Referring to Java API Specifications http java sun
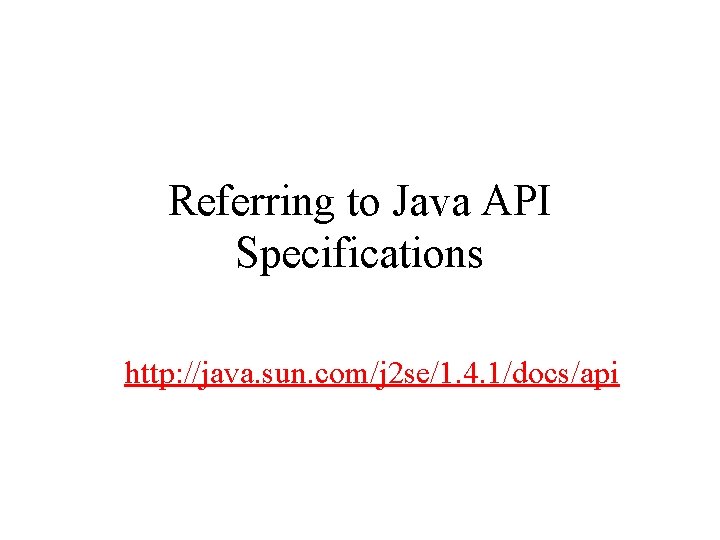
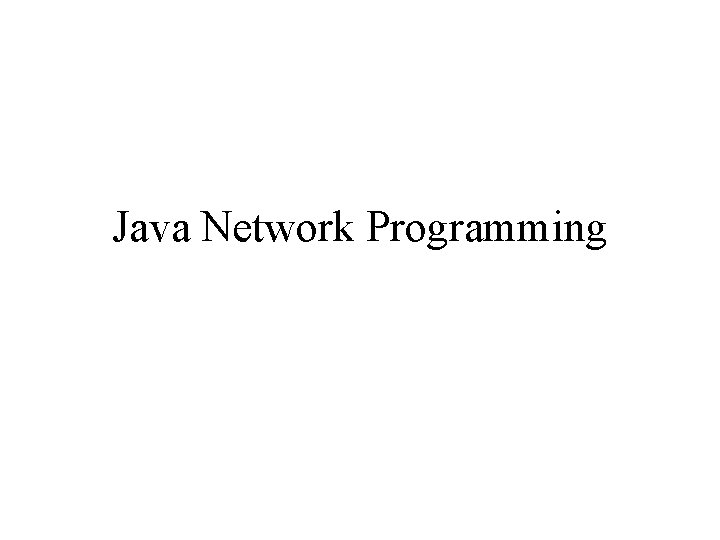
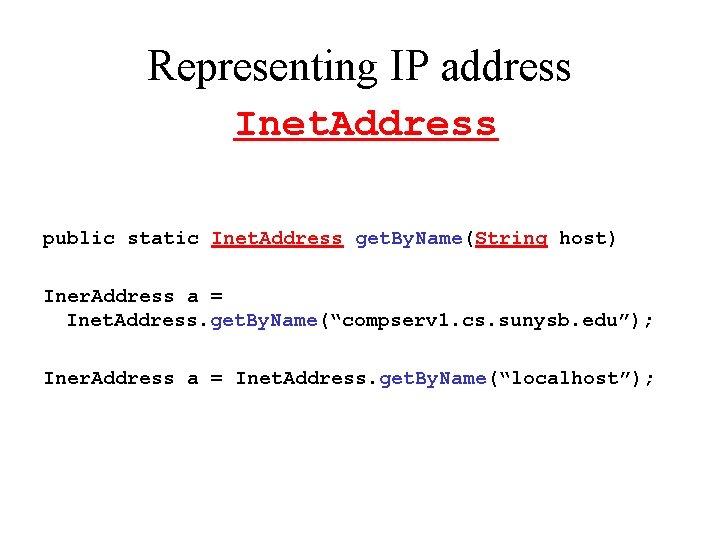
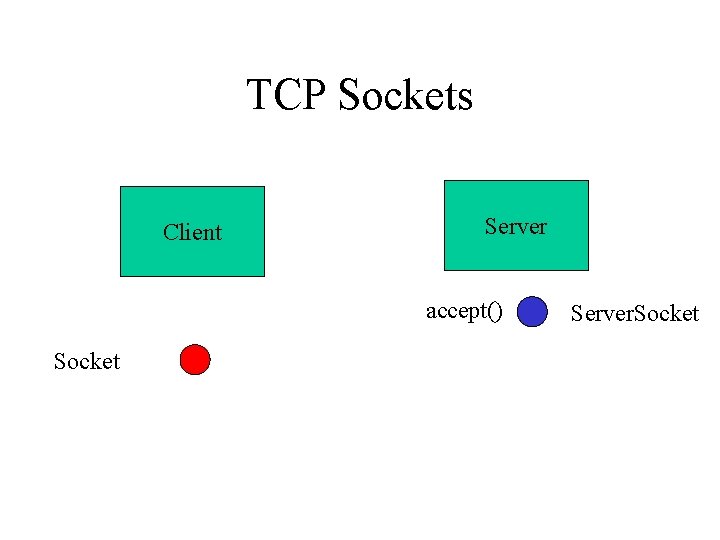
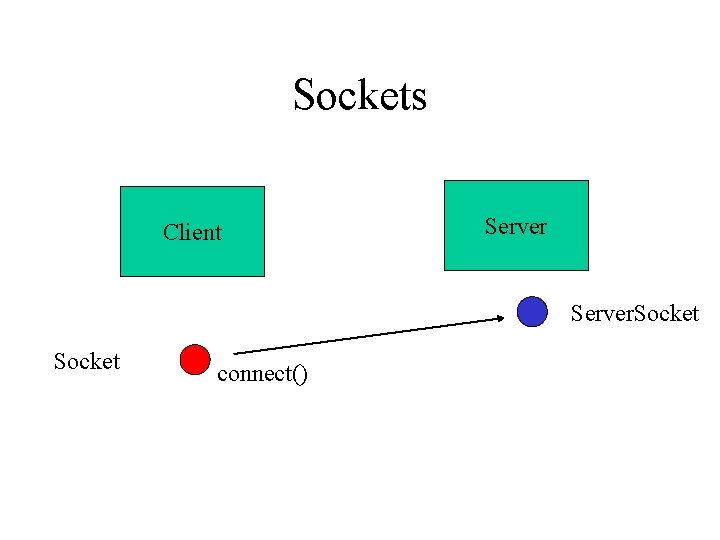
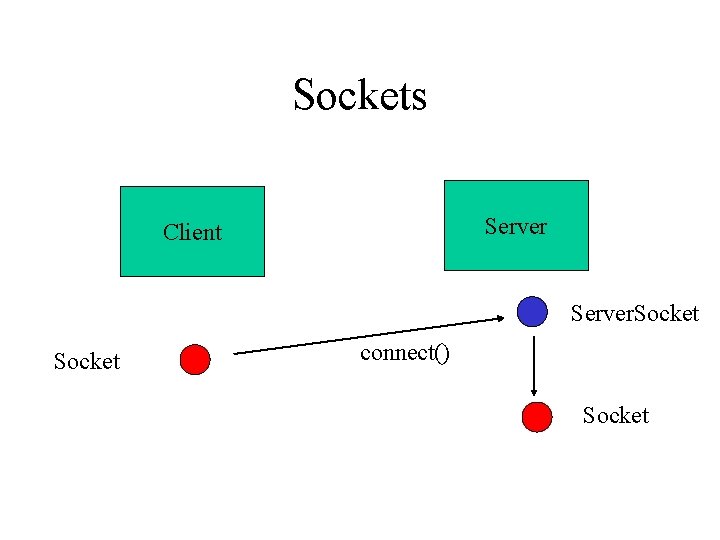
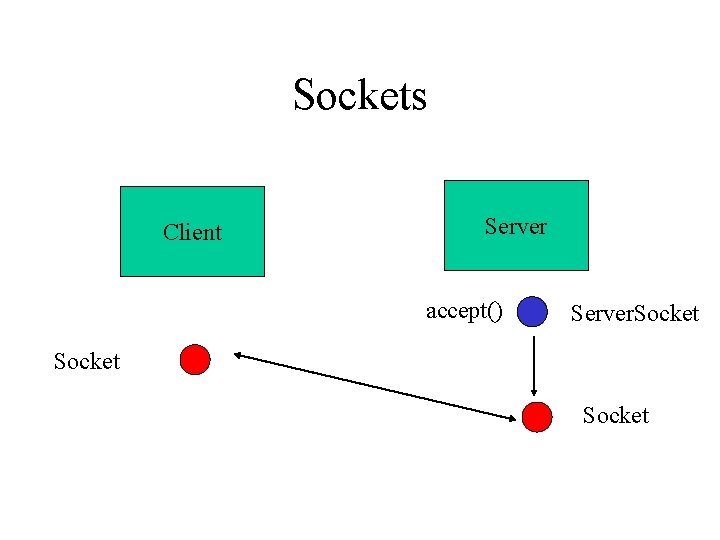
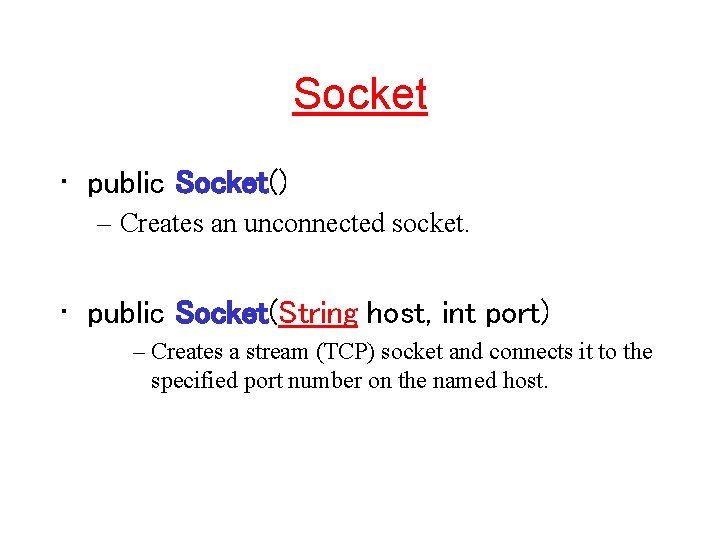
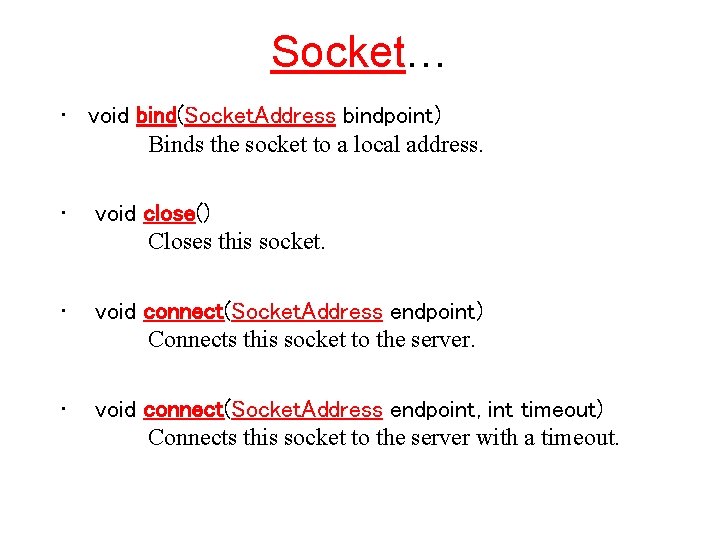
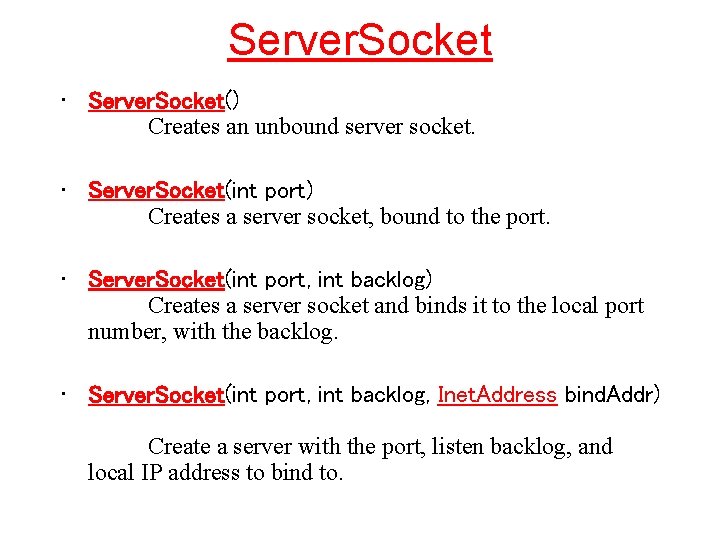
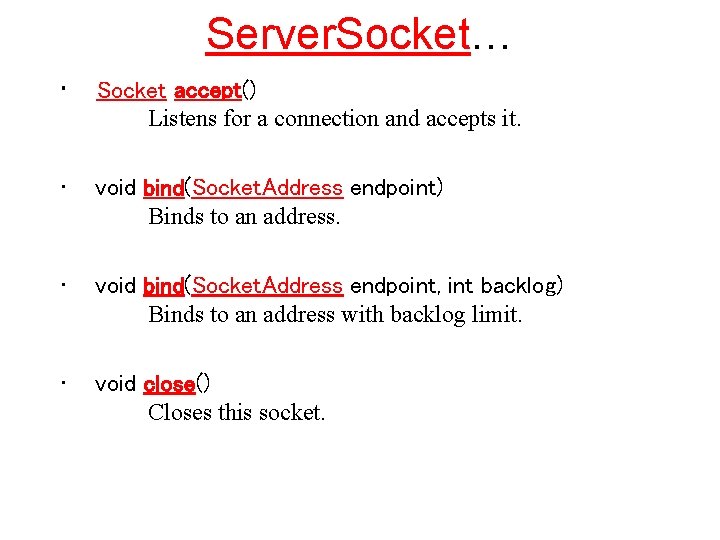
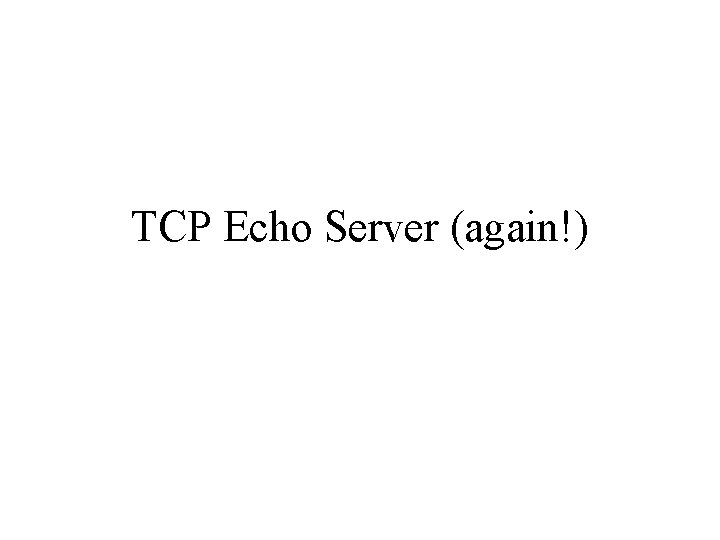
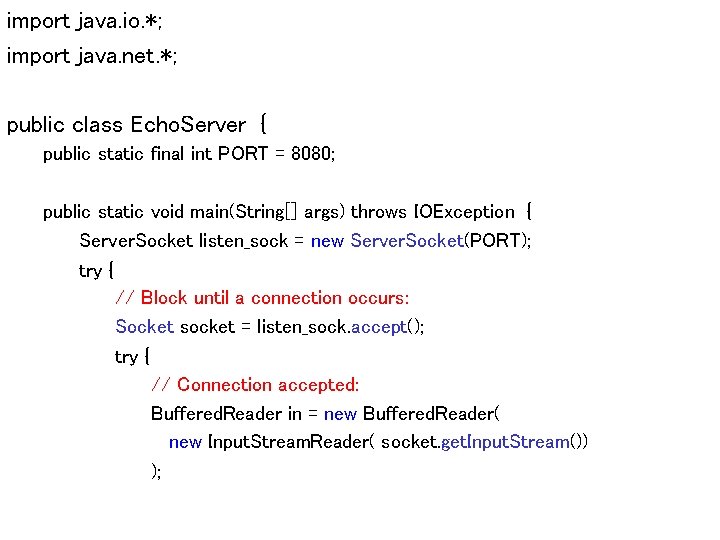
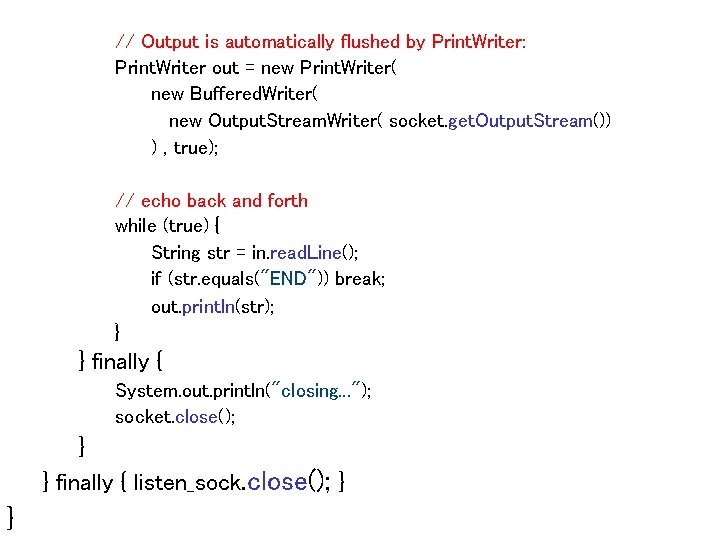
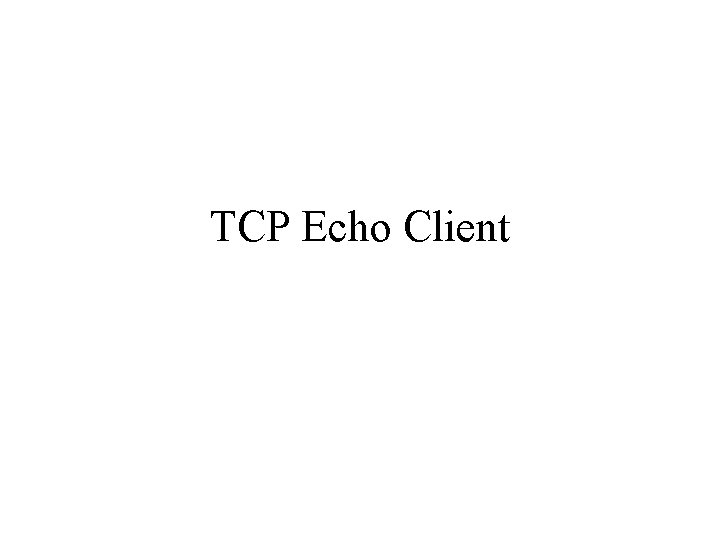
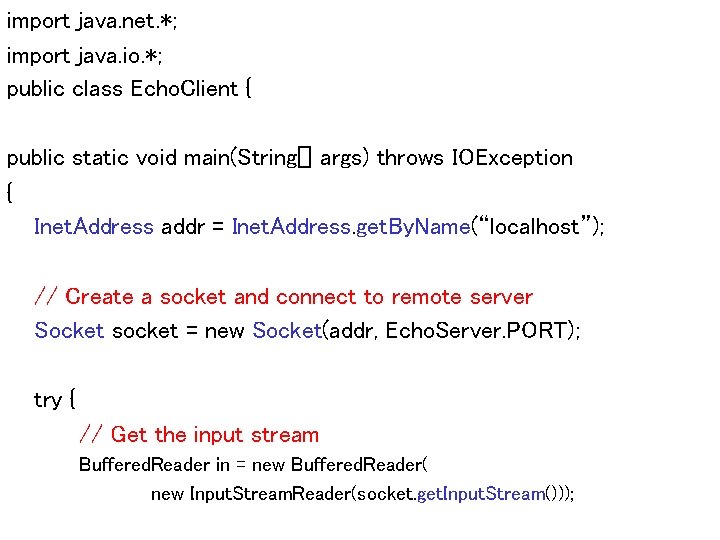
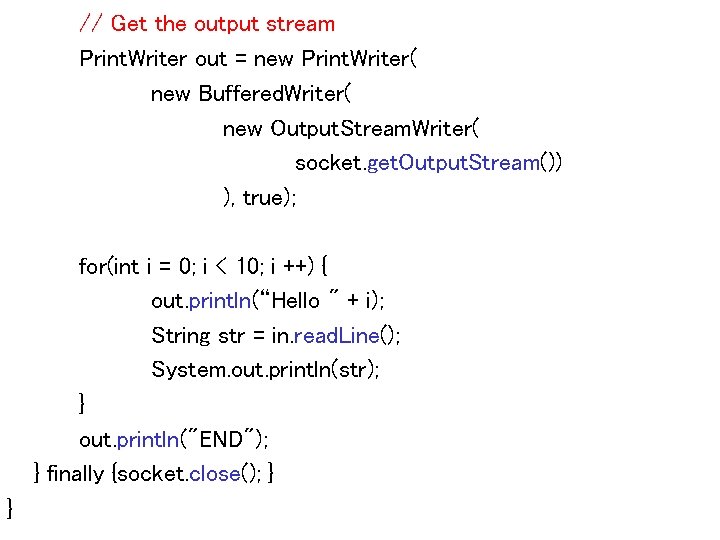
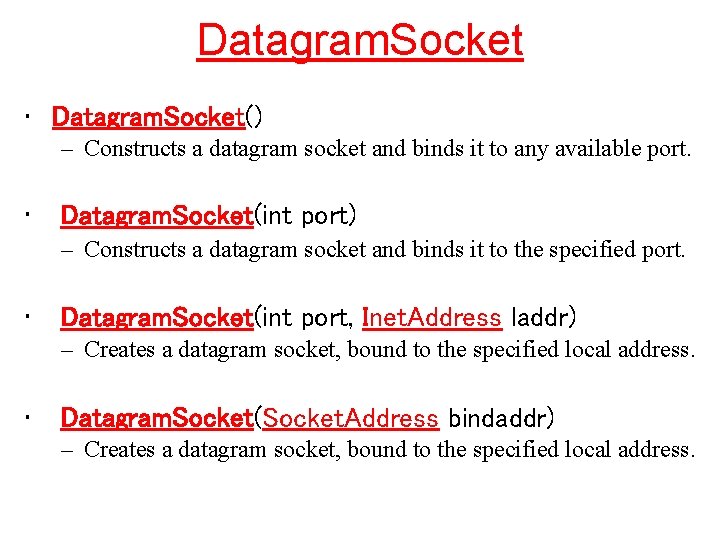
![Datagram. Packet • Datagram. Packet(byte[] buf, int length) – Constructs a Datagram. Packet for Datagram. Packet • Datagram. Packet(byte[] buf, int length) – Constructs a Datagram. Packet for](https://slidetodoc.com/presentation_image_h2/968462f65629d24fa69e3656366a6e8f/image-19.jpg)
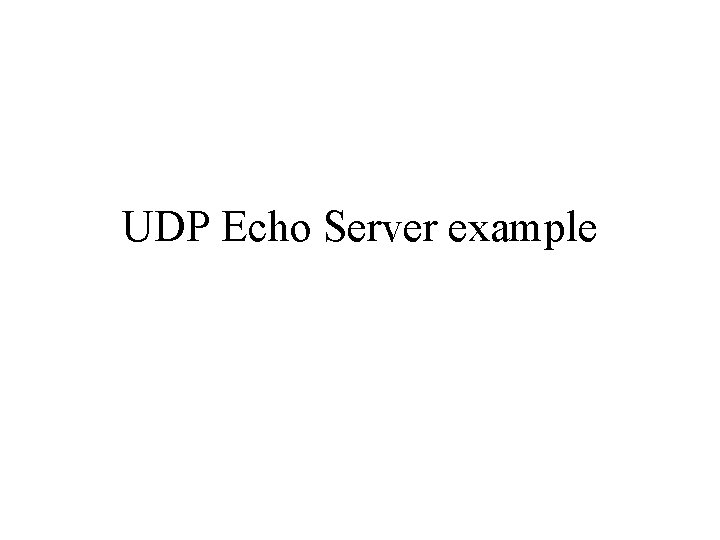
![// buffer to receive data byte[] buf = new byte[1000]; // buffer // create // buffer to receive data byte[] buf = new byte[1000]; // buffer // create](https://slidetodoc.com/presentation_image_h2/968462f65629d24fa69e3656366a6e8f/image-21.jpg)
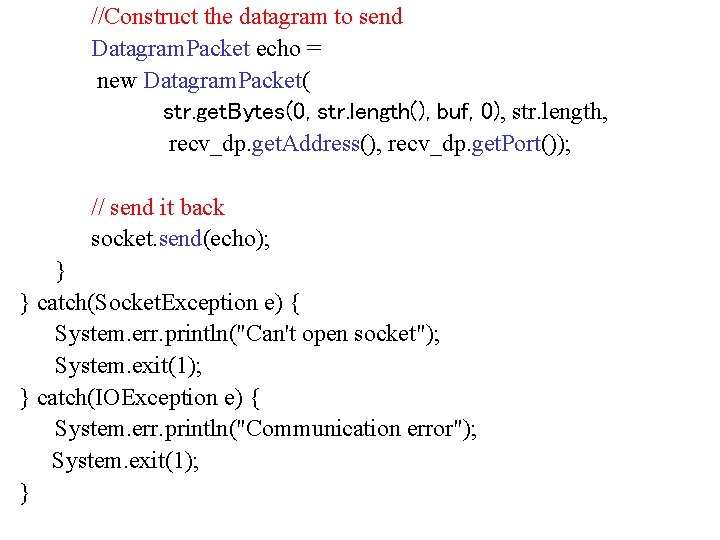
- Slides: 22
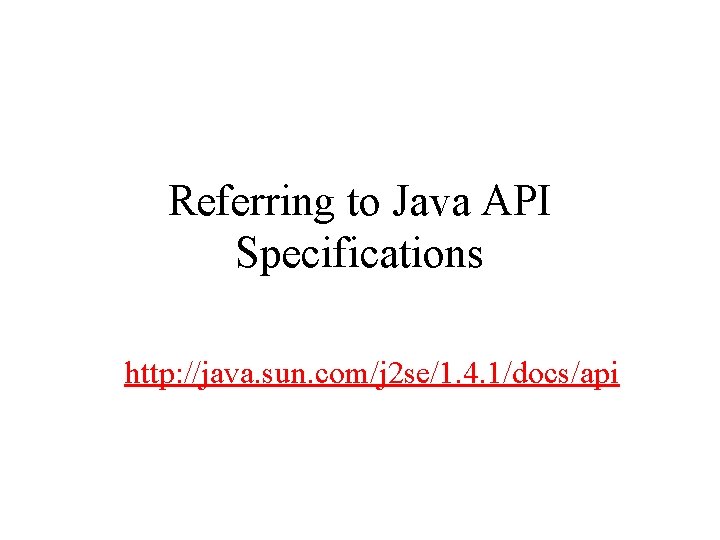
Referring to Java API Specifications http: //java. sun. com/j 2 se/1. 4. 1/docs/api
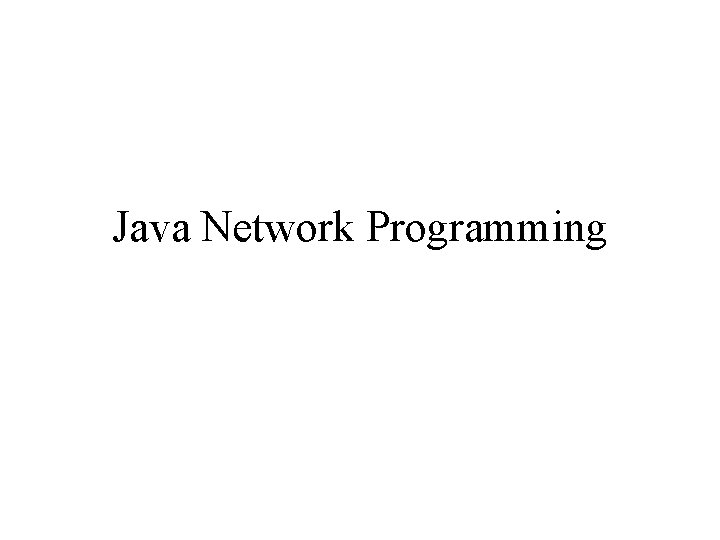
Java Network Programming
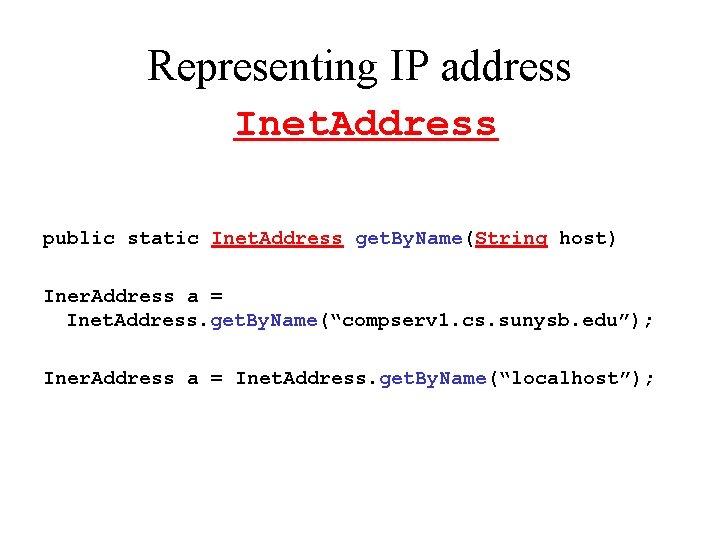
Representing IP address Inet. Address public static Inet. Address get. By. Name(String host) Iner. Address a = Inet. Address. get. By. Name(“compserv 1. cs. sunysb. edu”); Iner. Address a = Inet. Address. get. By. Name(“localhost”);
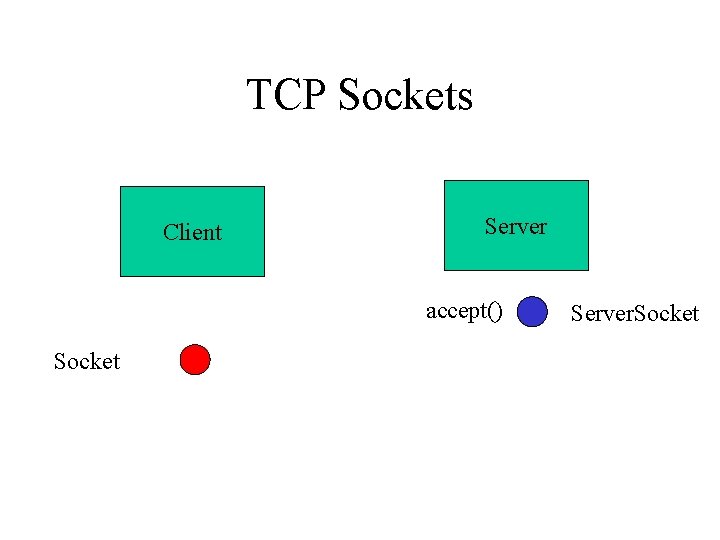
TCP Sockets Client Server accept() Socket Server. Socket
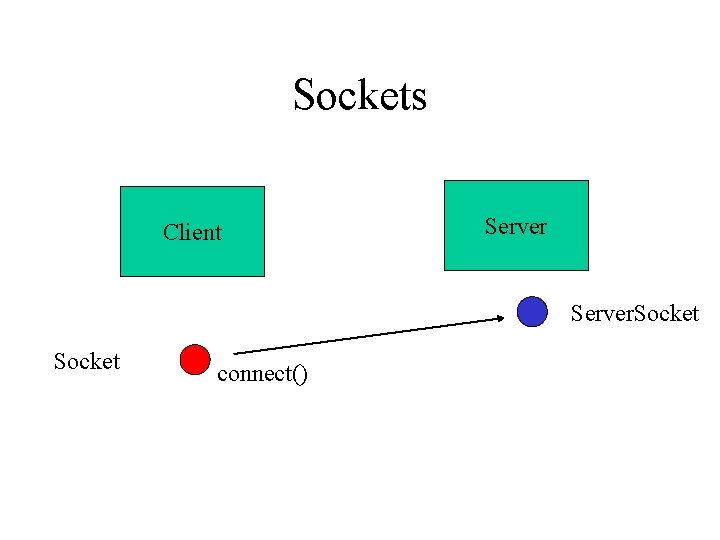
Sockets Client Server. Socket connect()
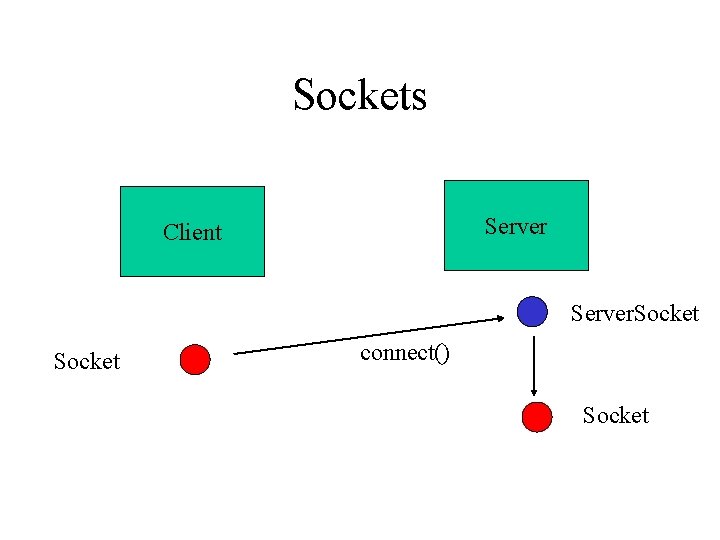
Sockets Server Client Server. Socket connect() Socket
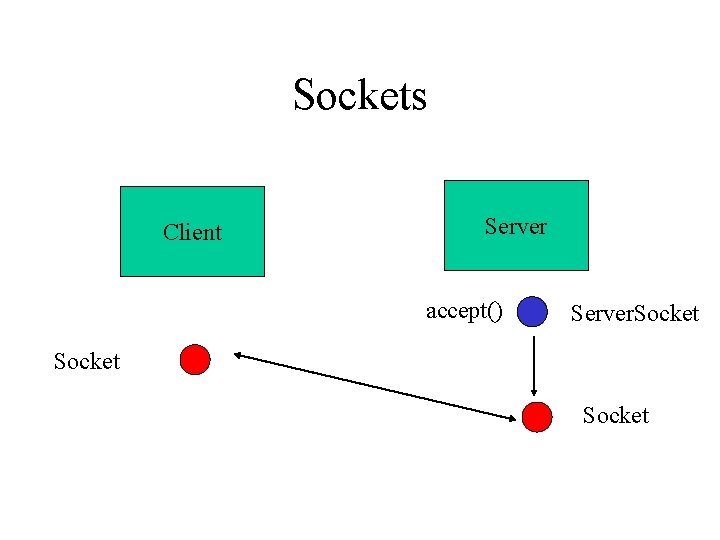
Sockets Client Server accept() Server. Socket
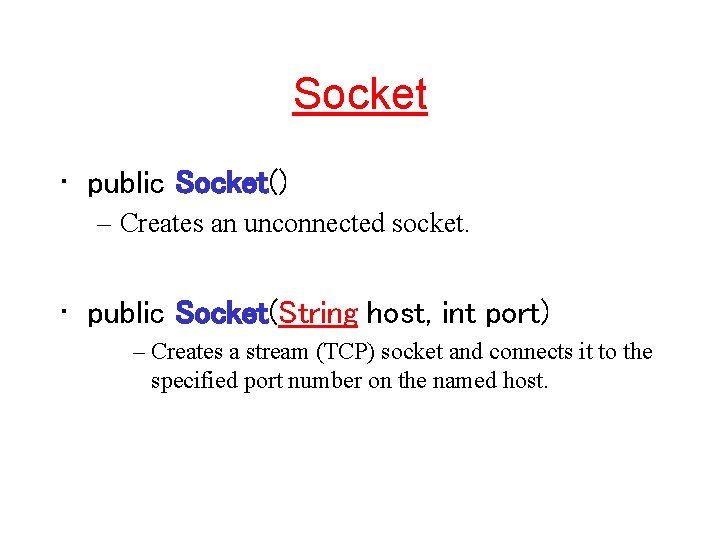
Socket • public Socket() – Creates an unconnected socket. • public Socket(String host, int port) – Creates a stream (TCP) socket and connects it to the specified port number on the named host.
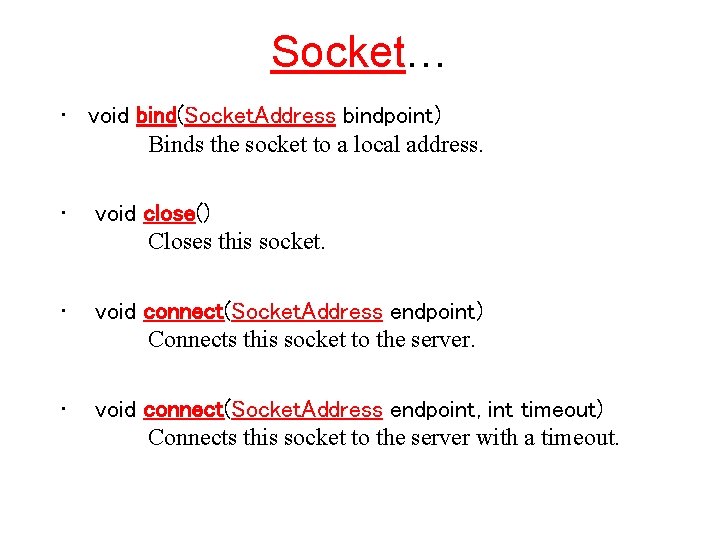
Socket… • void bind(Socket. Address bindpoint) Binds the socket to a local address. • void close() Closes this socket. • void connect(Socket. Address endpoint) Connects this socket to the server. • void connect(Socket. Address endpoint, int timeout) Connects this socket to the server with a timeout.
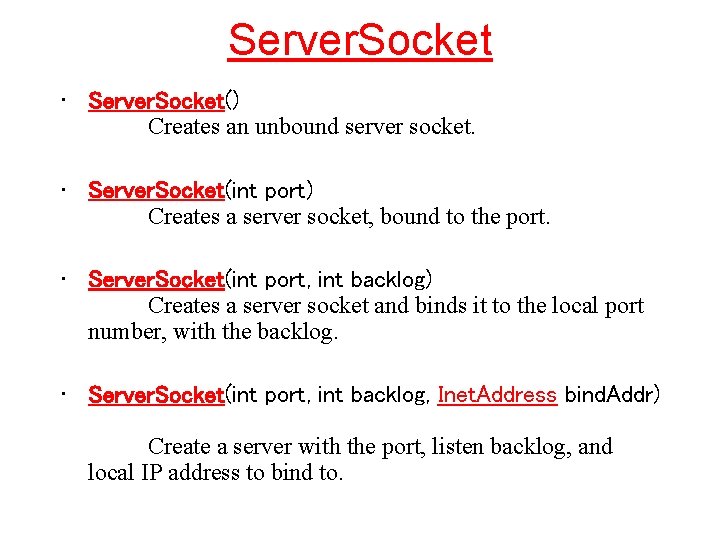
Server. Socket • Server. Socket() Creates an unbound server socket. • Server. Socket(int port) Creates a server socket, bound to the port. • Server. Socket(int port, int backlog) Creates a server socket and binds it to the local port number, with the backlog. • Server. Socket(int port, int backlog, Inet. Address bind. Addr) Create a server with the port, listen backlog, and local IP address to bind to.
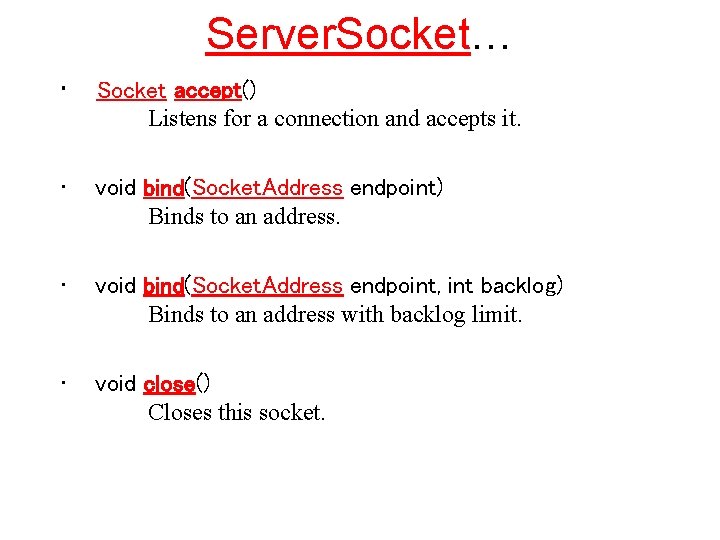
Server. Socket… • Socket accept() Listens for a connection and accepts it. • void bind(Socket. Address endpoint) Binds to an address. • void bind(Socket. Address endpoint, int backlog) Binds to an address with backlog limit. • void close() Closes this socket.
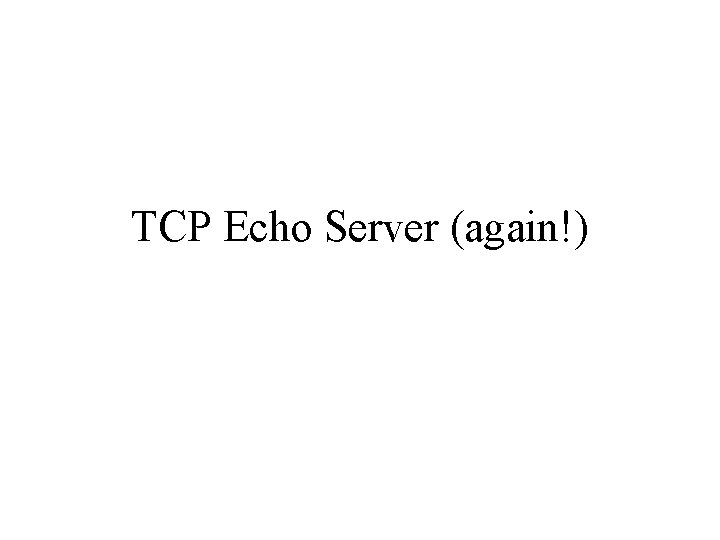
TCP Echo Server (again!)
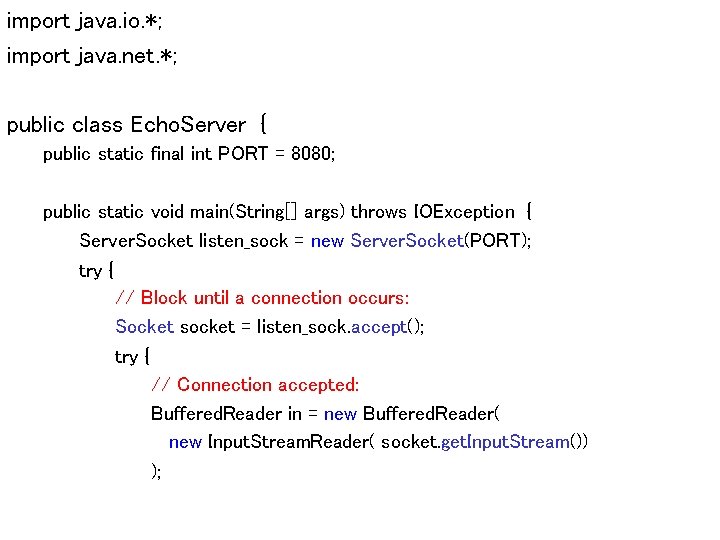
import java. io. *; import java. net. *; public class Echo. Server { public static final int PORT = 8080; public static void main(String[] args) throws IOException { Server. Socket listen_sock = new Server. Socket(PORT); try { // Block until a connection occurs: Socket socket = listen_sock. accept(); try { // Connection accepted: Buffered. Reader in = new Buffered. Reader( new Input. Stream. Reader( socket. get. Input. Stream()) );
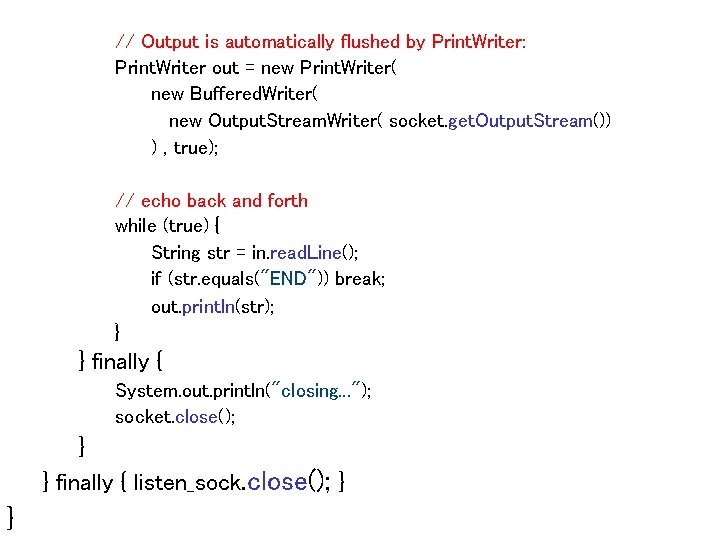
// Output is automatically flushed by Print. Writer: Print. Writer out = new Print. Writer( new Buffered. Writer( new Output. Stream. Writer( socket. get. Output. Stream()) ) , true); // echo back and forth while (true) { String str = in. read. Line(); if (str. equals("END")) break; out. println(str); } } finally { System. out. println("closing. . . "); socket. close(); } } finally { listen_sock. close(); } }
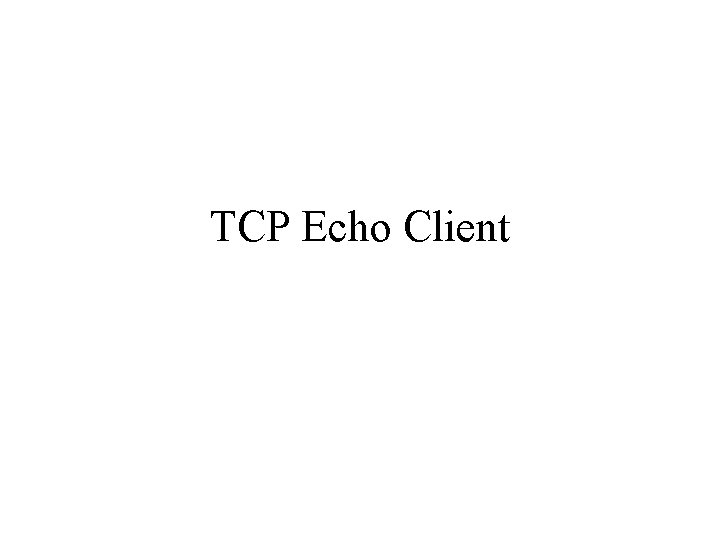
TCP Echo Client
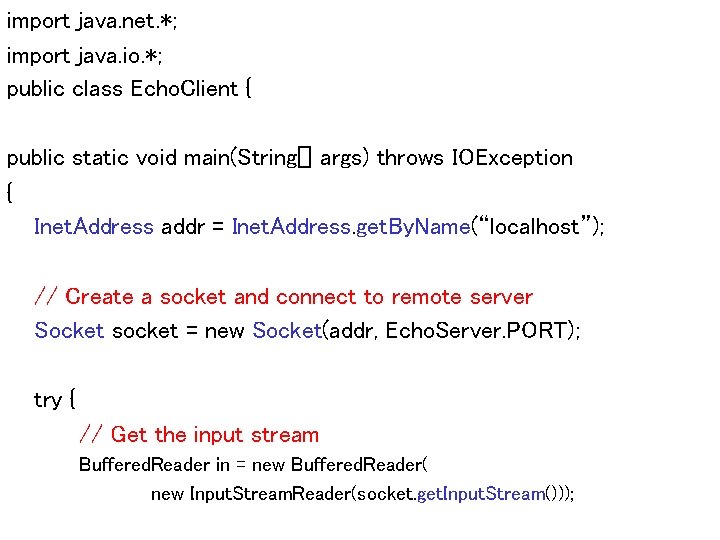
import java. net. *; import java. io. *; public class Echo. Client { public static void main(String[] args) throws IOException { Inet. Address addr = Inet. Address. get. By. Name(“localhost”); // Create a socket and connect to remote server Socket socket = new Socket(addr, Echo. Server. PORT); try { // Get the input stream Buffered. Reader in = new Buffered. Reader( new Input. Stream. Reader(socket. get. Input. Stream()));
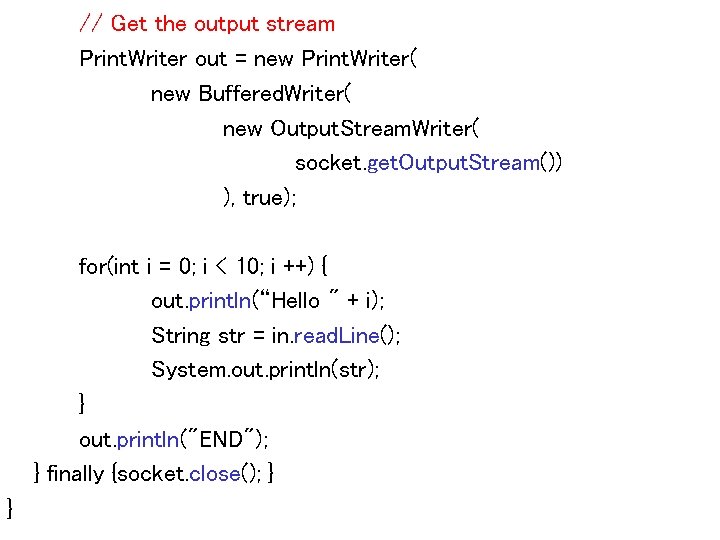
// Get the output stream Print. Writer out = new Print. Writer( new Buffered. Writer( new Output. Stream. Writer( socket. get. Output. Stream()) ), true); for(int i = 0; i < 10; i ++) { out. println(“Hello " + i); String str = in. read. Line(); System. out. println(str); } out. println("END"); } finally {socket. close(); } }
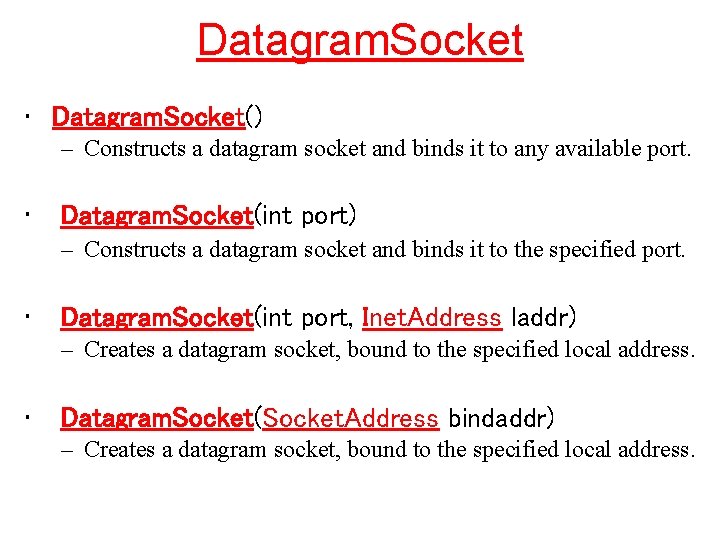
Datagram. Socket • Datagram. Socket() – Constructs a datagram socket and binds it to any available port. • Datagram. Socket(int port) – Constructs a datagram socket and binds it to the specified port. • Datagram. Socket(int port, Inet. Address laddr) – Creates a datagram socket, bound to the specified local address. • Datagram. Socket(Socket. Address bindaddr) – Creates a datagram socket, bound to the specified local address.
![Datagram Packet Datagram Packetbyte buf int length Constructs a Datagram Packet for Datagram. Packet • Datagram. Packet(byte[] buf, int length) – Constructs a Datagram. Packet for](https://slidetodoc.com/presentation_image_h2/968462f65629d24fa69e3656366a6e8f/image-19.jpg)
Datagram. Packet • Datagram. Packet(byte[] buf, int length) – Constructs a Datagram. Packet for receiving packets. • Datagram. Packet(byte[] buf, int length, Inet. Address address, int port) – Constructs a datagram packet for sending packets to the specified address and port.
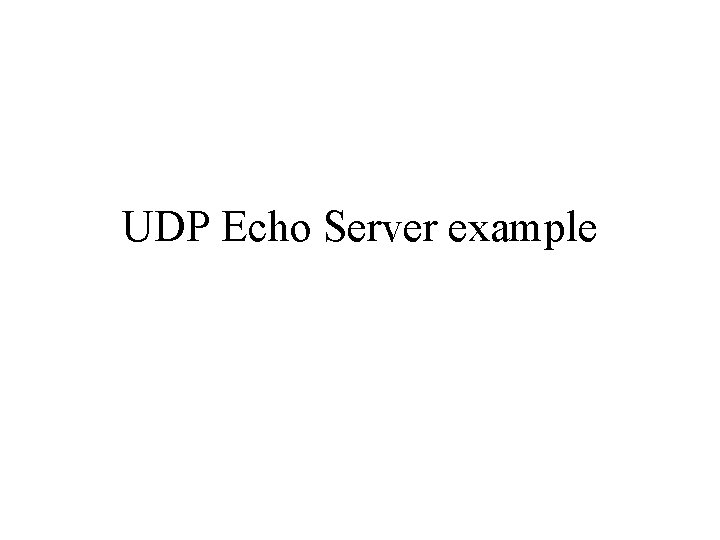
UDP Echo Server example
![buffer to receive data byte buf new byte1000 buffer create // buffer to receive data byte[] buf = new byte[1000]; // buffer // create](https://slidetodoc.com/presentation_image_h2/968462f65629d24fa69e3656366a6e8f/image-21.jpg)
// buffer to receive data byte[] buf = new byte[1000]; // buffer // create packet for receiving Datagram. Packet recv_dp = new Datagram. Packet(buf, buf. length); // create socket for sending Datagram. Socket socket; try { socket = new Datagram. Socket(INPORT); while(true) { // Block until a datagram appears: socket. receive(recv_dp); // Extract the string received String str = new String(recv_dp. get. Data(), 0, recv_dp. get. Length());
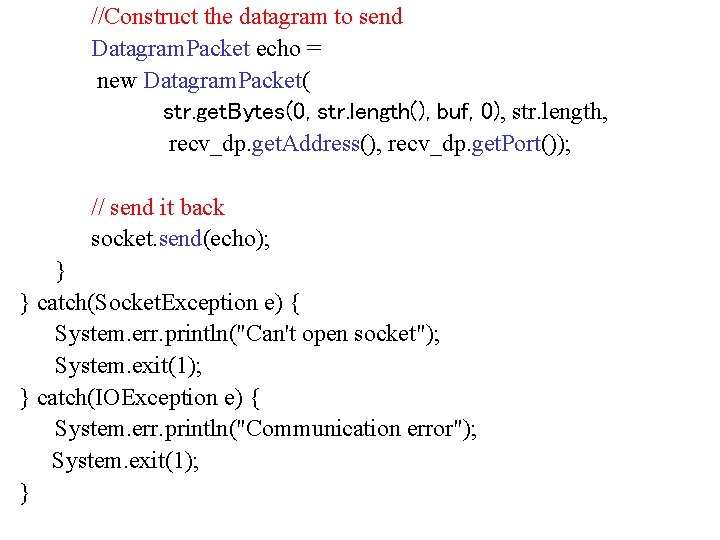
//Construct the datagram to send Datagram. Packet echo = new Datagram. Packet( str. get. Bytes(0, str. length(), buf, 0), str. length, recv_dp. get. Address(), recv_dp. get. Port()); // send it back socket. send(echo); } } catch(Socket. Exception e) { System. err. println("Can't open socket"); System. exit(1); } catch(IOException e) { System. err. println("Communication error"); System. exit(1); }
Java sun api
Http://java.sun.com/
Referring expression
Referring expressions examples
On referring strawson
Reference words
Referring expression
Maximuim
Referring others
Referring expression definition
We are usually referring to species diversity
14 lcp principles
تحليل قصيدة to daffodils
Proclaiming and referring tones
The second moroccan crisis of 1911 resulted in
Http //mbs.meb.gov.tr/ http //www.alantercihleri.com
Siat ung sistem informasi akademik
Java database connectivity api
Lotus notes java api
Java sound api
Java sound api
Java printer api
Graphics java api