Computer Science 209 Software Development Inheritance and Composition
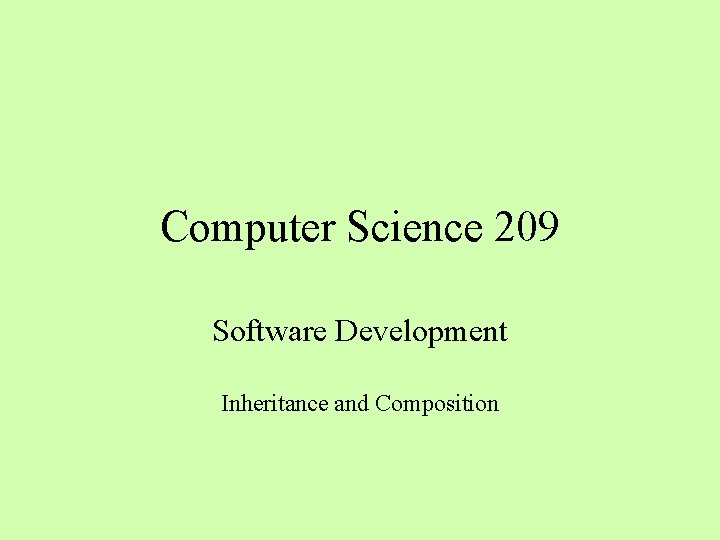
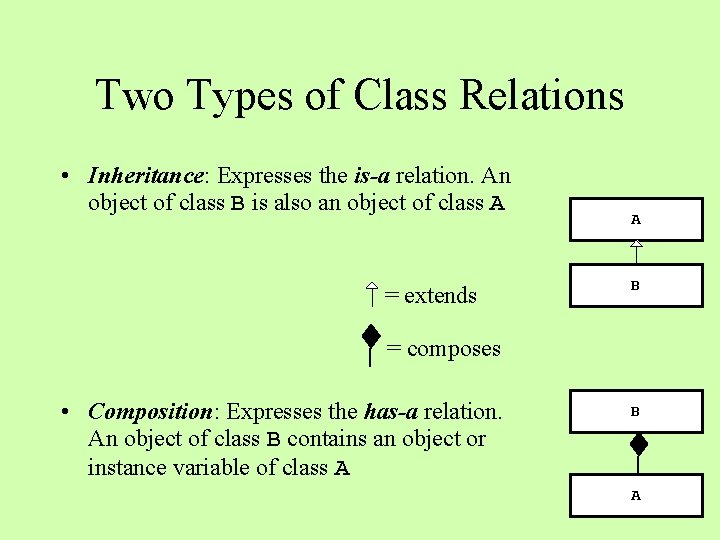
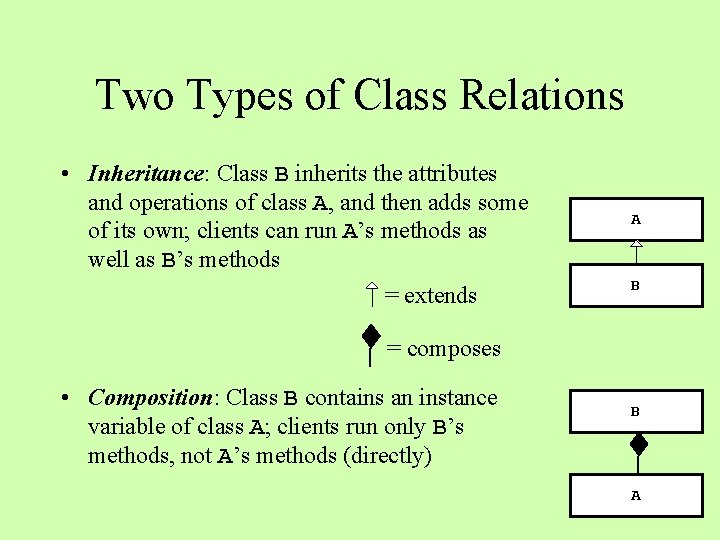
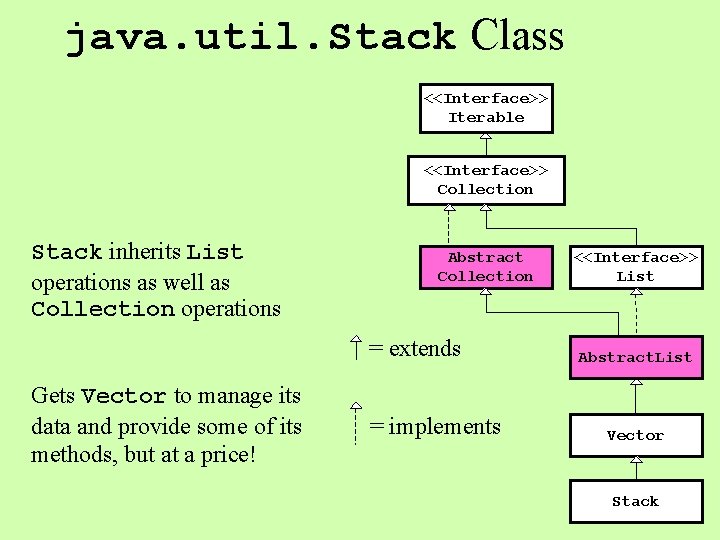
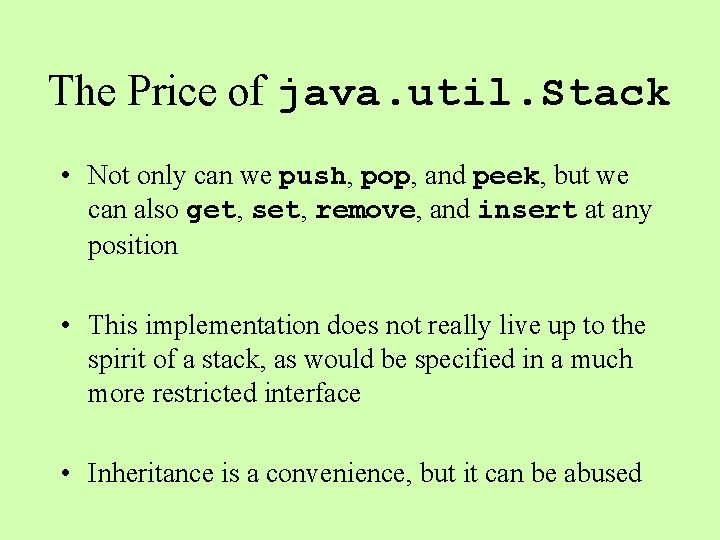
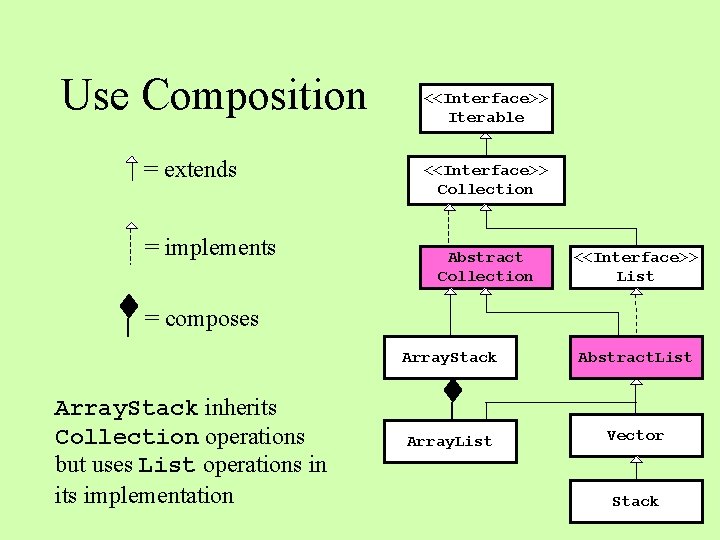
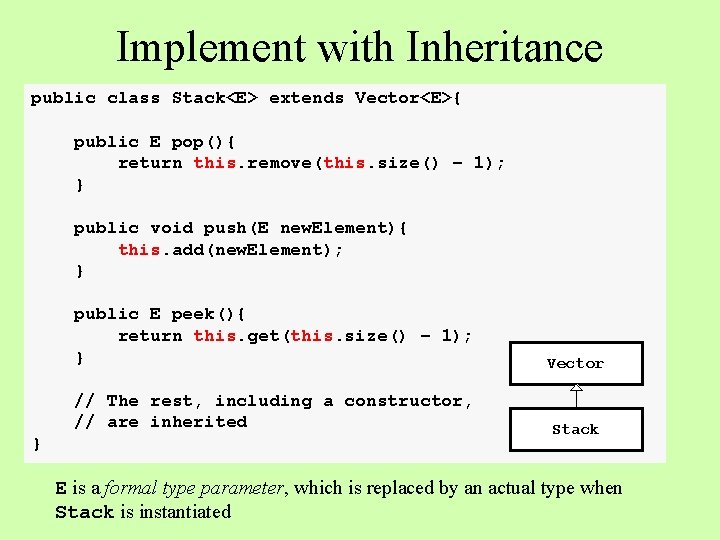
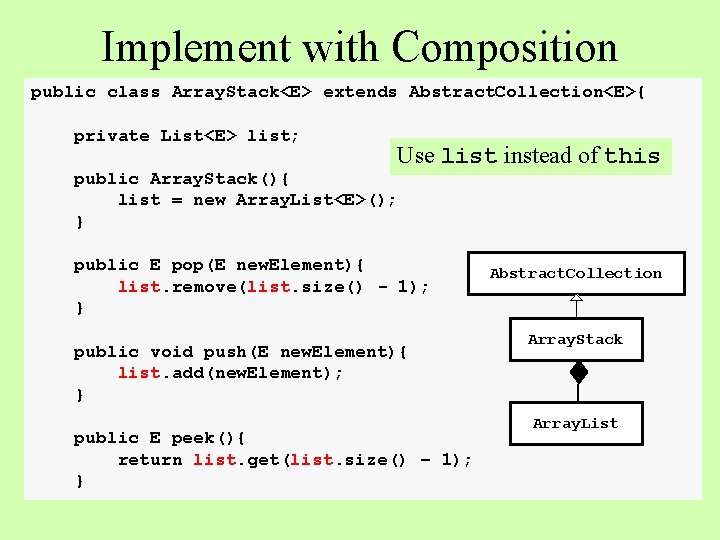
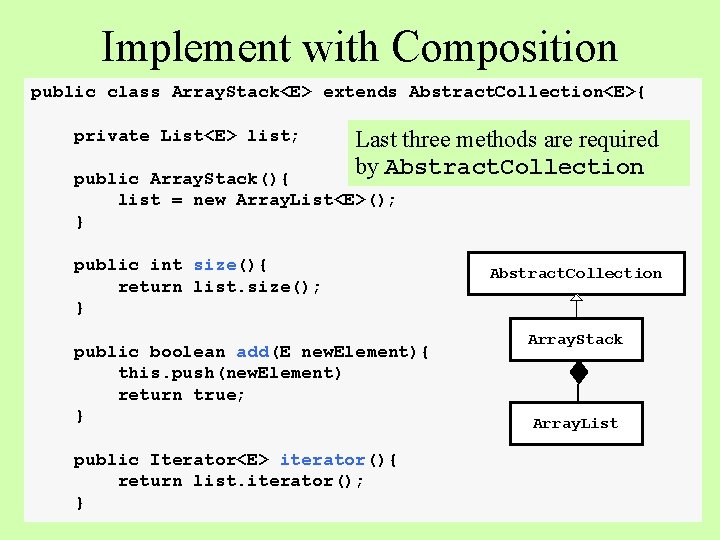
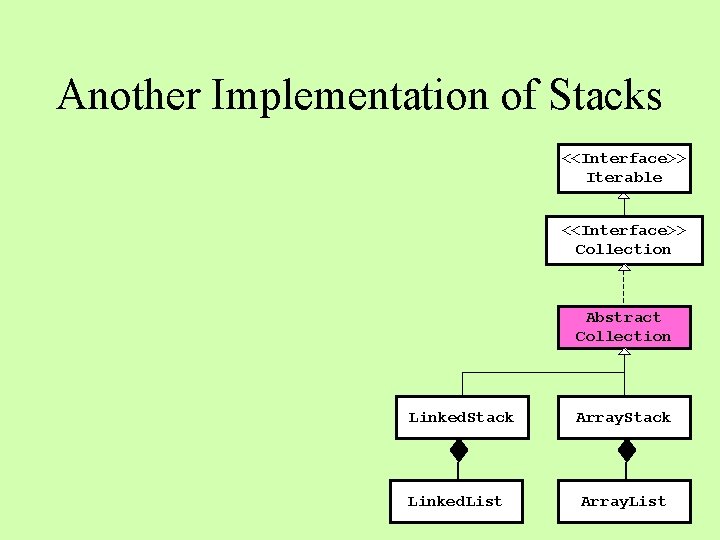
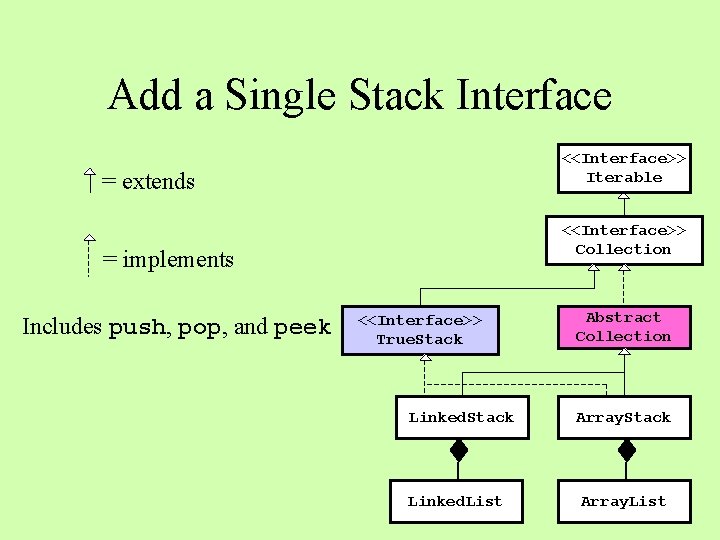
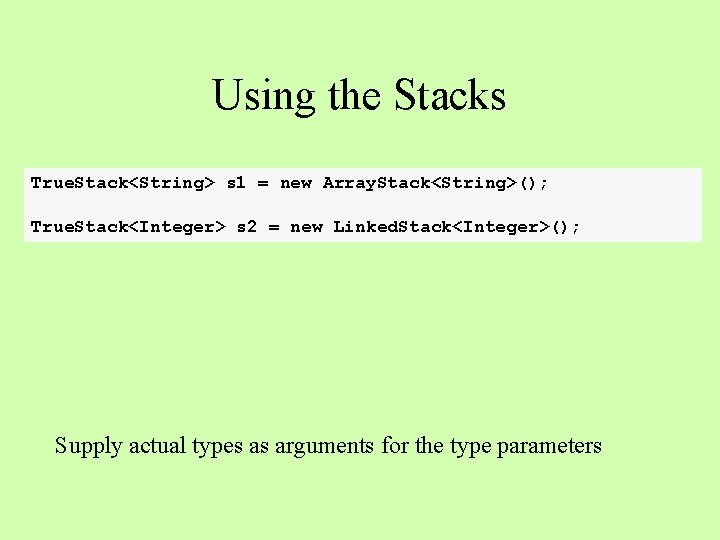
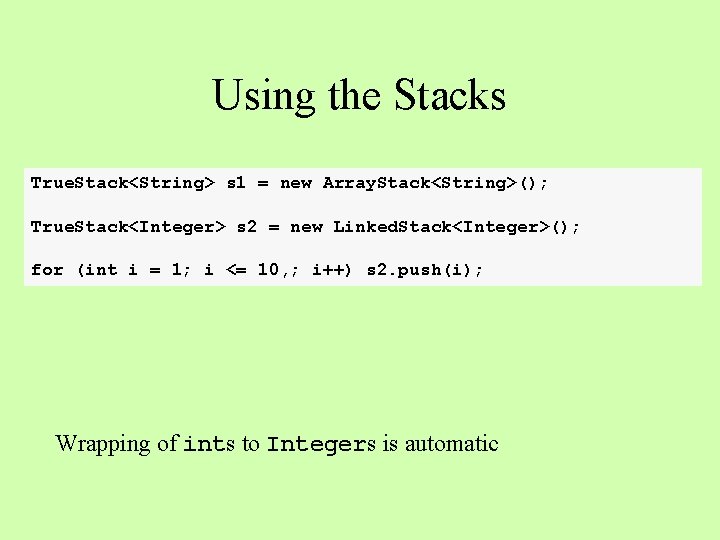
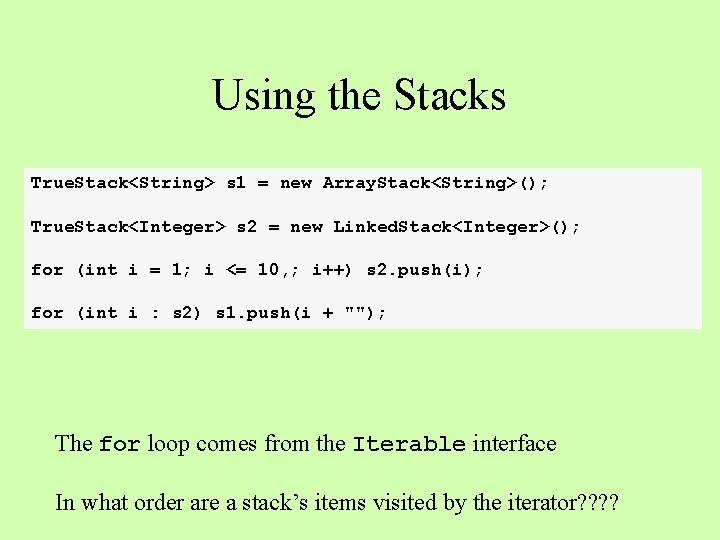
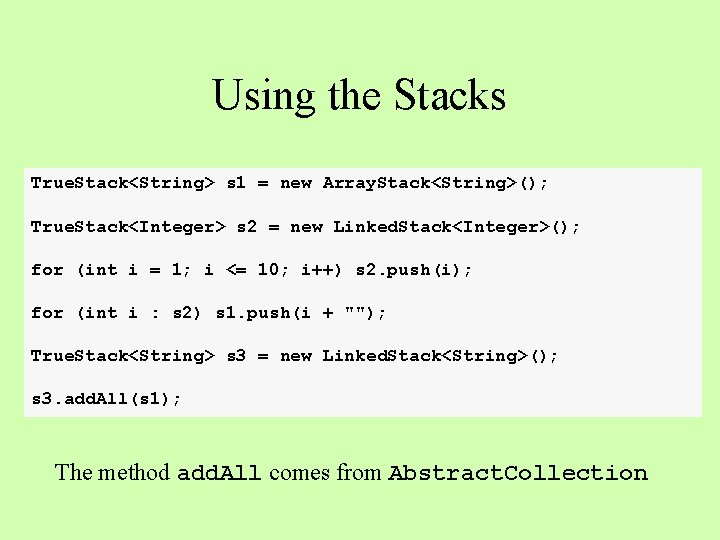
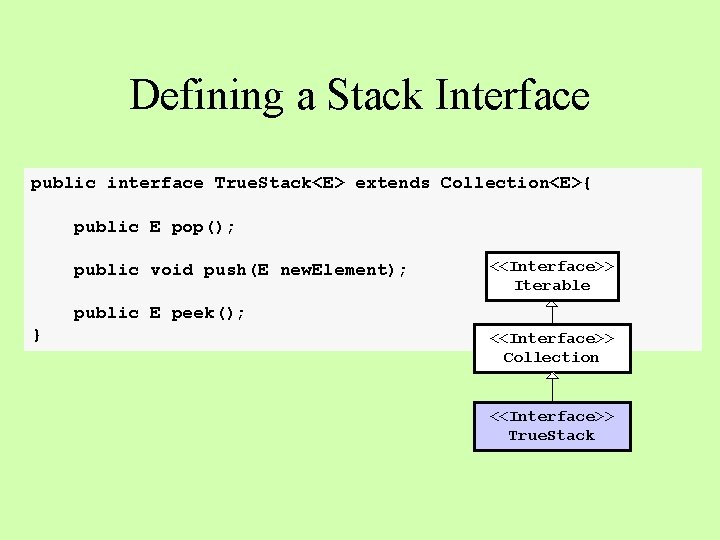
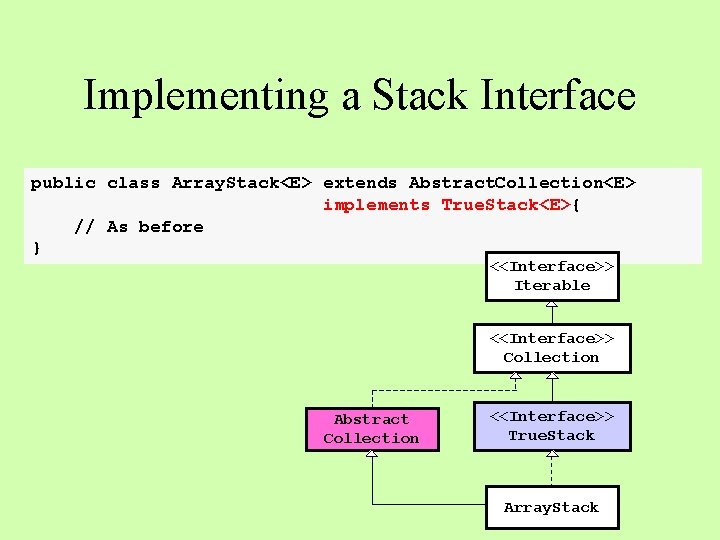
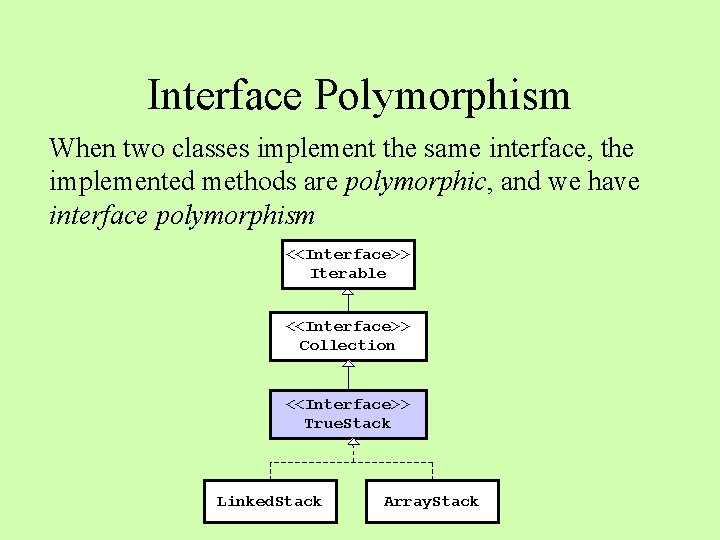
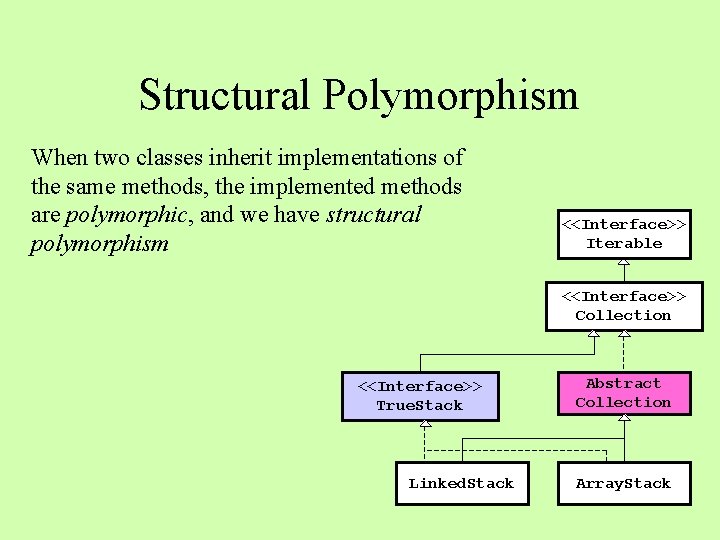
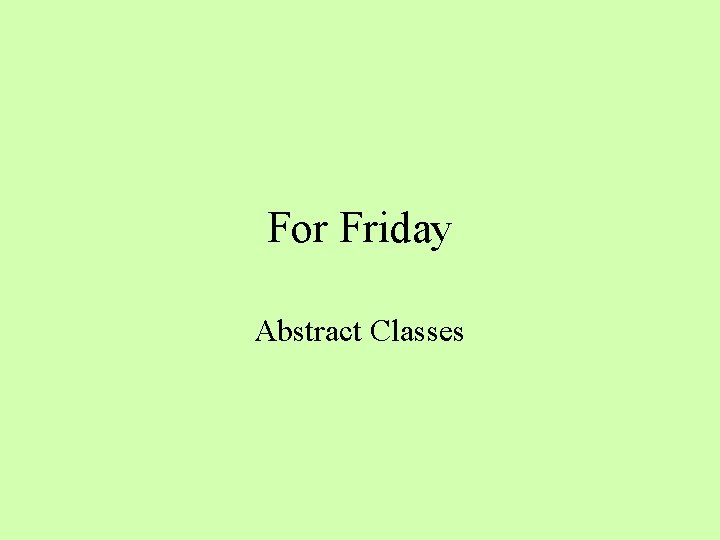
- Slides: 20
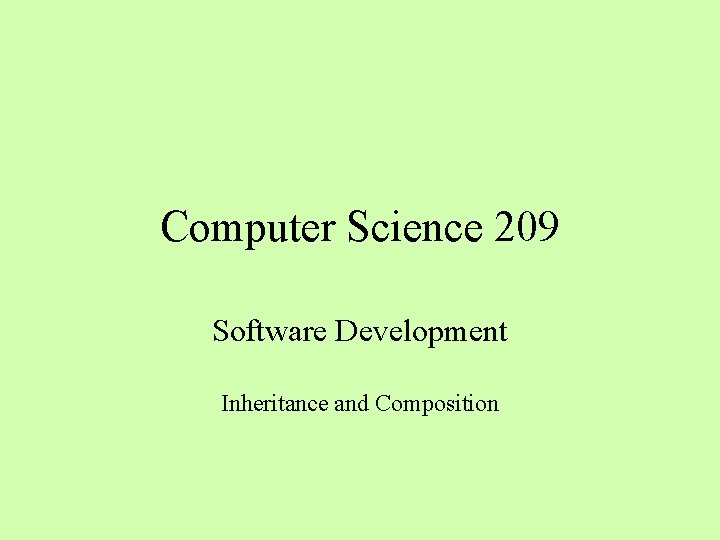
Computer Science 209 Software Development Inheritance and Composition
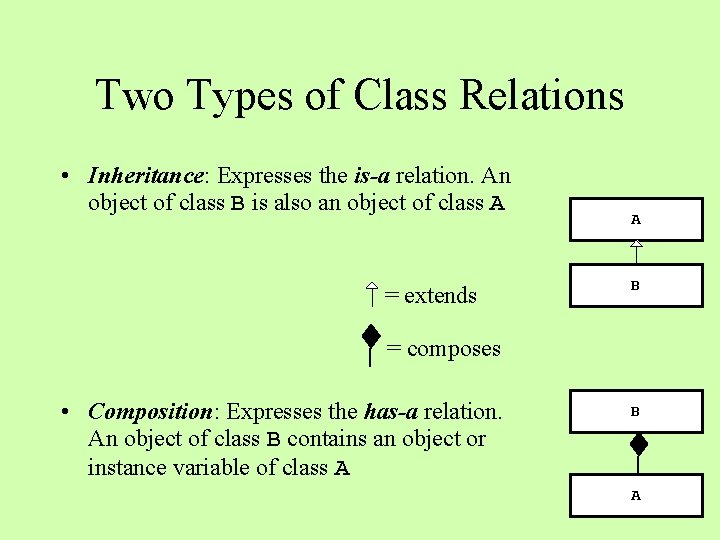
Two Types of Class Relations • Inheritance: Expresses the is-a relation. An object of class B is also an object of class A = extends A B = composes • Composition: Expresses the has-a relation. An object of class B contains an object or instance variable of class A B A
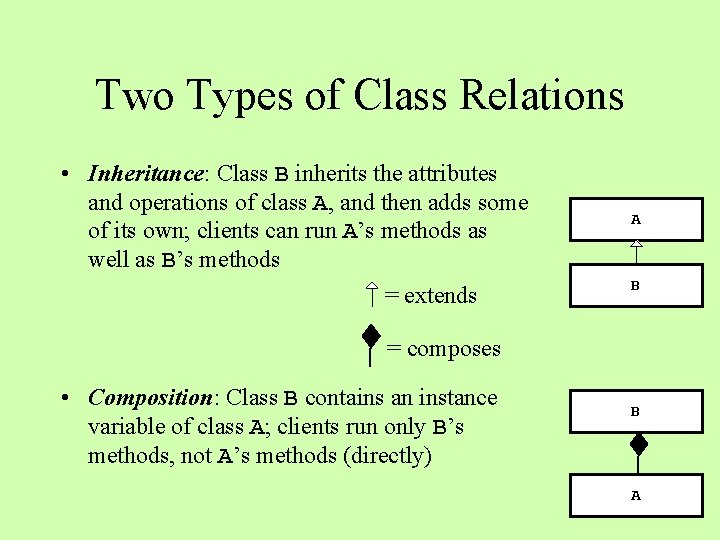
Two Types of Class Relations • Inheritance: Class B inherits the attributes and operations of class A, and then adds some of its own; clients can run A’s methods as well as B’s methods = extends A B = composes • Composition: Class B contains an instance variable of class A; clients run only B’s methods, not A’s methods (directly) B A
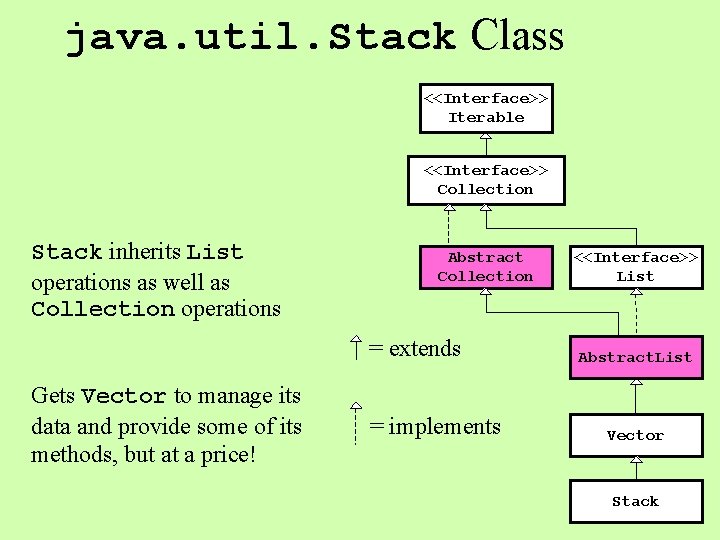
java. util. Stack Class <<Interface>> Iterable <<Interface>> Collection Stack inherits List operations as well as Collection operations Abstract Collection = extends Gets Vector to manage its data and provide some of its methods, but at a price! = implements <<Interface>> List Abstract. List Vector Stack
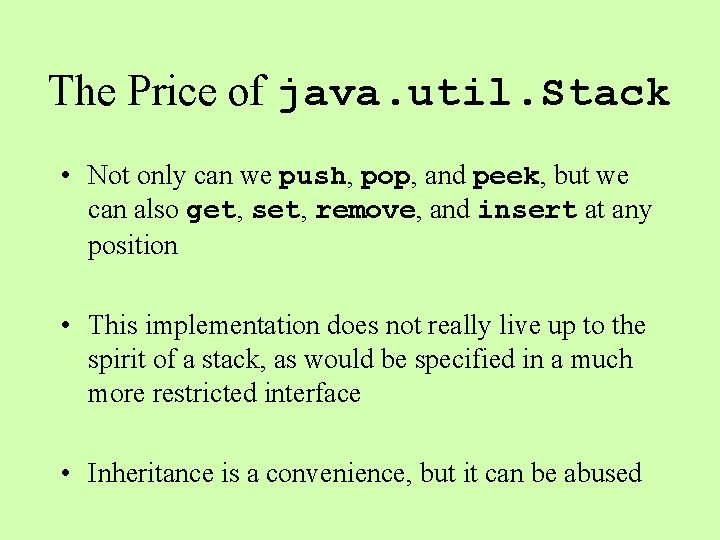
The Price of java. util. Stack • Not only can we push, pop, and peek, but we can also get, set, remove, and insert at any position • This implementation does not really live up to the spirit of a stack, as would be specified in a much more restricted interface • Inheritance is a convenience, but it can be abused
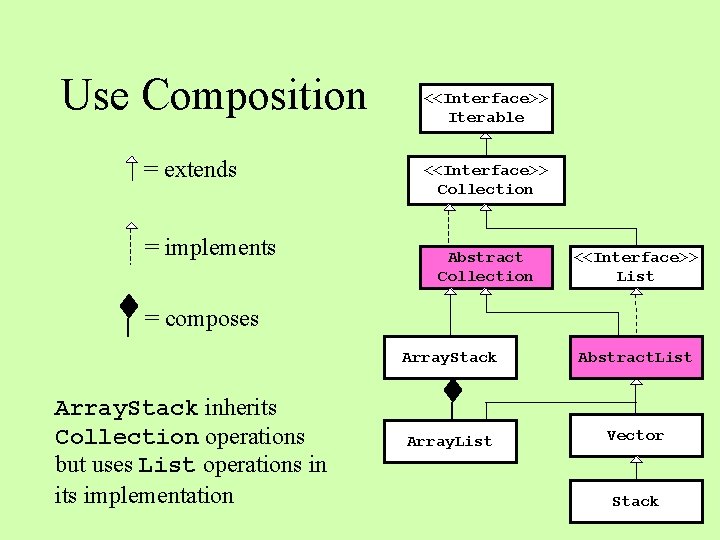
Use Composition = extends = implements <<Interface>> Iterable <<Interface>> Collection Abstract Collection <<Interface>> List = composes Array. Stack inherits Collection operations but uses List operations in its implementation Array. Stack Abstract. List Array. List Vector Stack
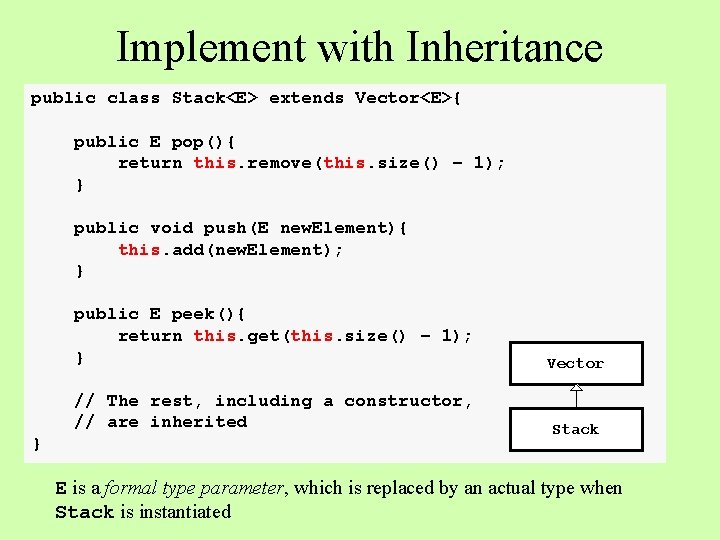
Implement with Inheritance public class Stack<E> extends Vector<E>{ public E pop(){ return this. remove(this. size() – 1); } public void push(E new. Element){ this. add(new. Element); } } public E peek(){ return this. get(this. size() – 1); } Vector // The rest, including a constructor, // are inherited Stack E is a formal type parameter, which is replaced by an actual type when Stack is instantiated
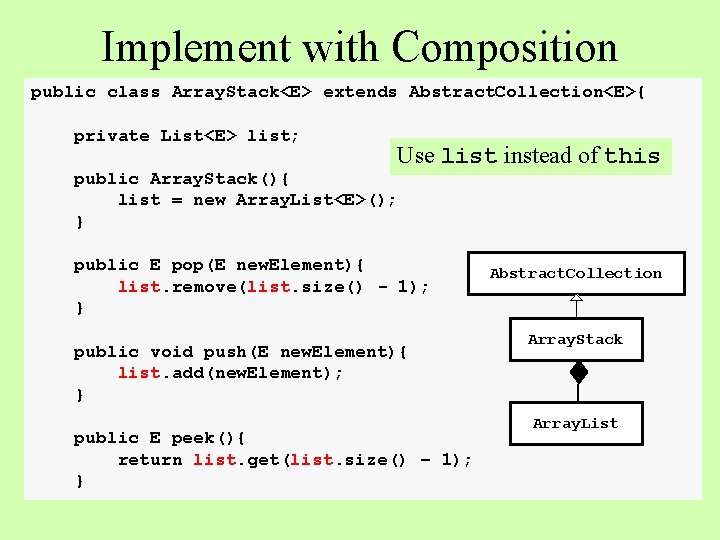
Implement with Composition public class Array. Stack<E> extends Abstract. Collection<E>{ private List<E> list; Use list instead of this public Array. Stack(){ list = new Array. List<E>(); } public E pop(E new. Element){ list. remove(list. size() - 1); } public void push(E new. Element){ list. add(new. Element); } public E peek(){ return list. get(list. size() – 1); } Abstract. Collection Array. Stack Array. List
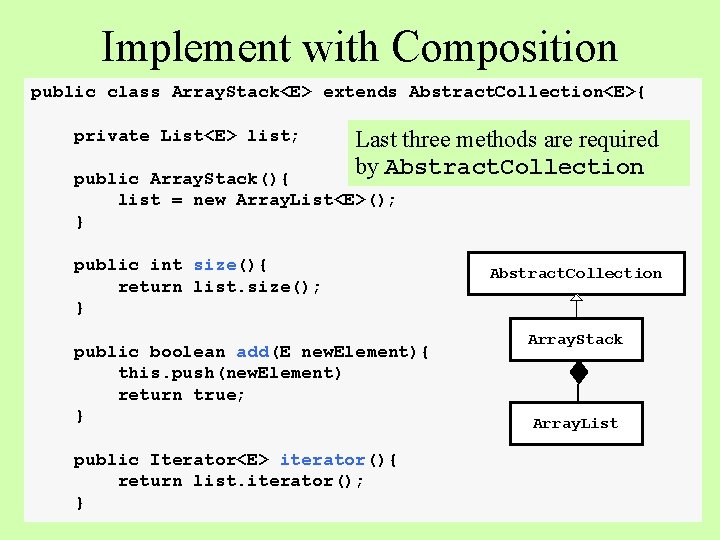
Implement with Composition public class Array. Stack<E> extends Abstract. Collection<E>{ private List<E> list; Last three methods are required by Abstract. Collection public Array. Stack(){ list = new Array. List<E>(); } public int size(){ return list. size(); } public boolean add(E new. Element){ this. push(new. Element) return true; } public Iterator<E> iterator(){ return list. iterator(); } Abstract. Collection Array. Stack Array. List
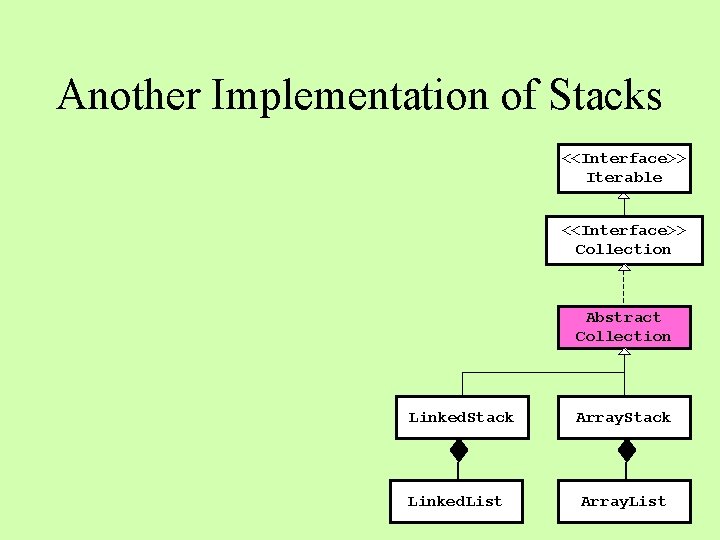
Another Implementation of Stacks <<Interface>> Iterable <<Interface>> Collection Abstract Collection Linked. Stack Linked. List Array. Stack Array. List
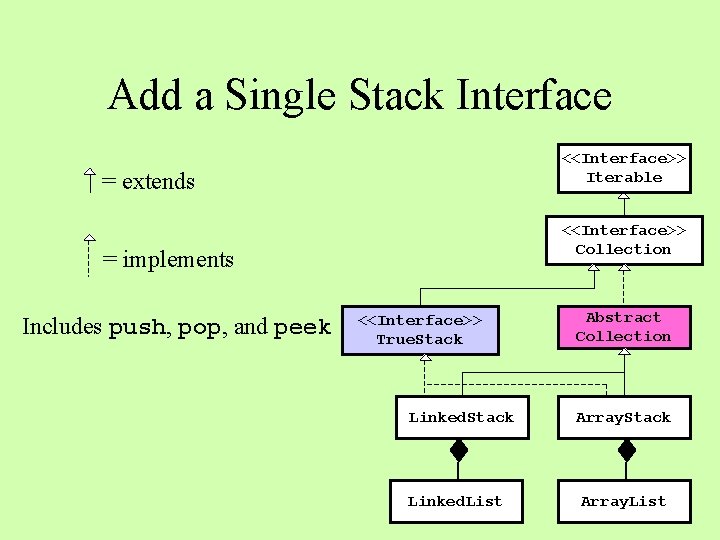
Add a Single Stack Interface <<Interface>> Iterable = extends <<Interface>> Collection = implements Includes push, pop, and peek <<Interface>> True. Stack Linked. List Abstract Collection Array. Stack Array. List
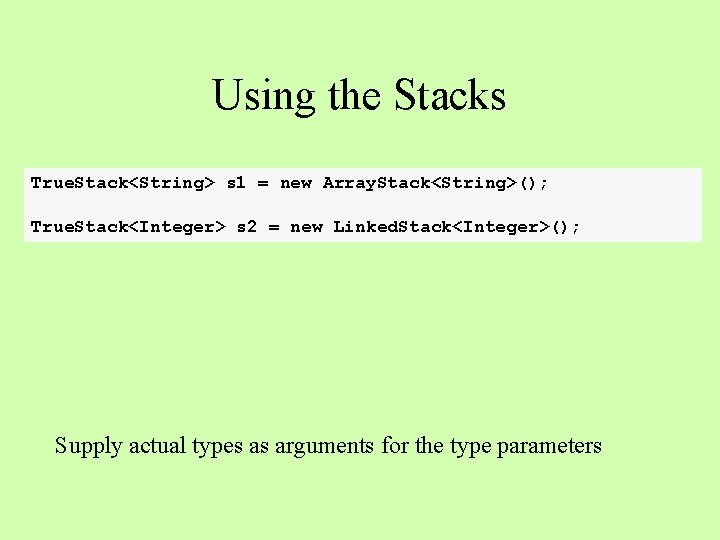
Using the Stacks True. Stack<String> s 1 = new Array. Stack<String>(); True. Stack<Integer> s 2 = new Linked. Stack<Integer>(); Supply actual types as arguments for the type parameters
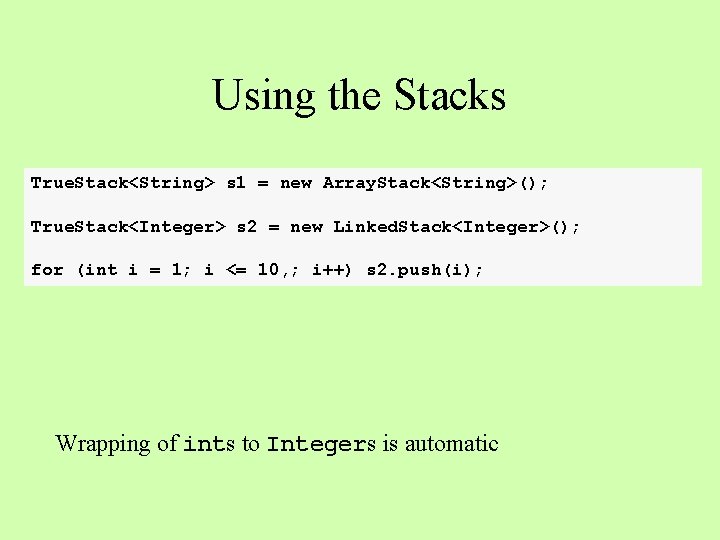
Using the Stacks True. Stack<String> s 1 = new Array. Stack<String>(); True. Stack<Integer> s 2 = new Linked. Stack<Integer>(); for (int i = 1; i <= 10, ; i++) s 2. push(i); Wrapping of ints to Integers is automatic
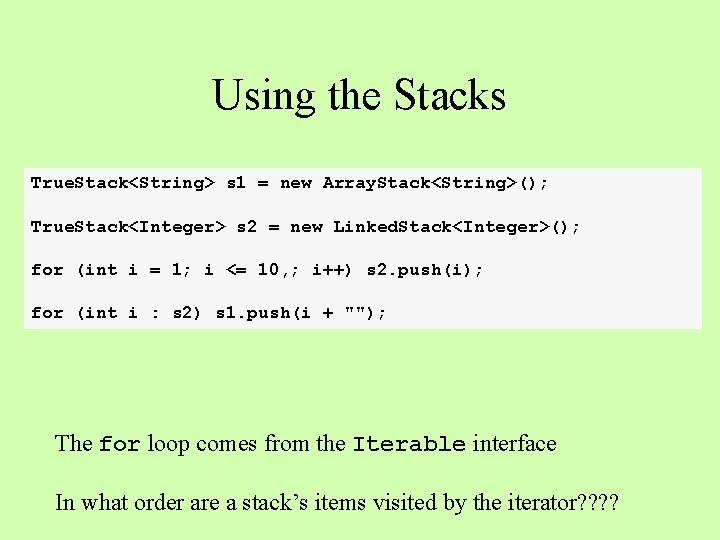
Using the Stacks True. Stack<String> s 1 = new Array. Stack<String>(); True. Stack<Integer> s 2 = new Linked. Stack<Integer>(); for (int i = 1; i <= 10, ; i++) s 2. push(i); for (int i : s 2) s 1. push(i + ""); The for loop comes from the Iterable interface In what order are a stack’s items visited by the iterator? ?
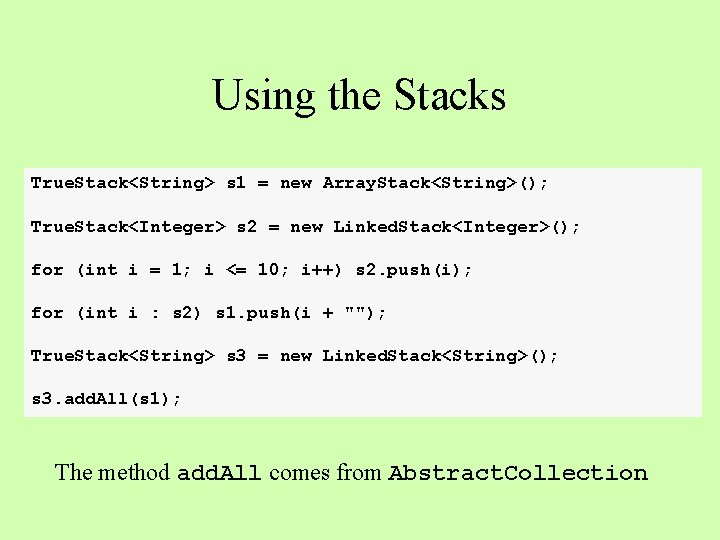
Using the Stacks True. Stack<String> s 1 = new Array. Stack<String>(); True. Stack<Integer> s 2 = new Linked. Stack<Integer>(); for (int i = 1; i <= 10; i++) s 2. push(i); for (int i : s 2) s 1. push(i + ""); True. Stack<String> s 3 = new Linked. Stack<String>(); s 3. add. All(s 1); The method add. All comes from Abstract. Collection
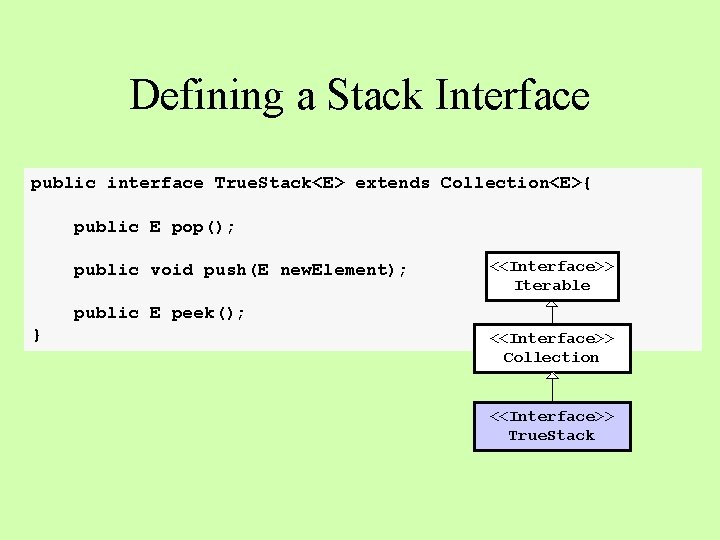
Defining a Stack Interface public interface True. Stack<E> extends Collection<E>{ public E pop(); public void push(E new. Element); <<Interface>> Iterable public E peek(); } <<Interface>> Collection <<Interface>> True. Stack
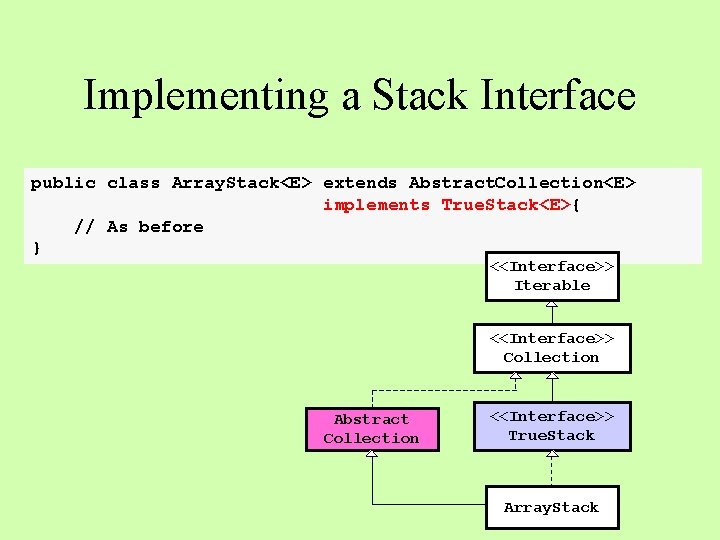
Implementing a Stack Interface public class Array. Stack<E> extends Abstract. Collection<E> implements True. Stack<E>{ // As before } <<Interface>> Iterable <<Interface>> Collection Abstract Collection <<Interface>> True. Stack Array. Stack
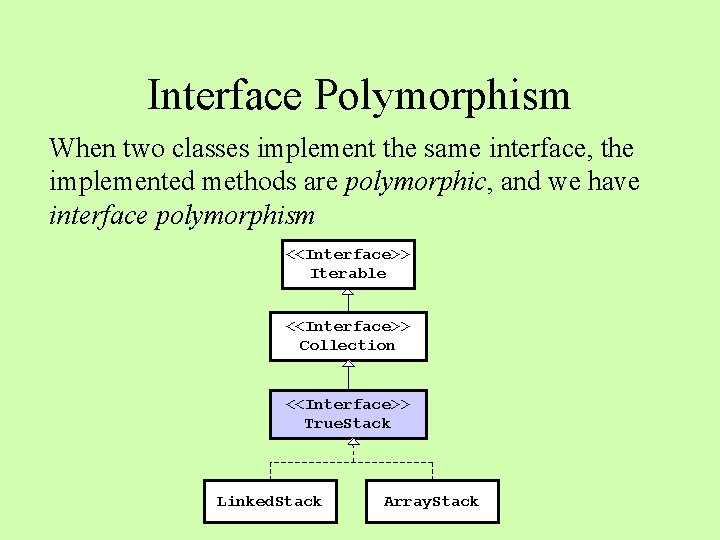
Interface Polymorphism When two classes implement the same interface, the implemented methods are polymorphic, and we have interface polymorphism <<Interface>> Iterable <<Interface>> Collection <<Interface>> True. Stack Linked. Stack Array. Stack
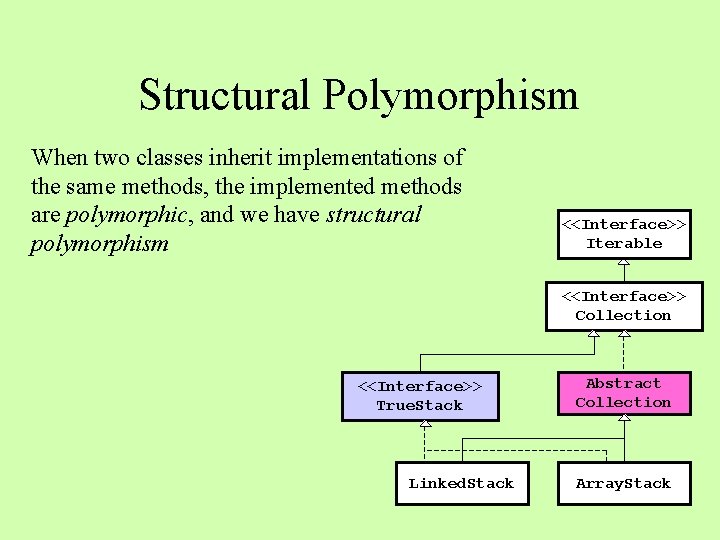
Structural Polymorphism When two classes inherit implementations of the same methods, the implemented methods are polymorphic, and we have structural polymorphism <<Interface>> Iterable <<Interface>> Collection <<Interface>> True. Stack Linked. Stack Abstract Collection Array. Stack
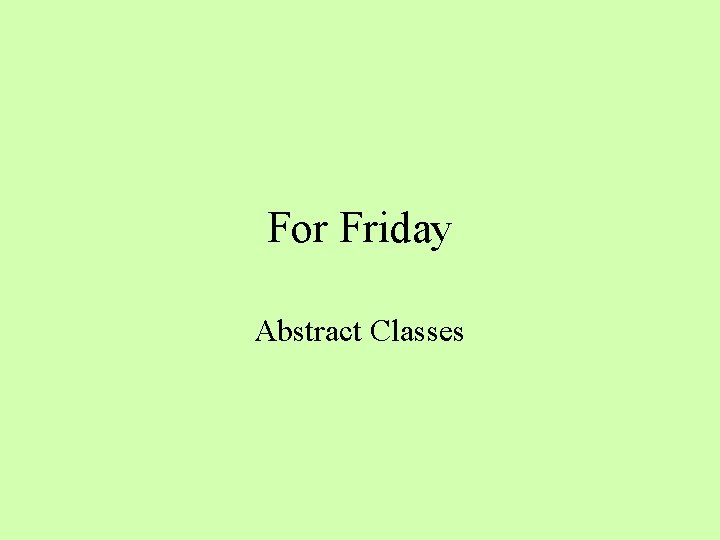
For Friday Abstract Classes
Inheritance definition computer science
Wordle 209
735 ilcs 5/13-209
Lesson 2 add integers page 209 answers
Ece 209
Participio presente activo griego
Whats a half life
Ece 209
Composition vs inheritance vs aggregation
Composition software development
What's your favourite school
Computer science vs software engineering
Computer science software engineering
Computer science software engineering
Computer aided software testing
Transovarial transmission คือ
Advantages of inheritance
Extranuclear inheritance
Section 12-1 chromosomes and inheritance
Chapter 11 complex inheritance and human heredity test
Encapsulation inheritance polymorphism