Chap 16 Transactions Transactions n n n Transaction
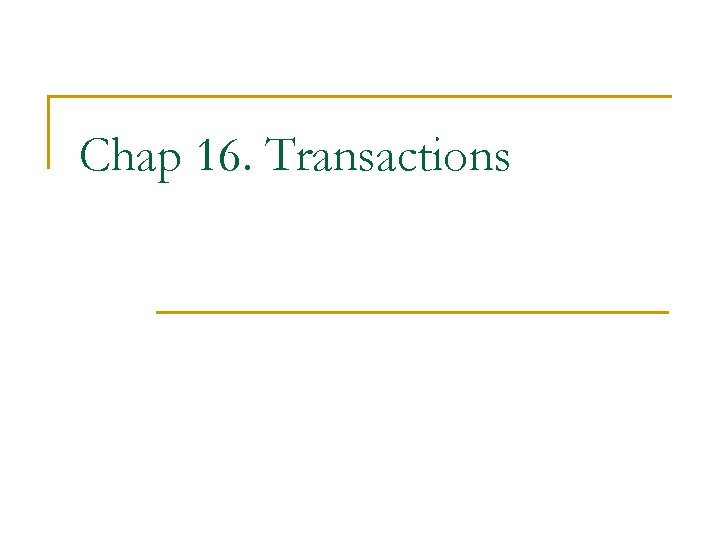
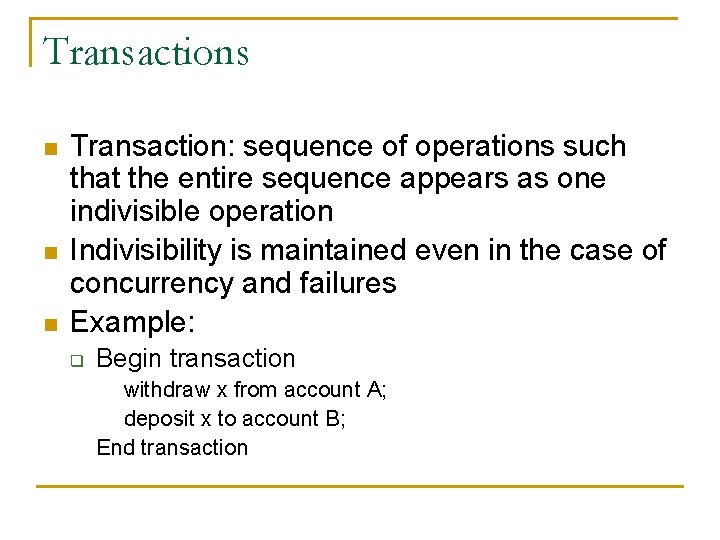
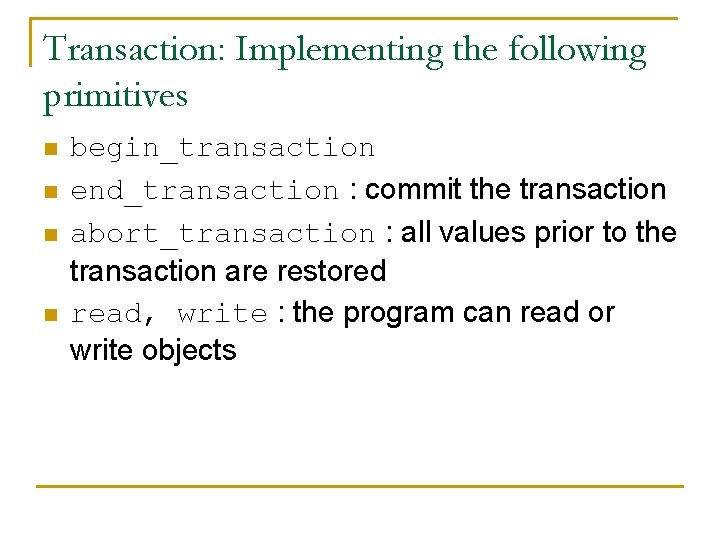
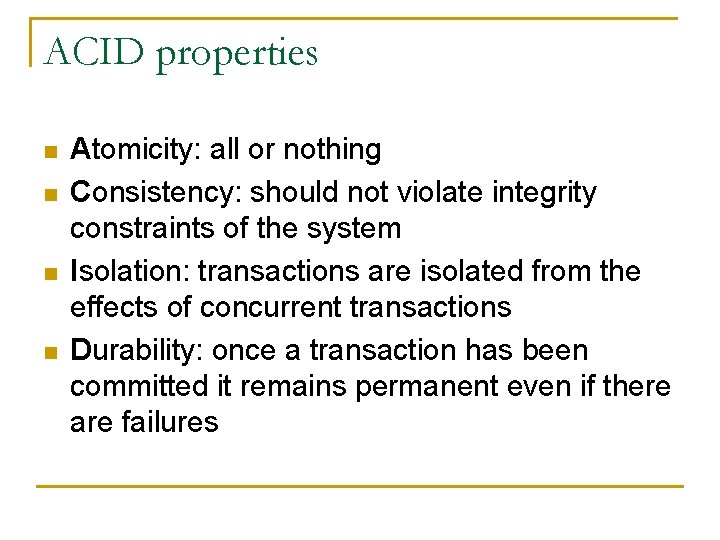
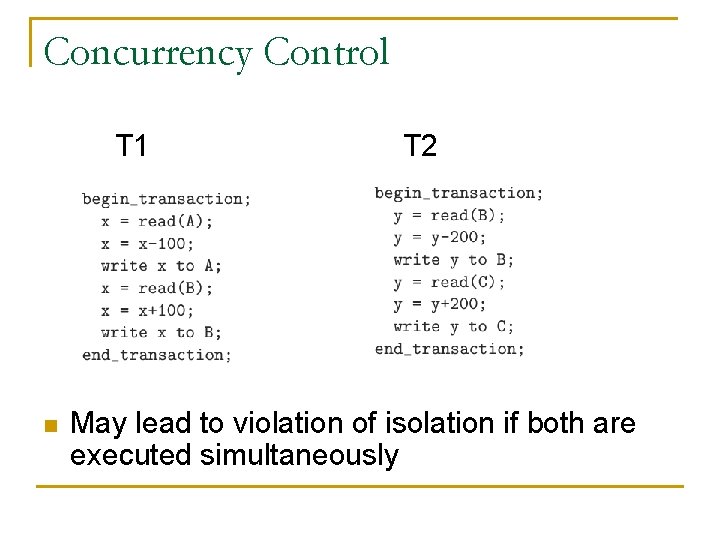
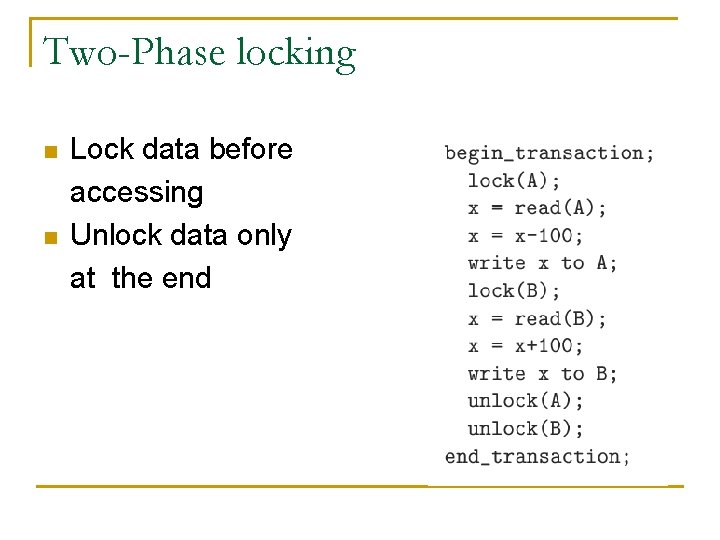
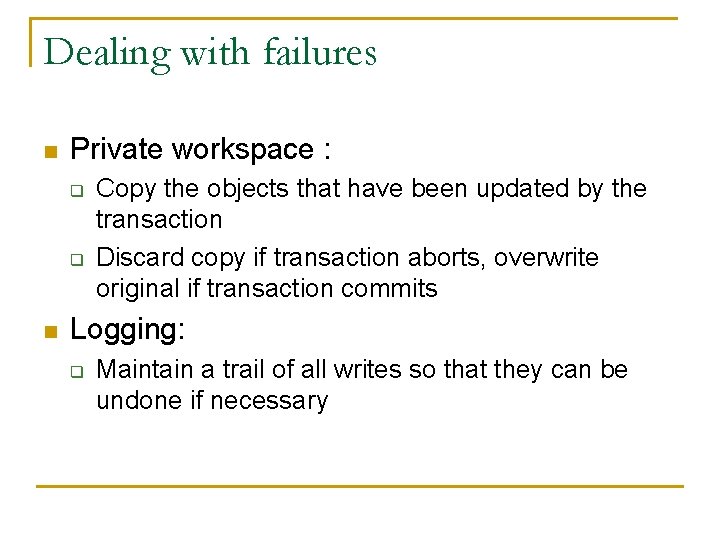
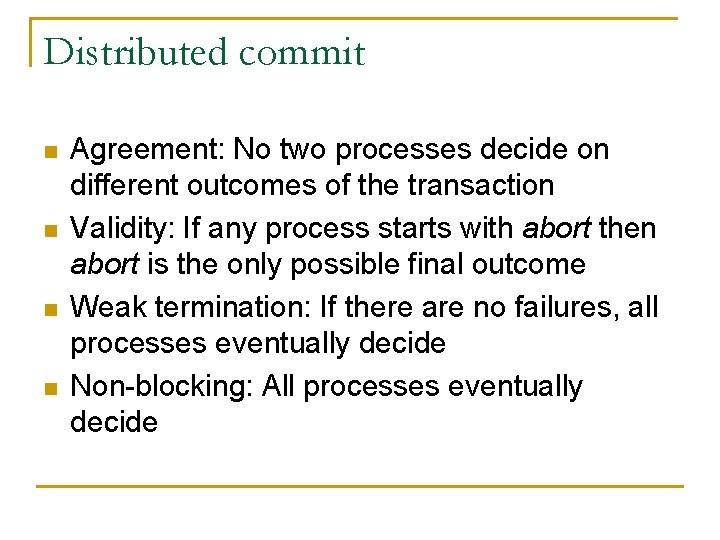
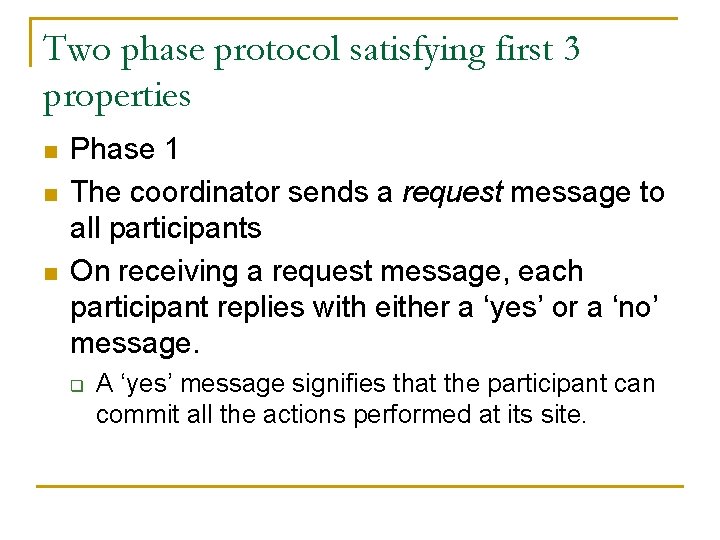
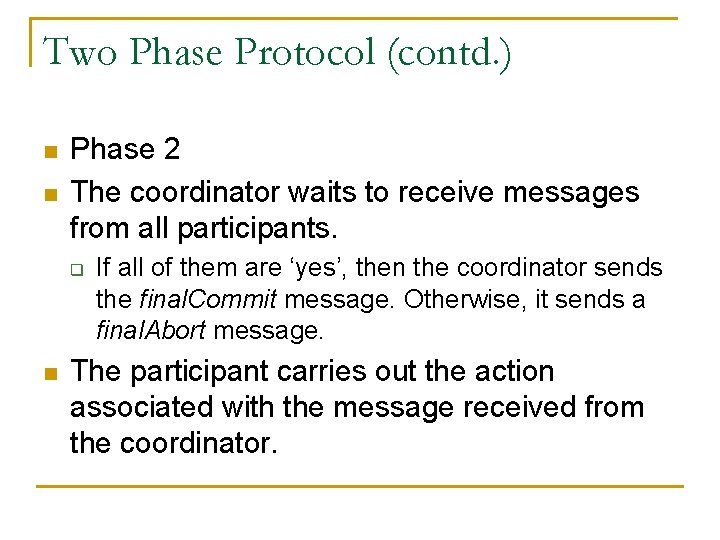
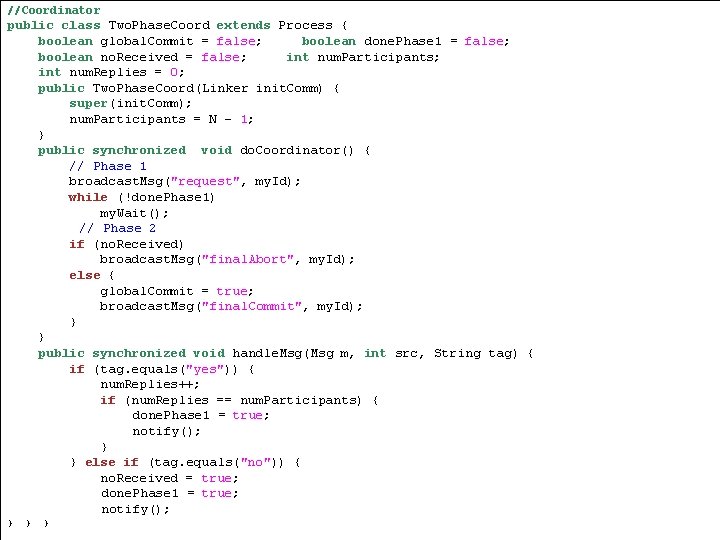
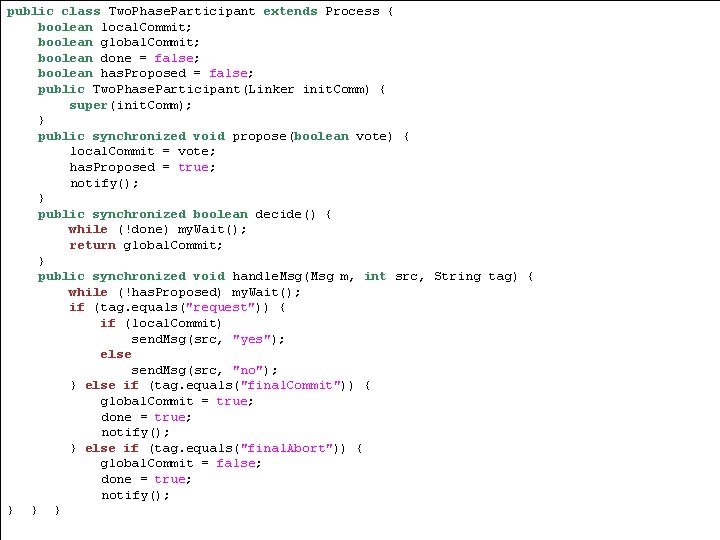
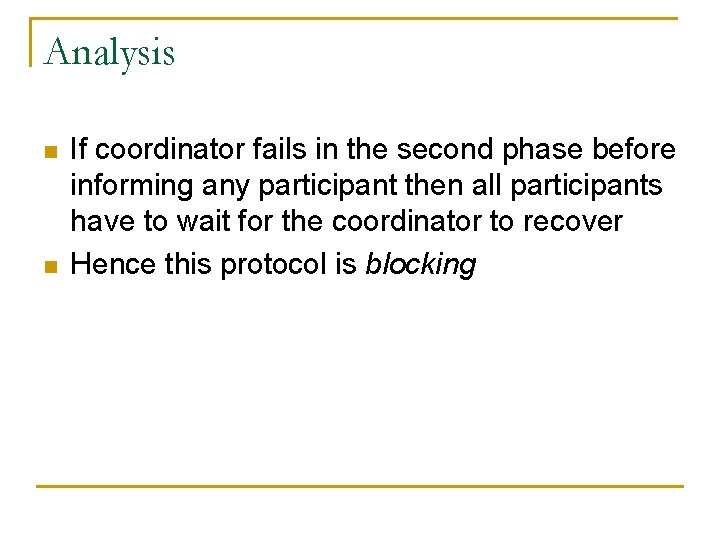
- Slides: 13
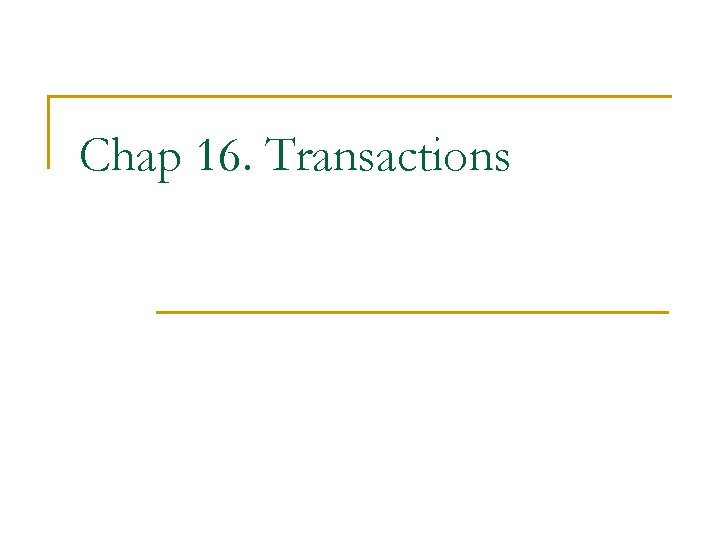
Chap 16. Transactions
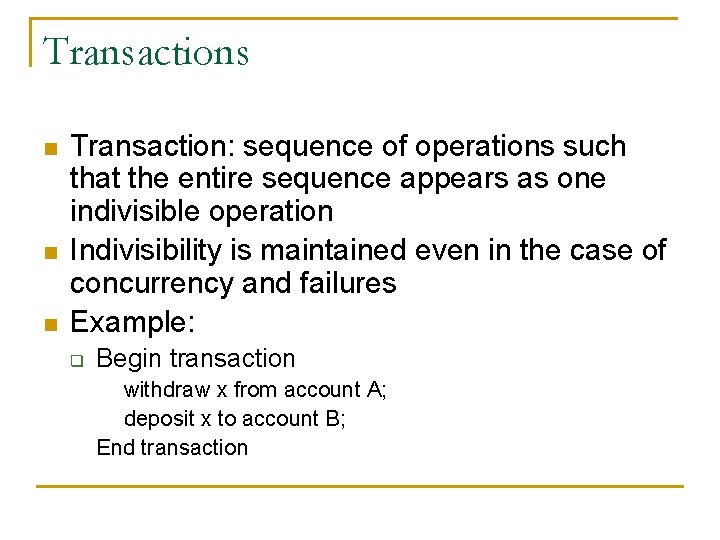
Transactions n n n Transaction: sequence of operations such that the entire sequence appears as one indivisible operation Indivisibility is maintained even in the case of concurrency and failures Example: q Begin transaction withdraw x from account A; deposit x to account B; End transaction
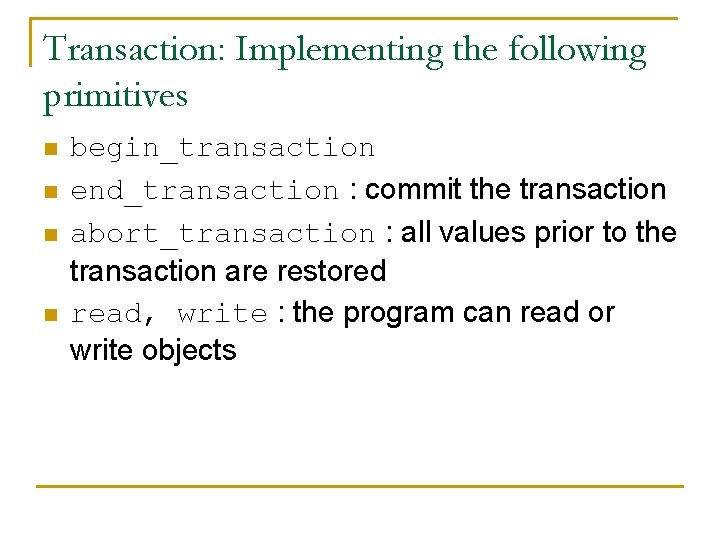
Transaction: Implementing the following primitives n n begin_transaction end_transaction : commit the transaction abort_transaction : all values prior to the transaction are restored read, write : the program can read or write objects
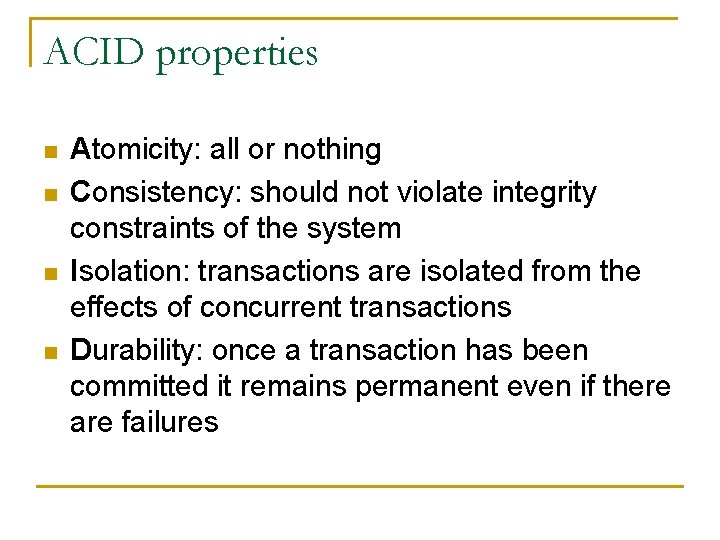
ACID properties n n Atomicity: all or nothing Consistency: should not violate integrity constraints of the system Isolation: transactions are isolated from the effects of concurrent transactions Durability: once a transaction has been committed it remains permanent even if there are failures
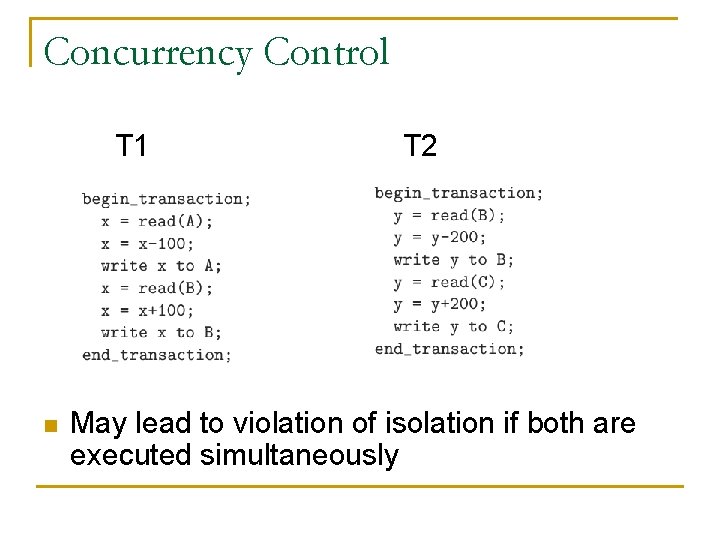
Concurrency Control T 1 n T 2 May lead to violation of isolation if both are executed simultaneously
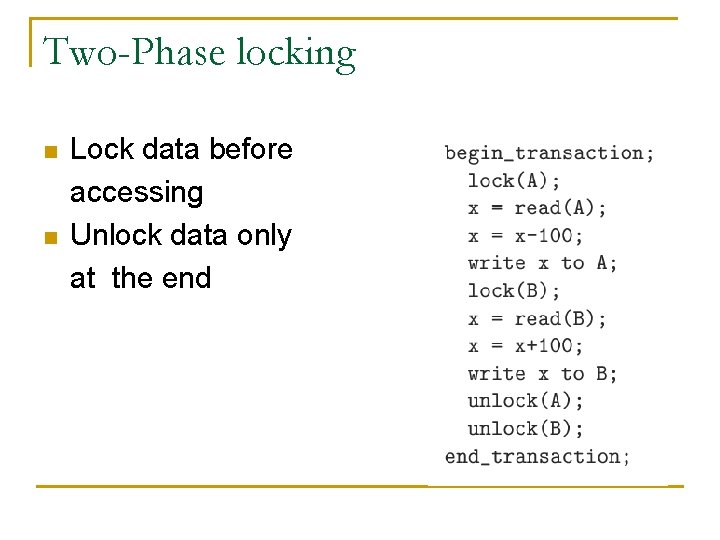
Two-Phase locking n n Lock data before accessing Unlock data only at the end
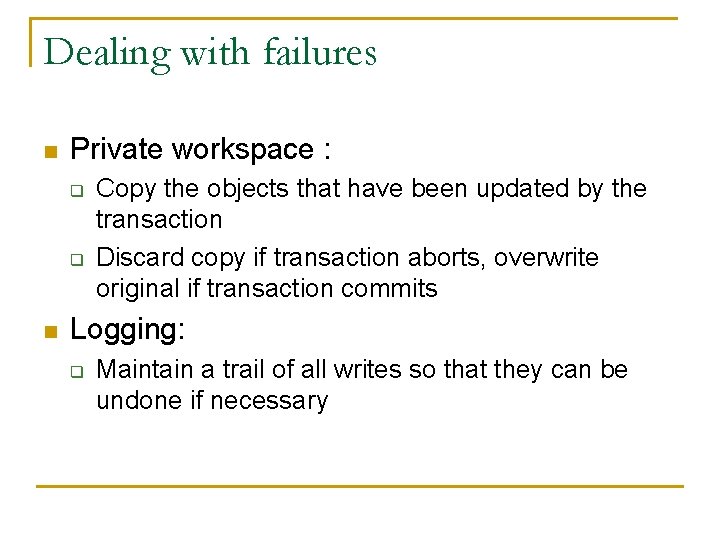
Dealing with failures n Private workspace : q q n Copy the objects that have been updated by the transaction Discard copy if transaction aborts, overwrite original if transaction commits Logging: q Maintain a trail of all writes so that they can be undone if necessary
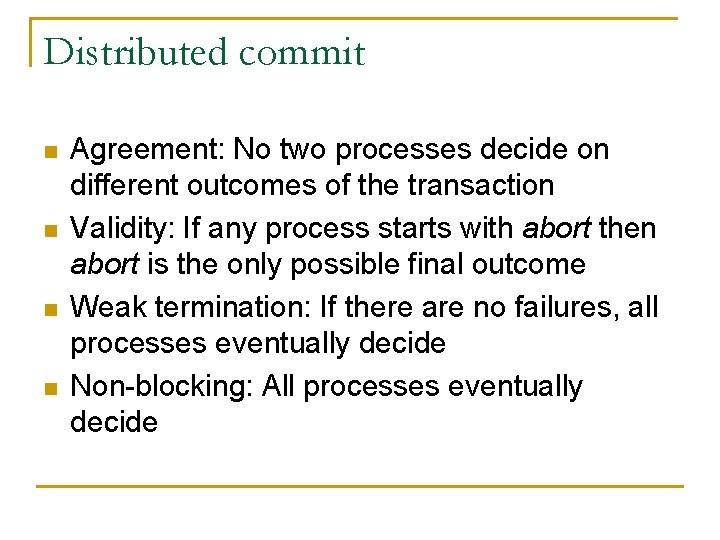
Distributed commit n n Agreement: No two processes decide on different outcomes of the transaction Validity: If any process starts with abort then abort is the only possible final outcome Weak termination: If there are no failures, all processes eventually decide Non-blocking: All processes eventually decide
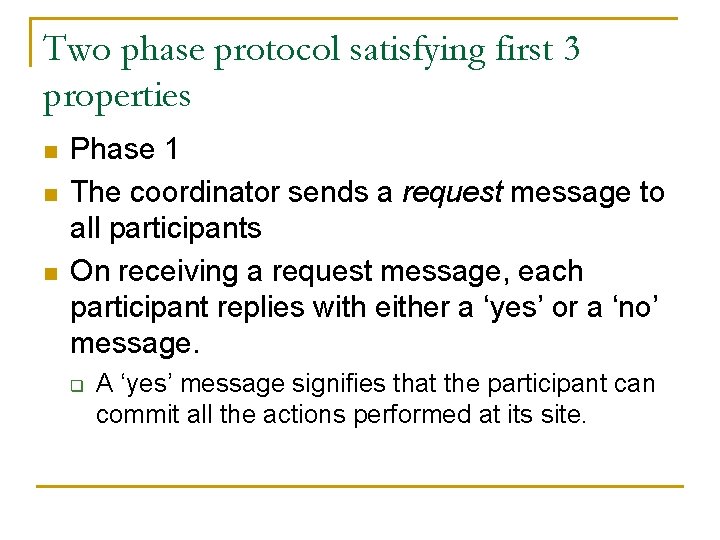
Two phase protocol satisfying first 3 properties n n n Phase 1 The coordinator sends a request message to all participants On receiving a request message, each participant replies with either a ‘yes’ or a ‘no’ message. q A ‘yes’ message signifies that the participant can commit all the actions performed at its site.
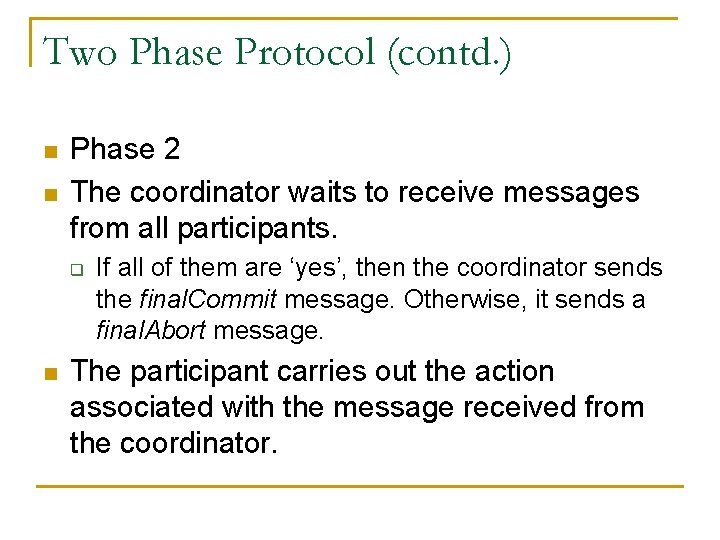
Two Phase Protocol (contd. ) n n Phase 2 The coordinator waits to receive messages from all participants. q n If all of them are ‘yes’, then the coordinator sends the final. Commit message. Otherwise, it sends a final. Abort message. The participant carries out the action associated with the message received from the coordinator.
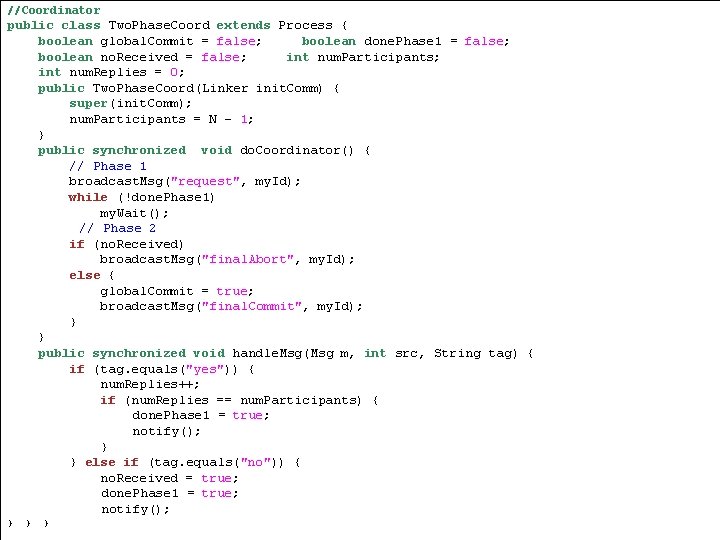
//Coordinator public class Two. Phase. Coord extends Process { boolean global. Commit = false; boolean done. Phase 1 = false; boolean no. Received = false; int num. Participants; int num. Replies = 0; public Two. Phase. Coord(Linker init. Comm) { super(init. Comm); num. Participants = N - 1; } public synchronized void do. Coordinator() { // Phase 1 broadcast. Msg("request", my. Id); while (!done. Phase 1) my. Wait(); // Phase 2 if (no. Received) broadcast. Msg("final. Abort", my. Id); else { global. Commit = true; broadcast. Msg("final. Commit", my. Id); } } public synchronized void handle. Msg(Msg m, int src, String tag) { if (tag. equals("yes")) { num. Replies++; if (num. Replies == num. Participants) { done. Phase 1 = true; notify(); } } else if (tag. equals("no")) { no. Received = true; done. Phase 1 = true; notify(); } } }
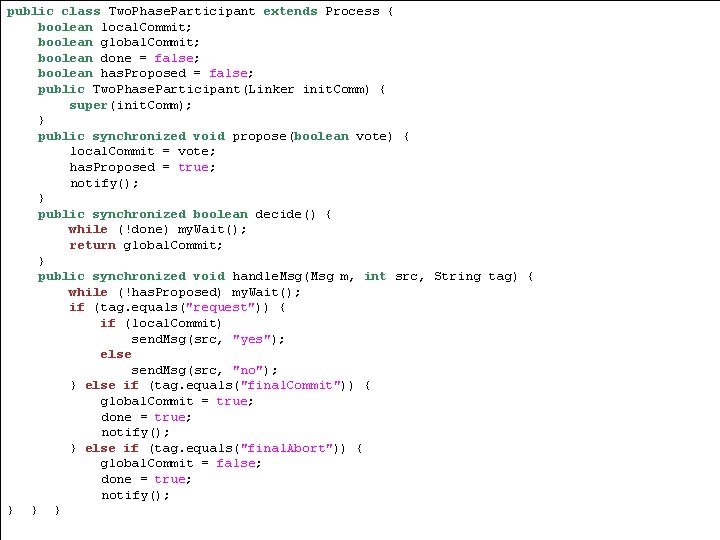
public class Two. Phase. Participant extends Process { boolean local. Commit; boolean global. Commit; boolean done = false; boolean has. Proposed = false; public Two. Phase. Participant(Linker init. Comm) { super(init. Comm); } public synchronized void propose(boolean vote) { local. Commit = vote; has. Proposed = true; notify(); } public synchronized boolean decide() { while (!done) my. Wait(); return global. Commit; } public synchronized void handle. Msg(Msg m, int src, String tag) { while (!has. Proposed) my. Wait(); if (tag. equals("request")) { if (local. Commit) send. Msg(src, "yes"); else send. Msg(src, "no"); } else if (tag. equals("final. Commit")) { global. Commit = true; done = true; notify(); } else if (tag. equals("final. Abort")) { global. Commit = false; done = true; notify(); } } }
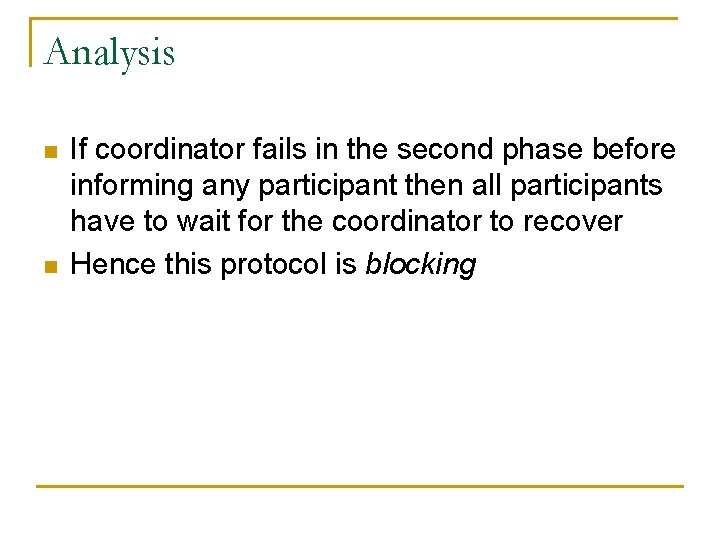
Analysis n n If coordinator fails in the second phase before informing any participant then all participants have to wait for the coordinator to recover Hence this protocol is blocking
Chap chap slide
Responsible relationships are based on
Autocorrelation in econometrics
To not die chap 18
Need for speed payback chapter 9
Friendly relationship chapter 12
I was in that state when a chap easily turns nasty analysis
Fitness chapter 1
The origin of species chapter 19 manhwa
Chap counter
Style chapter 1
What is the fundamental challenge of dashboard design?
Youjup
Chap 23