EEL 3801 Part VIII Fundamentals of C and
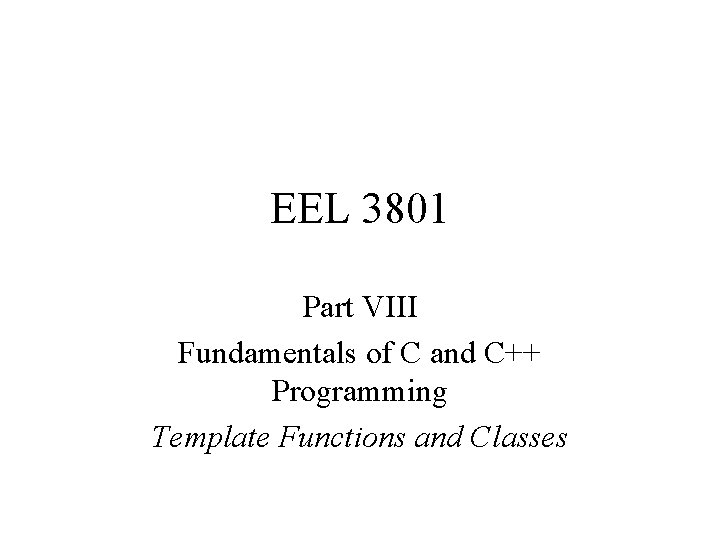
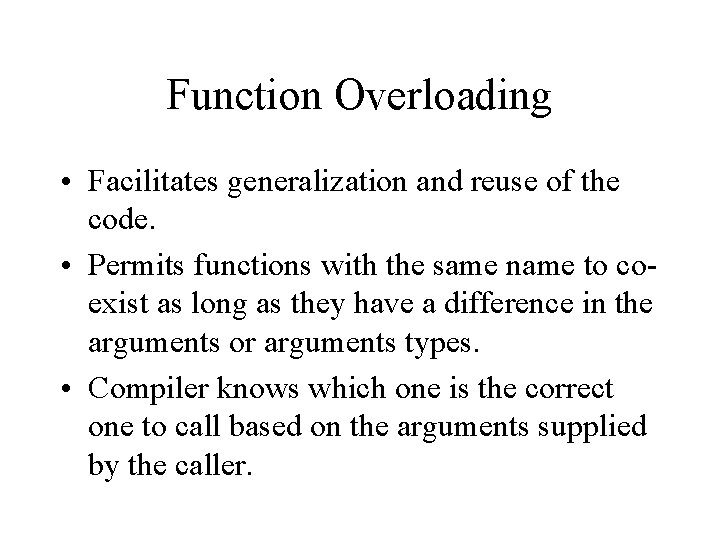
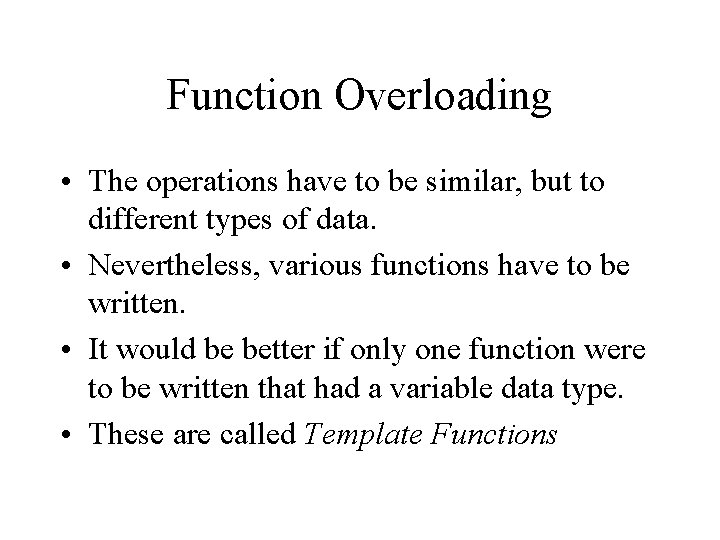
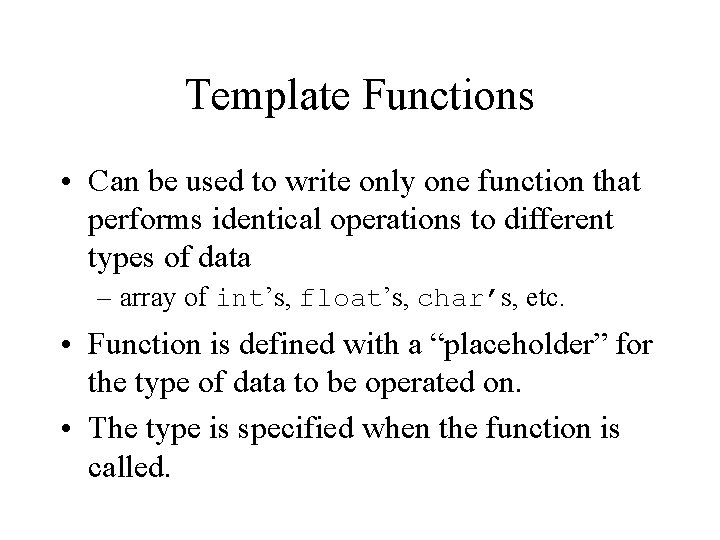
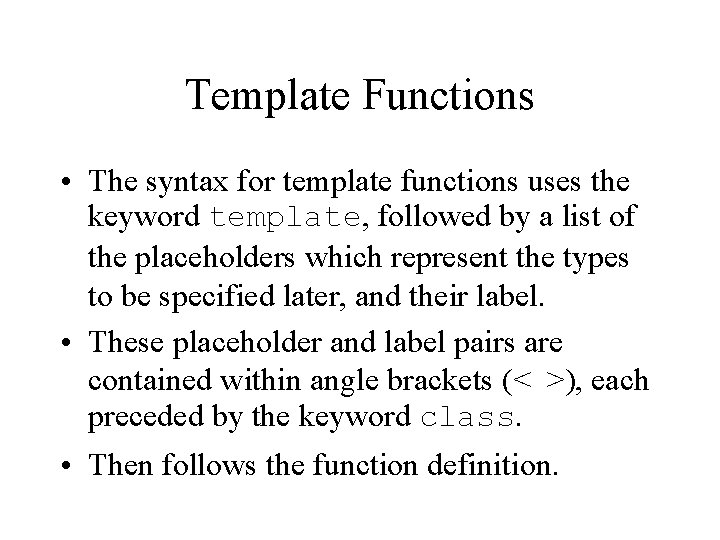
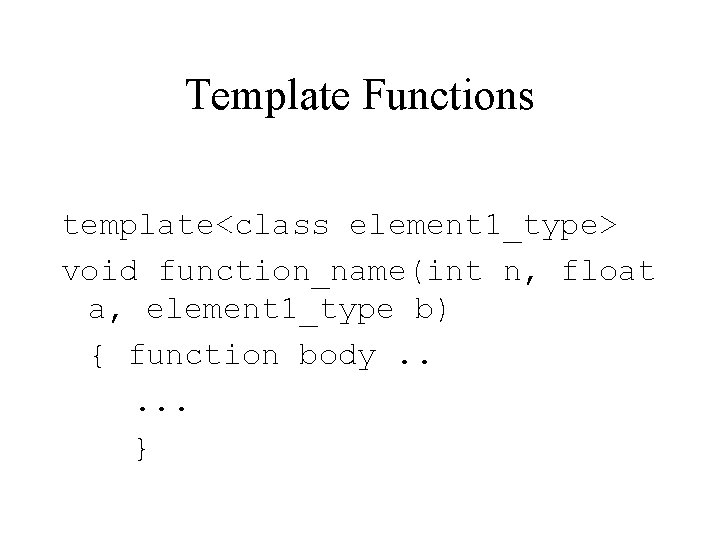
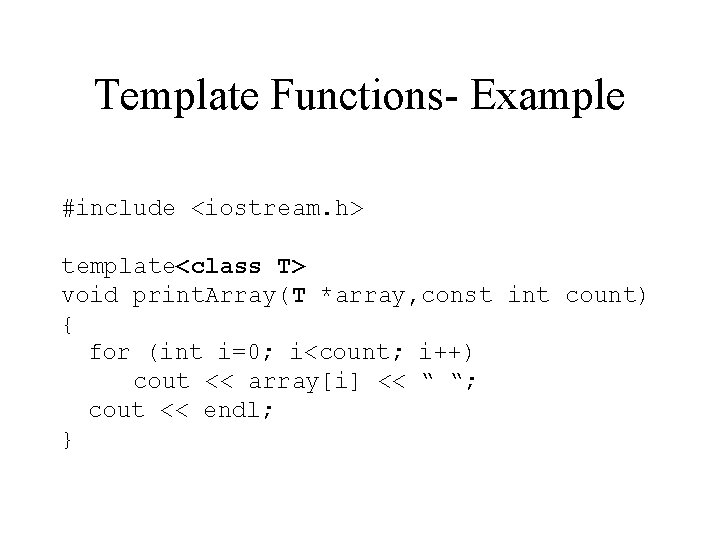
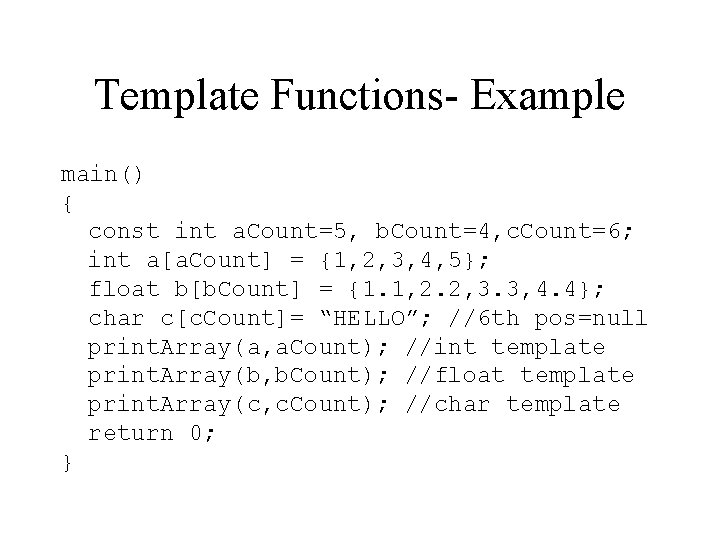
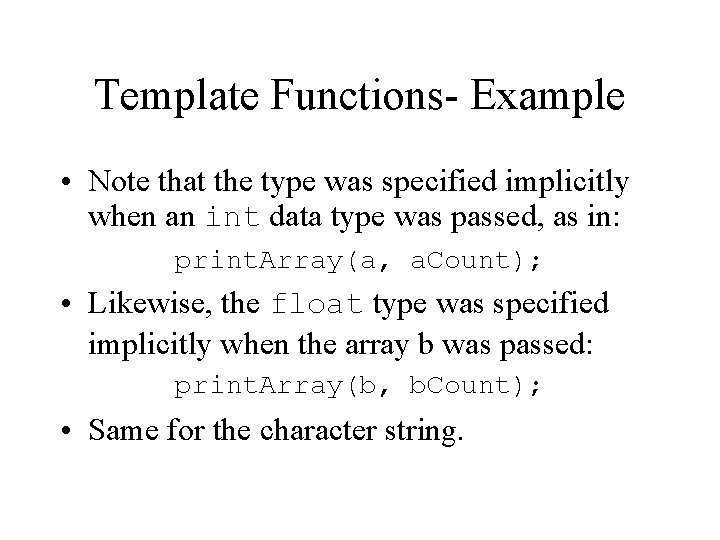
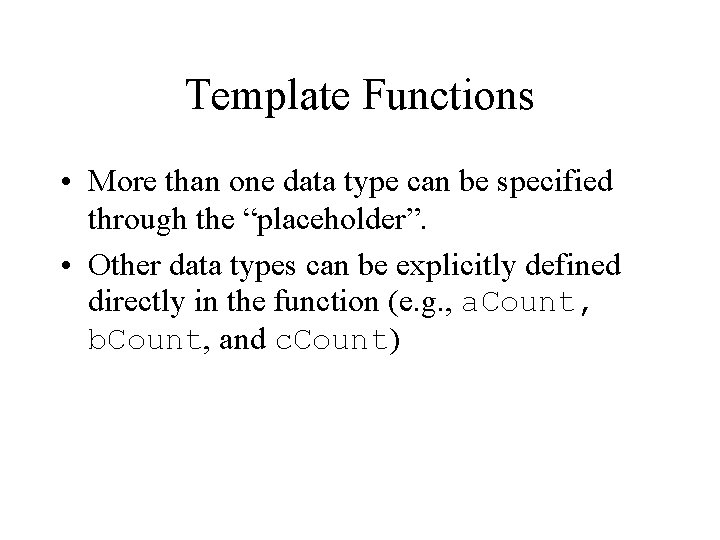
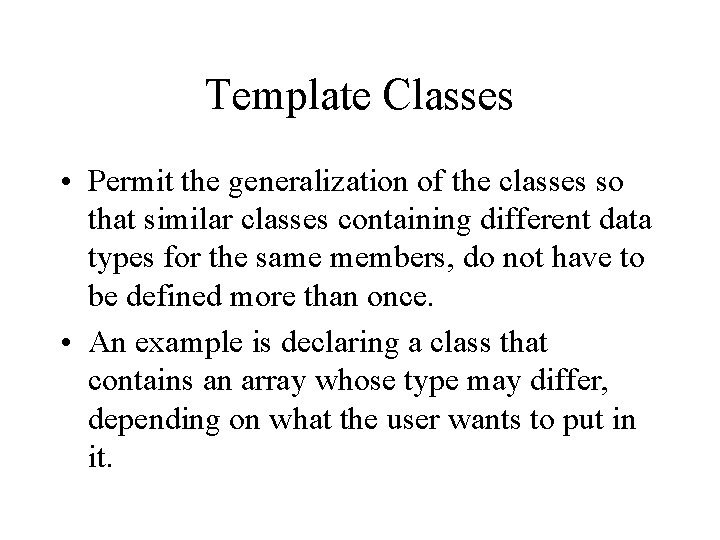
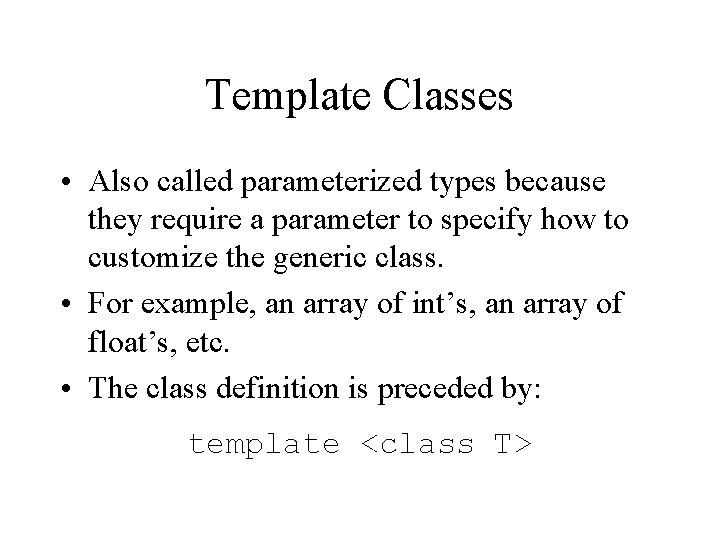
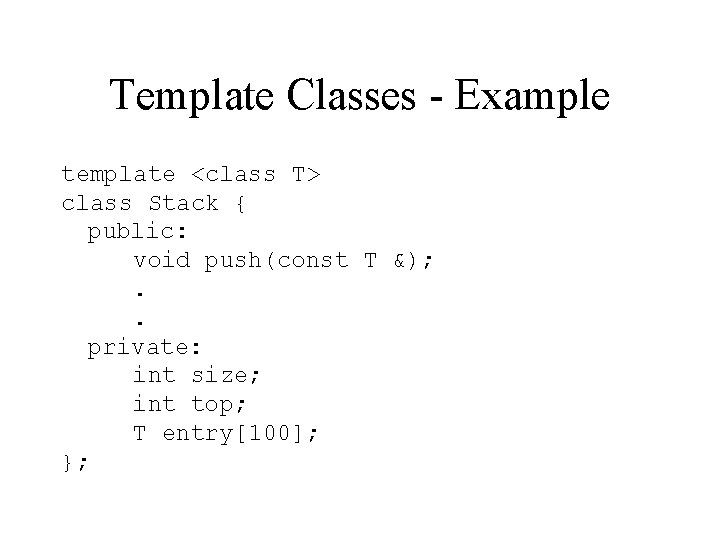
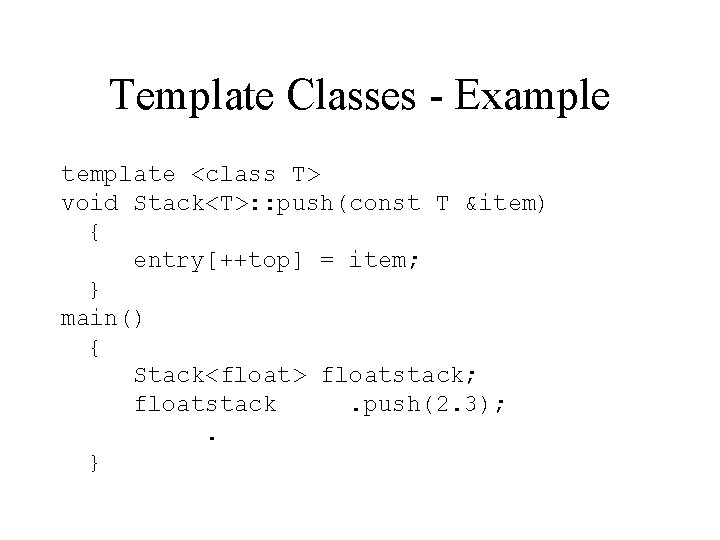
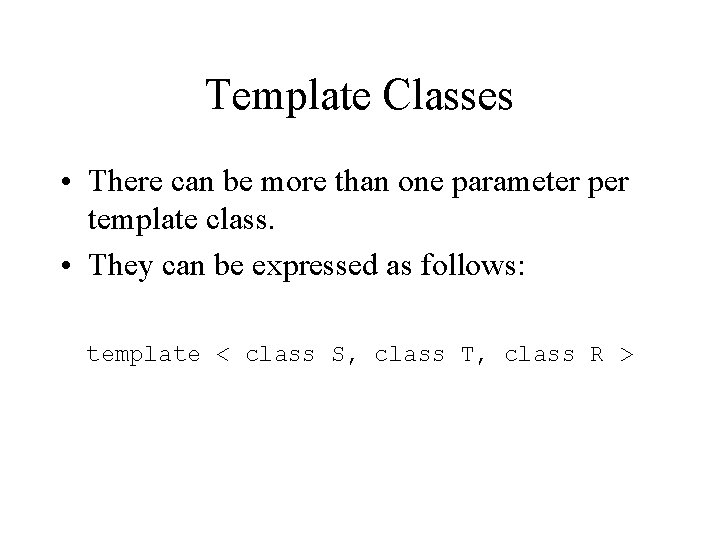
- Slides: 15
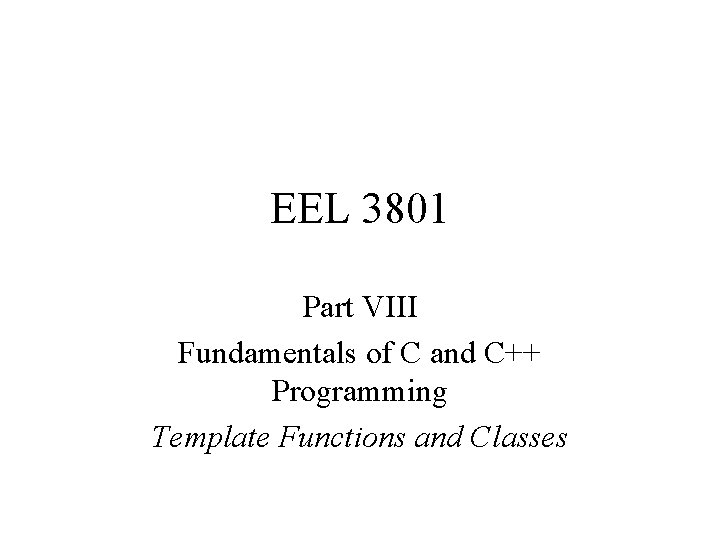
EEL 3801 Part VIII Fundamentals of C and C++ Programming Template Functions and Classes
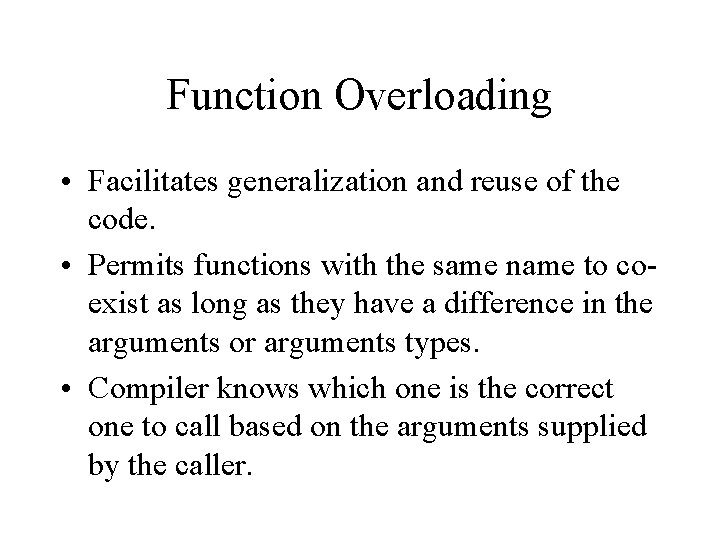
Function Overloading • Facilitates generalization and reuse of the code. • Permits functions with the same name to coexist as long as they have a difference in the arguments or arguments types. • Compiler knows which one is the correct one to call based on the arguments supplied by the caller.
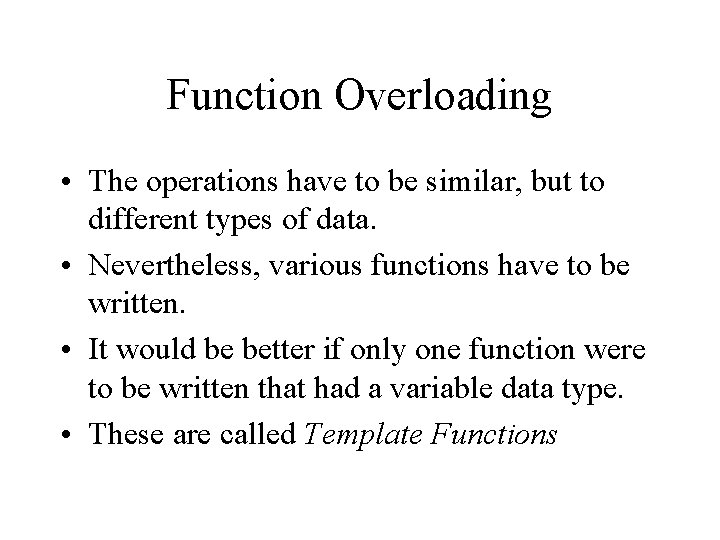
Function Overloading • The operations have to be similar, but to different types of data. • Nevertheless, various functions have to be written. • It would be better if only one function were to be written that had a variable data type. • These are called Template Functions
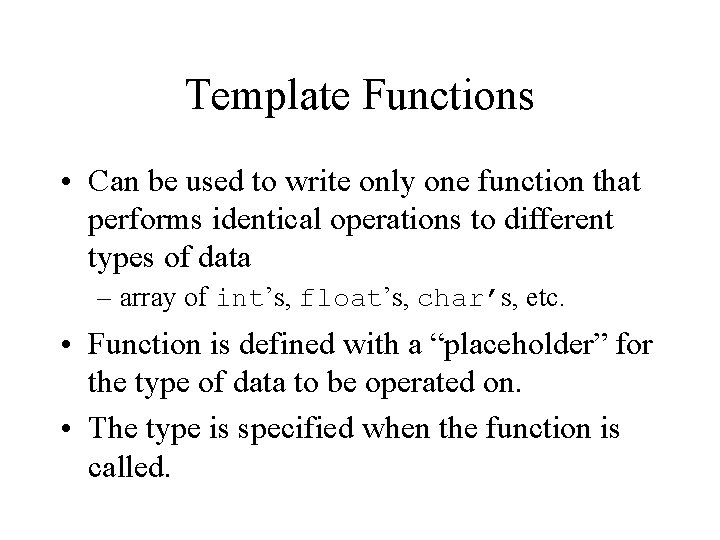
Template Functions • Can be used to write only one function that performs identical operations to different types of data – array of int’s, float’s, char’s, etc. • Function is defined with a “placeholder” for the type of data to be operated on. • The type is specified when the function is called.
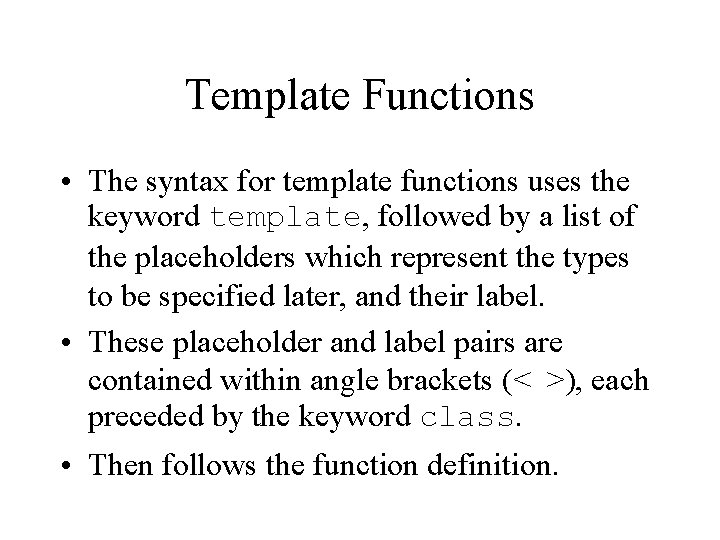
Template Functions • The syntax for template functions uses the keyword template, followed by a list of the placeholders which represent the types to be specified later, and their label. • These placeholder and label pairs are contained within angle brackets (< >), each preceded by the keyword class. • Then follows the function definition.
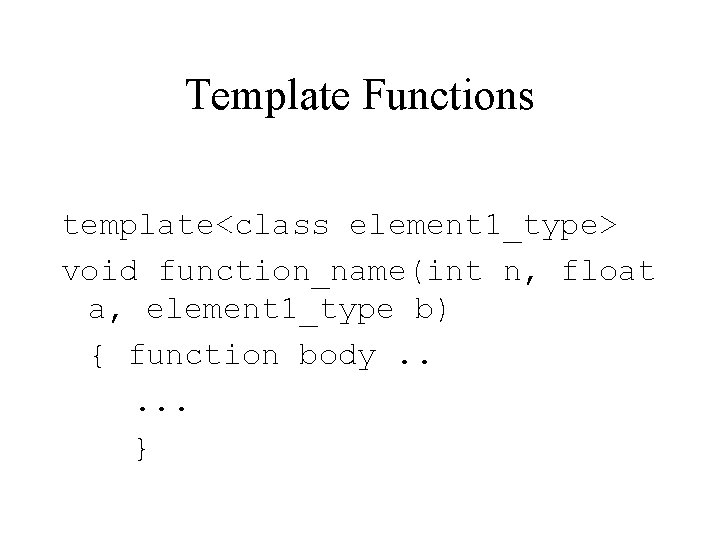
Template Functions template<class element 1_type> void function_name(int n, float a, element 1_type b) { function body. . . }
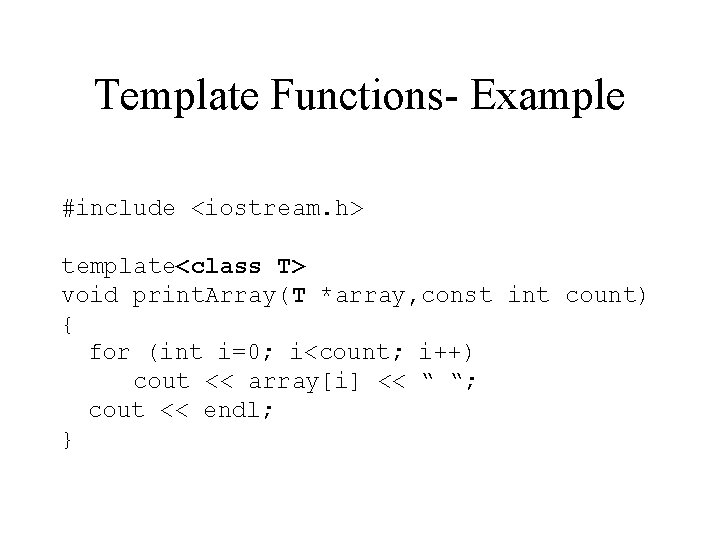
Template Functions- Example #include <iostream. h> template<class T> void print. Array(T *array, const int count) { for (int i=0; i<count; i++) cout << array[i] << “ “; cout << endl; }
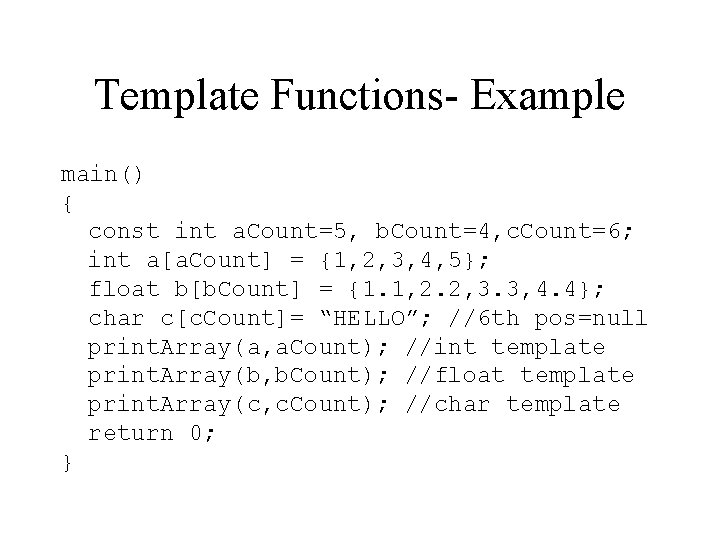
Template Functions- Example main() { const int a. Count=5, b. Count=4, c. Count=6; int a[a. Count] = {1, 2, 3, 4, 5}; float b[b. Count] = {1. 1, 2. 2, 3. 3, 4. 4}; char c[c. Count]= “HELLO”; //6 th pos=null print. Array(a, a. Count); //int template print. Array(b, b. Count); //float template print. Array(c, c. Count); //char template return 0; }
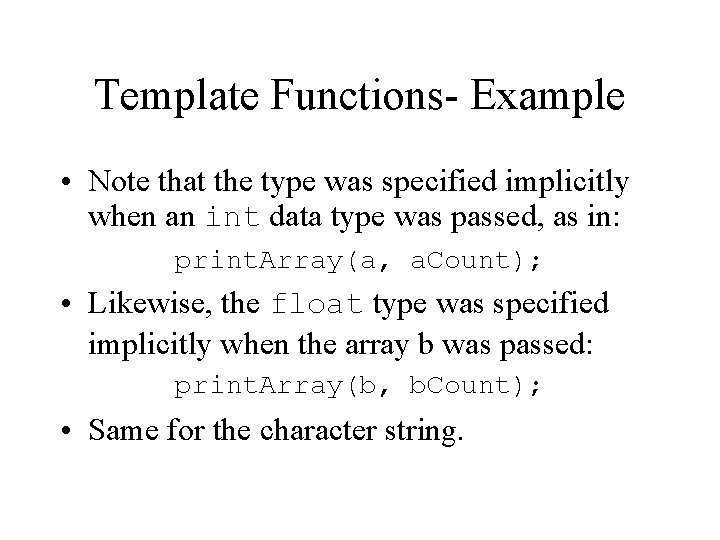
Template Functions- Example • Note that the type was specified implicitly when an int data type was passed, as in: print. Array(a, a. Count); • Likewise, the float type was specified implicitly when the array b was passed: print. Array(b, b. Count); • Same for the character string.
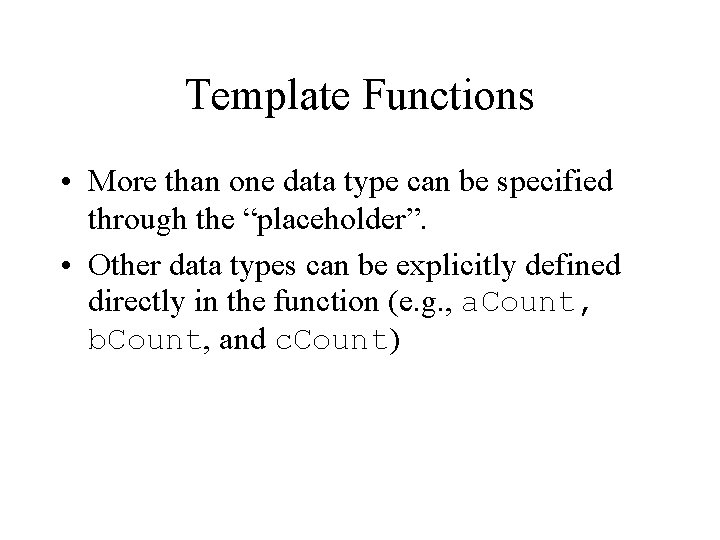
Template Functions • More than one data type can be specified through the “placeholder”. • Other data types can be explicitly defined directly in the function (e. g. , a. Count, b. Count, and c. Count)
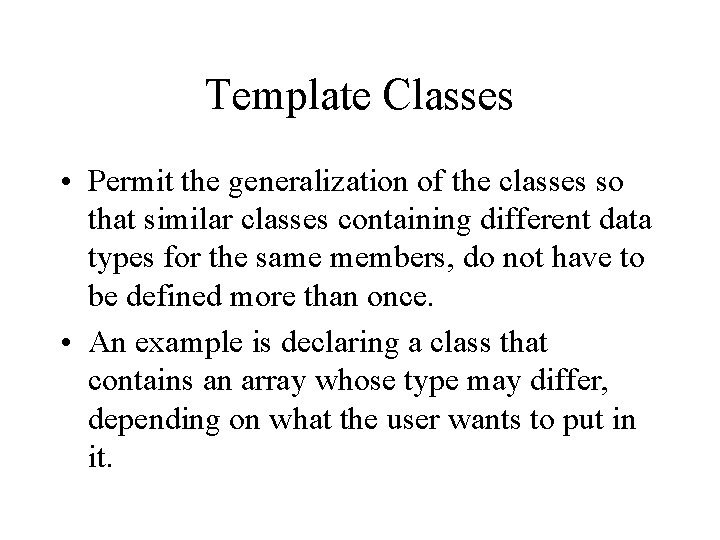
Template Classes • Permit the generalization of the classes so that similar classes containing different data types for the same members, do not have to be defined more than once. • An example is declaring a class that contains an array whose type may differ, depending on what the user wants to put in it.
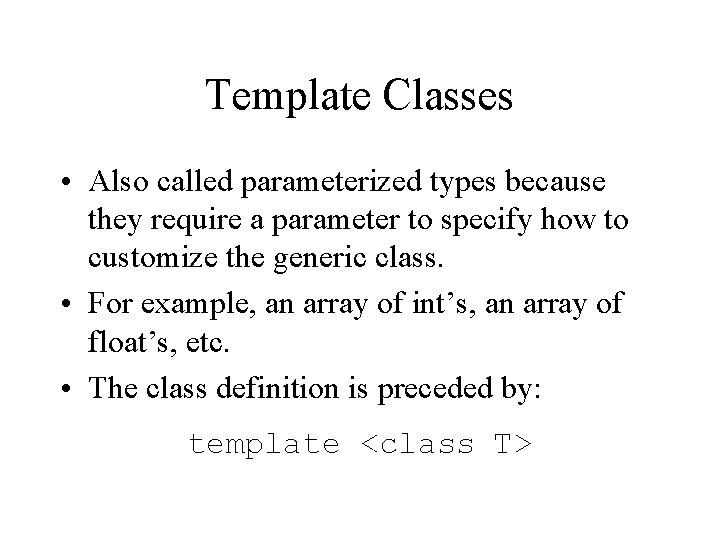
Template Classes • Also called parameterized types because they require a parameter to specify how to customize the generic class. • For example, an array of int’s, an array of float’s, etc. • The class definition is preceded by: template <class T>
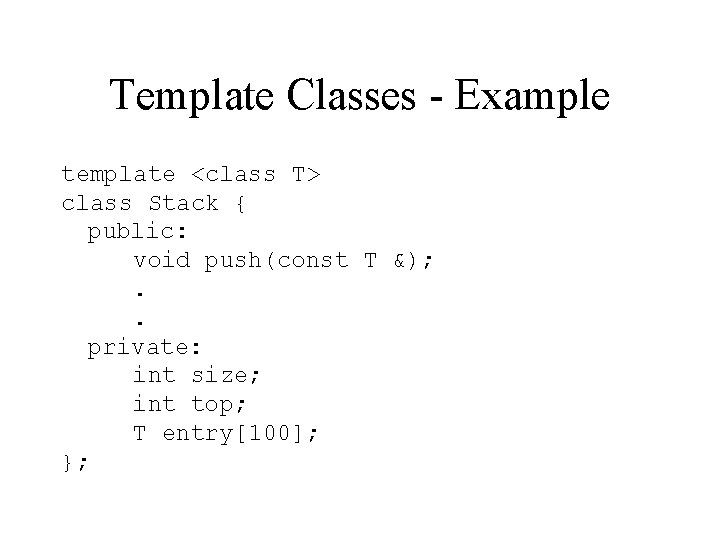
Template Classes - Example template <class T> class Stack { public: void push(const T &); . . private: int size; int top; T entry[100]; };
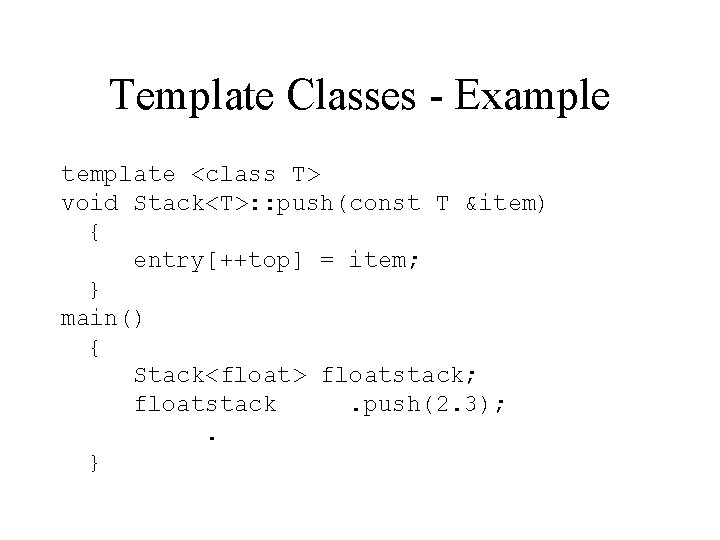
Template Classes - Example template <class T> void Stack<T>: : push(const T &item) { entry[++top] = item; } main() { Stack<float> floatstack; floatstack. push(2. 3); . }
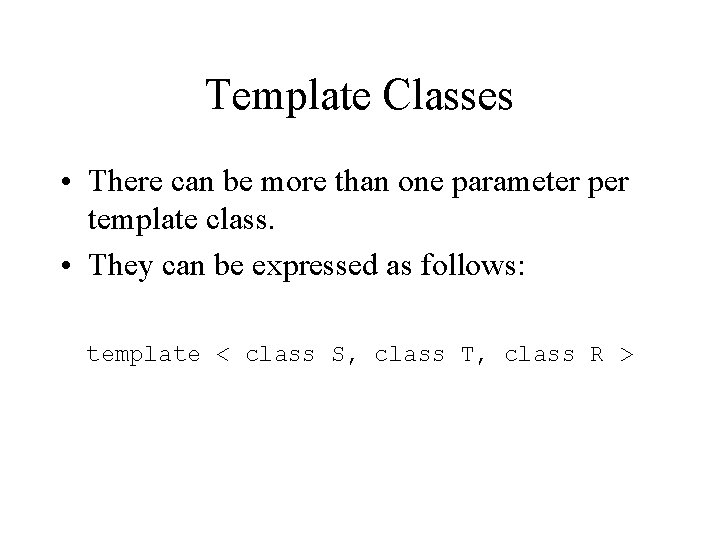
Template Classes • There can be more than one parameter per template class. • They can be expressed as follows: template < class S, class T, class R >