Inheritance Overview Inheritance polymorphism inheriting method definitions overriding
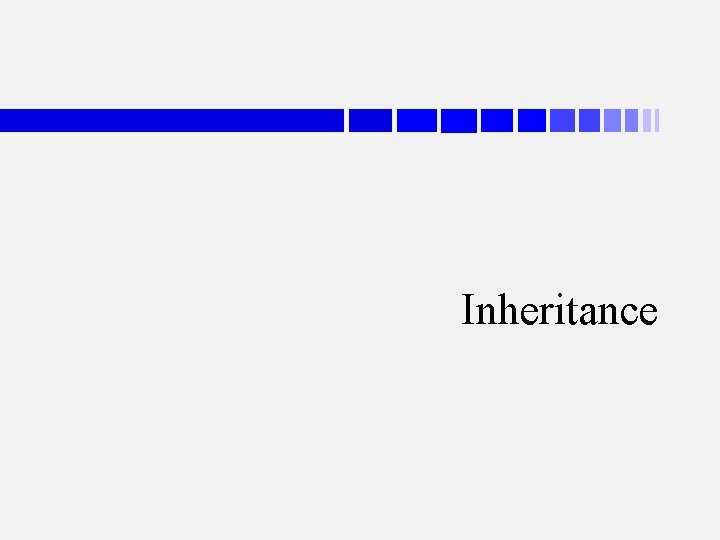
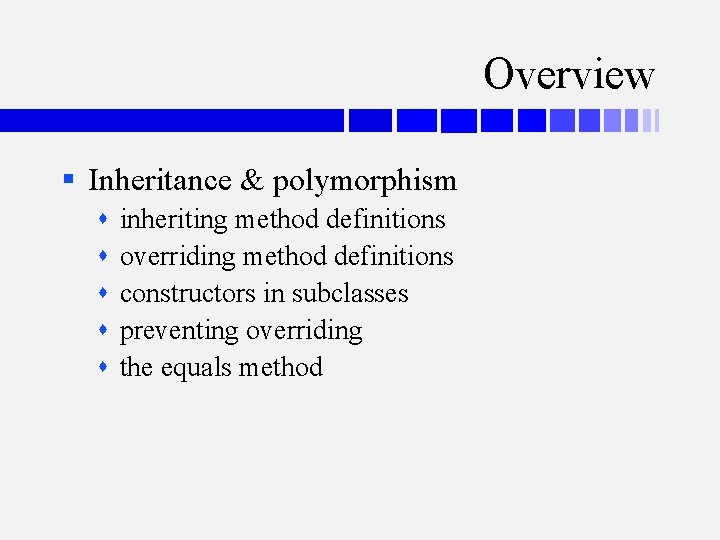
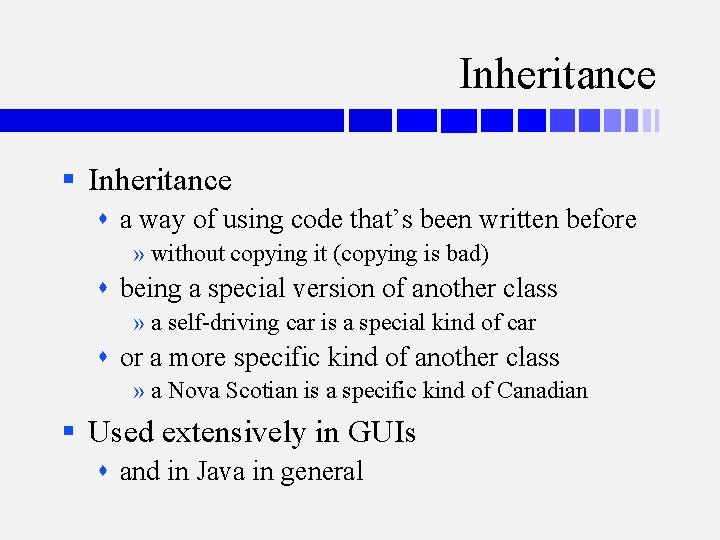
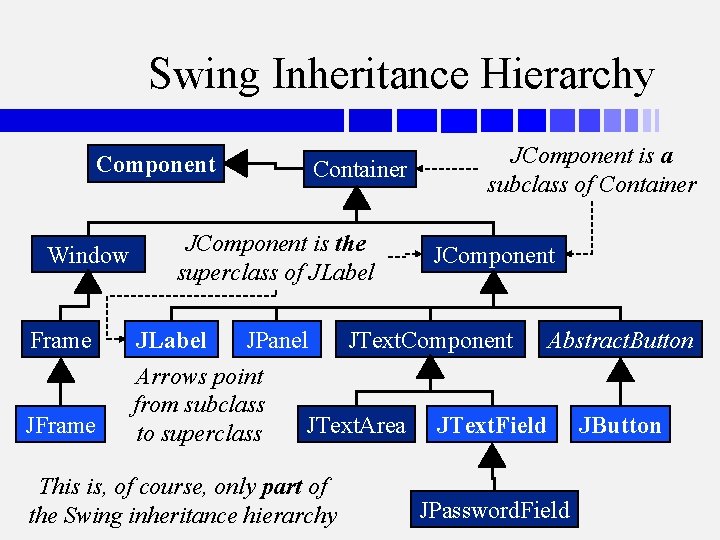
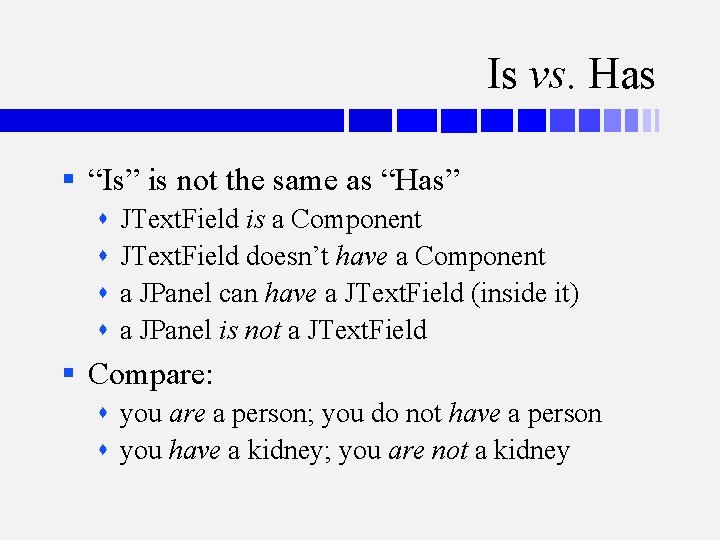
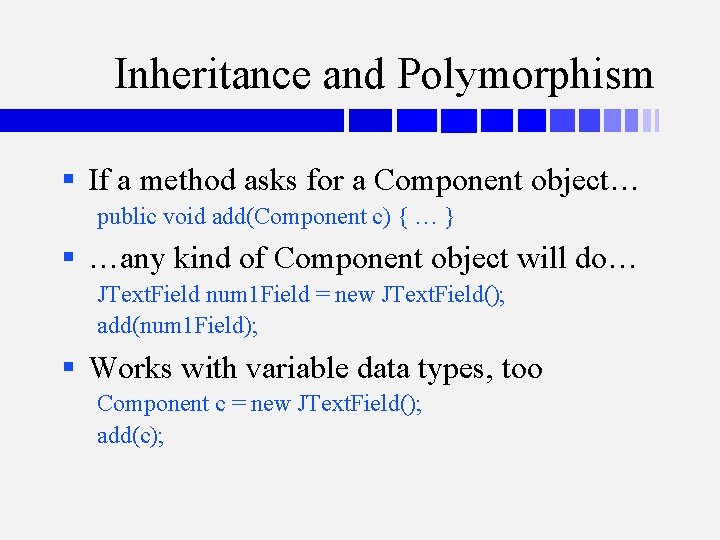
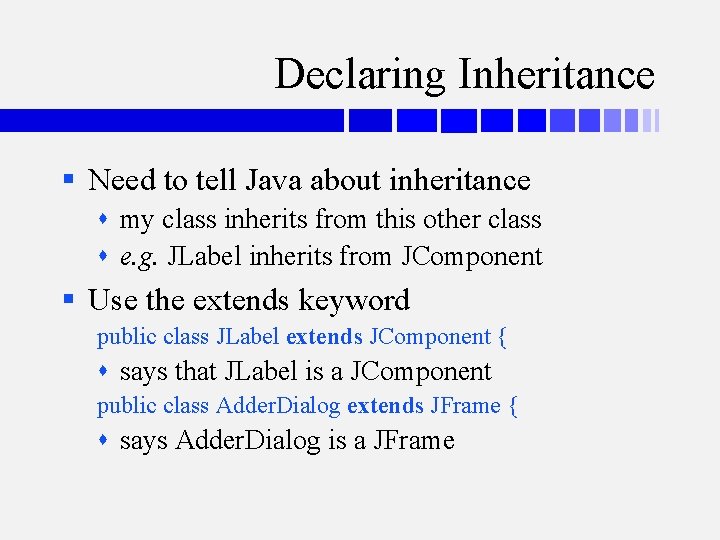
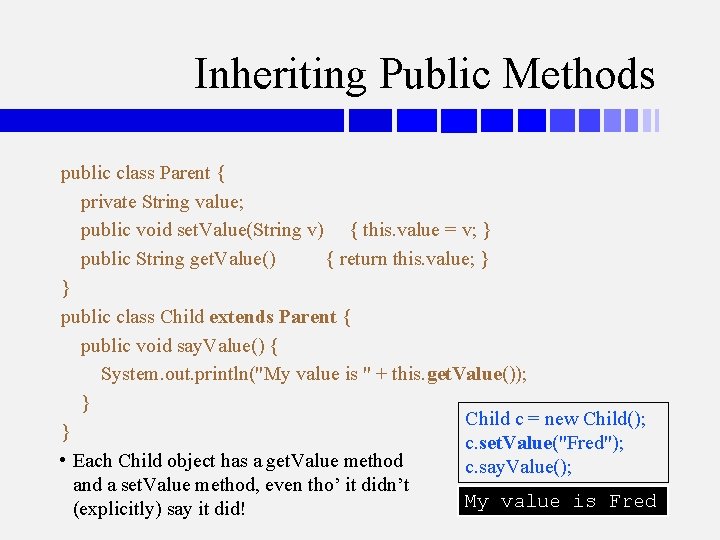
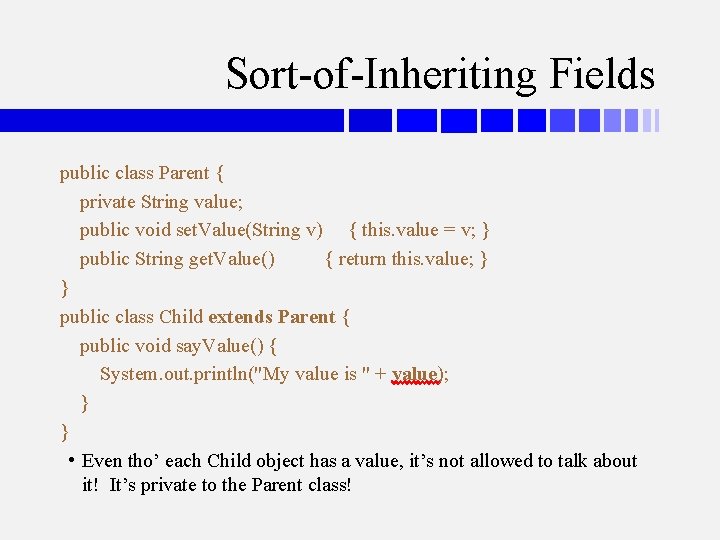
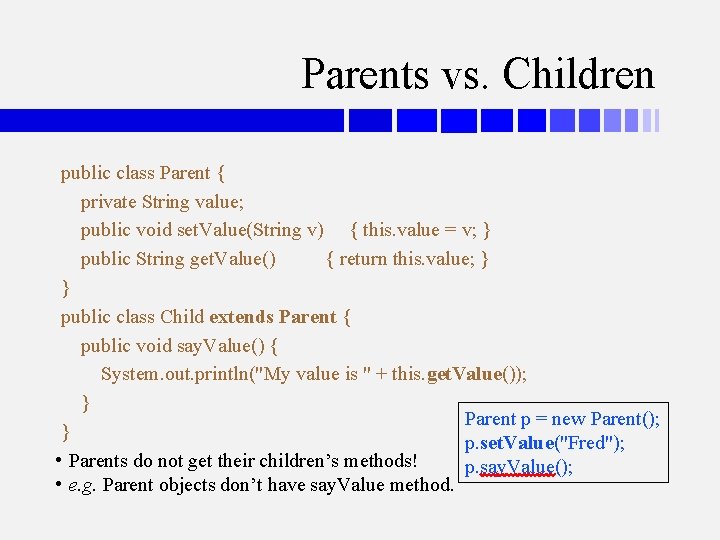
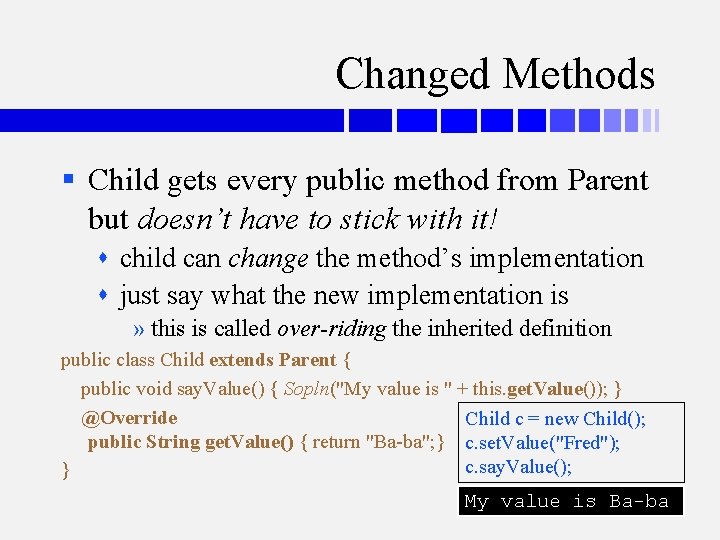
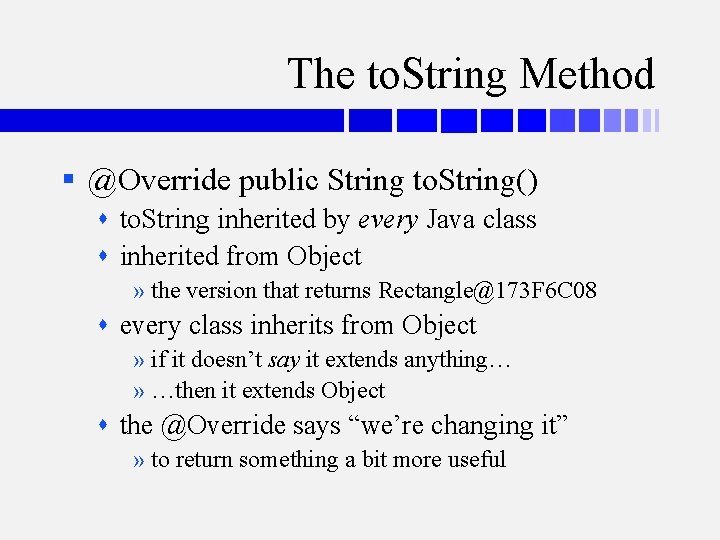
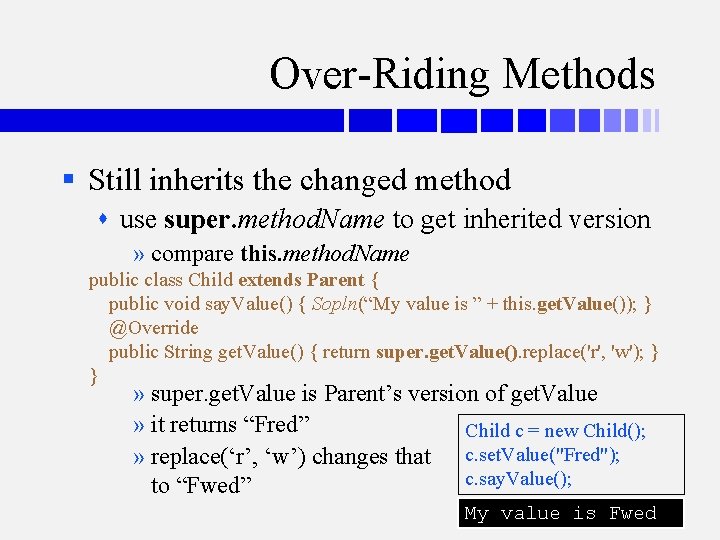
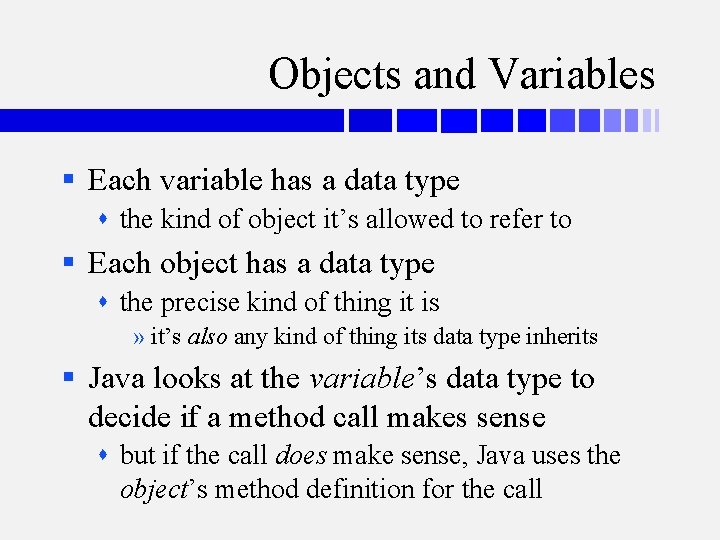
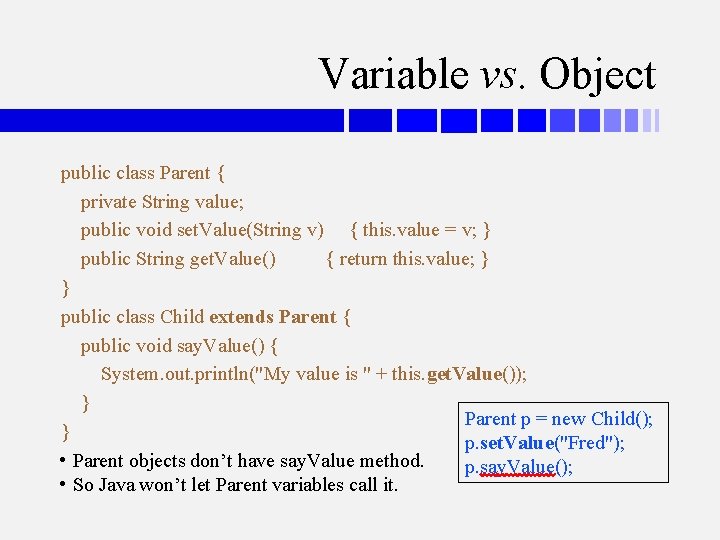
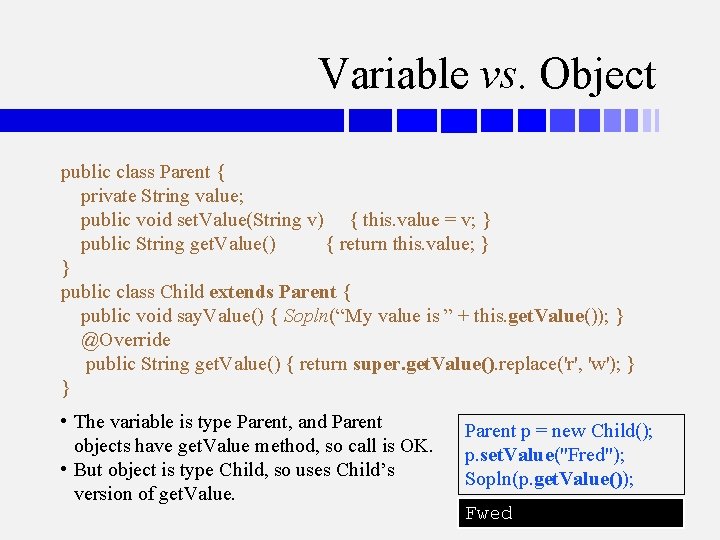
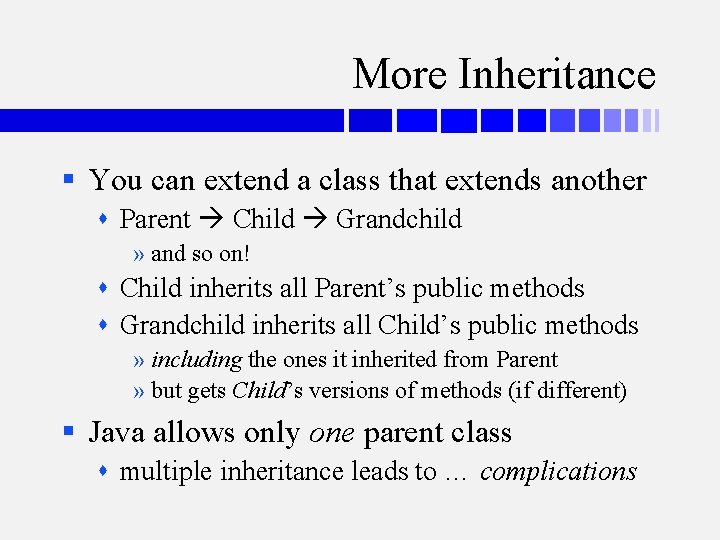
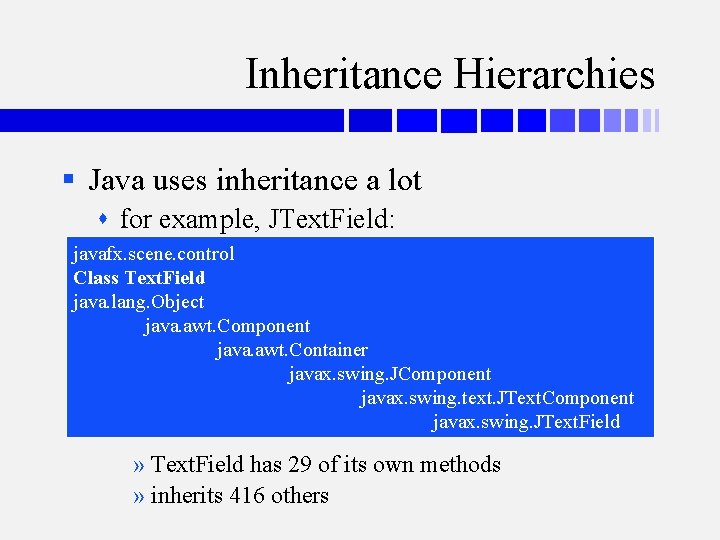
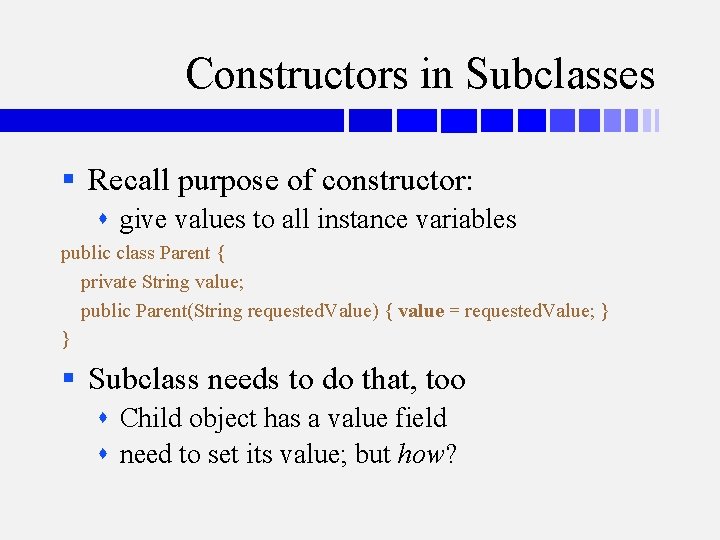
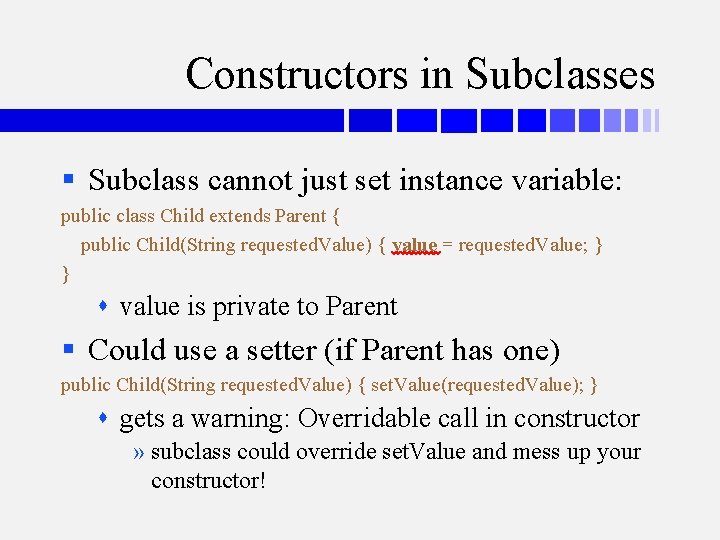
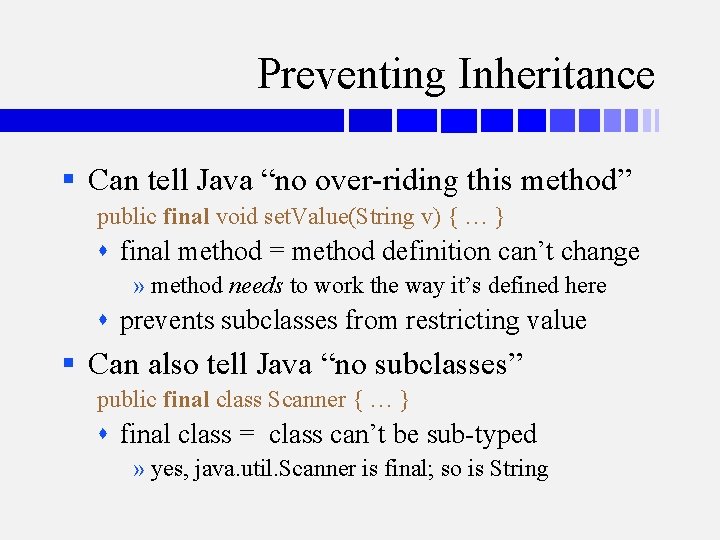
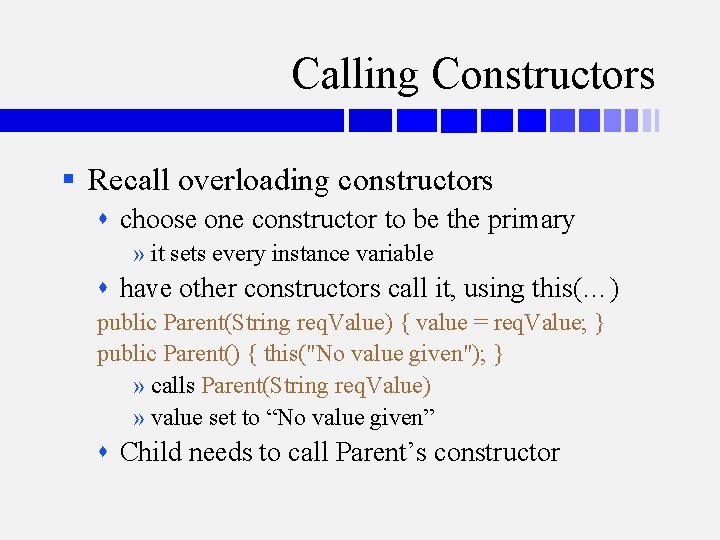
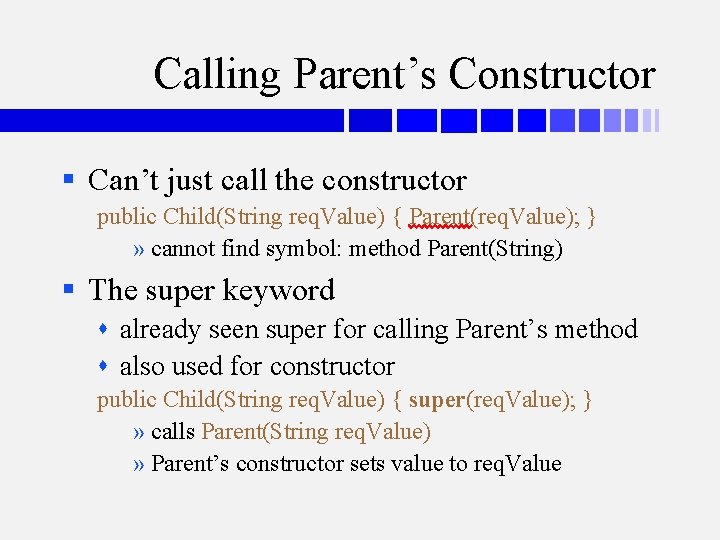
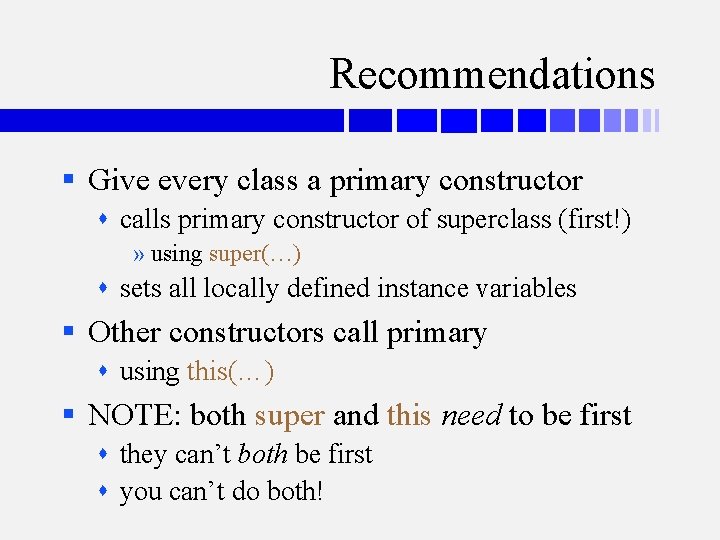
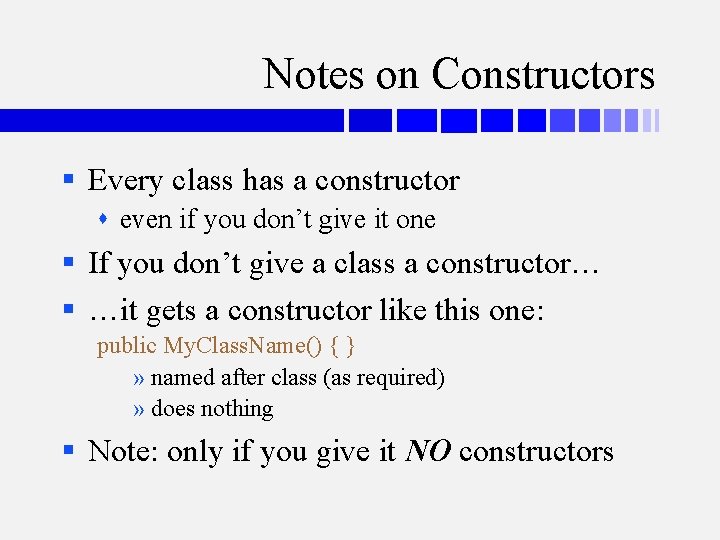
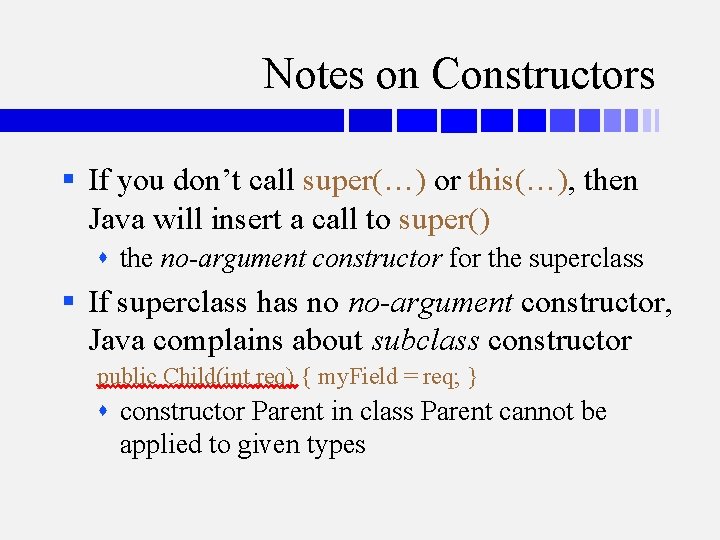
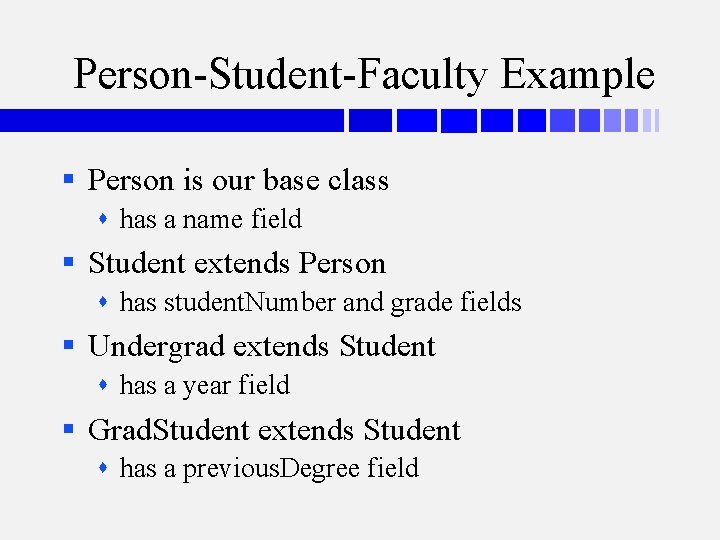
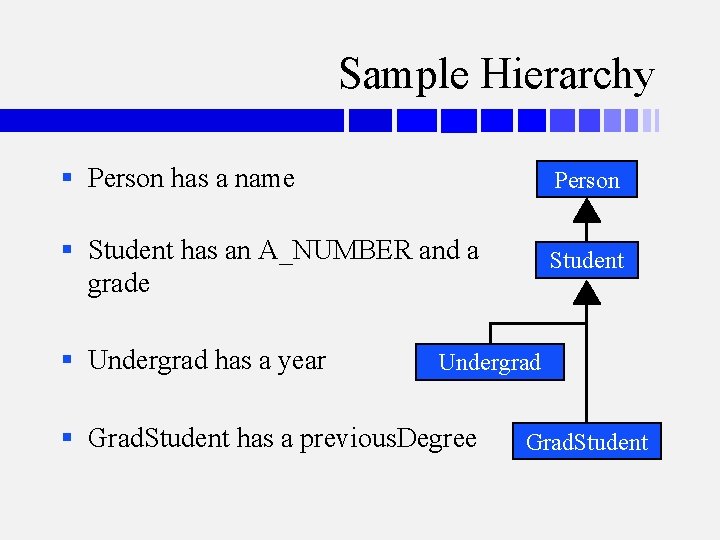
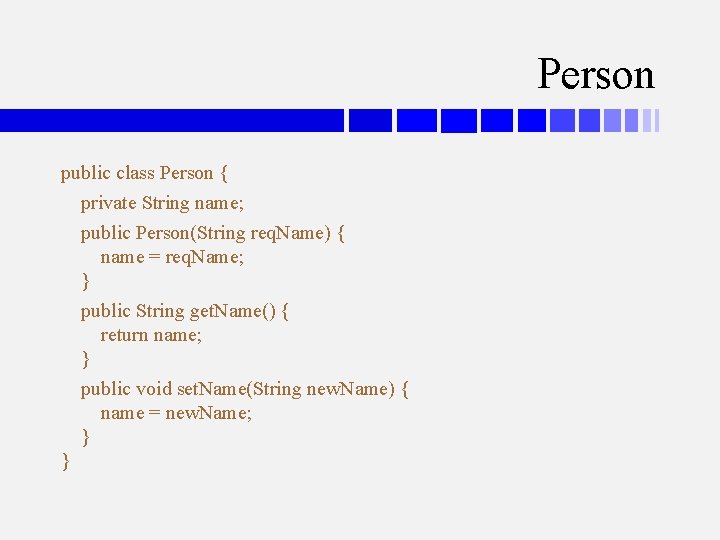
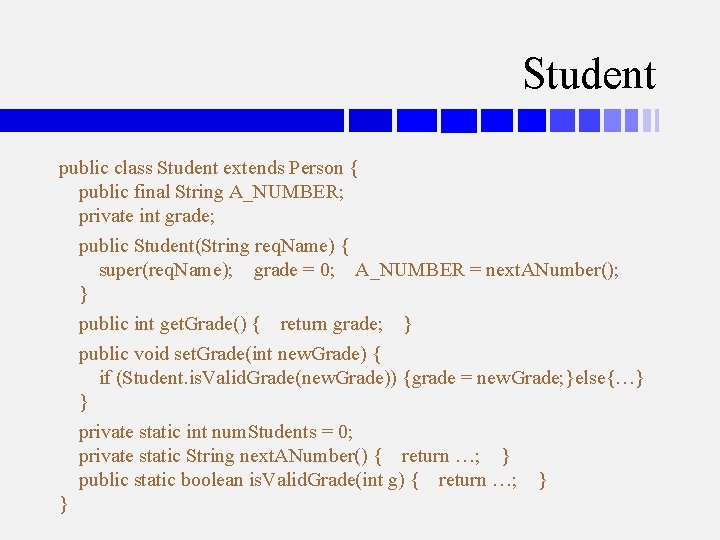
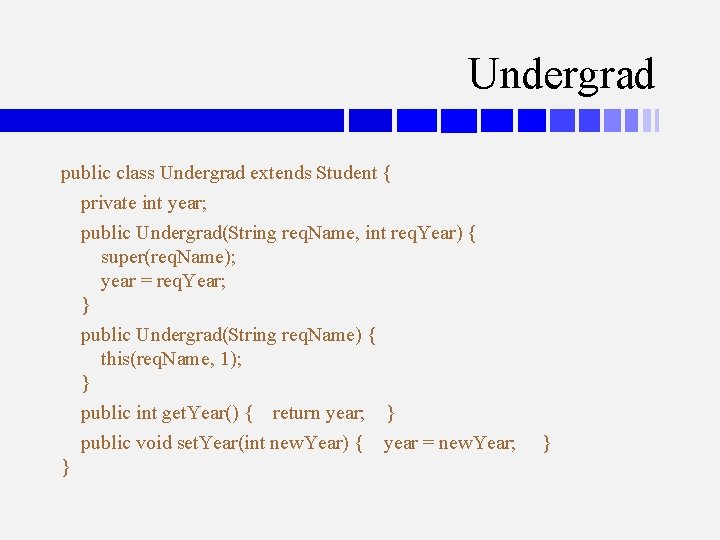
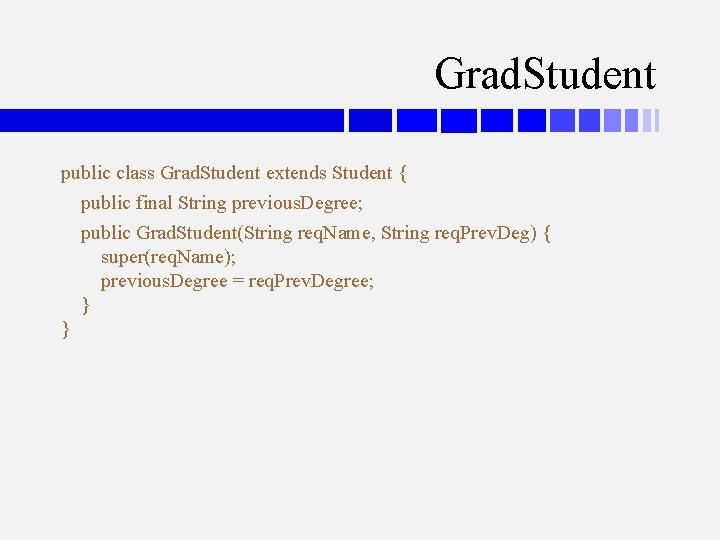
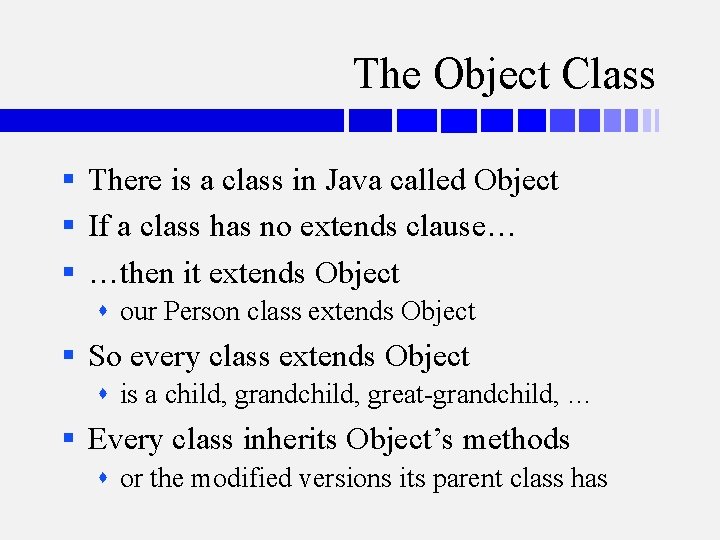
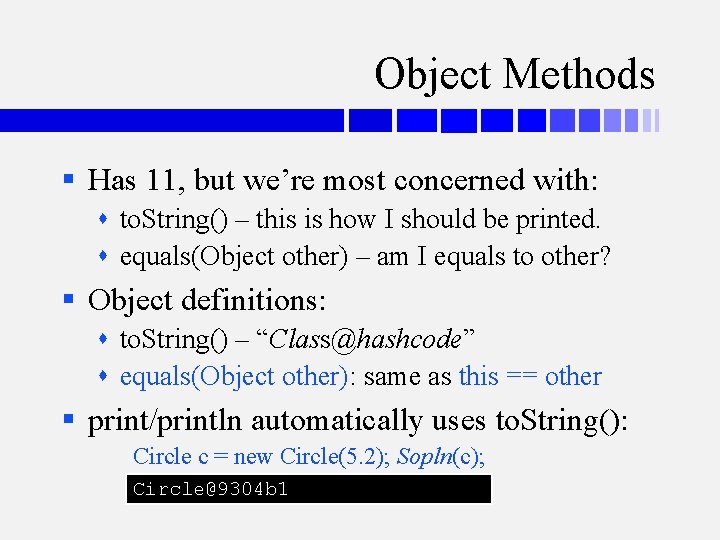
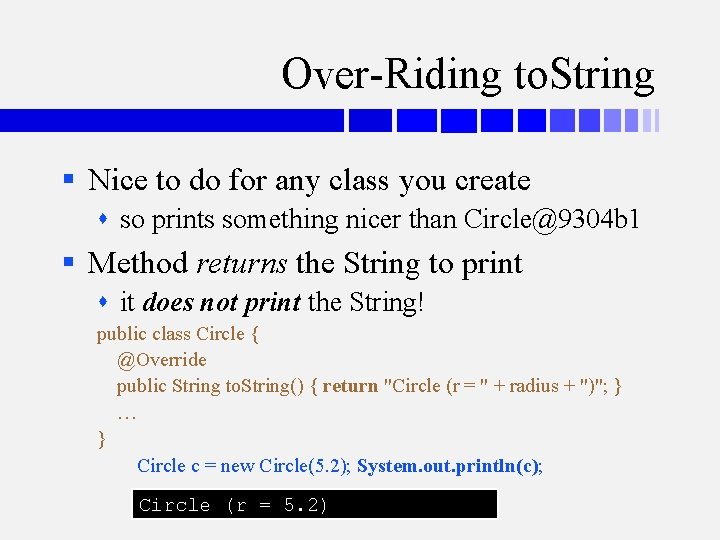
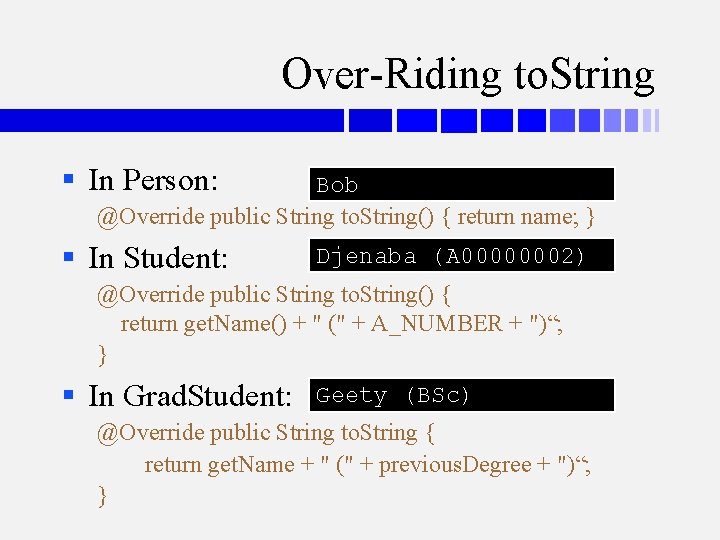
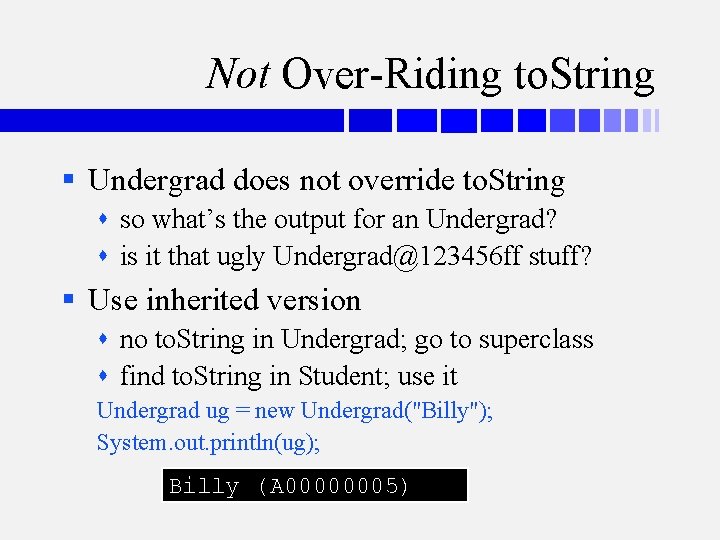
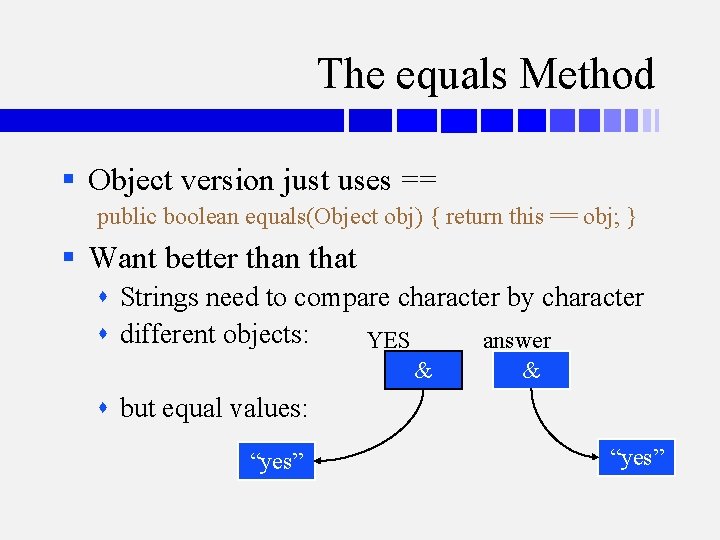
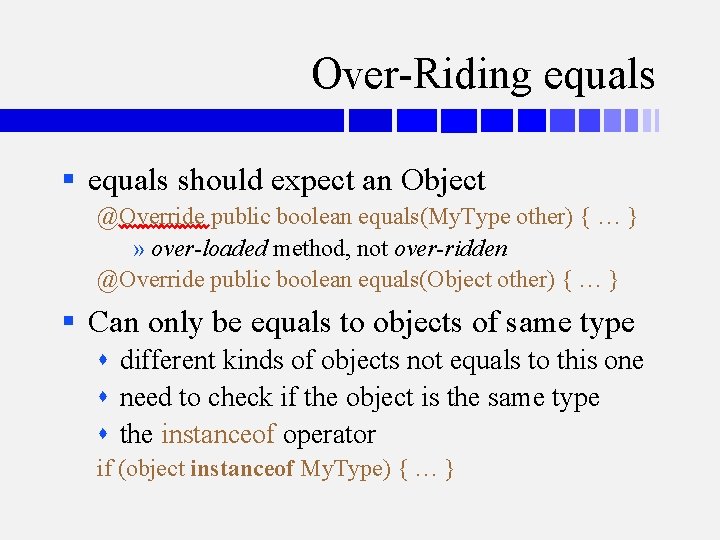
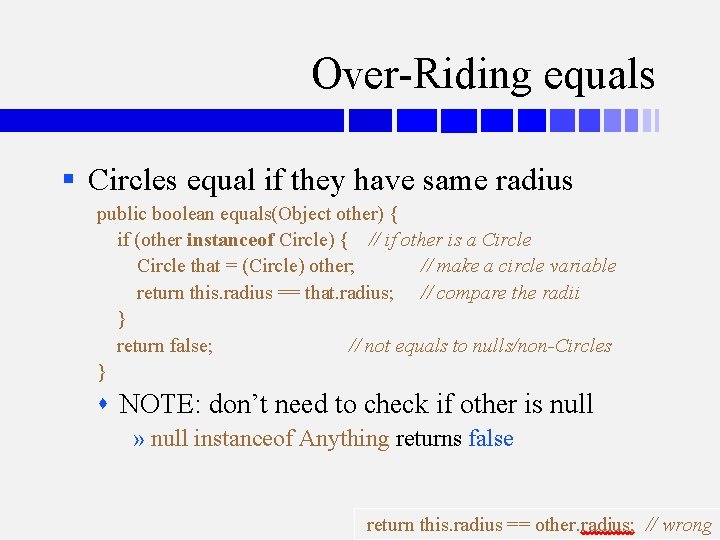
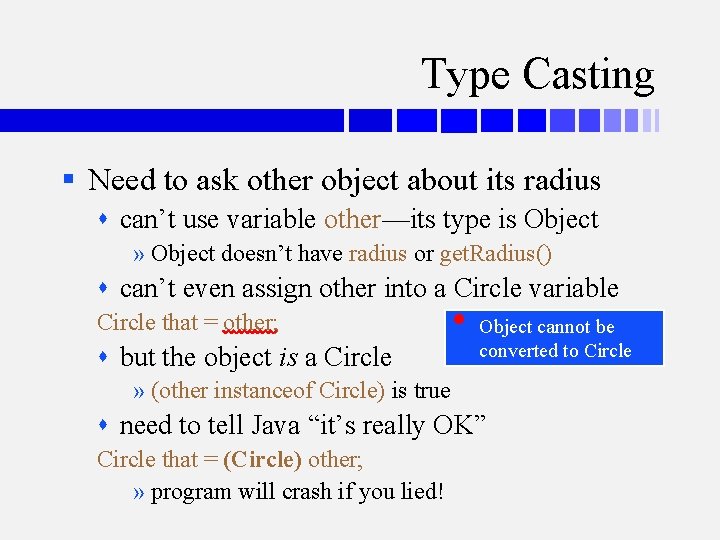
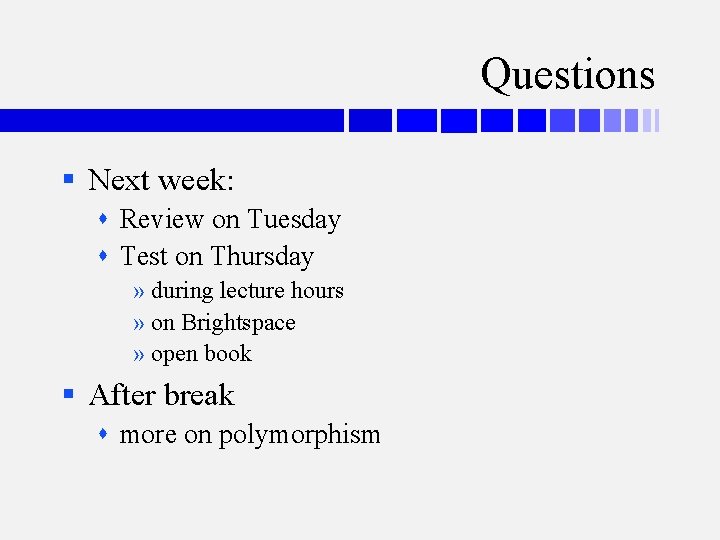
- Slides: 42
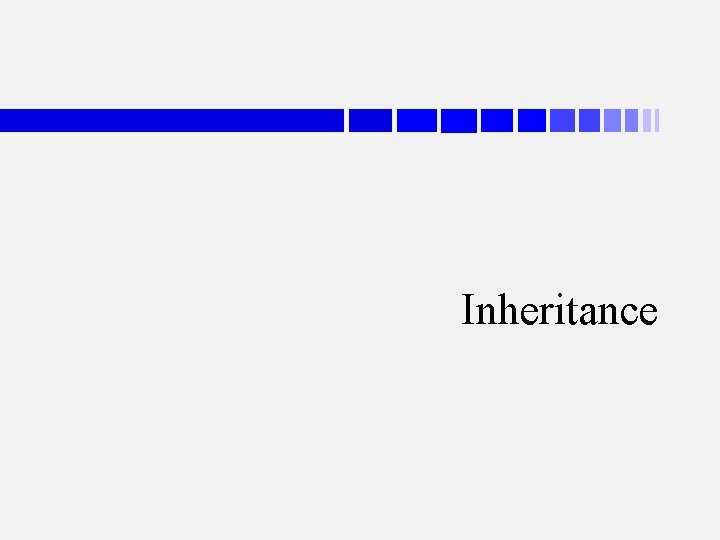
Inheritance
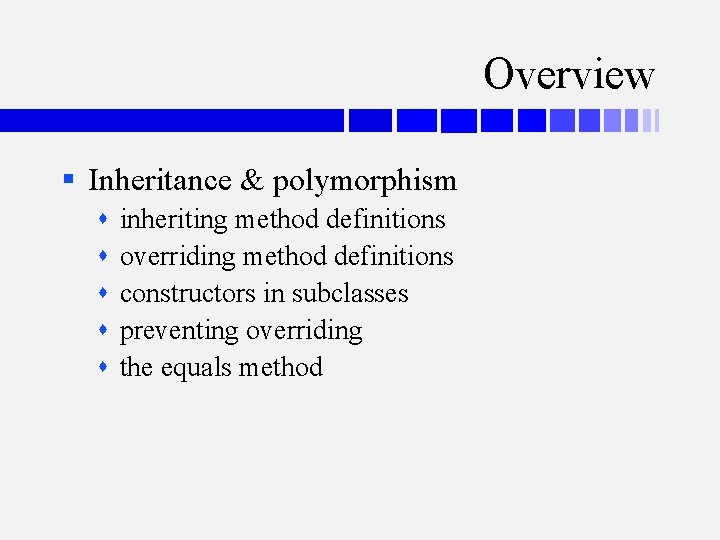
Overview § Inheritance & polymorphism inheriting method definitions overriding method definitions constructors in subclasses preventing overriding the equals method
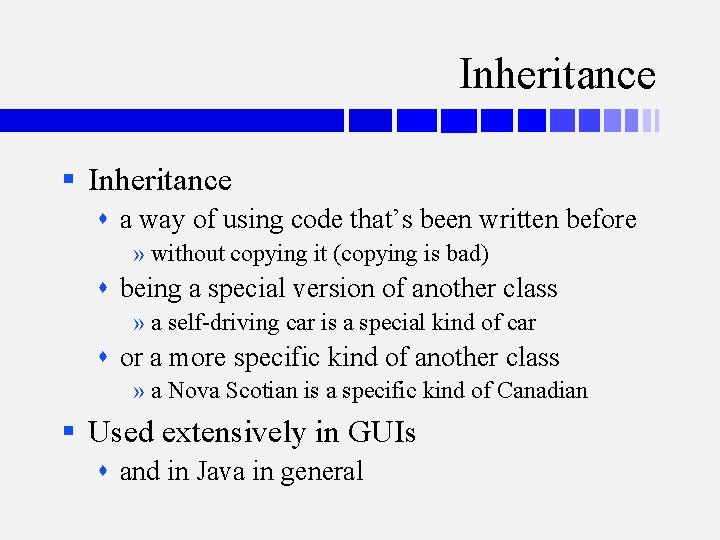
Inheritance § Inheritance a way of using code that’s been written before » without copying it (copying is bad) being a special version of another class » a self-driving car is a special kind of car or a more specific kind of another class » a Nova Scotian is a specific kind of Canadian § Used extensively in GUIs and in Java in general
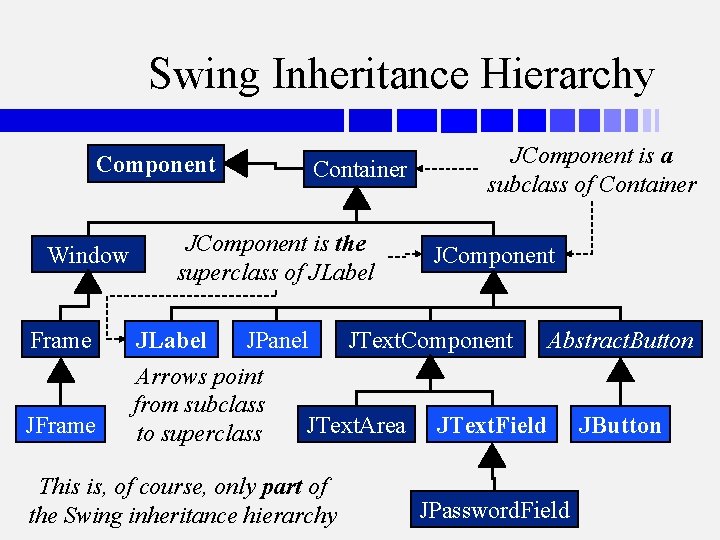
Swing Inheritance Hierarchy Component Window Container JComponent is the superclass of JLabel Frame JLabel JPanel JFrame Arrows point from subclass to superclass JComponent JText. Area This is, of course, only part of the Swing inheritance hierarchy JComponent is a subclass of Container Abstract. Button JText. Field JPassword. Field JButton
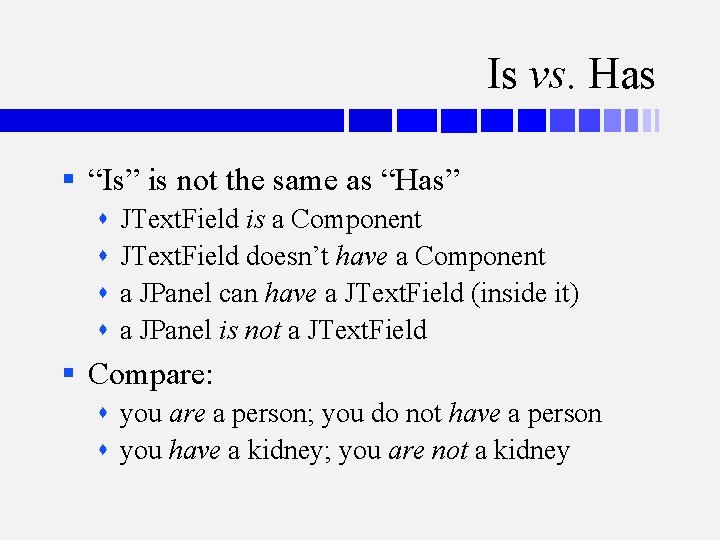
Is vs. Has § “Is” is not the same as “Has” JText. Field is a Component JText. Field doesn’t have a Component a JPanel can have a JText. Field (inside it) a JPanel is not a JText. Field § Compare: you are a person; you do not have a person you have a kidney; you are not a kidney
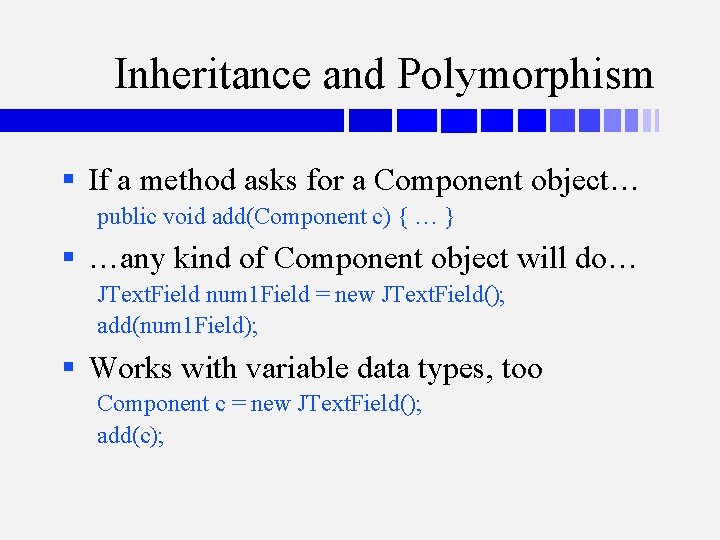
Inheritance and Polymorphism § If a method asks for a Component object… public void add(Component c) { … } § …any kind of Component object will do… JText. Field num 1 Field = new JText. Field(); add(num 1 Field); § Works with variable data types, too Component c = new JText. Field(); add(c);
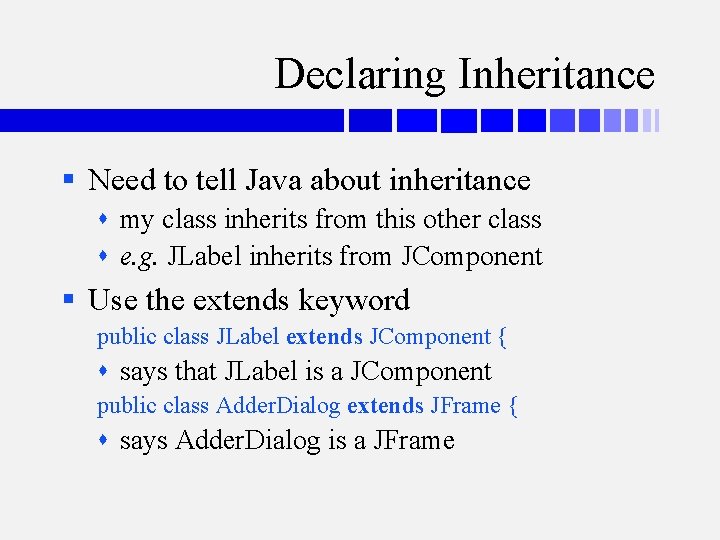
Declaring Inheritance § Need to tell Java about inheritance my class inherits from this other class e. g. JLabel inherits from JComponent § Use the extends keyword public class JLabel extends JComponent { says that JLabel is a JComponent public class Adder. Dialog extends JFrame { says Adder. Dialog is a JFrame
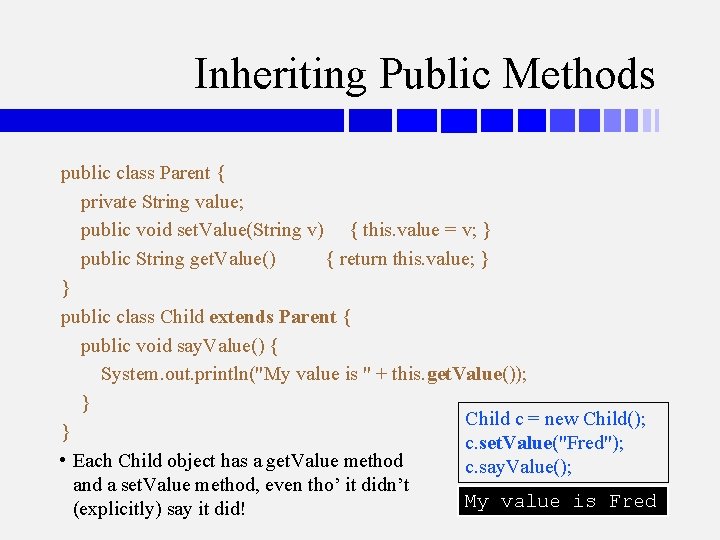
Inheriting Public Methods public class Parent { private String value; public void set. Value(String v) { this. value = v; } public String get. Value() { return this. value; } } public class Child extends Parent { public void say. Value() { System. out. println("My value is " + this. get. Value()); } Child c = new Child(); } c. set. Value("Fred"); • Each Child object has a get. Value method c. say. Value(); and a set. Value method, even tho’ it didn’t My value is Fred (explicitly) say it did!
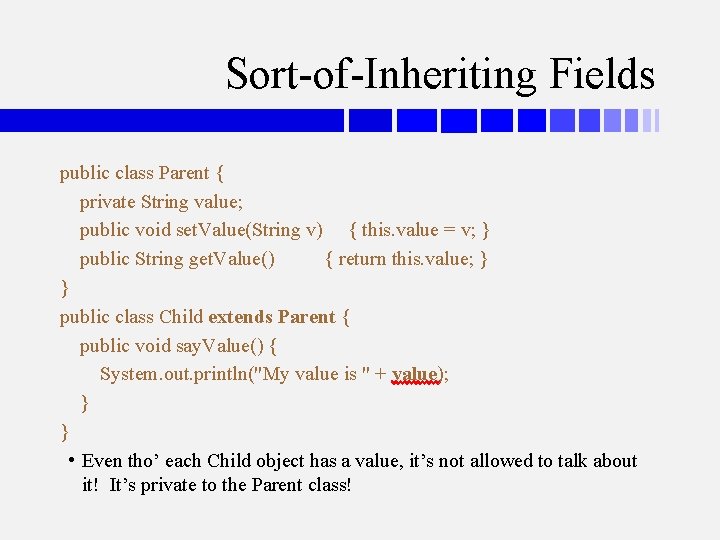
Sort-of-Inheriting Fields public class Parent { private String value; public void set. Value(String v) { this. value = v; } public String get. Value() { return this. value; } } public class Child extends Parent { public void say. Value() { System. out. println("My value is " + value); } } • Even tho’ each Child object has a value, it’s not allowed to talk about it! It’s private to the Parent class!
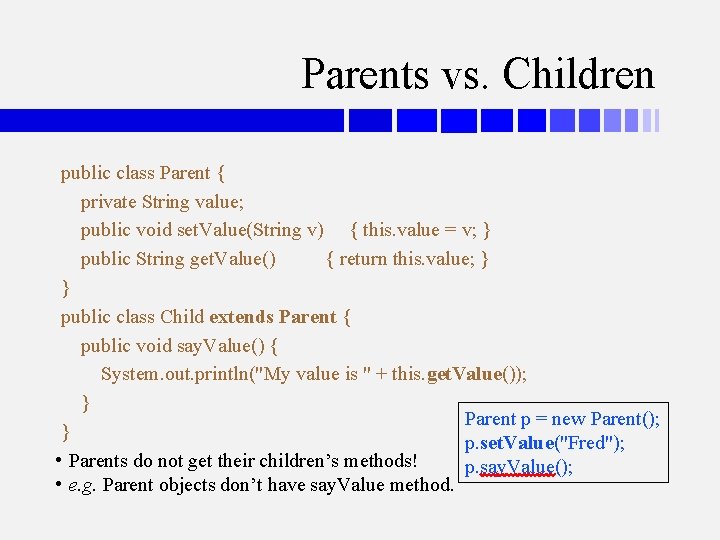
Parents vs. Children public class Parent { private String value; public void set. Value(String v) { this. value = v; } public String get. Value() { return this. value; } } public class Child extends Parent { public void say. Value() { System. out. println("My value is " + this. get. Value()); } Parent p = new Parent(); } p. set. Value("Fred"); • Parents do not get their children’s methods! p. say. Value(); • e. g. Parent objects don’t have say. Value method.
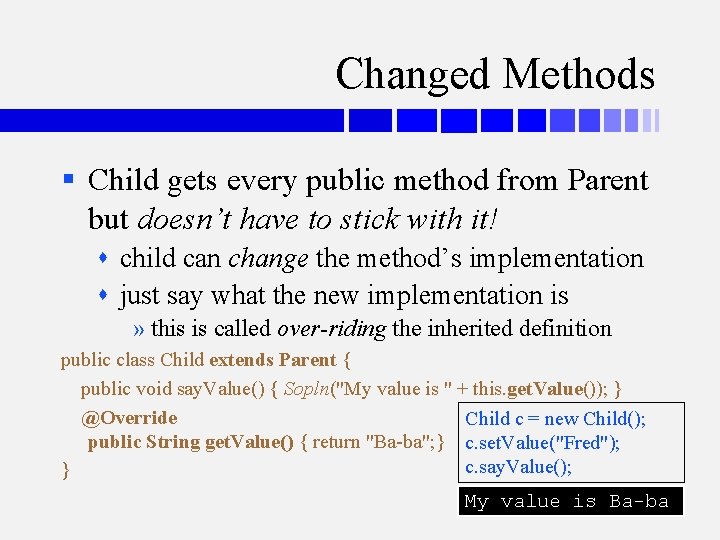
Changed Methods § Child gets every public method from Parent but doesn’t have to stick with it! child can change the method’s implementation just say what the new implementation is » this is called over-riding the inherited definition public class Child extends Parent { public void say. Value() { Sopln("My value is " + this. get. Value()); } @Override Child c = new Child(); public String get. Value() { return "Ba-ba"; } c. set. Value("Fred"); c. say. Value(); } My value is Ba-ba
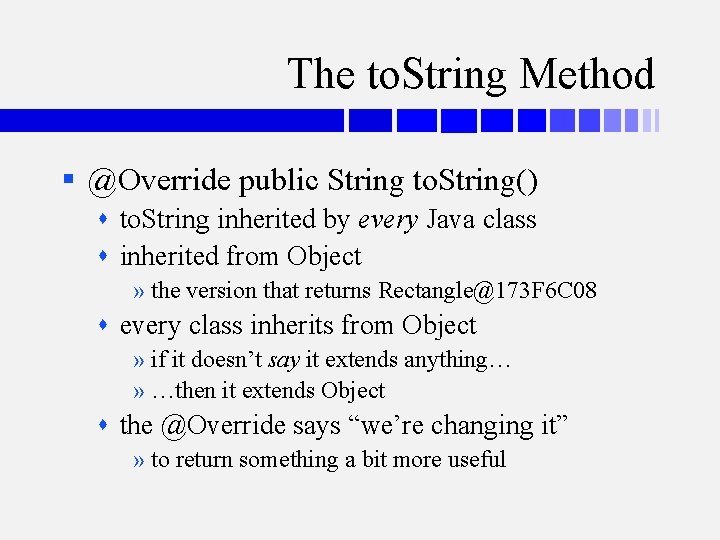
The to. String Method § @Override public String to. String() to. String inherited by every Java class inherited from Object » the version that returns Rectangle@173 F 6 C 08 every class inherits from Object » if it doesn’t say it extends anything… » …then it extends Object the @Override says “we’re changing it” » to return something a bit more useful
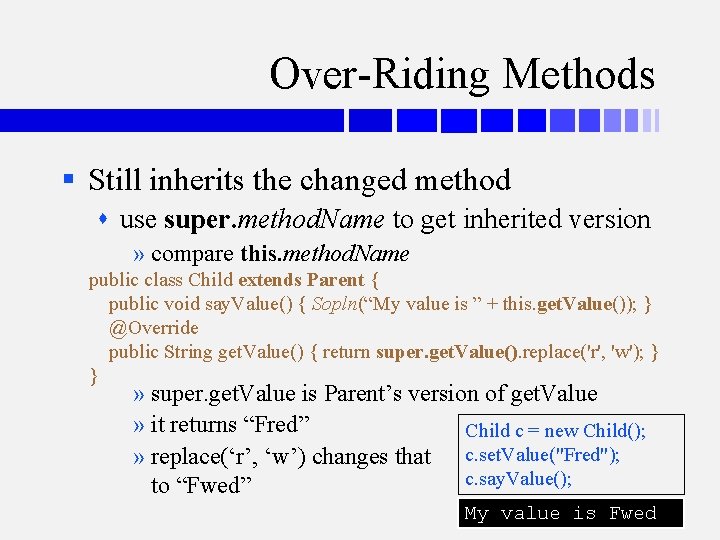
Over-Riding Methods § Still inherits the changed method use super. method. Name to get inherited version » compare this. method. Name public class Child extends Parent { public void say. Value() { Sopln(“My value is ” + this. get. Value()); } @Override public String get. Value() { return super. get. Value(). replace('r', 'w'); } } » super. get. Value is Parent’s version of get. Value » it returns “Fred” Child c = new Child(); » replace(‘r’, ‘w’) changes that c. set. Value("Fred"); c. say. Value(); to “Fwed” My value is Fwed
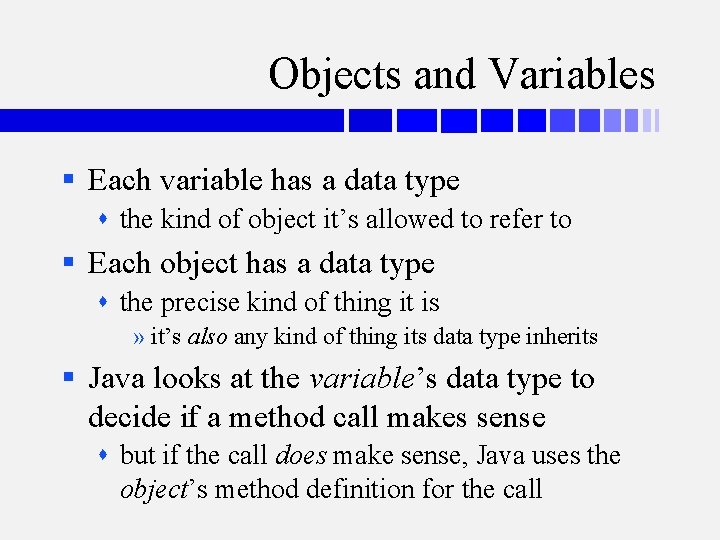
Objects and Variables § Each variable has a data type the kind of object it’s allowed to refer to § Each object has a data type the precise kind of thing it is » it’s also any kind of thing its data type inherits § Java looks at the variable’s data type to decide if a method call makes sense but if the call does make sense, Java uses the object’s method definition for the call
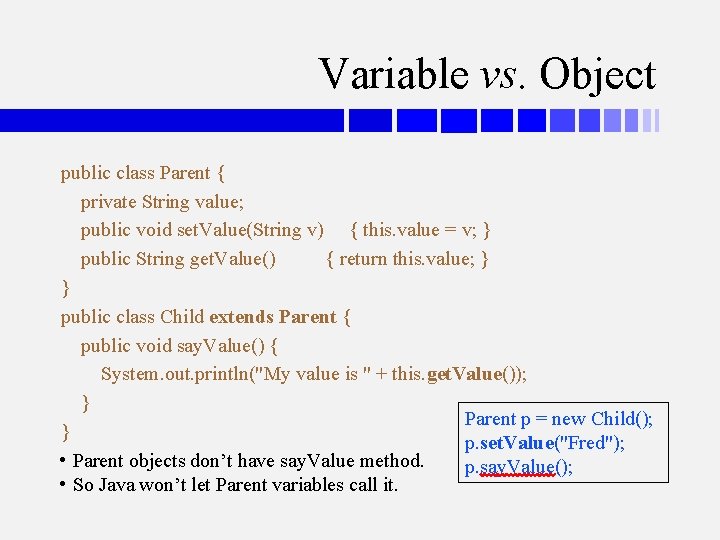
Variable vs. Object public class Parent { private String value; public void set. Value(String v) { this. value = v; } public String get. Value() { return this. value; } } public class Child extends Parent { public void say. Value() { System. out. println("My value is " + this. get. Value()); } Parent p = new Child(); } p. set. Value("Fred"); • Parent objects don’t have say. Value method. p. say. Value(); • So Java won’t let Parent variables call it.
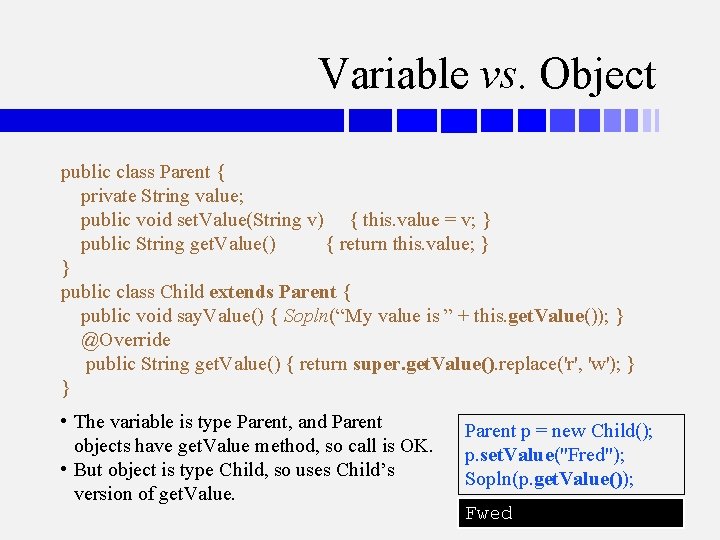
Variable vs. Object public class Parent { private String value; public void set. Value(String v) { this. value = v; } public String get. Value() { return this. value; } } public class Child extends Parent { public void say. Value() { Sopln(“My value is ” + this. get. Value()); } @Override public String get. Value() { return super. get. Value(). replace('r', 'w'); } } • The variable is type Parent, and Parent objects have get. Value method, so call is OK. • But object is type Child, so uses Child’s version of get. Value. Parent p = new Child(); p. set. Value("Fred"); Sopln(p. get. Value()); Fwed
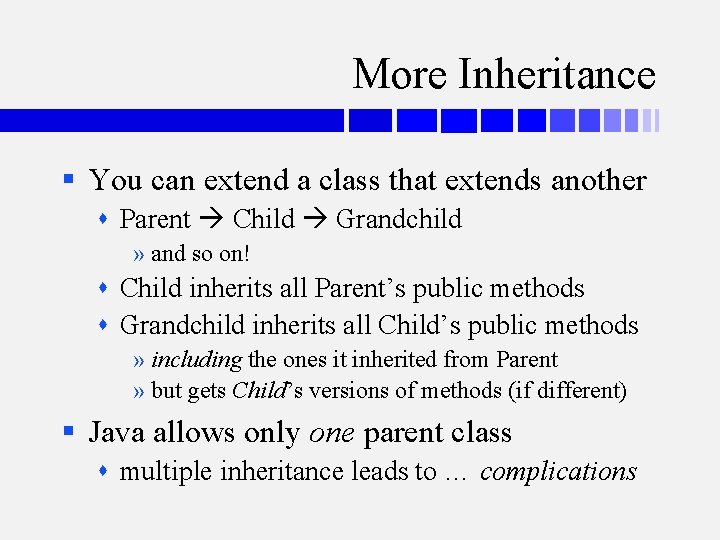
More Inheritance § You can extend a class that extends another Parent Child Grandchild » and so on! Child inherits all Parent’s public methods Grandchild inherits all Child’s public methods » including the ones it inherited from Parent » but gets Child’s versions of methods (if different) § Java allows only one parent class multiple inheritance leads to … complications
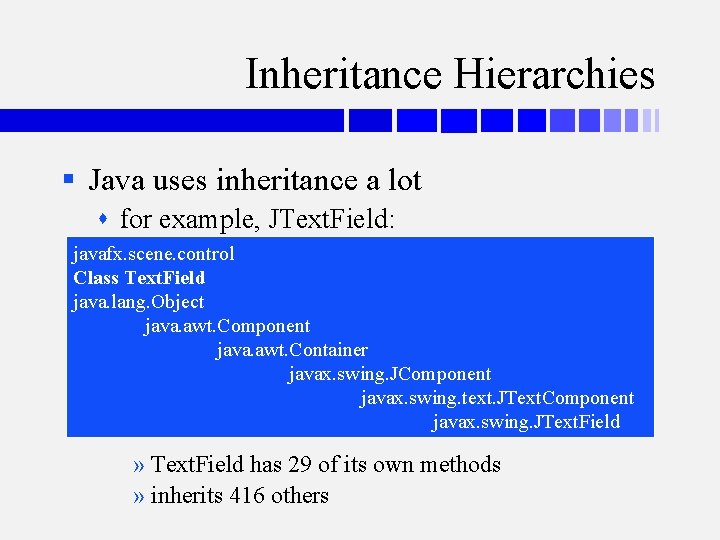
Inheritance Hierarchies § Java uses inheritance a lot for example, JText. Field: javafx. scene. control Class Text. Field java. lang. Object java. awt. Component java. awt. Container javax. swing. JComponent javax. swing. text. JText. Component javax. swing. JText. Field » Text. Field has 29 of its own methods » inherits 416 others
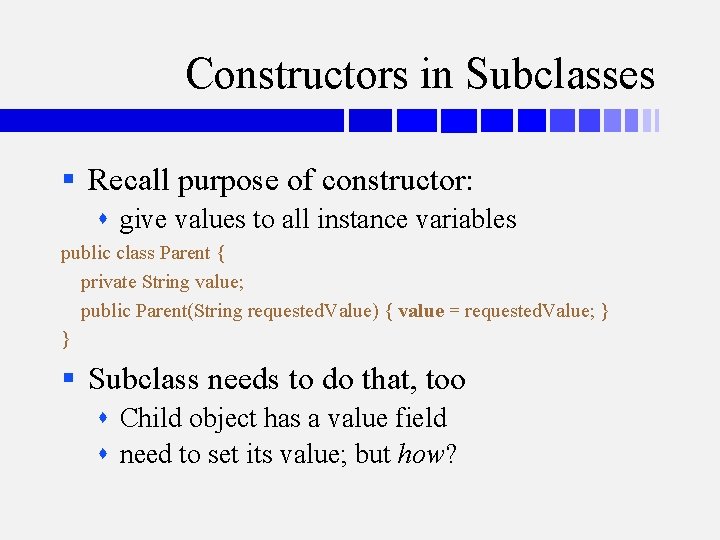
Constructors in Subclasses § Recall purpose of constructor: give values to all instance variables public class Parent { private String value; public Parent(String requested. Value) { value = requested. Value; } } § Subclass needs to do that, too Child object has a value field need to set its value; but how?
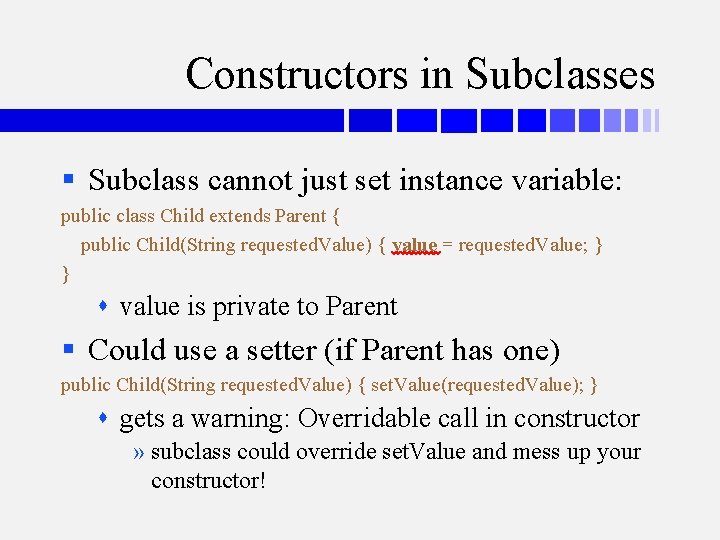
Constructors in Subclasses § Subclass cannot just set instance variable: public class Child extends Parent { public Child(String requested. Value) { value = requested. Value; } } value is private to Parent § Could use a setter (if Parent has one) public Child(String requested. Value) { set. Value(requested. Value); } gets a warning: Overridable call in constructor » subclass could override set. Value and mess up your constructor!
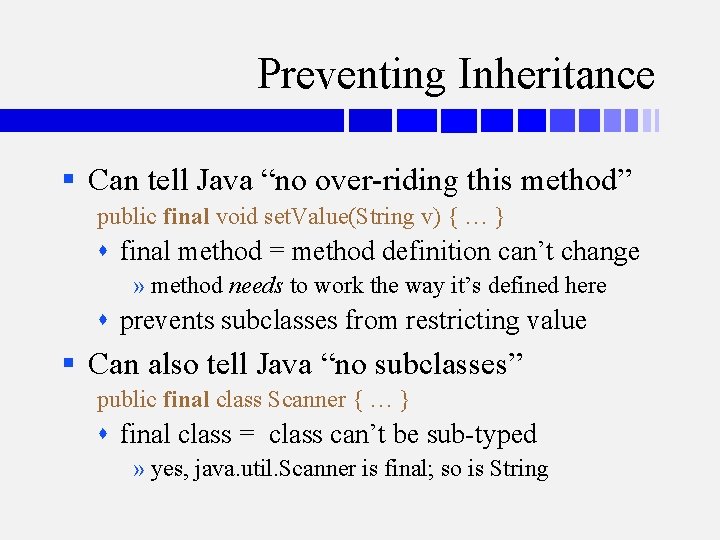
Preventing Inheritance § Can tell Java “no over-riding this method” public final void set. Value(String v) { … } final method = method definition can’t change » method needs to work the way it’s defined here prevents subclasses from restricting value § Can also tell Java “no subclasses” public final class Scanner { … } final class = class can’t be sub-typed » yes, java. util. Scanner is final; so is String
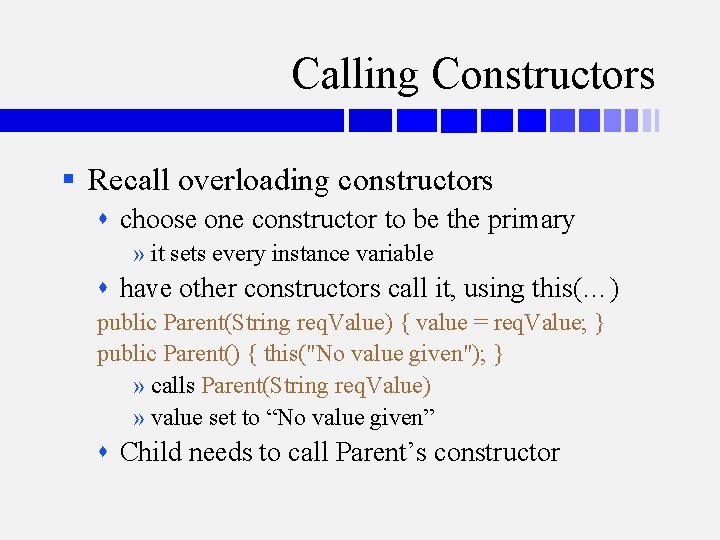
Calling Constructors § Recall overloading constructors choose one constructor to be the primary » it sets every instance variable have other constructors call it, using this(…) public Parent(String req. Value) { value = req. Value; } public Parent() { this("No value given"); } » calls Parent(String req. Value) » value set to “No value given” Child needs to call Parent’s constructor
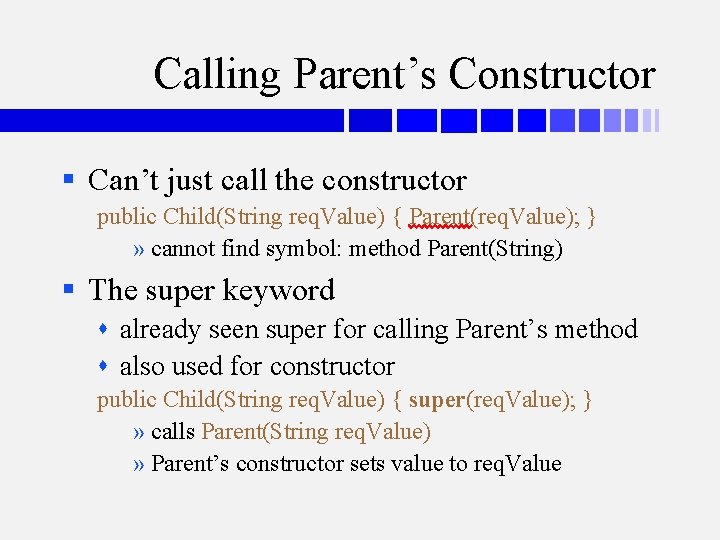
Calling Parent’s Constructor § Can’t just call the constructor public Child(String req. Value) { Parent(req. Value); } » cannot find symbol: method Parent(String) § The super keyword already seen super for calling Parent’s method also used for constructor public Child(String req. Value) { super(req. Value); } » calls Parent(String req. Value) » Parent’s constructor sets value to req. Value
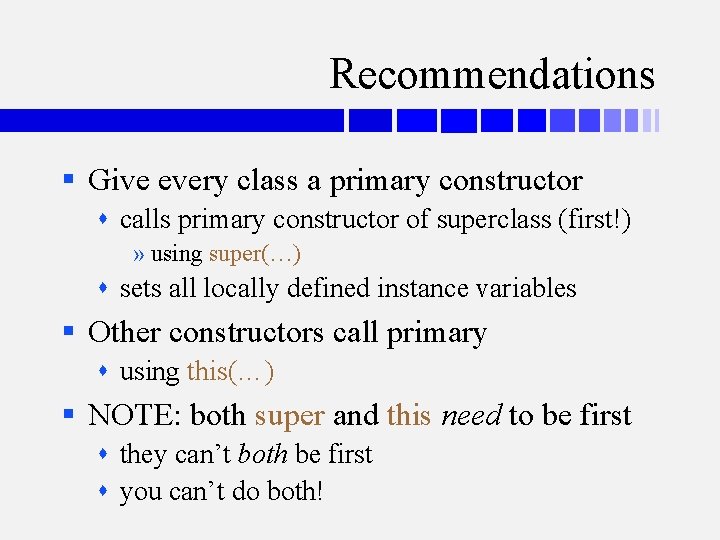
Recommendations § Give every class a primary constructor calls primary constructor of superclass (first!) » using super(…) sets all locally defined instance variables § Other constructors call primary using this(…) § NOTE: both super and this need to be first they can’t both be first you can’t do both!
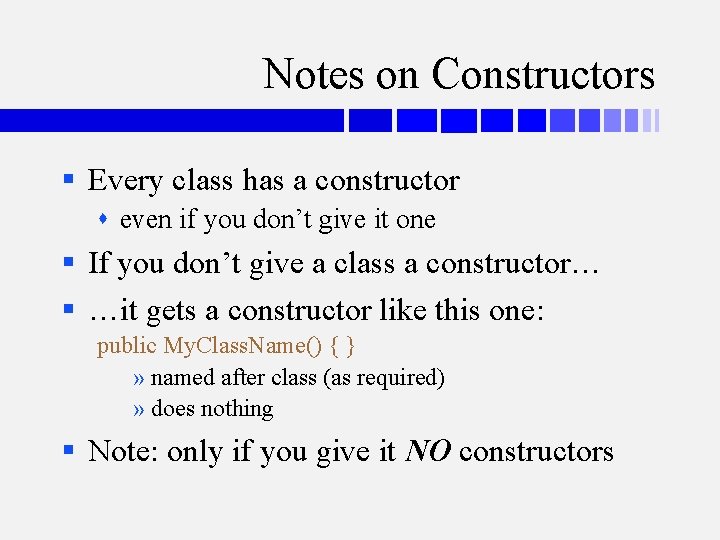
Notes on Constructors § Every class has a constructor even if you don’t give it one § If you don’t give a class a constructor… § …it gets a constructor like this one: public My. Class. Name() { } » named after class (as required) » does nothing § Note: only if you give it NO constructors
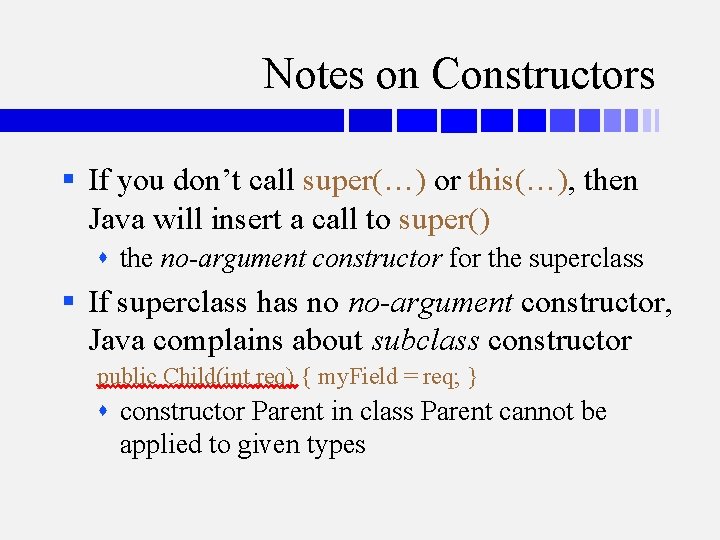
Notes on Constructors § If you don’t call super(…) or this(…), then Java will insert a call to super() the no-argument constructor for the superclass § If superclass has no no-argument constructor, Java complains about subclass constructor public Child(int req) { my. Field = req; } constructor Parent in class Parent cannot be applied to given types
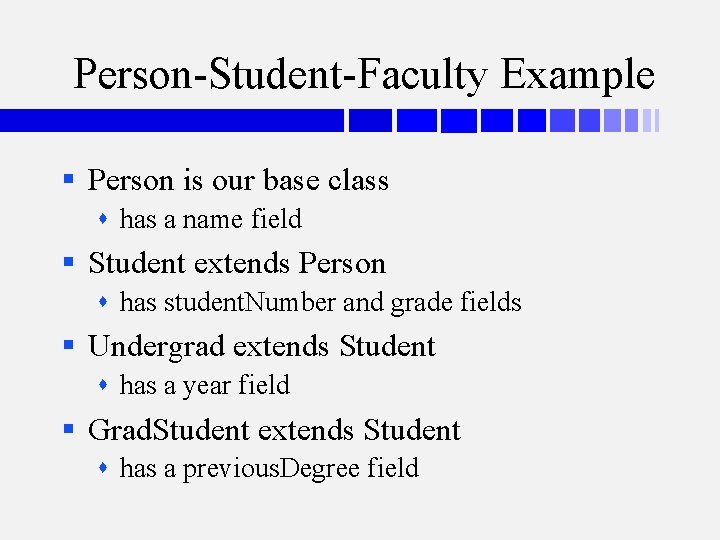
Person-Student-Faculty Example § Person is our base class has a name field § Student extends Person has student. Number and grade fields § Undergrad extends Student has a year field § Grad. Student extends Student has a previous. Degree field
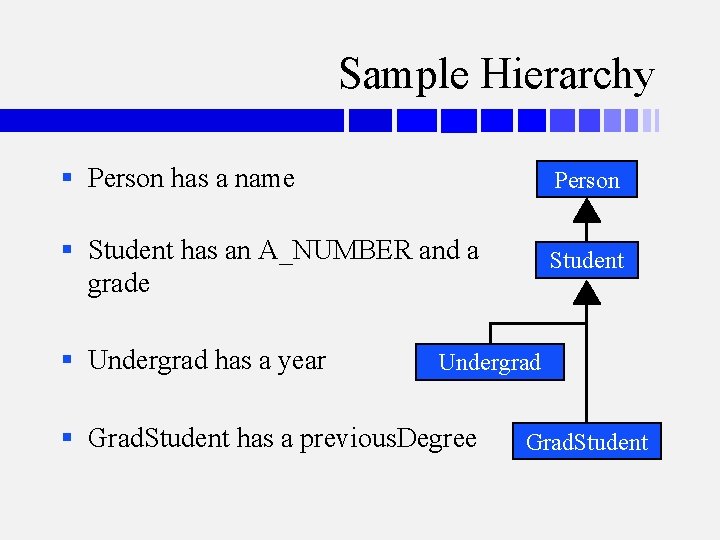
Sample Hierarchy § Person has a name Person § Student has an A_NUMBER and a grade Student § Undergrad has a year Undergrad § Grad. Student has a previous. Degree Grad. Student
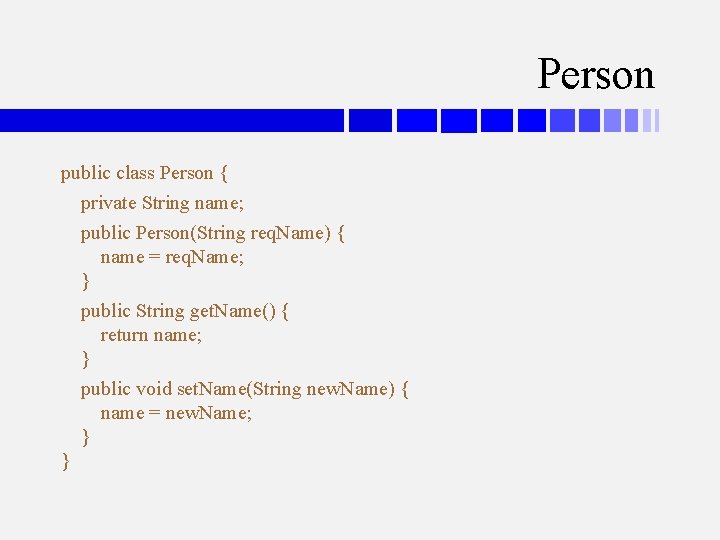
Person public class Person { private String name; public Person(String req. Name) { name = req. Name; } public String get. Name() { return name; } public void set. Name(String new. Name) { name = new. Name; } }
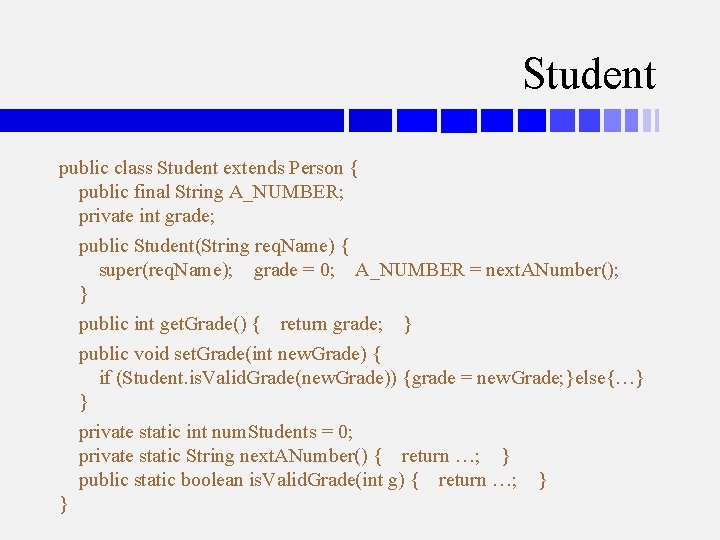
Student public class Student extends Person { public final String A_NUMBER; private int grade; public Student(String req. Name) { super(req. Name); grade = 0; A_NUMBER = next. ANumber(); } public int get. Grade() { return grade; } public void set. Grade(int new. Grade) { if (Student. is. Valid. Grade(new. Grade)) {grade = new. Grade; }else{…} } private static int num. Students = 0; private static String next. ANumber() { return …; } public static boolean is. Valid. Grade(int g) { return …; } }
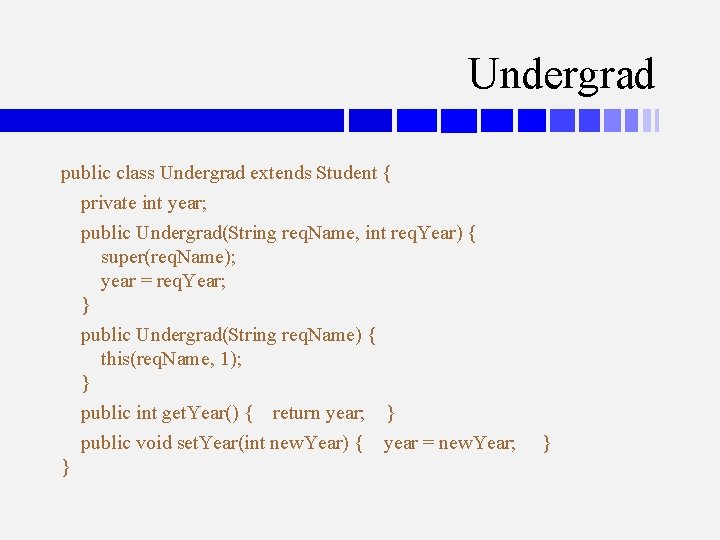
Undergrad public class Undergrad extends Student { private int year; public Undergrad(String req. Name, int req. Year) { super(req. Name); year = req. Year; } public Undergrad(String req. Name) { this(req. Name, 1); } public int get. Year() { return year; } public void set. Year(int new. Year) { year = new. Year; } }
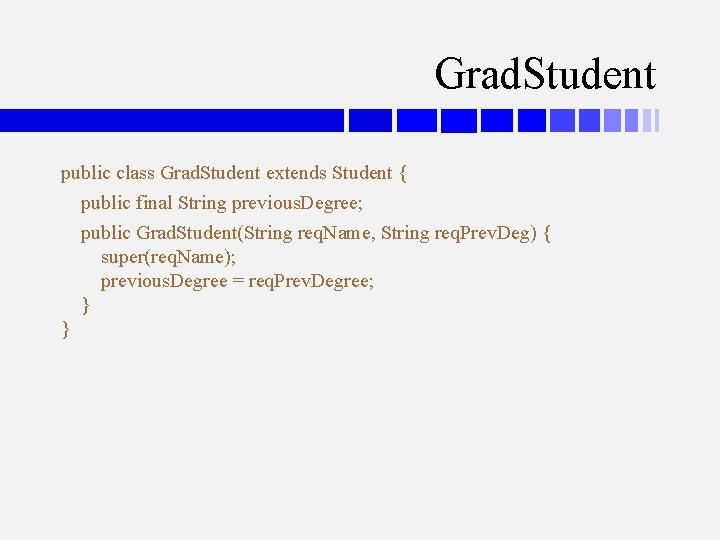
Grad. Student public class Grad. Student extends Student { public final String previous. Degree; public Grad. Student(String req. Name, String req. Prev. Deg) { super(req. Name); previous. Degree = req. Prev. Degree; } }
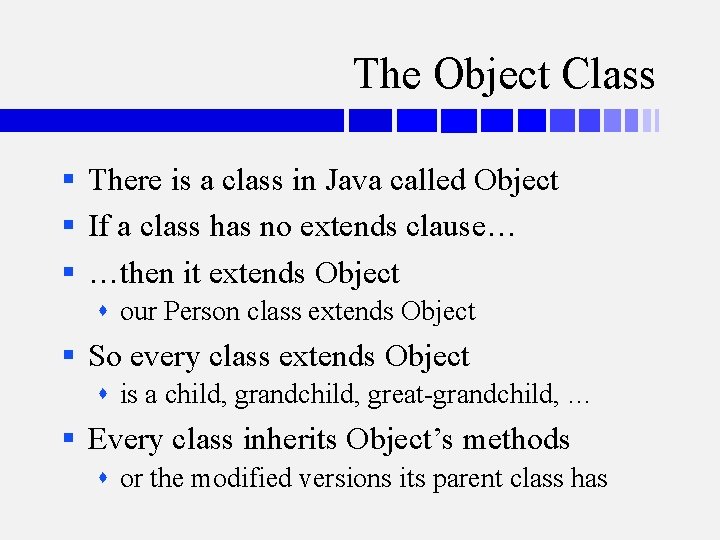
The Object Class § There is a class in Java called Object § If a class has no extends clause… § …then it extends Object our Person class extends Object § So every class extends Object is a child, grandchild, great-grandchild, … § Every class inherits Object’s methods or the modified versions its parent class has
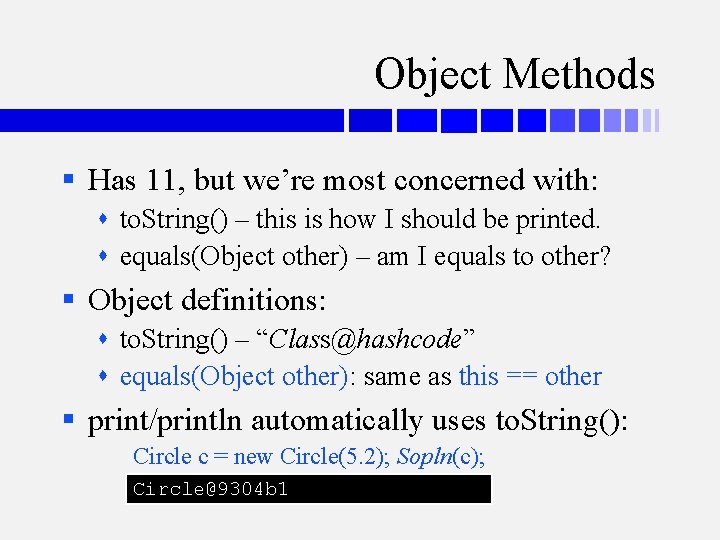
Object Methods § Has 11, but we’re most concerned with: to. String() – this is how I should be printed. equals(Object other) – am I equals to other? § Object definitions: to. String() – “Class@hashcode” equals(Object other): same as this == other § print/println automatically uses to. String(): Circle c = new Circle(5. 2); Sopln(c); Circle@9304 b 1
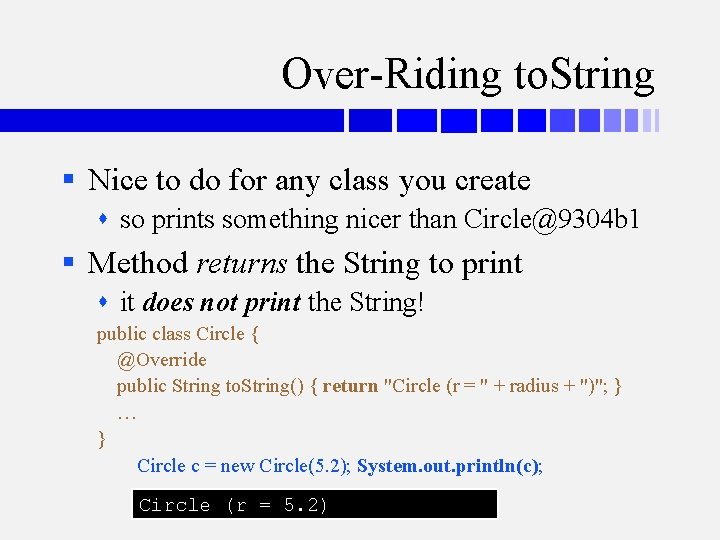
Over-Riding to. String § Nice to do for any class you create so prints something nicer than Circle@9304 b 1 § Method returns the String to print it does not print the String! public class Circle { @Override public String to. String() { return "Circle (r = " + radius + ")"; } … } Circle c = new Circle(5. 2); System. out. println(c); Circle (r = 5. 2)
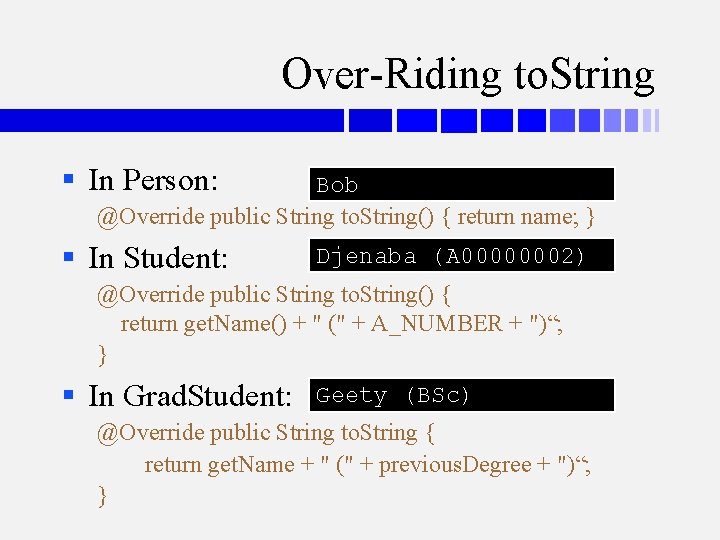
Over-Riding to. String § In Person: Bob @Override public String to. String() { return name; } § In Student: Djenaba (A 00000002) @Override public String to. String() { return get. Name() + " (" + A_NUMBER + ")“; } § In Grad. Student: Geety (BSc) @Override public String to. String { return get. Name + " (" + previous. Degree + ")“; }
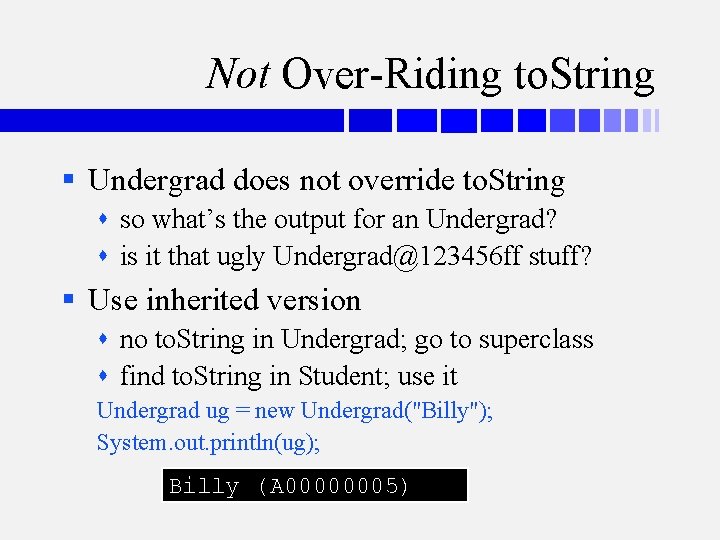
Not Over-Riding to. String § Undergrad does not override to. String so what’s the output for an Undergrad? is it that ugly Undergrad@123456 ff stuff? § Use inherited version no to. String in Undergrad; go to superclass find to. String in Student; use it Undergrad ug = new Undergrad("Billy"); System. out. println(ug); Billy (A 00000005)
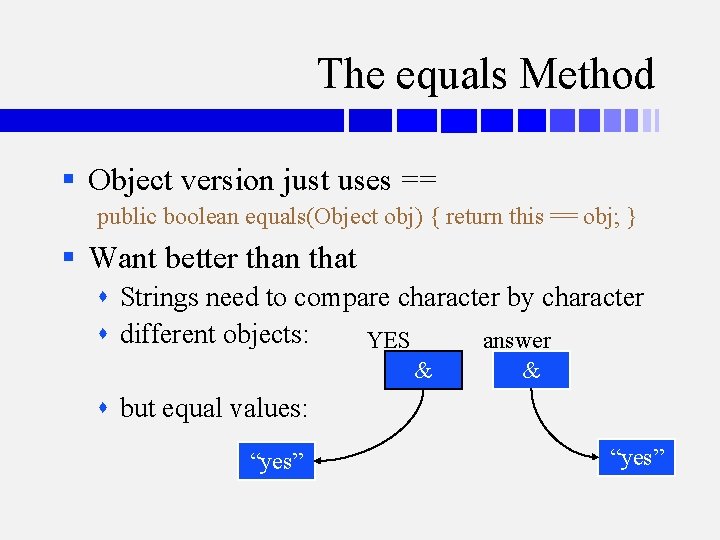
The equals Method § Object version just uses == public boolean equals(Object obj) { return this == obj; } § Want better than that Strings need to compare character by character different objects: YES answer & & but equal values: “yes”
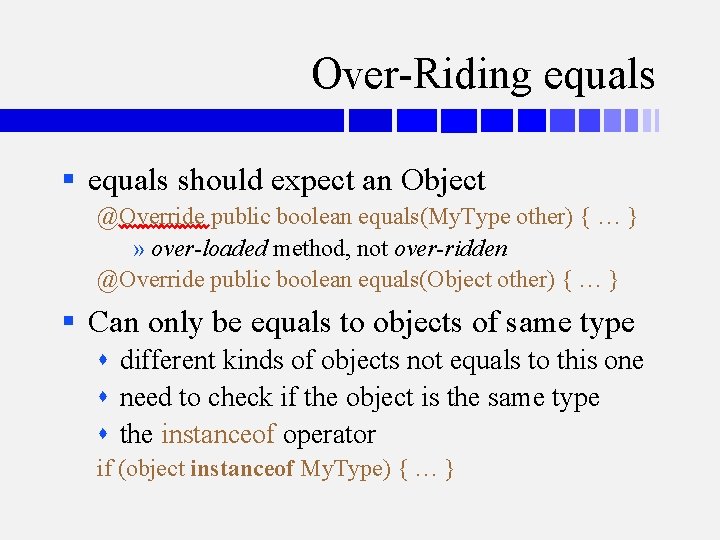
Over-Riding equals § equals should expect an Object @Override public boolean equals(My. Type other) { … } » over-loaded method, not over-ridden @Override public boolean equals(Object other) { … } § Can only be equals to objects of same type different kinds of objects not equals to this one need to check if the object is the same type the instanceof operator if (object instanceof My. Type) { … }
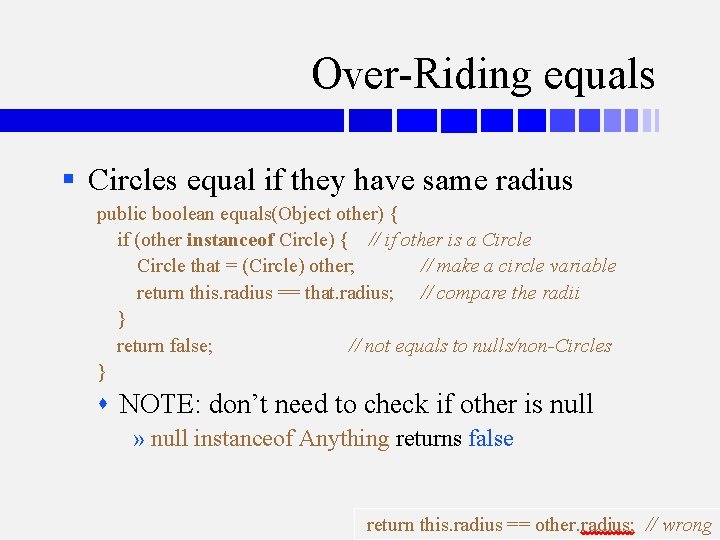
Over-Riding equals § Circles equal if they have same radius public boolean equals(Object other) { if (other instanceof Circle) { // if other is a Circle that = (Circle) other; // make a circle variable return this. radius == that. radius; // compare the radii } return false; // not equals to nulls/non-Circles } NOTE: don’t need to check if other is null » null instanceof Anything returns false return this. radius == other. radius; // wrong
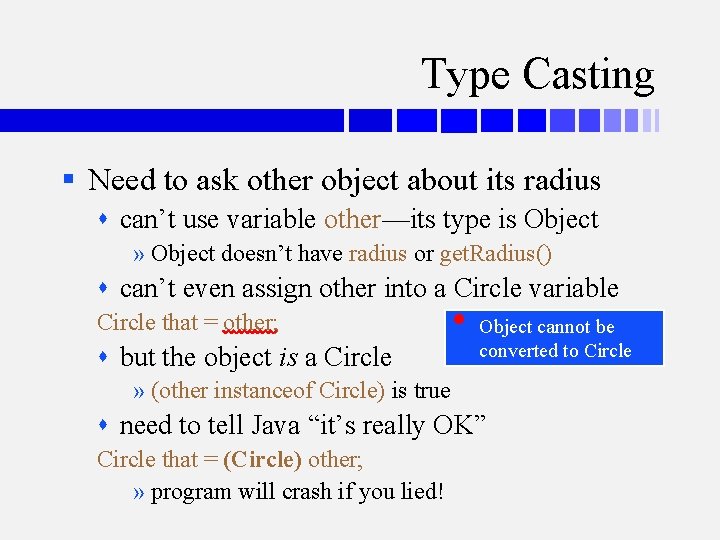
Type Casting § Need to ask other object about its radius can’t use variable other—its type is Object » Object doesn’t have radius or get. Radius() can’t even assign other into a Circle variable Circle that = other; but the object is a Circle • Object cannot be converted to Circle » (other instanceof Circle) is true need to tell Java “it’s really OK” Circle that = (Circle) other; » program will crash if you lied!
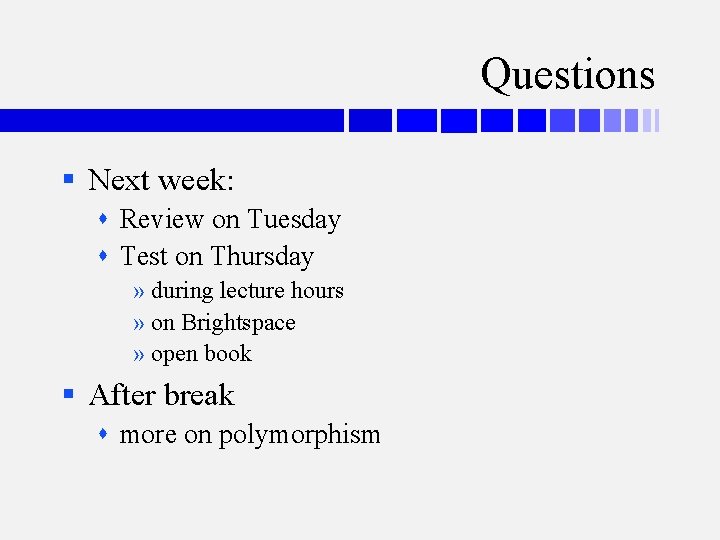
Questions § Next week: Review on Tuesday Test on Thursday » during lecture hours » on Brightspace » open book § After break more on polymorphism