Type Systems CSE 340 Principles of Programming Languages
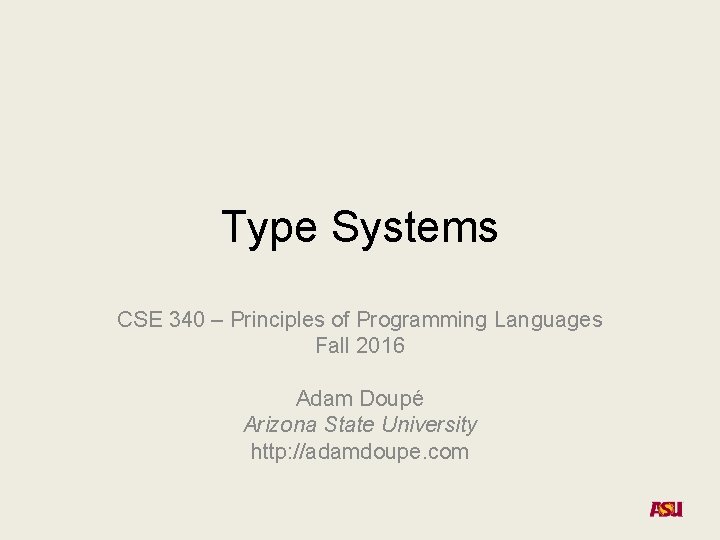
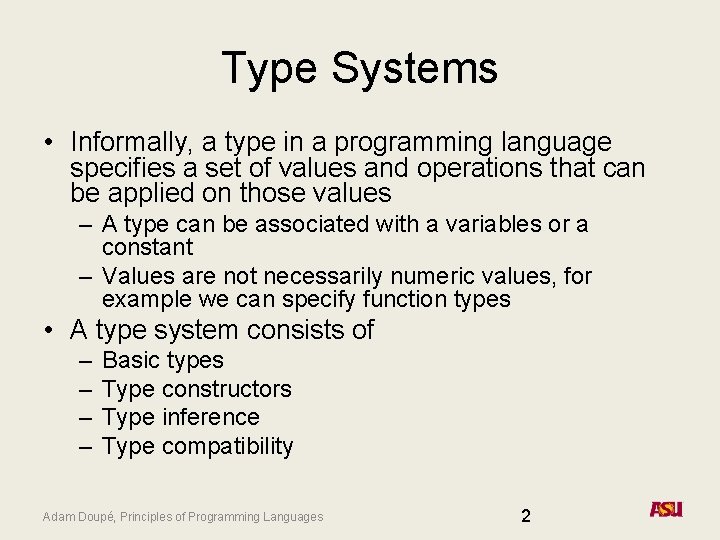
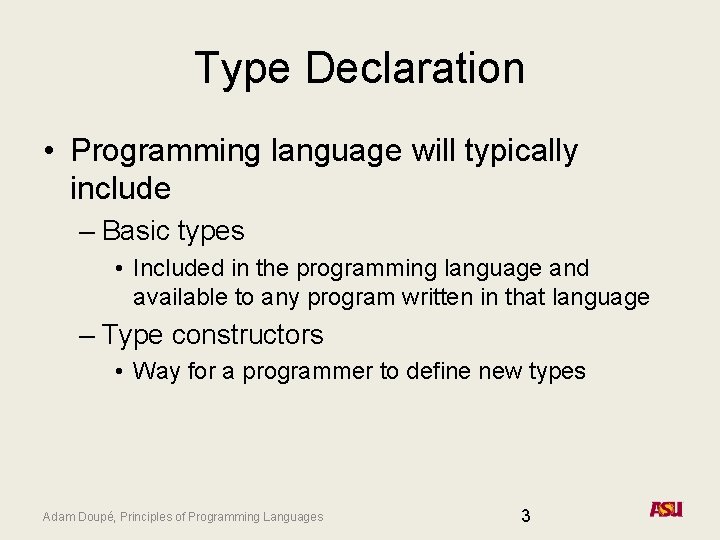
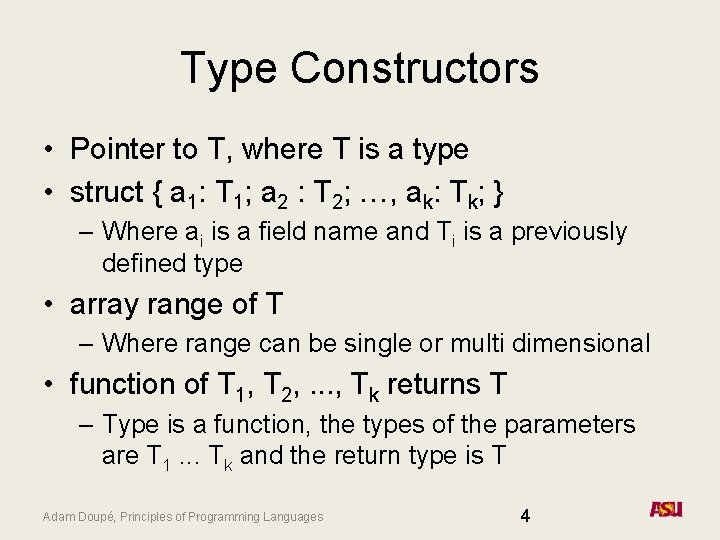
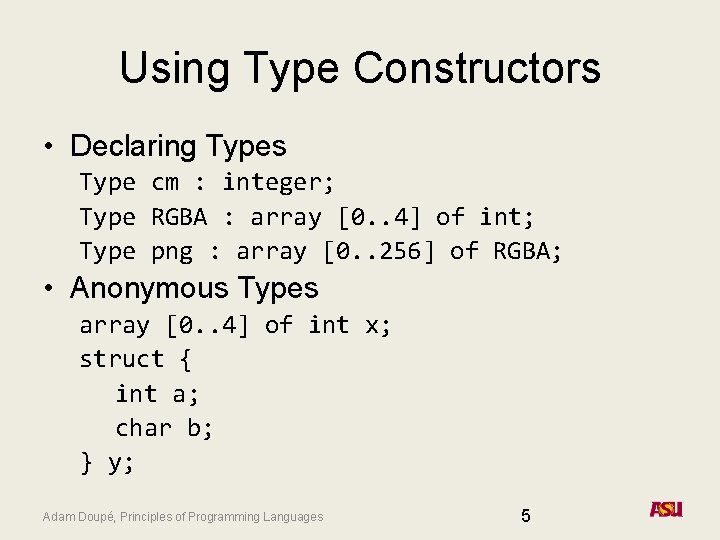
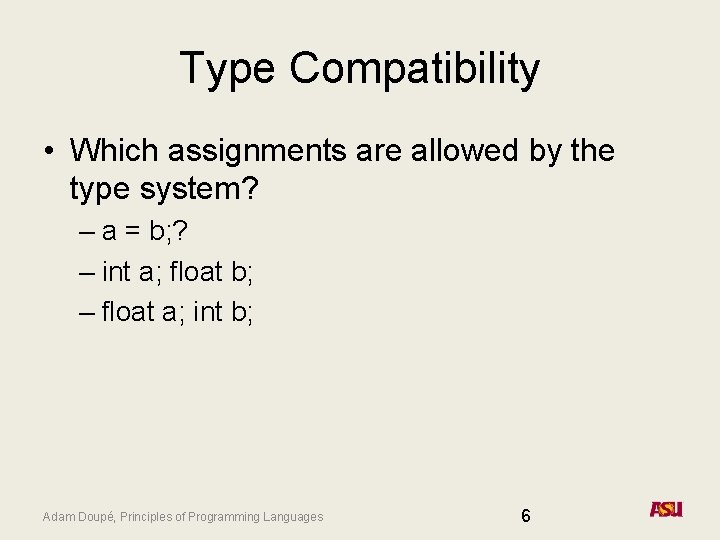
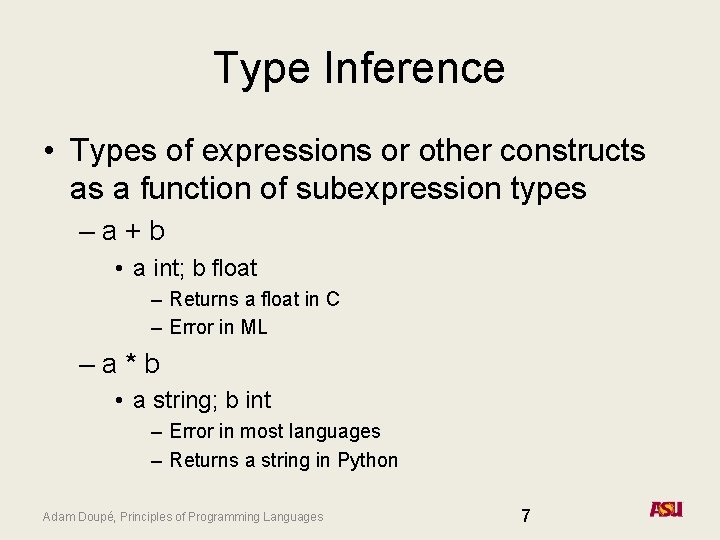
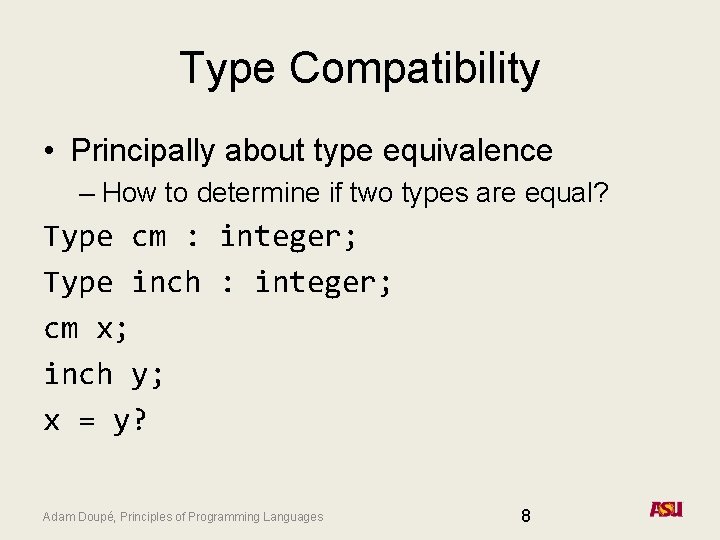
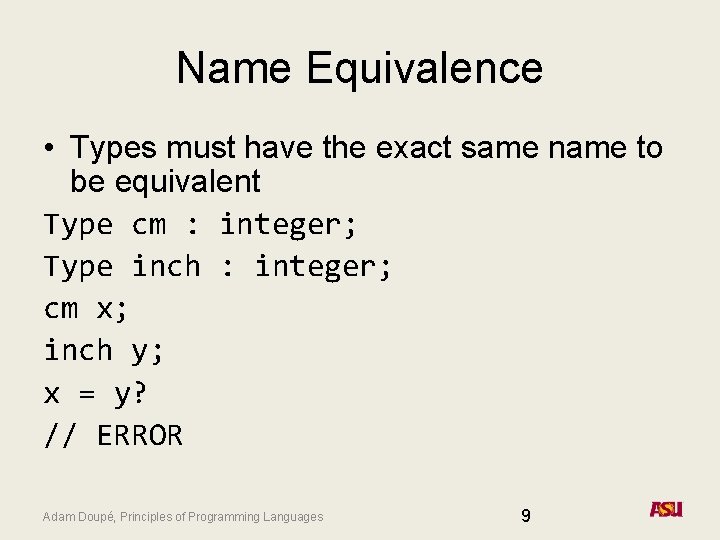
![Name Equivalence a: array [0. . 4] of int; b: array [0. . 4] Name Equivalence a: array [0. . 4] of int; b: array [0. . 4]](https://slidetodoc.com/presentation_image/251eeed9f29c508d15da5f09a586288a/image-10.jpg)
![Name Equivalence a, b: array [0. . 4] of int; • a = b? Name Equivalence a, b: array [0. . 4] of int; • a = b?](https://slidetodoc.com/presentation_image/251eeed9f29c508d15da5f09a586288a/image-11.jpg)
![Name Equivalence Type A: array [0. . 4] of int; a: A; b: A; Name Equivalence Type A: array [0. . 4] of int; a: A; b: A;](https://slidetodoc.com/presentation_image/251eeed9f29c508d15da5f09a586288a/image-12.jpg)
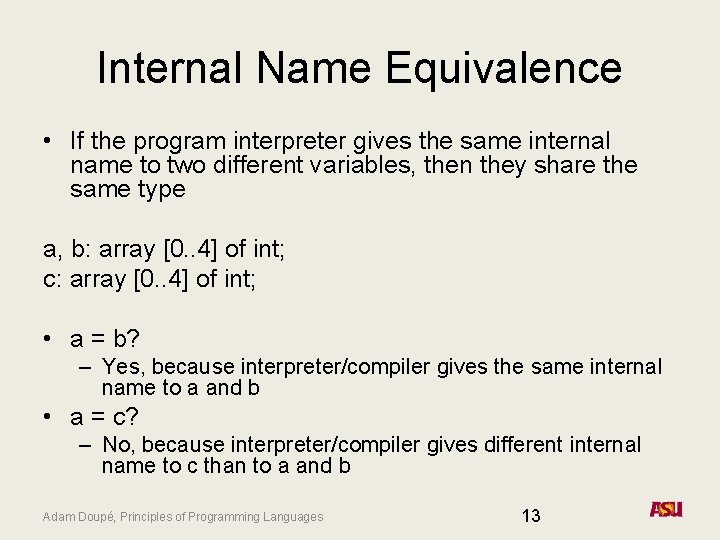
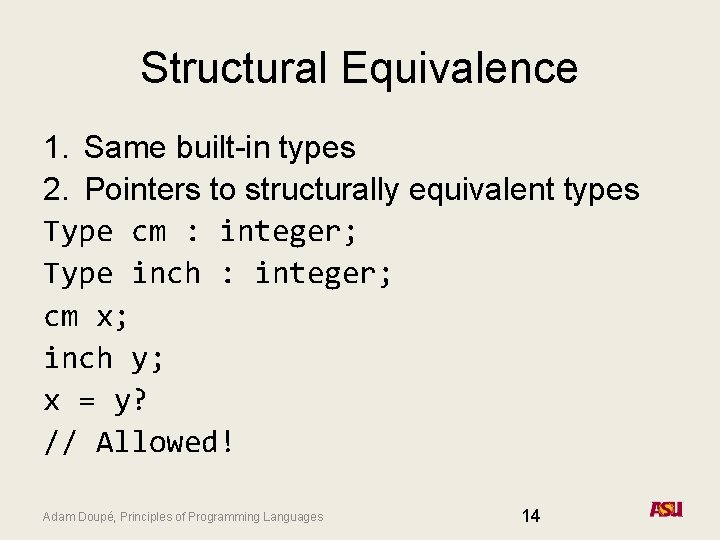
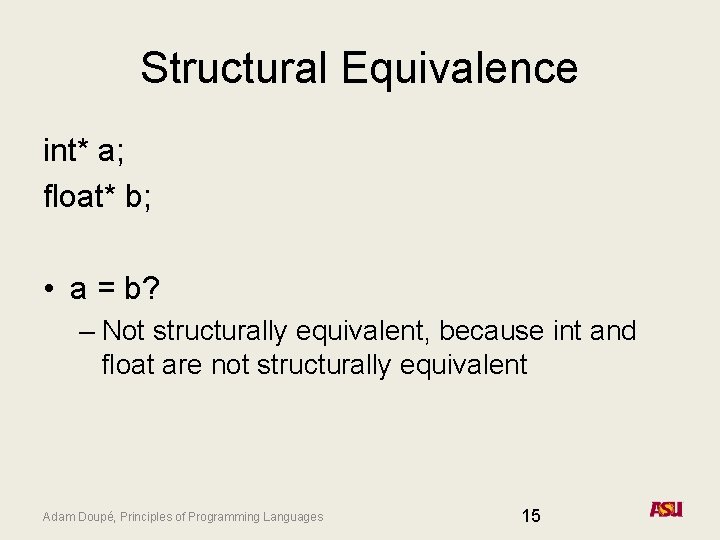
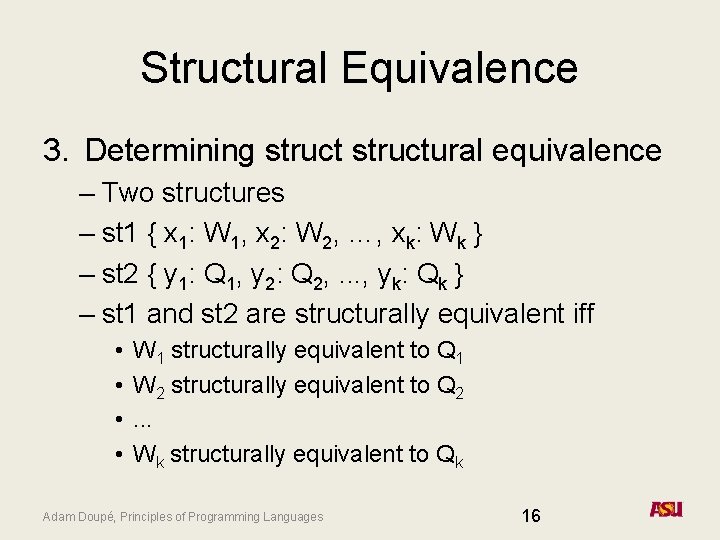
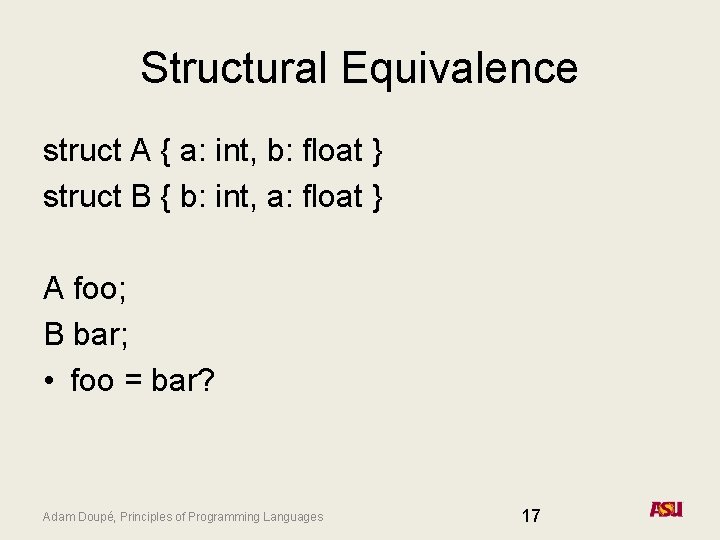
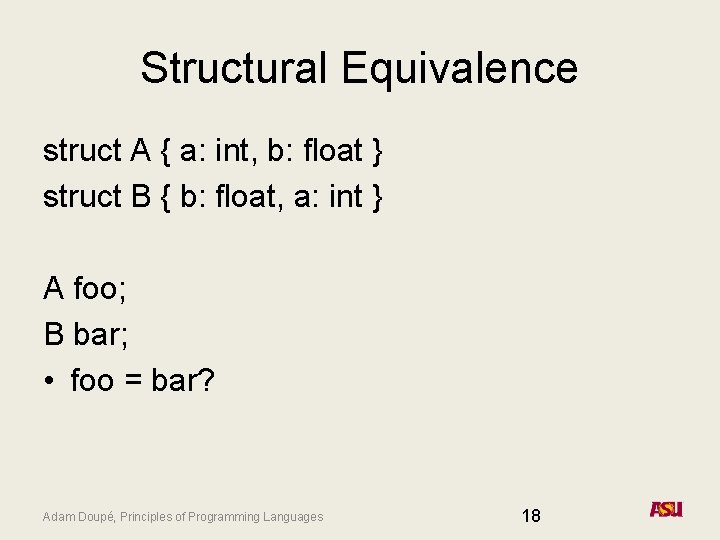
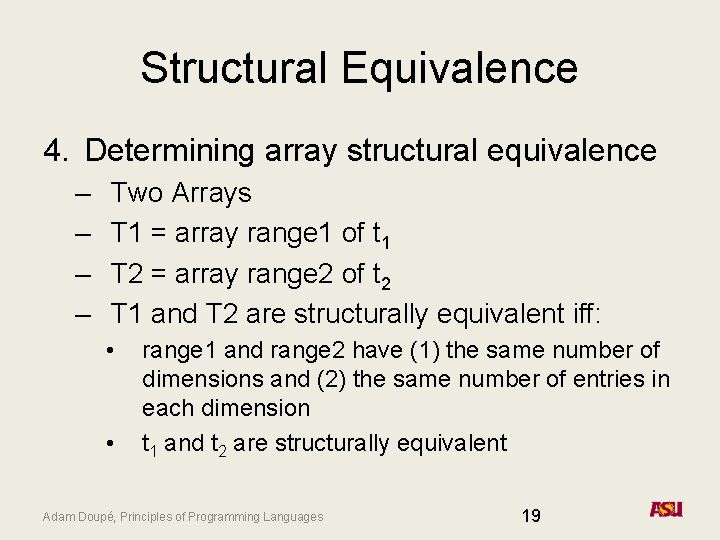
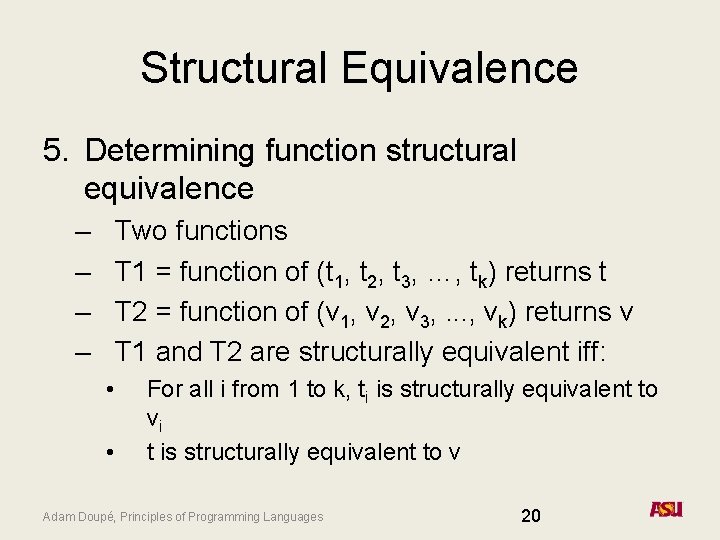
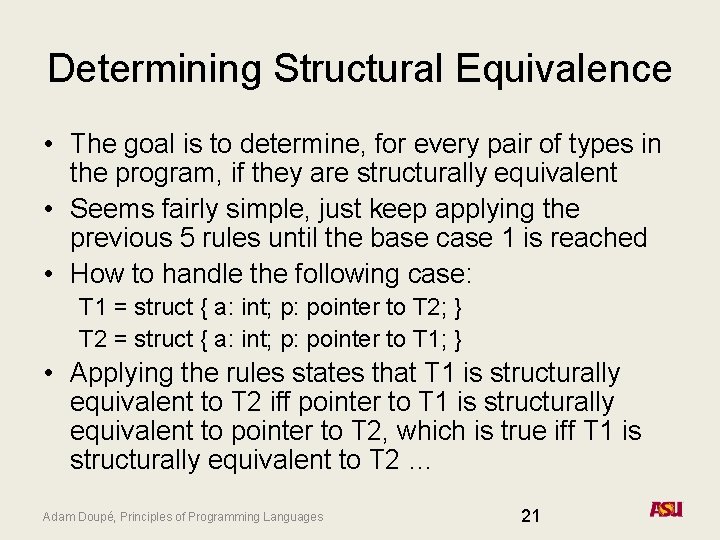
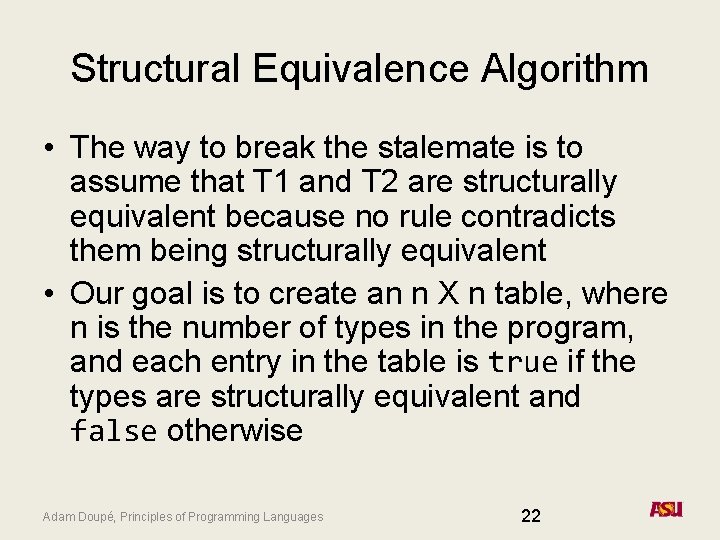
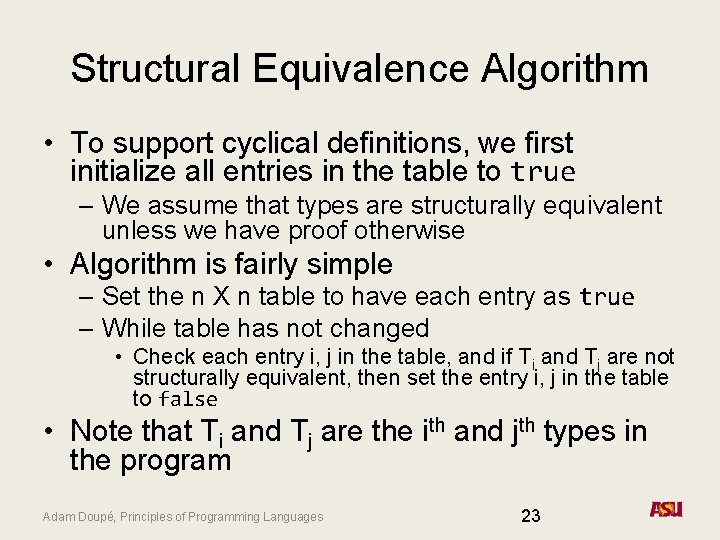
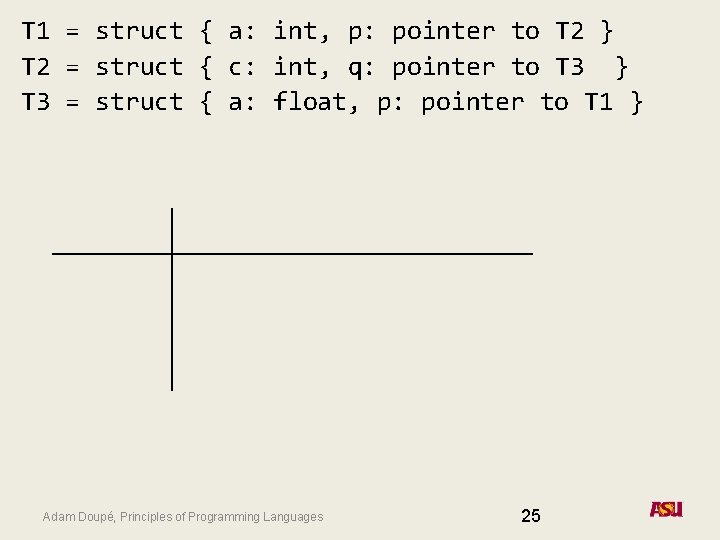
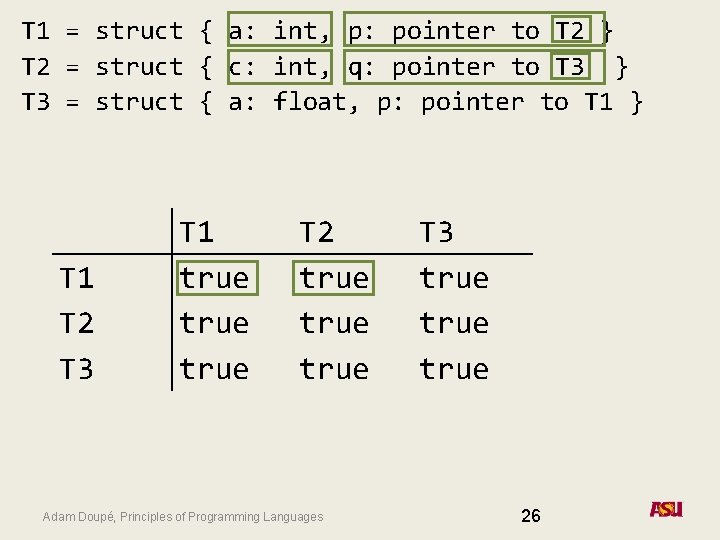
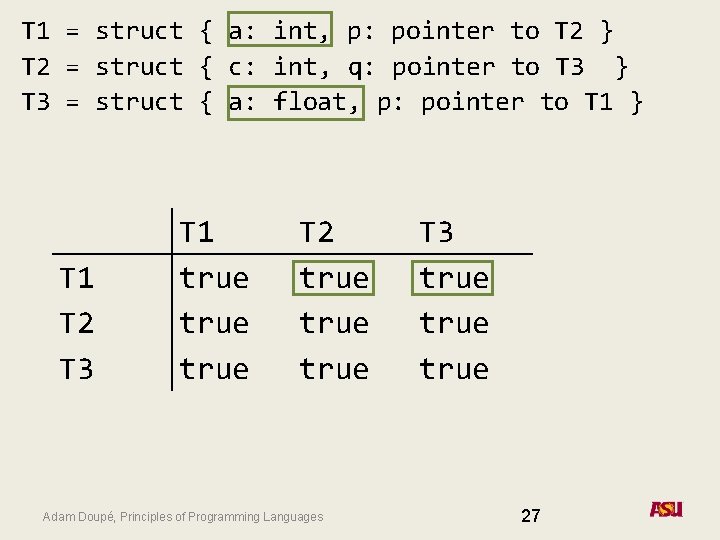
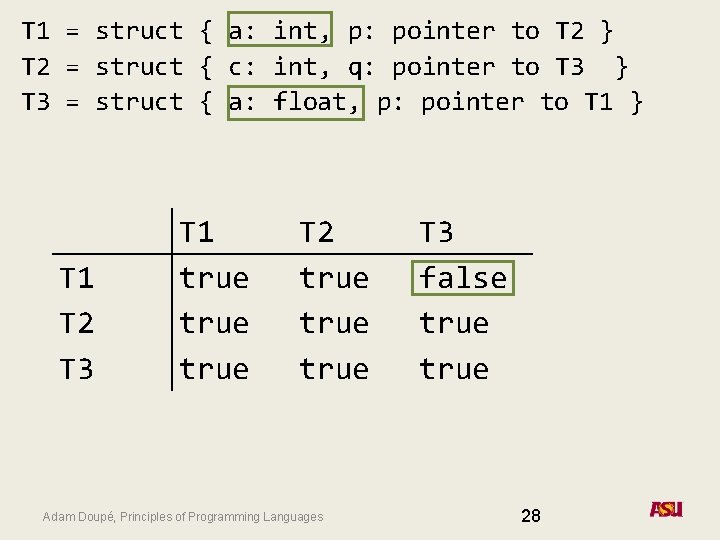
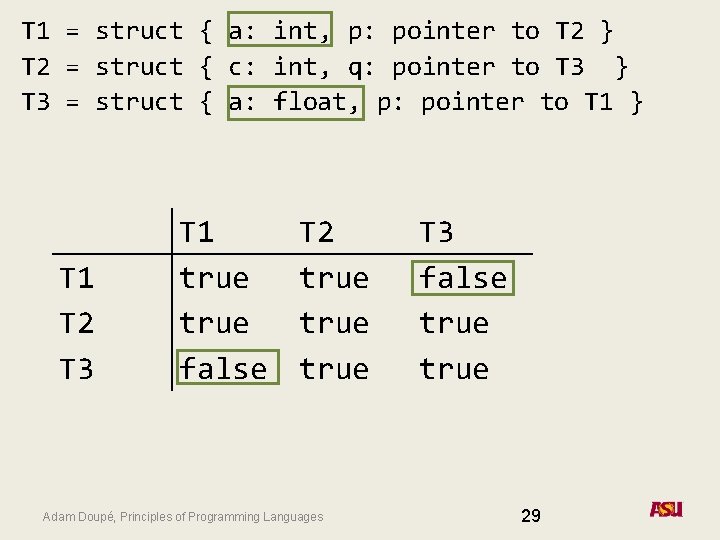
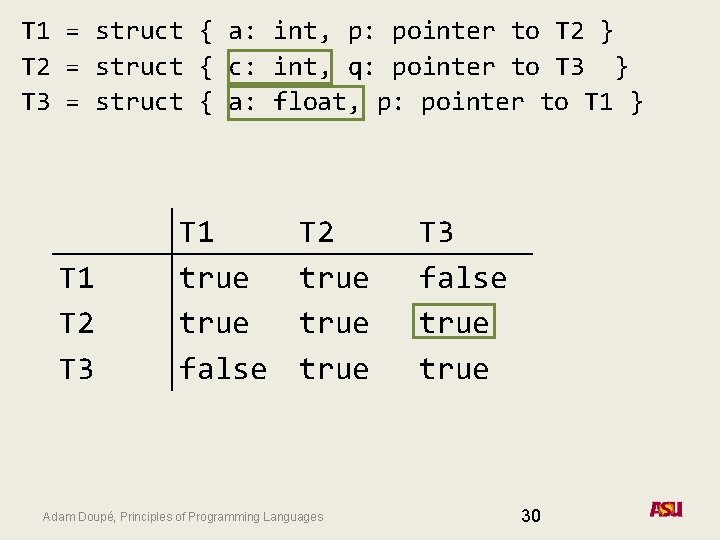
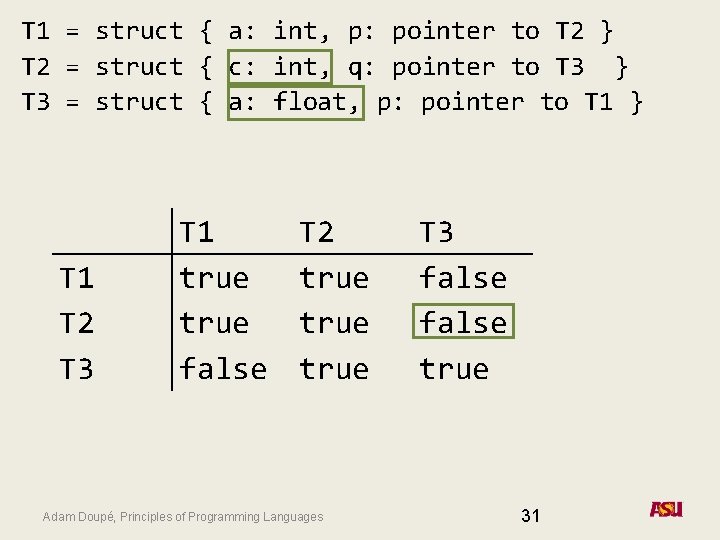
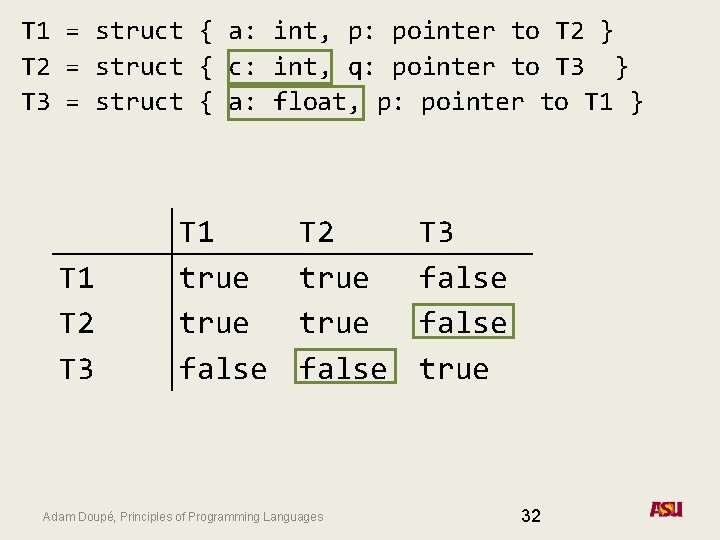
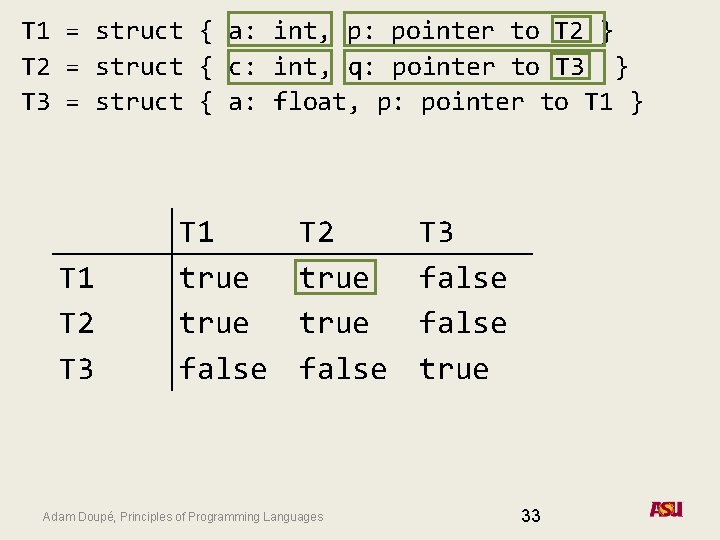
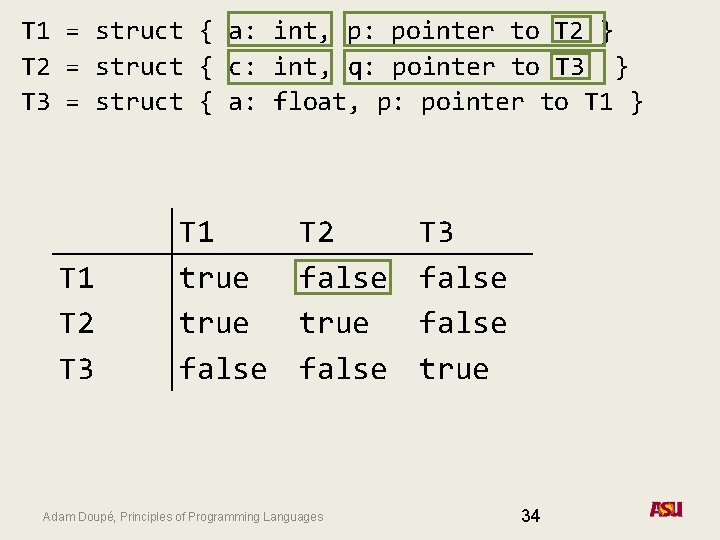
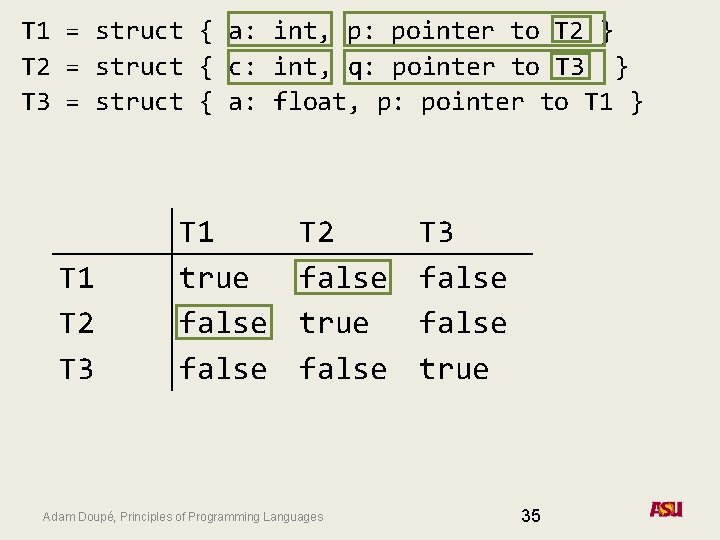
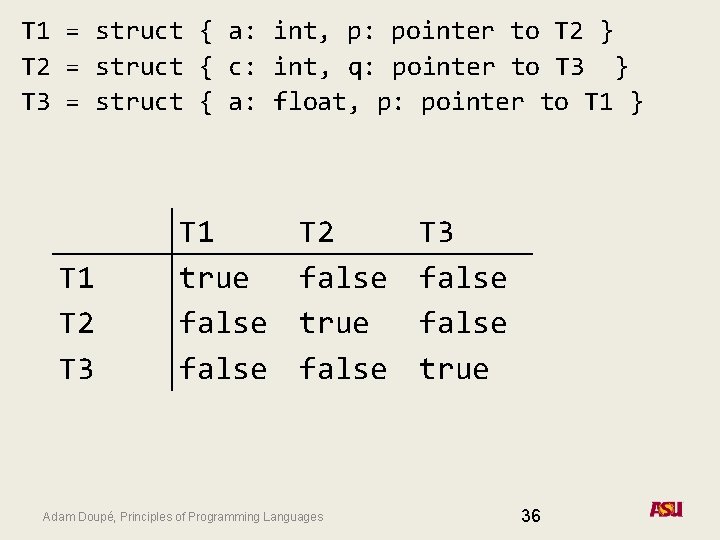
- Slides: 35
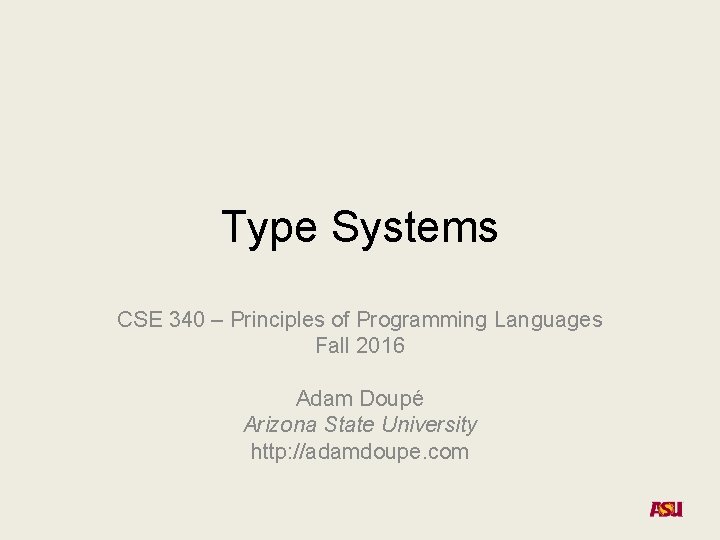
Type Systems CSE 340 – Principles of Programming Languages Fall 2016 Adam Doupé Arizona State University http: //adamdoupe. com
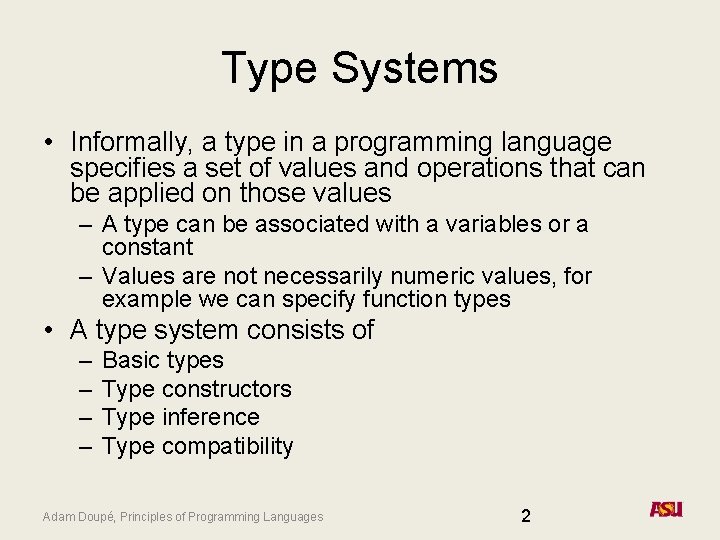
Type Systems • Informally, a type in a programming language specifies a set of values and operations that can be applied on those values – A type can be associated with a variables or a constant – Values are not necessarily numeric values, for example we can specify function types • A type system consists of – – Basic types Type constructors Type inference Type compatibility Adam Doupé, Principles of Programming Languages 2
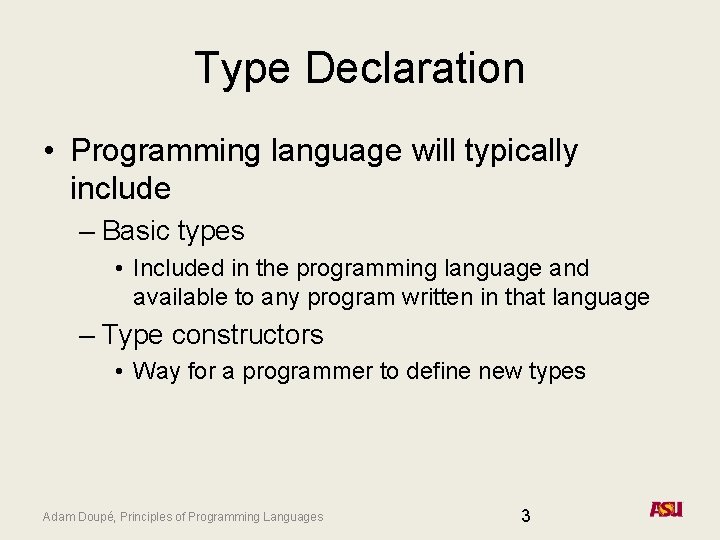
Type Declaration • Programming language will typically include – Basic types • Included in the programming language and available to any program written in that language – Type constructors • Way for a programmer to define new types Adam Doupé, Principles of Programming Languages 3
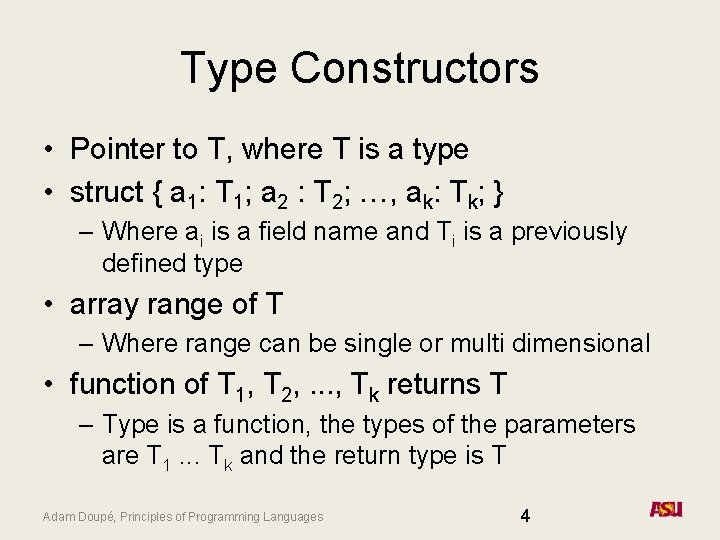
Type Constructors • Pointer to T, where T is a type • struct { a 1: T 1; a 2 : T 2; …, ak: Tk; } – Where ai is a field name and Ti is a previously defined type • array range of T – Where range can be single or multi dimensional • function of T 1, T 2, . . . , Tk returns T – Type is a function, the types of the parameters are T 1. . . Tk and the return type is T Adam Doupé, Principles of Programming Languages 4
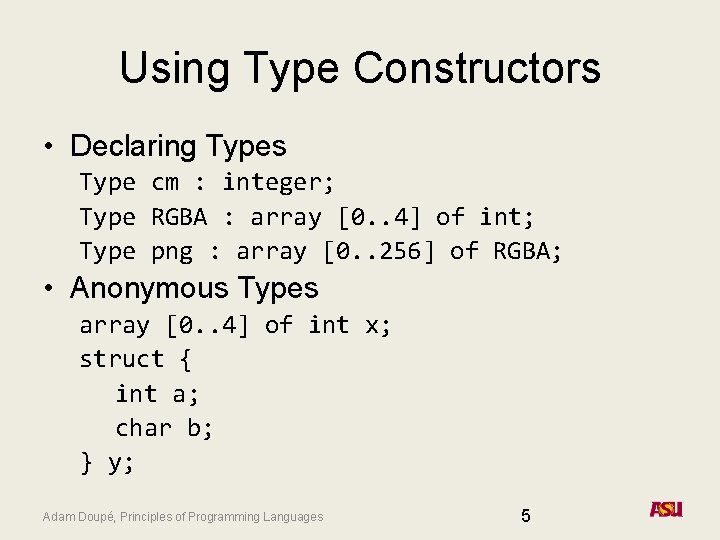
Using Type Constructors • Declaring Types Type cm : integer; Type RGBA : array [0. . 4] of int; Type png : array [0. . 256] of RGBA; • Anonymous Types array [0. . 4] of int x; struct { int a; char b; } y; Adam Doupé, Principles of Programming Languages 5
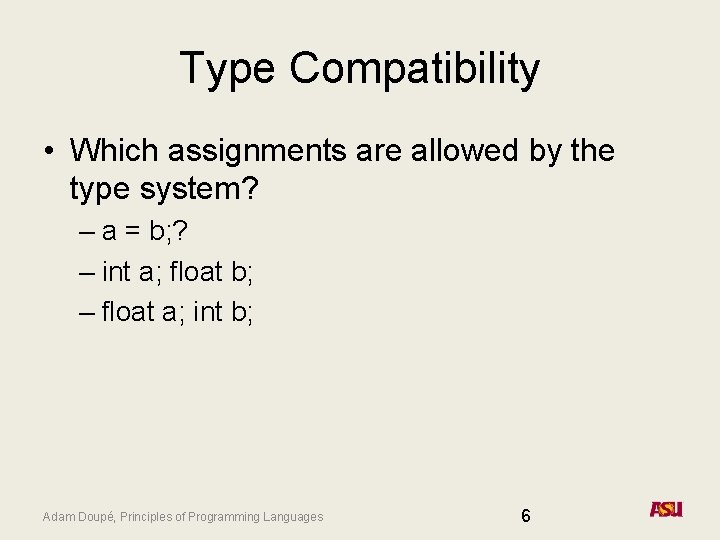
Type Compatibility • Which assignments are allowed by the type system? – a = b; ? – int a; float b; – float a; int b; Adam Doupé, Principles of Programming Languages 6
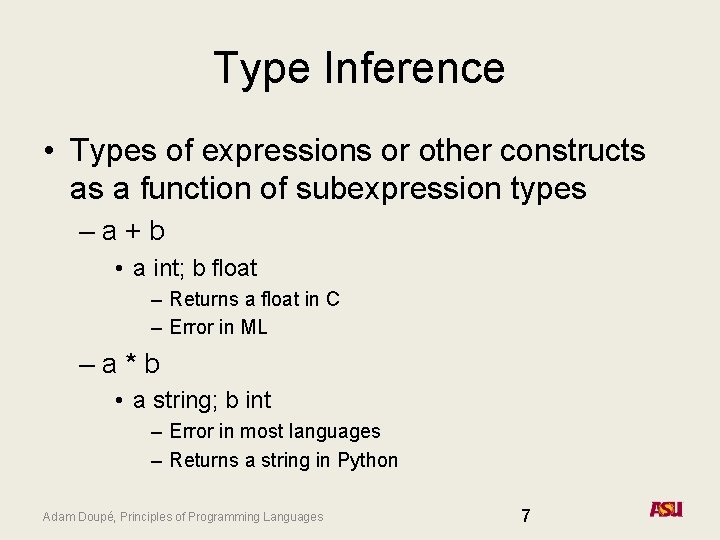
Type Inference • Types of expressions or other constructs as a function of subexpression types –a+b • a int; b float – Returns a float in C – Error in ML –a*b • a string; b int – Error in most languages – Returns a string in Python Adam Doupé, Principles of Programming Languages 7
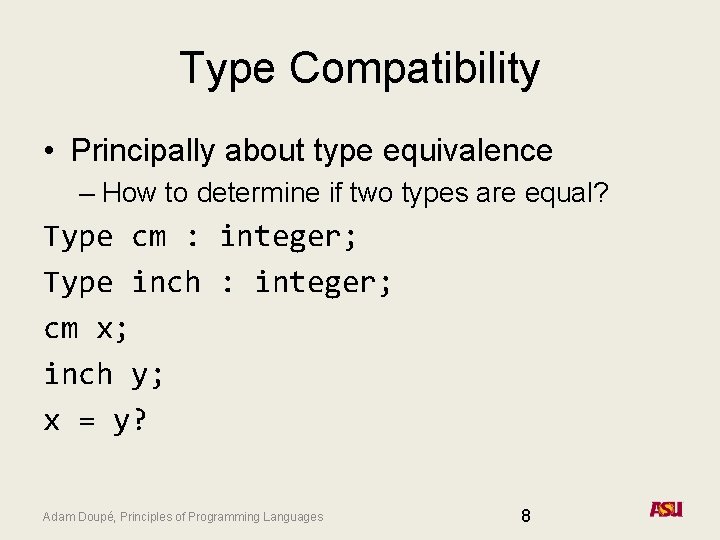
Type Compatibility • Principally about type equivalence – How to determine if two types are equal? Type cm : integer; Type inch : integer; cm x; inch y; x = y? Adam Doupé, Principles of Programming Languages 8
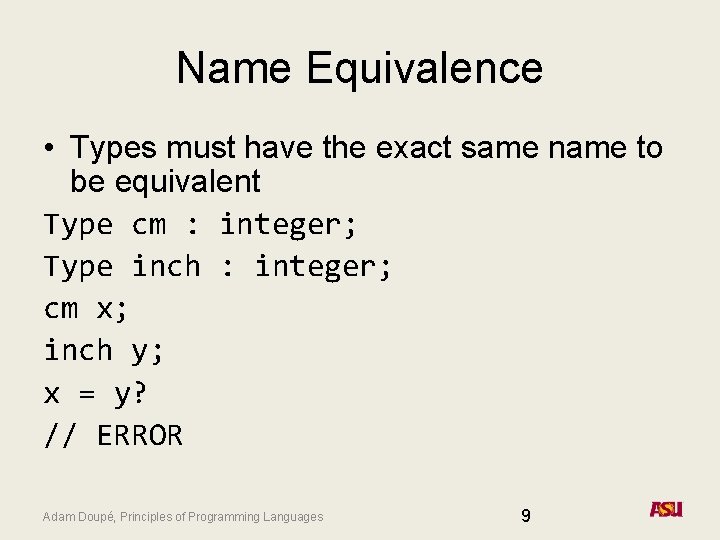
Name Equivalence • Types must have the exact same name to be equivalent Type cm : integer; Type inch : integer; cm x; inch y; x = y? // ERROR Adam Doupé, Principles of Programming Languages 9
![Name Equivalence a array 0 4 of int b array 0 4 Name Equivalence a: array [0. . 4] of int; b: array [0. . 4]](https://slidetodoc.com/presentation_image/251eeed9f29c508d15da5f09a586288a/image-10.jpg)
Name Equivalence a: array [0. . 4] of int; b: array [0. . 4] of int; • a = b? – Not allowed under name equivalence Adam Doupé, Principles of Programming Languages 10
![Name Equivalence a b array 0 4 of int a b Name Equivalence a, b: array [0. . 4] of int; • a = b?](https://slidetodoc.com/presentation_image/251eeed9f29c508d15da5f09a586288a/image-11.jpg)
Name Equivalence a, b: array [0. . 4] of int; • a = b? – Not allowed because array [0. . 4] of int is not named Adam Doupé, Principles of Programming Languages 11
![Name Equivalence Type A array 0 4 of int a A b A Name Equivalence Type A: array [0. . 4] of int; a: A; b: A;](https://slidetodoc.com/presentation_image/251eeed9f29c508d15da5f09a586288a/image-12.jpg)
Name Equivalence Type A: array [0. . 4] of int; a: A; b: A; • a = b? – Allowed, because both a and b have the same name Adam Doupé, Principles of Programming Languages 12
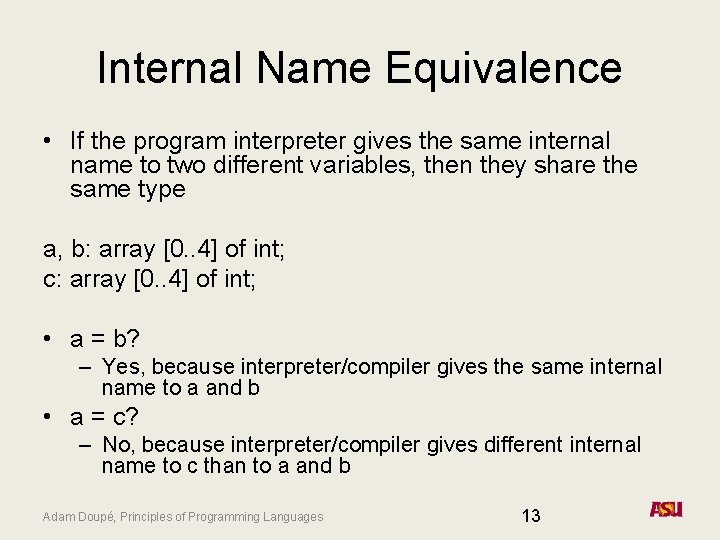
Internal Name Equivalence • If the program interpreter gives the same internal name to two different variables, then they share the same type a, b: array [0. . 4] of int; c: array [0. . 4] of int; • a = b? – Yes, because interpreter/compiler gives the same internal name to a and b • a = c? – No, because interpreter/compiler gives different internal name to c than to a and b Adam Doupé, Principles of Programming Languages 13
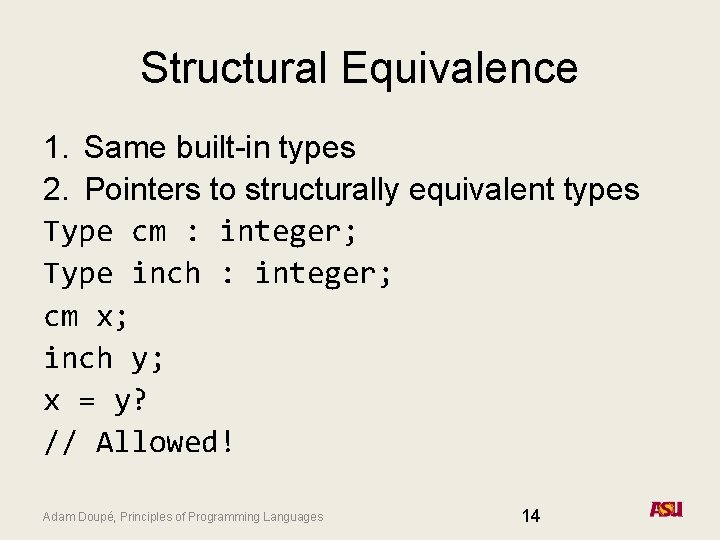
Structural Equivalence 1. Same built-in types 2. Pointers to structurally equivalent types Type cm : integer; Type inch : integer; cm x; inch y; x = y? // Allowed! Adam Doupé, Principles of Programming Languages 14
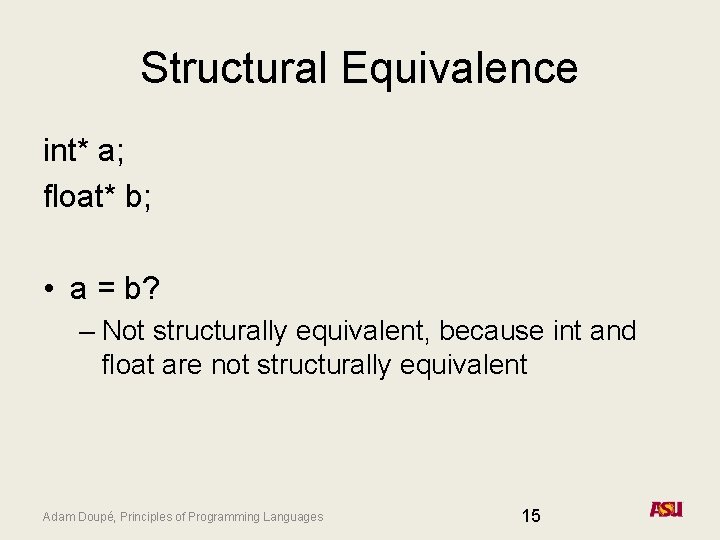
Structural Equivalence int* a; float* b; • a = b? – Not structurally equivalent, because int and float are not structurally equivalent Adam Doupé, Principles of Programming Languages 15
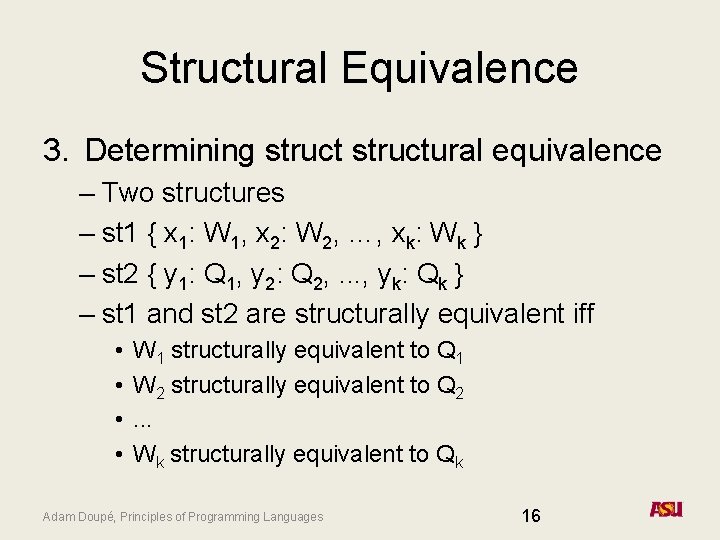
Structural Equivalence 3. Determining structural equivalence – Two structures – st 1 { x 1: W 1, x 2: W 2, …, xk: Wk } – st 2 { y 1: Q 1, y 2: Q 2, . . . , yk: Qk } – st 1 and st 2 are structurally equivalent iff • • W 1 structurally equivalent to Q 1 W 2 structurally equivalent to Q 2. . . Wk structurally equivalent to Qk Adam Doupé, Principles of Programming Languages 16
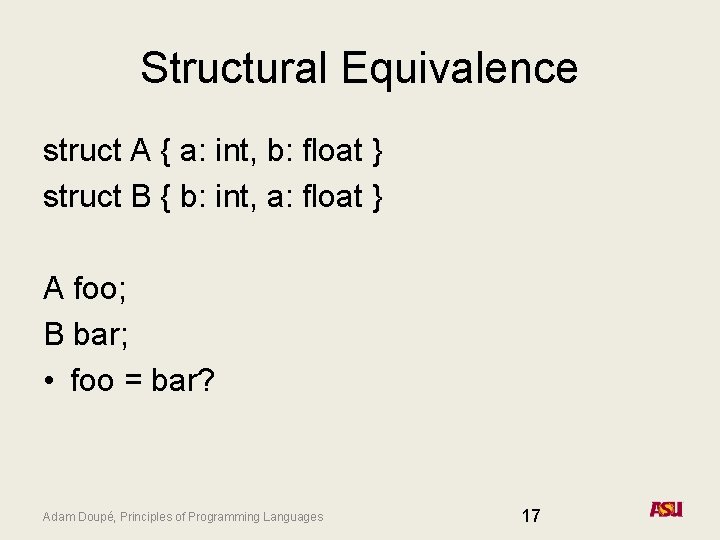
Structural Equivalence struct A { a: int, b: float } struct B { b: int, a: float } A foo; B bar; • foo = bar? Adam Doupé, Principles of Programming Languages 17
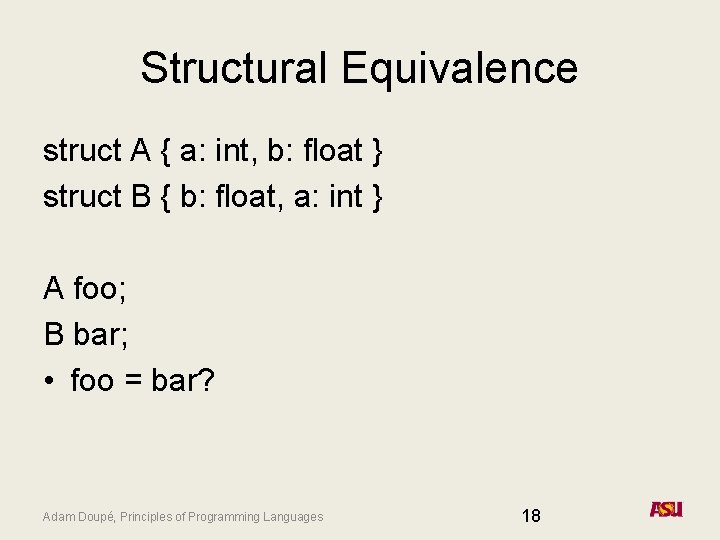
Structural Equivalence struct A { a: int, b: float } struct B { b: float, a: int } A foo; B bar; • foo = bar? Adam Doupé, Principles of Programming Languages 18
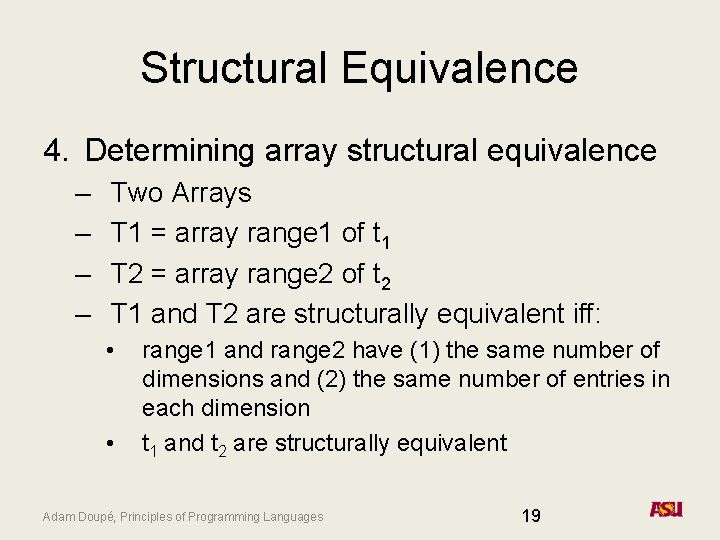
Structural Equivalence 4. Determining array structural equivalence – – Two Arrays T 1 = array range 1 of t 1 T 2 = array range 2 of t 2 T 1 and T 2 are structurally equivalent iff: • • range 1 and range 2 have (1) the same number of dimensions and (2) the same number of entries in each dimension t 1 and t 2 are structurally equivalent Adam Doupé, Principles of Programming Languages 19
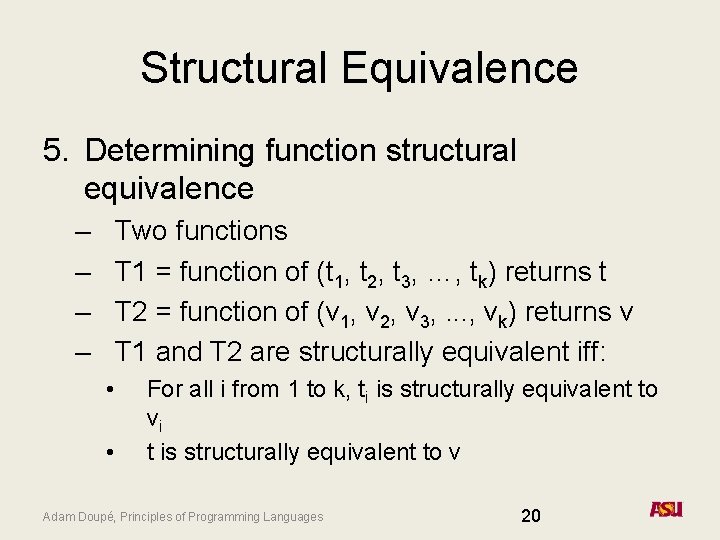
Structural Equivalence 5. Determining function structural equivalence – – Two functions T 1 = function of (t 1, t 2, t 3, …, tk) returns t T 2 = function of (v 1, v 2, v 3, . . . , vk) returns v T 1 and T 2 are structurally equivalent iff: • • For all i from 1 to k, ti is structurally equivalent to vi t is structurally equivalent to v Adam Doupé, Principles of Programming Languages 20
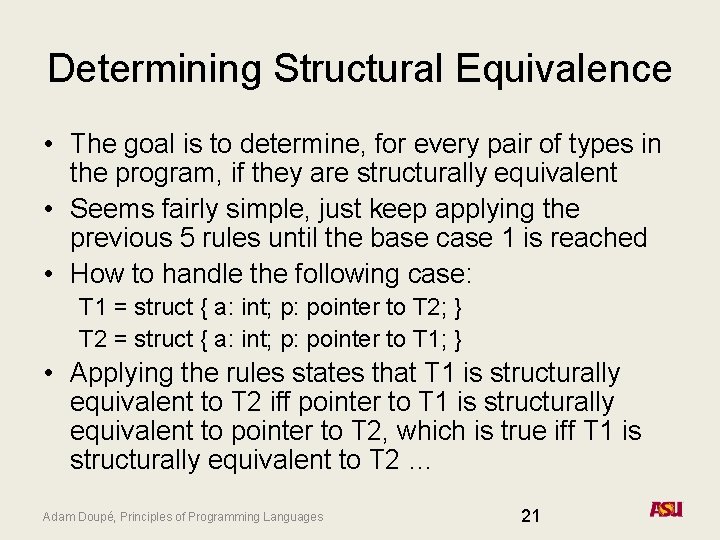
Determining Structural Equivalence • The goal is to determine, for every pair of types in the program, if they are structurally equivalent • Seems fairly simple, just keep applying the previous 5 rules until the base case 1 is reached • How to handle the following case: T 1 = struct { a: int; p: pointer to T 2; } T 2 = struct { a: int; p: pointer to T 1; } • Applying the rules states that T 1 is structurally equivalent to T 2 iff pointer to T 1 is structurally equivalent to pointer to T 2, which is true iff T 1 is structurally equivalent to T 2 … Adam Doupé, Principles of Programming Languages 21
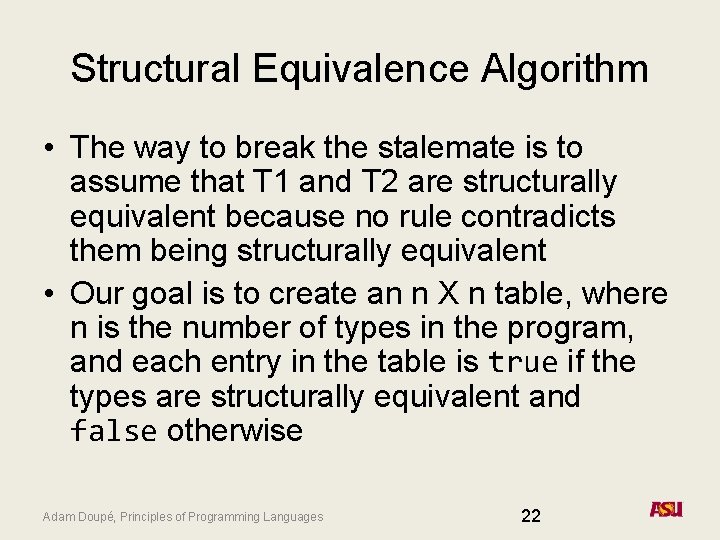
Structural Equivalence Algorithm • The way to break the stalemate is to assume that T 1 and T 2 are structurally equivalent because no rule contradicts them being structurally equivalent • Our goal is to create an n X n table, where n is the number of types in the program, and each entry in the table is true if the types are structurally equivalent and false otherwise Adam Doupé, Principles of Programming Languages 22
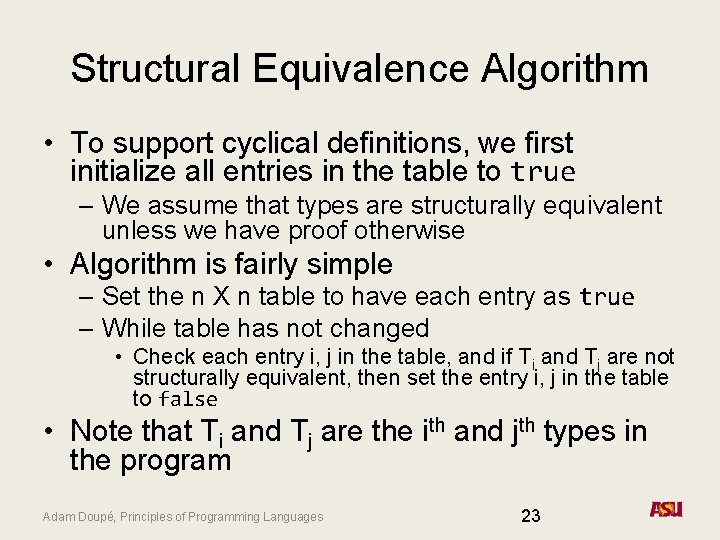
Structural Equivalence Algorithm • To support cyclical definitions, we first initialize all entries in the table to true – We assume that types are structurally equivalent unless we have proof otherwise • Algorithm is fairly simple – Set the n X n table to have each entry as true – While table has not changed • Check each entry i, j in the table, and if Ti and Tj are not structurally equivalent, then set the entry i, j in the table to false • Note that Ti and Tj are the ith and jth types in the program Adam Doupé, Principles of Programming Languages 23
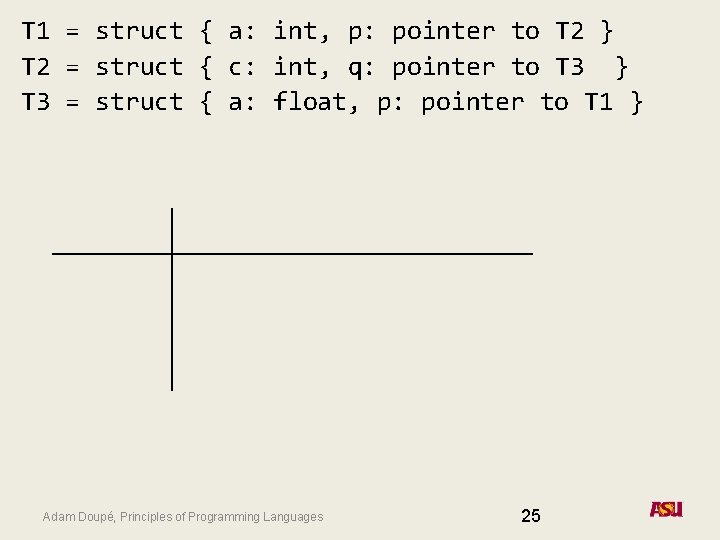
T 1 = struct { a: int, p: pointer to T 2 } T 2 = struct { c: int, q: pointer to T 3 } T 3 = struct { a: float, p: pointer to T 1 } T 1 T 2 T 3 T 1 true T 2 true Adam Doupé, Principles of Programming Languages T 3 true 25
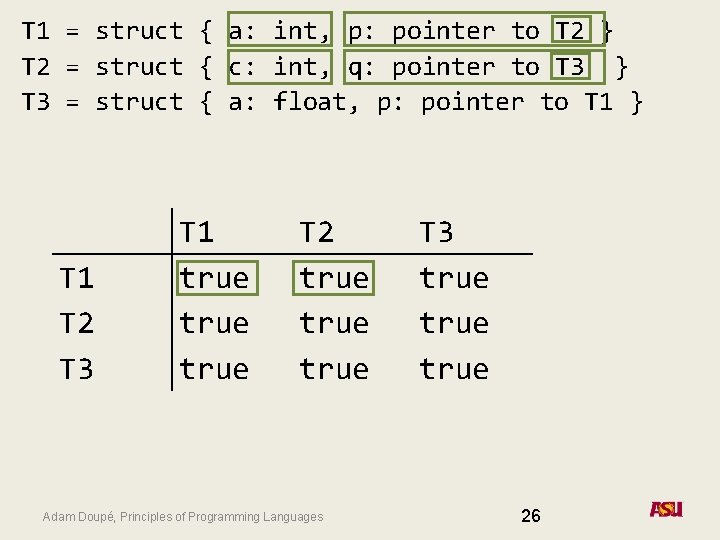
T 1 = struct { a: int, p: pointer to T 2 } T 2 = struct { c: int, q: pointer to T 3 } T 3 = struct { a: float, p: pointer to T 1 } T 1 T 2 T 3 T 1 true T 2 true Adam Doupé, Principles of Programming Languages T 3 true 26
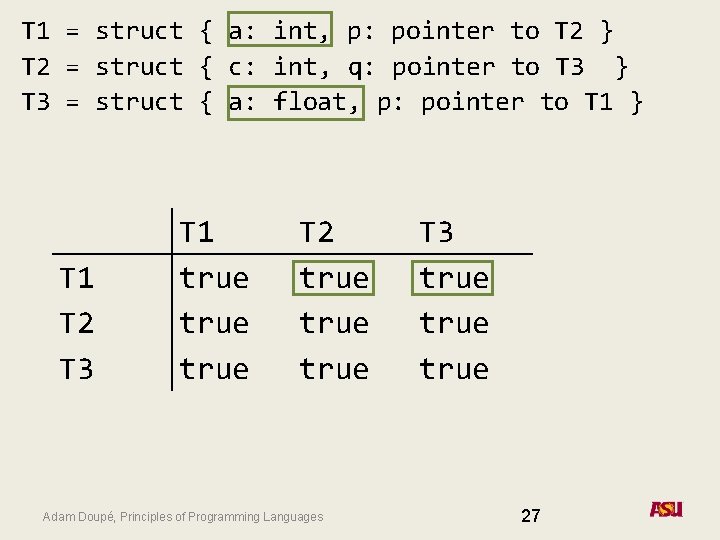
T 1 = struct { a: int, p: pointer to T 2 } T 2 = struct { c: int, q: pointer to T 3 } T 3 = struct { a: float, p: pointer to T 1 } T 1 T 2 T 3 T 1 true T 2 true Adam Doupé, Principles of Programming Languages T 3 true 27
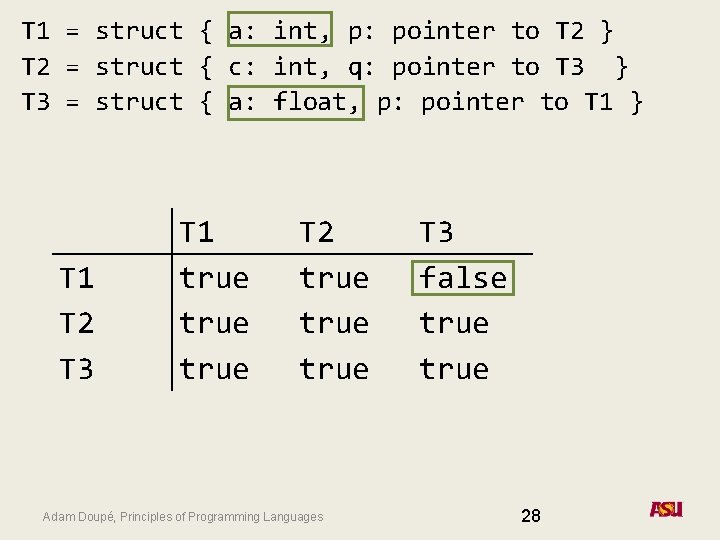
T 1 = struct { a: int, p: pointer to T 2 } T 2 = struct { c: int, q: pointer to T 3 } T 3 = struct { a: float, p: pointer to T 1 } T 1 T 2 T 3 T 1 true T 2 true Adam Doupé, Principles of Programming Languages T 3 false true 28
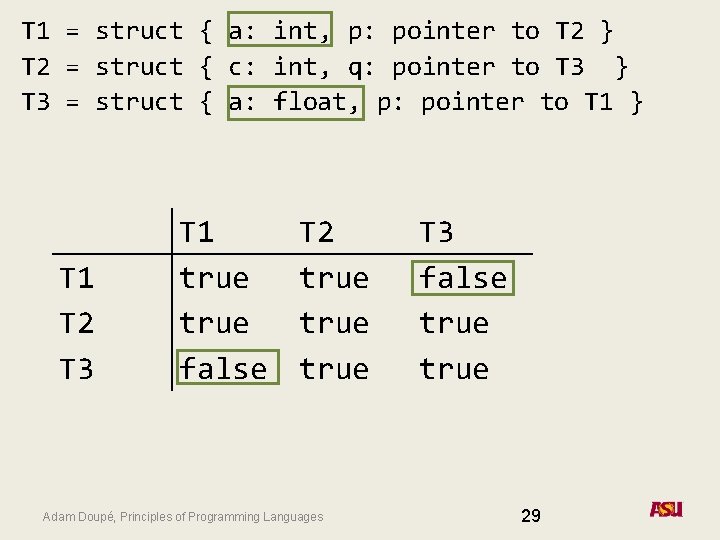
T 1 = struct { a: int, p: pointer to T 2 } T 2 = struct { c: int, q: pointer to T 3 } T 3 = struct { a: float, p: pointer to T 1 } T 1 T 2 T 3 T 1 true false T 2 true Adam Doupé, Principles of Programming Languages T 3 false true 29
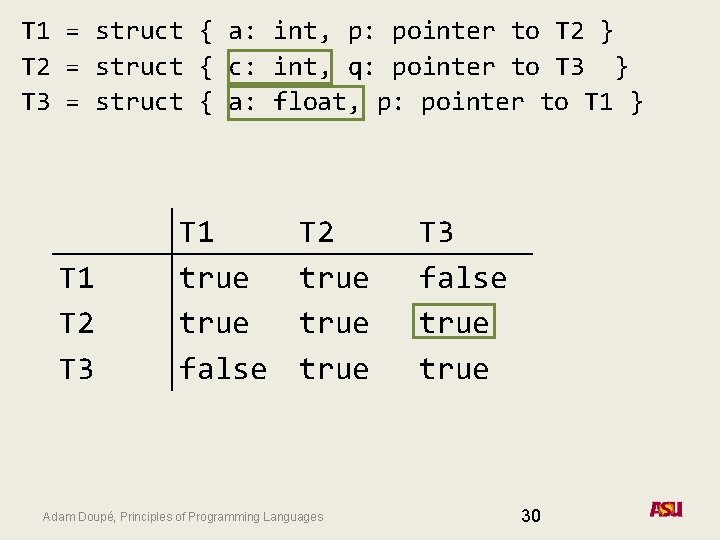
T 1 = struct { a: int, p: pointer to T 2 } T 2 = struct { c: int, q: pointer to T 3 } T 3 = struct { a: float, p: pointer to T 1 } T 1 T 2 T 3 T 1 true false T 2 true Adam Doupé, Principles of Programming Languages T 3 false true 30
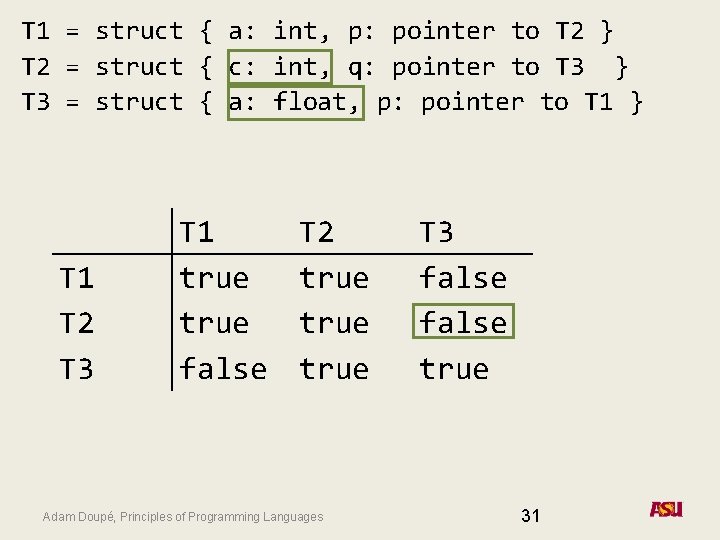
T 1 = struct { a: int, p: pointer to T 2 } T 2 = struct { c: int, q: pointer to T 3 } T 3 = struct { a: float, p: pointer to T 1 } T 1 T 2 T 3 T 1 true false T 2 true Adam Doupé, Principles of Programming Languages T 3 false true 31
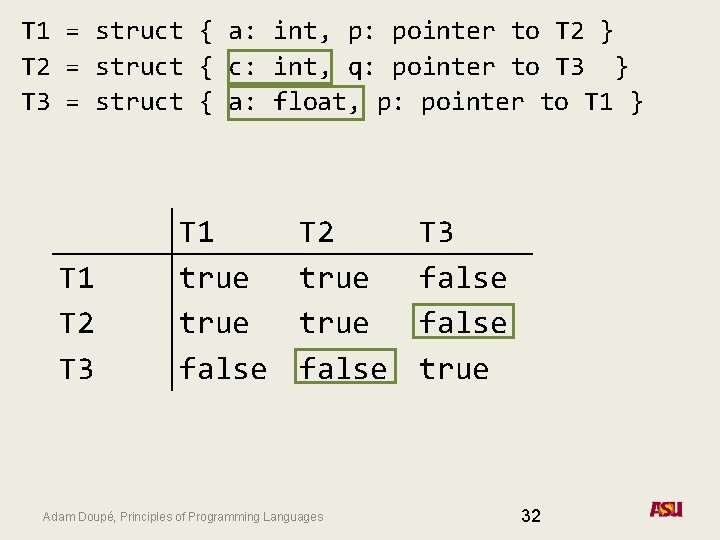
T 1 = struct { a: int, p: pointer to T 2 } T 2 = struct { c: int, q: pointer to T 3 } T 3 = struct { a: float, p: pointer to T 1 } T 1 T 2 T 3 T 1 true false T 2 true false Adam Doupé, Principles of Programming Languages T 3 false true 32
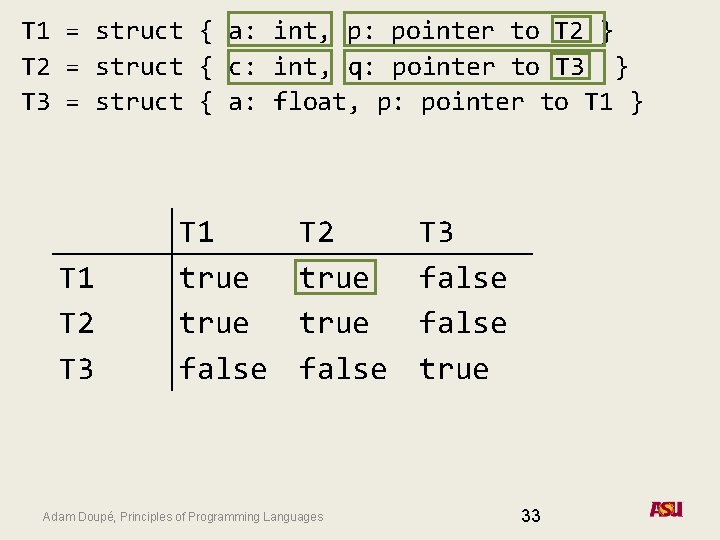
T 1 = struct { a: int, p: pointer to T 2 } T 2 = struct { c: int, q: pointer to T 3 } T 3 = struct { a: float, p: pointer to T 1 } T 1 T 2 T 3 T 1 true false T 2 true false Adam Doupé, Principles of Programming Languages T 3 false true 33
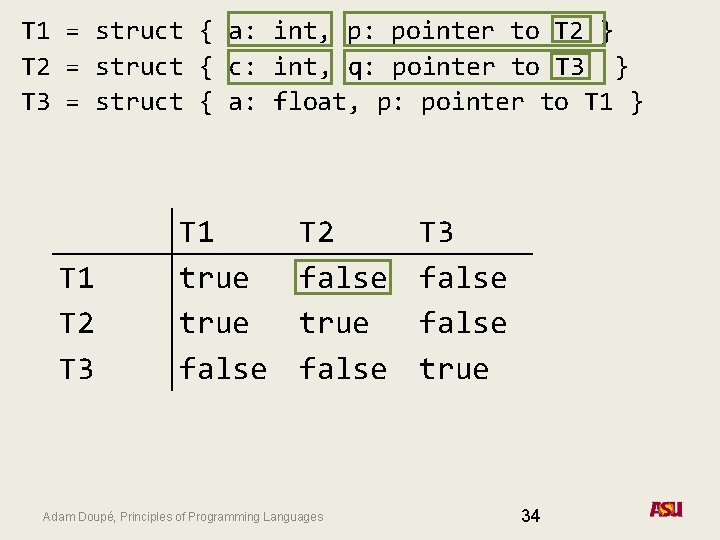
T 1 = struct { a: int, p: pointer to T 2 } T 2 = struct { c: int, q: pointer to T 3 } T 3 = struct { a: float, p: pointer to T 1 } T 1 T 2 T 3 T 1 true false T 2 false true false Adam Doupé, Principles of Programming Languages T 3 false true 34
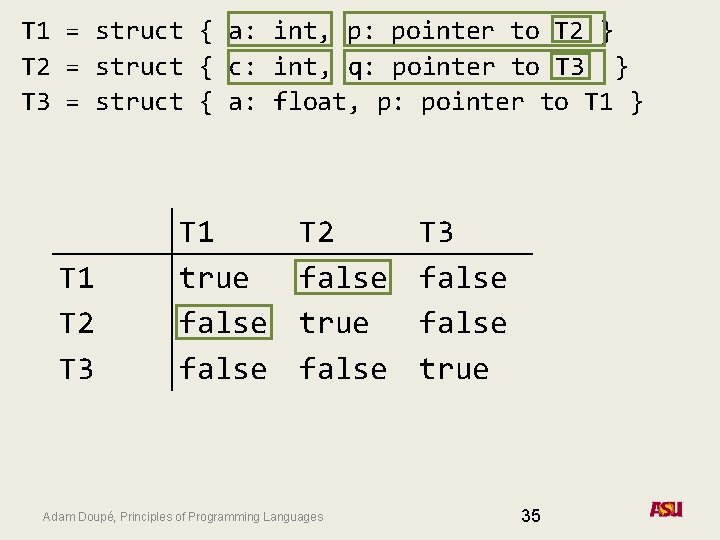
T 1 = struct { a: int, p: pointer to T 2 } T 2 = struct { c: int, q: pointer to T 3 } T 3 = struct { a: float, p: pointer to T 1 } T 1 T 2 T 3 T 1 true false T 2 false true false Adam Doupé, Principles of Programming Languages T 3 false true 35
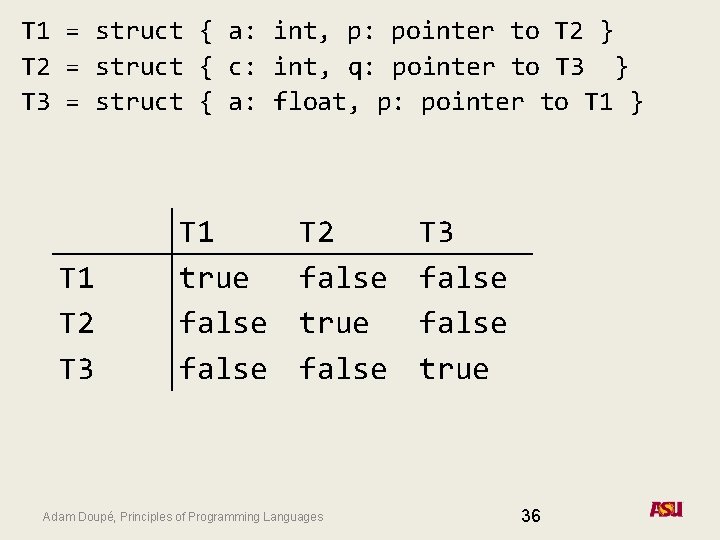
T 1 = struct { a: int, p: pointer to T 2 } T 2 = struct { c: int, q: pointer to T 3 } T 3 = struct { a: float, p: pointer to T 1 } T 1 T 2 T 3 T 1 true false T 2 false true false Adam Doupé, Principles of Programming Languages T 3 false true 36
Cse 340 principles of programming languages
Cse 340 principles of programming languages
Cse 340 asu
Cse 340 project 1
Adam doupe cse 340
Adam doupe cse 340
Tiny programming language
Real-time systems and programming languages
Real-time systems and programming languages
Expert systems: principles and programming, fourth edition
Elsa gunter uiuc
Real time example of multithreading in java
Programming languages levels
Introduction to programming languages
Plc
Joey paquet
Comparative programming languages
Alternative programming languages
Strongly typed vs weakly typed
Transmission programming languages
Integral data type example
Xenia programming languages
Advantages and disadvantages of programming languages
Mainstream programming languages
Programing languages
Programming languages
Programming languages
Programming languages
Brief history of programming languages
Lisp_q
Programming xkcd
If programming languages were cars
Reasons for studying concepts of programming languages
Cornell programming languages
Low level programming languages
Middle level programming languages