826 class Car def driveself self speed 10
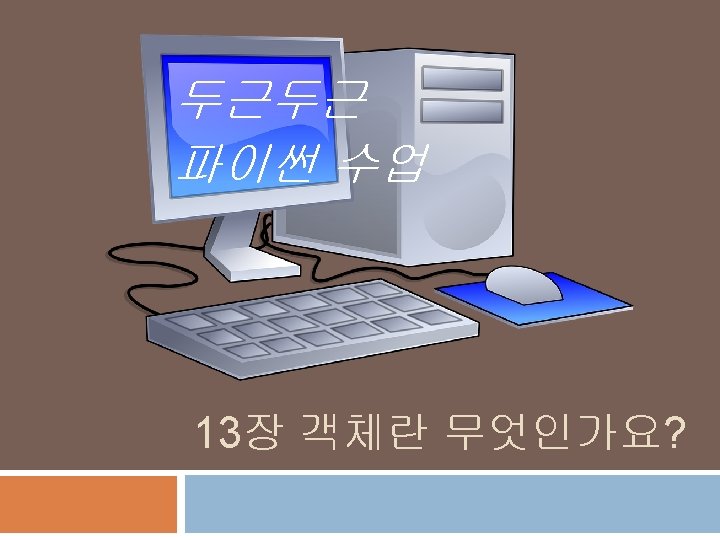
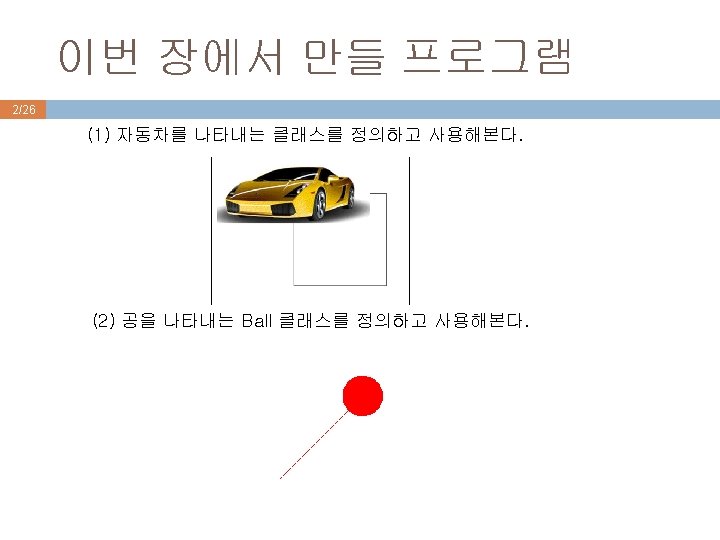
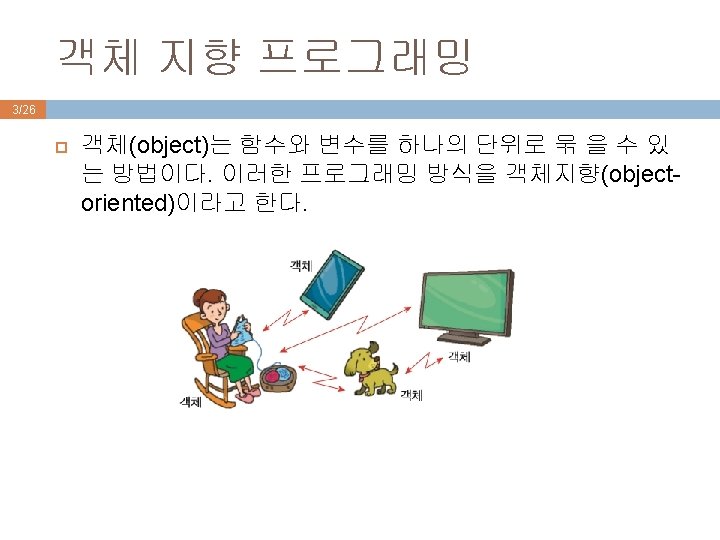
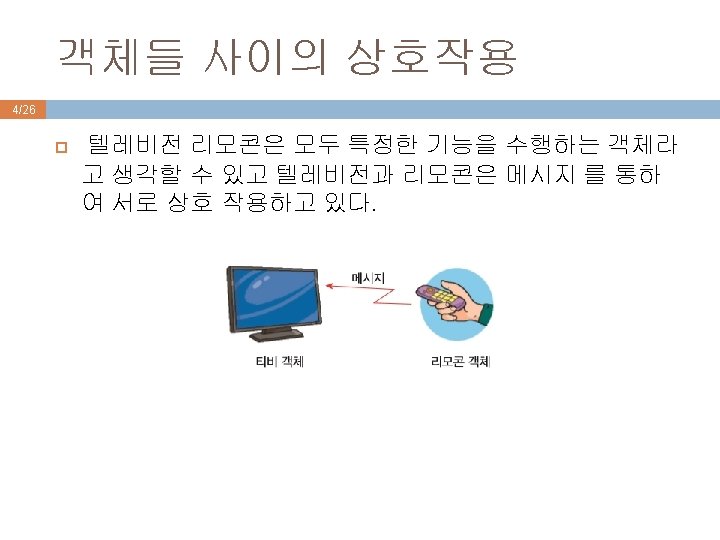
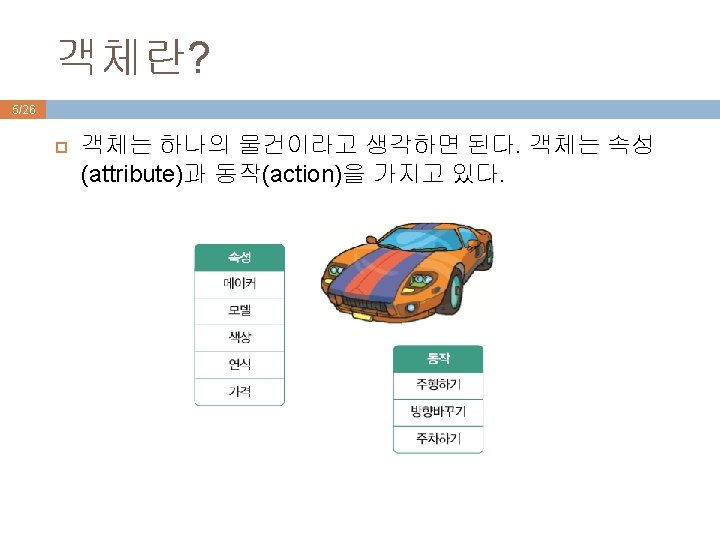
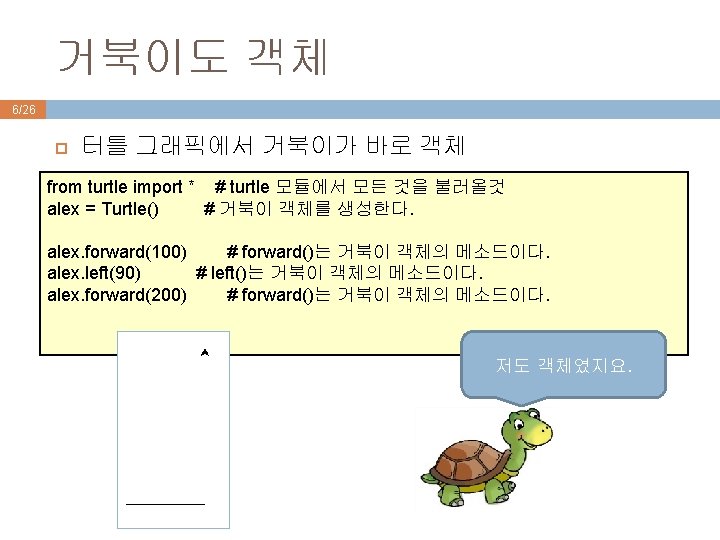
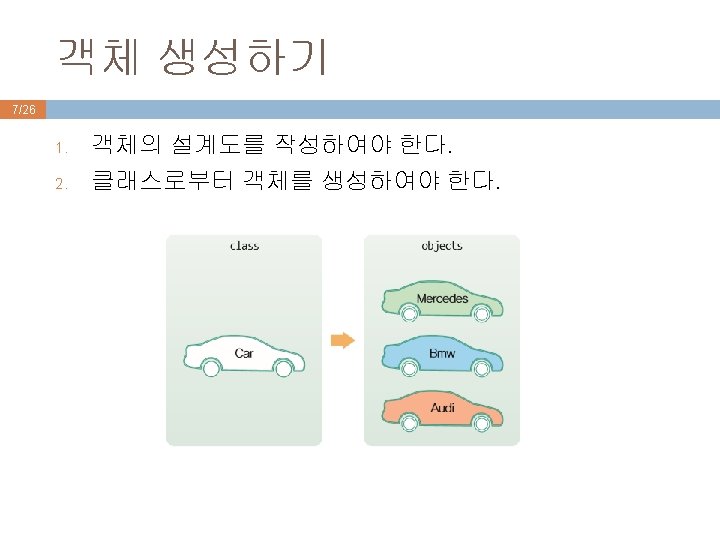
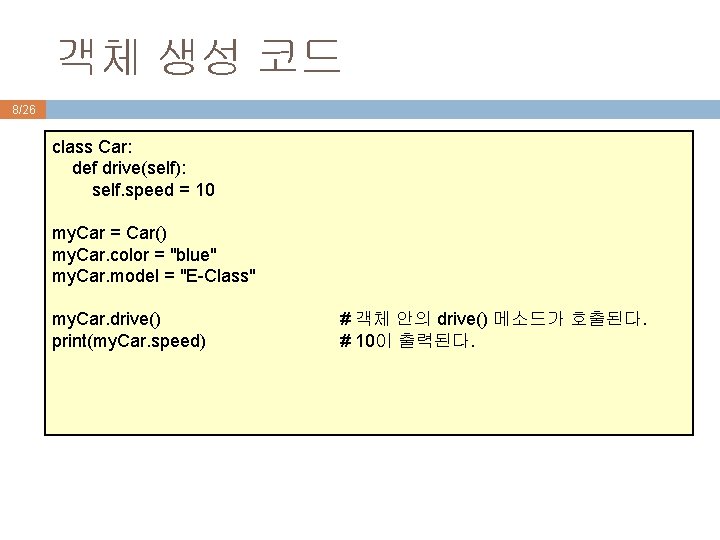
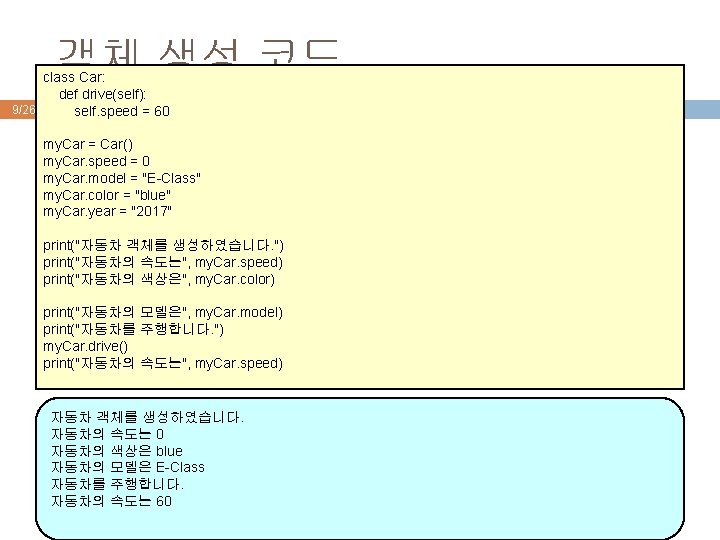
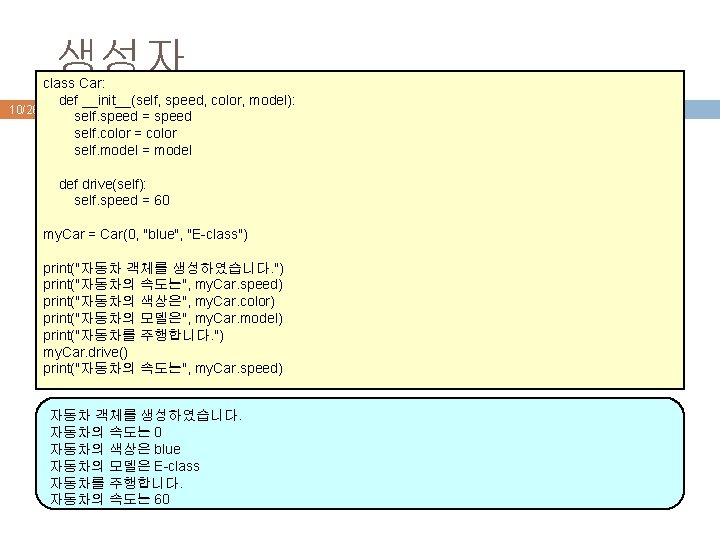
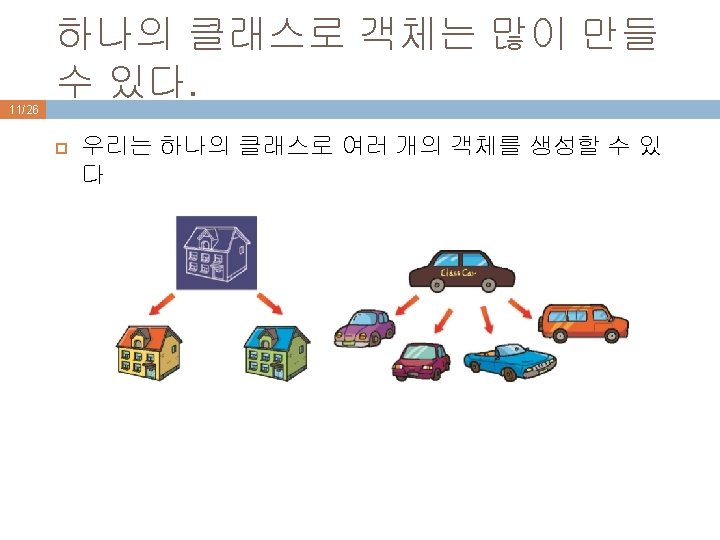
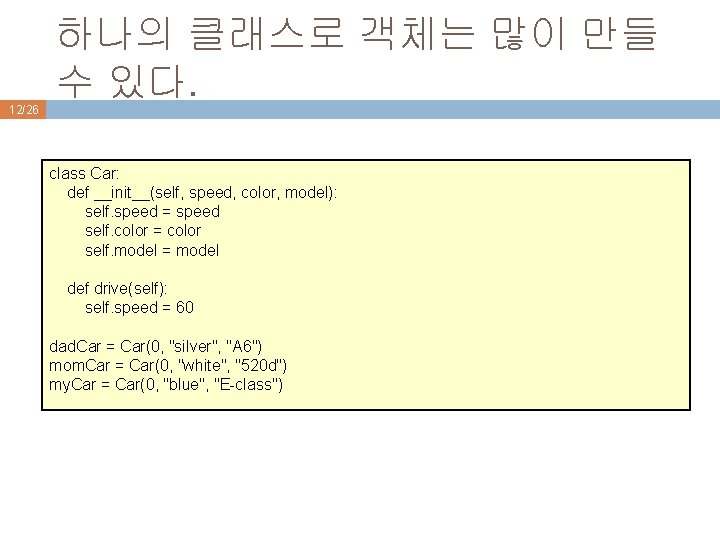
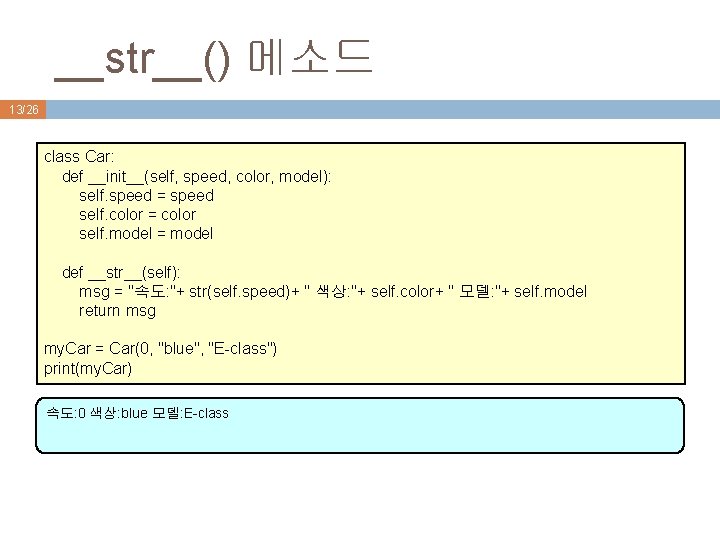
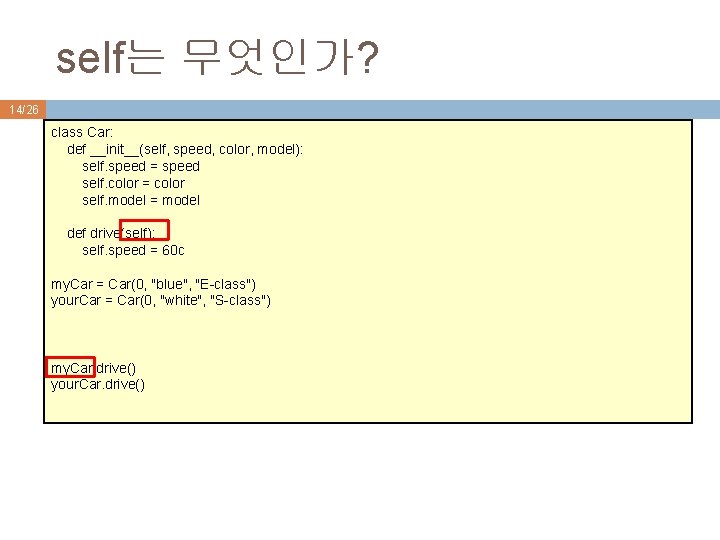
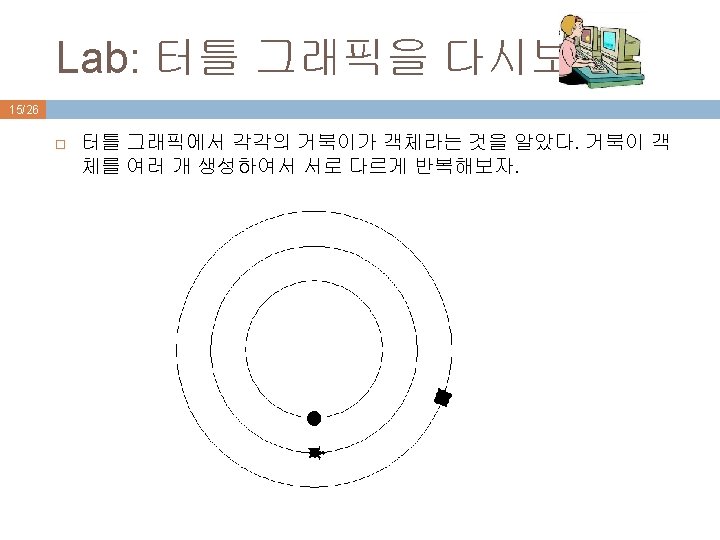
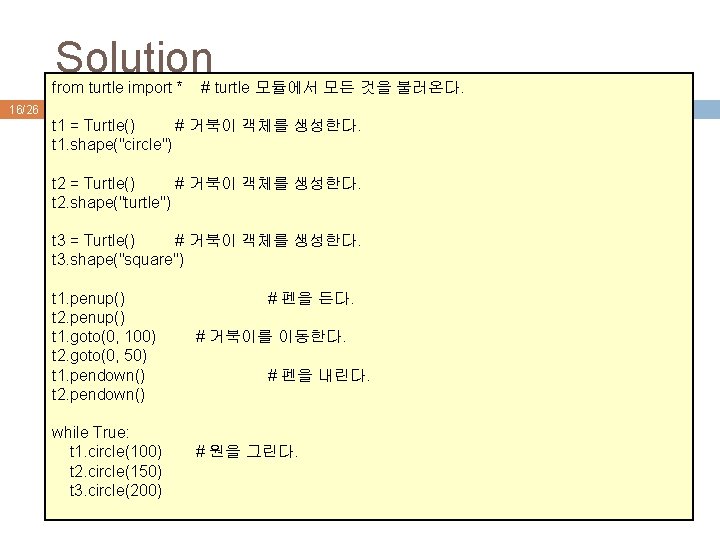
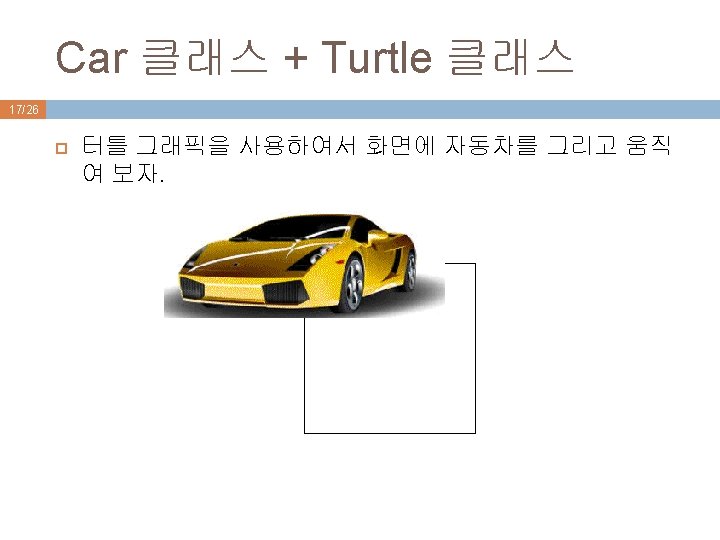
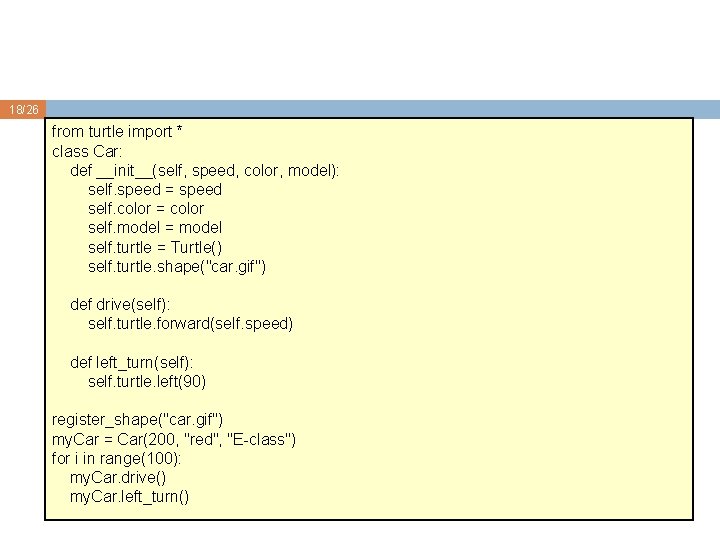
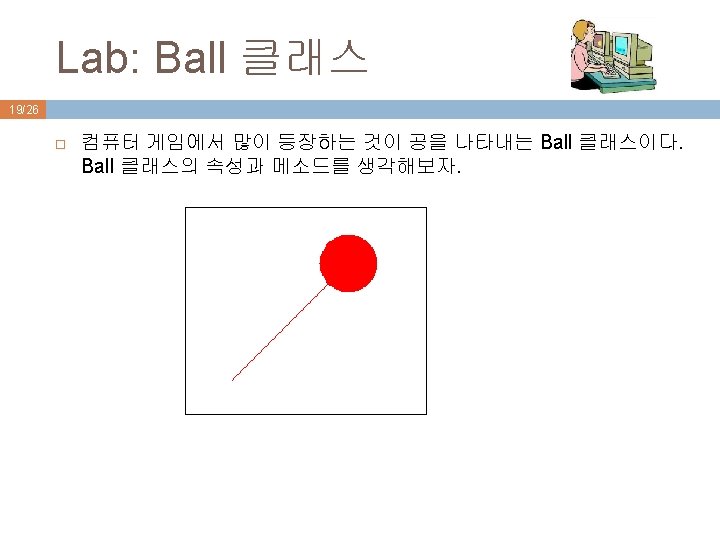
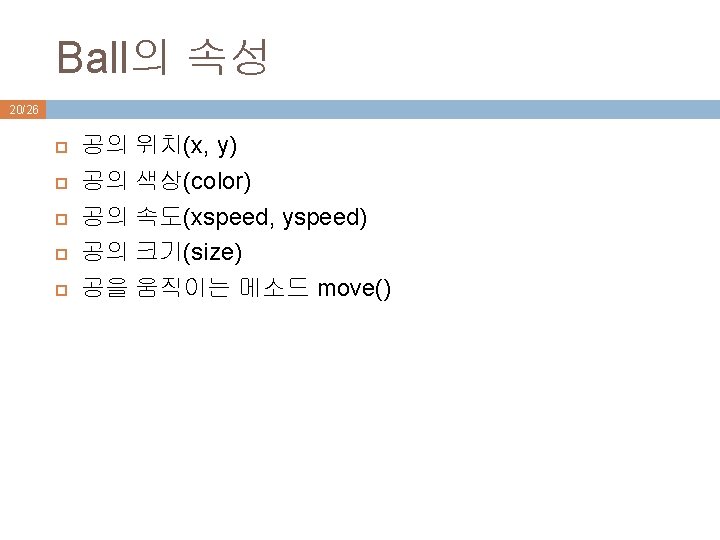
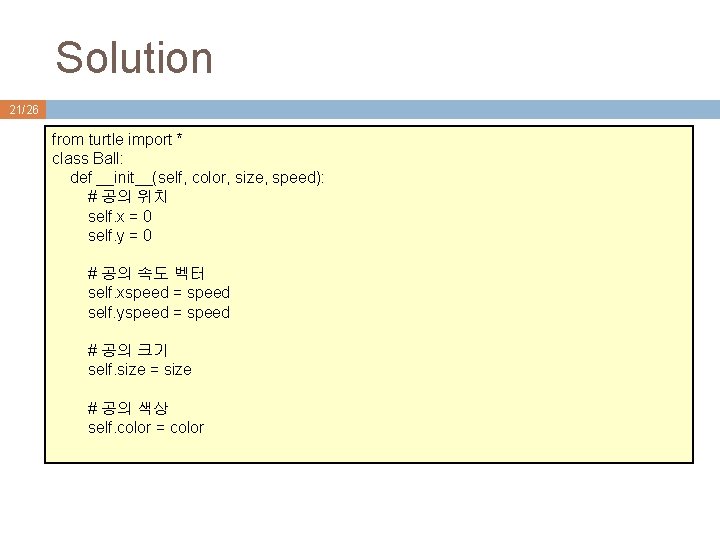
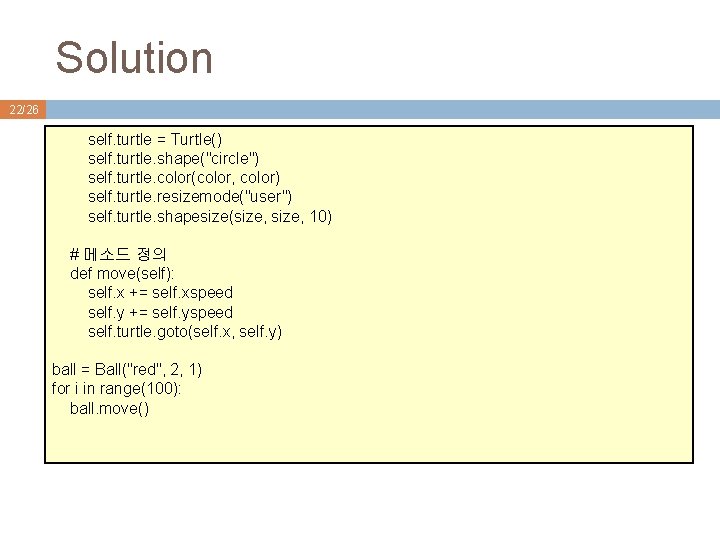
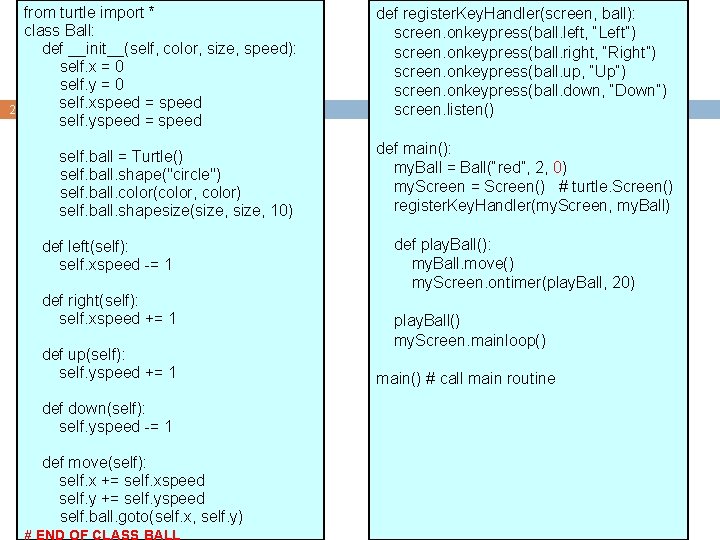
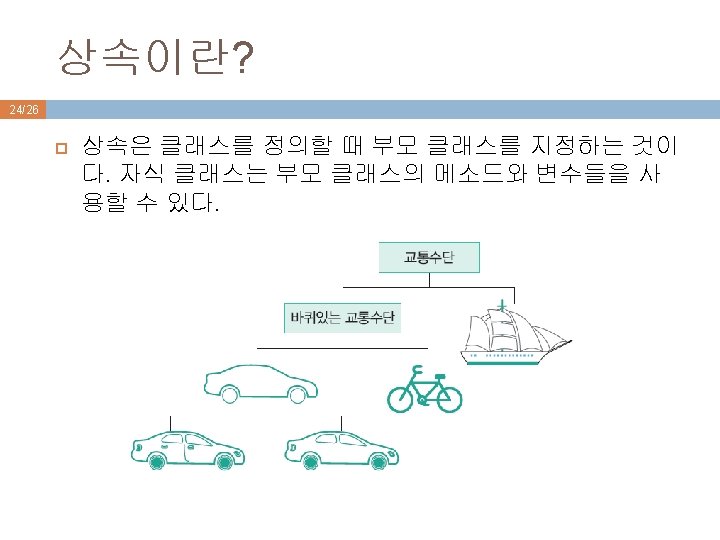
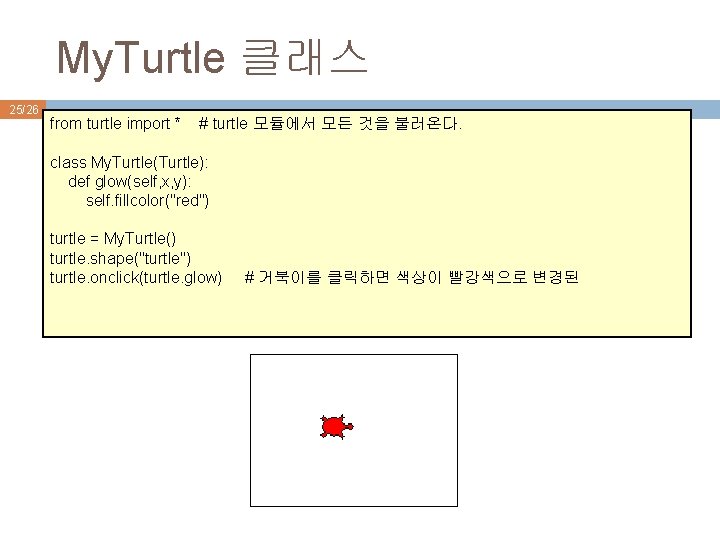
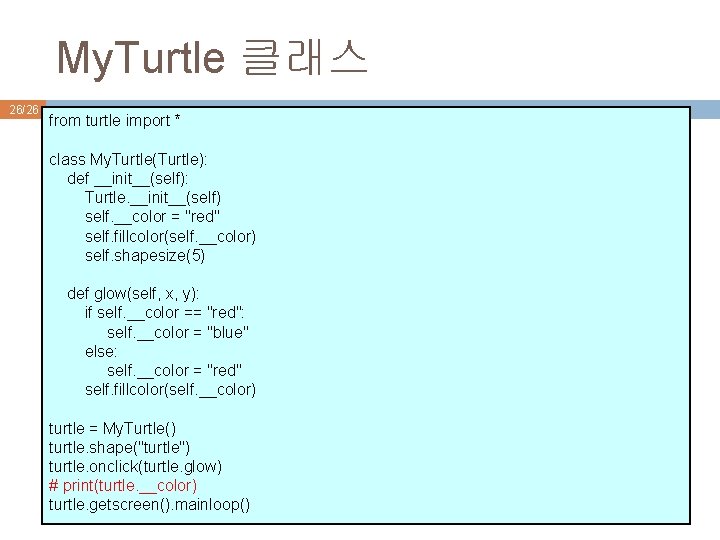
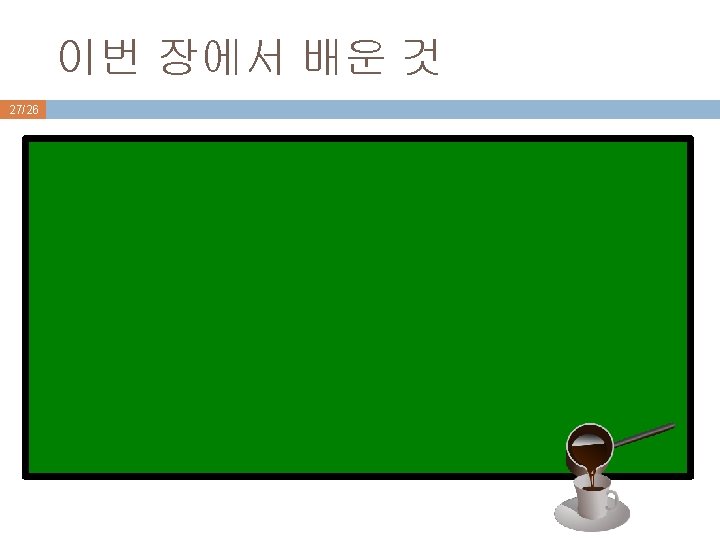
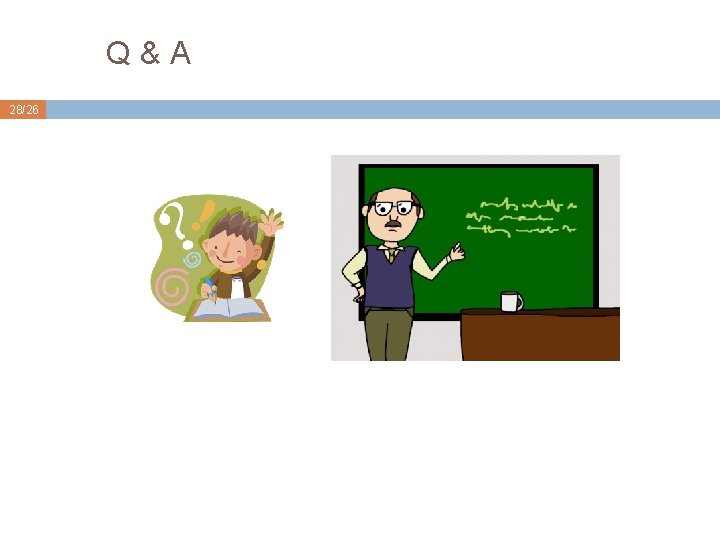
- Slides: 28
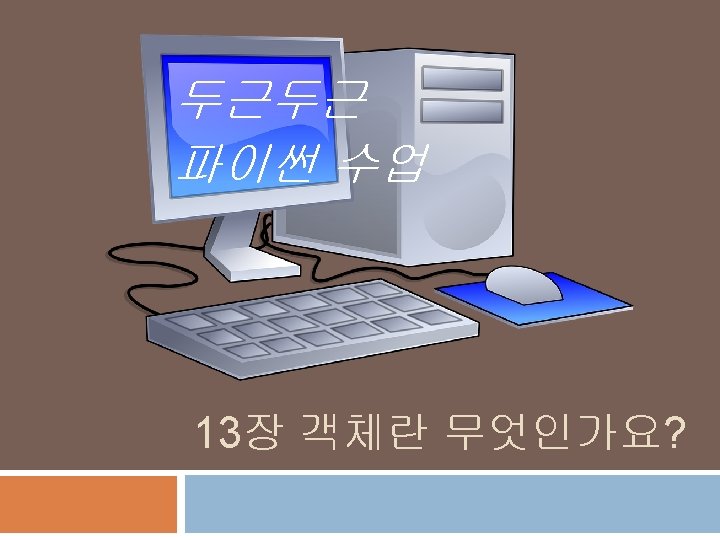
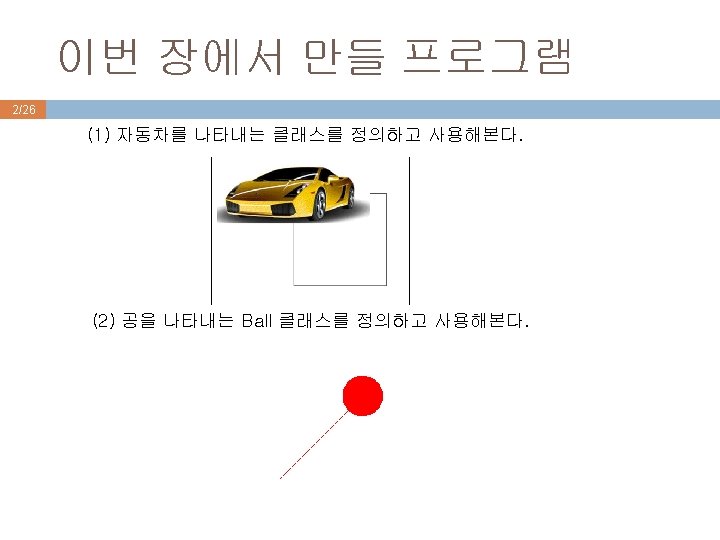
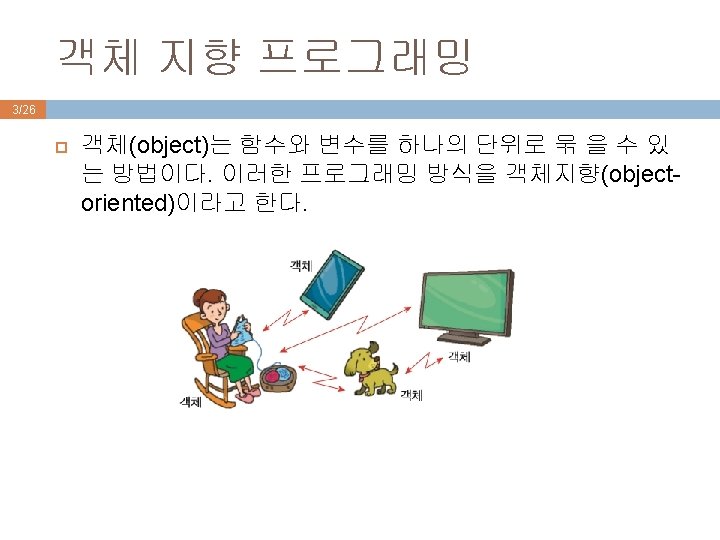
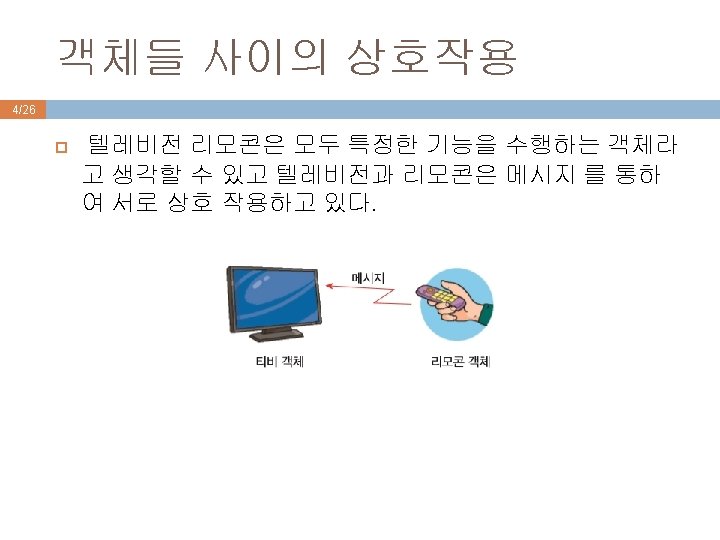
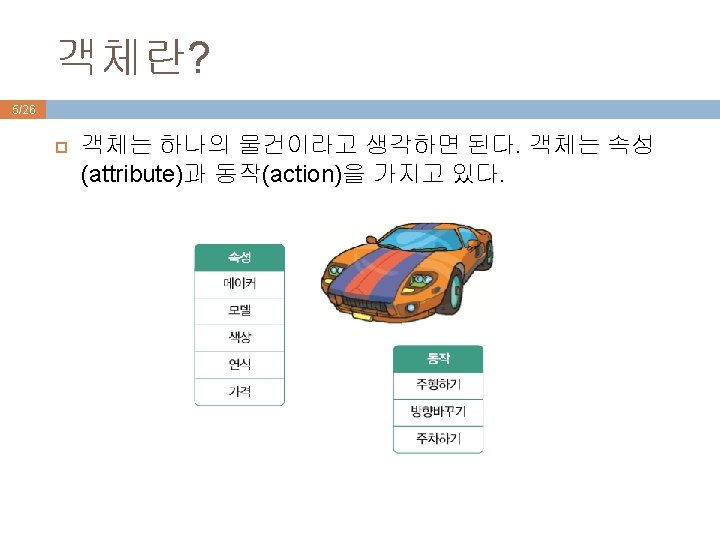
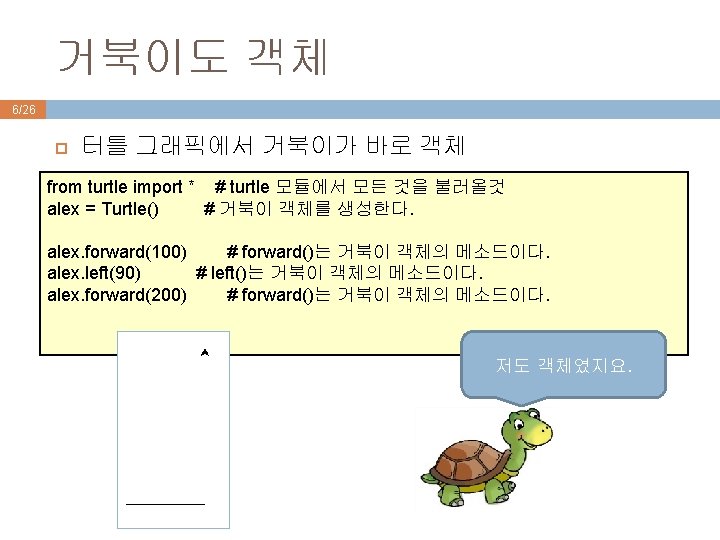
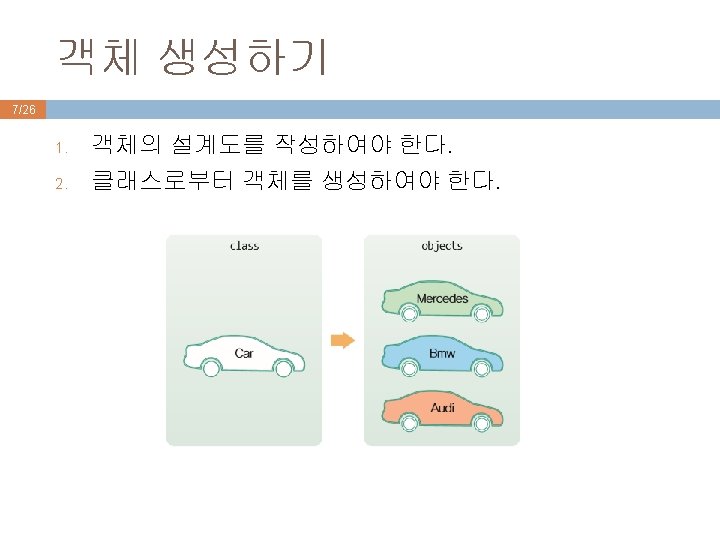
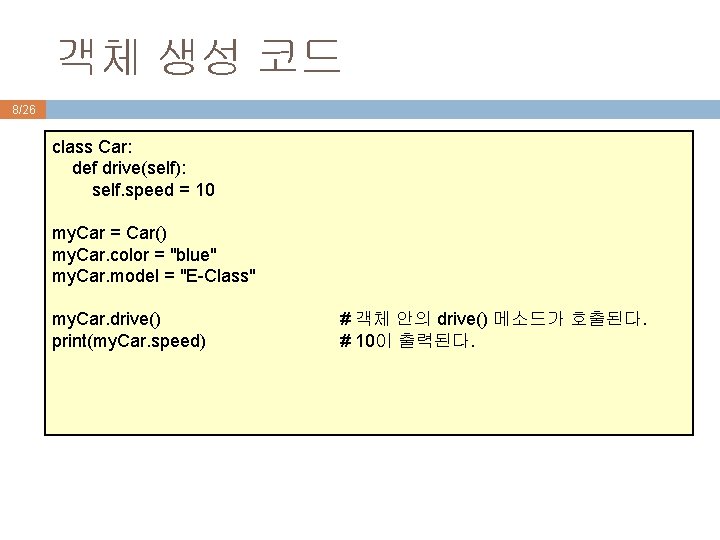
객체 생성 코드 8/26 class Car: def drive(self): self. speed = 10 my. Car = Car() my. Car. color = "blue" my. Car. model = "E-Class" my. Car. drive() print(my. Car. speed) # 객체 안의 drive() 메소드가 호출된다. # 10이 출력된다.
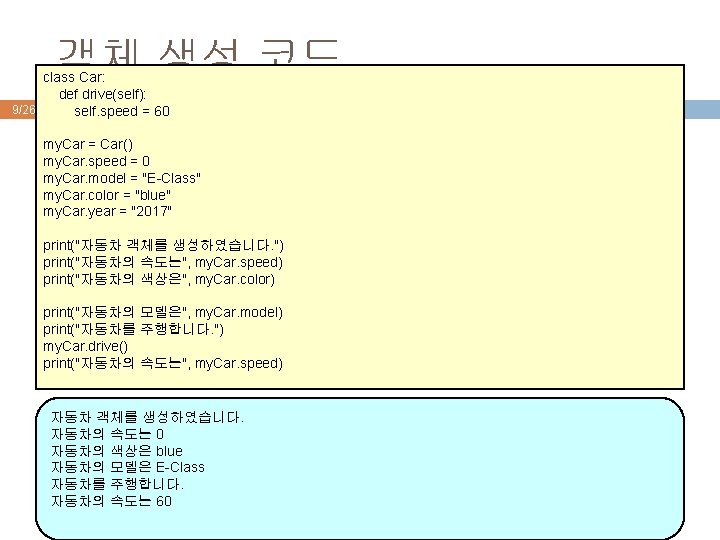
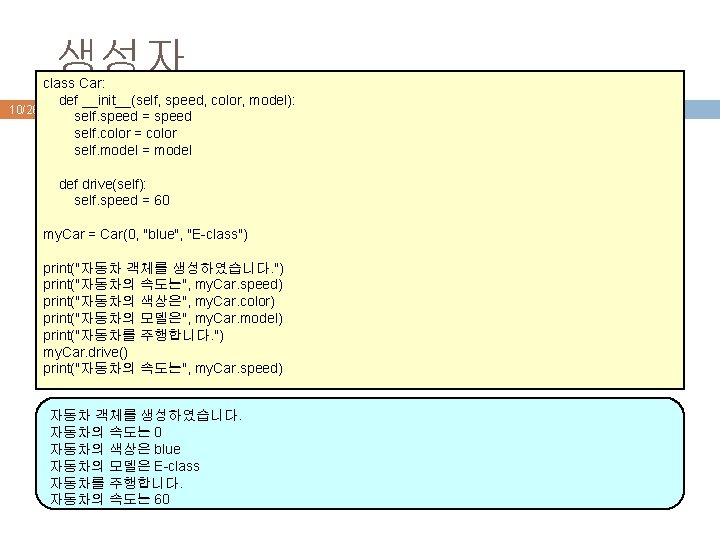
생성자 class Car: def __init__(self, speed, color, model): 10/26 self. speed = speed self. color = color self. model = model def drive(self): self. speed = 60 my. Car = Car(0, "blue", "E-class") print("자동차 객체를 생성하였습니다. ") print("자동차의 속도는", my. Car. speed) print("자동차의 색상은", my. Car. color) print("자동차의 모델은", my. Car. model) print("자동차를 주행합니다. ") my. Car. drive() print("자동차의 속도는", my. Car. speed) 자동차 객체를 생성하였습니다. 자동차의 속도는 0 자동차의 색상은 blue 자동차의 모델은 E-class 자동차를 주행합니다. 자동차의 속도는 60
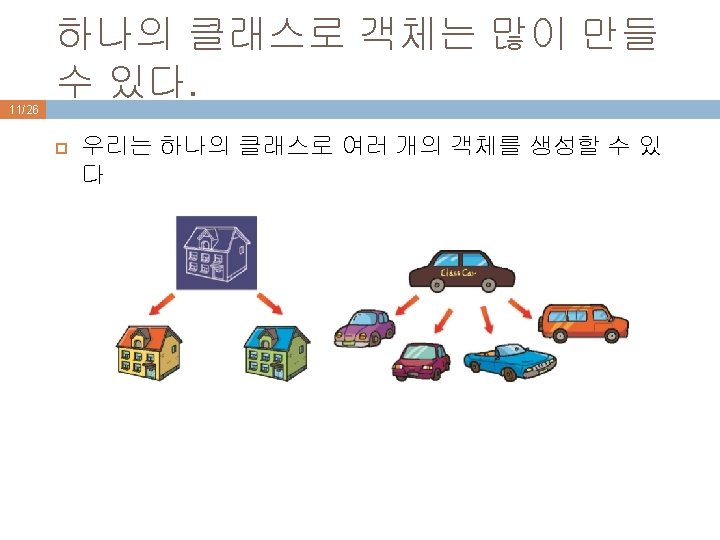
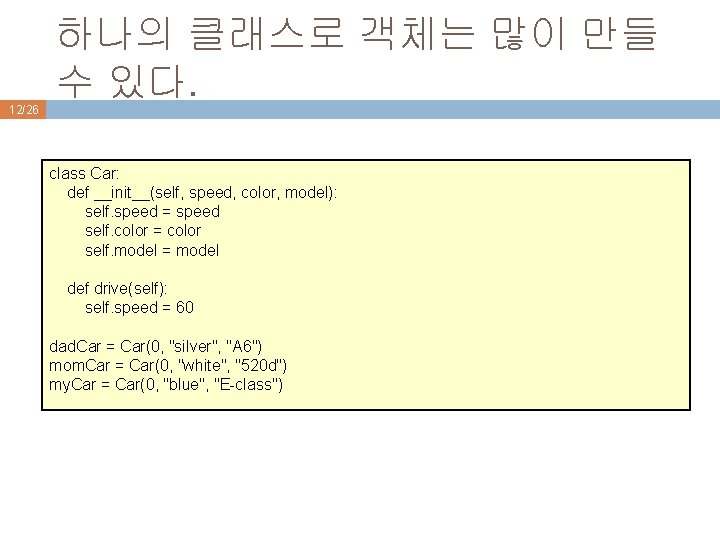
하나의 클래스로 객체는 많이 만들 수 있다. 12/26 class Car: def __init__(self, speed, color, model): self. speed = speed self. color = color self. model = model def drive(self): self. speed = 60 dad. Car = Car(0, "silver", "A 6") mom. Car = Car(0, "white", "520 d") my. Car = Car(0, "blue", "E-class")
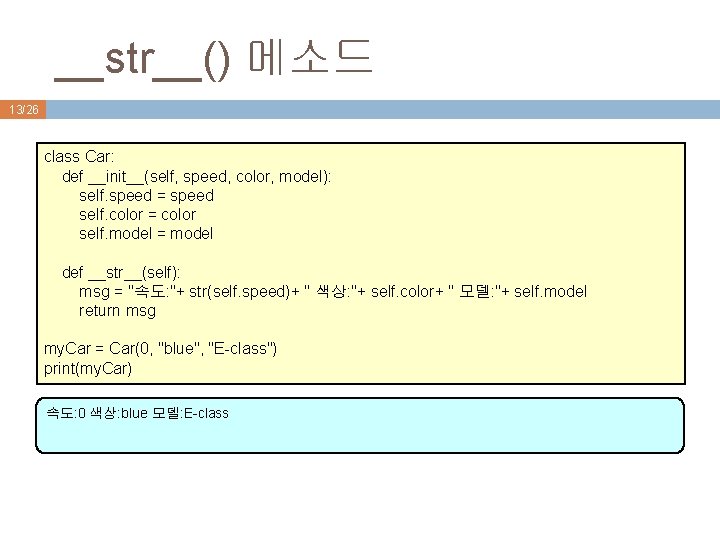
__str__() 메소드 13/26 class Car: def __init__(self, speed, color, model): self. speed = speed self. color = color self. model = model def __str__(self): msg = "속도: "+ str(self. speed)+ " 색상: "+ self. color+ " 모델: "+ self. model return msg my. Car = Car(0, "blue", "E-class") print(my. Car) 속도: 0 색상: blue 모델: E-class
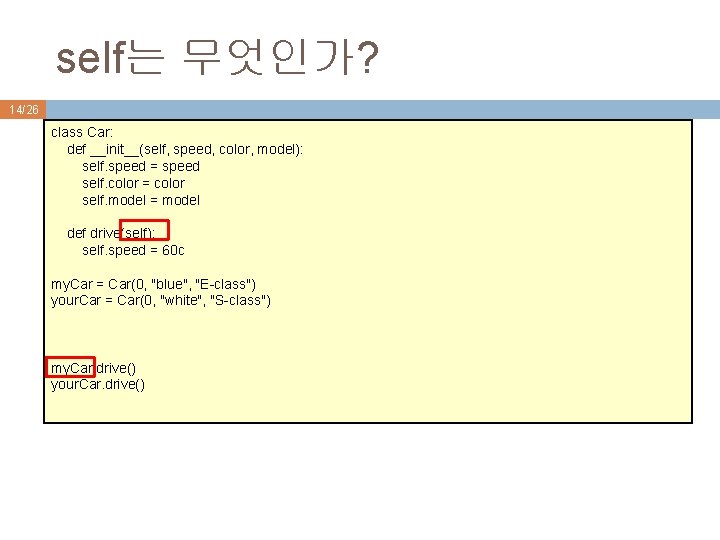
self는 무엇인가? 14/26 class Car: def __init__(self, speed, color, model): self. speed = speed self. color = color self. model = model def drive(self): self. speed = 60 c my. Car = Car(0, "blue", "E-class") your. Car = Car(0, "white", "S-class") my. Car. drive() your. Car. drive()
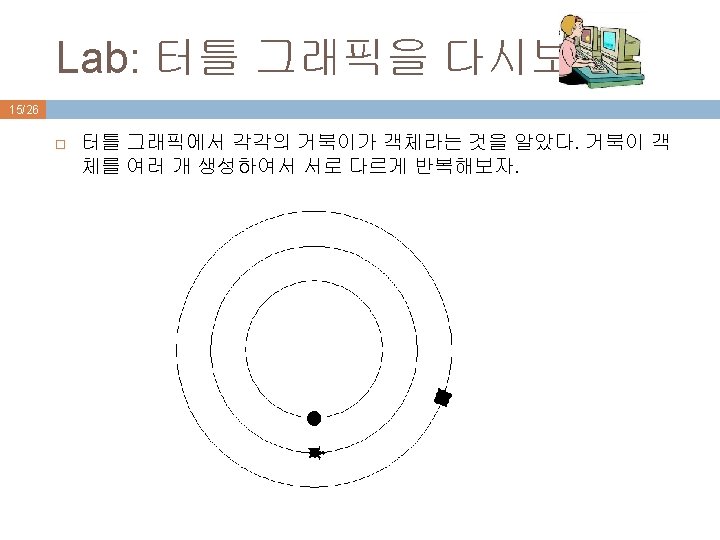
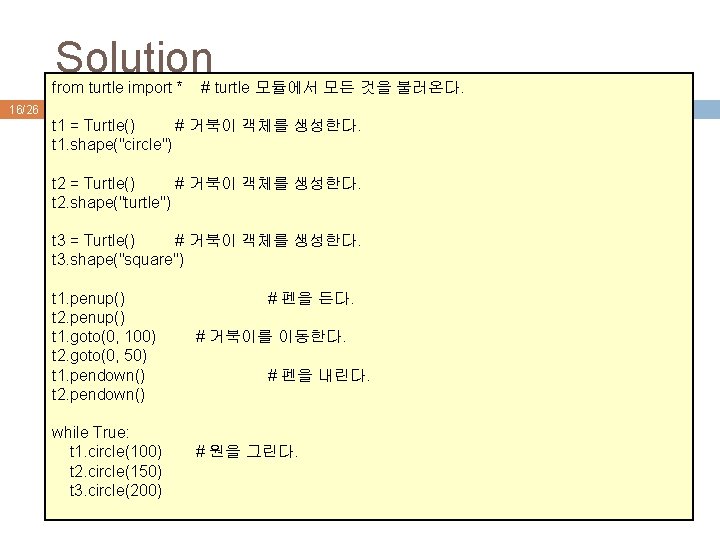
Solution from turtle import * 16/26 # turtle 모듈에서 모든 것을 불러온다. t 1 = Turtle() # 거북이 객체를 생성한다. t 1. shape("circle") t 2 = Turtle() # 거북이 객체를 생성한다. t 2. shape("turtle") t 3 = Turtle() # 거북이 객체를 생성한다. t 3. shape("square") t 1. penup() t 2. penup() t 1. goto(0, 100) t 2. goto(0, 50) t 1. pendown() t 2. pendown() while True: t 1. circle(100) t 2. circle(150) t 3. circle(200) # 펜을 든다. # 거북이를 이동한다. # 펜을 내린다. # 원을 그린다.
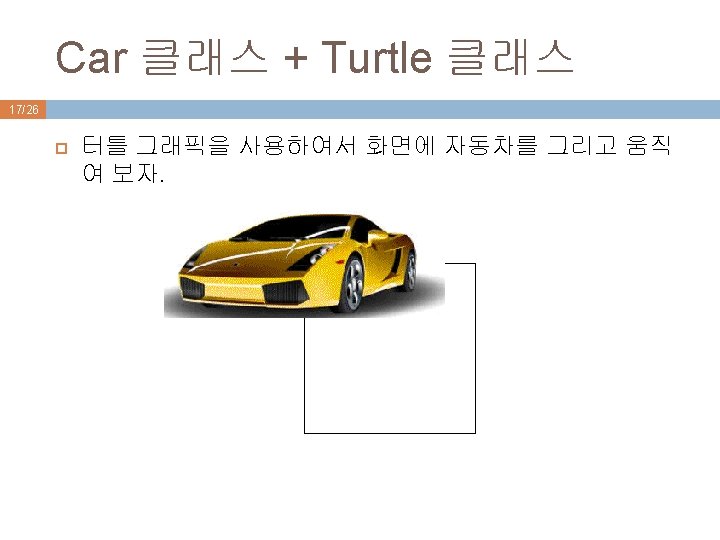
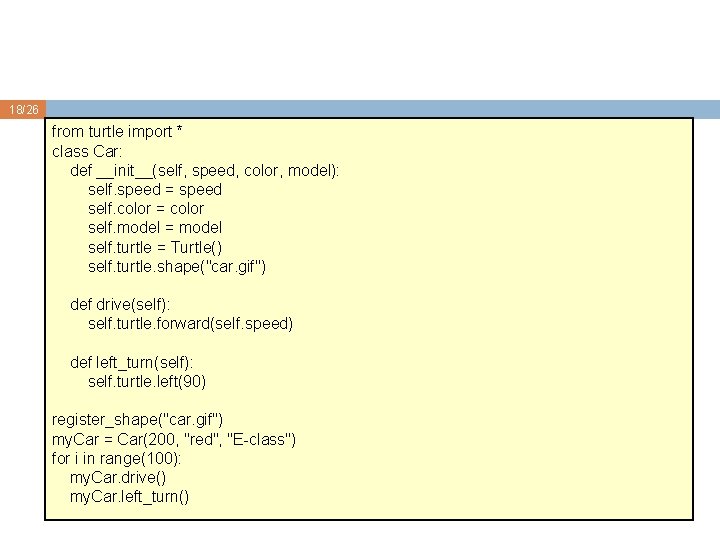
18/26 from turtle import * class Car: def __init__(self, speed, color, model): self. speed = speed self. color = color self. model = model self. turtle = Turtle() self. turtle. shape("car. gif") def drive(self): self. turtle. forward(self. speed) def left_turn(self): self. turtle. left(90) register_shape("car. gif") my. Car = Car(200, "red", "E-class") for i in range(100): my. Car. drive() my. Car. left_turn()
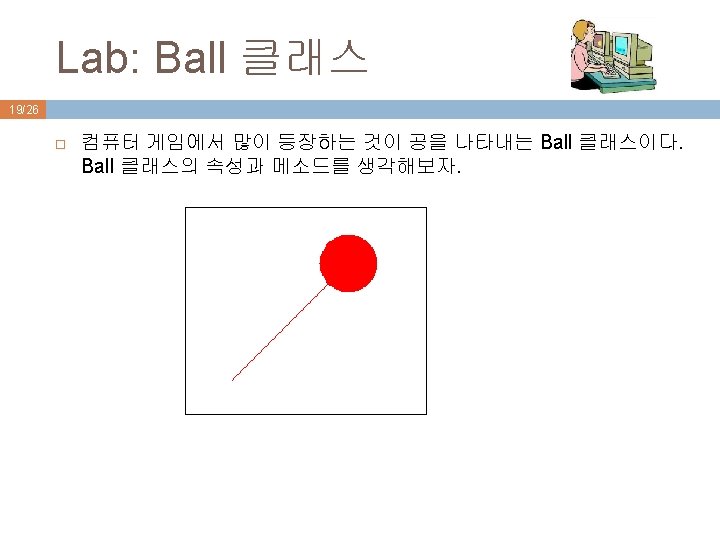
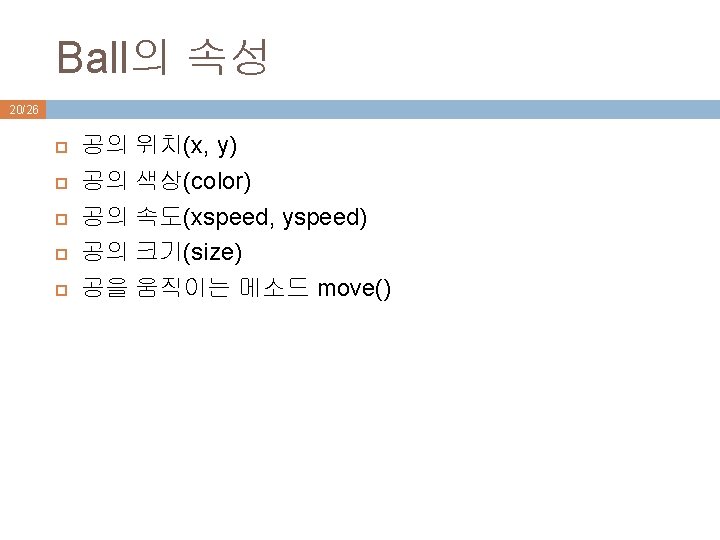
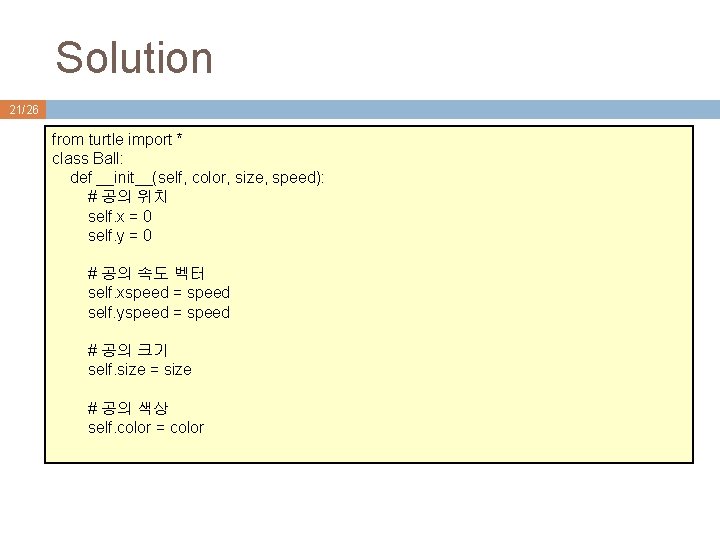
Solution 21/26 from turtle import * class Ball: def __init__(self, color, size, speed): # 공의 위치 self. x = 0 self. y = 0 # 공의 속도 벡터 self. xspeed = speed self. yspeed = speed # 공의 크기 self. size = size # 공의 색상 self. color = color
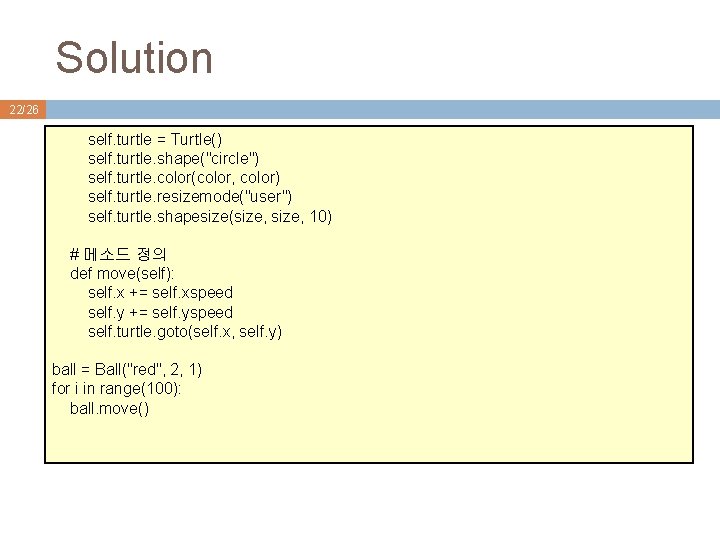
Solution 22/26 self. turtle = Turtle() self. turtle. shape("circle") self. turtle. color(color, color) self. turtle. resizemode("user") self. turtle. shapesize(size, 10) # 메소드 정의 def move(self): self. x += self. xspeed self. y += self. yspeed self. turtle. goto(self. x, self. y) ball = Ball("red", 2, 1) for i in range(100): ball. move()
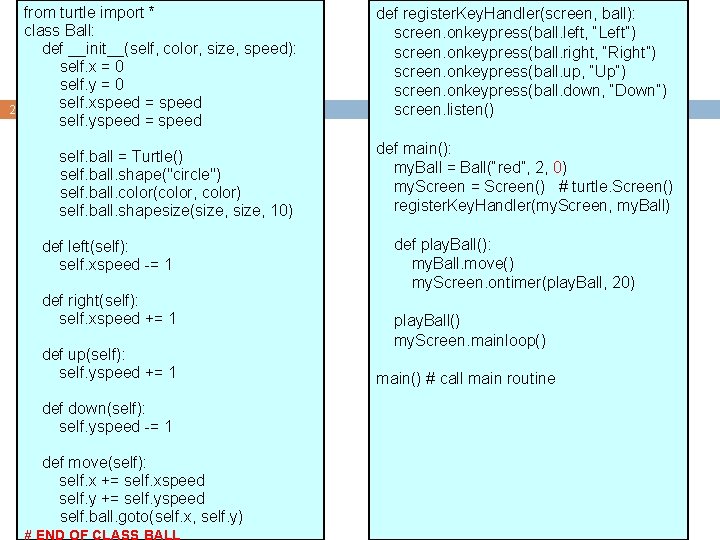
from turtle import * class Ball: def __init__(self, color, size, speed): self. x = 0 self. y = 0 self. xspeed = speed 23/26 self. yspeed = speed self. ball = Turtle() self. ball. shape("circle") self. ball. color(color, color) self. ball. shapesize(size, 10) def left(self): self. xspeed -= 1 def right(self): self. xspeed += 1 def up(self): self. yspeed += 1 def down(self): self. yspeed -= 1 def move(self): self. x += self. xspeed self. y += self. yspeed self. ball. goto(self. x, self. y) def register. Key. Handler(screen, ball): screen. onkeypress(ball. left, “Left”) screen. onkeypress(ball. right, “Right”) screen. onkeypress(ball. up, “Up”) screen. onkeypress(ball. down, “Down”) screen. listen() def main(): my. Ball = Ball(“red”, 2, 0) my. Screen = Screen() # turtle. Screen() register. Key. Handler(my. Screen, my. Ball) def play. Ball(): my. Ball. move() my. Screen. ontimer(play. Ball, 20) play. Ball() my. Screen. mainloop() main() # call main routine
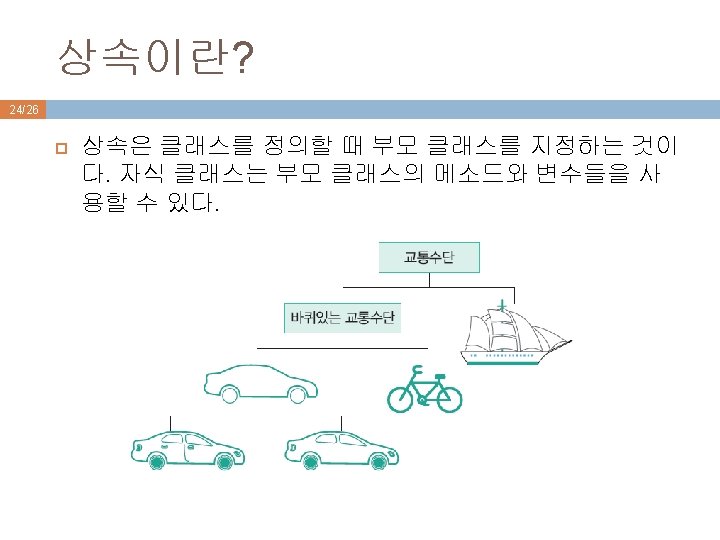
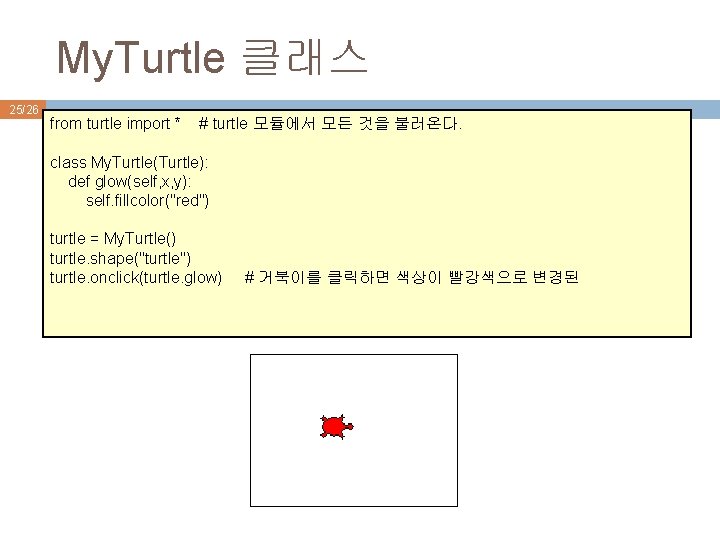
My. Turtle 클래스 25/26 from turtle import * # turtle 모듈에서 모든 것을 불러온다. class My. Turtle(Turtle): def glow(self, x, y): self. fillcolor("red") turtle = My. Turtle() turtle. shape("turtle") turtle. onclick(turtle. glow) # 거북이를 클릭하면 색상이 빨강색으로 변경된
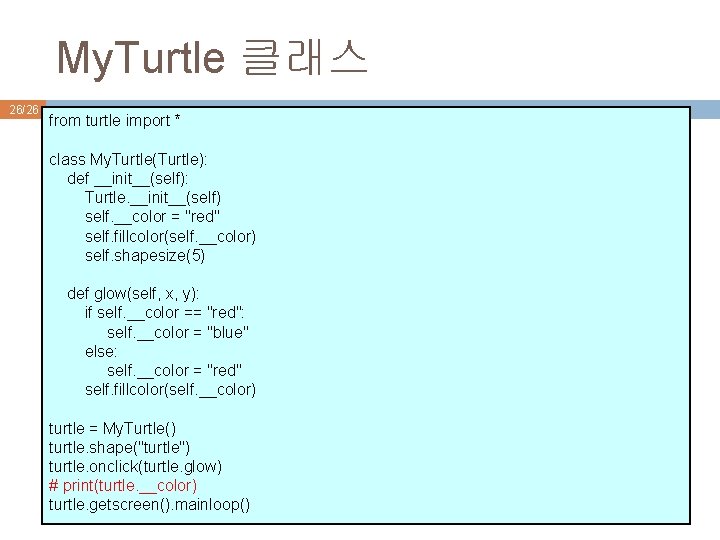
My. Turtle 클래스 26/26 from turtle import * class My. Turtle(Turtle): def __init__(self): Turtle. __init__(self) self. __color = "red" self. fillcolor(self. __color) self. shapesize(5) def glow(self, x, y): if self. __color == "red": self. __color = "blue" else: self. __color = "red" self. fillcolor(self. __color) turtle = My. Turtle() turtle. shape("turtle") turtle. onclick(turtle. glow) # print(turtle. __color) turtle. getscreen(). mainloop()
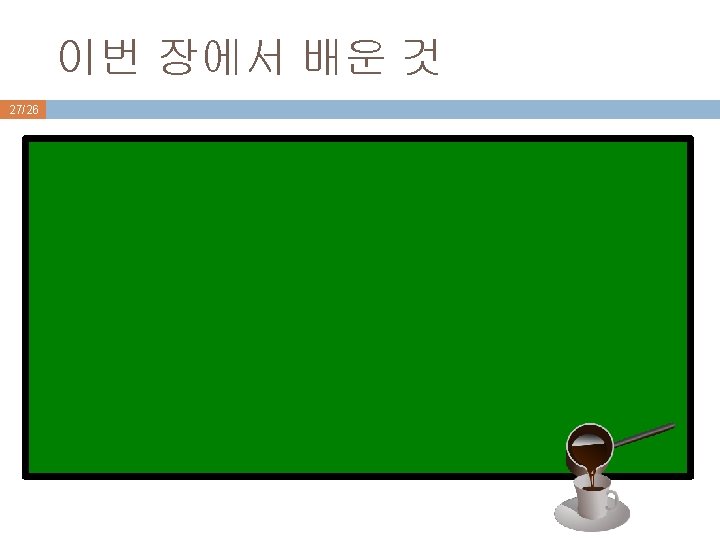
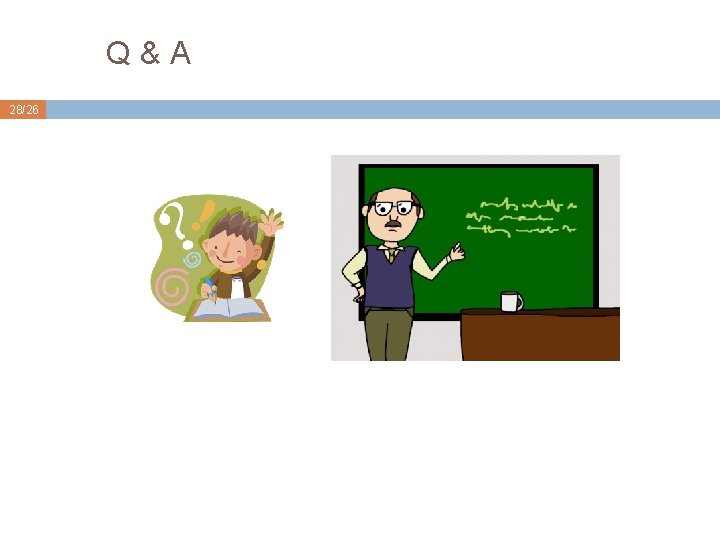
Q&A 28/26
If car a passes car b, then car a must be ____.
Rfc 826
Round 763 to the nearest hundred
Real-self meaning
Car 2 car consortium
Ch7on
Time distance/speed formula
What is fringe vision
Speed detection of moving vehicle
Self fulfilling prophecy betekenis
Google self driving car ppt
Car dealership class diagram
Public class vehicle private string name protected vehicle
Decision tree example of car type, shirt size and class
Public class car
Schedule of dimensions
Example of inheritance in java
Velocity
Difference between speed and velocity
What is the difference between speed and velocity
Self concept vs self esteem
Self concept vs self esteem
Different self concept
Self concept vs self esteem
It is the misalignment of the ideal and real self.
I self and me self difference
Contoh ideal self dan real self
Procedural self-knowledge includes ________.
Eyfs self confidence and self awareness