Computer Science 209 Software Development Java File Processing
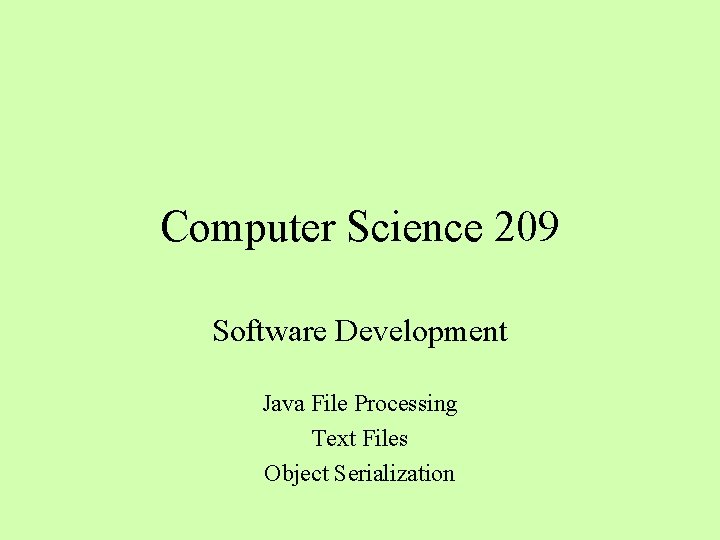
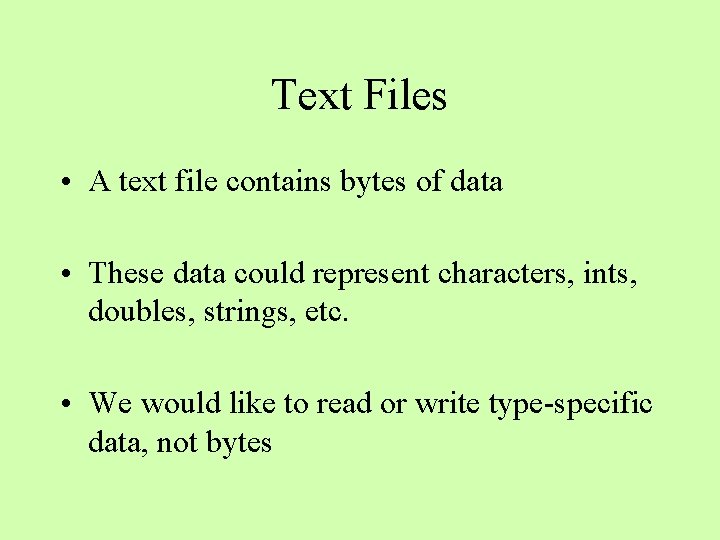
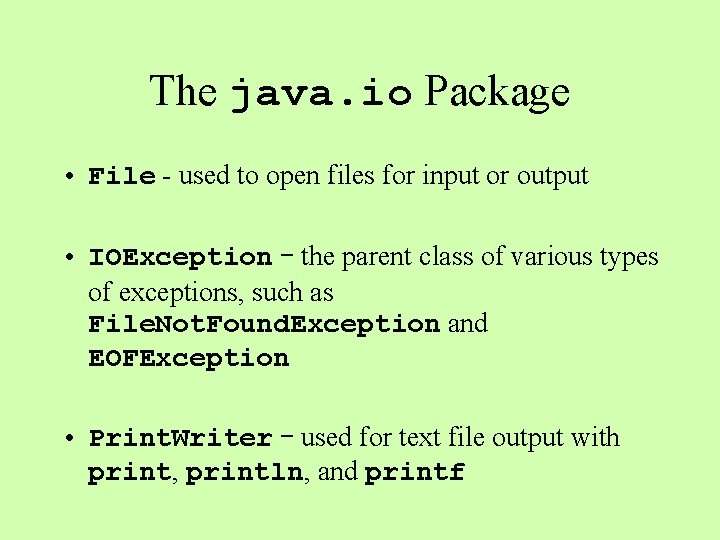
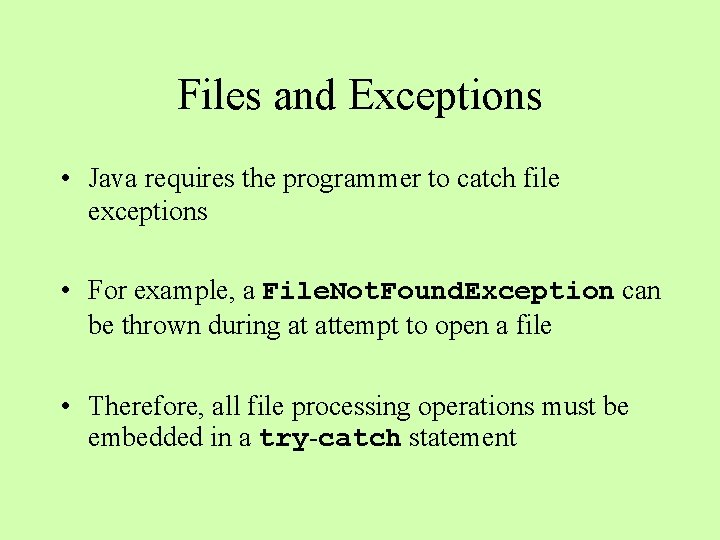
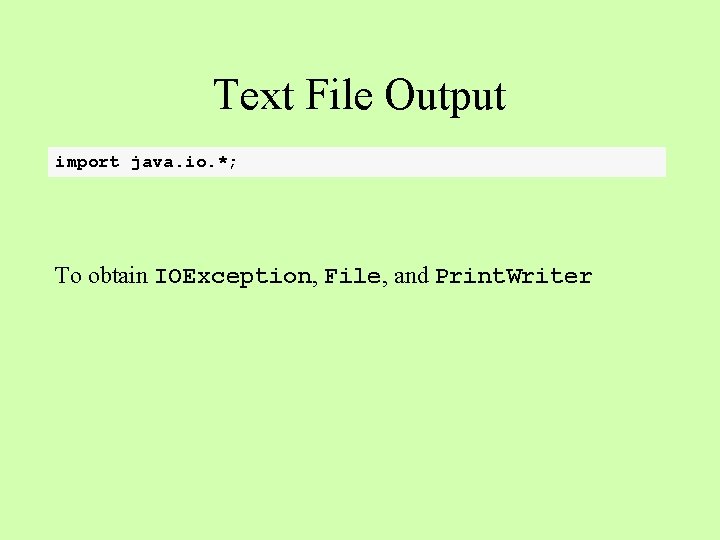
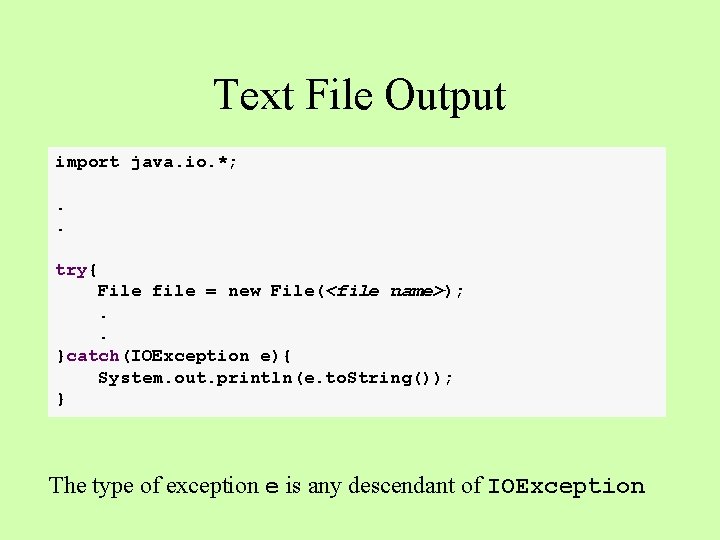
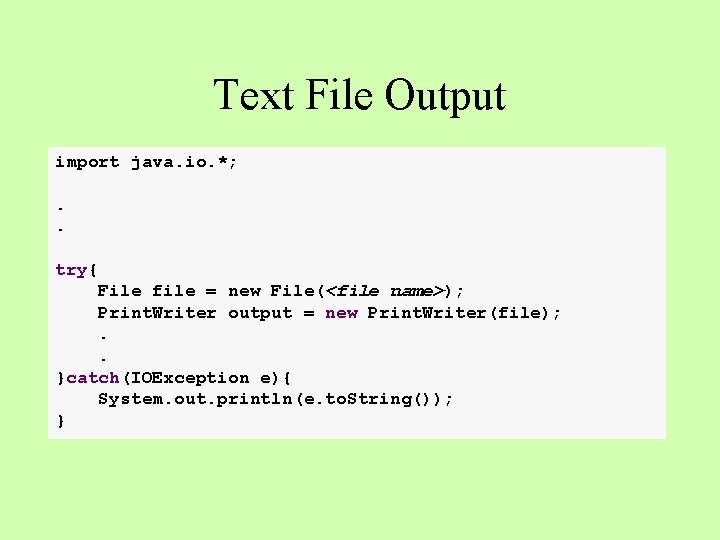
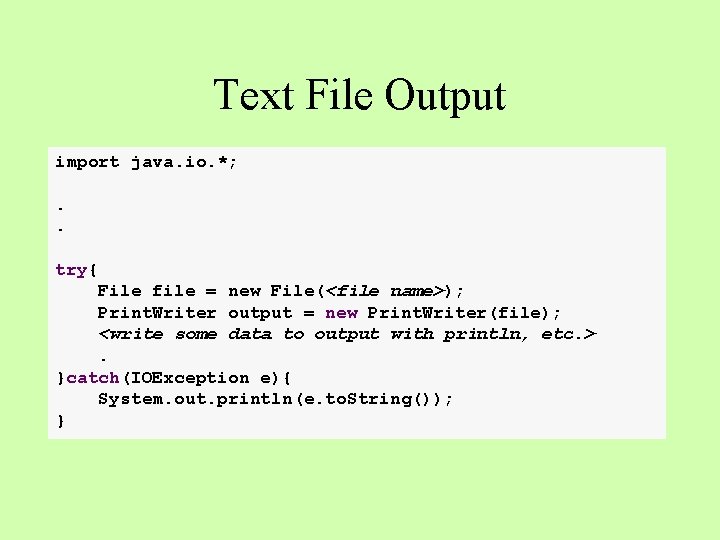
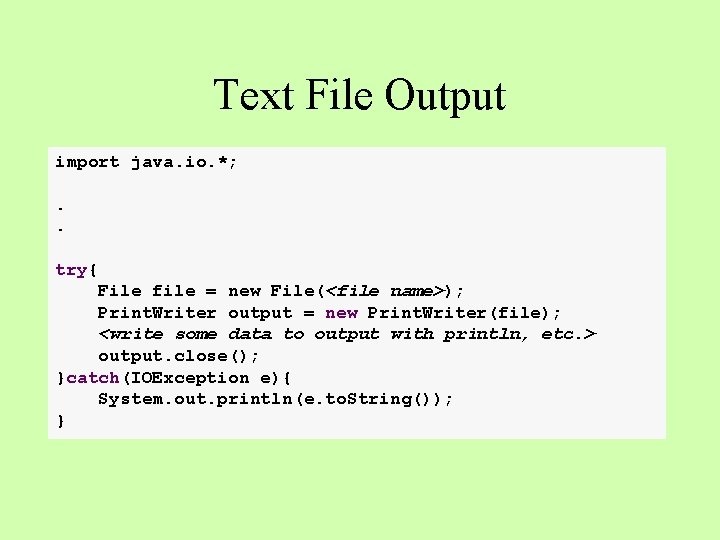
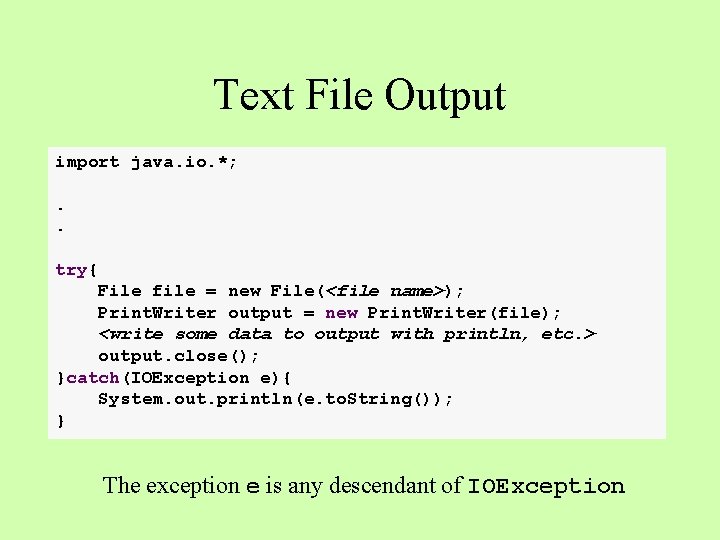
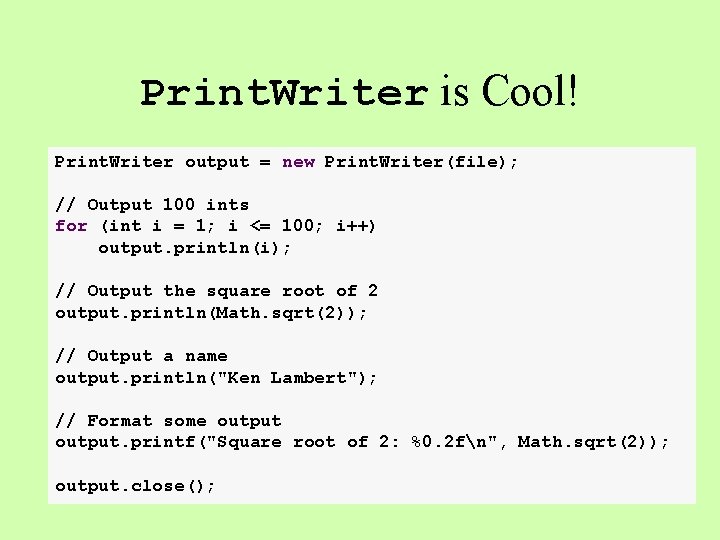
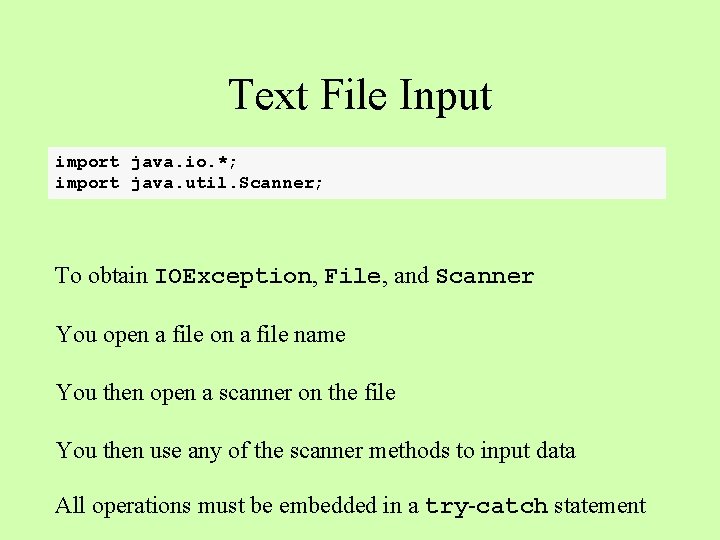
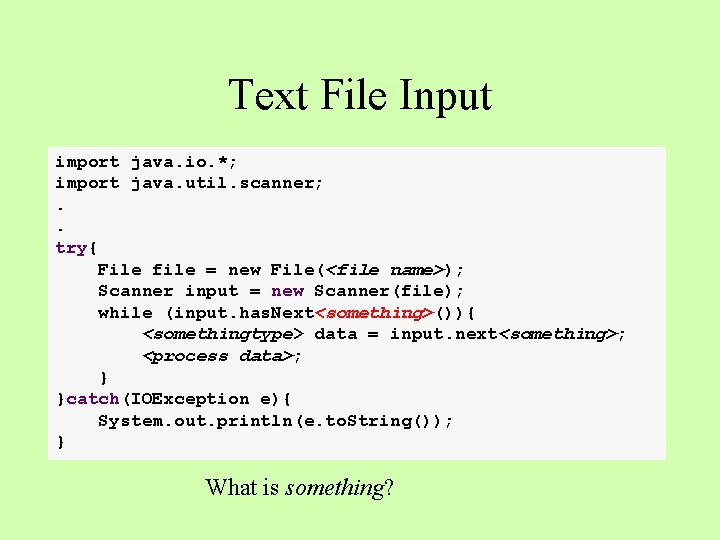
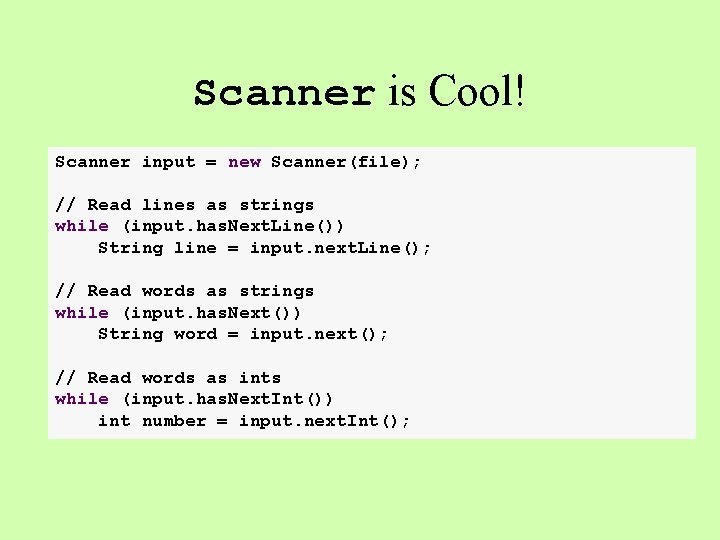
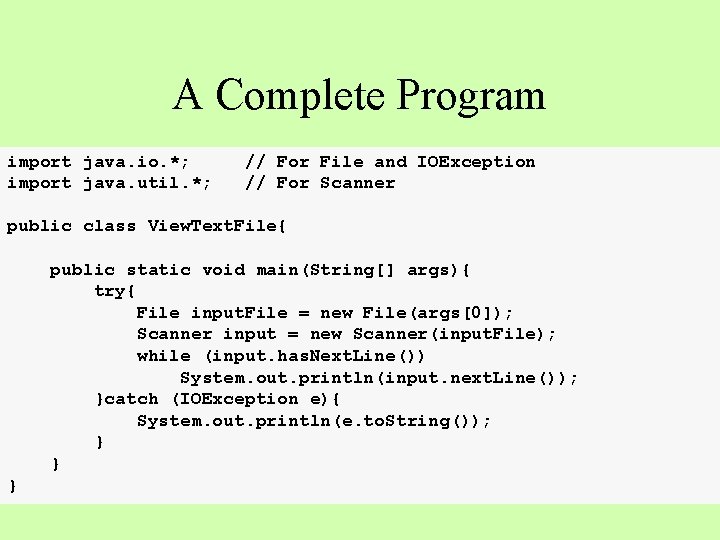
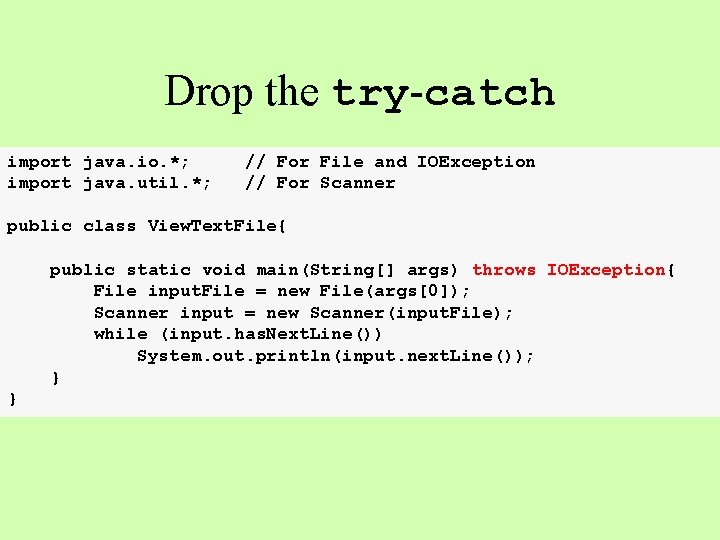
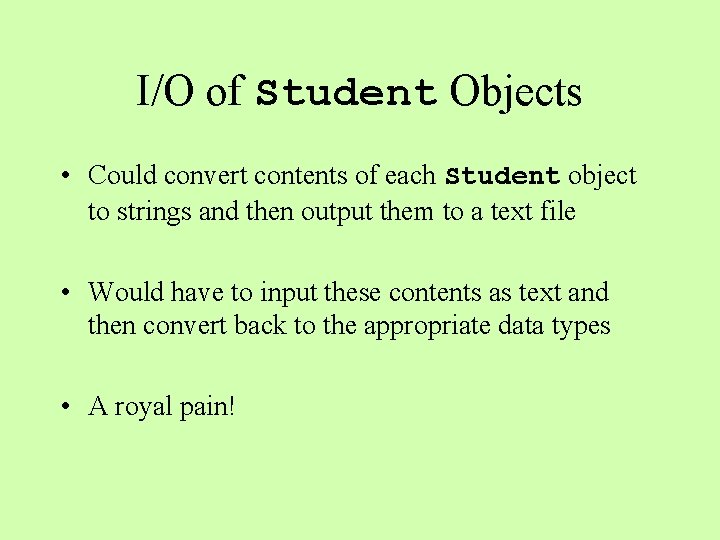
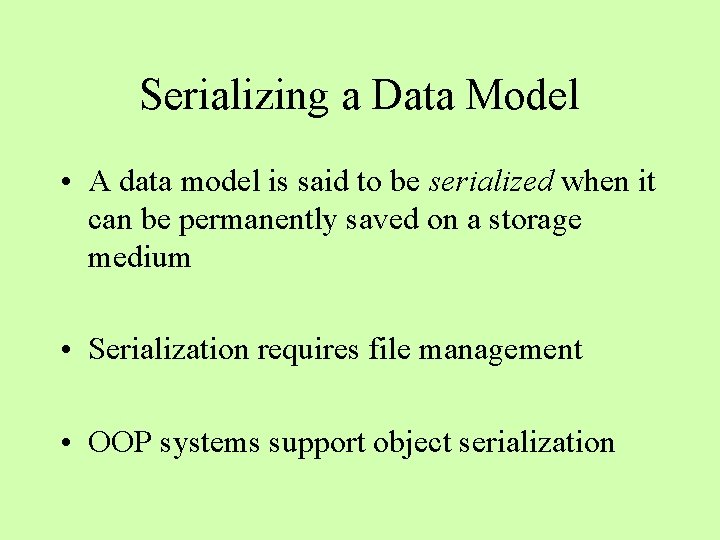
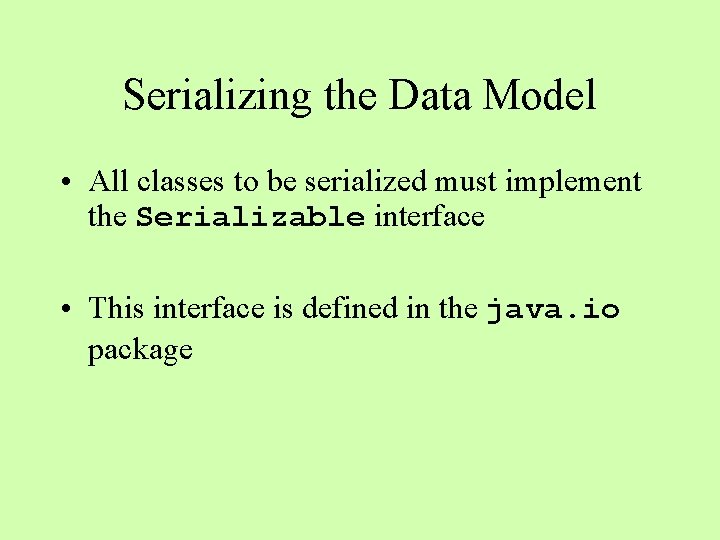
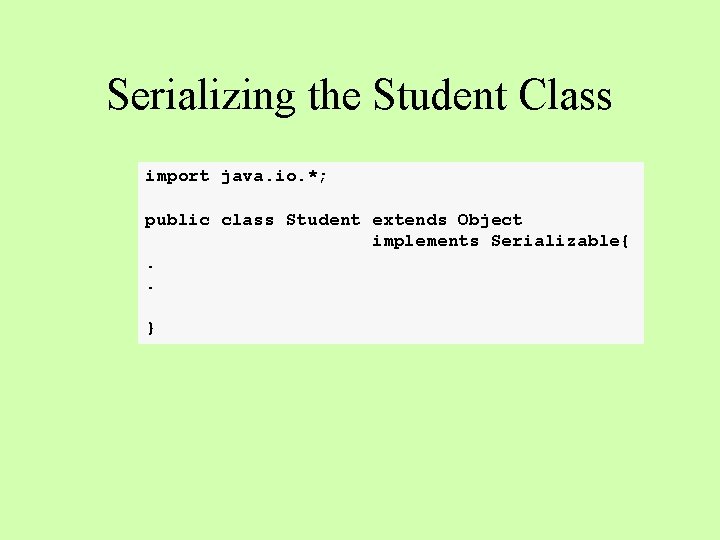
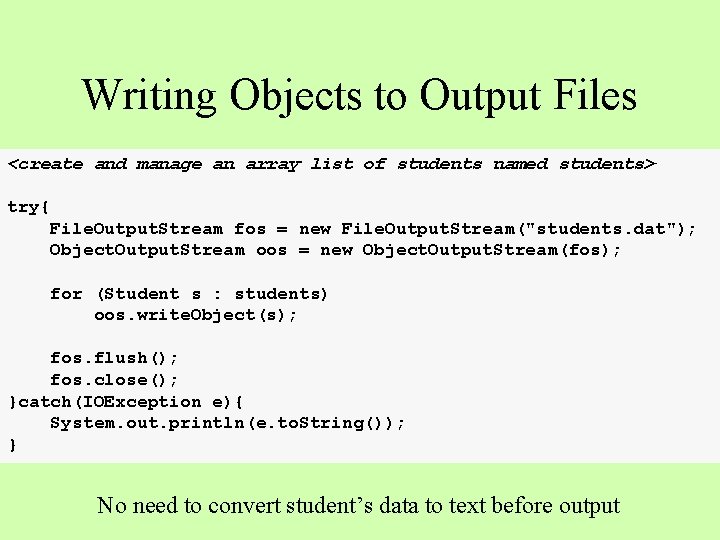
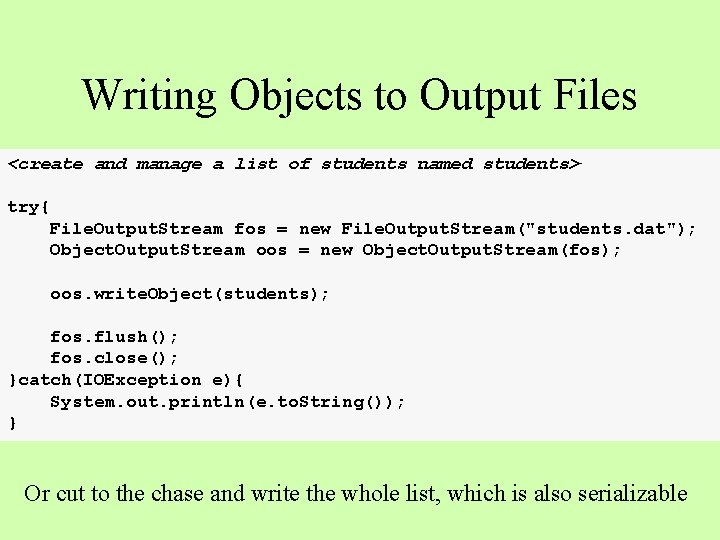
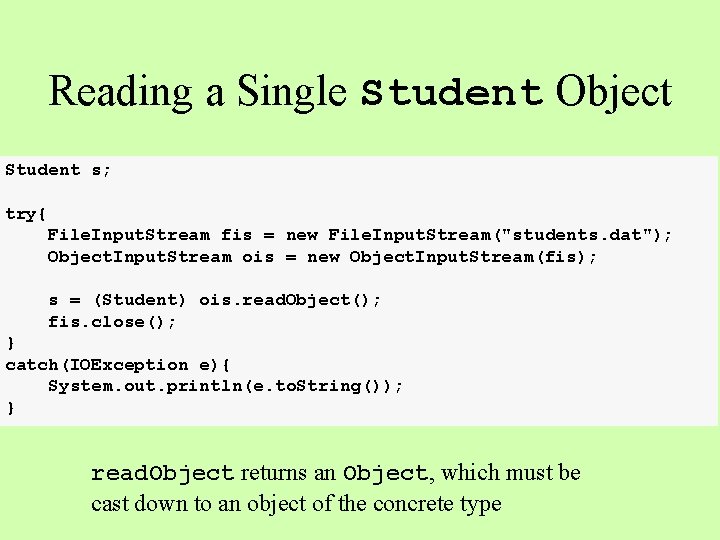
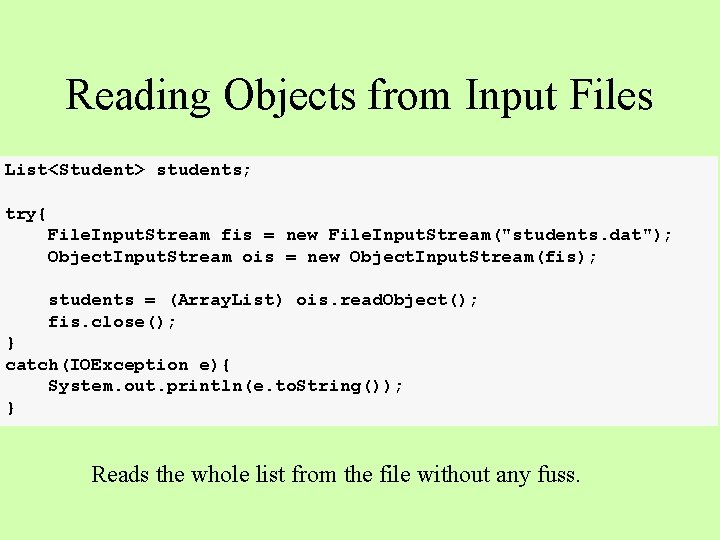
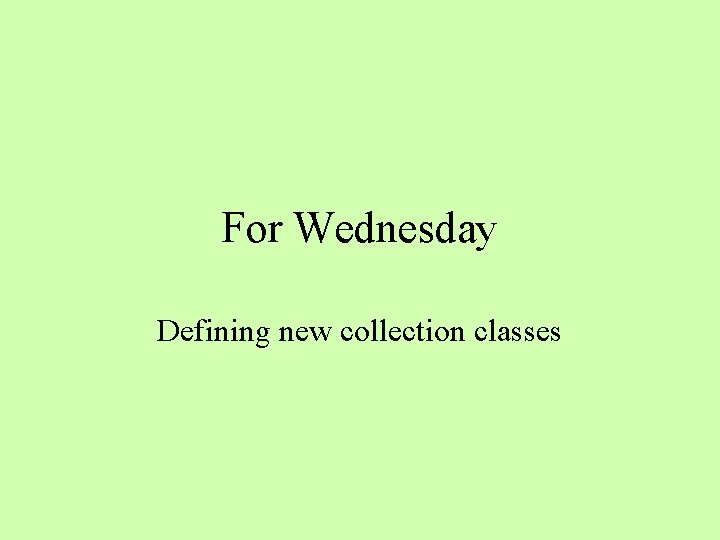
- Slides: 25
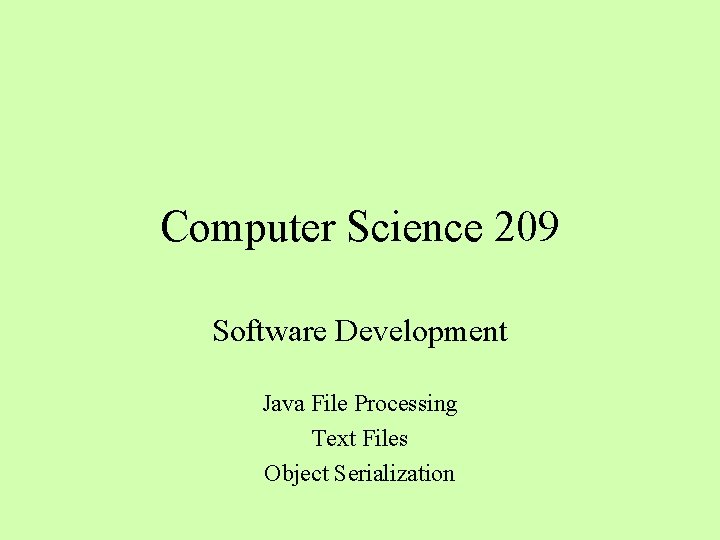
Computer Science 209 Software Development Java File Processing Text Files Object Serialization
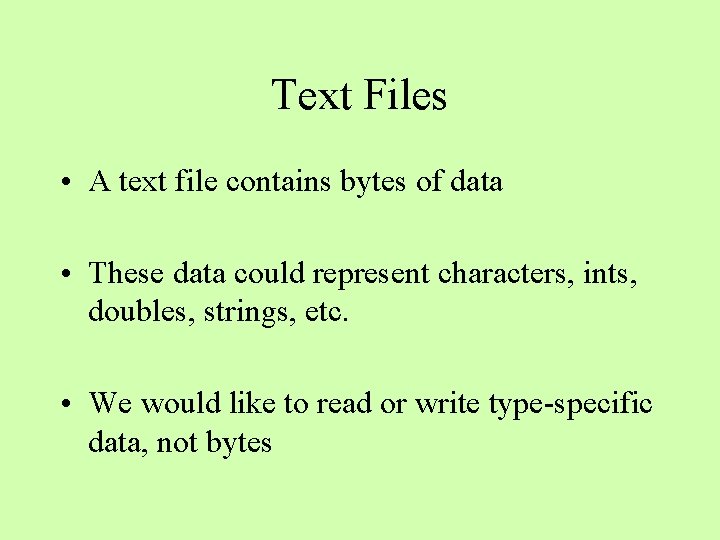
Text Files • A text file contains bytes of data • These data could represent characters, ints, doubles, strings, etc. • We would like to read or write type-specific data, not bytes
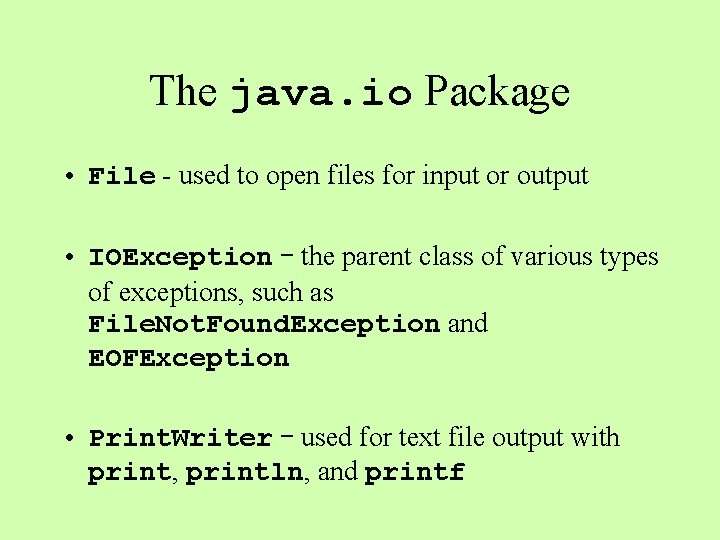
The java. io Package • File - used to open files for input or output • IOException – the parent class of various types of exceptions, such as File. Not. Found. Exception and EOFException • Print. Writer – used for text file output with print, println, and printf
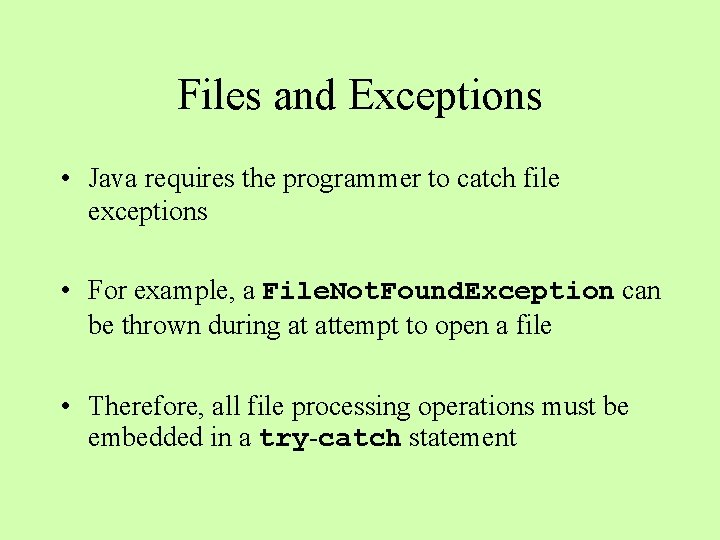
Files and Exceptions • Java requires the programmer to catch file exceptions • For example, a File. Not. Found. Exception can be thrown during at attempt to open a file • Therefore, all file processing operations must be embedded in a try-catch statement
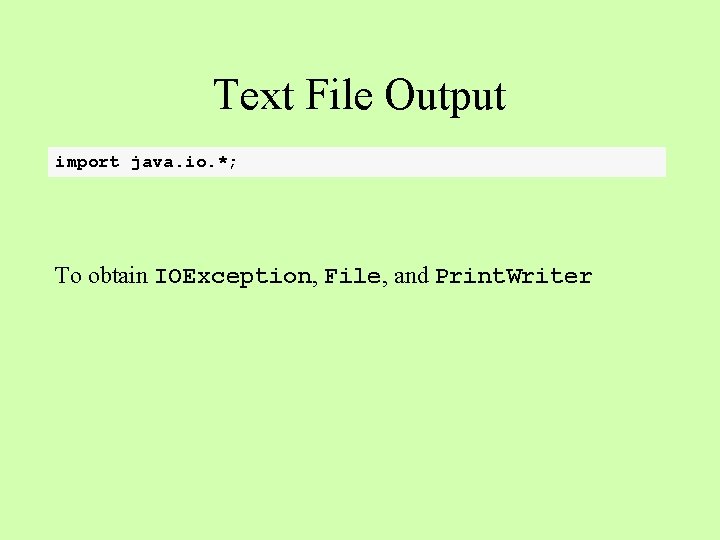
Text File Output import java. io. *; To obtain IOException, File, and Print. Writer
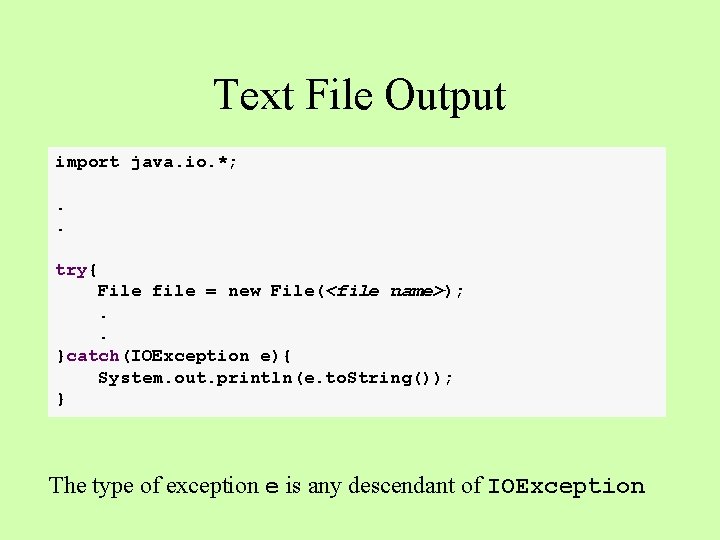
Text File Output import java. io. *; . . try{ File file = new File(<file name>); . . }catch(IOException e){ System. out. println(e. to. String()); } The type of exception e is any descendant of IOException
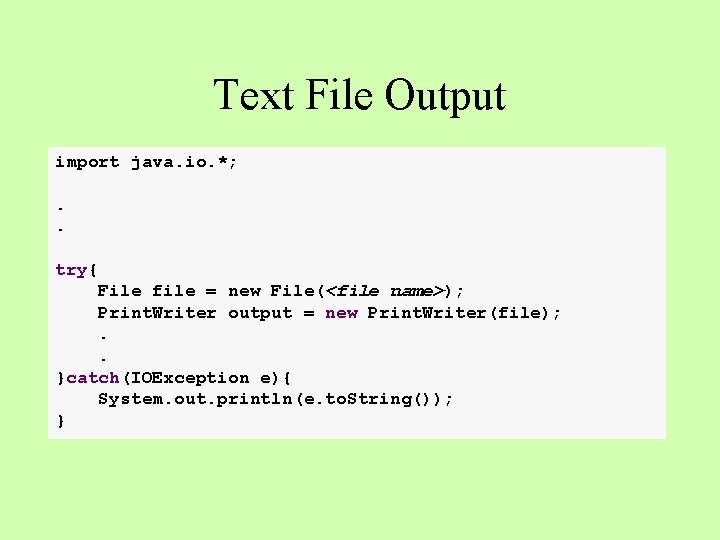
Text File Output import java. io. *; . . try{ File file = new File(<file name>); Print. Writer output = new Print. Writer(file); . . }catch(IOException e){ System. out. println(e. to. String()); }
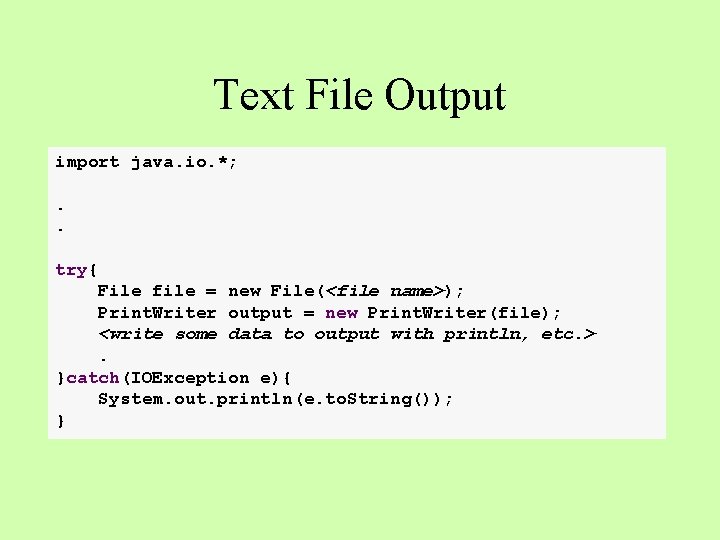
Text File Output import java. io. *; . . try{ File file = new File(<file name>); Print. Writer output = new Print. Writer(file); <write some data to output with println, etc. >. }catch(IOException e){ System. out. println(e. to. String()); }
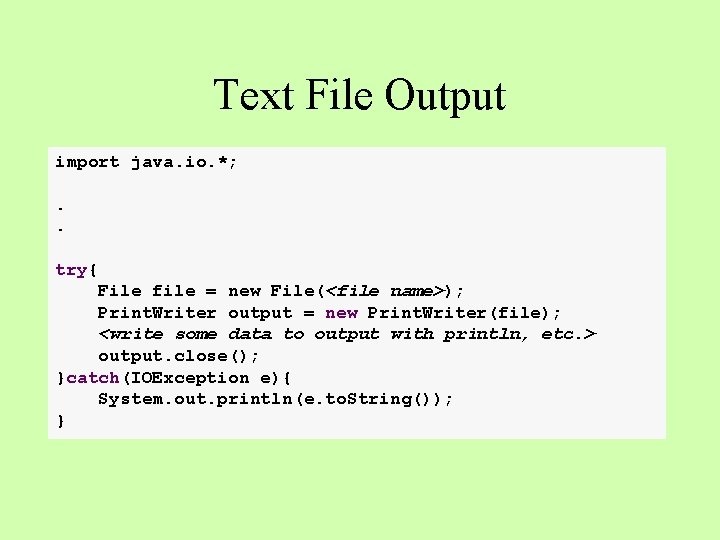
Text File Output import java. io. *; . . try{ File file = new File(<file name>); Print. Writer output = new Print. Writer(file); <write some data to output with println, etc. > output. close(); }catch(IOException e){ System. out. println(e. to. String()); }
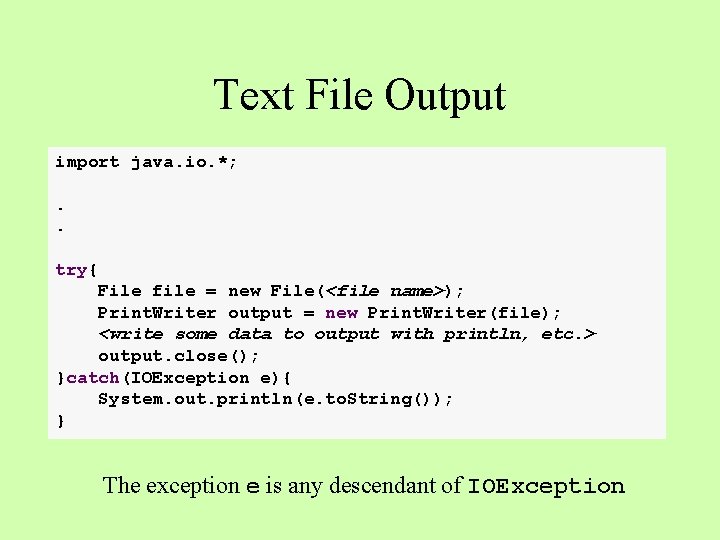
Text File Output import java. io. *; . . try{ File file = new File(<file name>); Print. Writer output = new Print. Writer(file); <write some data to output with println, etc. > output. close(); }catch(IOException e){ System. out. println(e. to. String()); } The exception e is any descendant of IOException
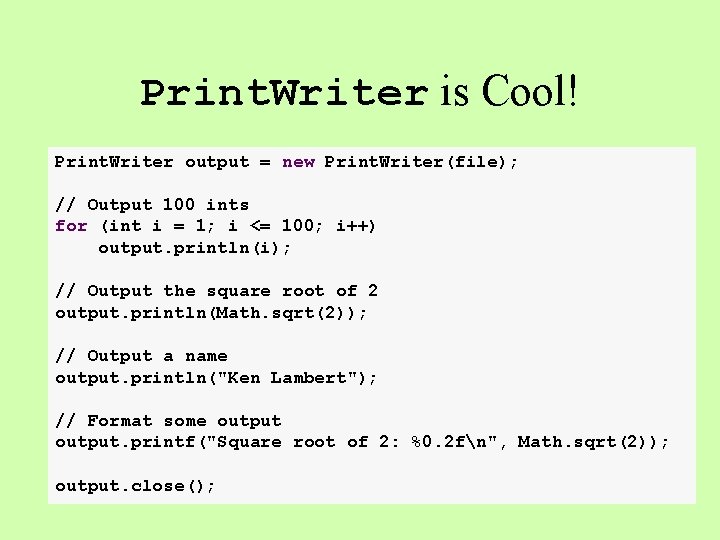
Print. Writer is Cool! Print. Writer output = new Print. Writer(file); // Output 100 ints for (int i = 1; i <= 100; i++) output. println(i); // Output the square root of 2 output. println(Math. sqrt(2)); // Output a name output. println("Ken Lambert"); // Format some output. printf("Square root of 2: %0. 2 fn", Math. sqrt(2)); output. close();
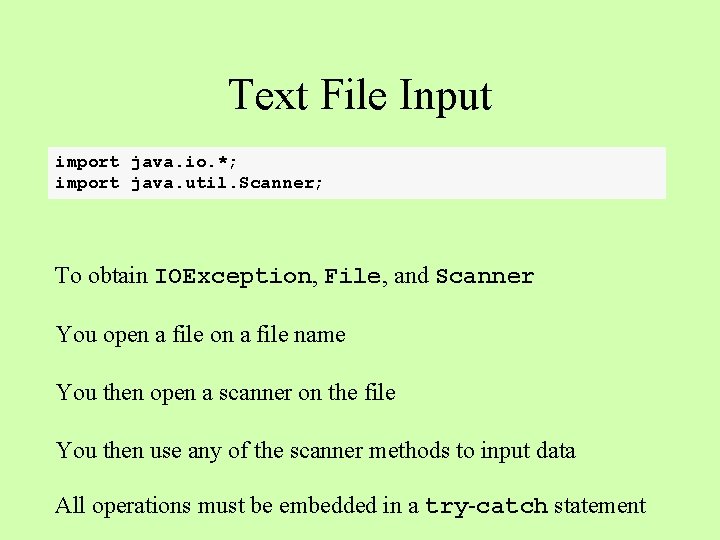
Text File Input import java. io. *; import java. util. Scanner; To obtain IOException, File, and Scanner You open a file on a file name You then open a scanner on the file You then use any of the scanner methods to input data All operations must be embedded in a try-catch statement
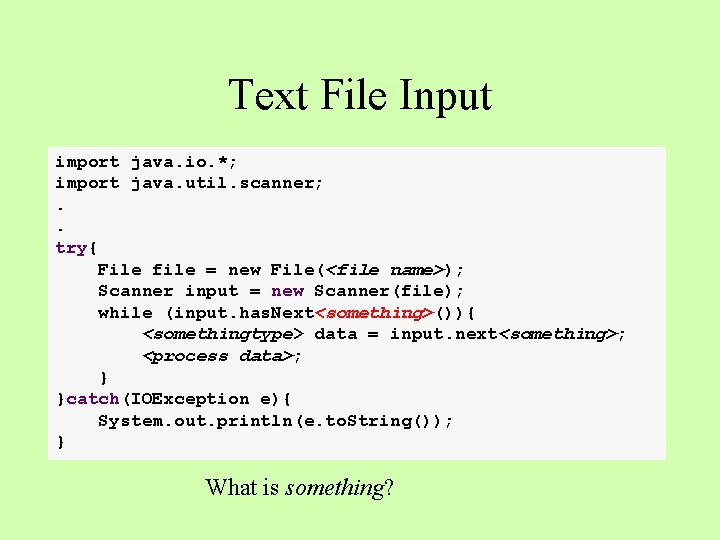
Text File Input import java. io. *; import java. util. scanner; . . try{ File file = new File(<file name>); Scanner input = new Scanner(file); while (input. has. Next<something>()){ <somethingtype> data = input. next<something>; <process data>; } }catch(IOException e){ System. out. println(e. to. String()); } What is something?
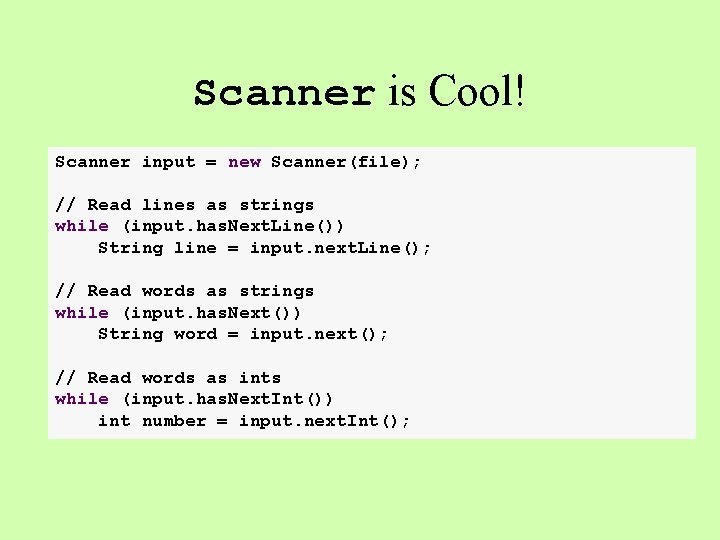
Scanner is Cool! Scanner input = new Scanner(file); // Read lines as strings while (input. has. Next. Line()) String line = input. next. Line(); // Read words as strings while (input. has. Next()) String word = input. next(); // Read words as ints while (input. has. Next. Int()) int number = input. next. Int();
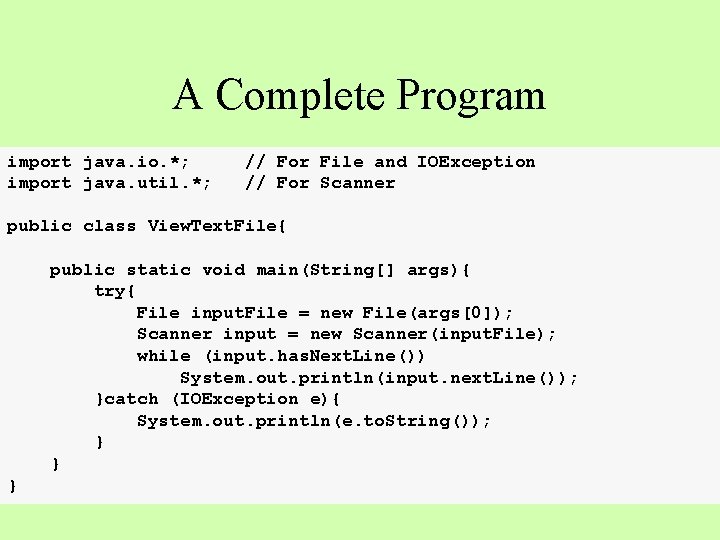
A Complete Program import java. io. *; import java. util. *; // For File and IOException // For Scanner public class View. Text. File{ public static void main(String[] args){ try{ File input. File = new File(args[0]); Scanner input = new Scanner(input. File); while (input. has. Next. Line()) System. out. println(input. next. Line()); }catch (IOException e){ System. out. println(e. to. String()); } } }
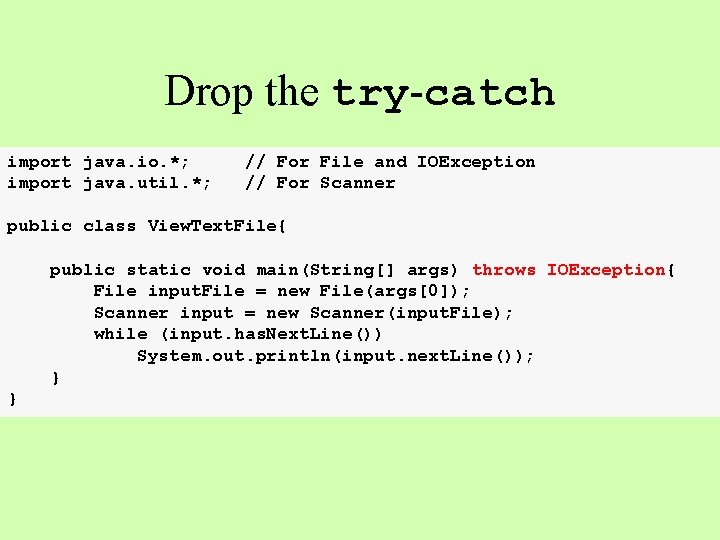
Drop the try-catch import java. io. *; import java. util. *; // For File and IOException // For Scanner public class View. Text. File{ public static void main(String[] args) throws IOException{ File input. File = new File(args[0]); Scanner input = new Scanner(input. File); while (input. has. Next. Line()) System. out. println(input. next. Line()); } }
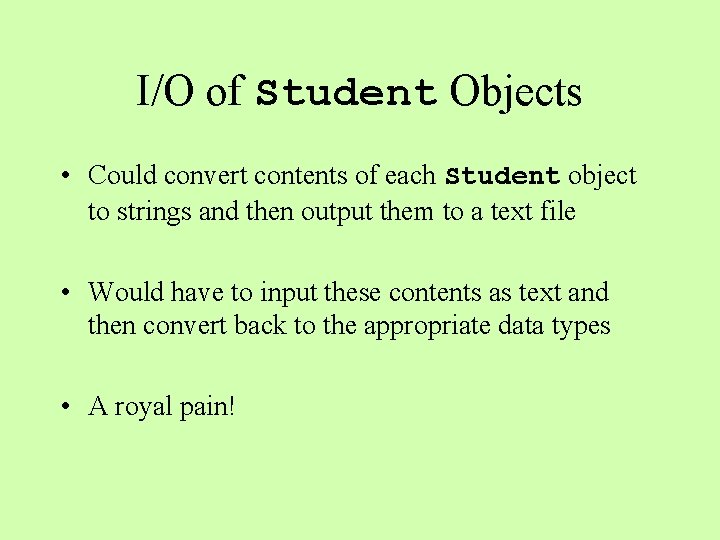
I/O of Student Objects • Could convert contents of each Student object to strings and then output them to a text file • Would have to input these contents as text and then convert back to the appropriate data types • A royal pain!
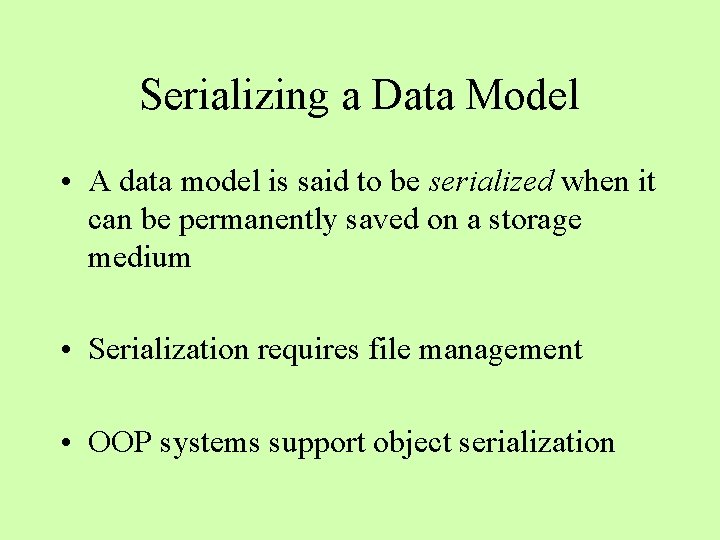
Serializing a Data Model • A data model is said to be serialized when it can be permanently saved on a storage medium • Serialization requires file management • OOP systems support object serialization
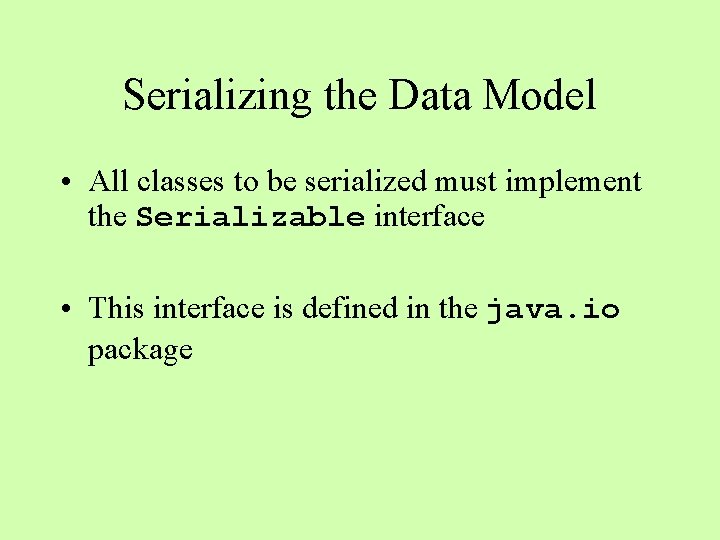
Serializing the Data Model • All classes to be serialized must implement the Serializable interface • This interface is defined in the java. io package
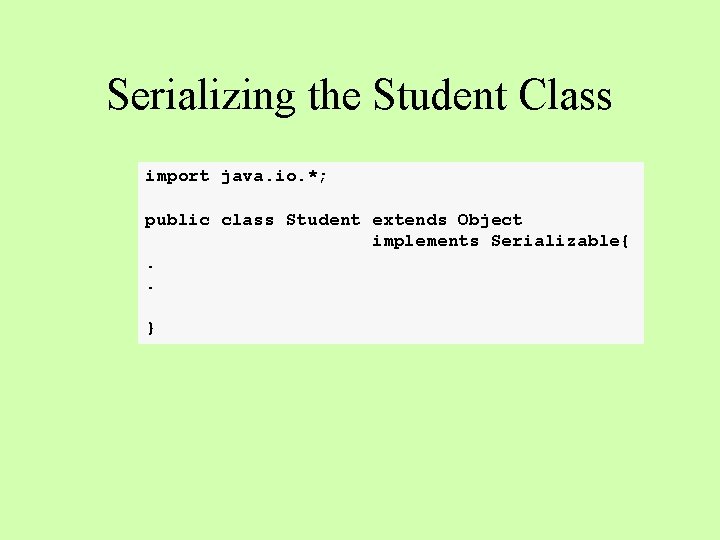
Serializing the Student Class import java. io. *; public class Student extends Object implements Serializable{. . }
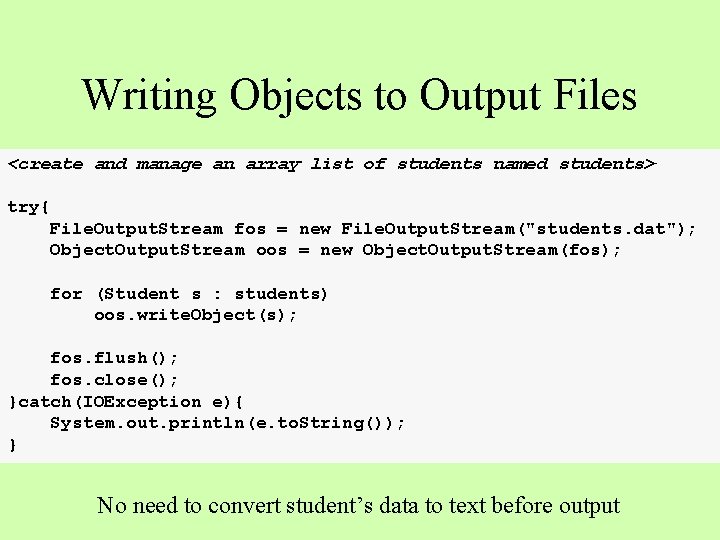
Writing Objects to Output Files <create and manage an array list of students named students> try{ File. Output. Stream fos = new File. Output. Stream("students. dat"); Object. Output. Stream oos = new Object. Output. Stream(fos); for (Student s : students) oos. write. Object(s); fos. flush(); fos. close(); }catch(IOException e){ System. out. println(e. to. String()); } No need to convert student’s data to text before output
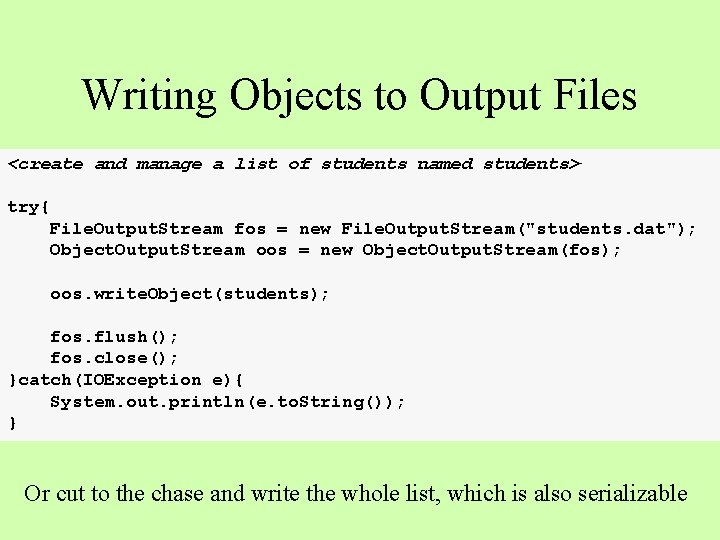
Writing Objects to Output Files <create and manage a list of students named students> try{ File. Output. Stream fos = new File. Output. Stream("students. dat"); Object. Output. Stream oos = new Object. Output. Stream(fos); oos. write. Object(students); fos. flush(); fos. close(); }catch(IOException e){ System. out. println(e. to. String()); } Or cut to the chase and write the whole list, which is also serializable
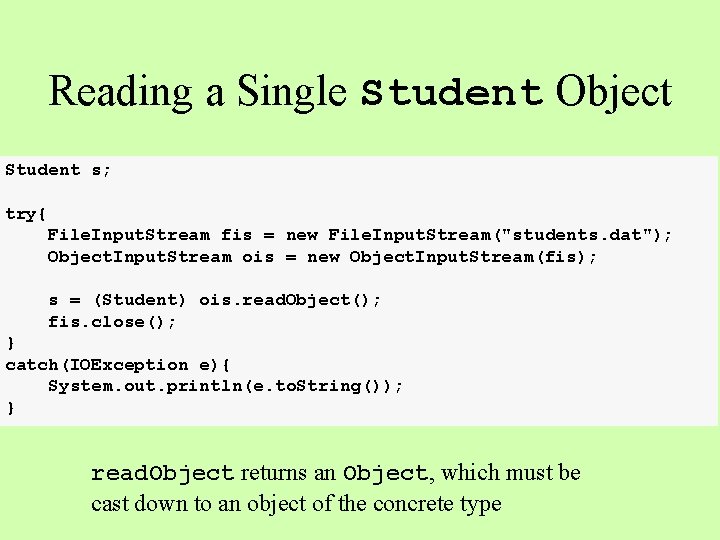
Reading a Single Student Object Student s; try{ File. Input. Stream fis = new File. Input. Stream("students. dat"); Object. Input. Stream ois = new Object. Input. Stream(fis); s = (Student) ois. read. Object(); fis. close(); } catch(IOException e){ System. out. println(e. to. String()); } read. Object returns an Object, which must be cast down to an object of the concrete type
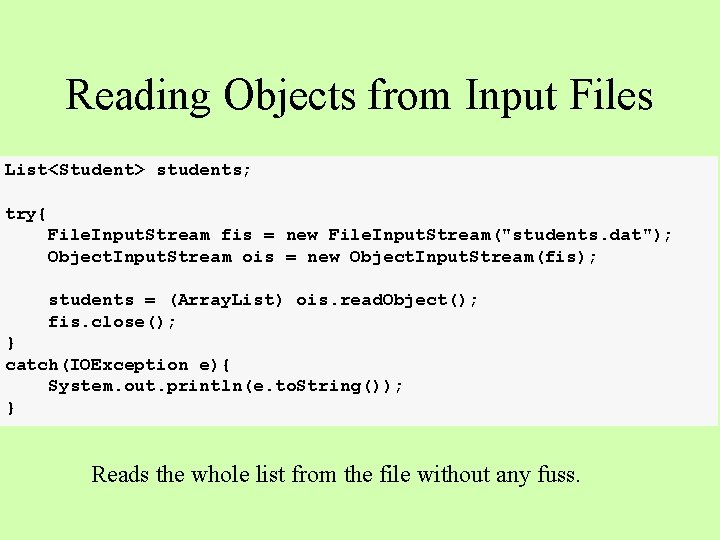
Reading Objects from Input Files List<Student> students; try{ File. Input. Stream fis = new File. Input. Stream("students. dat"); Object. Input. Stream ois = new Object. Input. Stream(fis); students = (Array. List) ois. read. Object(); fis. close(); } catch(IOException e){ System. out. println(e. to. String()); } Reads the whole list from the file without any fuss.
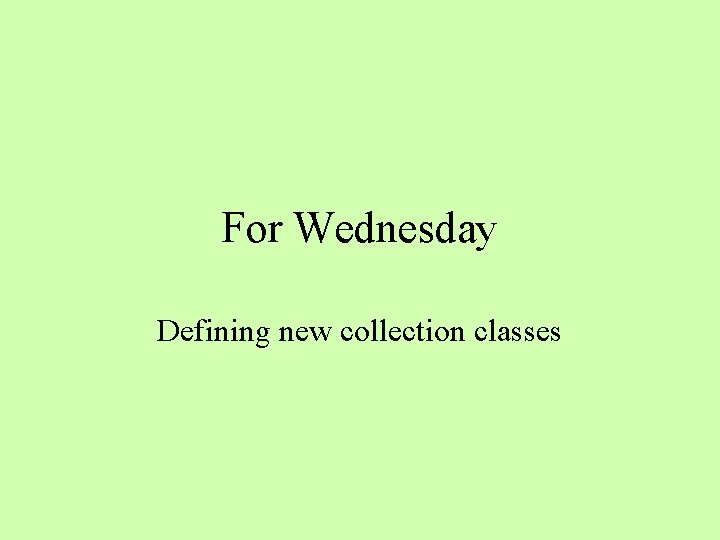
For Wednesday Defining new collection classes