Scripting Languages and CShell What is a scripting
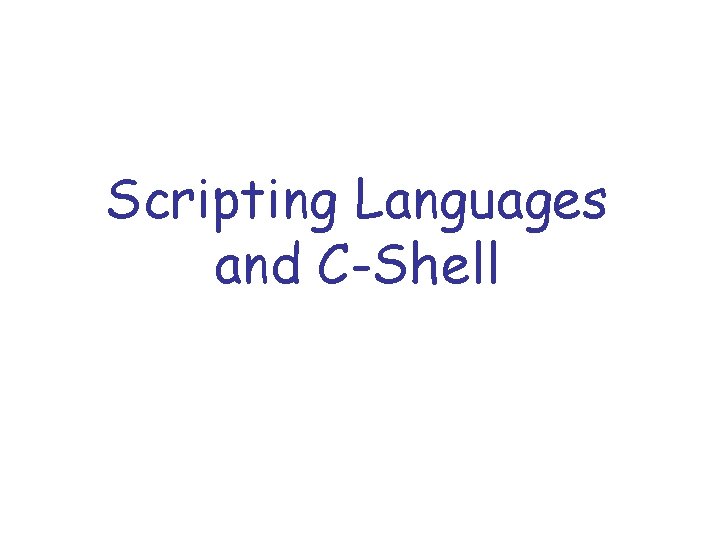
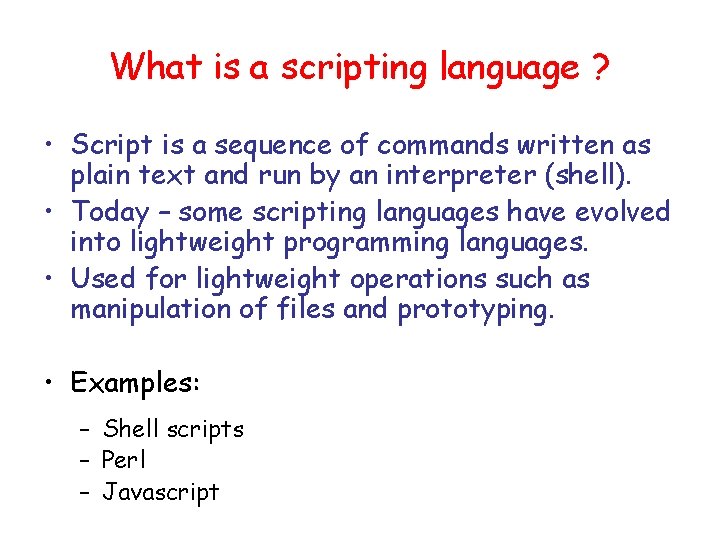
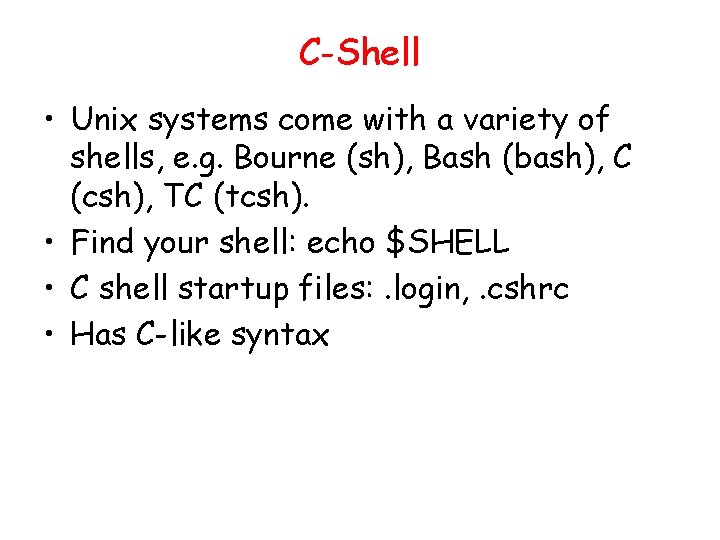
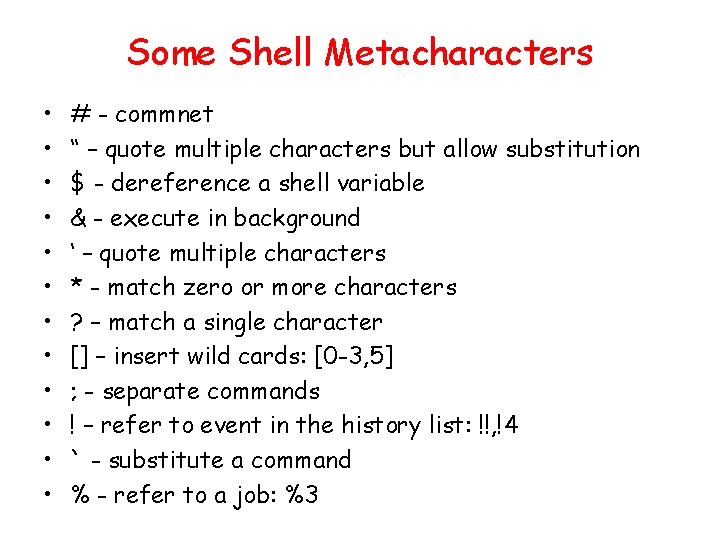
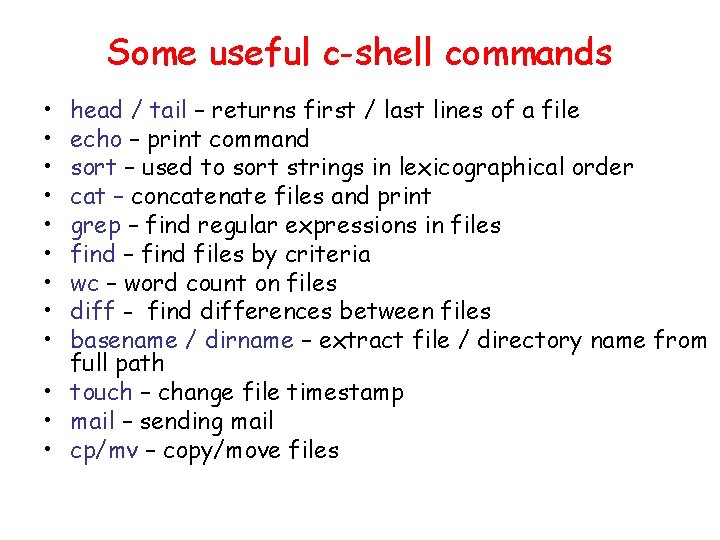
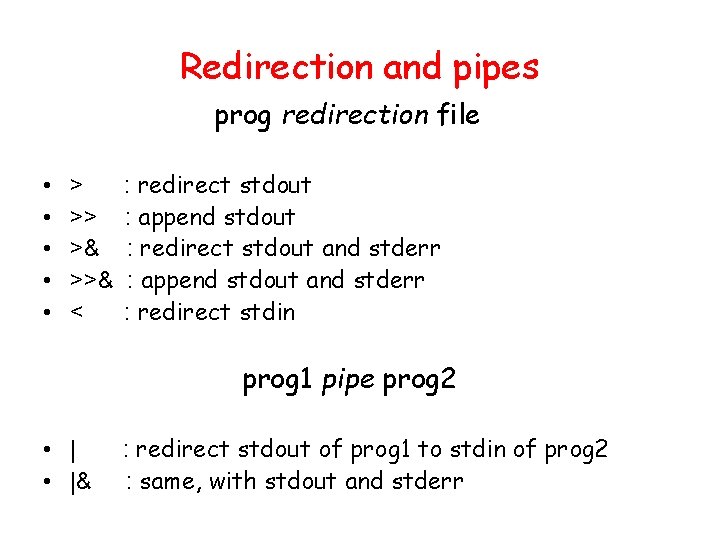
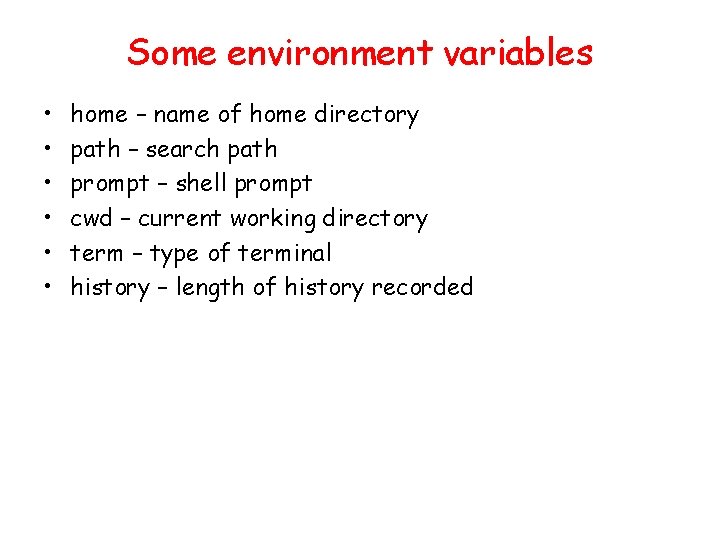
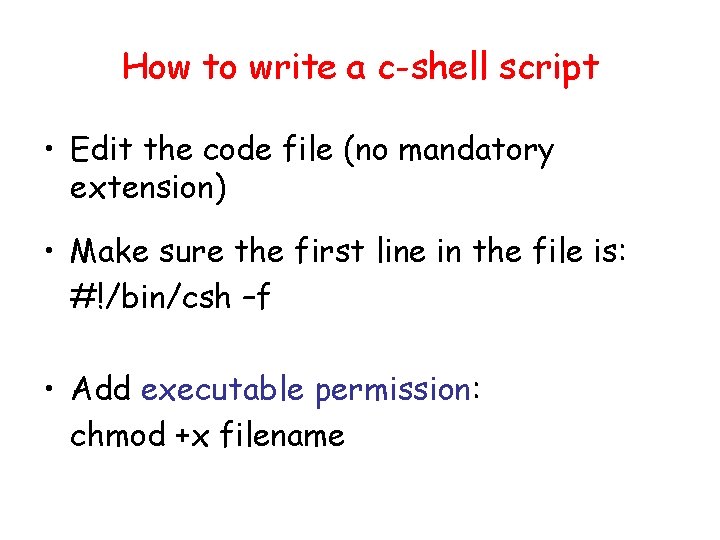
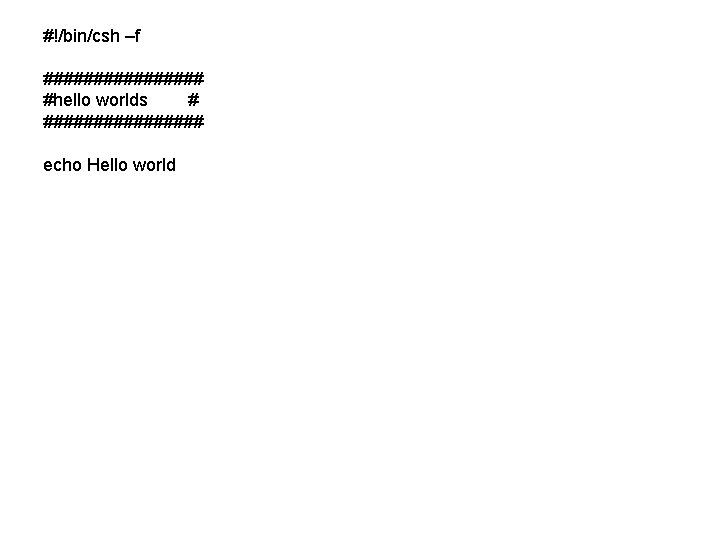
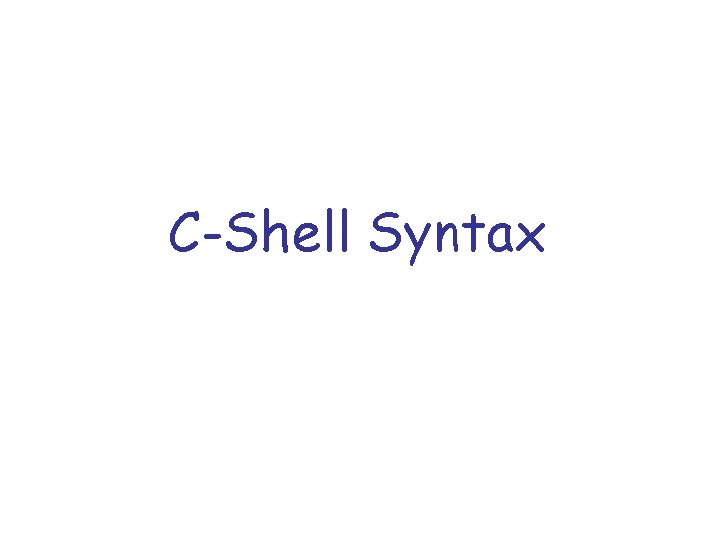
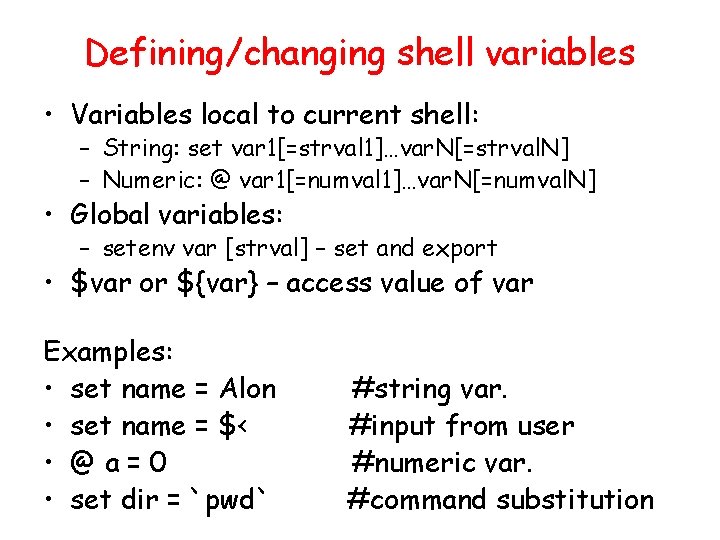
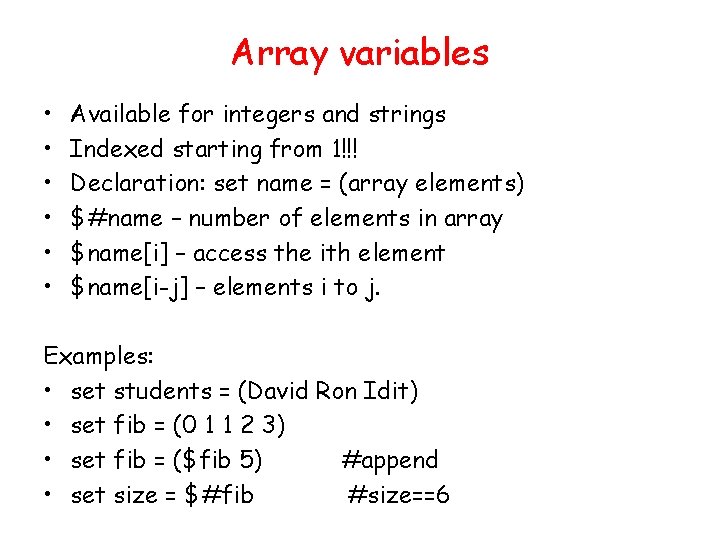
![Passing arguments to scripts • $argv[1]…$argv[9] are assigned the command line arguments • $#argv Passing arguments to scripts • $argv[1]…$argv[9] are assigned the command line arguments • $#argv](https://slidetodoc.com/presentation_image_h2/0c4741c79aeffd633d1e310e9aa13f79/image-13.jpg)
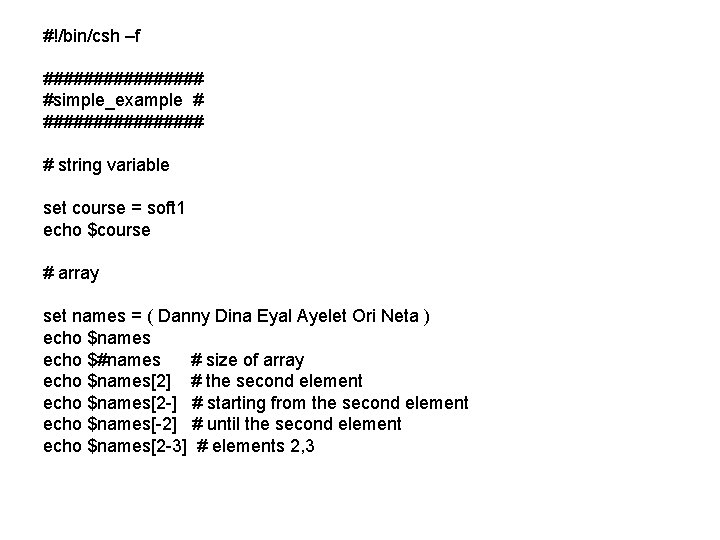
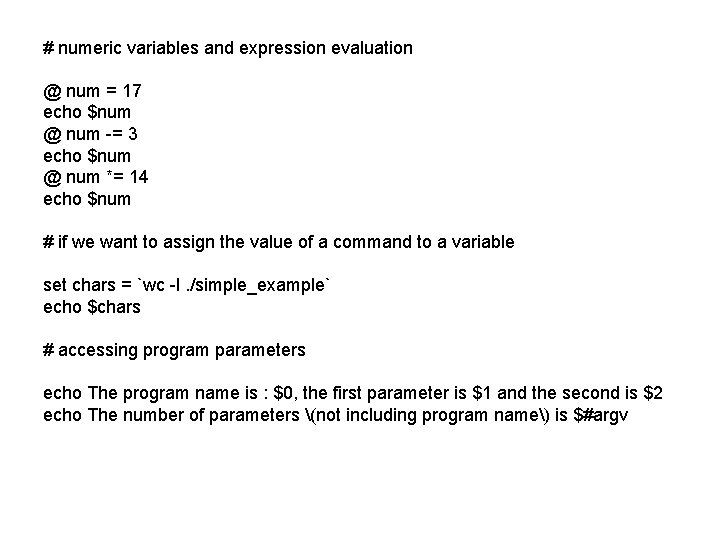
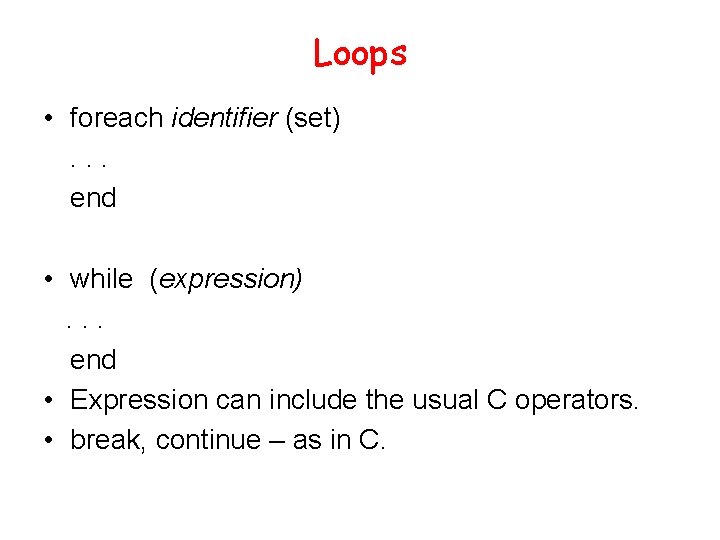
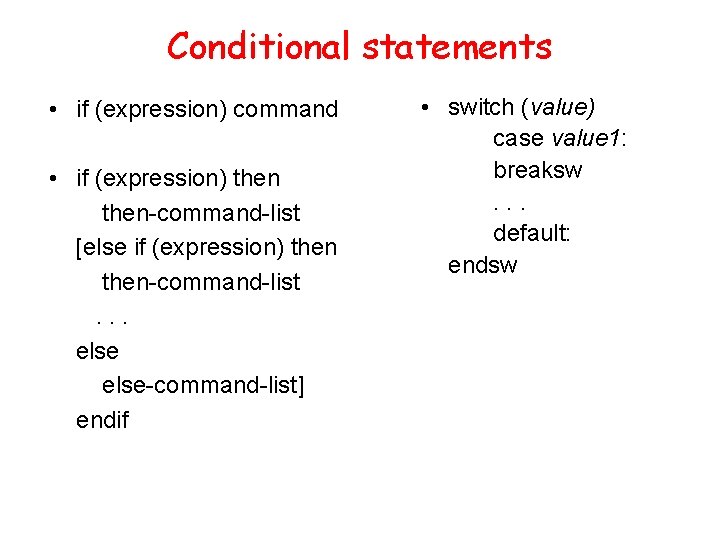
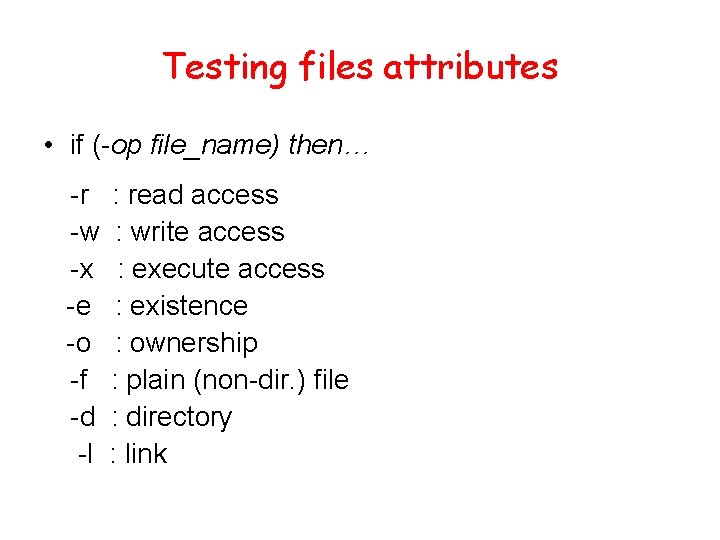
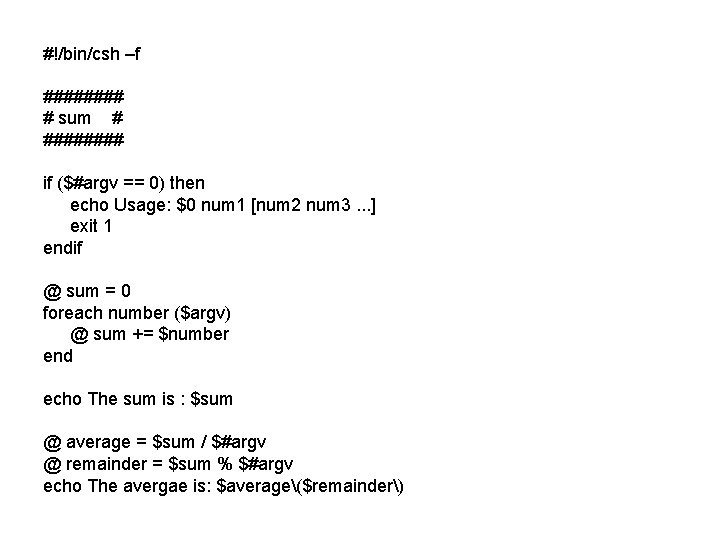
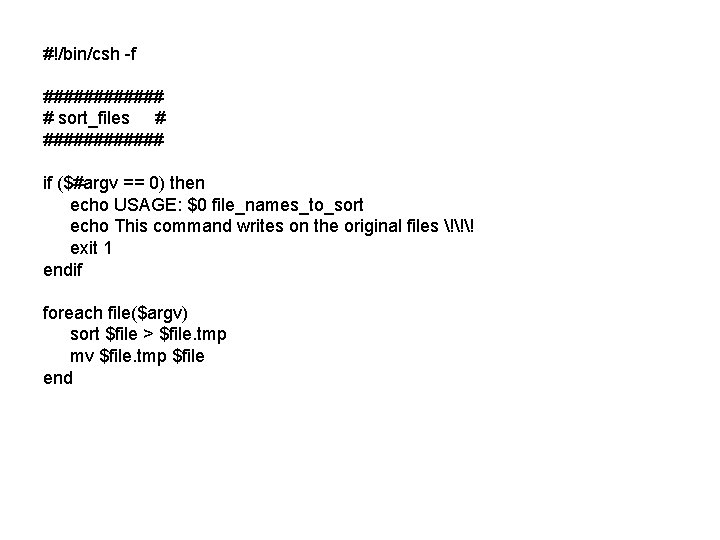
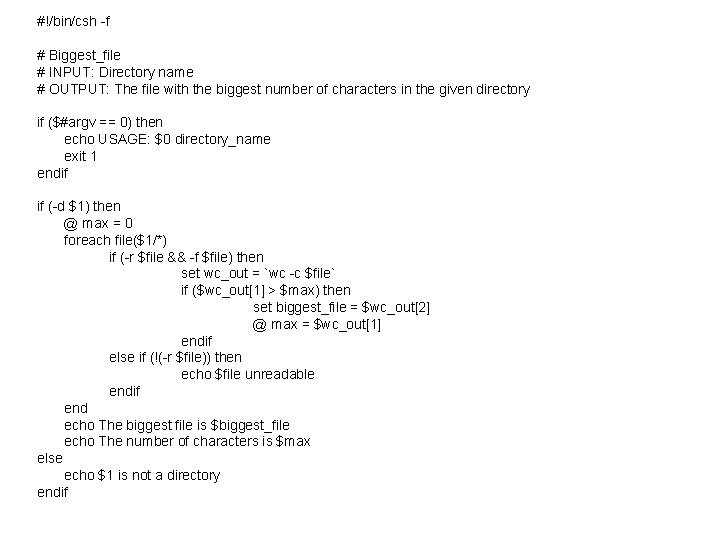
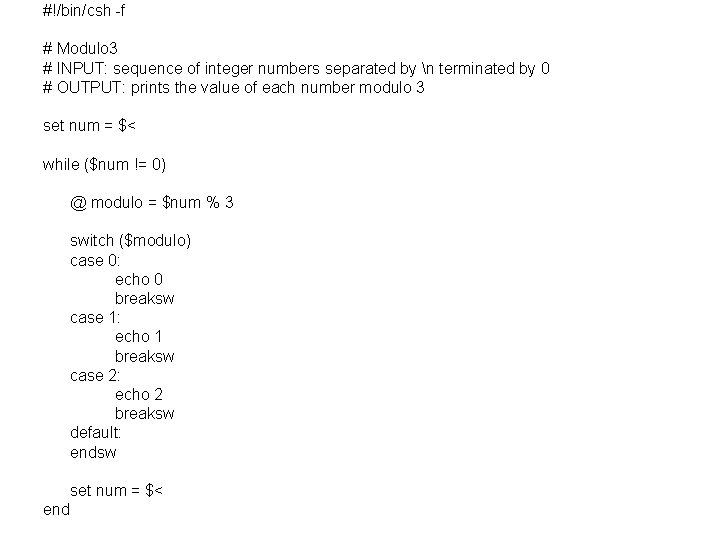
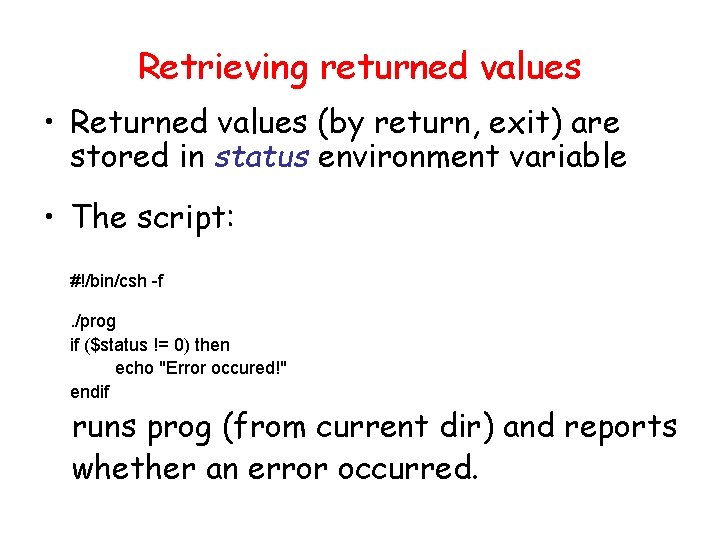
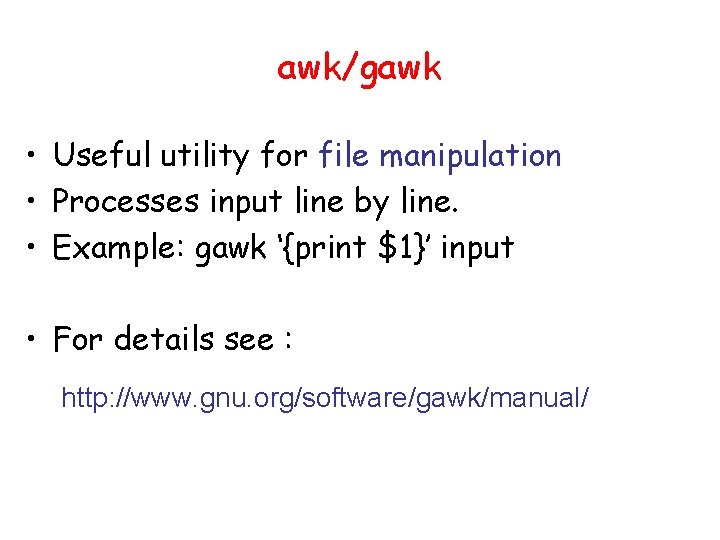
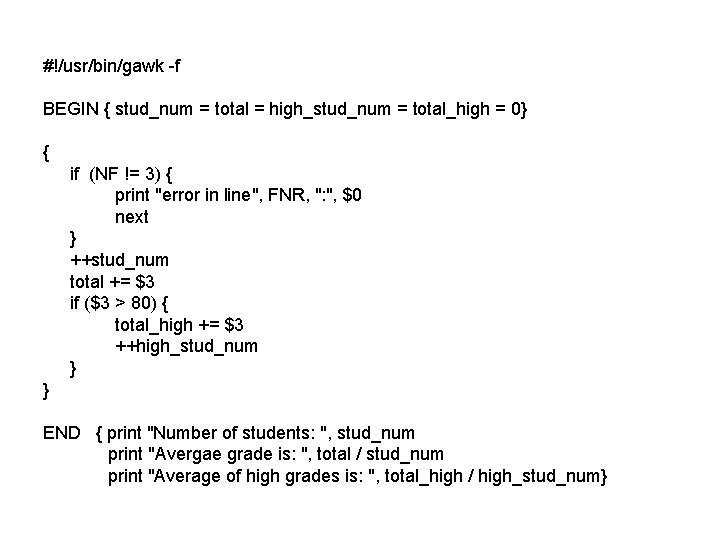
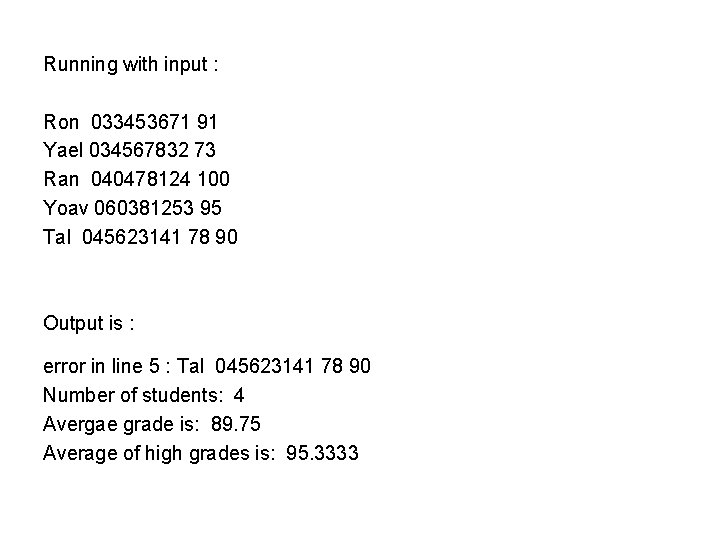
- Slides: 26
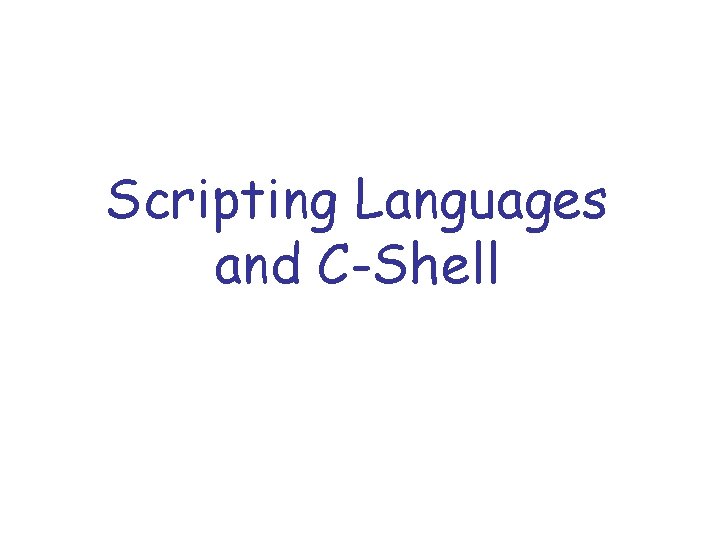
Scripting Languages and C-Shell
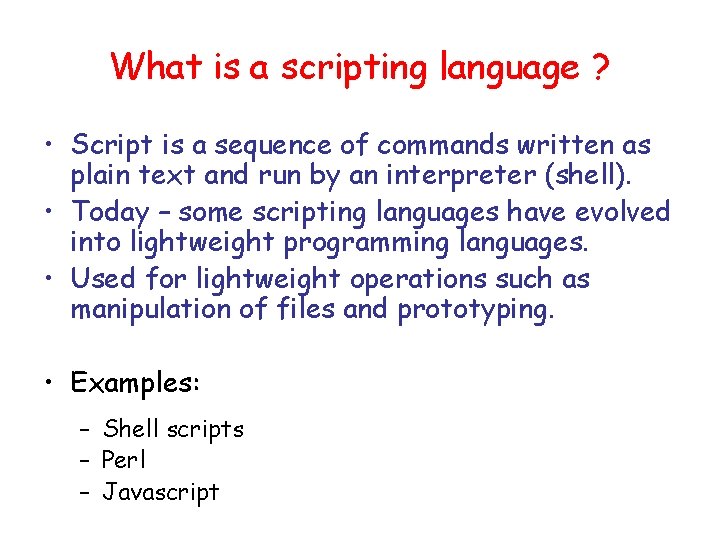
What is a scripting language ? • Script is a sequence of commands written as plain text and run by an interpreter (shell). • Today – some scripting languages have evolved into lightweight programming languages. • Used for lightweight operations such as manipulation of files and prototyping. • Examples: – Shell scripts – Perl – Javascript
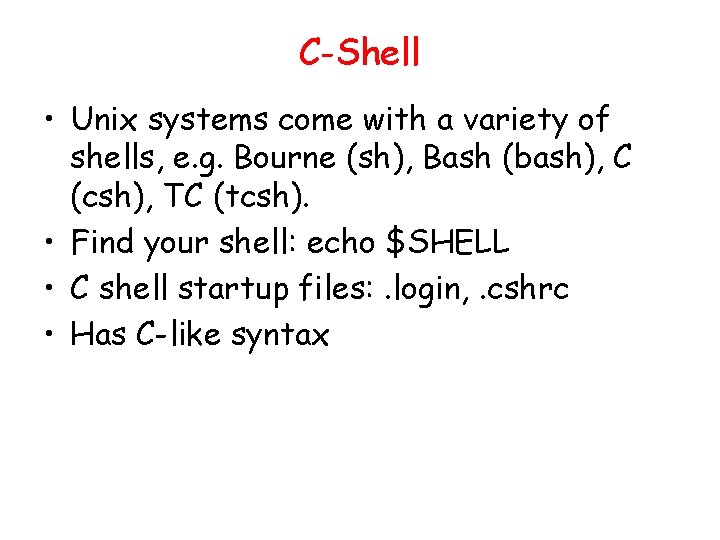
C-Shell • Unix systems come with a variety of shells, e. g. Bourne (sh), Bash (bash), C (csh), TC (tcsh). • Find your shell: echo $SHELL • C shell startup files: . login, . cshrc • Has C-like syntax
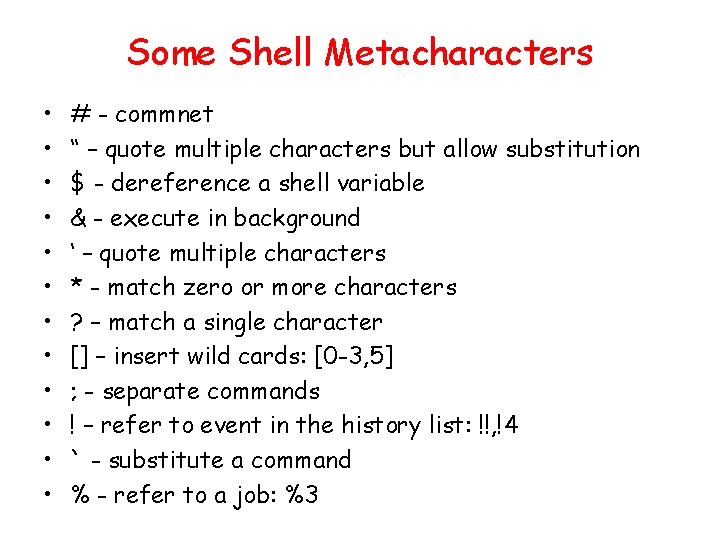
Some Shell Metacharacters • • • # - commnet “ – quote multiple characters but allow substitution $ - dereference a shell variable & - execute in background ‘ – quote multiple characters * - match zero or more characters ? – match a single character [] – insert wild cards: [0 -3, 5] ; - separate commands ! – refer to event in the history list: !!, !4 ` - substitute a command % - refer to a job: %3
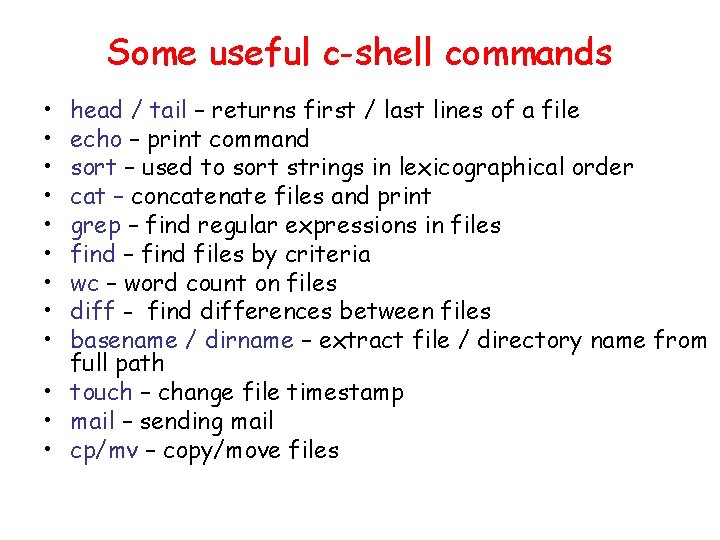
Some useful c-shell commands • • • head / tail – returns first / last lines of a file echo – print command sort – used to sort strings in lexicographical order cat – concatenate files and print grep – find regular expressions in files find – find files by criteria wc – word count on files diff - find differences between files basename / dirname – extract file / directory name from full path • touch – change file timestamp • mail – sending mail • cp/mv – copy/move files
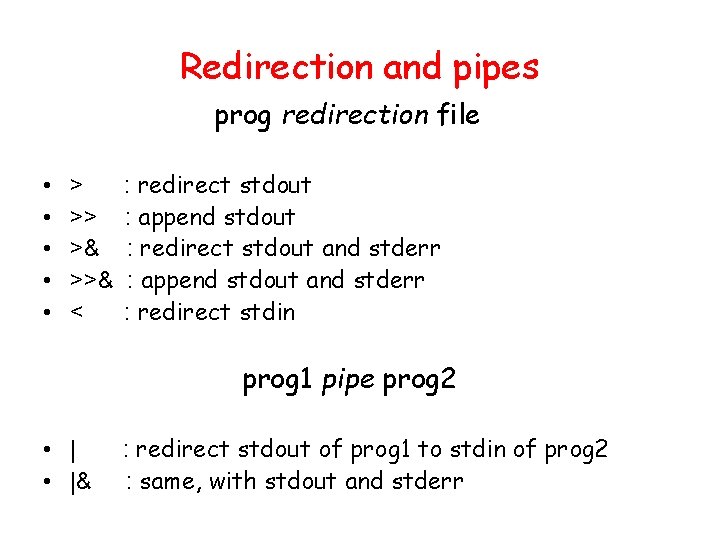
Redirection and pipes prog redirection file • • • > >> >& >>& < : redirect stdout : append stdout : redirect stdout and stderr : append stdout and stderr : redirect stdin prog 1 pipe prog 2 • |& : redirect stdout of prog 1 to stdin of prog 2 : same, with stdout and stderr
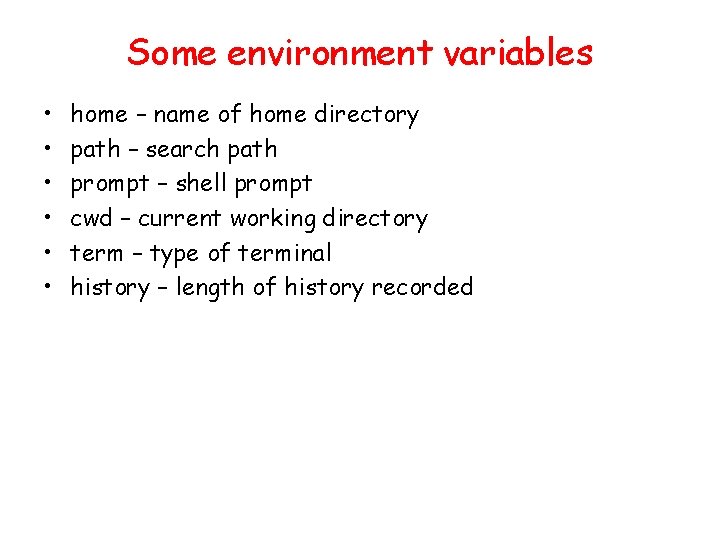
Some environment variables • • • home – name of home directory path – search path prompt – shell prompt cwd – current working directory term – type of terminal history – length of history recorded
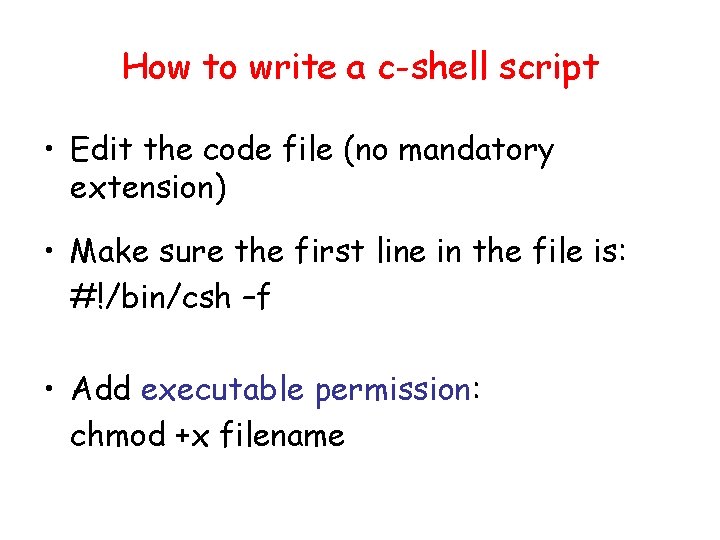
How to write a c-shell script • Edit the code file (no mandatory extension) • Make sure the first line in the file is: #!/bin/csh –f • Add executable permission: chmod +x filename
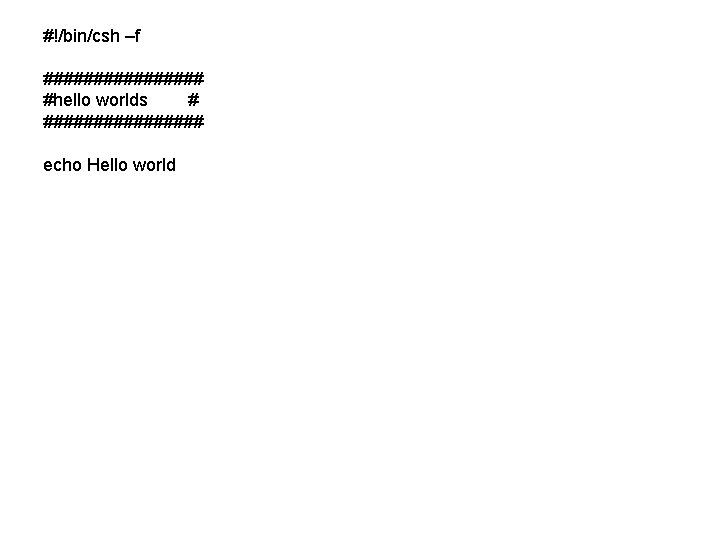
#!/bin/csh –f ######## #hello worlds # ######## echo Hello world
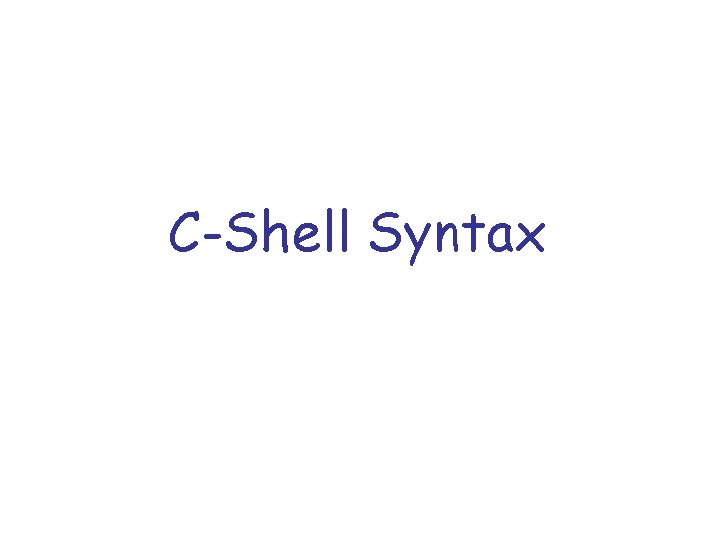
C-Shell Syntax
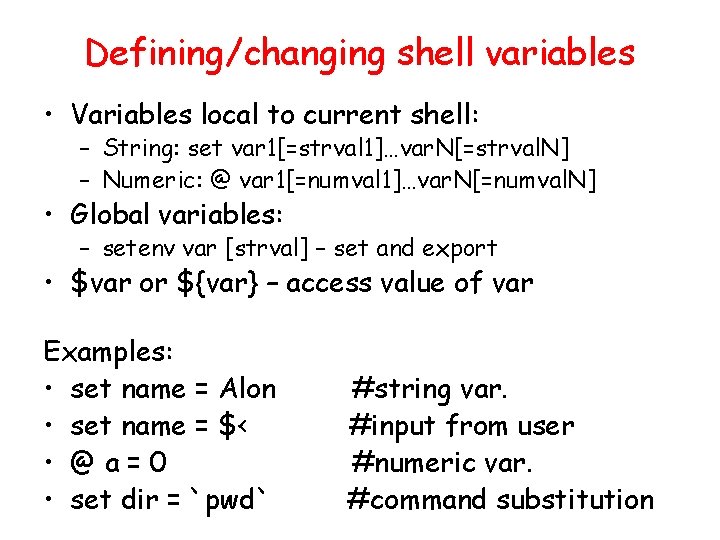
Defining/changing shell variables • Variables local to current shell: – String: set var 1[=strval 1]…var. N[=strval. N] – Numeric: @ var 1[=numval 1]…var. N[=numval. N] • Global variables: – setenv var [strval] – set and export • $var or ${var} – access value of var Examples: • set name = Alon • set name = $< • @a=0 • set dir = `pwd` #string var. #input from user #numeric var. #command substitution
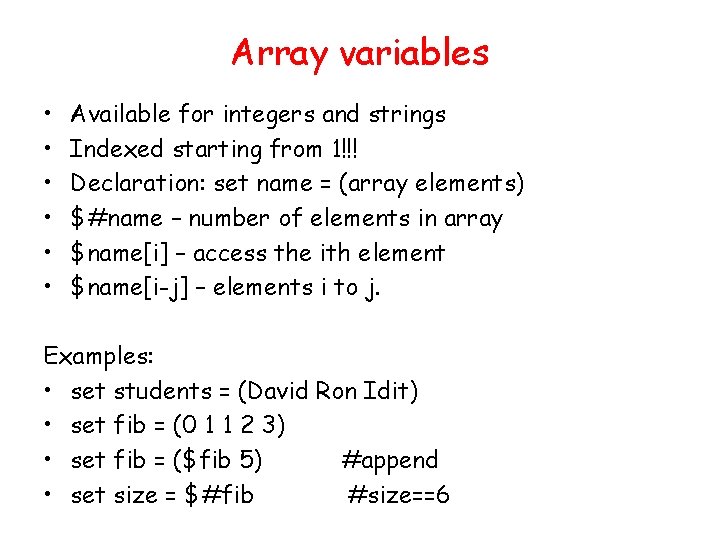
Array variables • • • Available for integers and strings Indexed starting from 1!!! Declaration: set name = (array elements) $#name – number of elements in array $name[i] – access the ith element $name[i-j] – elements i to j. Examples: • set students = (David Ron Idit) • set fib = (0 1 1 2 3) • set fib = ($fib 5) #append • set size = $#fib #size==6
![Passing arguments to scripts argv1argv9 are assigned the command line arguments argv Passing arguments to scripts • $argv[1]…$argv[9] are assigned the command line arguments • $#argv](https://slidetodoc.com/presentation_image_h2/0c4741c79aeffd633d1e310e9aa13f79/image-13.jpg)
Passing arguments to scripts • $argv[1]…$argv[9] are assigned the command line arguments • $#argv or $# - total number of arguments • $argv[*] or $* - values of all arguments • $argv[0] or $0 – name of script • shift [variable_name] – in case there are more than 9 arguments – shifts words one position to the left in variable (argv is the default).
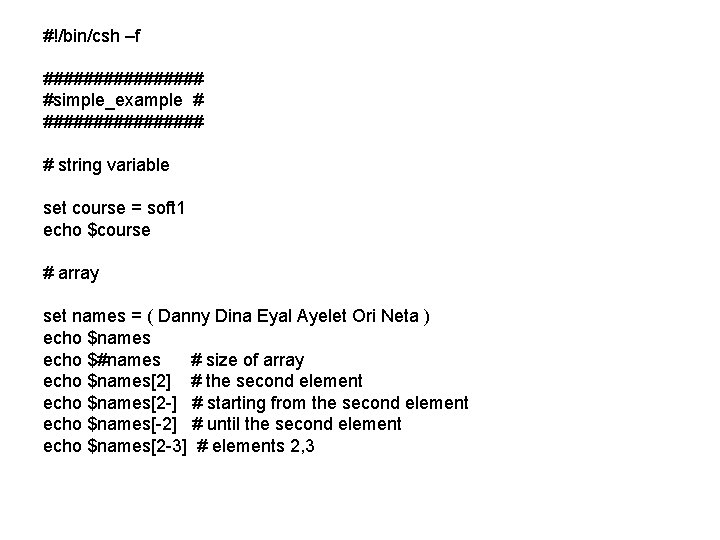
#!/bin/csh –f ######## #simple_example # ######## # string variable set course = soft 1 echo $course # array set names = ( Danny Dina Eyal Ayelet Ori Neta ) echo $names echo $#names # size of array echo $names[2] # the second element echo $names[2 -] # starting from the second element echo $names[-2] # until the second element echo $names[2 -3] # elements 2, 3
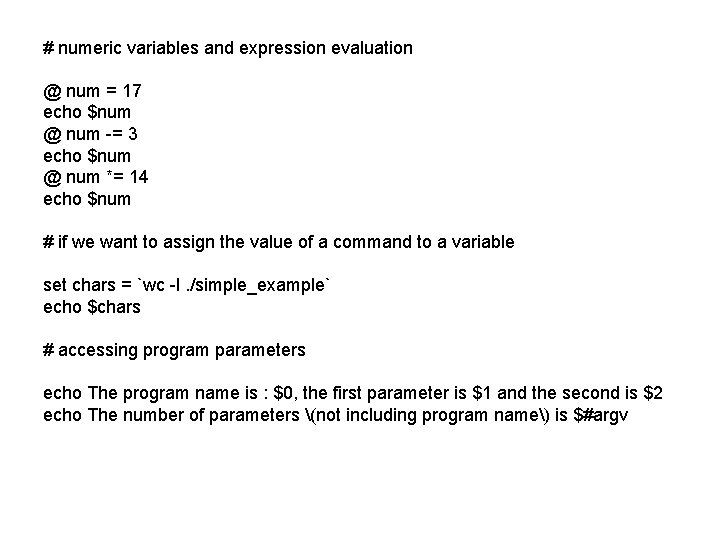
# numeric variables and expression evaluation @ num = 17 echo $num @ num -= 3 echo $num @ num *= 14 echo $num # if we want to assign the value of a command to a variable set chars = `wc -l. /simple_example` echo $chars # accessing program parameters echo The program name is : $0, the first parameter is $1 and the second is $2 echo The number of parameters (not including program name) is $#argv
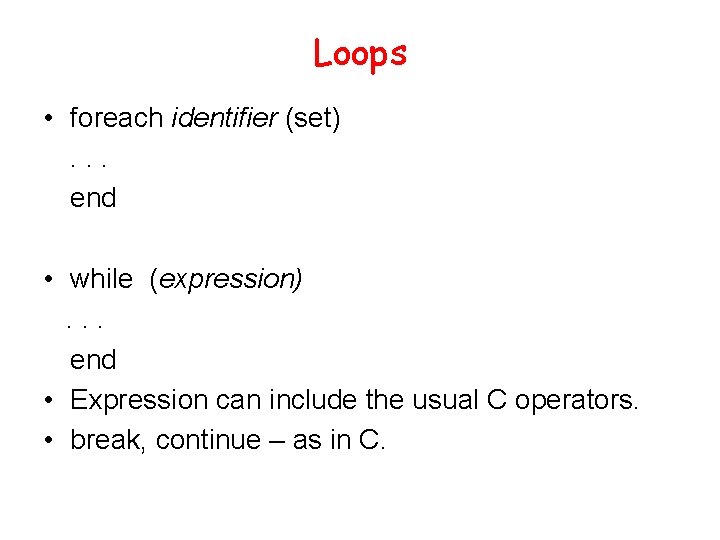
Loops • foreach identifier (set). . . end • while (expression). . . end • Expression can include the usual C operators. • break, continue – as in C.
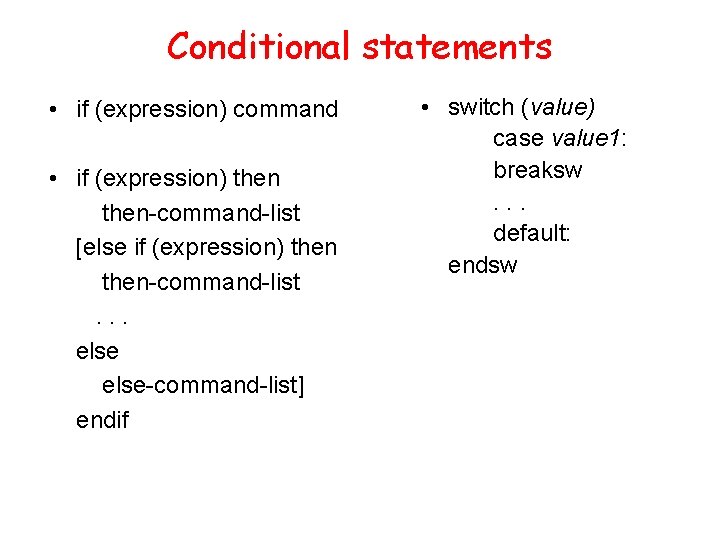
Conditional statements • if (expression) command • if (expression) then-command-list [else if (expression) then-command-list. . . else-command-list] endif • switch (value) case value 1: breaksw. . . default: endsw
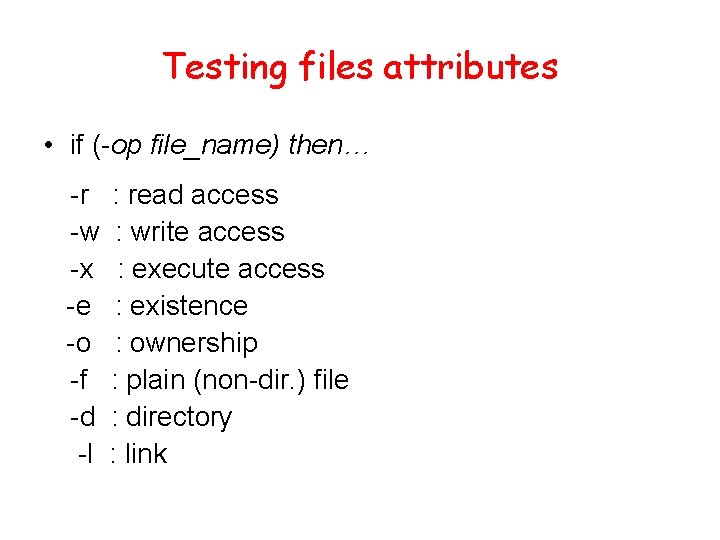
Testing files attributes • if (-op file_name) then… -r -w -x -e -o -f -d -l : read access : write access : execute access : existence : ownership : plain (non-dir. ) file : directory : link
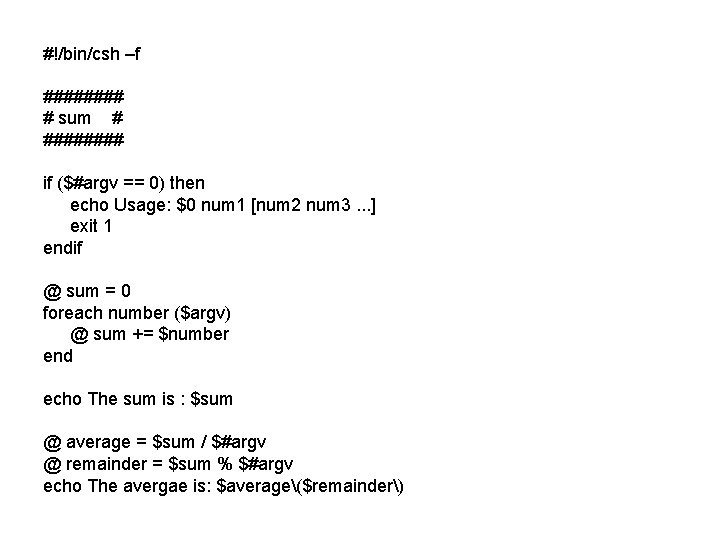
#!/bin/csh –f #### # sum # #### if ($#argv == 0) then echo Usage: $0 num 1 [num 2 num 3. . . ] exit 1 endif @ sum = 0 foreach number ($argv) @ sum += $number end echo The sum is : $sum @ average = $sum / $#argv @ remainder = $sum % $#argv echo The avergae is: $average($remainder)
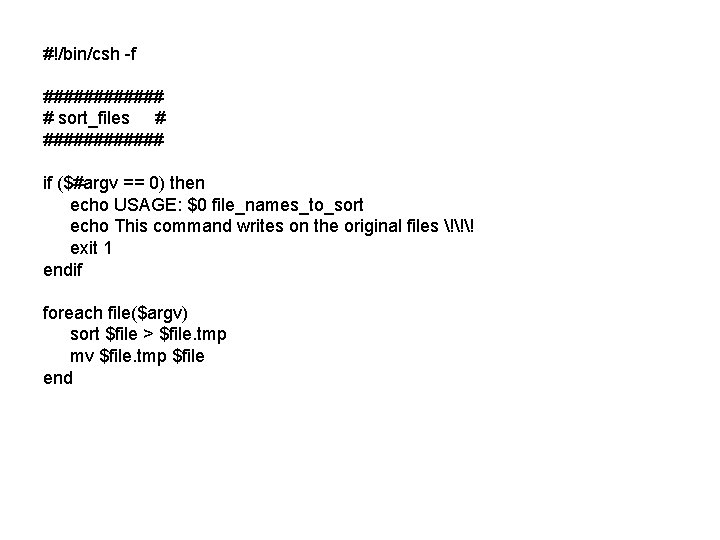
#!/bin/csh -f ###### # sort_files # ###### if ($#argv == 0) then echo USAGE: $0 file_names_to_sort echo This command writes on the original files !!! exit 1 endif foreach file($argv) sort $file > $file. tmp mv $file. tmp $file end
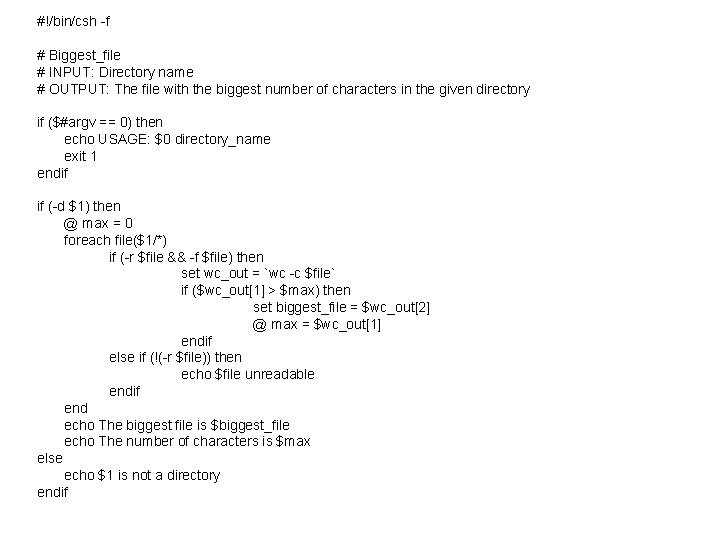
#!/bin/csh -f # Biggest_file # INPUT: Directory name # OUTPUT: The file with the biggest number of characters in the given directory if ($#argv == 0) then echo USAGE: $0 directory_name exit 1 endif if (-d $1) then @ max = 0 foreach file($1/*) if (-r $file && -f $file) then set wc_out = `wc -c $file` if ($wc_out[1] > $max) then set biggest_file = $wc_out[2] @ max = $wc_out[1] endif else if (!(-r $file)) then echo $file unreadable endif end echo The biggest file is $biggest_file echo The number of characters is $max else echo $1 is not a directory endif
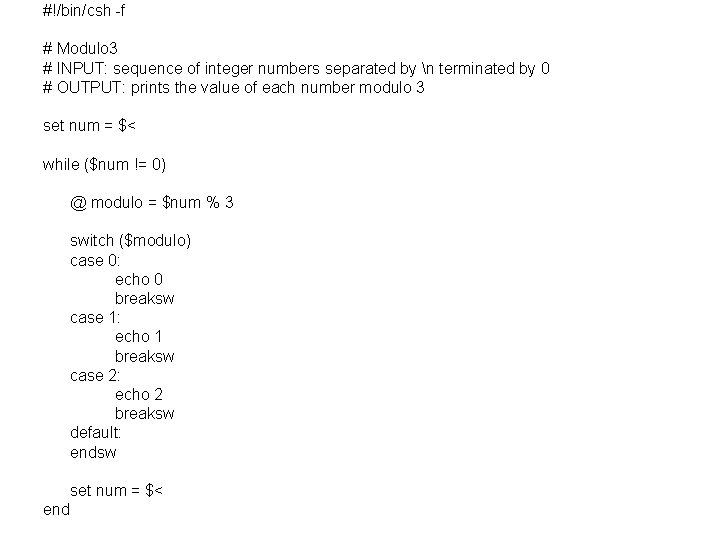
#!/bin/csh -f # Modulo 3 # INPUT: sequence of integer numbers separated by n terminated by 0 # OUTPUT: prints the value of each number modulo 3 set num = $< while ($num != 0) @ modulo = $num % 3 switch ($modulo) case 0: echo 0 breaksw case 1: echo 1 breaksw case 2: echo 2 breaksw default: endsw set num = $< end
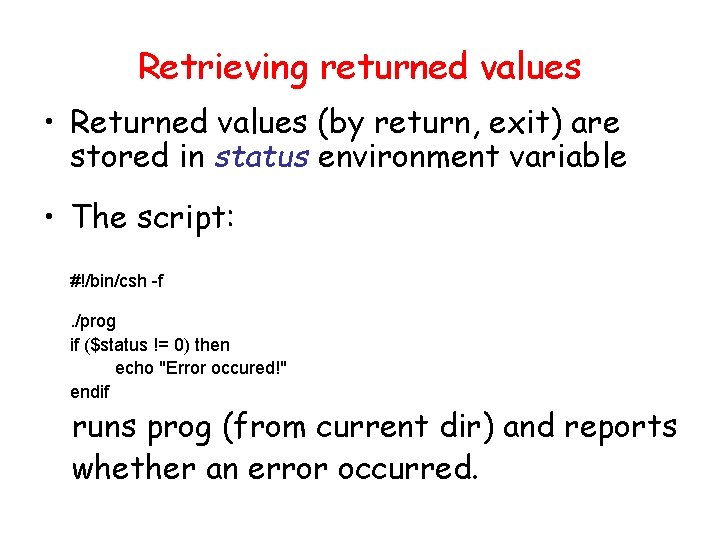
Retrieving returned values • Returned values (by return, exit) are stored in status environment variable • The script: #!/bin/csh -f. /prog if ($status != 0) then echo "Error occured!" endif runs prog (from current dir) and reports whether an error occurred.
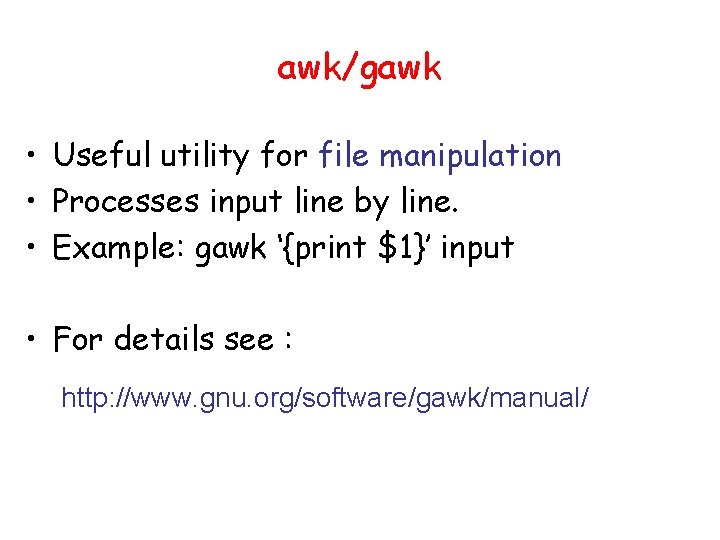
awk/gawk • Useful utility for file manipulation • Processes input line by line. • Example: gawk ‘{print $1}’ input • For details see : http: //www. gnu. org/software/gawk/manual/
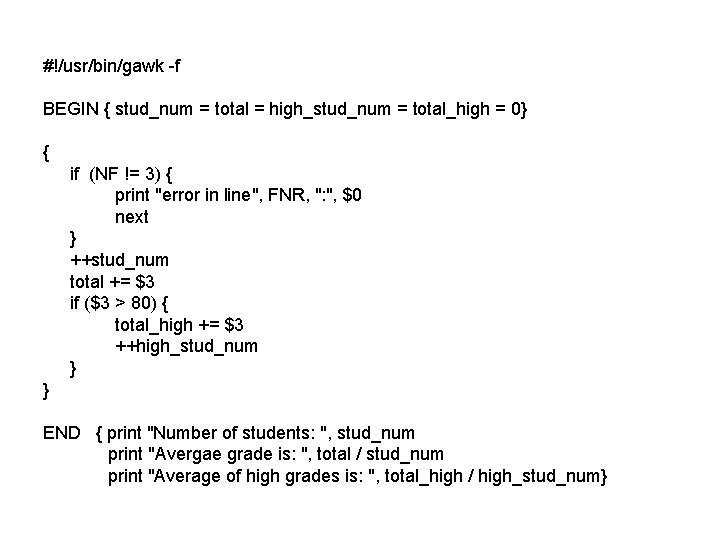
#!/usr/bin/gawk -f BEGIN { stud_num = total = high_stud_num = total_high = 0} { if (NF != 3) { print "error in line", FNR, ": ", $0 next } ++stud_num total += $3 if ($3 > 80) { total_high += $3 ++high_stud_num } } END { print "Number of students: ", stud_num print "Avergae grade is: ", total / stud_num print "Average of high grades is: ", total_high / high_stud_num}
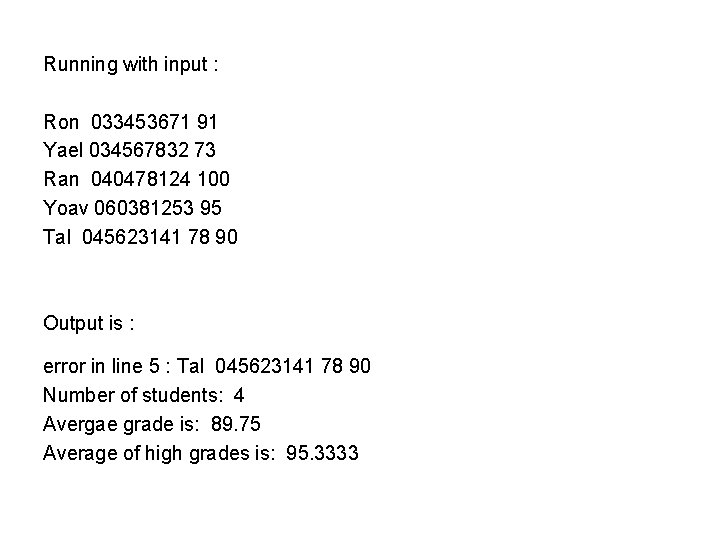
Running with input : Ron 033453671 91 Yael 034567832 73 Ran 040478124 100 Yoav 060381253 95 Tal 045623141 78 90 Output is : error in line 5 : Tal 045623141 78 90 Number of students: 4 Avergae grade is: 89. 75 Average of high grades is: 95. 3333
Introduction to scripting languages
Common characteristics of scripting languages
Explain the innovative features of scripting languages.
Innovative features of scripting language
Language
Innovative features of scripting languages
Lab 7-7 shells scripting and data management
Advantages of client side scripting
Inventor scripting
Linden scripting language
Loadrunner scripting language
Gelscripting
Client-side scripting examples
Tabular editor perspectives
Elongday
Sage 100 scripting
Paraview python scripting
Xss steal cookie
Swift (parallel scripting language)
Pop_subcomp
Common cause of buffer overflow cross-site scripting
Scripting image
Scripting image
Neoload scripting
Boolean algebra
Linear scripting framework
What is server side programming