Simple Input n Interactive Input The Class Scanner
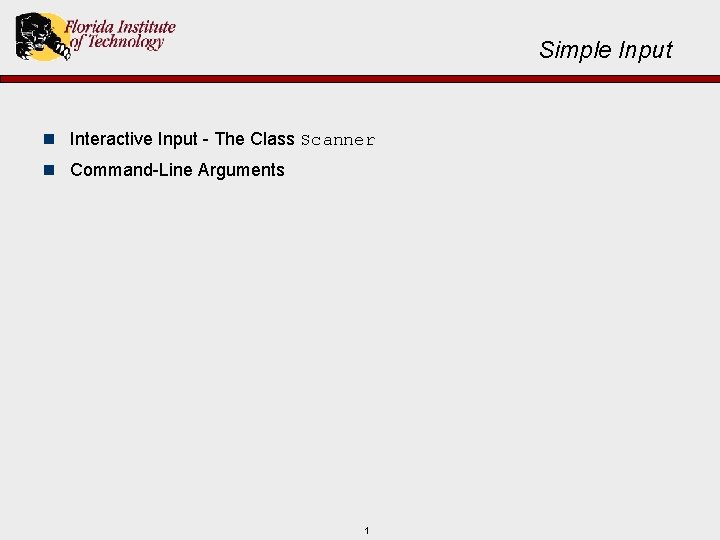
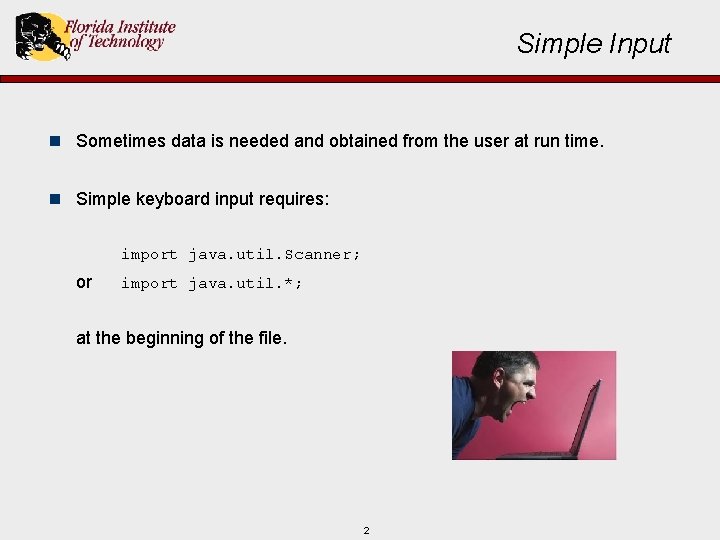
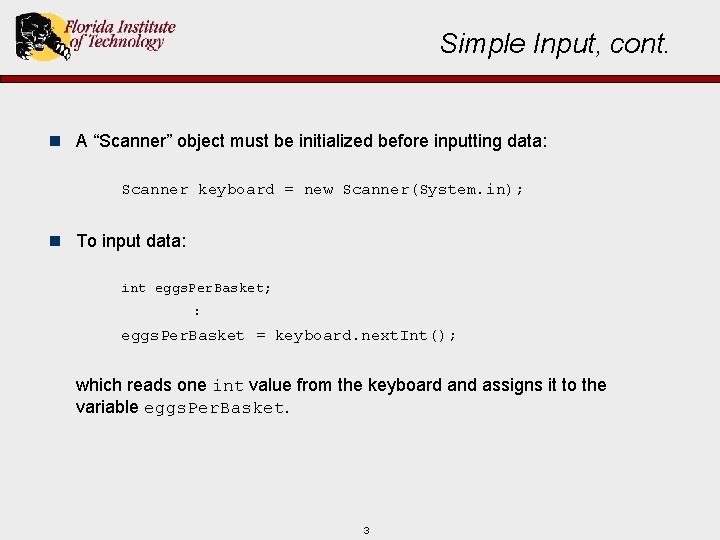
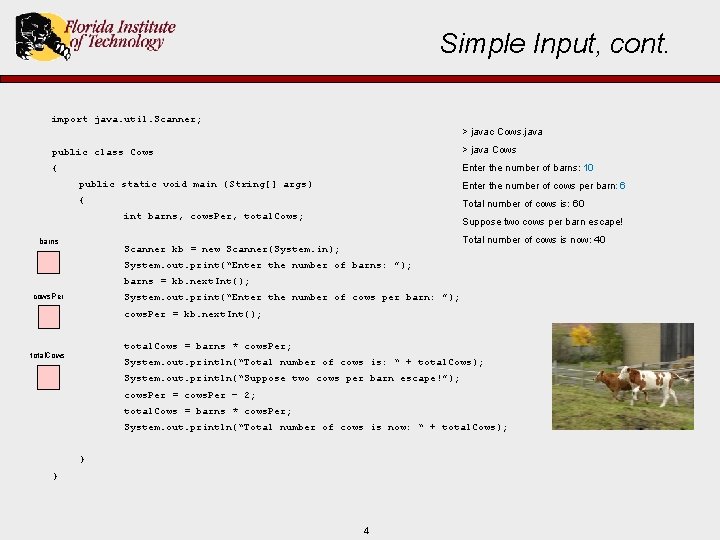
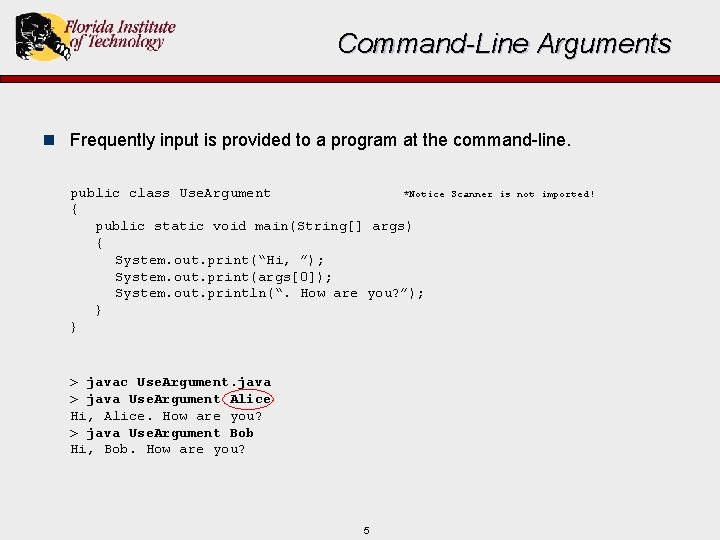
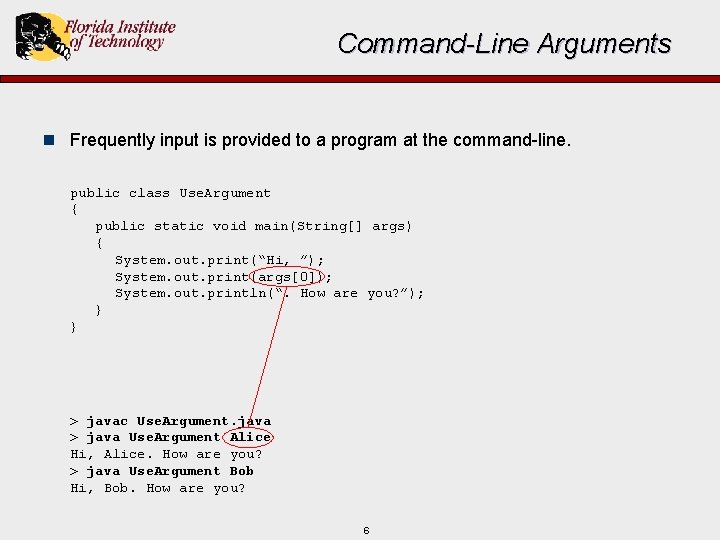
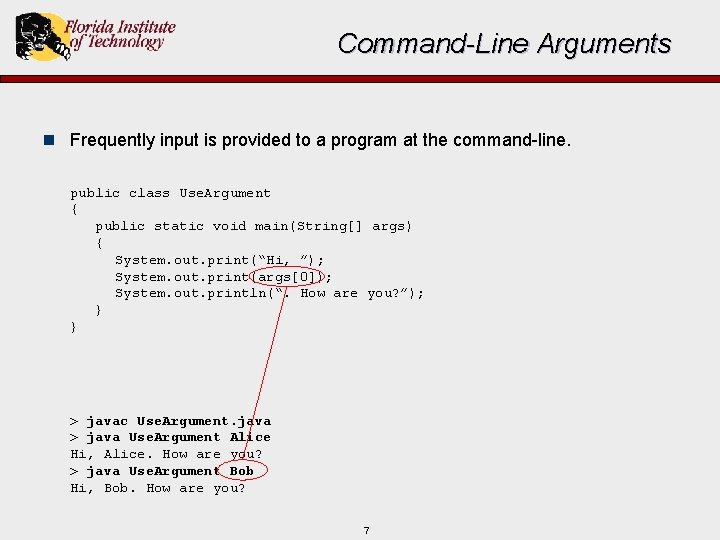
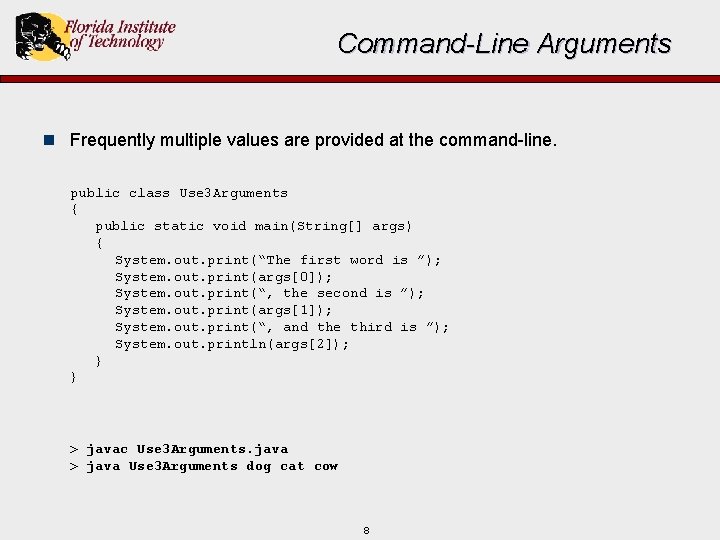
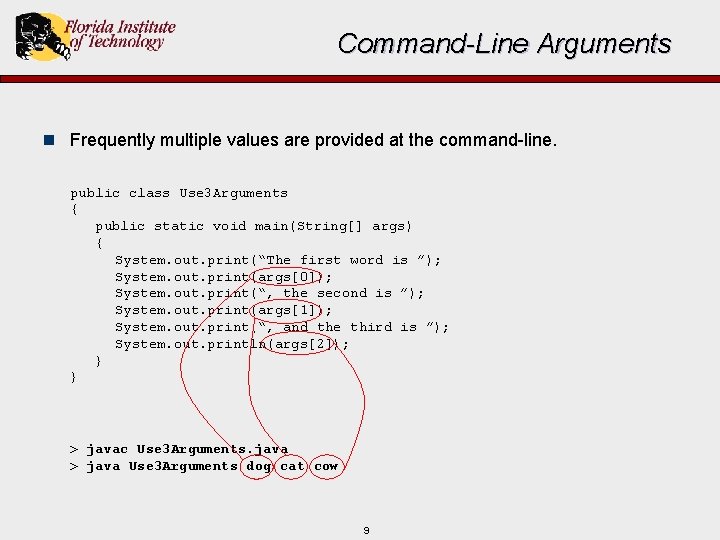
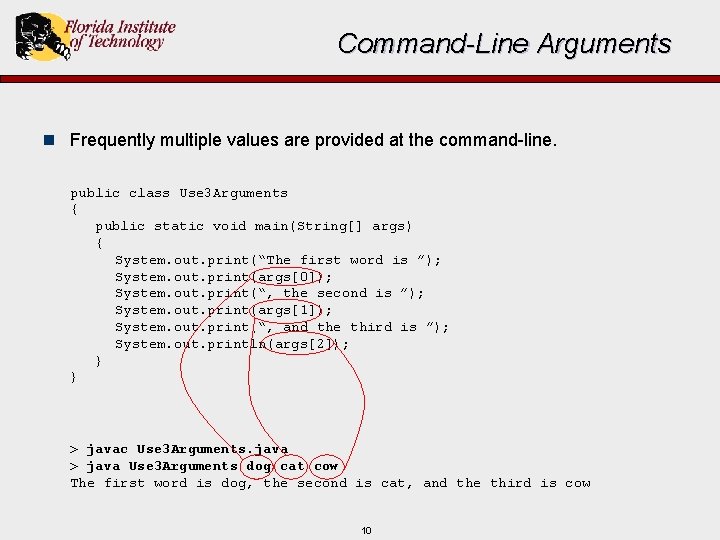
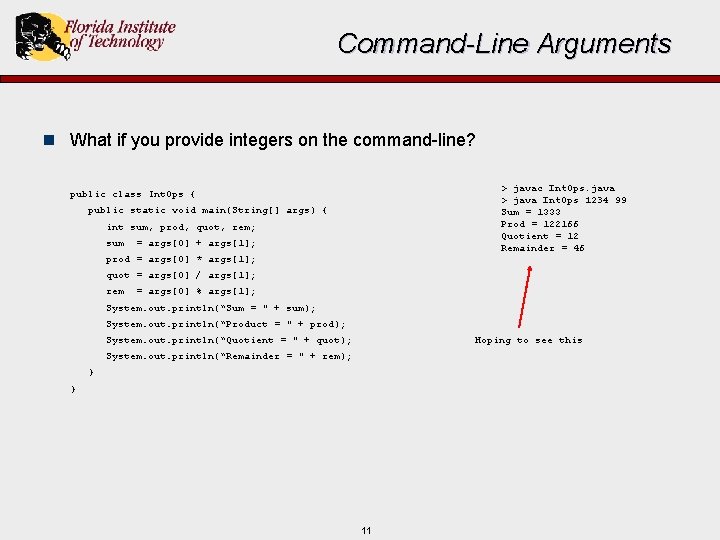
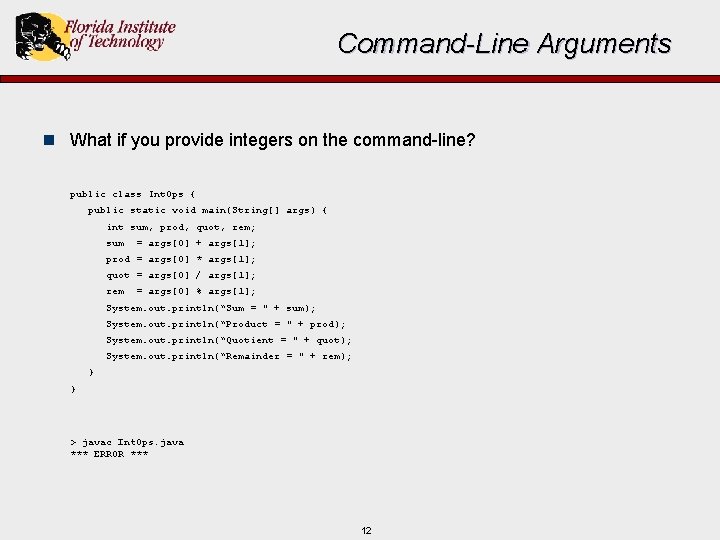
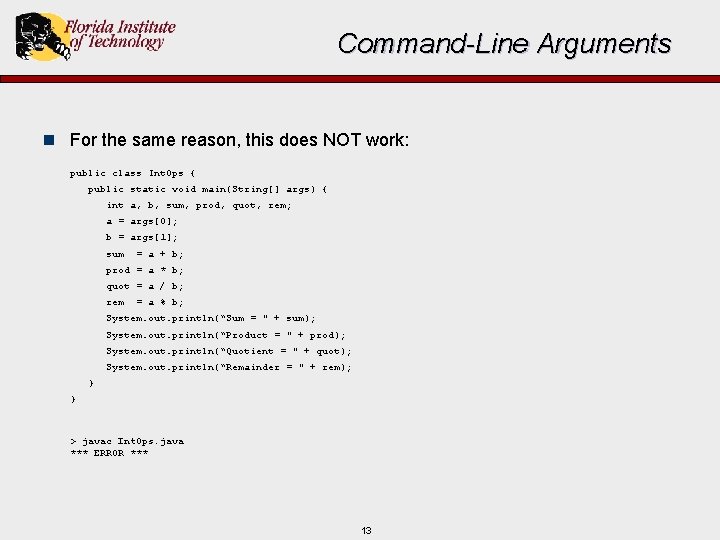
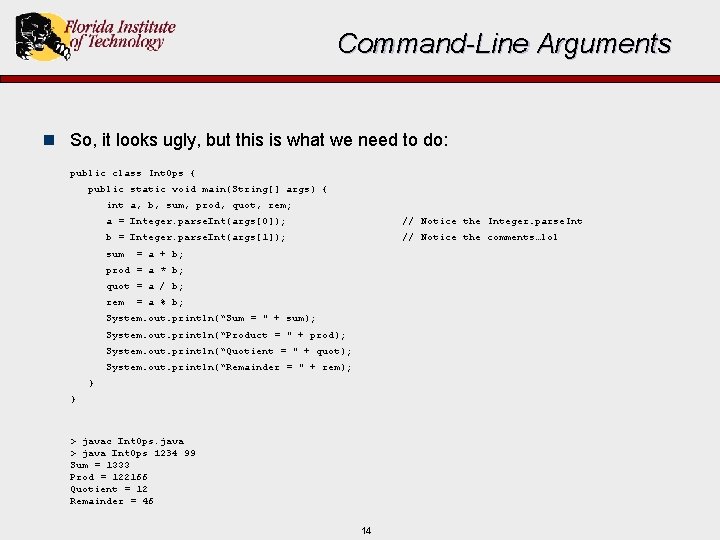
- Slides: 14
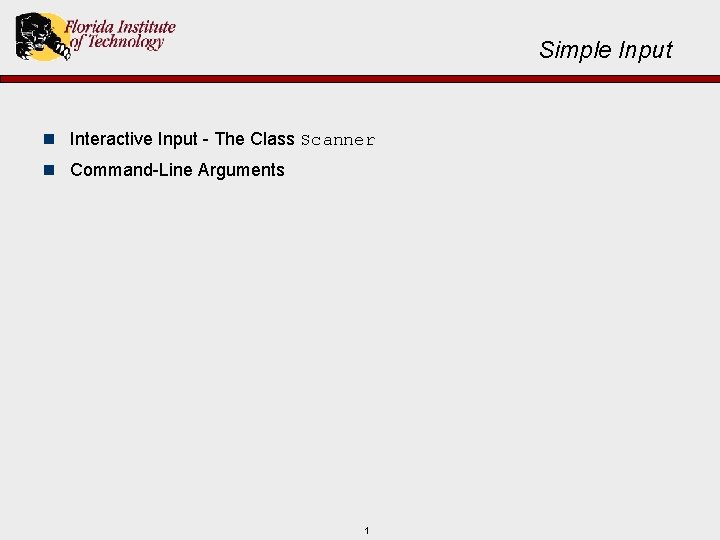
Simple Input n Interactive Input - The Class Scanner n Command-Line Arguments 1
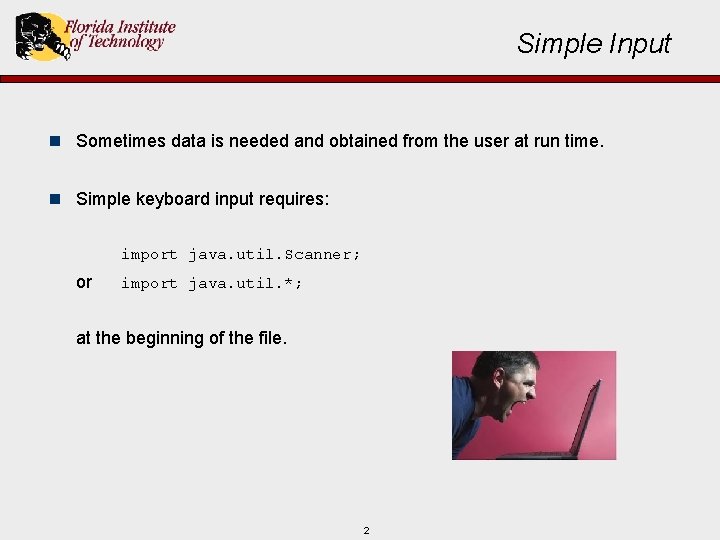
Simple Input n Sometimes data is needed and obtained from the user at run time. n Simple keyboard input requires: import java. util. Scanner; or import java. util. *; at the beginning of the file. 2
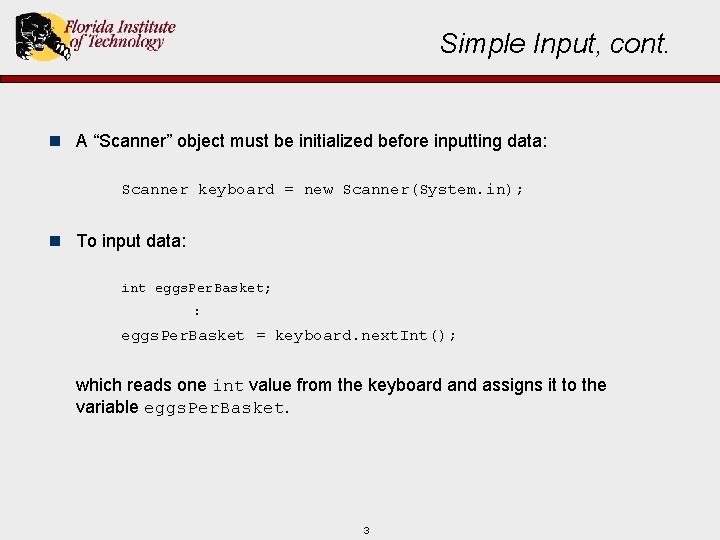
Simple Input, cont. n A “Scanner” object must be initialized before inputting data: Scanner keyboard = new Scanner(System. in); n To input data: int eggs. Per. Basket; : eggs. Per. Basket = keyboard. next. Int(); which reads one int value from the keyboard and assigns it to the variable eggs. Per. Basket. 3
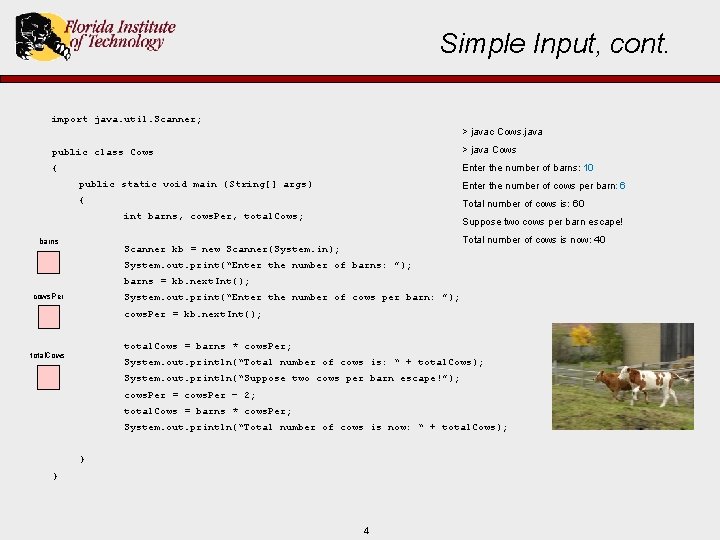
Simple Input, cont. import java. util. Scanner; > javac Cows. java public class Cows > java Cows { Enter the number of barns: 10 public static void main (String[] args) Enter the number of cows per barn: 6 { Total number of cows is: 60 int barns, cows. Per, total. Cows; barns Suppose two cows per barn escape! Total number of cows is now: 40 Scanner kb = new Scanner(System. in); System. out. print(“Enter the number of barns: ”); barns = kb. next. Int(); cows. Per System. out. print(“Enter the number of cows per barn: ”); cows. Per = kb. next. Int(); total. Cows = barns * cows. Per; total. Cows System. out. println(“Total number of cows is: “ + total. Cows); System. out. println(“Suppose two cows per barn escape!”); cows. Per = cows. Per – 2; total. Cows = barns * cows. Per; System. out. println(“Total number of cows is now: “ + total. Cows); } } 4
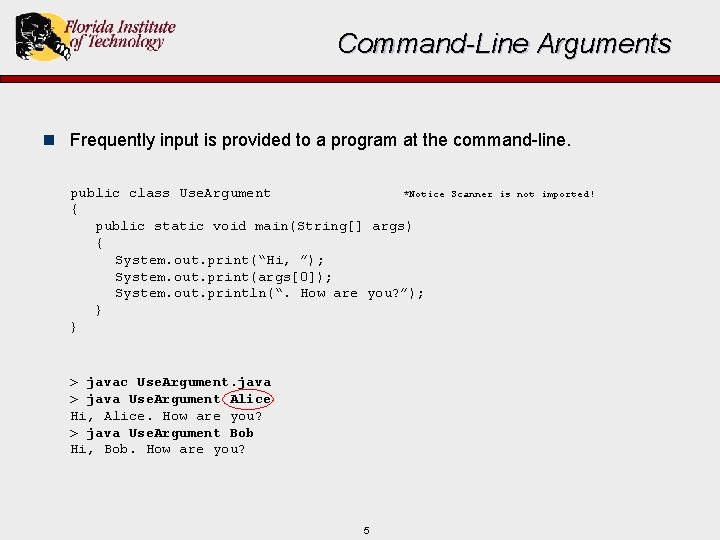
Command-Line Arguments n Frequently input is provided to a program at the command-line. public class Use. Argument *Notice { public static void main(String[] args) { System. out. print(“Hi, ”); System. out. print(args[0]); System. out. println(“. How are you? ”); } } > javac Use. Argument. java > java Use. Argument Alice Hi, Alice. How are you? > java Use. Argument Bob Hi, Bob. How are you? 5 Scanner is not imported!
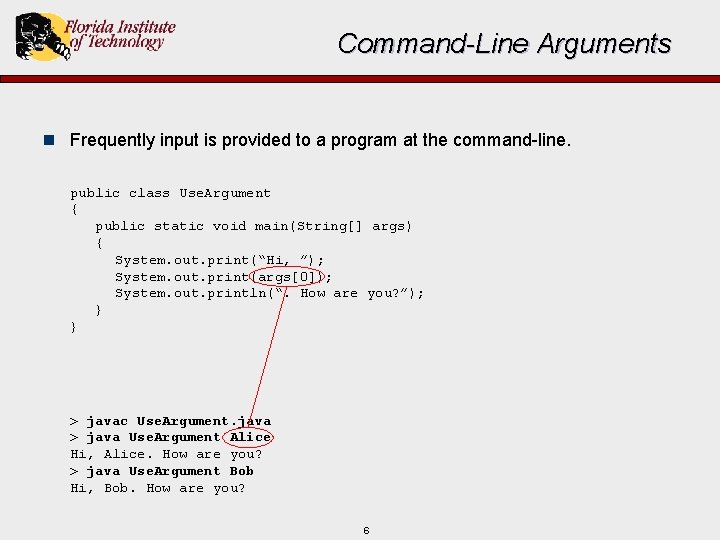
Command-Line Arguments n Frequently input is provided to a program at the command-line. public class Use. Argument { public static void main(String[] args) { System. out. print(“Hi, ”); System. out. print(args[0]); System. out. println(“. How are you? ”); } } > javac Use. Argument. java > java Use. Argument Alice Hi, Alice. How are you? > java Use. Argument Bob Hi, Bob. How are you? 6
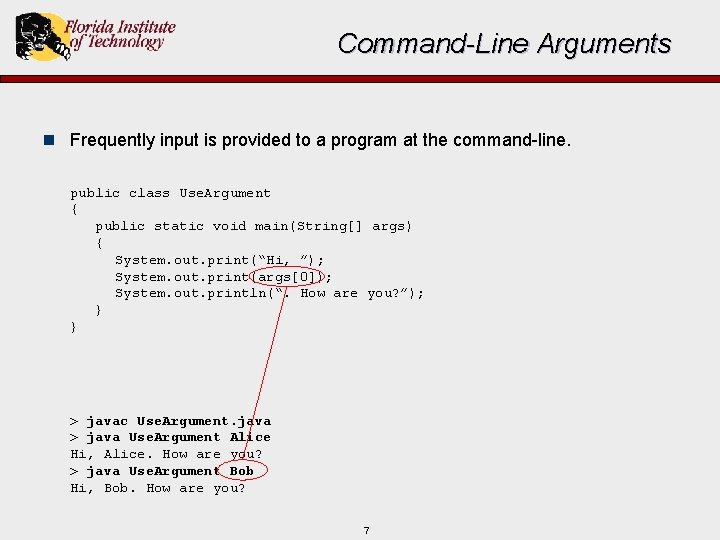
Command-Line Arguments n Frequently input is provided to a program at the command-line. public class Use. Argument { public static void main(String[] args) { System. out. print(“Hi, ”); System. out. print(args[0]); System. out. println(“. How are you? ”); } } > javac Use. Argument. java > java Use. Argument Alice Hi, Alice. How are you? > java Use. Argument Bob Hi, Bob. How are you? 7
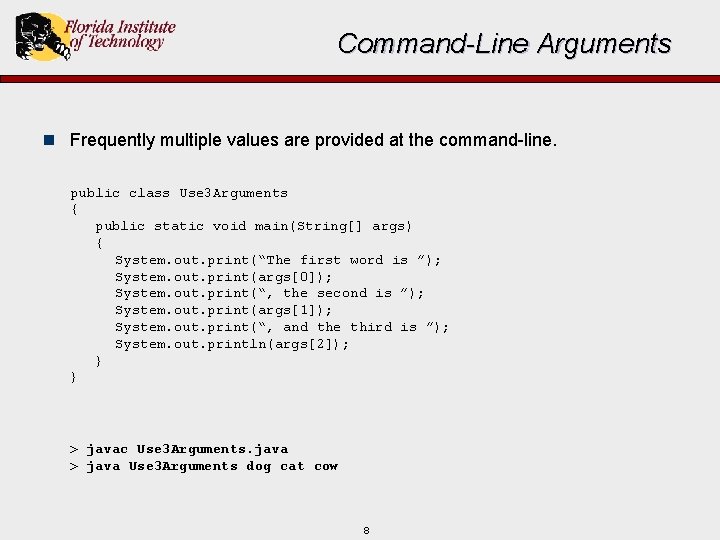
Command-Line Arguments n Frequently multiple values are provided at the command-line. public class Use 3 Arguments { public static void main(String[] args) { System. out. print(“The first word is ”); System. out. print(args[0]); System. out. print(“, the second is ”); System. out. print(args[1]); System. out. print(“, and the third is ”); System. out. println(args[2]); } } > javac Use 3 Arguments. java > java Use 3 Arguments dog cat cow 8
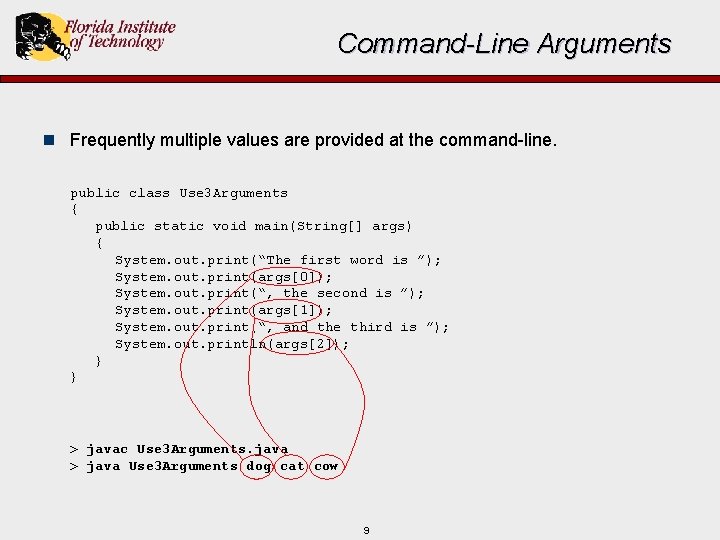
Command-Line Arguments n Frequently multiple values are provided at the command-line. public class Use 3 Arguments { public static void main(String[] args) { System. out. print(“The first word is ”); System. out. print(args[0]); System. out. print(“, the second is ”); System. out. print(args[1]); System. out. print(“, and the third is ”); System. out. println(args[2]); } } > javac Use 3 Arguments. java > java Use 3 Arguments dog cat cow 9
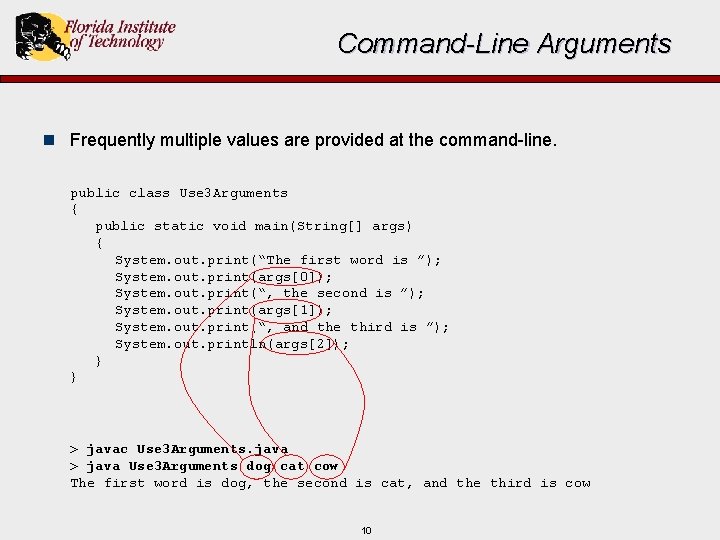
Command-Line Arguments n Frequently multiple values are provided at the command-line. public class Use 3 Arguments { public static void main(String[] args) { System. out. print(“The first word is ”); System. out. print(args[0]); System. out. print(“, the second is ”); System. out. print(args[1]); System. out. print(“, and the third is ”); System. out. println(args[2]); } } > javac Use 3 Arguments. java > java Use 3 Arguments dog cat cow The first word is dog, the second is cat, and the third is cow 10
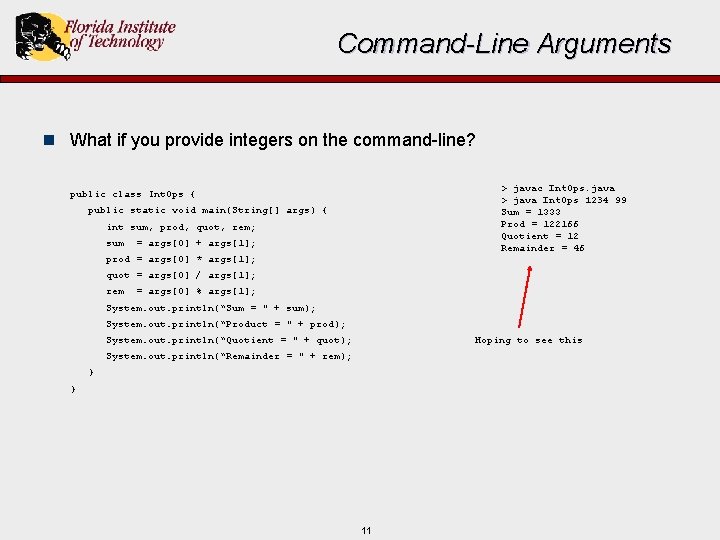
Command-Line Arguments n What if you provide integers on the command-line? > javac Int. Ops. java > java Int. Ops 1234 99 Sum = 1333 Prod = 122166 Quotient = 12 Remainder = 46 public class Int. Ops { public static void main(String[] args) { int sum, prod, quot, rem; sum = args[0] + args[1]; prod = args[0] * args[1]; quot = args[0] / args[1]; rem = args[0] % args[1]; System. out. println(“Sum = " + sum); System. out. println(“Product = " + prod); System. out. println(“Quotient = " + quot); Hoping to see this System. out. println(“Remainder = " + rem); } } 11
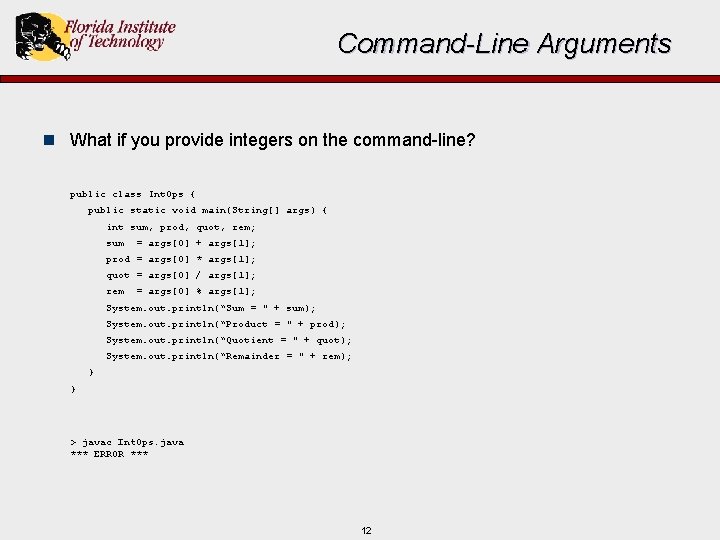
Command-Line Arguments n What if you provide integers on the command-line? public class Int. Ops { public static void main(String[] args) { int sum, prod, quot, rem; sum = args[0] + args[1]; prod = args[0] * args[1]; quot = args[0] / args[1]; rem = args[0] % args[1]; System. out. println(“Sum = " + sum); System. out. println(“Product = " + prod); System. out. println(“Quotient = " + quot); System. out. println(“Remainder = " + rem); } } > javac Int. Ops. java *** ERROR *** 12
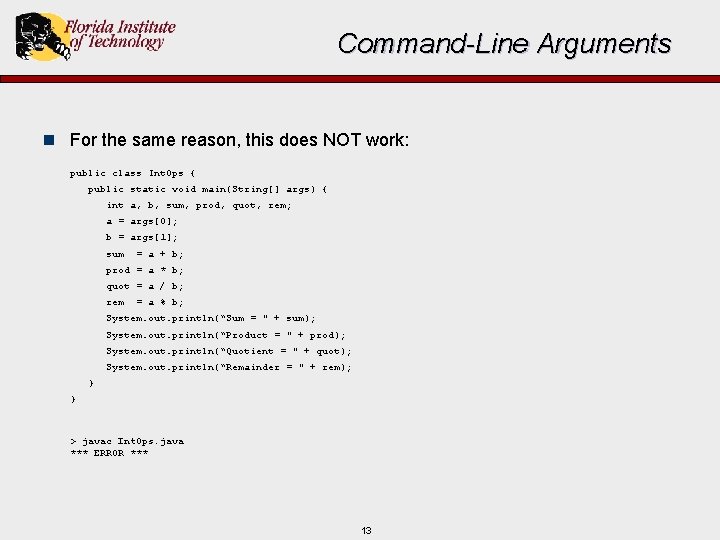
Command-Line Arguments n For the same reason, this does NOT work: public class Int. Ops { public static void main(String[] args) { int a, b, sum, prod, quot, rem; a = args[0]; b = args[1]; sum = a + b; prod = a * b; quot = a / b; rem = a % b; System. out. println(“Sum = " + sum); System. out. println(“Product = " + prod); System. out. println(“Quotient = " + quot); System. out. println(“Remainder = " + rem); } } > javac Int. Ops. java *** ERROR *** 13
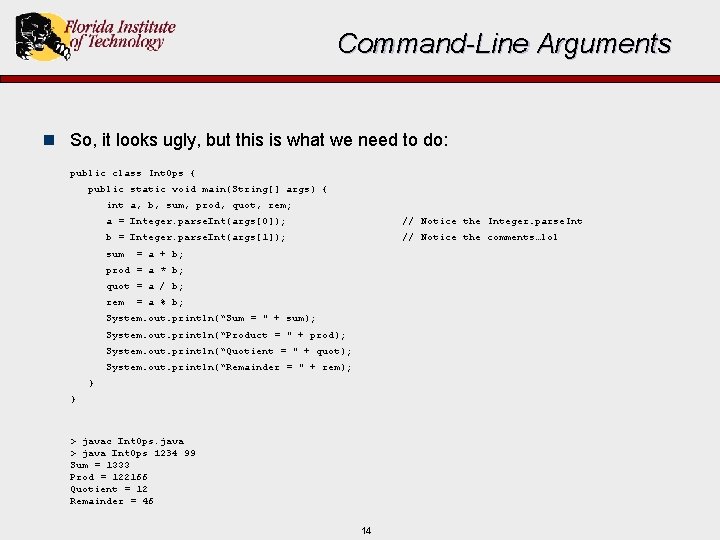
Command-Line Arguments n So, it looks ugly, but this is what we need to do: public class Int. Ops { public static void main(String[] args) { int a, b, sum, prod, quot, rem; a = Integer. parse. Int(args[0]); // Notice the Integer. parse. Int b = Integer. parse. Int(args[1]); // Notice the comments…lol sum = a + b; prod = a * b; quot = a / b; rem = a % b; System. out. println(“Sum = " + sum); System. out. println(“Product = " + prod); System. out. println(“Quotient = " + quot); System. out. println(“Remainder = " + rem); } } > javac Int. Ops. java > java Int. Ops 1234 99 Sum = 1333 Prod = 122166 Quotient = 12 Remainder = 46 14
Scanner keyboard = new scanner(system.in);
Scanner input device
Literary device scanner
Is a scanner an input or output device
Definition of input and output devices
Dot
Logical classification of input devices
Hát kết hợp bộ gõ cơ thể
Bổ thể
Tỉ lệ cơ thể trẻ em
Gấu đi như thế nào
Thang điểm glasgow
Chúa sống lại
Môn thể thao bắt đầu bằng chữ f