Textual Data Many computer applications manipulate textual data
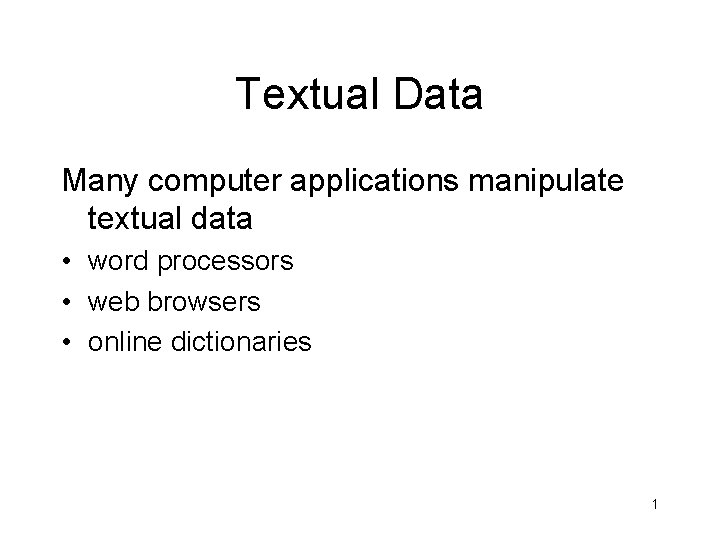
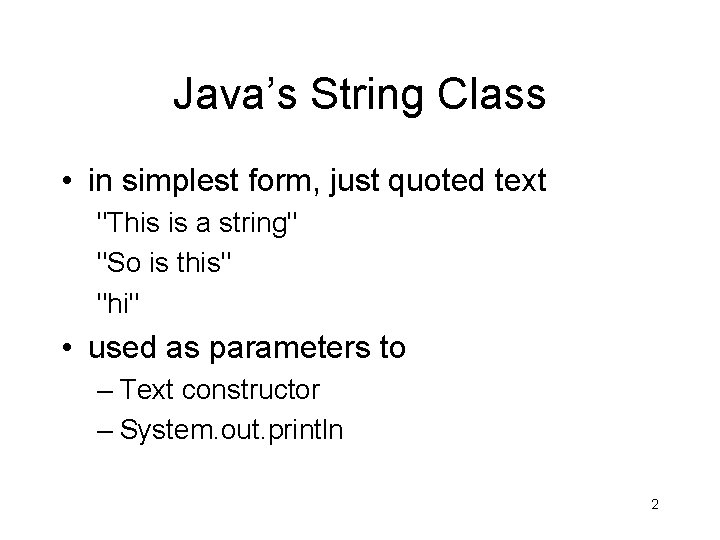
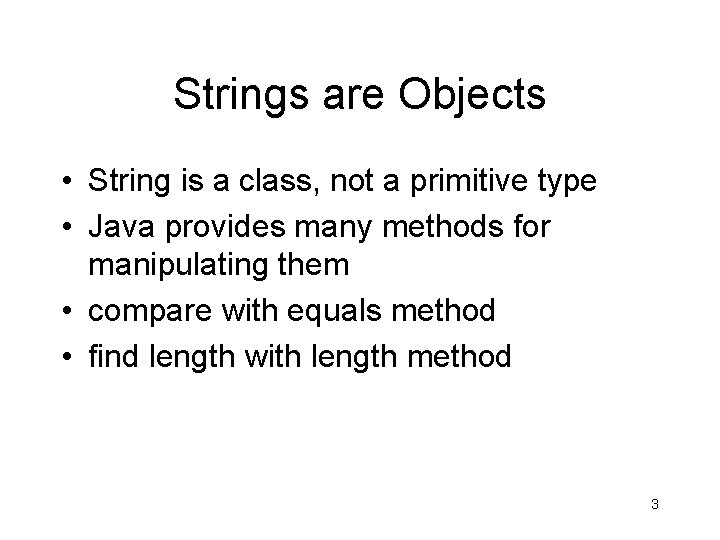
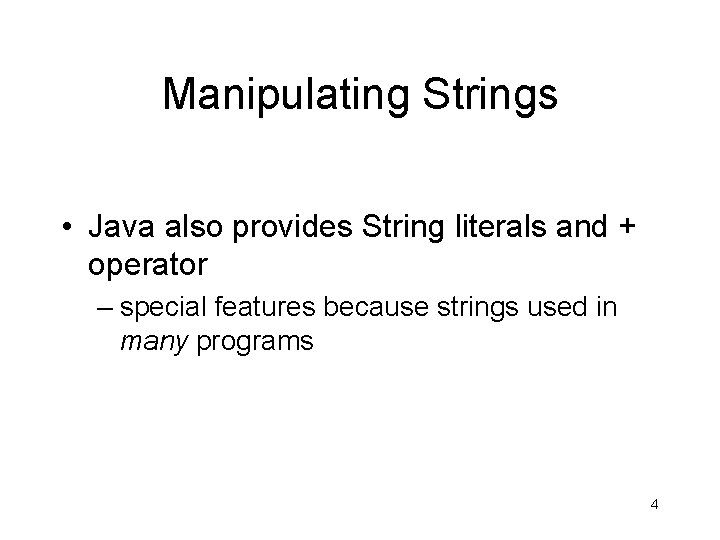
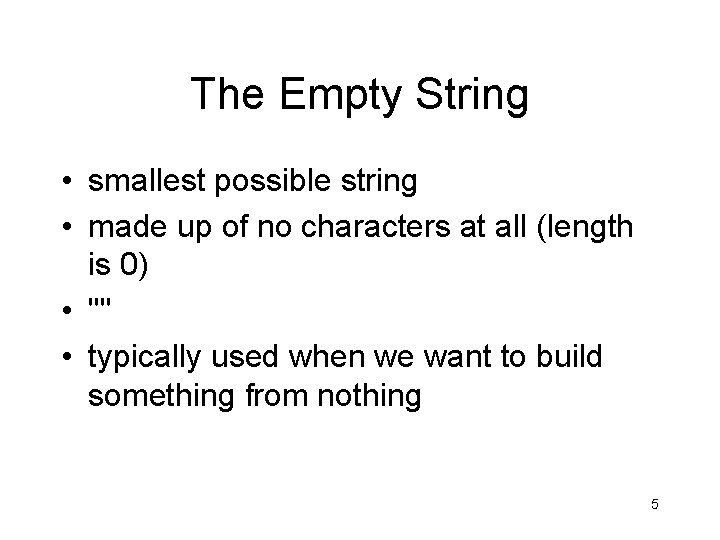
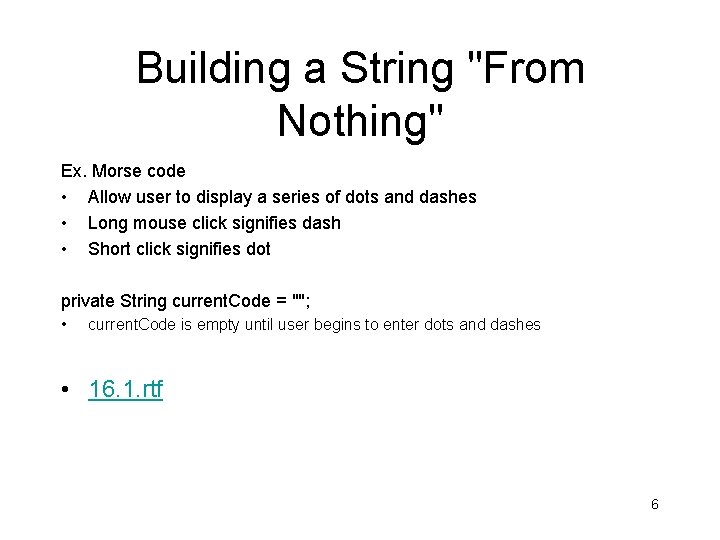
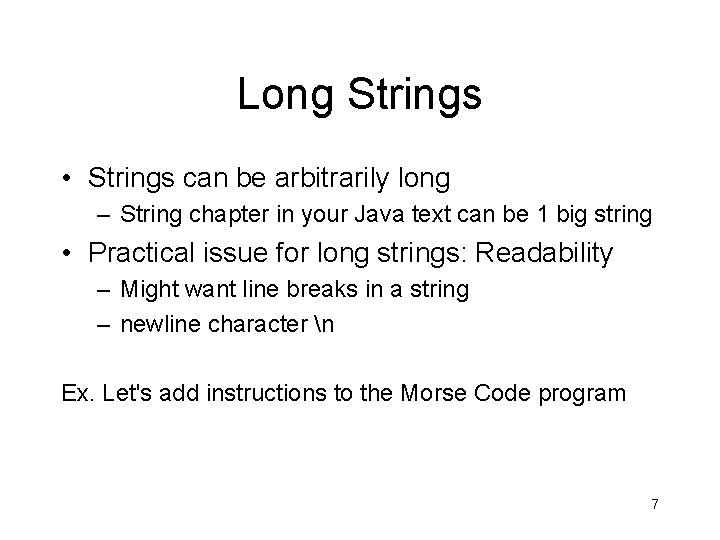
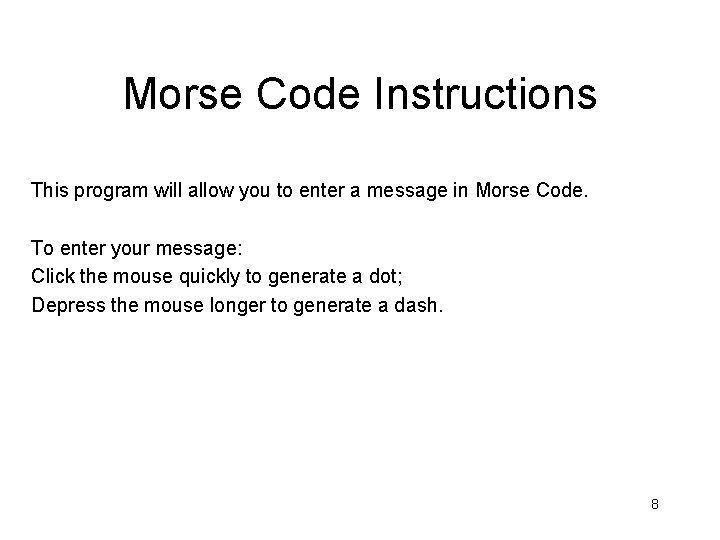
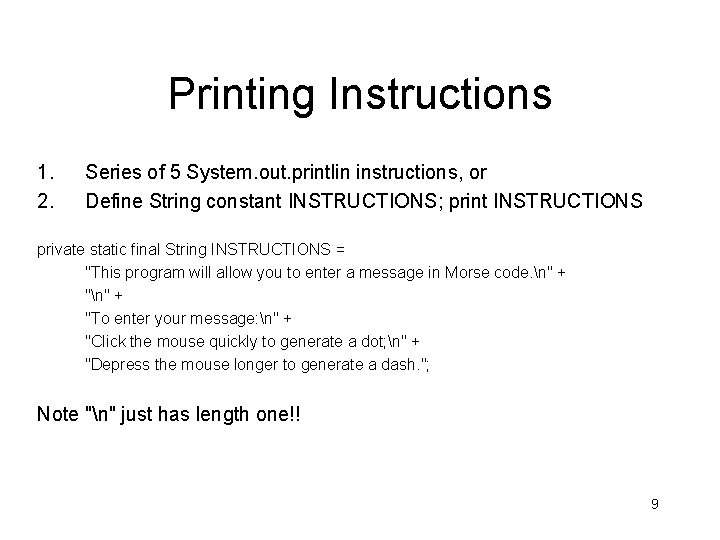
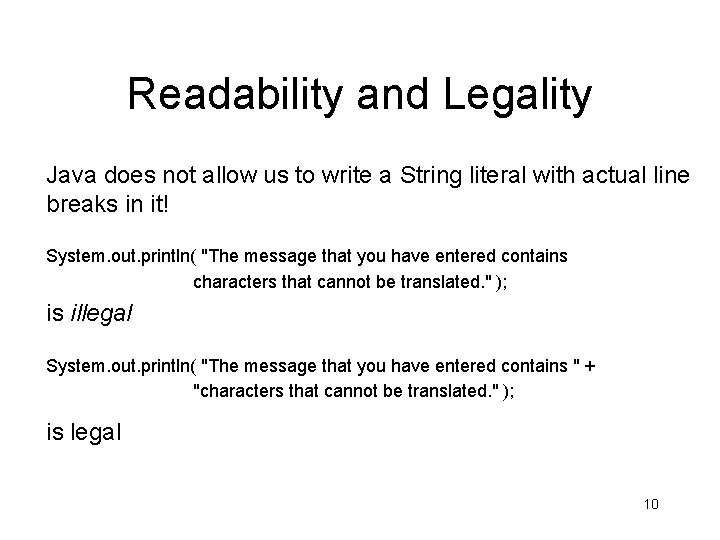
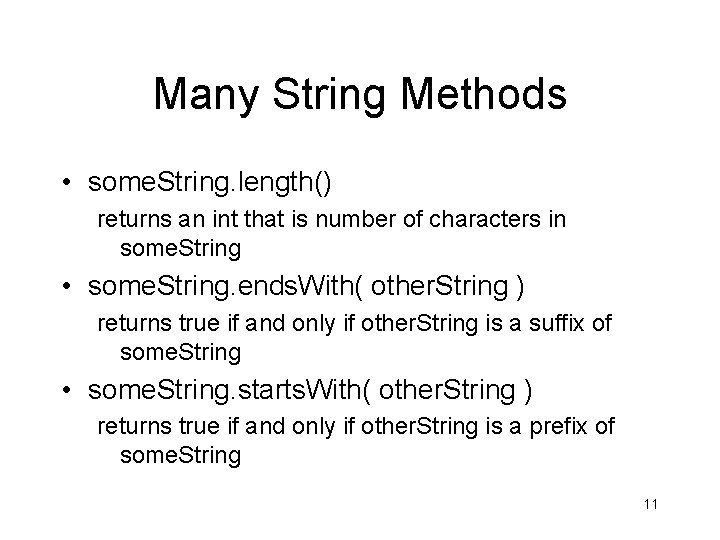
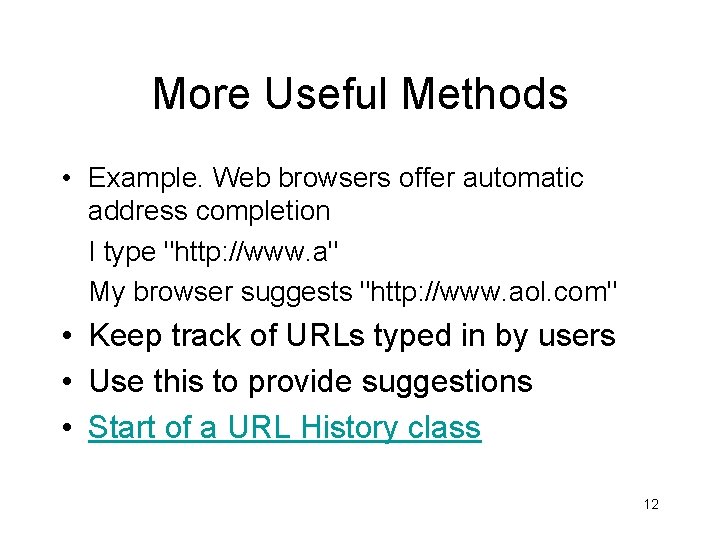
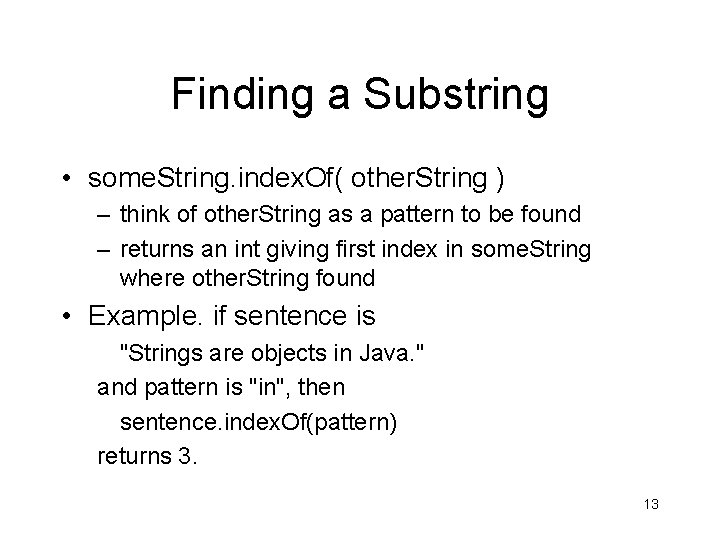
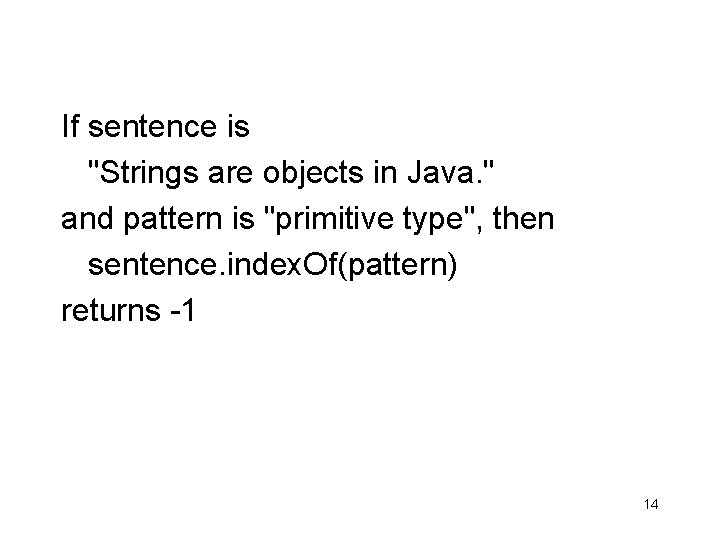
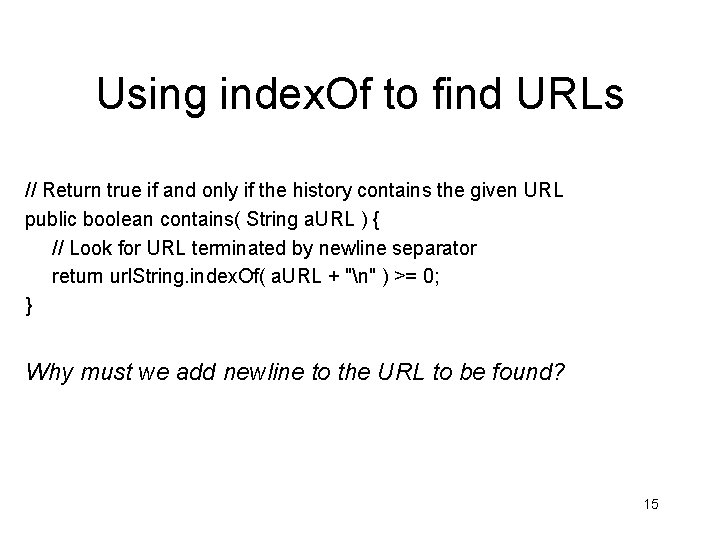
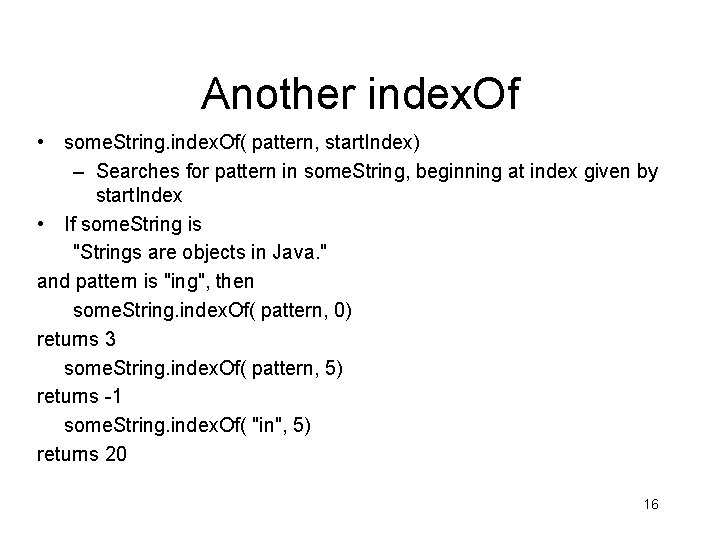
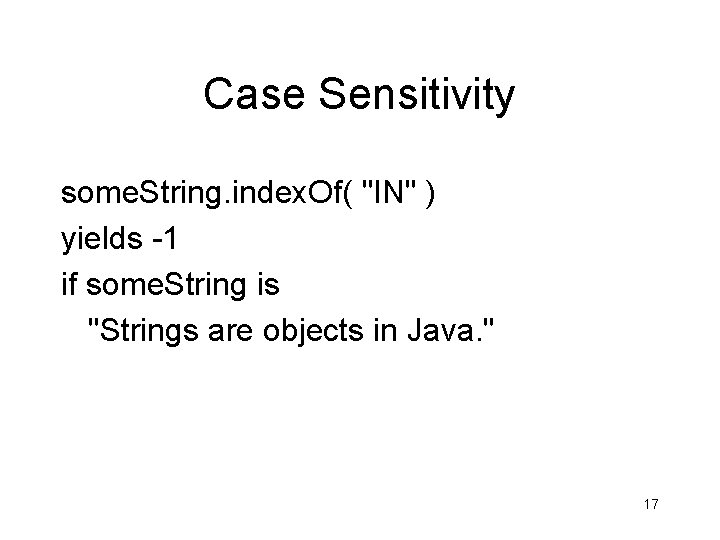
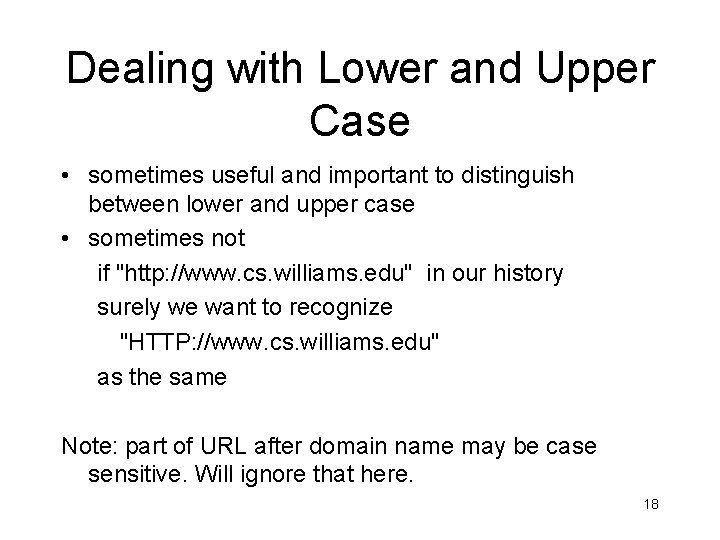
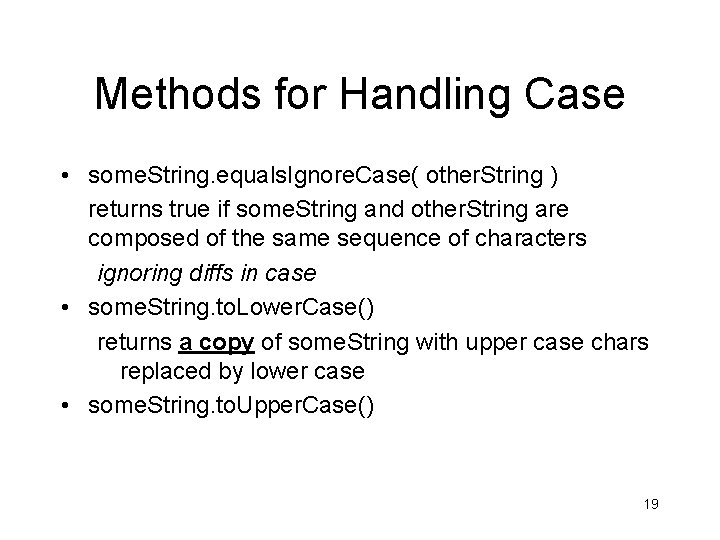
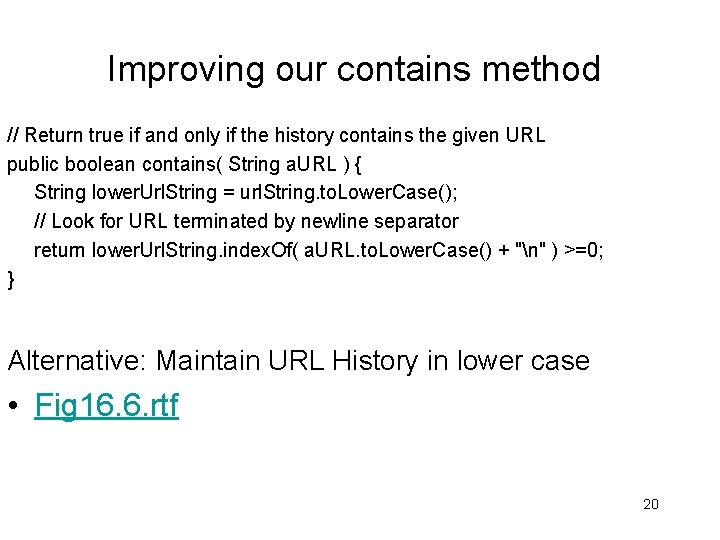
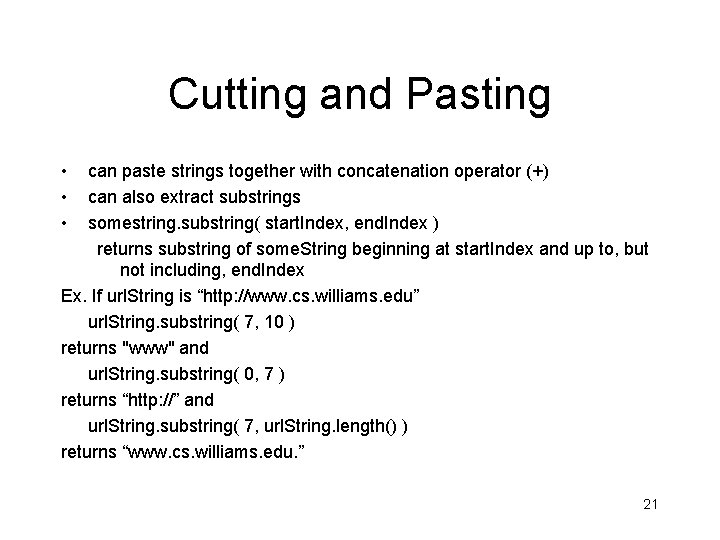
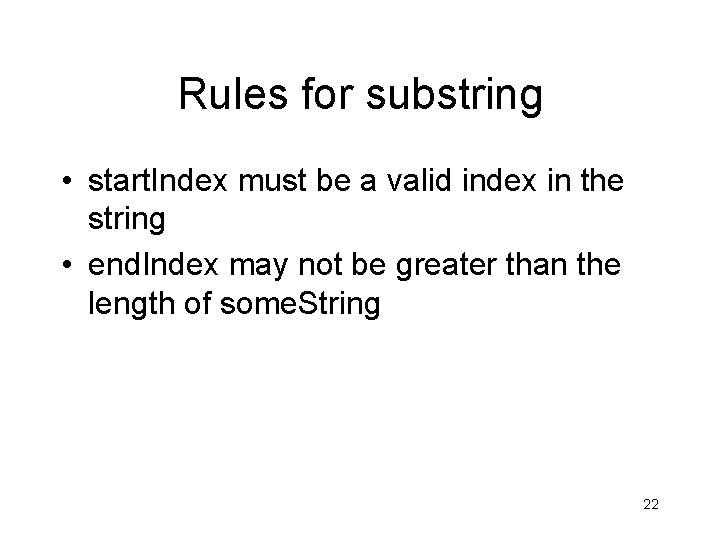
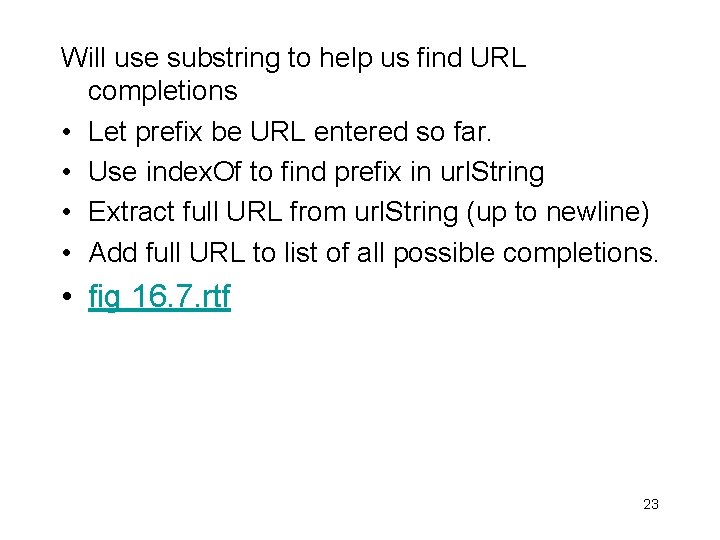
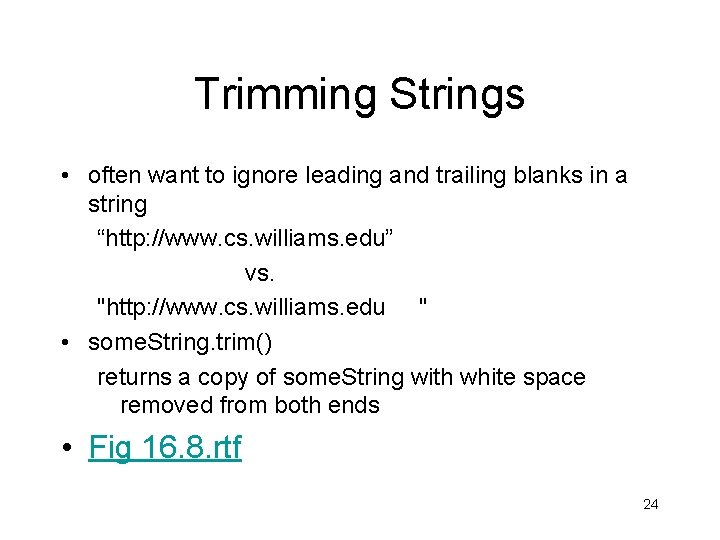
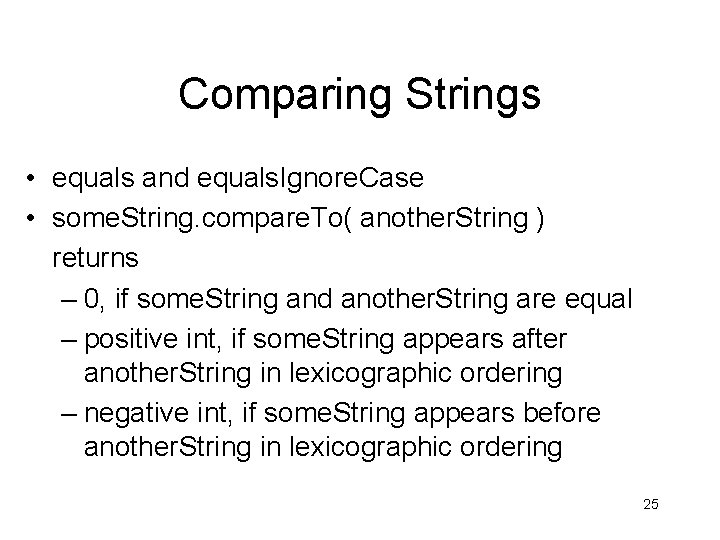
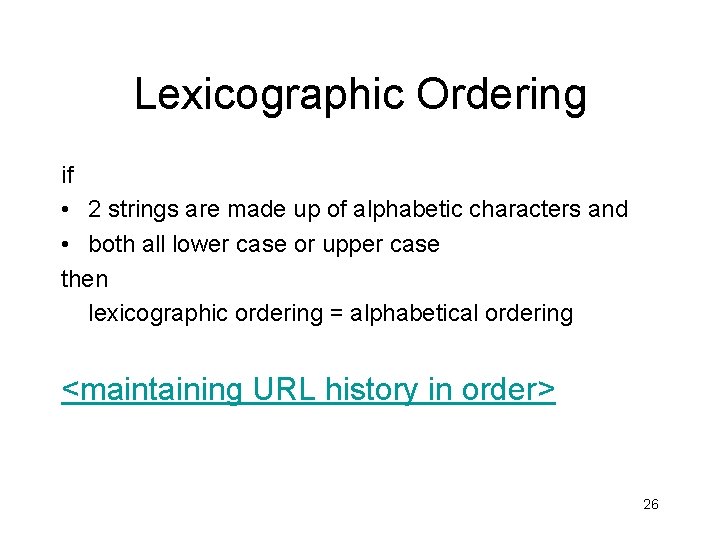
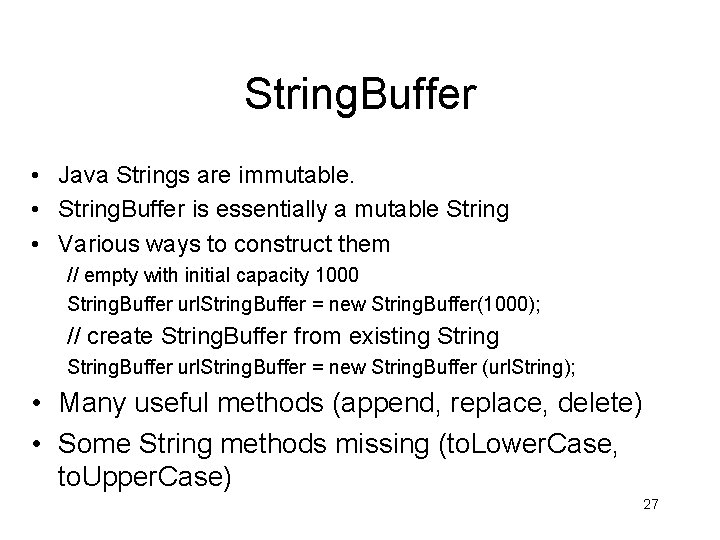
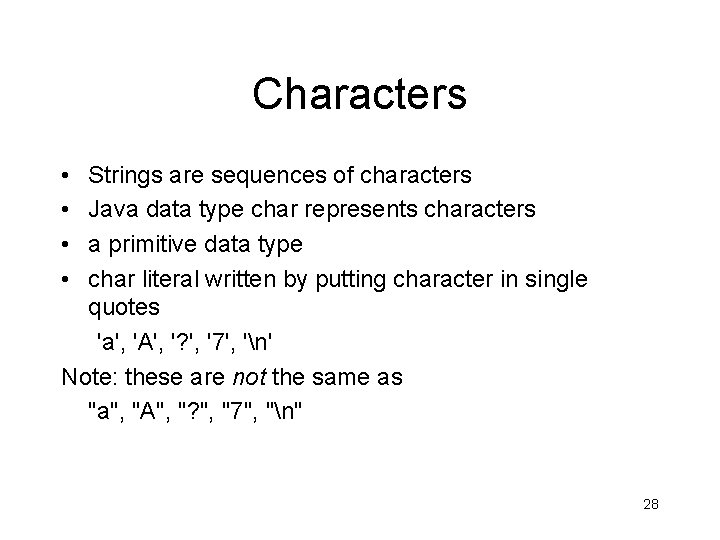
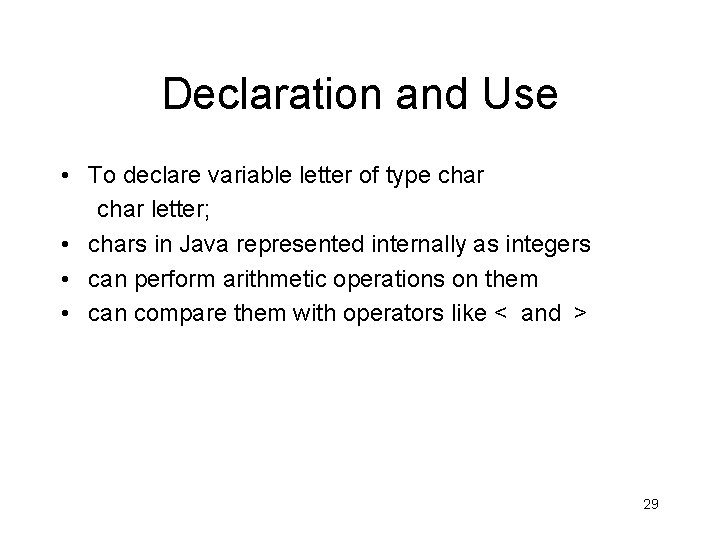
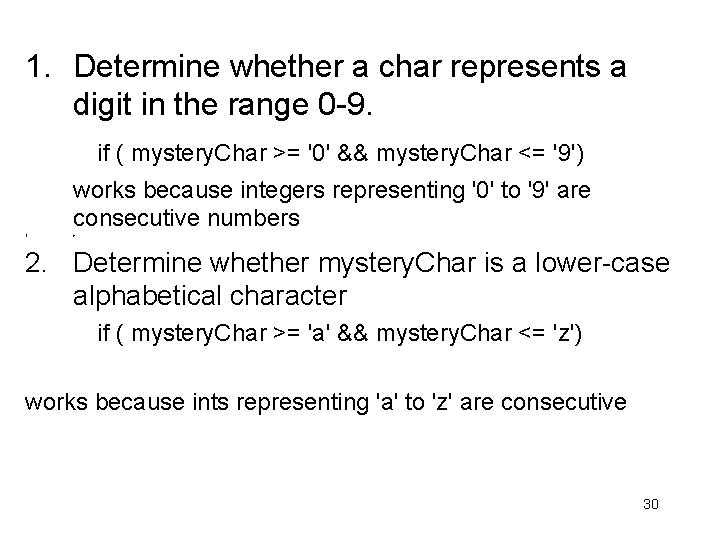
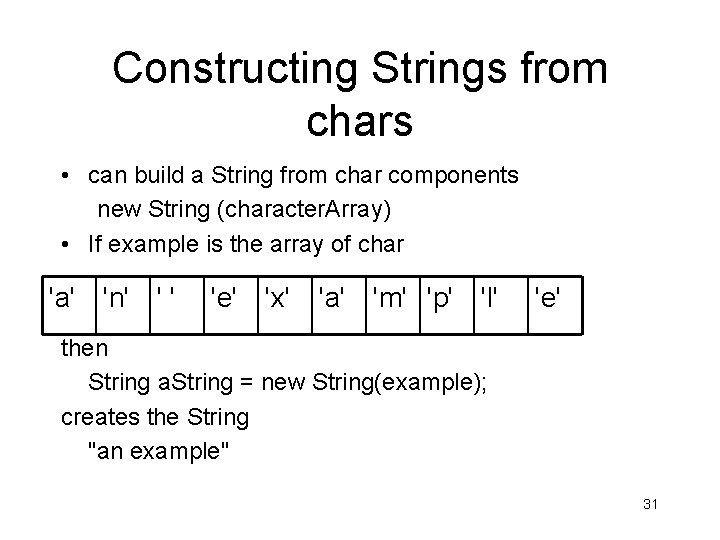
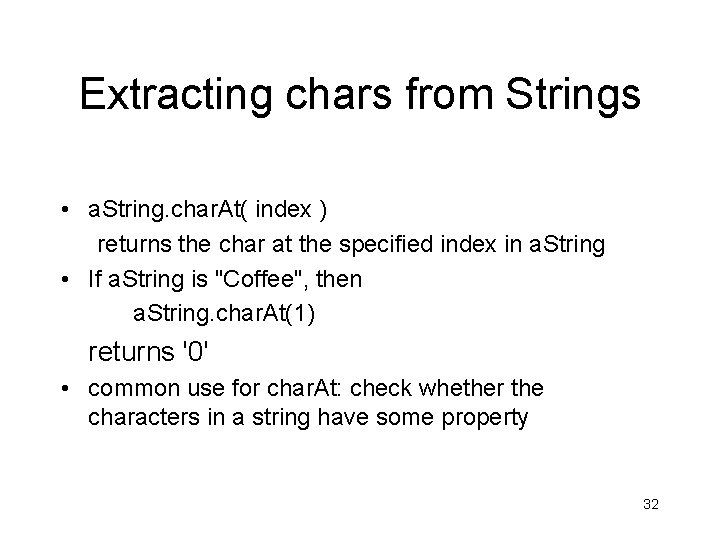
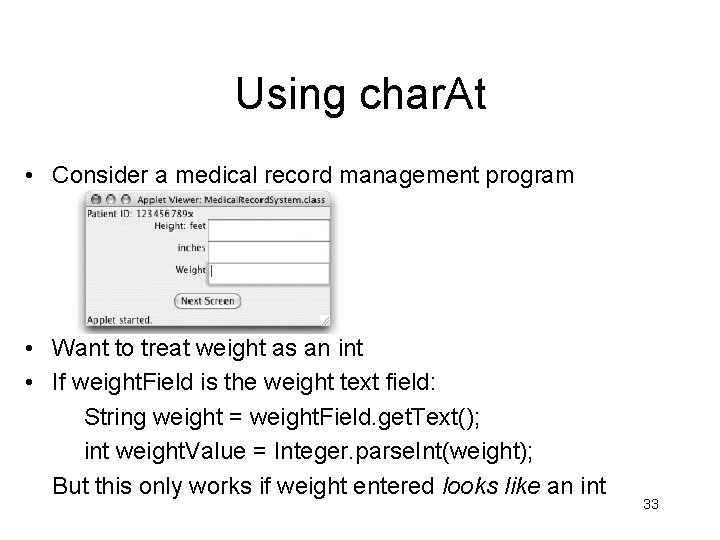
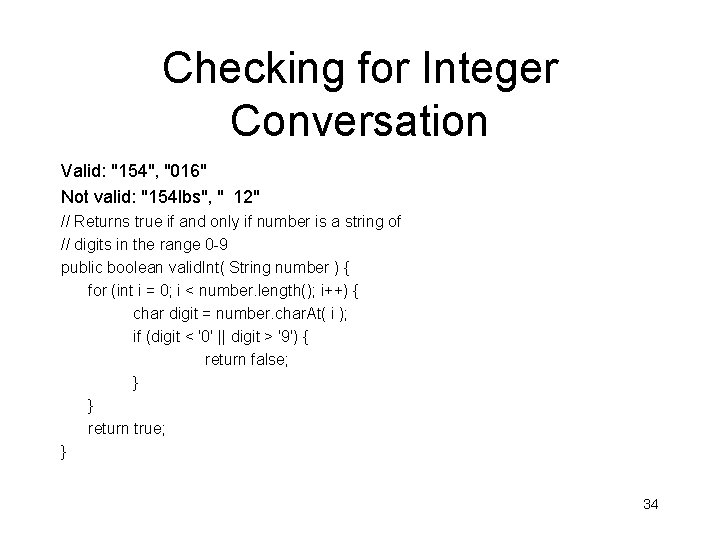
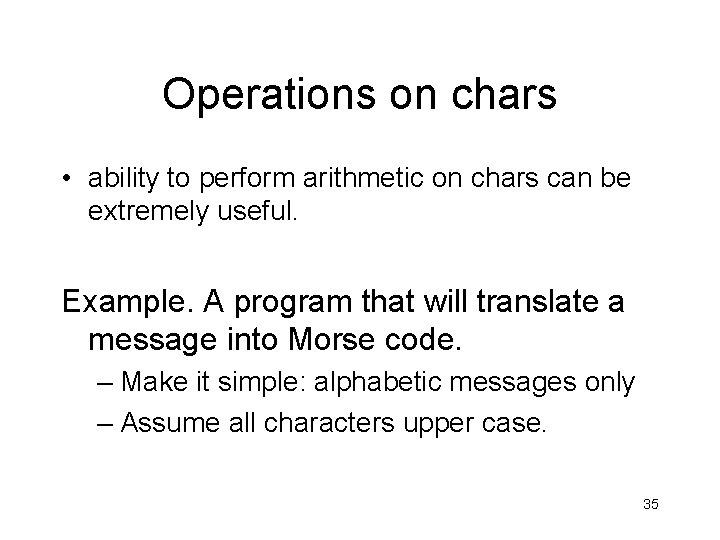
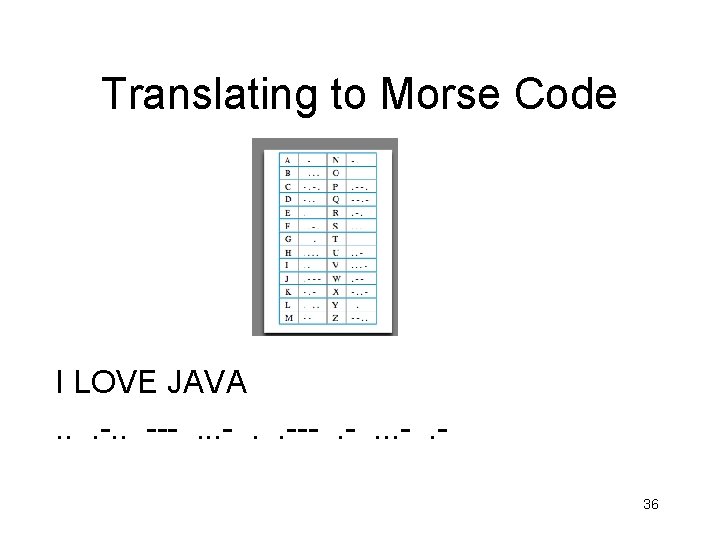
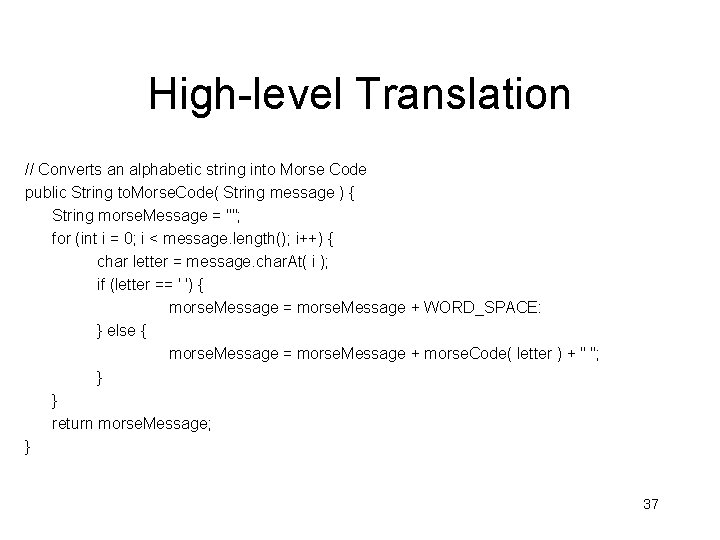
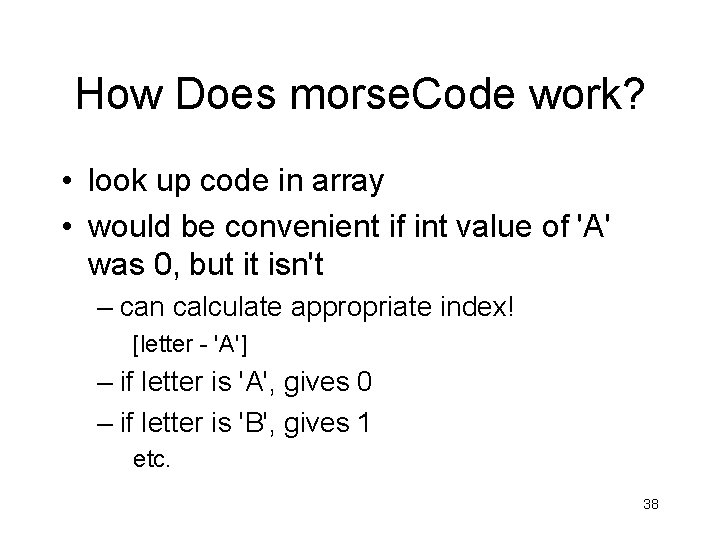
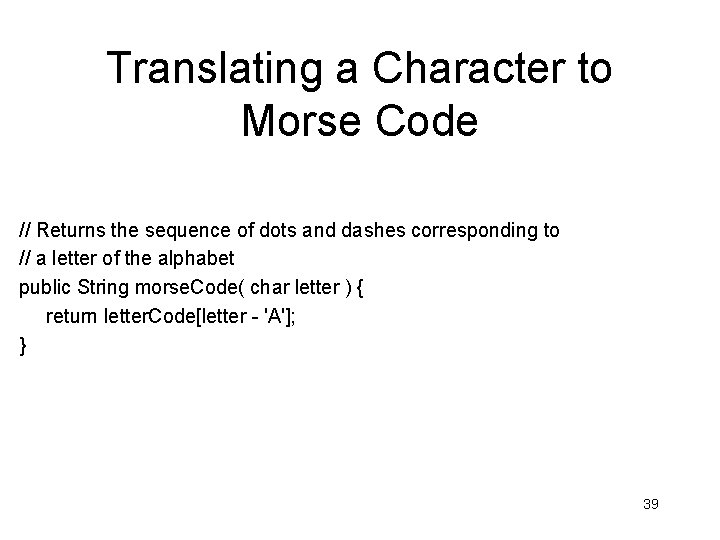
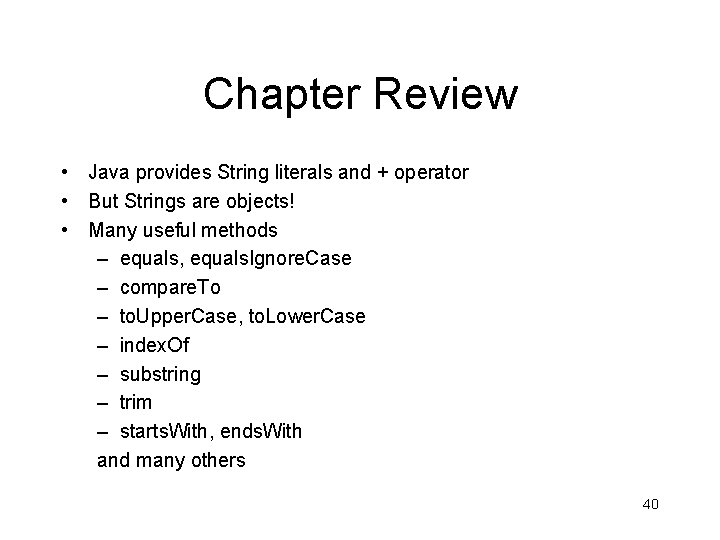
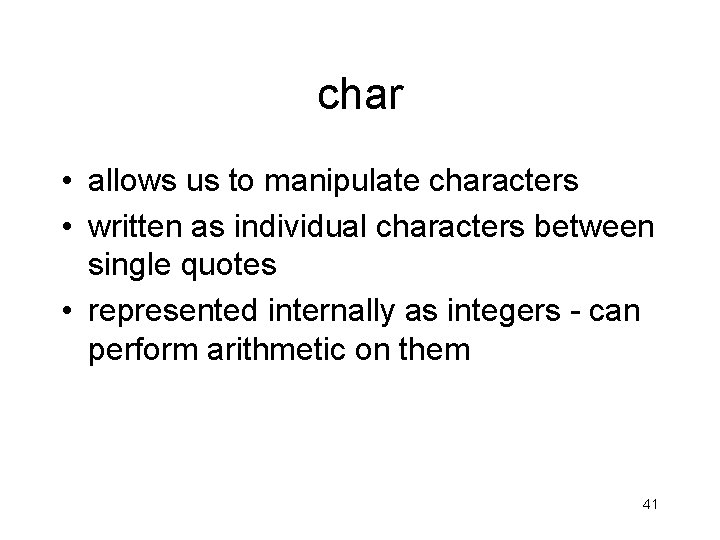
- Slides: 41
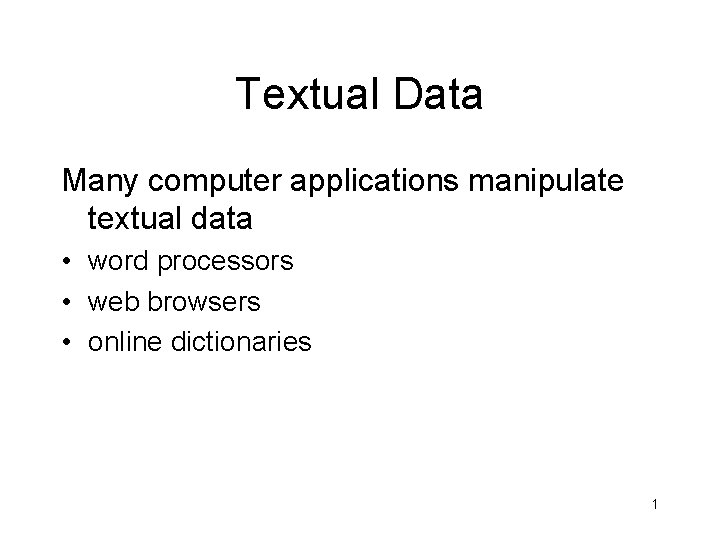
Textual Data Many computer applications manipulate textual data • word processors • web browsers • online dictionaries 1
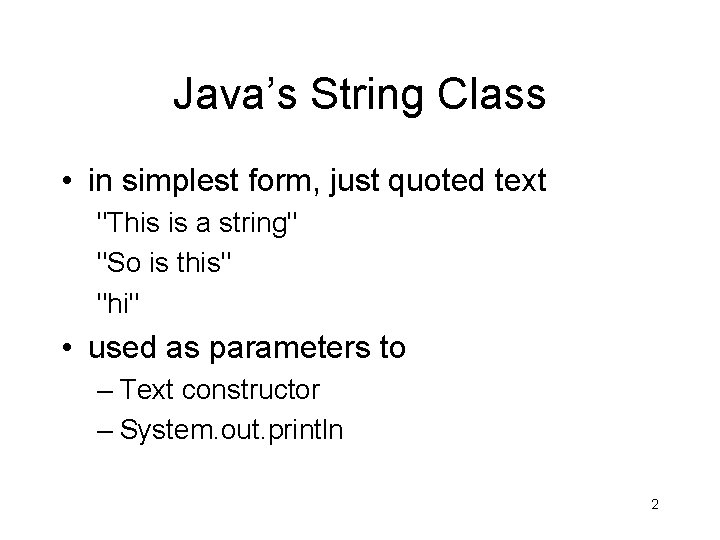
Java’s String Class • in simplest form, just quoted text "This is a string" "So is this" "hi" • used as parameters to – Text constructor – System. out. println 2
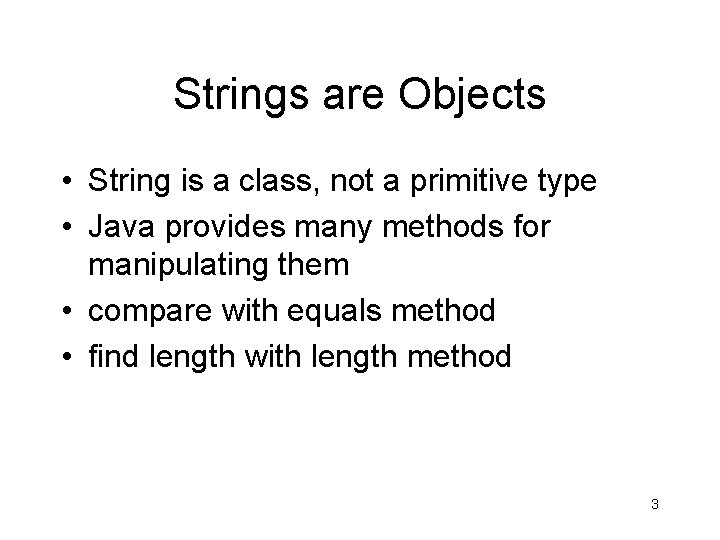
Strings are Objects • String is a class, not a primitive type • Java provides many methods for manipulating them • compare with equals method • find length with length method 3
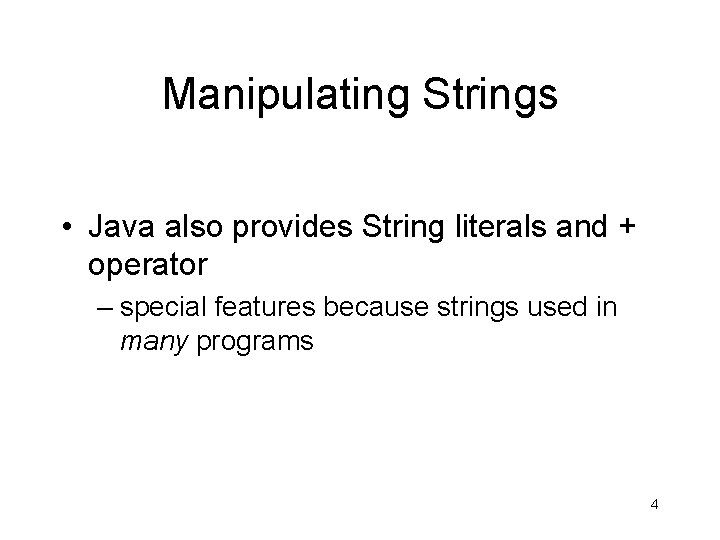
Manipulating Strings • Java also provides String literals and + operator – special features because strings used in many programs 4
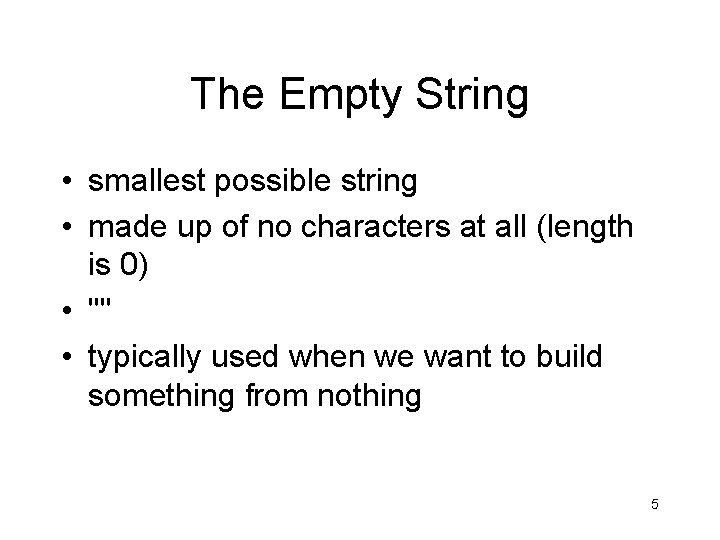
The Empty String • smallest possible string • made up of no characters at all (length is 0) • "" • typically used when we want to build something from nothing 5
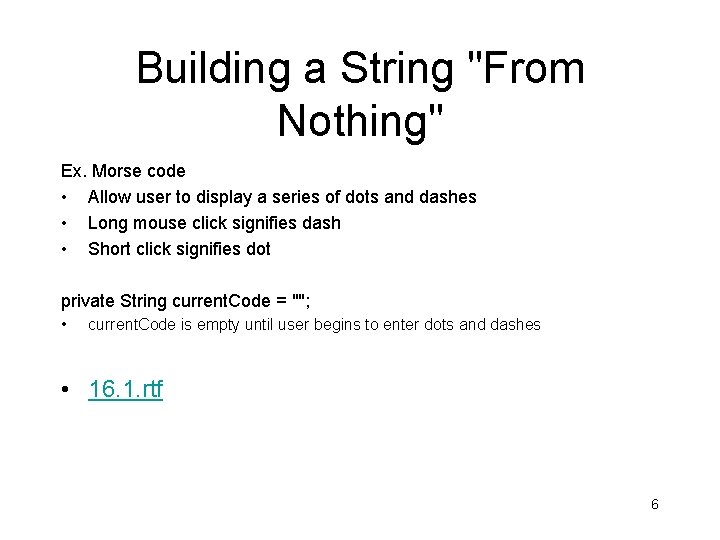
Building a String "From Nothing" Ex. Morse code • Allow user to display a series of dots and dashes • Long mouse click signifies dash • Short click signifies dot private String current. Code = ""; • current. Code is empty until user begins to enter dots and dashes • 16. 1. rtf 6
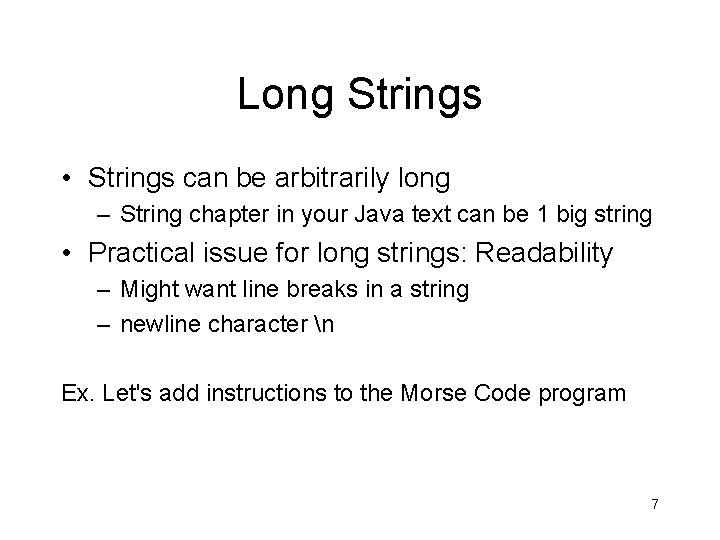
Long Strings • Strings can be arbitrarily long – String chapter in your Java text can be 1 big string • Practical issue for long strings: Readability – Might want line breaks in a string – newline character n Ex. Let's add instructions to the Morse Code program 7
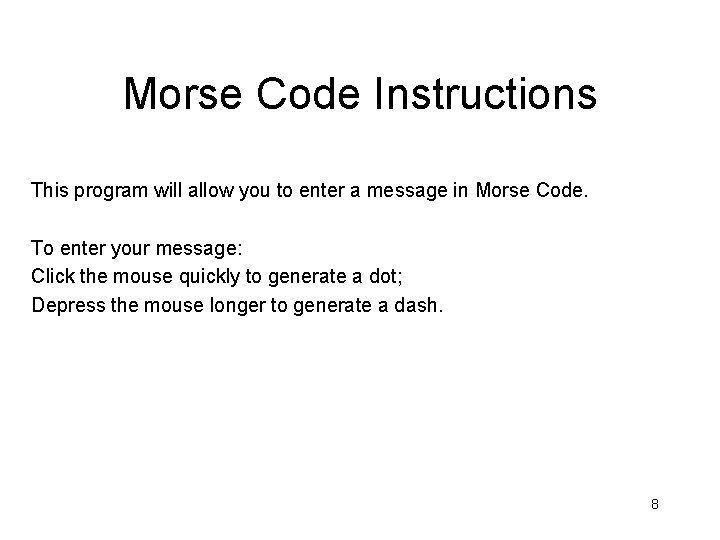
Morse Code Instructions This program will allow you to enter a message in Morse Code. To enter your message: Click the mouse quickly to generate a dot; Depress the mouse longer to generate a dash. 8
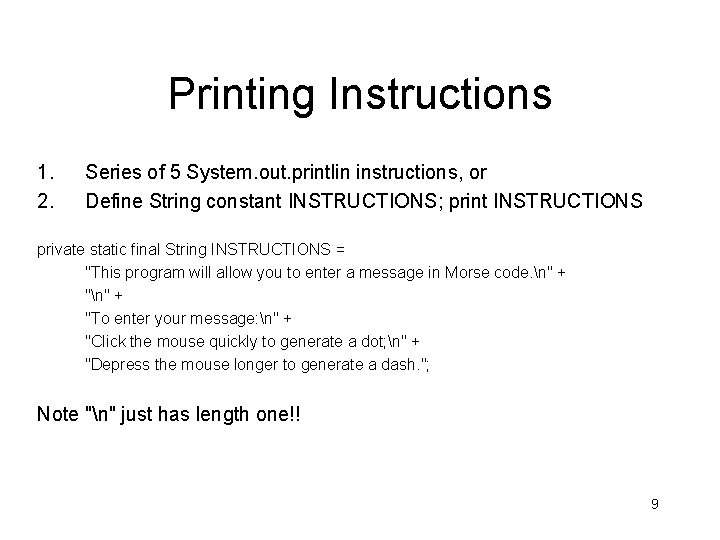
Printing Instructions 1. 2. Series of 5 System. out. printlin instructions, or Define String constant INSTRUCTIONS; print INSTRUCTIONS private static final String INSTRUCTIONS = "This program will allow you to enter a message in Morse code. n" + "To enter your message: n" + "Click the mouse quickly to generate a dot; n" + "Depress the mouse longer to generate a dash. "; Note "n" just has length one!! 9
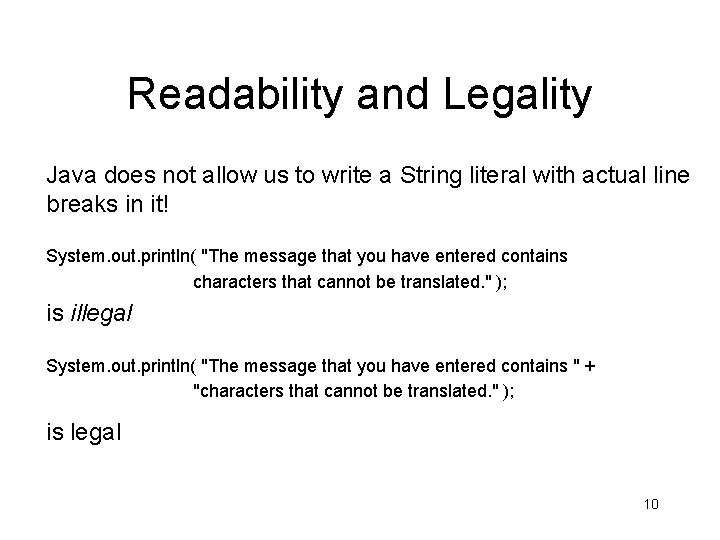
Readability and Legality Java does not allow us to write a String literal with actual line breaks in it! System. out. println( "The message that you have entered contains characters that cannot be translated. " ); is illegal System. out. println( "The message that you have entered contains " + "characters that cannot be translated. " ); is legal 10
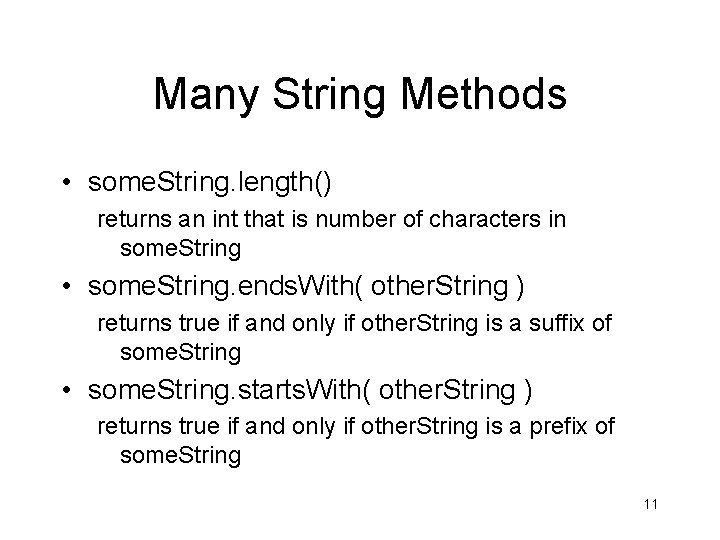
Many String Methods • some. String. length() returns an int that is number of characters in some. String • some. String. ends. With( other. String ) returns true if and only if other. String is a suffix of some. String • some. String. starts. With( other. String ) returns true if and only if other. String is a prefix of some. String 11
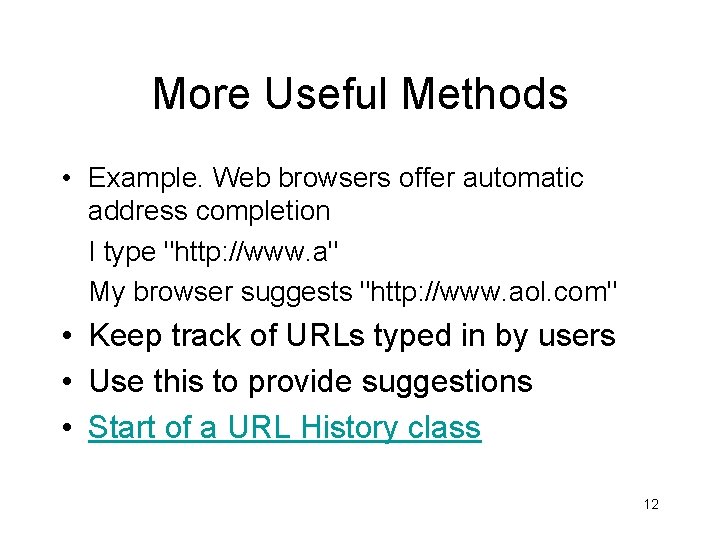
More Useful Methods • Example. Web browsers offer automatic address completion I type "http: //www. a" My browser suggests "http: //www. aol. com" • Keep track of URLs typed in by users • Use this to provide suggestions • Start of a URL History class 12
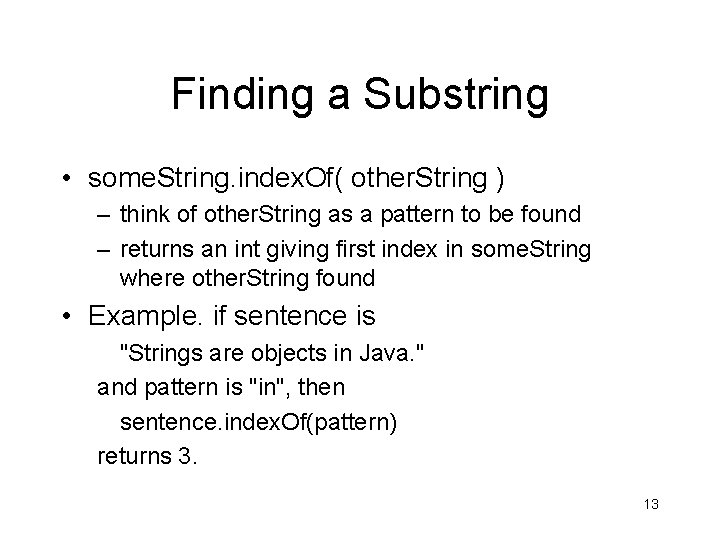
Finding a Substring • some. String. index. Of( other. String ) – think of other. String as a pattern to be found – returns an int giving first index in some. String where other. String found • Example. if sentence is "Strings are objects in Java. " and pattern is "in", then sentence. index. Of(pattern) returns 3. 13
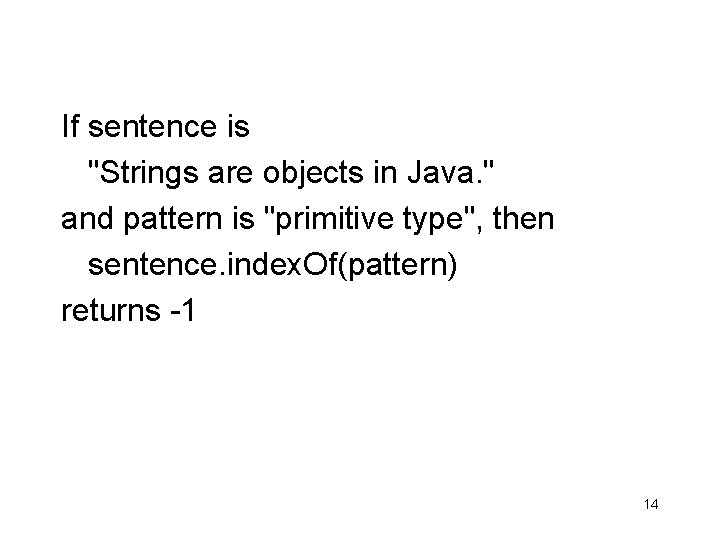
If sentence is "Strings are objects in Java. " and pattern is "primitive type", then sentence. index. Of(pattern) returns -1 14
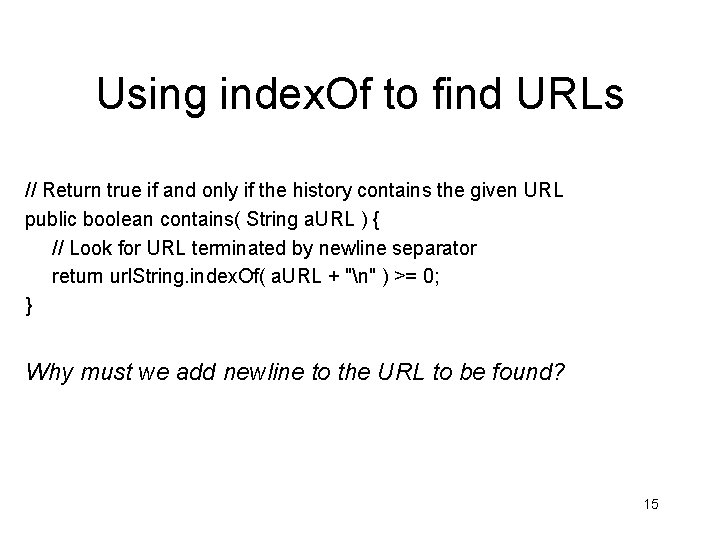
Using index. Of to find URLs // Return true if and only if the history contains the given URL public boolean contains( String a. URL ) { // Look for URL terminated by newline separator return url. String. index. Of( a. URL + "n" ) >= 0; } Why must we add newline to the URL to be found? 15
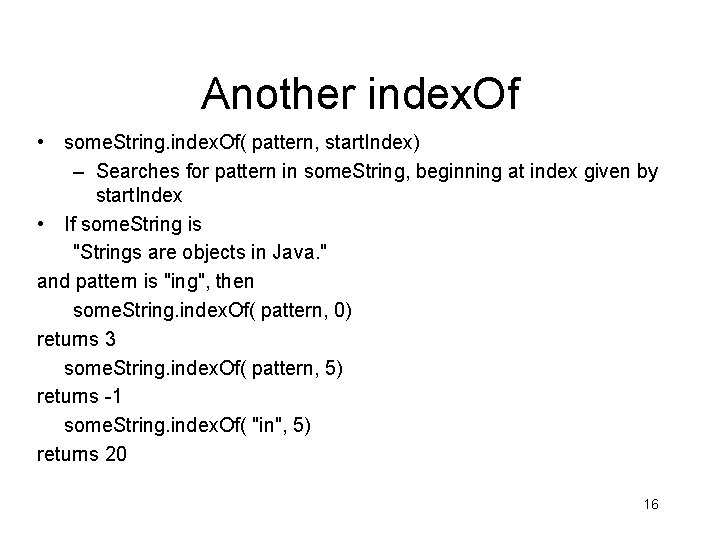
Another index. Of • some. String. index. Of( pattern, start. Index) – Searches for pattern in some. String, beginning at index given by start. Index • If some. String is "Strings are objects in Java. " and pattern is "ing", then some. String. index. Of( pattern, 0) returns 3 some. String. index. Of( pattern, 5) returns -1 some. String. index. Of( "in", 5) returns 20 16
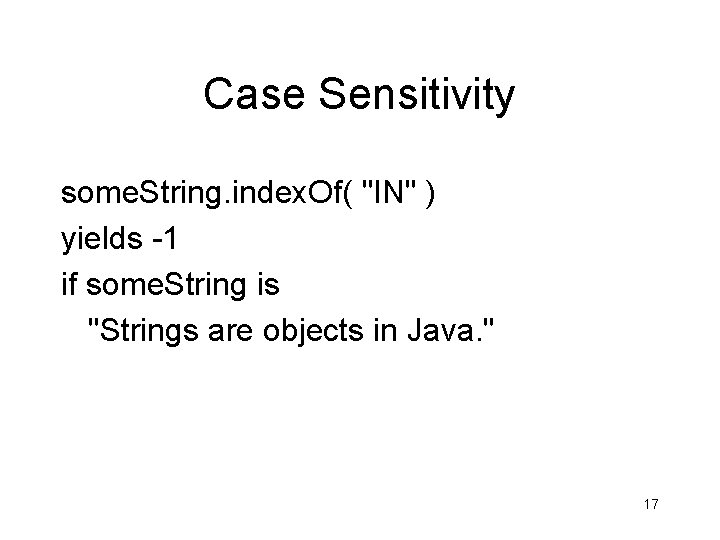
Case Sensitivity some. String. index. Of( "IN" ) yields -1 if some. String is "Strings are objects in Java. " 17
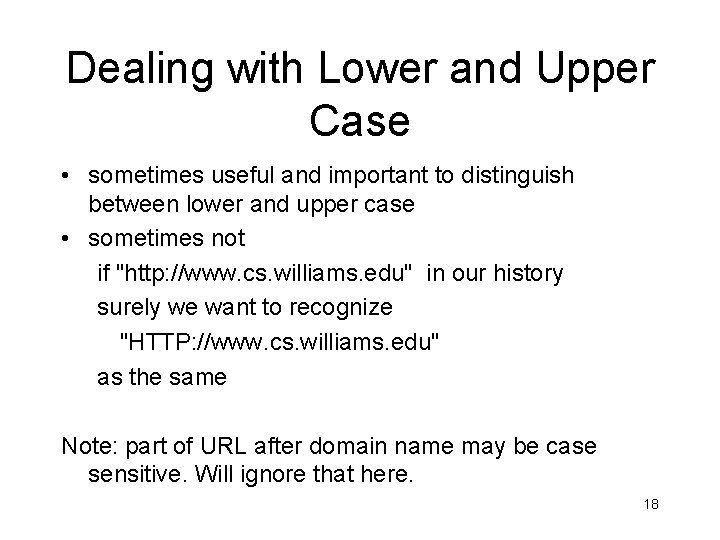
Dealing with Lower and Upper Case • sometimes useful and important to distinguish between lower and upper case • sometimes not if "http: //www. cs. williams. edu" in our history surely we want to recognize "HTTP: //www. cs. williams. edu" as the same Note: part of URL after domain name may be case sensitive. Will ignore that here. 18
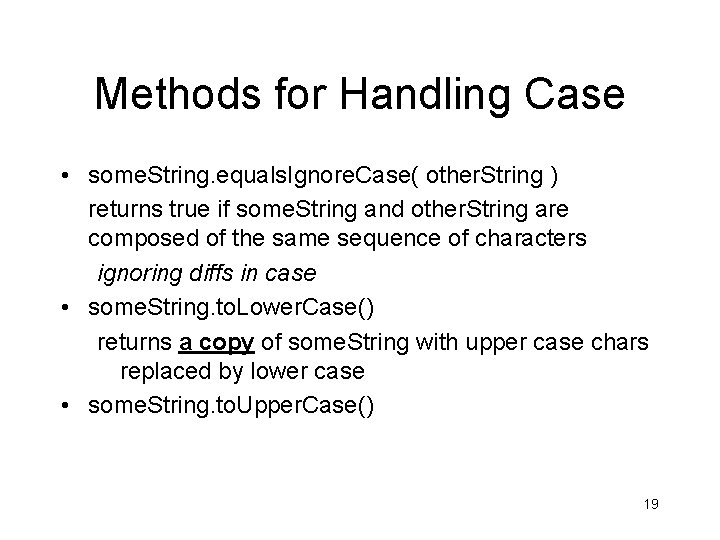
Methods for Handling Case • some. String. equals. Ignore. Case( other. String ) returns true if some. String and other. String are composed of the same sequence of characters ignoring diffs in case • some. String. to. Lower. Case() returns a copy of some. String with upper case chars replaced by lower case • some. String. to. Upper. Case() 19
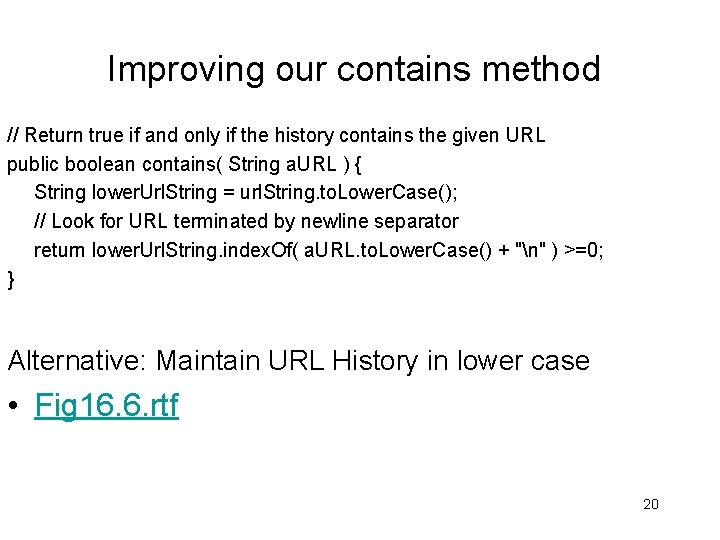
Improving our contains method // Return true if and only if the history contains the given URL public boolean contains( String a. URL ) { String lower. Url. String = url. String. to. Lower. Case(); // Look for URL terminated by newline separator return lower. Url. String. index. Of( a. URL. to. Lower. Case() + "n" ) >=0; } Alternative: Maintain URL History in lower case • Fig 16. 6. rtf 20
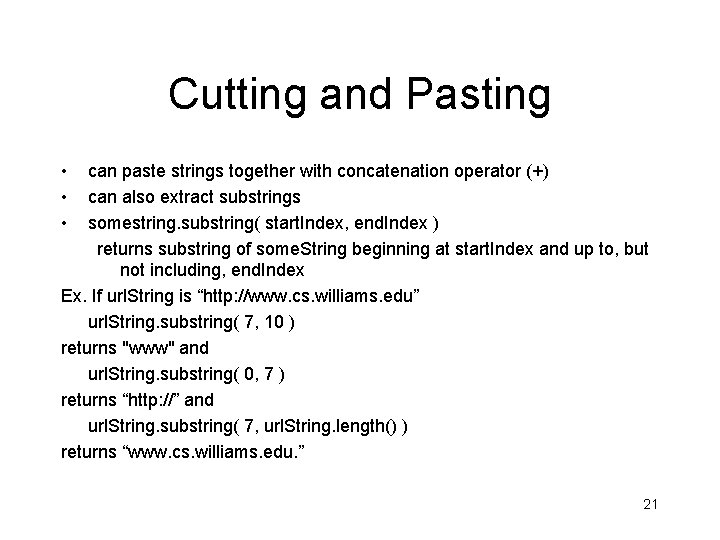
Cutting and Pasting • • • can paste strings together with concatenation operator (+) can also extract substrings somestring. substring( start. Index, end. Index ) returns substring of some. String beginning at start. Index and up to, but not including, end. Index Ex. If url. String is “http: //www. cs. williams. edu” url. String. substring( 7, 10 ) returns "www" and url. String. substring( 0, 7 ) returns “http: //” and url. String. substring( 7, url. String. length() ) returns “www. cs. williams. edu. ” 21
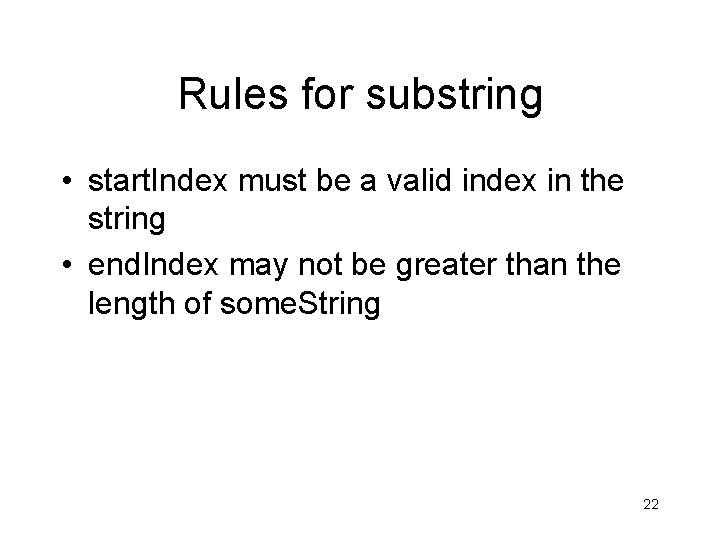
Rules for substring • start. Index must be a valid index in the string • end. Index may not be greater than the length of some. String 22
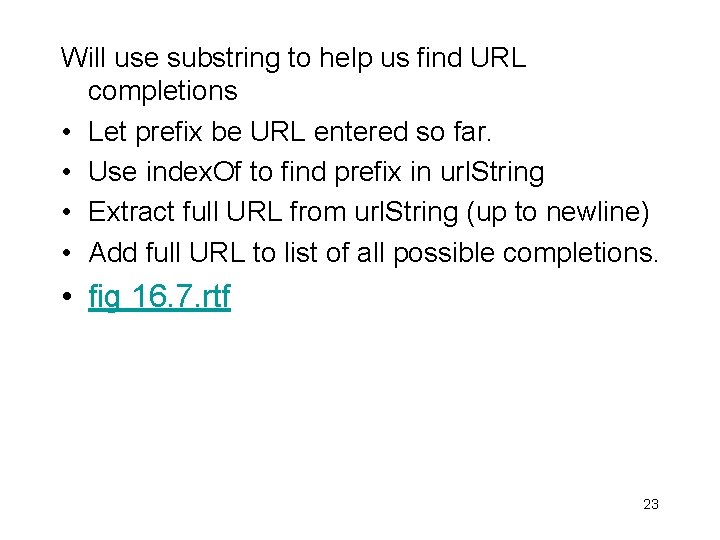
Will use substring to help us find URL completions • Let prefix be URL entered so far. • Use index. Of to find prefix in url. String • Extract full URL from url. String (up to newline) • Add full URL to list of all possible completions. • fig 16. 7. rtf 23
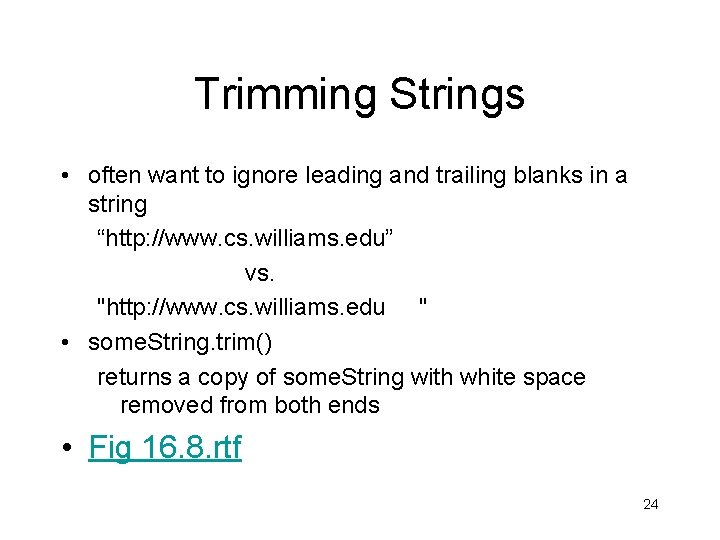
Trimming Strings • often want to ignore leading and trailing blanks in a string “http: //www. cs. williams. edu” vs. "http: //www. cs. williams. edu " • some. String. trim() returns a copy of some. String with white space removed from both ends • Fig 16. 8. rtf 24
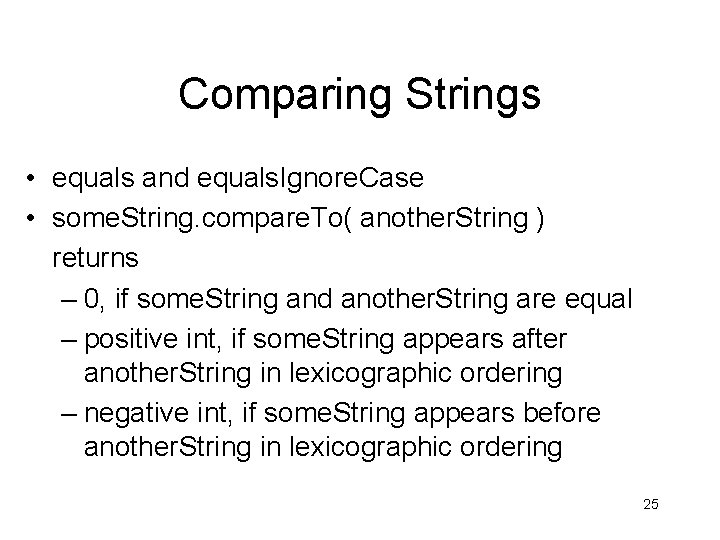
Comparing Strings • equals and equals. Ignore. Case • some. String. compare. To( another. String ) returns – 0, if some. String and another. String are equal – positive int, if some. String appears after another. String in lexicographic ordering – negative int, if some. String appears before another. String in lexicographic ordering 25
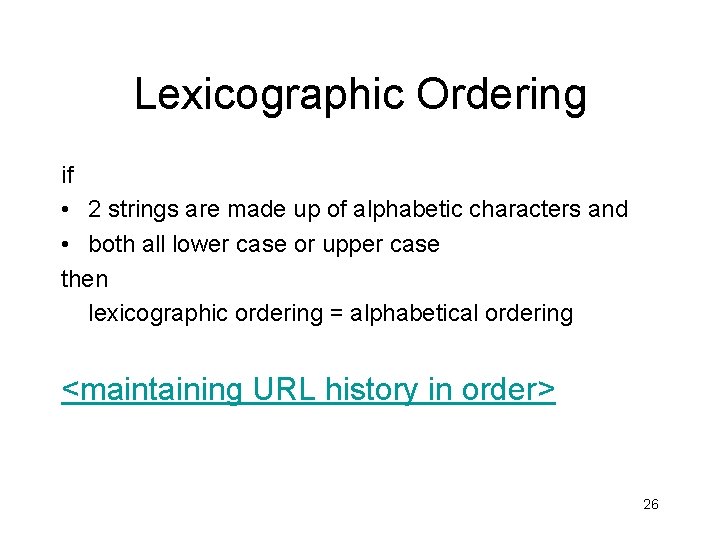
Lexicographic Ordering if • 2 strings are made up of alphabetic characters and • both all lower case or upper case then lexicographic ordering = alphabetical ordering <maintaining URL history in order> 26
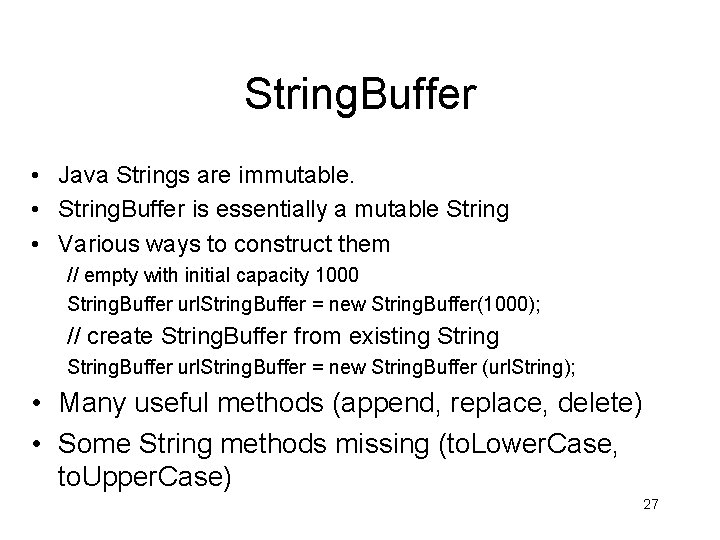
String. Buffer • Java Strings are immutable. • String. Buffer is essentially a mutable String • Various ways to construct them // empty with initial capacity 1000 String. Buffer url. String. Buffer = new String. Buffer(1000); // create String. Buffer from existing String. Buffer url. String. Buffer = new String. Buffer (url. String); • Many useful methods (append, replace, delete) • Some String methods missing (to. Lower. Case, to. Upper. Case) 27
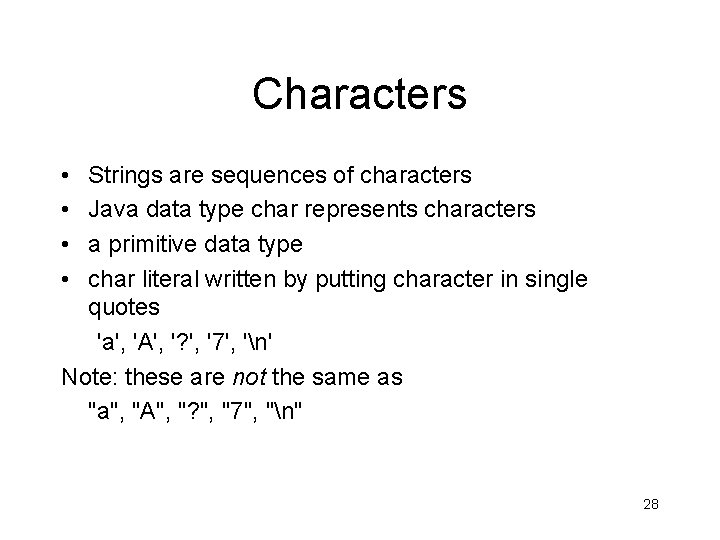
Characters • • Strings are sequences of characters Java data type char represents characters a primitive data type char literal written by putting character in single quotes 'a', 'A', '? ', '7', 'n' Note: these are not the same as "a", "A", "? ", "7", "n" 28
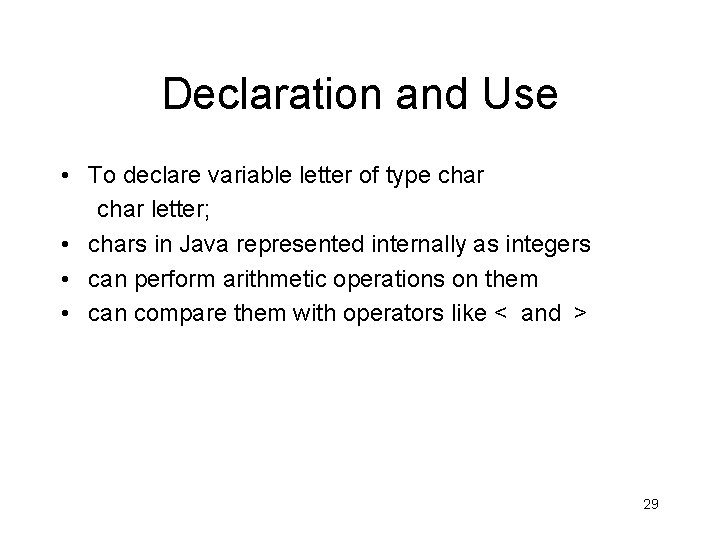
Declaration and Use • To declare variable letter of type char letter; • chars in Java represented internally as integers • can perform arithmetic operations on them • can compare them with operators like < and > 29
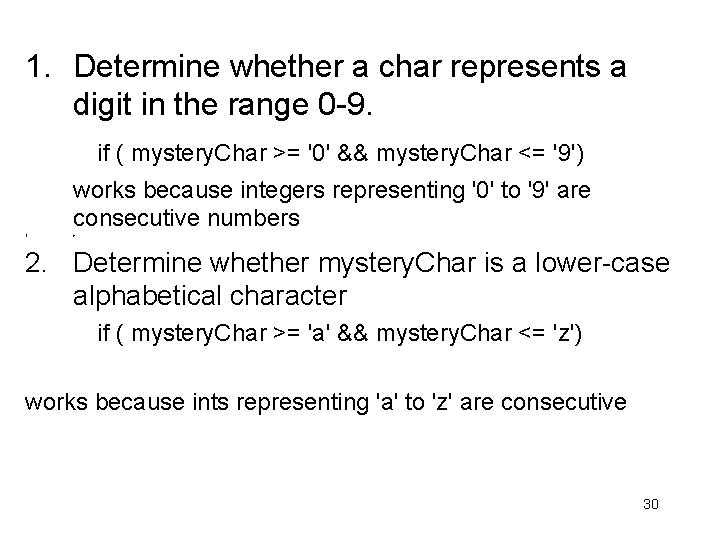
1. Determine whether a char represents a digit in the range 0 -9. if ( mystery. Char >= '0' && mystery. Char <= '9') works because integers representing '0' to '9' are consecutive numbers 1. e 2. Determine whether mystery. Char is a lower-case alphabetical character if ( mystery. Char >= 'a' && mystery. Char <= 'z') works because ints representing 'a' to 'z' are consecutive 30
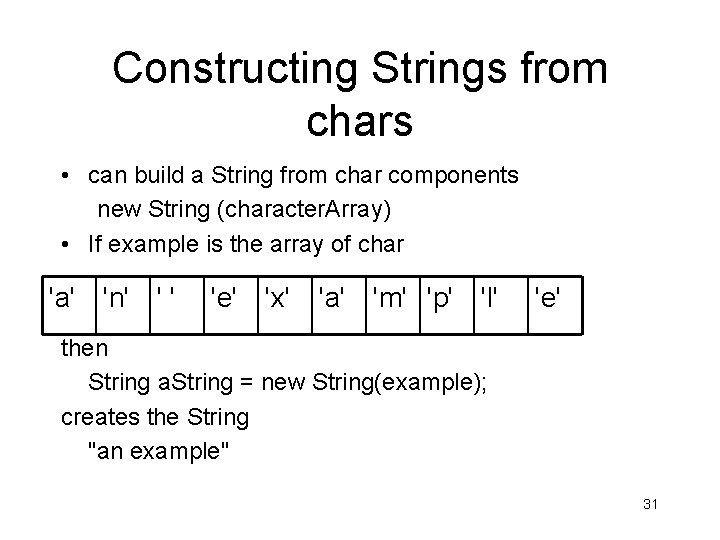
Constructing Strings from chars • can build a String from char components new String (character. Array) • If example is the array of char 'a' 'n' ' ' 'e' 'x' 'a' 'm' 'p' 'l' 'e' then String a. String = new String(example); creates the String "an example" 31
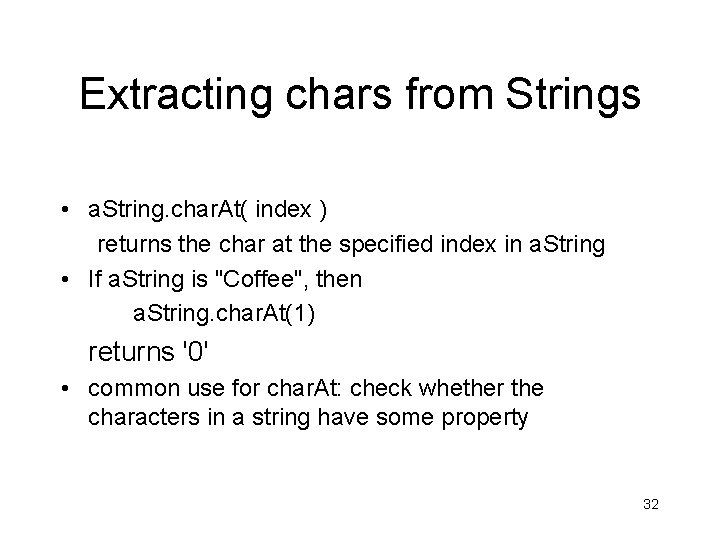
Extracting chars from Strings • a. String. char. At( index ) returns the char at the specified index in a. String • If a. String is "Coffee", then a. String. char. At(1) returns '0' • common use for char. At: check whether the characters in a string have some property 32
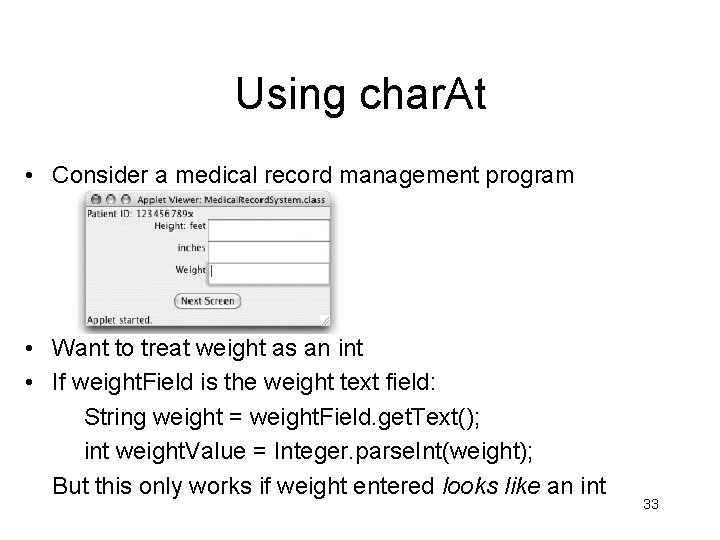
Using char. At • Consider a medical record management program • Want to treat weight as an int • If weight. Field is the weight text field: String weight = weight. Field. get. Text(); int weight. Value = Integer. parse. Int(weight); But this only works if weight entered looks like an int 33
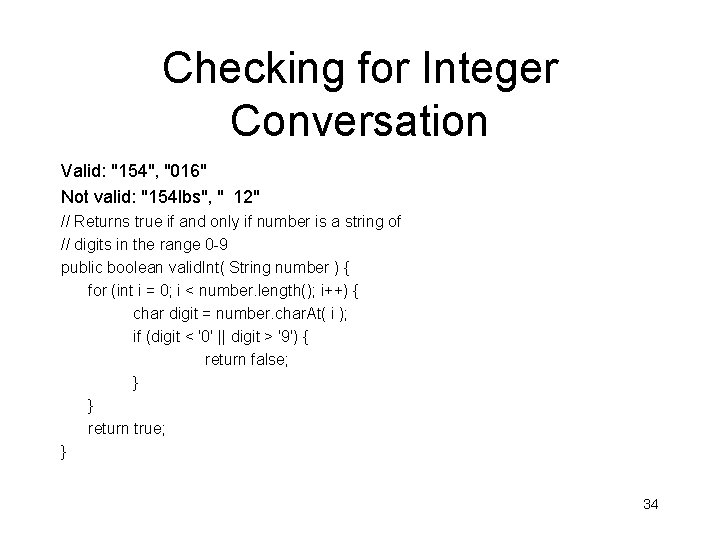
Checking for Integer Conversation Valid: "154", "016" Not valid: "154 lbs", " 12" // Returns true if and only if number is a string of // digits in the range 0 -9 public boolean valid. Int( String number ) { for (int i = 0; i < number. length(); i++) { char digit = number. char. At( i ); if (digit < '0' || digit > '9') { return false; } } return true; } 34
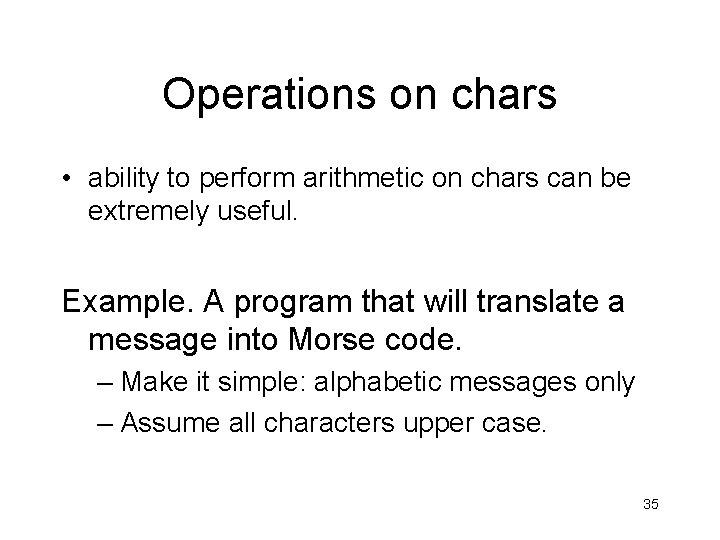
Operations on chars • ability to perform arithmetic on chars can be extremely useful. Example. A program that will translate a message into Morse code. – Make it simple: alphabetic messages only – Assume all characters upper case. 35
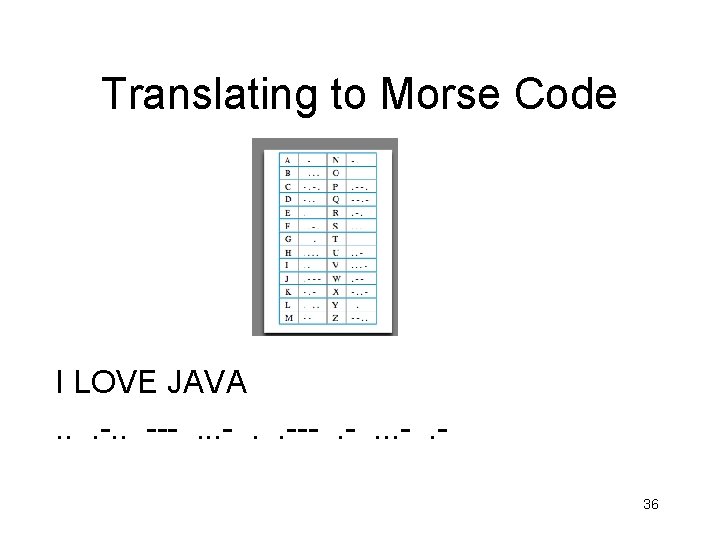
Translating to Morse Code I LOVE JAVA. . . ---. -. 36
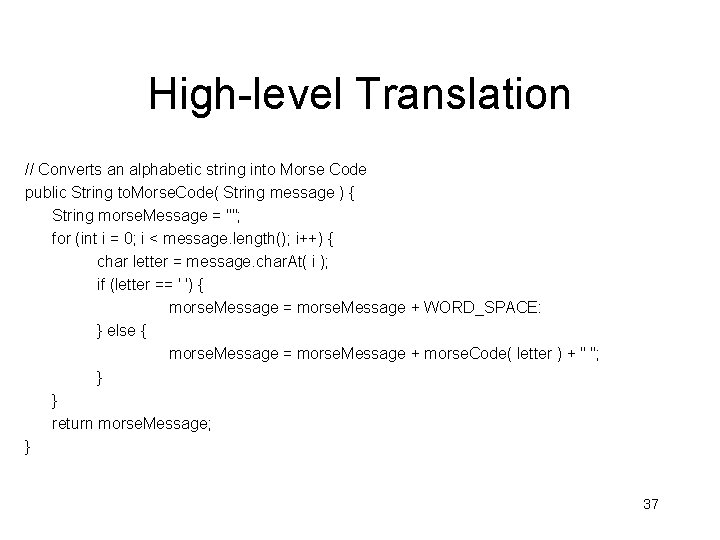
High-level Translation // Converts an alphabetic string into Morse Code public String to. Morse. Code( String message ) { String morse. Message = ""; for (int i = 0; i < message. length(); i++) { char letter = message. char. At( i ); if (letter == ' ') { morse. Message = morse. Message + WORD_SPACE: } else { morse. Message = morse. Message + morse. Code( letter ) + " "; } } return morse. Message; } 37
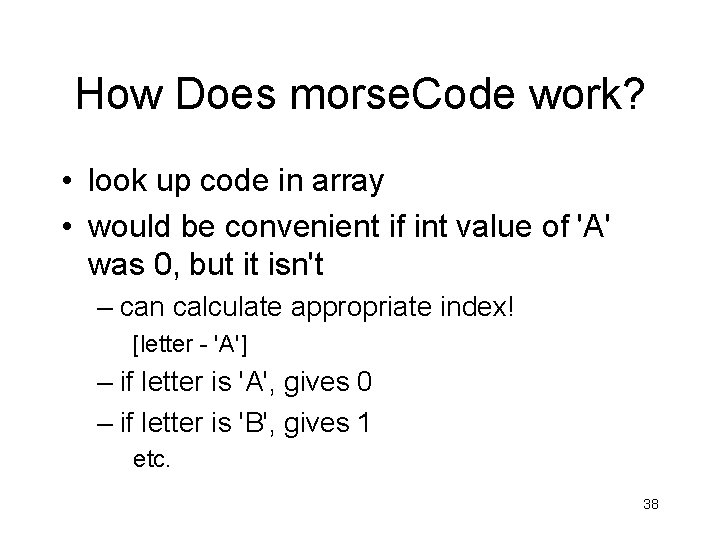
How Does morse. Code work? • look up code in array • would be convenient if int value of 'A' was 0, but it isn't – can calculate appropriate index! [letter - 'A'] – if letter is 'A', gives 0 – if letter is 'B', gives 1 etc. 38
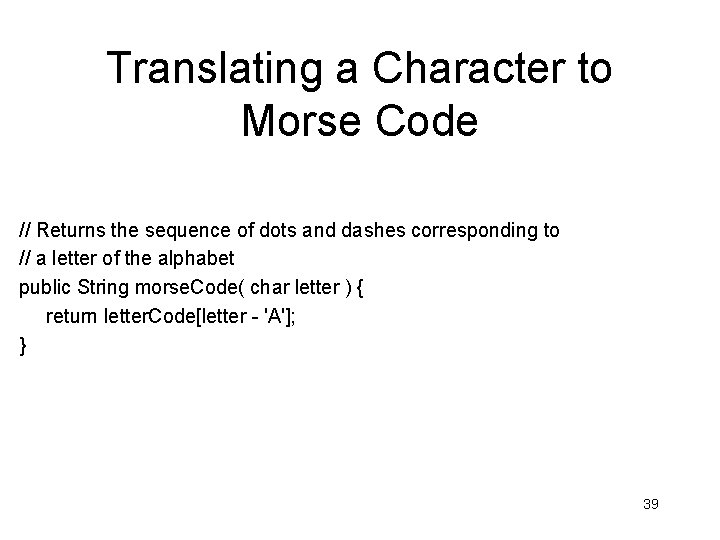
Translating a Character to Morse Code // Returns the sequence of dots and dashes corresponding to // a letter of the alphabet public String morse. Code( char letter ) { return letter. Code[letter - 'A']; } 39
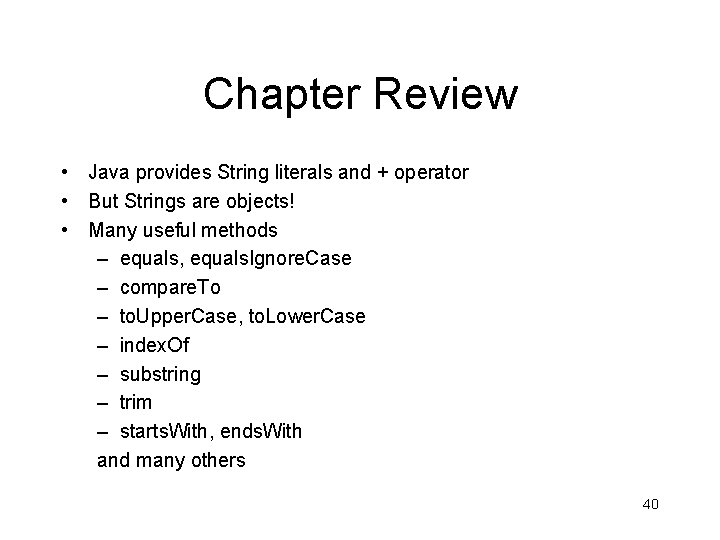
Chapter Review • Java provides String literals and + operator • But Strings are objects! • Many useful methods – equals, equals. Ignore. Case – compare. To – to. Upper. Case, to. Lower. Case – index. Of – substring – trim – starts. With, ends. With and many others 40
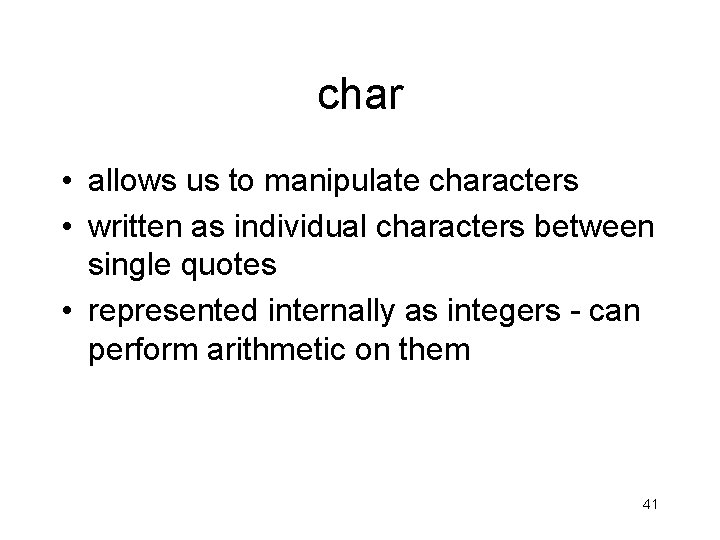
char • allows us to manipulate characters • written as individual characters between single quotes • represented internally as integers - can perform arithmetic on them 41
Computers manipulate data in many ways
How does lady macbeth manipulate macbeth
How does nurse ratched manipulate the patients
How does claudius manipulate laertes
How does montresor manipulate fortunato
Algebraic fractions simplify
Fortunato's carnival costume symbolizes
Business application in computer network
Subtraction rule example
Uses of microcomputer
Principles of network applications
Objectives of computer applications
Computer integrated manufacturing applications
Computer applications - understanding computers unit
Computer networks and internets with internet applications
Business applications of computer networks
Business applications of computer networks
What is computer network and its applications
Perfect competition 4 conditions
Er diagram many to many
Convert conceptual model to logical model
Difference between erm and erd
Unary many to many
Erd ratio
Unary many to many
Many-to-many communication
Sqlbi many to many
Ternary relationship database
Many sellers and many buyers
How many fields in computer science
How many fields in computer science
Jellyfish syllables
How many parts of computer
Software is divided into two categories
How many syllables in mummy
How many parts are there in computer
Systems applications and products in data processing
Systems applications & products in data processing
Spatial data mining applications
Building data mining applications for crm
Characteristics of big data applications
Data driven web applications