Methods Why methods n A method gives a
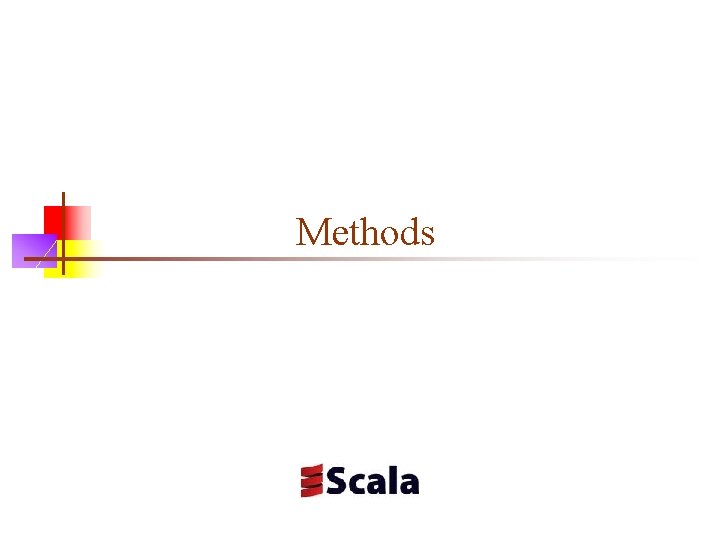
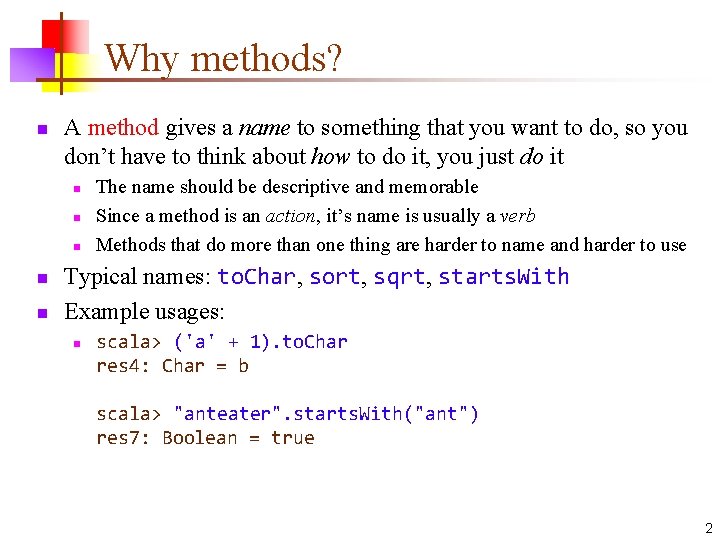
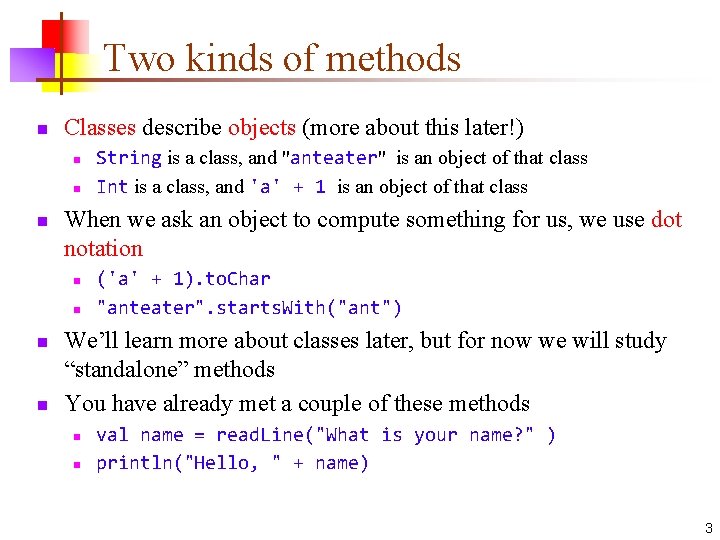
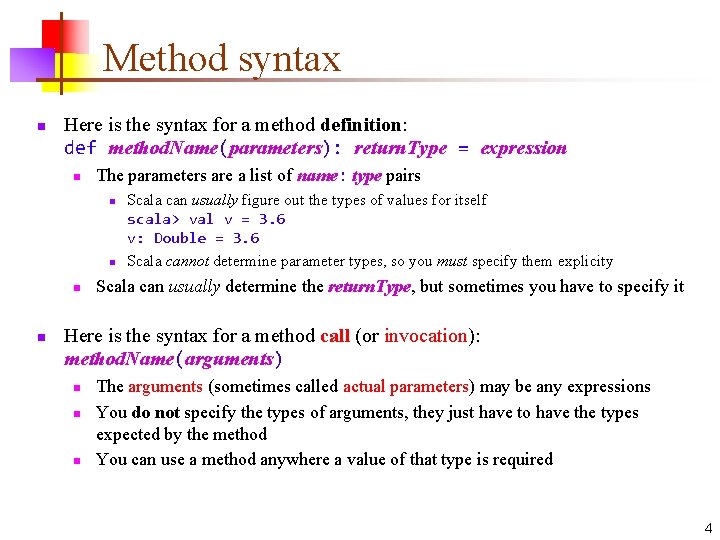
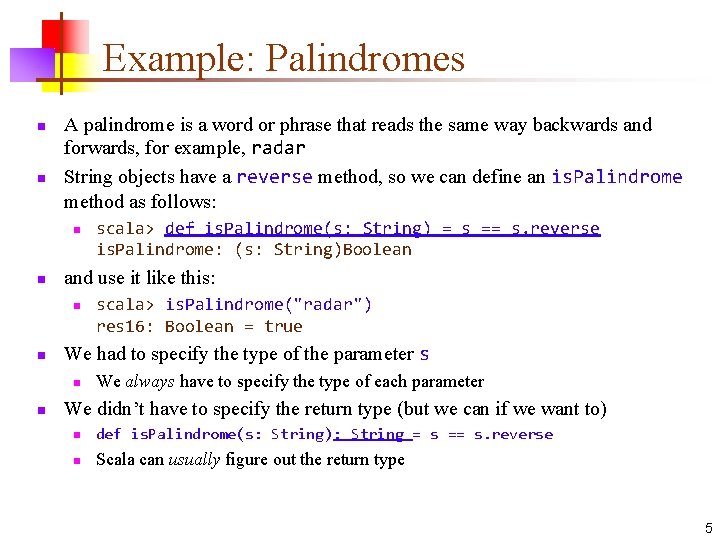
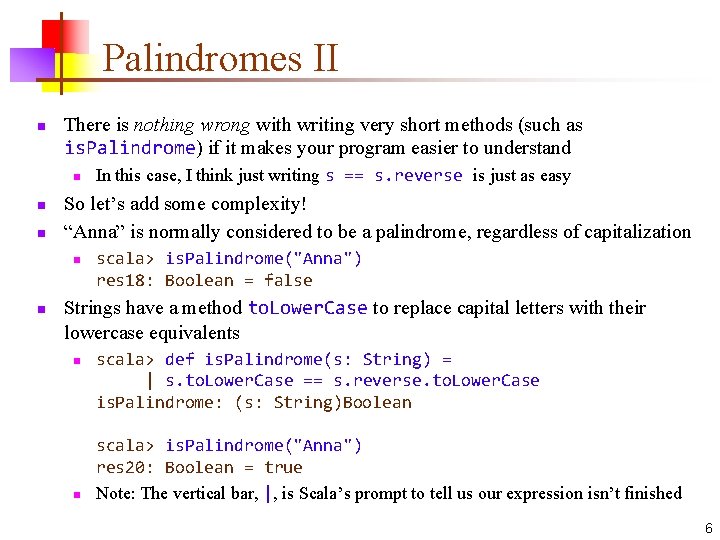
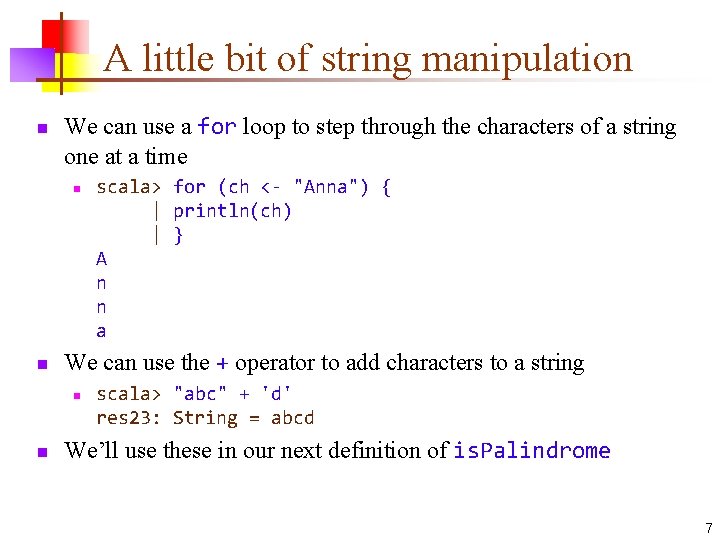
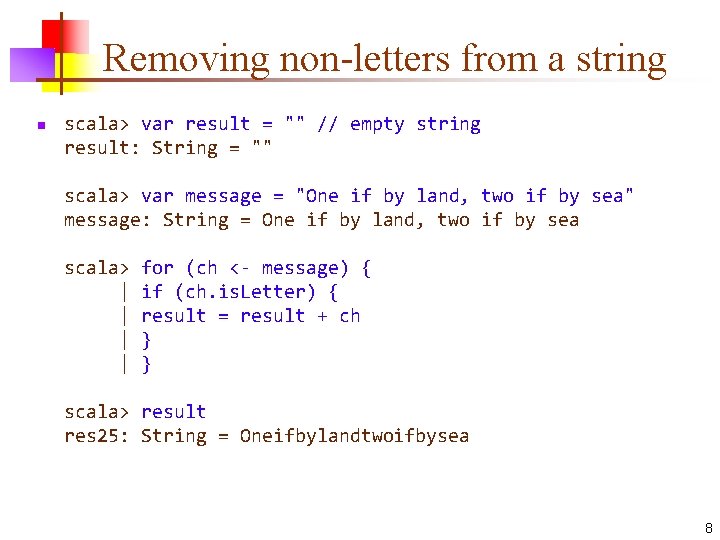
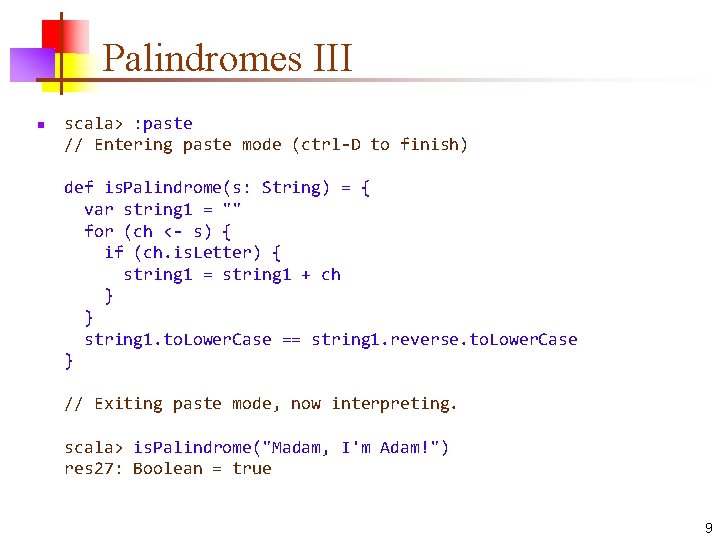
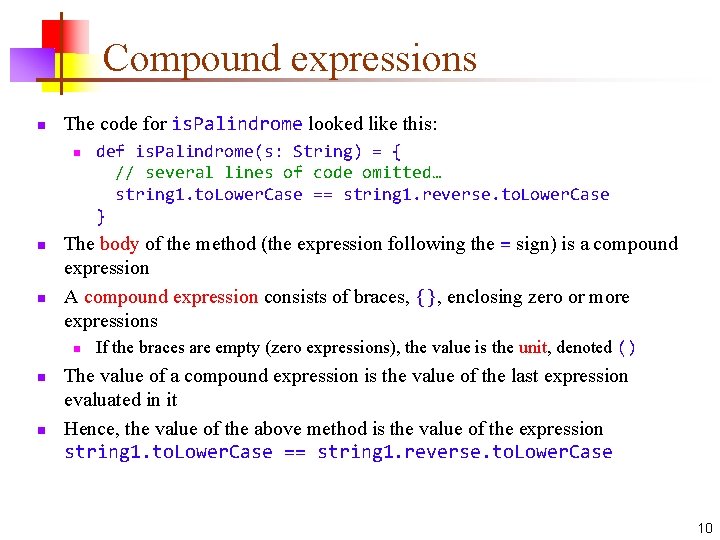
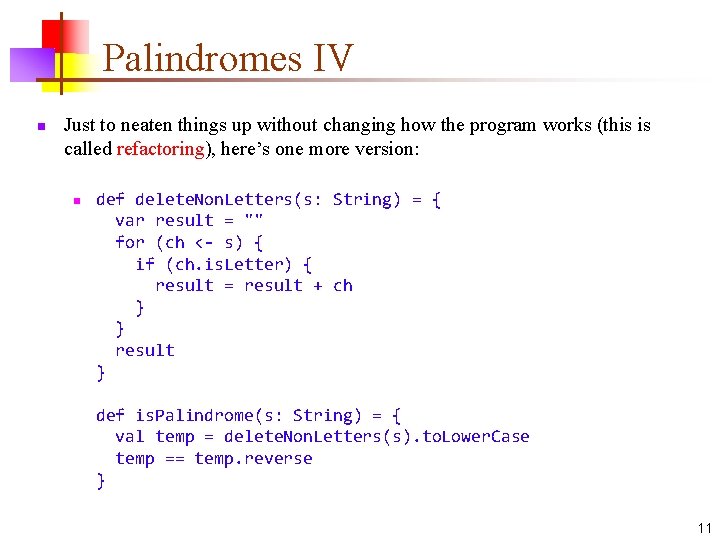
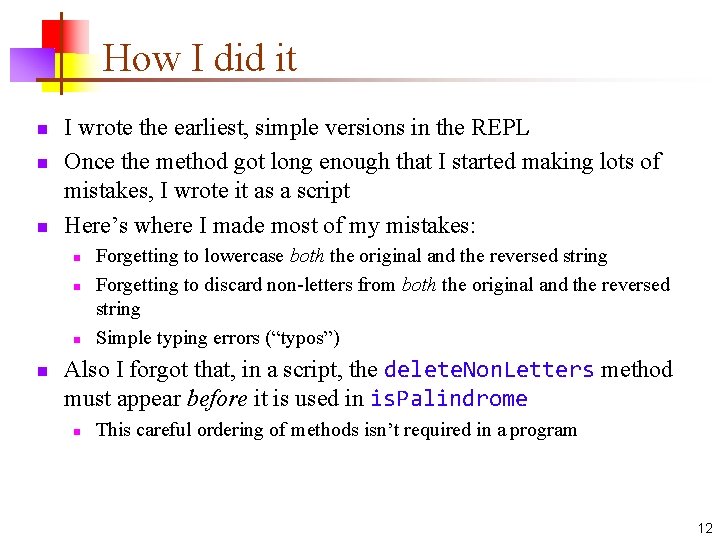
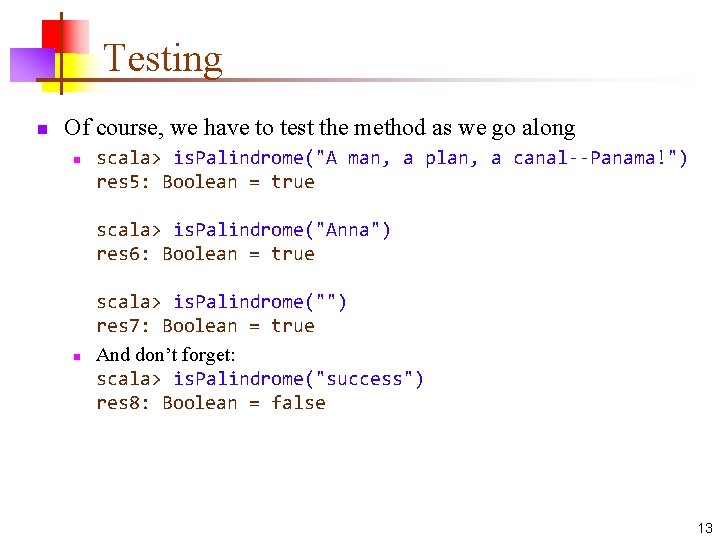
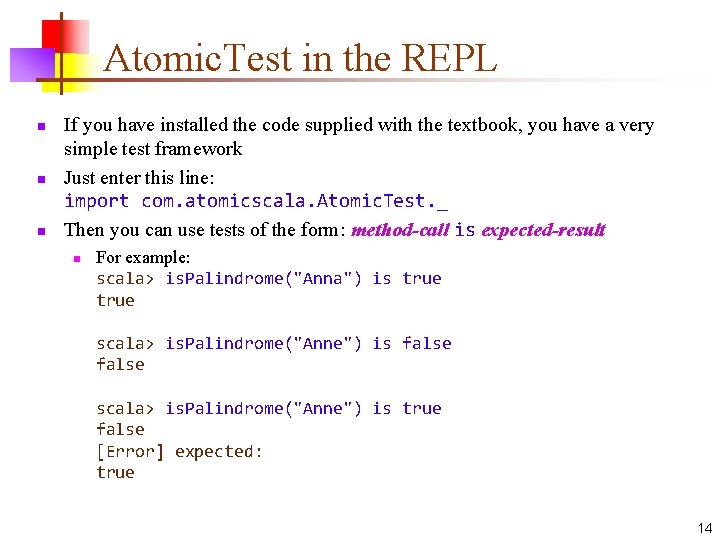
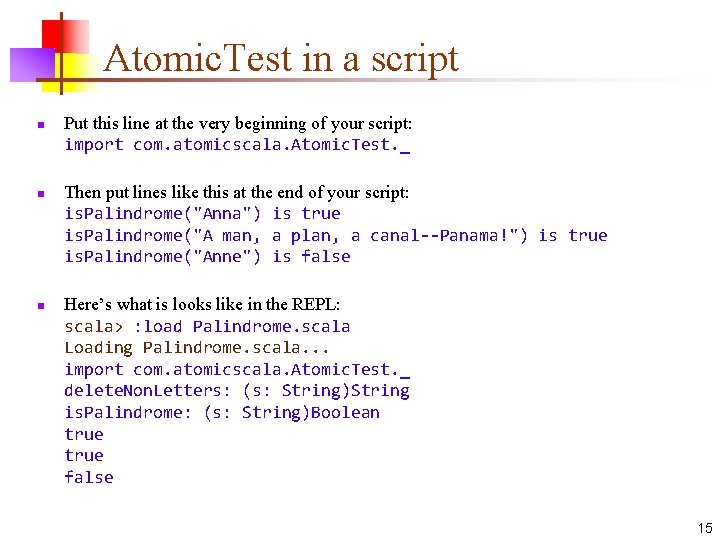
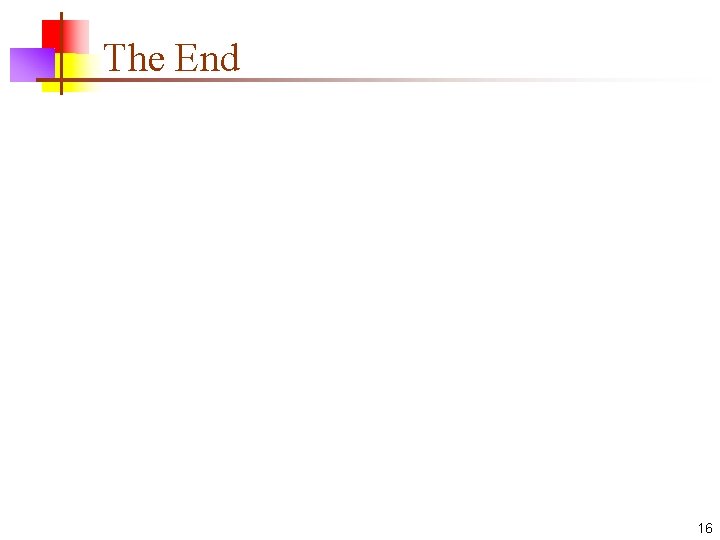
- Slides: 16
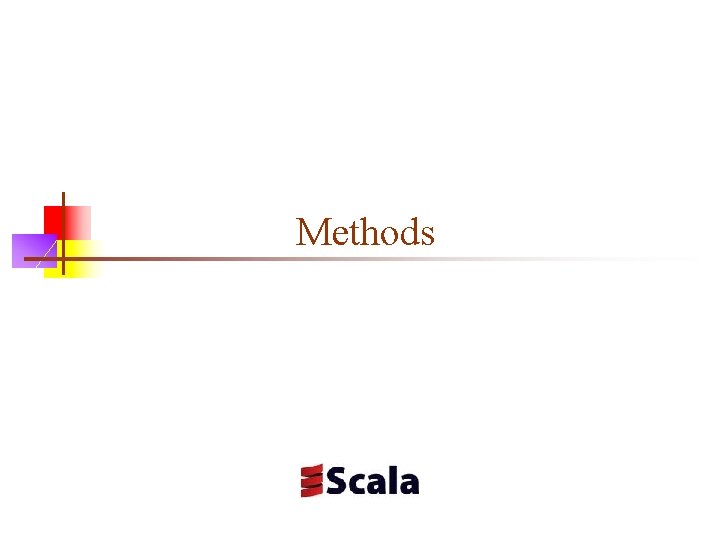
Methods
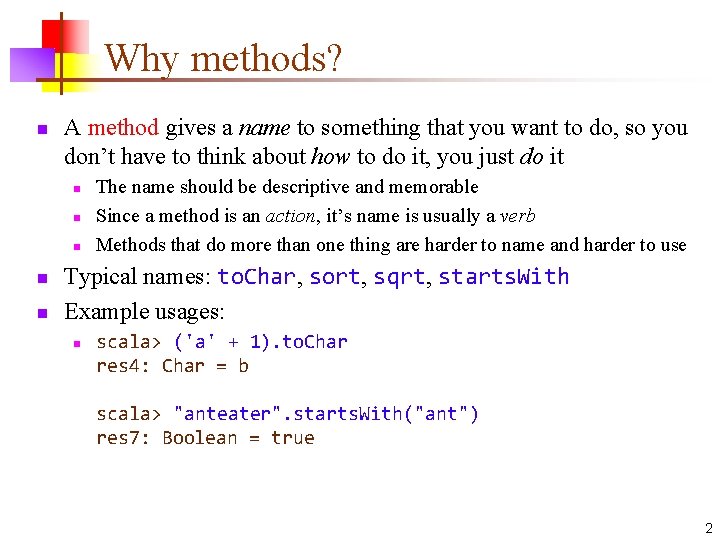
Why methods? n A method gives a name to something that you want to do, so you don’t have to think about how to do it, you just do it n n n The name should be descriptive and memorable Since a method is an action, it’s name is usually a verb Methods that do more than one thing are harder to name and harder to use Typical names: to. Char, sort, sqrt, starts. With Example usages: n scala> ('a' + 1). to. Char res 4: Char = b scala> "anteater". starts. With("ant") res 7: Boolean = true 2
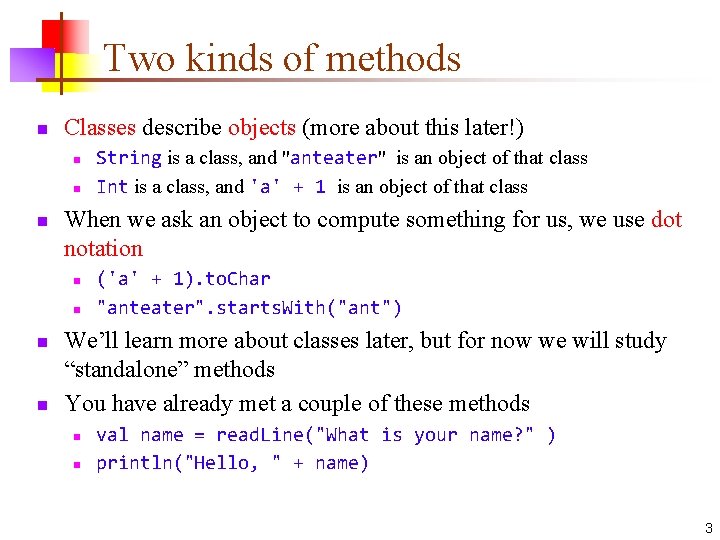
Two kinds of methods n Classes describe objects (more about this later!) n n n When we ask an object to compute something for us, we use dot notation n n String is a class, and "anteater" is an object of that class Int is a class, and 'a' + 1 is an object of that class ('a' + 1). to. Char "anteater". starts. With("ant") We’ll learn more about classes later, but for now we will study “standalone” methods You have already met a couple of these methods n n val name = read. Line("What is your name? " ) println("Hello, " + name) 3
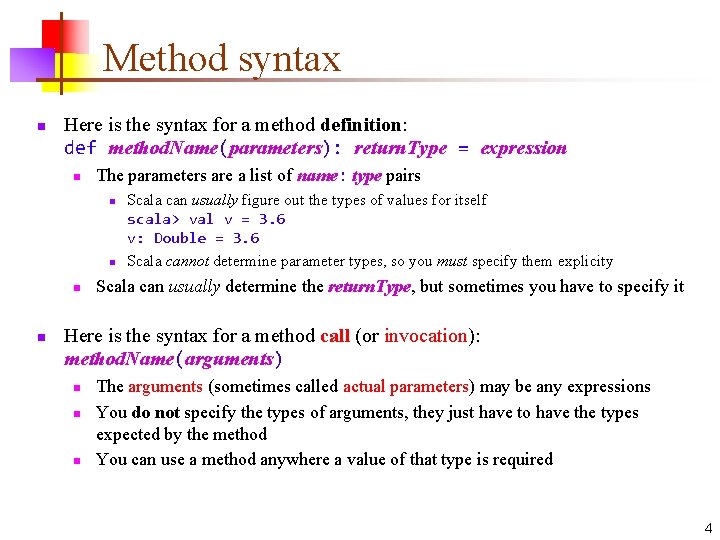
Method syntax n Here is the syntax for a method definition: def method. Name(parameters): return. Type = expression n The parameters are a list of name: type pairs n n Scala can usually figure out the types of values for itself scala> val v = 3. 6 v: Double = 3. 6 Scala cannot determine parameter types, so you must specify them explicity Scala can usually determine the return. Type, but sometimes you have to specify it Here is the syntax for a method call (or invocation): method. Name(arguments) n n n The arguments (sometimes called actual parameters) may be any expressions You do not specify the types of arguments, they just have to have the types expected by the method You can use a method anywhere a value of that type is required 4
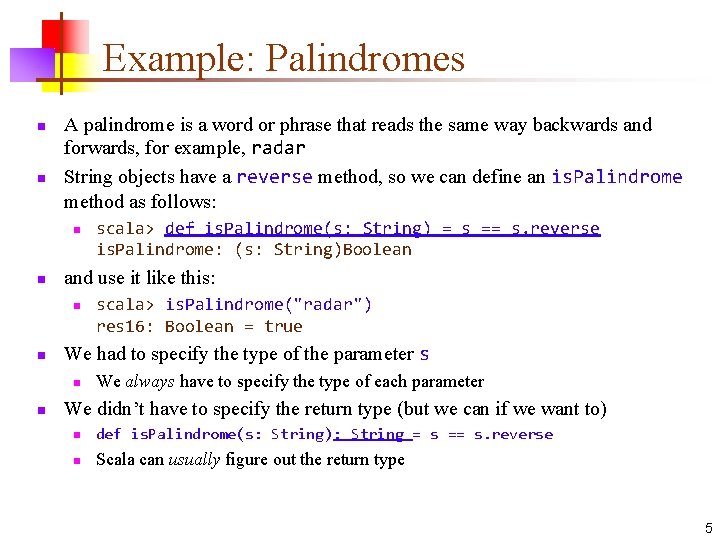
Example: Palindromes n n A palindrome is a word or phrase that reads the same way backwards and forwards, for example, radar String objects have a reverse method, so we can define an is. Palindrome method as follows: n n and use it like this: n n scala> is. Palindrome("radar") res 16: Boolean = true We had to specify the type of the parameter s n n scala> def is. Palindrome(s: String) = s == s. reverse is. Palindrome: (s: String)Boolean We always have to specify the type of each parameter We didn’t have to specify the return type (but we can if we want to) n def is. Palindrome(s: String): String = s == s. reverse n Scala can usually figure out the return type 5
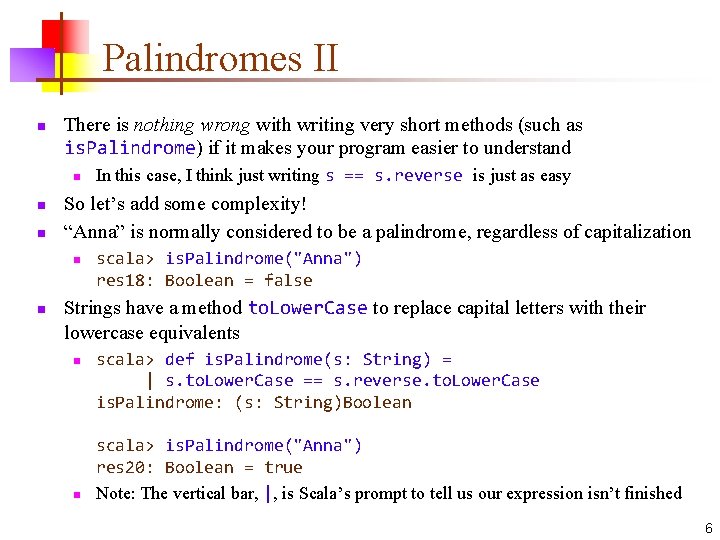
Palindromes II n There is nothing wrong with writing very short methods (such as is. Palindrome) if it makes your program easier to understand n n n So let’s add some complexity! “Anna” is normally considered to be a palindrome, regardless of capitalization n n In this case, I think just writing s == s. reverse is just as easy scala> is. Palindrome("Anna") res 18: Boolean = false Strings have a method to. Lower. Case to replace capital letters with their lowercase equivalents n n scala> def is. Palindrome(s: String) = | s. to. Lower. Case == s. reverse. to. Lower. Case is. Palindrome: (s: String)Boolean scala> is. Palindrome("Anna") res 20: Boolean = true Note: The vertical bar, |, is Scala’s prompt to tell us our expression isn’t finished 6
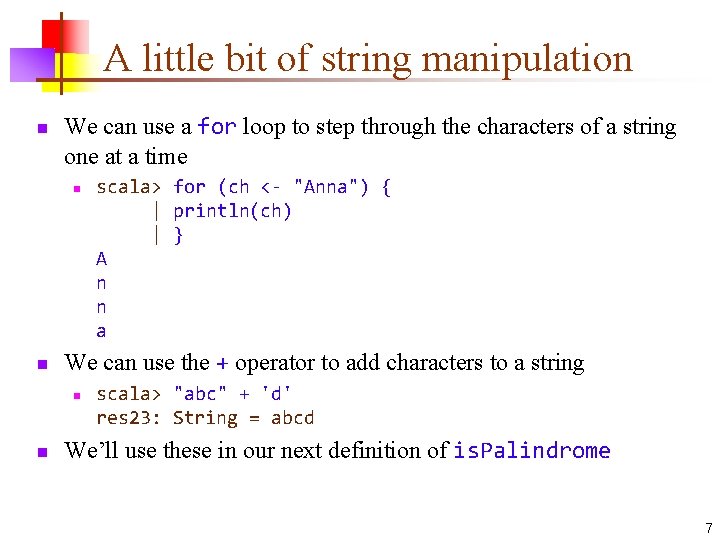
A little bit of string manipulation n We can use a for loop to step through the characters of a string one at a time n n We can use the + operator to add characters to a string n n scala> for (ch <- "Anna") { | println(ch) | } A n n a scala> "abc" + 'd' res 23: String = abcd We’ll use these in our next definition of is. Palindrome 7
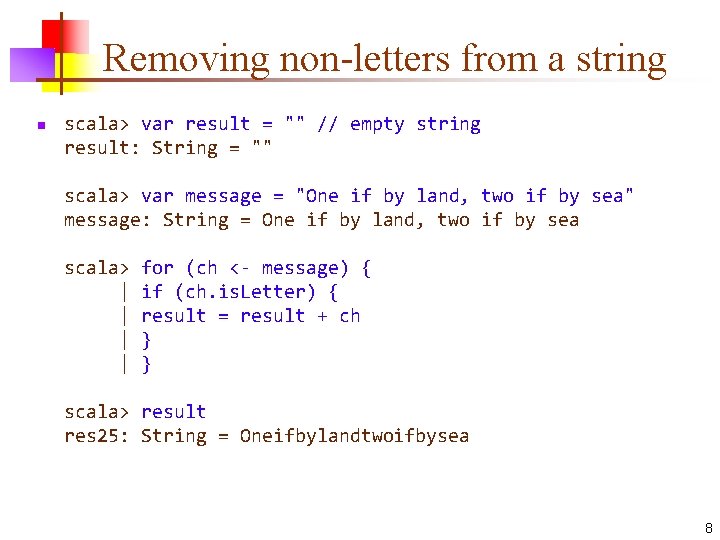
Removing non-letters from a string n scala> var result = "" // empty string result: String = "" scala> var message = "One if by land, two if by sea" message: String = One if by land, two if by sea scala> | | for (ch <- message) { if (ch. is. Letter) { result = result + ch } } scala> result res 25: String = Oneifbylandtwoifbysea 8
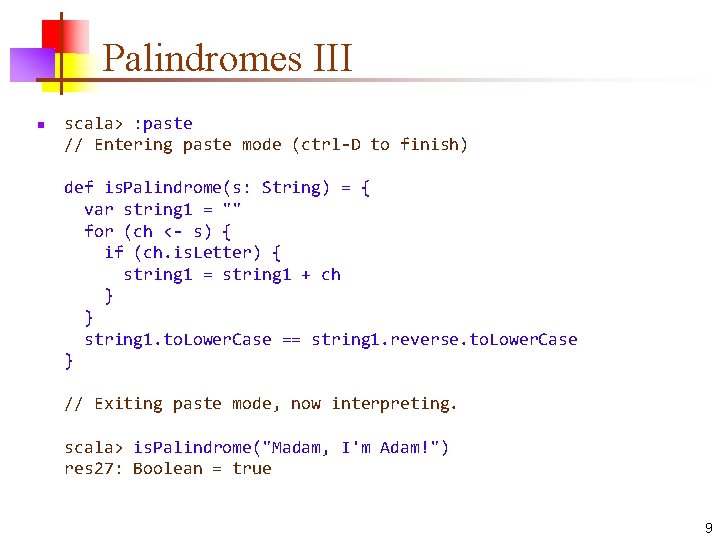
Palindromes III n scala> : paste // Entering paste mode (ctrl-D to finish) def is. Palindrome(s: String) = { var string 1 = "" for (ch <- s) { if (ch. is. Letter) { string 1 = string 1 + ch } } string 1. to. Lower. Case == string 1. reverse. to. Lower. Case } // Exiting paste mode, now interpreting. scala> is. Palindrome("Madam, I'm Adam!") res 27: Boolean = true 9
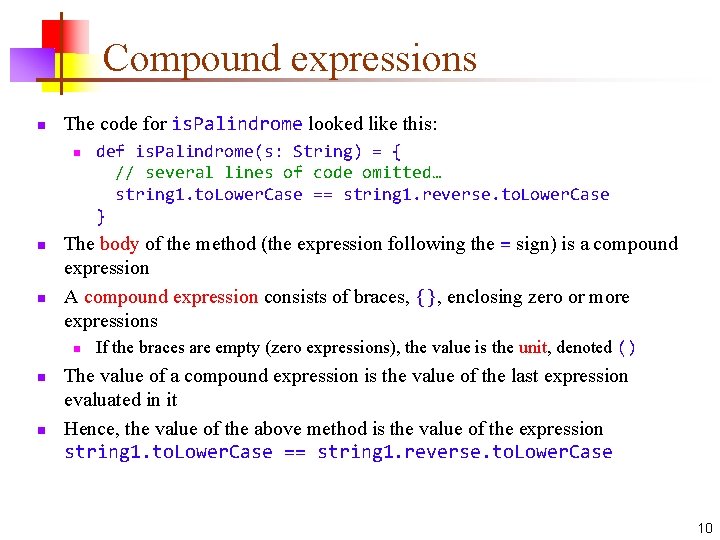
Compound expressions n The code for is. Palindrome looked like this: n n n The body of the method (the expression following the = sign) is a compound expression A compound expression consists of braces, {}, enclosing zero or more expressions n n n def is. Palindrome(s: String) = { // several lines of code omitted… string 1. to. Lower. Case == string 1. reverse. to. Lower. Case } If the braces are empty (zero expressions), the value is the unit, denoted () The value of a compound expression is the value of the last expression evaluated in it Hence, the value of the above method is the value of the expression string 1. to. Lower. Case == string 1. reverse. to. Lower. Case 10
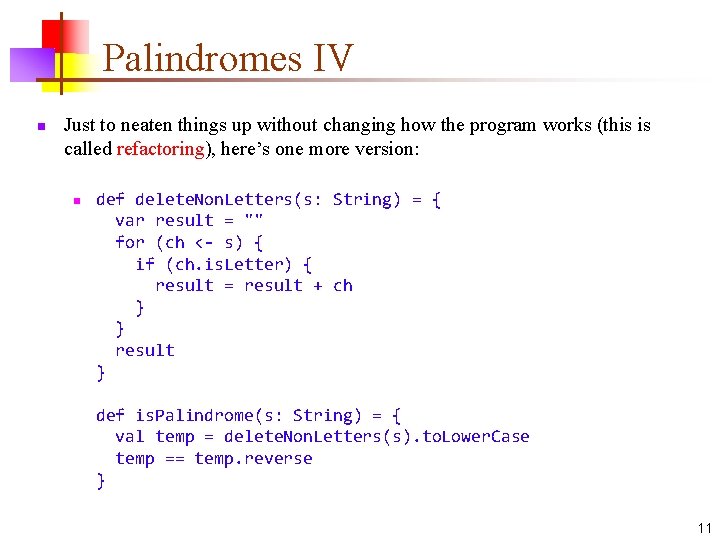
Palindromes IV n Just to neaten things up without changing how the program works (this is called refactoring), here’s one more version: n def delete. Non. Letters(s: String) = { var result = "" for (ch <- s) { if (ch. is. Letter) { result = result + ch } } result } def is. Palindrome(s: String) = { val temp = delete. Non. Letters(s). to. Lower. Case temp == temp. reverse } 11
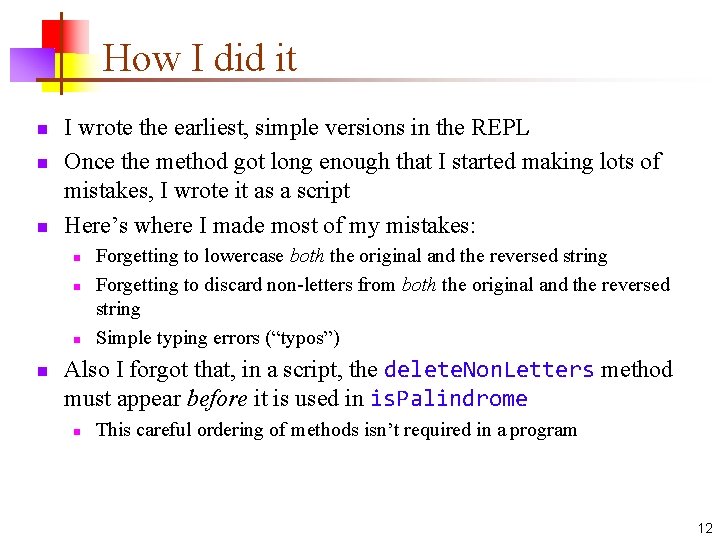
How I did it n n n I wrote the earliest, simple versions in the REPL Once the method got long enough that I started making lots of mistakes, I wrote it as a script Here’s where I made most of my mistakes: n n Forgetting to lowercase both the original and the reversed string Forgetting to discard non-letters from both the original and the reversed string Simple typing errors (“typos”) Also I forgot that, in a script, the delete. Non. Letters method must appear before it is used in is. Palindrome n This careful ordering of methods isn’t required in a program 12
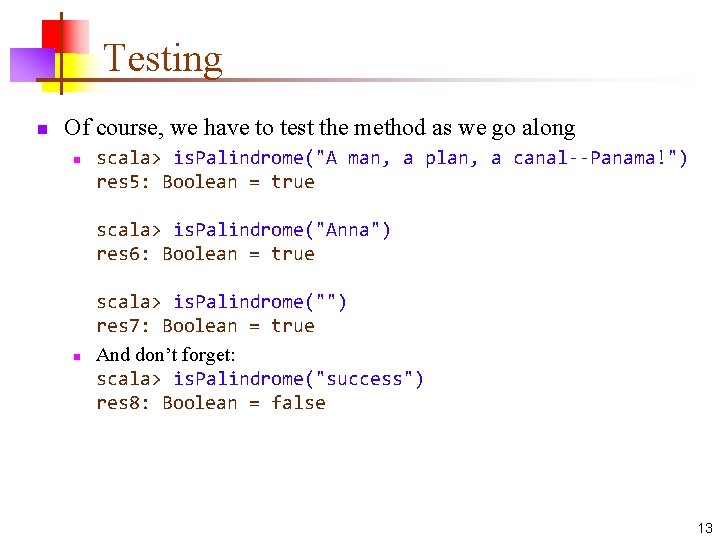
Testing n Of course, we have to test the method as we go along n scala> is. Palindrome("A man, a plan, a canal--Panama!") res 5: Boolean = true scala> is. Palindrome("Anna") res 6: Boolean = true n scala> is. Palindrome("") res 7: Boolean = true And don’t forget: scala> is. Palindrome("success") res 8: Boolean = false 13
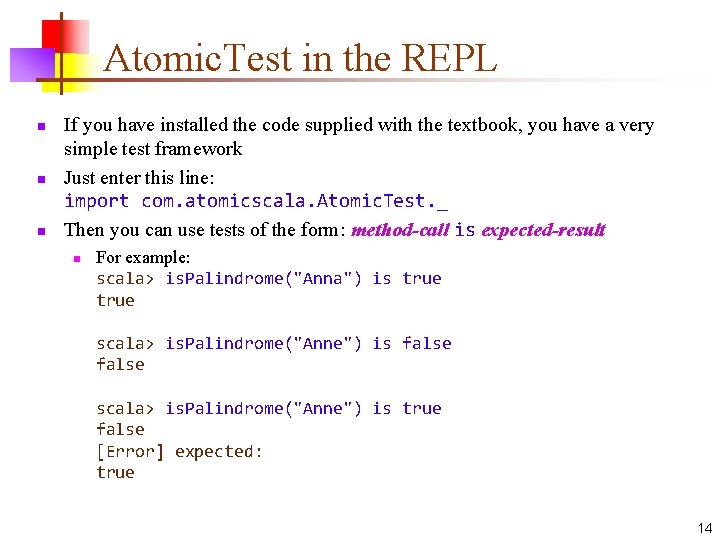
Atomic. Test in the REPL n n n If you have installed the code supplied with the textbook, you have a very simple test framework Just enter this line: import com. atomicscala. Atomic. Test. _ Then you can use tests of the form: method-call is expected-result n For example: scala> is. Palindrome("Anna") is true scala> is. Palindrome("Anne") is false scala> is. Palindrome("Anne") is true false [Error] expected: true 14
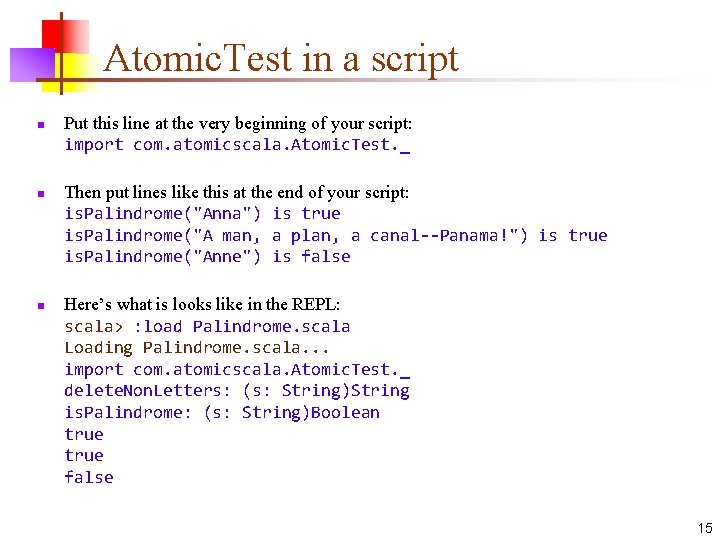
Atomic. Test in a script n n n Put this line at the very beginning of your script: import com. atomicscala. Atomic. Test. _ Then put lines like this at the end of your script: is. Palindrome("Anna") is true is. Palindrome("A man, a plan, a canal--Panama!") is true is. Palindrome("Anne") is false Here’s what is looks like in the REPL: scala> : load Palindrome. scala Loading Palindrome. scala. . . import com. atomicscala. Atomic. Test. _ delete. Non. Letters: (s: String)String is. Palindrome: (s: String)Boolean true false 15
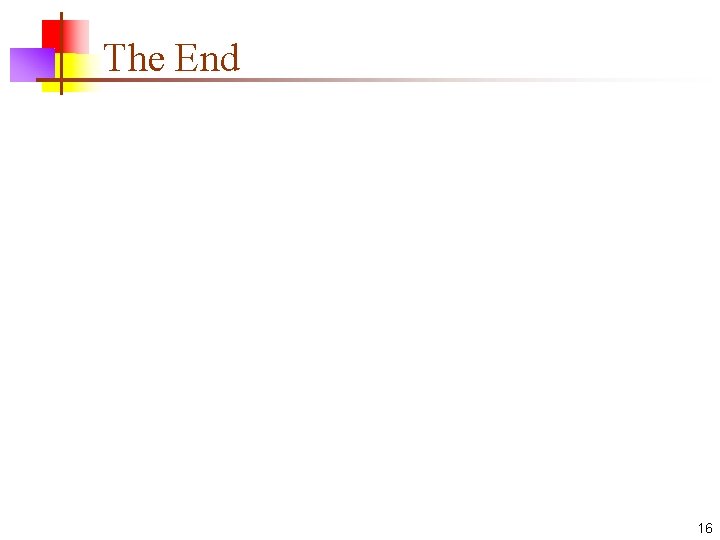
The End 16
Why why why why
Dont ask why why why
Conclusion of symposium
Direct and indirect wax pattern
Your love never gives up
Chapter 16 catcher in the rye
Kinetic theory of solids
Nuptial in romeo and juliet
A cash-flow statement gives you important feedback on your:
Non essential amino acids in food
Rima puts the tub in the sun
Primitive streak is formed from
Dental papilla gives rise to
When an author gives hints or clues
A woman gives a beggar 50 cents
The ground gives way question answers
Acid halide