Programing Paradigms Lecturer Hamza Azeem Lecture 2 C
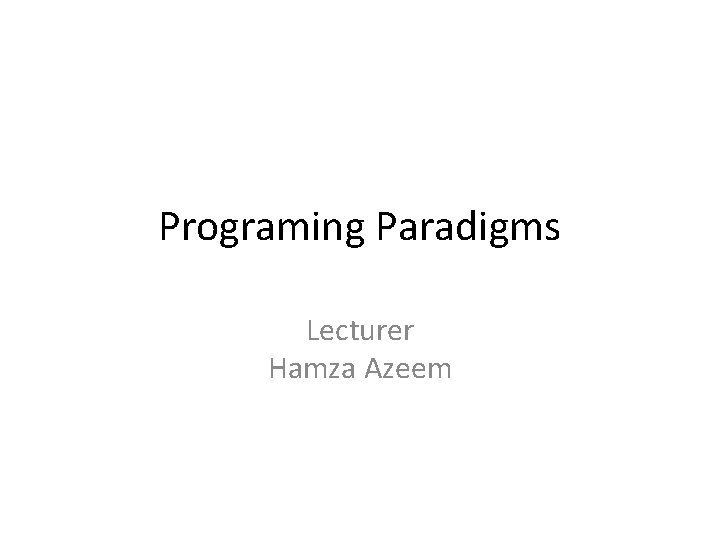
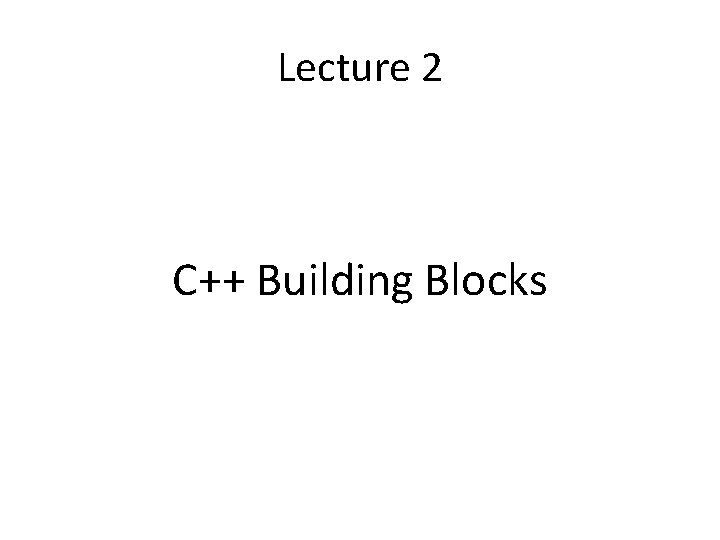
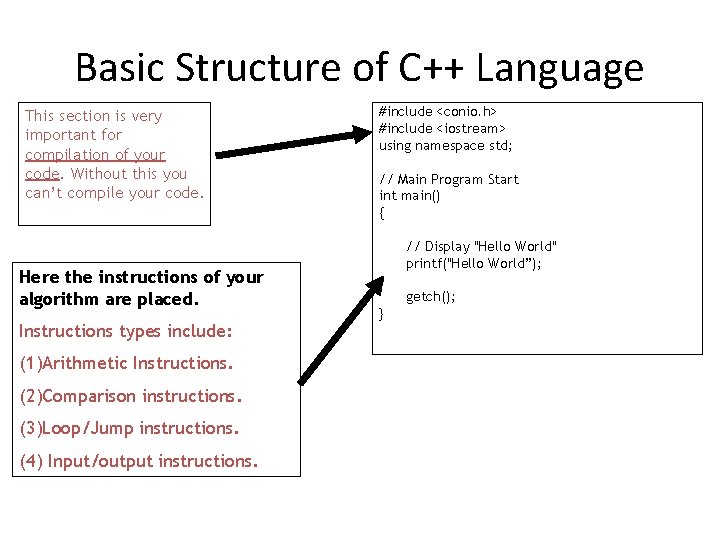
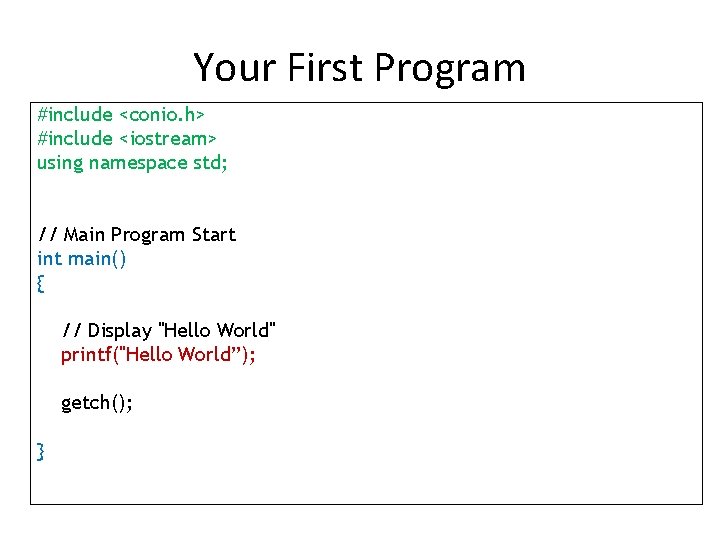
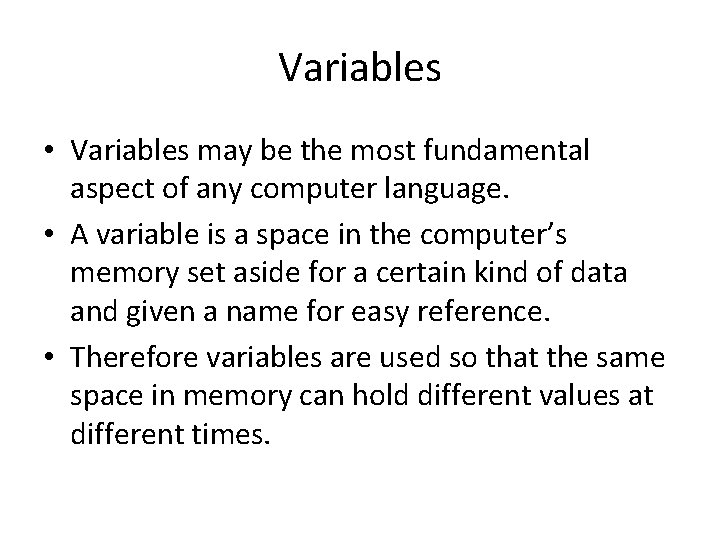
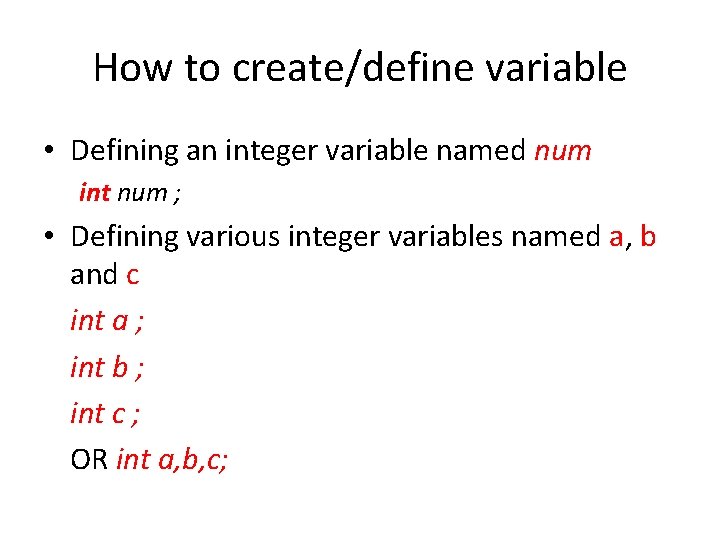
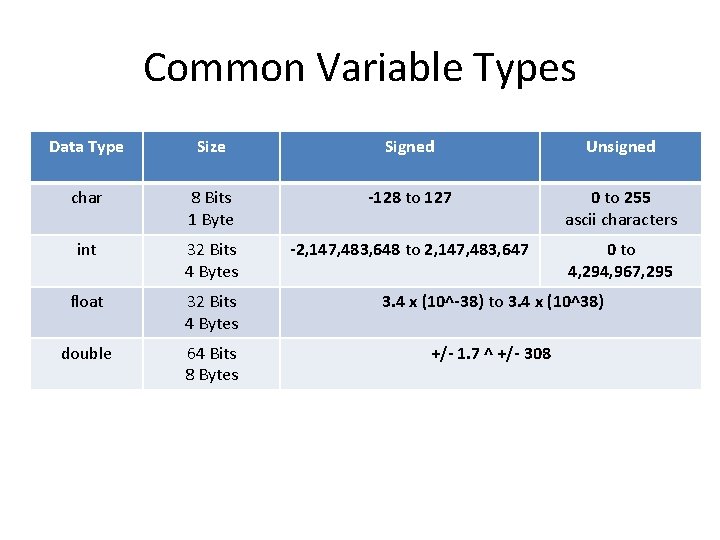
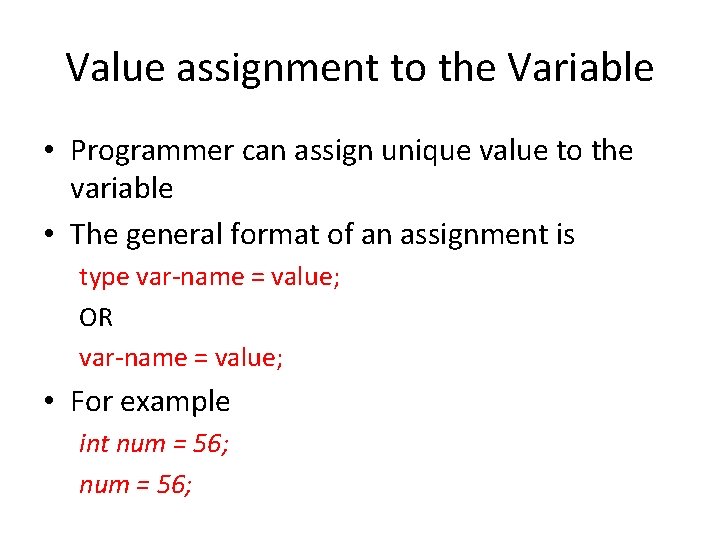
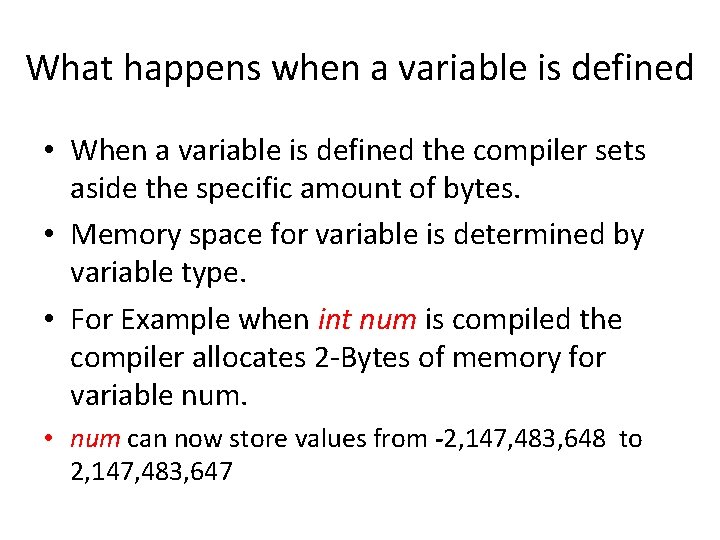
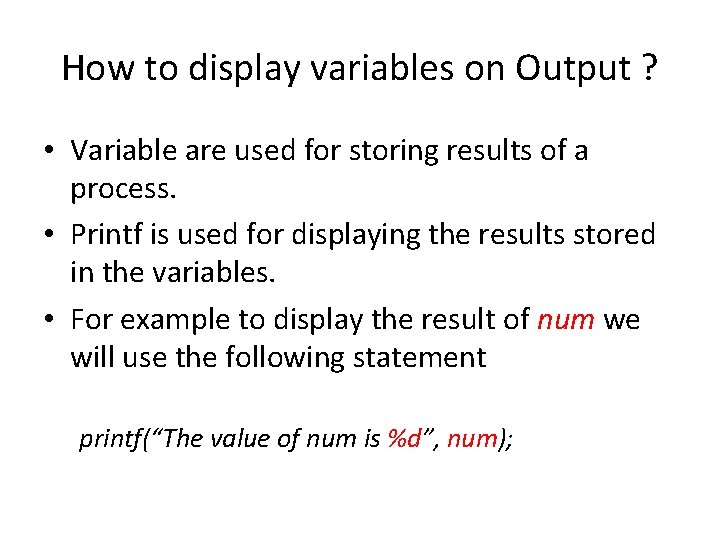
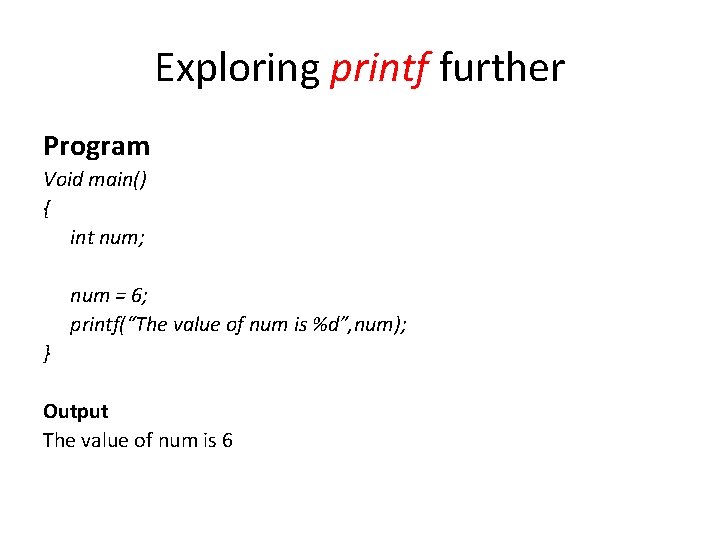
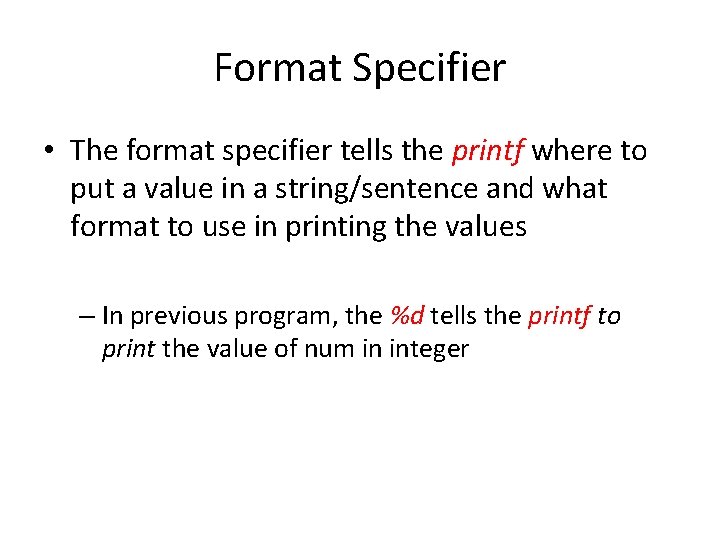
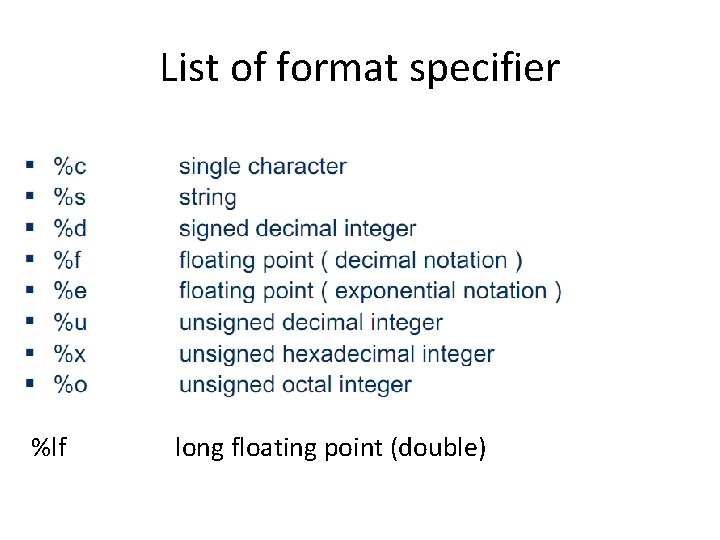
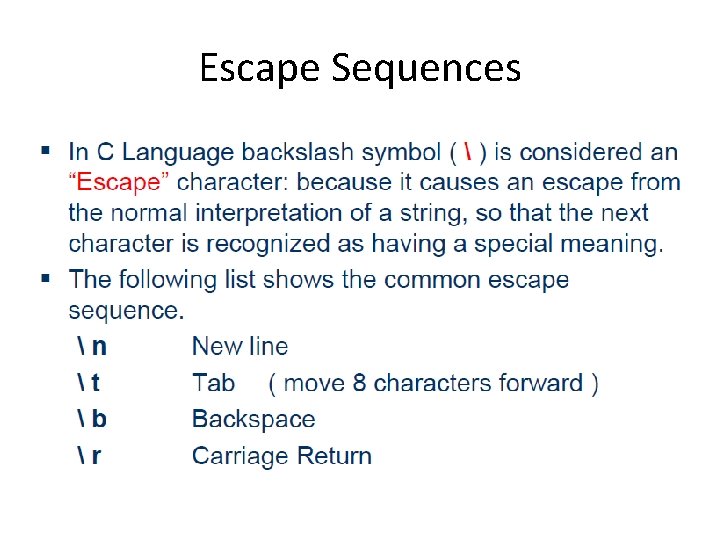
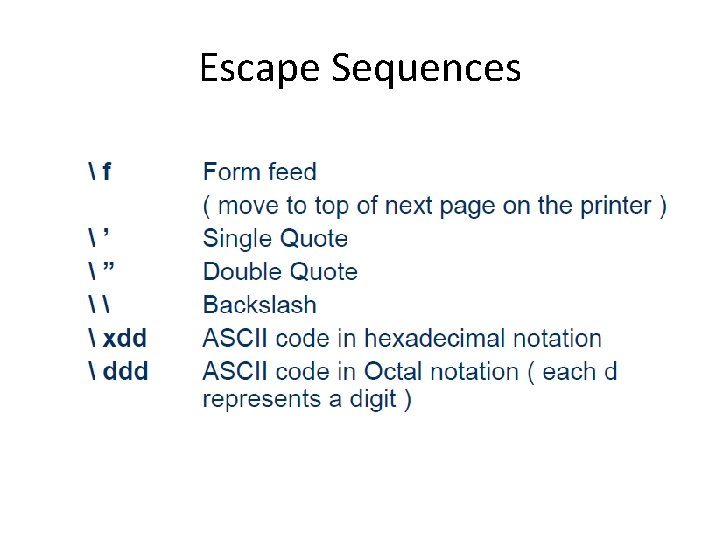
- Slides: 15
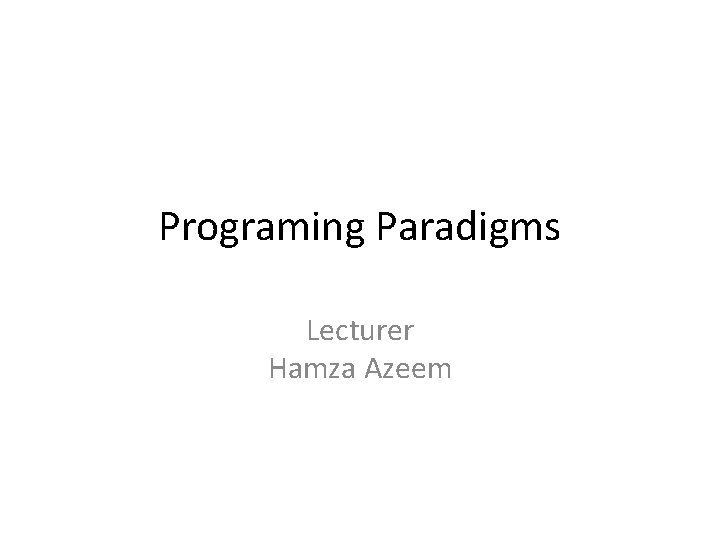
Programing Paradigms Lecturer Hamza Azeem
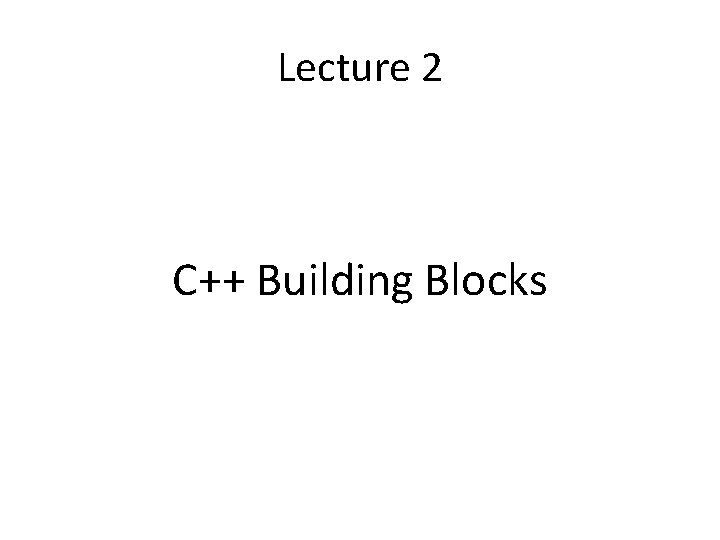
Lecture 2 C++ Building Blocks
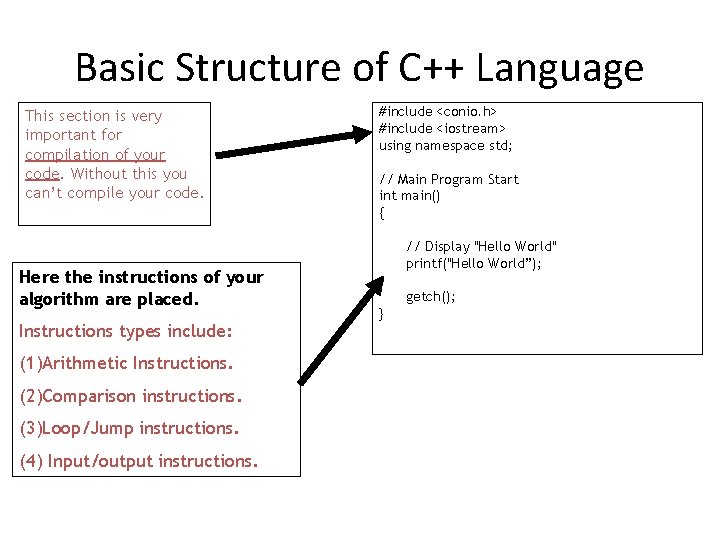
Basic Structure of C++ Language This section is very important for compilation of your code. Without this you can’t compile your code. Here the instructions of your algorithm are placed. Instructions types include: (1)Arithmetic Instructions. (2)Comparison instructions. (3)Loop/Jump instructions. (4) Input/output instructions. #include <conio. h> #include <iostream> using namespace std; // Main Program Start int main() { // Display "Hello World" printf("Hello World”); getch(); }
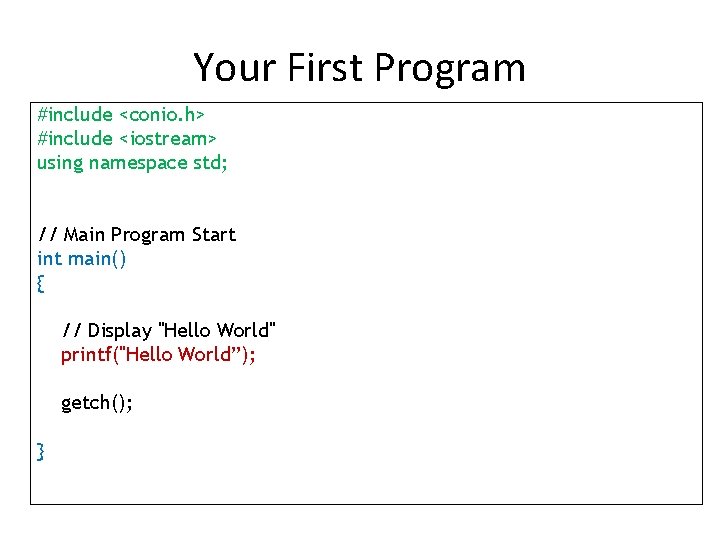
Your First Program #include <conio. h> #include <iostream> using namespace std; // Main Program Start int main() { // Display "Hello World" printf("Hello World”); getch(); }
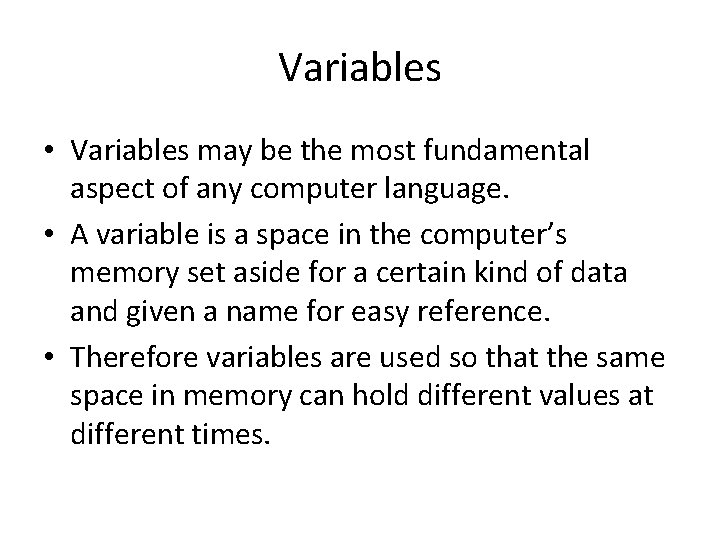
Variables • Variables may be the most fundamental aspect of any computer language. • A variable is a space in the computer’s memory set aside for a certain kind of data and given a name for easy reference. • Therefore variables are used so that the same space in memory can hold different values at different times.
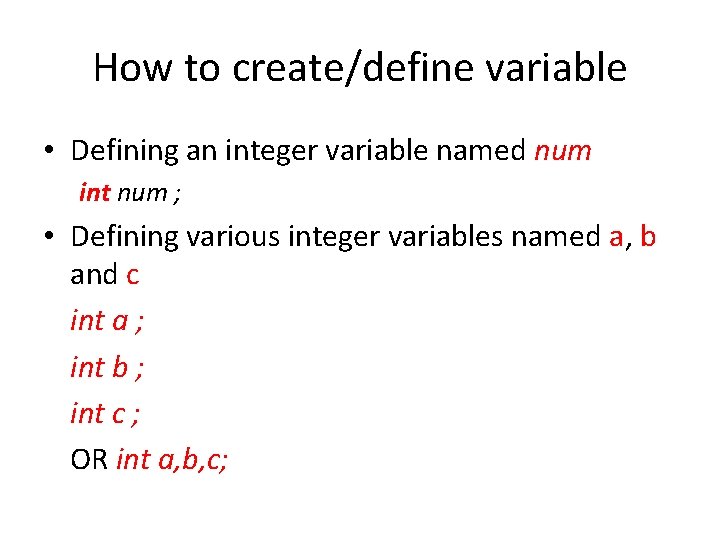
How to create/define variable • Defining an integer variable named num int num ; • Defining various integer variables named a, b and c int a ; int b ; int c ; OR int a, b, c;
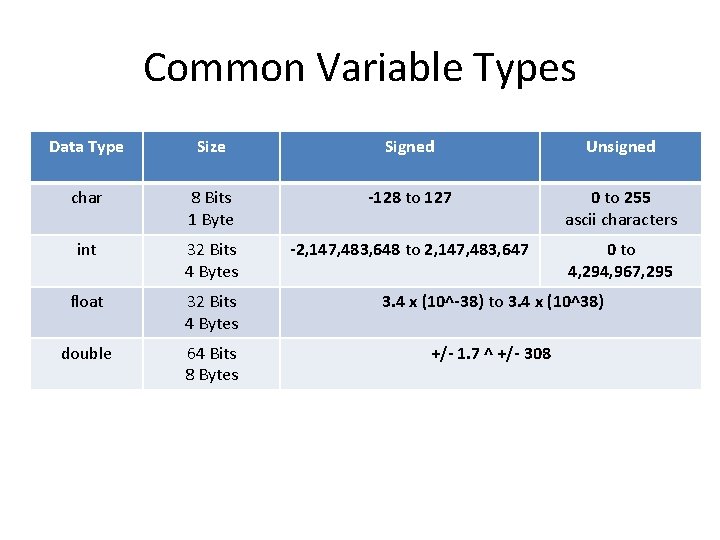
Common Variable Types Data Type Size Signed Unsigned char 8 Bits 1 Byte -128 to 127 0 to 255 ascii characters int 32 Bits 4 Bytes -2, 147, 483, 648 to 2, 147, 483, 647 0 to 4, 294, 967, 295 float 32 Bits 4 Bytes 3. 4 x (10^-38) to 3. 4 x (10^38) double 64 Bits 8 Bytes +/- 1. 7 ^ +/- 308
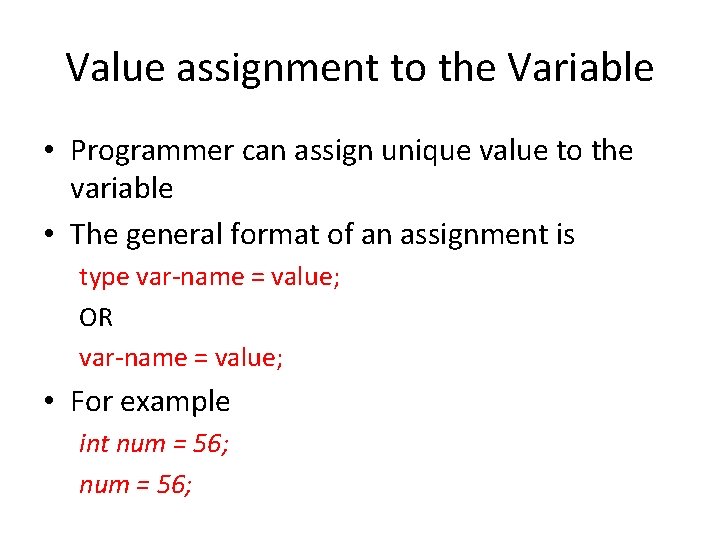
Value assignment to the Variable • Programmer can assign unique value to the variable • The general format of an assignment is type var-name = value; OR var-name = value; • For example int num = 56;
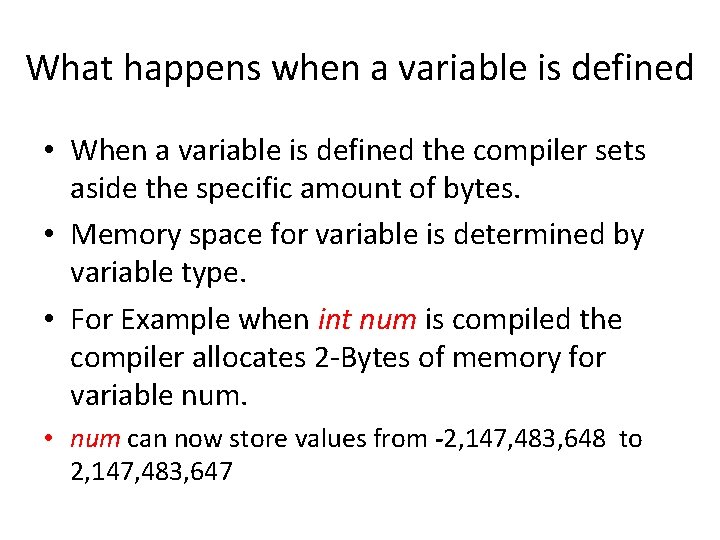
What happens when a variable is defined • When a variable is defined the compiler sets aside the specific amount of bytes. • Memory space for variable is determined by variable type. • For Example when int num is compiled the compiler allocates 2 -Bytes of memory for variable num. • num can now store values from -2, 147, 483, 648 to 2, 147, 483, 647
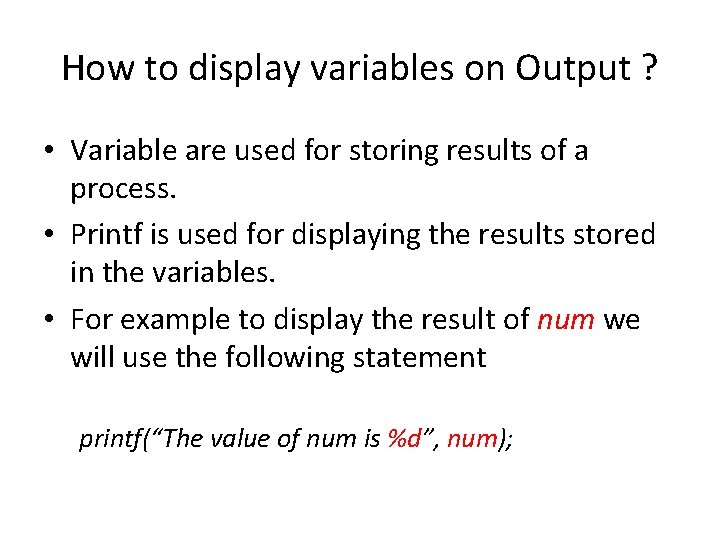
How to display variables on Output ? • Variable are used for storing results of a process. • Printf is used for displaying the results stored in the variables. • For example to display the result of num we will use the following statement printf(“The value of num is %d”, num);
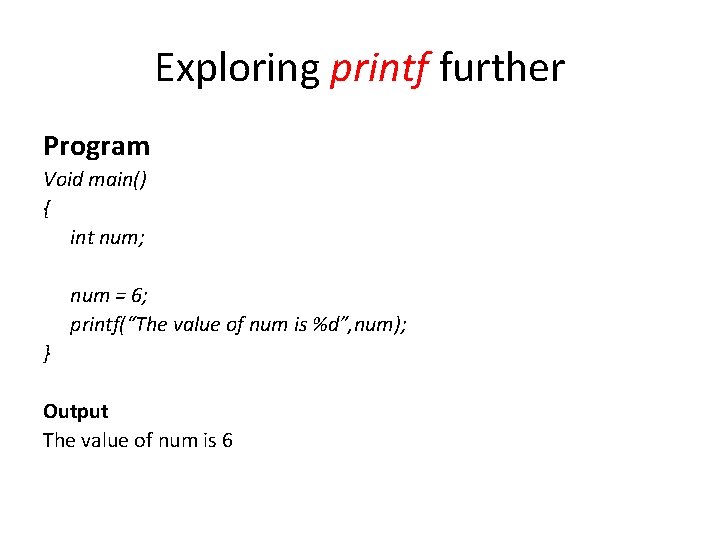
Exploring printf further Program Void main() { int num; num = 6; printf(“The value of num is %d”, num); } Output The value of num is 6
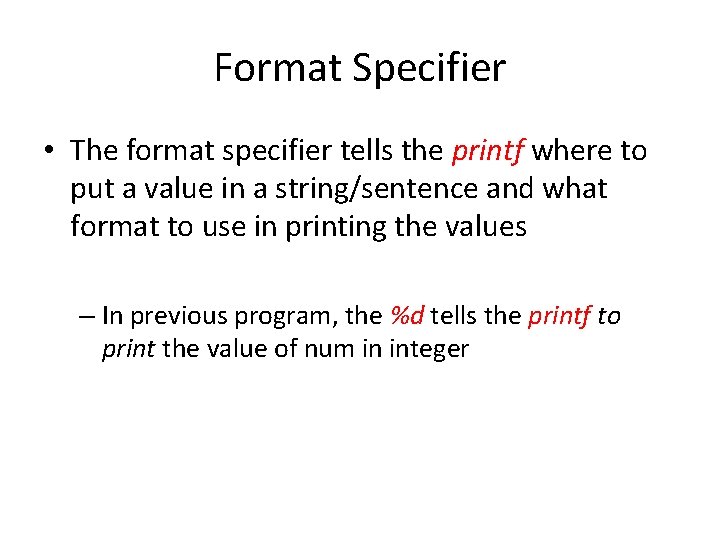
Format Specifier • The format specifier tells the printf where to put a value in a string/sentence and what format to use in printing the values – In previous program, the %d tells the printf to print the value of num in integer
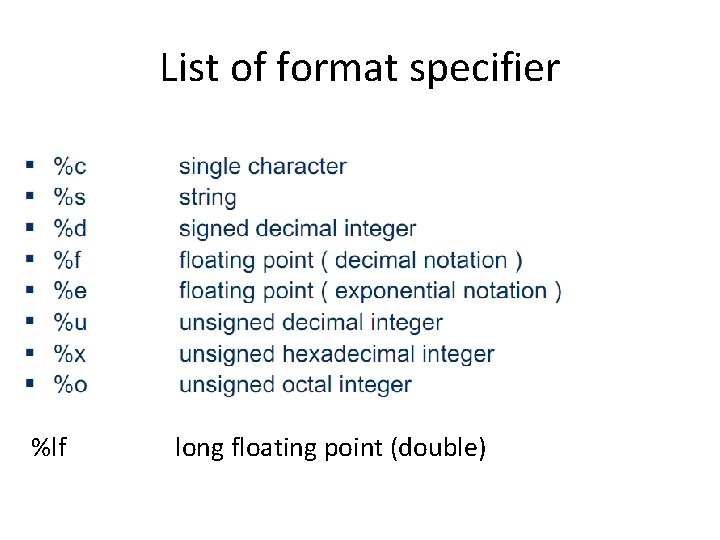
List of format specifier %lf long floating point (double)
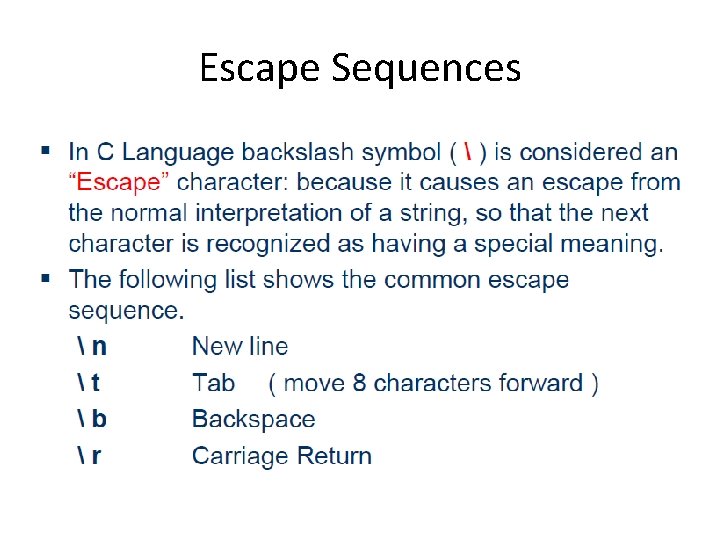
Escape Sequences
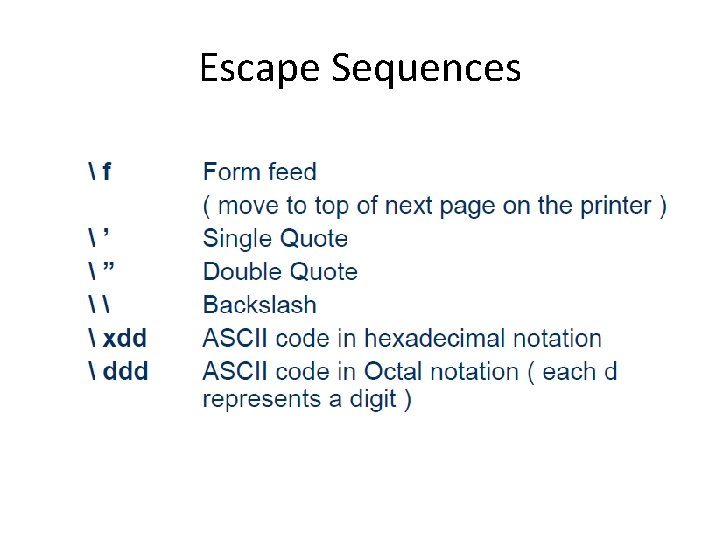
Escape Sequences