Repetition In todays lesson we will look at
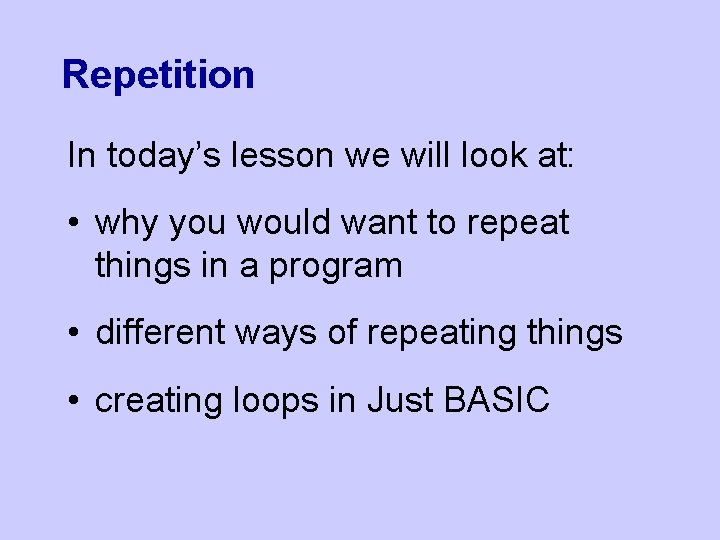
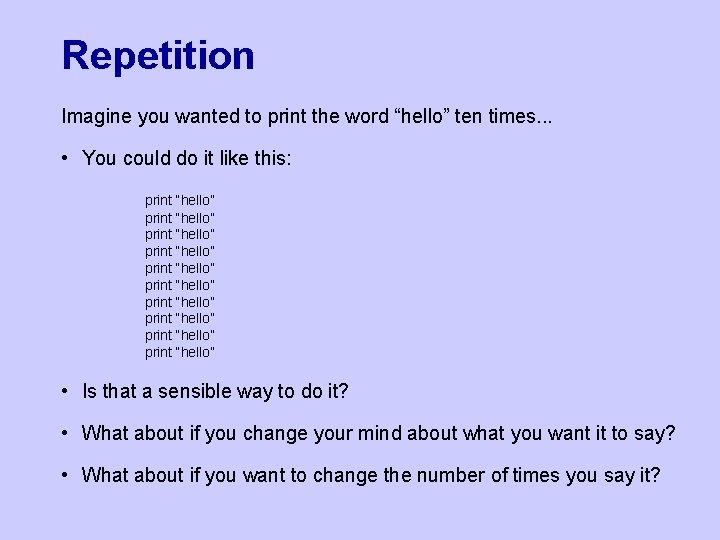
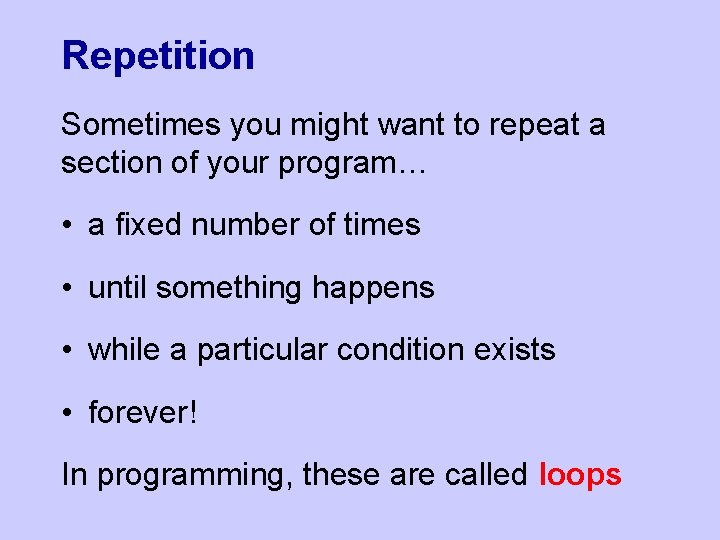
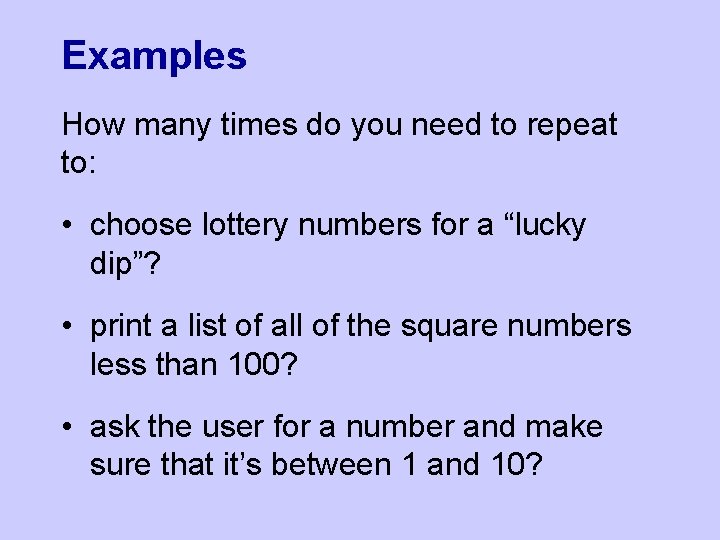
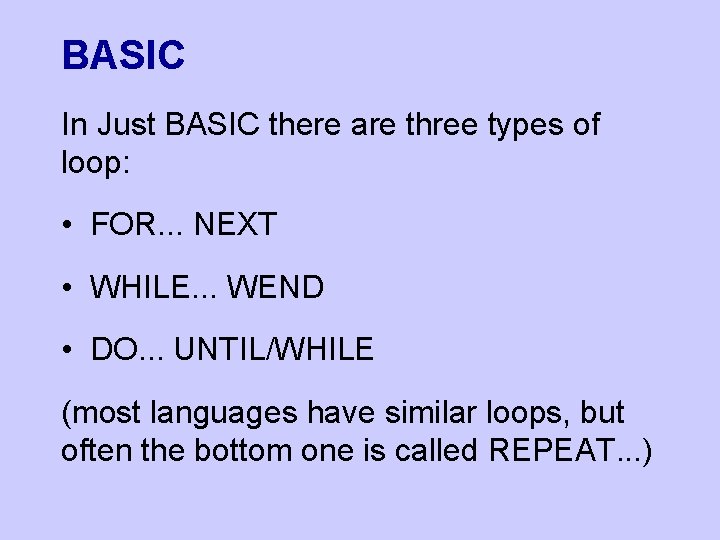
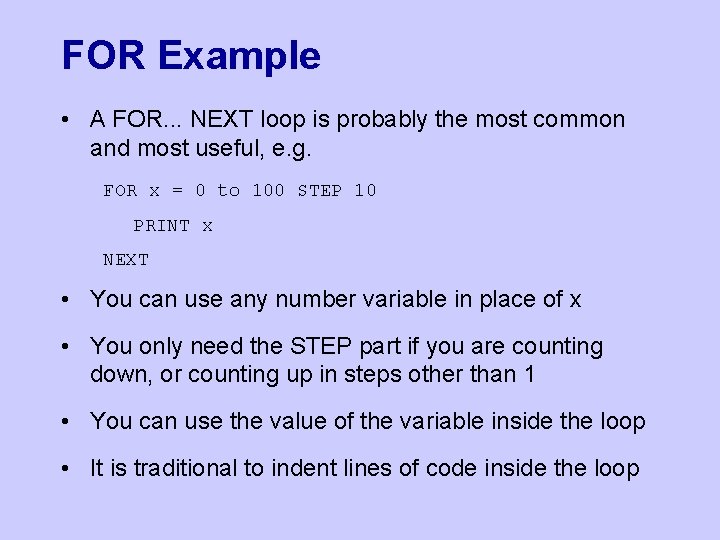
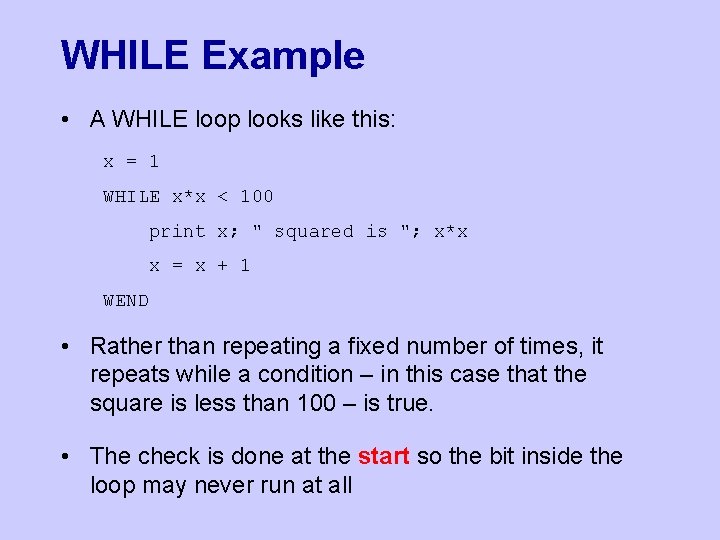
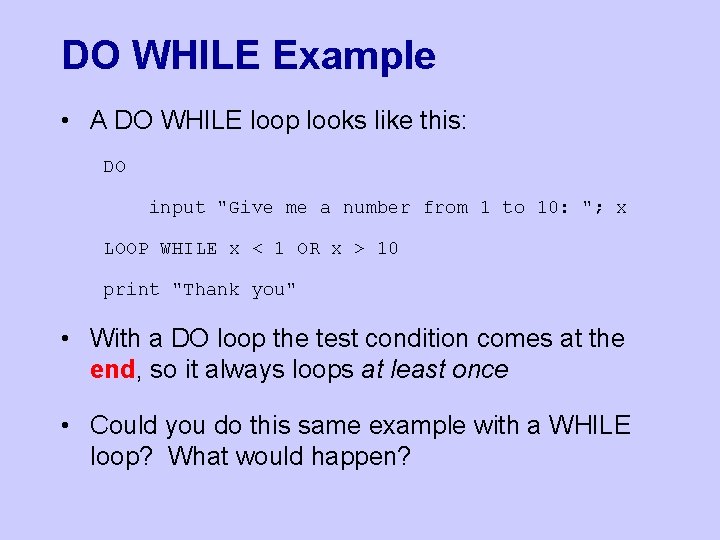
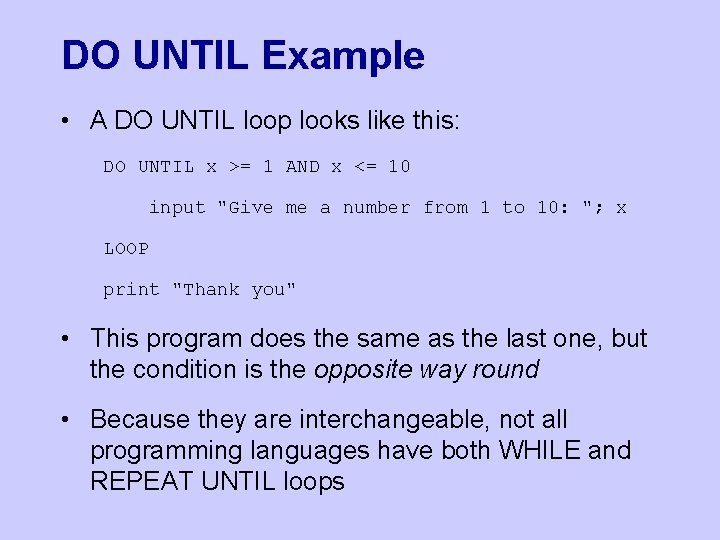
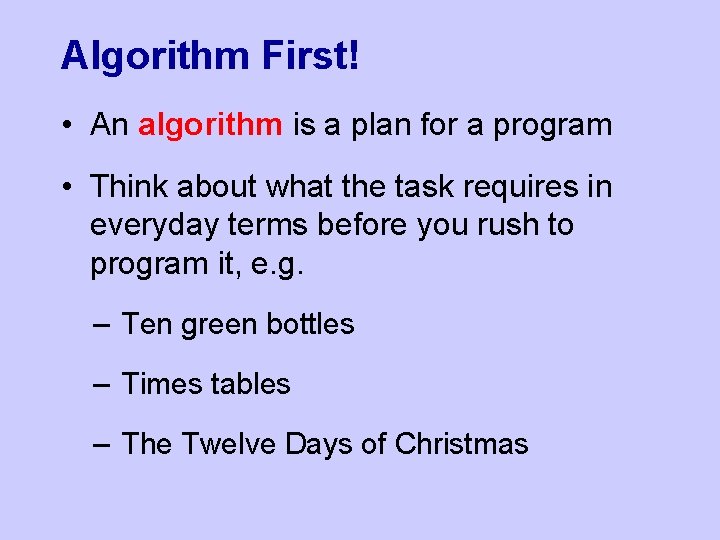
- Slides: 10
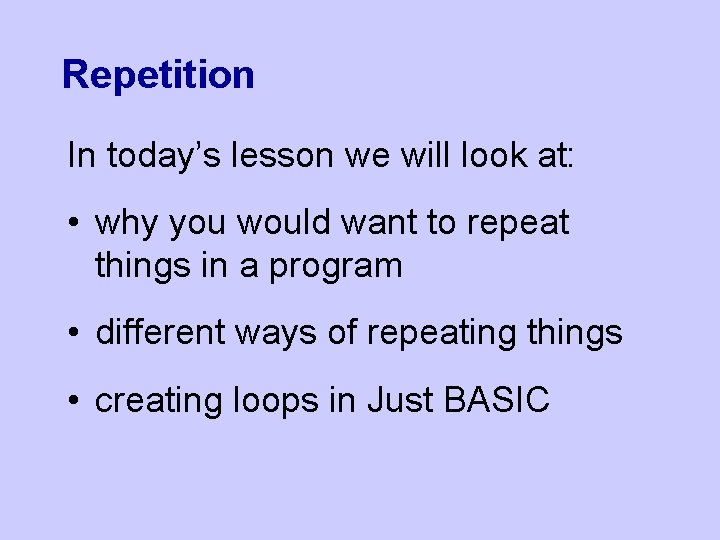
Repetition In today’s lesson we will look at: • why you would want to repeat things in a program • different ways of repeating things • creating loops in Just BASIC
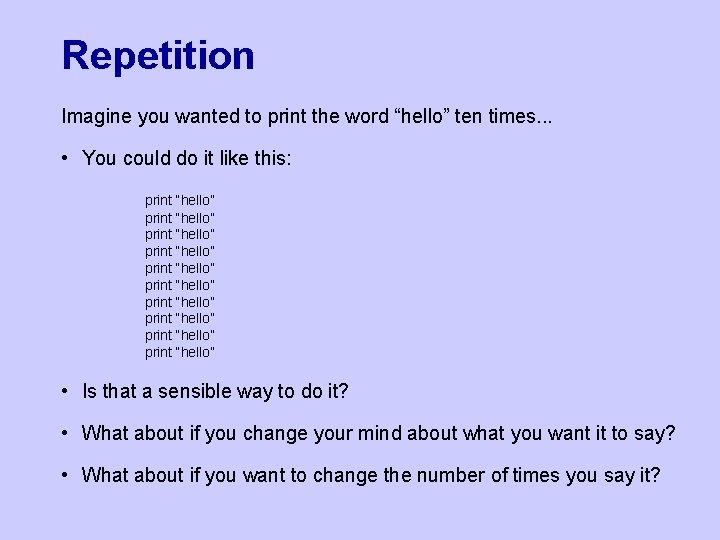
Repetition Imagine you wanted to print the word “hello” ten times. . . • You could do it like this: print “hello” print “hello” print “hello” • Is that a sensible way to do it? • What about if you change your mind about what you want it to say? • What about if you want to change the number of times you say it?
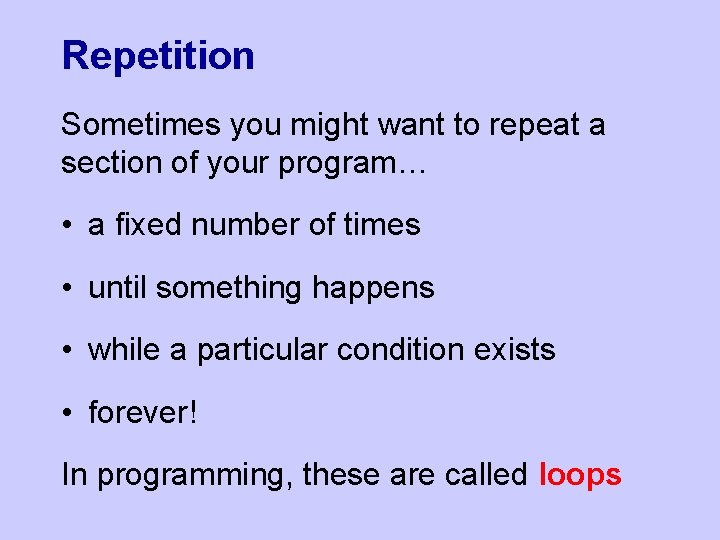
Repetition Sometimes you might want to repeat a section of your program… • a fixed number of times • until something happens • while a particular condition exists • forever! In programming, these are called loops
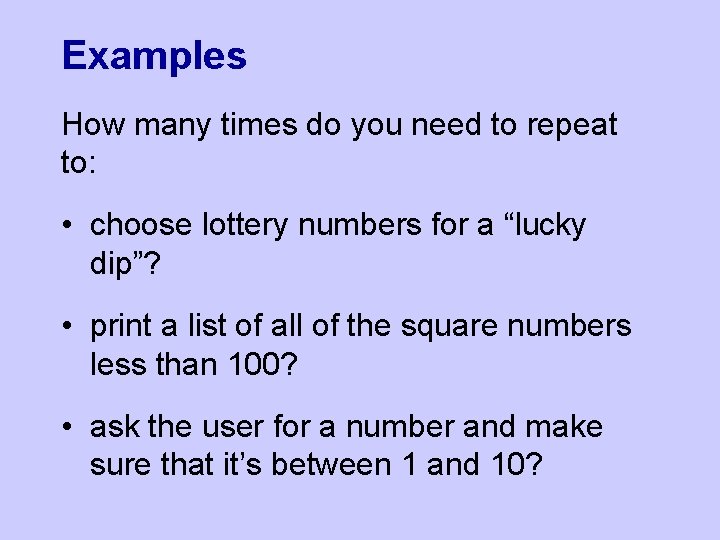
Examples How many times do you need to repeat to: • choose lottery numbers for a “lucky dip”? • print a list of all of the square numbers less than 100? • ask the user for a number and make sure that it’s between 1 and 10?
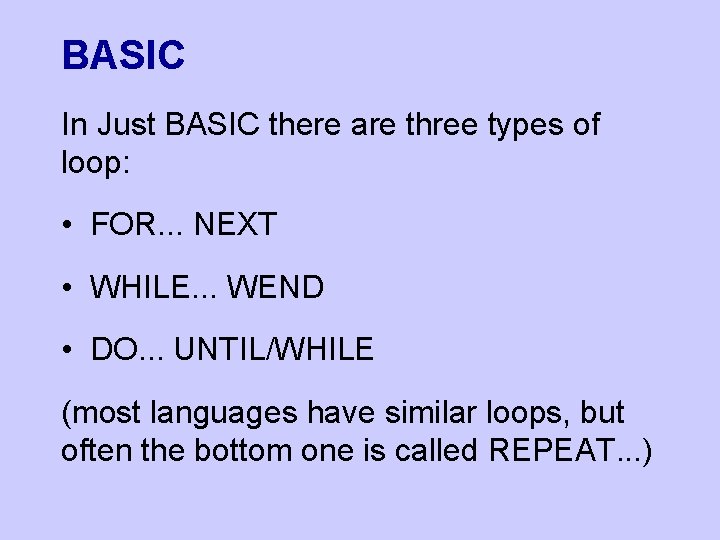
BASIC In Just BASIC there are three types of loop: • FOR. . . NEXT • WHILE. . . WEND • DO. . . UNTIL/WHILE (most languages have similar loops, but often the bottom one is called REPEAT. . . )
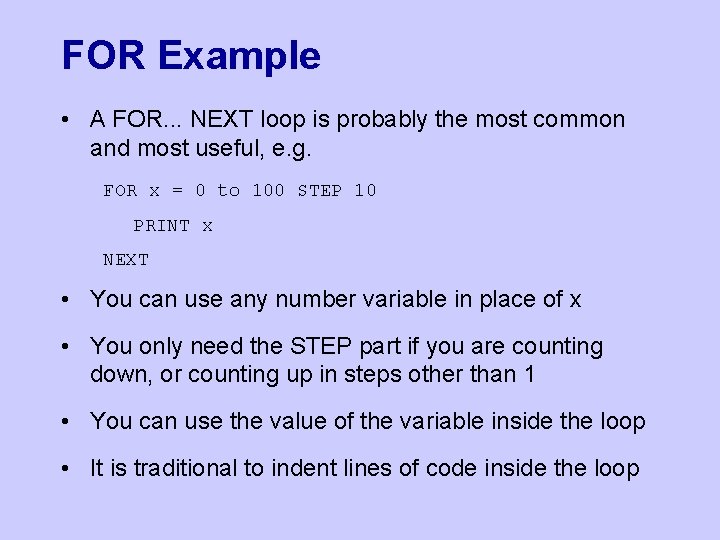
FOR Example • A FOR. . . NEXT loop is probably the most common and most useful, e. g. FOR x = 0 to 100 STEP 10 PRINT x NEXT • You can use any number variable in place of x • You only need the STEP part if you are counting down, or counting up in steps other than 1 • You can use the value of the variable inside the loop • It is traditional to indent lines of code inside the loop
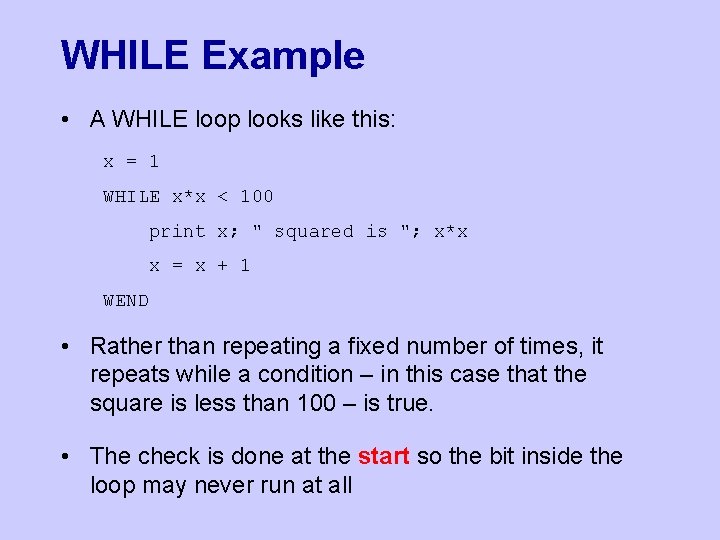
WHILE Example • A WHILE loop looks like this: x = 1 WHILE x*x < 100 print x; " squared is "; x*x x = x + 1 WEND • Rather than repeating a fixed number of times, it repeats while a condition – in this case that the square is less than 100 – is true. • The check is done at the start so the bit inside the loop may never run at all
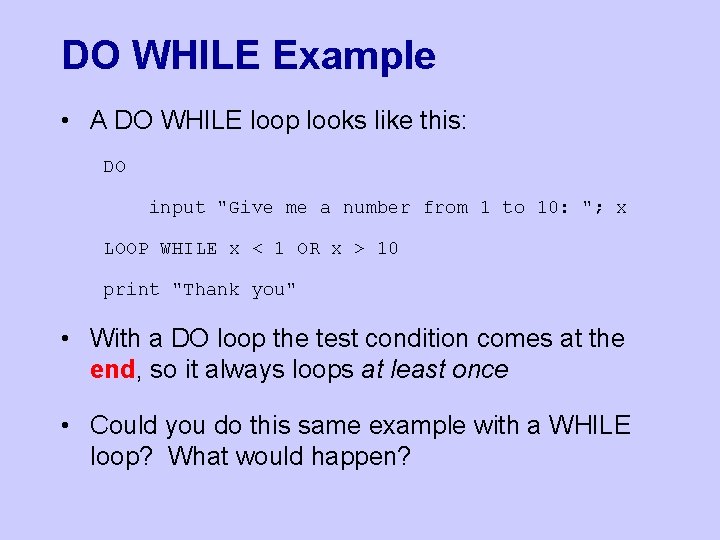
DO WHILE Example • A DO WHILE loop looks like this: DO input "Give me a number from 1 to 10: "; x LOOP WHILE x < 1 OR x > 10 print "Thank you" • With a DO loop the test condition comes at the end, so it always loops at least once • Could you do this same example with a WHILE loop? What would happen?
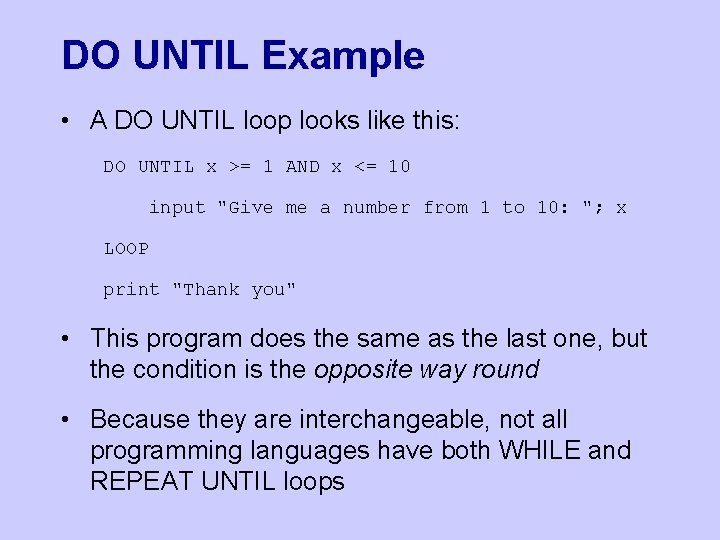
DO UNTIL Example • A DO UNTIL loop looks like this: DO UNTIL x >= 1 AND x <= 10 input "Give me a number from 1 to 10: "; x LOOP print "Thank you" • This program does the same as the last one, but the condition is the opposite way round • Because they are interchangeable, not all programming languages have both WHILE and REPEAT UNTIL loops
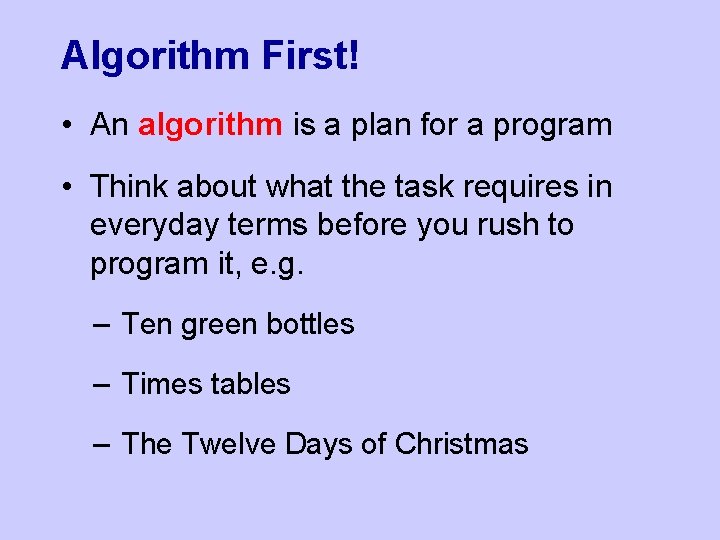
Algorithm First! • An algorithm is a plan for a program • Think about what the task requires in everyday terms before you rush to program it, e. g. – Ten green bottles – Times tables – The Twelve Days of Christmas