09 Iterative Execution Mark Dixon Page 1 Questions
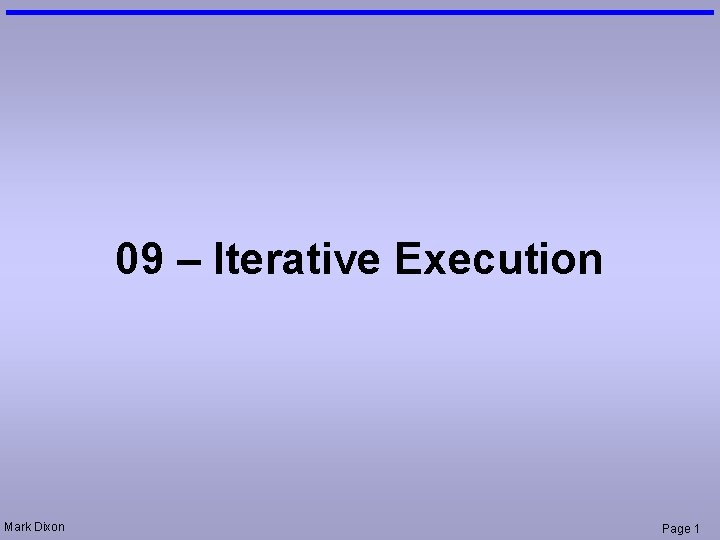
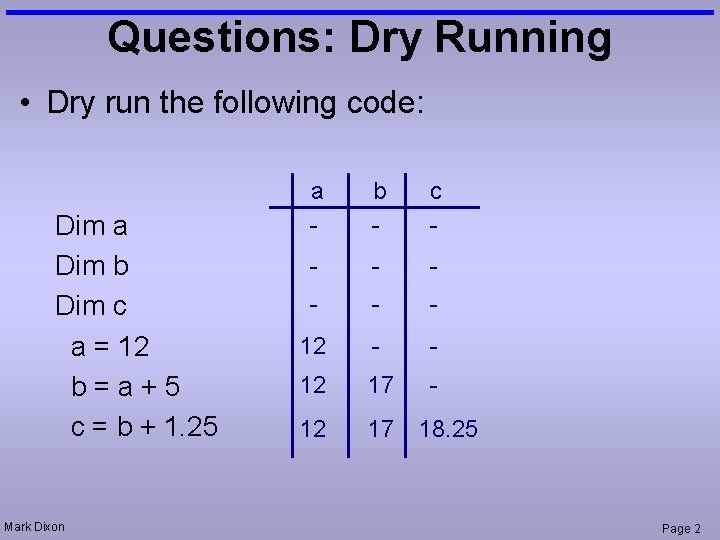
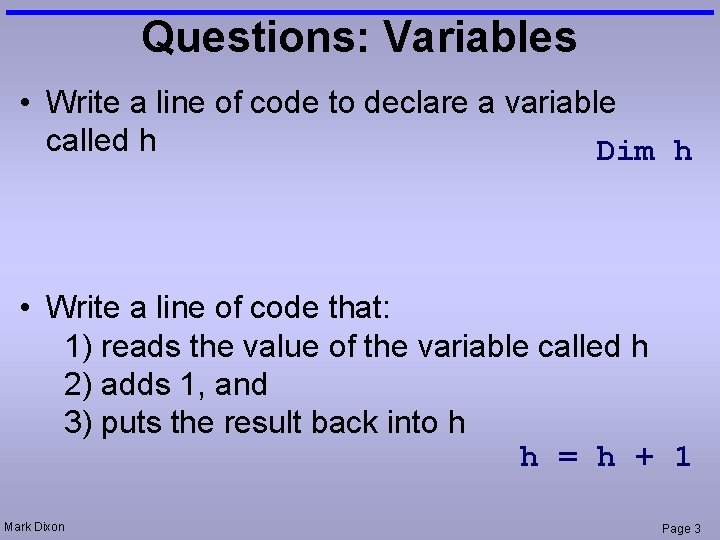
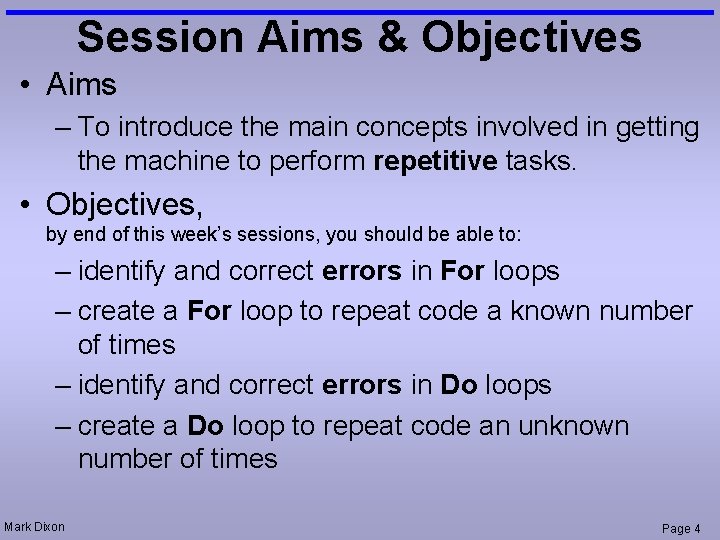
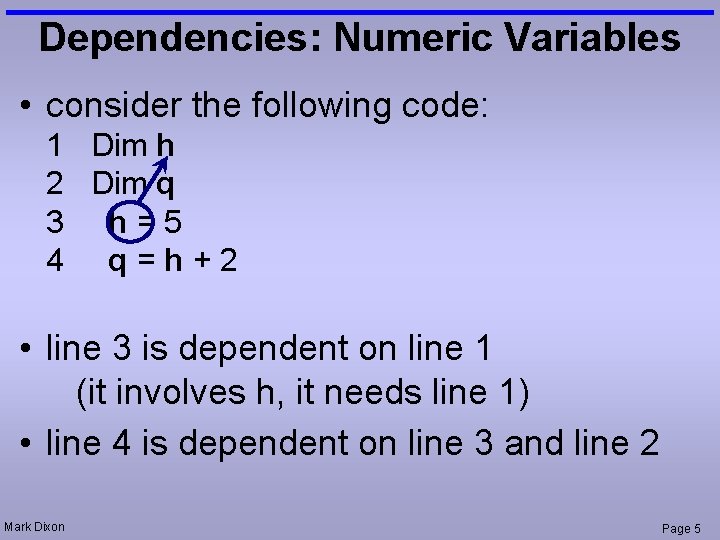
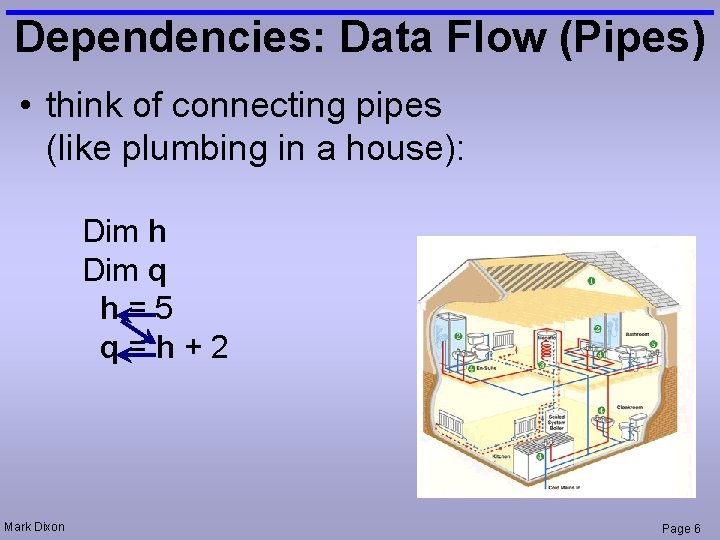
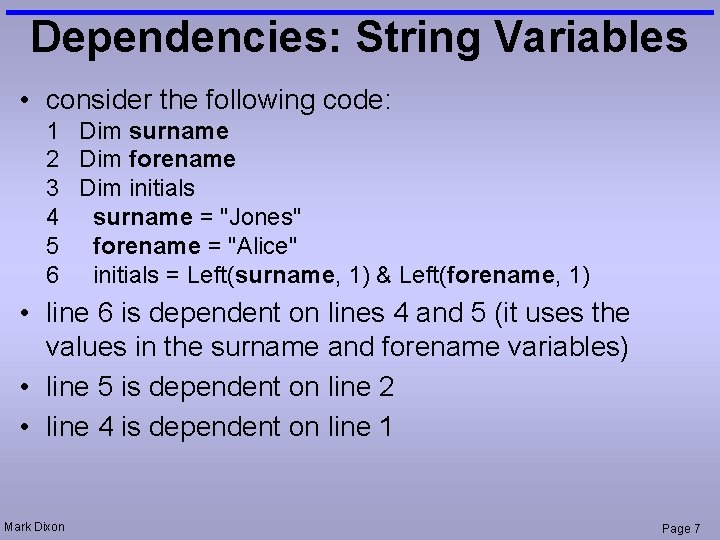
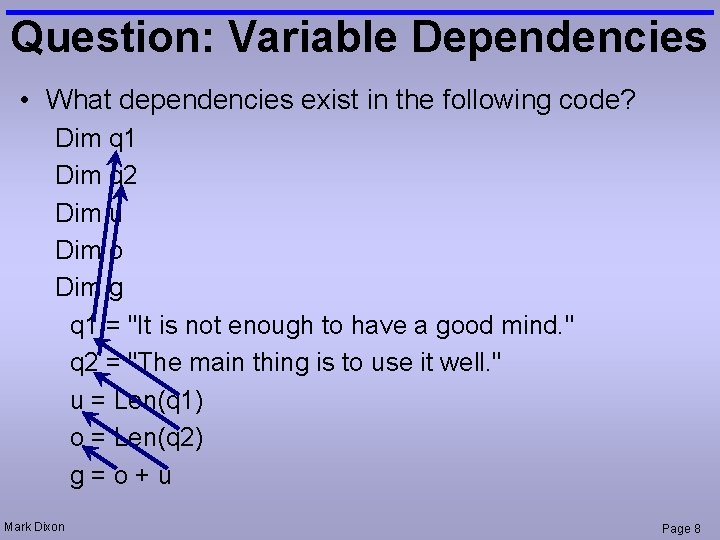
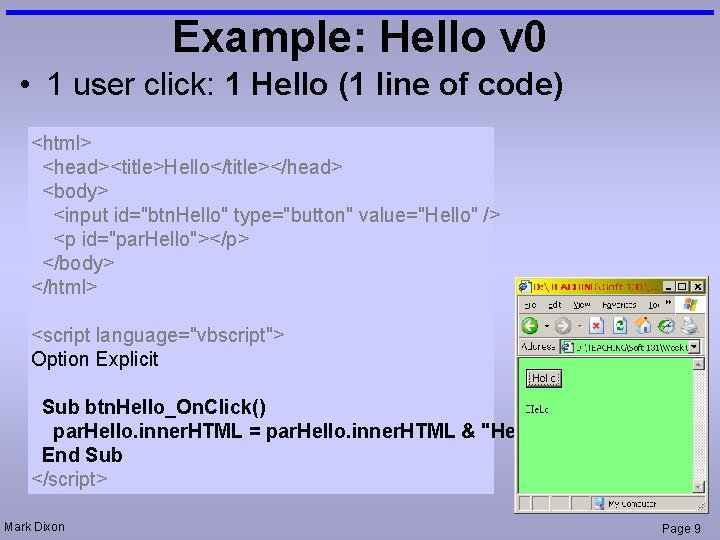
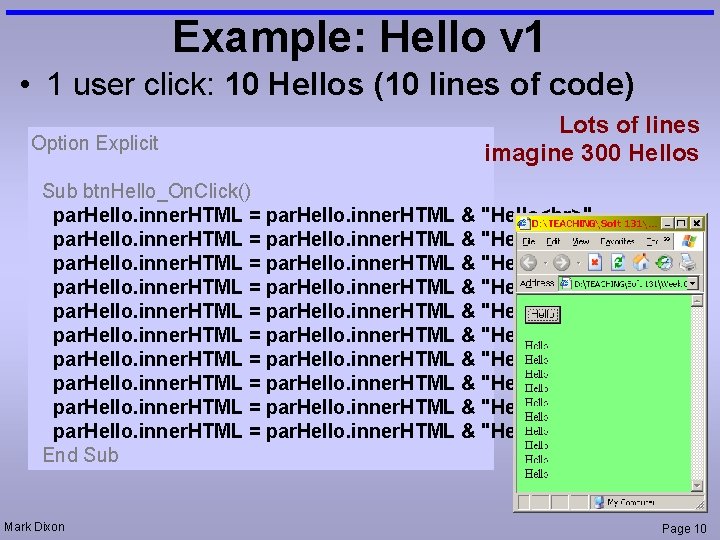
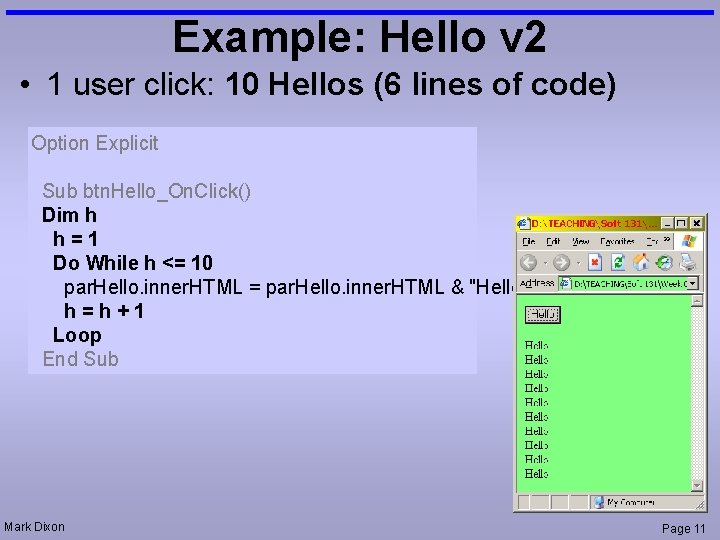
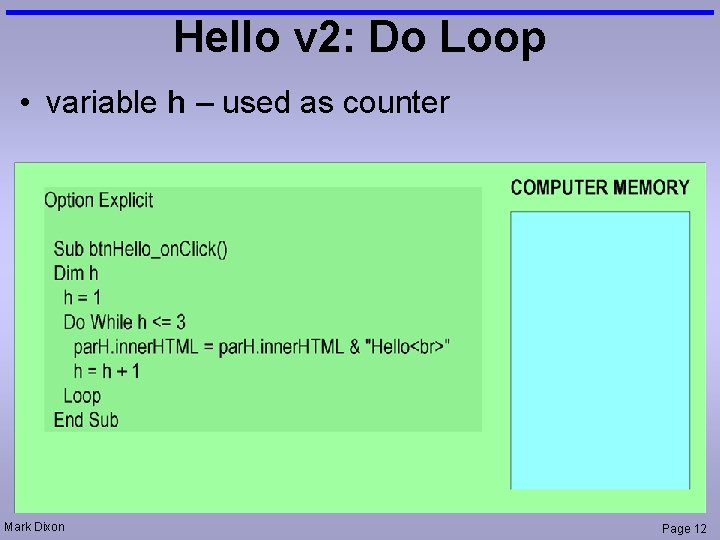
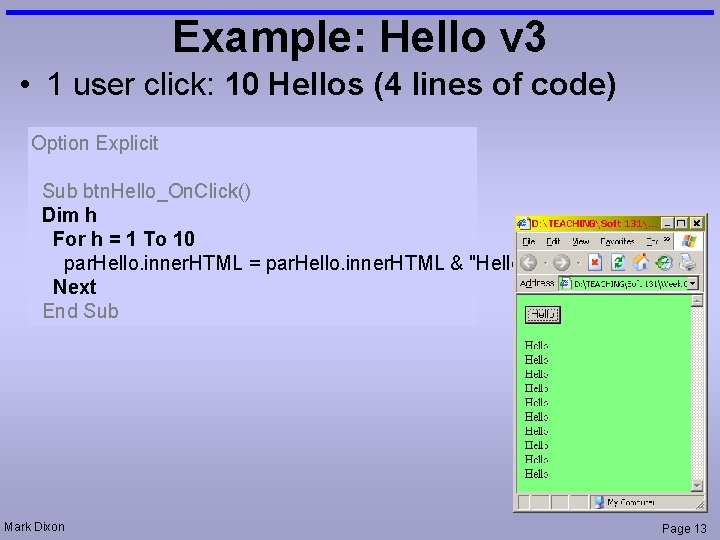
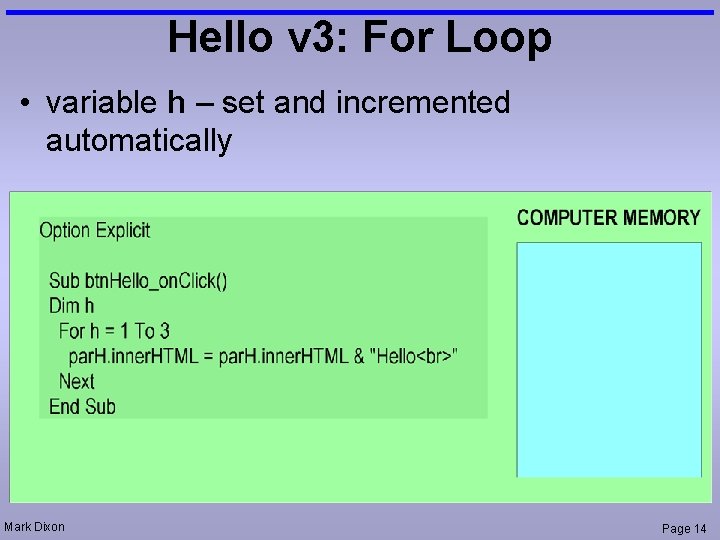
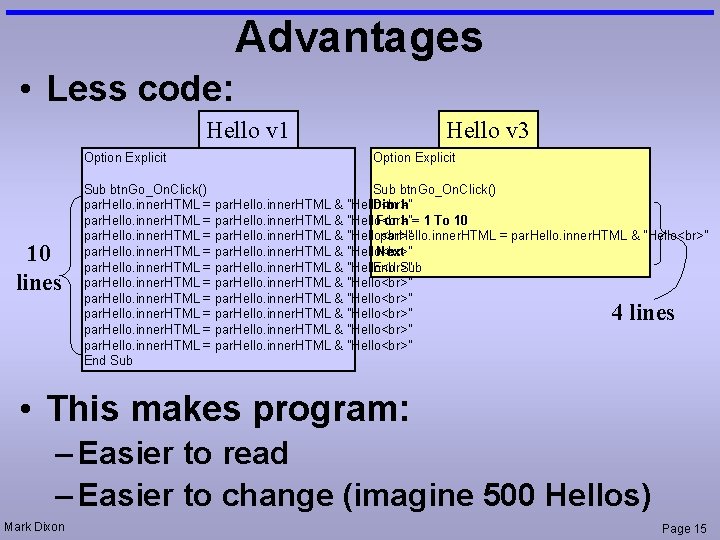
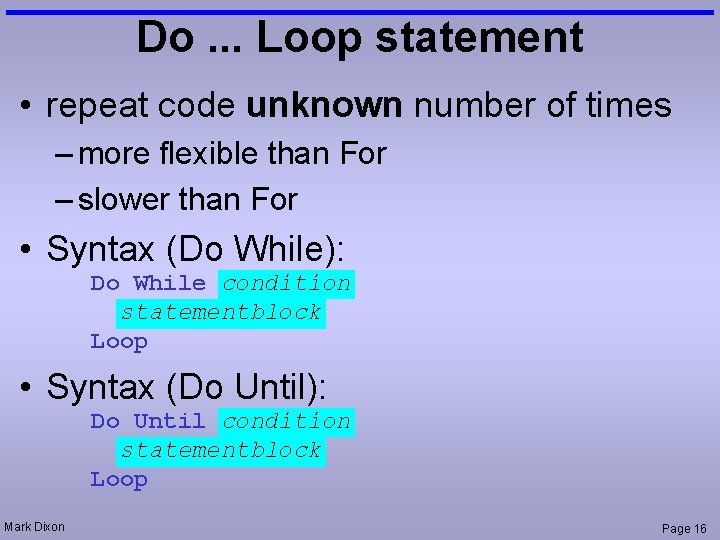
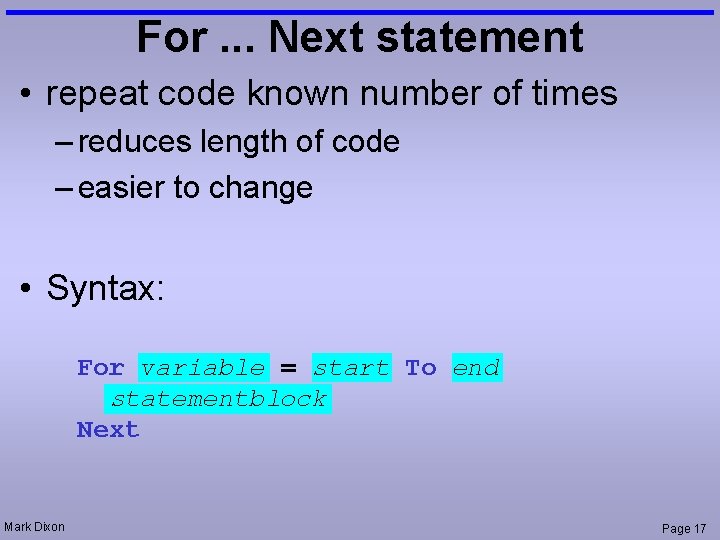
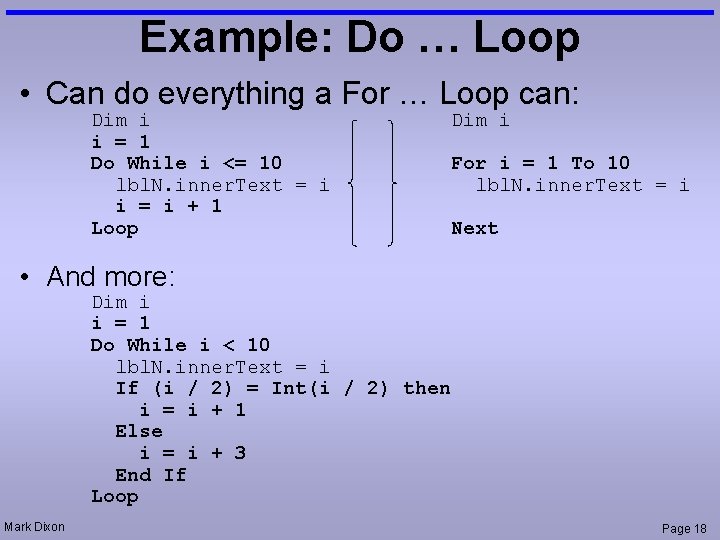
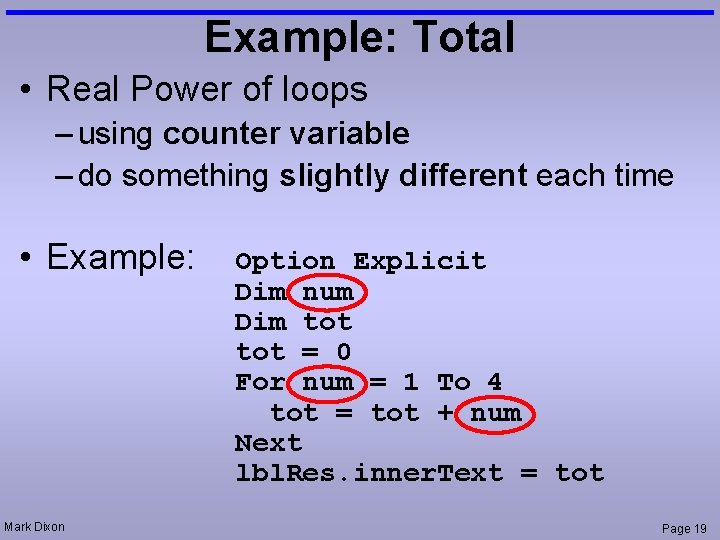
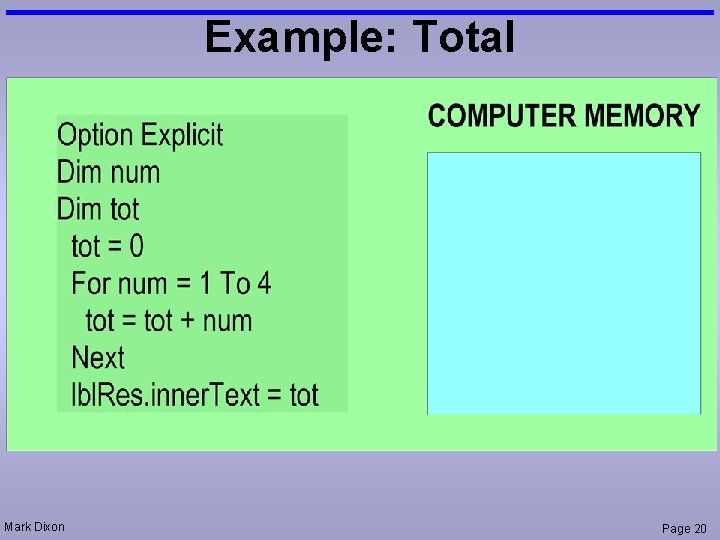
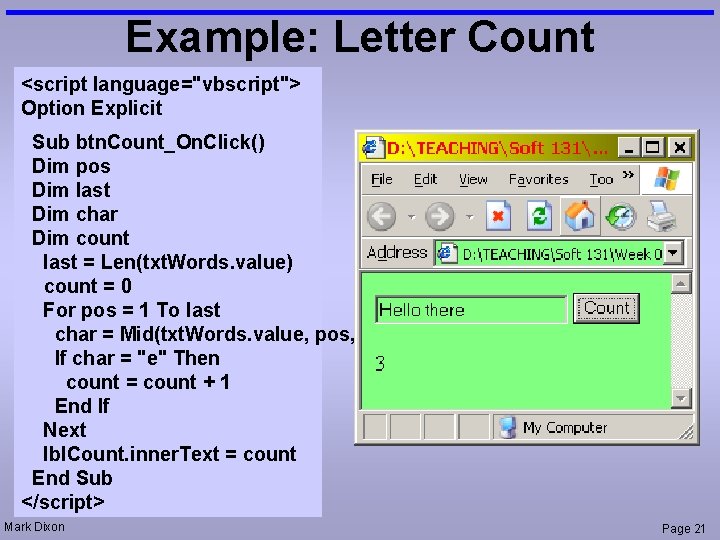
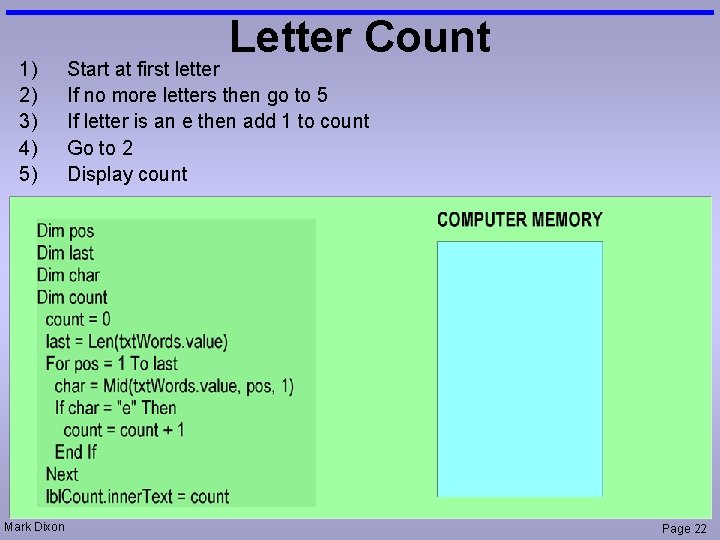
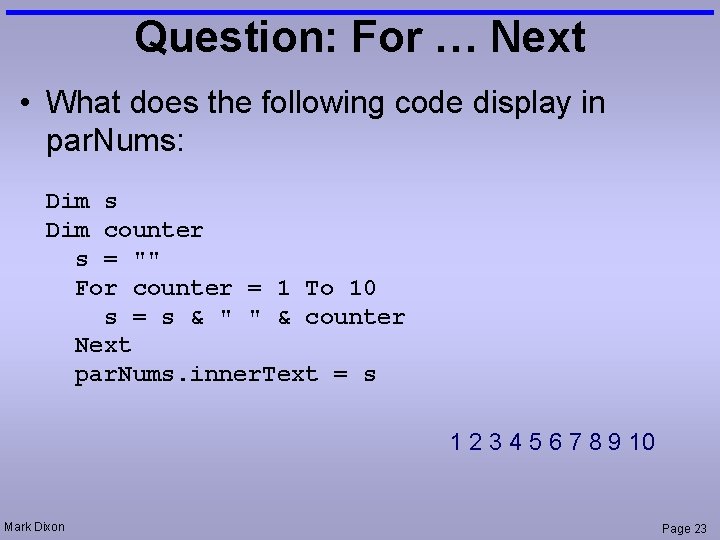
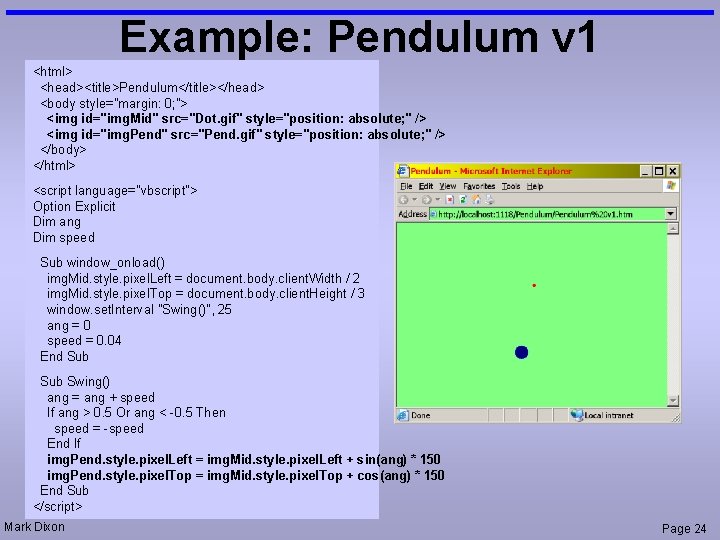
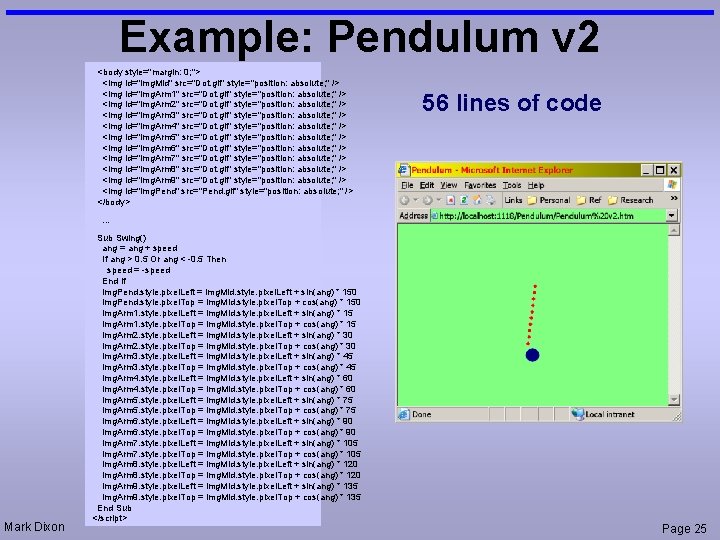
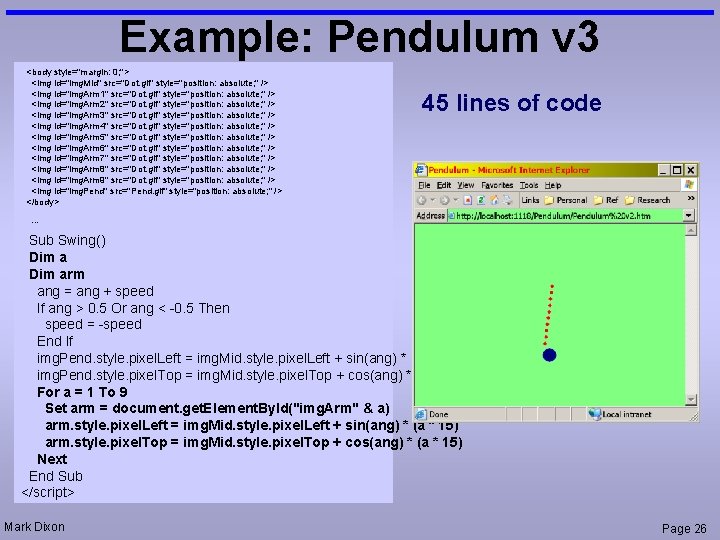
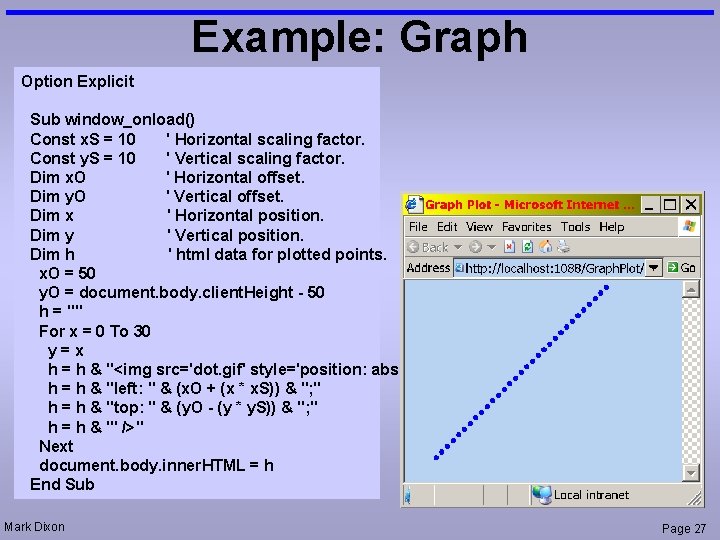
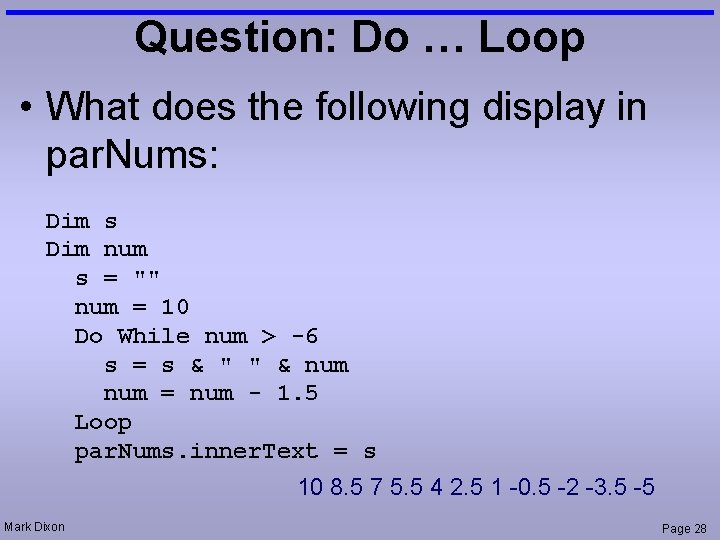
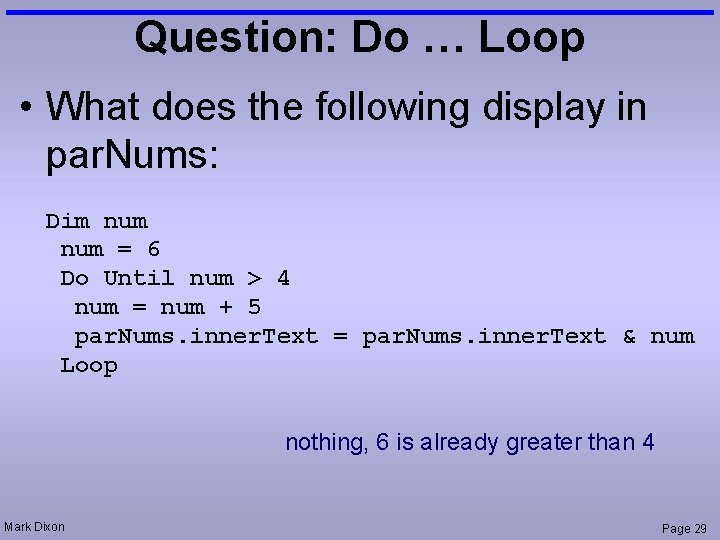
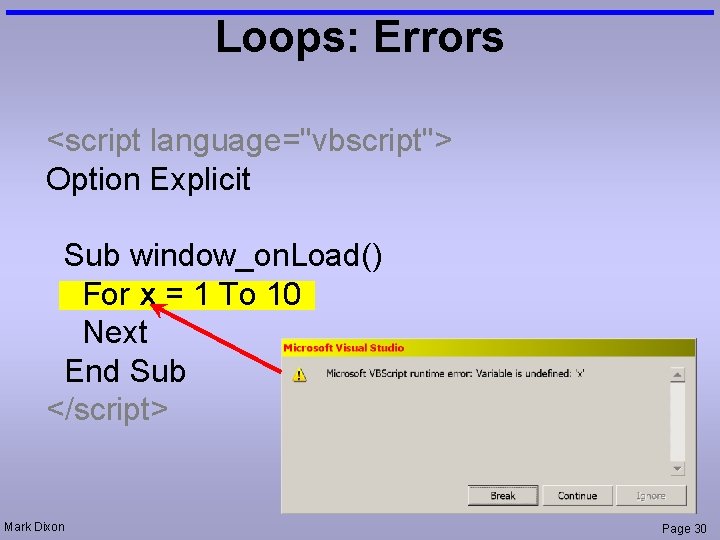
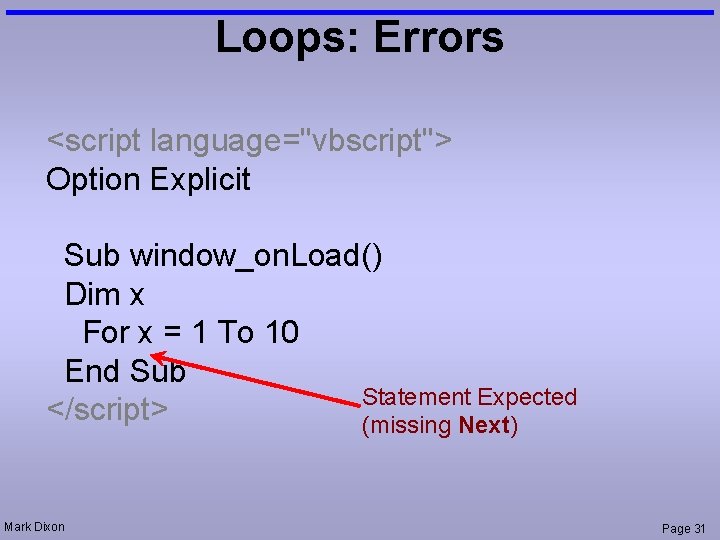
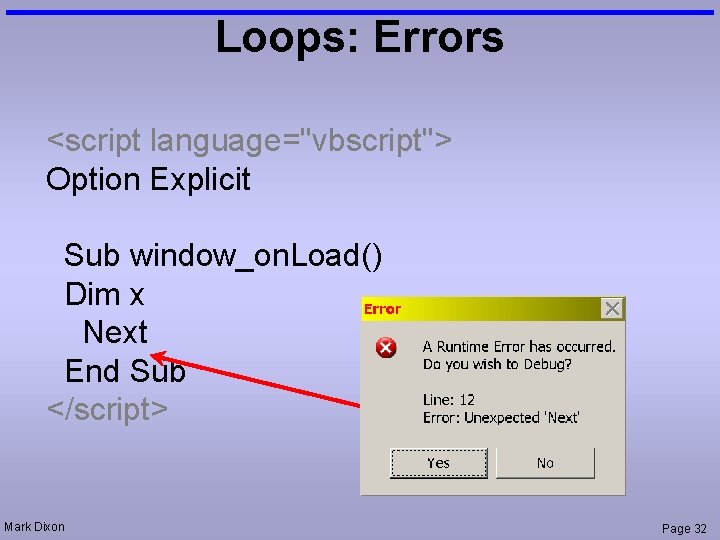
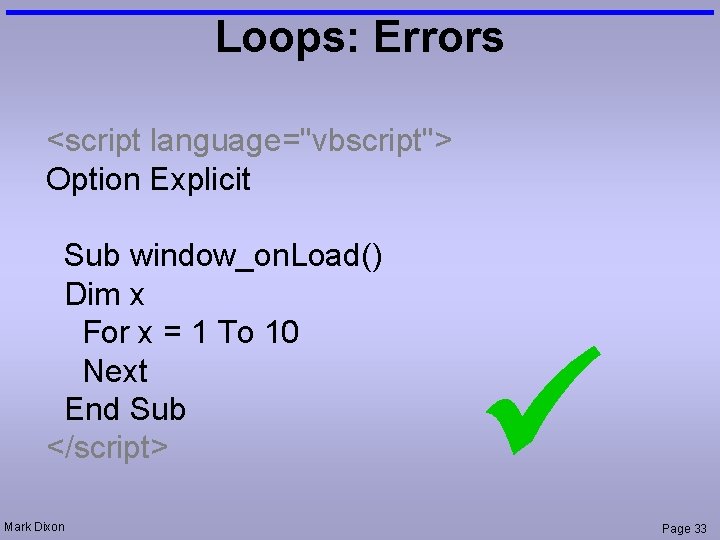
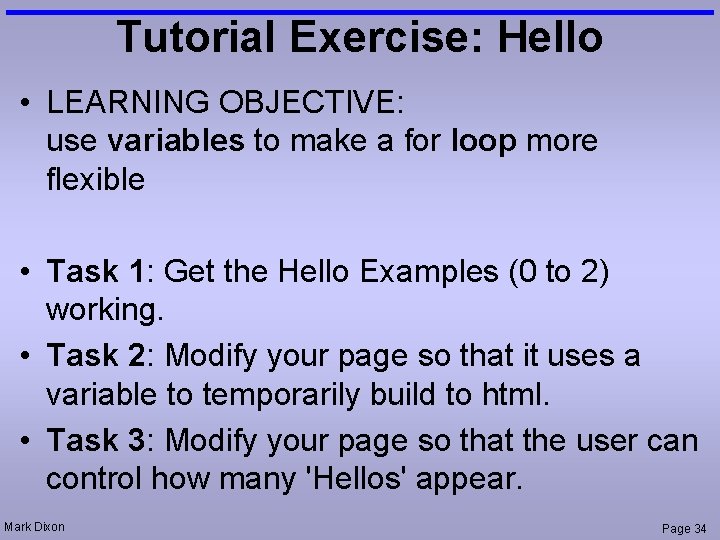
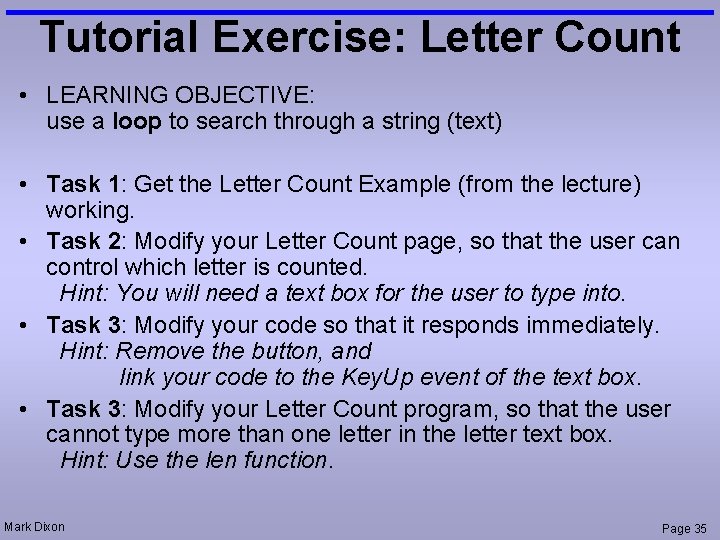
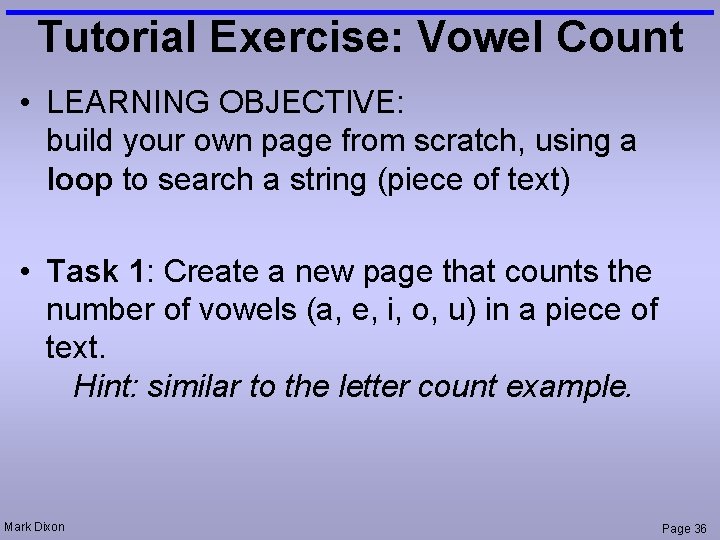
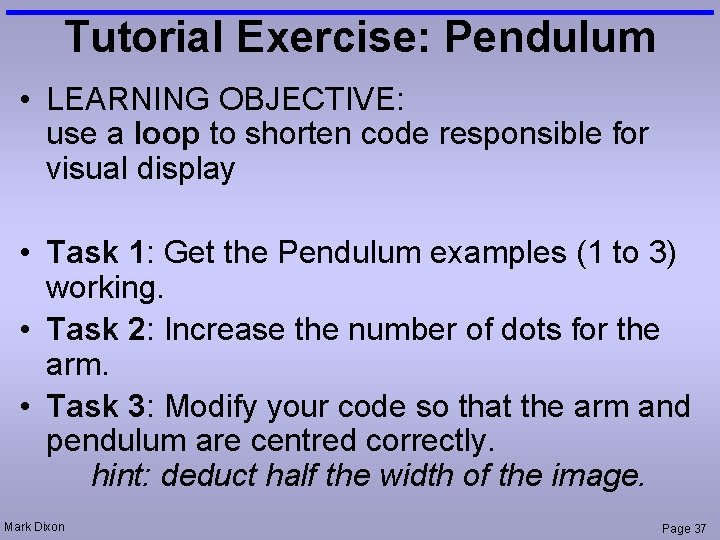
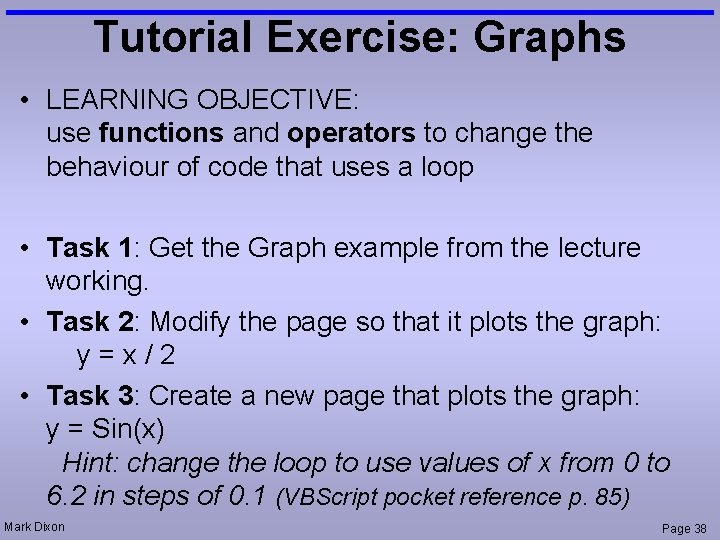
- Slides: 38
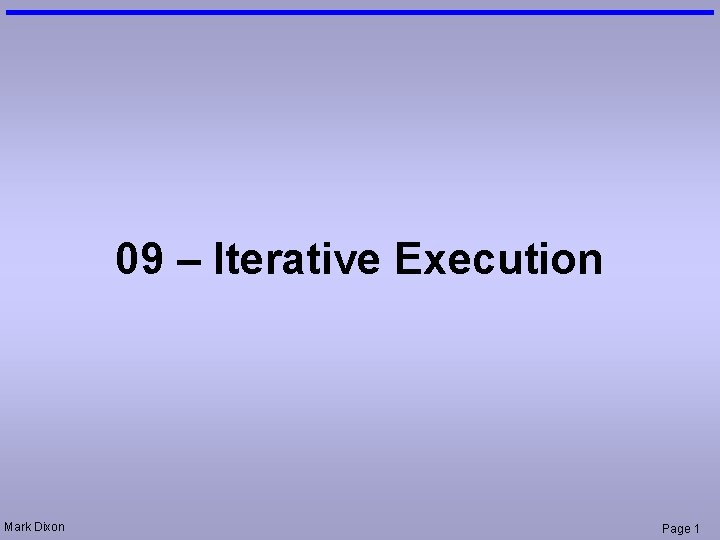
09 – Iterative Execution Mark Dixon Page 1
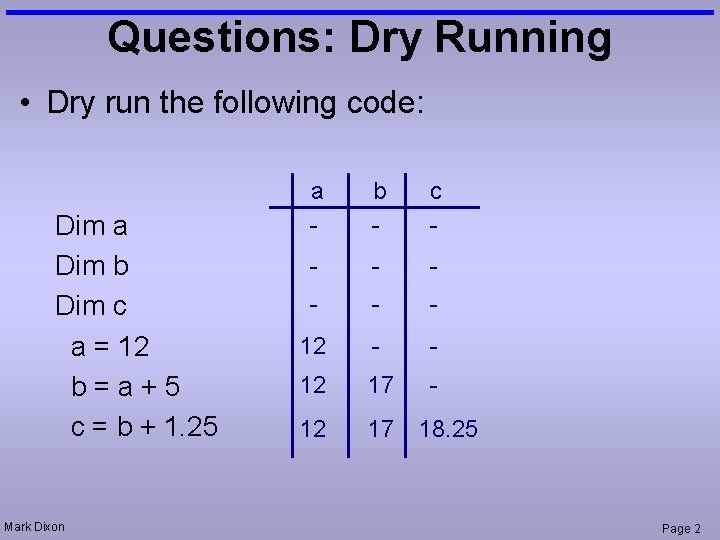
Questions: Dry Running • Dry run the following code: Dim a Dim b Dim c a = 12 b=a+5 c = b + 1. 25 Mark Dixon a - b - c - - - - 12 17 18. 25 Page 2
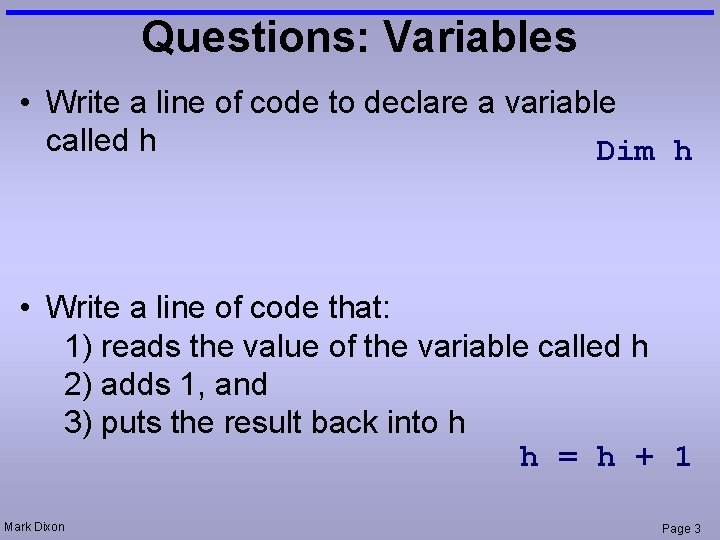
Questions: Variables • Write a line of code to declare a variable called h Dim h • Write a line of code that: 1) reads the value of the variable called h 2) adds 1, and 3) puts the result back into h h = h + 1 Mark Dixon Page 3
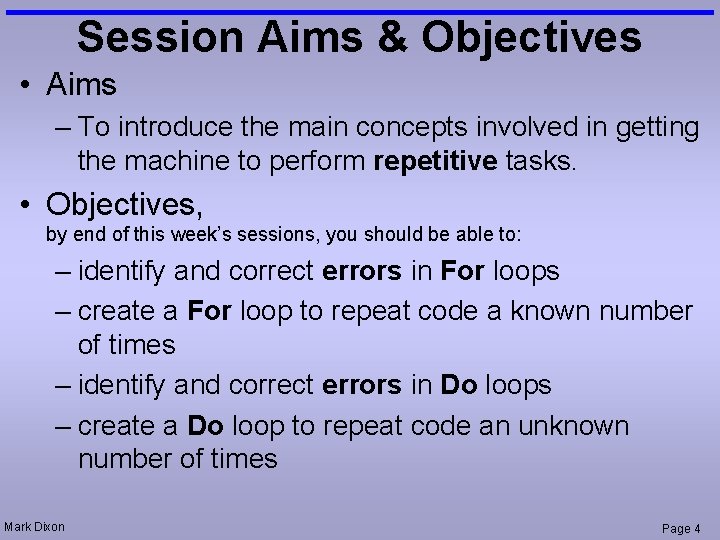
Session Aims & Objectives • Aims – To introduce the main concepts involved in getting the machine to perform repetitive tasks. • Objectives, by end of this week’s sessions, you should be able to: – identify and correct errors in For loops – create a For loop to repeat code a known number of times – identify and correct errors in Do loops – create a Do loop to repeat code an unknown number of times Mark Dixon Page 4
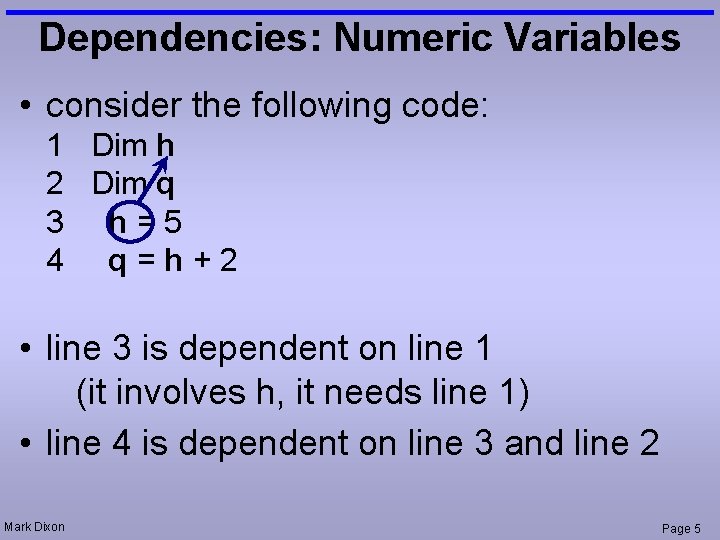
Dependencies: Numeric Variables • consider the following code: 1 Dim h 2 Dim q 3 h=5 4 q=h+2 • line 3 is dependent on line 1 (it involves h, it needs line 1) • line 4 is dependent on line 3 and line 2 Mark Dixon Page 5
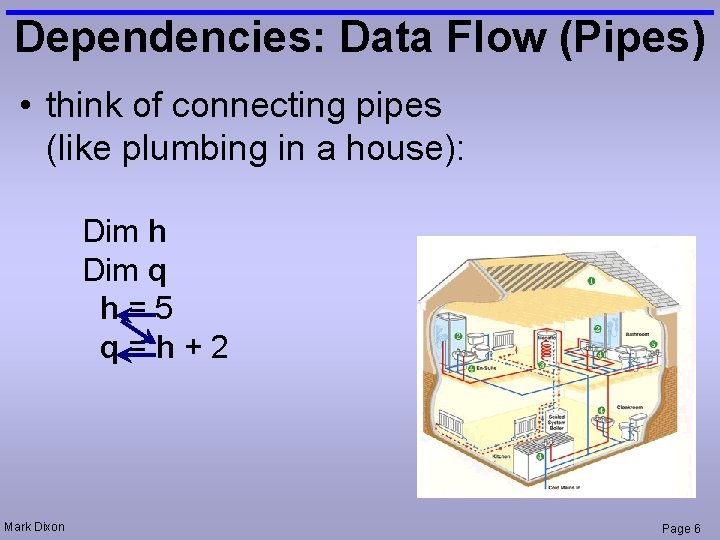
Dependencies: Data Flow (Pipes) • think of connecting pipes (like plumbing in a house): Dim h Dim q h=5 q=h+2 Mark Dixon Page 6
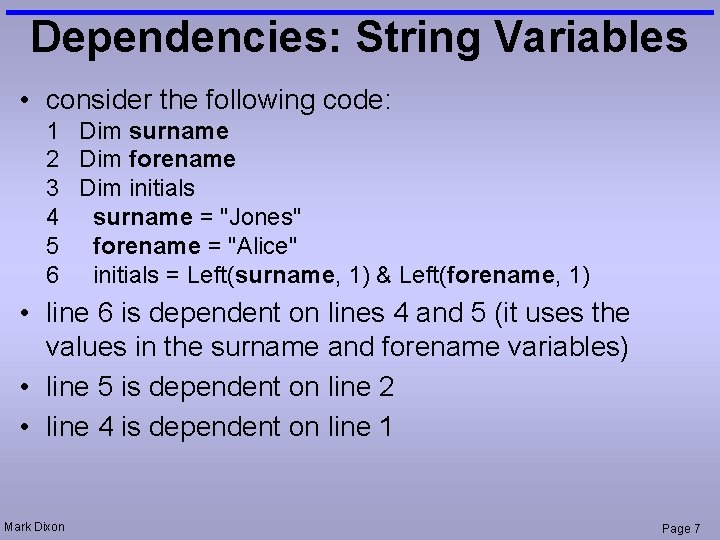
Dependencies: String Variables • consider the following code: 1 Dim surname 2 Dim forename 3 Dim initials 4 surname = "Jones" 5 forename = "Alice" 6 initials = Left(surname, 1) & Left(forename, 1) • line 6 is dependent on lines 4 and 5 (it uses the values in the surname and forename variables) • line 5 is dependent on line 2 • line 4 is dependent on line 1 Mark Dixon Page 7
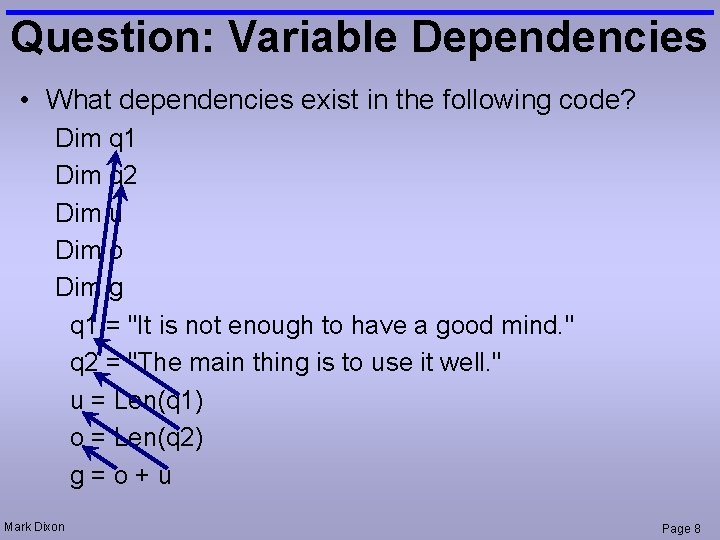
Question: Variable Dependencies • What dependencies exist in the following code? Dim q 1 Dim q 2 Dim u Dim o Dim g q 1 = "It is not enough to have a good mind. " q 2 = "The main thing is to use it well. " u = Len(q 1) o = Len(q 2) g=o+u Mark Dixon Page 8
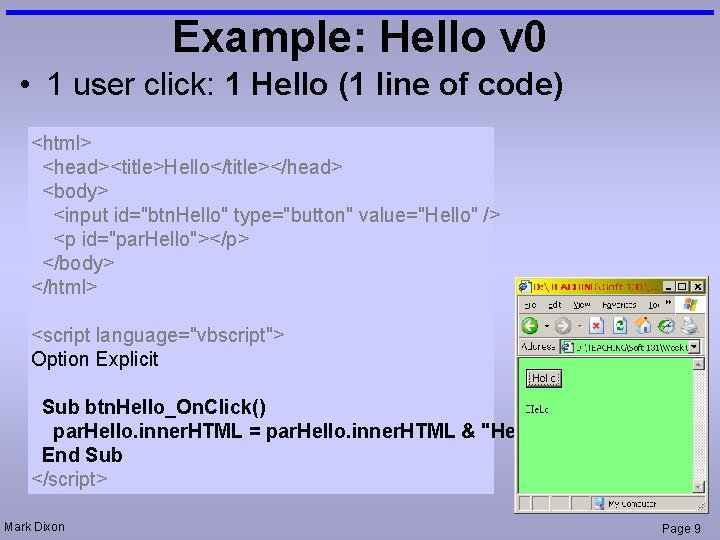
Example: Hello v 0 • 1 user click: 1 Hello (1 line of code) <html> <head><title>Hello</title></head> <body> <input id="btn. Hello" type="button" value="Hello" /> <p id="par. Hello"></p> </body> </html> <script language="vbscript"> Option Explicit Sub btn. Hello_On. Click() par. Hello. inner. HTML = par. Hello. inner. HTML & "Hello " End Sub </script> Mark Dixon Page 9
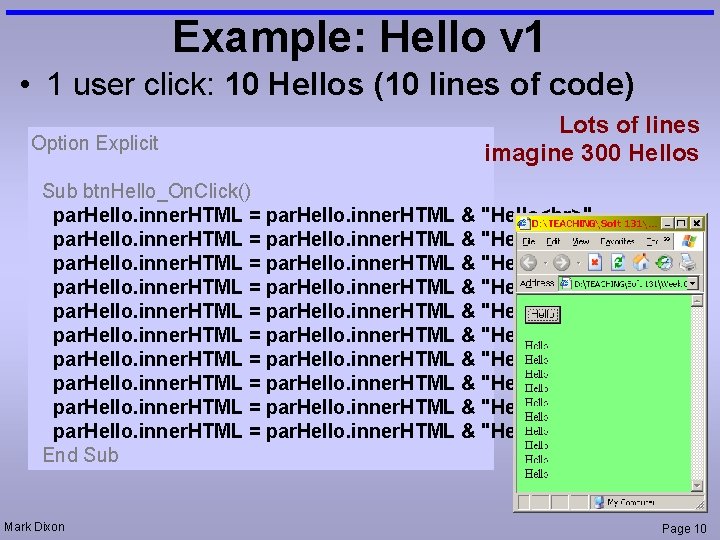
Example: Hello v 1 • 1 user click: 10 Hellos (10 lines of code) Option Explicit Lots of lines imagine 300 Hellos Sub btn. Hello_On. Click() par. Hello. inner. HTML = par. Hello. inner. HTML & "Hello " par. Hello. inner. HTML = par. Hello. inner. HTML & "Hello " End Sub Mark Dixon Page 10
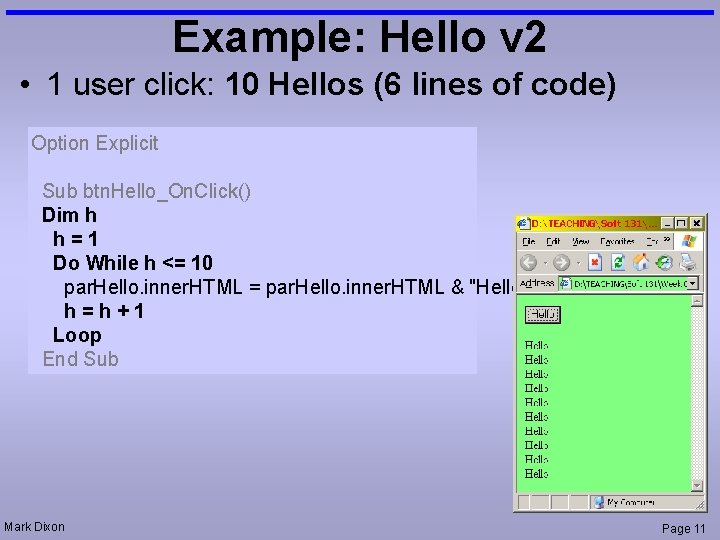
Example: Hello v 2 • 1 user click: 10 Hellos (6 lines of code) Option Explicit Sub btn. Hello_On. Click() Dim h h=1 Do While h <= 10 par. Hello. inner. HTML = par. Hello. inner. HTML & "Hello " h=h+1 Loop End Sub Mark Dixon Page 11
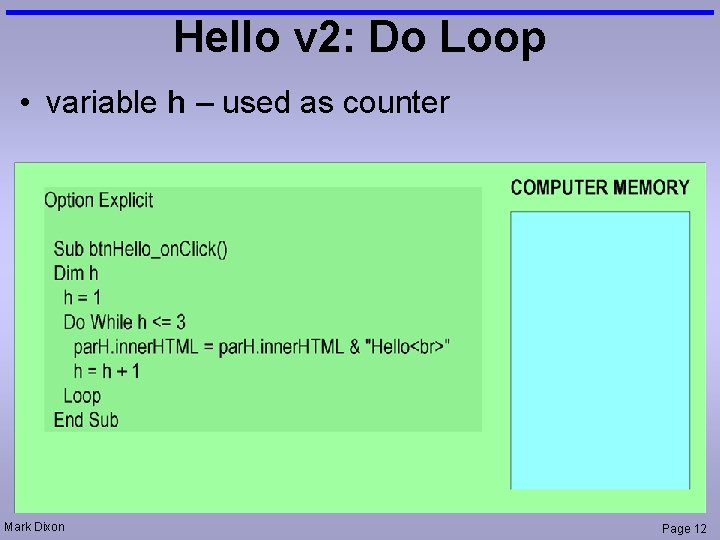
Hello v 2: Do Loop • variable h – used as counter Mark Dixon Page 12
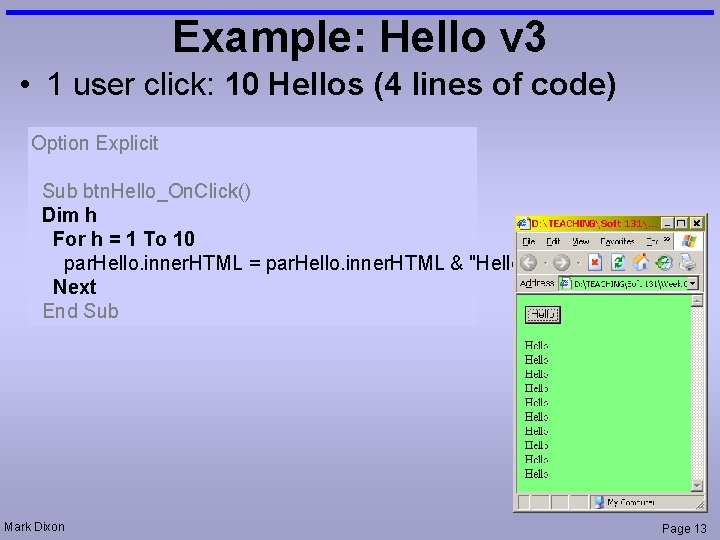
Example: Hello v 3 • 1 user click: 10 Hellos (4 lines of code) Option Explicit Sub btn. Hello_On. Click() Dim h For h = 1 To 10 par. Hello. inner. HTML = par. Hello. inner. HTML & "Hello " Next End Sub Mark Dixon Page 13
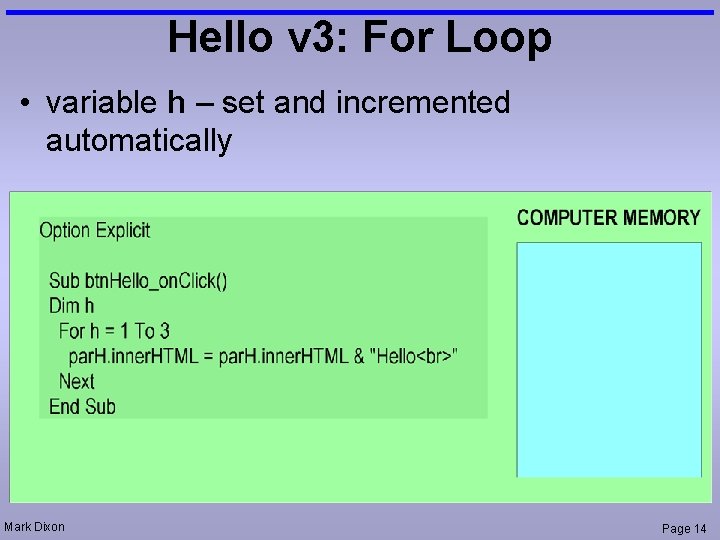
Hello v 3: For Loop • variable h – set and incremented automatically Mark Dixon Page 14
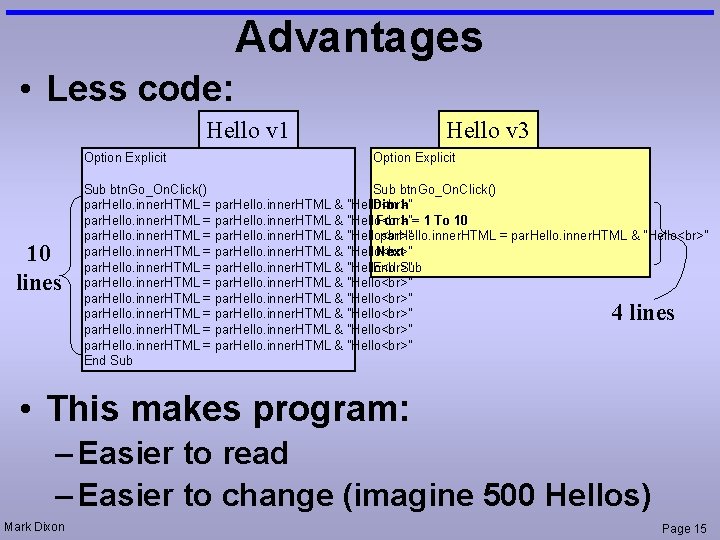
Advantages • Less code: Hello v 1 Option Explicit 10 lines Hello v 3 Option Explicit Sub btn. Go_On. Click() par. Hello. inner. HTML = par. Hello. inner. HTML & "Hello " Dim h par. Hello. inner. HTML = par. Hello. inner. HTML & "Hello " For h = 1 To 10 par. Hello. inner. HTML = par. Hello. inner. HTML & "Hello " Next par. Hello. inner. HTML = par. Hello. inner. HTML & "Hello " End Sub par. Hello. inner. HTML = par. Hello. inner. HTML & "Hello " End Sub 4 lines • This makes program: – Easier to read – Easier to change (imagine 500 Hellos) Mark Dixon Page 15
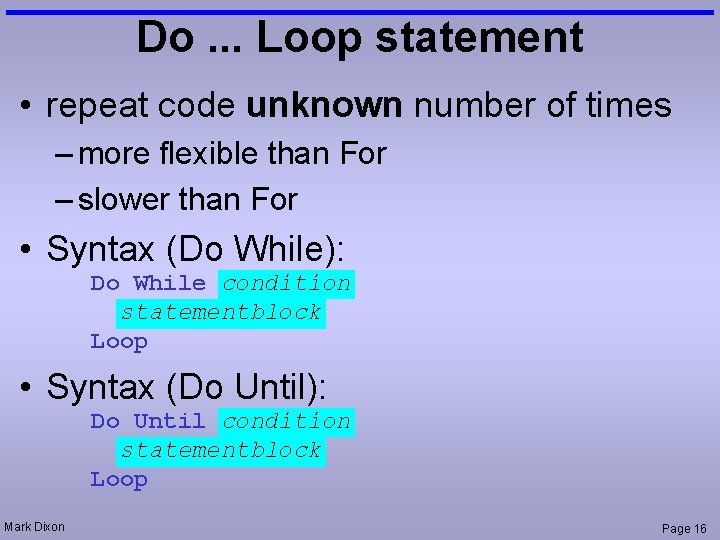
Do. . . Loop statement • repeat code unknown number of times – more flexible than For – slower than For • Syntax (Do While): Do While condition statementblock Loop • Syntax (Do Until): Do Until condition statementblock Loop Mark Dixon Page 16
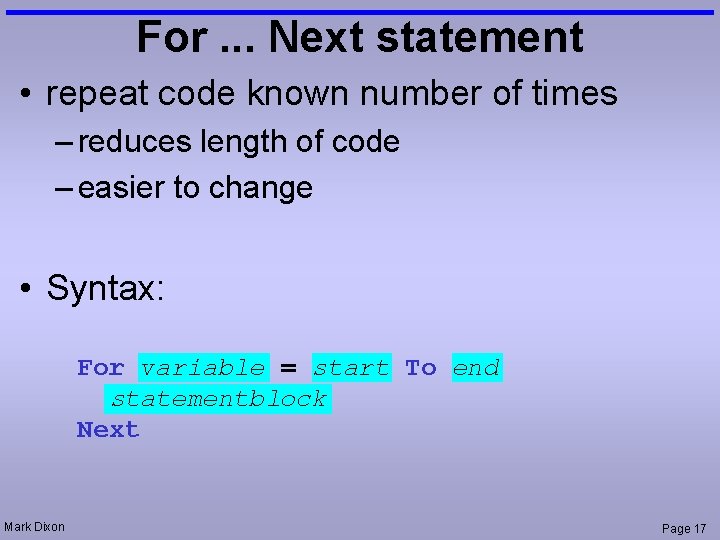
For. . . Next statement • repeat code known number of times – reduces length of code – easier to change • Syntax: For variable = start To end statementblock Next Mark Dixon Page 17
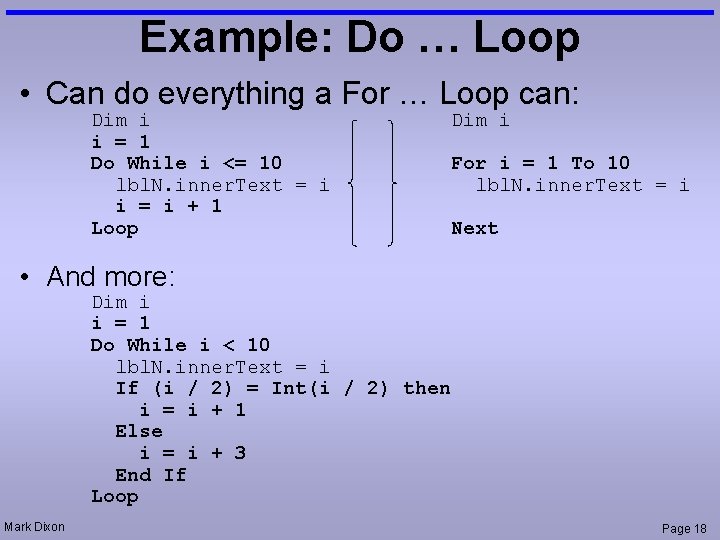
Example: Do … Loop • Can do everything a For … Loop can: Dim i i = 1 Do While i <= 10 lbl. N. inner. Text = i i = i + 1 Loop Dim i For i = 1 To 10 lbl. N. inner. Text = i Next • And more: Dim i i = 1 Do While i < 10 lbl. N. inner. Text = i If (i / 2) = Int(i / 2) then i = i + 1 Else i = i + 3 End If Loop Mark Dixon Page 18
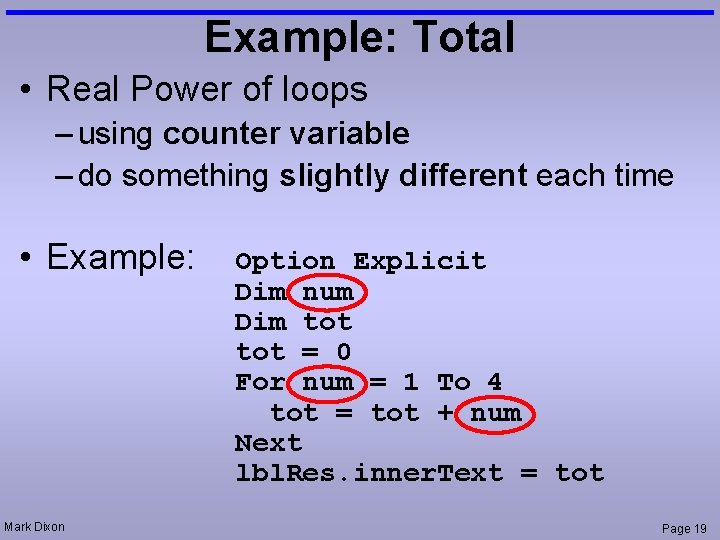
Example: Total • Real Power of loops – using counter variable – do something slightly different each time • Example: Mark Dixon Option Explicit Dim num Dim tot = 0 For num = 1 To 4 tot = tot + num Next lbl. Res. inner. Text = tot Page 19
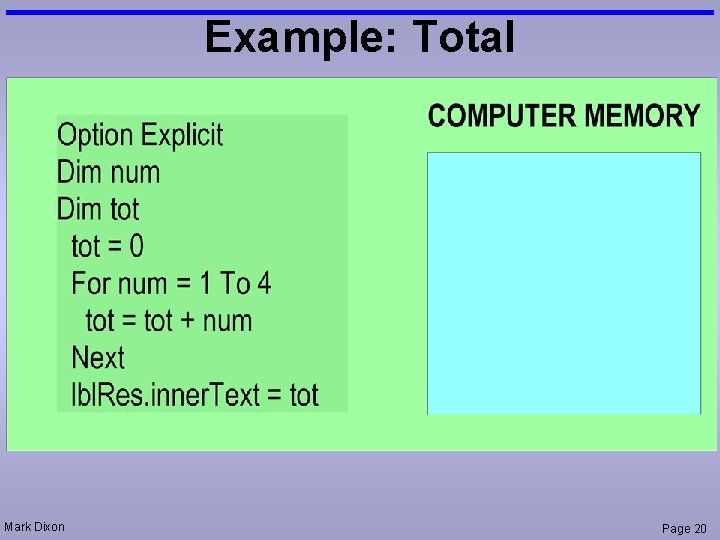
Example: Total Mark Dixon Page 20
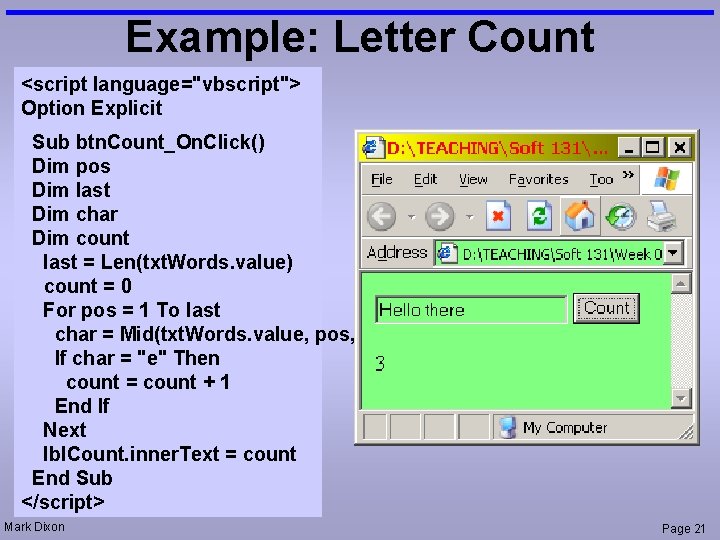
Example: Letter Count <script language="vbscript"> Option Explicit Sub btn. Count_On. Click() Dim pos Dim last Dim char Dim count last = Len(txt. Words. value) count = 0 For pos = 1 To last char = Mid(txt. Words. value, pos, 1) If char = "e" Then count = count + 1 End If Next lbl. Count. inner. Text = count End Sub </script> Mark Dixon Page 21
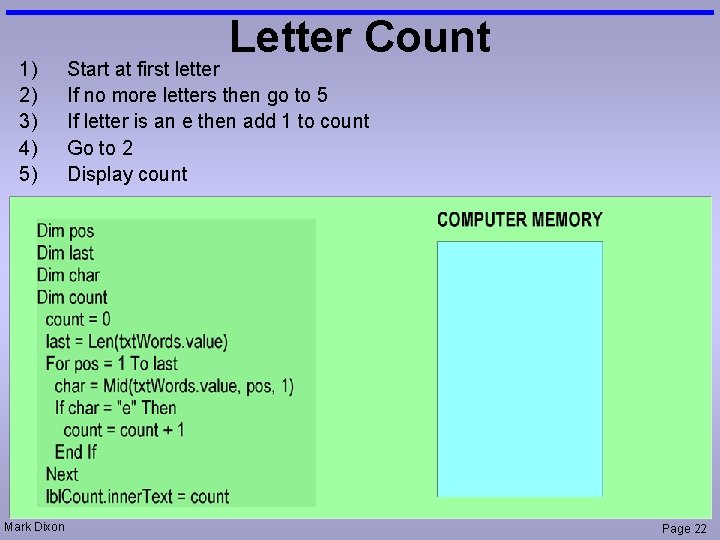
1) 2) 3) 4) 5) Mark Dixon Letter Count Start at first letter If no more letters then go to 5 If letter is an e then add 1 to count Go to 2 Display count Page 22
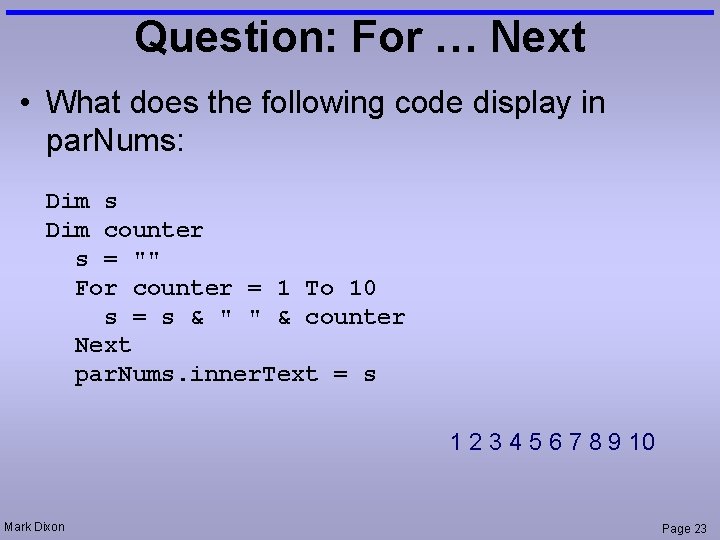
Question: For … Next • What does the following code display in par. Nums: Dim s Dim counter s = "" For counter = 1 To 10 s = s & " " & counter Next par. Nums. inner. Text = s 1 2 3 4 5 6 7 8 9 10 Mark Dixon Page 23
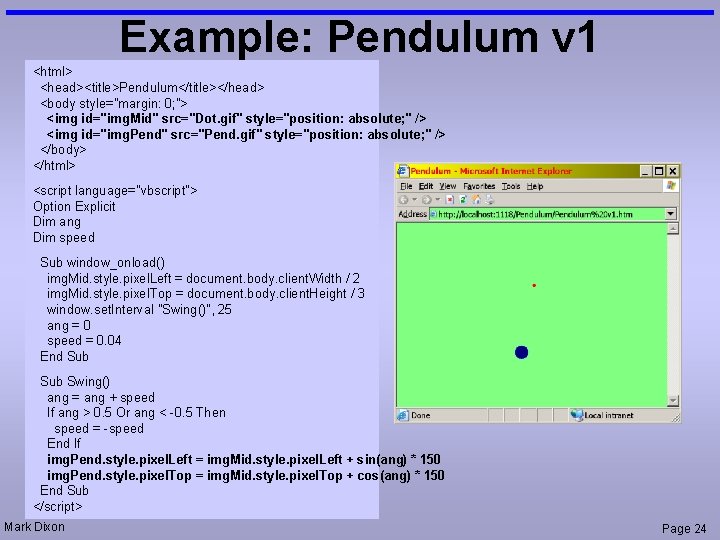
Example: Pendulum v 1 <html> <head><title>Pendulum</title></head> <body style="margin: 0; "> <img id="img. Mid" src="Dot. gif" style="position: absolute; " /> <img id="img. Pend" src="Pend. gif" style="position: absolute; " /> </body> </html> <script language="vbscript"> Option Explicit Dim ang Dim speed Sub window_onload() img. Mid. style. pixel. Left = document. body. client. Width / 2 img. Mid. style. pixel. Top = document. body. client. Height / 3 window. set. Interval "Swing()", 25 ang = 0 speed = 0. 04 End Sub Swing() ang = ang + speed If ang > 0. 5 Or ang < -0. 5 Then speed = -speed End If img. Pend. style. pixel. Left = img. Mid. style. pixel. Left + sin(ang) * 150 img. Pend. style. pixel. Top = img. Mid. style. pixel. Top + cos(ang) * 150 End Sub </script> Mark Dixon Page 24
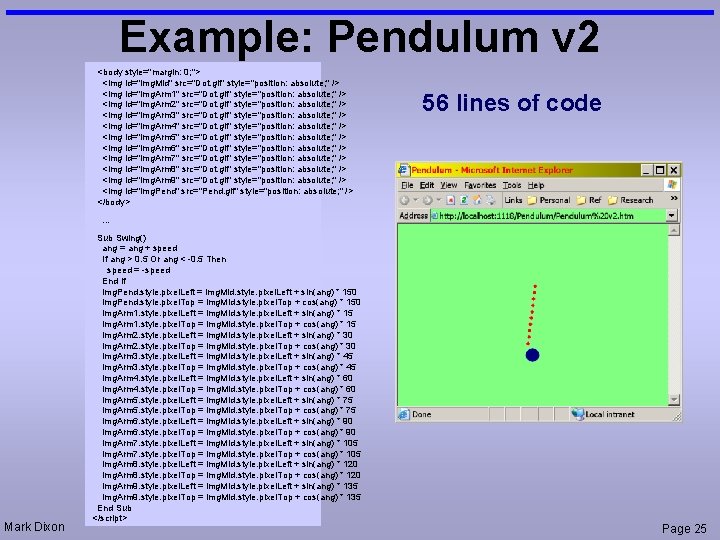
Example: Pendulum v 2 <body style="margin: 0; "> <img id="img. Mid" src="Dot. gif" style="position: absolute; " /> <img id="img. Arm 1" src="Dot. gif" style="position: absolute; " /> <img id="img. Arm 2" src="Dot. gif" style="position: absolute; " /> <img id="img. Arm 3" src="Dot. gif" style="position: absolute; " /> <img id="img. Arm 4" src="Dot. gif" style="position: absolute; " /> <img id="img. Arm 5" src="Dot. gif" style="position: absolute; " /> <img id="img. Arm 6" src="Dot. gif" style="position: absolute; " /> <img id="img. Arm 7" src="Dot. gif" style="position: absolute; " /> <img id="img. Arm 8" src="Dot. gif" style="position: absolute; " /> <img id="img. Arm 9" src="Dot. gif" style="position: absolute; " /> <img id="img. Pend" src="Pend. gif" style="position: absolute; " /> </body> 56 lines of code … Mark Dixon Sub Swing() ang = ang + speed If ang > 0. 5 Or ang < -0. 5 Then speed = -speed End If img. Pend. style. pixel. Left = img. Mid. style. pixel. Left + sin(ang) * 150 img. Pend. style. pixel. Top = img. Mid. style. pixel. Top + cos(ang) * 150 img. Arm 1. style. pixel. Left = img. Mid. style. pixel. Left + sin(ang) * 15 img. Arm 1. style. pixel. Top = img. Mid. style. pixel. Top + cos(ang) * 15 img. Arm 2. style. pixel. Left = img. Mid. style. pixel. Left + sin(ang) * 30 img. Arm 2. style. pixel. Top = img. Mid. style. pixel. Top + cos(ang) * 30 img. Arm 3. style. pixel. Left = img. Mid. style. pixel. Left + sin(ang) * 45 img. Arm 3. style. pixel. Top = img. Mid. style. pixel. Top + cos(ang) * 45 img. Arm 4. style. pixel. Left = img. Mid. style. pixel. Left + sin(ang) * 60 img. Arm 4. style. pixel. Top = img. Mid. style. pixel. Top + cos(ang) * 60 img. Arm 5. style. pixel. Left = img. Mid. style. pixel. Left + sin(ang) * 75 img. Arm 5. style. pixel. Top = img. Mid. style. pixel. Top + cos(ang) * 75 img. Arm 6. style. pixel. Left = img. Mid. style. pixel. Left + sin(ang) * 90 img. Arm 6. style. pixel. Top = img. Mid. style. pixel. Top + cos(ang) * 90 img. Arm 7. style. pixel. Left = img. Mid. style. pixel. Left + sin(ang) * 105 img. Arm 7. style. pixel. Top = img. Mid. style. pixel. Top + cos(ang) * 105 img. Arm 8. style. pixel. Left = img. Mid. style. pixel. Left + sin(ang) * 120 img. Arm 8. style. pixel. Top = img. Mid. style. pixel. Top + cos(ang) * 120 img. Arm 9. style. pixel. Left = img. Mid. style. pixel. Left + sin(ang) * 135 img. Arm 9. style. pixel. Top = img. Mid. style. pixel. Top + cos(ang) * 135 End Sub </script> Page 25
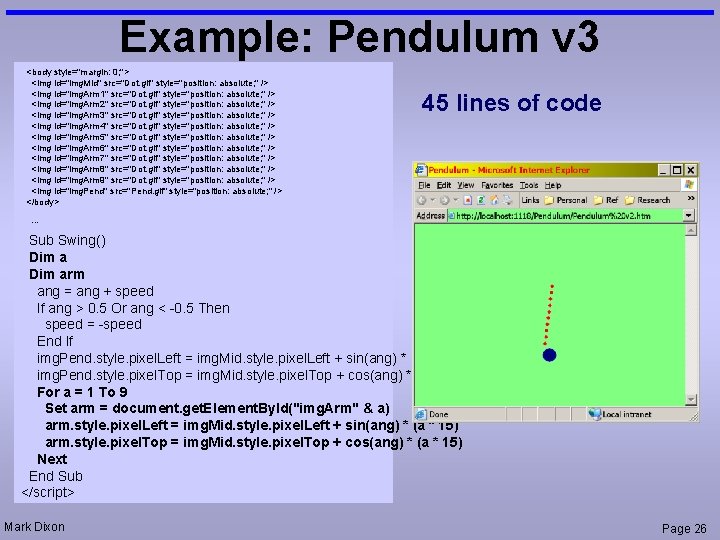
Example: Pendulum v 3 <body style="margin: 0; "> <img id="img. Mid" src="Dot. gif" style="position: absolute; " /> <img id="img. Arm 1" src="Dot. gif" style="position: absolute; " /> <img id="img. Arm 2" src="Dot. gif" style="position: absolute; " /> <img id="img. Arm 3" src="Dot. gif" style="position: absolute; " /> <img id="img. Arm 4" src="Dot. gif" style="position: absolute; " /> <img id="img. Arm 5" src="Dot. gif" style="position: absolute; " /> <img id="img. Arm 6" src="Dot. gif" style="position: absolute; " /> <img id="img. Arm 7" src="Dot. gif" style="position: absolute; " /> <img id="img. Arm 8" src="Dot. gif" style="position: absolute; " /> <img id="img. Arm 9" src="Dot. gif" style="position: absolute; " /> <img id="img. Pend" src="Pend. gif" style="position: absolute; " /> </body> 45 lines of code … Sub Swing() Dim arm ang = ang + speed If ang > 0. 5 Or ang < -0. 5 Then speed = -speed End If img. Pend. style. pixel. Left = img. Mid. style. pixel. Left + sin(ang) * 150 img. Pend. style. pixel. Top = img. Mid. style. pixel. Top + cos(ang) * 150 For a = 1 To 9 Set arm = document. get. Element. By. Id("img. Arm" & a) arm. style. pixel. Left = img. Mid. style. pixel. Left + sin(ang) * (a * 15) arm. style. pixel. Top = img. Mid. style. pixel. Top + cos(ang) * (a * 15) Next End Sub </script> Mark Dixon Page 26
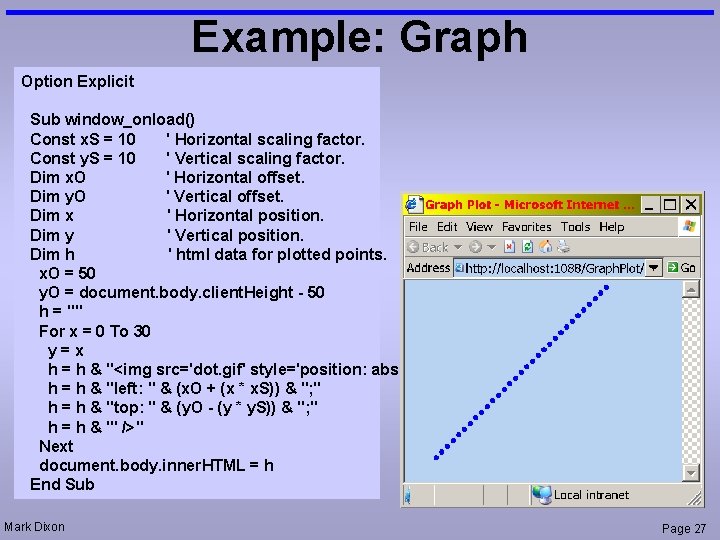
Example: Graph Option Explicit Sub window_onload() Const x. S = 10 ' Horizontal scaling factor. Const y. S = 10 ' Vertical scaling factor. Dim x. O ' Horizontal offset. Dim y. O ' Vertical offset. Dim x ' Horizontal position. Dim y ' Vertical position. Dim h ' html data for plotted points. x. O = 50 y. O = document. body. client. Height - 50 h = "" For x = 0 To 30 y=x h = h & "<img src='dot. gif' style='position: absolute; " h = h & "left: " & (x. O + (x * x. S)) & "; " h = h & "top: " & (y. O - (y * y. S)) & "; " h = h & "' />" Next document. body. inner. HTML = h End Sub Mark Dixon Page 27
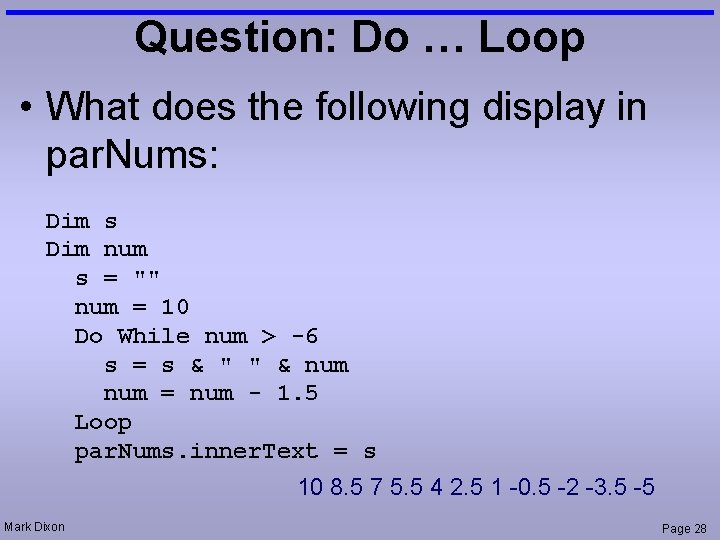
Question: Do … Loop • What does the following display in par. Nums: Dim s Dim num s = "" num = 10 Do While num > -6 s = s & " " & num = num - 1. 5 Loop par. Nums. inner. Text = s 10 8. 5 7 5. 5 4 2. 5 1 -0. 5 -2 -3. 5 -5 Mark Dixon Page 28
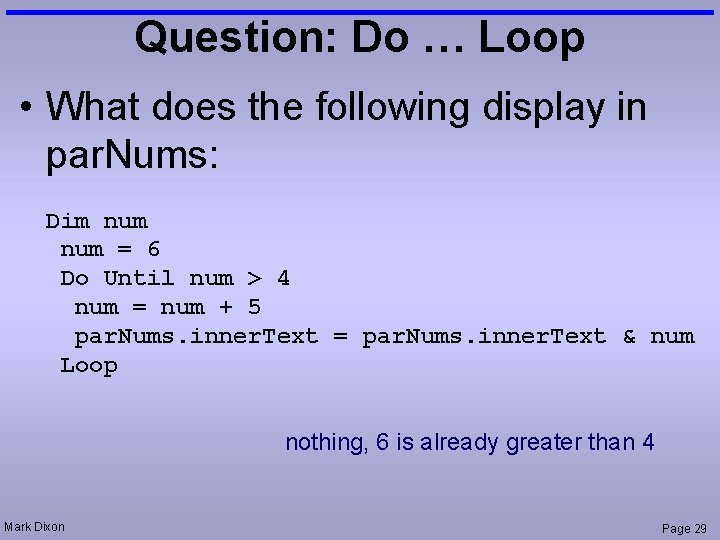
Question: Do … Loop • What does the following display in par. Nums: Dim num = 6 Do Until num > 4 num = num + 5 par. Nums. inner. Text = par. Nums. inner. Text & num Loop nothing, 6 is already greater than 4 Mark Dixon Page 29
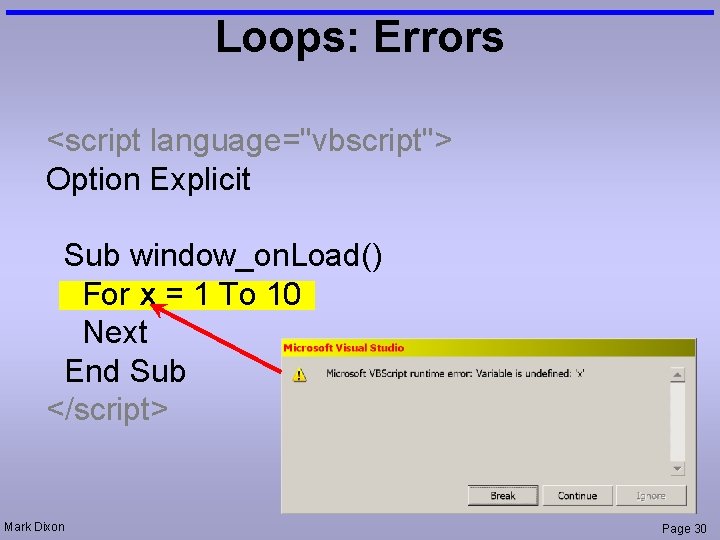
Loops: Errors <script language="vbscript"> Option Explicit Sub window_on. Load() For x = 1 To 10 Next End Sub </script> Mark Dixon Page 30
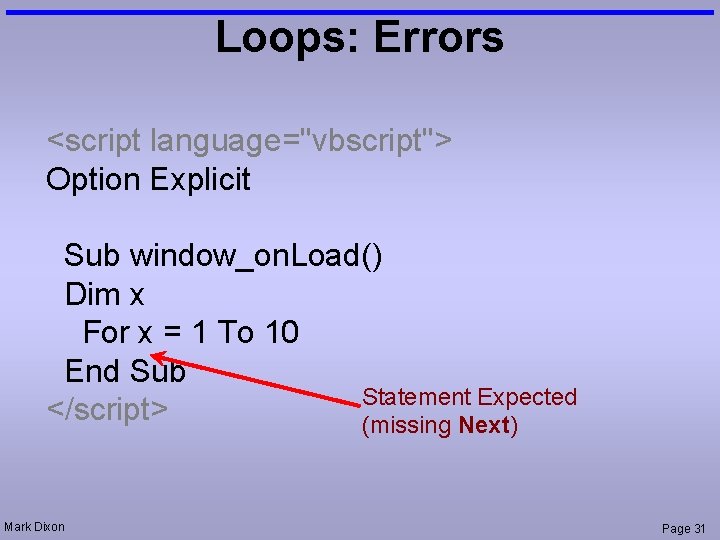
Loops: Errors <script language="vbscript"> Option Explicit Sub window_on. Load() Dim x For x = 1 To 10 End Sub Statement Expected </script> (missing Next) Mark Dixon Page 31
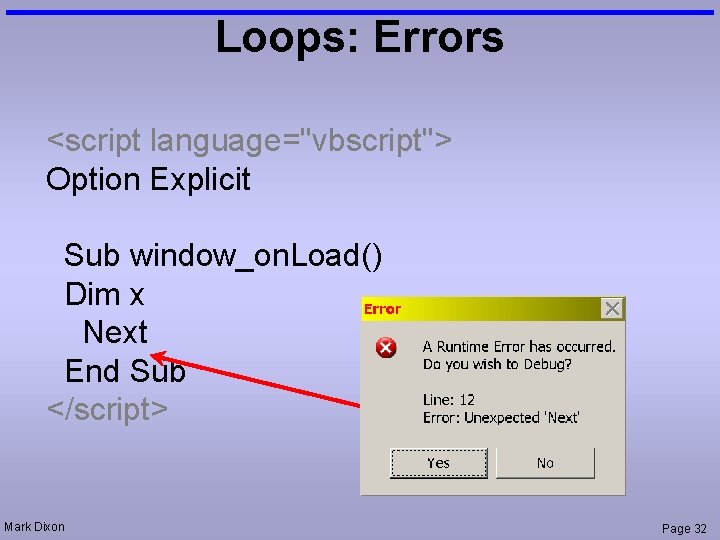
Loops: Errors <script language="vbscript"> Option Explicit Sub window_on. Load() Dim x Next End Sub </script> Mark Dixon Page 32
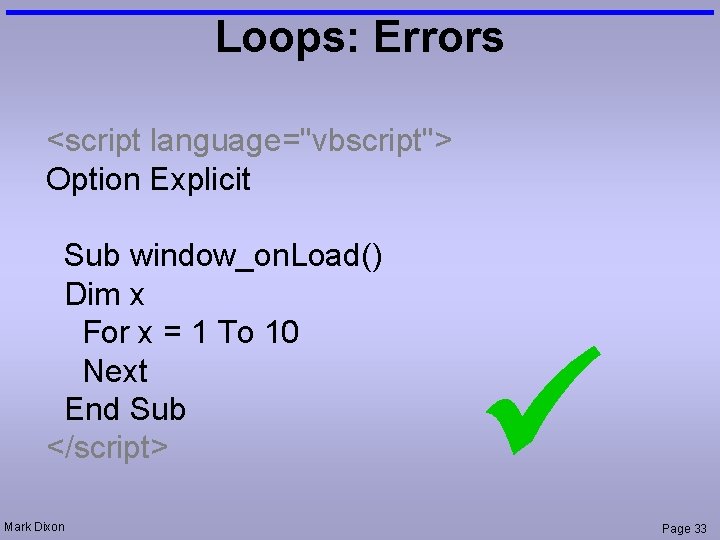
Loops: Errors <script language="vbscript"> Option Explicit Sub window_on. Load() Dim x For x = 1 To 10 Next End Sub </script> Mark Dixon Page 33
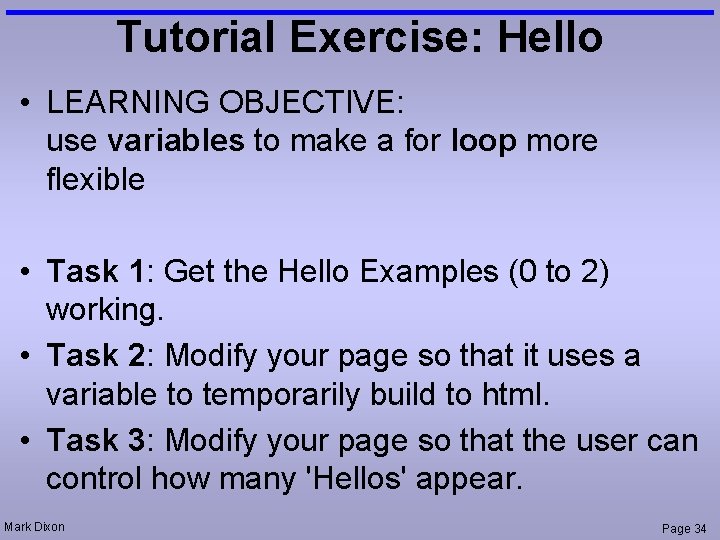
Tutorial Exercise: Hello • LEARNING OBJECTIVE: use variables to make a for loop more flexible • Task 1: Get the Hello Examples (0 to 2) working. • Task 2: Modify your page so that it uses a variable to temporarily build to html. • Task 3: Modify your page so that the user can control how many 'Hellos' appear. Mark Dixon Page 34
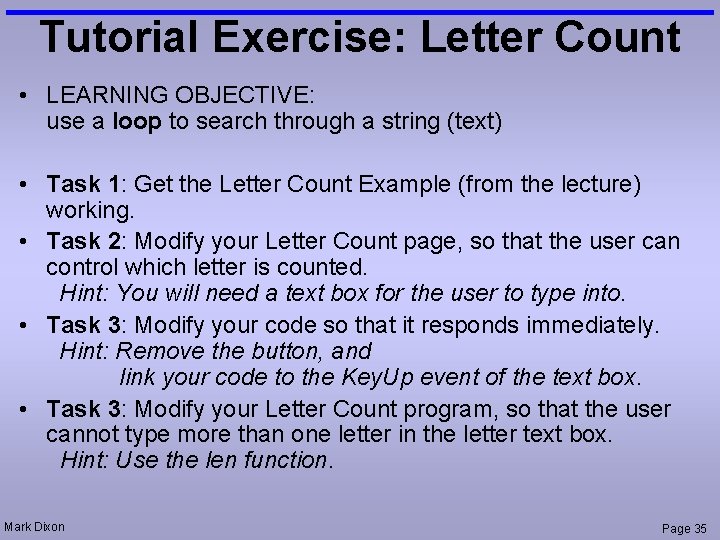
Tutorial Exercise: Letter Count • LEARNING OBJECTIVE: use a loop to search through a string (text) • Task 1: Get the Letter Count Example (from the lecture) working. • Task 2: Modify your Letter Count page, so that the user can control which letter is counted. Hint: You will need a text box for the user to type into. • Task 3: Modify your code so that it responds immediately. Hint: Remove the button, and link your code to the Key. Up event of the text box. • Task 3: Modify your Letter Count program, so that the user cannot type more than one letter in the letter text box. Hint: Use the len function. Mark Dixon Page 35
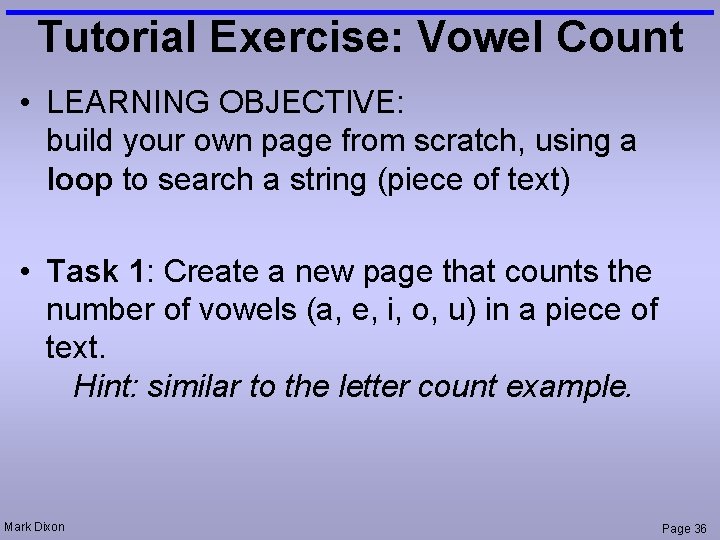
Tutorial Exercise: Vowel Count • LEARNING OBJECTIVE: build your own page from scratch, using a loop to search a string (piece of text) • Task 1: Create a new page that counts the number of vowels (a, e, i, o, u) in a piece of text. Hint: similar to the letter count example. Mark Dixon Page 36
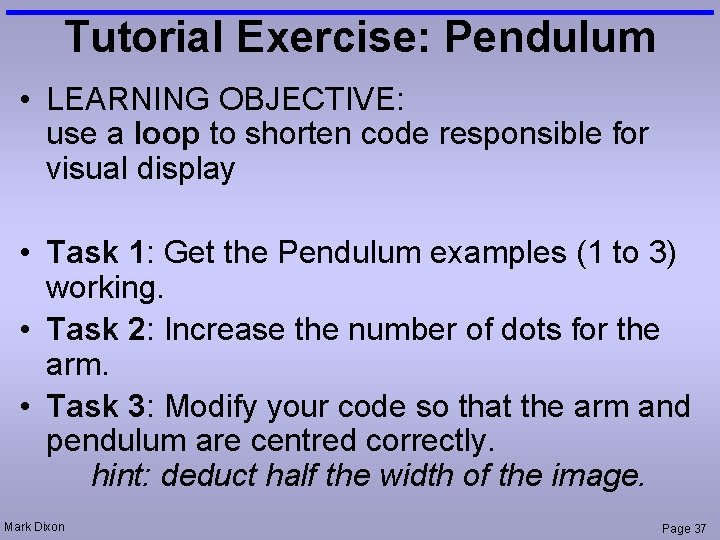
Tutorial Exercise: Pendulum • LEARNING OBJECTIVE: use a loop to shorten code responsible for visual display • Task 1: Get the Pendulum examples (1 to 3) working. • Task 2: Increase the number of dots for the arm. • Task 3: Modify your code so that the arm and pendulum are centred correctly. hint: deduct half the width of the image. Mark Dixon Page 37
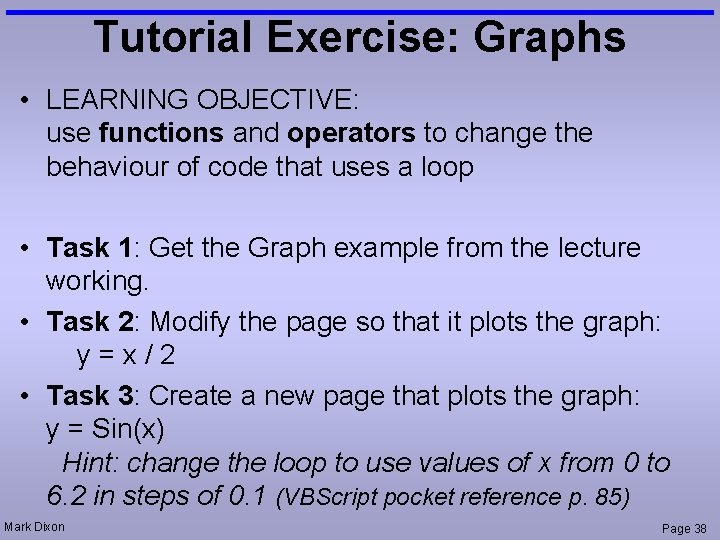
Tutorial Exercise: Graphs • LEARNING OBJECTIVE: use functions and operators to change the behaviour of code that uses a loop • Task 1: Get the Graph example from the lecture working. • Task 2: Modify the page so that it plots the graph: y=x/2 • Task 3: Create a new page that plots the graph: y = Sin(x) Hint: change the loop to use values of x from 0 to 6. 2 in steps of 0. 1 (VBScript pocket reference p. 85) Mark Dixon Page 38
Mark dixon artist
Mark dixon
How far down is the title page in apa
Spreidingsbreedte
George washington dixon
Gda academy
Dixon montessori charter school
David dixon ubc
A mason dixon memory
Paul dixon smu
Karen k dixon
Teoria de dixon y joly
Dixon case study
Potky
Grafico de dixon
The boy does nothing dance
Dixon campus jefferson
Jennifer dixon accident
Dixon swivel joints
Recursion and loop difference
Iterative deepening a* search
Incremental project management
Iterative project management
Conventional and evolutionary work breakdown structure
Iterative patterns
Iterative inorder traversal
Iterative improvement algorithm
Iterations
Progressive deepening search
Iterative deepening a* search
Recursive and iterative query
Recursive and iterative query
Iterative server
Iterative statements
Recursive and iterative query
Iterative techniques in matrix algebra
Iterative techniques in matrix algebra
Iterative deepening a* search
Application layer