Outline n n n Processes Threads Interprocess communication
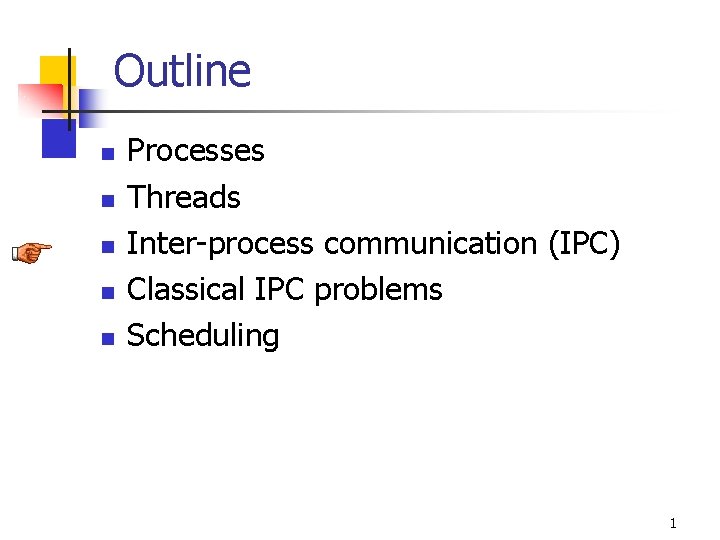
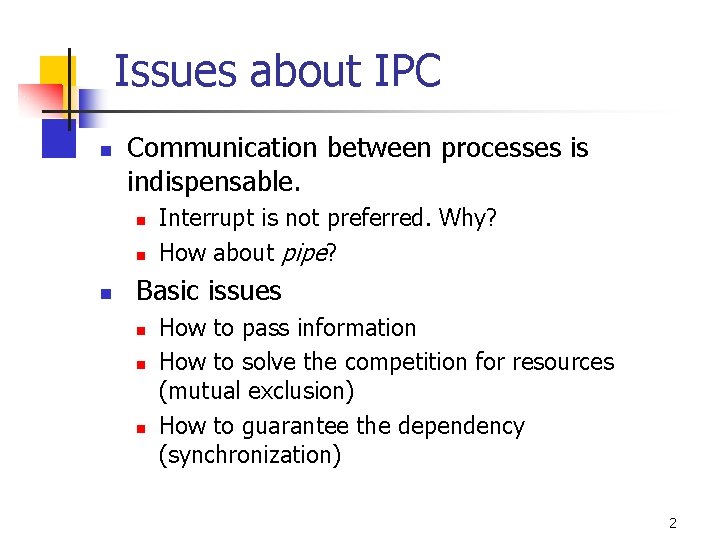
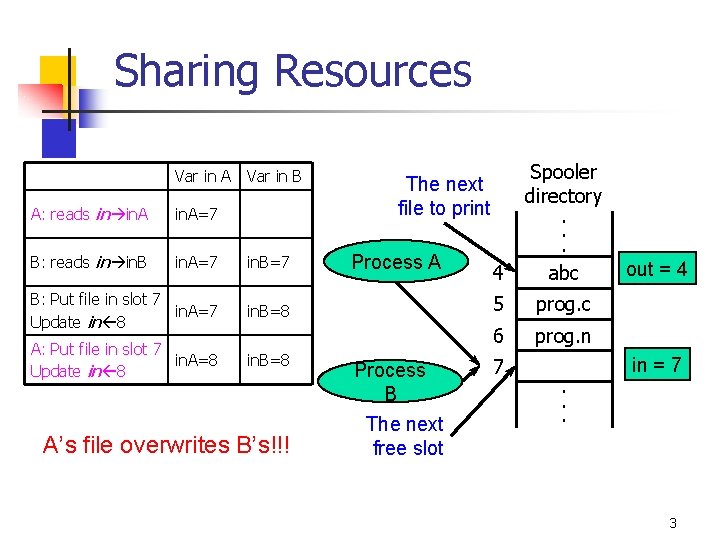
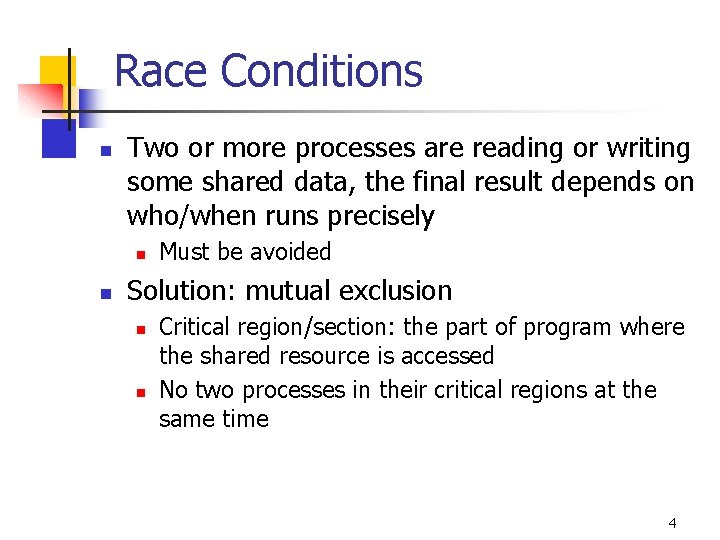
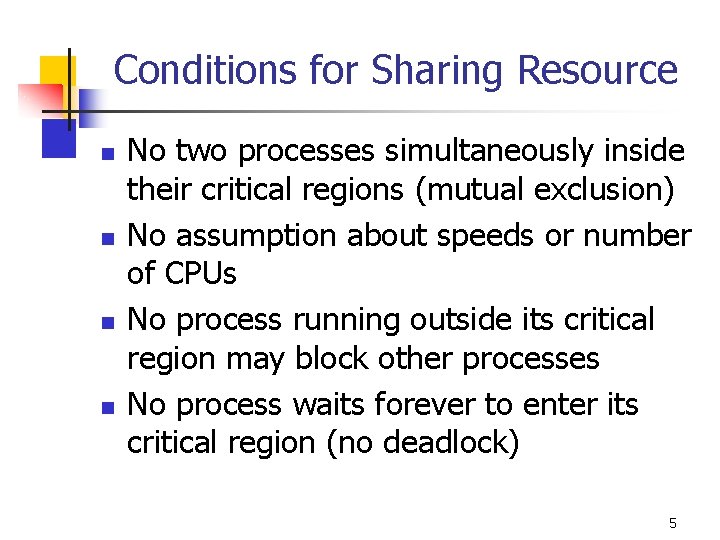
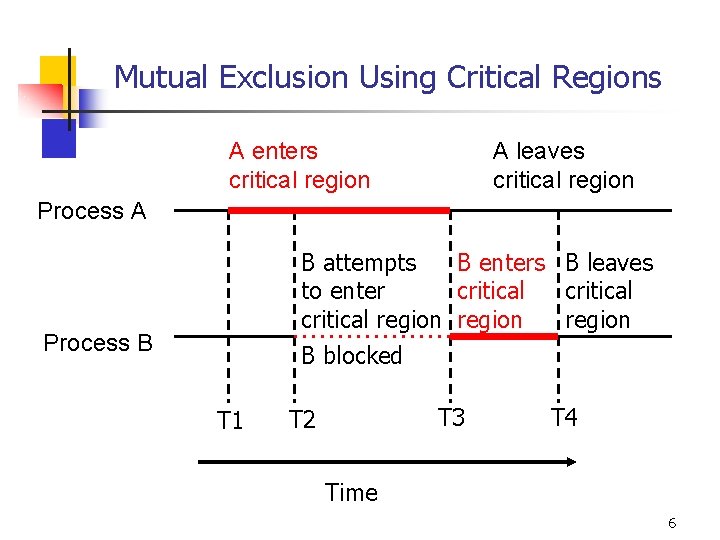
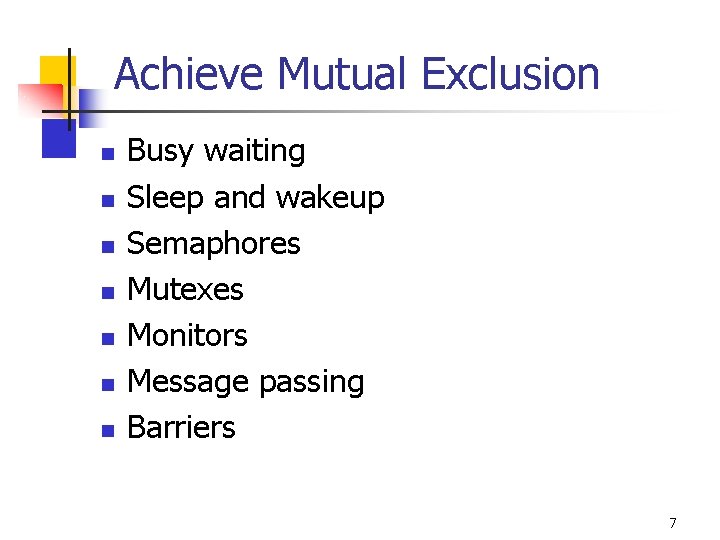
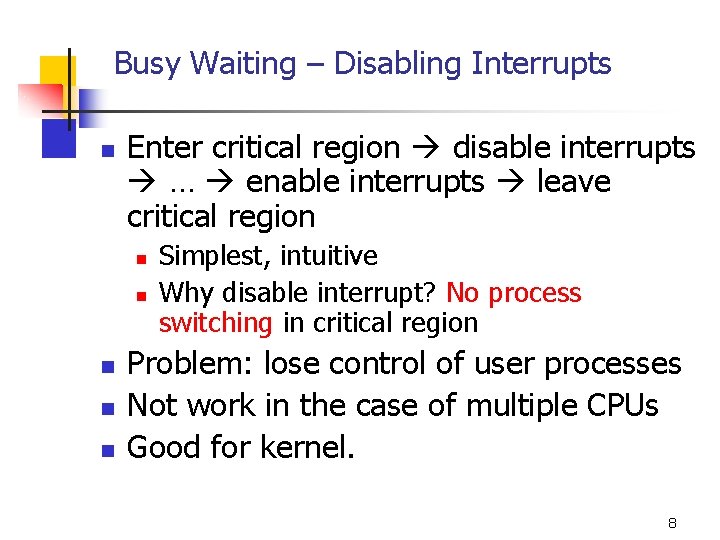
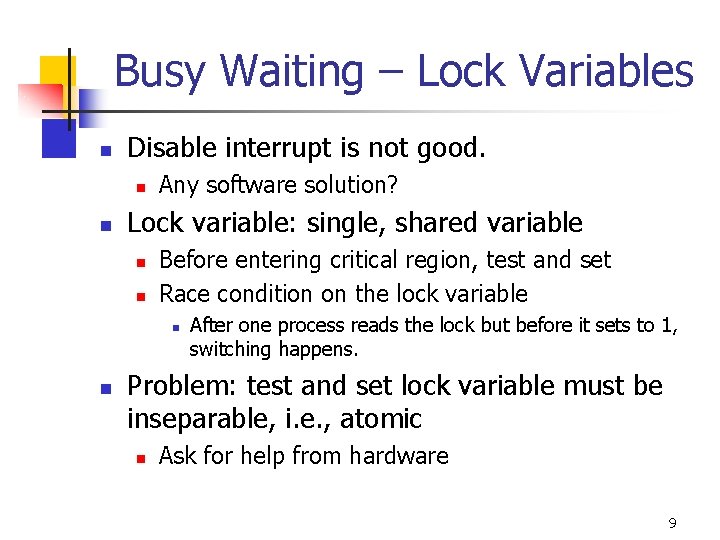
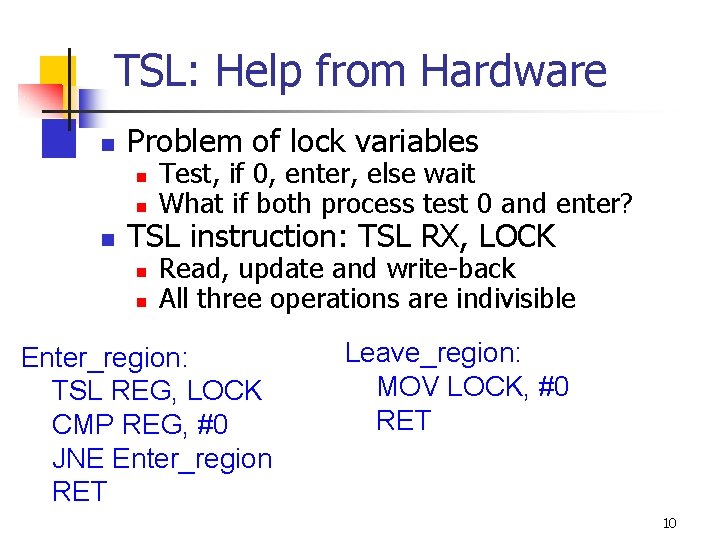
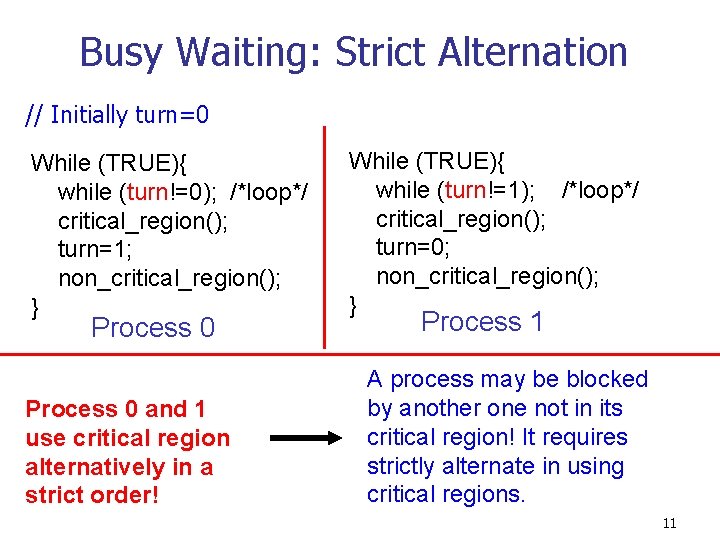
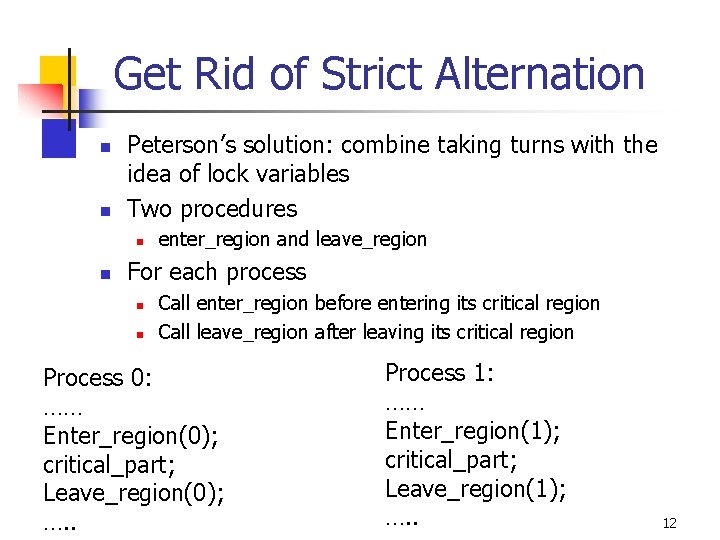
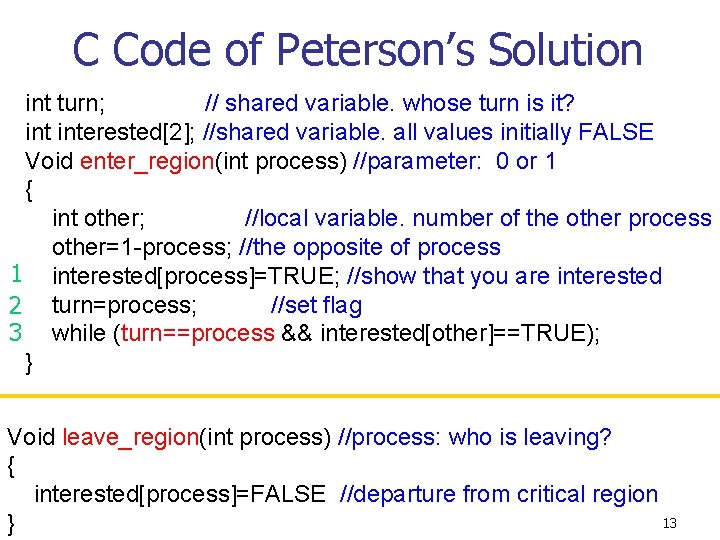
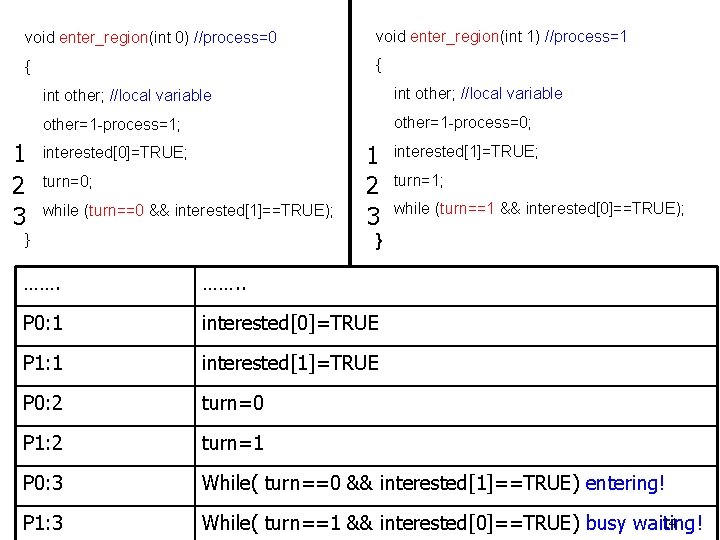
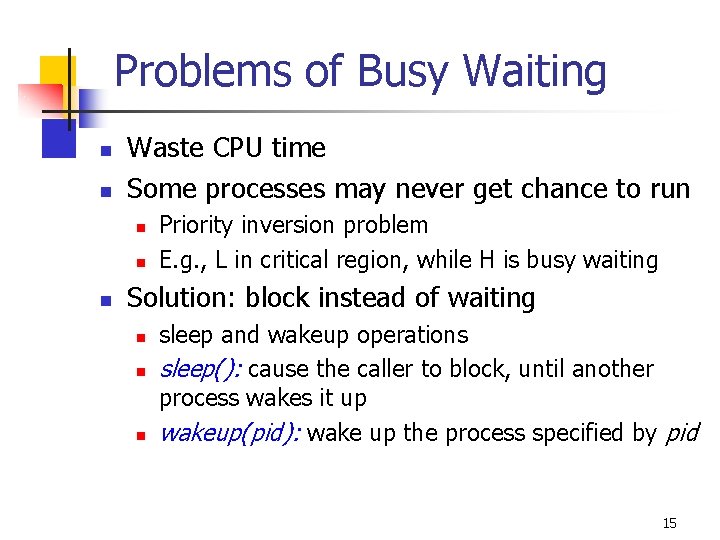
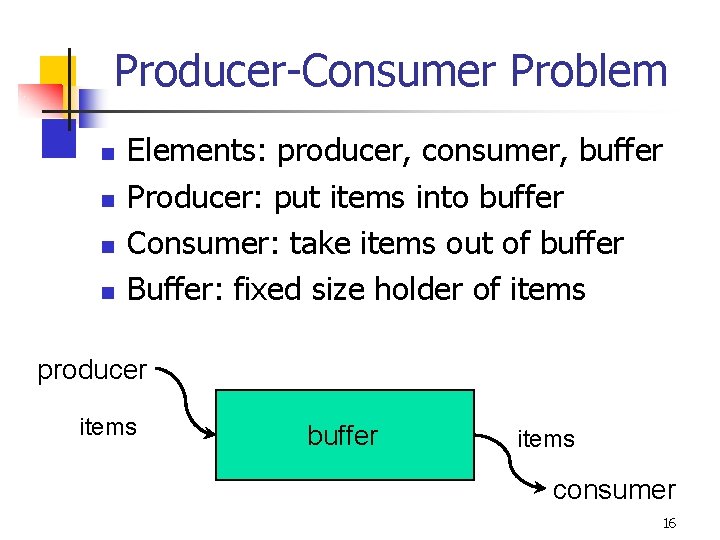
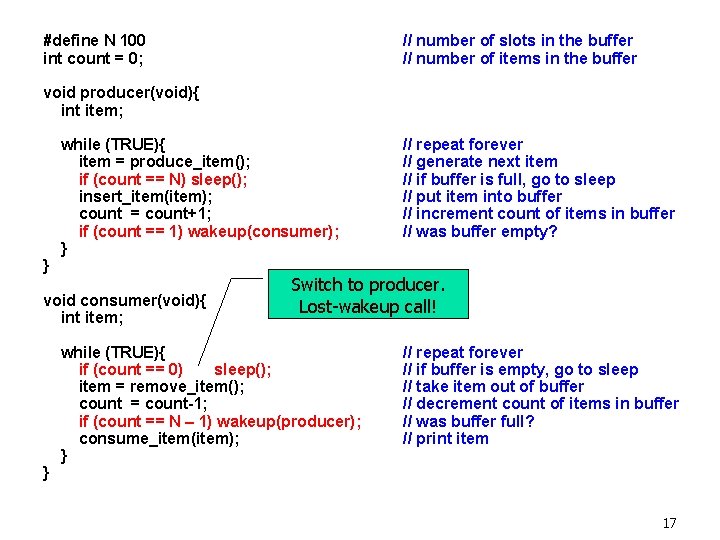
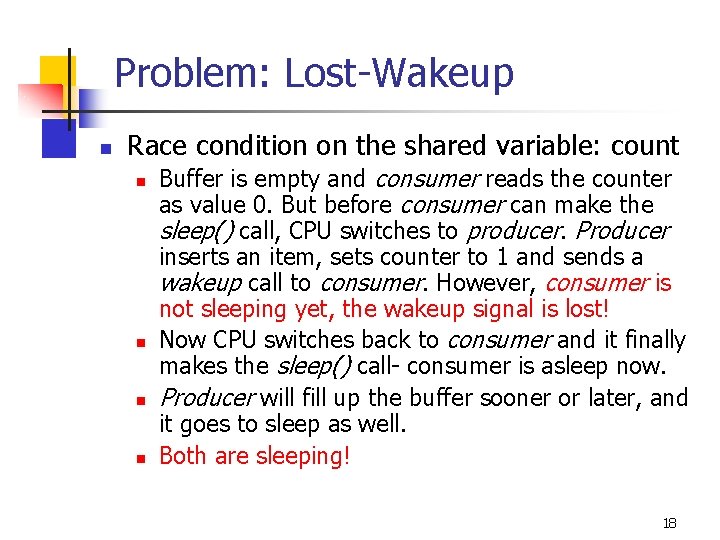
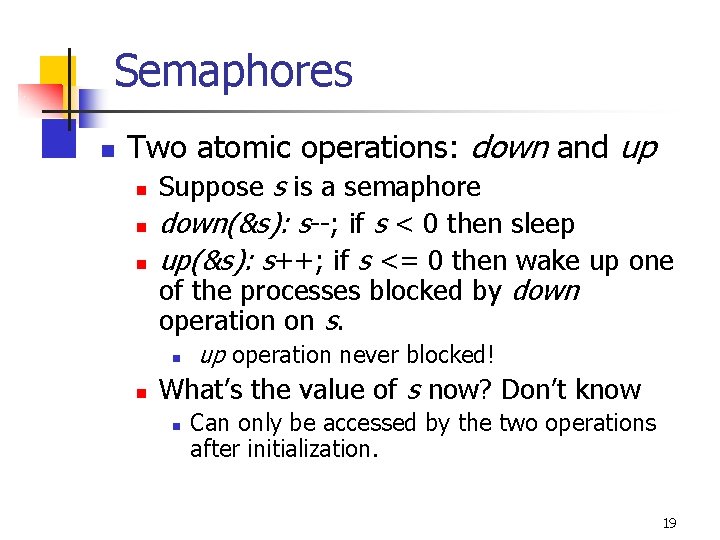
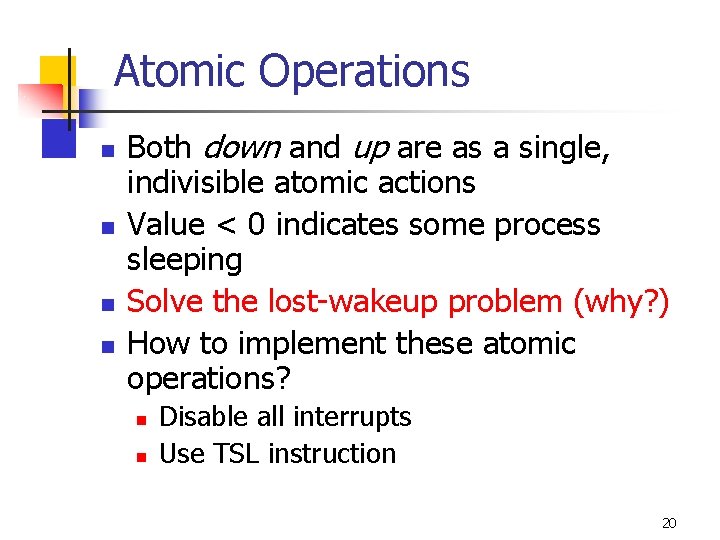
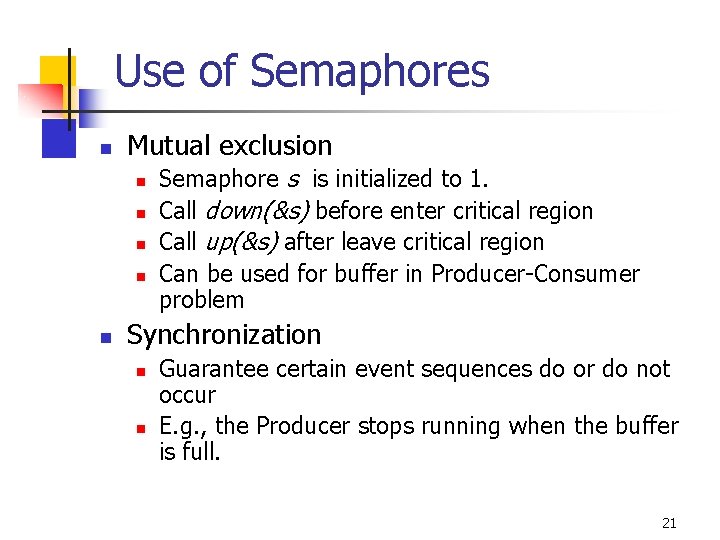
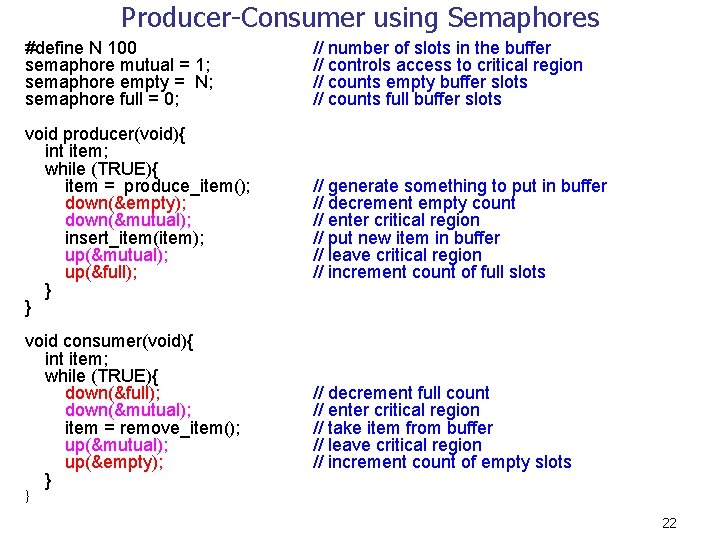
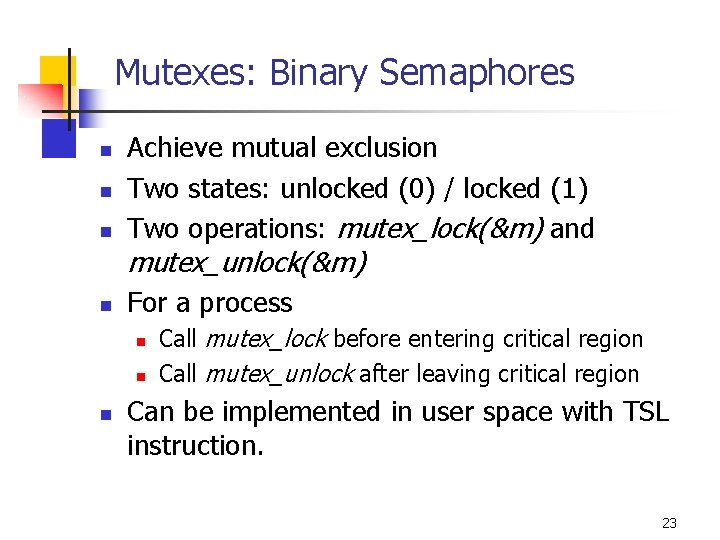
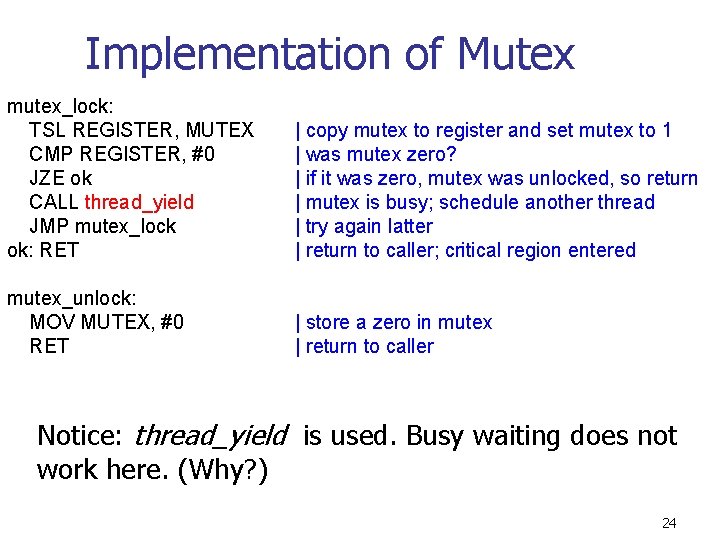
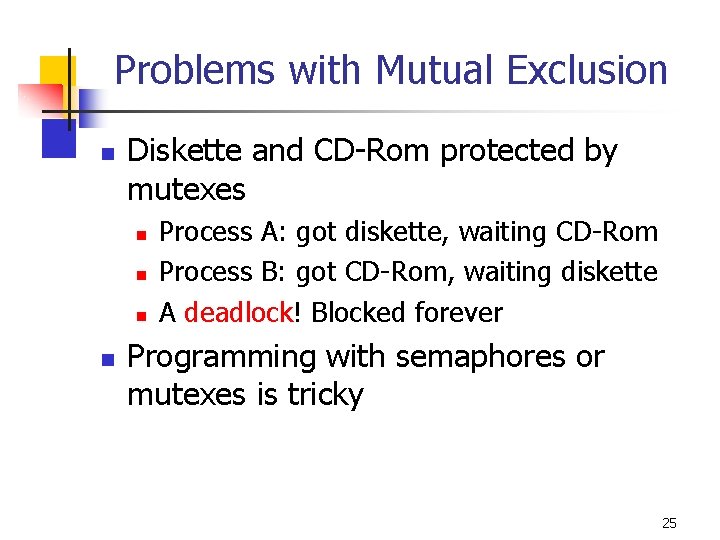
- Slides: 25
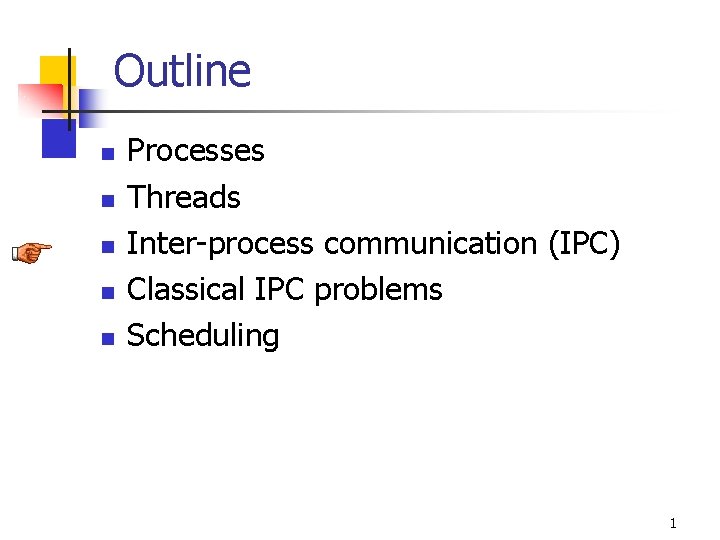
Outline n n n Processes Threads Inter-process communication (IPC) Classical IPC problems Scheduling 1
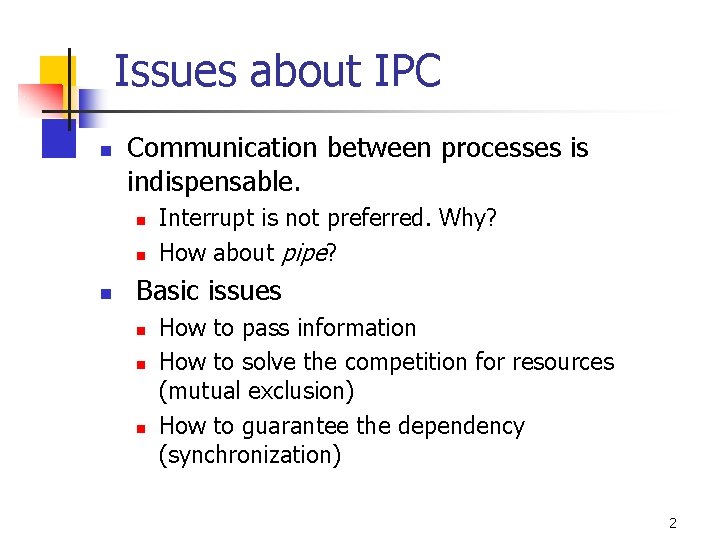
Issues about IPC n Communication between processes is indispensable. n n n Interrupt is not preferred. Why? How about pipe? Basic issues n n n How to pass information How to solve the competition for resources (mutual exclusion) How to guarantee the dependency (synchronization) 2
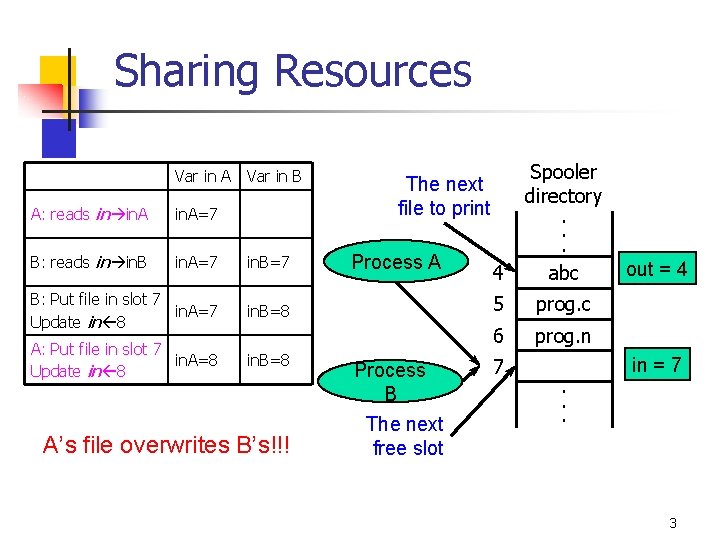
Sharing Resources Var in A Var in B A: reads in in. A=7 B: reads in in. B in. A=7 in. B=7 B: Put file in slot 7 in. A=7 Update in 8 in. B=8 A: Put file in slot 7 in. A=8 Update in 8 in. B=8 A’s file overwrites B’s!!! 4 Spooler directory. . . abc 5 prog. c 6 prog. n The next file to print Process A Process B The next free slot 7 . . . out = 4 in = 7 3
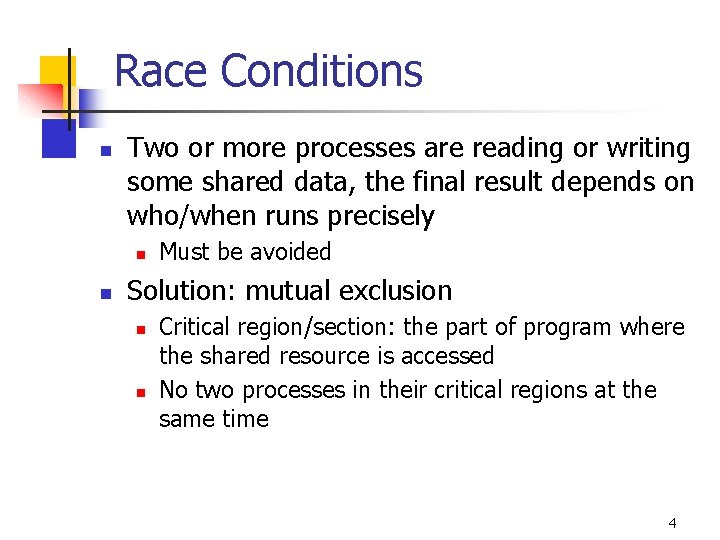
Race Conditions n Two or more processes are reading or writing some shared data, the final result depends on who/when runs precisely n n Must be avoided Solution: mutual exclusion n n Critical region/section: the part of program where the shared resource is accessed No two processes in their critical regions at the same time 4
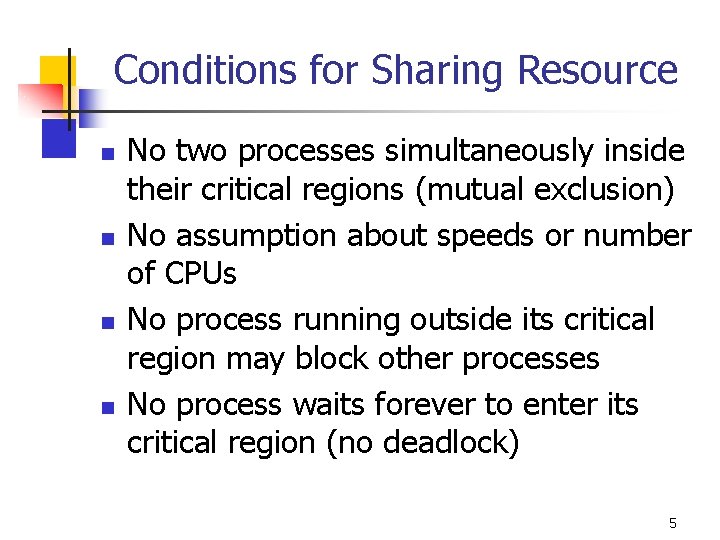
Conditions for Sharing Resource n n No two processes simultaneously inside their critical regions (mutual exclusion) No assumption about speeds or number of CPUs No process running outside its critical region may block other processes No process waits forever to enter its critical region (no deadlock) 5
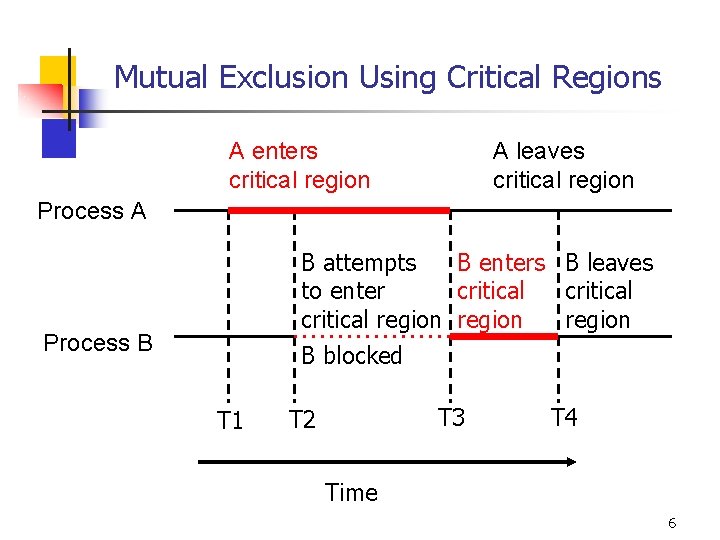
Mutual Exclusion Using Critical Regions A enters critical region A leaves critical region Process A B attempts B enters B leaves to enter critical region Process B B blocked T 1 T 3 T 2 T 4 Time 6
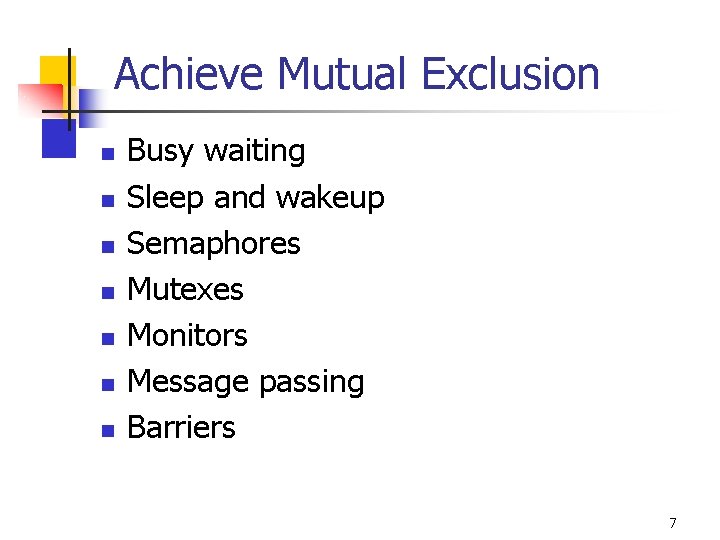
Achieve Mutual Exclusion n n n Busy waiting Sleep and wakeup Semaphores Mutexes Monitors Message passing Barriers 7
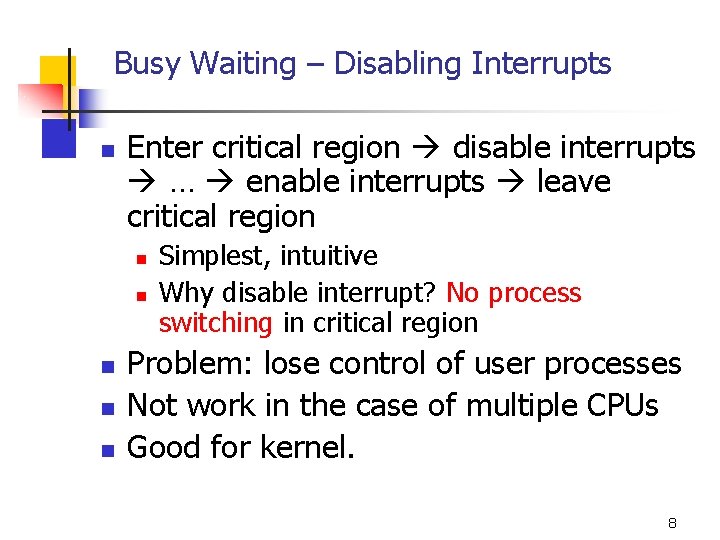
Busy Waiting – Disabling Interrupts n Enter critical region disable interrupts … enable interrupts leave critical region n n Simplest, intuitive Why disable interrupt? No process switching in critical region Problem: lose control of user processes Not work in the case of multiple CPUs Good for kernel. 8
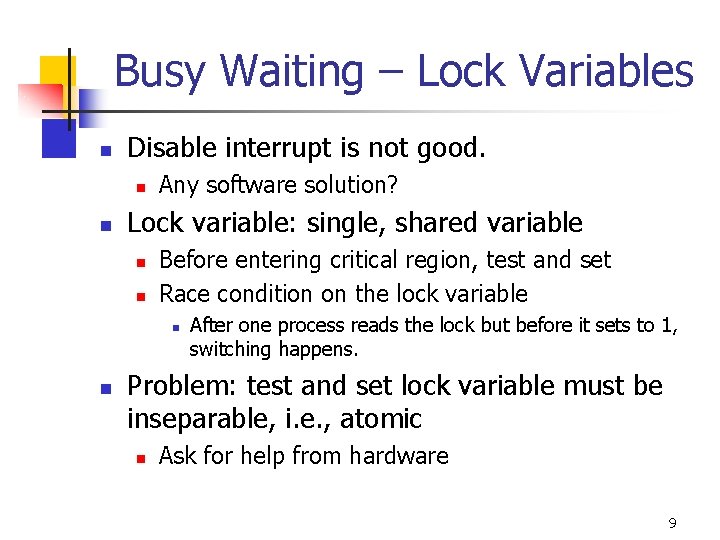
Busy Waiting – Lock Variables n Disable interrupt is not good. n n Any software solution? Lock variable: single, shared variable n n Before entering critical region, test and set Race condition on the lock variable n n After one process reads the lock but before it sets to 1, switching happens. Problem: test and set lock variable must be inseparable, i. e. , atomic n Ask for help from hardware 9
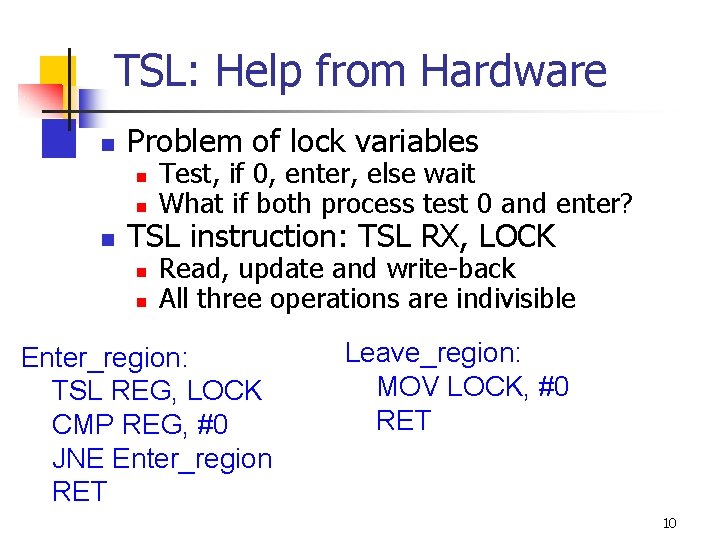
TSL: Help from Hardware n Problem of lock variables n n n Test, if 0, enter, else wait What if both process test 0 and enter? TSL instruction: TSL RX, LOCK n n Read, update and write-back All three operations are indivisible Enter_region: TSL REG, LOCK CMP REG, #0 JNE Enter_region RET Leave_region: MOV LOCK, #0 RET 10
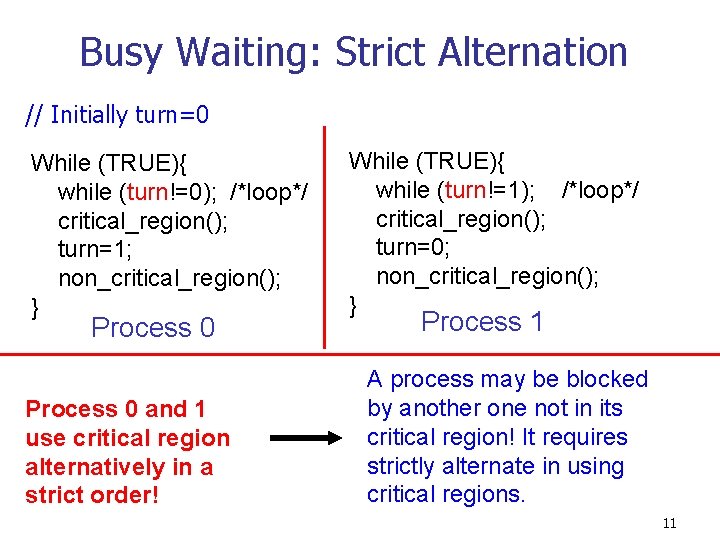
Busy Waiting: Strict Alternation // Initially turn=0 While (TRUE){ while (turn!=0); /*loop*/ critical_region(); turn=1; non_critical_region(); } Process 0 and 1 use critical region alternatively in a strict order! While (TRUE){ while (turn!=1); /*loop*/ critical_region(); turn=0; non_critical_region(); } Process 1 A process may be blocked by another one not in its critical region! It requires strictly alternate in using critical regions. 11
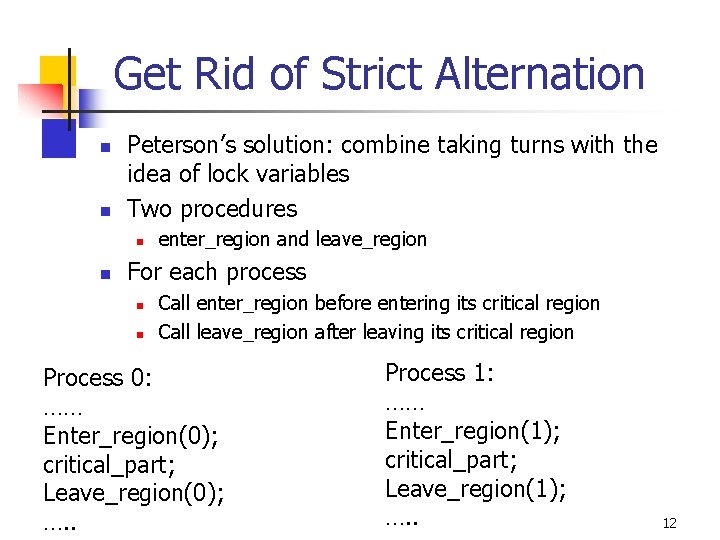
Get Rid of Strict Alternation n n Peterson’s solution: combine taking turns with the idea of lock variables Two procedures n n enter_region and leave_region For each process n n Call enter_region before entering its critical region Call leave_region after leaving its critical region Process 0: …… Enter_region(0); critical_part; Leave_region(0); …. . Process 1: …… Enter_region(1); critical_part; Leave_region(1); …. . 12
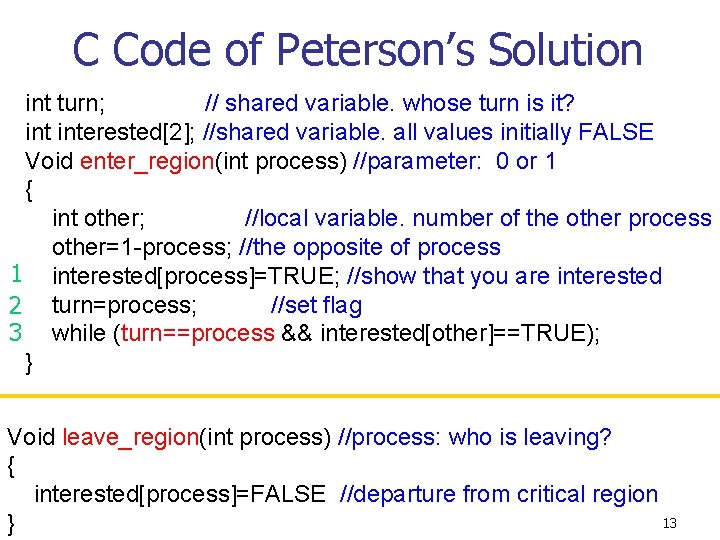
C Code of Peterson’s Solution int turn; // shared variable. whose turn is it? interested[2]; //shared variable. all values initially FALSE Void enter_region(int process) //parameter: 0 or 1 { int other; //local variable. number of the other process other=1 -process; //the opposite of process 1 interested[process]=TRUE; //show that you are interested //set flag 2 turn=process; 3 while (turn==process && interested[other]==TRUE); } Void leave_region(int process) //process: who is leaving? { interested[process]=FALSE //departure from critical region 13 }
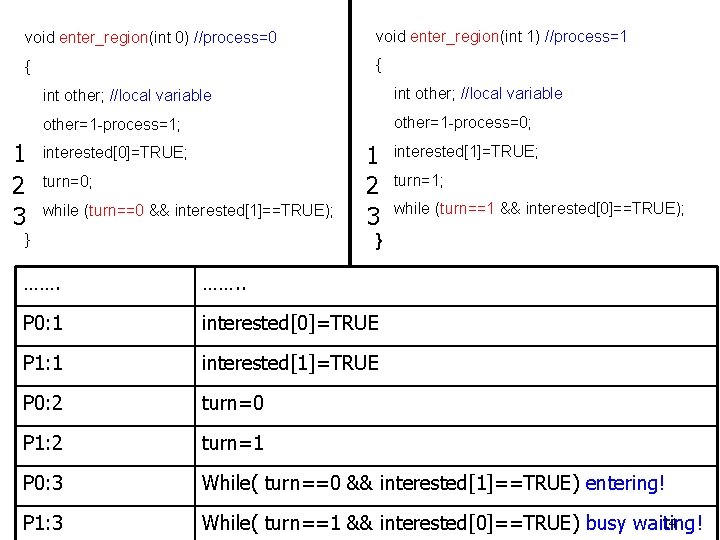
void enter_region(int 0) //process=0 void enter_region(int 1) //process=1 { { 1 2 3 int other; //local variable other=1 -process=1; other=1 -process=0; interested[0]=TRUE; turn=0; while (turn==0 && interested[1]==TRUE); 1 2 3 interested[1]=TRUE; turn=1; while (turn==1 && interested[0]==TRUE); } } ……. . P 0: 1 interested[0]=TRUE P 1: 1 interested[1]=TRUE P 0: 2 turn=0 P 1: 2 turn=1 P 0: 3 While( turn==0 && interested[1]==TRUE) entering! P 1: 3 14 While( turn==1 && interested[0]==TRUE) busy waiting!
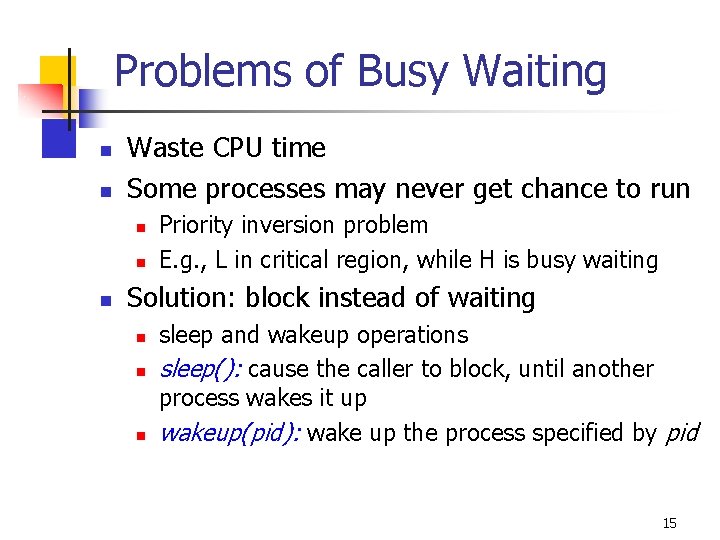
Problems of Busy Waiting n n Waste CPU time Some processes may never get chance to run n Priority inversion problem E. g. , L in critical region, while H is busy waiting Solution: block instead of waiting n n n sleep and wakeup operations sleep(): cause the caller to block, until another process wakes it up wakeup(pid): wake up the process specified by pid 15
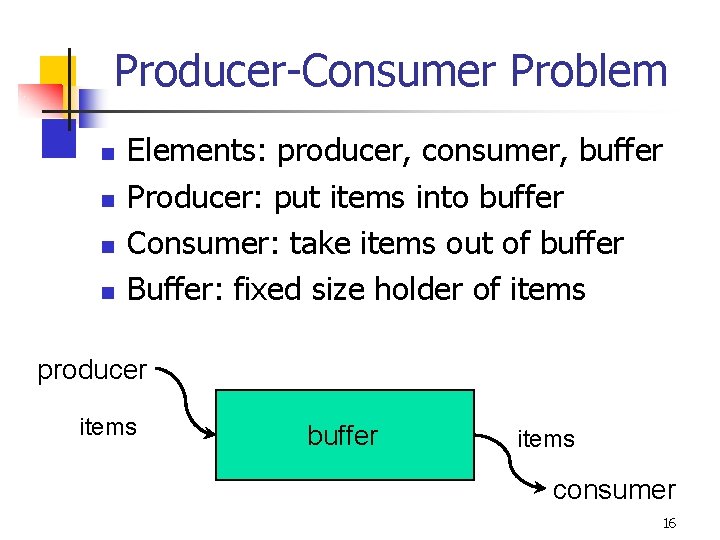
Producer-Consumer Problem n n Elements: producer, consumer, buffer Producer: put items into buffer Consumer: take items out of buffer Buffer: fixed size holder of items producer items buffer items consumer 16
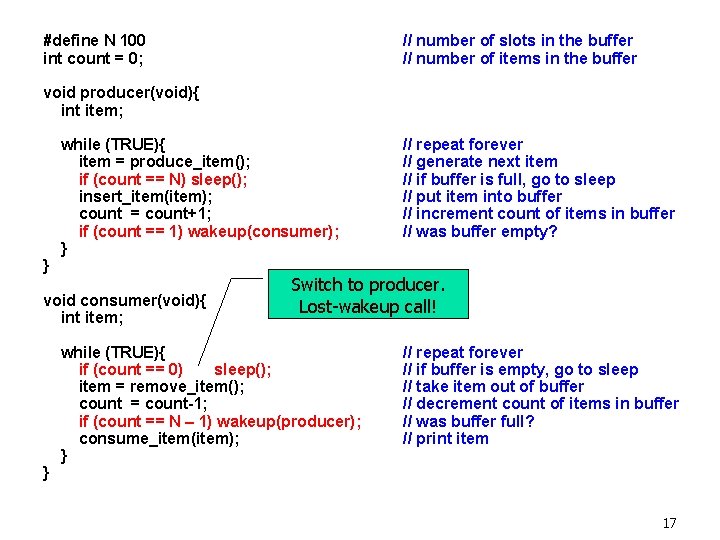
#define N 100 int count = 0; // number of slots in the buffer // number of items in the buffer void producer(void){ int item; } while (TRUE){ item = produce_item(); if (count == N) sleep(); insert_item(item); count = count+1; if (count == 1) wakeup(consumer); } void consumer(void){ int item; } // repeat forever // generate next item // if buffer is full, go to sleep // put item into buffer // increment count of items in buffer // was buffer empty? Switch to producer. Lost-wakeup call! while (TRUE){ if (count == 0) sleep(); item = remove_item(); count = count-1; if (count == N – 1) wakeup(producer); consume_item(item); } // repeat forever // if buffer is empty, go to sleep // take item out of buffer // decrement count of items in buffer // was buffer full? // print item 17
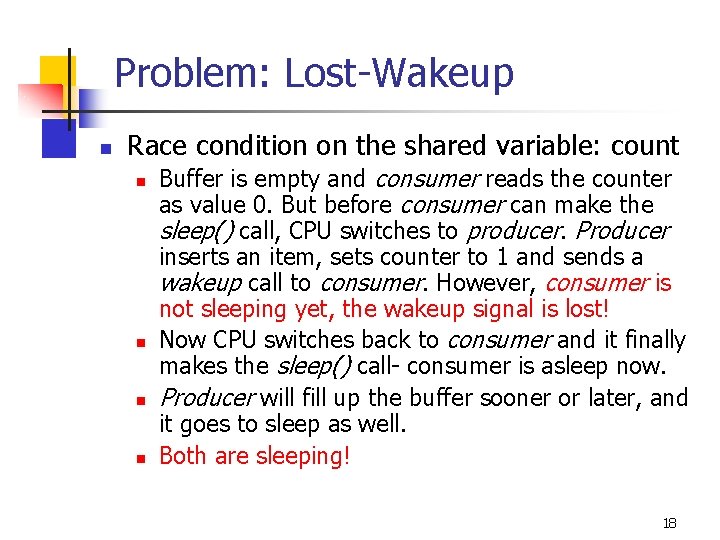
Problem: Lost-Wakeup n Race condition on the shared variable: count n n Buffer is empty and consumer reads the counter as value 0. But before consumer can make the sleep() call, CPU switches to producer. Producer inserts an item, sets counter to 1 and sends a wakeup call to consumer. However, consumer is not sleeping yet, the wakeup signal is lost! Now CPU switches back to consumer and it finally makes the sleep() call- consumer is asleep now. Producer will fill up the buffer sooner or later, and it goes to sleep as well. Both are sleeping! 18
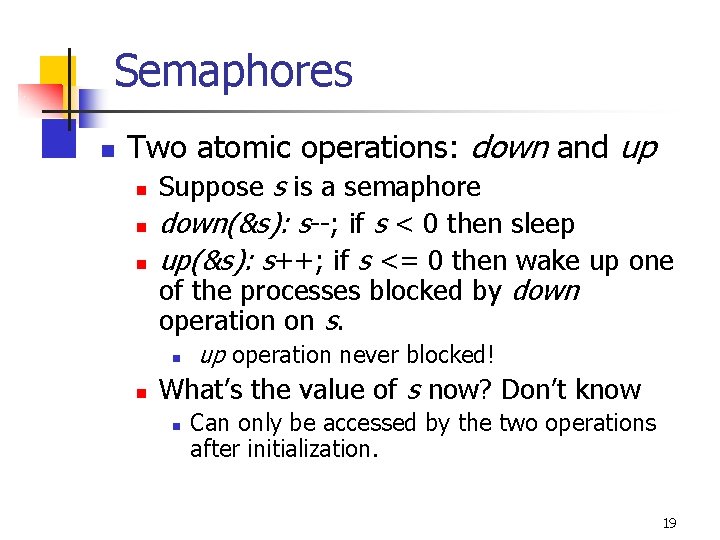
Semaphores n Two atomic operations: down and up n n n Suppose s is a semaphore down(&s): s--; if s < 0 then sleep up(&s): s++; if s <= 0 then wake up one of the processes blocked by down operation on s. n n up operation never blocked! What’s the value of s now? Don’t know n Can only be accessed by the two operations after initialization. 19
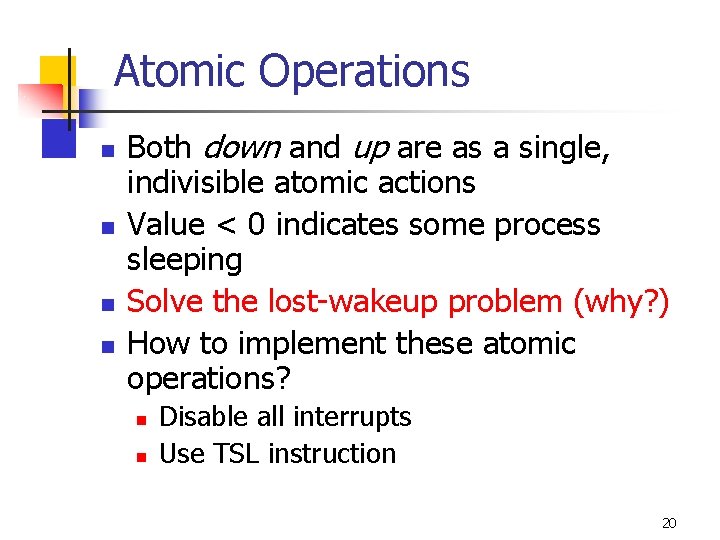
Atomic Operations n n Both down and up are as a single, indivisible atomic actions Value < 0 indicates some process sleeping Solve the lost-wakeup problem (why? ) How to implement these atomic operations? n n Disable all interrupts Use TSL instruction 20
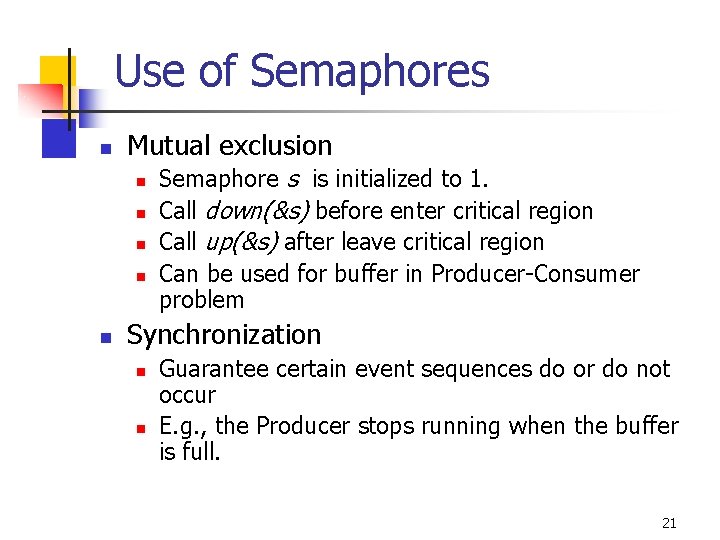
Use of Semaphores n Mutual exclusion n n Semaphore s is initialized to 1. Call down(&s) before enter critical region Call up(&s) after leave critical region Can be used for buffer in Producer-Consumer problem Synchronization n n Guarantee certain event sequences do or do not occur E. g. , the Producer stops running when the buffer is full. 21
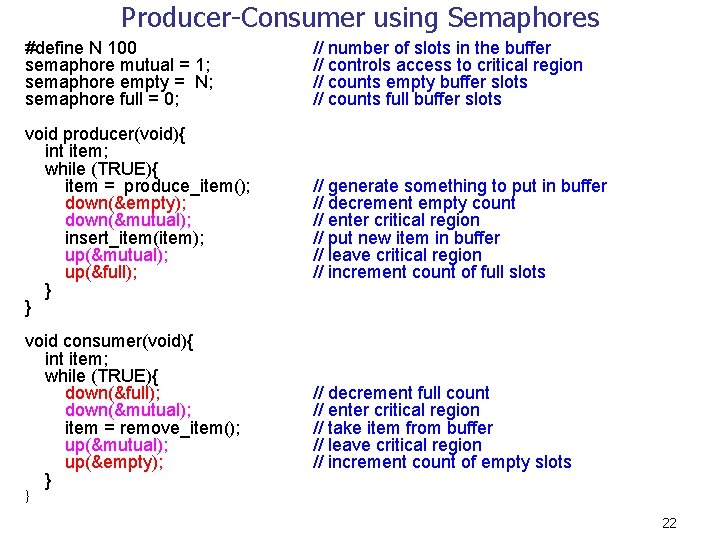
Producer-Consumer using Semaphores #define N 100 semaphore mutual = 1; semaphore empty = N; semaphore full = 0; // number of slots in the buffer // controls access to critical region // counts empty buffer slots // counts full buffer slots void producer(void){ int item; while (TRUE){ item = produce_item(); down(&empty); down(&mutual); insert_item(item); up(&mutual); up(&full); } } // generate something to put in buffer // decrement empty count // enter critical region // put new item in buffer // leave critical region // increment count of full slots void consumer(void){ int item; while (TRUE){ down(&full); down(&mutual); item = remove_item(); up(&mutual); up(&empty); } // decrement full count // enter critical region // take item from buffer // leave critical region // increment count of empty slots } 22
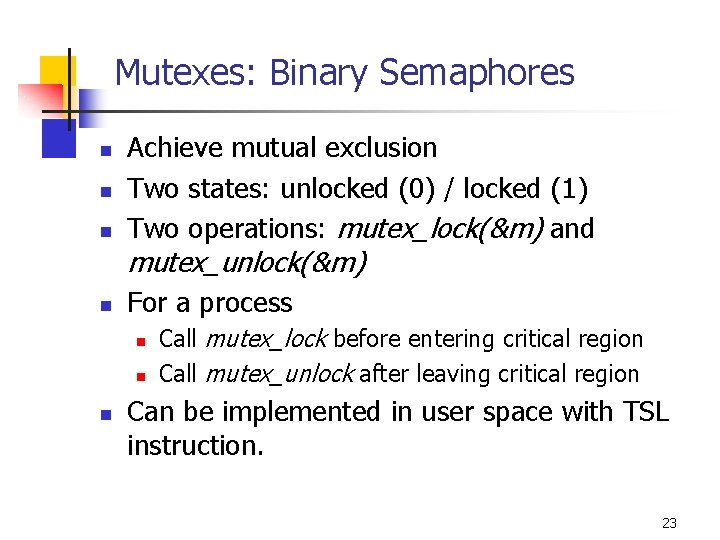
Mutexes: Binary Semaphores n n n Achieve mutual exclusion Two states: unlocked (0) / locked (1) Two operations: mutex_lock(&m) and mutex_unlock(&m) n For a process n n n Call mutex_lock before entering critical region Call mutex_unlock after leaving critical region Can be implemented in user space with TSL instruction. 23
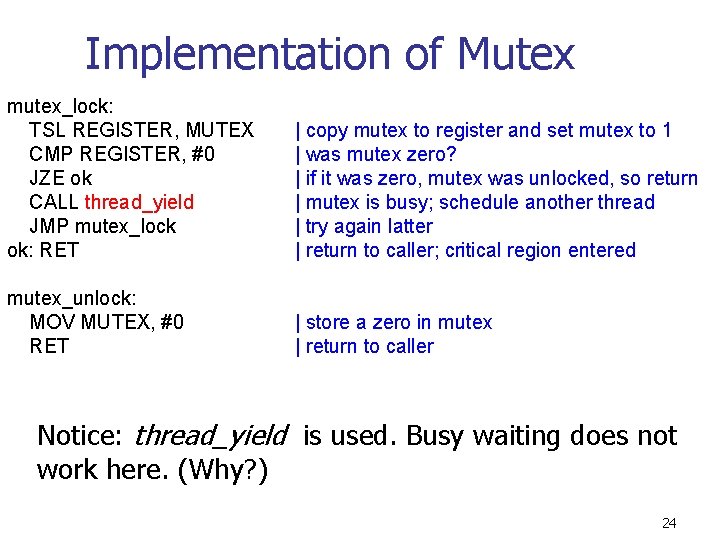
Implementation of Mutex mutex_lock: TSL REGISTER, MUTEX CMP REGISTER, #0 JZE ok CALL thread_yield JMP mutex_lock ok: RET | copy mutex to register and set mutex to 1 | was mutex zero? | if it was zero, mutex was unlocked, so return | mutex is busy; schedule another thread | try again latter | return to caller; critical region entered mutex_unlock: MOV MUTEX, #0 RET | store a zero in mutex | return to caller Notice: thread_yield is used. Busy waiting does not work here. (Why? ) 24
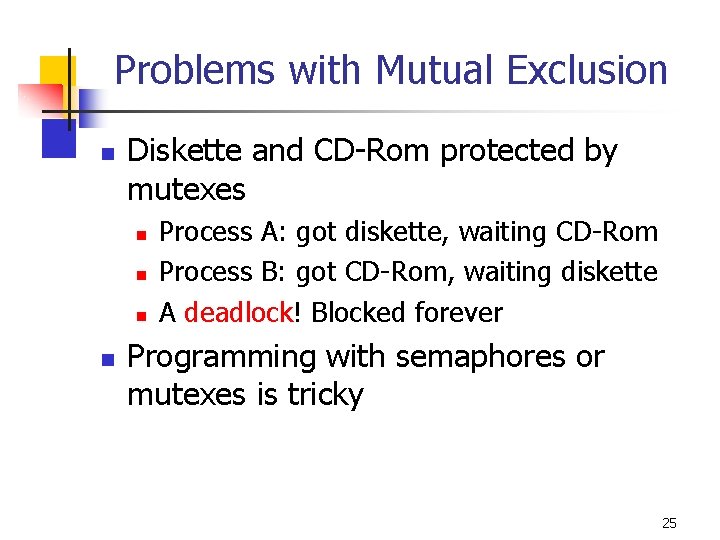
Problems with Mutual Exclusion n Diskette and CD-Rom protected by mutexes n n Process A: got diskette, waiting CD-Rom Process B: got CD-Rom, waiting diskette A deadlock! Blocked forever Programming with semaphores or mutexes is tricky 25
Interprocess communication in linux
Java interprocess communication
Message passing os
Interprocess communication in os
Race condition in interprocess communication
External data representation in distributed system
Android interprocess communication
Interprocess communication
Process thread
Threads vs processes
Special relativity vs general relativity
Concurrent processes are processes that
Examples of quote sandwiches
Communication integration processes
Katherine miller organizational communication
C11 thread
Shared memory java
Conventional representation of threads
Process and threads
A flexible flat material made by interlacing threads/fibers
Escalonamento de threads
Process and threads
Os threads
The vinaya pitaka is a sacred text of………….
Needle like threads of spongy bone
Helps press seams in tubes like sleeves