CMSC 202 Polymorphism 2 nd Lecture Aug 6
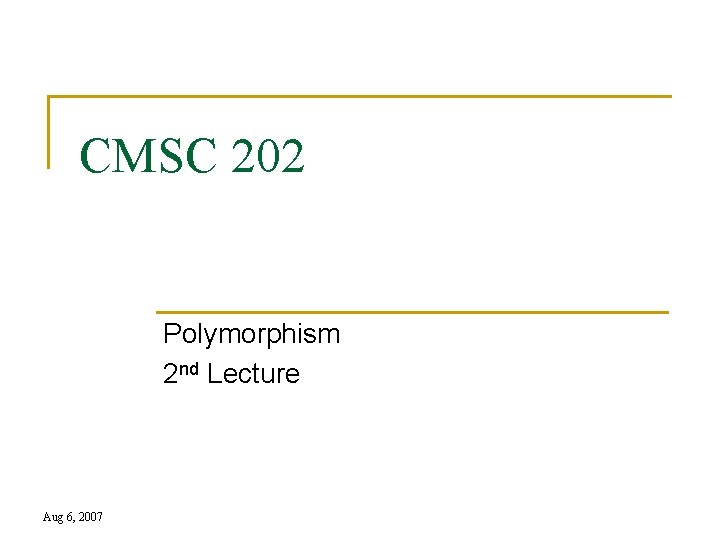
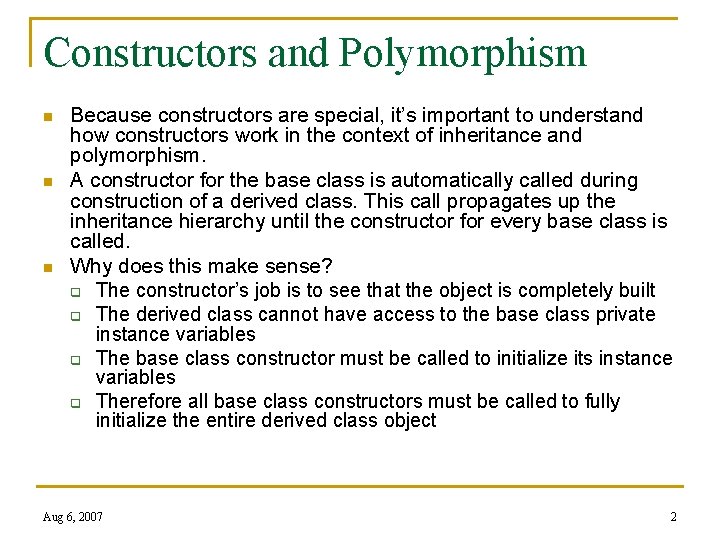
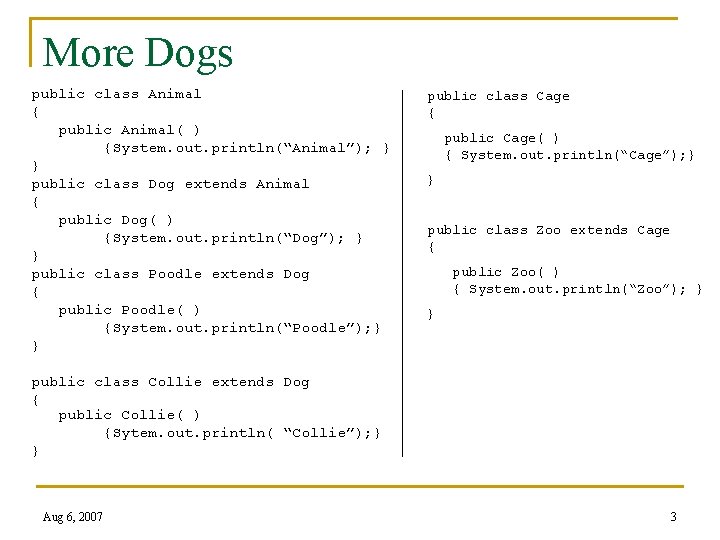
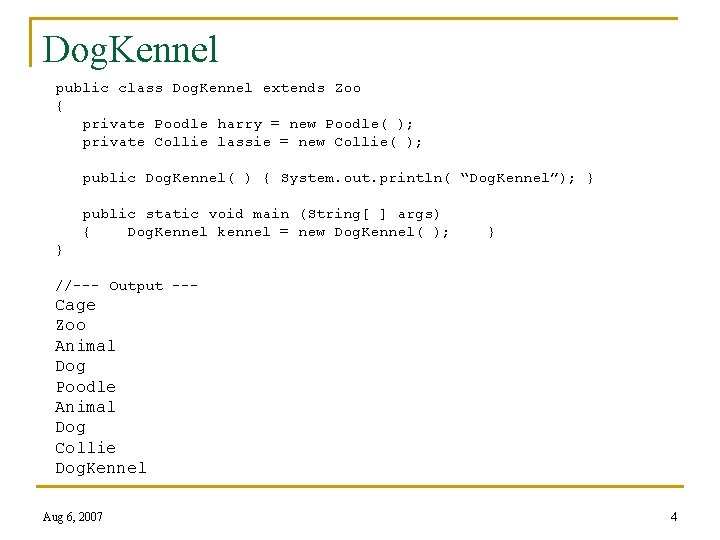
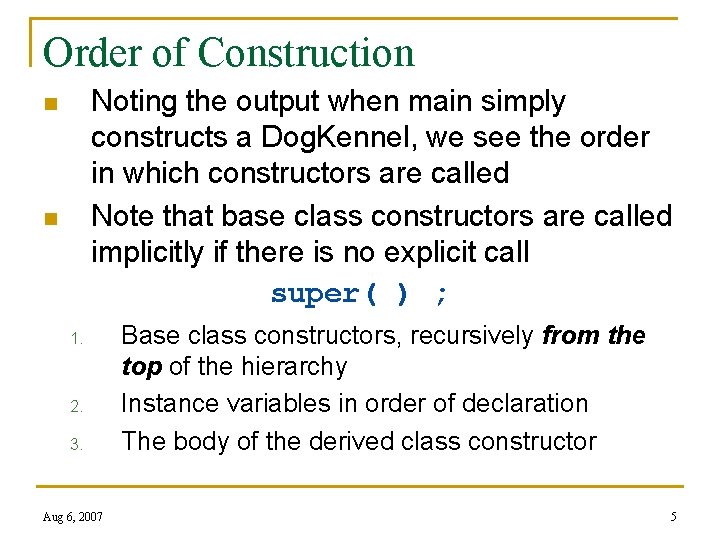
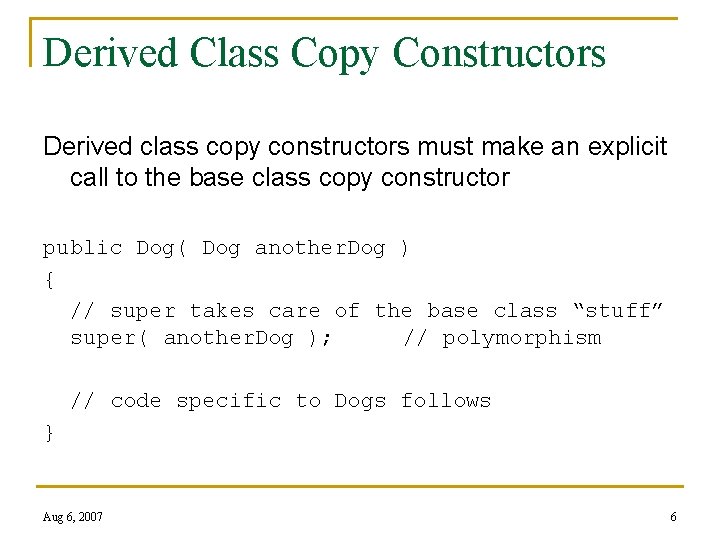
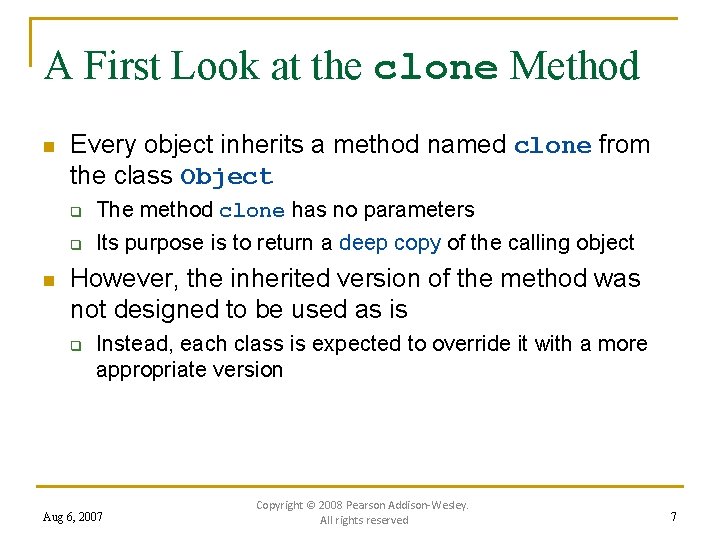
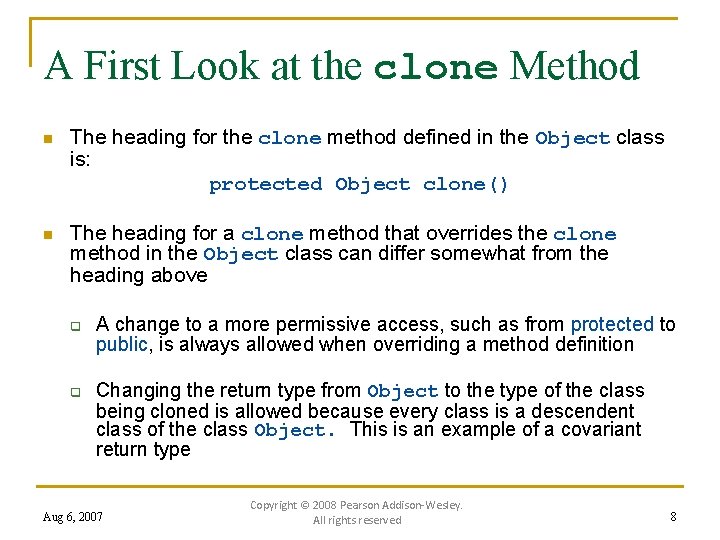
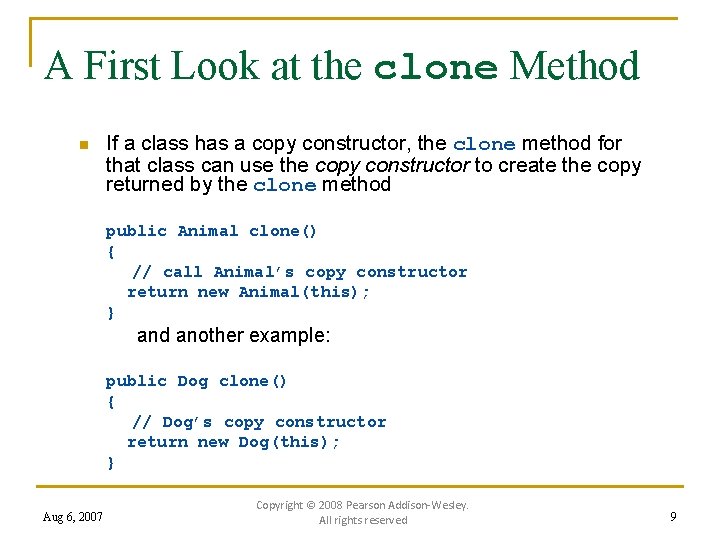
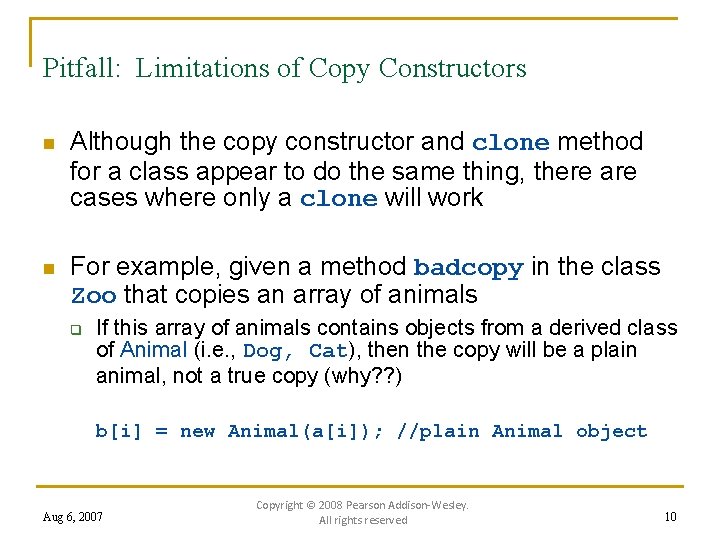
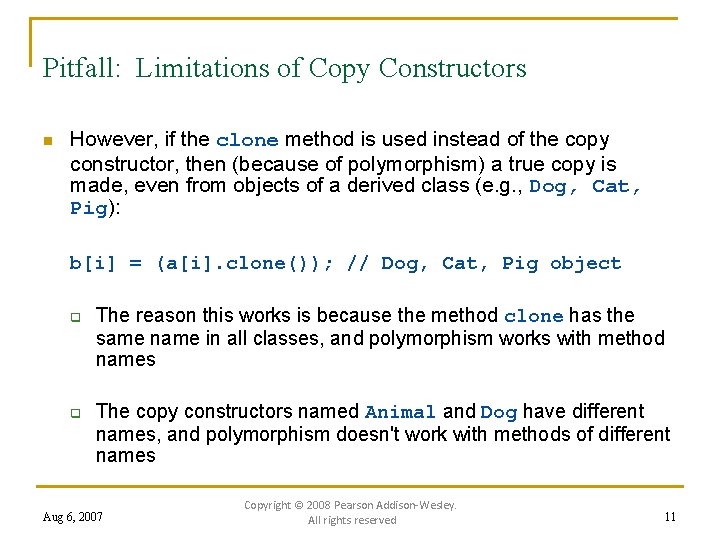
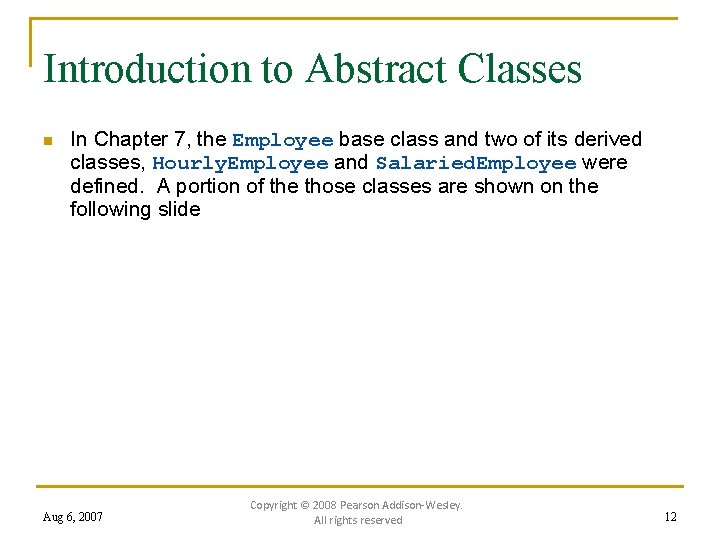
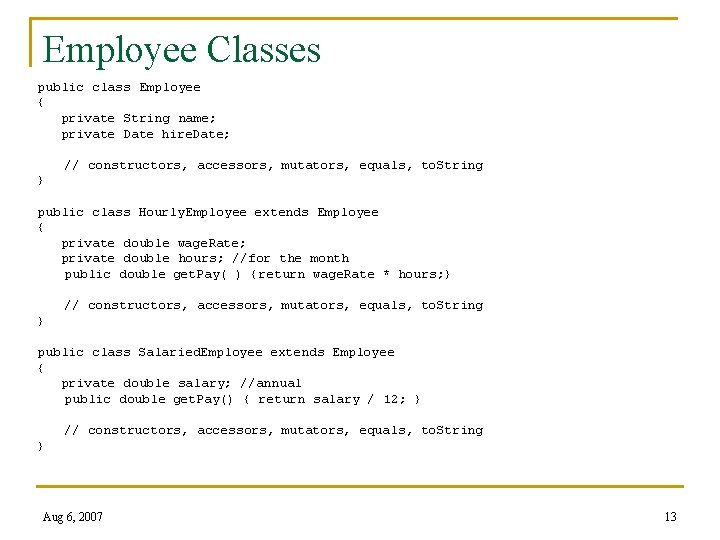
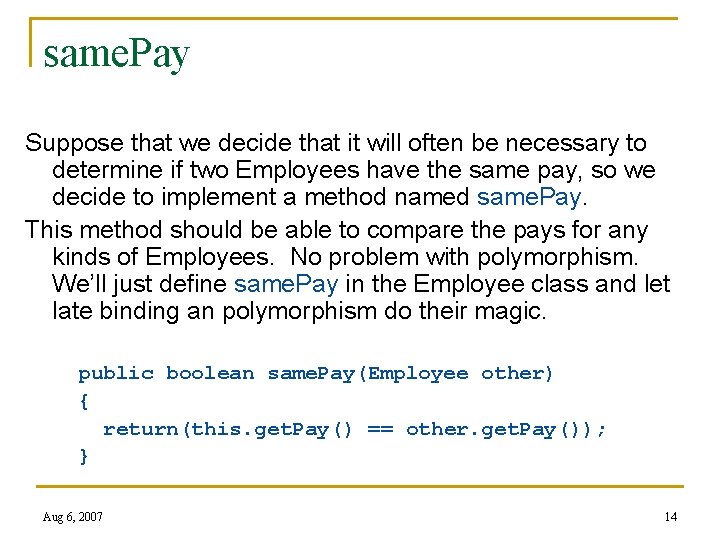
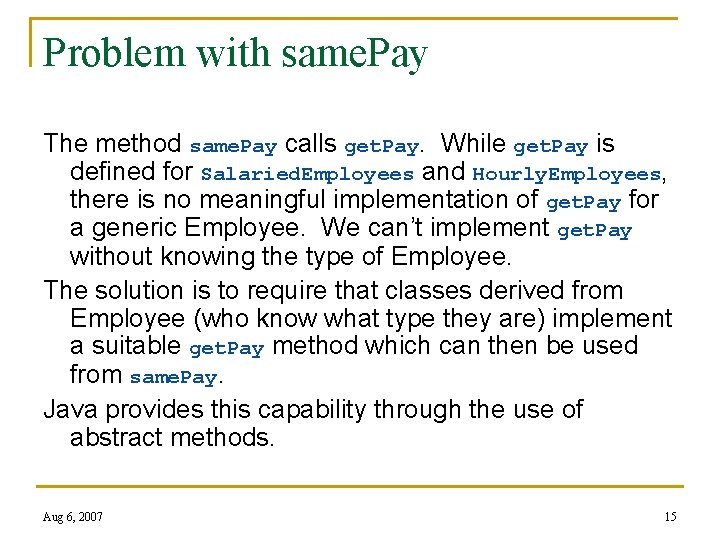
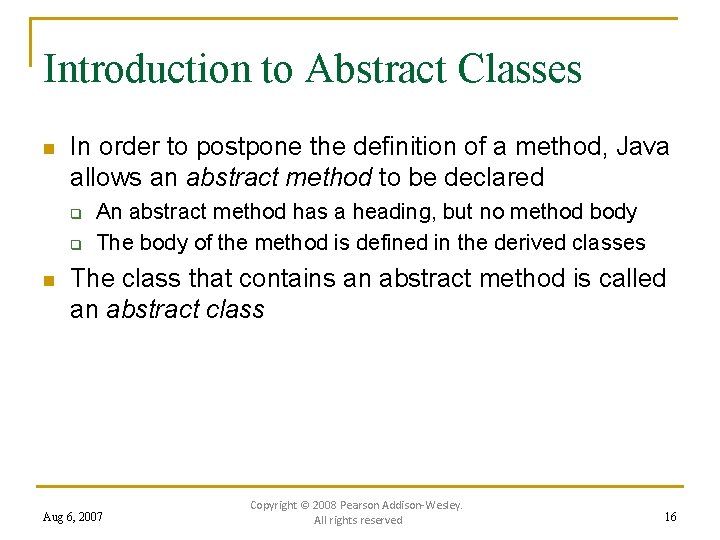
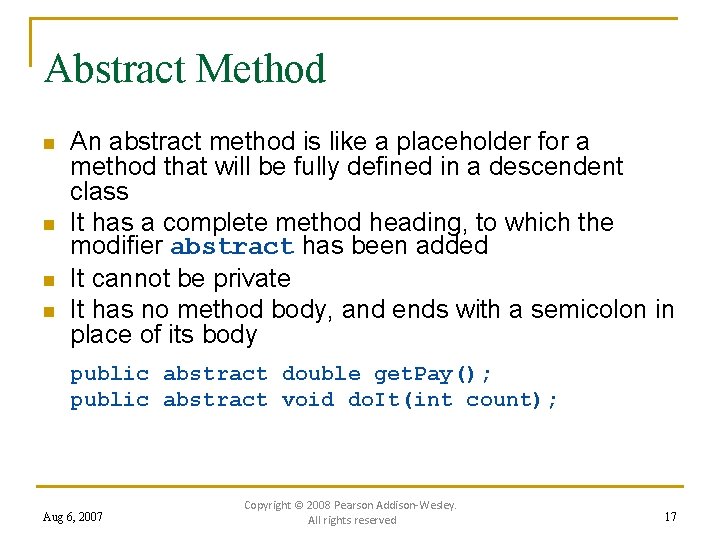
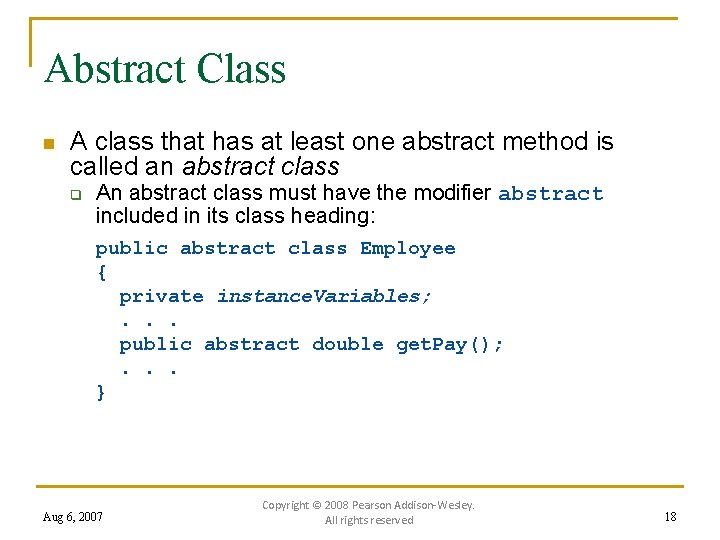
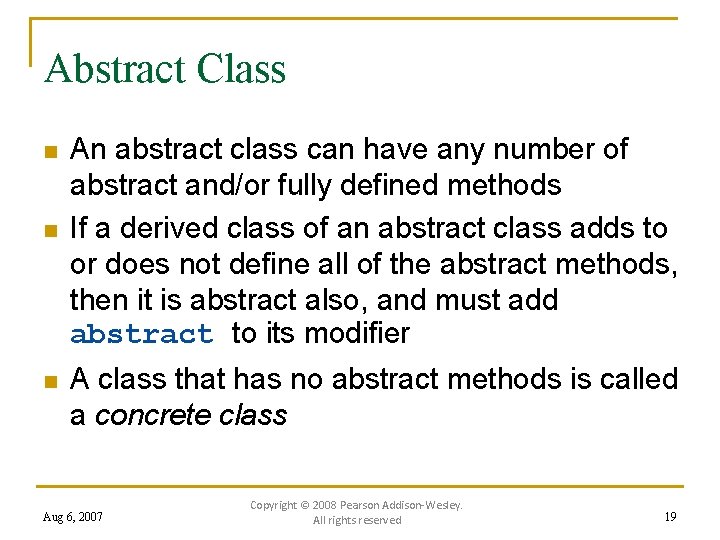
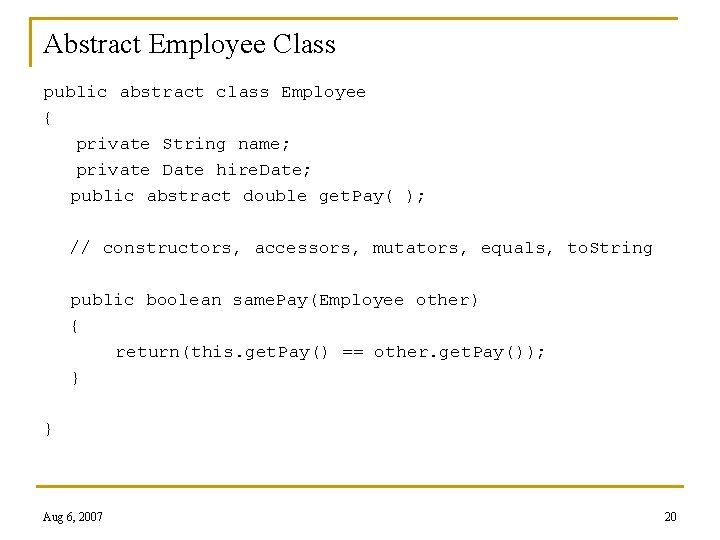
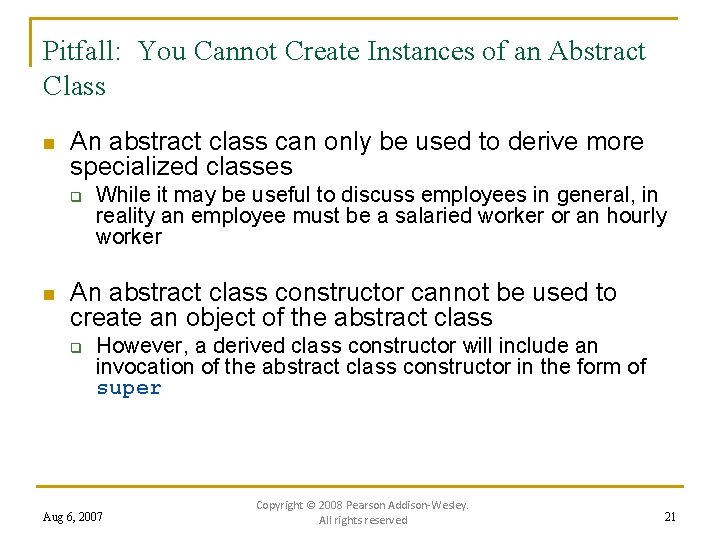
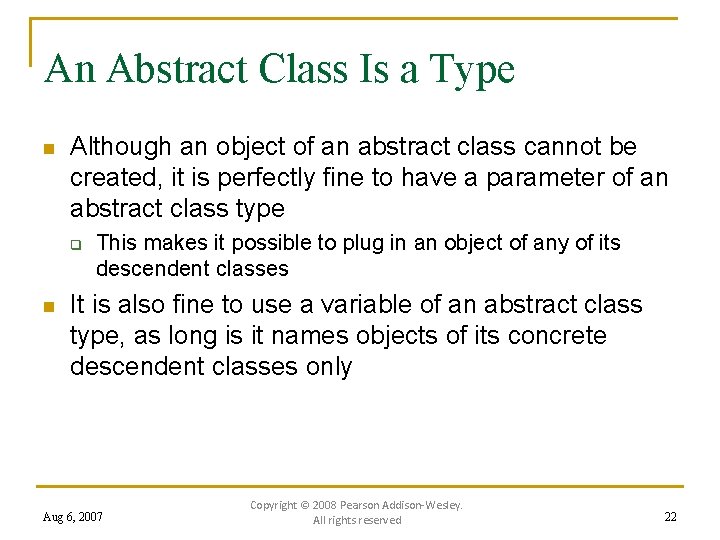
- Slides: 22
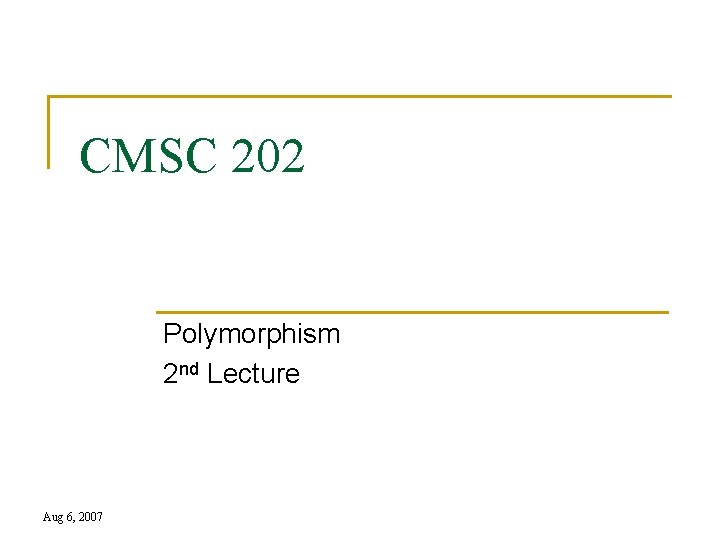
CMSC 202 Polymorphism 2 nd Lecture Aug 6, 2007
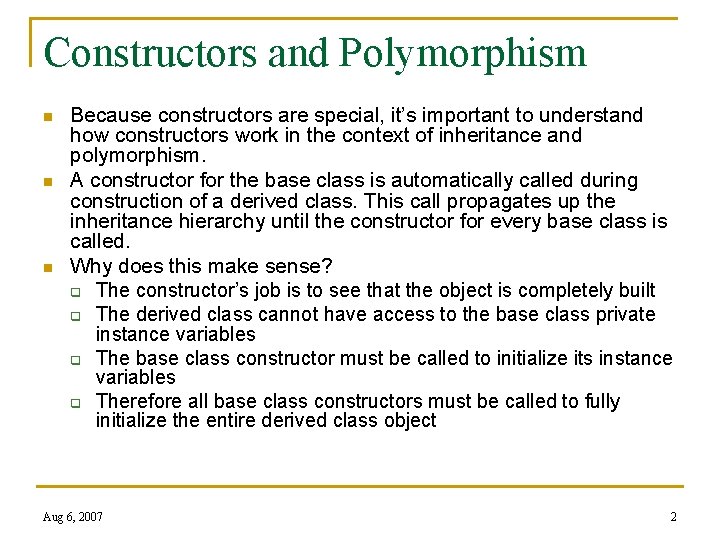
Constructors and Polymorphism n n n Because constructors are special, it’s important to understand how constructors work in the context of inheritance and polymorphism. A constructor for the base class is automatically called during construction of a derived class. This call propagates up the inheritance hierarchy until the constructor for every base class is called. Why does this make sense? q The constructor’s job is to see that the object is completely built q The derived class cannot have access to the base class private instance variables q The base class constructor must be called to initialize its instance variables q Therefore all base class constructors must be called to fully initialize the entire derived class object Aug 6, 2007 2
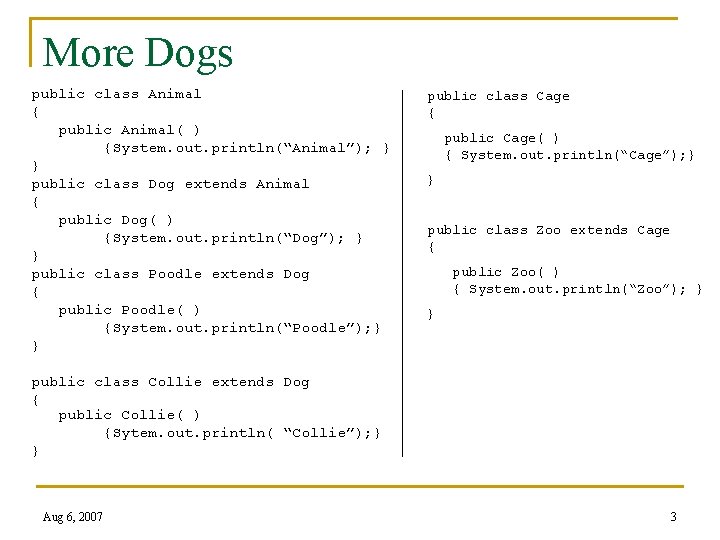
More Dogs public class Animal { public Animal( ) {System. out. println(“Animal”); } } public class Dog extends Animal { public Dog( ) {System. out. println(“Dog”); } } public class Poodle extends Dog { public Poodle( ) {System. out. println(“Poodle”); } } public class Cage { public Cage( ) { System. out. println(“Cage”); } } public class Zoo extends Cage { public Zoo( ) { System. out. println(“Zoo”); } } public class Collie extends Dog { public Collie( ) {Sytem. out. println( “Collie”); } } Aug 6, 2007 3
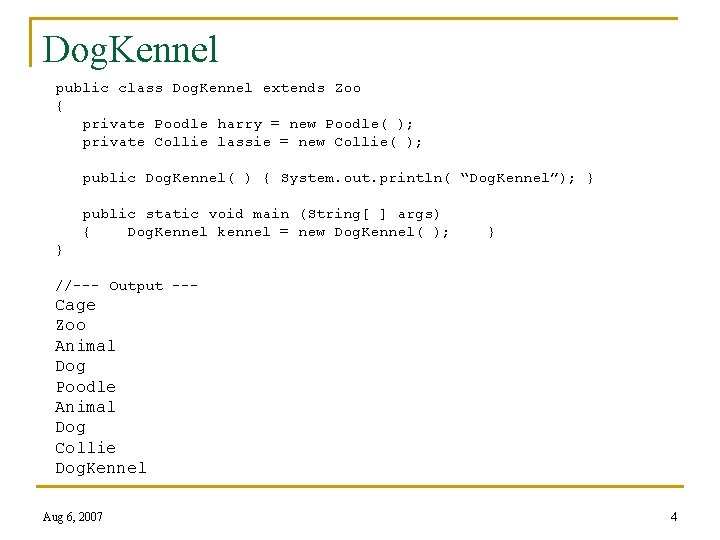
Dog. Kennel public class Dog. Kennel extends Zoo { private Poodle harry = new Poodle( ); private Collie lassie = new Collie( ); public Dog. Kennel( ) { System. out. println( “Dog. Kennel”); } public static void main (String[ ] args) { Dog. Kennel kennel = new Dog. Kennel( ); } } //--- Output --- Cage Zoo Animal Dog Poodle Animal Dog Collie Dog. Kennel Aug 6, 2007 4
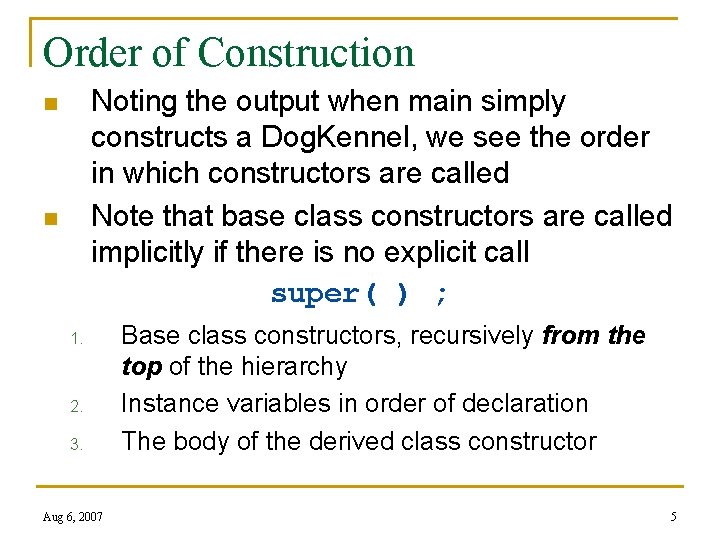
Order of Construction Noting the output when main simply constructs a Dog. Kennel, we see the order in which constructors are called Note that base class constructors are called implicitly if there is no explicit call super( ) ; n n 1. 2. 3. Aug 6, 2007 Base class constructors, recursively from the top of the hierarchy Instance variables in order of declaration The body of the derived class constructor 5
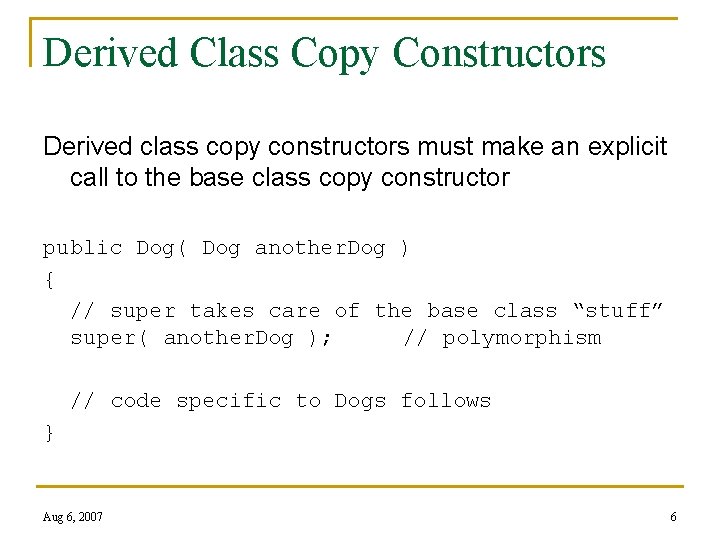
Derived Class Copy Constructors Derived class copy constructors must make an explicit call to the base class copy constructor public Dog( Dog another. Dog ) { // super takes care of the base class “stuff” super( another. Dog ); // polymorphism // code specific to Dogs follows } Aug 6, 2007 6
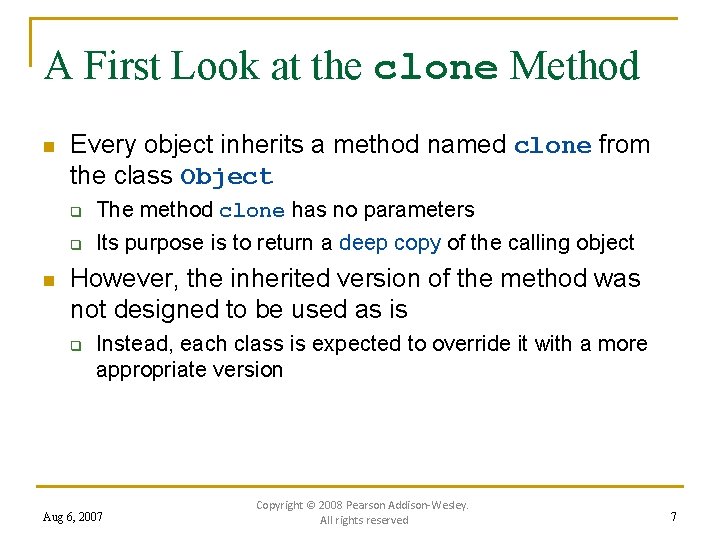
A First Look at the clone Method n n Every object inherits a method named clone from the class Object q The method clone has no parameters q Its purpose is to return a deep copy of the calling object However, the inherited version of the method was not designed to be used as is q Instead, each class is expected to override it with a more appropriate version Aug 6, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 7
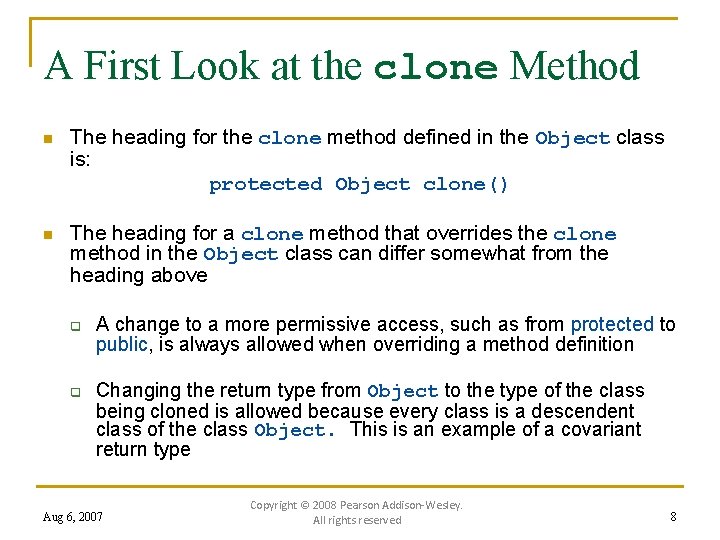
A First Look at the clone Method n The heading for the clone method defined in the Object class is: protected Object clone() n The heading for a clone method that overrides the clone method in the Object class can differ somewhat from the heading above q q A change to a more permissive access, such as from protected to public, is always allowed when overriding a method definition Changing the return type from Object to the type of the class being cloned is allowed because every class is a descendent class of the class Object. This is an example of a covariant return type Aug 6, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 8
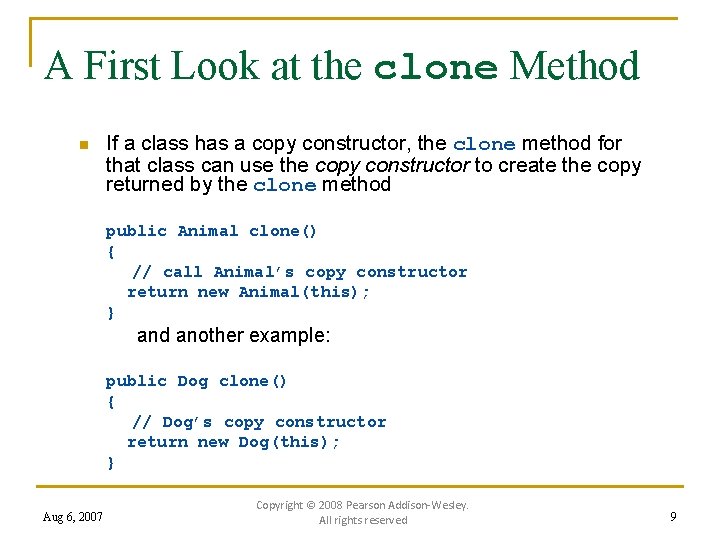
A First Look at the clone Method n If a class has a copy constructor, the clone method for that class can use the copy constructor to create the copy returned by the clone method public Animal clone() { // call Animal’s copy constructor return new Animal(this); } and another example: public Dog clone() { // Dog’s copy constructor return new Dog(this); } Aug 6, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 9
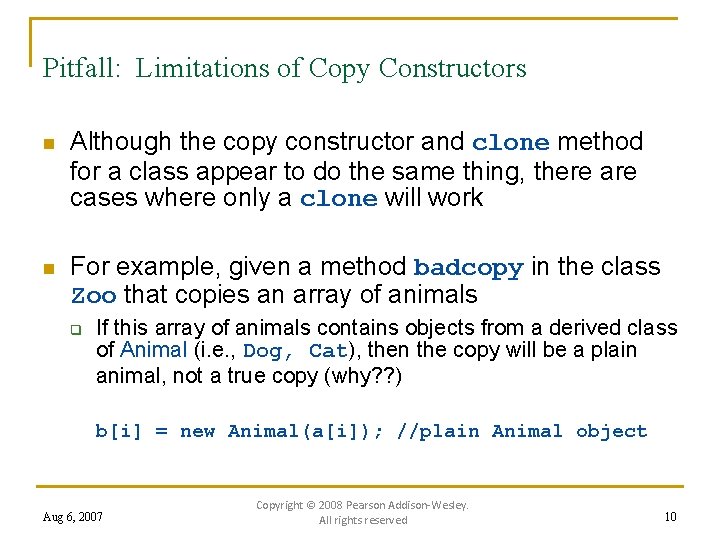
Pitfall: Limitations of Copy Constructors n Although the copy constructor and clone method for a class appear to do the same thing, there are cases where only a clone will work n For example, given a method badcopy in the class Zoo that copies an array of animals q If this array of animals contains objects from a derived class of Animal (i. e. , Dog, Cat), then the copy will be a plain animal, not a true copy (why? ? ) b[i] = new Animal(a[i]); //plain Animal object Aug 6, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 10
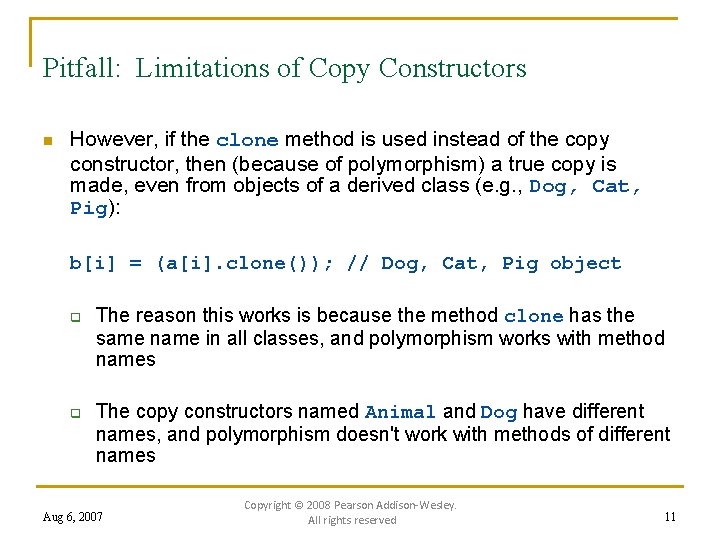
Pitfall: Limitations of Copy Constructors n However, if the clone method is used instead of the copy constructor, then (because of polymorphism) a true copy is made, even from objects of a derived class (e. g. , Dog, Cat, Pig): b[i] = (a[i]. clone()); // Dog, Cat, Pig object q q The reason this works is because the method clone has the same name in all classes, and polymorphism works with method names The copy constructors named Animal and Dog have different names, and polymorphism doesn't work with methods of different names Aug 6, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 11
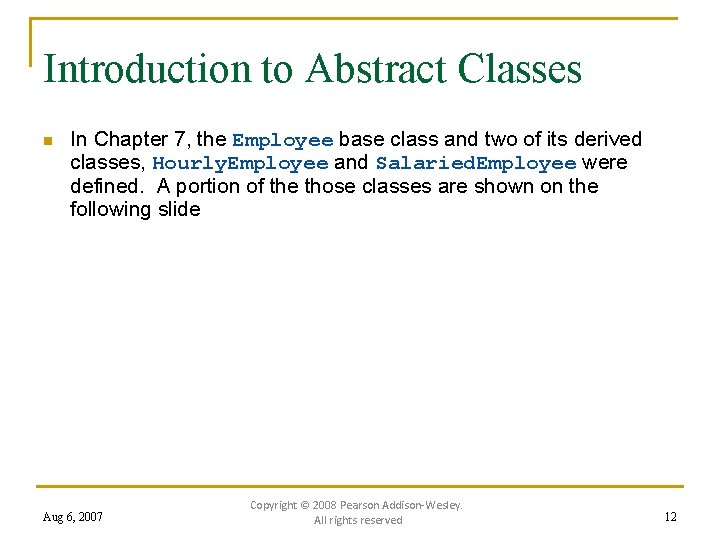
Introduction to Abstract Classes n In Chapter 7, the Employee base class and two of its derived classes, Hourly. Employee and Salaried. Employee were defined. A portion of the those classes are shown on the following slide Aug 6, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 12
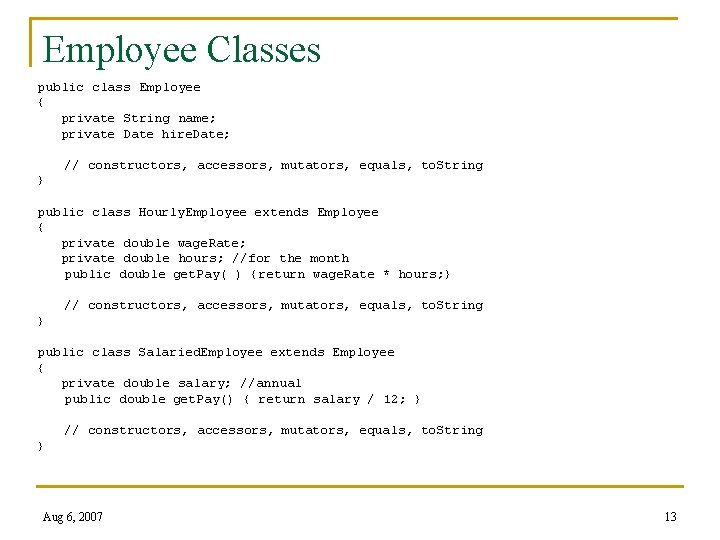
Employee Classes public class Employee { private String name; private Date hire. Date; // constructors, accessors, mutators, equals, to. String } public class Hourly. Employee extends Employee { private double wage. Rate; private double hours; //for the month public double get. Pay( ) {return wage. Rate * hours; } // constructors, accessors, mutators, equals, to. String } public class Salaried. Employee extends Employee { private double salary; //annual public double get. Pay() { return salary / 12; } // constructors, accessors, mutators, equals, to. String } Aug 6, 2007 13
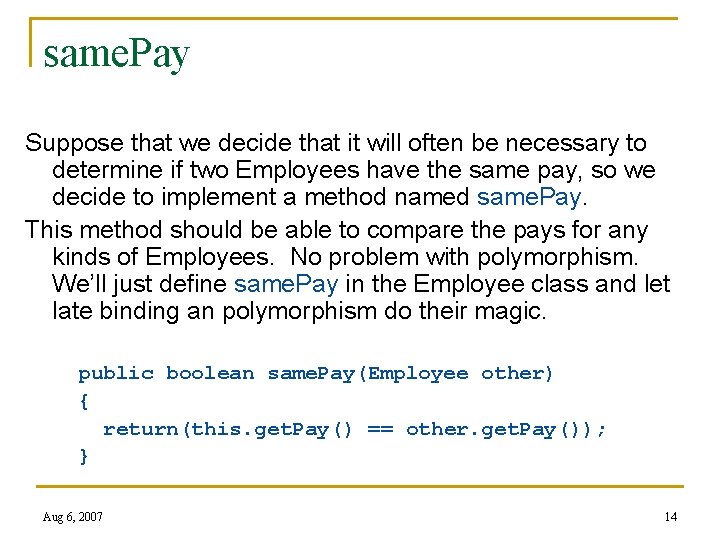
same. Pay Suppose that we decide that it will often be necessary to determine if two Employees have the same pay, so we decide to implement a method named same. Pay. This method should be able to compare the pays for any kinds of Employees. No problem with polymorphism. We’ll just define same. Pay in the Employee class and let late binding an polymorphism do their magic. public boolean same. Pay(Employee other) { return(this. get. Pay() == other. get. Pay()); } Aug 6, 2007 14
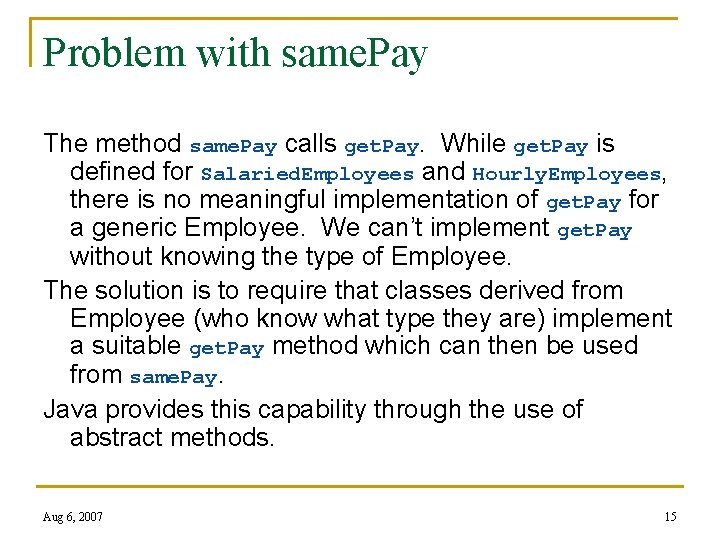
Problem with same. Pay The method same. Pay calls get. Pay. While get. Pay is defined for Salaried. Employees and Hourly. Employees, there is no meaningful implementation of get. Pay for a generic Employee. We can’t implement get. Pay without knowing the type of Employee. The solution is to require that classes derived from Employee (who know what type they are) implement a suitable get. Pay method which can then be used from same. Pay. Java provides this capability through the use of abstract methods. Aug 6, 2007 15
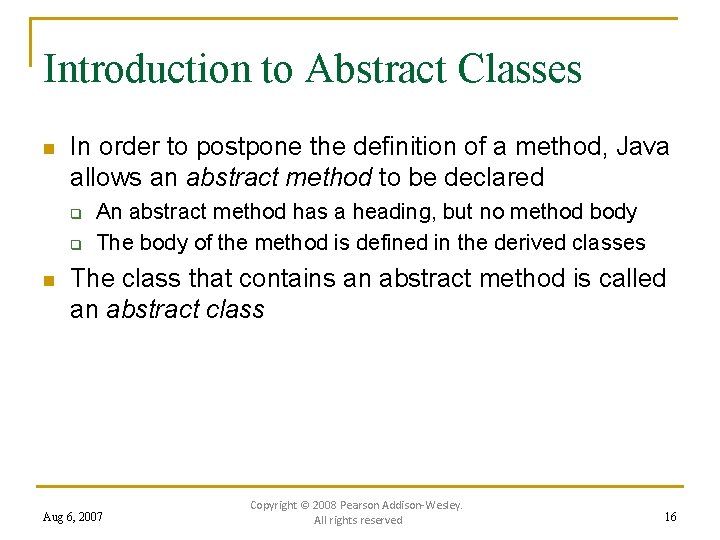
Introduction to Abstract Classes n In order to postpone the definition of a method, Java allows an abstract method to be declared q q n An abstract method has a heading, but no method body The body of the method is defined in the derived classes The class that contains an abstract method is called an abstract class Aug 6, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 16
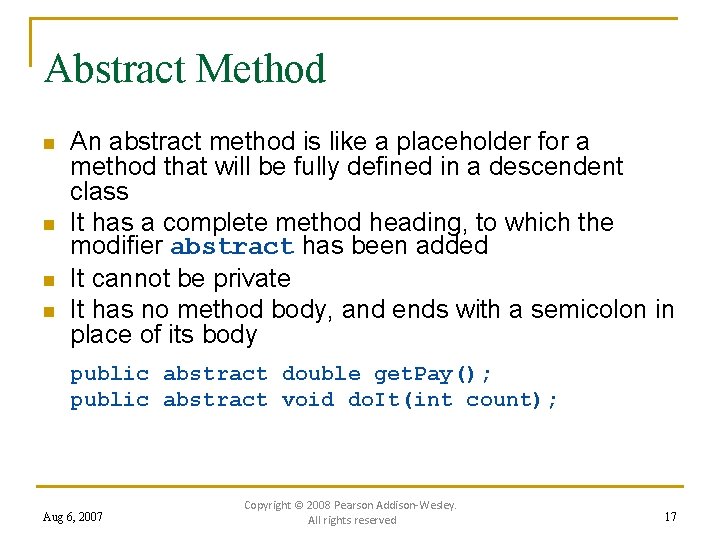
Abstract Method n n An abstract method is like a placeholder for a method that will be fully defined in a descendent class It has a complete method heading, to which the modifier abstract has been added It cannot be private It has no method body, and ends with a semicolon in place of its body public abstract double get. Pay(); public abstract void do. It(int count); Aug 6, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 17
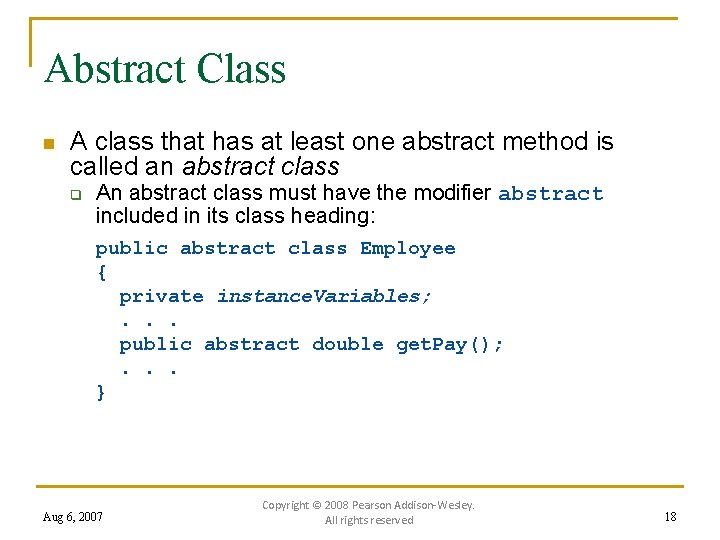
Abstract Class n A class that has at least one abstract method is called an abstract class q An abstract class must have the modifier abstract included in its class heading: public abstract class Employee { private instance. Variables; . . . public abstract double get. Pay(); . . . } Aug 6, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 18
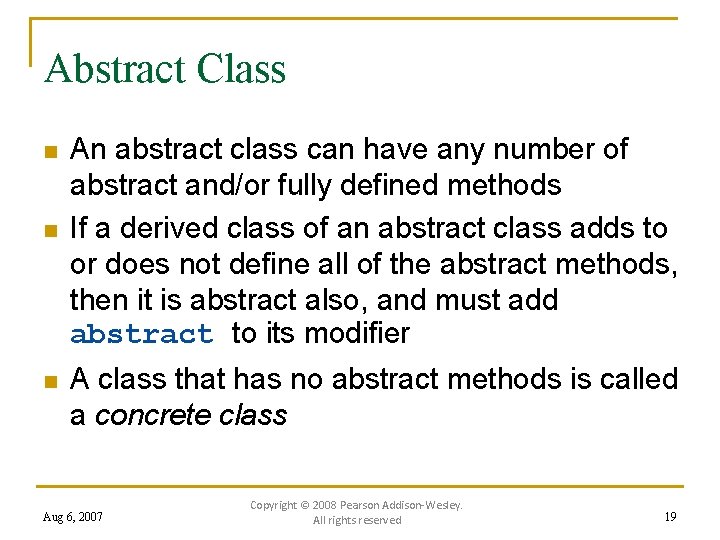
Abstract Class n n n An abstract class can have any number of abstract and/or fully defined methods If a derived class of an abstract class adds to or does not define all of the abstract methods, then it is abstract also, and must add abstract to its modifier A class that has no abstract methods is called a concrete class Aug 6, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 19
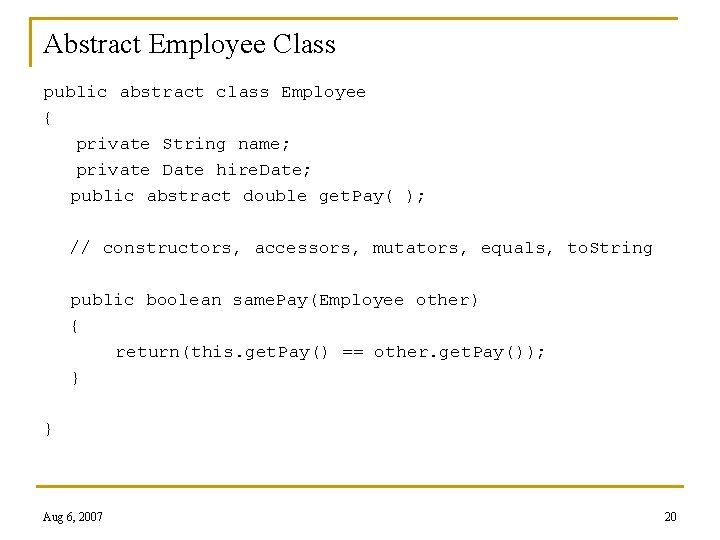
Abstract Employee Class public abstract class Employee { private String name; private Date hire. Date; public abstract double get. Pay( ); // constructors, accessors, mutators, equals, to. String public boolean same. Pay(Employee other) { return(this. get. Pay() == other. get. Pay()); } } Aug 6, 2007 20
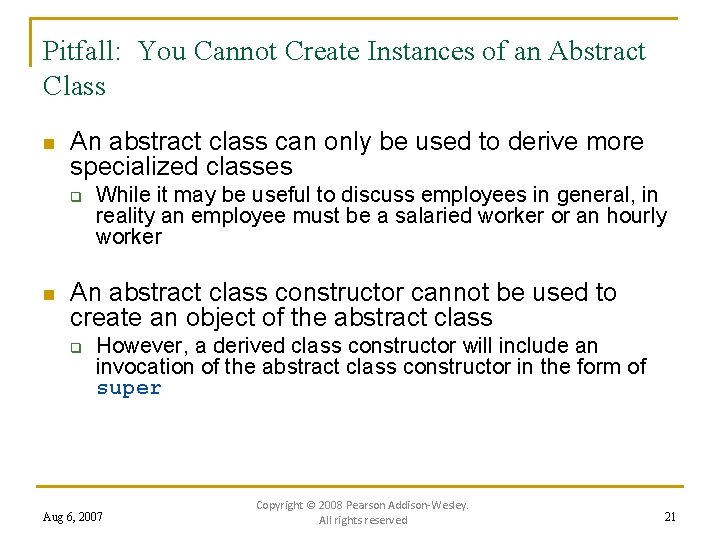
Pitfall: You Cannot Create Instances of an Abstract Class n An abstract class can only be used to derive more specialized classes q n While it may be useful to discuss employees in general, in reality an employee must be a salaried worker or an hourly worker An abstract class constructor cannot be used to create an object of the abstract class q However, a derived class constructor will include an invocation of the abstract class constructor in the form of super Aug 6, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 21
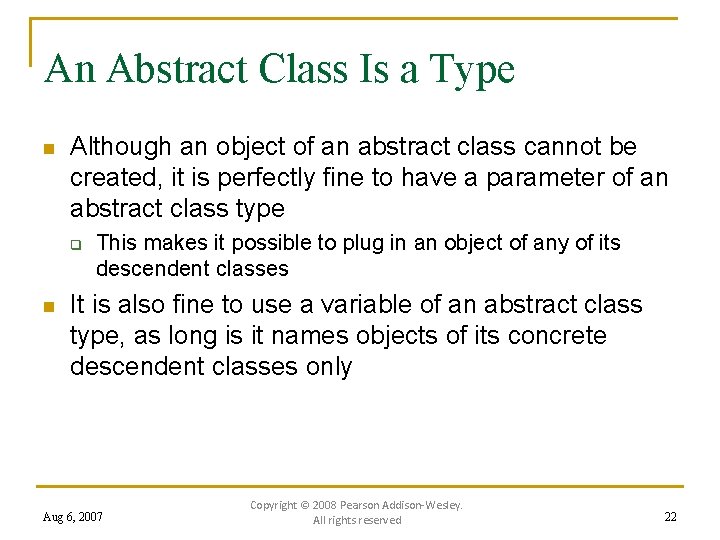
An Abstract Class Is a Type n Although an object of an abstract class cannot be created, it is perfectly fine to have a parameter of an abstract class type q n This makes it possible to plug in an object of any of its descendent classes It is also fine to use a variable of an abstract class type, as long is it names objects of its concrete descendent classes only Aug 6, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 22
Umbc cmsc 202
Umbc cmsc 202
Aug q
Translation
Aug comm device
Dna and rna chart
Initiell
Agosto 6 1891
Aug root word
01:640:244 lecture notes - lecture 15: plat, idah, farad
Bio 202
Coe202
Cse 202
Mt 202
Ribofuranosa
192,168,0,202
Hasil dari 202-152 adalah
Cve 202
Chn-202
Bcd to excess 3
Aud-202
Superposition electric circuits
Lcm of 2 and 12