More on Functions Intro to Computer Science CS
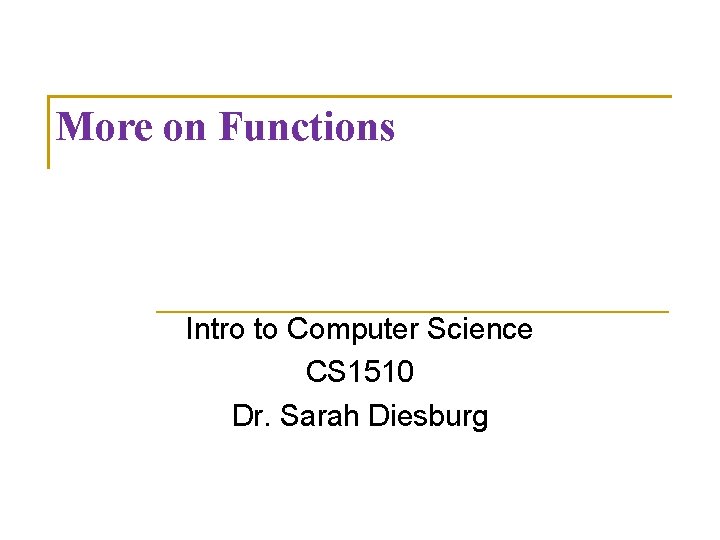
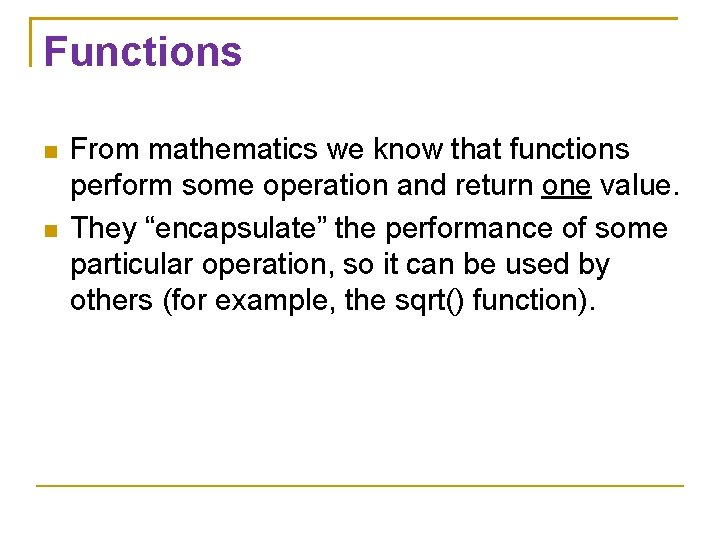
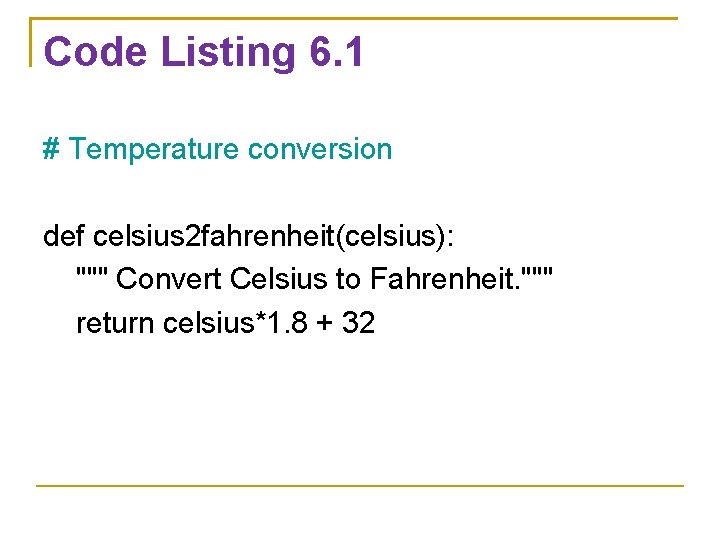
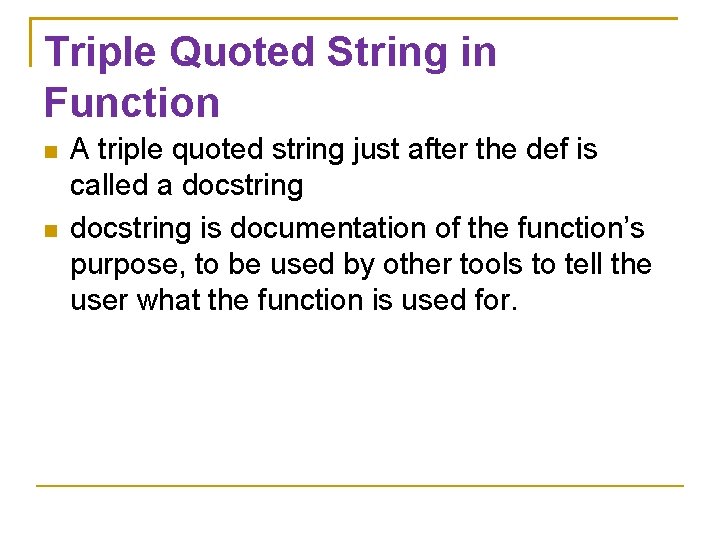
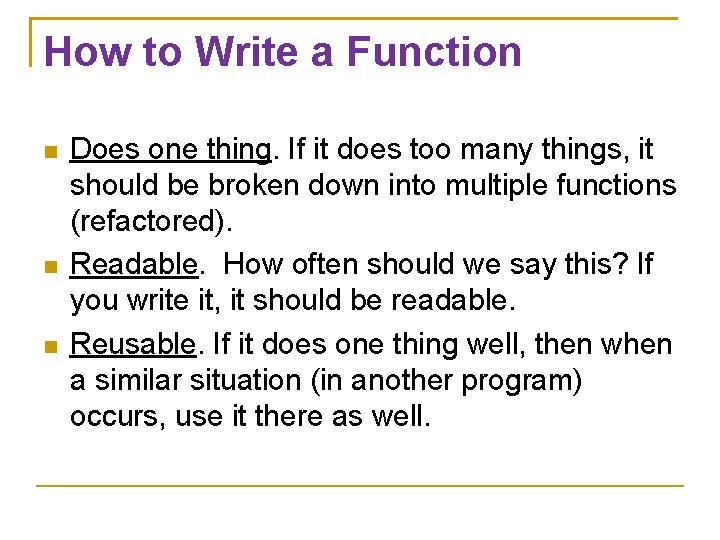
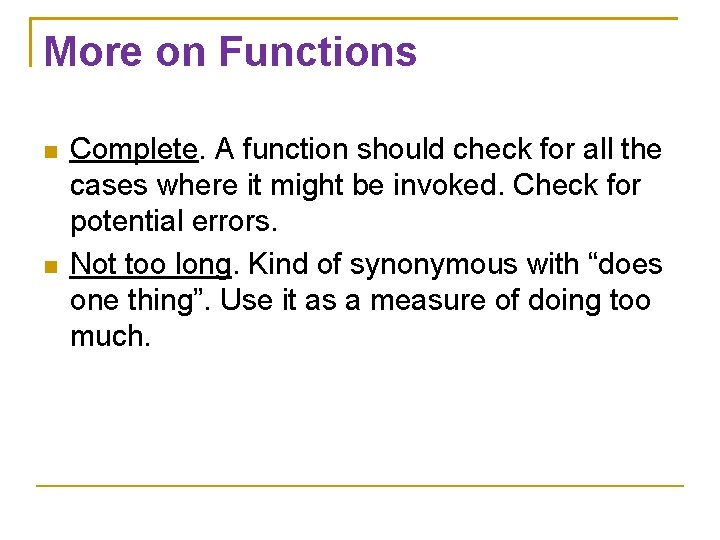
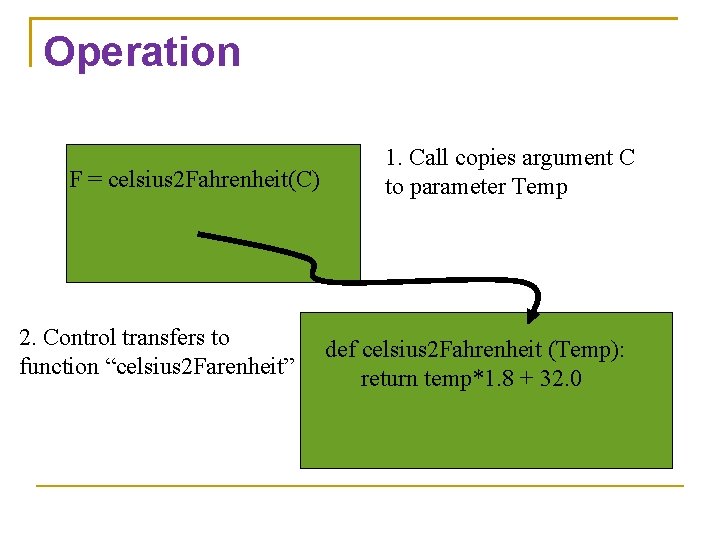
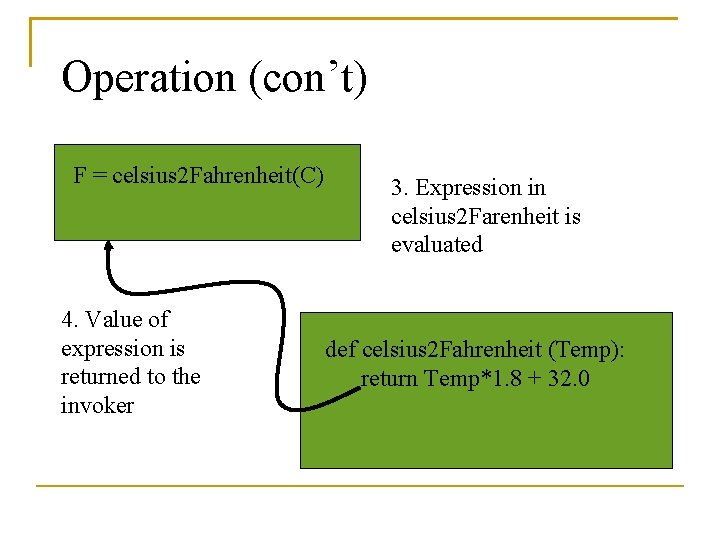
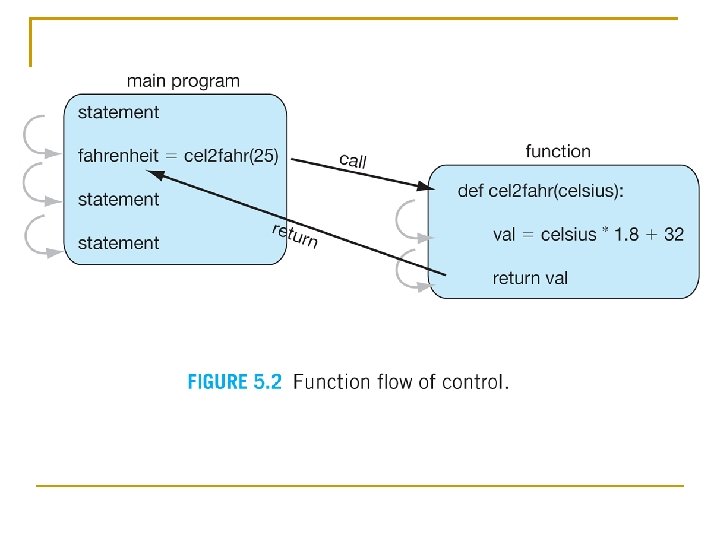
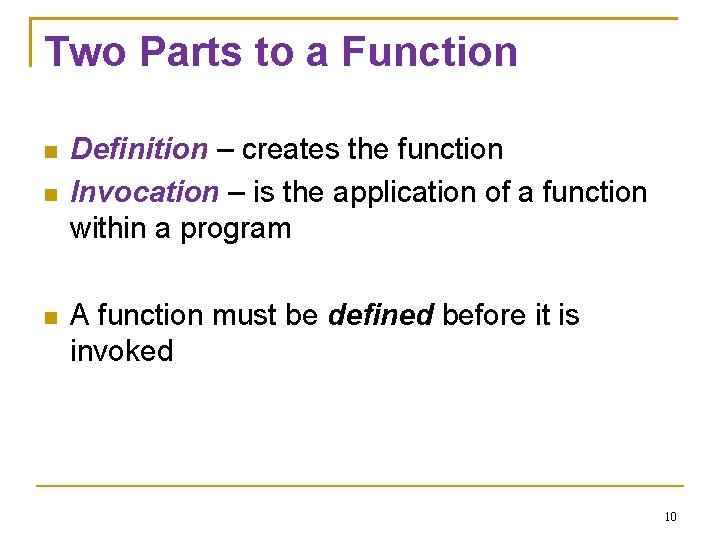
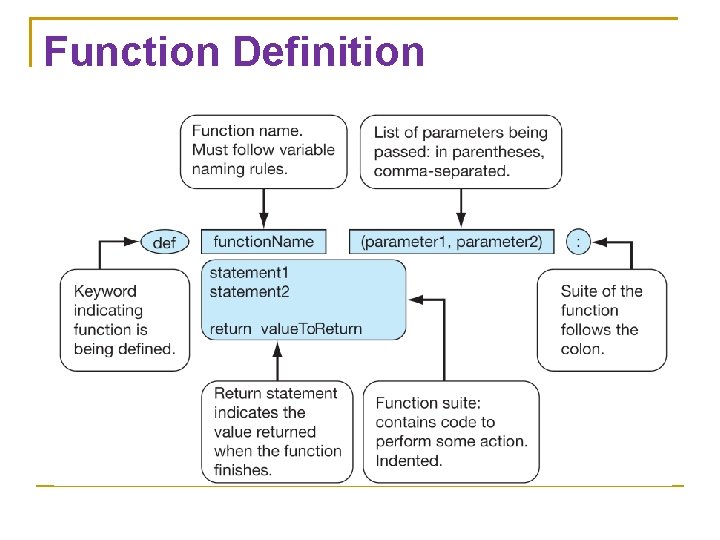
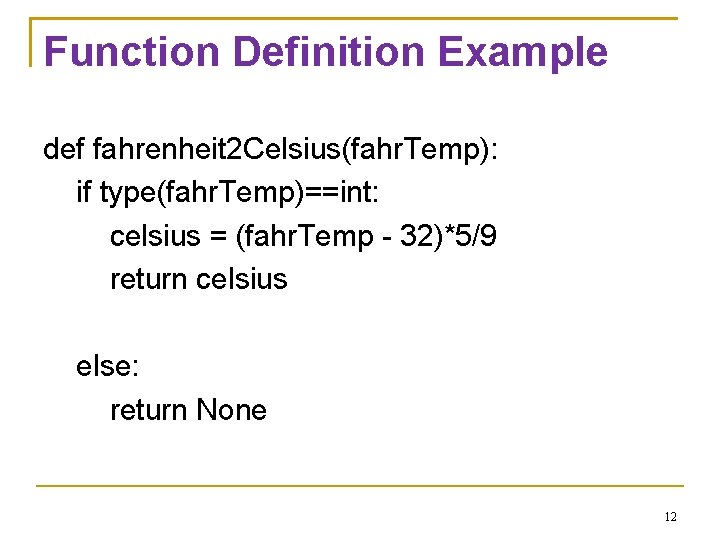
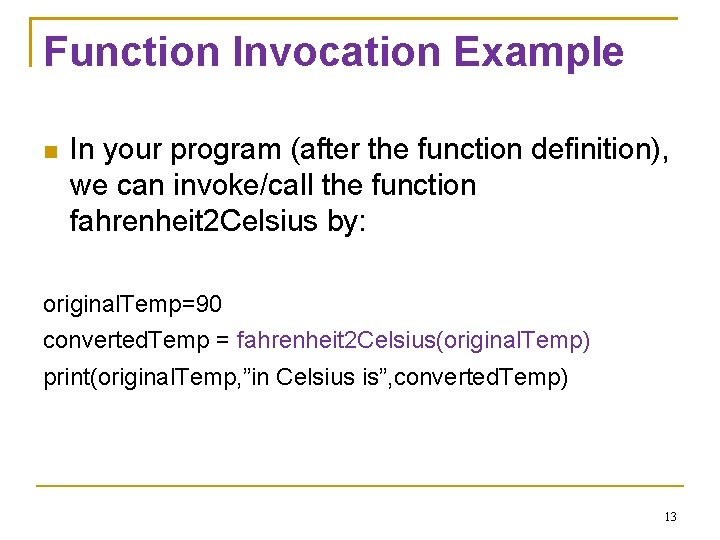
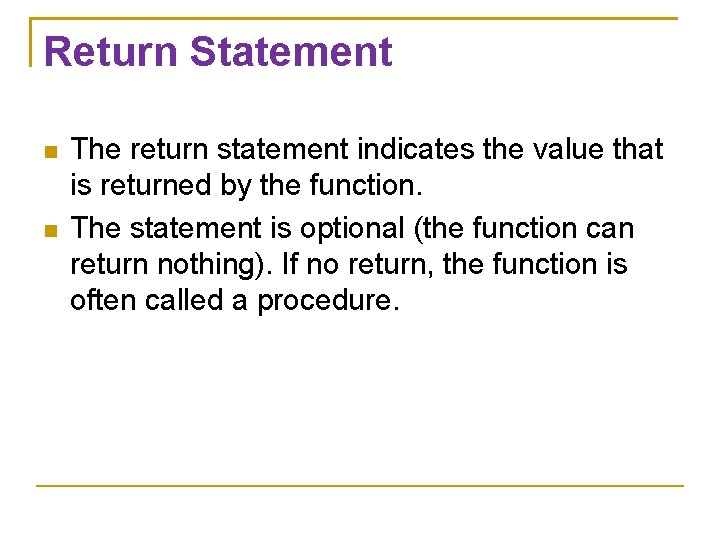
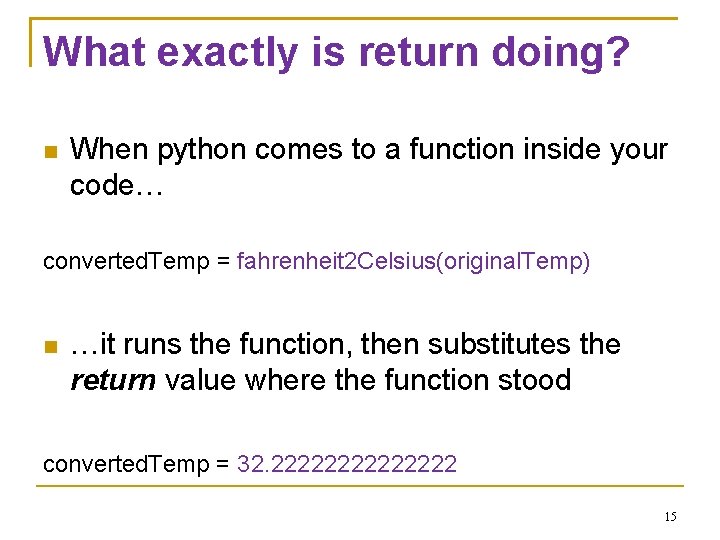
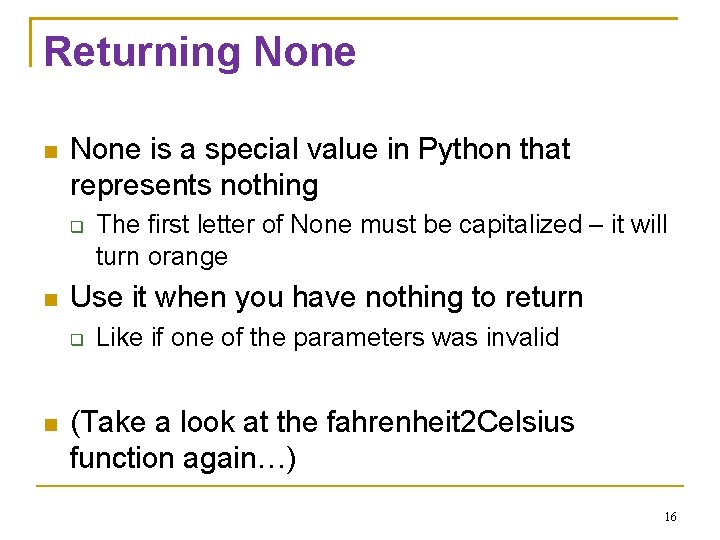
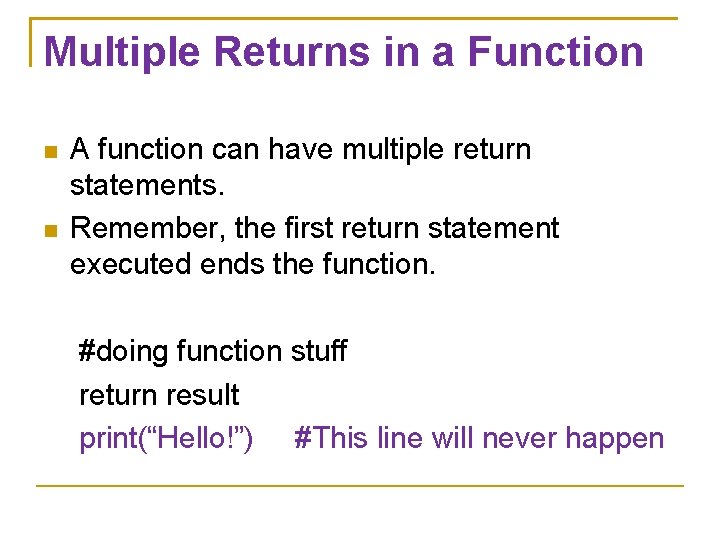
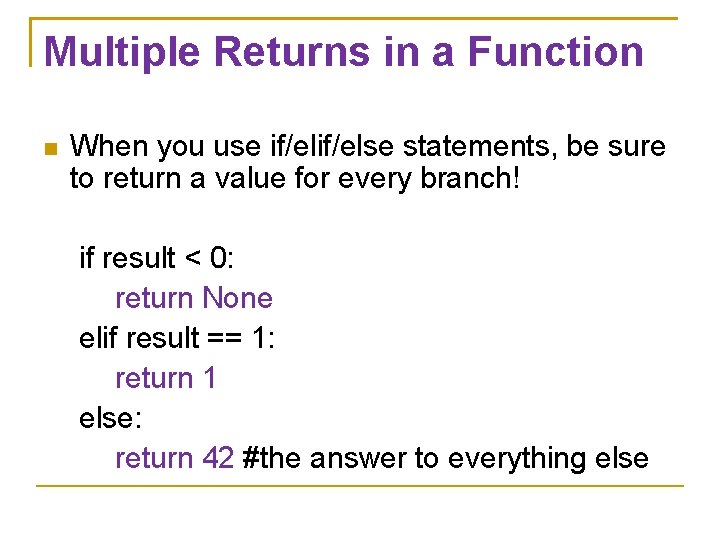
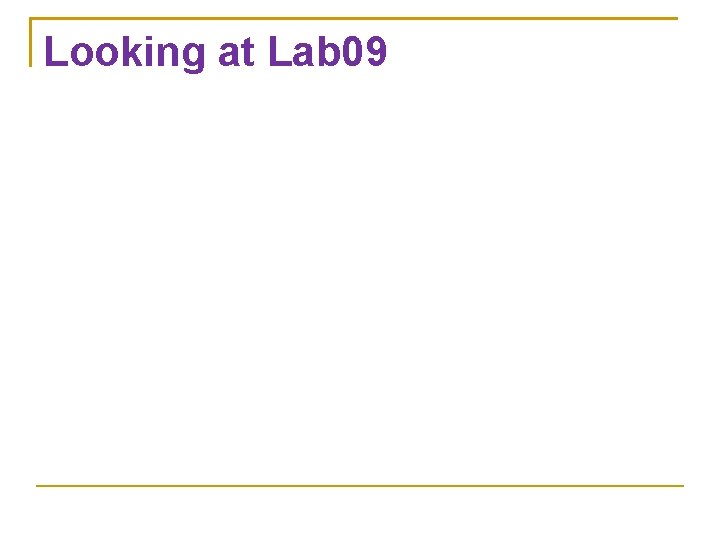
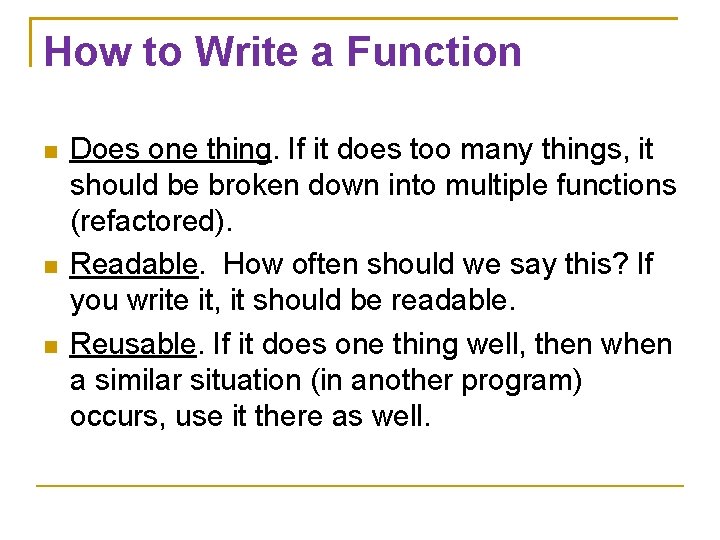
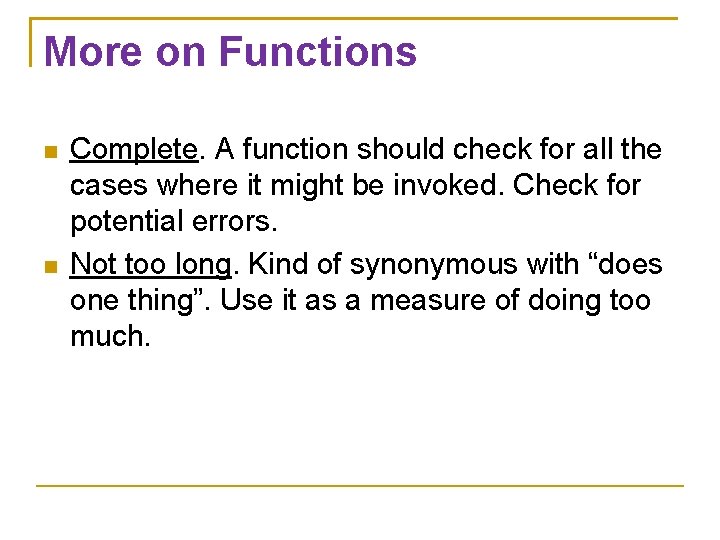
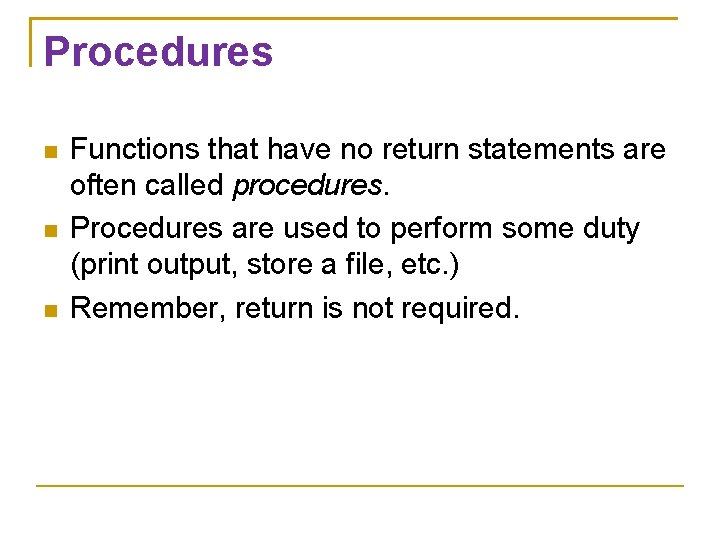
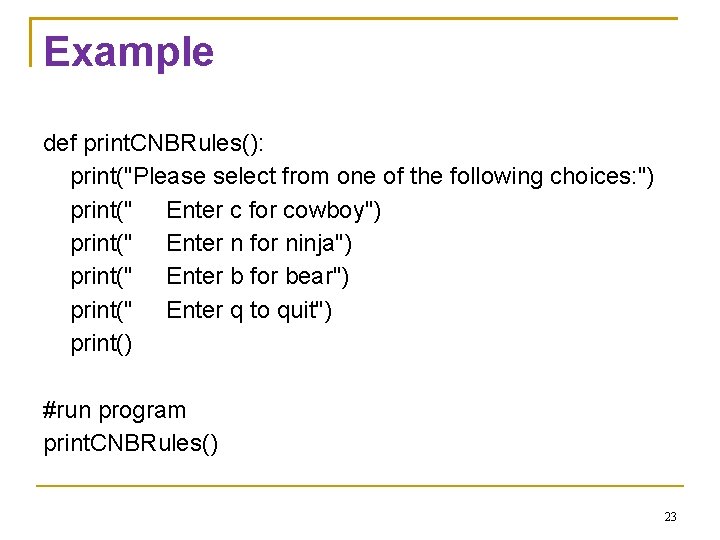
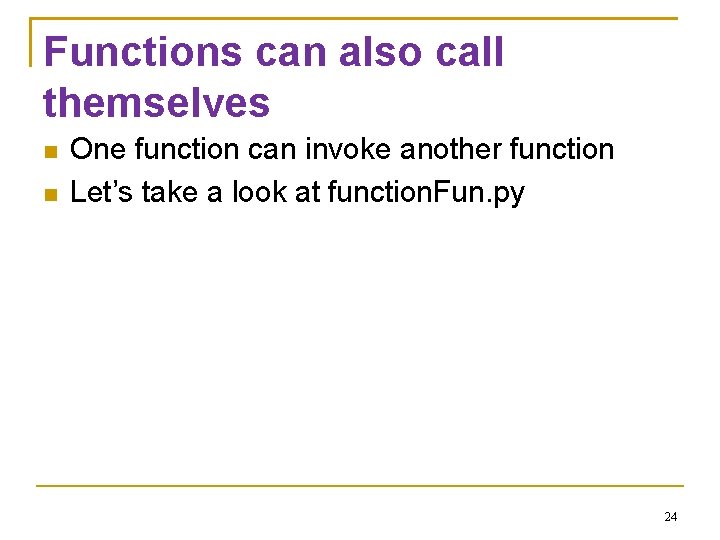
- Slides: 24
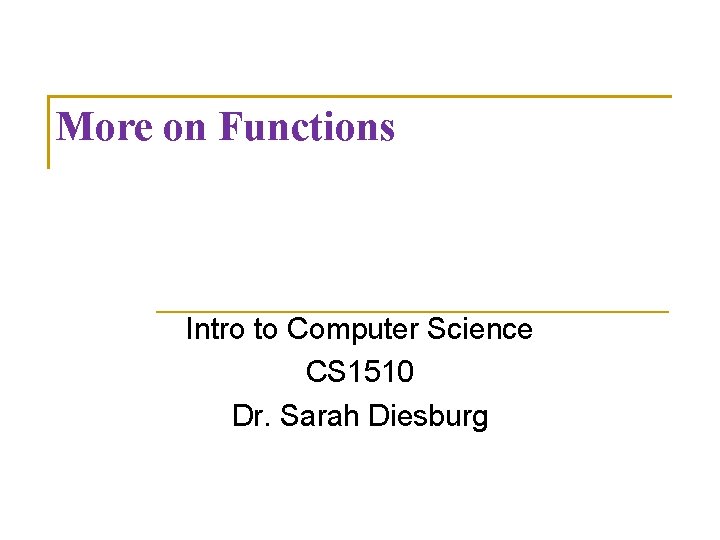
More on Functions Intro to Computer Science CS 1510 Dr. Sarah Diesburg
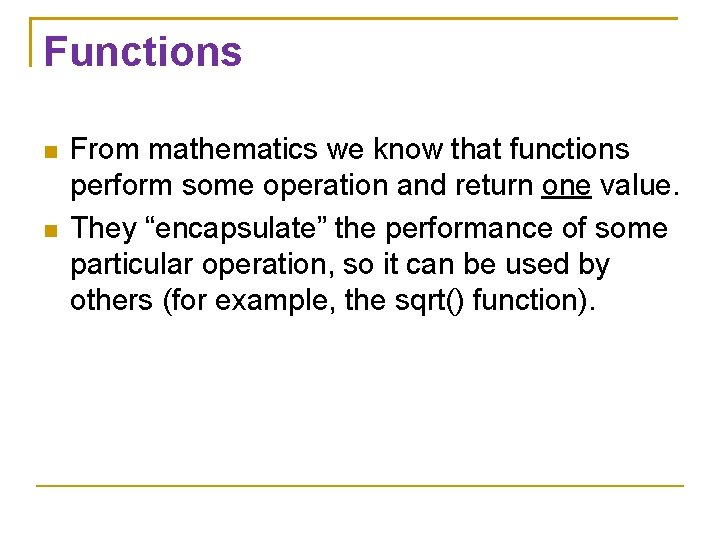
Functions From mathematics we know that functions perform some operation and return one value. They “encapsulate” the performance of some particular operation, so it can be used by others (for example, the sqrt() function).
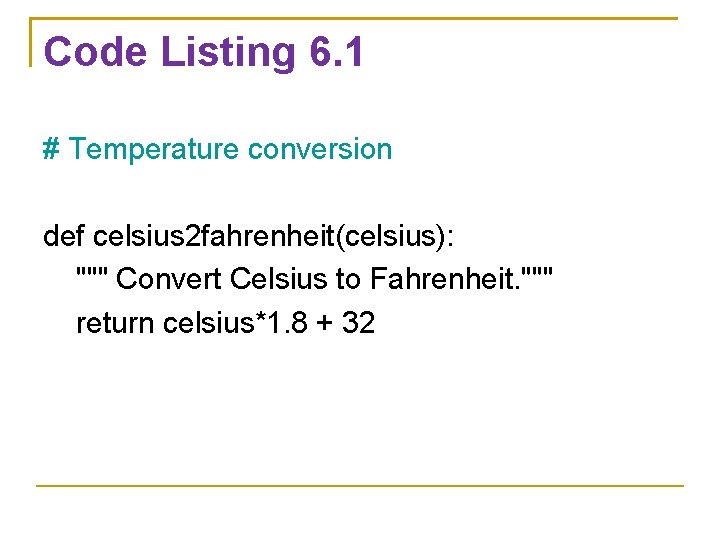
Code Listing 6. 1 # Temperature conversion def celsius 2 fahrenheit(celsius): """ Convert Celsius to Fahrenheit. """ return celsius*1. 8 + 32
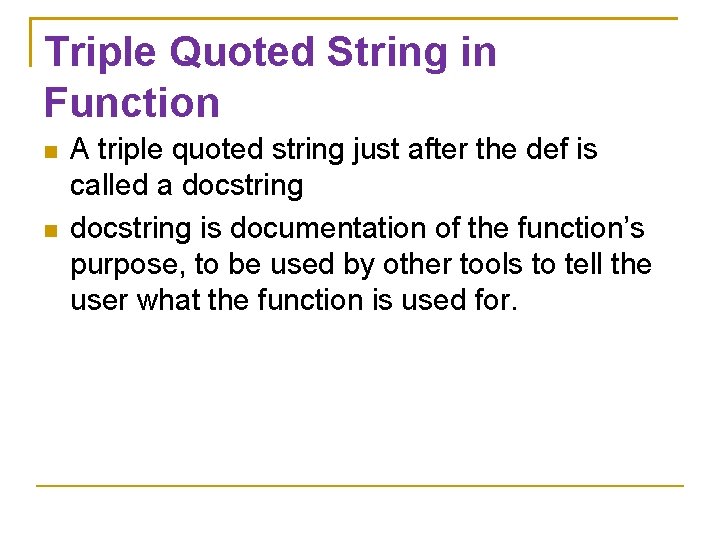
Triple Quoted String in Function A triple quoted string just after the def is called a docstring is documentation of the function’s purpose, to be used by other tools to tell the user what the function is used for.
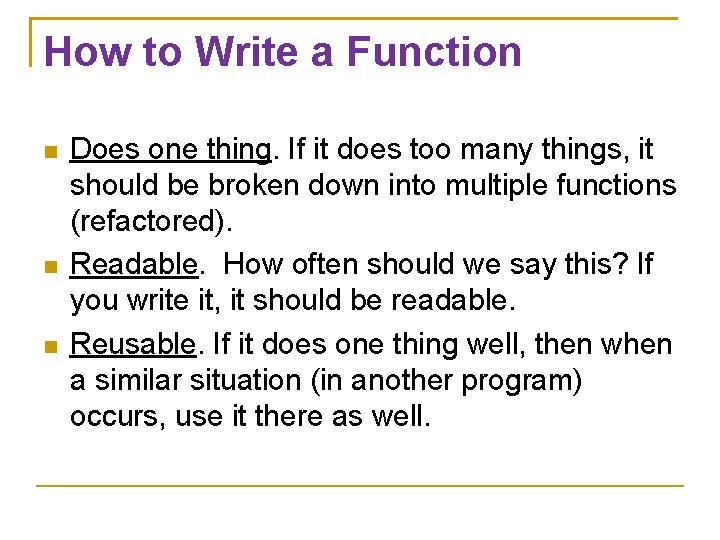
How to Write a Function Does one thing. If it does too many things, it should be broken down into multiple functions (refactored). Readable. How often should we say this? If you write it, it should be readable. Reusable. If it does one thing well, then when a similar situation (in another program) occurs, use it there as well.
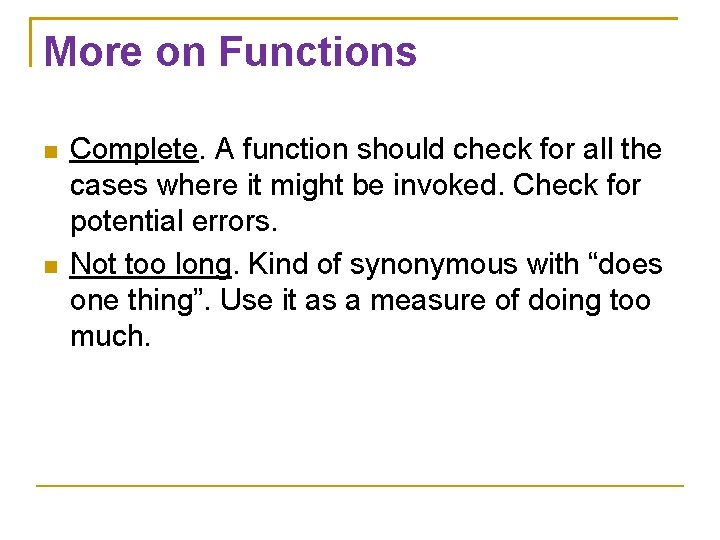
More on Functions Complete. A function should check for all the cases where it might be invoked. Check for potential errors. Not too long. Kind of synonymous with “does one thing”. Use it as a measure of doing too much.
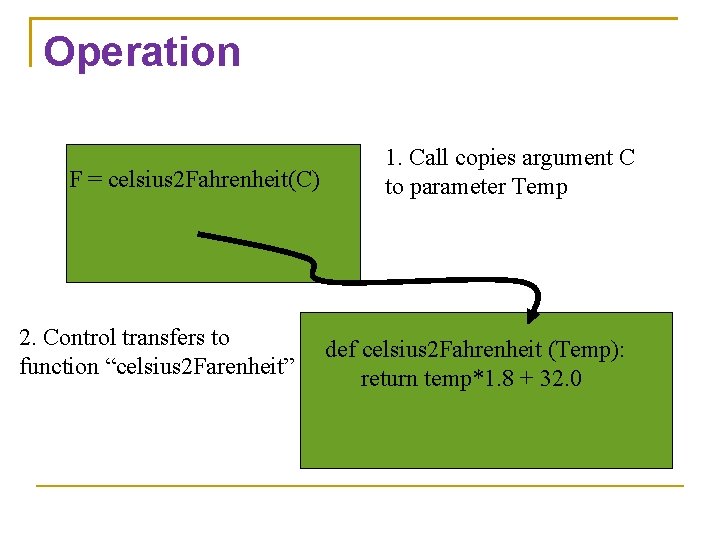
Operation F = celsius 2 Fahrenheit(C) 2. Control transfers to function “celsius 2 Farenheit” 1. Call copies argument C to parameter Temp def celsius 2 Fahrenheit (Temp): return temp*1. 8 + 32. 0
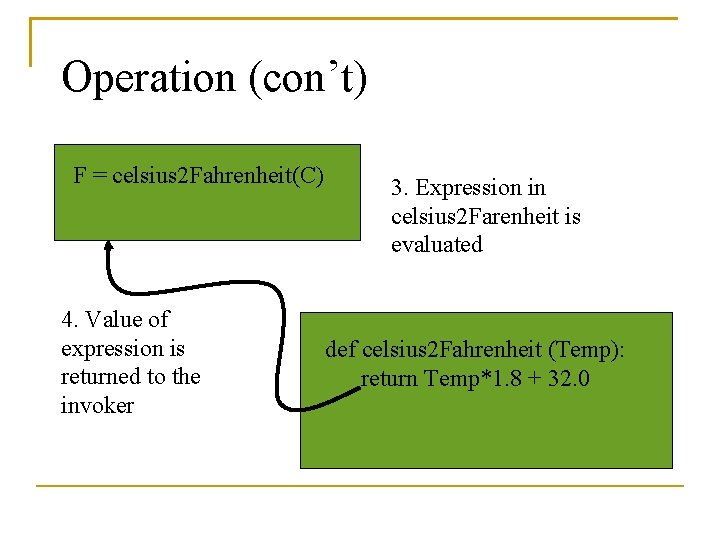
Operation (con’t) F = celsius 2 Fahrenheit(C) 4. Value of expression is returned to the invoker 3. Expression in celsius 2 Farenheit is evaluated def celsius 2 Fahrenheit (Temp): return Temp*1. 8 + 32. 0
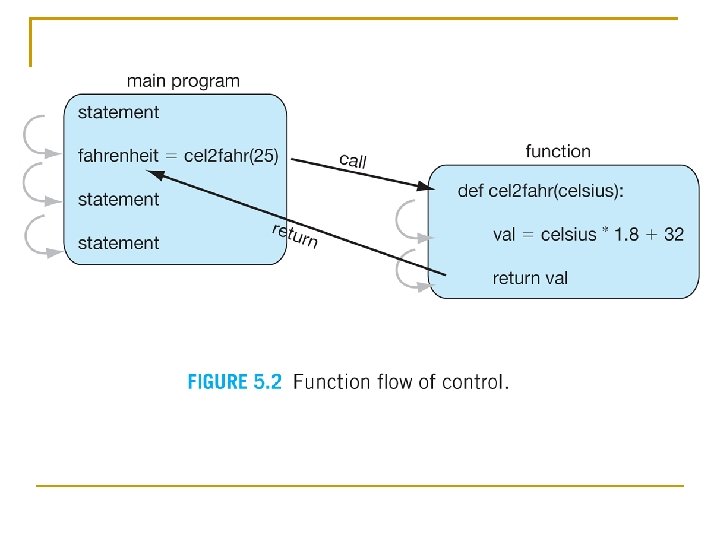
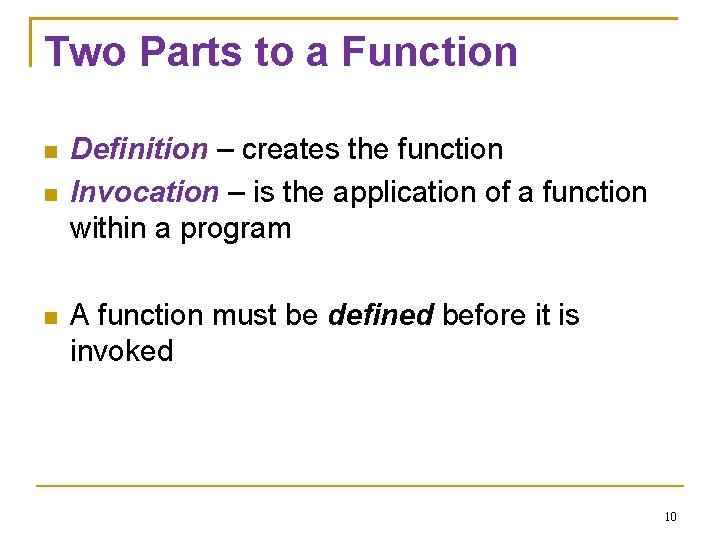
Two Parts to a Function Definition – creates the function Invocation – is the application of a function within a program A function must be defined before it is invoked 10
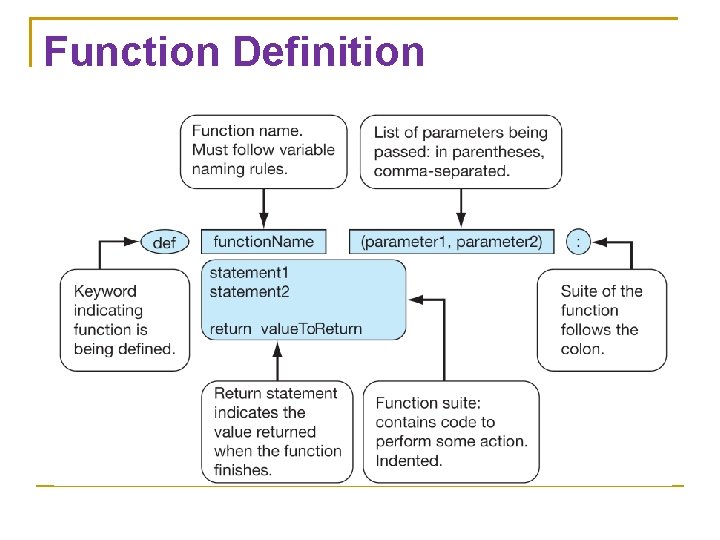
Function Definition
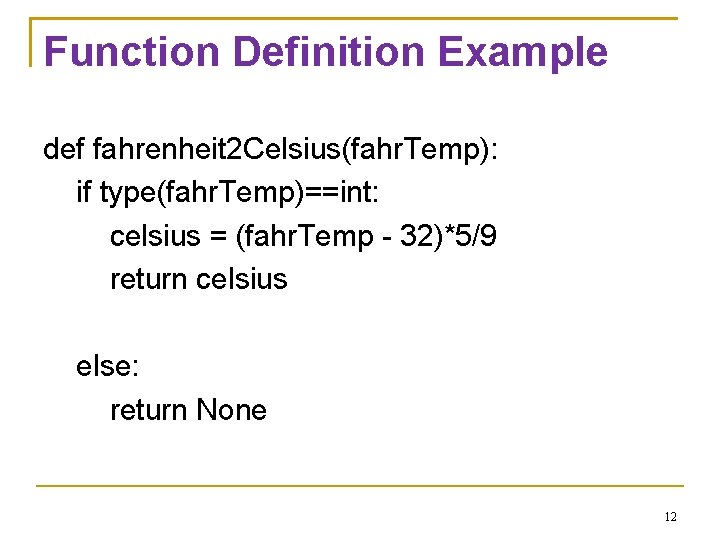
Function Definition Example def fahrenheit 2 Celsius(fahr. Temp): if type(fahr. Temp)==int: celsius = (fahr. Temp - 32)*5/9 return celsius else: return None 12
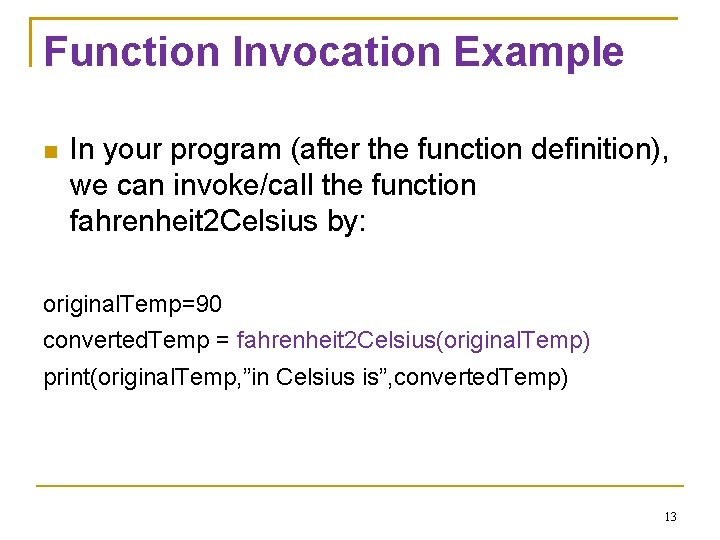
Function Invocation Example In your program (after the function definition), we can invoke/call the function fahrenheit 2 Celsius by: original. Temp=90 converted. Temp = fahrenheit 2 Celsius(original. Temp) print(original. Temp, ”in Celsius is”, converted. Temp) 13
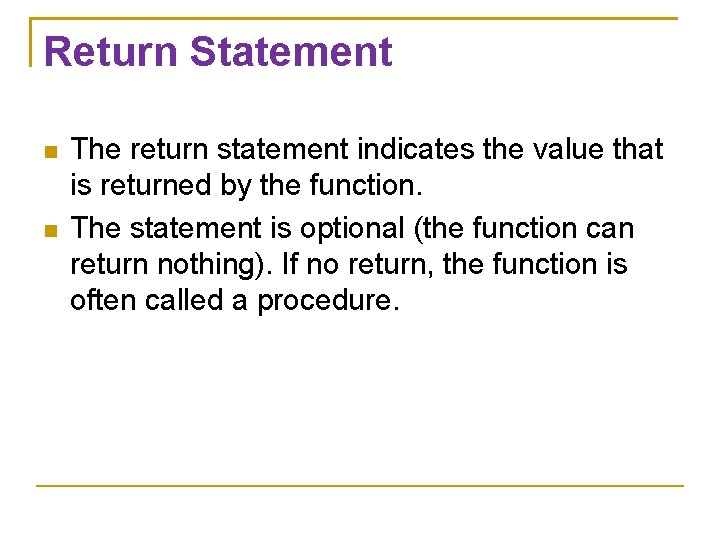
Return Statement The return statement indicates the value that is returned by the function. The statement is optional (the function can return nothing). If no return, the function is often called a procedure.
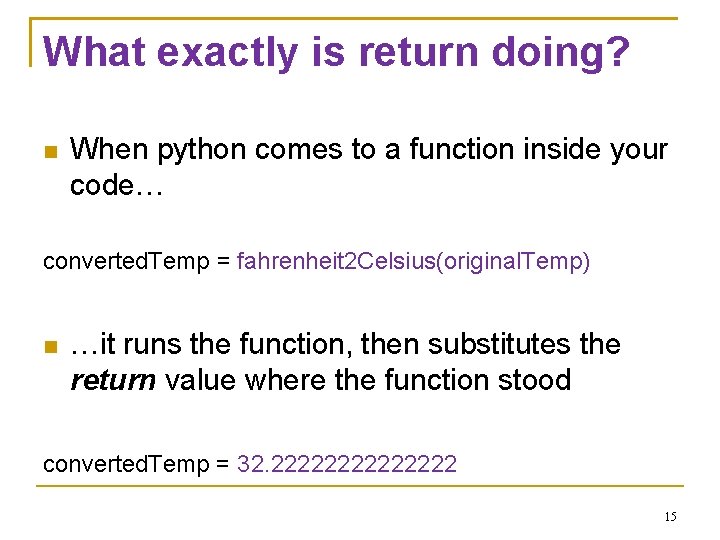
What exactly is return doing? When python comes to a function inside your code… converted. Temp = fahrenheit 2 Celsius(original. Temp) …it runs the function, then substitutes the return value where the function stood converted. Temp = 32. 2222222 15
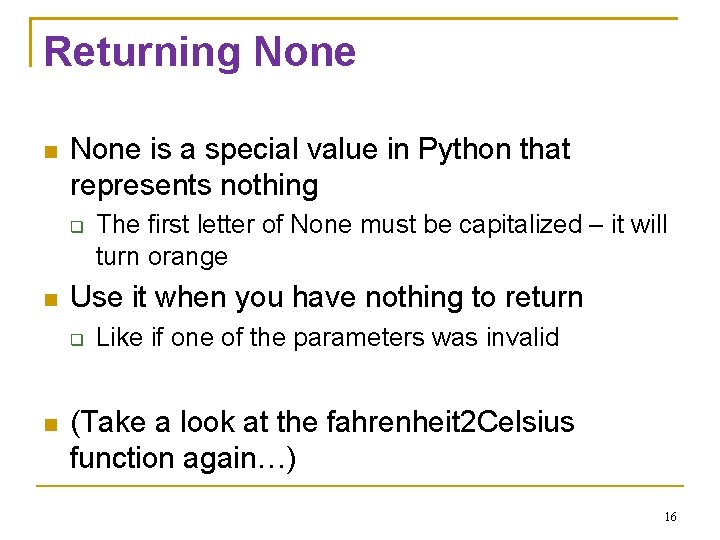
Returning None is a special value in Python that represents nothing Use it when you have nothing to return The first letter of None must be capitalized – it will turn orange Like if one of the parameters was invalid (Take a look at the fahrenheit 2 Celsius function again…) 16
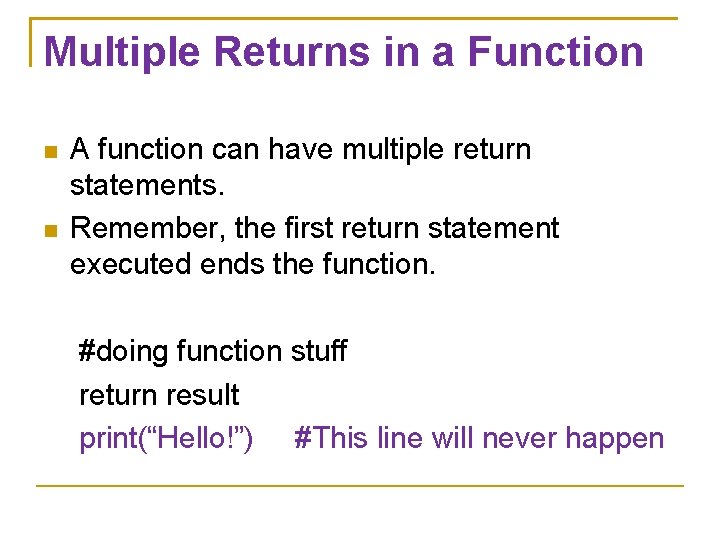
Multiple Returns in a Function A function can have multiple return statements. Remember, the first return statement executed ends the function. #doing function stuff return result print(“Hello!”) #This line will never happen
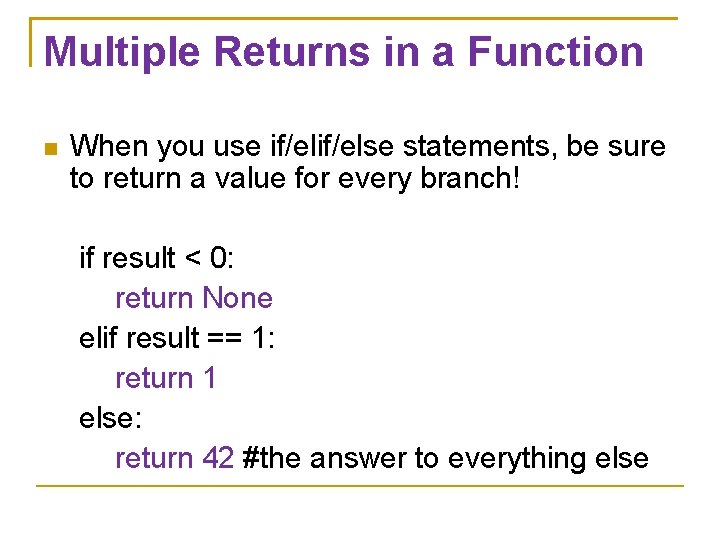
Multiple Returns in a Function When you use if/else statements, be sure to return a value for every branch! if result < 0: return None elif result == 1: return 1 else: return 42 #the answer to everything else
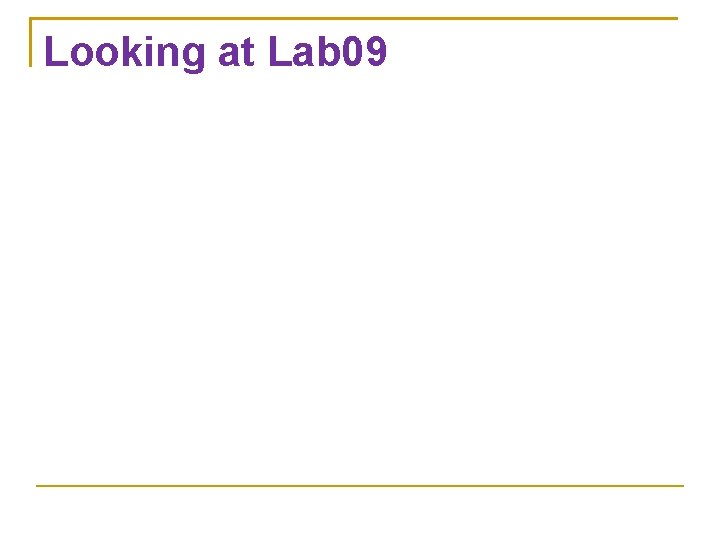
Looking at Lab 09
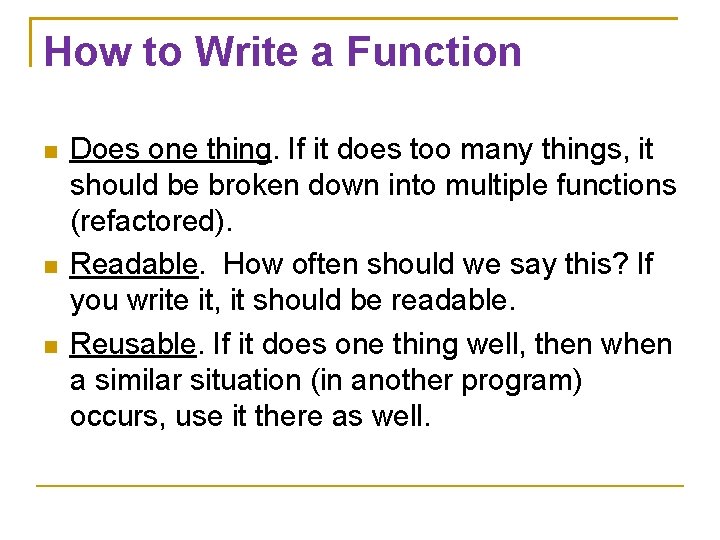
How to Write a Function Does one thing. If it does too many things, it should be broken down into multiple functions (refactored). Readable. How often should we say this? If you write it, it should be readable. Reusable. If it does one thing well, then when a similar situation (in another program) occurs, use it there as well.
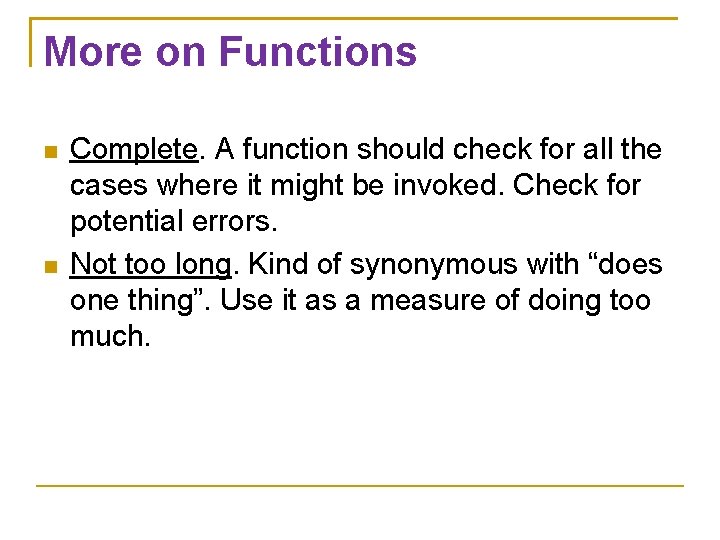
More on Functions Complete. A function should check for all the cases where it might be invoked. Check for potential errors. Not too long. Kind of synonymous with “does one thing”. Use it as a measure of doing too much.
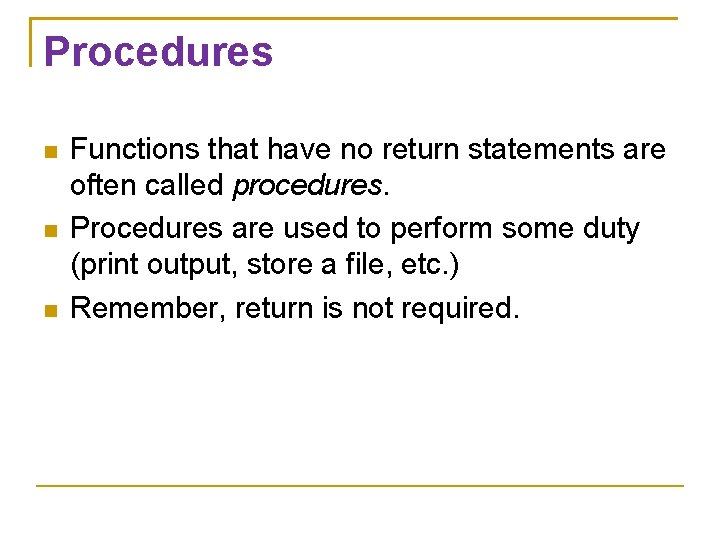
Procedures Functions that have no return statements are often called procedures. Procedures are used to perform some duty (print output, store a file, etc. ) Remember, return is not required.
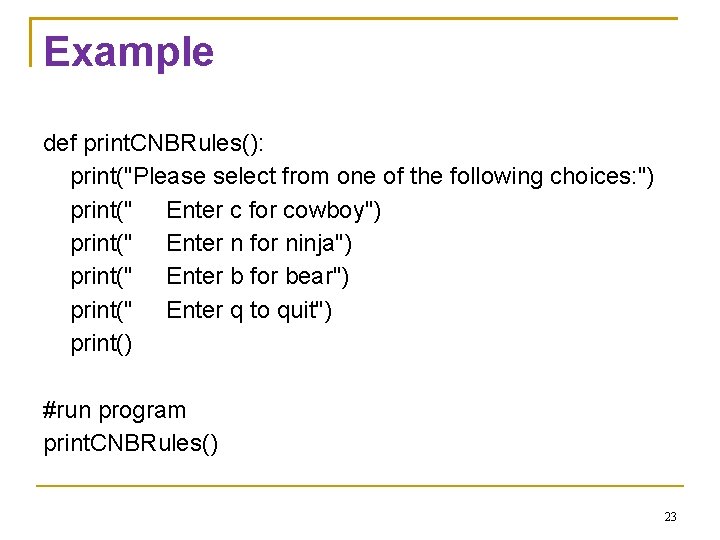
Example def print. CNBRules(): print("Please select from one of the following choices: ") print(" Enter c for cowboy") print(" Enter n for ninja") print(" Enter b for bear") print(" Enter q to quit") print() #run program print. CNBRules() 23
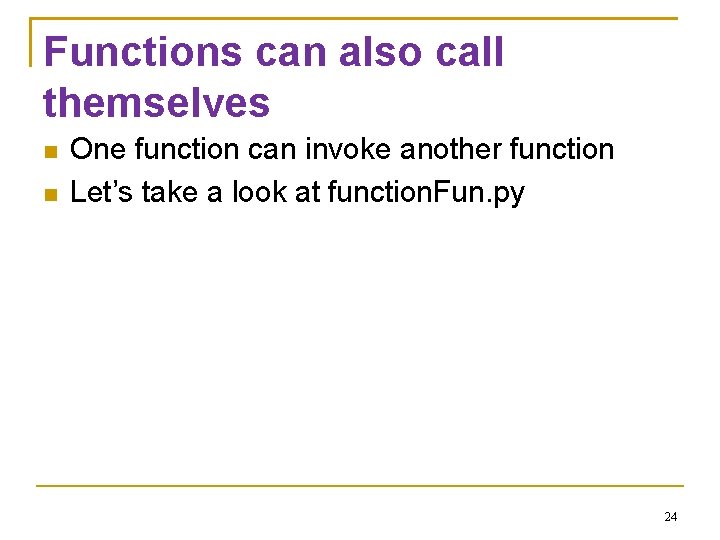
Functions can also call themselves One function can invoke another function Let’s take a look at function. Fun. py 24
More more more i want more more more more we praise you
More more more i want more more more more we praise you
My favorite subject is p.e
Introduction to piecewise functions
Chapter 1 introduction to forensic science and the law
The more you take the more you leave behind
The more you study the more you learn
Aspire not to
Examples of newton's first law
Knowing more remembering more
The more i give to thee the more i have
More choices more chances
Human history becomes more and more a race
Piecewise functions absolute value
How to evaluate function
Evaluating functions and operations on functions
Social science vs natural science
3 branches of science
Natural science vs physical science
Applied science vs pure science
Natural science and social science similarities
Science fusion online
Rule of 70 population growth
Julie lundquist
Soft science definition