int tree Leaf Node Leaf 1 Leaf Node
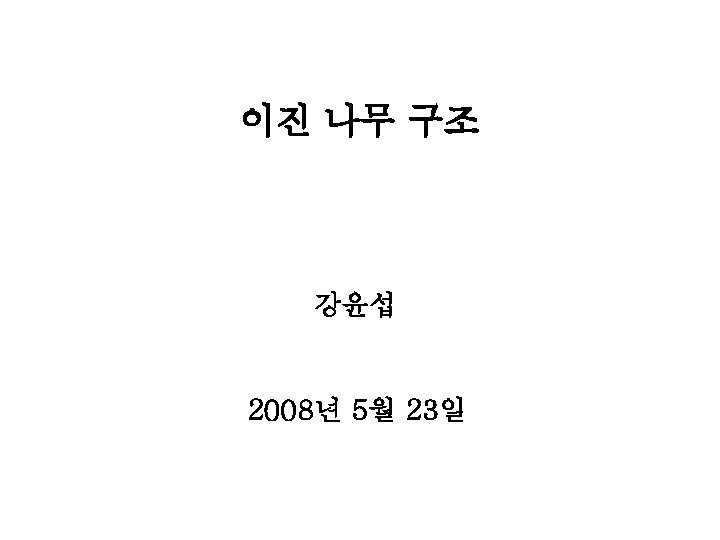
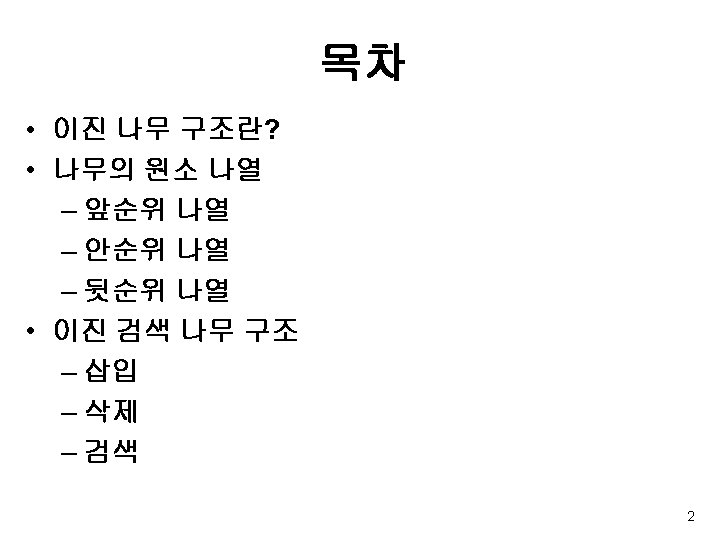
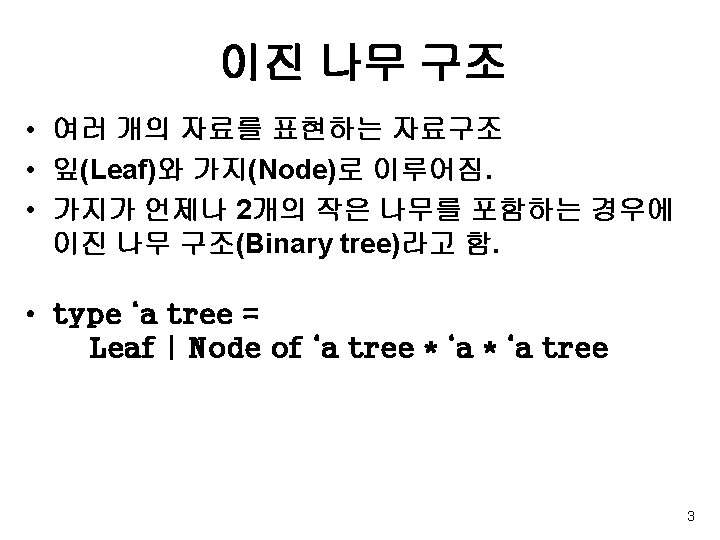
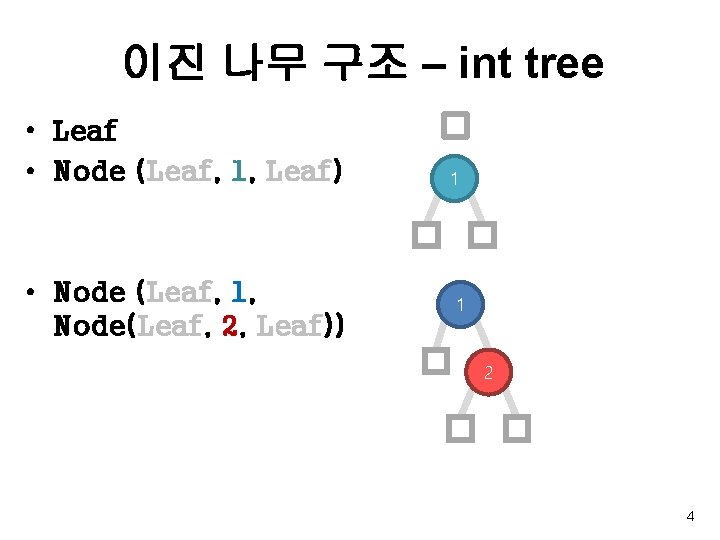
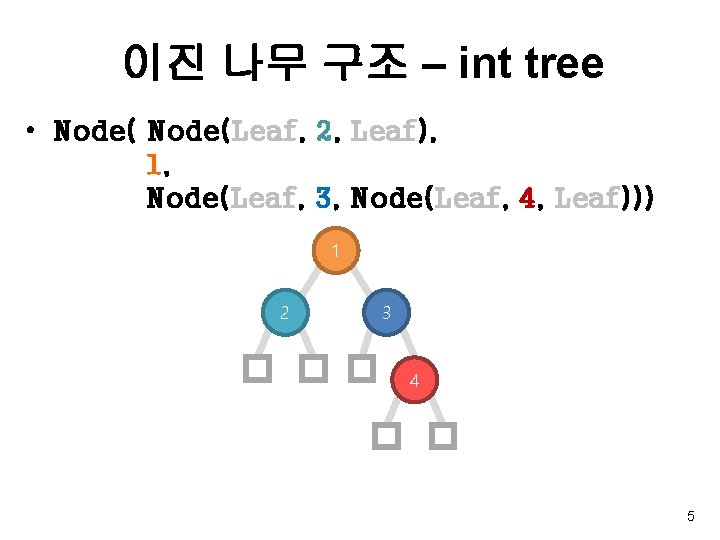
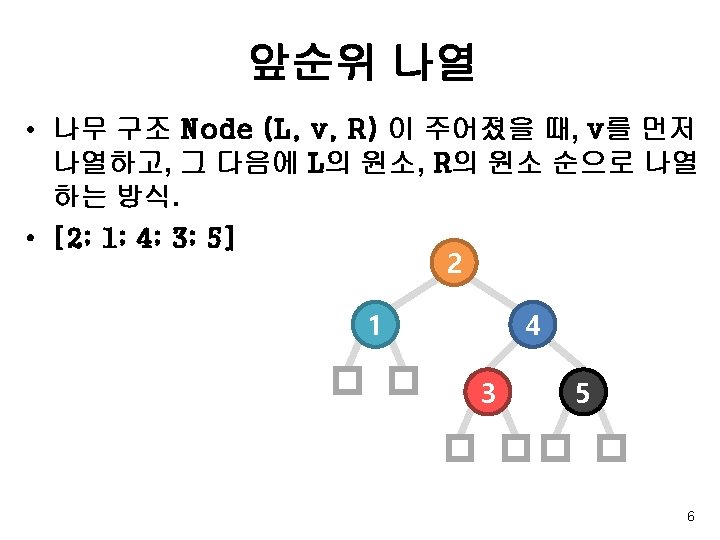
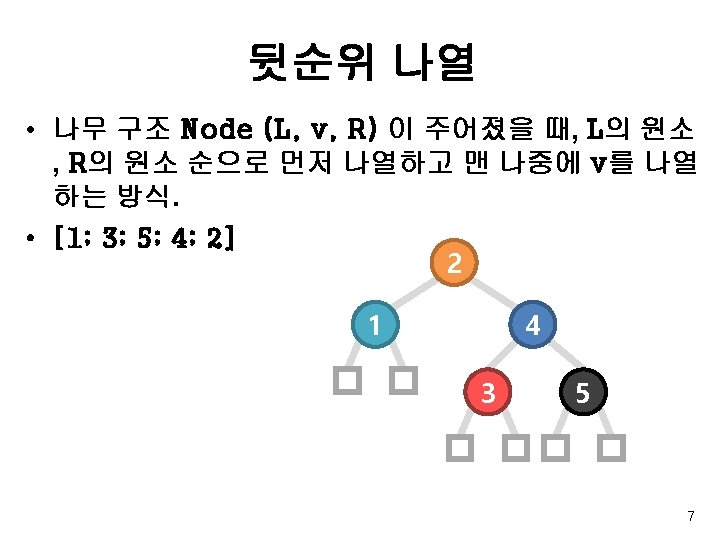
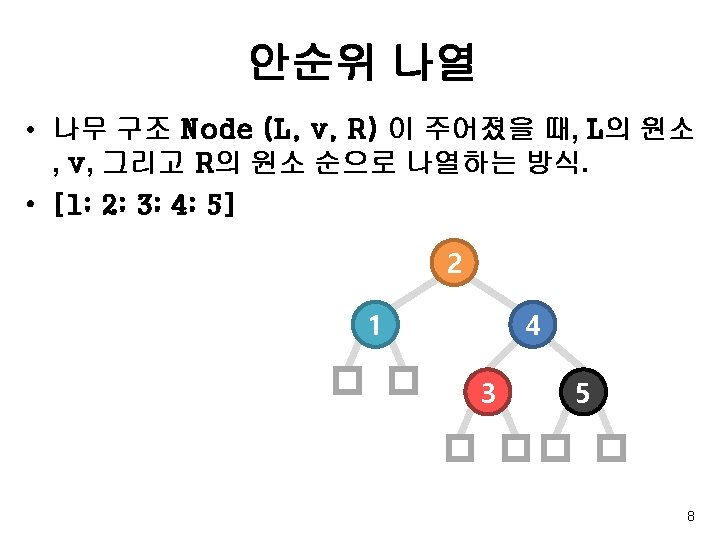
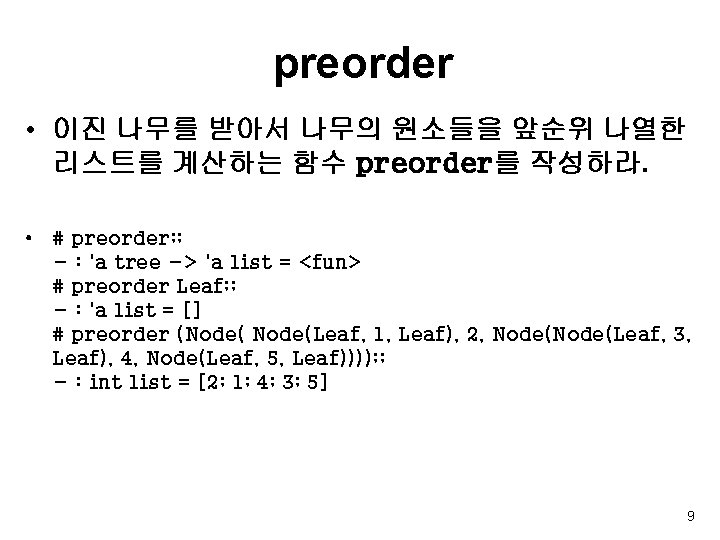
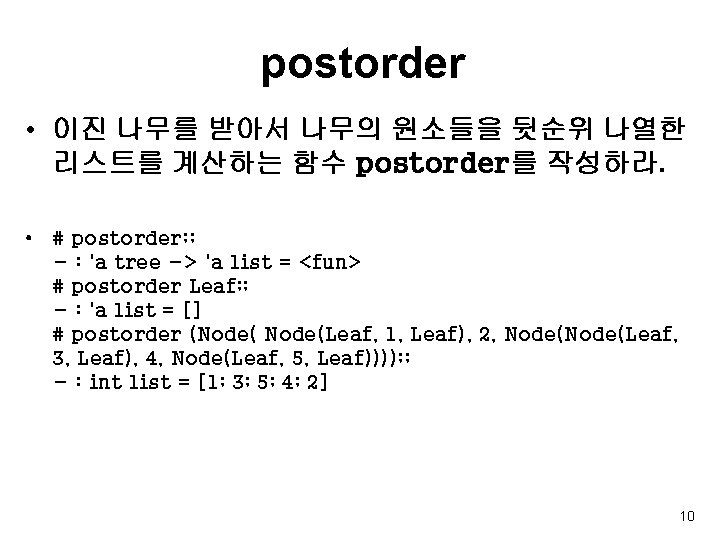
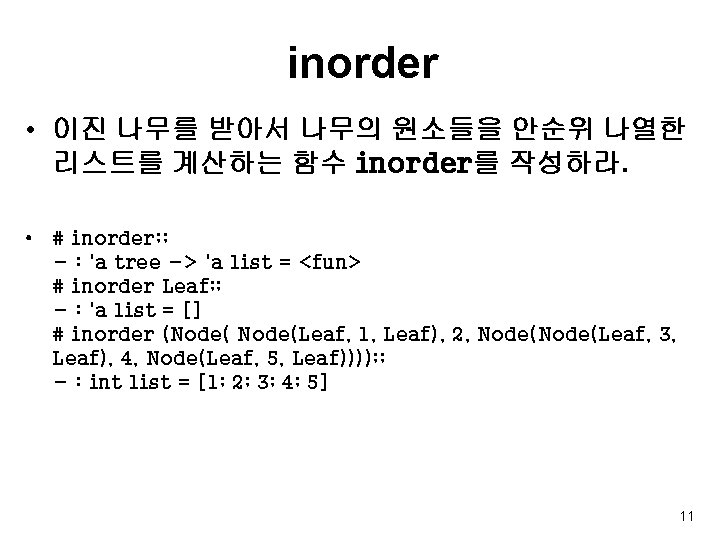
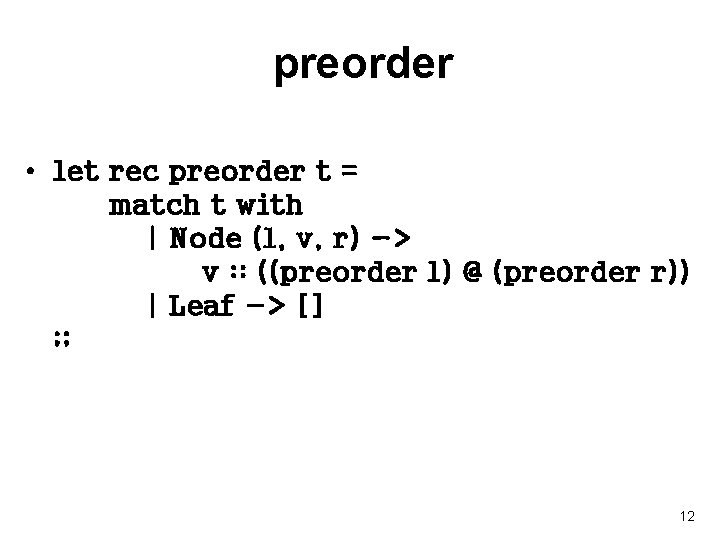
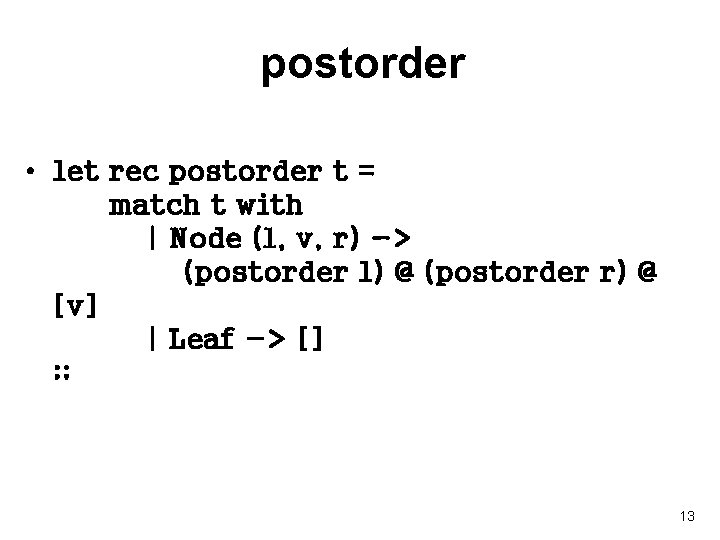
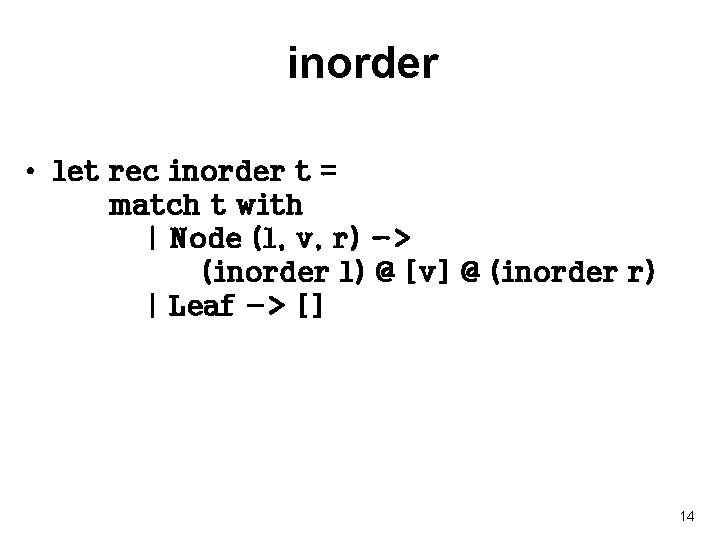
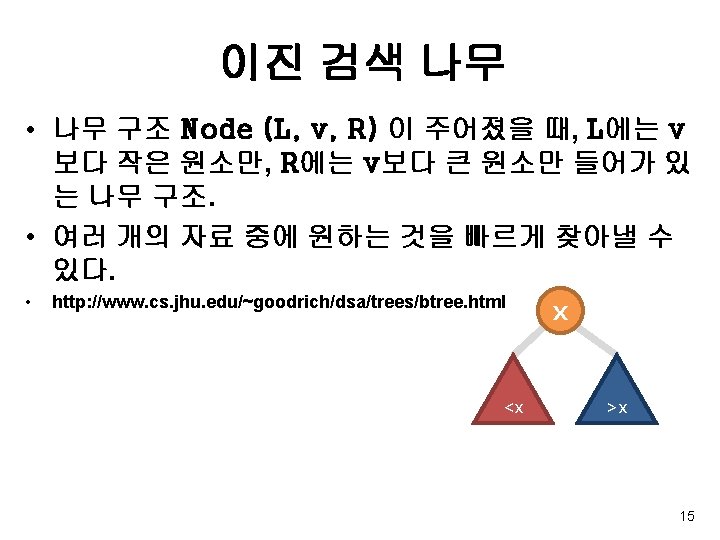
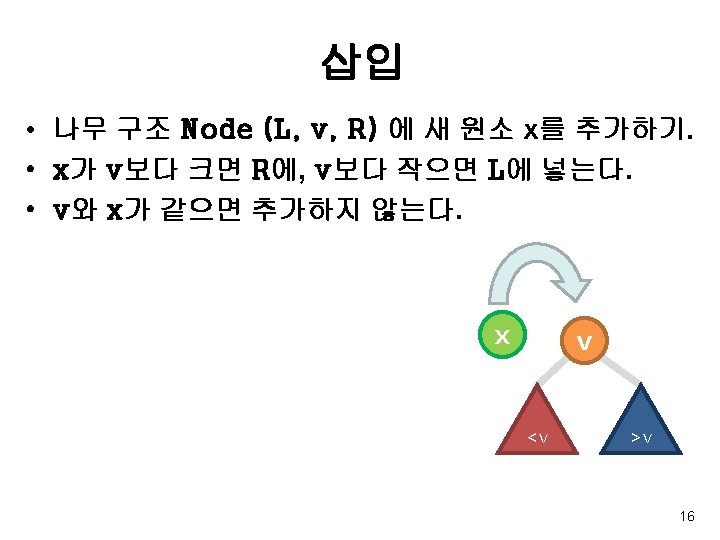
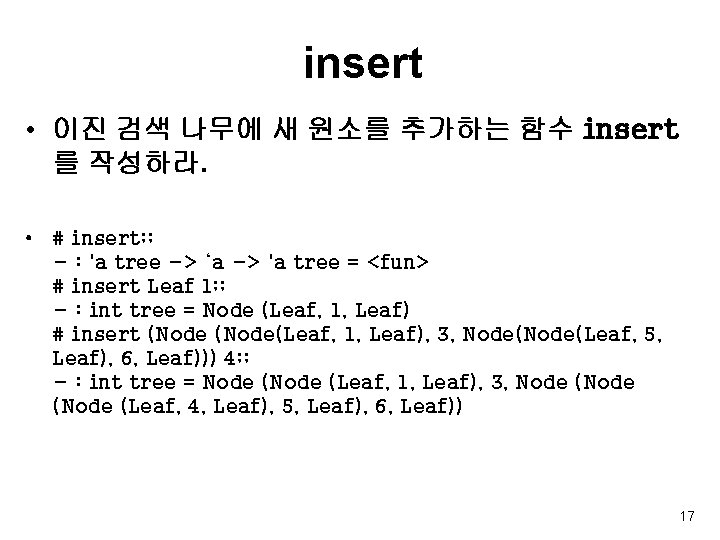
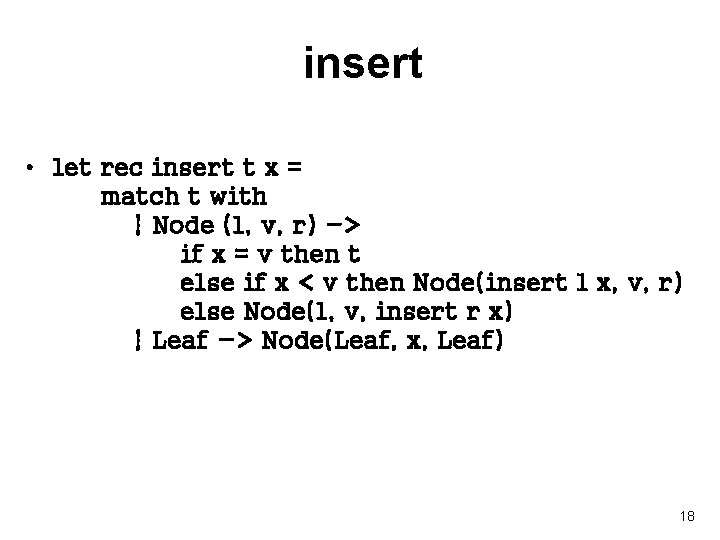
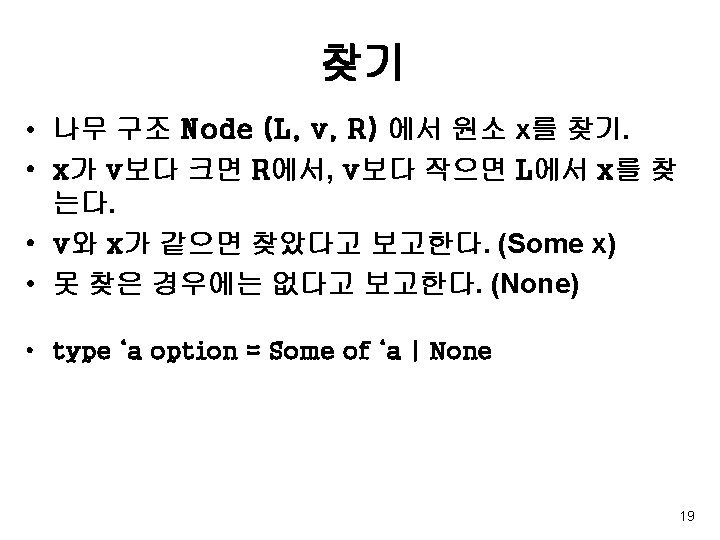
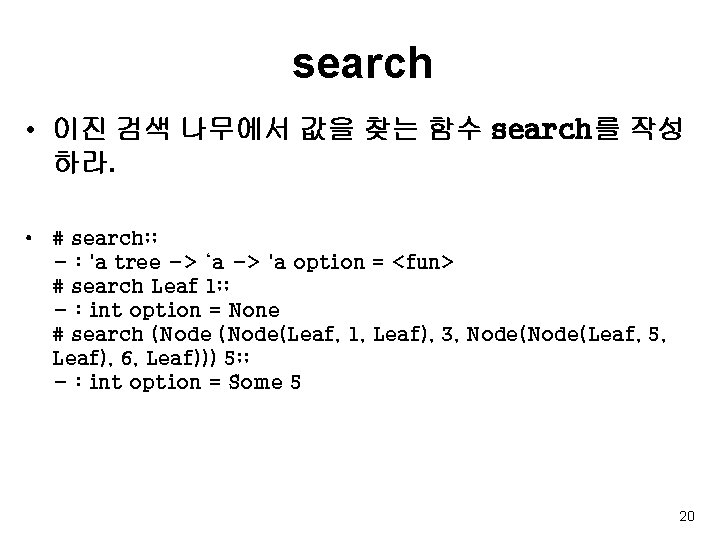
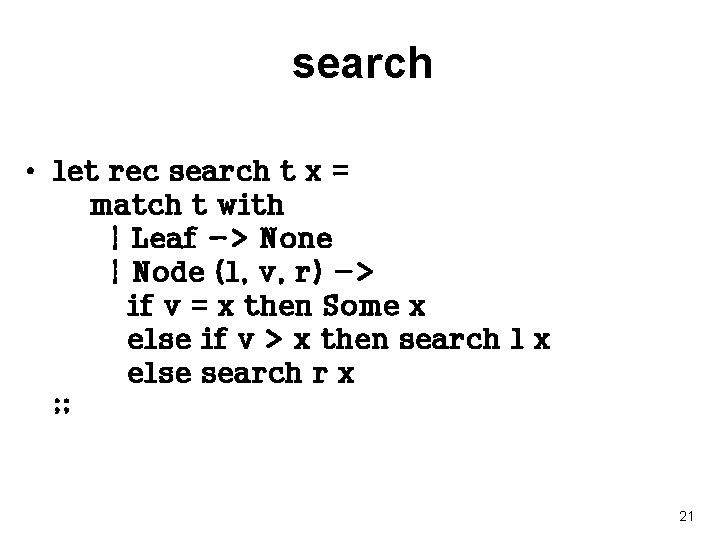
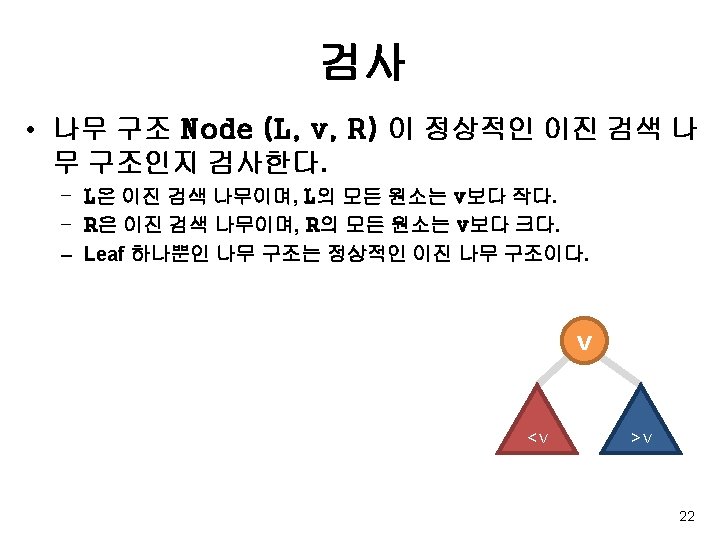
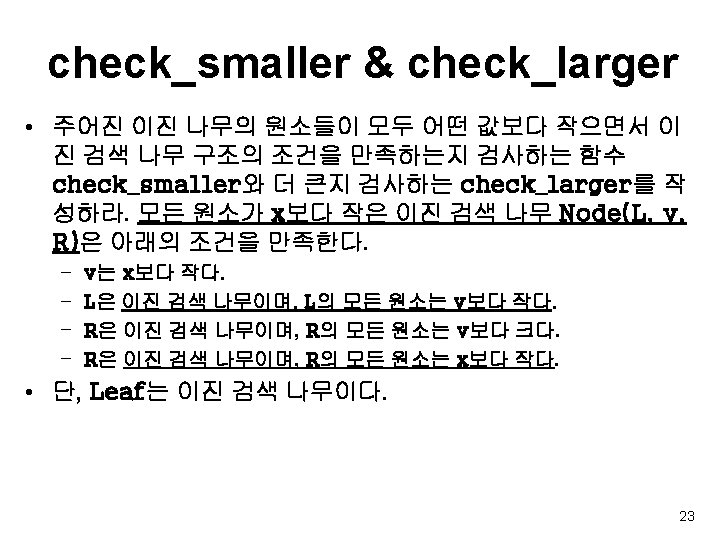
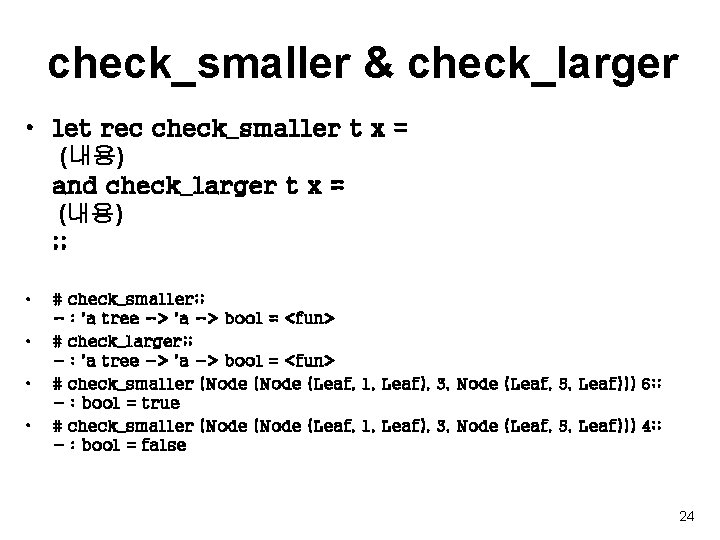
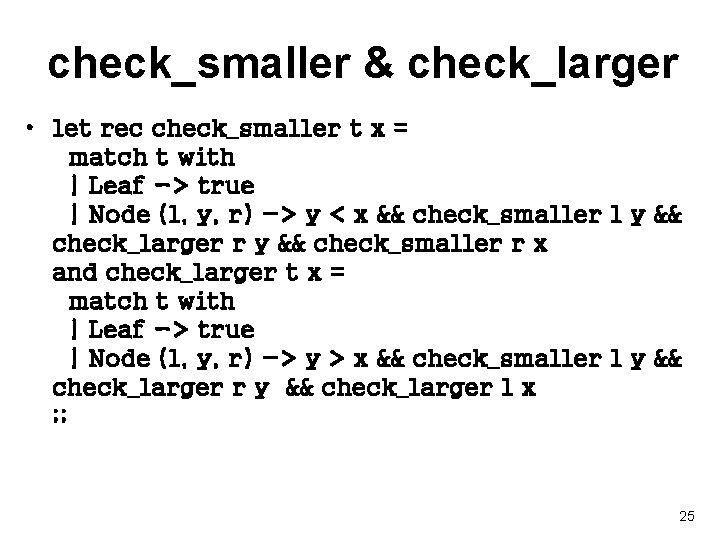
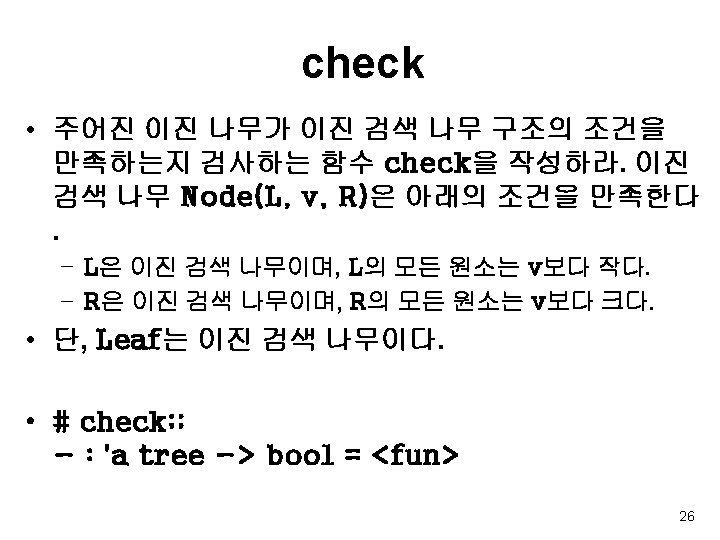
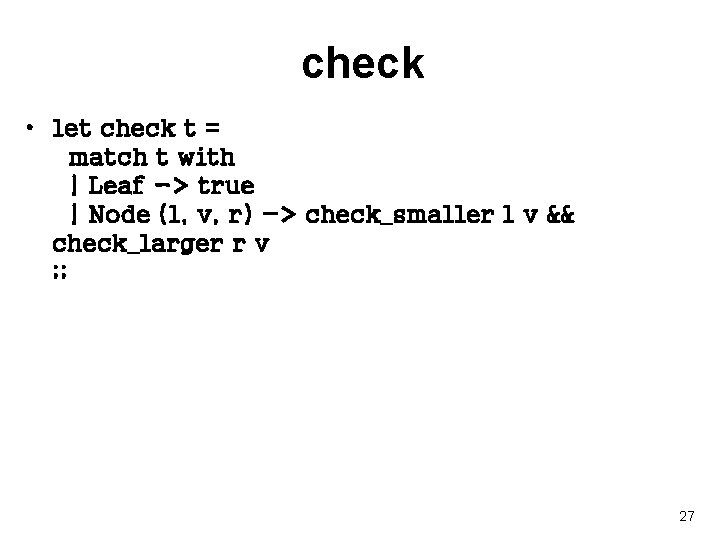
- Slides: 27
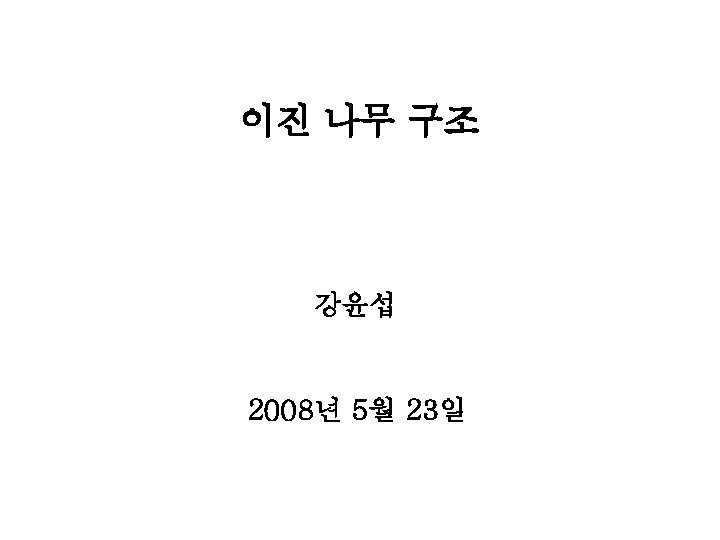
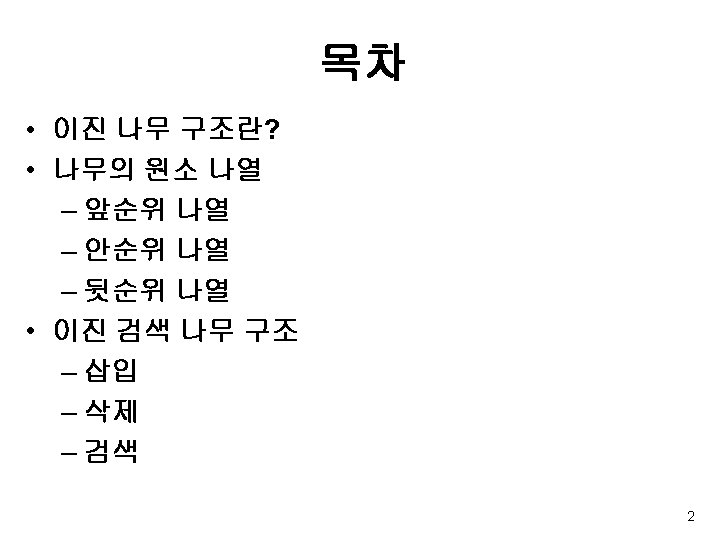
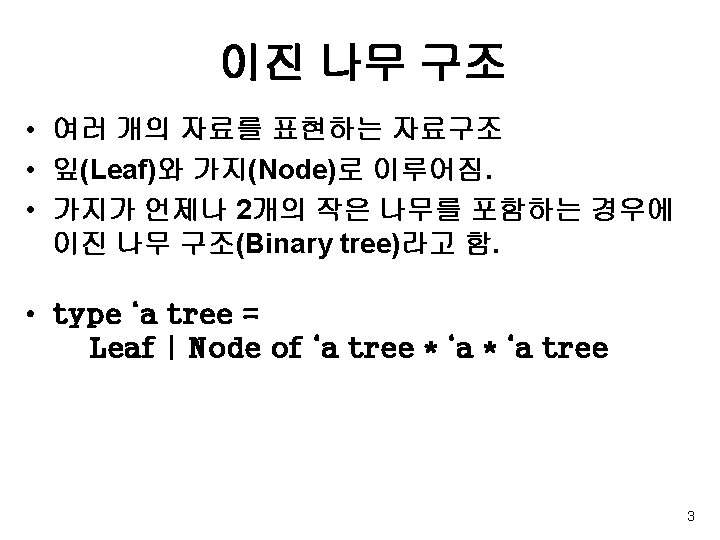
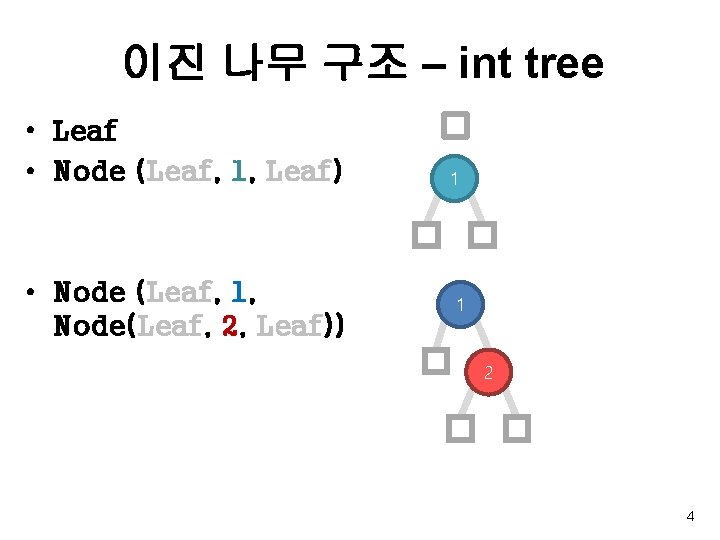
이진 나무 구조 – int tree • Leaf • Node (Leaf, 1, Leaf) • Node (Leaf, 1, Node(Leaf, 2, Leaf)) 1 1 2 4
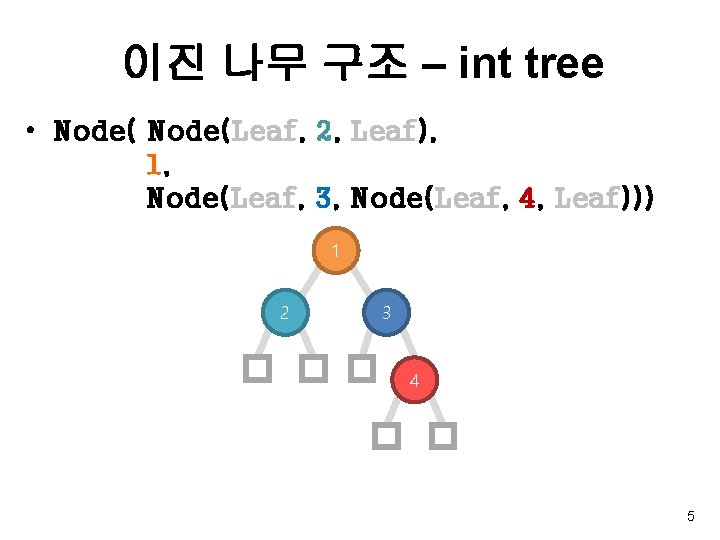
이진 나무 구조 – int tree • Node(Leaf, 2, Leaf), 1, Node(Leaf, 3, Node(Leaf, 4, Leaf))) 1 2 3 4 5
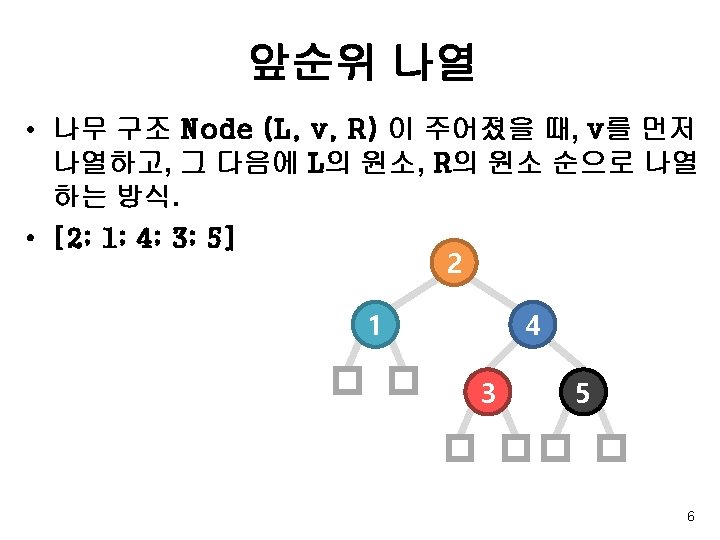
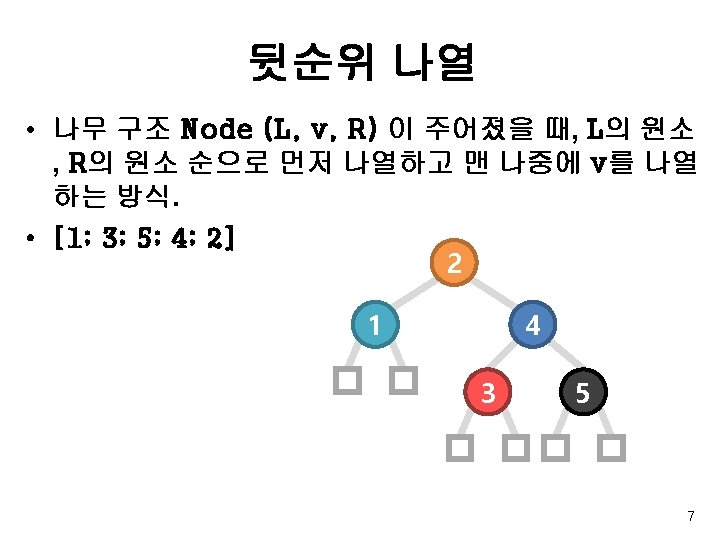
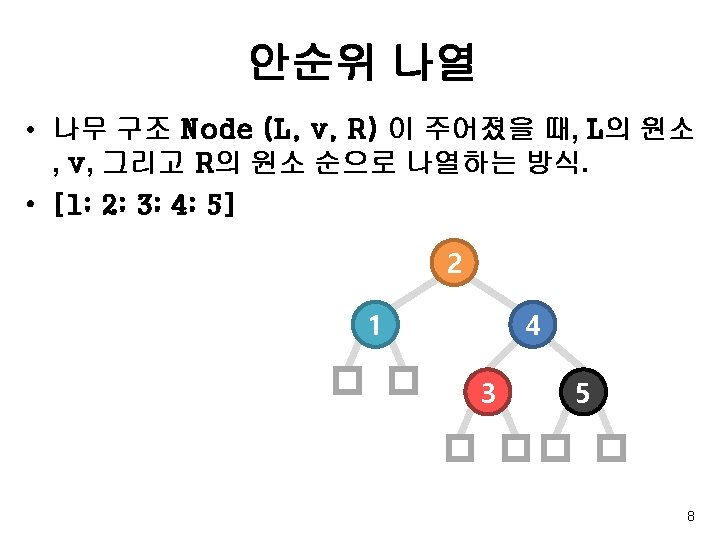
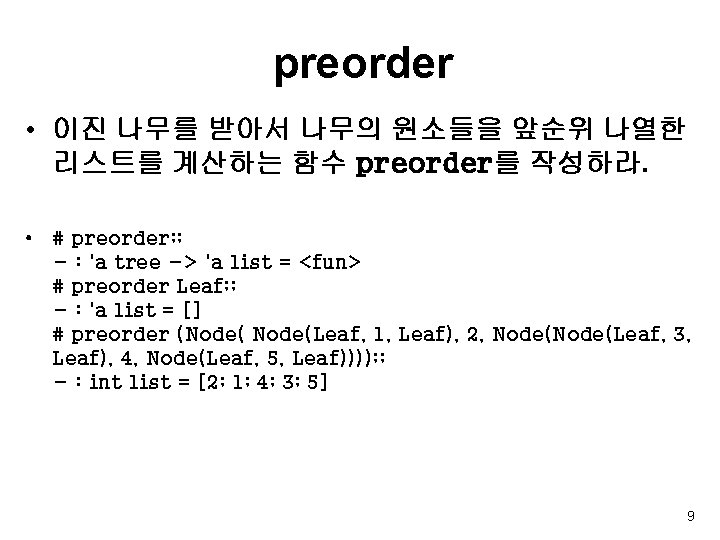
preorder • 이진 나무를 받아서 나무의 원소들을 앞순위 나열한 리스트를 계산하는 함수 preorder를 작성하라. • # preorder; ; - : 'a tree -> 'a list = <fun> # preorder Leaf; ; - : 'a list = [] # preorder (Node(Leaf, 1, Leaf), 2, Node(Leaf, 3, Leaf), 4, Node(Leaf, 5, Leaf)))); ; - : int list = [2; 1; 4; 3; 5] 9
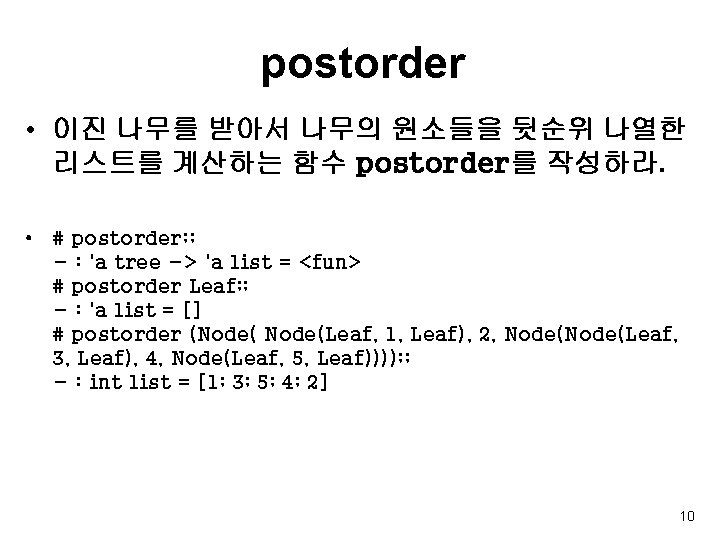
postorder • 이진 나무를 받아서 나무의 원소들을 뒷순위 나열한 리스트를 계산하는 함수 postorder를 작성하라. • # postorder; ; - : 'a tree -> 'a list = <fun> # postorder Leaf; ; - : 'a list = [] # postorder (Node(Leaf, 1, Leaf), 2, Node(Leaf, 3, Leaf), 4, Node(Leaf, 5, Leaf)))); ; - : int list = [1; 3; 5; 4; 2] 10
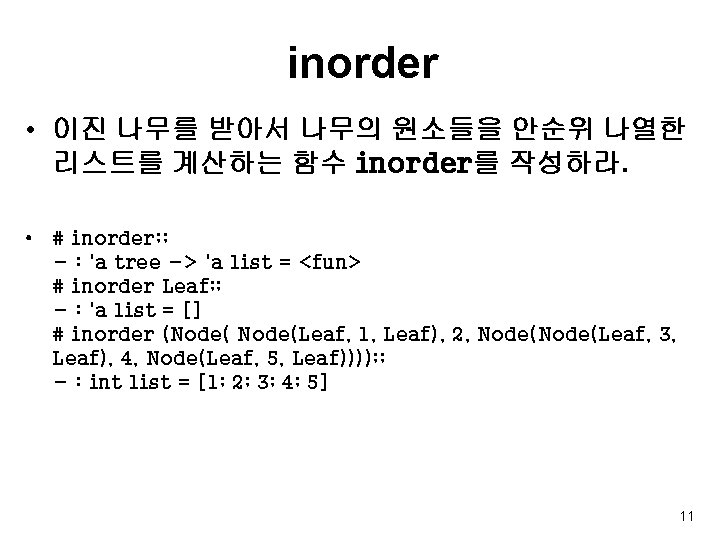
inorder • 이진 나무를 받아서 나무의 원소들을 안순위 나열한 리스트를 계산하는 함수 inorder를 작성하라. • # inorder; ; - : 'a tree -> 'a list = <fun> # inorder Leaf; ; - : 'a list = [] # inorder (Node(Leaf, 1, Leaf), 2, Node(Leaf, 3, Leaf), 4, Node(Leaf, 5, Leaf)))); ; - : int list = [1; 2; 3; 4; 5] 11
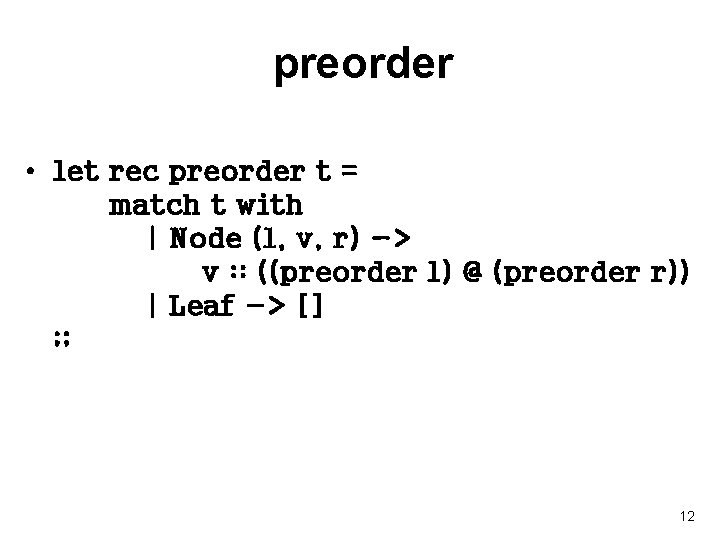
preorder • let rec preorder t = match t with | Node (l, v, r) -> v : : ((preorder l) @ (preorder r)) | Leaf -> [] ; ; 12
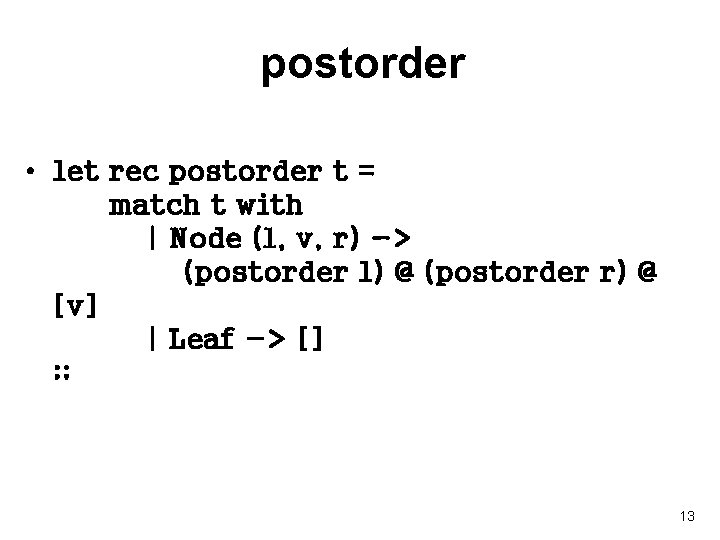
postorder • let rec postorder t = match t with | Node (l, v, r) -> (postorder l) @ (postorder r) @ [v] | Leaf -> [] ; ; 13
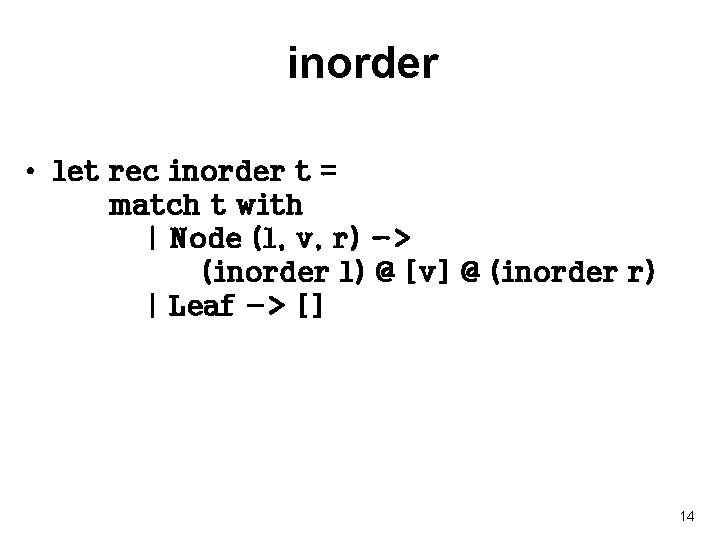
inorder • let rec inorder t = match t with | Node (l, v, r) -> (inorder l) @ [v] @ (inorder r) | Leaf -> [] 14
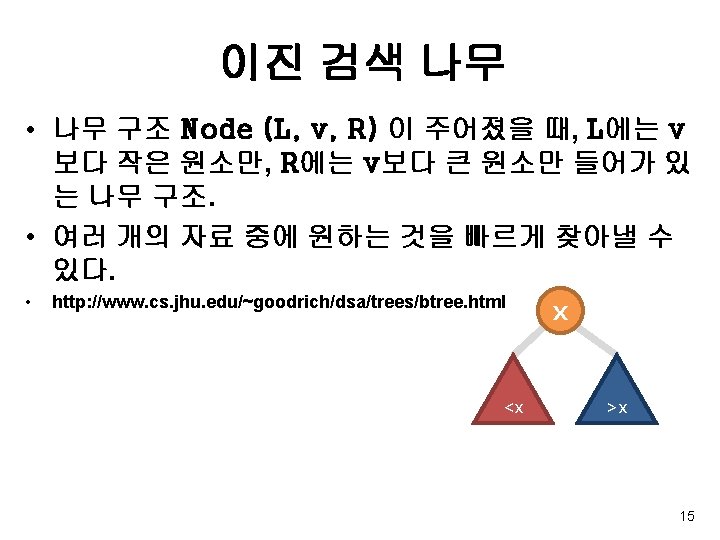
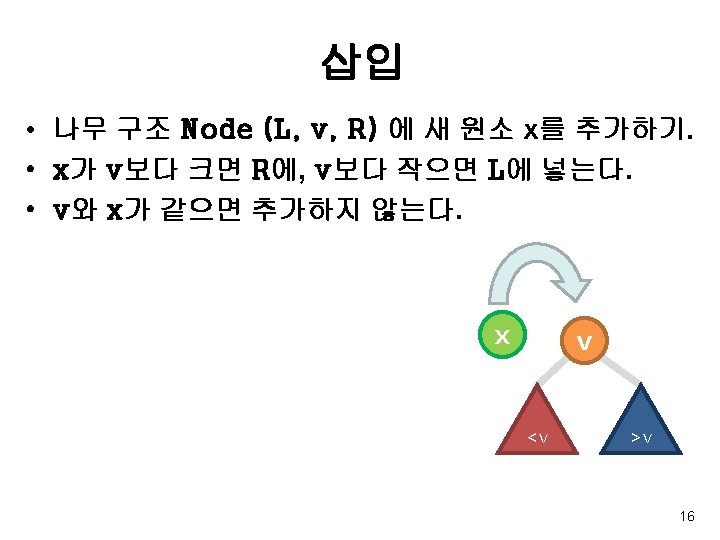
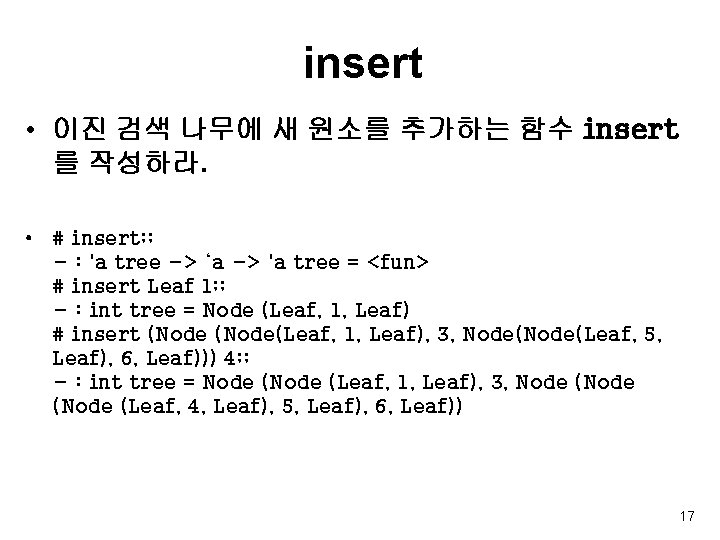
insert • 이진 검색 나무에 새 원소를 추가하는 함수 insert 를 작성하라. • # insert; ; - : 'a tree -> ‘a -> 'a tree = <fun> # insert Leaf 1; ; - : int tree = Node (Leaf, 1, Leaf) # insert (Node(Leaf, 1, Leaf), 3, Node(Leaf, 5, Leaf), 6, Leaf))) 4; ; - : int tree = Node (Leaf, 1, Leaf), 3, Node (Node (Leaf, 4, Leaf), 5, Leaf), 6, Leaf)) 17
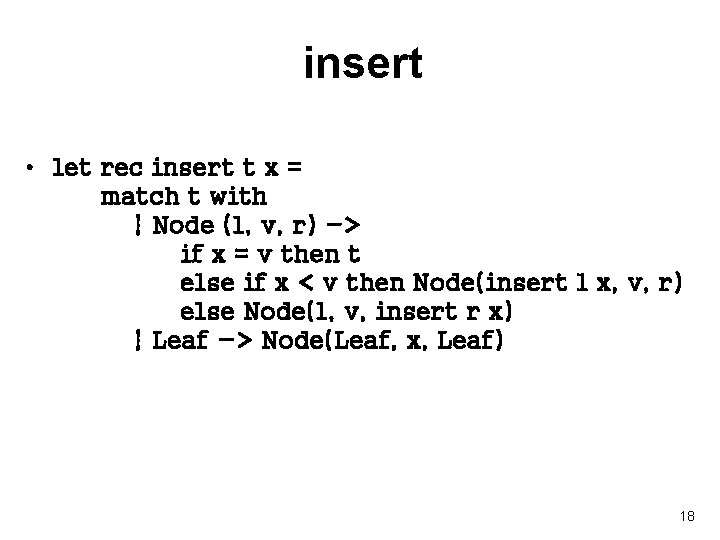
insert • let rec insert t x = match t with | Node (l, v, r) -> if x = v then t else if x < v then Node(insert l x, v, r) else Node(l, v, insert r x) | Leaf -> Node(Leaf, x, Leaf) 18
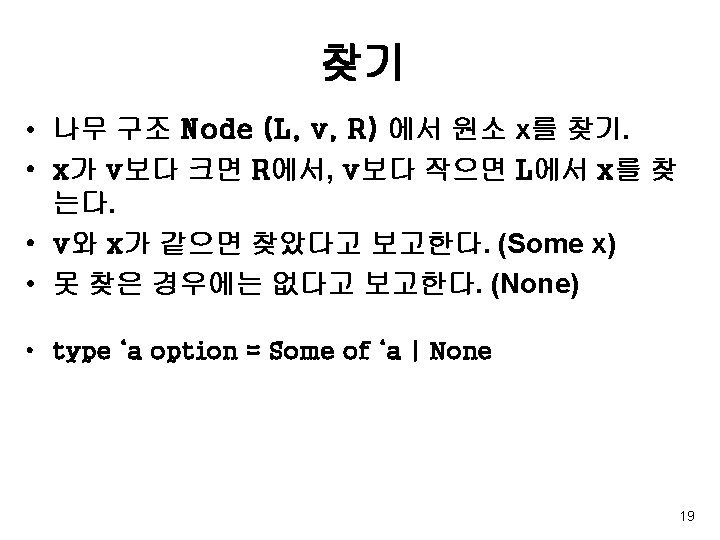
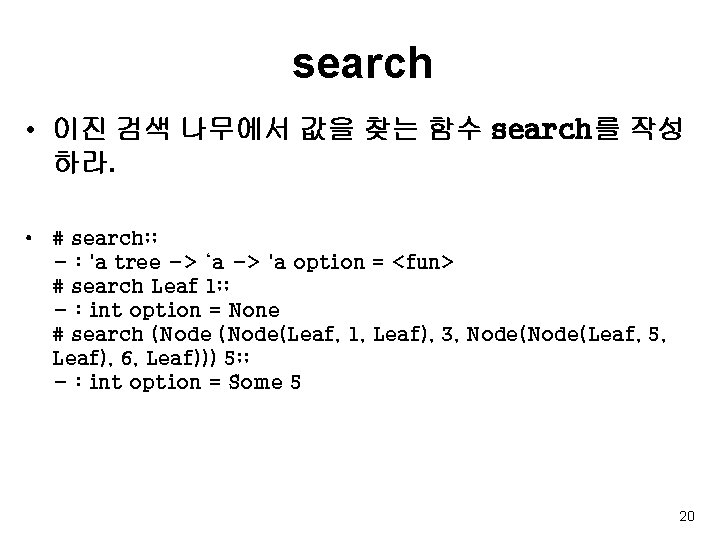
search • 이진 검색 나무에서 값을 찾는 함수 search를 작성 하라. • # search; ; - : 'a tree -> ‘a -> 'a option = <fun> # search Leaf 1; ; - : int option = None # search (Node(Leaf, 1, Leaf), 3, Node(Leaf, 5, Leaf), 6, Leaf))) 5; ; - : int option = Some 5 20
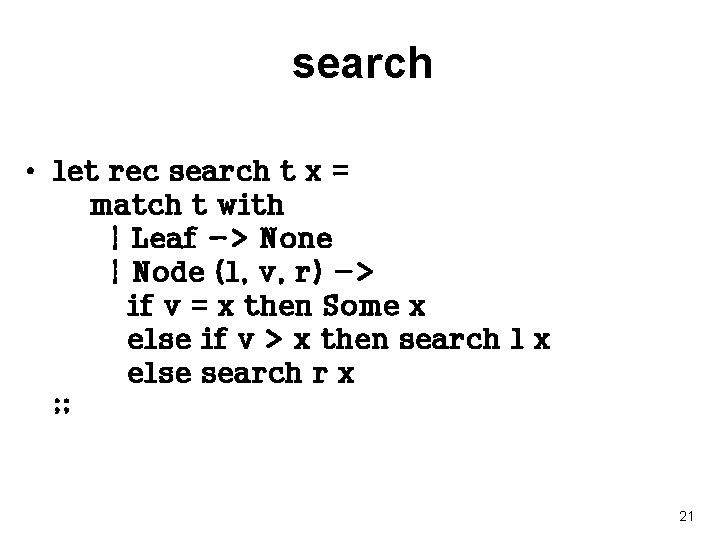
search • let rec search t x = match t with | Leaf -> None | Node (l, v, r) -> if v = x then Some x else if v > x then search l x else search r x ; ; 21
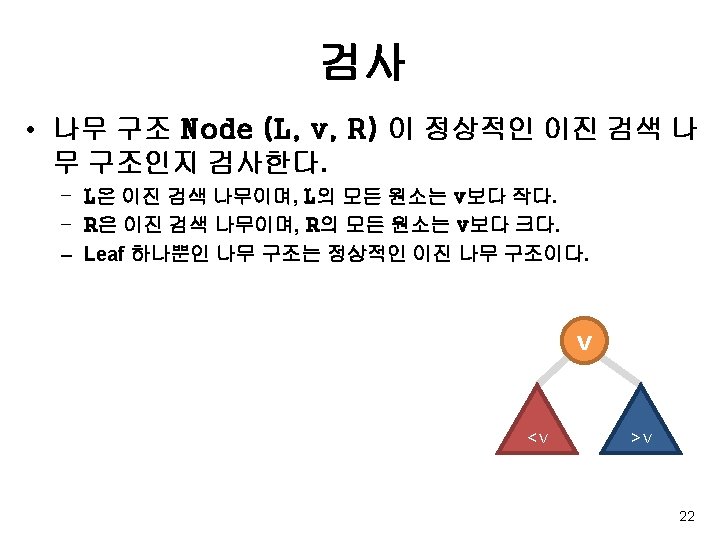
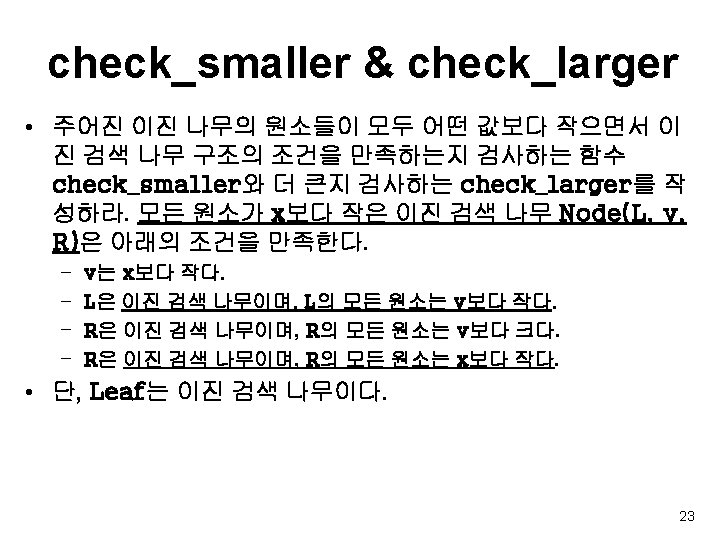
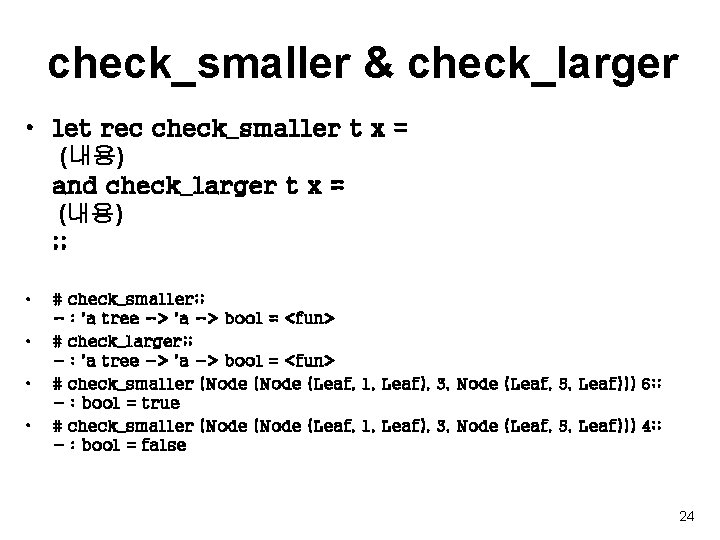
check_smaller & check_larger • let rec check_smaller t x = (내용) and check_larger t x = (내용) ; ; • • # check_smaller; ; - : 'a tree -> 'a -> bool = <fun> # check_larger; ; - : 'a tree -> 'a -> bool = <fun> # check_smaller (Node (Leaf, 1, Leaf), 3, Node (Leaf, 5, Leaf))) 6; ; - : bool = true # check_smaller (Node (Leaf, 1, Leaf), 3, Node (Leaf, 5, Leaf))) 4; ; - : bool = false 24
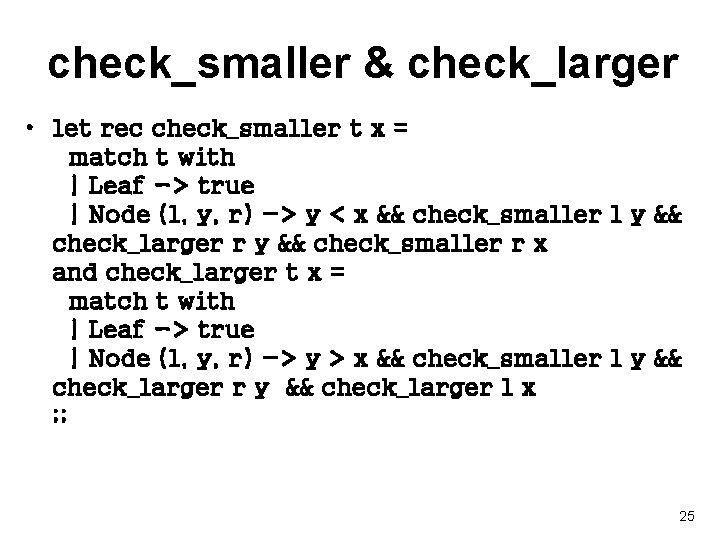
check_smaller & check_larger • let rec check_smaller t x = match t with | Leaf -> true | Node (l, y, r) -> y < x && check_smaller l y && check_larger r y && check_smaller r x and check_larger t x = match t with | Leaf -> true | Node (l, y, r) -> y > x && check_smaller l y && check_larger r y && check_larger l x ; ; 25
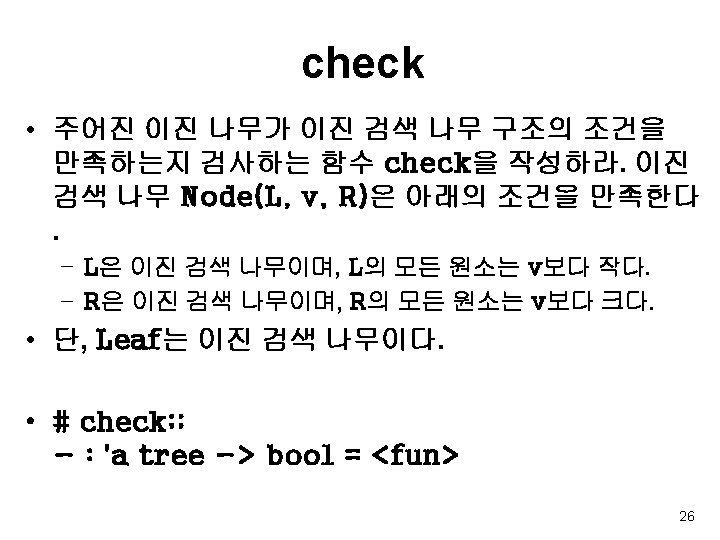
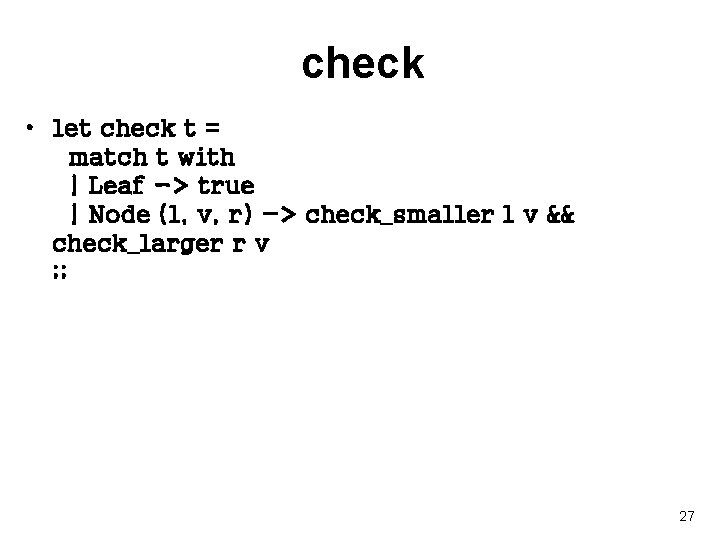
check • let check t = match t with | Leaf -> true | Node (l, v, r) -> check_smaller l v && check_larger r v ; ; 27
Sum0
Divideint
Int max(int x int y)
Inheritance calculator
Public void drawsquare(int x, int y, int len)
Struct node int data struct node* next
Char argv
Int.max
Int main int argc char argv
Int main int num 4
Void swap
Int factorial(int n)
Int f(int n)
Interface myinterface int foo(int x)
Const int arduino adalah
Struct node int i float j
Typedef struct in c
Struct node int data
Struct node int i float j
Typedef struct node node
Reference node and non reference node
Parallel spin
Reference node and non reference node
Constructive interference of standing waves
Nodeleaf
Maple leaf and oak leaf homologous
Leaf and non leaf procedure