9 3 include iostream h void main char
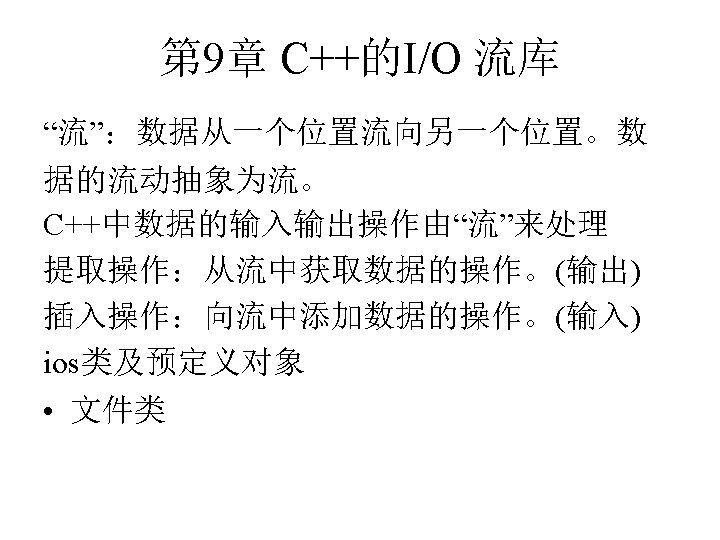
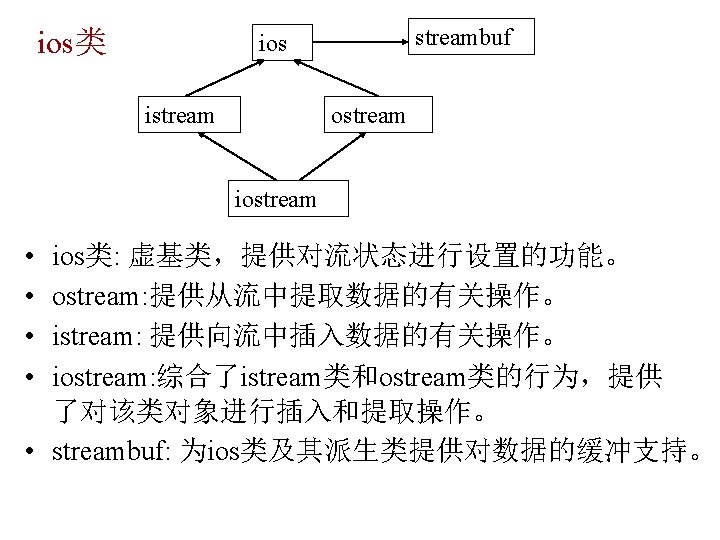
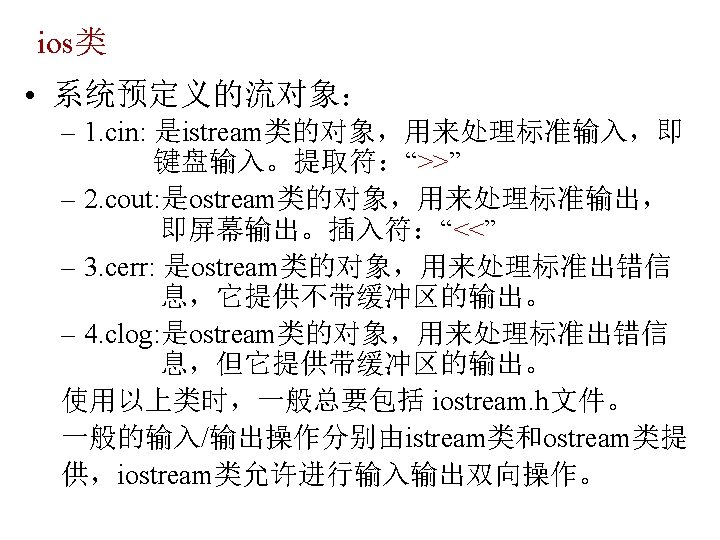
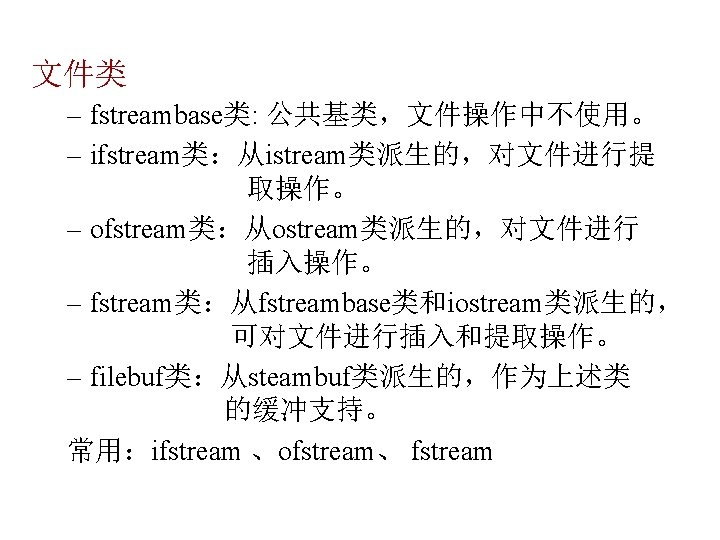
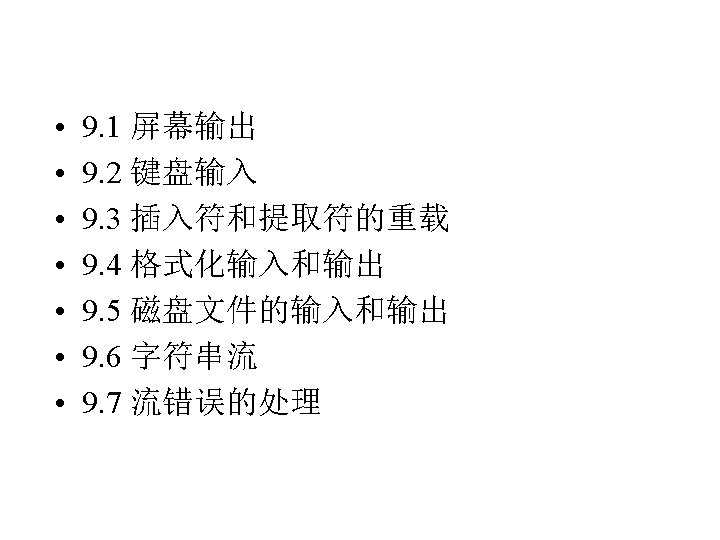
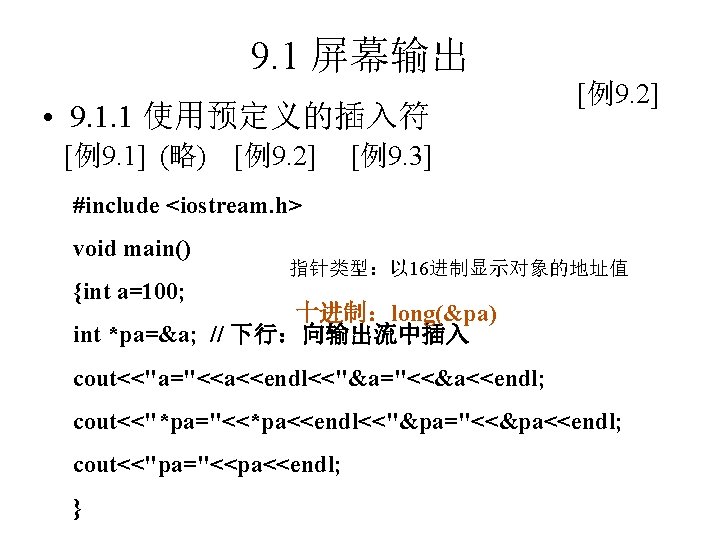
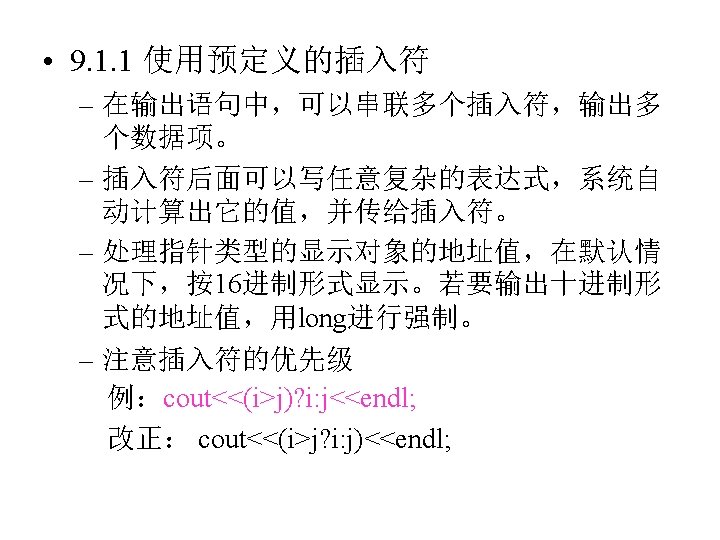
![[例9. 3] #include <iostream. h> void main() { char *str="string"; cout<<“the string is: ”<<str<<endl; [例9. 3] #include <iostream. h> void main() { char *str="string"; cout<<“the string is: ”<<str<<endl;](https://slidetodoc.com/presentation_image_h2/7c66b15f1f13deff940824e0bc0eb3a8/image-8.jpg)
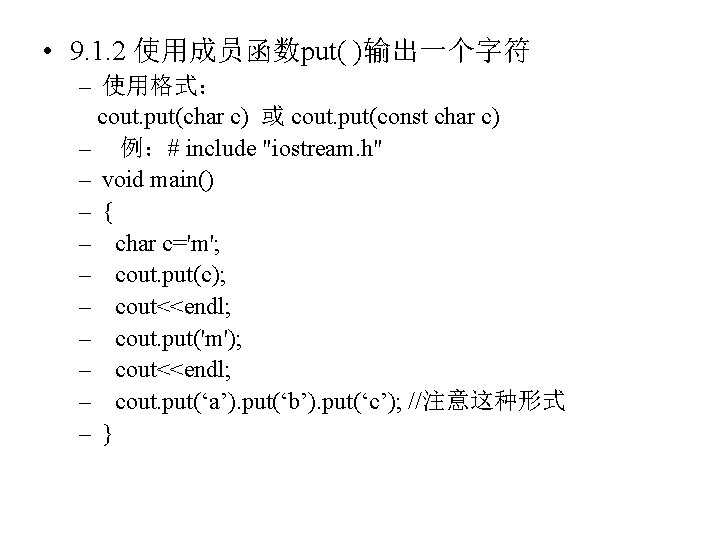
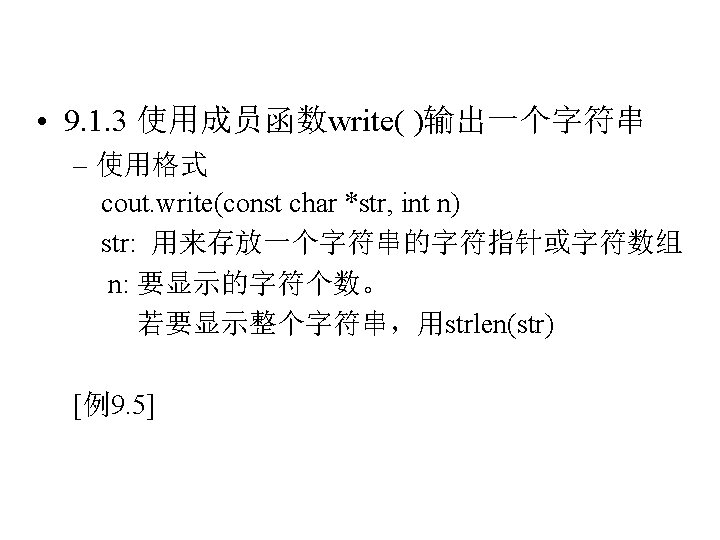
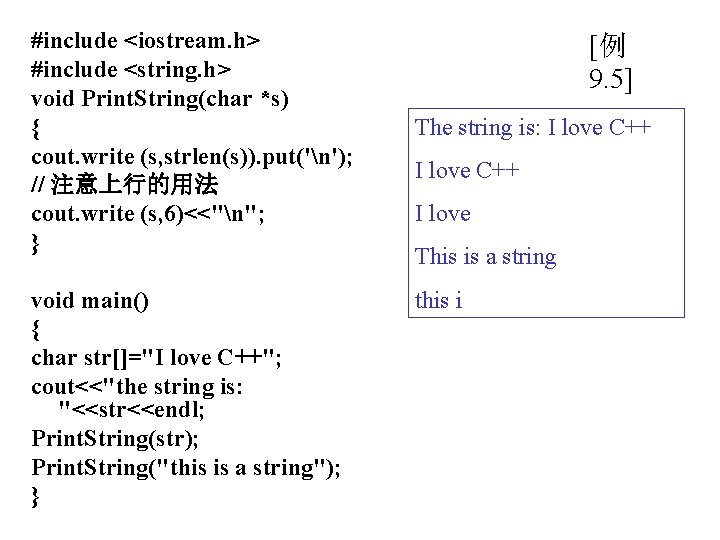
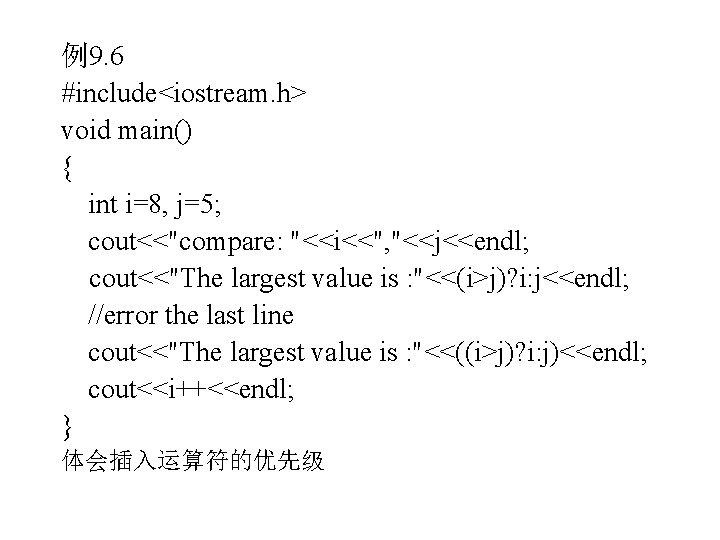
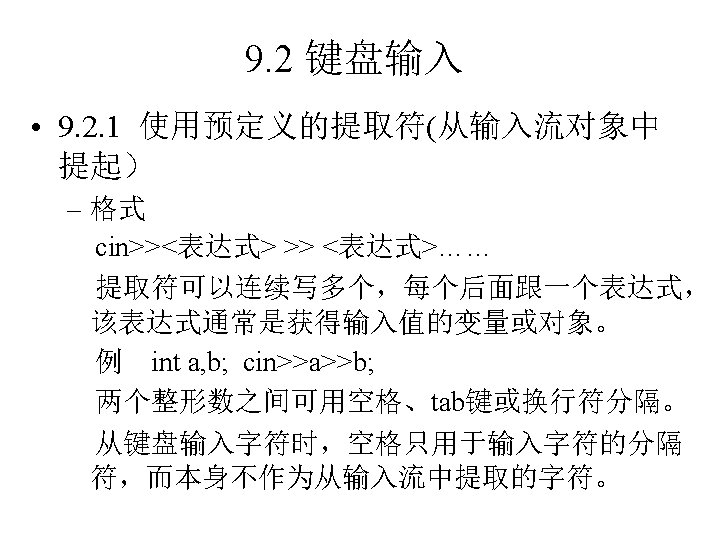
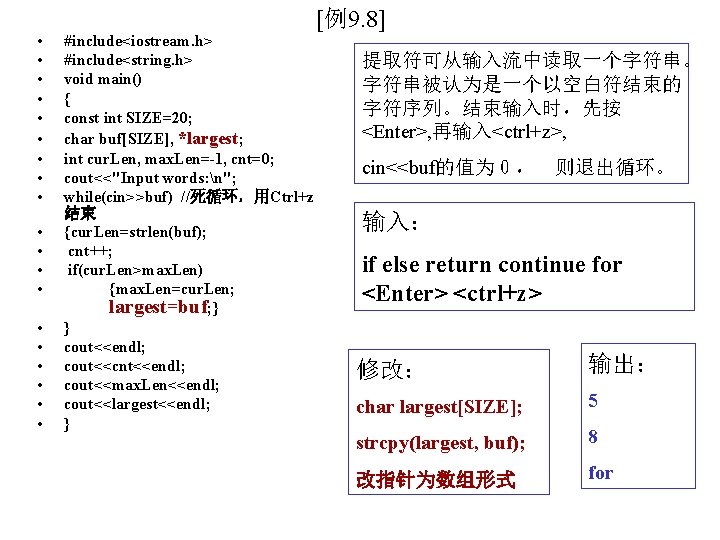
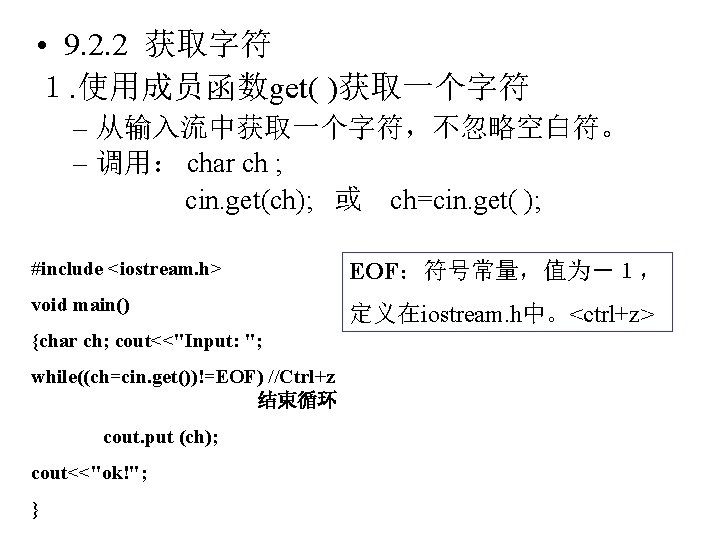
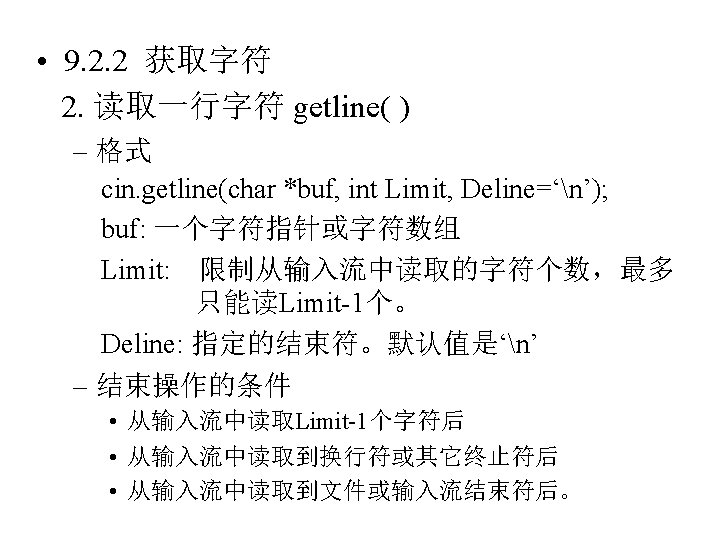
![#include <iostream. h> const int SIZE=80; void main() {int lcnt=0, lmax=-1; char buf[SIZE]; cout<<"Input. #include <iostream. h> const int SIZE=80; void main() {int lcnt=0, lmax=-1; char buf[SIZE]; cout<<"Input.](https://slidetodoc.com/presentation_image_h2/7c66b15f1f13deff940824e0bc0eb3a8/image-17.jpg)
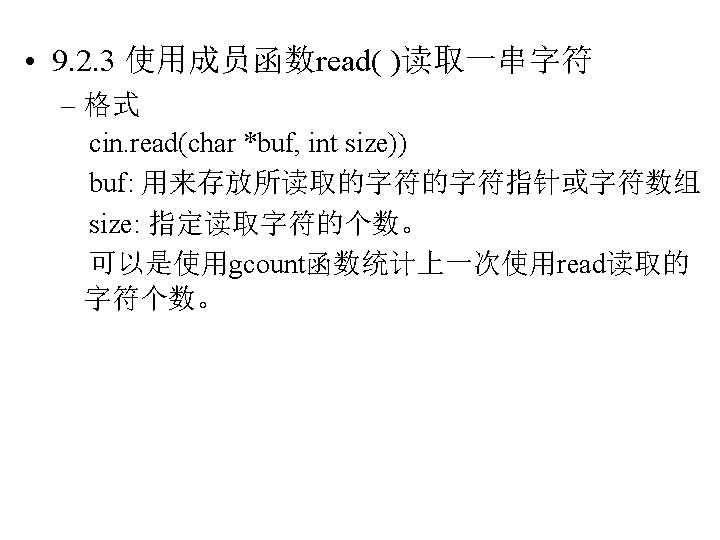
![[例9. 11] Input… #include <iostream. h> void main() { const int S=80; char buf[S]=""; [例9. 11] Input… #include <iostream. h> void main() { const int S=80; char buf[S]="";](https://slidetodoc.com/presentation_image_h2/7c66b15f1f13deff940824e0bc0eb3a8/image-19.jpg)
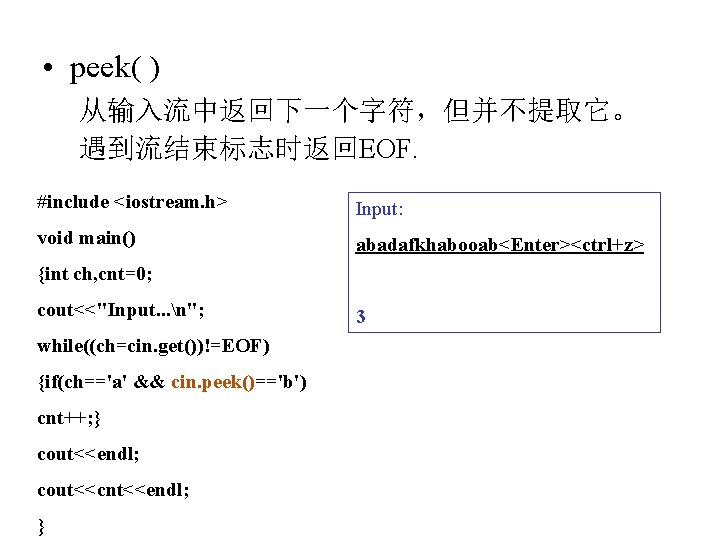
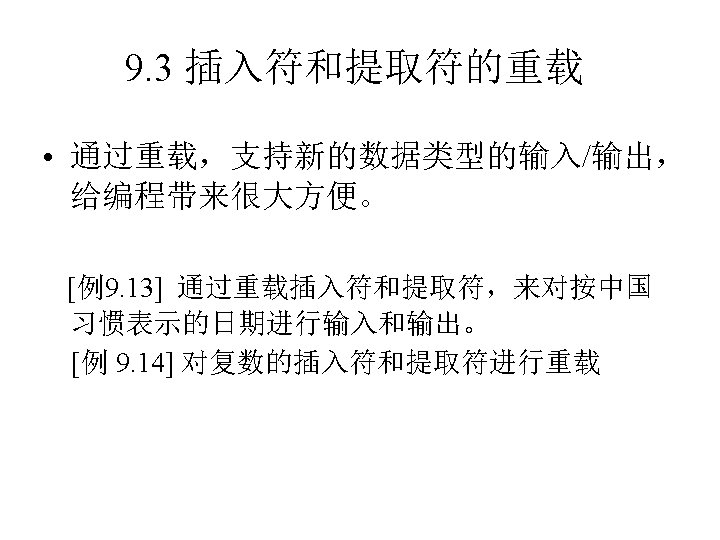
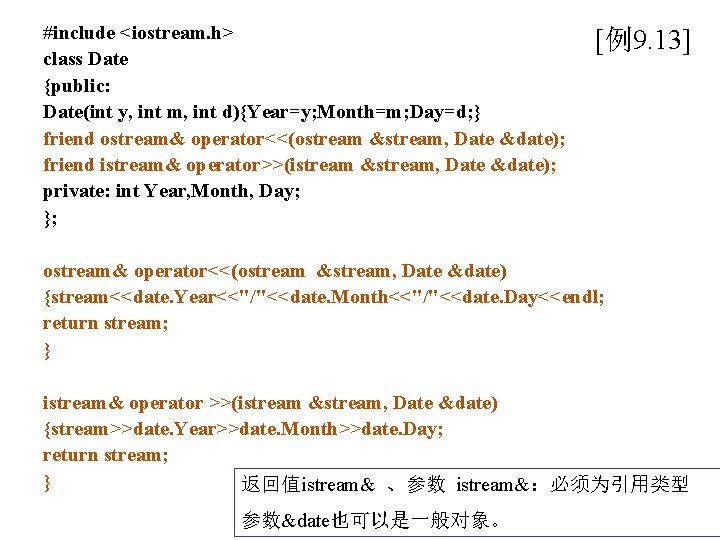
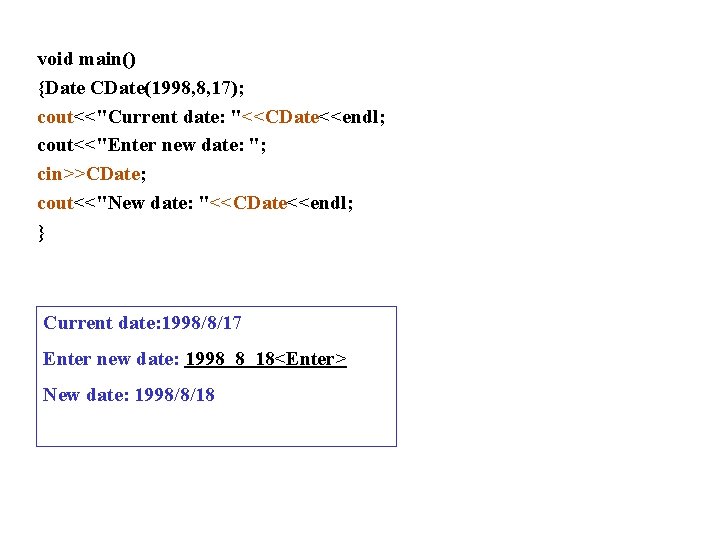
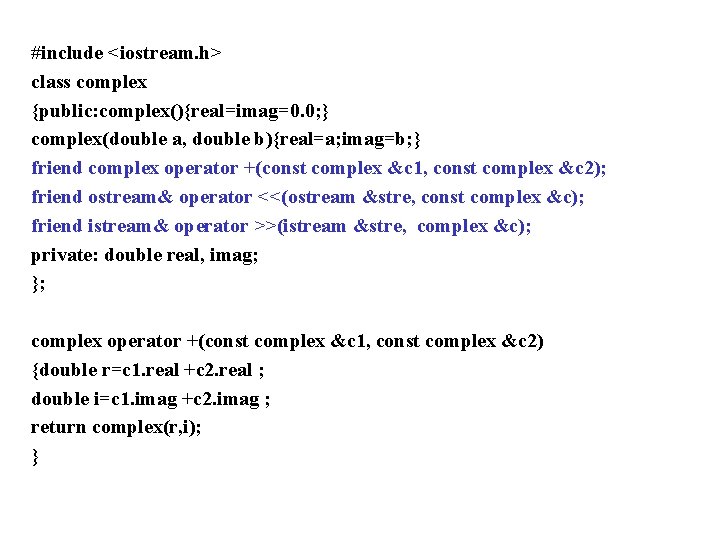
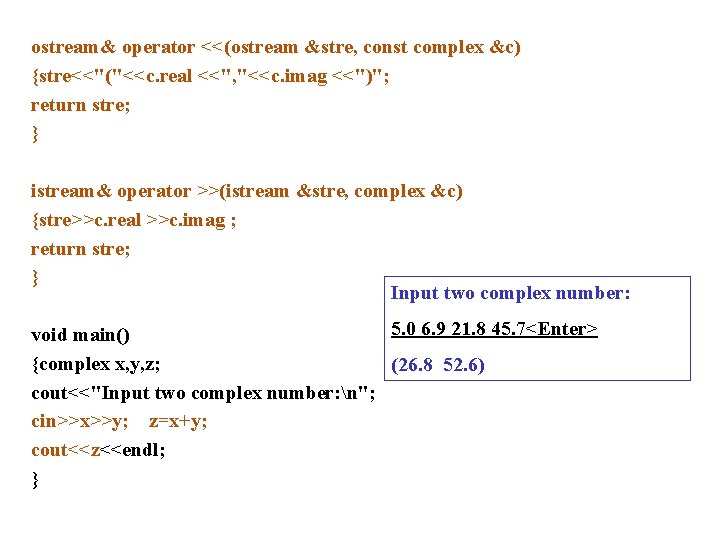
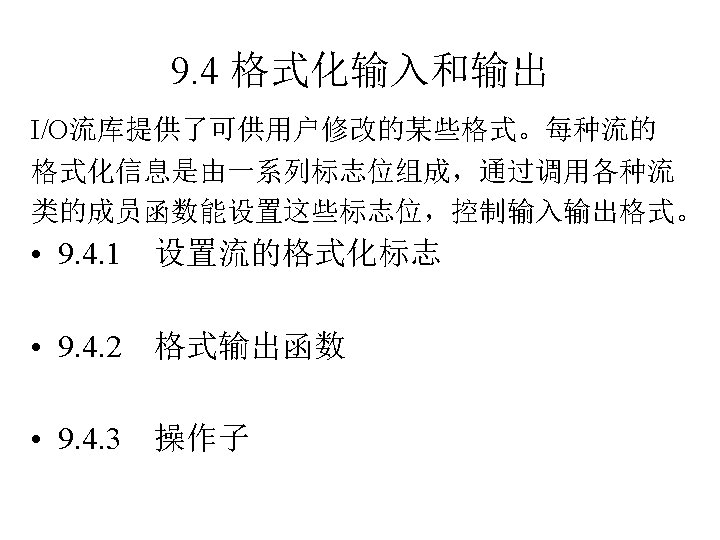
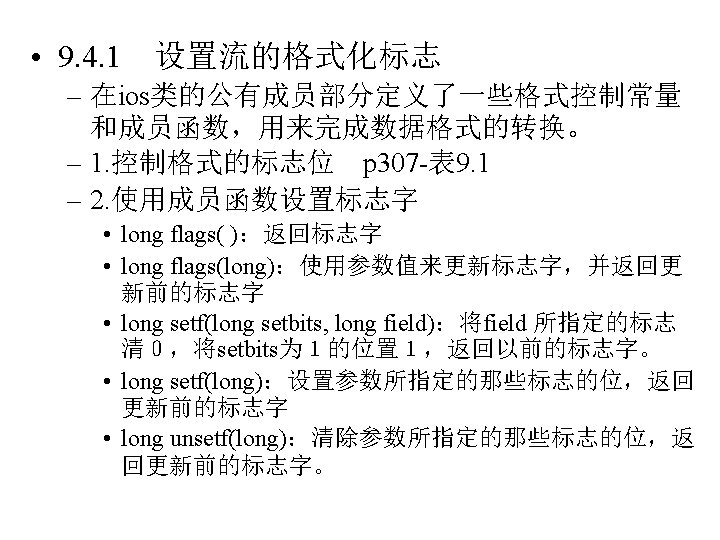
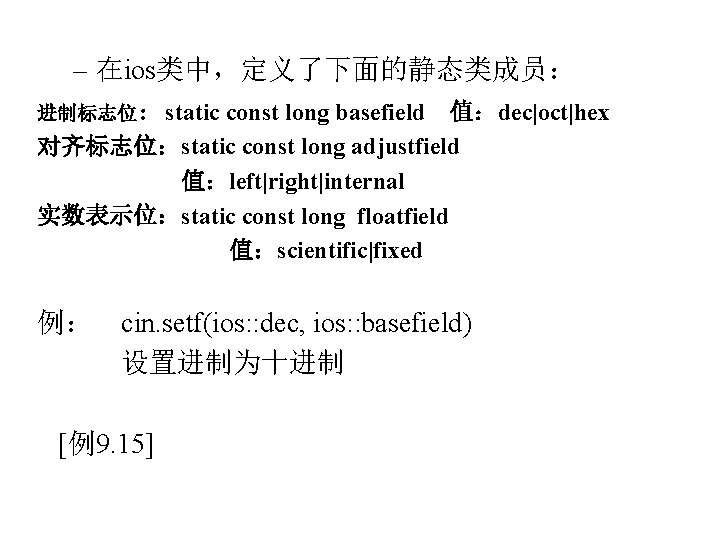
![[例9. 15] #include <iostream. h> void main() { cout. setf (ios: : oct, ios: [例9. 15] #include <iostream. h> void main() { cout. setf (ios: : oct, ios:](https://slidetodoc.com/presentation_image_h2/7c66b15f1f13deff940824e0bc0eb3a8/image-29.jpg)
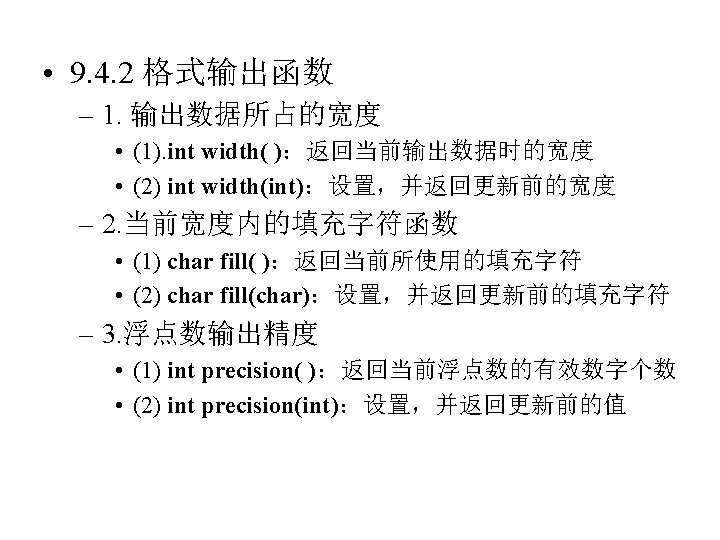
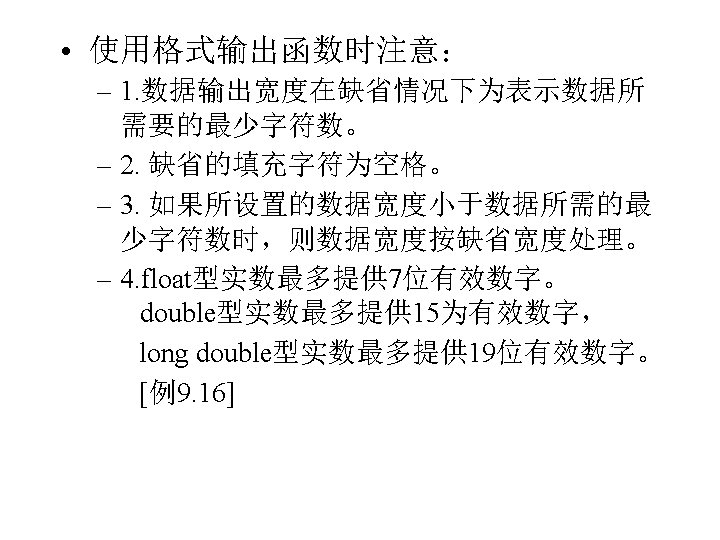
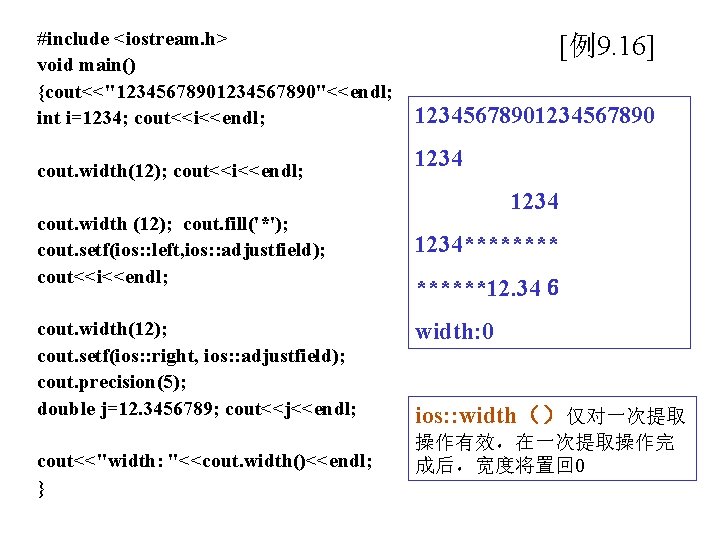
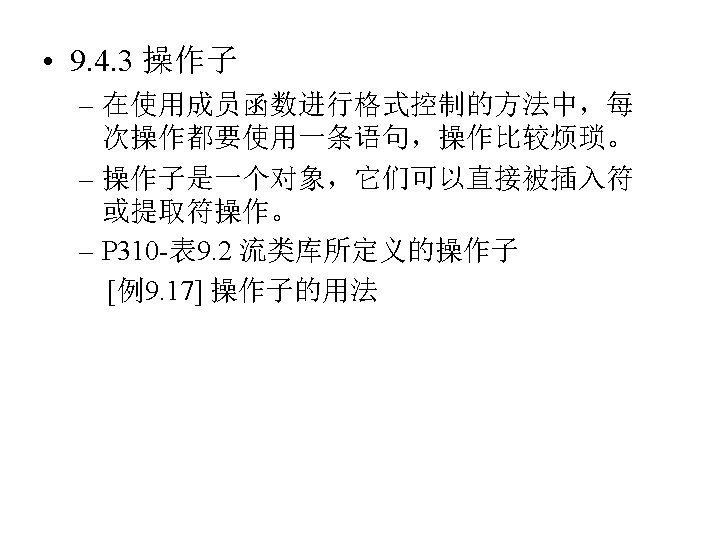
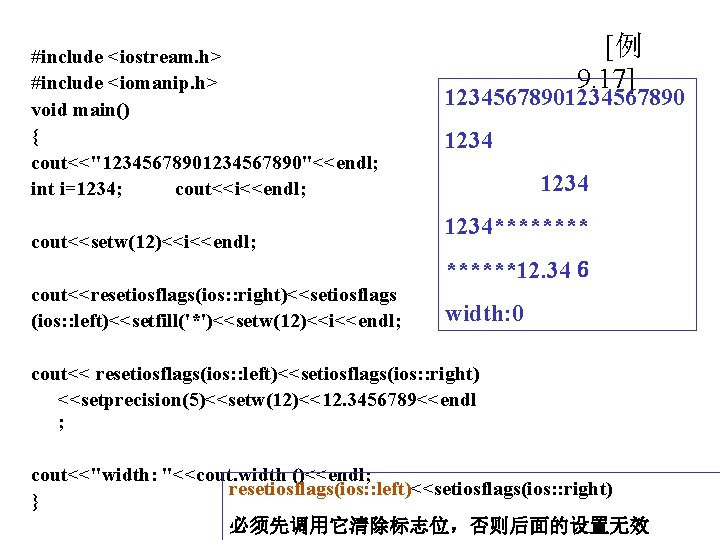
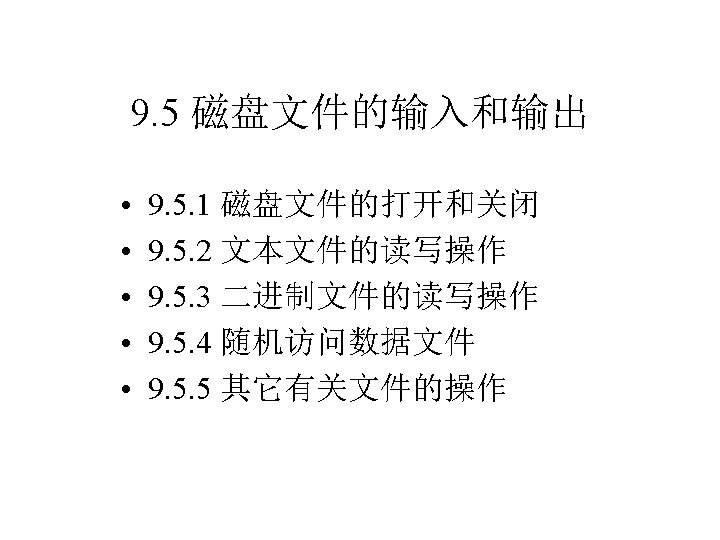
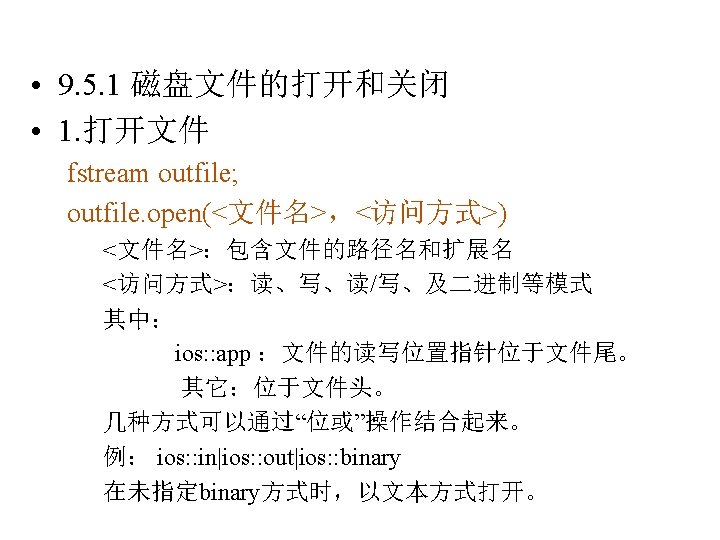
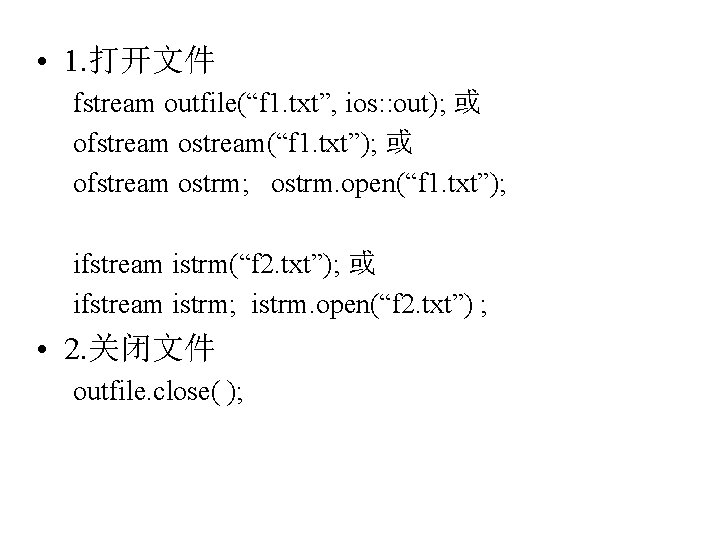
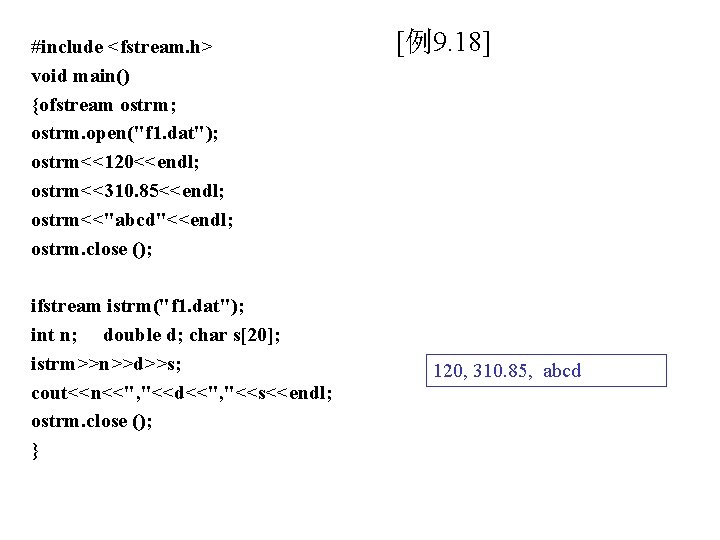
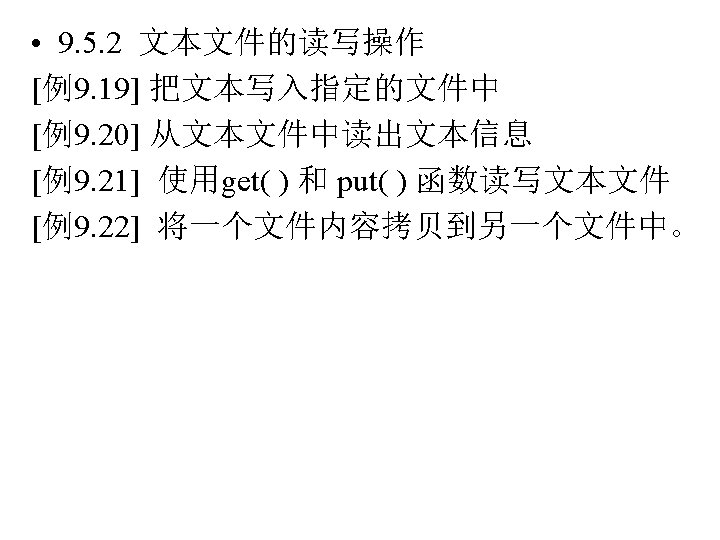
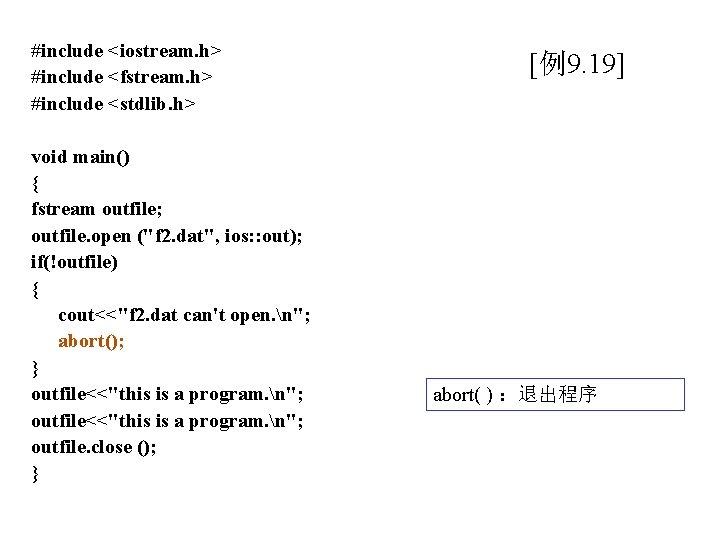
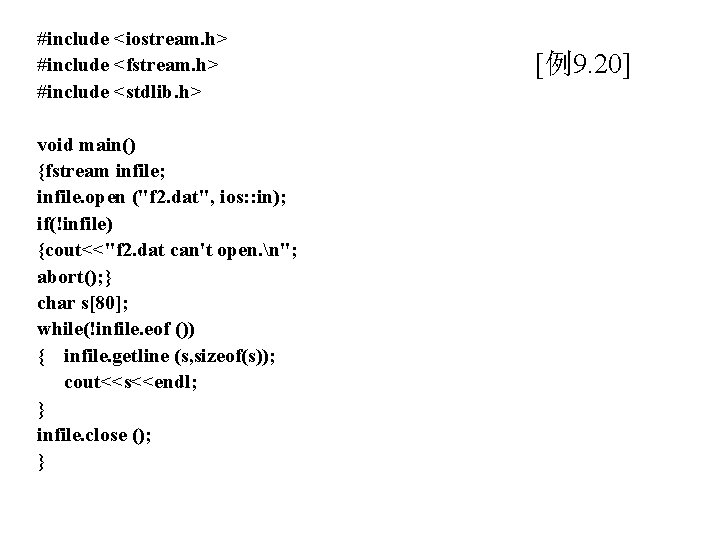
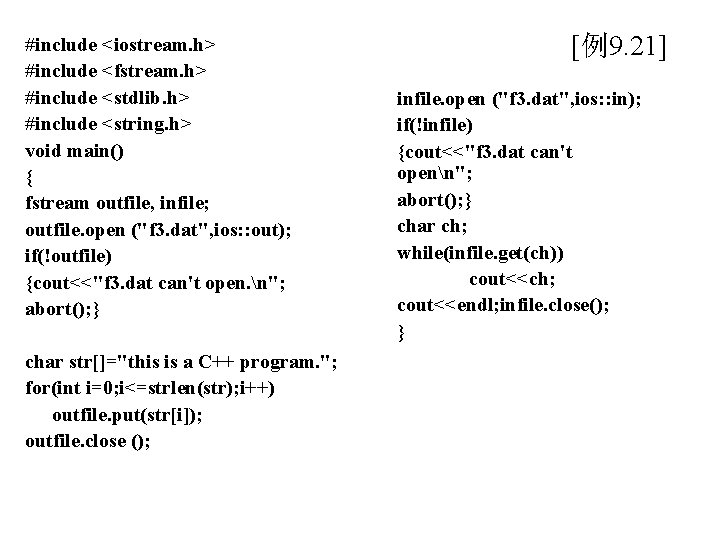
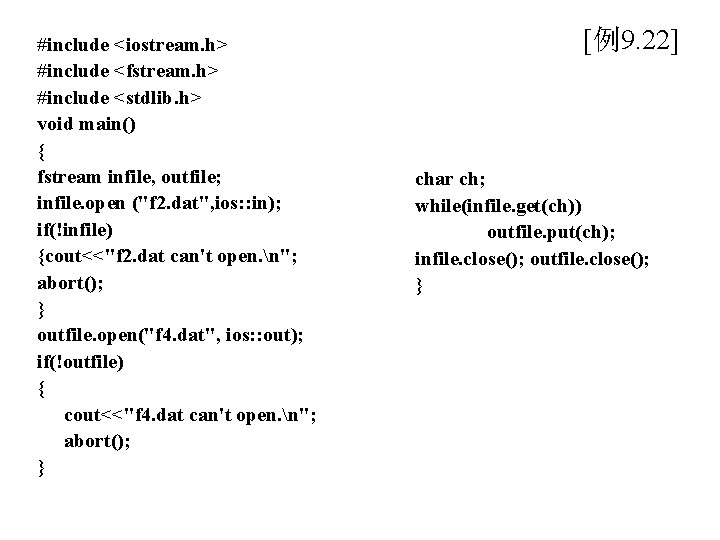
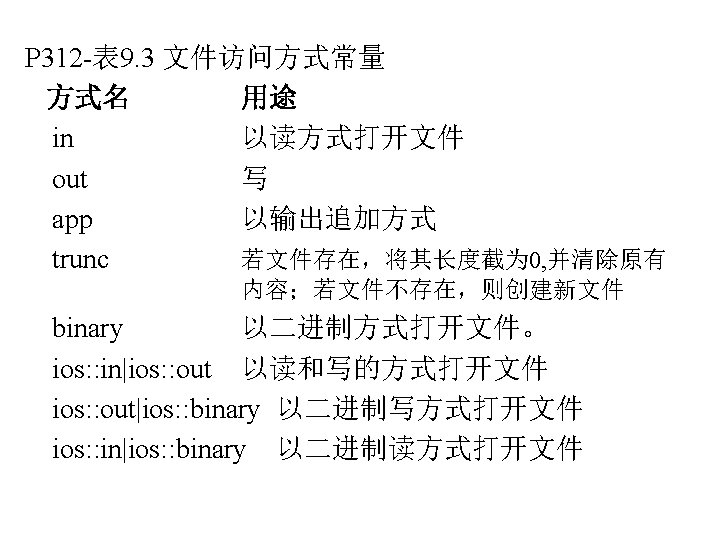
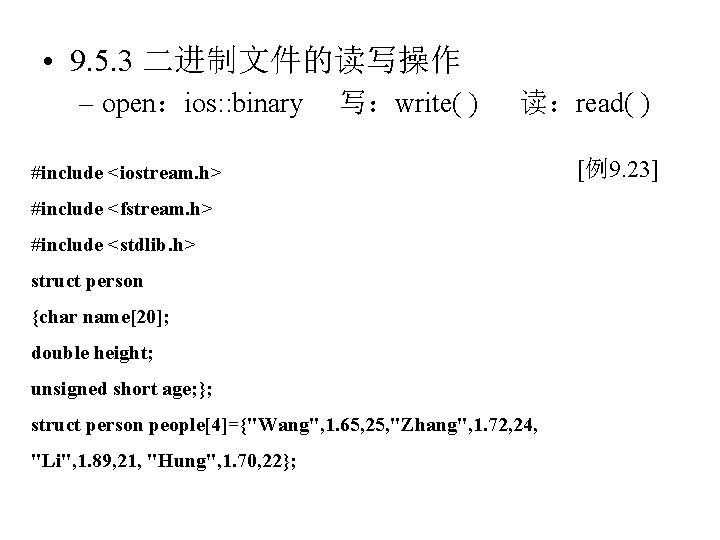
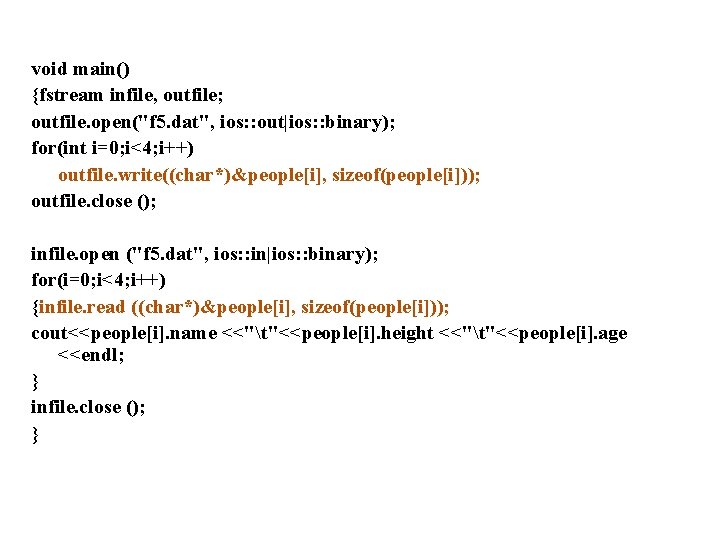
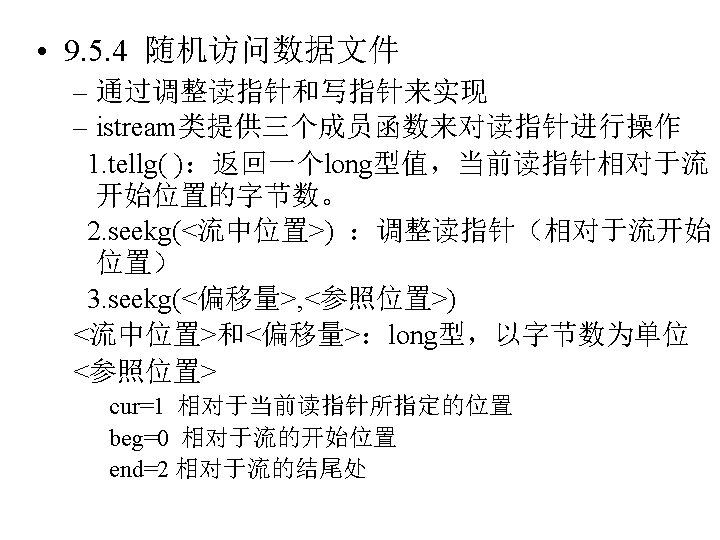
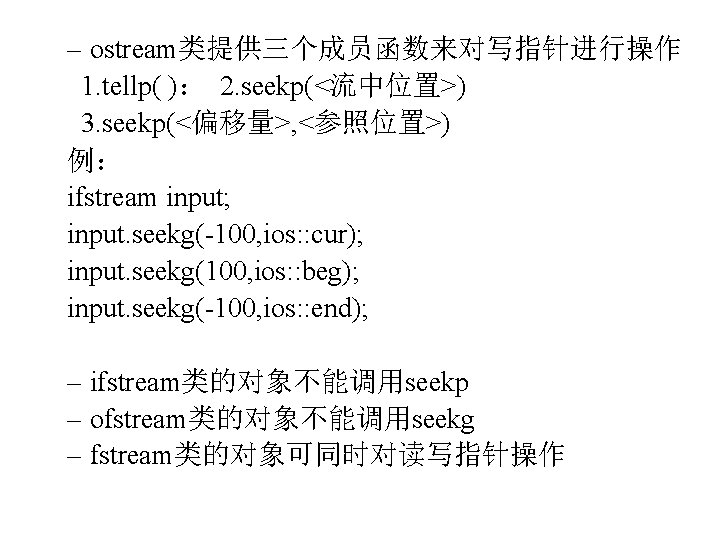
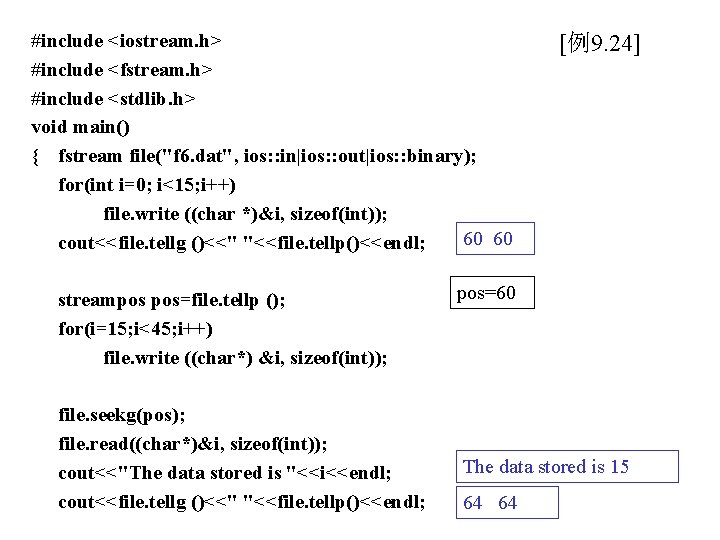
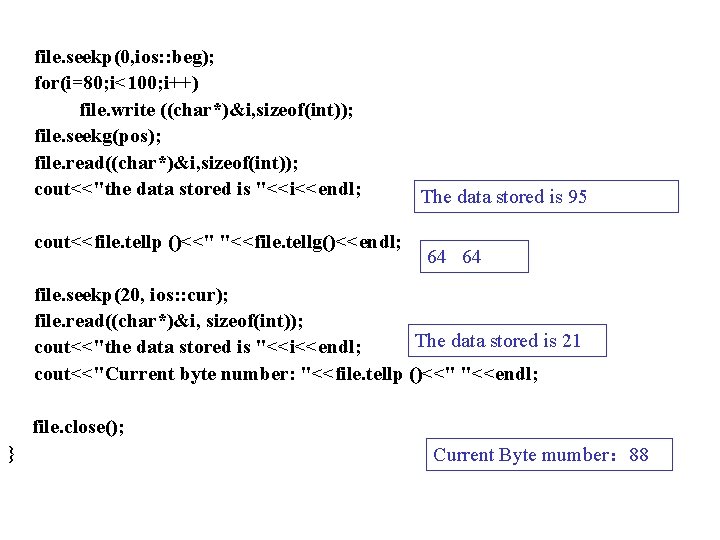
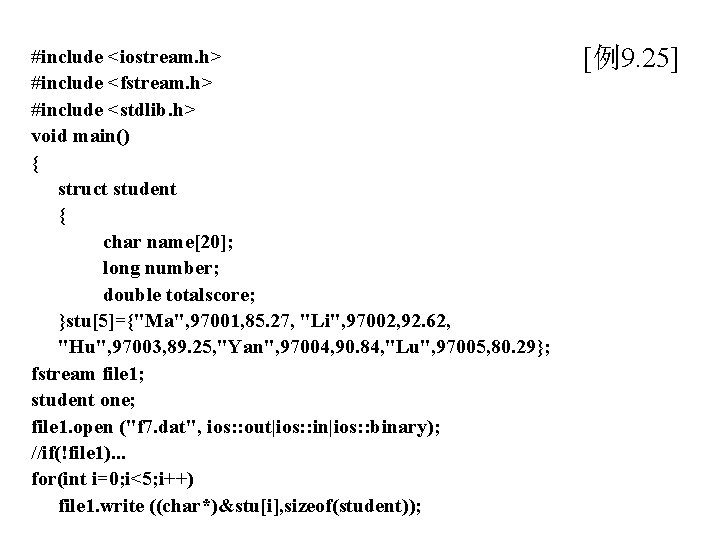
![file 1. seekp (sizeof(student)*4); file 1. read((char*)&one, sizeof(stu[i])); cout<<one. name<<"t"<<one. number<<"t"<<one. totalscore<<endl; Lu 97005 file 1. seekp (sizeof(student)*4); file 1. read((char*)&one, sizeof(stu[i])); cout<<one. name<<"t"<<one. number<<"t"<<one. totalscore<<endl; Lu 97005](https://slidetodoc.com/presentation_image_h2/7c66b15f1f13deff940824e0bc0eb3a8/image-52.jpg)
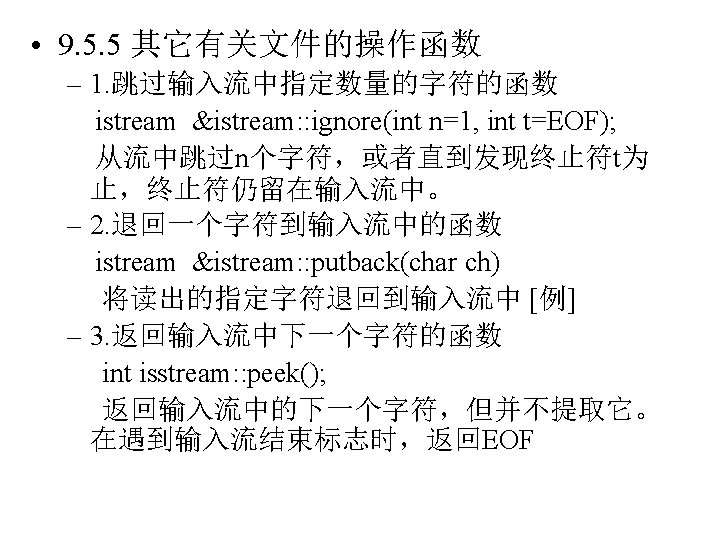
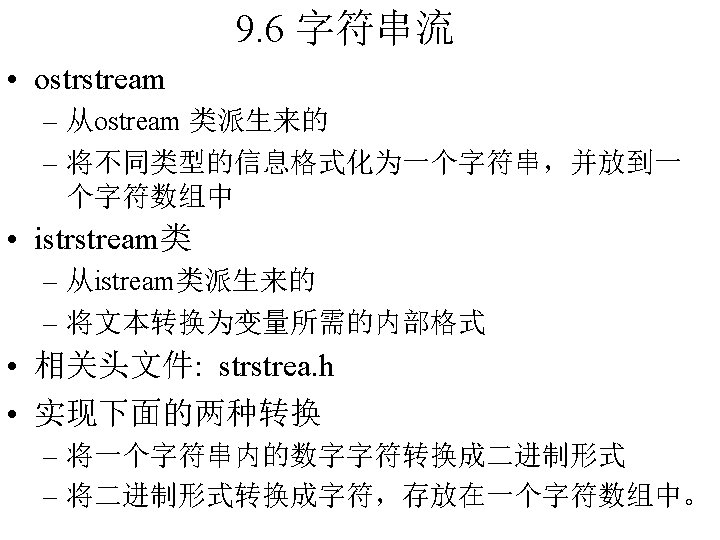
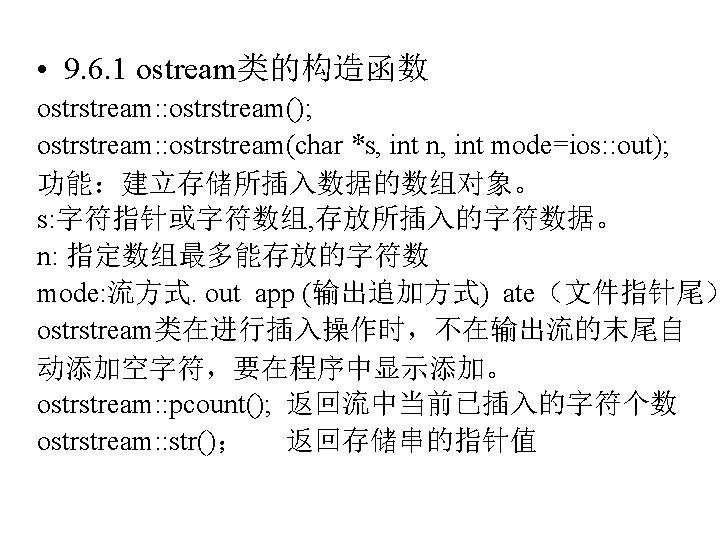
![#include <fstream. h> #include <strstrea. h> const int N=80; void main() { char buf[N]; #include <fstream. h> #include <strstrea. h> const int N=80; void main() { char buf[N];](https://slidetodoc.com/presentation_image_h2/7c66b15f1f13deff940824e0bc0eb3a8/image-56.jpg)
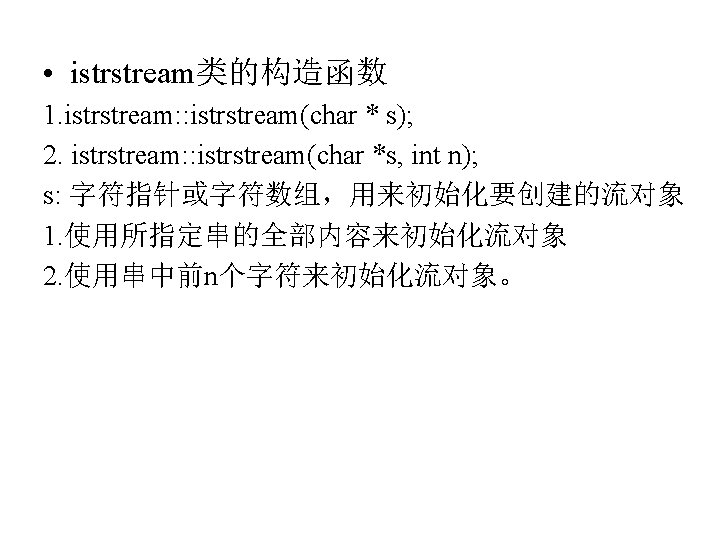
![[例9. 29] #include <iostream. h> #include <strstrea. h> void main() {char buf[]="123 45. 67"; [例9. 29] #include <iostream. h> #include <strstrea. h> void main() {char buf[]="123 45. 67";](https://slidetodoc.com/presentation_image_h2/7c66b15f1f13deff940824e0bc0eb3a8/image-58.jpg)
![#include <iostream. h> #include <strstrea. h> void main() { char buf[]="12345"; int i, j; #include <iostream. h> #include <strstrea. h> void main() { char buf[]="12345"; int i, j;](https://slidetodoc.com/presentation_image_h2/7c66b15f1f13deff940824e0bc0eb3a8/image-59.jpg)
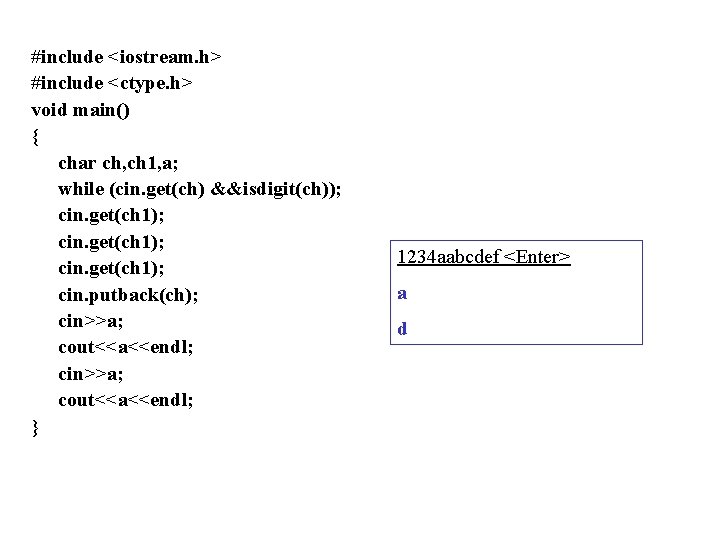
- Slides: 60
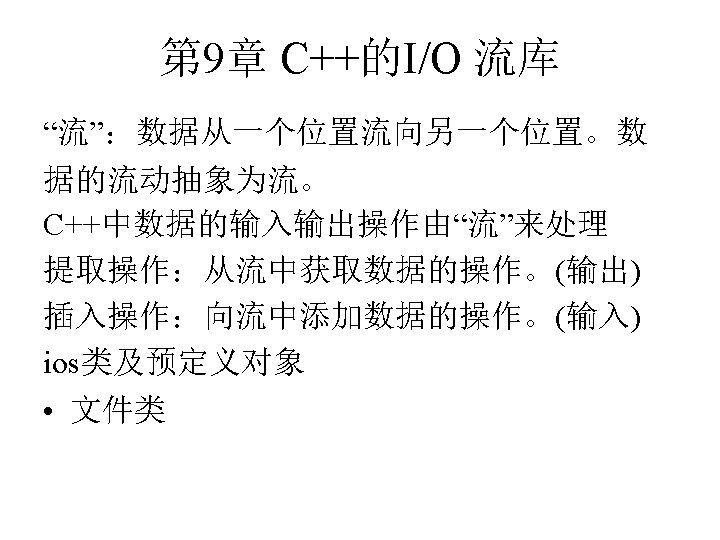
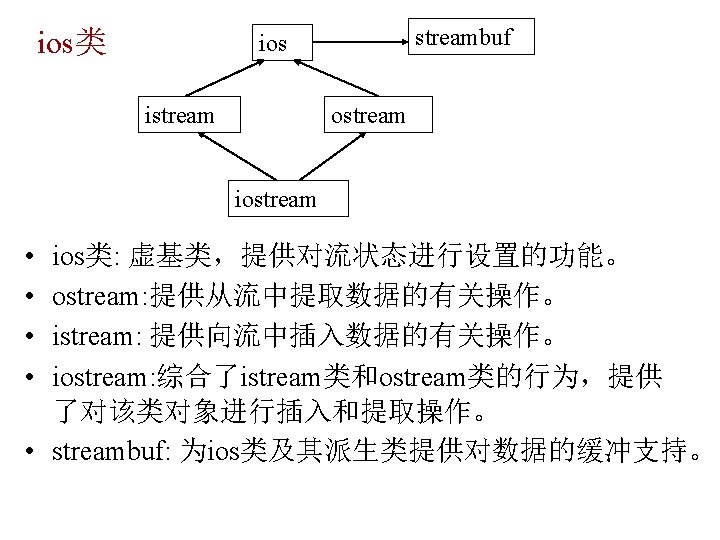
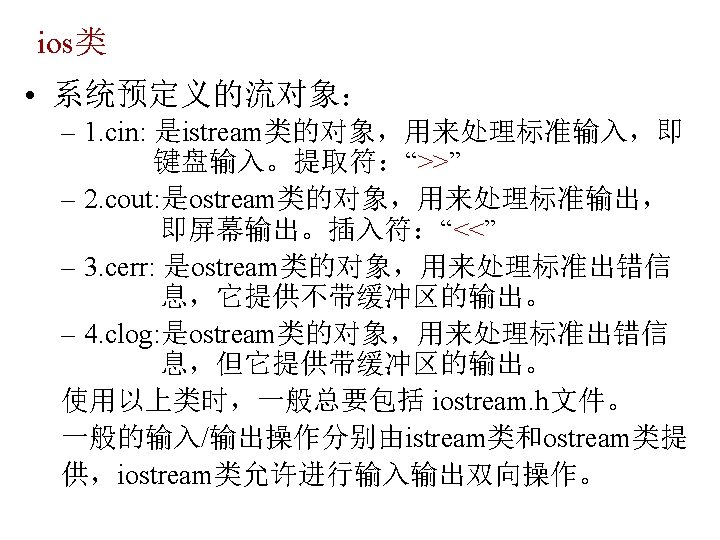
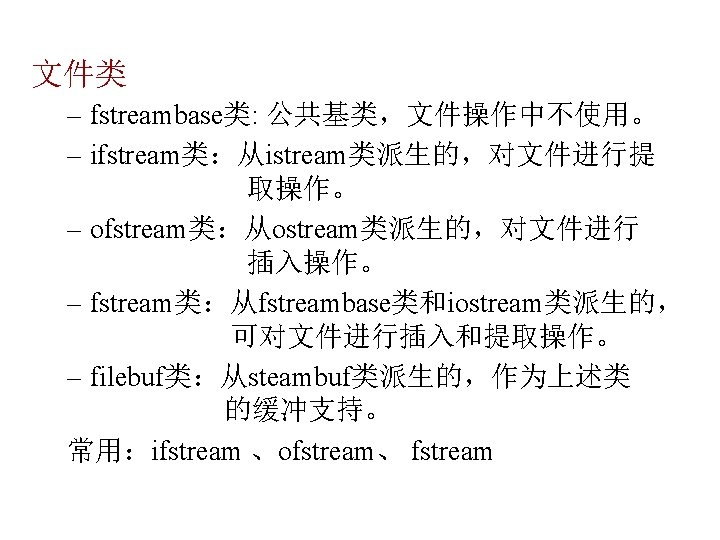
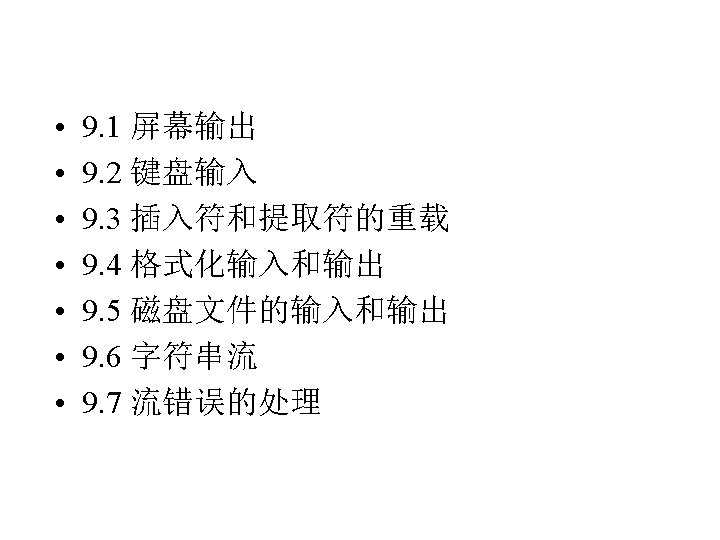
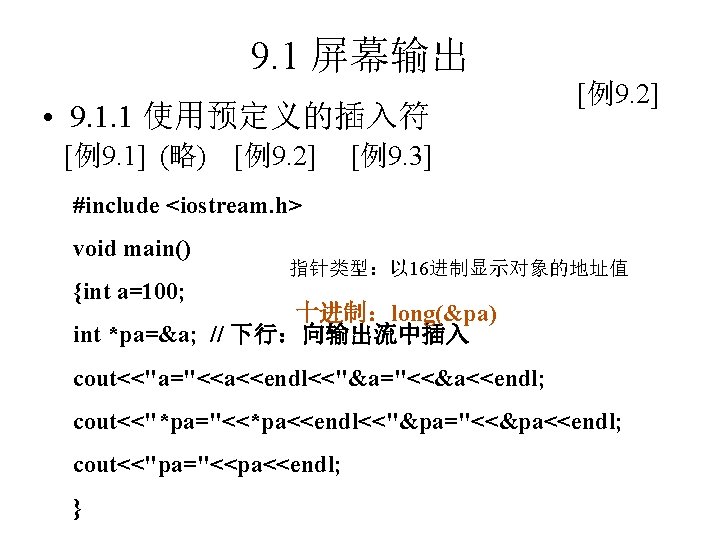
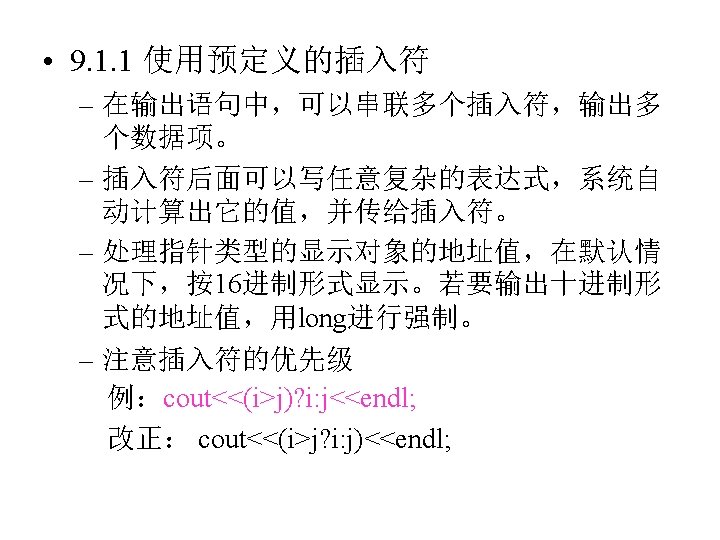
![例9 3 include iostream h void main char strstring coutthe string is strendl [例9. 3] #include <iostream. h> void main() { char *str="string"; cout<<“the string is: ”<<str<<endl;](https://slidetodoc.com/presentation_image_h2/7c66b15f1f13deff940824e0bc0eb3a8/image-8.jpg)
[例9. 3] #include <iostream. h> void main() { char *str="string"; cout<<“the string is: ”<<str<<endl; //输出字符指针所指向的字符串 cout<<“the addrrss is: ”<<(void*)str<<endl; //输出字符串的地址 } The string is: string The address is 0 x….
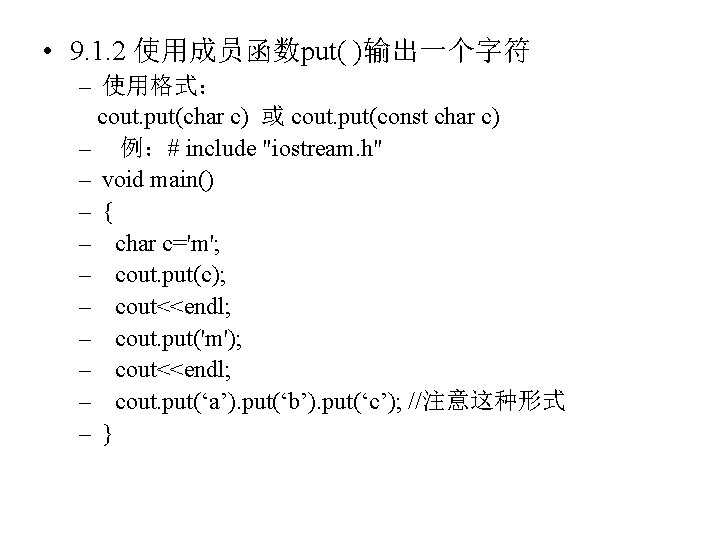
• 9. 1. 2 使用成员函数put( )输出一个字符 – 使用格式: cout. put(char c) 或 cout. put(const char c) – 例:# include "iostream. h" – void main() – { – char c='m'; – cout. put(c); – cout<<endl; – cout. put('m'); – cout<<endl; – cout. put(‘a’). put(‘b’). put(‘c’); //注意这种形式 – }
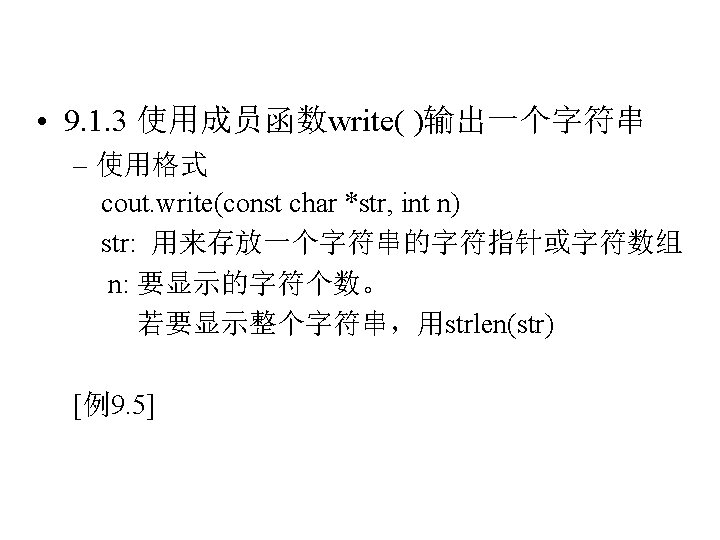
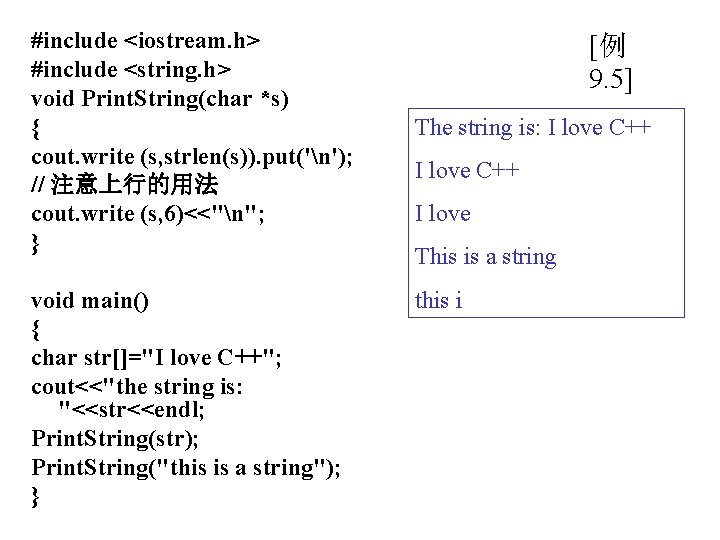
#include <iostream. h> #include <string. h> void Print. String(char *s) { cout. write (s, strlen(s)). put('n'); // 注意上行的用法 cout. write (s, 6)<<"n"; } void main() { char str[]="I love C++"; cout<<"the string is: "<<str<<endl; Print. String(str); Print. String("this is a string"); } [例 9. 5] The string is: I love C++ I love This is a string this i
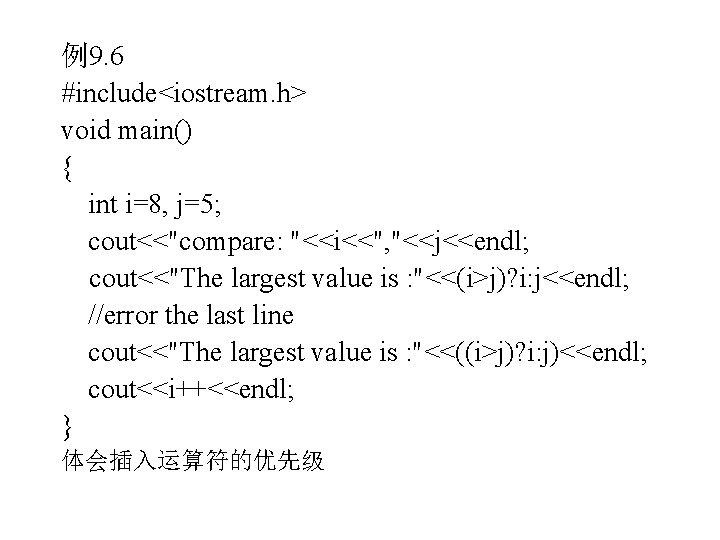
例9. 6 #include<iostream. h> void main() { int i=8, j=5; cout<<"compare: "<<i<<", "<<j<<endl; cout<<"The largest value is : "<<(i>j)? i: j<<endl; //error the last line cout<<"The largest value is : "<<((i>j)? i: j)<<endl; cout<<i++<<endl; } 体会插入运算符的优先级
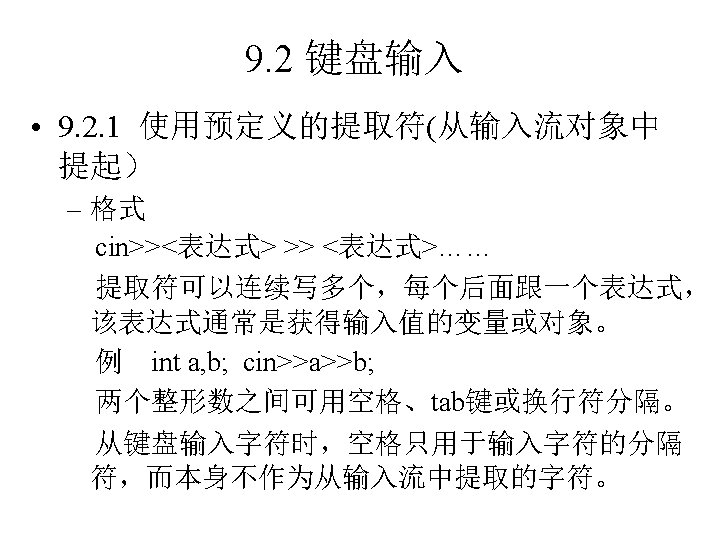
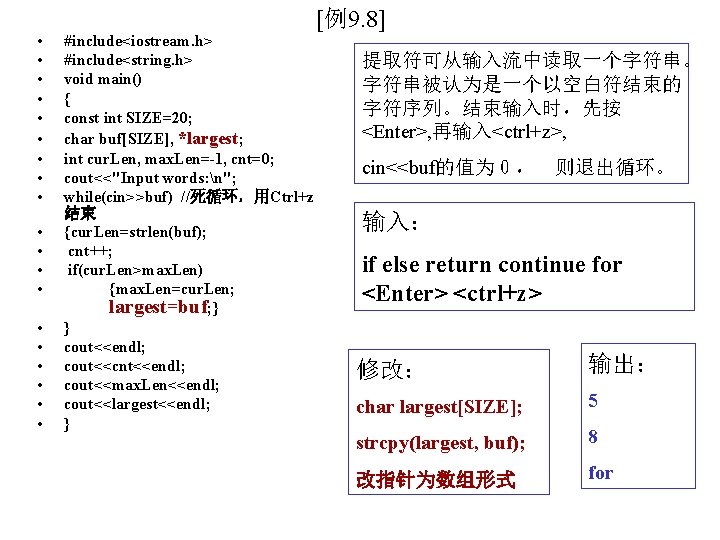
• • • • • #include<iostream. h> #include<string. h> void main() { const int SIZE=20; char buf[SIZE], *largest; int cur. Len, max. Len=-1, cnt=0; cout<<"Input words: n"; while(cin>>buf) //死循环,用Ctrl+z 结束 {cur. Len=strlen(buf); cnt++; if(cur. Len>max. Len) {max. Len=cur. Len; largest=buf; } } cout<<endl; cout<<cnt<<endl; cout<<max. Len<<endl; cout<<largest<<endl; } [例9. 8] 提取符可从输入流中读取一个字符串。 字符串被认为是一个以空白符结束的 字符序列。结束输入时,先按 <Enter>, 再输入<ctrl+z>, cin<<buf的值为0, 则退出循环。 输入: if else return continue for <Enter> <ctrl+z> 修改: 输出: char largest[SIZE]; 5 strcpy(largest, buf); 8 改指针为数组形式 for
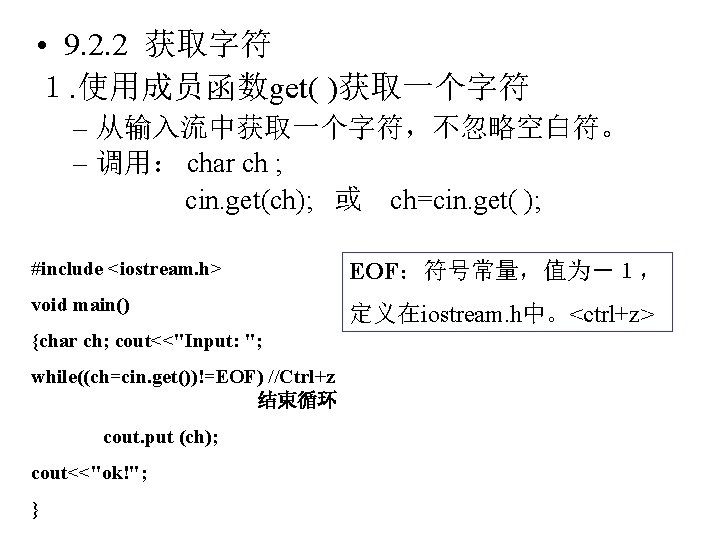
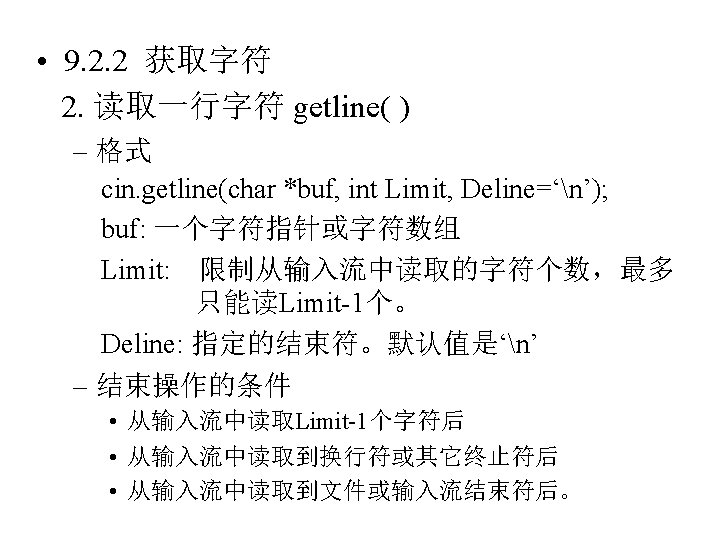
![include iostream h const int SIZE80 void main int lcnt0 lmax1 char bufSIZE coutInput #include <iostream. h> const int SIZE=80; void main() {int lcnt=0, lmax=-1; char buf[SIZE]; cout<<"Input.](https://slidetodoc.com/presentation_image_h2/7c66b15f1f13deff940824e0bc0eb3a8/image-17.jpg)
#include <iostream. h> const int SIZE=80; void main() {int lcnt=0, lmax=-1; char buf[SIZE]; cout<<"Input. . . n"; while(cin. getline(buf, SIZE)) {int count=cin. gcount(); lcnt++; if(count>lmax) lmax=count; cout<<"Line # "<<lcnt<<"t" <<"chars read: "<<count<<endl; cout. write(buf, count). put('n'); } cout<<endl; cout<<"Total line: "<<lcnt<<endl; cout<<"Longest line: "<<lmax<<endl; } [例9. 9] istream类的成员函数 gcount( ): 返回上一次getline( )函数 实际读入的字符个数, 包括空白符。 while(cin. getline(buf, SIZE) ) 每次从输入流中读取一行 字符放在buf中结束输入时 使用<ctrl+z>
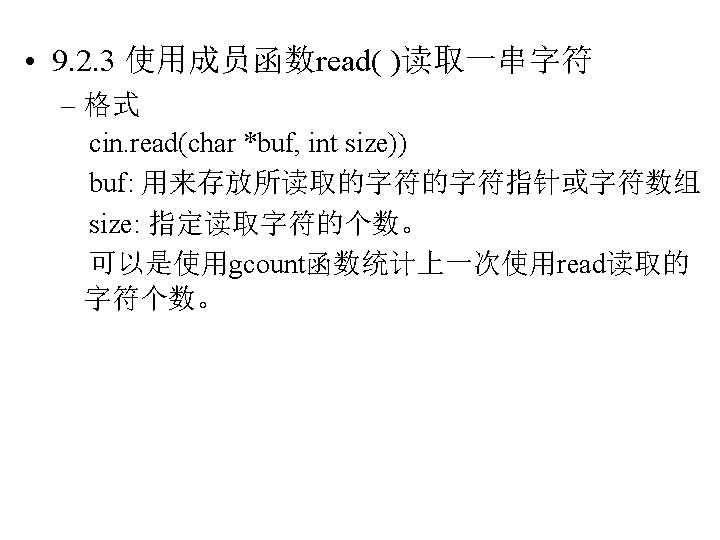
![例9 11 Input include iostream h void main const int S80 char bufS [例9. 11] Input… #include <iostream. h> void main() { const int S=80; char buf[S]="";](https://slidetodoc.com/presentation_image_h2/7c66b15f1f13deff940824e0bc0eb3a8/image-19.jpg)
[例9. 11] Input… #include <iostream. h> void main() { const int S=80; char buf[S]=""; cout<<"Input. . . n"; cin. read (buf, S); cout<<endl; cout<<buf<<endl; cout<<cin. gcount (); } abcd<Enter> efghijk<Enter> <ctrl+z> abcd efghijk 13
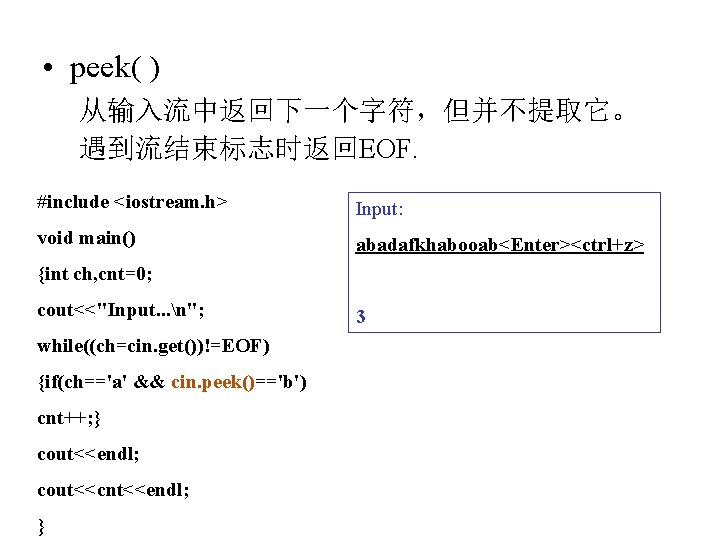
• peek( ) 从输入流中返回下一个字符,但并不提取它。 遇到流结束标志时返回EOF. #include <iostream. h> Input: void main() abadafkhabooab<Enter><ctrl+z> {int ch, cnt=0; cout<<"Input. . . n"; while((ch=cin. get())!=EOF) {if(ch=='a' && cin. peek()=='b') cnt++; } cout<<endl; cout<<cnt<<endl; } 3
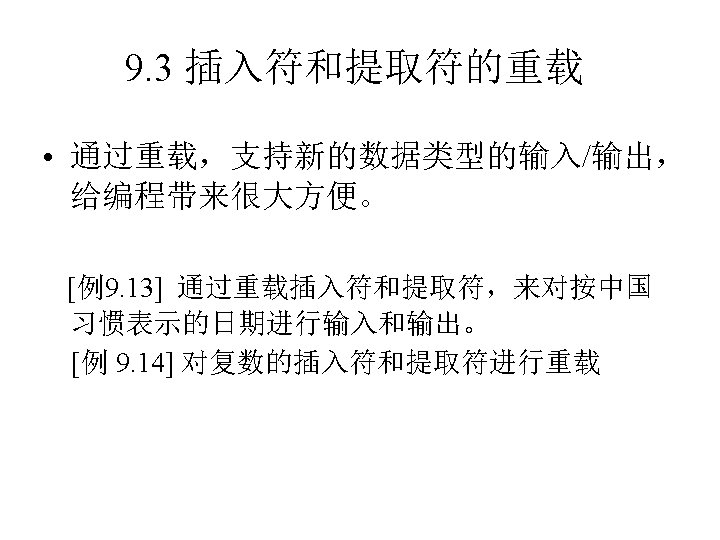
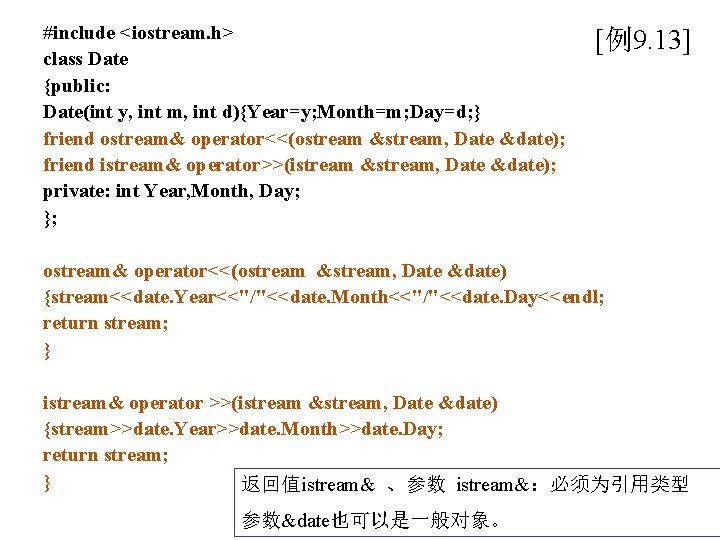
#include <iostream. h> class Date {public: Date(int y, int m, int d){Year=y; Month=m; Day=d; } friend ostream& operator<<(ostream &stream, Date &date); friend istream& operator>>(istream &stream, Date &date); private: int Year, Month, Day; }; [例9. 13] ostream& operator<<(ostream &stream, Date &date) {stream<<date. Year<<"/"<<date. Month<<"/"<<date. Day<<endl; return stream; } istream& operator >>(istream &stream, Date &date) {stream>>date. Year>>date. Month>>date. Day; return stream; } 返回值istream& 、参数 istream&:必须为引用类型 参数&date也可以是一般对象。
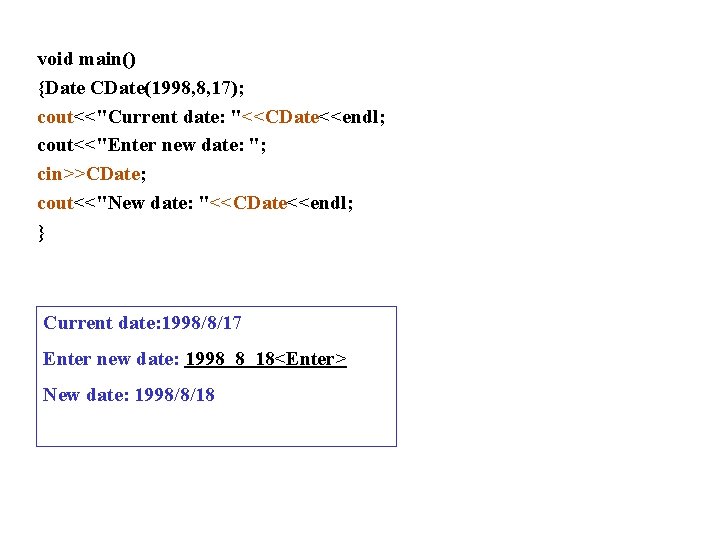
void main() {Date CDate(1998, 8, 17); cout<<"Current date: "<<CDate<<endl; cout<<"Enter new date: "; cin>>CDate; cout<<"New date: "<<CDate<<endl; } Current date: 1998/8/17 Enter new date: 1998 8 18<Enter> New date: 1998/8/18
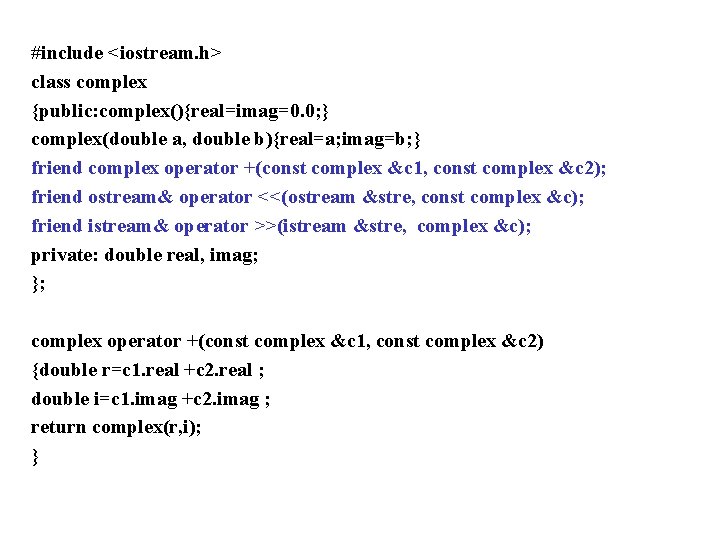
#include <iostream. h> class complex {public: complex(){real=imag=0. 0; } complex(double a, double b){real=a; imag=b; } friend complex operator +(const complex &c 1, const complex &c 2); friend ostream& operator <<(ostream &stre, const complex &c); friend istream& operator >>(istream &stre, complex &c); private: double real, imag; }; complex operator +(const complex &c 1, const complex &c 2) {double r=c 1. real +c 2. real ; double i=c 1. imag +c 2. imag ; return complex(r, i); }
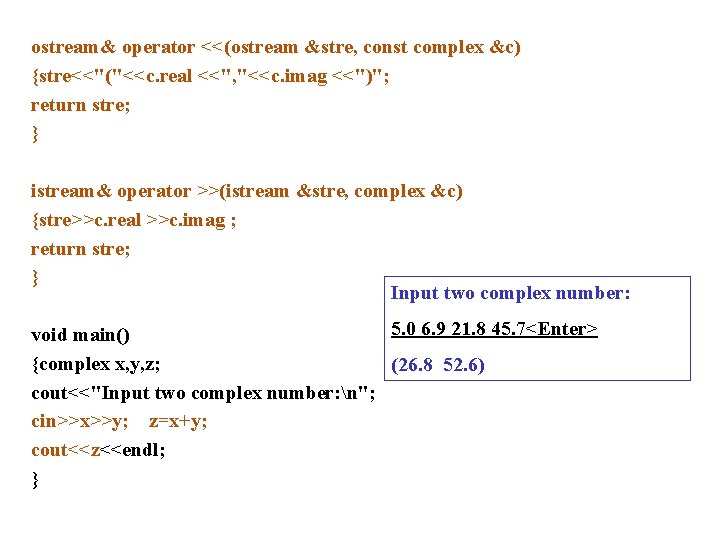
ostream& operator <<(ostream &stre, const complex &c) {stre<<"("<<c. real <<", "<<c. imag <<")"; return stre; } istream& operator >>(istream &stre, complex &c) {stre>>c. real >>c. imag ; return stre; } Input two complex number: 5. 0 6. 9 21. 8 45. 7<Enter> void main() {complex x, y, z; (26. 8 52. 6) cout<<"Input two complex number: n"; cin>>x>>y; z=x+y; cout<<z<<endl; }
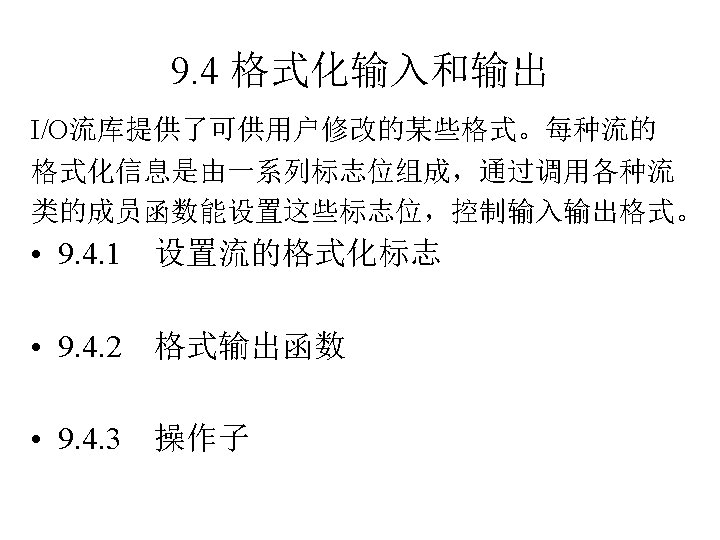
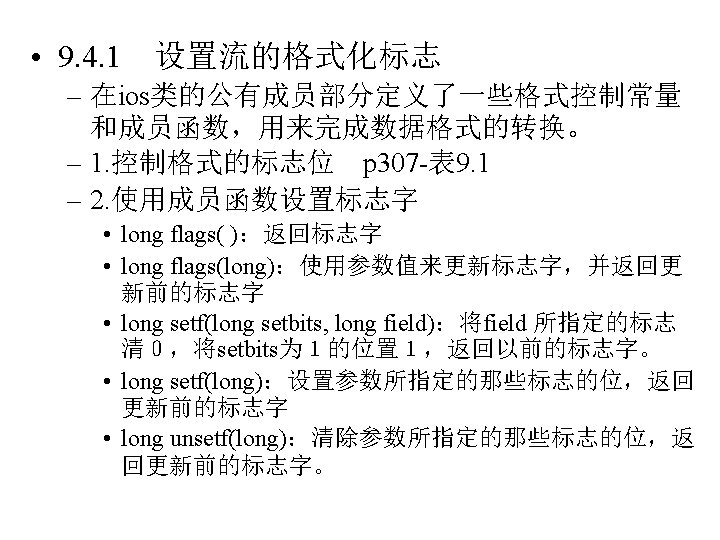
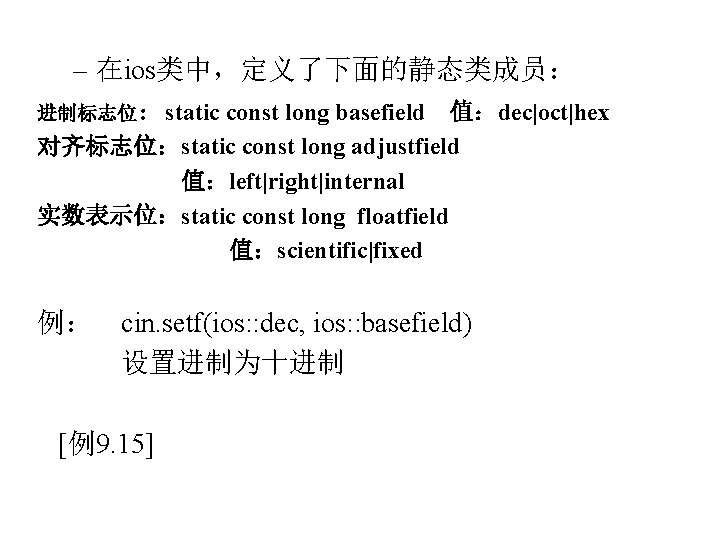
– 在ios类中,定义了下面的静态类成员: 进制标志位:static const long basefield 值:dec|oct|hex 对齐标志位:static const long adjustfield 值:left|right|internal 实数表示位:static const long floatfield 值:scientific|fixed 例: cin. setf(ios: : dec, ios: : basefield) 设置进制为十进制 [例9. 15]
![例9 15 include iostream h void main cout setf ios oct ios [例9. 15] #include <iostream. h> void main() { cout. setf (ios: : oct, ios:](https://slidetodoc.com/presentation_image_h2/7c66b15f1f13deff940824e0bc0eb3a8/image-29.jpg)
[例9. 15] #include <iostream. h> void main() { cout. setf (ios: : oct, ios: : basefield ); cout<<"OCT: 48 -->"<<48<<endl; OCT: 48 -->60 cout. setf (ios: : dec, ios: : basefield ); cout<<"DEC: 48 -->"<<48<<endl; DEC: 48 -->48 cout. setf (ios: : hex, ios: : basefield ); cout<<"HEX: 48 -->"<<48<<endl; HEX: 32 -->0 x 20 HEX: 48 -->30 HEX: 254 -->0 XFE cout. setf (ios: : showbase); //显示基数符号 cout<<"HEX: 32 -->"<<32<<endl; cout. setf(ios: : uppercase); //用大写字母输出十六进制数值 cout<<"HEX: 254 -->"<<254<<endl; }
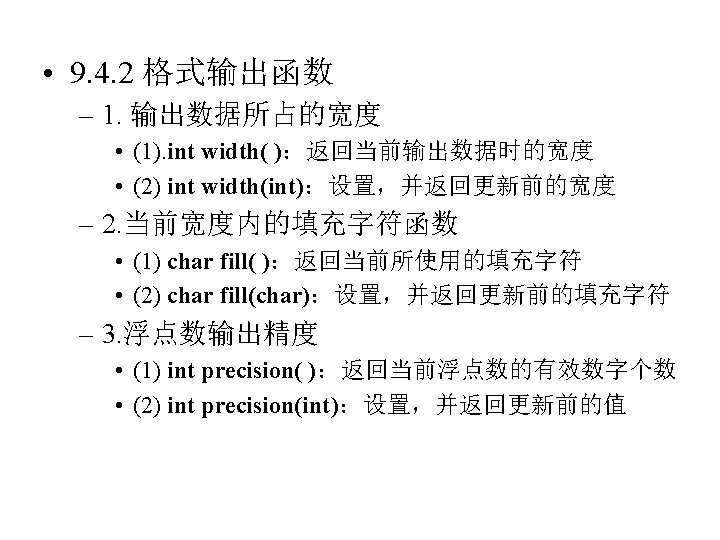
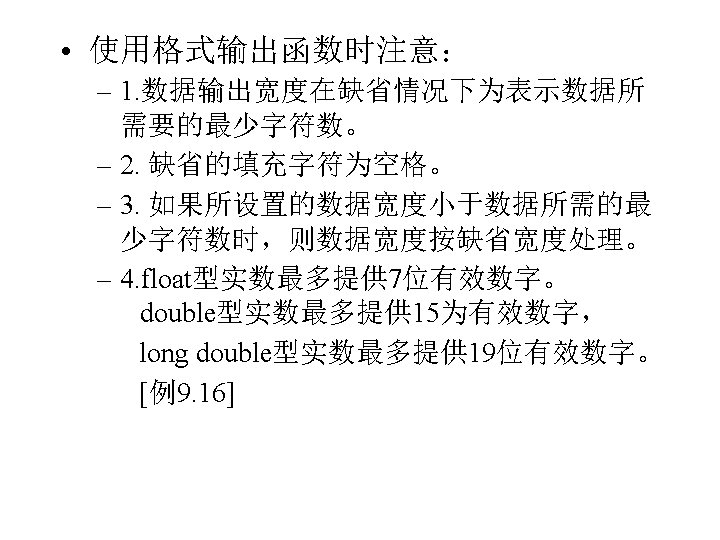
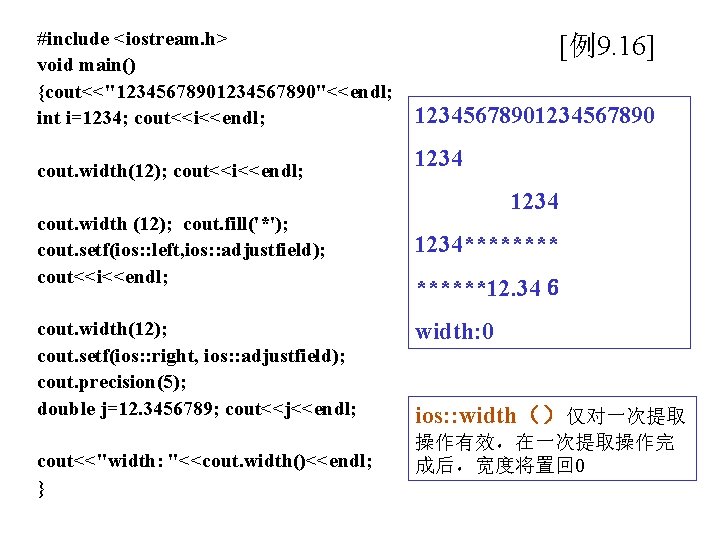
#include <iostream. h> void main() {cout<<"1234567890"<<endl; int i=1234; cout<<i<<endl; cout. width(12); cout<<i<<endl; cout. width (12); cout. fill('*'); cout. setf(ios: : left, ios: : adjustfield); cout<<i<<endl; cout. width(12); cout. setf(ios: : right, ios: : adjustfield); cout. precision(5); double j=12. 3456789; cout<<j<<endl; cout<<"width: "<<cout. width()<<endl; } [例9. 16] 1234567890 1234******12. 346 width: 0 ios: : width()仅对一次提取 操作有效,在一次提取操作完 成后,宽度将置回 0
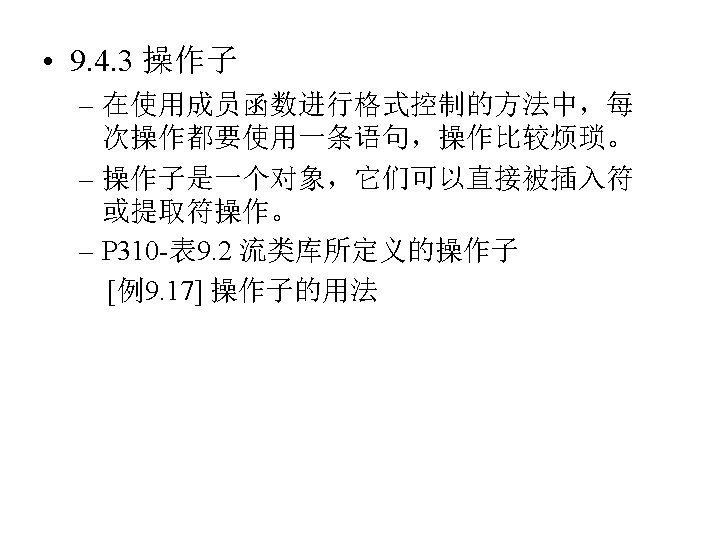
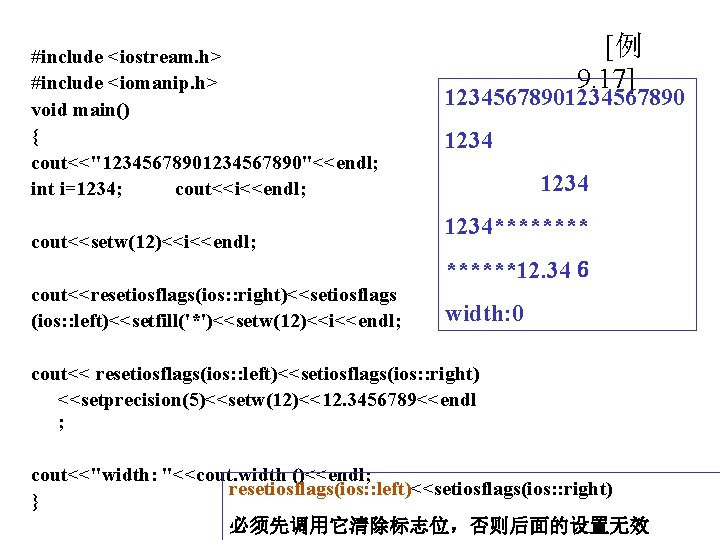
#include <iostream. h> #include <iomanip. h> void main() { cout<<"1234567890"<<endl; int i=1234; cout<<i<<endl; cout<<setw(12)<<i<<endl; [例 9. 17] 1234567890 1234******12. 346 cout<<resetiosflags(ios: : right)<<setiosflags (ios: : left)<<setfill('*')<<setw(12)<<i<<endl; width: 0 cout<< resetiosflags(ios: : left)<<setiosflags(ios: : right) <<setprecision(5)<<setw(12)<<12. 3456789<<endl ; cout<<"width: "<<cout. width ()<<endl; resetiosflags(ios: : left)<<setiosflags(ios: : right) } 必须先调用它清除标志位,否则后面的设置无效
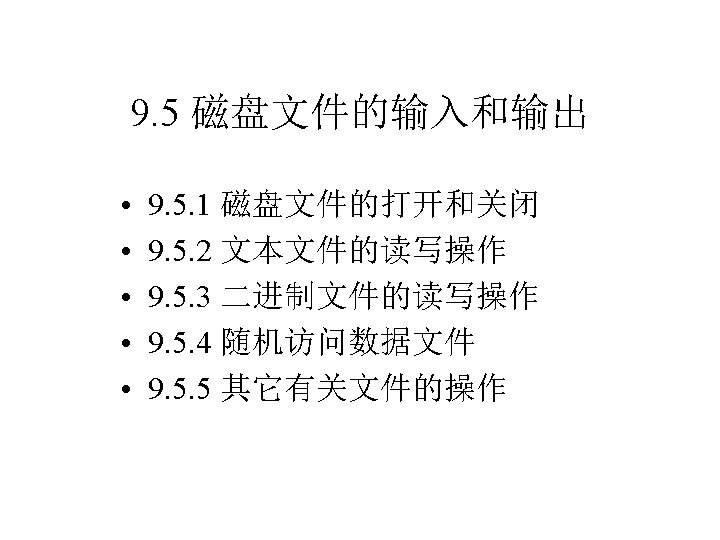
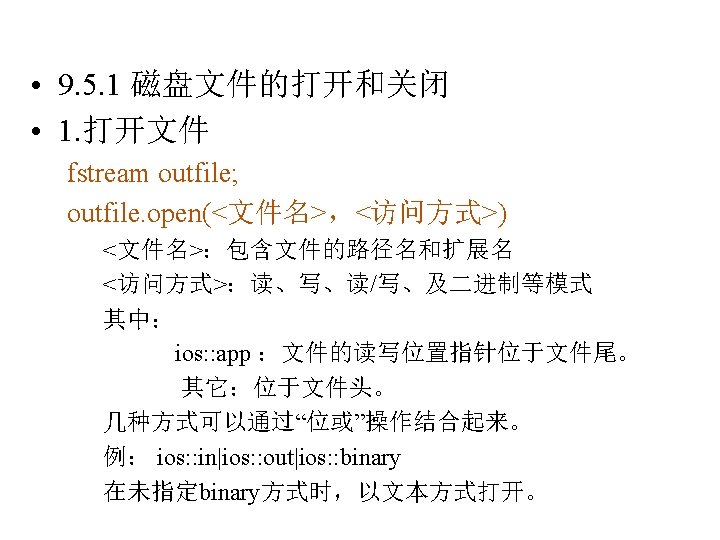
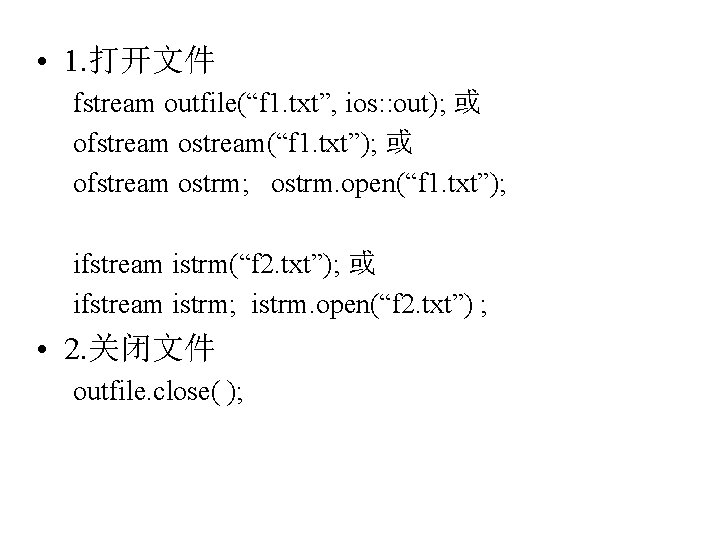
• 1. 打开文件 fstream outfile(“f 1. txt”, ios: : out); 或 ofstream ostream(“f 1. txt”); 或 ofstream ostrm; ostrm. open(“f 1. txt”); ifstream istrm(“f 2. txt”); 或 ifstream istrm; istrm. open(“f 2. txt”) ; • 2. 关闭文件 outfile. close( );
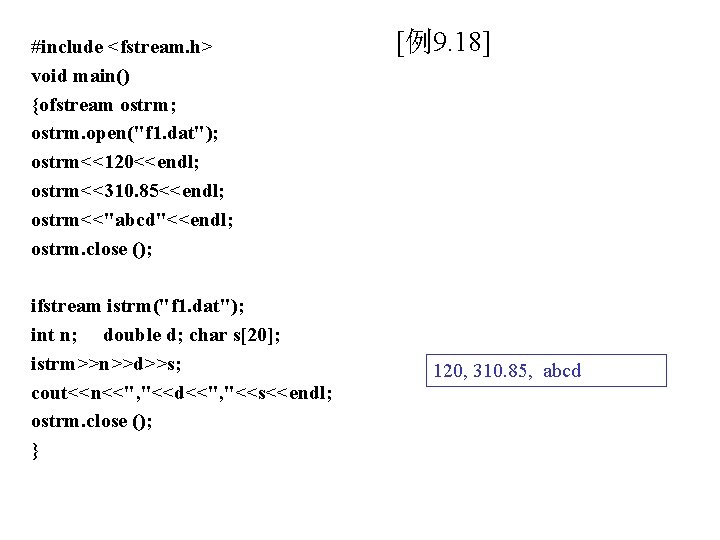
#include <fstream. h> void main() {ofstream ostrm; ostrm. open("f 1. dat"); ostrm<<120<<endl; ostrm<<310. 85<<endl; ostrm<<"abcd"<<endl; ostrm. close (); ifstream istrm("f 1. dat"); int n; double d; char s[20]; istrm>>n>>d>>s; cout<<n<<", "<<d<<", "<<s<<endl; ostrm. close (); } [例9. 18] 120, 310. 85, abcd
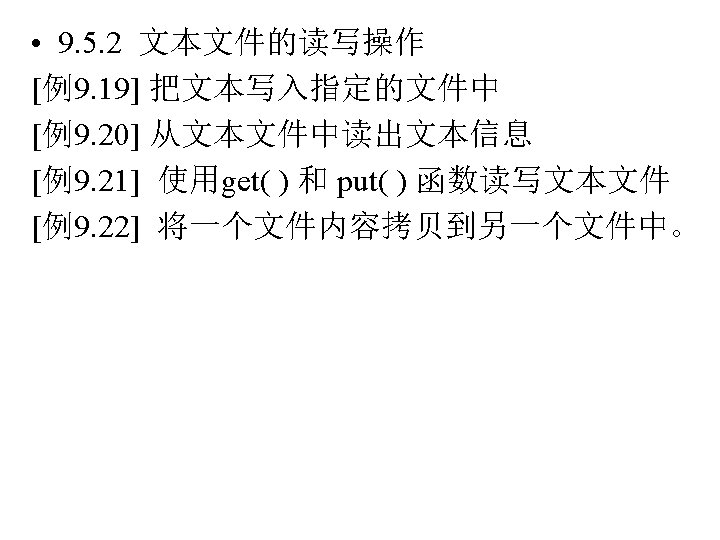
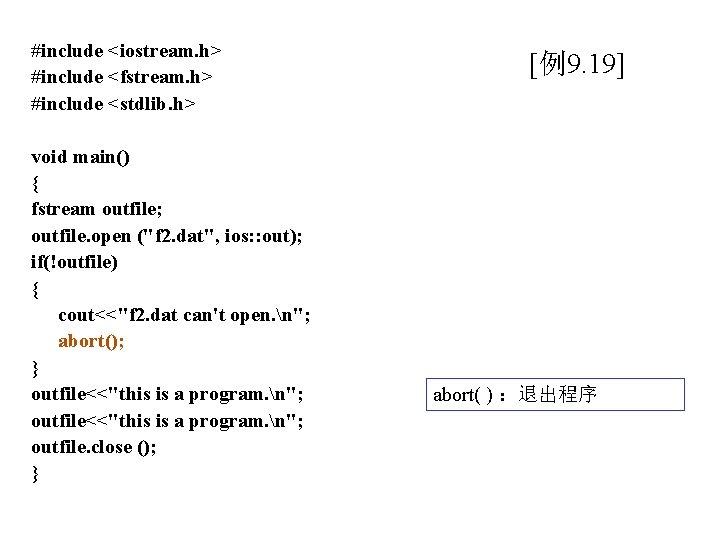
#include <iostream. h> #include <fstream. h> #include <stdlib. h> void main() { fstream outfile; outfile. open ("f 2. dat", ios: : out); if(!outfile) { cout<<"f 2. dat can't open. n"; abort(); } outfile<<"this is a program. n"; outfile. close (); } [例9. 19] abort( ) :退出程序
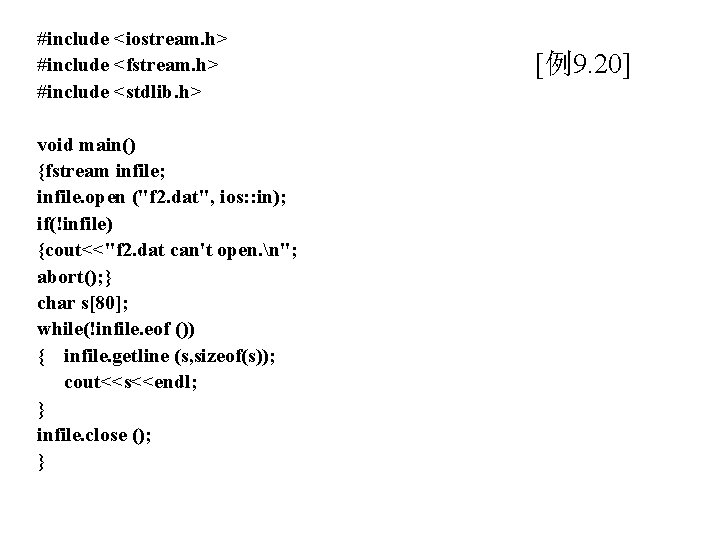
#include <iostream. h> #include <fstream. h> #include <stdlib. h> void main() {fstream infile; infile. open ("f 2. dat", ios: : in); if(!infile) {cout<<"f 2. dat can't open. n"; abort(); } char s[80]; while(!infile. eof ()) { infile. getline (s, sizeof(s)); cout<<s<<endl; } infile. close (); } [例9. 20]
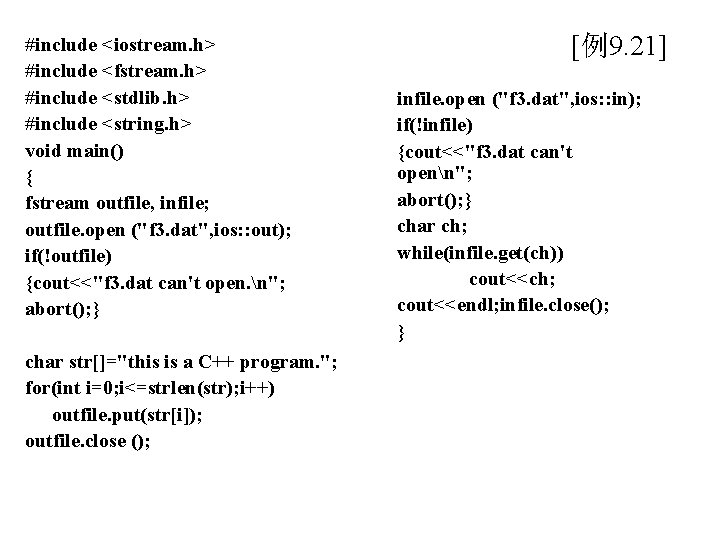
#include <iostream. h> #include <fstream. h> #include <stdlib. h> #include <string. h> void main() { fstream outfile, infile; outfile. open ("f 3. dat", ios: : out); if(!outfile) {cout<<"f 3. dat can't open. n"; abort(); } char str[]="this is a C++ program. "; for(int i=0; i<=strlen(str); i++) outfile. put(str[i]); outfile. close (); [例9. 21] infile. open ("f 3. dat", ios: : in); if(!infile) {cout<<"f 3. dat can't openn"; abort(); } char ch; while(infile. get(ch)) cout<<ch; cout<<endl; infile. close(); }
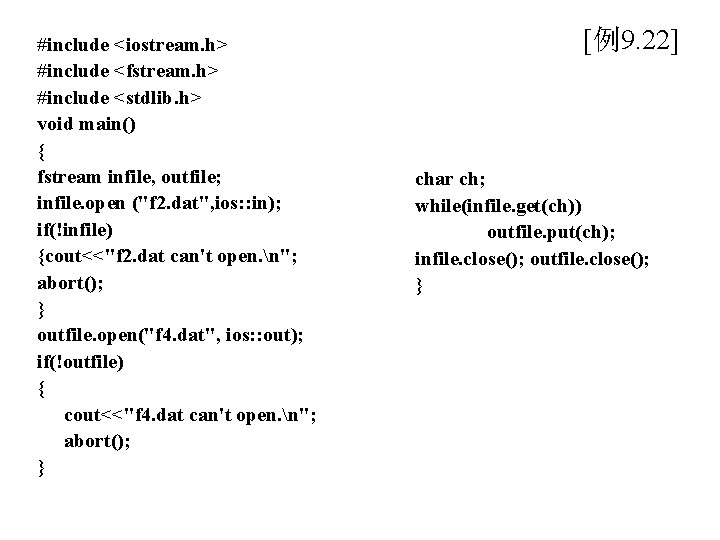
#include <iostream. h> #include <fstream. h> #include <stdlib. h> void main() { fstream infile, outfile; infile. open ("f 2. dat", ios: : in); if(!infile) {cout<<"f 2. dat can't open. n"; abort(); } outfile. open("f 4. dat", ios: : out); if(!outfile) { cout<<"f 4. dat can't open. n"; abort(); } [例9. 22] char ch; while(infile. get(ch)) outfile. put(ch); infile. close(); outfile. close(); }
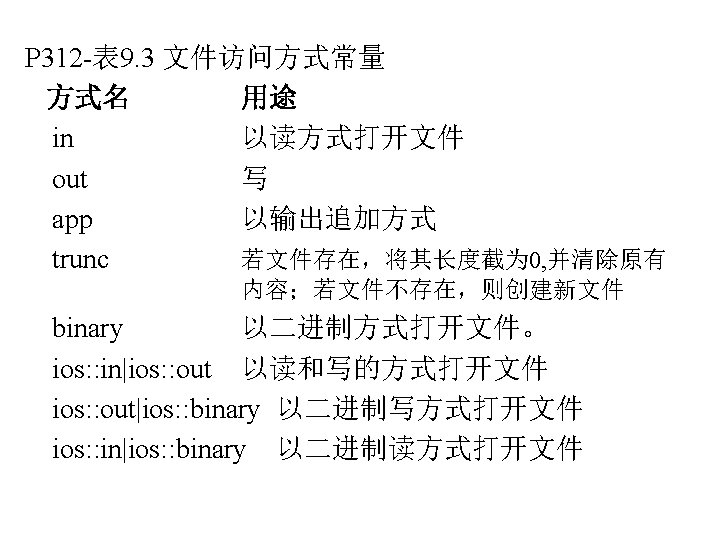
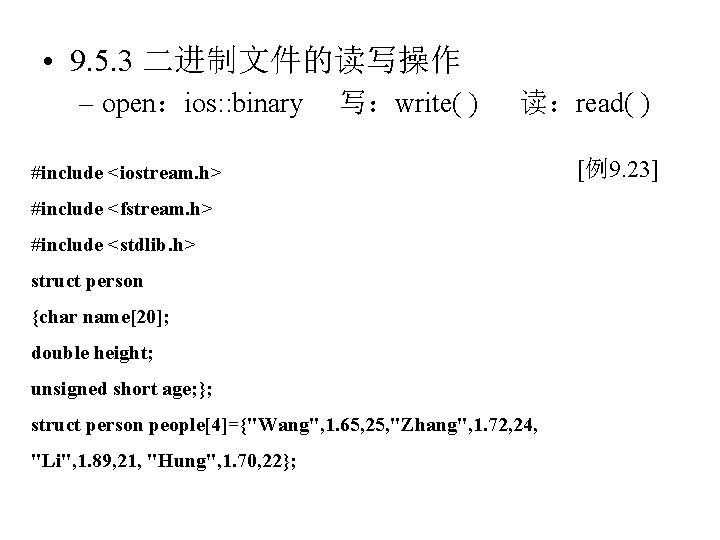
• 9. 5. 3 二进制文件的读写操作 – open:ios: : binary 写:write( ) 读:read( ) #include <iostream. h> #include <fstream. h> #include <stdlib. h> struct person {char name[20]; double height; unsigned short age; }; struct person people[4]={"Wang", 1. 65, 25, "Zhang", 1. 72, 24, "Li", 1. 89, 21, "Hung", 1. 70, 22}; [例9. 23]
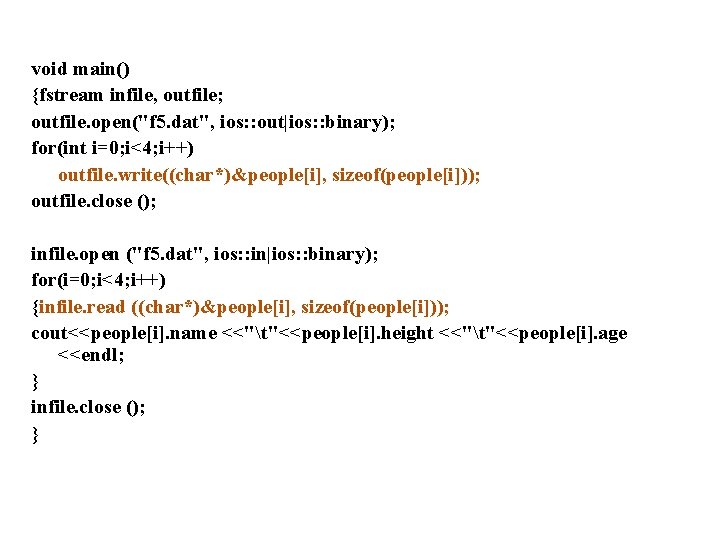
void main() {fstream infile, outfile; outfile. open("f 5. dat", ios: : out|ios: : binary); for(int i=0; i<4; i++) outfile. write((char*)&people[i], sizeof(people[i])); outfile. close (); infile. open ("f 5. dat", ios: : in|ios: : binary); for(i=0; i<4; i++) {infile. read ((char*)&people[i], sizeof(people[i])); cout<<people[i]. name <<"t"<<people[i]. height <<"t"<<people[i]. age <<endl; } infile. close (); }
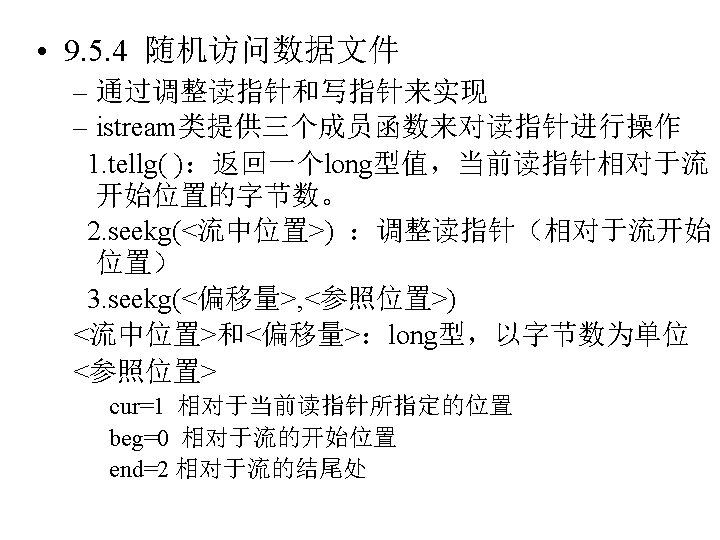
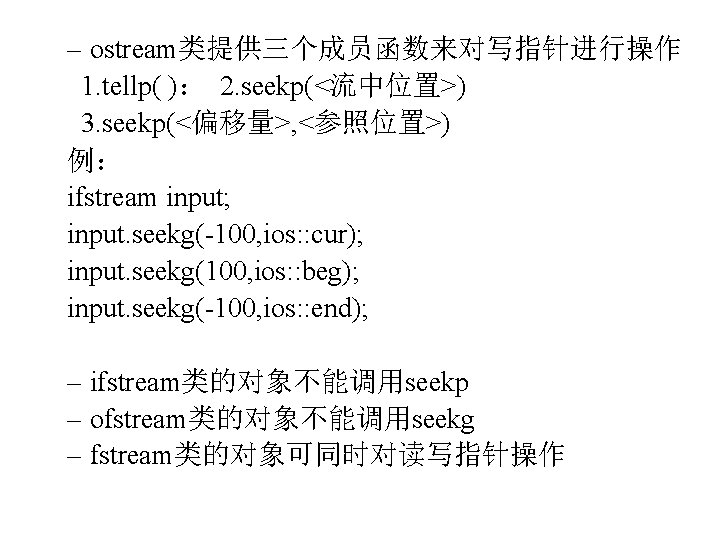
– ostream类提供三个成员函数来对写指针进行操作 1. tellp( ): 2. seekp(<流中位置>) 3. seekp(<偏移量>, <参照位置>) 例: ifstream input; input. seekg(-100, ios: : cur); input. seekg(100, ios: : beg); input. seekg(-100, ios: : end); – ifstream类的对象不能调用seekp – ofstream类的对象不能调用seekg – fstream类的对象可同时对读写指针操作
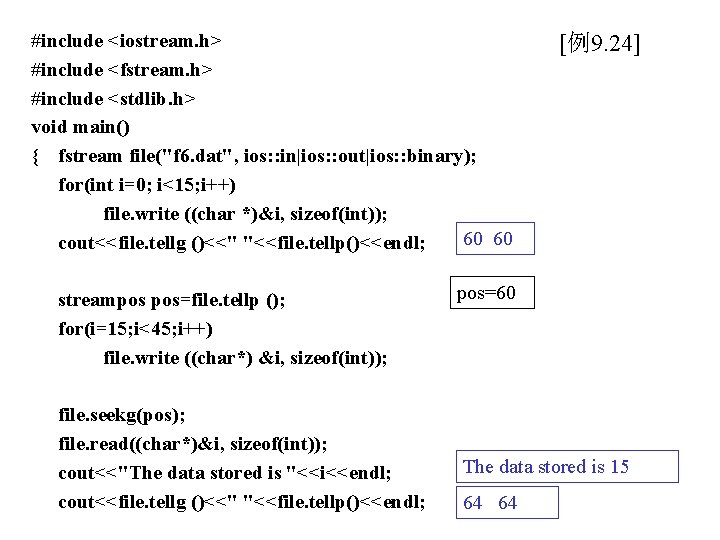
#include <iostream. h> #include <fstream. h> #include <stdlib. h> void main() { fstream file("f 6. dat", ios: : in|ios: : out|ios: : binary); for(int i=0; i<15; i++) file. write ((char *)&i, sizeof(int)); 60 60 cout<<file. tellg ()<<" "<<file. tellp()<<endl; streampos pos=file. tellp (); for(i=15; i<45; i++) file. write ((char*) &i, sizeof(int)); file. seekg(pos); file. read((char*)&i, sizeof(int)); cout<<"The data stored is "<<i<<endl; cout<<file. tellg ()<<" "<<file. tellp()<<endl; [例9. 24] pos=60 The data stored is 15 64 64
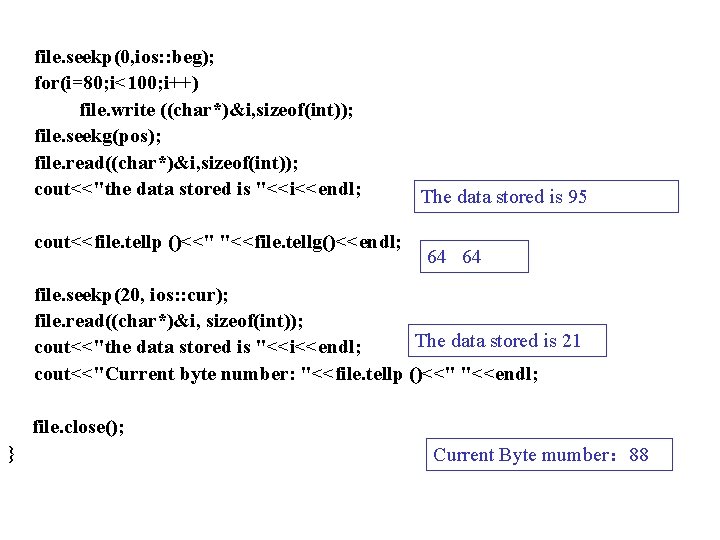
file. seekp(0, ios: : beg); for(i=80; i<100; i++) file. write ((char*)&i, sizeof(int)); file. seekg(pos); file. read((char*)&i, sizeof(int)); cout<<"the data stored is "<<i<<endl; cout<<file. tellp ()<<" "<<file. tellg()<<endl; The data stored is 95 64 64 file. seekp(20, ios: : cur); file. read((char*)&i, sizeof(int)); The data stored is 21 cout<<"the data stored is "<<i<<endl; cout<<"Current byte number: "<<file. tellp ()<<" "<<endl; file. close(); } Current Byte mumber: 88
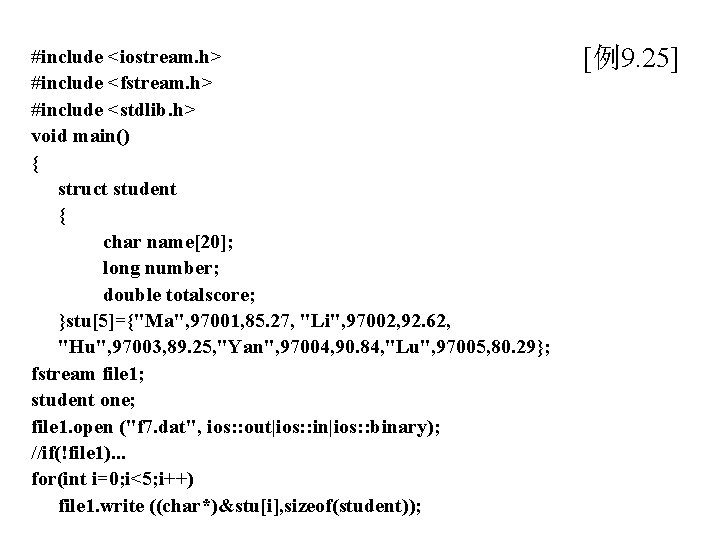
#include <iostream. h> #include <fstream. h> #include <stdlib. h> void main() { struct student { char name[20]; long number; double totalscore; }stu[5]={"Ma", 97001, 85. 27, "Li", 97002, 92. 62, "Hu", 97003, 89. 25, "Yan", 97004, 90. 84, "Lu", 97005, 80. 29}; fstream file 1; student one; file 1. open ("f 7. dat", ios: : out|ios: : in|ios: : binary); //if(!file 1). . . for(int i=0; i<5; i++) file 1. write ((char*)&stu[i], sizeof(student)); [例9. 25]
![file 1 seekp sizeofstudent4 file 1 readcharone sizeofstui coutone nametone numbertone totalscoreendl Lu 97005 file 1. seekp (sizeof(student)*4); file 1. read((char*)&one, sizeof(stu[i])); cout<<one. name<<"t"<<one. number<<"t"<<one. totalscore<<endl; Lu 97005](https://slidetodoc.com/presentation_image_h2/7c66b15f1f13deff940824e0bc0eb3a8/image-52.jpg)
file 1. seekp (sizeof(student)*4); file 1. read((char*)&one, sizeof(stu[i])); cout<<one. name<<"t"<<one. number<<"t"<<one. totalscore<<endl; Lu 97005 80. 92 file 1. seekp(sizeof(student)*1); file 1. read ((char*)&one, sizeof(stu[i])); cout<<one. name<<"t"<<one. number<<"t"<<one. totalscore<<endl; Li 97002 92. 62 file 1. close (); }
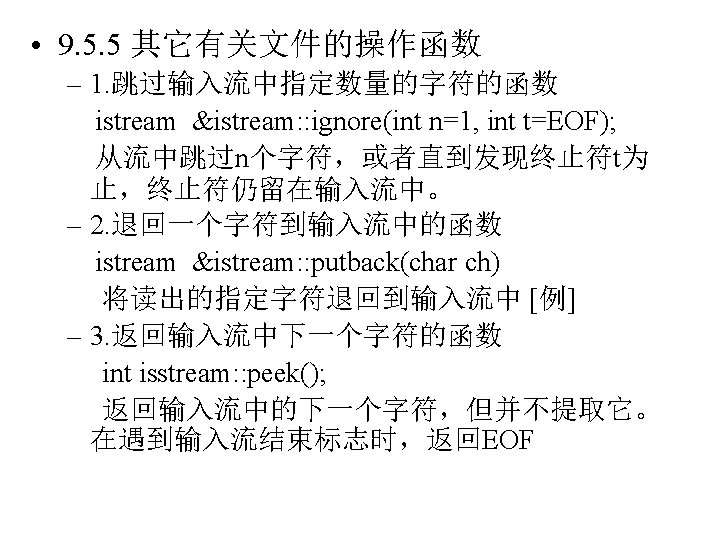
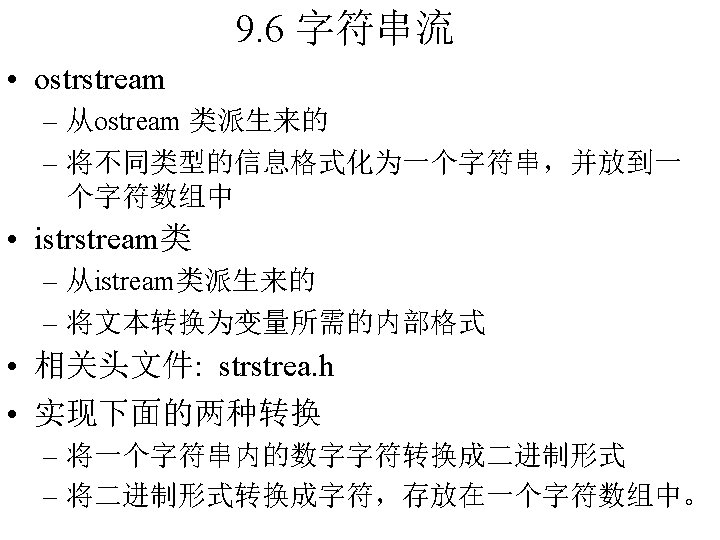
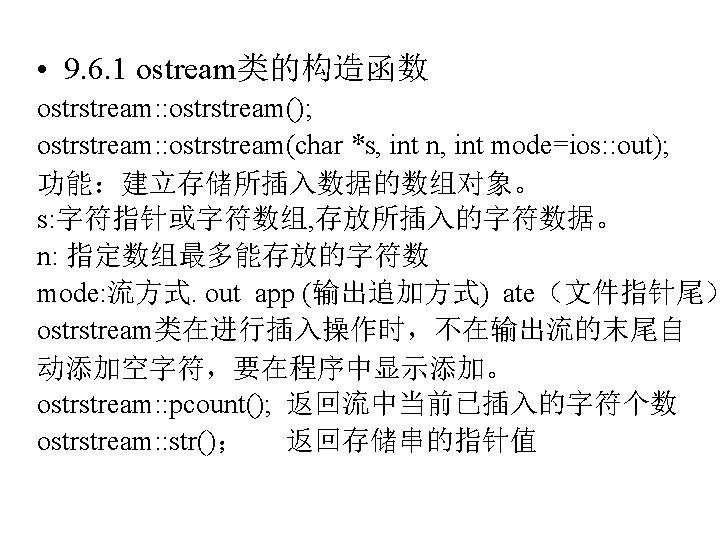
![include fstream h include strstrea h const int N80 void main char bufN #include <fstream. h> #include <strstrea. h> const int N=80; void main() { char buf[N];](https://slidetodoc.com/presentation_image_h2/7c66b15f1f13deff940824e0bc0eb3a8/image-56.jpg)
#include <fstream. h> #include <strstrea. h> const int N=80; void main() { char buf[N]; ostrstream out 1(buf, sizeof(buf)); int a=50; for(int i=0; i<6; i++, a+=10) out 1<<"a="<<a<<"; "; out 1<<'