Applications of Shift and Rotate Instructions Sahar Mosleh
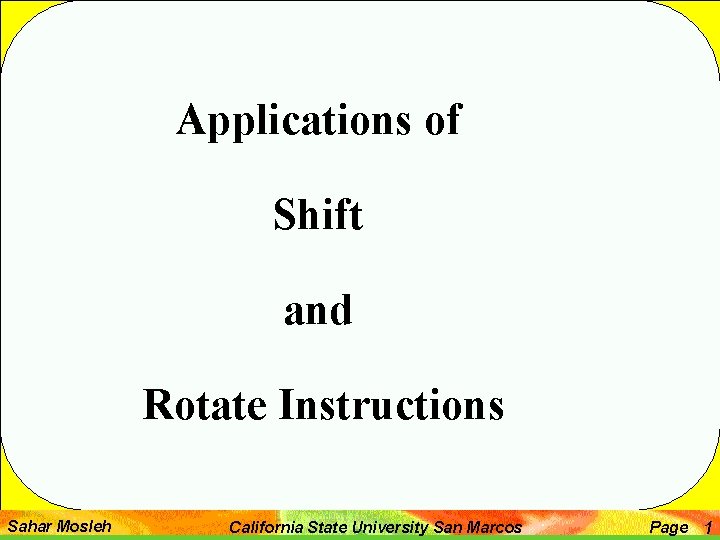
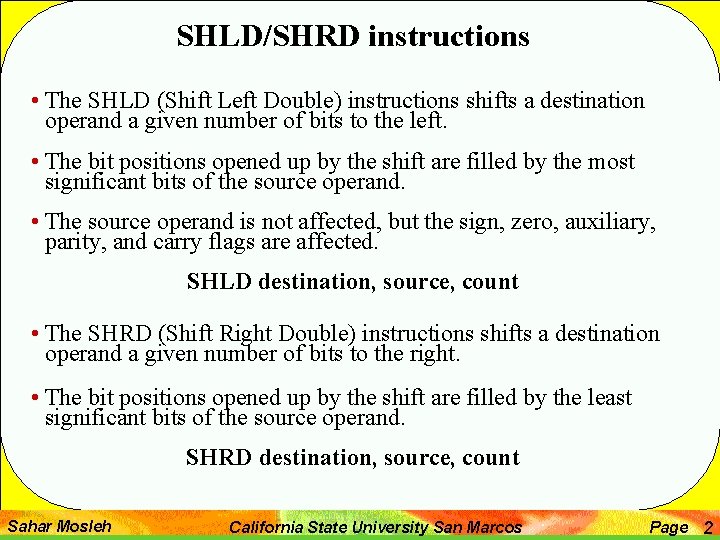
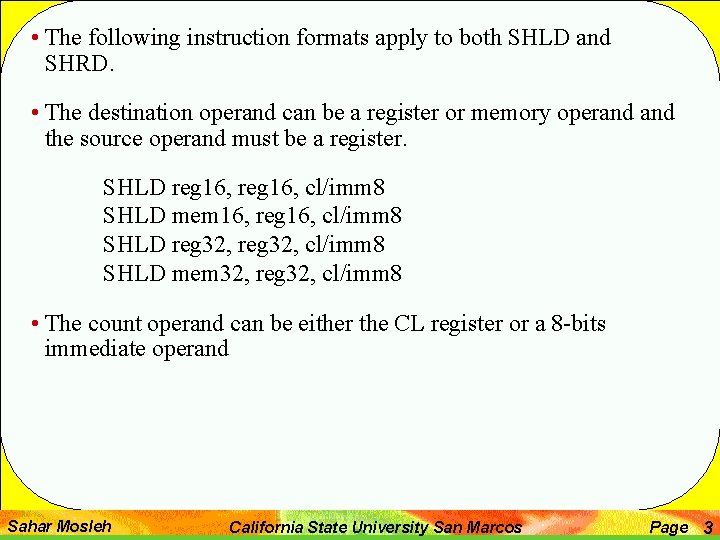
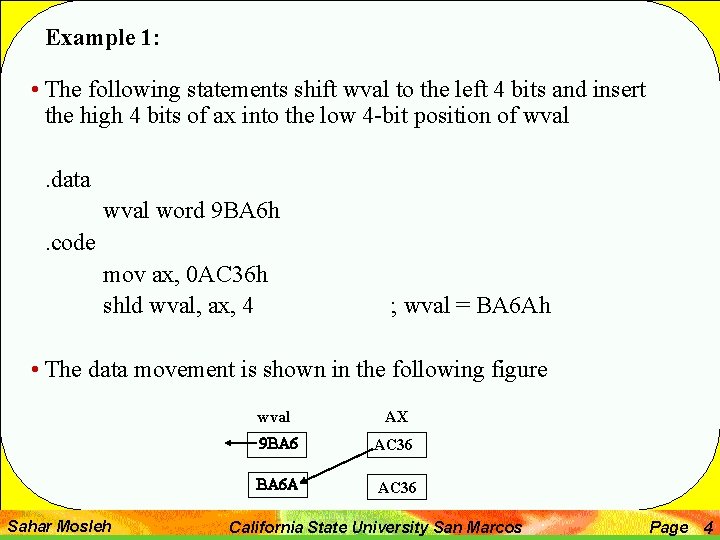
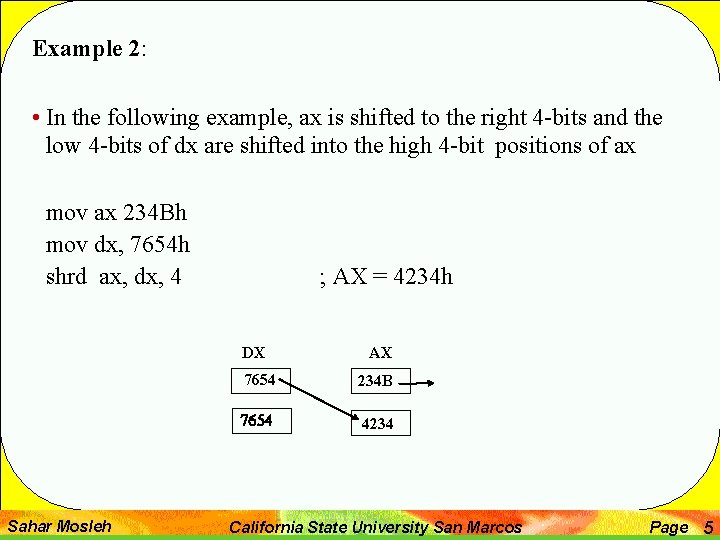
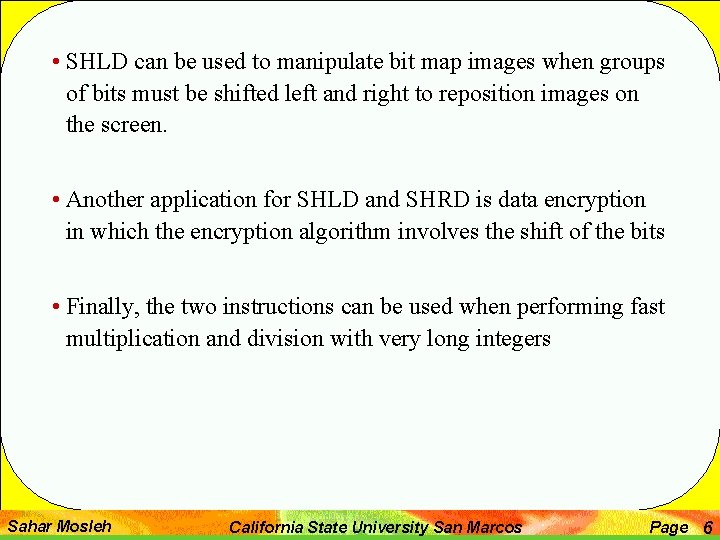
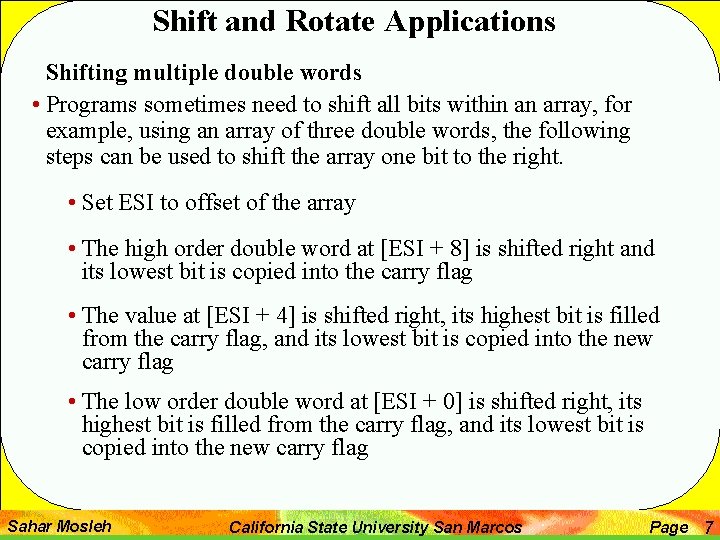
![99999999 h [esi] [esi+4] 9999 h [esi+8] . data array. Size = 3 array 99999999 h [esi] [esi+4] 9999 h [esi+8] . data array. Size = 3 array](https://slidetodoc.com/presentation_image_h2/375d517c96e0934f281b1e632d24261b/image-8.jpg)
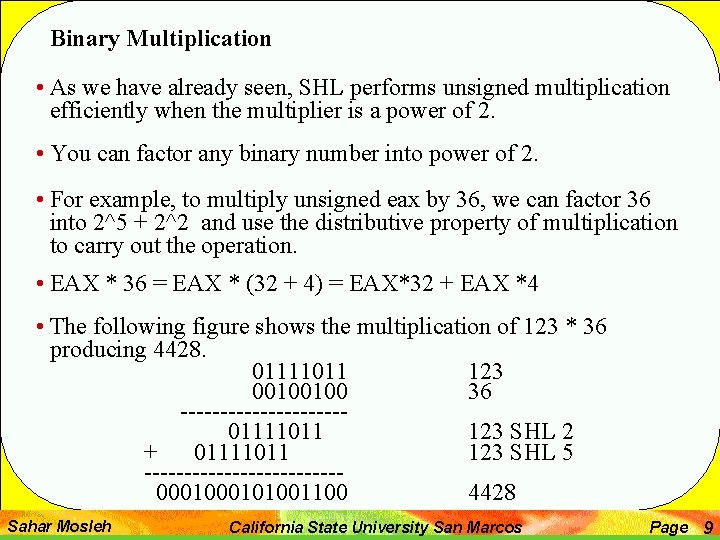
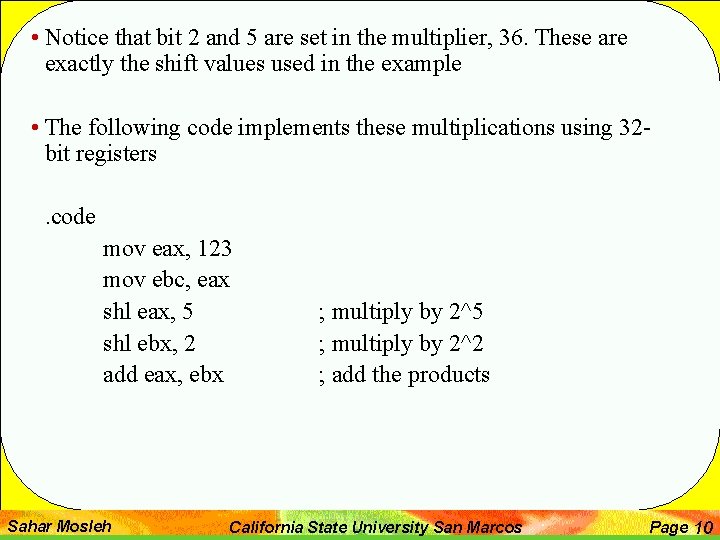
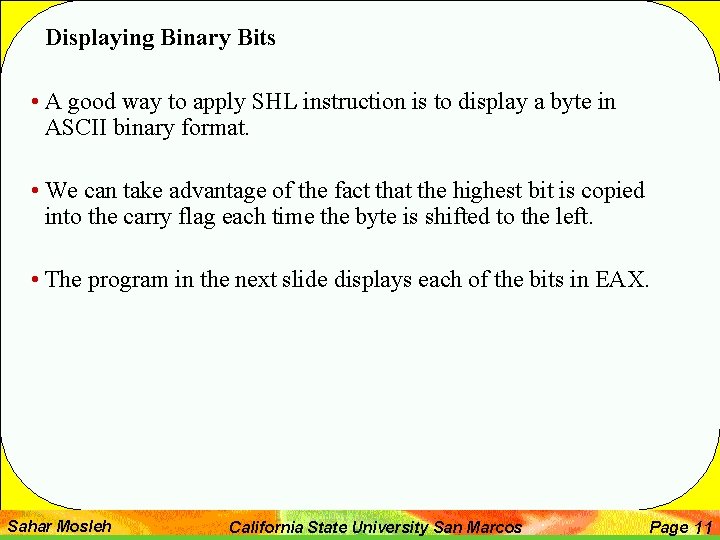
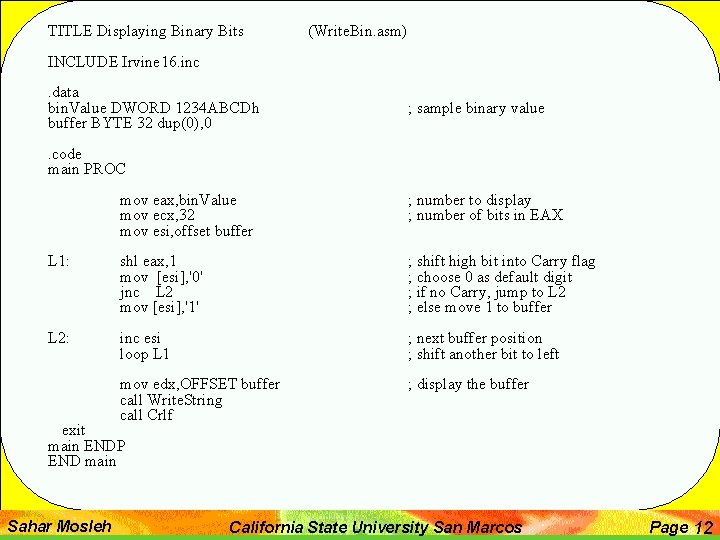
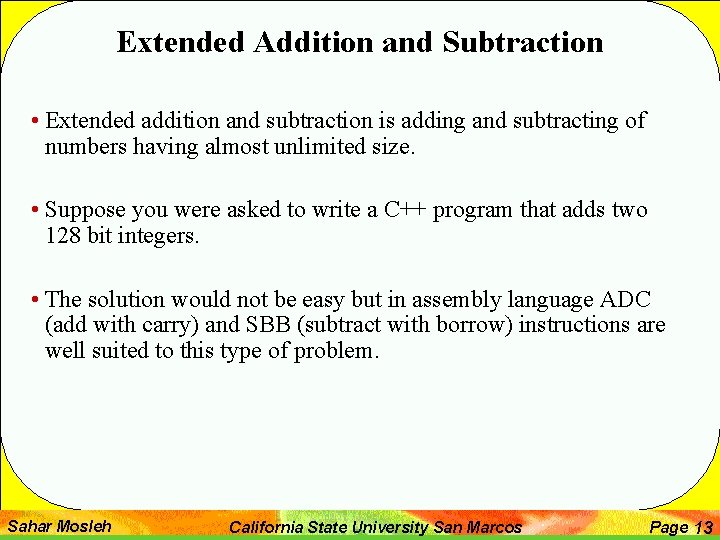
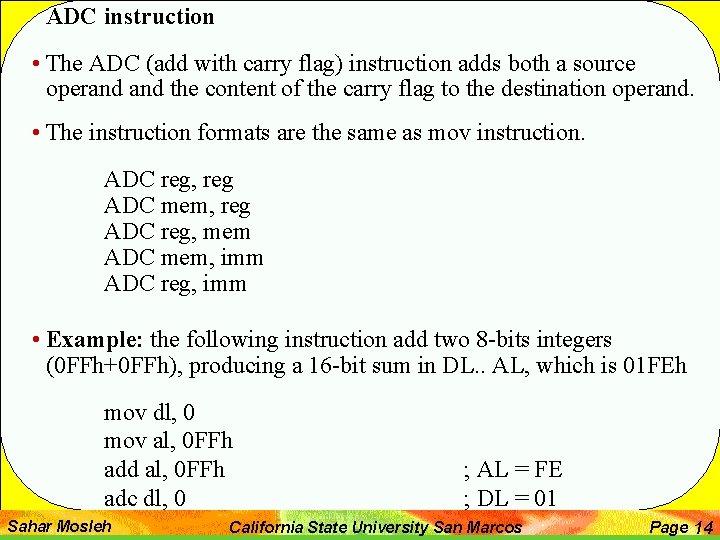
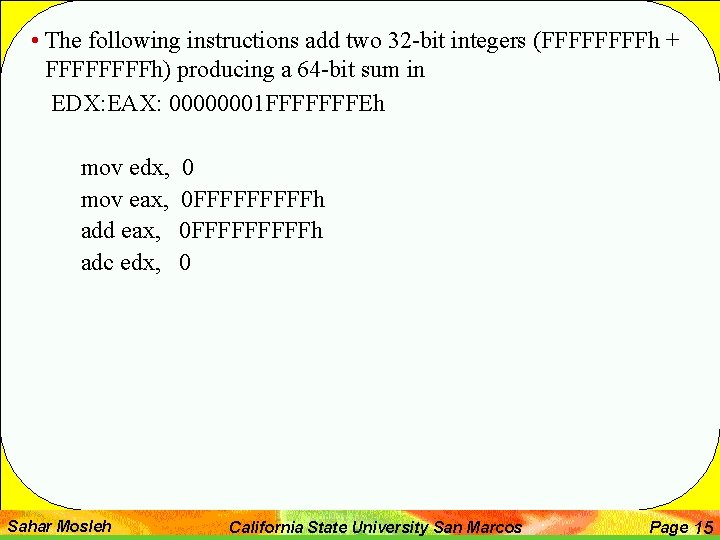
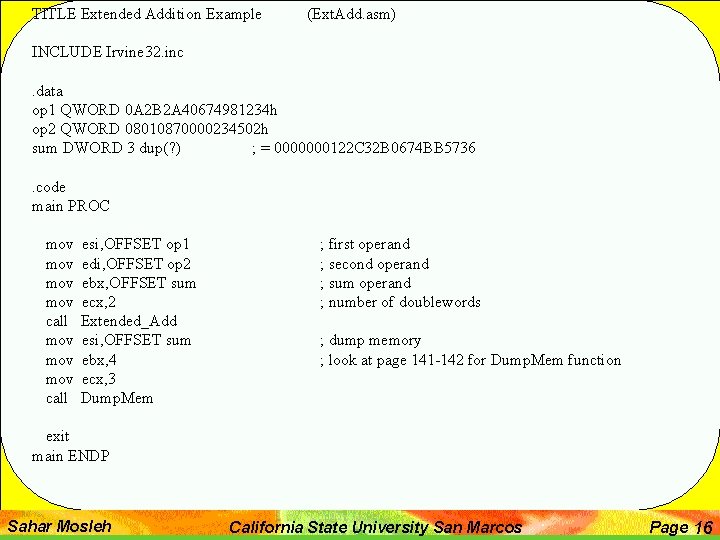
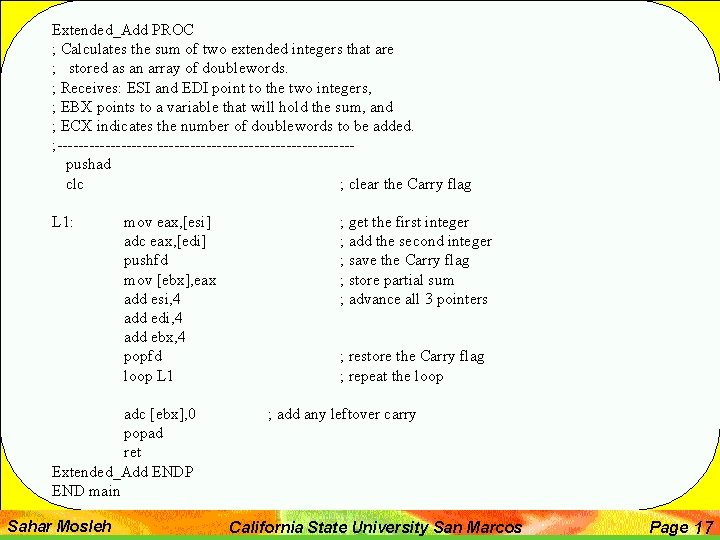
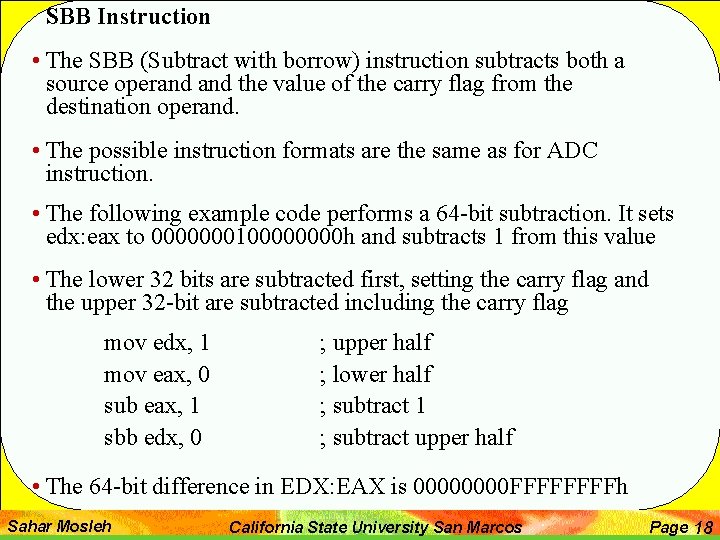
- Slides: 18
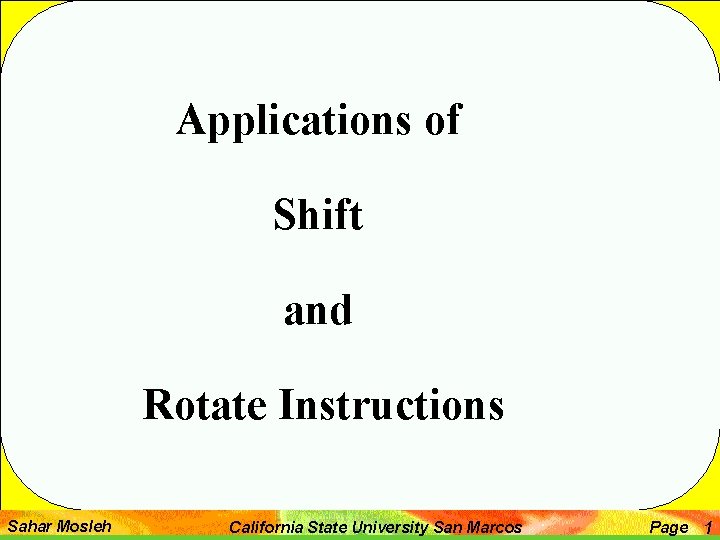
Applications of Shift and Rotate Instructions Sahar Mosleh California State University San Marcos Page 1
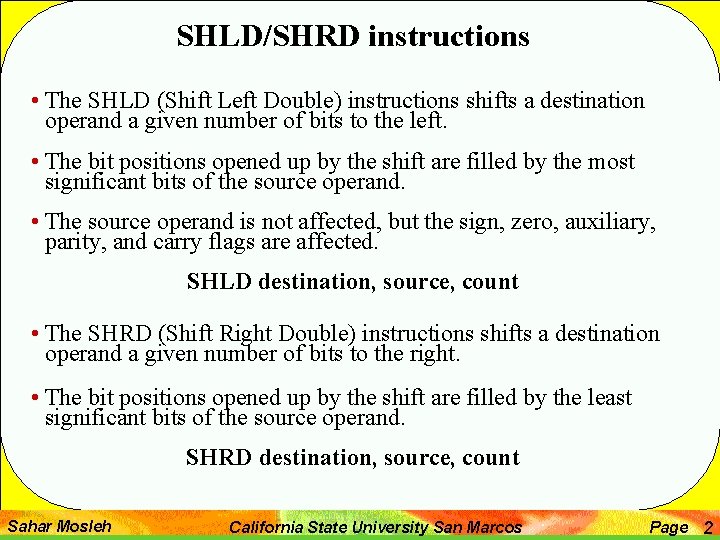
SHLD/SHRD instructions • The SHLD (Shift Left Double) instructions shifts a destination operand a given number of bits to the left. • The bit positions opened up by the shift are filled by the most significant bits of the source operand. • The source operand is not affected, but the sign, zero, auxiliary, parity, and carry flags are affected. SHLD destination, source, count • The SHRD (Shift Right Double) instructions shifts a destination operand a given number of bits to the right. • The bit positions opened up by the shift are filled by the least significant bits of the source operand. SHRD destination, source, count Sahar Mosleh California State University San Marcos Page 2
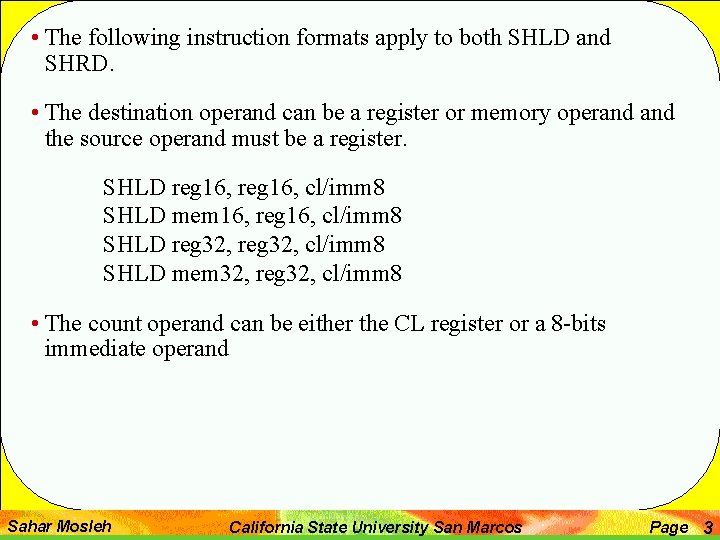
• The following instruction formats apply to both SHLD and SHRD. • The destination operand can be a register or memory operand the source operand must be a register. SHLD reg 16, cl/imm 8 SHLD mem 16, reg 16, cl/imm 8 SHLD reg 32, cl/imm 8 SHLD mem 32, reg 32, cl/imm 8 • The count operand can be either the CL register or a 8 -bits immediate operand Sahar Mosleh California State University San Marcos Page 3
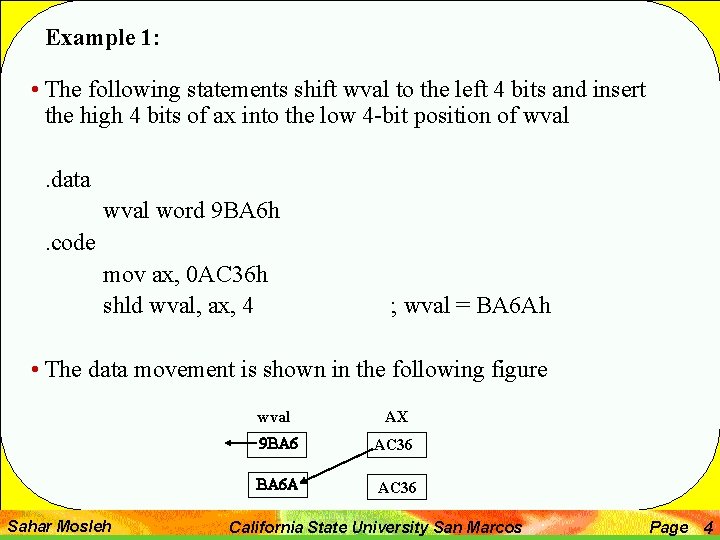
Example 1: • The following statements shift wval to the left 4 bits and insert the high 4 bits of ax into the low 4 -bit position of wval. data wval word 9 BA 6 h. code mov ax, 0 AC 36 h shld wval, ax, 4 ; wval = BA 6 Ah • The data movement is shown in the following figure wval Sahar Mosleh AX 9 BA 6 AC 36 BA 6 A AC 36 California State University San Marcos Page 4
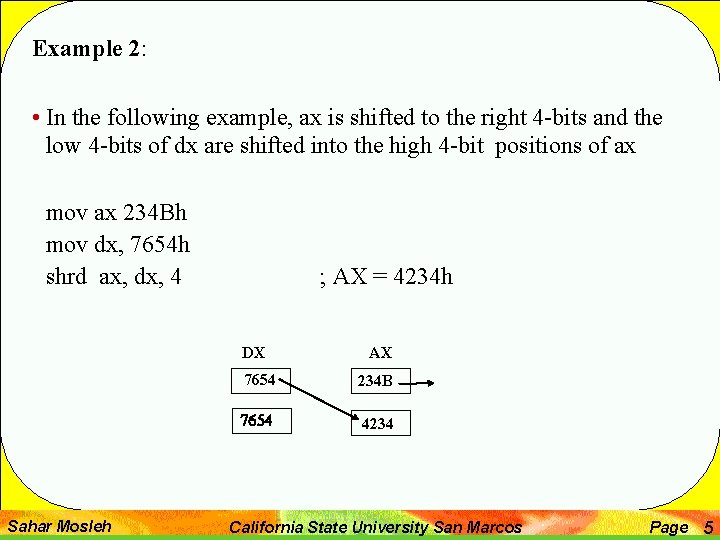
Example 2: • In the following example, ax is shifted to the right 4 -bits and the low 4 -bits of dx are shifted into the high 4 -bit positions of ax mov ax 234 Bh mov dx, 7654 h shrd ax, dx, 4 ; AX = 4234 h DX Sahar Mosleh AX 7654 234 B 7654 4234 California State University San Marcos Page 5
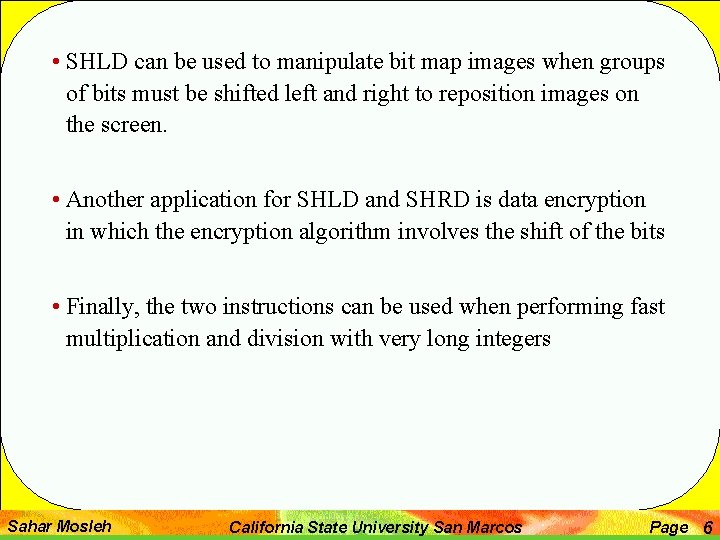
• SHLD can be used to manipulate bit map images when groups of bits must be shifted left and right to reposition images on the screen. • Another application for SHLD and SHRD is data encryption in which the encryption algorithm involves the shift of the bits • Finally, the two instructions can be used when performing fast multiplication and division with very long integers Sahar Mosleh California State University San Marcos Page 6
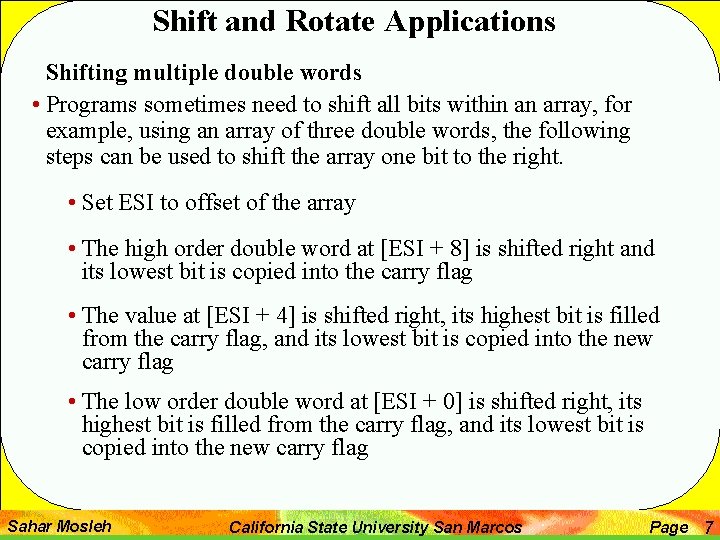
Shift and Rotate Applications Shifting multiple double words • Programs sometimes need to shift all bits within an array, for example, using an array of three double words, the following steps can be used to shift the array one bit to the right. • Set ESI to offset of the array • The high order double word at [ESI + 8] is shifted right and its lowest bit is copied into the carry flag • The value at [ESI + 4] is shifted right, its highest bit is filled from the carry flag, and its lowest bit is copied into the new carry flag • The low order double word at [ESI + 0] is shifted right, its highest bit is filled from the carry flag, and its lowest bit is copied into the new carry flag Sahar Mosleh California State University San Marcos Page 7
![99999999 h esi esi4 9999 h esi8 data array Size 3 array 99999999 h [esi] [esi+4] 9999 h [esi+8] . data array. Size = 3 array](https://slidetodoc.com/presentation_image_h2/375d517c96e0934f281b1e632d24261b/image-8.jpg)
99999999 h [esi] [esi+4] 9999 h [esi+8] . data array. Size = 3 array DWORD array. Size DUP (9999 h) ; 1001. code mov esi, offset array add esi, 8 mov ebx, [esi] ; high dword shr ebx, 1 sub esi, 4 mov ebx, [esi] ; middle dword, include carry rcr ebx, 1 sub esi, 4 mov ebx, [esi] ; low dword, include Carry rcr ebx, 1 The program output shows the number in binary before and after shift 1001 1001 1001. . . ect 0100 1100 1100 1100. . . ect Sahar Mosleh California State University San Marcos Page 8
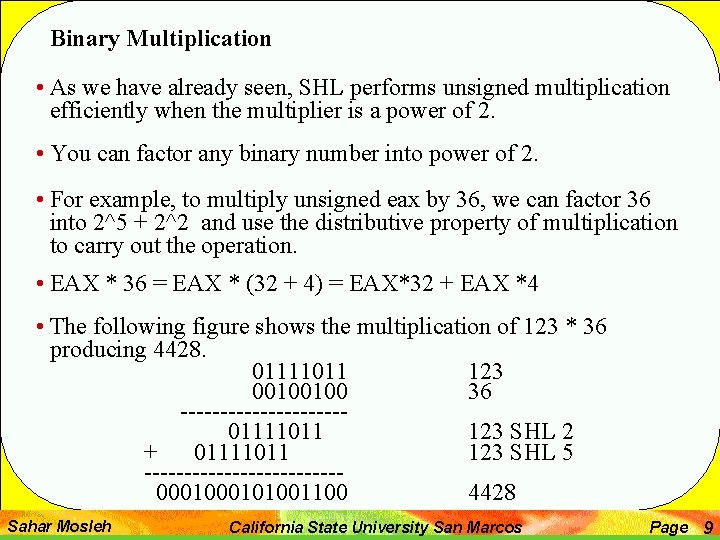
Binary Multiplication • As we have already seen, SHL performs unsigned multiplication efficiently when the multiplier is a power of 2. • You can factor any binary number into power of 2. • For example, to multiply unsigned eax by 36, we can factor 36 into 2^5 + 2^2 and use the distributive property of multiplication to carry out the operation. • EAX * 36 = EAX * (32 + 4) = EAX*32 + EAX *4 • The following figure shows the multiplication of 123 * 36 producing 4428. 01111011 123 00100100 36 ----------01111011 123 SHL 2 + 01111011 123 SHL 5 ------------000101001100 4428 Sahar Mosleh California State University San Marcos Page 9
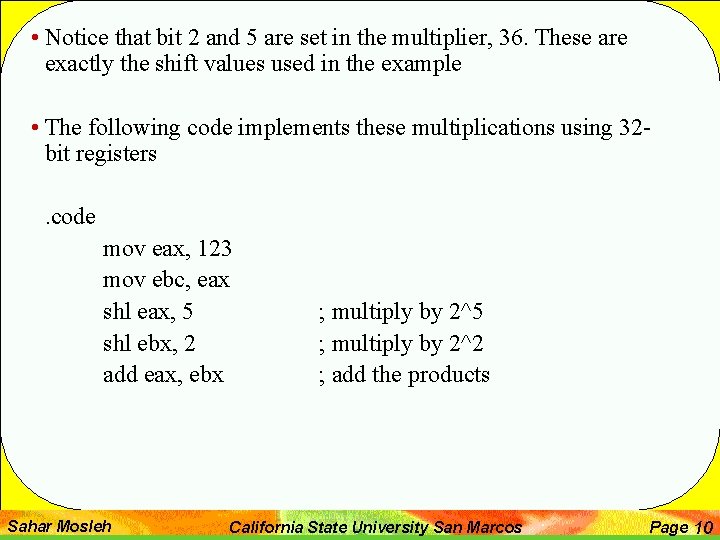
• Notice that bit 2 and 5 are set in the multiplier, 36. These are exactly the shift values used in the example • The following code implements these multiplications using 32 bit registers. code mov eax, 123 mov ebc, eax shl eax, 5 shl ebx, 2 add eax, ebx Sahar Mosleh ; multiply by 2^5 ; multiply by 2^2 ; add the products California State University San Marcos Page 10
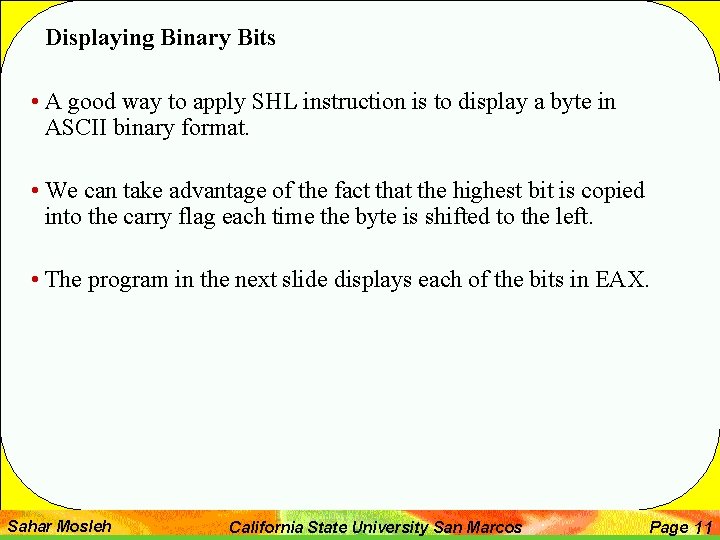
Displaying Binary Bits • A good way to apply SHL instruction is to display a byte in ASCII binary format. • We can take advantage of the fact that the highest bit is copied into the carry flag each time the byte is shifted to the left. • The program in the next slide displays each of the bits in EAX. Sahar Mosleh California State University San Marcos Page 11
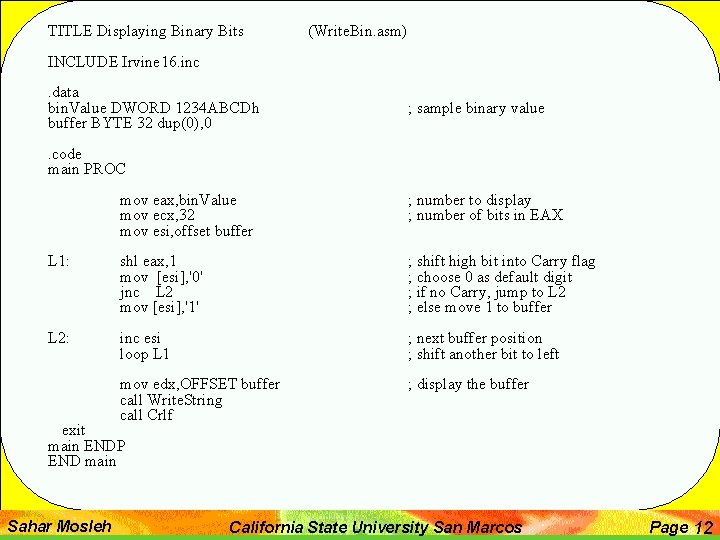
TITLE Displaying Binary Bits (Write. Bin. asm) INCLUDE Irvine 16. inc. data bin. Value DWORD 1234 ABCDh buffer BYTE 32 dup(0), 0 ; sample binary value . code main PROC mov eax, bin. Value mov ecx, 32 mov esi, offset buffer ; number to display ; number of bits in EAX L 1: shl eax, 1 mov [esi], '0' jnc L 2 mov [esi], '1' ; shift high bit into Carry flag ; choose 0 as default digit ; if no Carry, jump to L 2 ; else move 1 to buffer L 2: inc esi loop L 1 ; next buffer position ; shift another bit to left mov edx, OFFSET buffer call Write. String call Crlf ; display the buffer exit main ENDP END main Sahar Mosleh California State University San Marcos Page 12
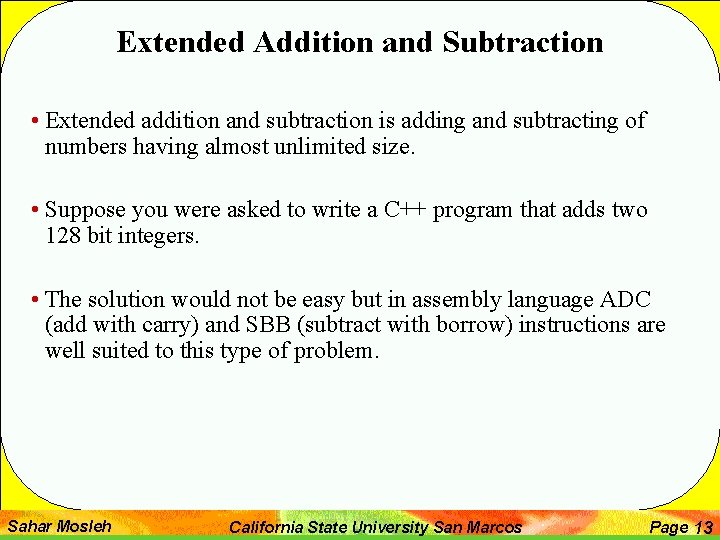
Extended Addition and Subtraction • Extended addition and subtraction is adding and subtracting of numbers having almost unlimited size. • Suppose you were asked to write a C++ program that adds two 128 bit integers. • The solution would not be easy but in assembly language ADC (add with carry) and SBB (subtract with borrow) instructions are well suited to this type of problem. Sahar Mosleh California State University San Marcos Page 13
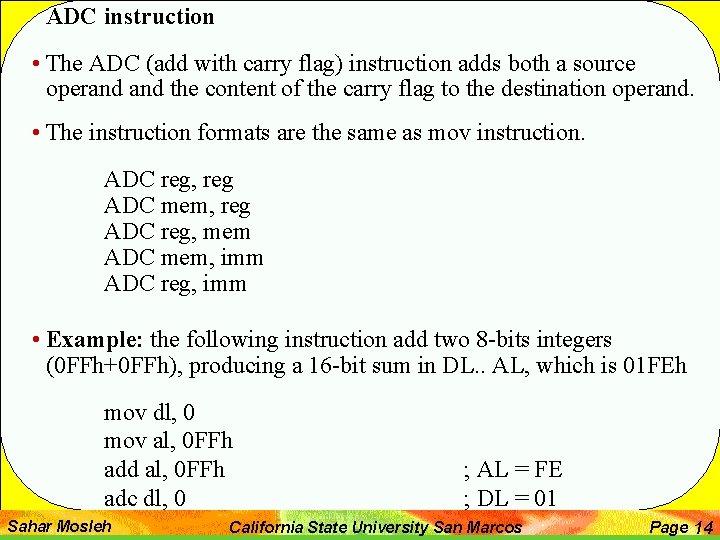
ADC instruction • The ADC (add with carry flag) instruction adds both a source operand the content of the carry flag to the destination operand. • The instruction formats are the same as mov instruction. ADC reg, reg ADC mem, reg ADC reg, mem ADC mem, imm ADC reg, imm • Example: the following instruction add two 8 -bits integers (0 FFh+0 FFh), producing a 16 -bit sum in DL. . AL, which is 01 FEh mov dl, 0 mov al, 0 FFh add al, 0 FFh adc dl, 0 Sahar Mosleh ; AL = FE ; DL = 01 California State University San Marcos Page 14
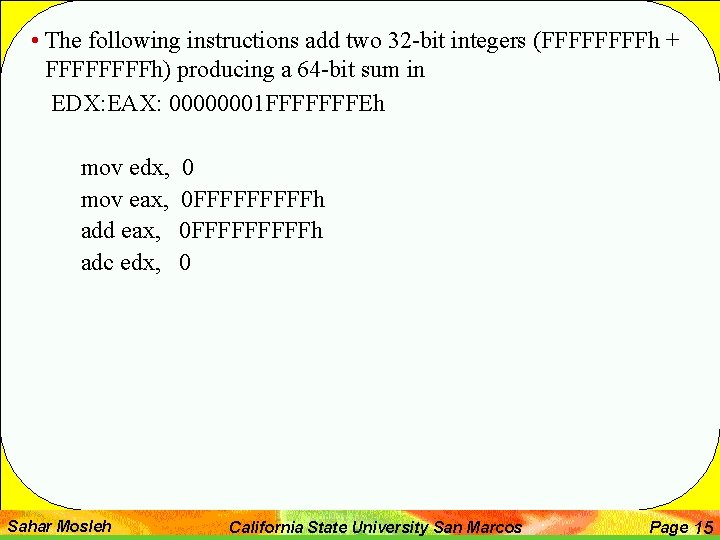
• The following instructions add two 32 -bit integers (FFFFh + FFFFh) producing a 64 -bit sum in EDX: EAX: 00000001 FFFFFFFEh mov edx, mov eax, add eax, adc edx, Sahar Mosleh 0 0 FFFFFFFFFh 0 California State University San Marcos Page 15
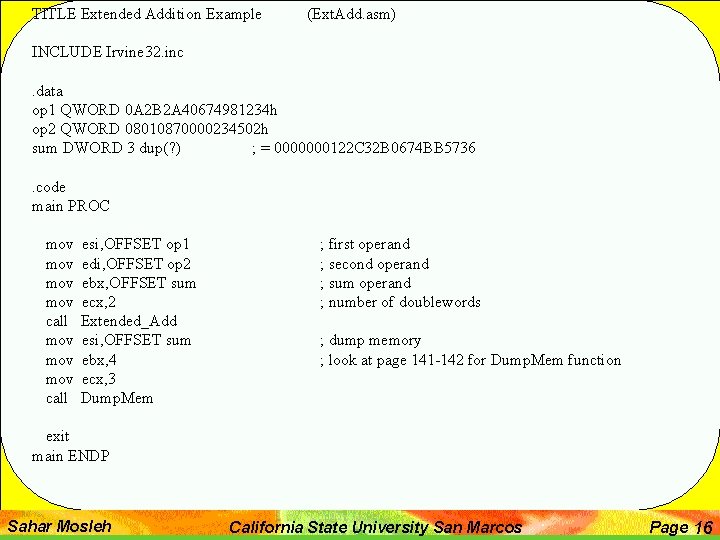
TITLE Extended Addition Example (Ext. Add. asm) INCLUDE Irvine 32. inc. data op 1 QWORD 0 A 2 B 2 A 40674981234 h op 2 QWORD 08010870000234502 h sum DWORD 3 dup(? ) ; = 0000000122 C 32 B 0674 BB 5736. code main PROC mov mov call esi, OFFSET op 1 edi, OFFSET op 2 ebx, OFFSET sum ecx, 2 Extended_Add esi, OFFSET sum ebx, 4 ecx, 3 Dump. Mem ; first operand ; second operand ; sum operand ; number of doublewords ; dump memory ; look at page 141 -142 for Dump. Mem function exit main ENDP Sahar Mosleh California State University San Marcos Page 16
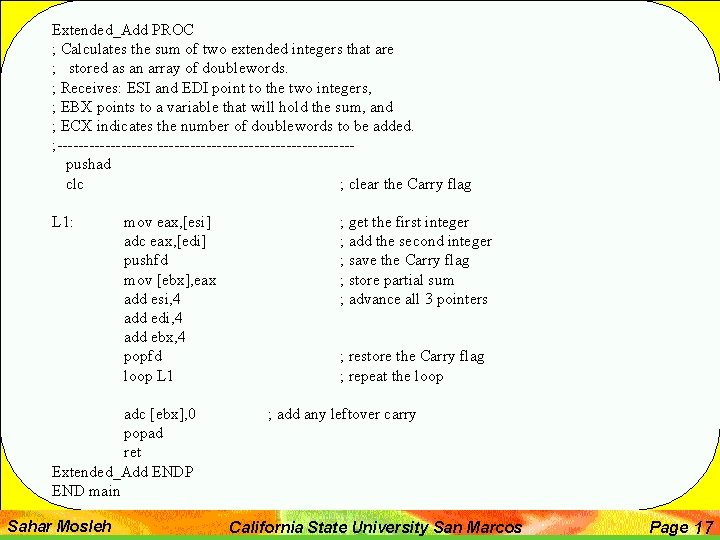
Extended_Add PROC ; Calculates the sum of two extended integers that are ; stored as an array of doublewords. ; Receives: ESI and EDI point to the two integers, ; EBX points to a variable that will hold the sum, and ; ECX indicates the number of doublewords to be added. ; ----------------------------pushad clc ; clear the Carry flag L 1: mov eax, [esi] adc eax, [edi] pushfd mov [ebx], eax add esi, 4 add edi, 4 add ebx, 4 popfd loop L 1 adc [ebx], 0 popad ret Extended_Add ENDP END main Sahar Mosleh ; get the first integer ; add the second integer ; save the Carry flag ; store partial sum ; advance all 3 pointers ; restore the Carry flag ; repeat the loop ; add any leftover carry California State University San Marcos Page 17
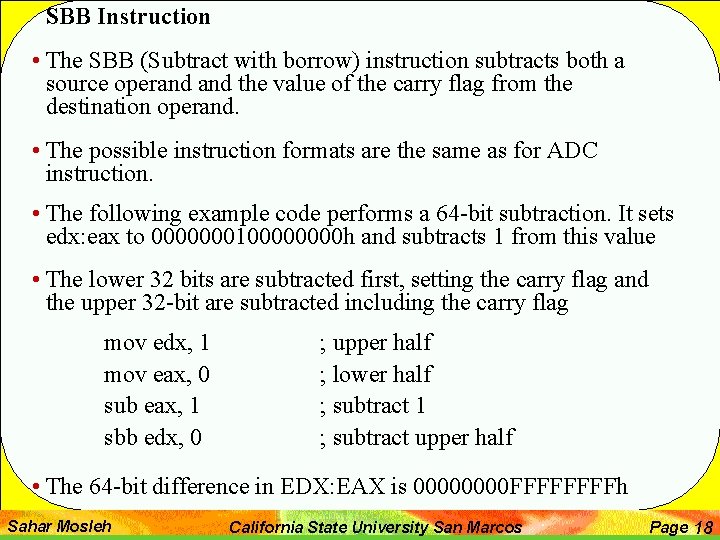
SBB Instruction • The SBB (Subtract with borrow) instruction subtracts both a source operand the value of the carry flag from the destination operand. • The possible instruction formats are the same as for ADC instruction. • The following example code performs a 64 -bit subtraction. It sets edx: eax to 000000010000 h and subtracts 1 from this value • The lower 32 bits are subtracted first, setting the carry flag and the upper 32 -bit are subtracted including the carry flag mov edx, 1 mov eax, 0 sub eax, 1 sbb edx, 0 ; upper half ; lower half ; subtract 1 ; subtract upper half • The 64 -bit difference in EDX: EAX is 0000 FFFFh Sahar Mosleh California State University San Marcos Page 18
In which instruction msb gets shifted to chf as well as lsb
Sahar mosleh
Sahar mosleh
Light linking blender
Sahar mosleh
Sahar mosleh
Sahar mosleh
Lambert beer law and its limitations
Aniline uv spectrum
Cap 221
Bathochromic shift and hypsochromic shift
Difference between arithmetic shift and logical shift
Logical shift and arithmetic shift
Gabriel hugh elkaim
Difference between arithmetic shift and logical shift
Condylar angle
Rotate and swap instruction in 8051
Fixed pulley
Paint.net rotate image