Repetition In todays lesson we will look at
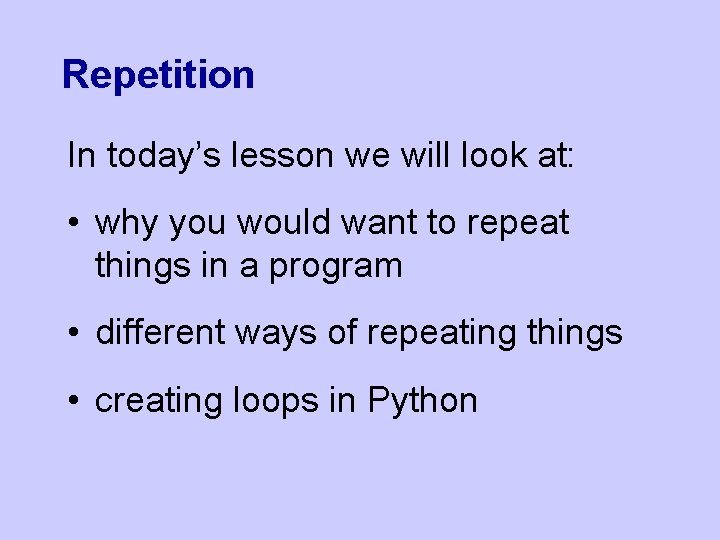
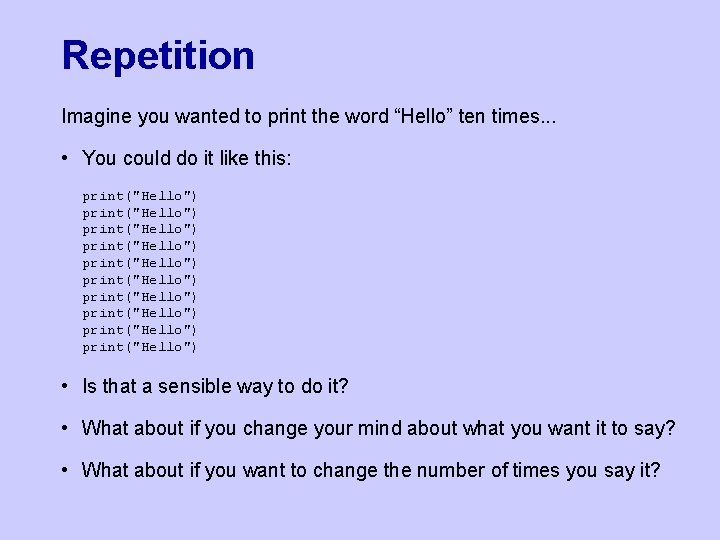
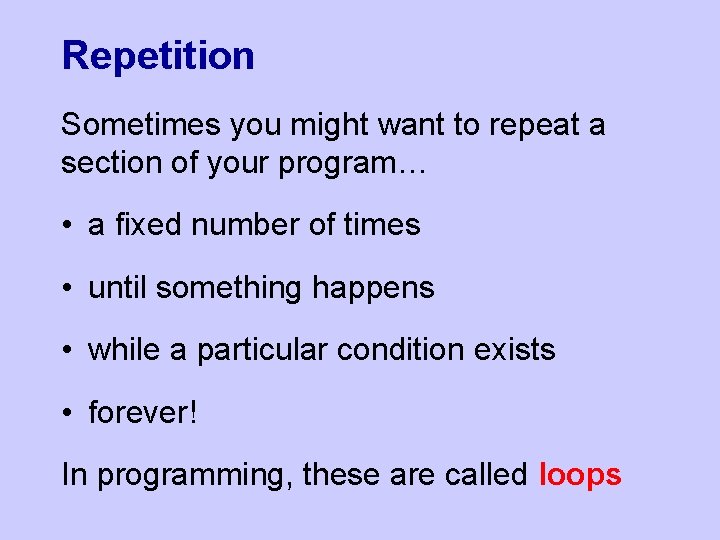
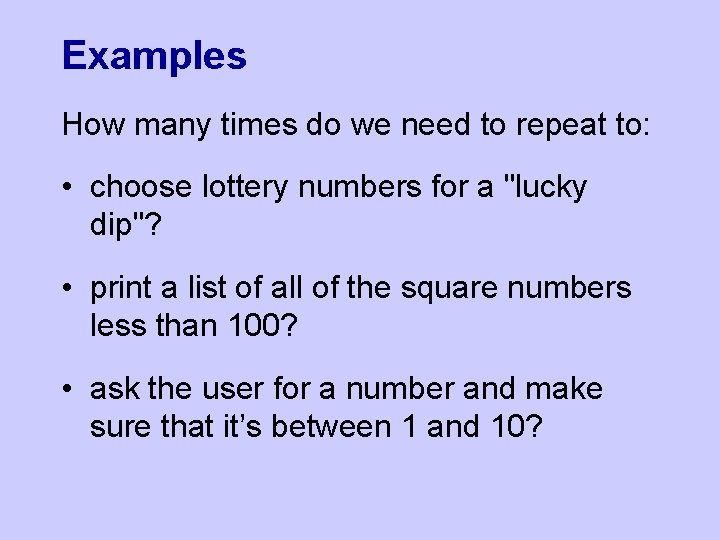
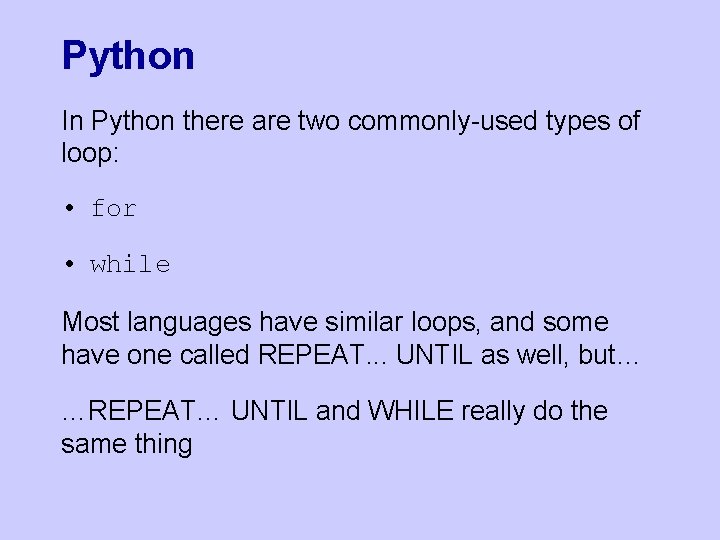
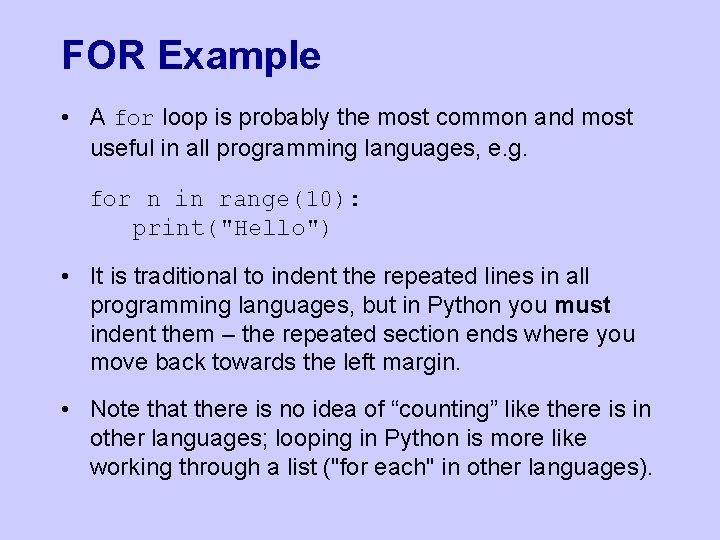
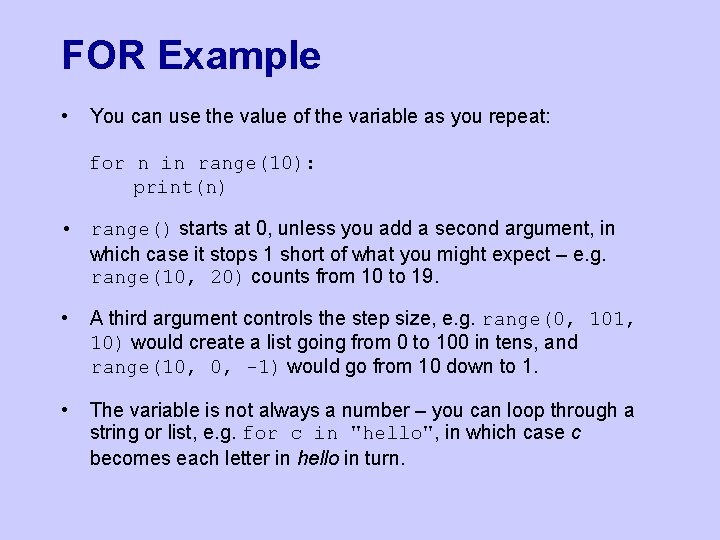
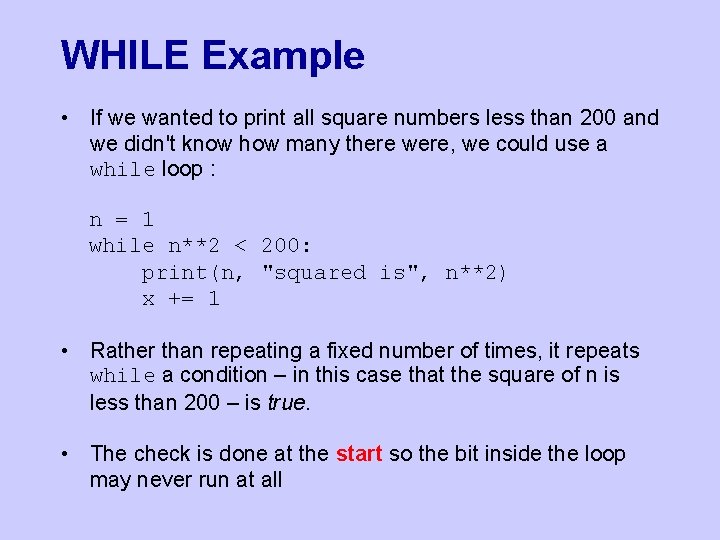
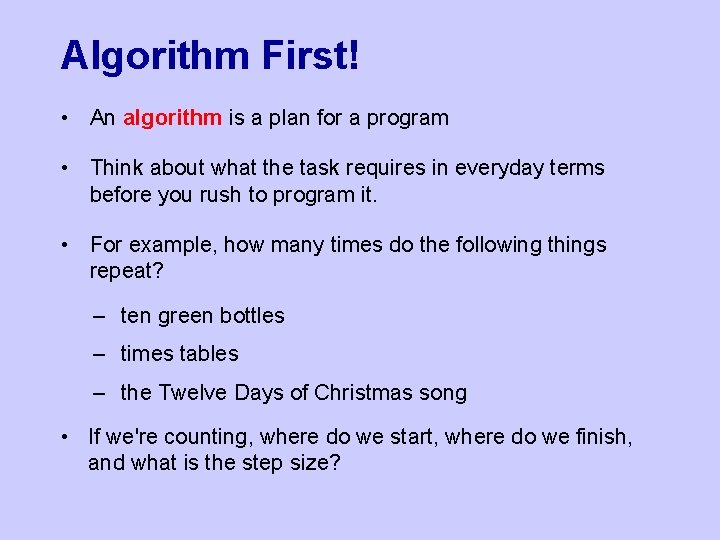
- Slides: 9
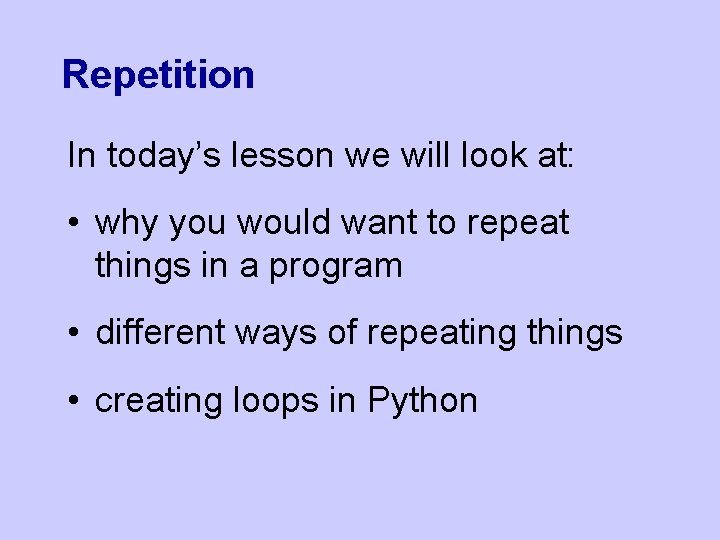
Repetition In today’s lesson we will look at: • why you would want to repeat things in a program • different ways of repeating things • creating loops in Python
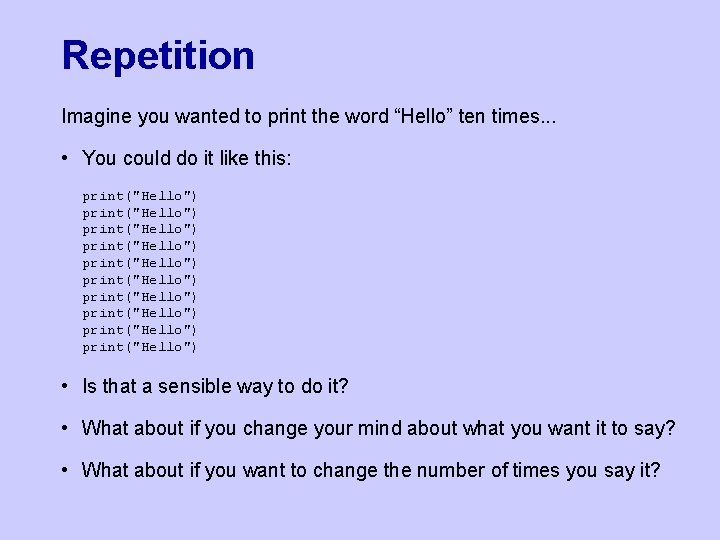
Repetition Imagine you wanted to print the word “Hello” ten times. . . • You could do it like this: print("Hello") print("Hello") print("Hello") • Is that a sensible way to do it? • What about if you change your mind about what you want it to say? • What about if you want to change the number of times you say it?
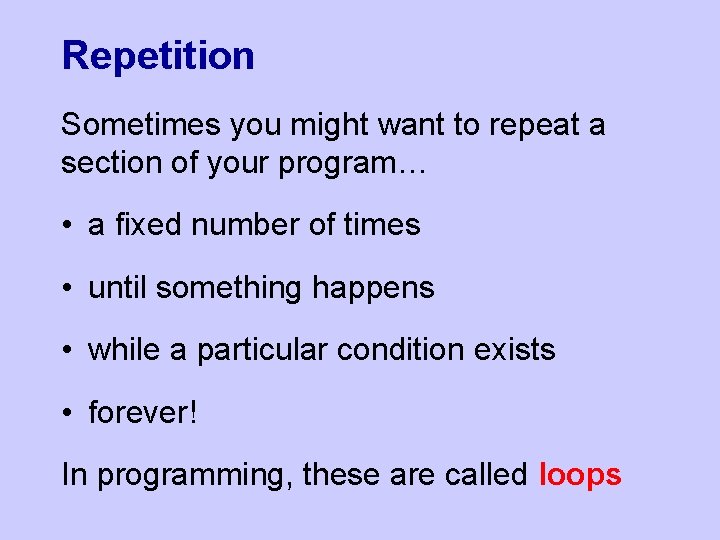
Repetition Sometimes you might want to repeat a section of your program… • a fixed number of times • until something happens • while a particular condition exists • forever! In programming, these are called loops
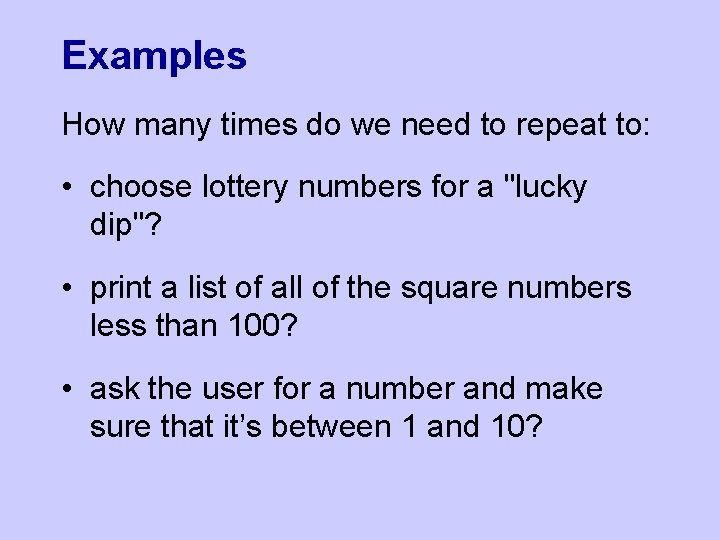
Examples How many times do we need to repeat to: • choose lottery numbers for a "lucky dip"? • print a list of all of the square numbers less than 100? • ask the user for a number and make sure that it’s between 1 and 10?
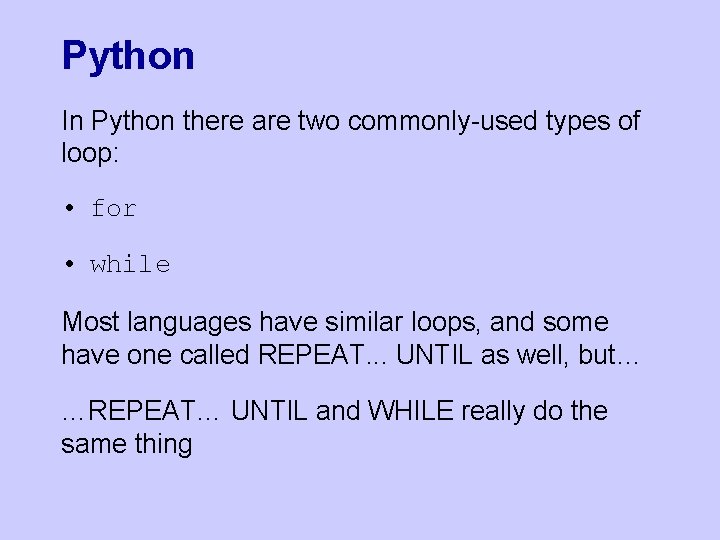
Python In Python there are two commonly-used types of loop: • for • while Most languages have similar loops, and some have one called REPEAT. . . UNTIL as well, but… …REPEAT… UNTIL and WHILE really do the same thing
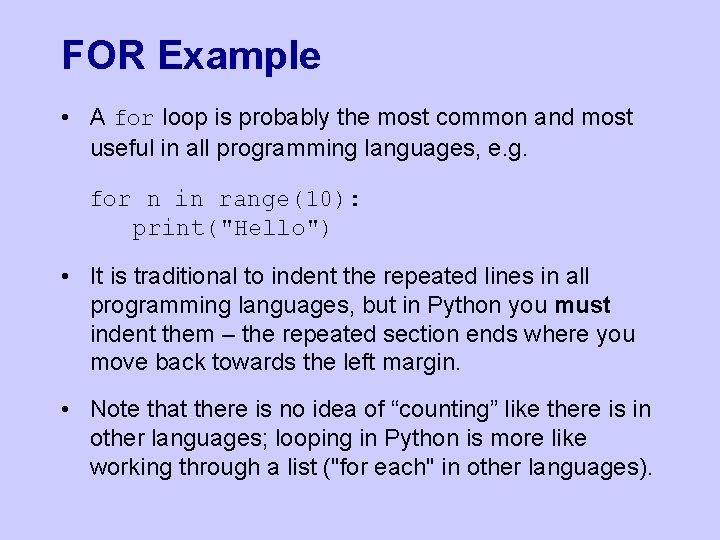
FOR Example • A for loop is probably the most common and most useful in all programming languages, e. g. for n in range(10): print("Hello") • It is traditional to indent the repeated lines in all programming languages, but in Python you must indent them – the repeated section ends where you move back towards the left margin. • Note that there is no idea of “counting” like there is in other languages; looping in Python is more like working through a list ("for each" in other languages).
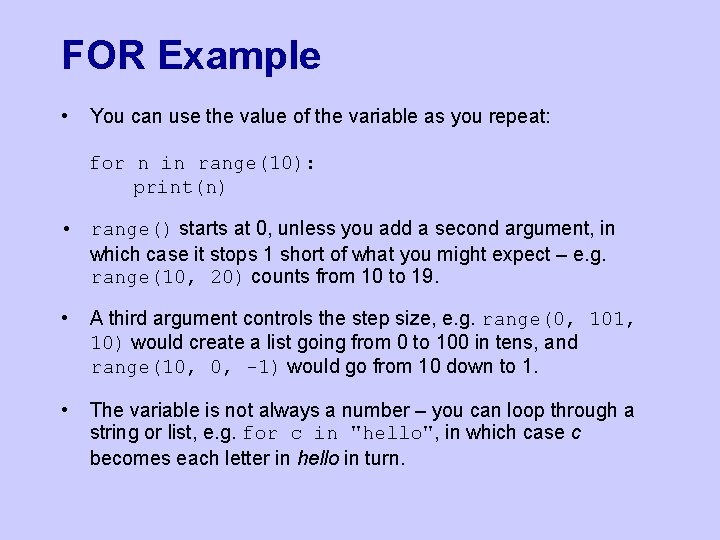
FOR Example • You can use the value of the variable as you repeat: for n in range(10): print(n) • range() starts at 0, unless you add a second argument, in which case it stops 1 short of what you might expect – e. g. range(10, 20) counts from 10 to 19. • A third argument controls the step size, e. g. range(0, 101, 10) would create a list going from 0 to 100 in tens, and range(10, 0, -1) would go from 10 down to 1. • The variable is not always a number – you can loop through a string or list, e. g. for c in "hello", in which case c becomes each letter in hello in turn.
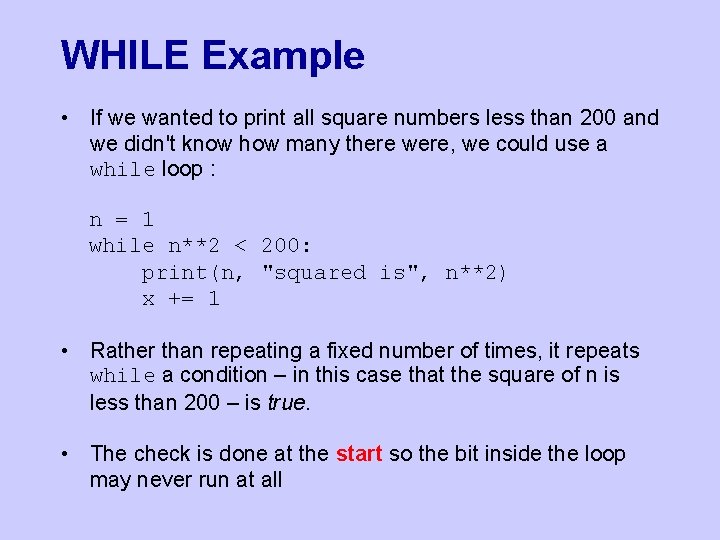
WHILE Example • If we wanted to print all square numbers less than 200 and we didn't know how many there were, we could use a while loop : n = 1 while n**2 < 200: print(n, "squared is", n**2) x += 1 • Rather than repeating a fixed number of times, it repeats while a condition – in this case that the square of n is less than 200 – is true. • The check is done at the start so the bit inside the loop may never run at all
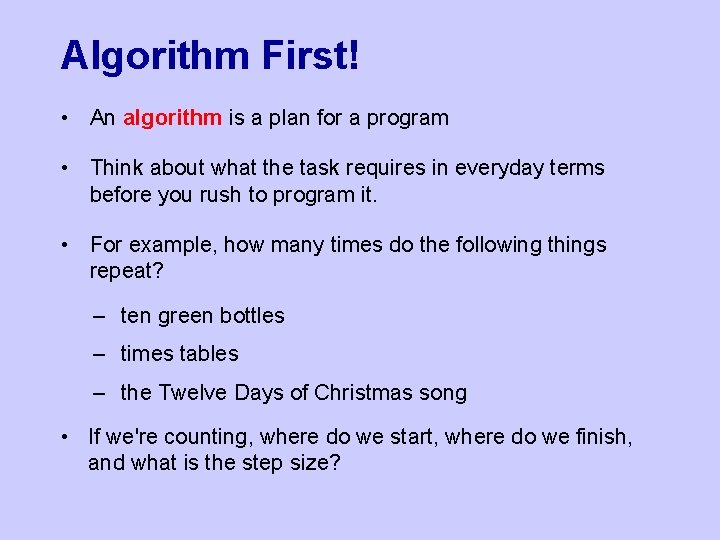
Algorithm First! • An algorithm is a plan for a program • Think about what the task requires in everyday terms before you rush to program it. • For example, how many times do the following things repeat? – ten green bottles – times tables – the Twelve Days of Christmas song • If we're counting, where do we start, where do we finish, and what is the step size?