ITC 250 Copyright 2008 ITC 1 Chapter 10
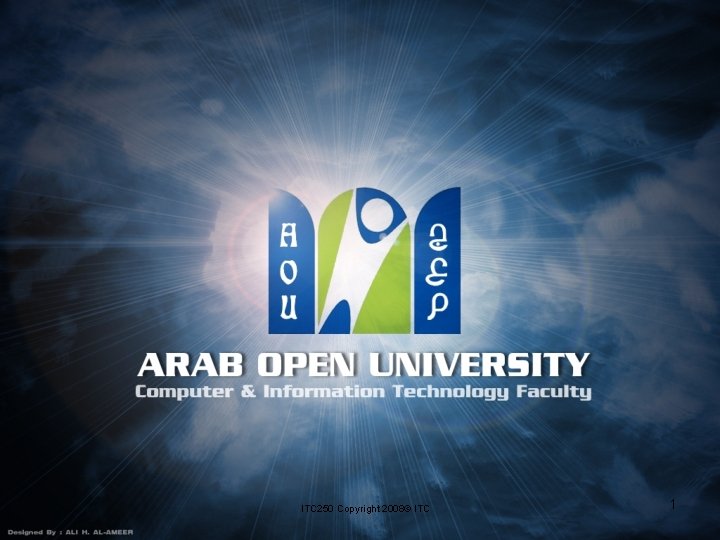
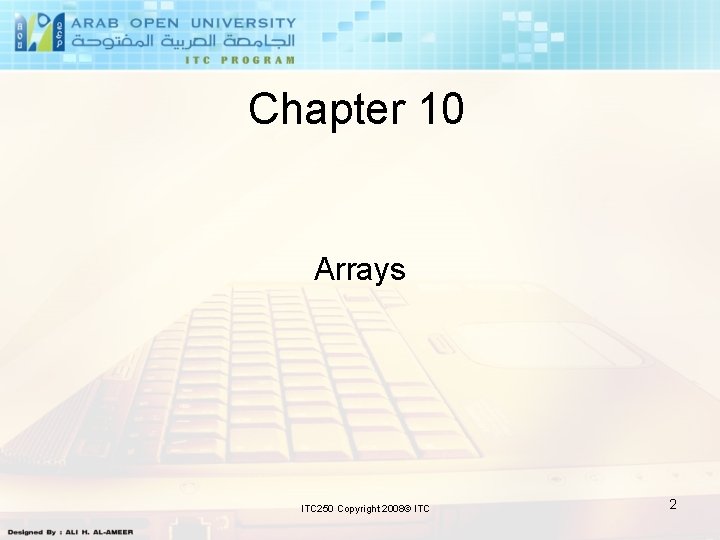
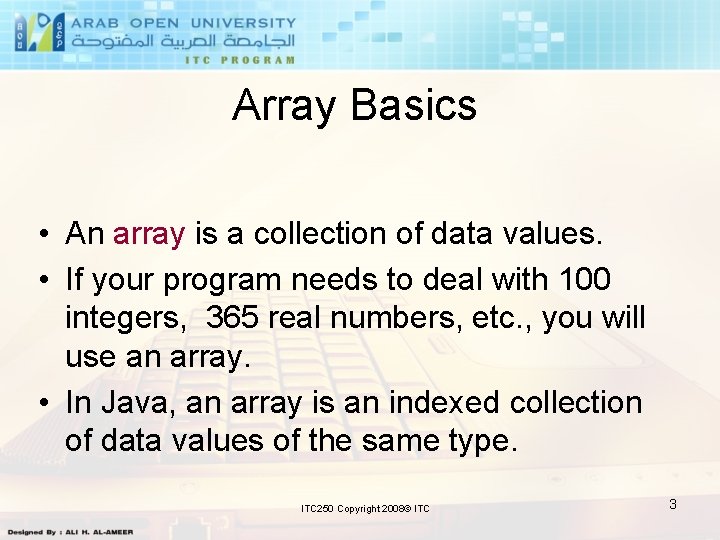
![Arrays of Primitive Data Types • Array Declaration <data type> [ ] <variable> 1 Arrays of Primitive Data Types • Array Declaration <data type> [ ] <variable> 1](https://slidetodoc.com/presentation_image_h2/04ddf621b2a0e443b33d7b566dd18367/image-4.jpg)
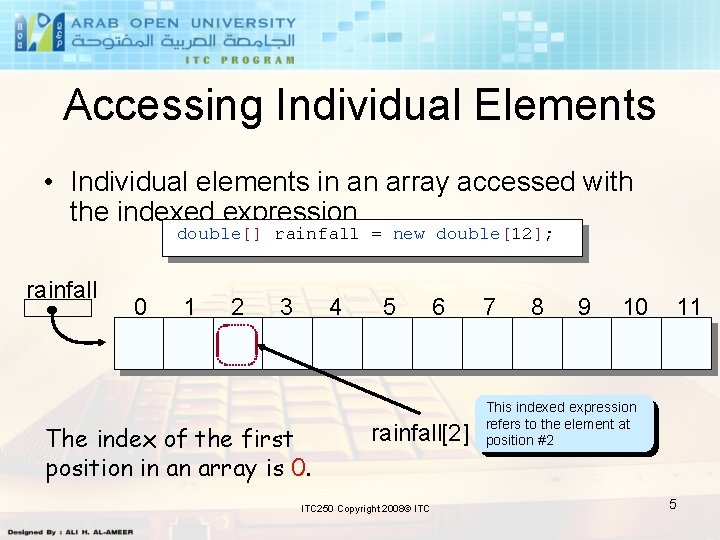
![Array Processing – Sample 1 double[] rainfall = new double[12]; double The public constant Array Processing – Sample 1 double[] rainfall = new double[12]; double The public constant](https://slidetodoc.com/presentation_image_h2/04ddf621b2a0e443b33d7b566dd18367/image-6.jpg)
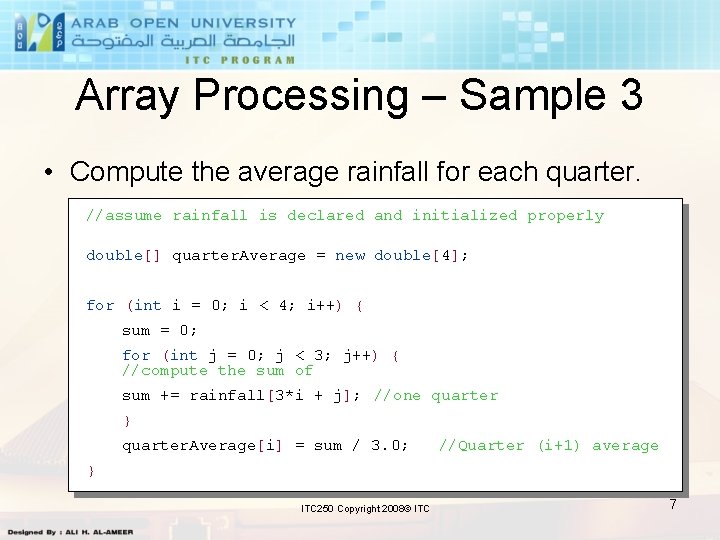
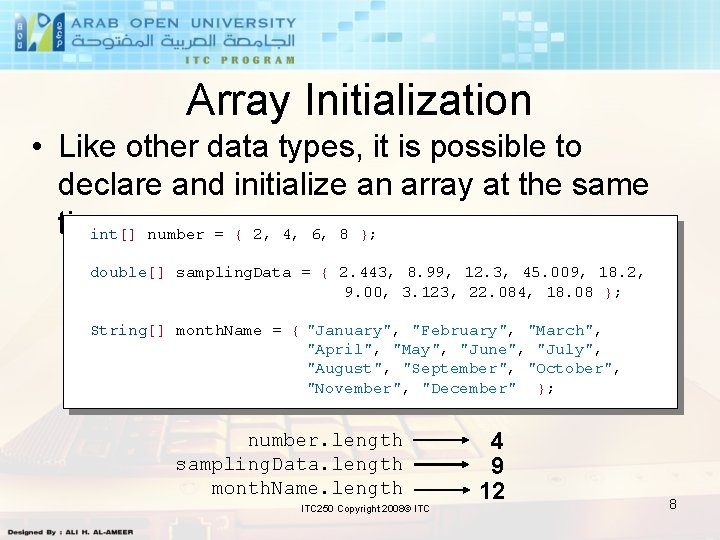
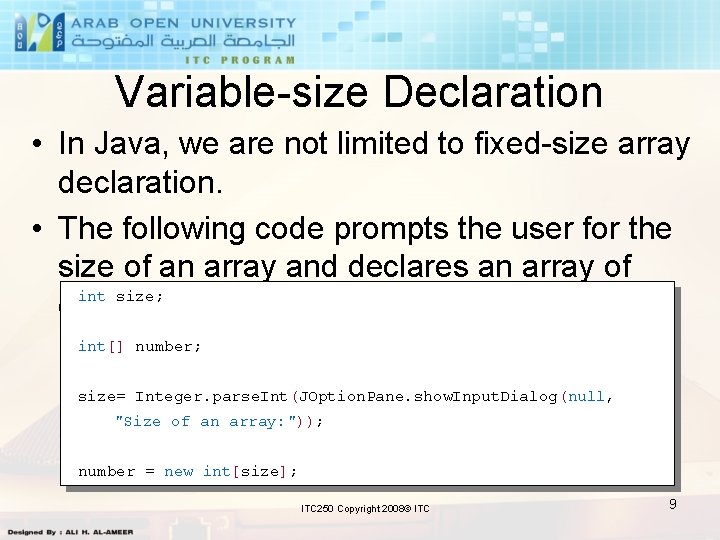
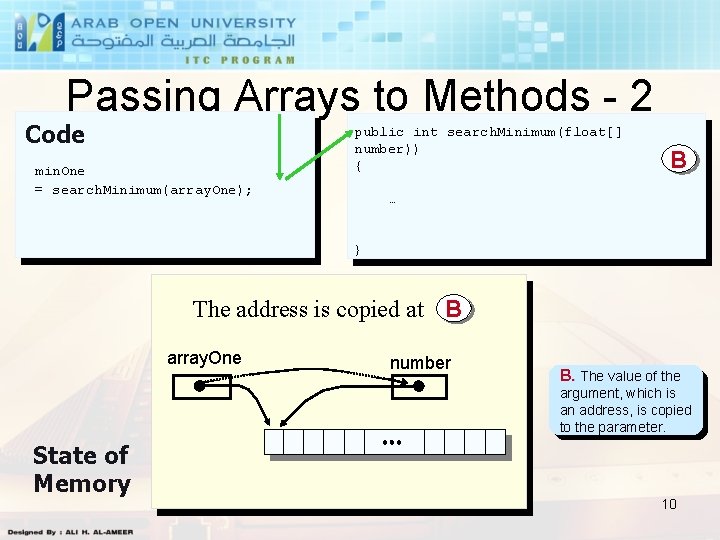
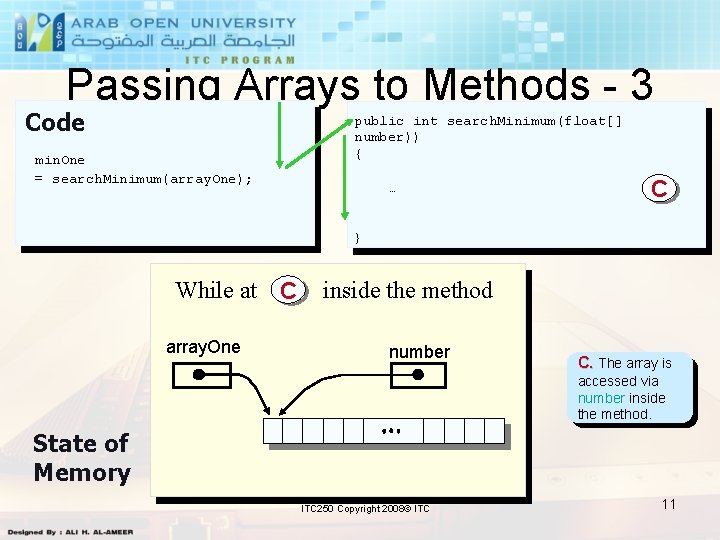
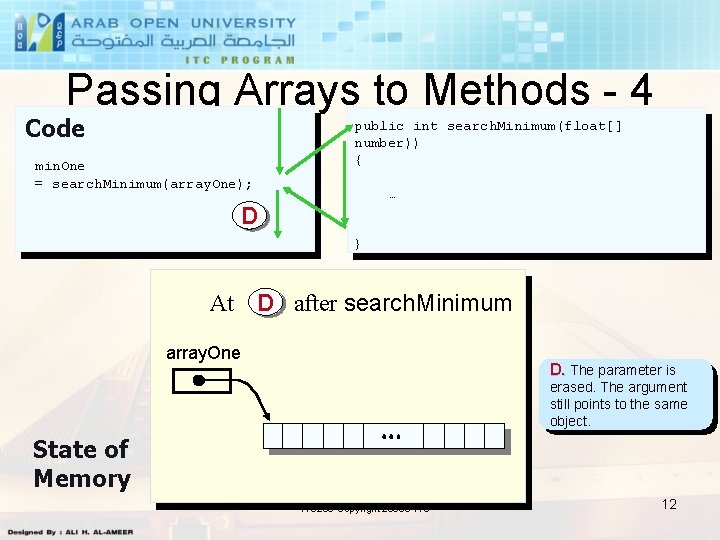
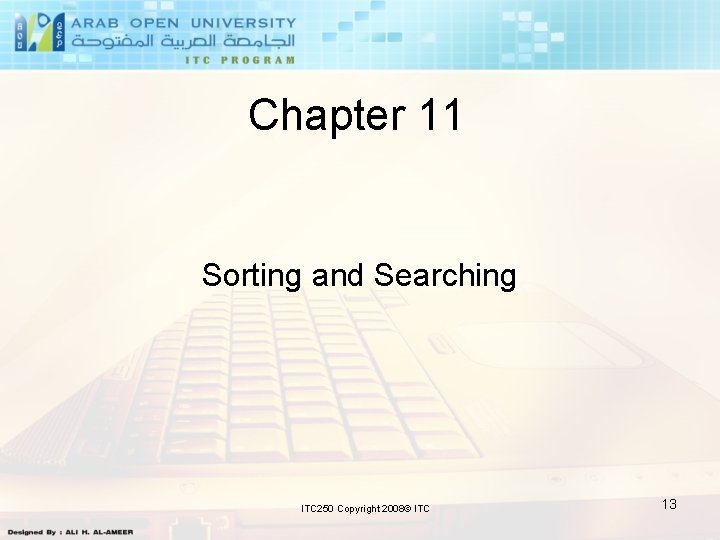
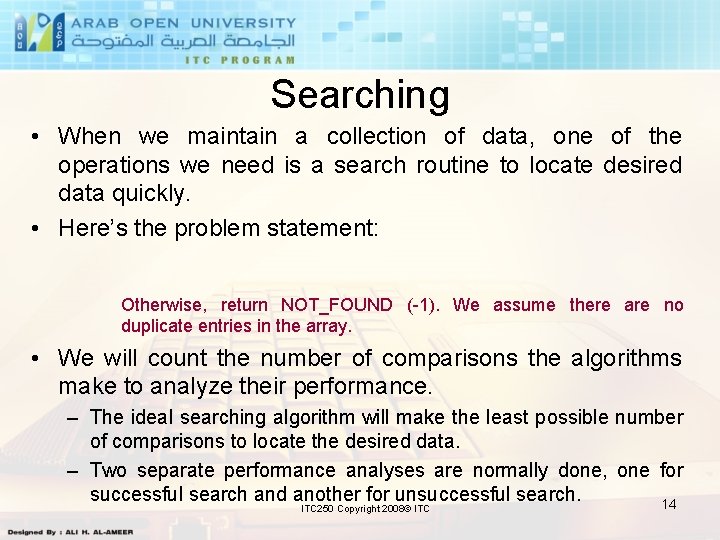
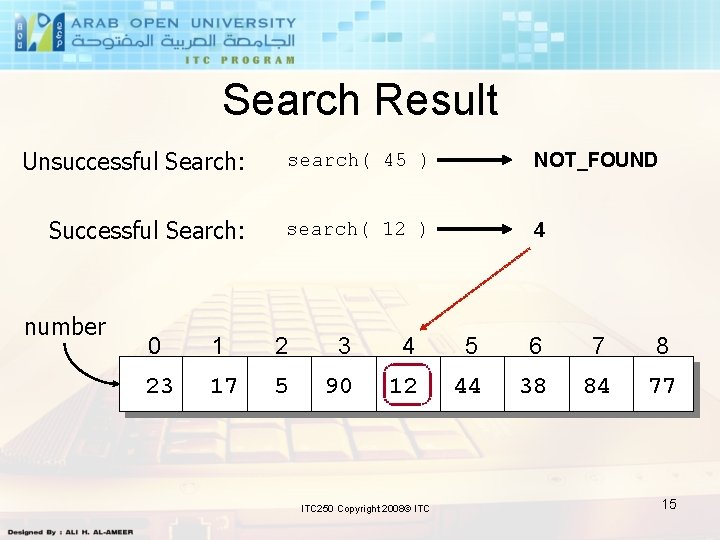
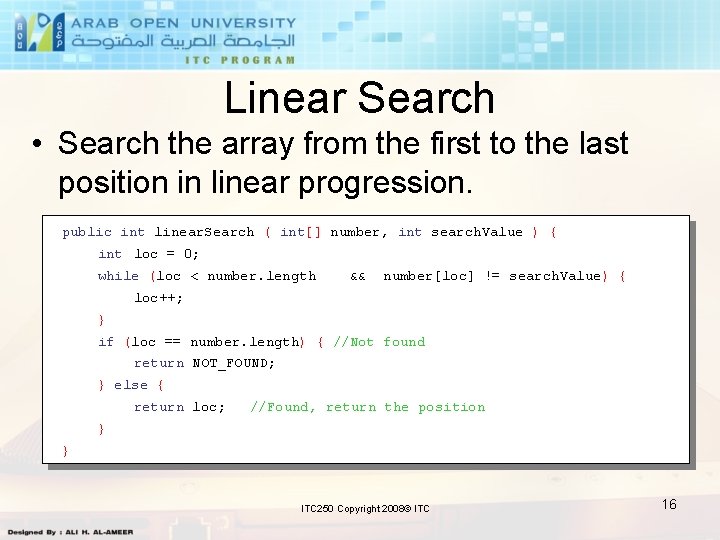
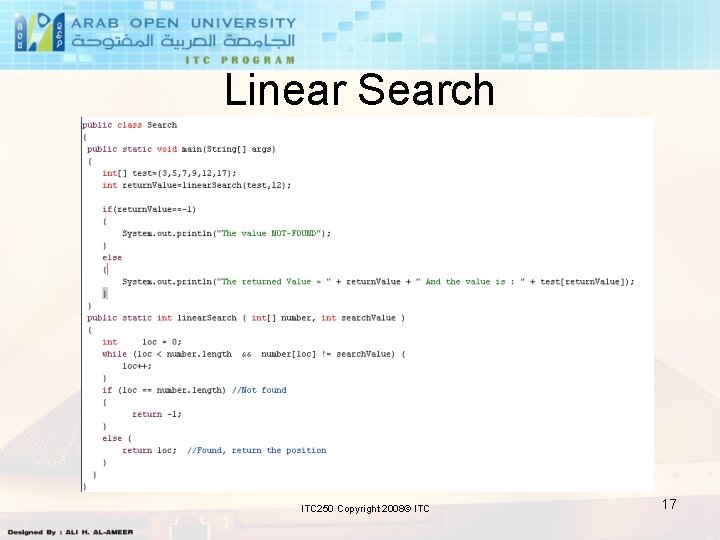
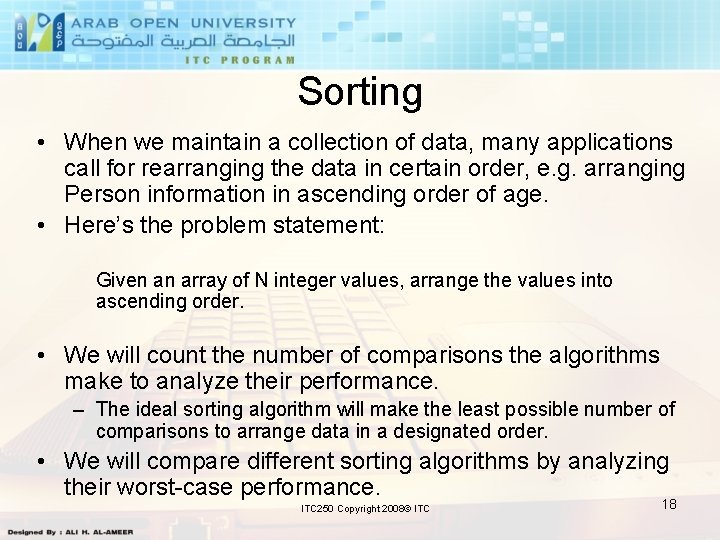
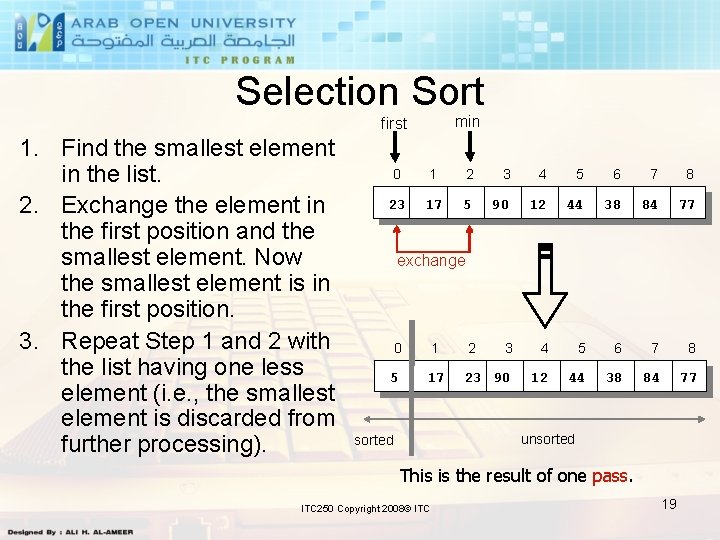
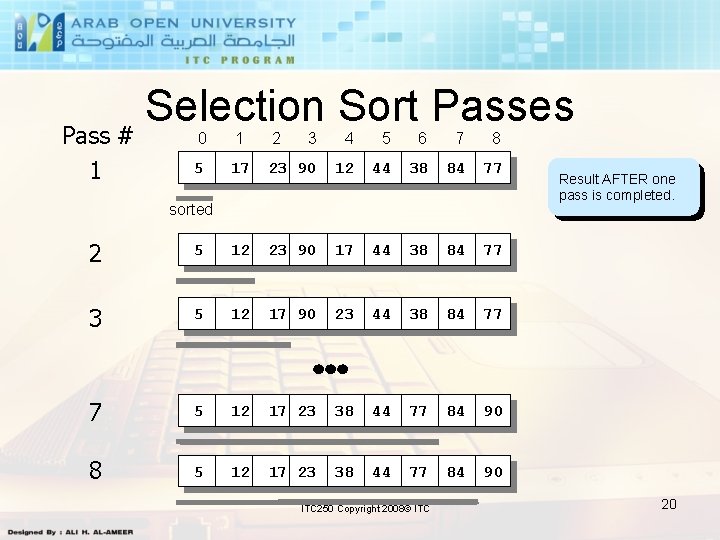
![Selection Sort Routine public void selection. Sort(int[] number) { int start. Index, min. Index, Selection Sort Routine public void selection. Sort(int[] number) { int start. Index, min. Index,](https://slidetodoc.com/presentation_image_h2/04ddf621b2a0e443b33d7b566dd18367/image-21.jpg)
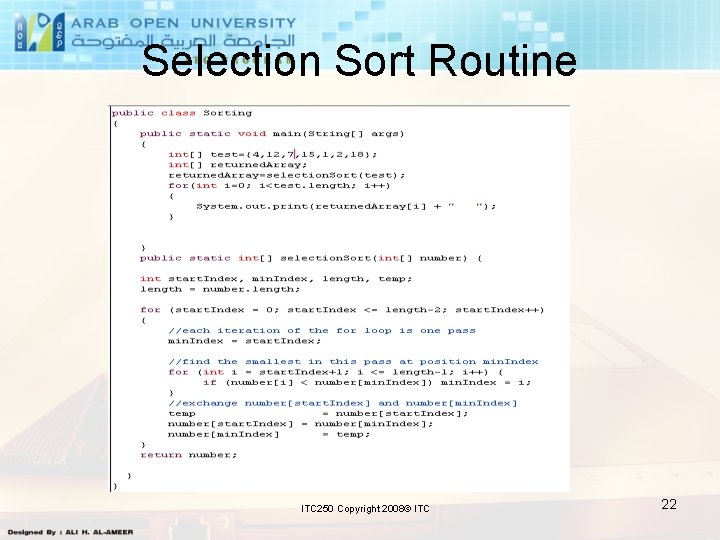
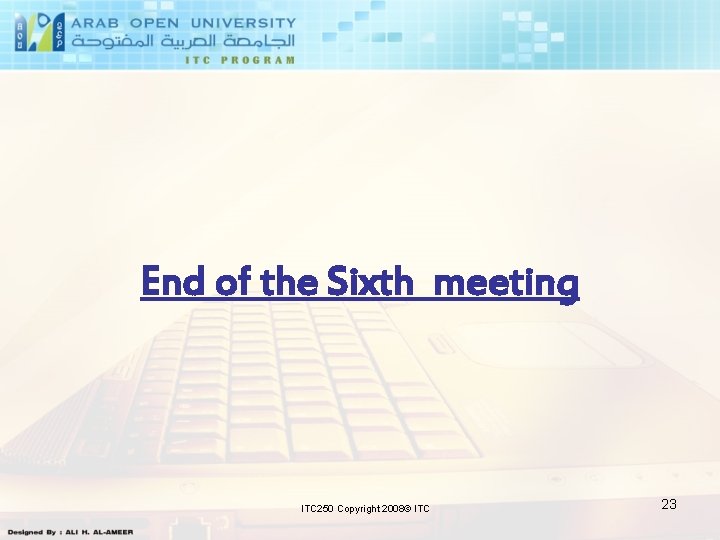
- Slides: 23
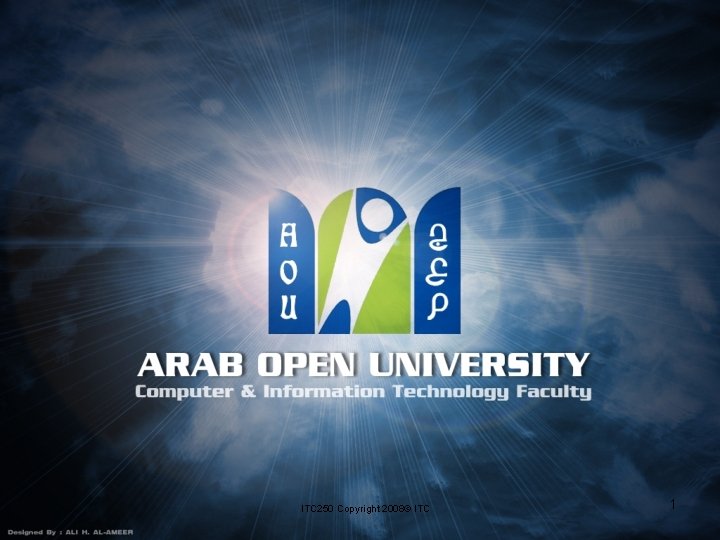
ITC 250 Copyright 2008© ITC 1
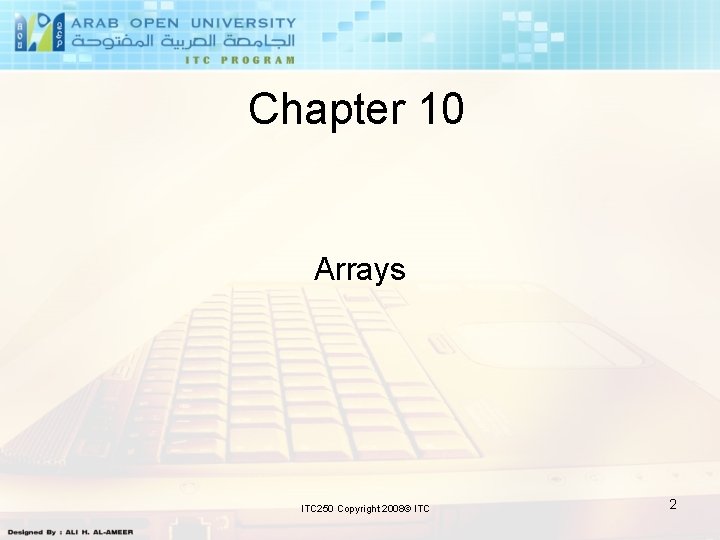
Chapter 10 Arrays ITC 250 Copyright 2008© ITC 2
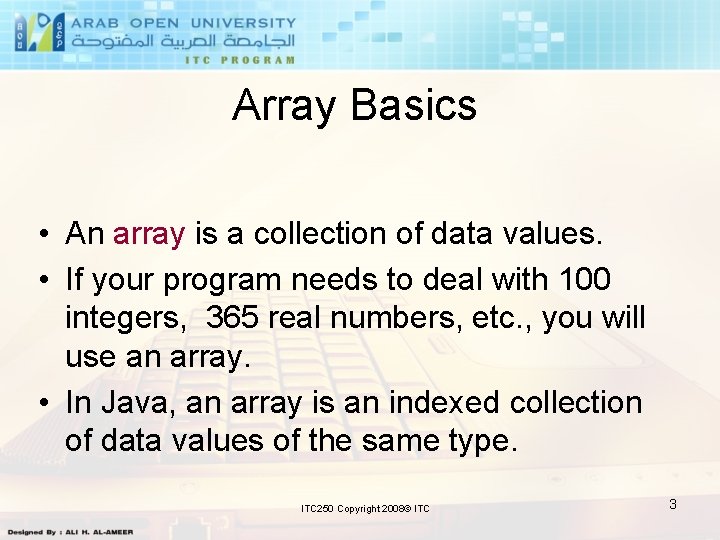
Array Basics • An array is a collection of data values. • If your program needs to deal with 100 integers, 365 real numbers, etc. , you will use an array. • In Java, an array is an indexed collection of data values of the same type. ITC 250 Copyright 2008© ITC 3
![Arrays of Primitive Data Types Array Declaration data type variable 1 Arrays of Primitive Data Types • Array Declaration <data type> [ ] <variable> 1](https://slidetodoc.com/presentation_image_h2/04ddf621b2a0e443b33d7b566dd18367/image-4.jpg)
Arrays of Primitive Data Types • Array Declaration <data type> [ ] <variable> 1 <data type> <variable>[ ] //variation 2 • Array Creation • Variation 2 <variable> = new 1 <data type> [ <size>Variation ] double[ ] rainfall; double rainfall [ ]; Example rainfall = new double[12]; Copyrightan 2008©object! ITC An array ITC 250 is like 4
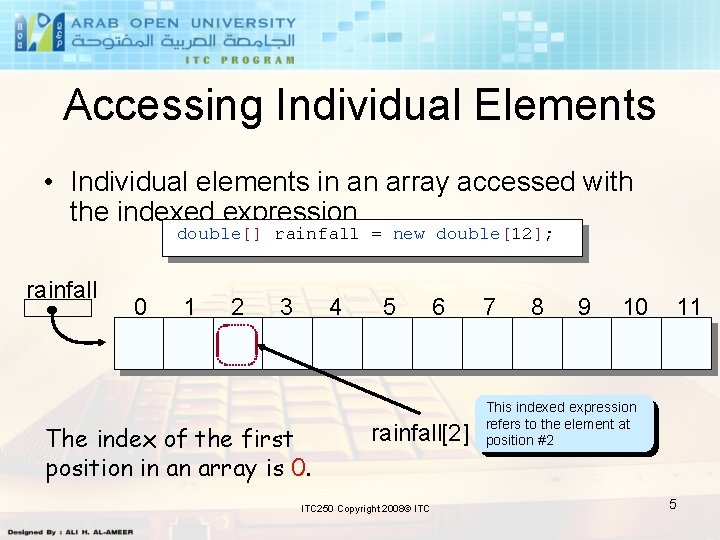
Accessing Individual Elements • Individual elements in an array accessed with the indexed expression. double[] rainfall = new double[12]; rainfall 0 1 2 3 4 The index of the first position in an array is 0. 5 6 rainfall[2] ITC 250 Copyright 2008© ITC 7 8 9 10 11 This indexed expression refers to the element at position #2 5
![Array Processing Sample 1 double rainfall new double12 double The public constant Array Processing – Sample 1 double[] rainfall = new double[12]; double The public constant](https://slidetodoc.com/presentation_image_h2/04ddf621b2a0e443b33d7b566dd18367/image-6.jpg)
Array Processing – Sample 1 double[] rainfall = new double[12]; double The public constant length returns the capacity of an array. annual. Average, sum = 0. 0; for (int i = 0; i < rainfall. length; i++) { rainfall[i] = Double. parse. Double( JOption. Pane. showinput. Dialog(null, "Rainfall for month " + (i+1) ) ); sum += rainfall[i]; } annual. Average = sum / rainfall. length; ITC 250 Copyright 2008© ITC 6
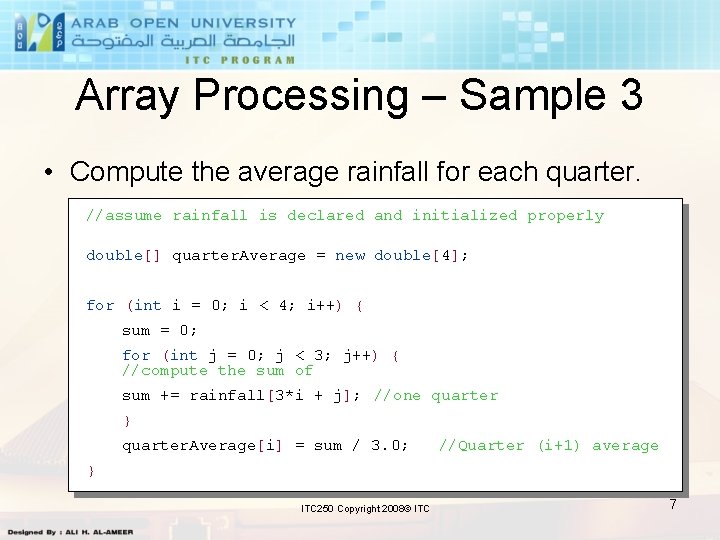
Array Processing – Sample 3 • Compute the average rainfall for each quarter. //assume rainfall is declared and initialized properly double[] quarter. Average = new double[4]; for (int i = 0; i < 4; i++) { sum = 0; for (int j = 0; j < 3; j++) { //compute the sum of sum += rainfall[3*i + j]; //one quarter } quarter. Average[i] = sum / 3. 0; //Quarter (i+1) average } ITC 250 Copyright 2008© ITC 7
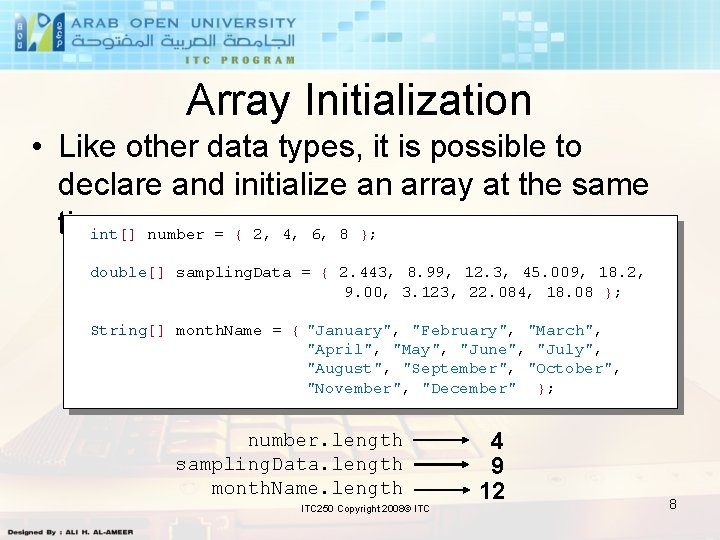
Array Initialization • Like other data types, it is possible to declare and initialize an array at the same time. int[] number = { 2, 4, 6, 8 }; double[] sampling. Data = { 2. 443, 8. 99, 12. 3, 45. 009, 18. 2, 9. 00, 3. 123, 22. 084, 18. 08 }; String[] month. Name = { "January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December" }; number. length sampling. Data. length month. Name. length ITC 250 Copyright 2008© ITC 4 9 12 8
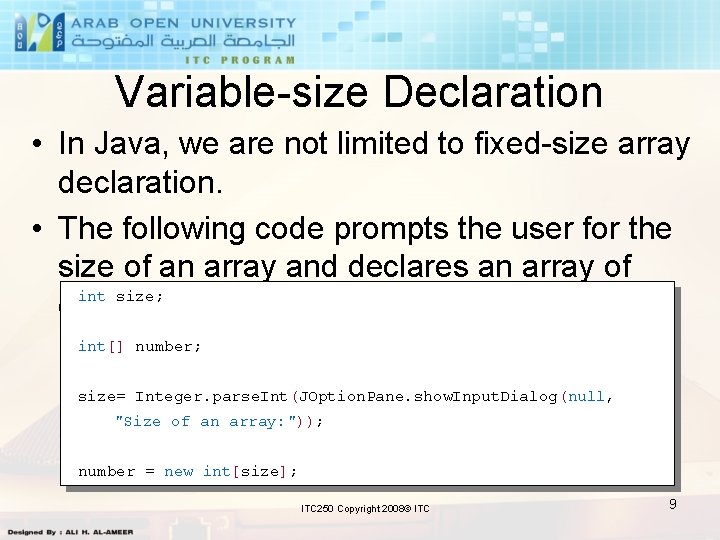
Variable-size Declaration • In Java, we are not limited to fixed-size array declaration. • The following code prompts the user for the size of an array and declares an array of int size; designated size: int[] number; size= Integer. parse. Int(JOption. Pane. show. Input. Dialog(null, "Size of an array: ")); number = new int[size]; ITC 250 Copyright 2008© ITC 9
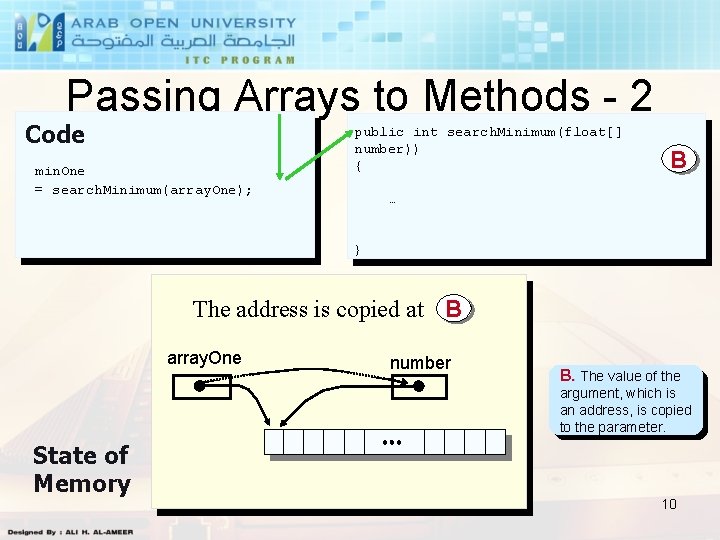
Passing Arrays to Methods - 2 Code min. One = search. Minimum(array. One); public int search. Minimum(float[] number)) { B … } The address is copied at B array. One number B. The value of the argument, which is an address, is copied to the parameter. State of Memory ITC 250 Copyright 2008© ITC 10
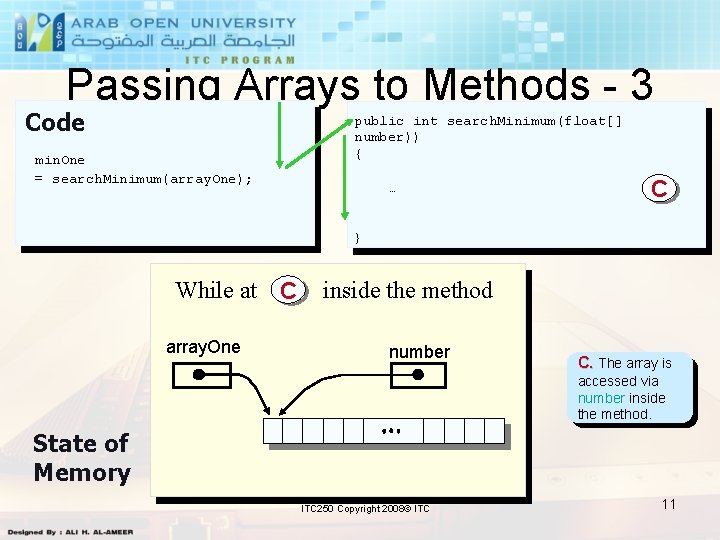
Passing Arrays to Methods - 3 Code min. One = search. Minimum(array. One); public int search. Minimum(float[] number)) { … C } While at C array. One inside the method number C. The array is accessed via number inside the method. State of Memory ITC 250 Copyright 2008© ITC 11
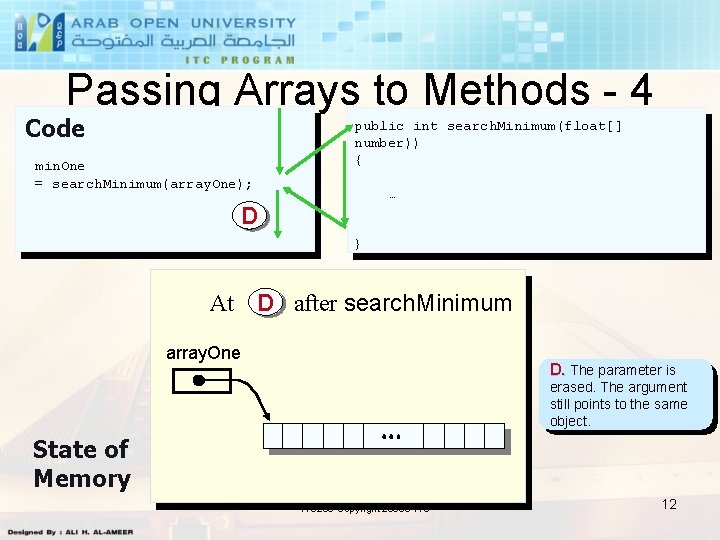
Passing Arrays to Methods - 4 Code min. One = search. Minimum(array. One); public int search. Minimum(float[] number)) { … D } At D after search. Minimum array. One number D. The parameter is erased. The argument still points to the same object. State of Memory ITC 250 Copyright 2008© ITC 12
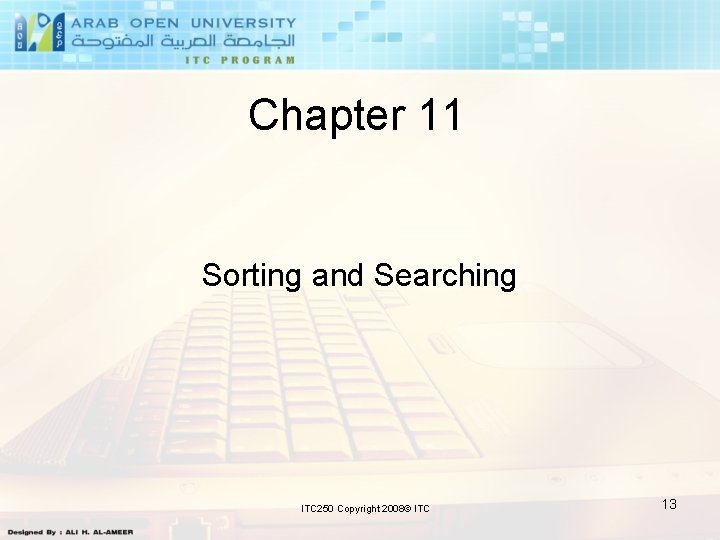
Chapter 11 Sorting and Searching ITC 250 Copyright 2008© ITC 13
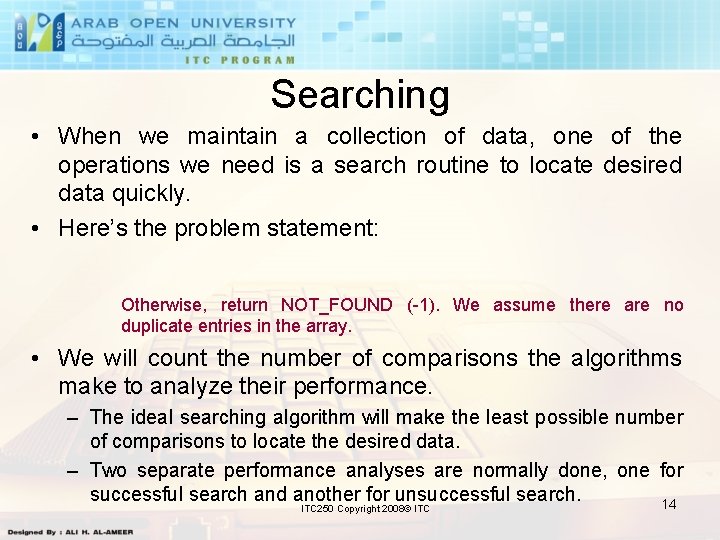
Searching • When we maintain a collection of data, one of the operations we need is a search routine to locate desired data quickly. • Here’s the problem statement: Otherwise, return NOT_FOUND (-1). We assume there are no duplicate entries in the array. • We will count the number of comparisons the algorithms make to analyze their performance. – The ideal searching algorithm will make the least possible number of comparisons to locate the desired data. – Two separate performance analyses are normally done, one for successful search and another for unsuccessful search. 14 ITC 250 Copyright 2008© ITC
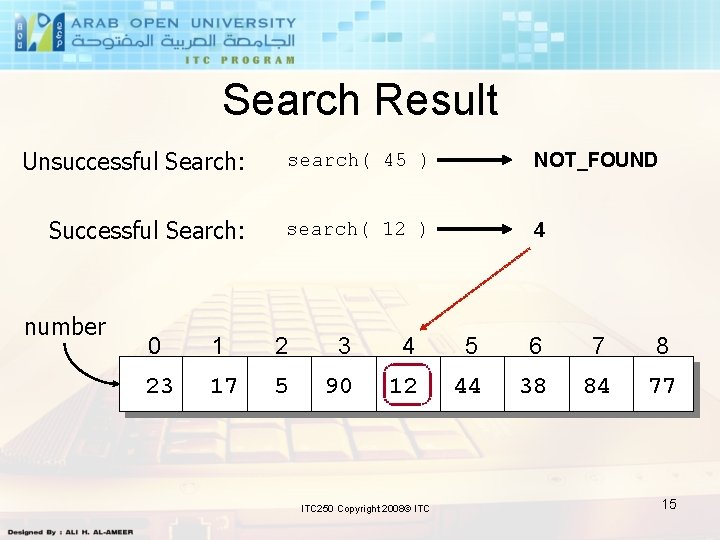
Search Result Unsuccessful Search: search( 45 ) NOT_FOUND Successful Search: search( 12 ) 4 number 0 1 2 3 4 5 6 7 8 23 17 5 90 12 44 38 84 77 ITC 250 Copyright 2008© ITC 15
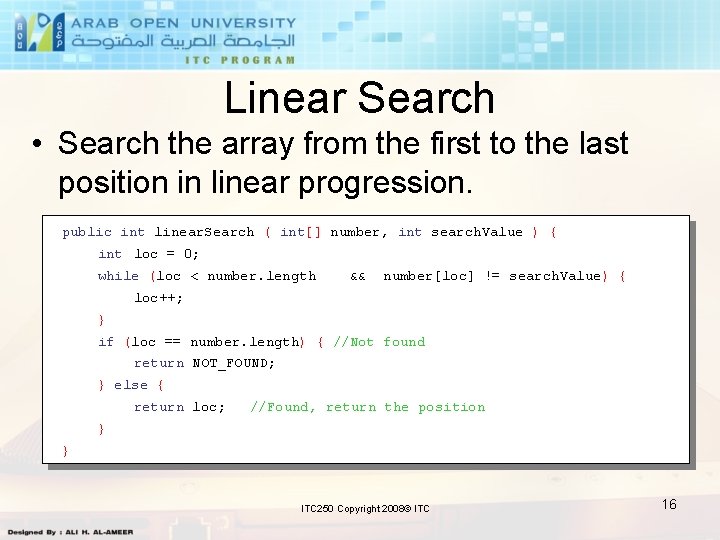
Linear Search • Search the array from the first to the last position in linear progression. public int linear. Search ( int[] number, int search. Value ) { int loc = 0; while (loc < number. length && number[loc] != search. Value) { loc++; } if (loc == number. length) { //Not found return NOT_FOUND; } else { return loc; //Found, return the position } } ITC 250 Copyright 2008© ITC 16
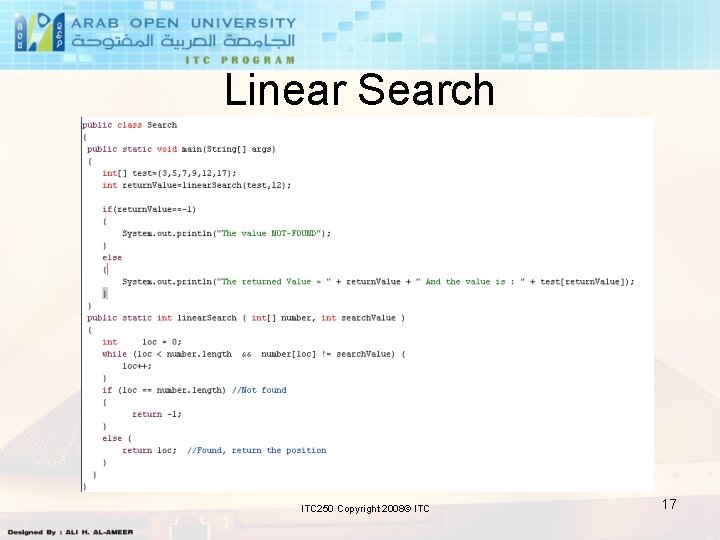
Linear Search ITC 250 Copyright 2008© ITC 17
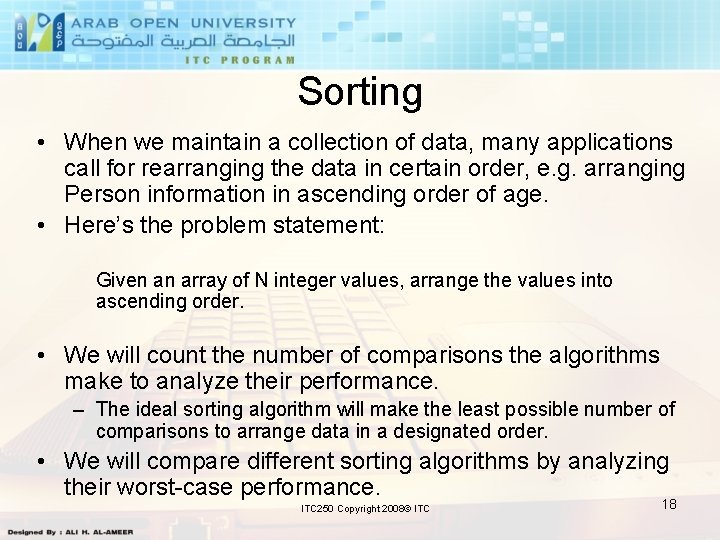
Sorting • When we maintain a collection of data, many applications call for rearranging the data in certain order, e. g. arranging Person information in ascending order of age. • Here’s the problem statement: Given an array of N integer values, arrange the values into ascending order. • We will count the number of comparisons the algorithms make to analyze their performance. – The ideal sorting algorithm will make the least possible number of comparisons to arrange data in a designated order. • We will compare different sorting algorithms by analyzing their worst-case performance. ITC 250 Copyright 2008© ITC 18
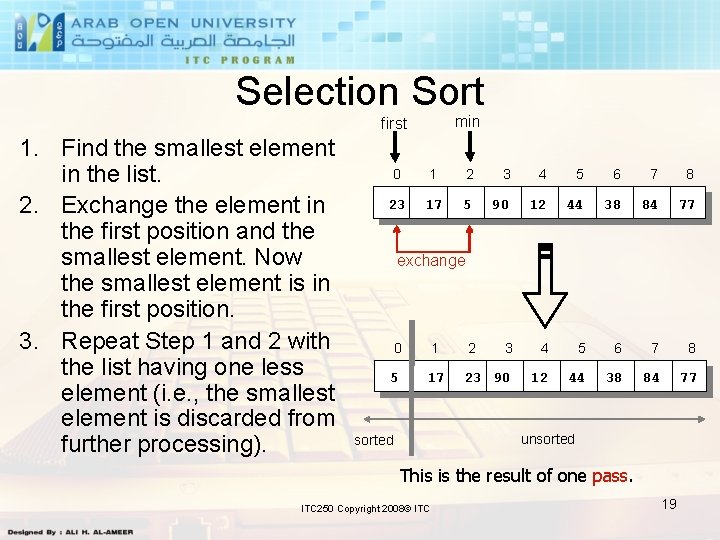
Selection Sort min first 1. Find the smallest element in the list. 2. Exchange the element in the first position and the smallest element. Now the smallest element is in the first position. 3. Repeat Step 1 and 2 with the list having one less element (i. e. , the smallest element is discarded from further processing). 0 1 2 3 4 5 6 7 8 23 17 5 90 12 44 38 84 77 exchange 0 1 2 3 4 5 6 7 8 5 17 23 90 12 44 38 84 77 unsorted This is the result of one pass. ITC 250 Copyright 2008© ITC 19
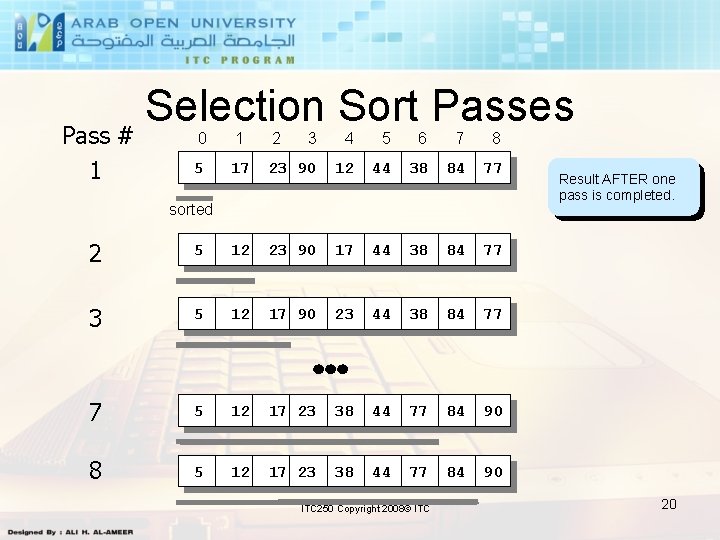
Pass # 1 Selection Sort Passes 0 1 2 3 4 5 6 7 8 5 17 23 90 12 44 38 84 77 sorted 2 5 12 23 90 17 44 38 84 77 3 5 12 17 90 23 44 38 84 77 7 5 12 17 23 38 44 77 84 90 8 5 12 17 23 38 44 77 84 90 ITC 250 Copyright 2008© ITC Result AFTER one pass is completed. 20
![Selection Sort Routine public void selection Sortint number int start Index min Index Selection Sort Routine public void selection. Sort(int[] number) { int start. Index, min. Index,](https://slidetodoc.com/presentation_image_h2/04ddf621b2a0e443b33d7b566dd18367/image-21.jpg)
Selection Sort Routine public void selection. Sort(int[] number) { int start. Index, min. Index, length, temp; length = number. length; for (start. Index = 0; start. Index <= length-2; start. Index++){ //each iteration of the for loop is one pass min. Index = start. Index; //find the smallest in this pass at position min. Index for (int i = start. Index+1; i <= length-1; i++) { if (number[i] < number[min. Index]) min. Index = i; } //exchange number[start. Index] and number[min. Index] temp = number[start. Index]; number[start. Index] = number[min. Index]; number[min. Index] = temp; } } ITC 250 Copyright 2008© ITC 21
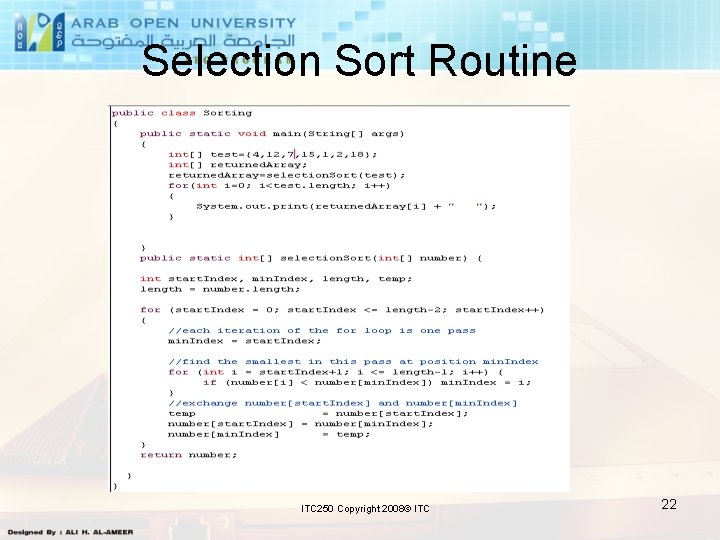
Selection Sort Routine ITC 250 Copyright 2008© ITC 22
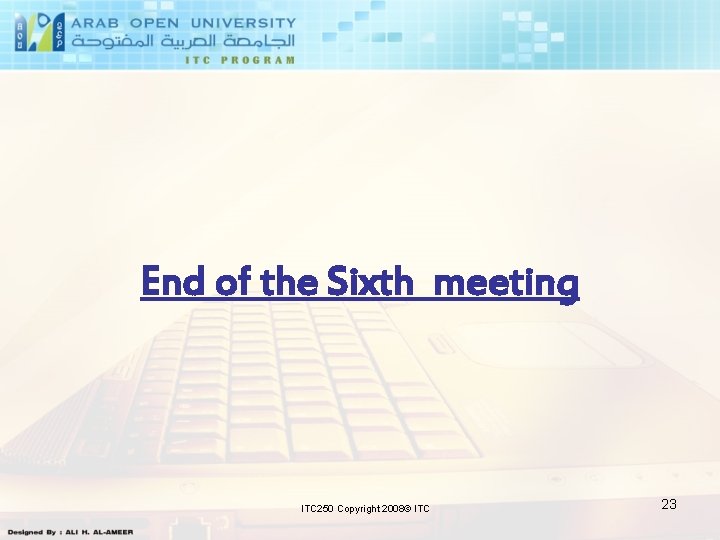
End of the Sixth meeting ITC 250 Copyright 2008© ITC 23
Itc-01 itc-02 itc-03 and itc-04
2008 2008
Copyright 2008
Copyright 2008
Copyright 2008
Copyright 2008
2008 pearson prentice hall inc
Copyright 2008
Copyright 2008
Coni retina
Itc vincenzo benini
Chamilo itc
Itc/2308/2007
Itc company vision and mission
Itc alessandrini montesilvano
Istituto ginanni ravenna
Scuola bodoni parma
Itc 103
Ilo turin courses
Federico cesi terni
Itc bitonto
Itc-icg 09
Itc logo
Bodoni parma