebp), %eax add %edx, %eax mov %eax, -0 x 8(%ebp) MIPS machine code cross-compile 20 a = 3; 24020003 afc 20008 b = 9; 24020009 afc 20004 c = a + b; 8 fc 30008 8 fc 20004 0000 00621021 afc 20000 li sw v 0, 3 v 0, 8(s 8) li sw v 0, 9 v 0, 4(s 8) lw lw nop addu sw v 1, 8(s 8) v 0, 4(s 8) v 0, v 1, v 0, 0(s 8) Korea Univ
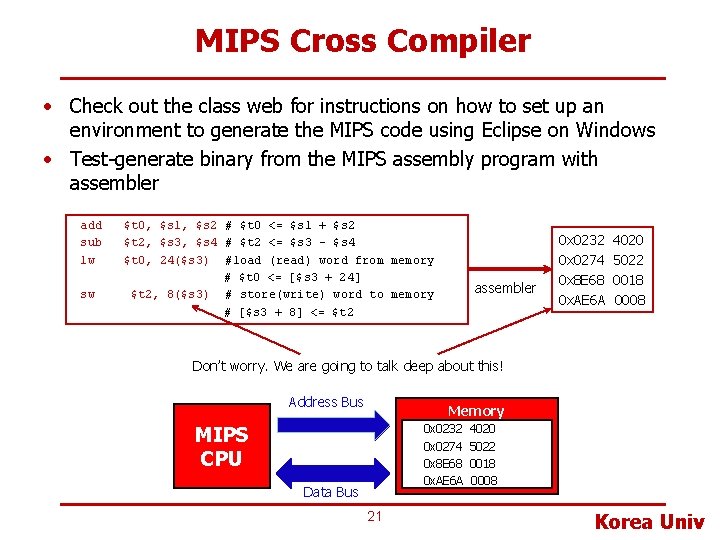
MIPS Cross Compiler • Check out the class web for instructions on how to set up an environment to generate the MIPS code using Eclipse on Windows • Test-generate binary from the MIPS assembly program with assembler add sub lw sw $t 0, $s 1, $s 2 # $t 0 <= $s 1 + $s 2 $t 2, $s 3, $s 4 # $t 2 <= $s 3 - $s 4 $t 0, 24($s 3) #load (read) word from memory # $t 0 <= [$s 3 + 24] $t 2, 8($s 3) # store(write) word to memory # [$s 3 + 8] <= $t 2 assembler 0 x 0232 0 x 0274 0 x 8 E 68 0 x. AE 6 A 4020 5022 0018 0008 Don’t worry. We are going to talk deep about this! Address Bus Memory 0 x 0232 0 x 0274 0 x 8 E 68 0 x. AE 6 A MIPS CPU Data Bus 21 4020 5022 0018 0008 Korea Univ
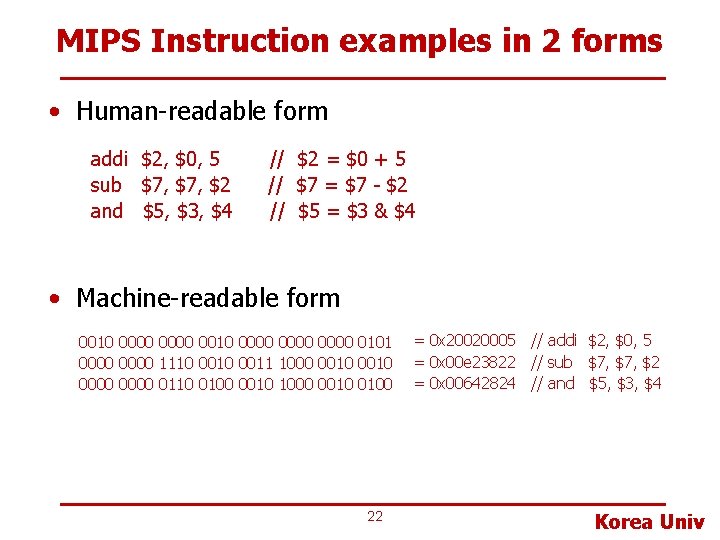
MIPS Instruction examples in 2 forms • Human-readable form addi $2, $0, 5 sub $7, $2 and $5, $3, $4 // $2 = $0 + 5 // $7 = $7 - $2 // $5 = $3 & $4 • Machine-readable form 0010 0000 0000 0101 0000 1110 0011 1000 0010 0000 0110 0100 0010 1000 0010 0100 22 = 0 x 20020005 // addi $2, $0, 5 = 0 x 00 e 23822 // sub $7, $2 = 0 x 00642824 // and $5, $3, $4 Korea Univ
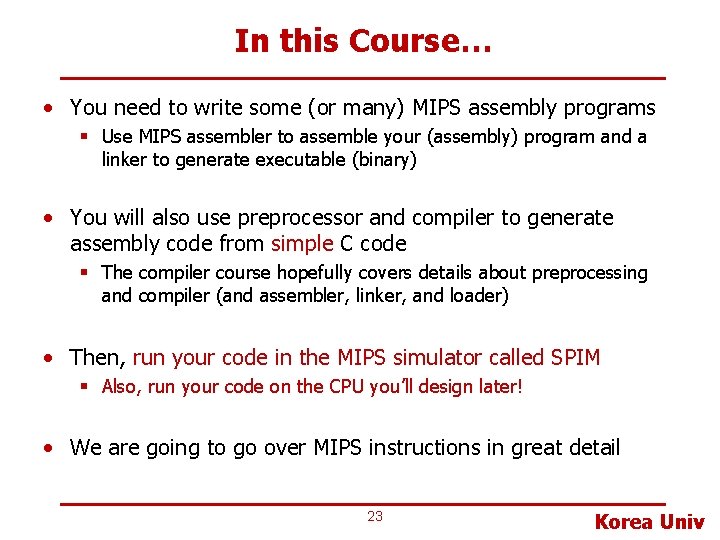
In this Course… • You need to write some (or many) MIPS assembly programs § Use MIPS assembler to assemble your (assembly) program and a linker to generate executable (binary) • You will also use preprocessor and compiler to generate assembly code from simple C code § The compiler course hopefully covers details about preprocessing and compiler (and assembler, linker, and loader) • Then, run your code in the MIPS simulator called SPIM § Also, run your code on the CPU you’ll design later! • We are going to go over MIPS instructions in great detail 23 Korea Univ
210 210 210
Dns 208 67 222 222
Le cose importanti della vita non sono cose
Bus architecture in computer architecture
Difference between computer organization and architecture
Interrupt cycle flow chart
Xxaxis matlab 222
Pineer species
Section 521.222 (d)(2) transportation code
Império do jogo do bicho
Log 2
222 third street cambridge ma
Mism 222
Ece 222 github
Skillbridge navadmin
Infosys 222
Blk 222
Mide-222
Psalmul 6
Mekila 222
Htppec
Eco 222
Csce 221
Flosoft fs 222