Overloading and Overriding Overloading reusing the same method
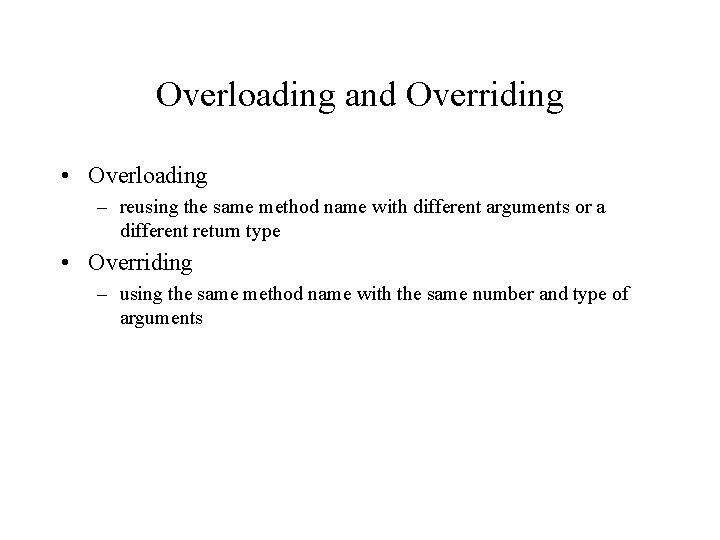
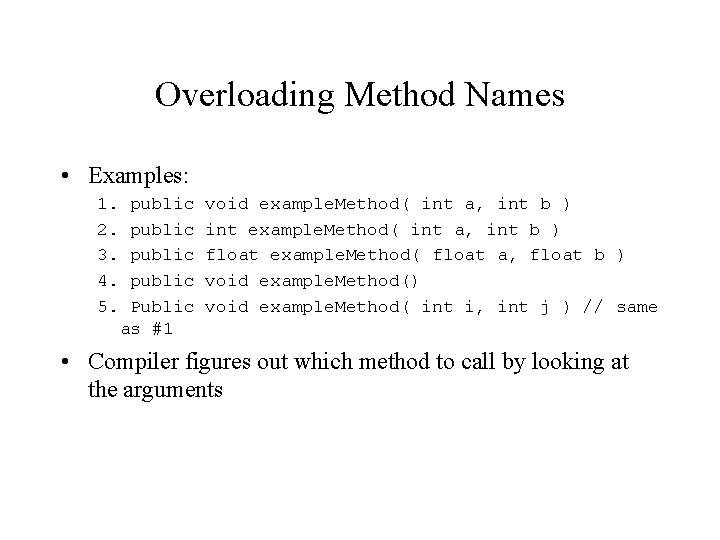
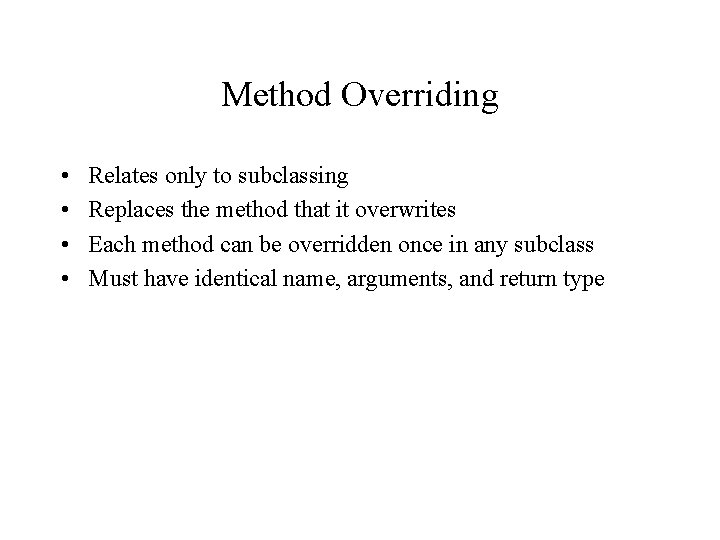
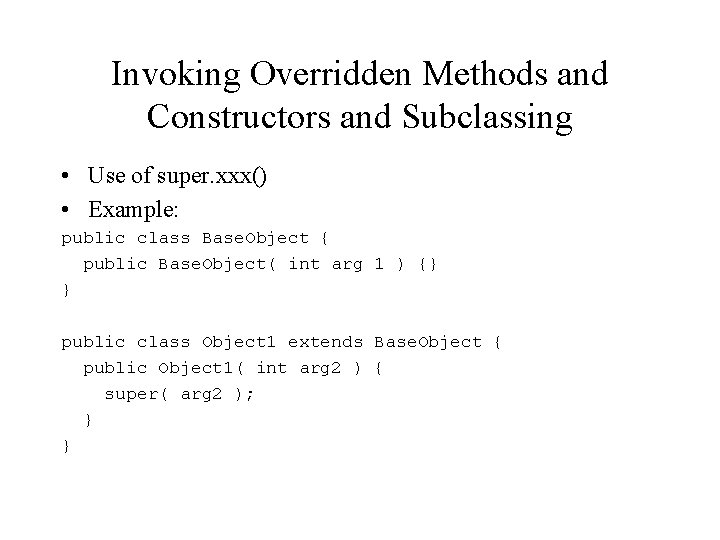
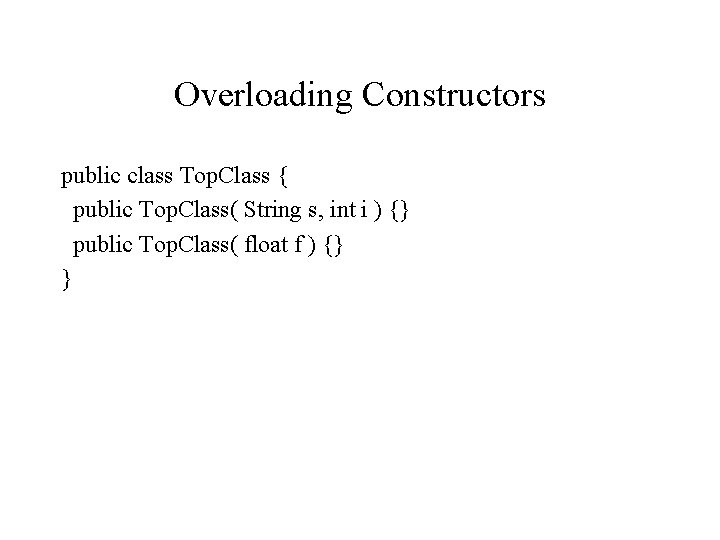
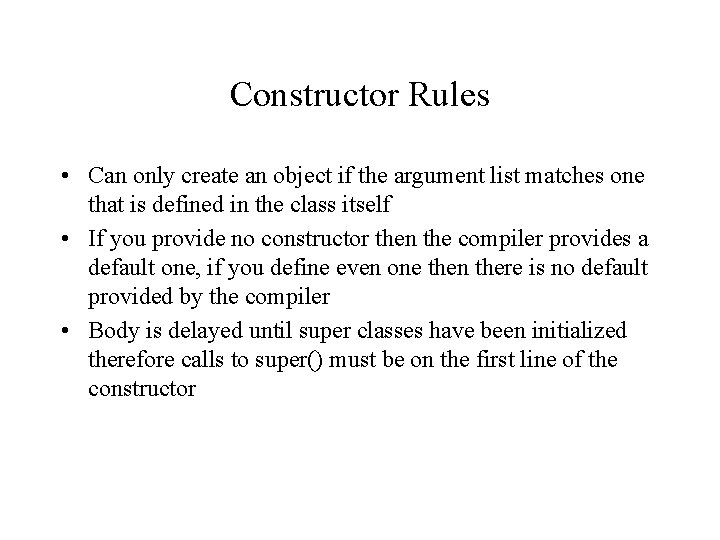
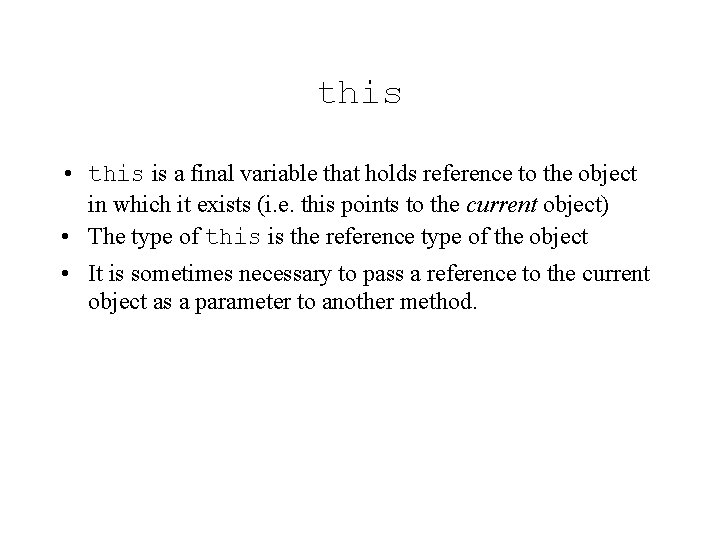
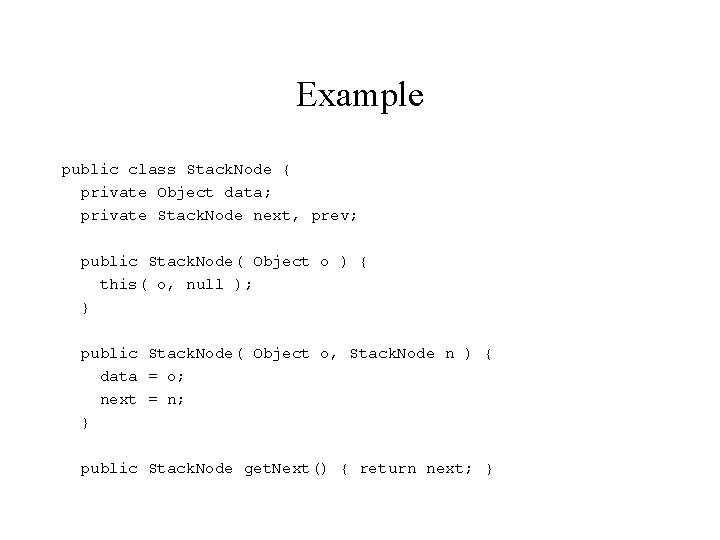
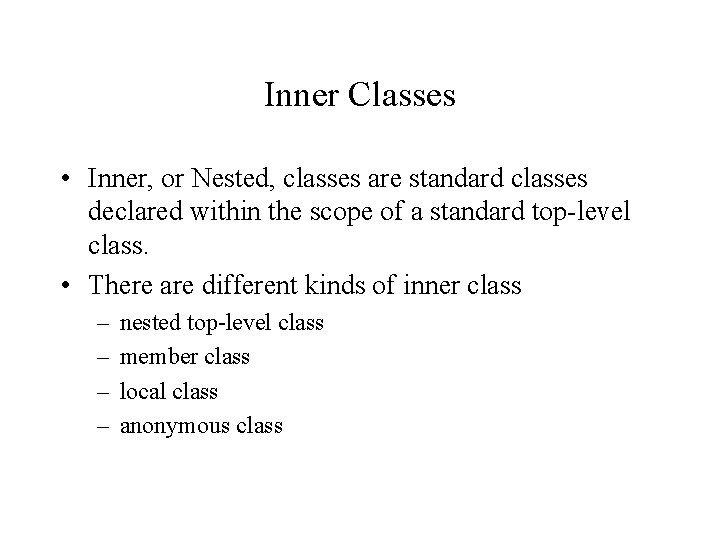
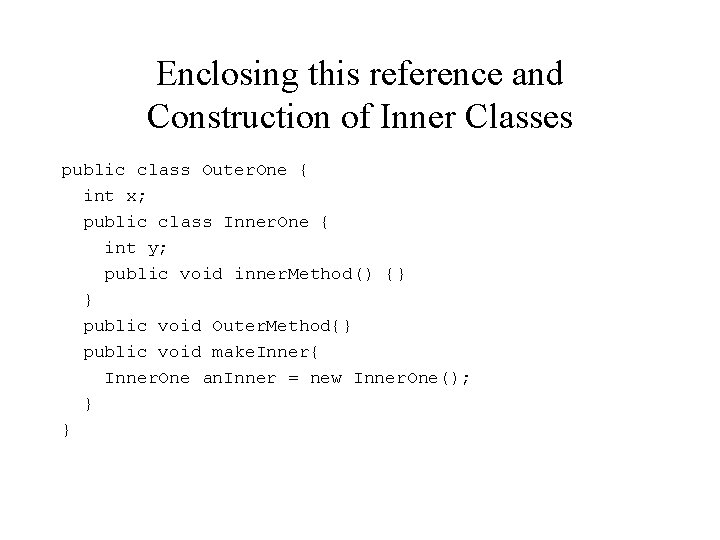
![Continued…. Public static void main( String args[] ) { Outer. One. Inner. One i Continued…. Public static void main( String args[] ) { Outer. One. Inner. One i](https://slidetodoc.com/presentation_image_h/1ee58508d3ba44cf4c054f165adf004f/image-11.jpg)
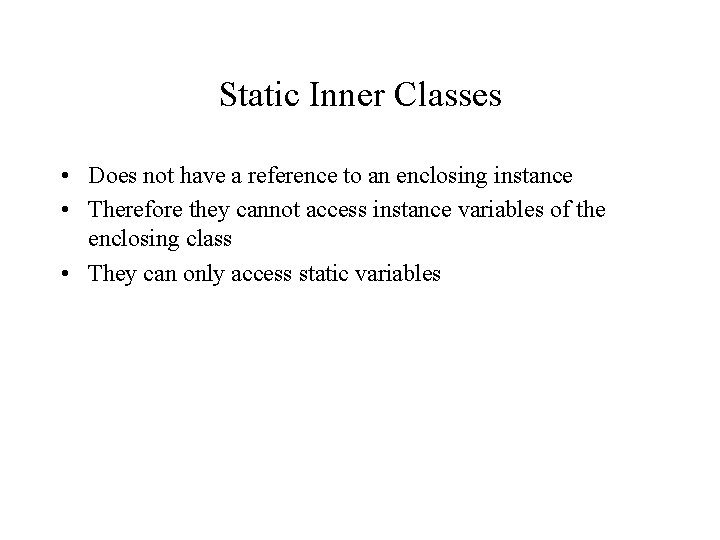
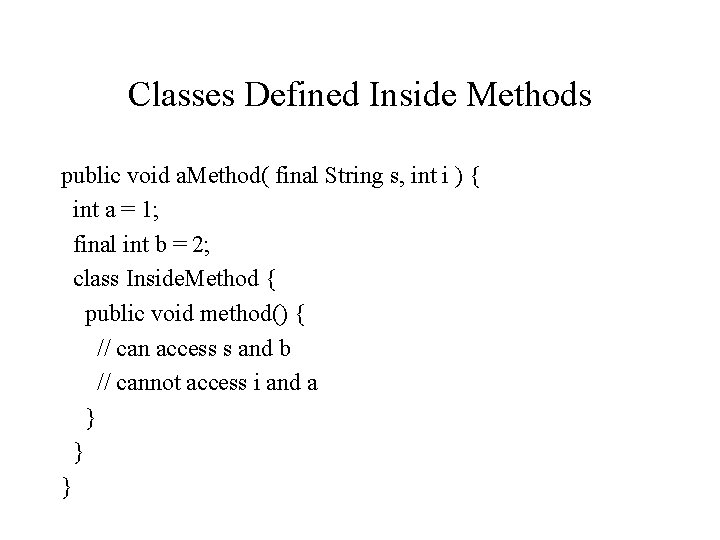
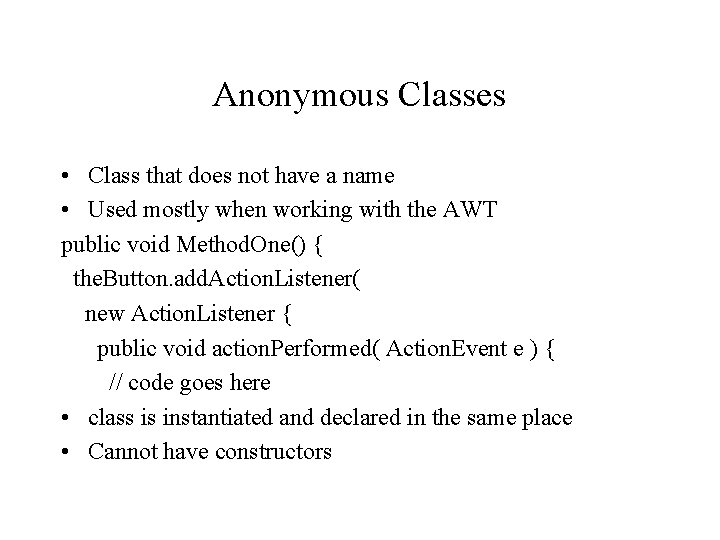
- Slides: 14
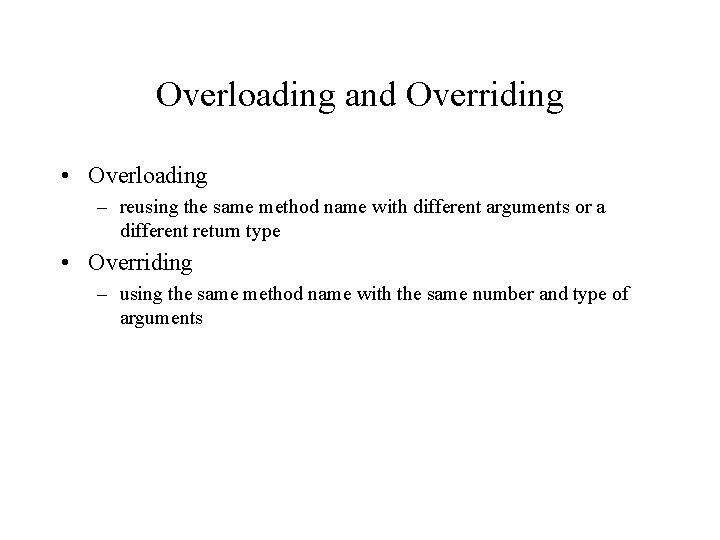
Overloading and Overriding • Overloading – reusing the same method name with different arguments or a different return type • Overriding – using the same method name with the same number and type of arguments
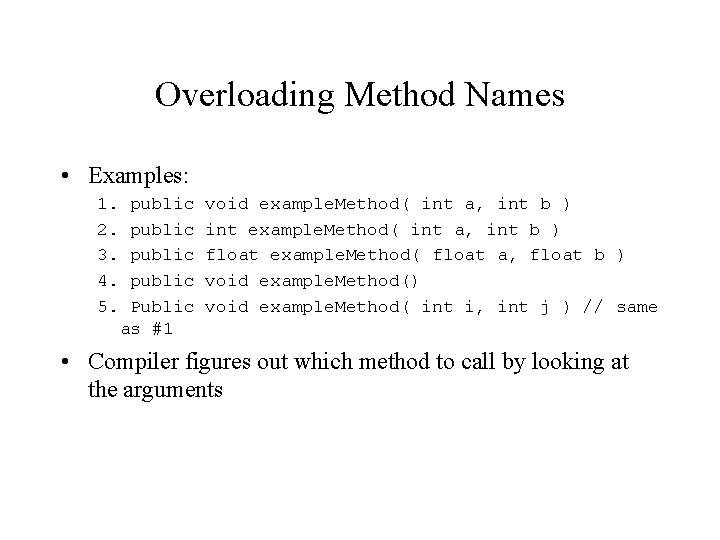
Overloading Method Names • Examples: 1. 2. 3. 4. 5. public Public as #1 void example. Method( int a, int b ) int example. Method( int a, int b ) float example. Method( float a, float b ) void example. Method( int i, int j ) // same • Compiler figures out which method to call by looking at the arguments
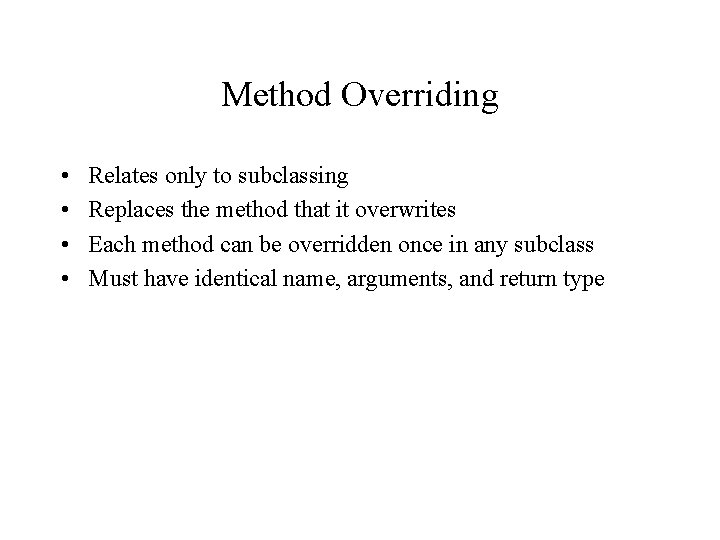
Method Overriding • • Relates only to subclassing Replaces the method that it overwrites Each method can be overridden once in any subclass Must have identical name, arguments, and return type
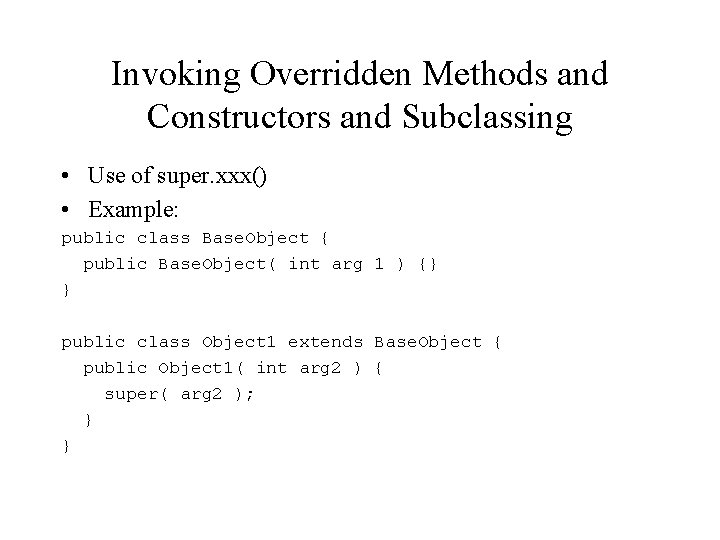
Invoking Overridden Methods and Constructors and Subclassing • Use of super. xxx() • Example: public class Base. Object { public Base. Object( int arg 1 ) {} } public class Object 1 extends Base. Object { public Object 1( int arg 2 ) { super( arg 2 ); } }
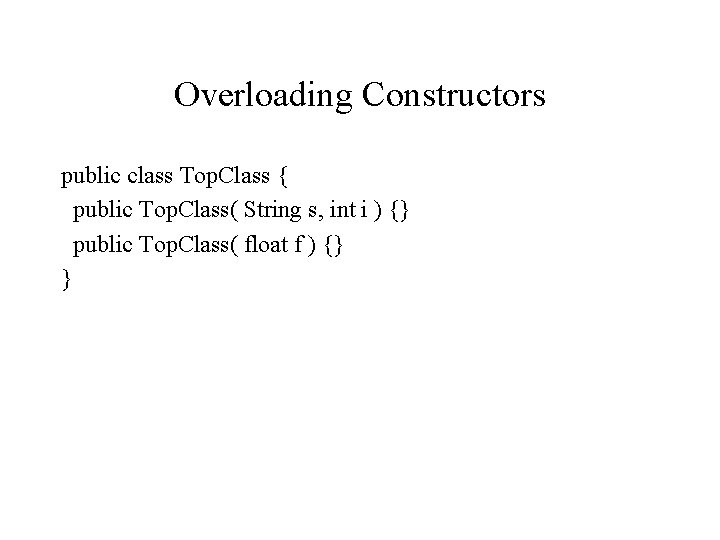
Overloading Constructors public class Top. Class { public Top. Class( String s, int i ) {} public Top. Class( float f ) {} }
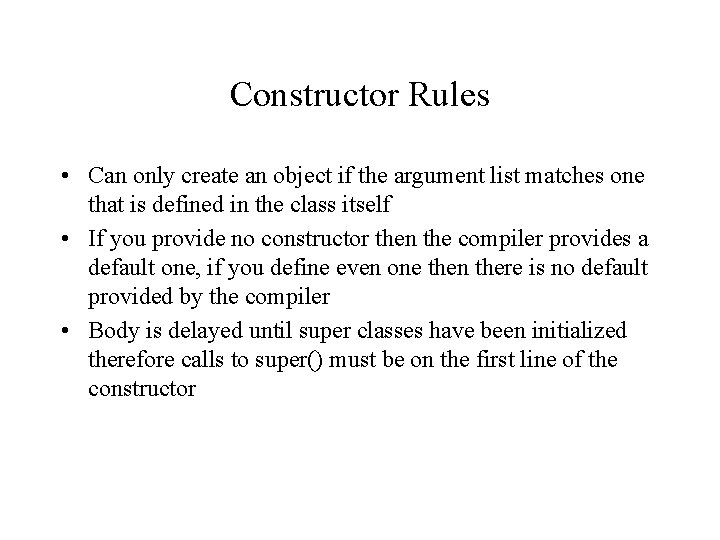
Constructor Rules • Can only create an object if the argument list matches one that is defined in the class itself • If you provide no constructor then the compiler provides a default one, if you define even one then there is no default provided by the compiler • Body is delayed until super classes have been initialized therefore calls to super() must be on the first line of the constructor
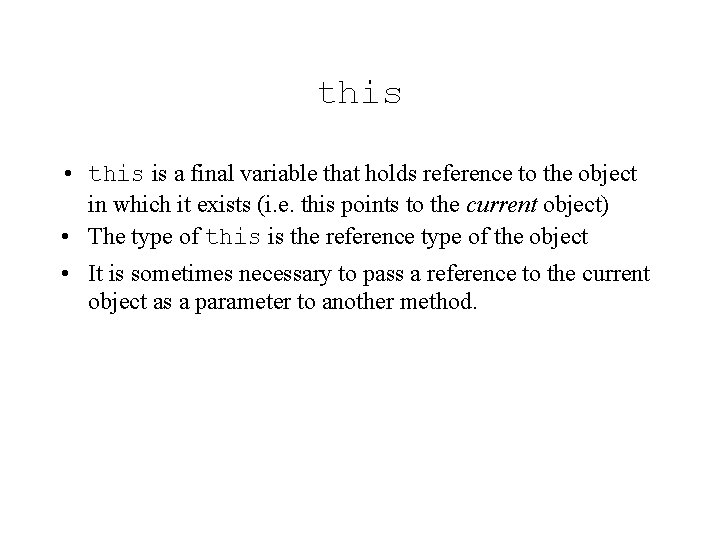
this • this is a final variable that holds reference to the object in which it exists (i. e. this points to the current object) • The type of this is the reference type of the object • It is sometimes necessary to pass a reference to the current object as a parameter to another method.
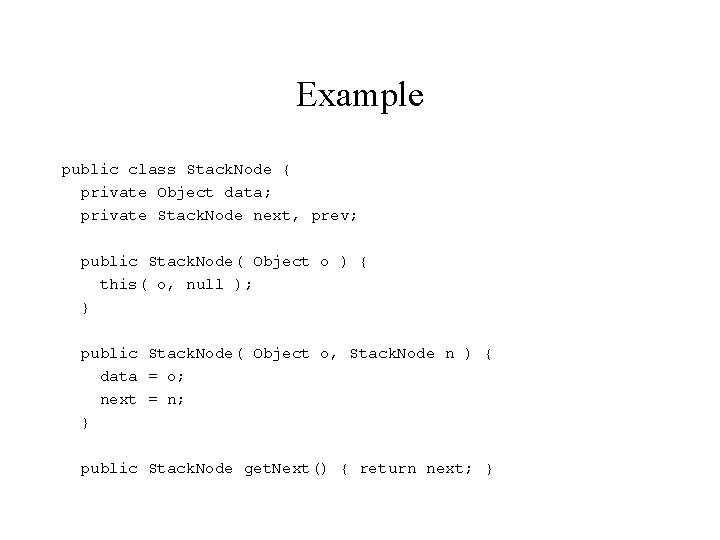
Example public class Stack. Node { private Object data; private Stack. Node next, prev; public Stack. Node( Object o ) { this( o, null ); } public Stack. Node( Object o, Stack. Node n ) { data = o; next = n; } public Stack. Node get. Next() { return next; }
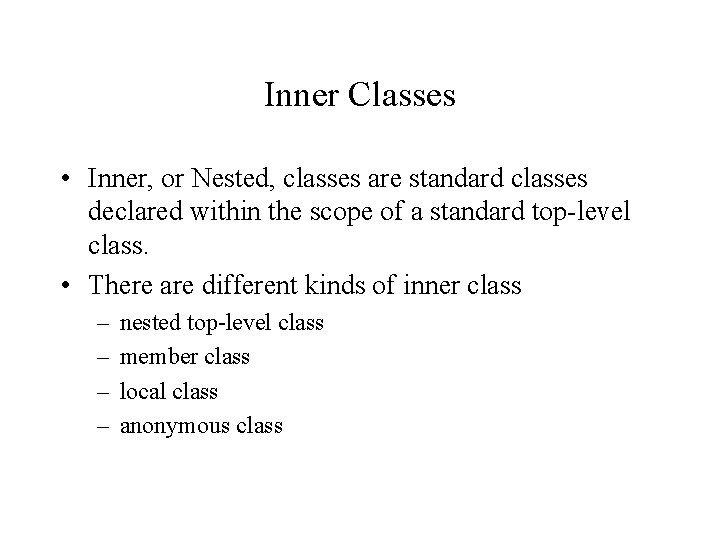
Inner Classes • Inner, or Nested, classes are standard classes declared within the scope of a standard top-level class. • There are different kinds of inner class – – nested top-level class member class local class anonymous class
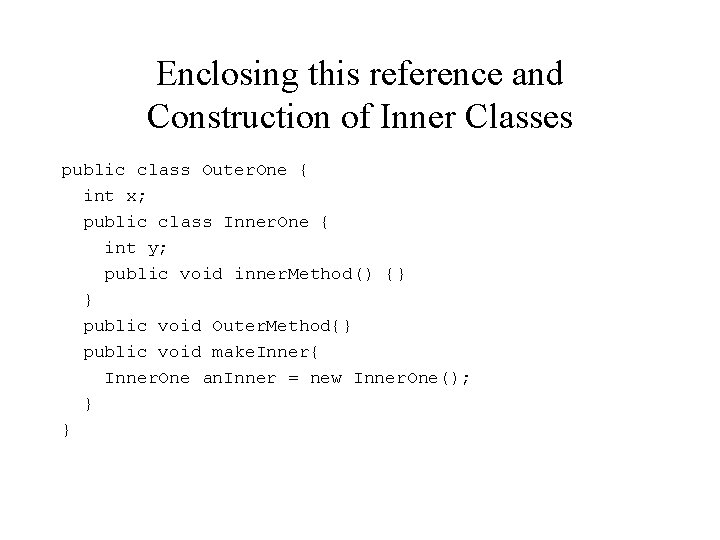
Enclosing this reference and Construction of Inner Classes public class Outer. One { int x; public class Inner. One { int y; public void inner. Method() {} } public void Outer. Method{} public void make. Inner{ Inner. One an. Inner = new Inner. One(); } }
![Continued Public static void main String args Outer One Inner One i Continued…. Public static void main( String args[] ) { Outer. One. Inner. One i](https://slidetodoc.com/presentation_image_h/1ee58508d3ba44cf4c054f165adf004f/image-11.jpg)
Continued…. Public static void main( String args[] ) { Outer. One. Inner. One i = new Outer. One(). new Inner. One(); }
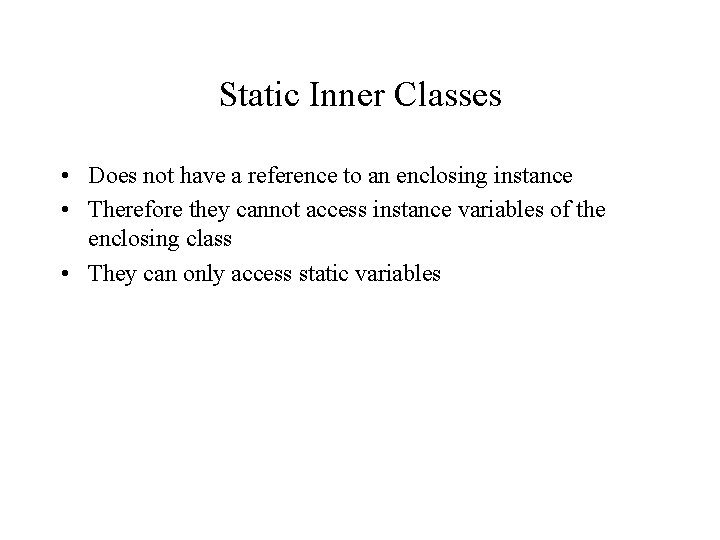
Static Inner Classes • Does not have a reference to an enclosing instance • Therefore they cannot access instance variables of the enclosing class • They can only access static variables
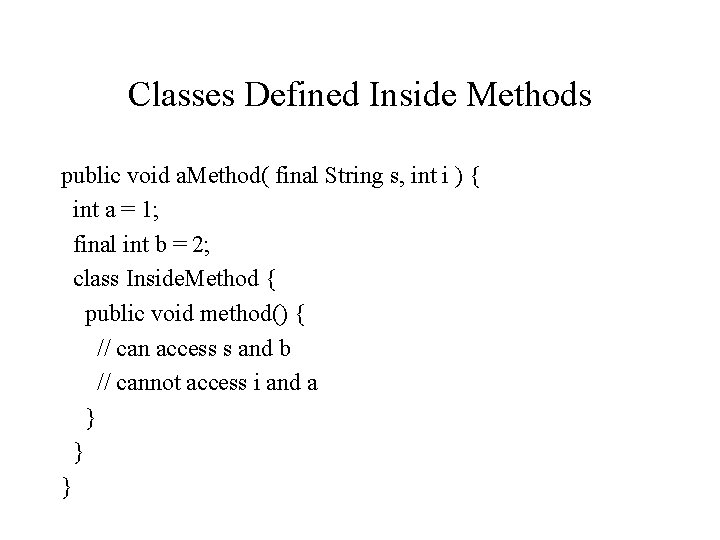
Classes Defined Inside Methods public void a. Method( final String s, int i ) { int a = 1; final int b = 2; class Inside. Method { public void method() { // can access s and b // cannot access i and a } } }
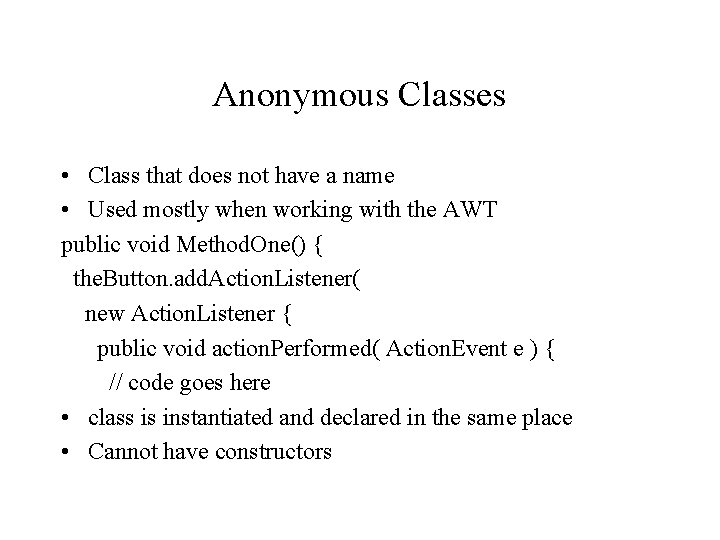
Anonymous Classes • Class that does not have a name • Used mostly when working with the AWT public void Method. One() { the. Button. add. Action. Listener( new Action. Listener { public void action. Performed( Action. Event e ) { // code goes here • class is instantiated and declared in the same place • Cannot have constructors
Overriding and overloading in java
Overriding and overloading
Hierarchical inheritance in java
Overriding vs overloading
Gomco clamp
Debugging has one overriding objective
Overriding member function in c++
Contoh method overloading
Same time same place แปลว่า
Same place same passion
Similar figures have the same but not necessarily the same
Similar polygons formula
Trans name
Method signature consists of
C++ overload parenthesis operator