Computer Systems InterProcess Communication via Sockets Interprocess Communication
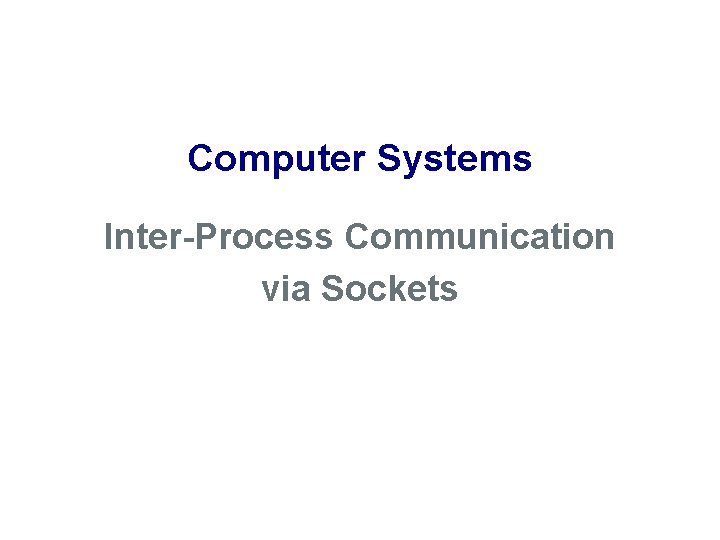
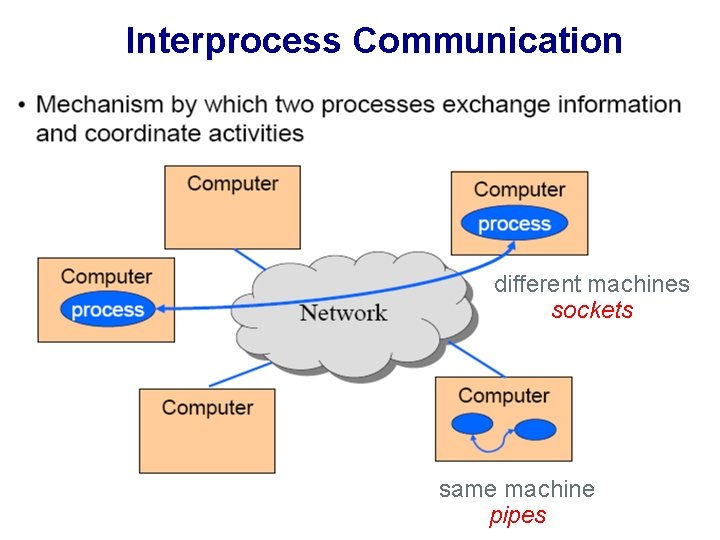
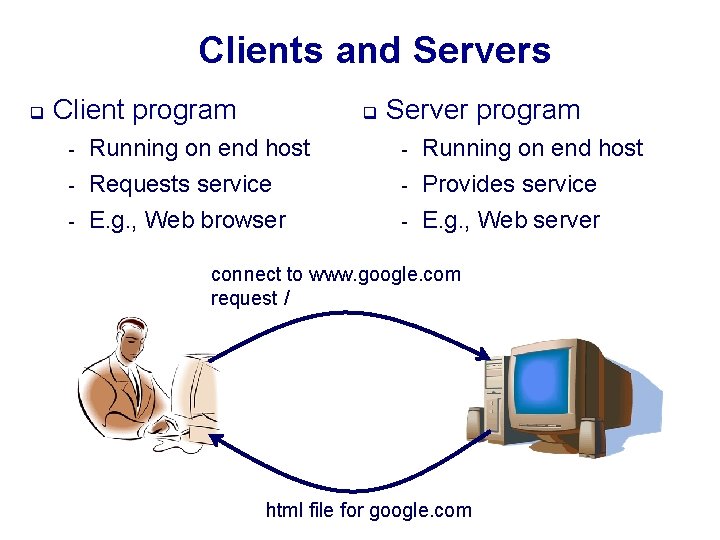
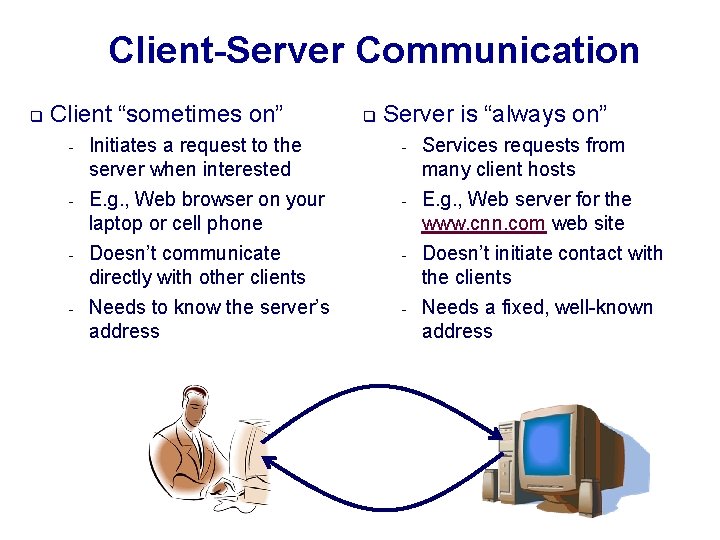
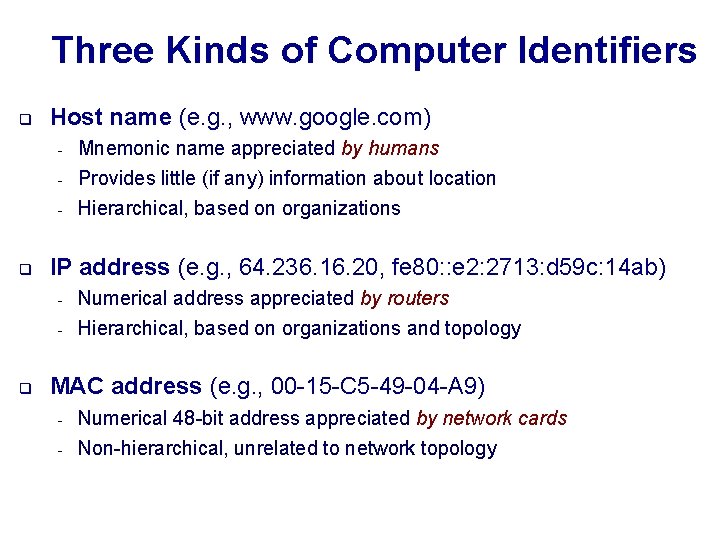
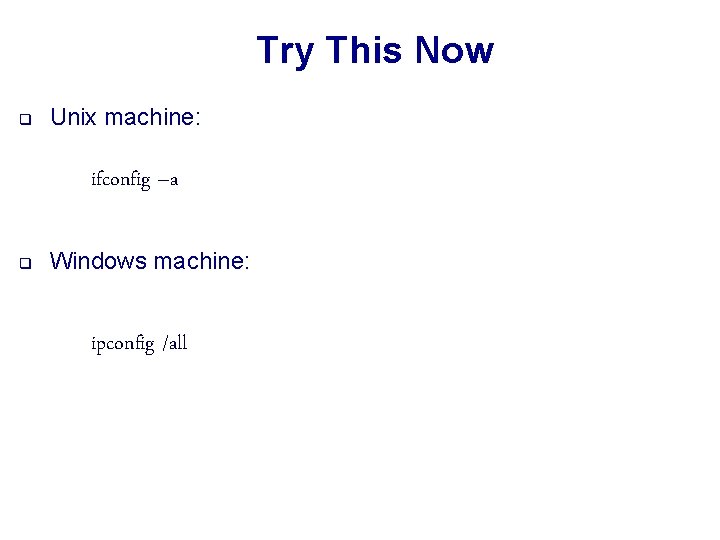
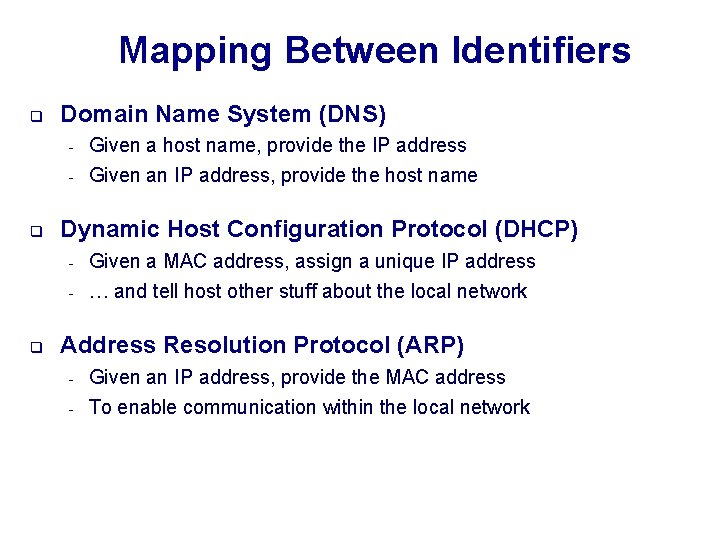
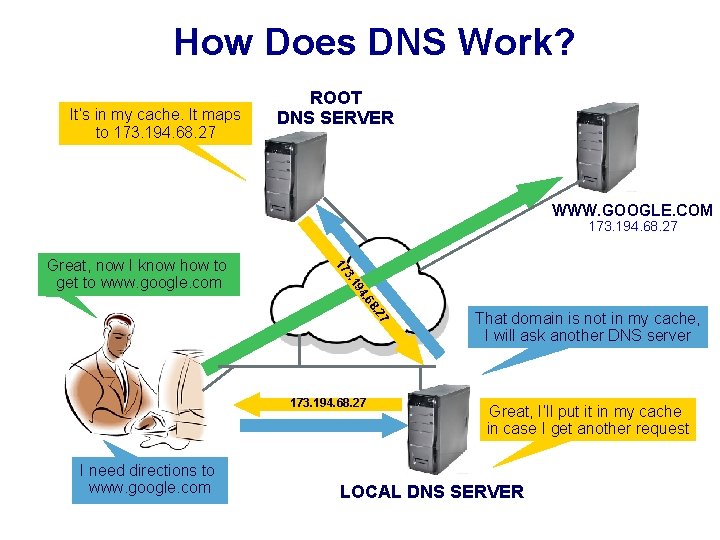
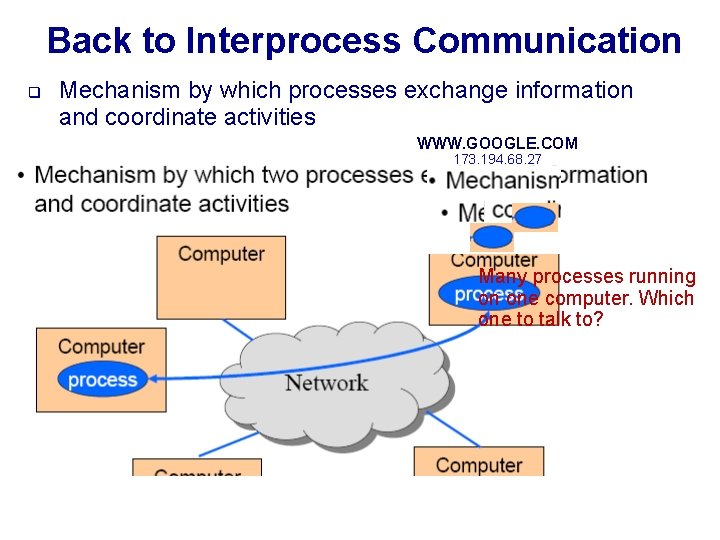
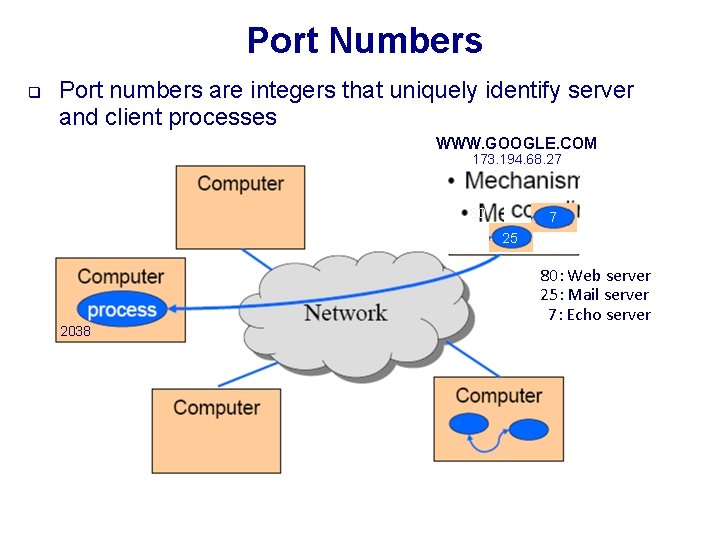
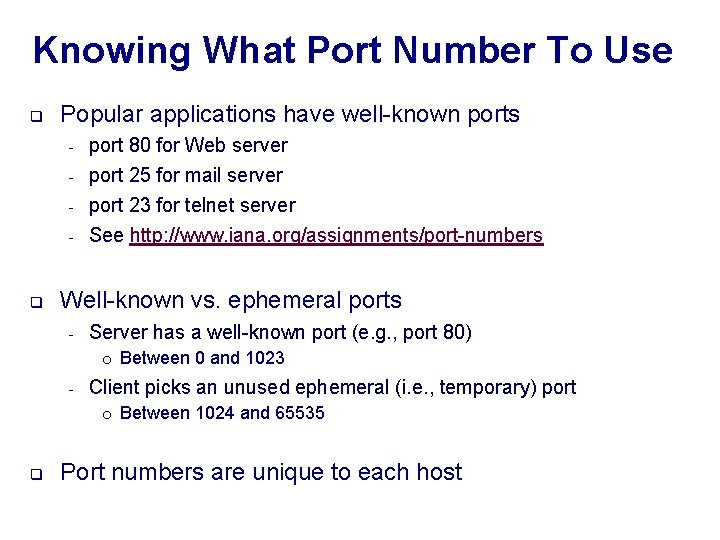
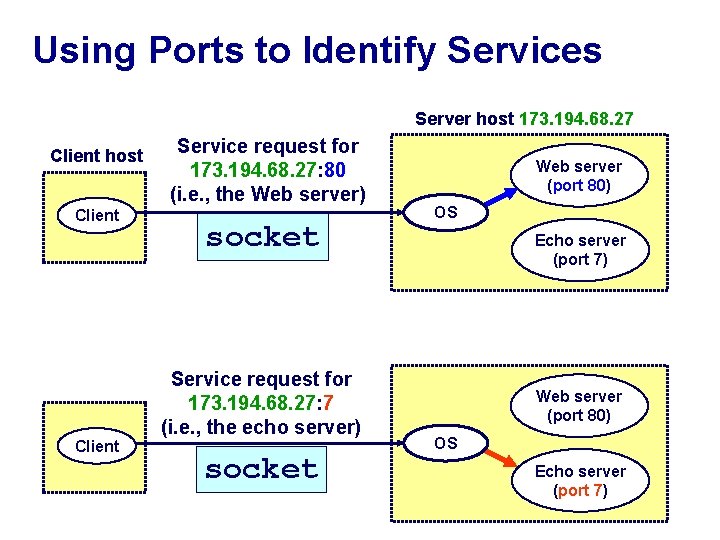
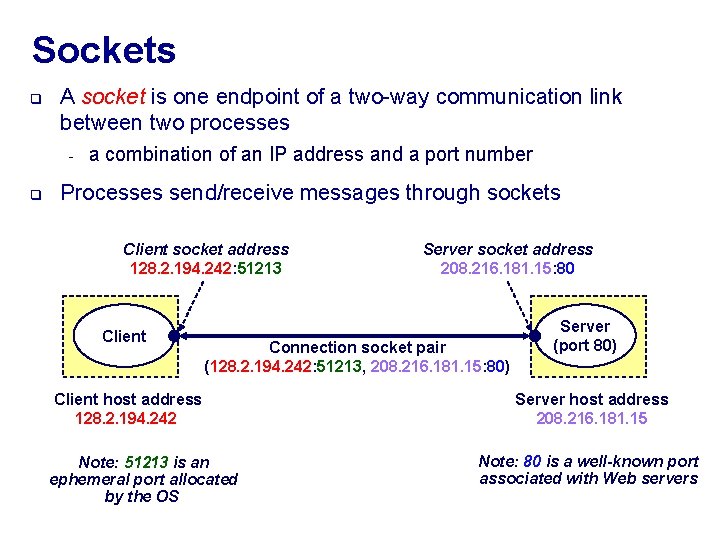
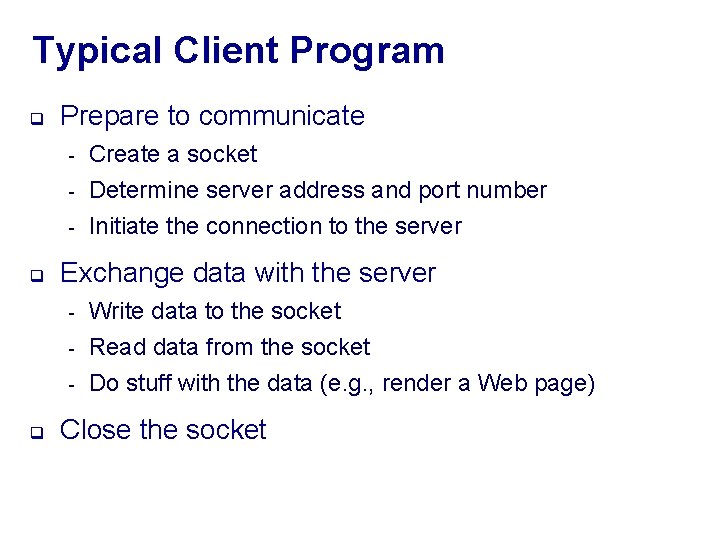
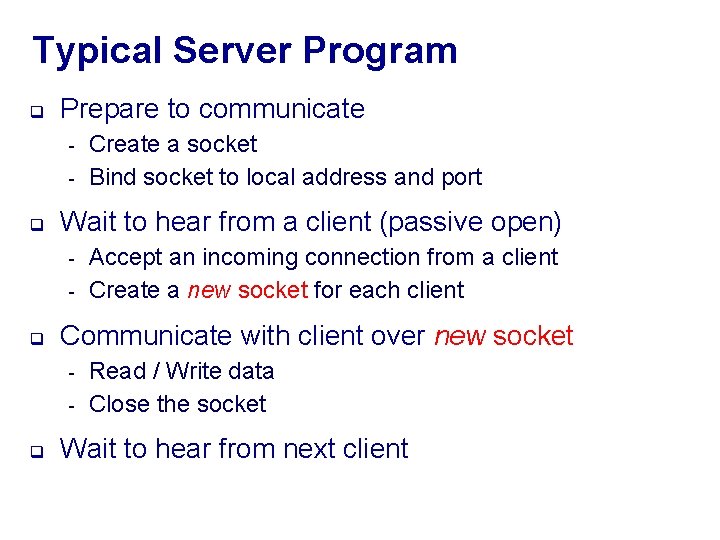
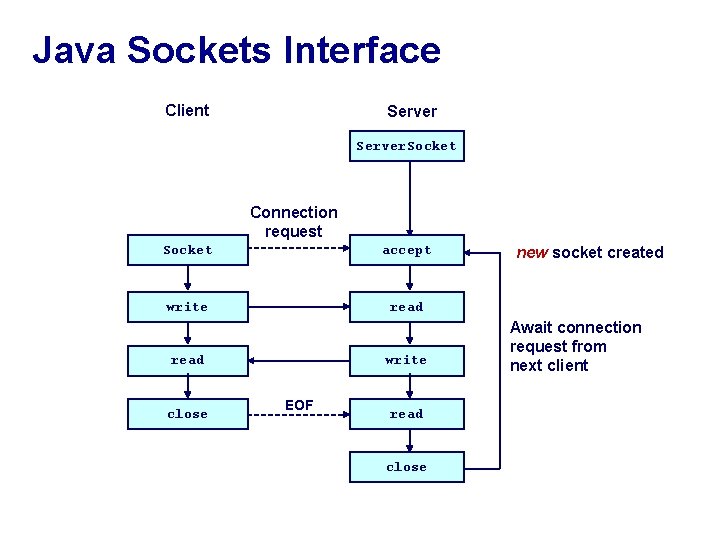
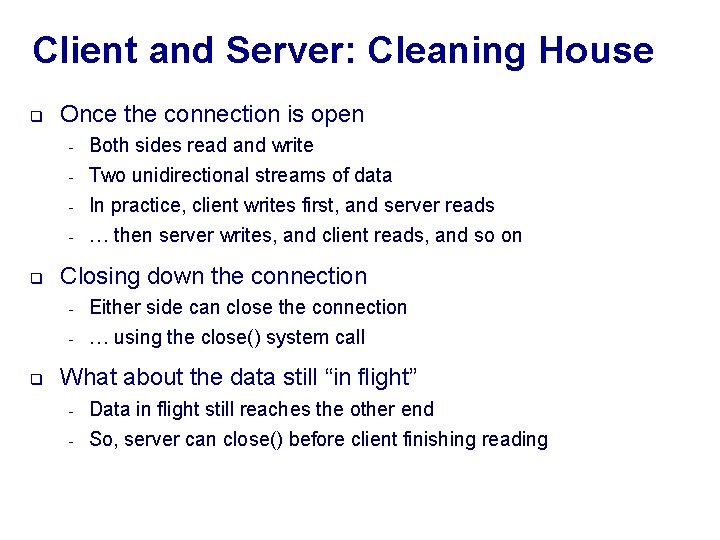
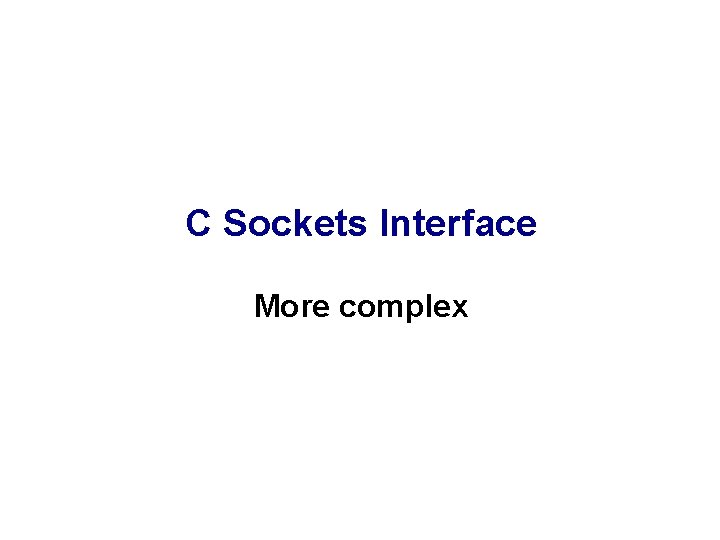
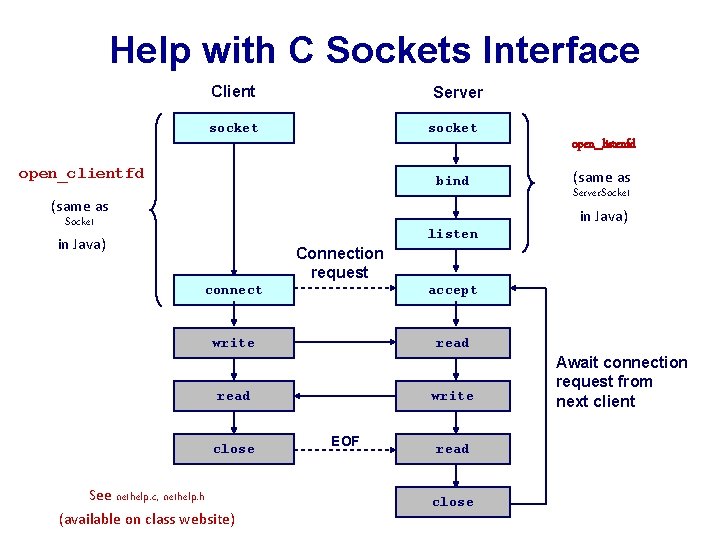
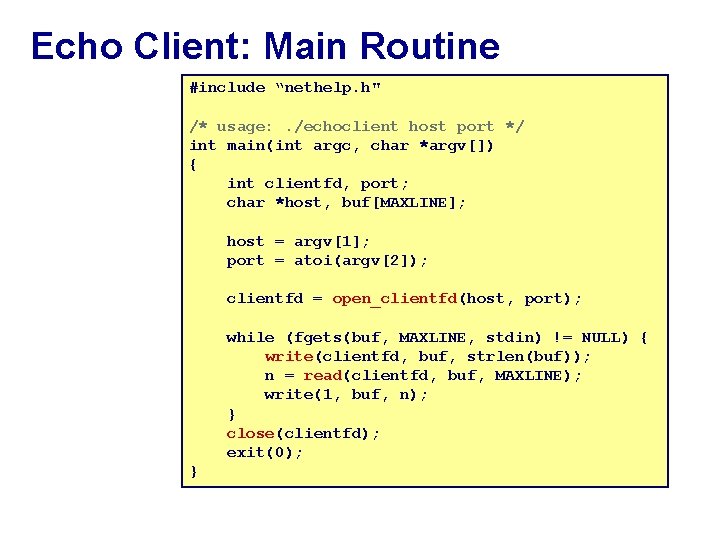
![Multi-threaded Echo Server: Main Routine int main(int argc, char *argv[]) { int listenfd, connfd, Multi-threaded Echo Server: Main Routine int main(int argc, char *argv[]) { int listenfd, connfd,](https://slidetodoc.com/presentation_image_h/0462c69286f8a1a90cf193c794f76d14/image-21.jpg)
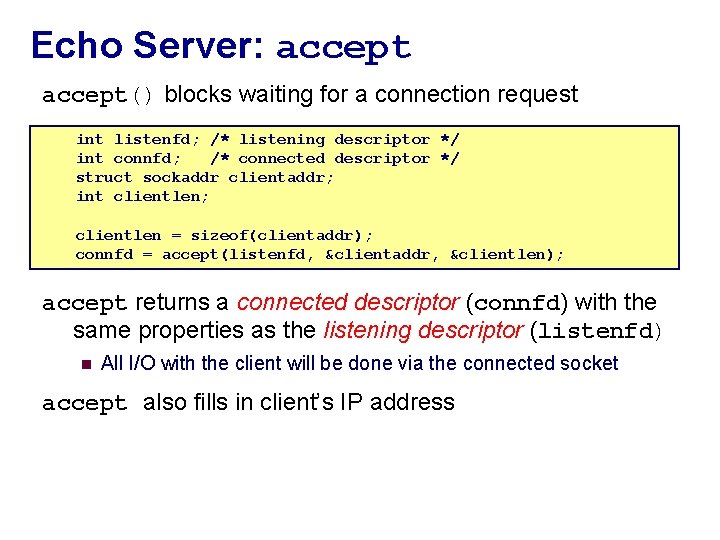
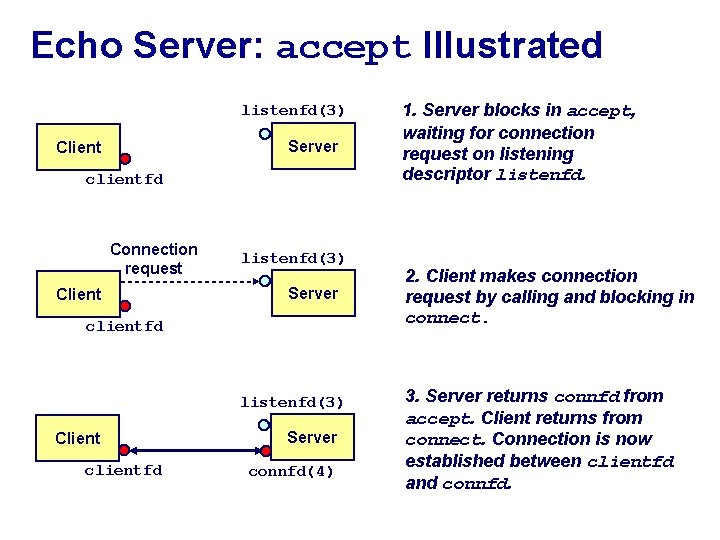
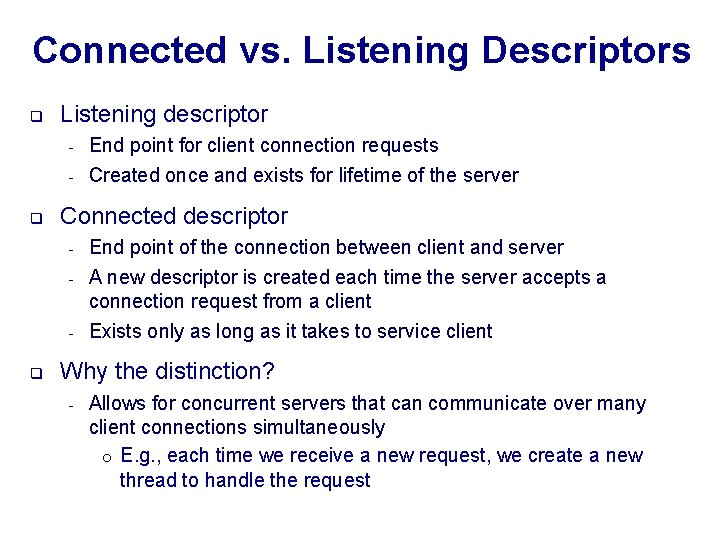
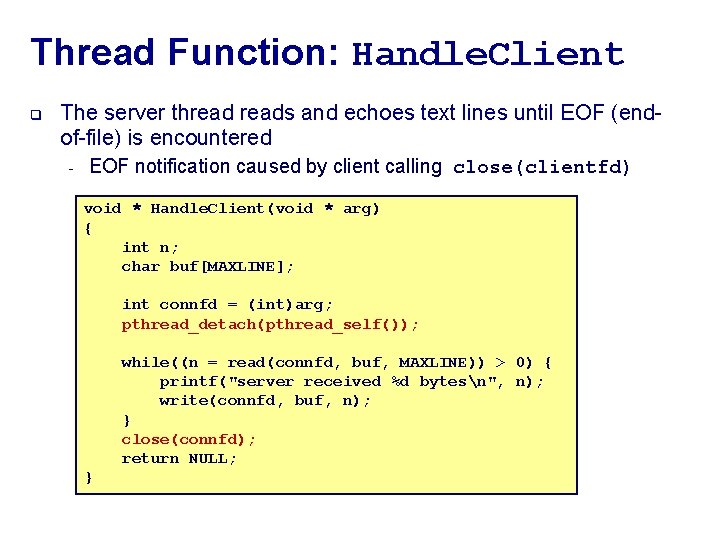
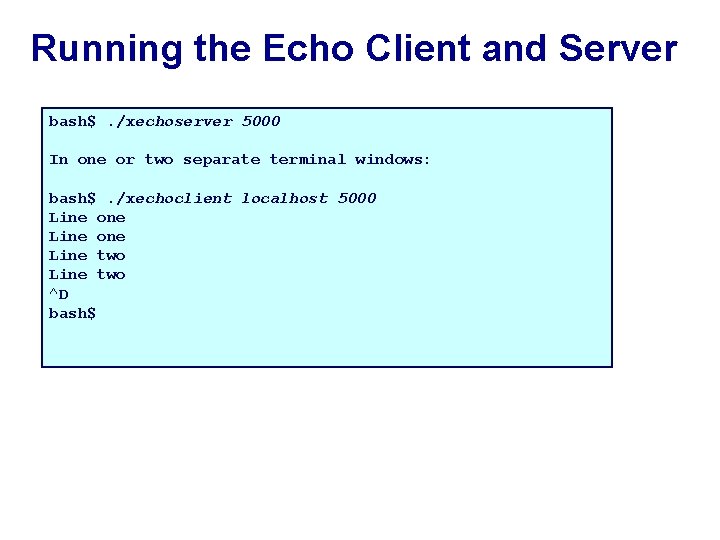
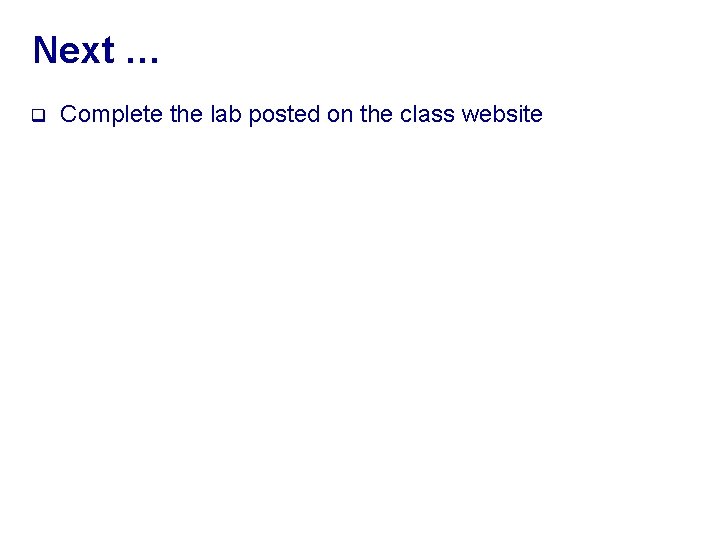
- Slides: 27
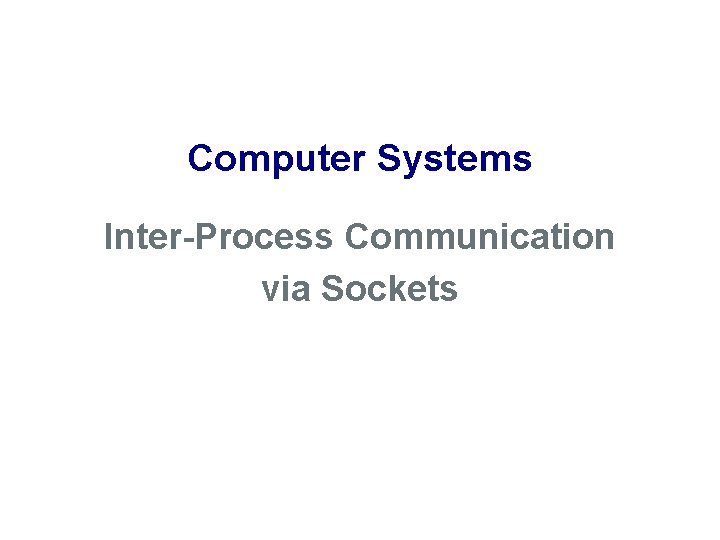
Computer Systems Inter-Process Communication via Sockets
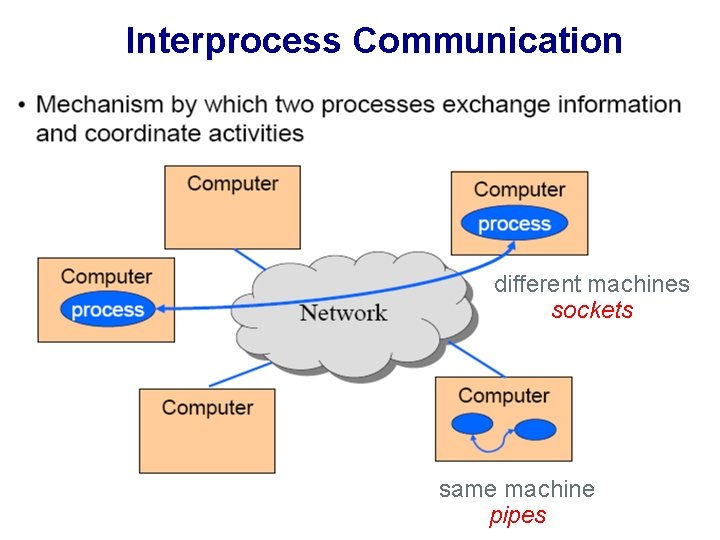
Interprocess Communication different machines sockets same machine pipes
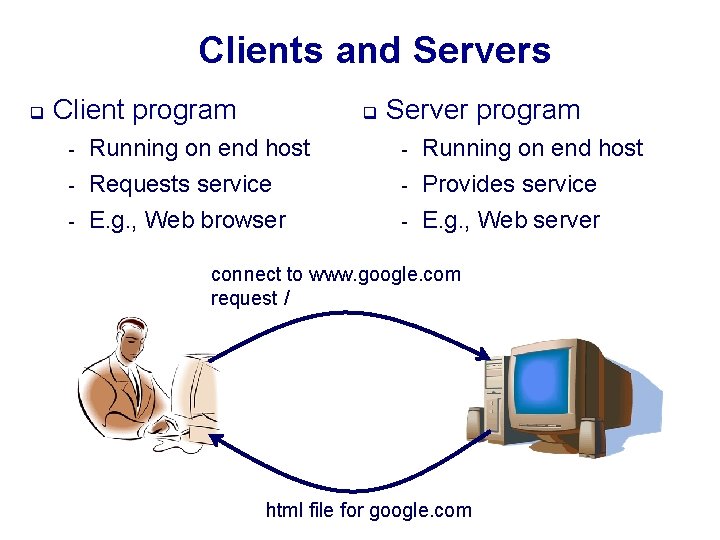
Clients and Servers q Client program - q Running on end host Requests service E. g. , Web browser Server program - Running on end host Provides service E. g. , Web server connect to www. google. com request / html file for google. com
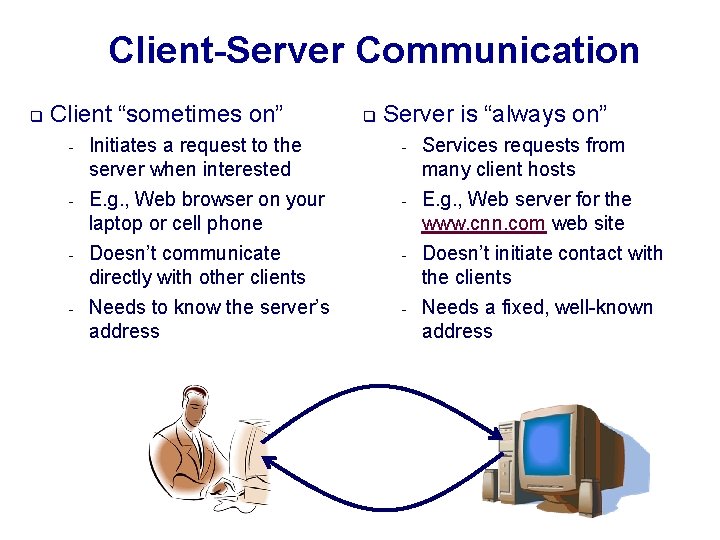
Client-Server Communication q Client “sometimes on” - Initiates a request to the server when interested E. g. , Web browser on your laptop or cell phone Doesn’t communicate directly with other clients Needs to know the server’s address q Server is “always on” - Services requests from many client hosts E. g. , Web server for the www. cnn. com web site Doesn’t initiate contact with the clients Needs a fixed, well-known address
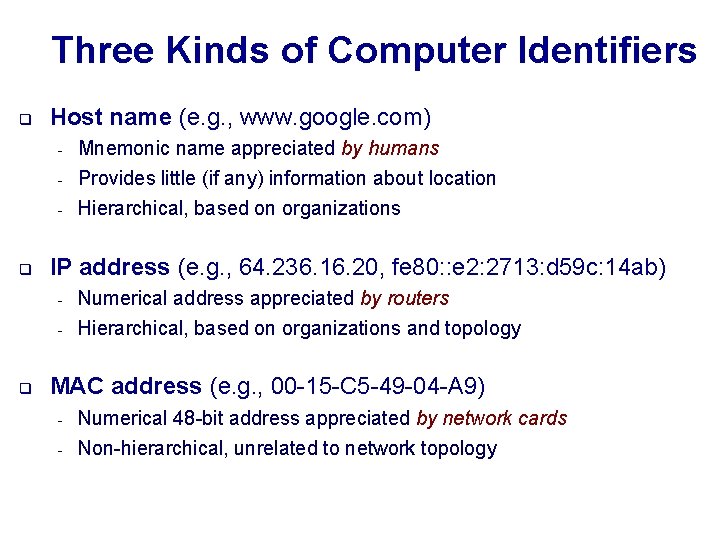
Three Kinds of Computer Identifiers q Host name (e. g. , www. google. com) - Mnemonic name appreciated by humans - Provides little (if any) information about location Hierarchical, based on organizations - q IP address (e. g. , 64. 236. 16. 20, fe 80: : e 2: 2713: d 59 c: 14 ab) - q Numerical address appreciated by routers Hierarchical, based on organizations and topology MAC address (e. g. , 00 -15 -C 5 -49 -04 -A 9) - Numerical 48 -bit address appreciated by network cards Non-hierarchical, unrelated to network topology
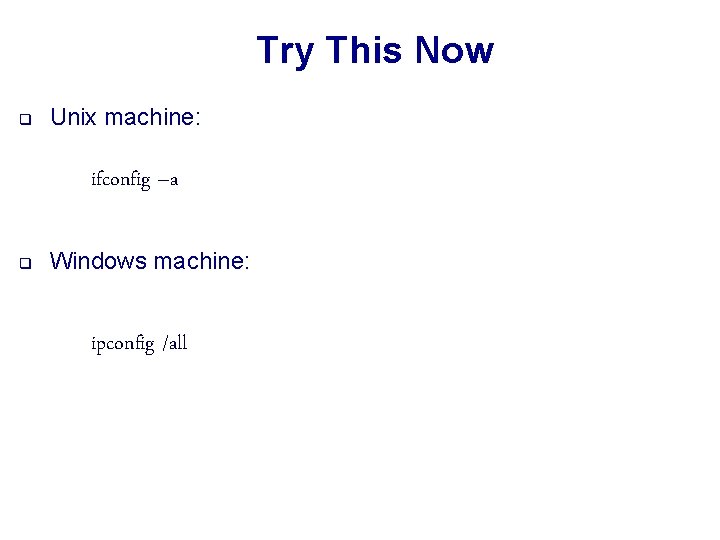
Try This Now q Unix machine: ifconfig –a q Windows machine: ipconfig /all
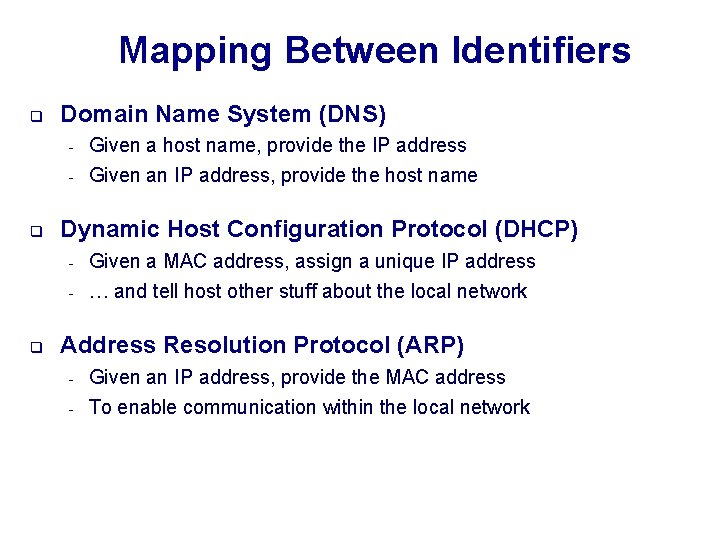
Mapping Between Identifiers q q Domain Name System (DNS) - Given a host name, provide the IP address - Given an IP address, provide the host name Dynamic Host Configuration Protocol (DHCP) - q Given a MAC address, assign a unique IP address … and tell host other stuff about the local network Address Resolution Protocol (ARP) - Given an IP address, provide the MAC address To enable communication within the local network
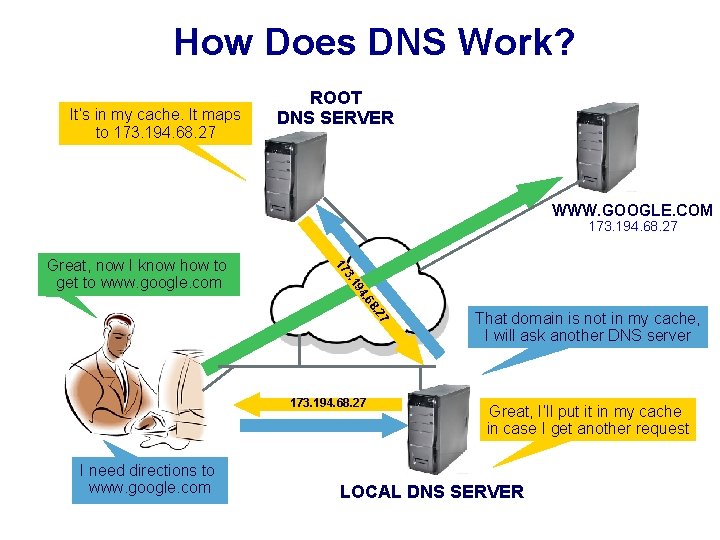
How Does DNS Work? It’s in my cache. It maps to 173. 194. 68. 27 ROOT DNS SERVER WWW. GOOGLE. COM 173. 194. 68. 27 19 3. 17 Great, now I know how to get to www. google. com 68 4. 7. 2 173. 194. 68. 27 I need directions to www. google. com That domain is not in my cache, I will ask another DNS server Great, I’ll put it in my cache in case I get another request LOCAL DNS SERVER
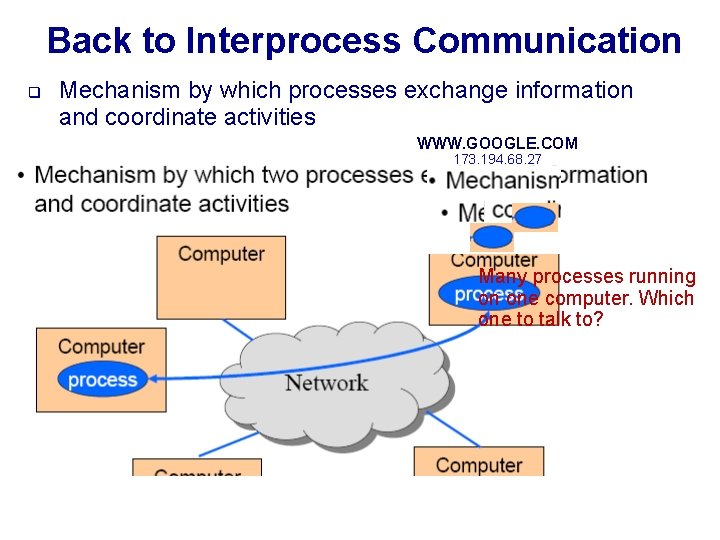
Back to Interprocess Communication q Mechanism by which processes exchange information and coordinate activities WWW. GOOGLE. COM 173. 194. 68. 27 Many processes running on one computer. Which one to talk to?
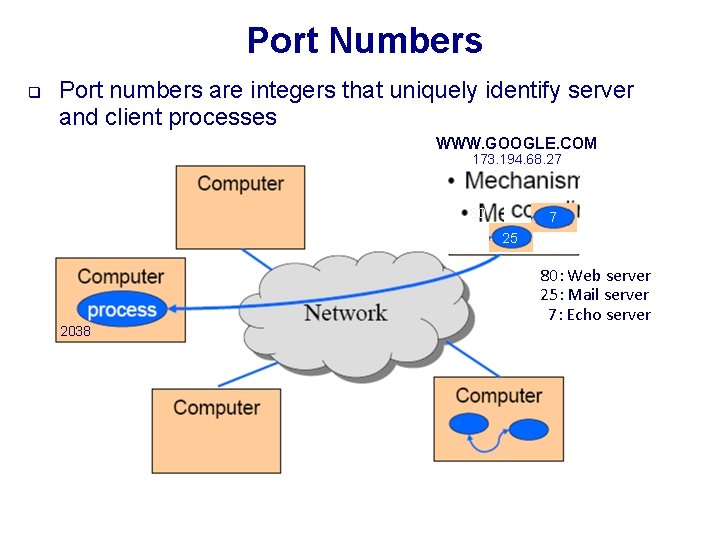
Port Numbers q Port numbers are integers that uniquely identify server and client processes WWW. GOOGLE. COM 173. 194. 68. 27 80 7 25 2038 80: Web server 25: Mail server 7: Echo server
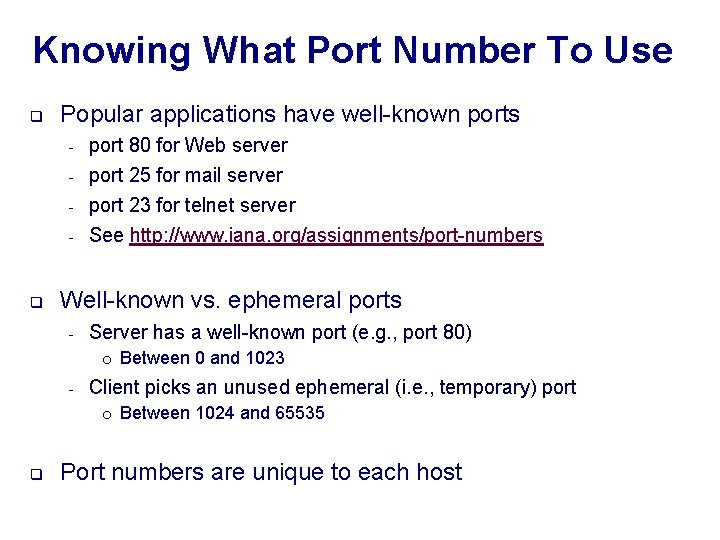
Knowing What Port Number To Use q q Popular applications have well-known ports - port 80 for Web server - port 25 for mail server port 23 for telnet server - See http: //www. iana. org/assignments/port-numbers Well-known vs. ephemeral ports - Server has a well-known port (e. g. , port 80) o Between 0 and 1023 - Client picks an unused ephemeral (i. e. , temporary) port o Between 1024 and 65535 q Port numbers are unique to each host
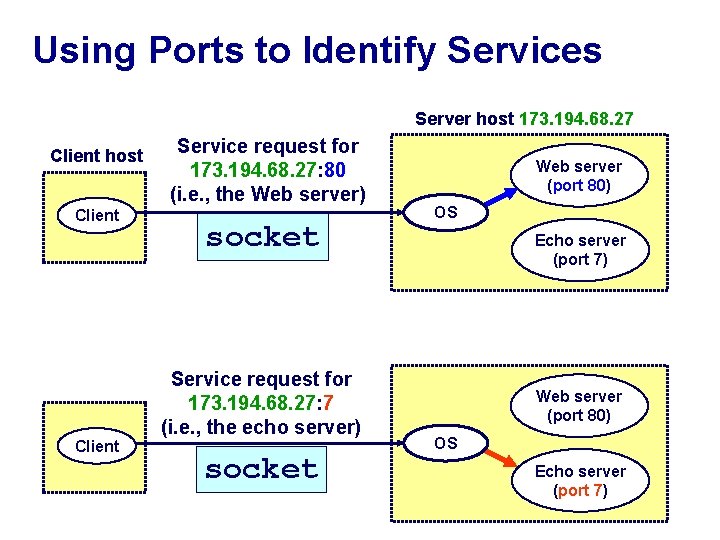
Using Ports to Identify Services Server host 173. 194. 68. 27 Client host Client Service request for 173. 194. 68. 27: 80 (i. e. , the Web server) socket Service request for 173. 194. 68. 27: 7 (i. e. , the echo server) socket Web server (port 80) OS Echo server (port 7)
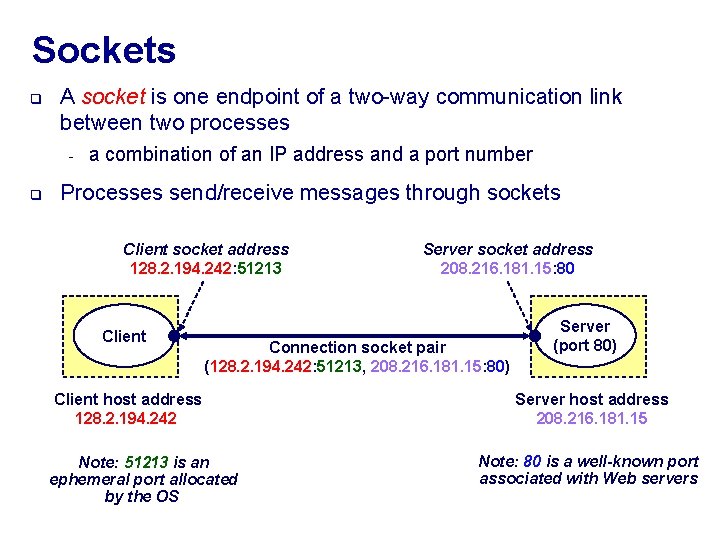
Sockets q A socket is one endpoint of a two-way communication link between two processes - q a combination of an IP address and a port number Processes send/receive messages through sockets Client socket address 128. 2. 194. 242: 51213 Client Server socket address 208. 216. 181. 15: 80 Connection socket pair (128. 2. 194. 242: 51213, 208. 216. 181. 15: 80) Client host address 128. 2. 194. 242 Note: 51213 is an ephemeral port allocated by the OS Server (port 80) Server host address 208. 216. 181. 15 Note: 80 is a well-known port associated with Web servers
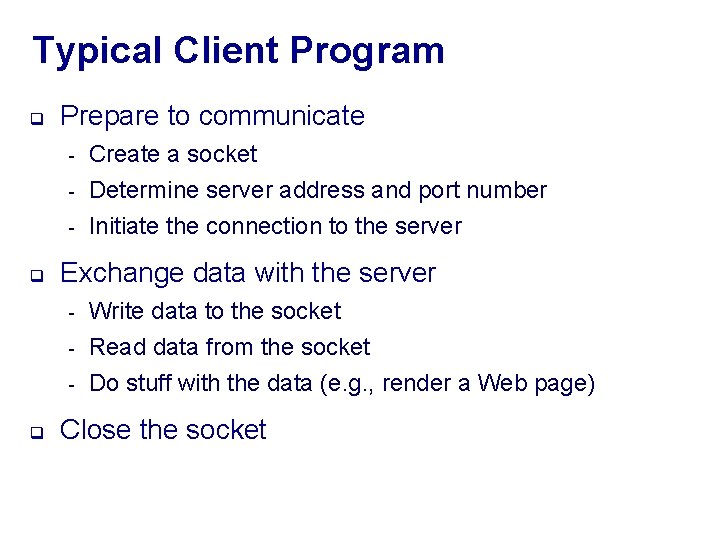
Typical Client Program q Prepare to communicate - q Exchange data with the server - Write data to the socket Read data from the socket - Do stuff with the data (e. g. , render a Web page) - q Create a socket Determine server address and port number Initiate the connection to the server Close the socket
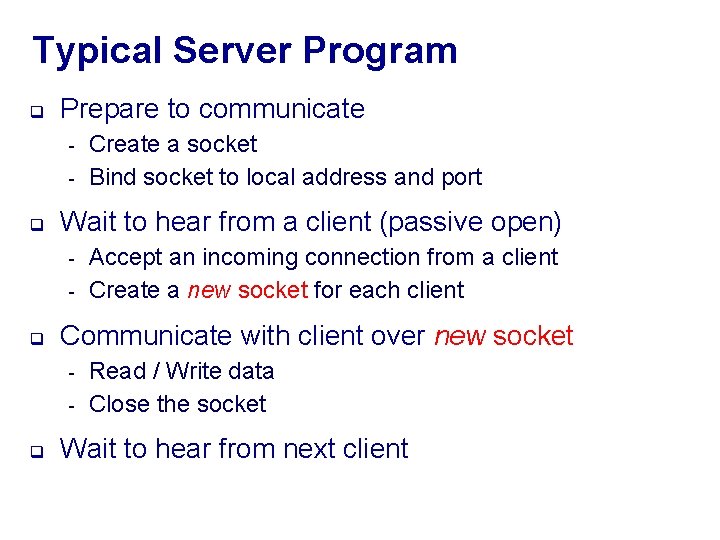
Typical Server Program q Prepare to communicate - q Wait to hear from a client (passive open) - q Accept an incoming connection from a client Create a new socket for each client Communicate with client over new socket - q Create a socket Bind socket to local address and port Read / Write data Close the socket Wait to hear from next client
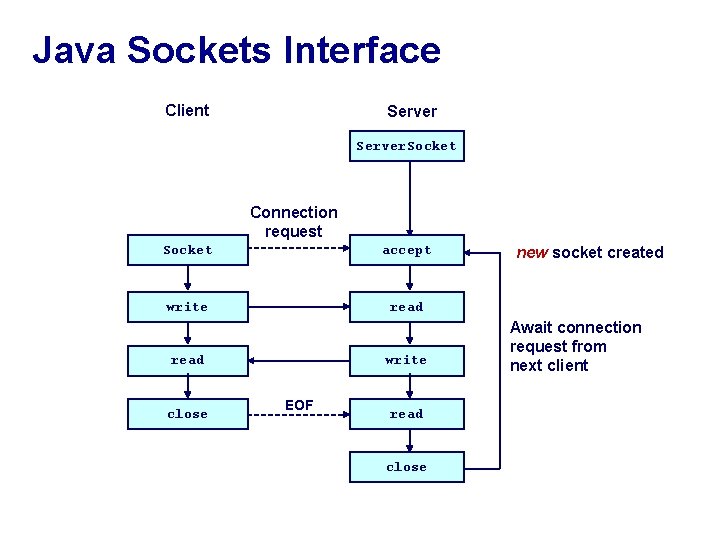
Java Sockets Interface Client Server. Socket Connection request Socket accept write read close write EOF read close new socket created Await connection request from next client
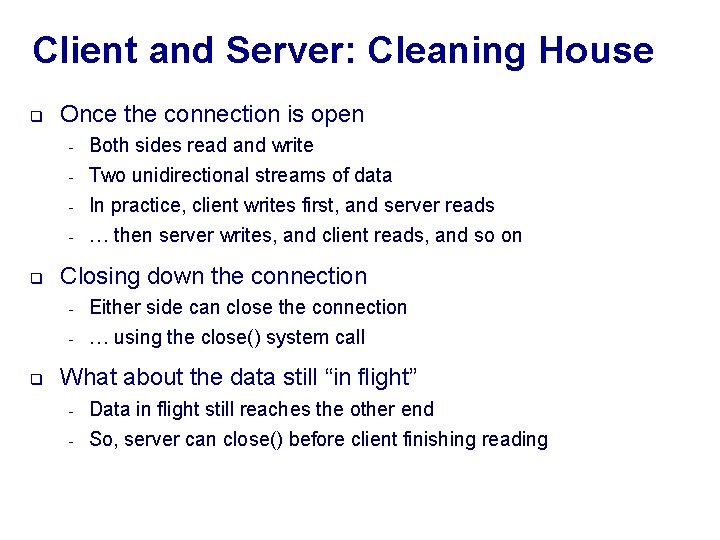
Client and Server: Cleaning House q q Once the connection is open - Both sides read and write - Two unidirectional streams of data In practice, client writes first, and server reads - … then server writes, and client reads, and so on Closing down the connection - q Either side can close the connection … using the close() system call What about the data still “in flight” - Data in flight still reaches the other end So, server can close() before client finishing reading
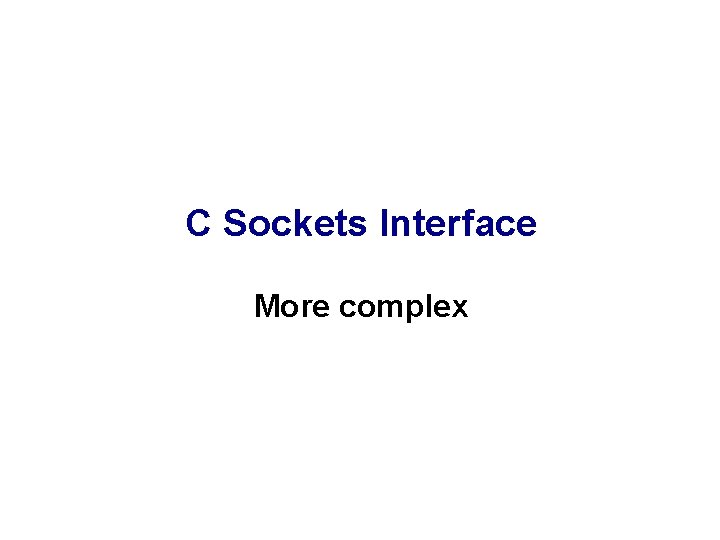
C Sockets Interface More complex
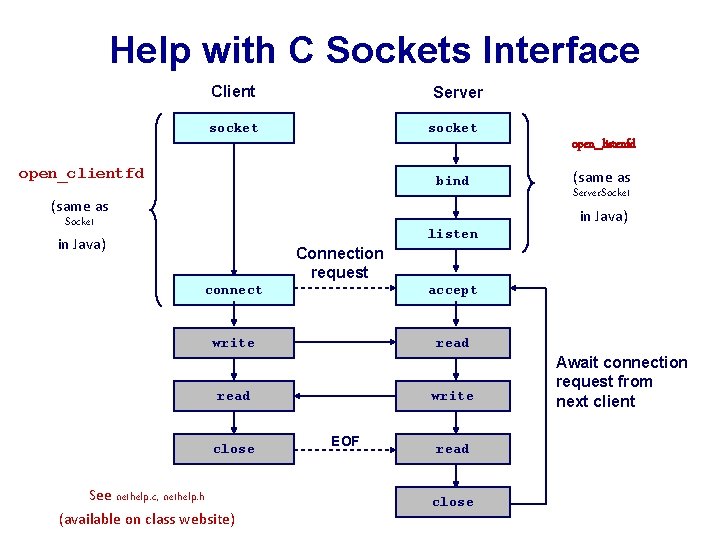
Help with C Sockets Interface Client Server socket open_listenfd open_clientfd bind (same as Socket (same as Server. Socket in Java) listen in Java) Connection request connect accept write read close See nethelp. c, nethelp. h (available on class website) write EOF read close Await connection request from next client
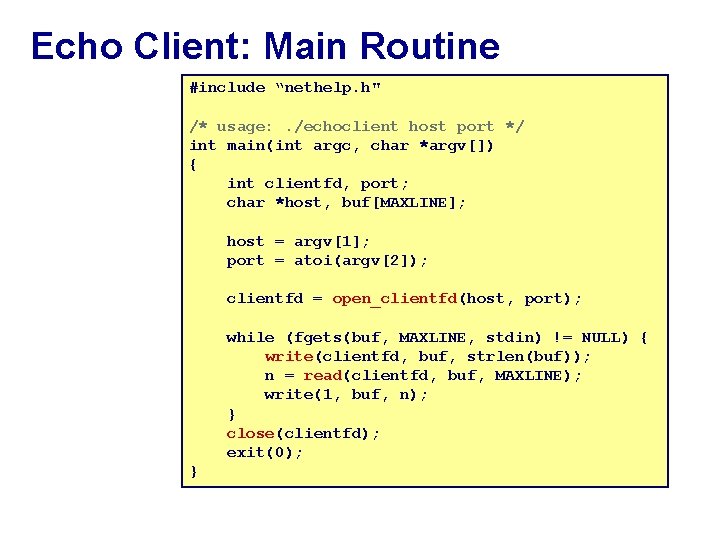
Echo Client: Main Routine #include “nethelp. h" /* usage: . /echoclient host port */ int main(int argc, char *argv[]) { int clientfd, port; char *host, buf[MAXLINE]; host = argv[1]; port = atoi(argv[2]); clientfd = open_clientfd(host, port); while (fgets(buf, MAXLINE, stdin) != NULL) { write(clientfd, buf, strlen(buf)); n = read(clientfd, buf, MAXLINE); write(1, buf, n); } close(clientfd); exit(0); }
![Multithreaded Echo Server Main Routine int mainint argc char argv int listenfd connfd Multi-threaded Echo Server: Main Routine int main(int argc, char *argv[]) { int listenfd, connfd,](https://slidetodoc.com/presentation_image_h/0462c69286f8a1a90cf193c794f76d14/image-21.jpg)
Multi-threaded Echo Server: Main Routine int main(int argc, char *argv[]) { int listenfd, connfd, port, clientlen, tid; struct sockaddr clientaddr; port = atoi(argv[1]); /* the server listens on a port passed on the command line */ listenfd = open_listenfd(port); clientlen = sizeof(clientaddr); while (1) { connfd = accept(listenfd, &clientaddr, &clientlen); pthread_create(&tid, NULL, Handle. Client, (void*)connfd); } } Must be implemented The server loops endlessly, waiting for connection request from client, then starting a new thread to handle the client request.
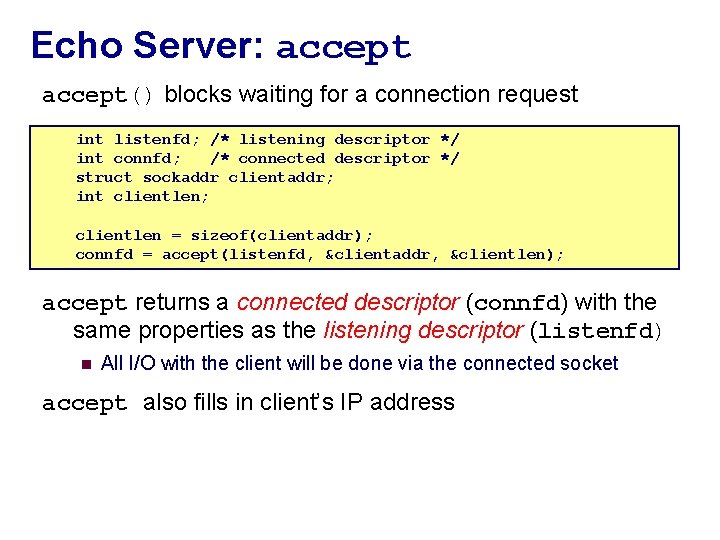
Echo Server: accept() blocks waiting for a connection request int listenfd; /* listening descriptor */ int connfd; /* connected descriptor */ struct sockaddr clientaddr; int clientlen; clientlen = sizeof(clientaddr); connfd = accept(listenfd, &clientaddr, &clientlen); accept returns a connected descriptor (connfd) with the same properties as the listening descriptor (listenfd) n All I/O with the client will be done via the connected socket accept also fills in client’s IP address
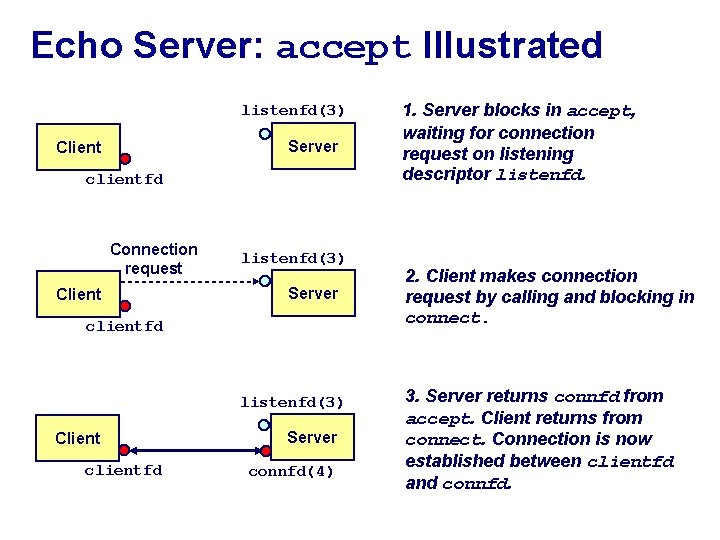
Echo Server: accept Illustrated listenfd(3) Server Client clientfd Connection request Client listenfd(3) Server clientfd listenfd(3) Client clientfd Server connfd(4) 1. Server blocks in accept, waiting for connection request on listening descriptor listenfd. 2. Client makes connection request by calling and blocking in connect. 3. Server returns connfd from accept. Client returns from connect. Connection is now established between clientfd and connfd.
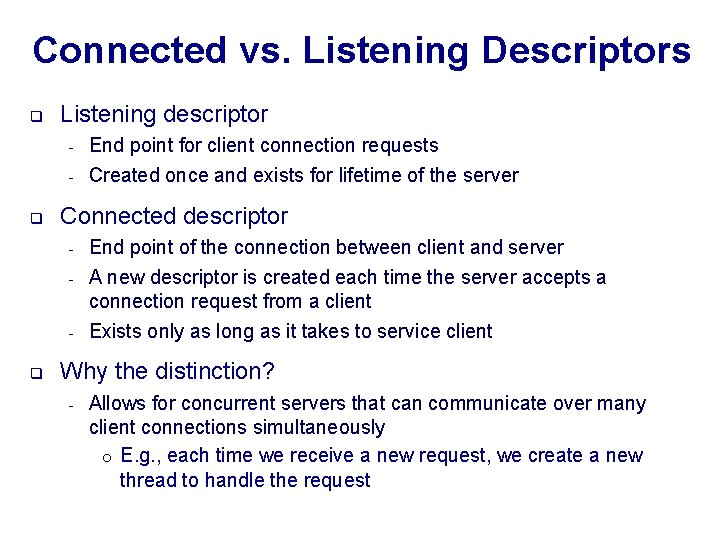
Connected vs. Listening Descriptors q q Listening descriptor - End point for client connection requests - Created once and exists for lifetime of the server Connected descriptor - q End point of the connection between client and server A new descriptor is created each time the server accepts a connection request from a client Exists only as long as it takes to service client Why the distinction? - Allows for concurrent servers that can communicate over many client connections simultaneously o E. g. , each time we receive a new request, we create a new thread to handle the request
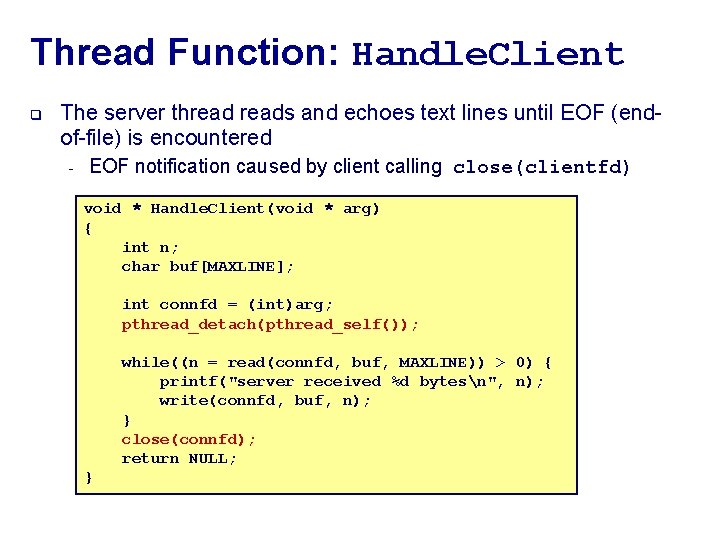
Thread Function: Handle. Client q The server threads and echoes text lines until EOF (endof-file) is encountered - EOF notification caused by client calling close(clientfd) void * Handle. Client(void * arg) { int n; char buf[MAXLINE]; int connfd = (int)arg; pthread_detach(pthread_self()); while((n = read(connfd, buf, MAXLINE)) > 0) { printf("server received %d bytesn", n); write(connfd, buf, n); } close(connfd); return NULL; }
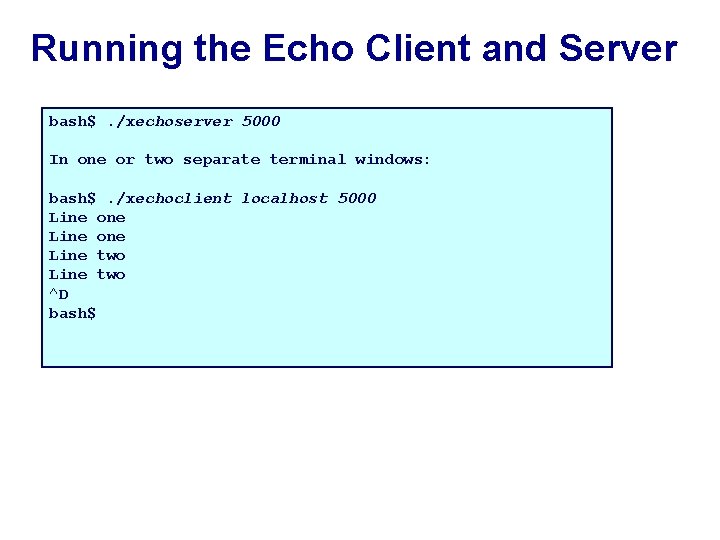
Running the Echo Client and Server bash$. /xechoserver 5000 In one or two separate terminal windows: bash$. /xechoclient localhost 5000 Line one Line two ^D bash$
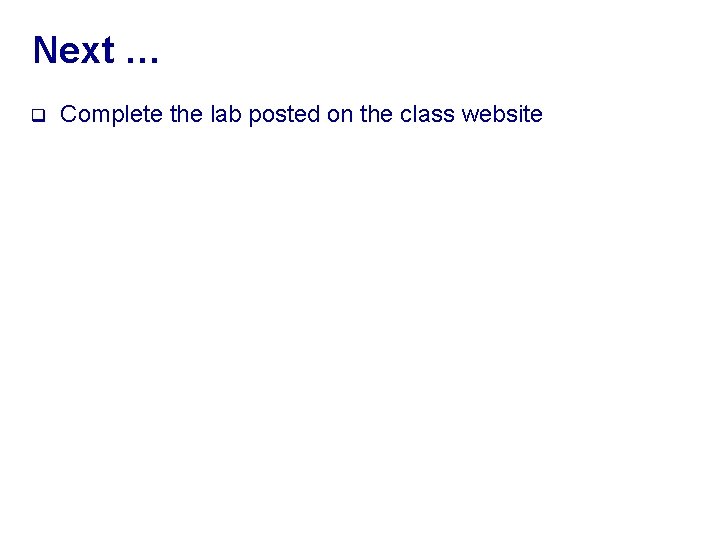
Next … q Complete the lab posted on the class website