Event Driven Programming wirh Graphical User Interfaces GUIs
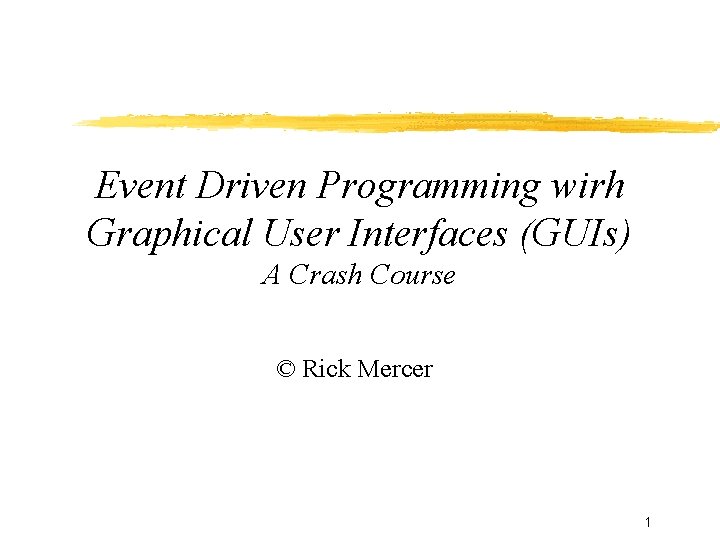
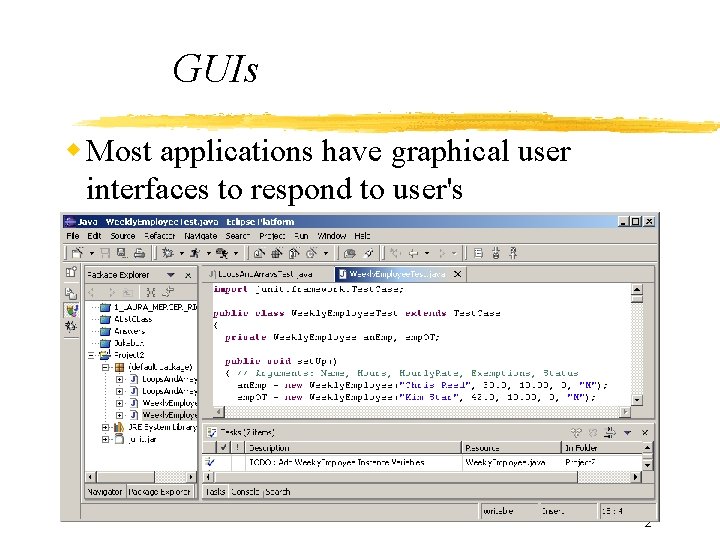
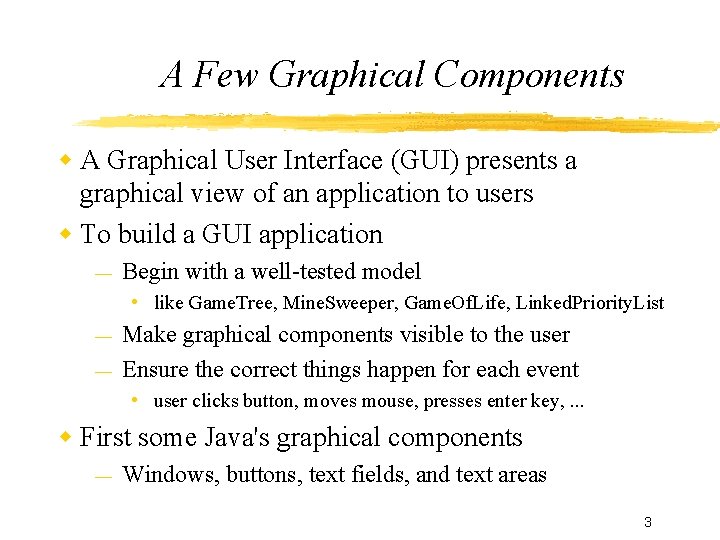
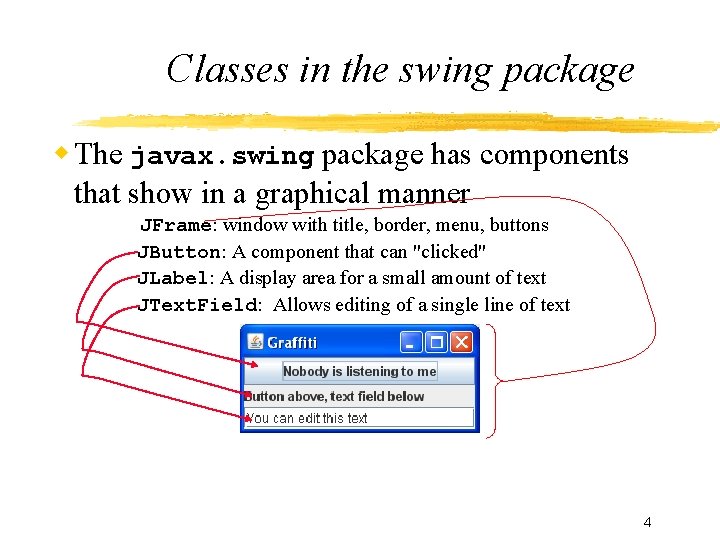
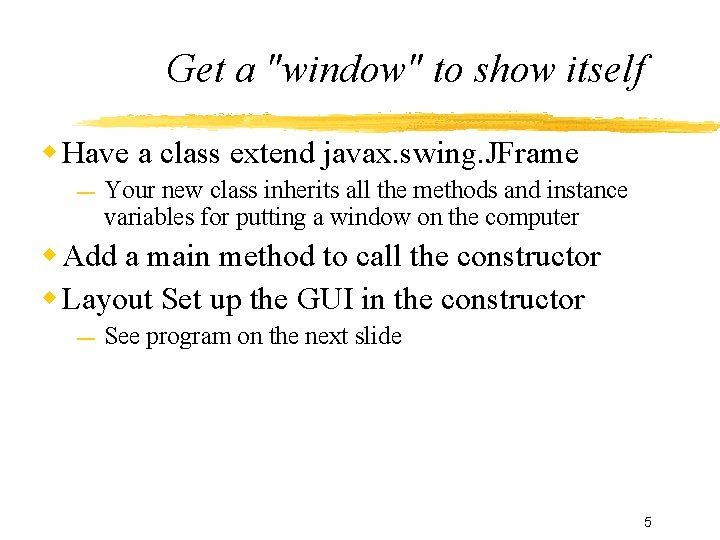
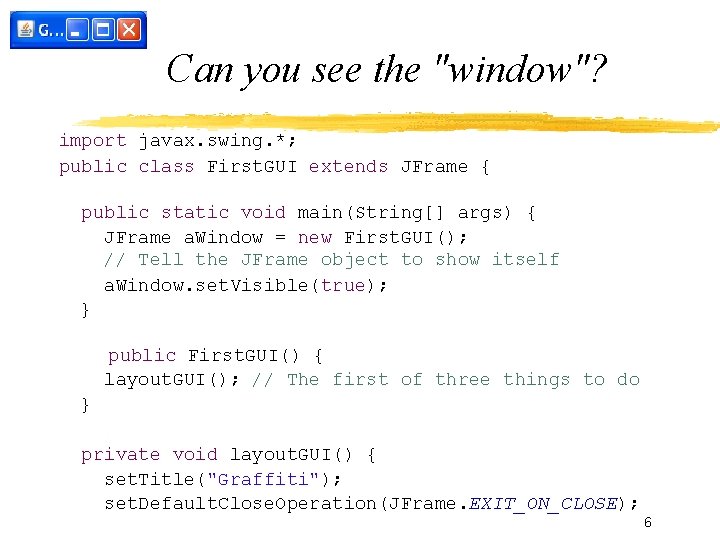
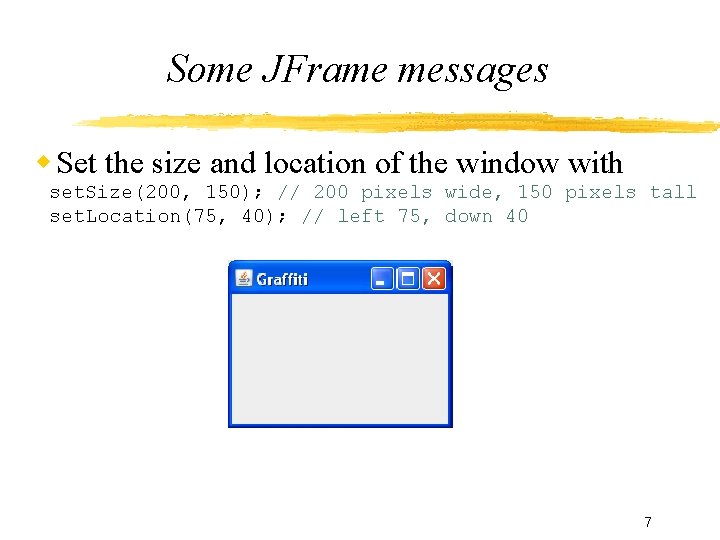
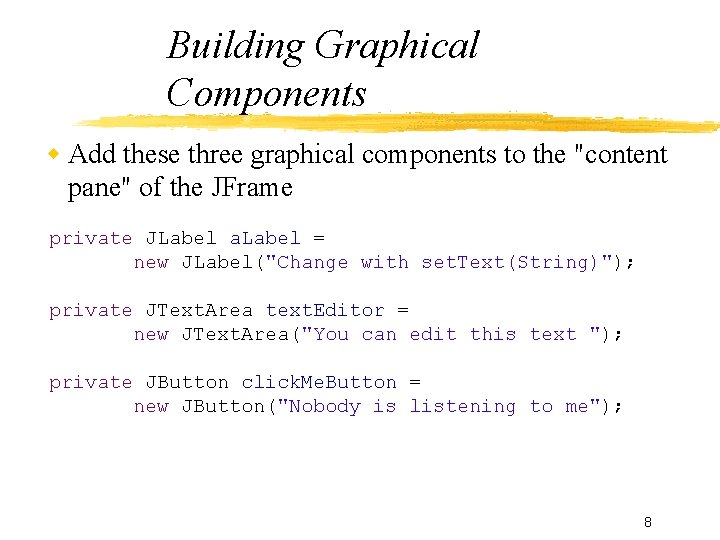
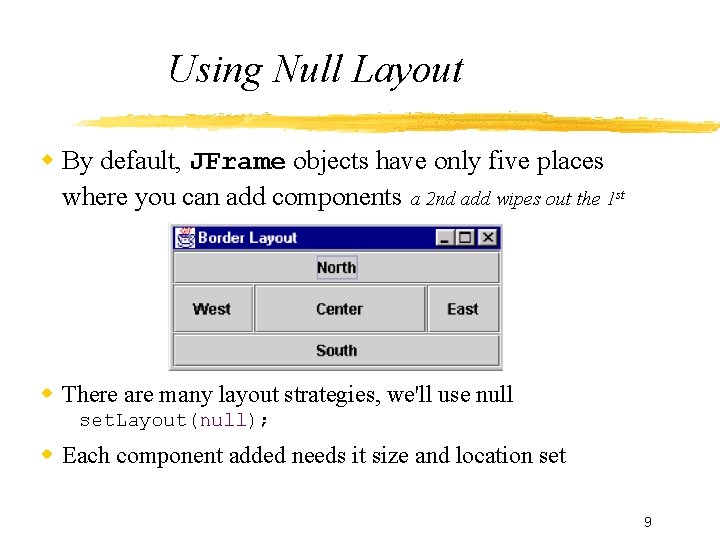
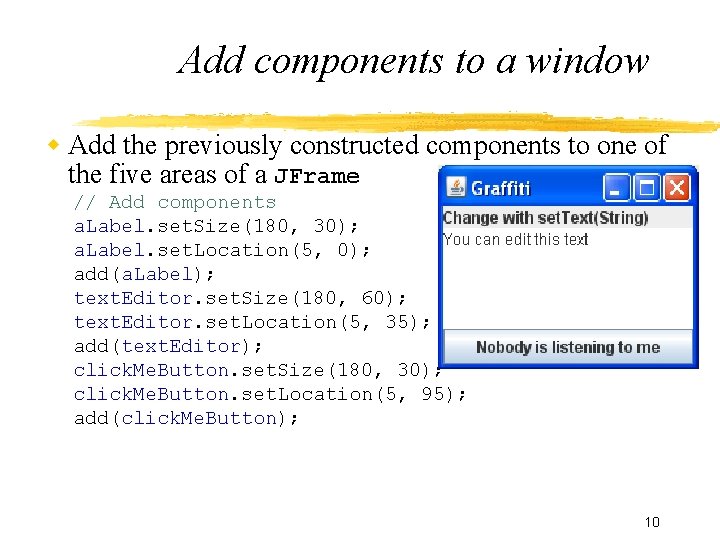
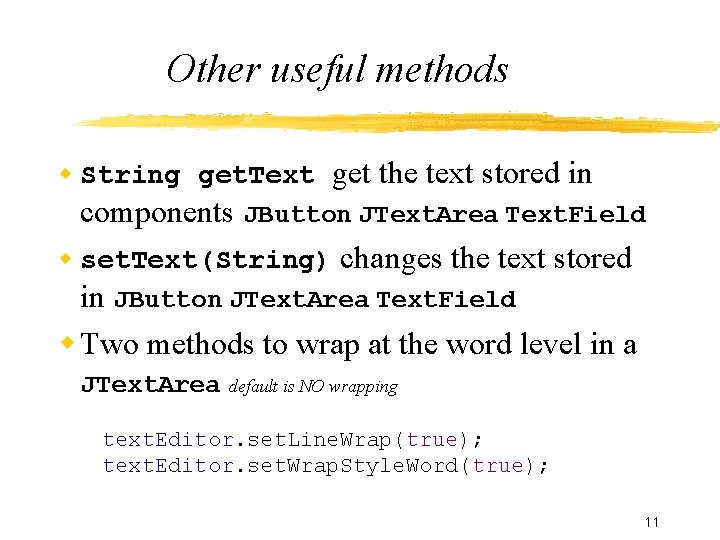
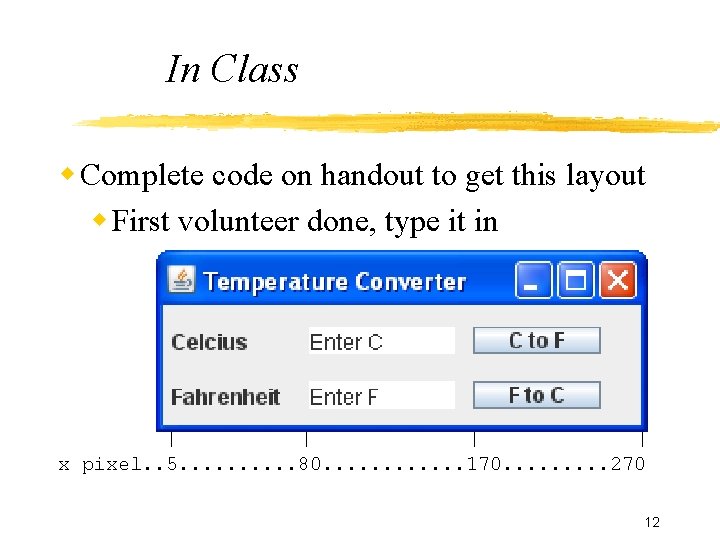
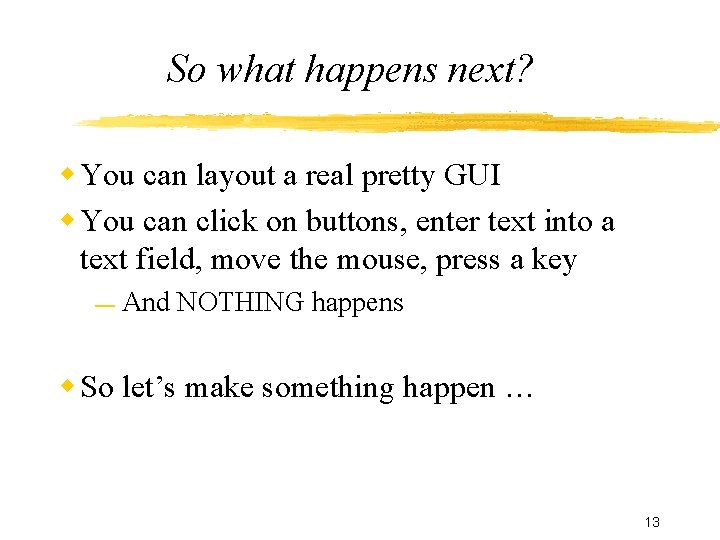
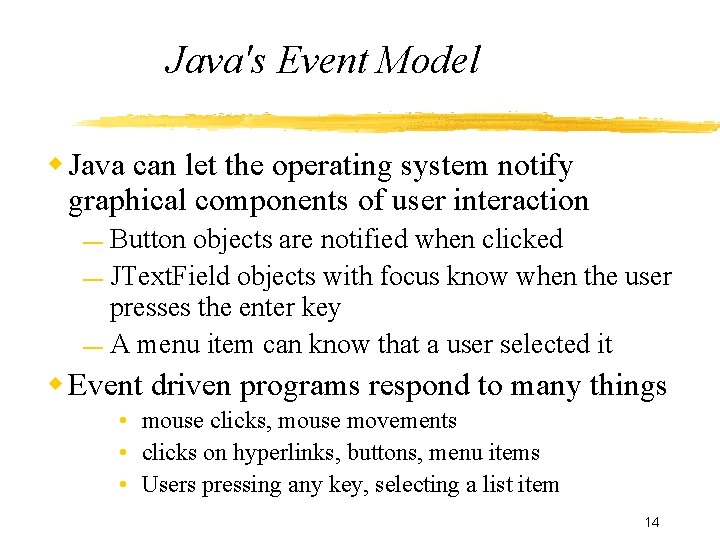
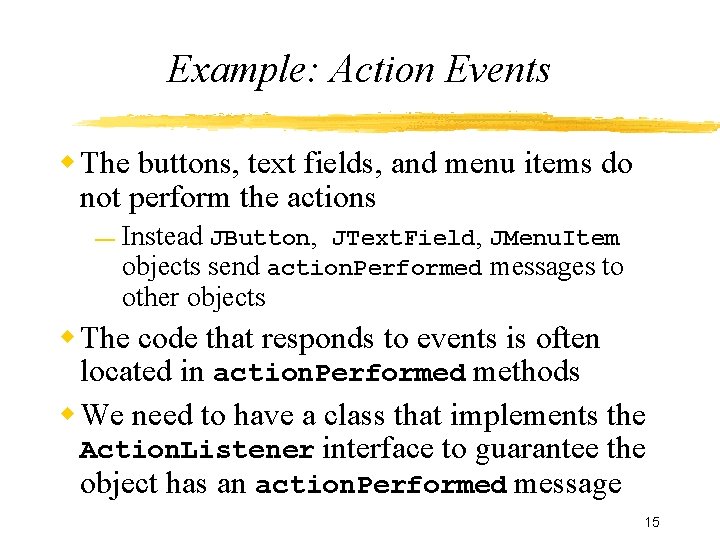
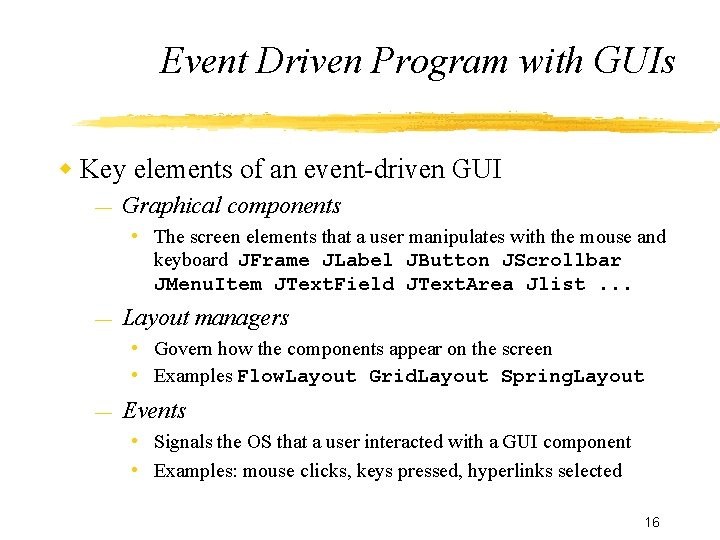
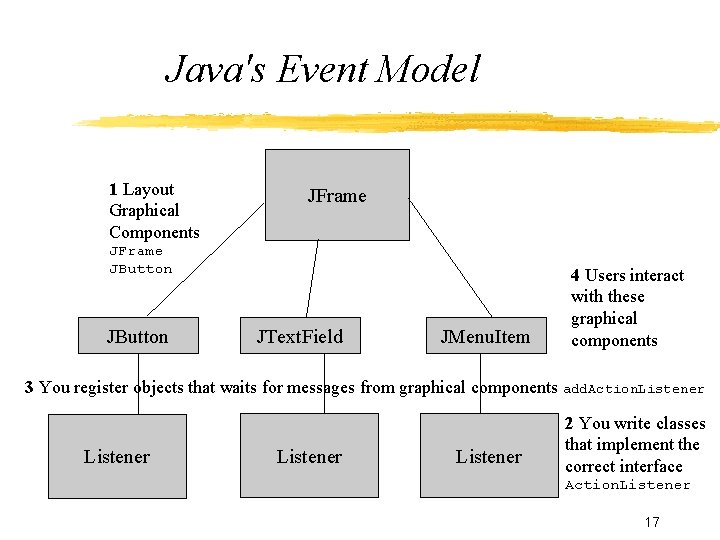
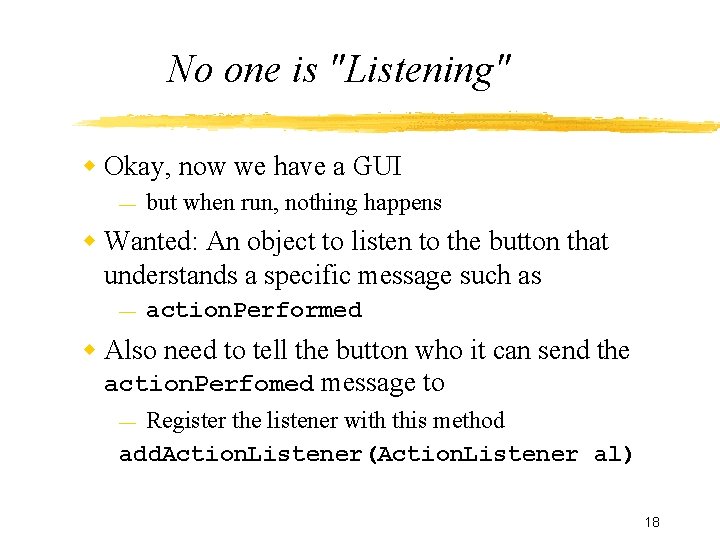
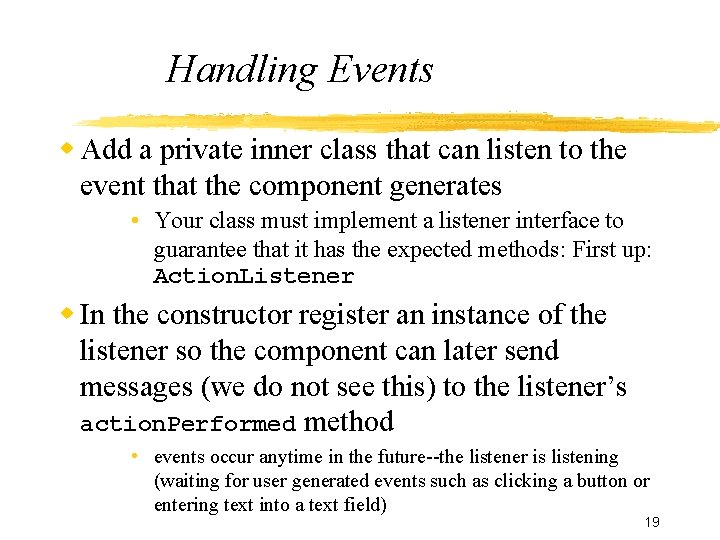
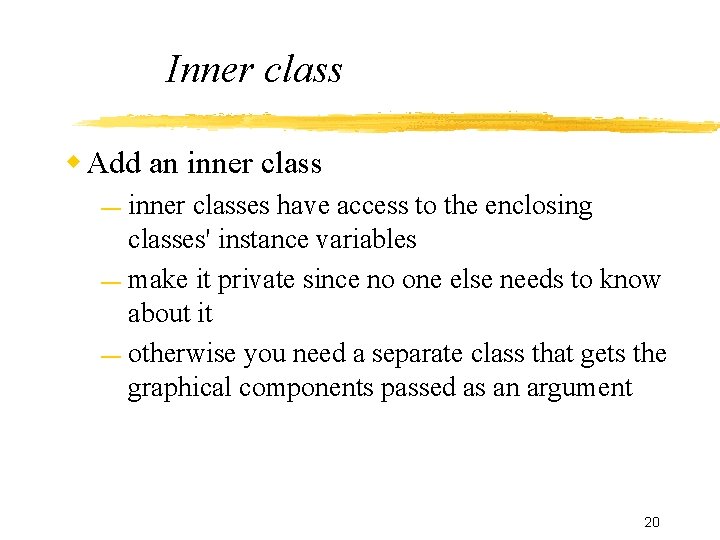
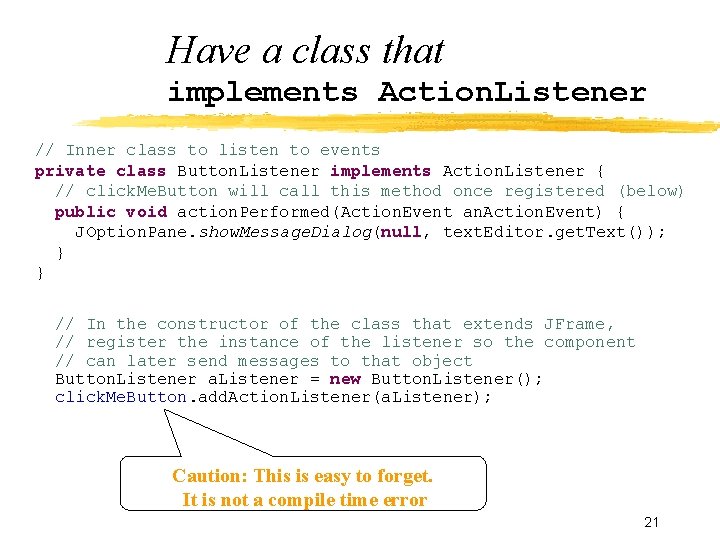
- Slides: 21
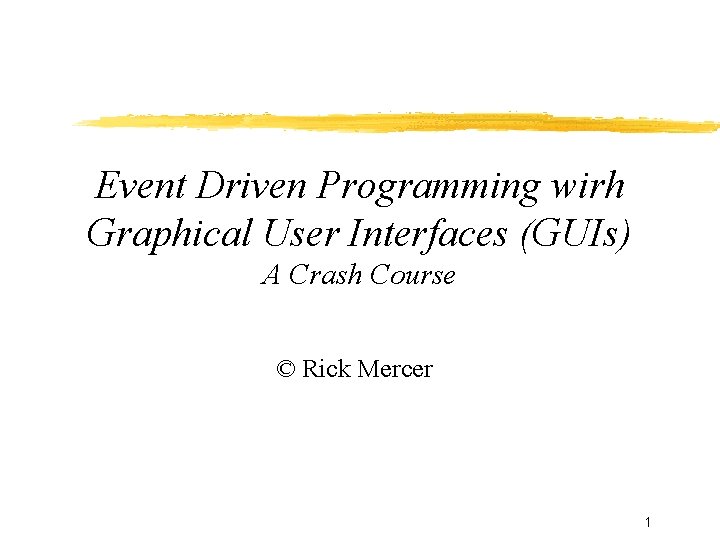
Event Driven Programming wirh Graphical User Interfaces (GUIs) A Crash Course © Rick Mercer 1
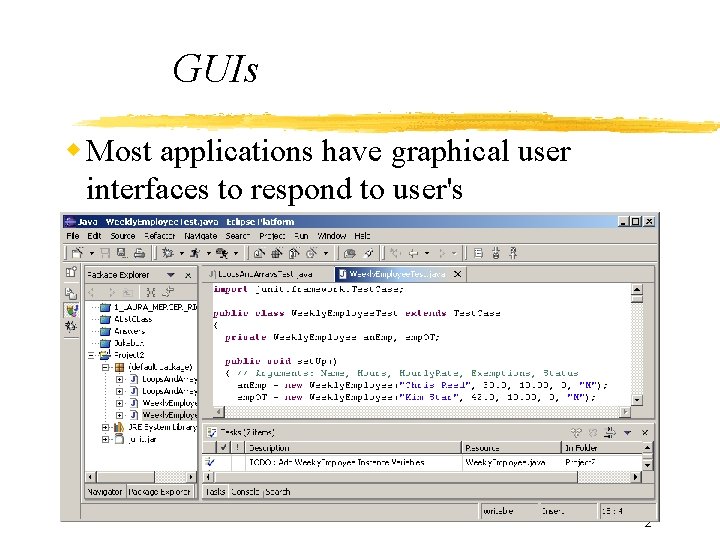
GUIs Most applications have graphical user interfaces to respond to user's 2
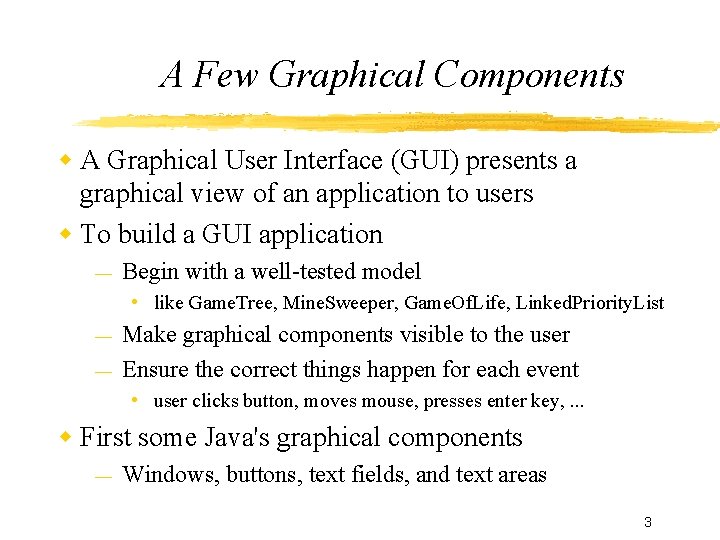
A Few Graphical Components A Graphical User Interface (GUI) presents a graphical view of an application to users To build a GUI application — Begin with a well-tested model • like Game. Tree, Mine. Sweeper, Game. Of. Life, Linked. Priority. List — — Make graphical components visible to the user Ensure the correct things happen for each event • user clicks button, moves mouse, presses enter key, . . . First some Java's graphical components — Windows, buttons, text fields, and text areas 3
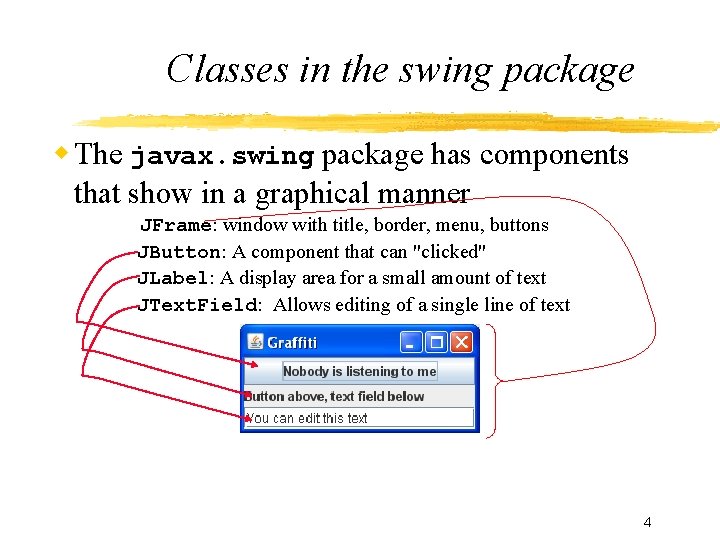
Classes in the swing package The javax. swing package has components that show in a graphical manner JFrame: window with title, border, menu, buttons JButton: A component that can "clicked" JLabel: A display area for a small amount of text JText. Field: Allows editing of a single line of text 4
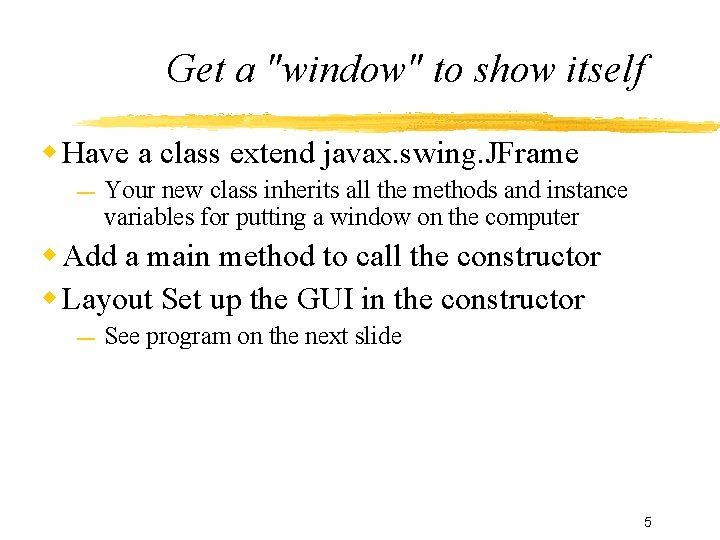
Get a "window" to show itself Have a class extend javax. swing. JFrame — Your new class inherits all the methods and instance variables for putting a window on the computer Add a main method to call the constructor Layout Set up the GUI in the constructor — See program on the next slide 5
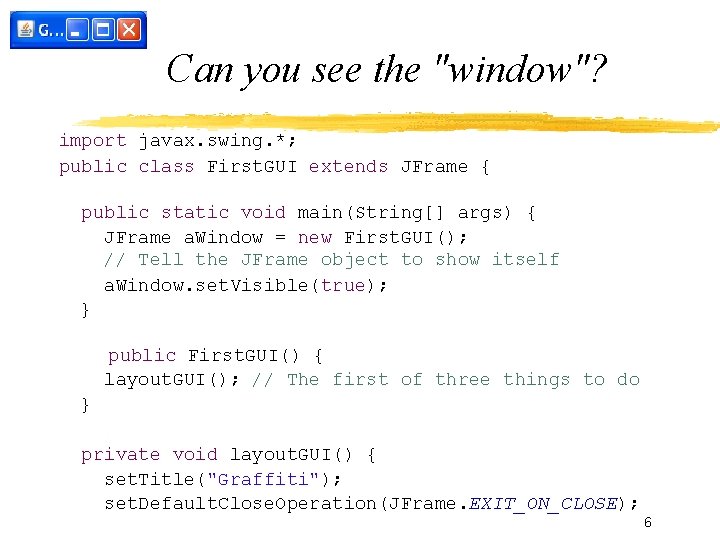
Can you see the "window"? import javax. swing. *; public class First. GUI extends JFrame { public static void main(String[] args) { JFrame a. Window = new First. GUI(); // Tell the JFrame object to show itself a. Window. set. Visible(true); } public First. GUI() { layout. GUI(); // The first of three things to do } private void layout. GUI() { set. Title("Graffiti"); set. Default. Close. Operation(JFrame. EXIT_ON_CLOSE); 6
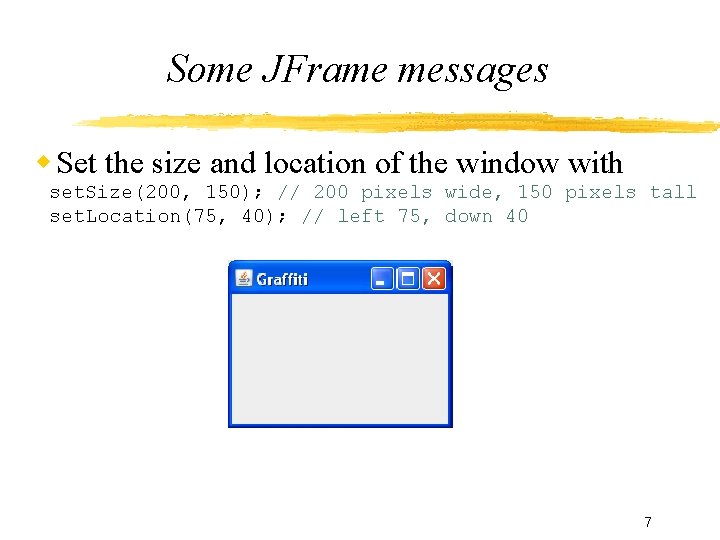
Some JFrame messages Set the size and location of the window with set. Size(200, 150); // 200 pixels wide, 150 pixels tall set. Location(75, 40); // left 75, down 40 7
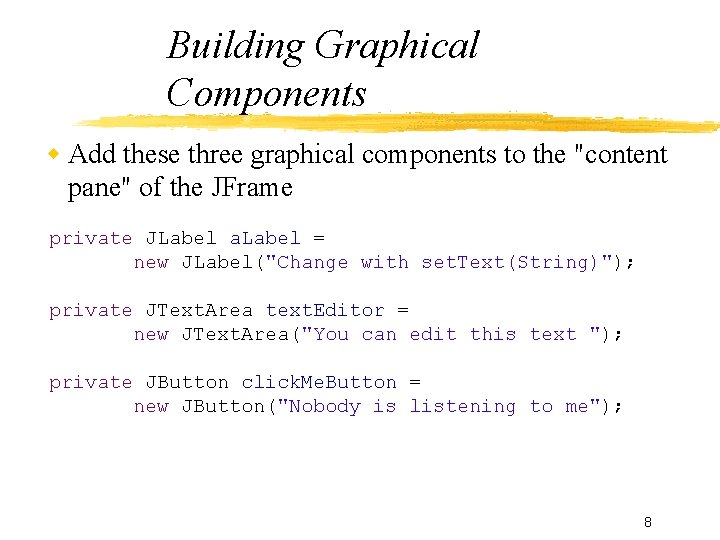
Building Graphical Components Add these three graphical components to the "content pane" of the JFrame private JLabel a. Label = new JLabel("Change with set. Text(String)"); private JText. Area text. Editor = new JText. Area("You can edit this text "); private JButton click. Me. Button = new JButton("Nobody is listening to me"); 8
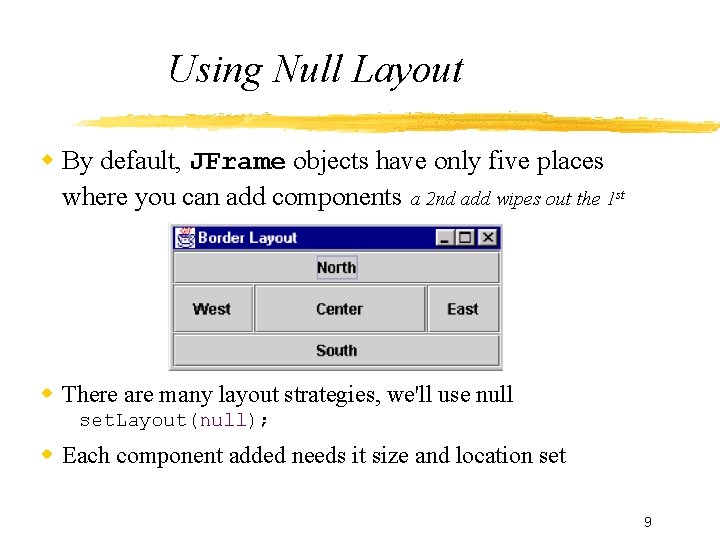
Using Null Layout By default, JFrame objects have only five places where you can add components a 2 nd add wipes out the 1 st There are many layout strategies, we'll use null set. Layout(null); Each component added needs it size and location set 9
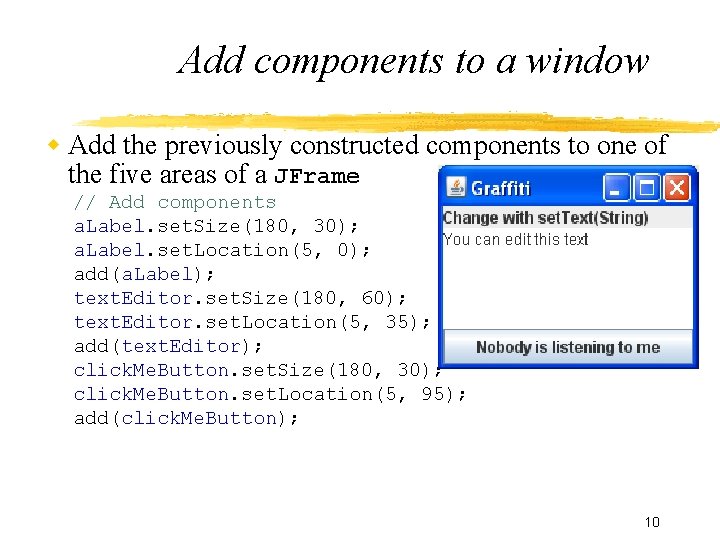
Add components to a window Add the previously constructed components to one of the five areas of a JFrame // Add components a. Label. set. Size(180, 30); a. Label. set. Location(5, 0); add(a. Label); text. Editor. set. Size(180, 60); text. Editor. set. Location(5, 35); add(text. Editor); click. Me. Button. set. Size(180, 30); click. Me. Button. set. Location(5, 95); add(click. Me. Button); 10
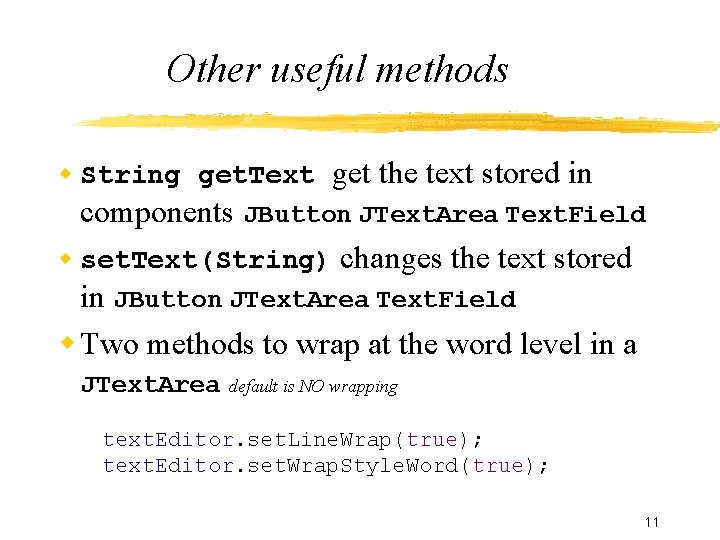
Other useful methods String get. Text get the text stored in components JButton JText. Area Text. Field set. Text(String) changes the text stored in JButton JText. Area Text. Field Two methods to wrap at the word level in a JText. Area default is NO wrapping text. Editor. set. Line. Wrap(true); text. Editor. set. Wrap. Style. Word(true); 11
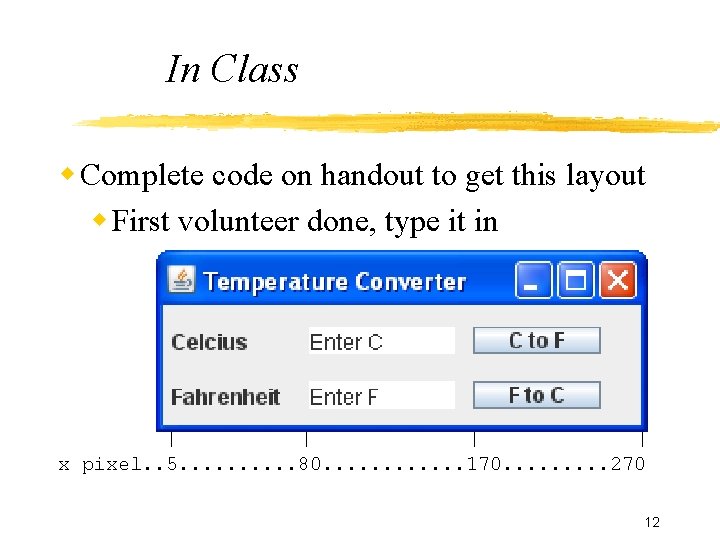
In Class Complete code on handout to get this layout First volunteer done, type it in | | x pixel. . 5. . 80. . . 170. . 270 12
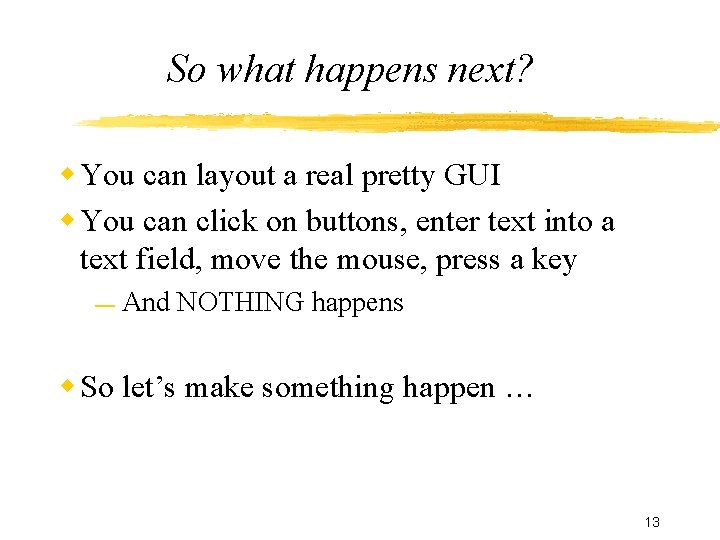
So what happens next? You can layout a real pretty GUI You can click on buttons, enter text into a text field, move the mouse, press a key — And NOTHING happens So let’s make something happen … 13
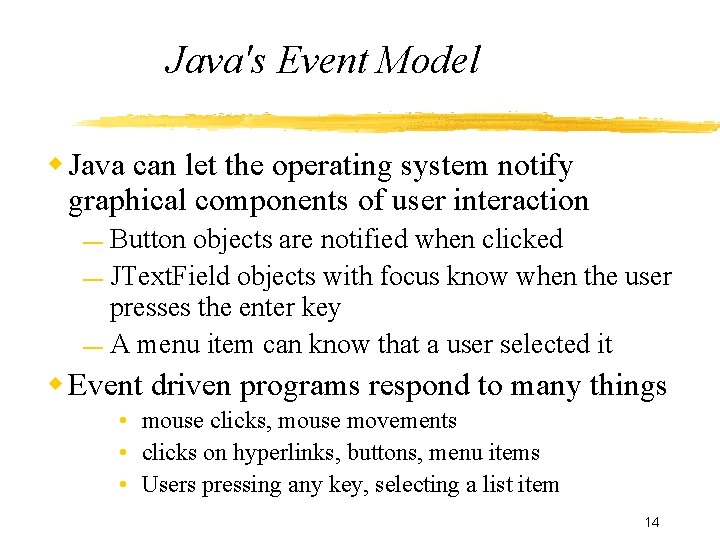
Java's Event Model Java can let the operating system notify graphical components of user interaction Button objects are notified when clicked — JText. Field objects with focus know when the user presses the enter key — A menu item can know that a user selected it — Event driven programs respond to many things • mouse clicks, mouse movements • clicks on hyperlinks, buttons, menu items • Users pressing any key, selecting a list item 14
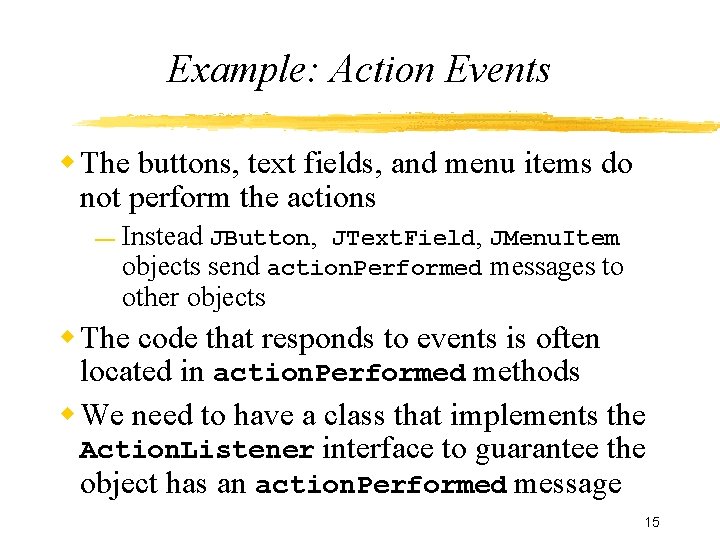
Example: Action Events The buttons, text fields, and menu items do not perform the actions — Instead JButton, JText. Field, JMenu. Item objects send action. Performed messages to other objects The code that responds to events is often located in action. Performed methods We need to have a class that implements the Action. Listener interface to guarantee the object has an action. Performed message 15
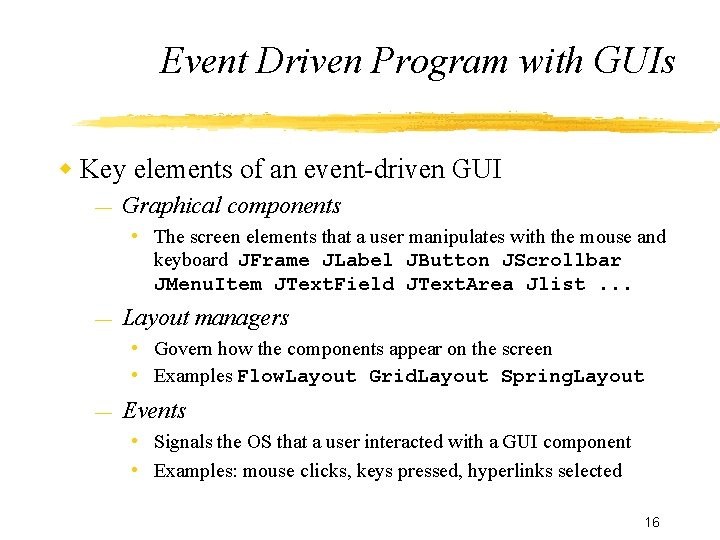
Event Driven Program with GUIs Key elements of an event-driven GUI — Graphical components • The screen elements that a user manipulates with the mouse and keyboard JFrame JLabel JButton JScrollbar JMenu. Item JText. Field JText. Area Jlist. . . — Layout managers • Govern how the components appear on the screen • Examples Flow. Layout Grid. Layout Spring. Layout — Events • Signals the OS that a user interacted with a GUI component • Examples: mouse clicks, keys pressed, hyperlinks selected 16
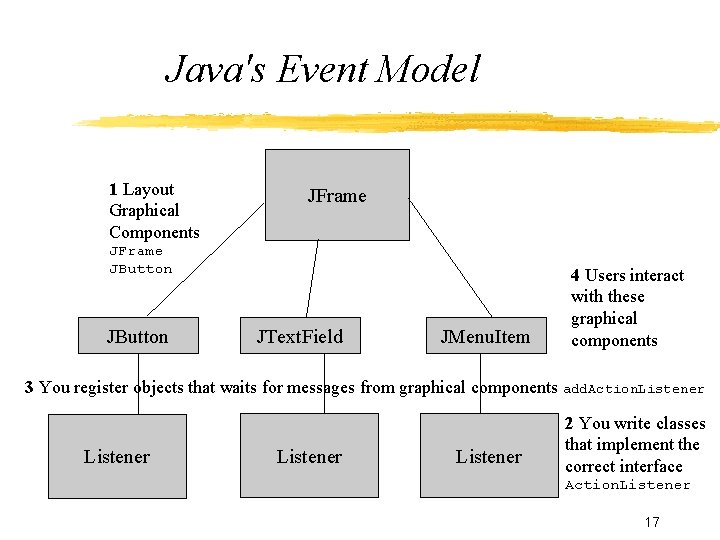
Java's Event Model 1 Layout Graphical Components JFrame JButton JText. Field JMenu. Item 4 Users interact with these graphical components 3 You register objects that waits for messages from graphical components add. Action. Listener 2 You write classes that implement the correct interface Action. Listener 17
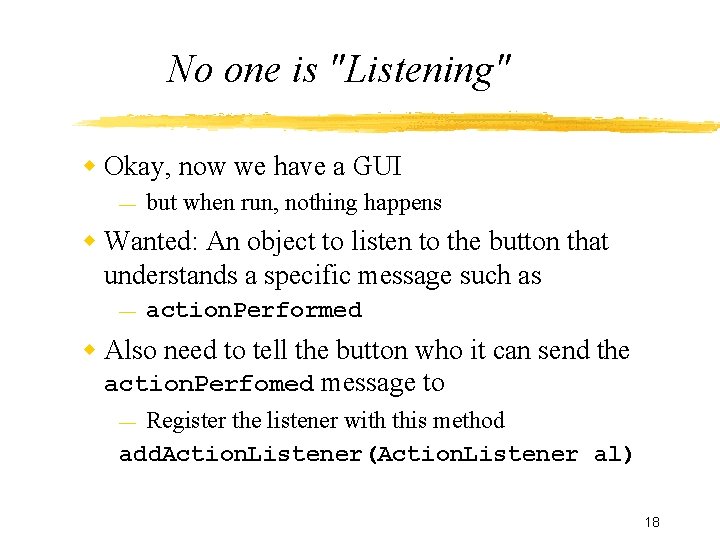
No one is "Listening" Okay, now we have a GUI — but when run, nothing happens Wanted: An object to listen to the button that understands a specific message such as — action. Performed Also need to tell the button who it can send the action. Perfomed message to Register the listener with this method add. Action. Listener(Action. Listener al) — 18
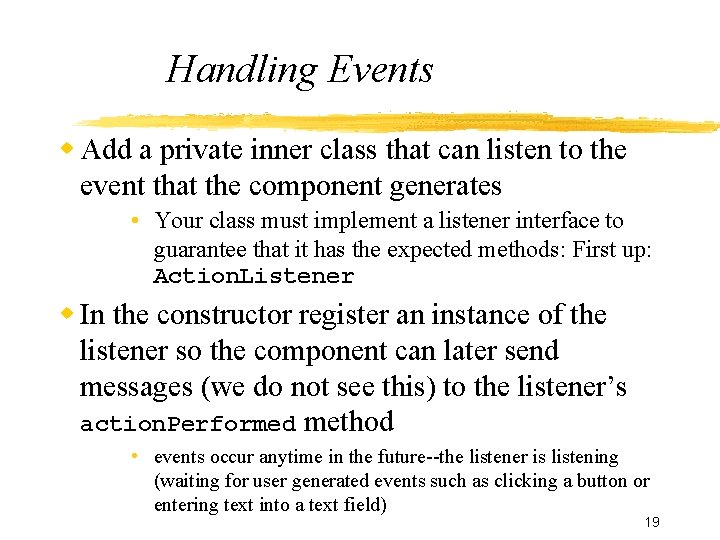
Handling Events Add a private inner class that can listen to the event that the component generates • Your class must implement a listener interface to guarantee that it has the expected methods: First up: Action. Listener In the constructor register an instance of the listener so the component can later send messages (we do not see this) to the listener’s action. Performed method • events occur anytime in the future--the listener is listening (waiting for user generated events such as clicking a button or entering text into a text field) 19
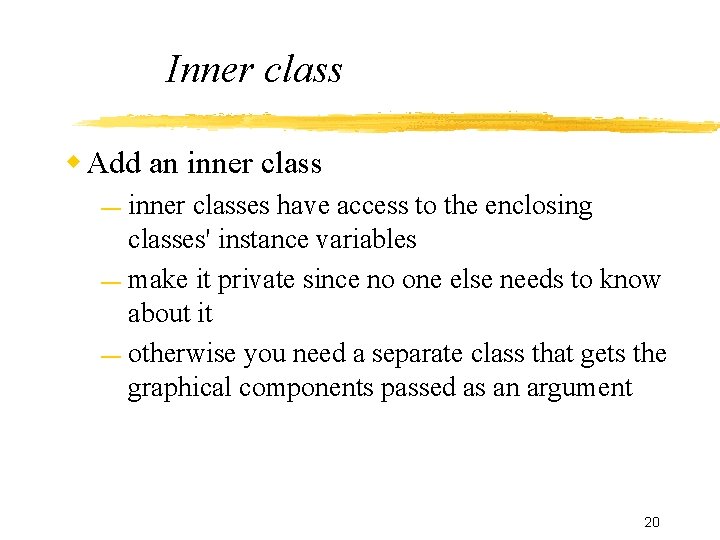
Inner class Add an inner classes have access to the enclosing classes' instance variables — make it private since no one else needs to know about it — otherwise you need a separate class that gets the graphical components passed as an argument — 20
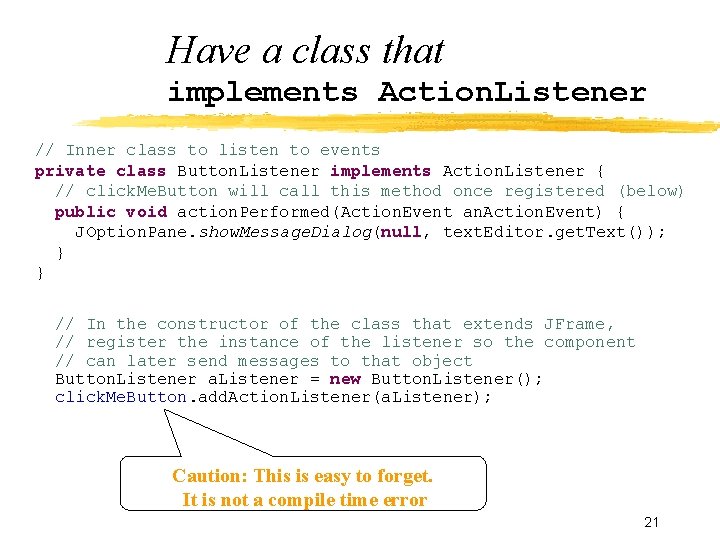
Have a class that implements Action. Listener // Inner class to listen to events private class Button. Listener implements Action. Listener { // click. Me. Button will call this method once registered (below) public void action. Performed(Action. Event an. Action. Event) { JOption. Pane. show. Message. Dialog(null, text. Editor. get. Text()); } } // In the constructor of the class that extends JFrame, // register the instance of the listener so the component // can later send messages to that object Button. Listener a. Listener = new Button. Listener(); click. Me. Button. add. Action. Listener(a. Listener); Caution: This is easy to forget. It is not a compile time error 21
Programming graphical user interfaces in r
Sequential program and an event-driven program?
Event driven programming paradigm
Event driven programming in java
Event driven programming in java
Heuristic evaluation of user interfaces
User interfaces design dc
Web based interface
Why are user interfaces hard to implement
Common gui event types and listener interfaces in java
Characteristics of graphical user interface
Gui graphics
Principles of user interface design
Characteristics of graphical user interface
Java graphical user interface
Jpanel
Graphical user interface testing
User interface history
Components of graphical user interface
Graphical user interface design principles
Graphical user interface testing tools
Idioms