Discussion 2 More to discuss CMPE 212 Fall
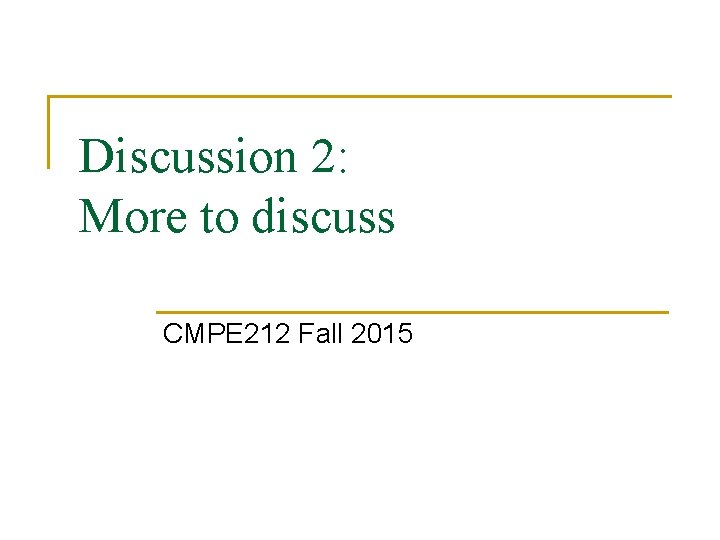
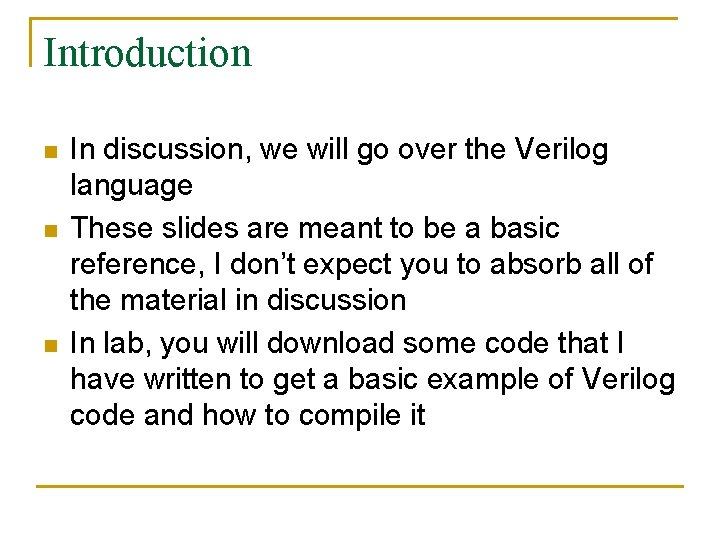
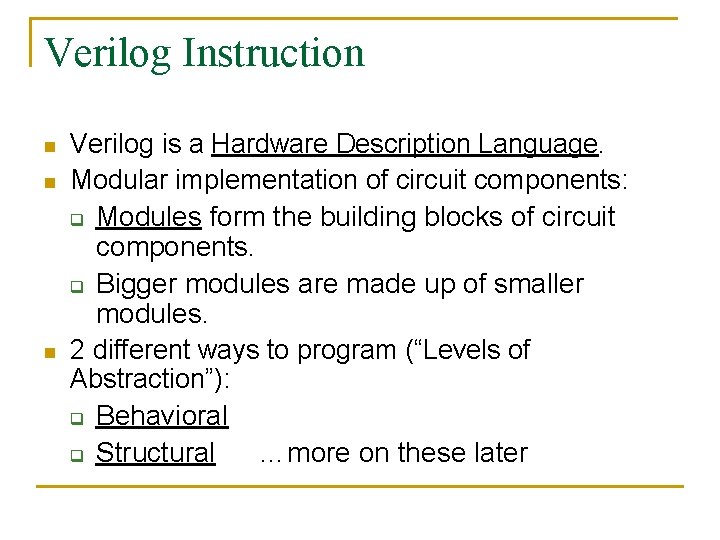
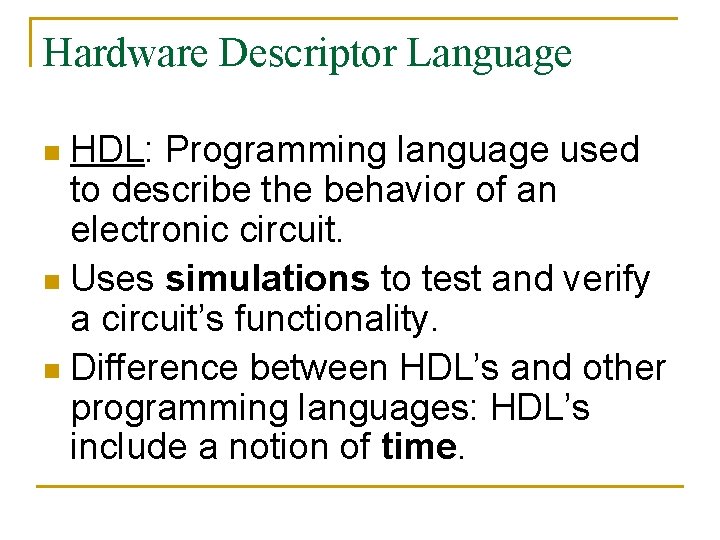
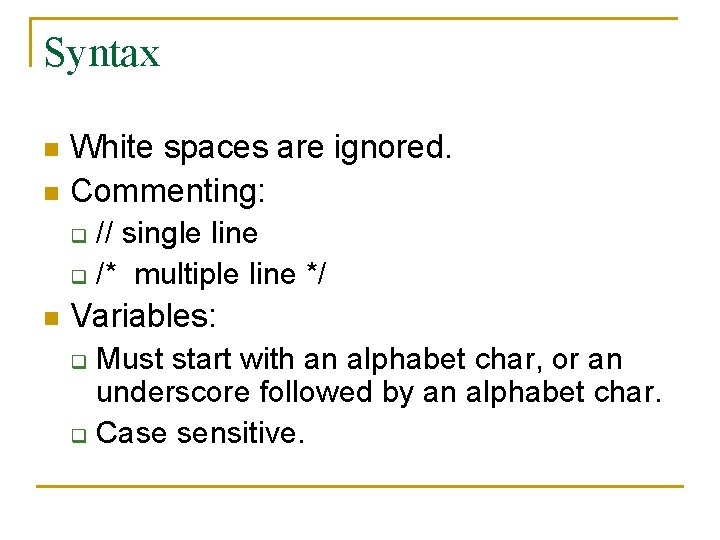
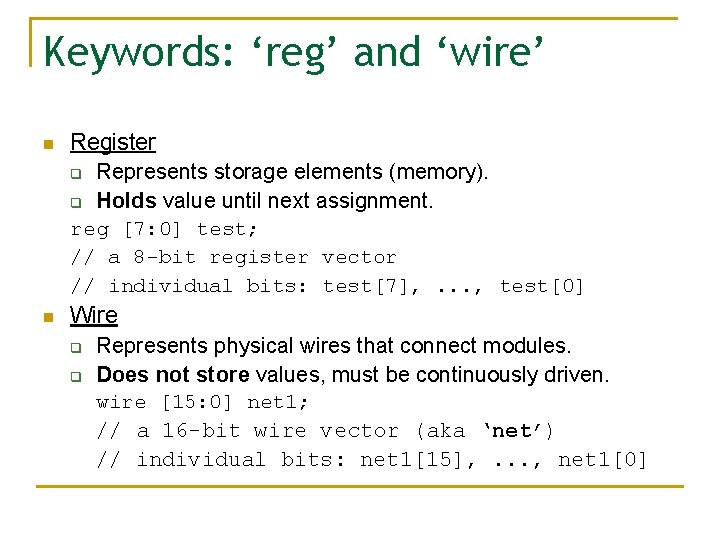
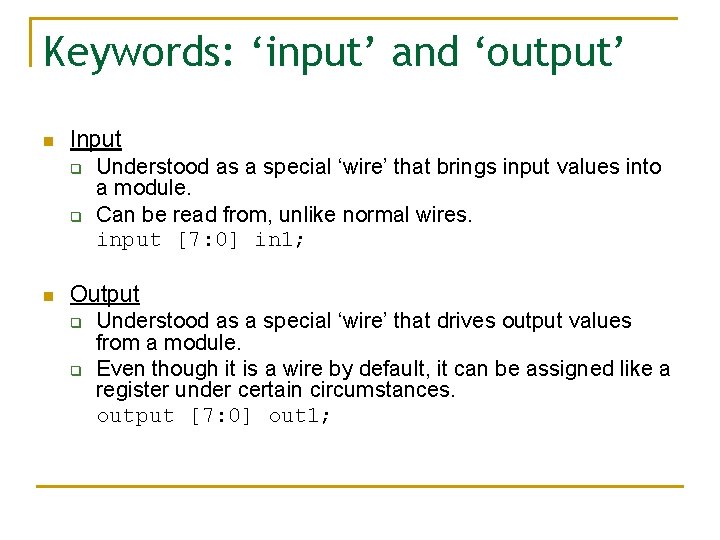
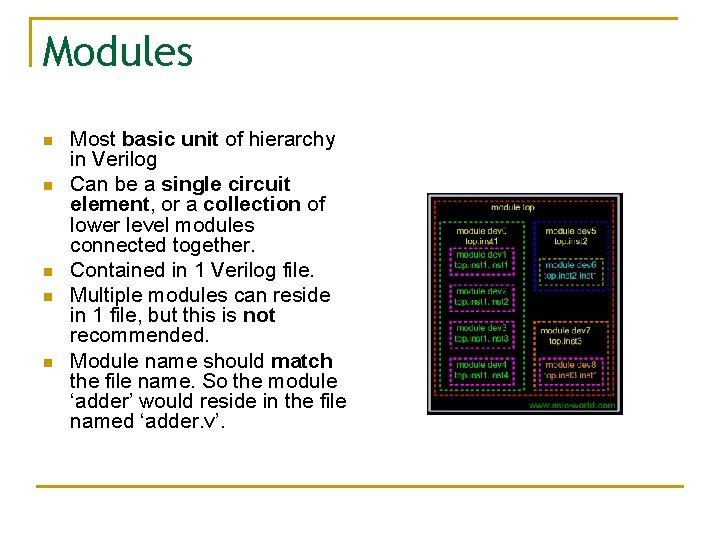
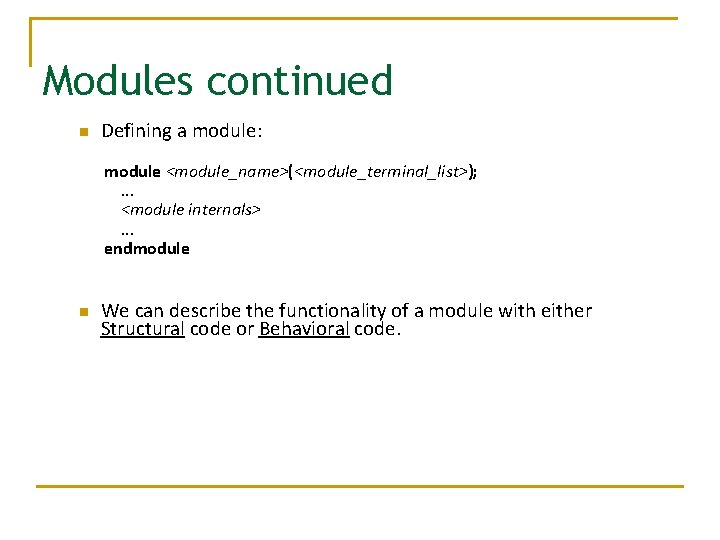
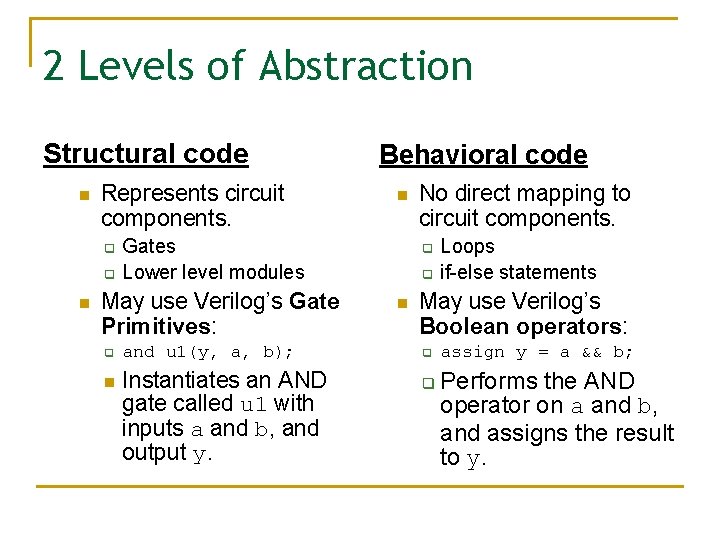
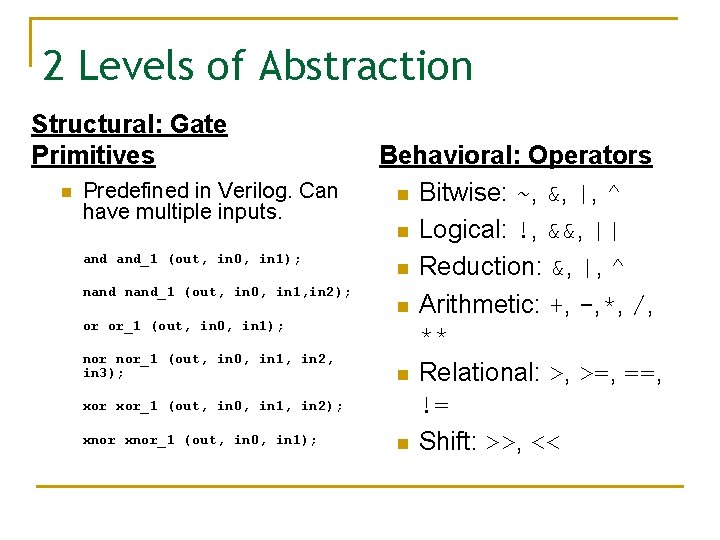
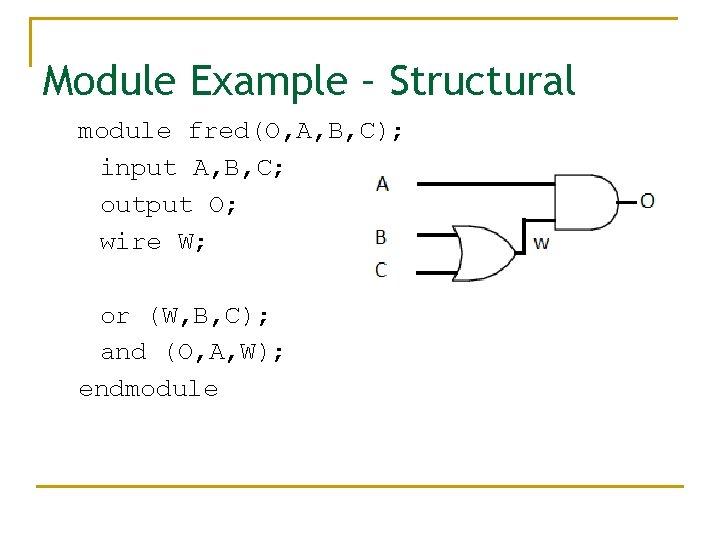
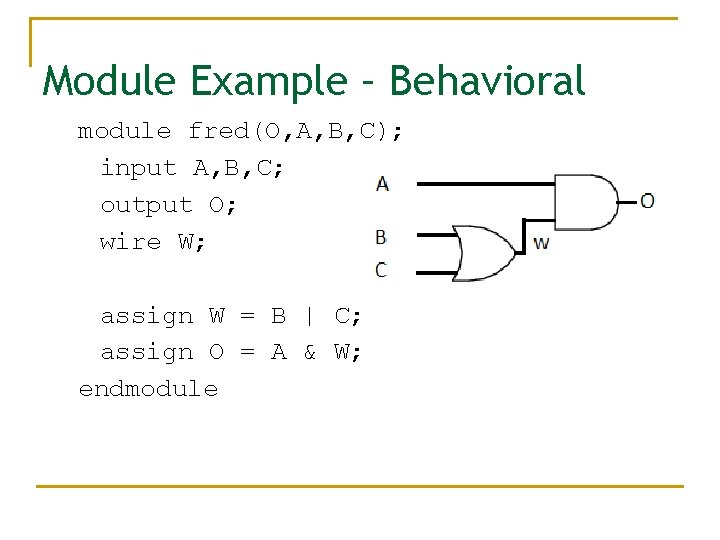
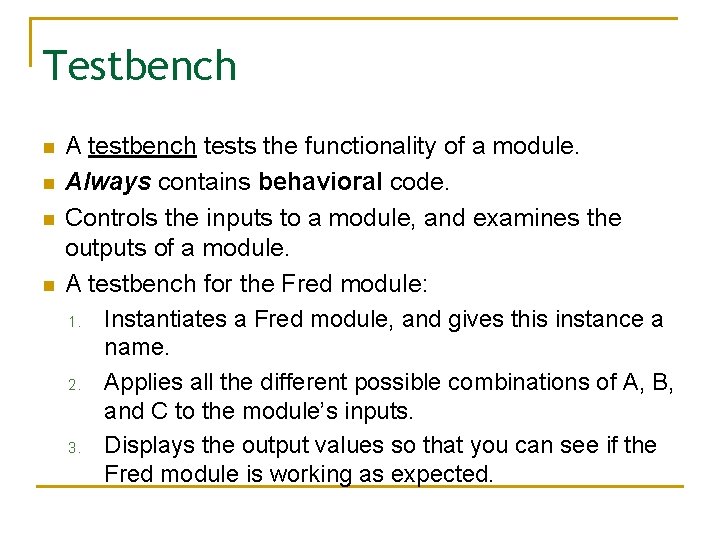
![Module Example – Test Bench module testbench 4 Fred(); reg [2: 0] switches; wire Module Example – Test Bench module testbench 4 Fred(); reg [2: 0] switches; wire](https://slidetodoc.com/presentation_image_h2/3126b5549633d43e64192b54b3de9523/image-15.jpg)
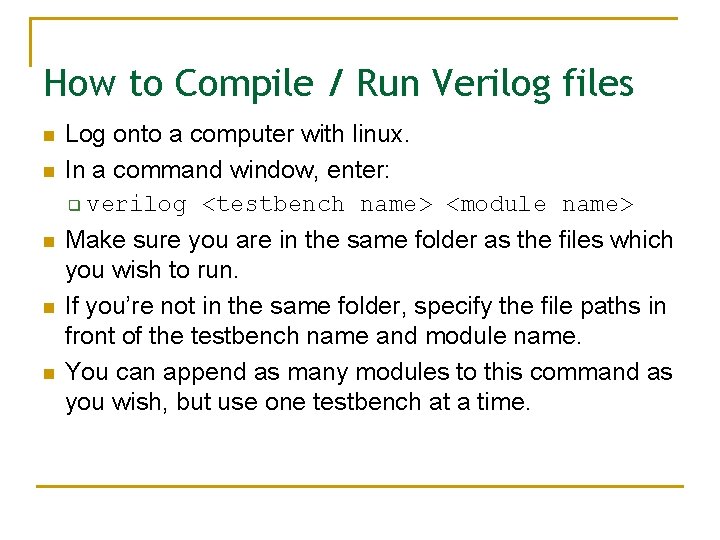
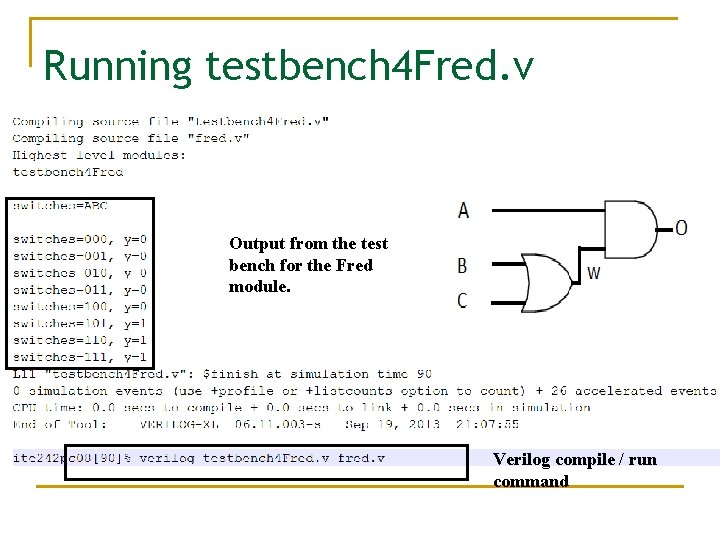
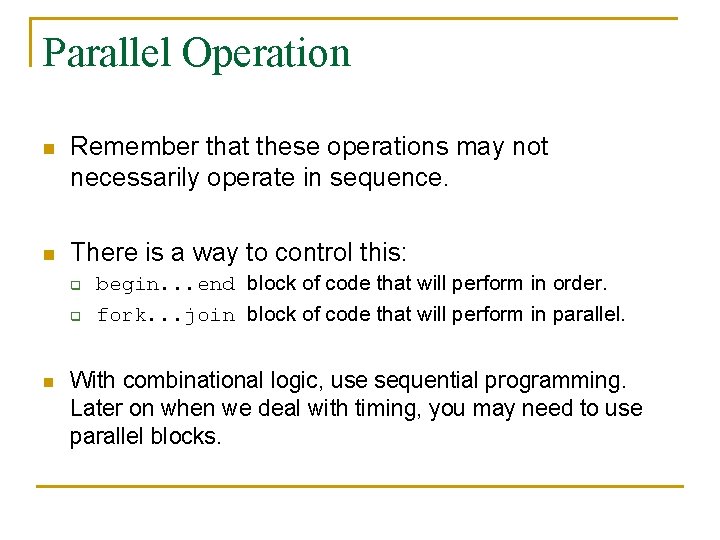
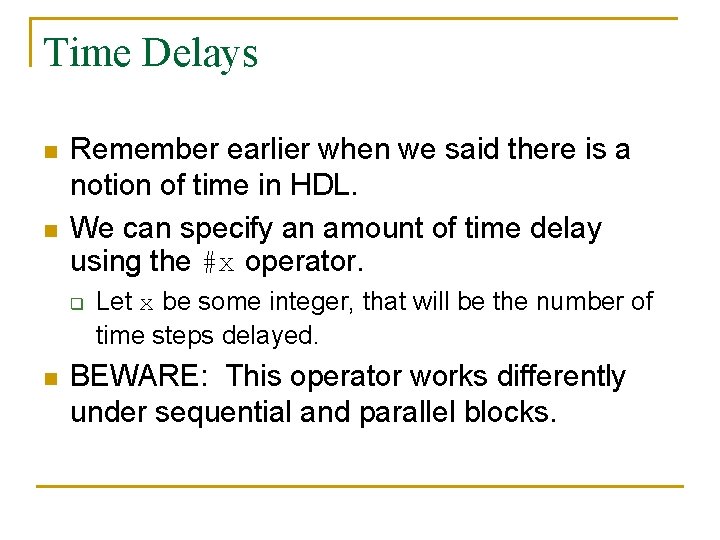
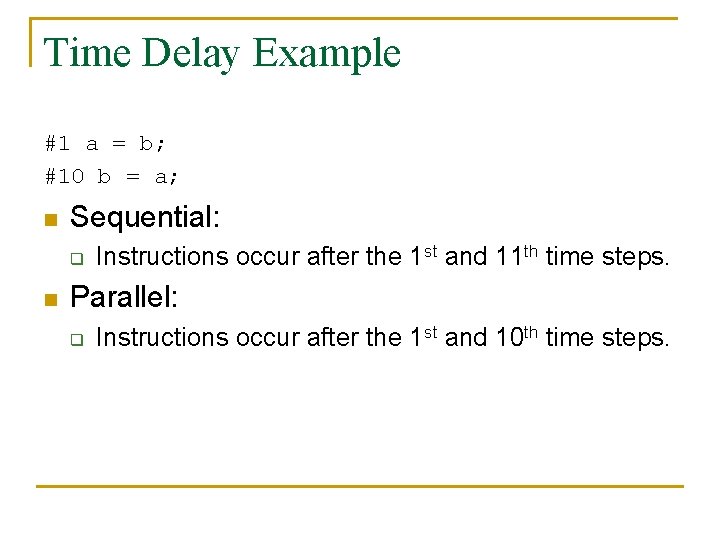
- Slides: 20
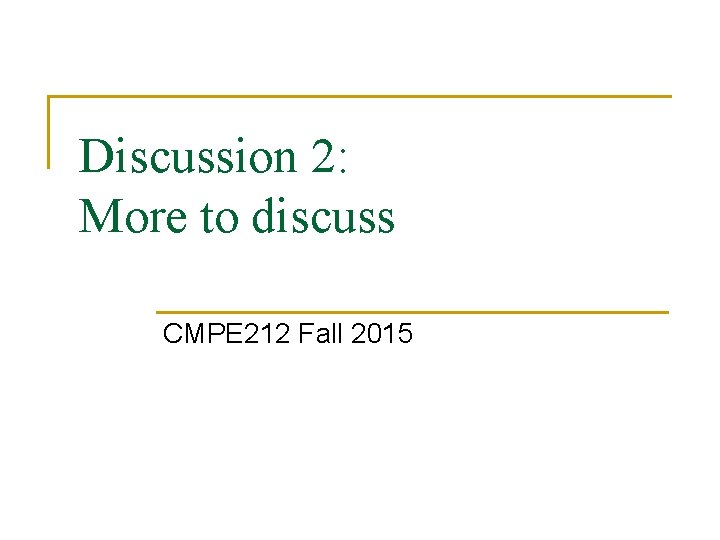
Discussion 2: More to discuss CMPE 212 Fall 2015
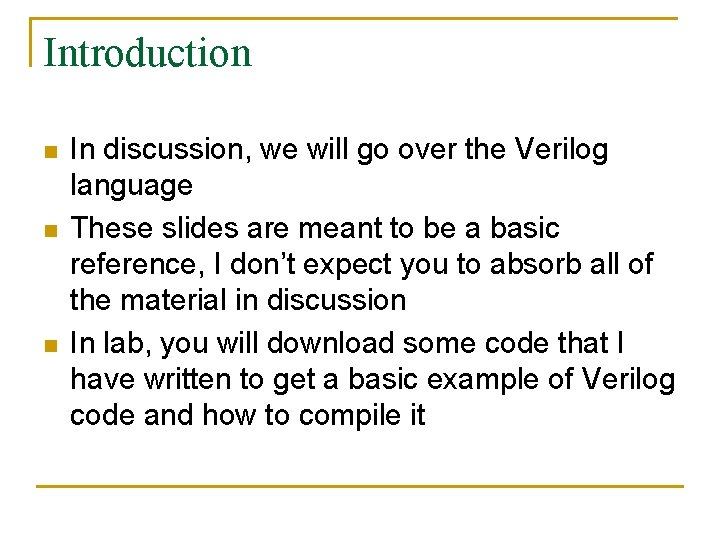
Introduction n In discussion, we will go over the Verilog language These slides are meant to be a basic reference, I don’t expect you to absorb all of the material in discussion In lab, you will download some code that I have written to get a basic example of Verilog code and how to compile it
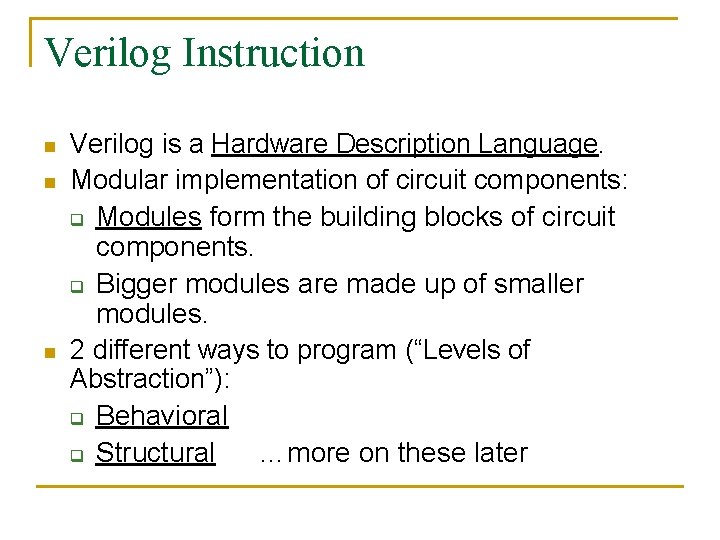
Verilog Instruction n Verilog is a Hardware Description Language. Modular implementation of circuit components: q Modules form the building blocks of circuit components. q Bigger modules are made up of smaller modules. 2 different ways to program (“Levels of Abstraction”): q Behavioral q Structural …more on these later
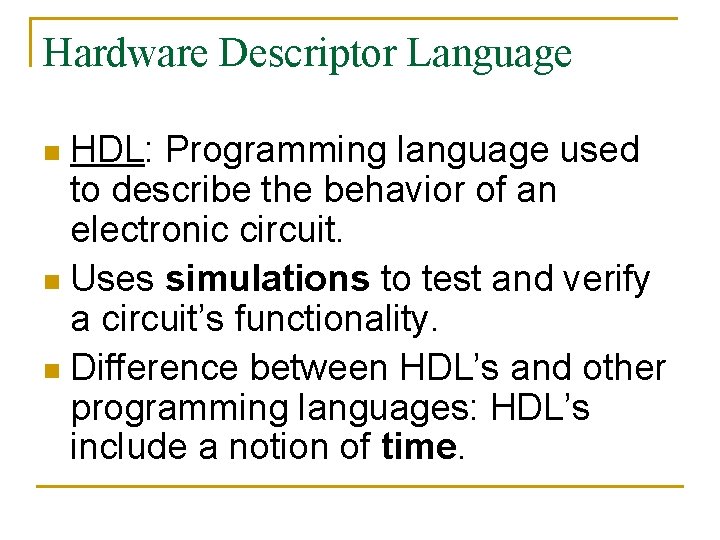
Hardware Descriptor Language HDL: Programming language used to describe the behavior of an electronic circuit. n Uses simulations to test and verify a circuit’s functionality. n Difference between HDL’s and other programming languages: HDL’s include a notion of time. n
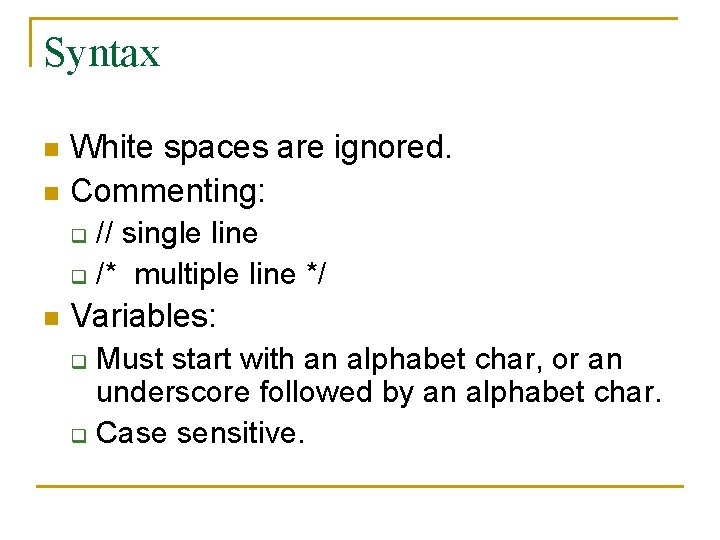
Syntax n n White spaces are ignored. Commenting: // single line q /* multiple line */ q n Variables: Must start with an alphabet char, or an underscore followed by an alphabet char. q Case sensitive. q
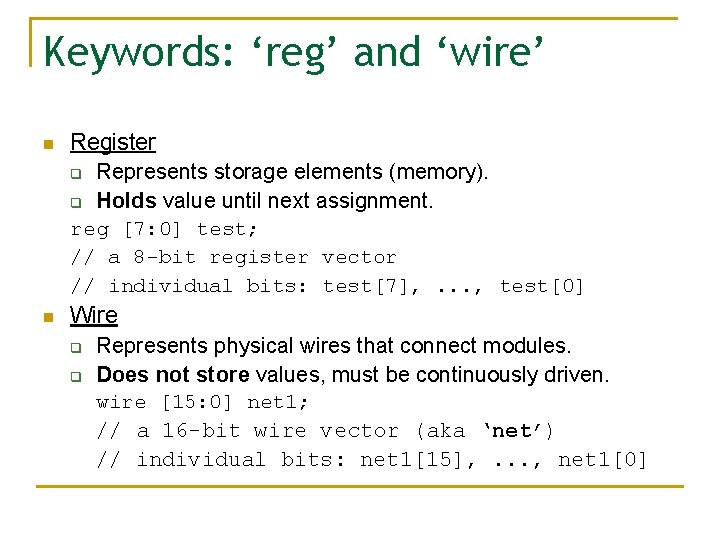
Keywords: ‘reg’ and ‘wire’ n Register q Represents storage elements (memory). q Holds value until next assignment. reg [7: 0] test; // a 8 -bit register vector // individual bits: test[7], . . . , test[0] n Wire q Represents physical wires that connect modules. q Does not store values, must be continuously driven. wire [15: 0] net 1; // a 16 -bit wire vector (aka ‘net’) // individual bits: net 1[15], . . . , net 1[0]
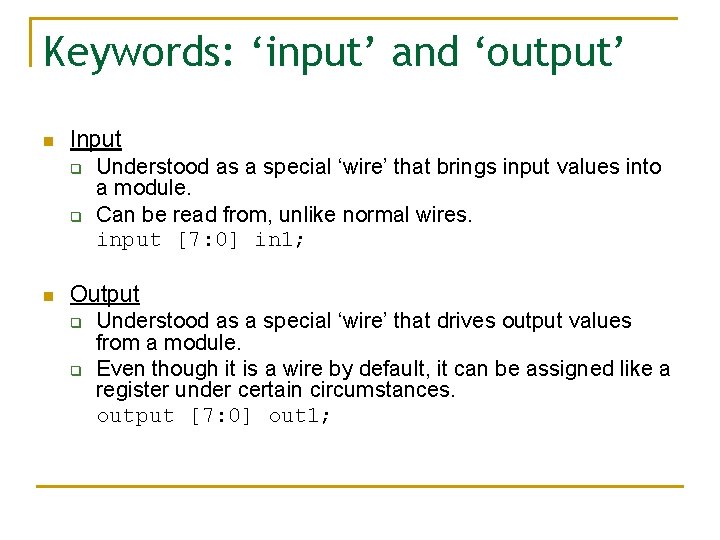
Keywords: ‘input’ and ‘output’ n Input q Understood as a special ‘wire’ that brings input values into a module. q Can be read from, unlike normal wires. input [7: 0] in 1; n Output q Understood as a special ‘wire’ that drives output values from a module. q Even though it is a wire by default, it can be assigned like a register under certain circumstances. output [7: 0] out 1;
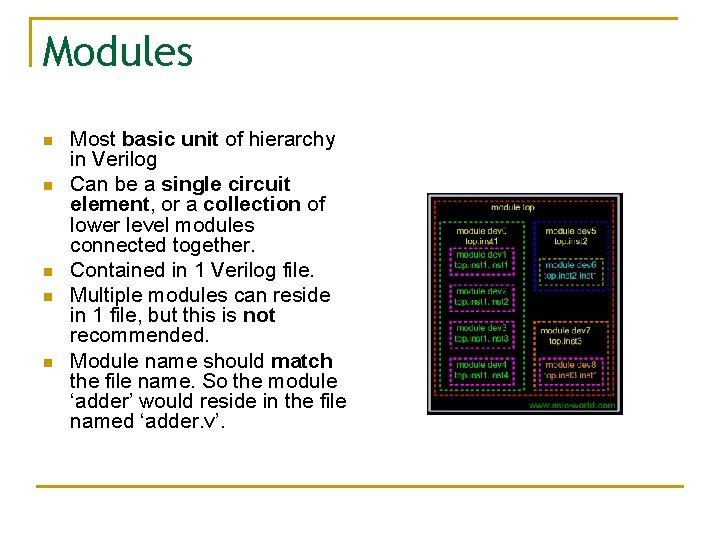
Modules n n n Most basic unit of hierarchy in Verilog Can be a single circuit element, or a collection of lower level modules connected together. Contained in 1 Verilog file. Multiple modules can reside in 1 file, but this is not recommended. Module name should match the file name. So the module ‘adder’ would reside in the file named ‘adder. v’.
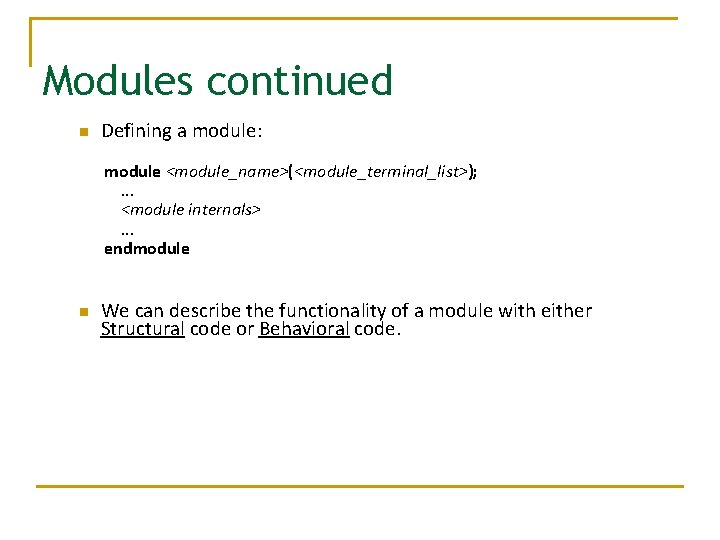
Modules continued n Defining a module: module <module_name>(<module_terminal_list>); . . . <module internals>. . . endmodule n We can describe the functionality of a module with either Structural code or Behavioral code.
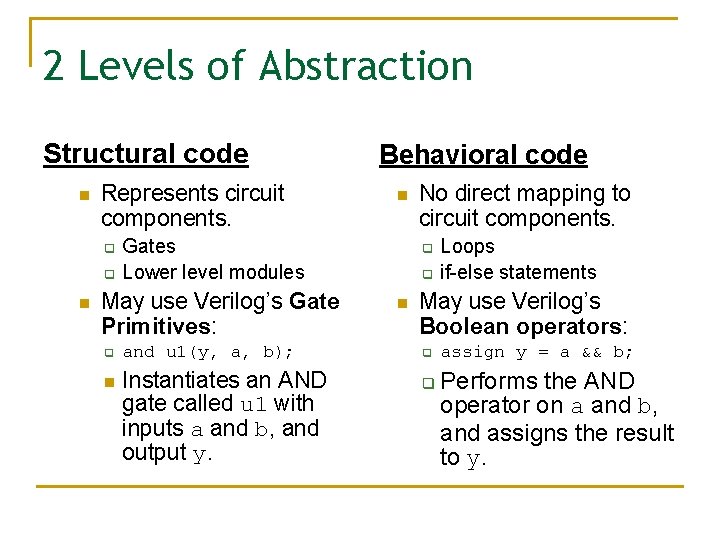
2 Levels of Abstraction Structural code n Represents circuit components. q q n n n Gates Lower level modules May use Verilog’s Gate Primitives: q Behavioral code and u 1(y, a, b); Instantiates an AND gate called u 1 with inputs a and b, and output y. No direct mapping to circuit components. q q n Loops if-else statements May use Verilog’s Boolean operators: q q assign y = a && b; Performs the AND operator on a and b, and assigns the result to y.
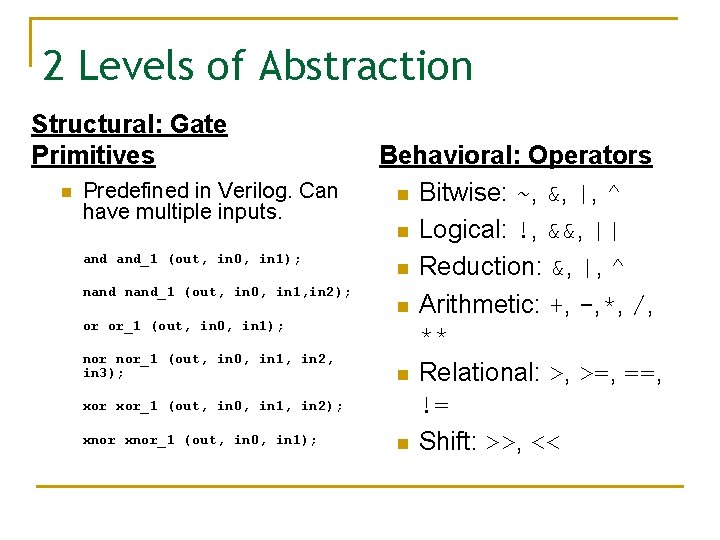
2 Levels of Abstraction Structural: Gate Primitives n Predefined in Verilog. Can have multiple inputs. and_1 (out, in 0, in 1); nand_1 (out, in 0, in 1, in 2); or or_1 (out, in 0, in 1); nor_1 (out, in 0, in 1, in 2, in 3); xor_1 (out, in 0, in 1, in 2); xnor_1 (out, in 0, in 1); Behavioral: Operators n Bitwise: ~, &, |, ^ n Logical: !, &&, || n Reduction: &, |, ^ n Arithmetic: +, -, *, /, ** n Relational: >, >=, ==, != n Shift: >>, <<
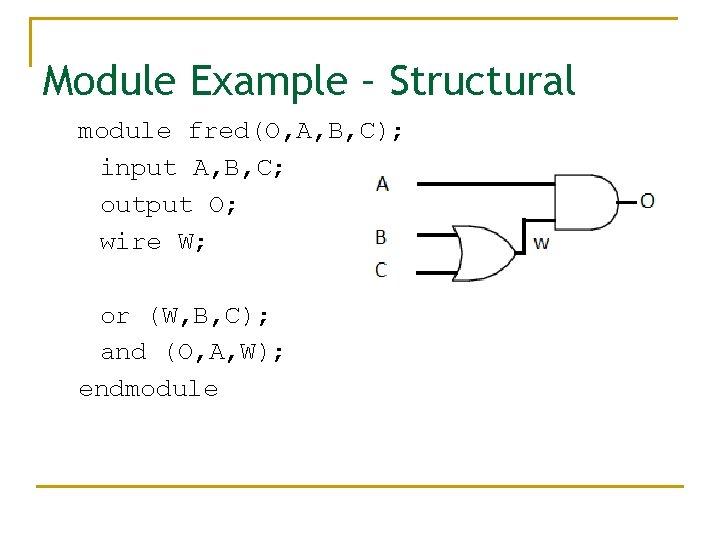
Module Example – Structural module fred(O, A, B, C); input A, B, C; output O; wire W; or (W, B, C); and (O, A, W); endmodule
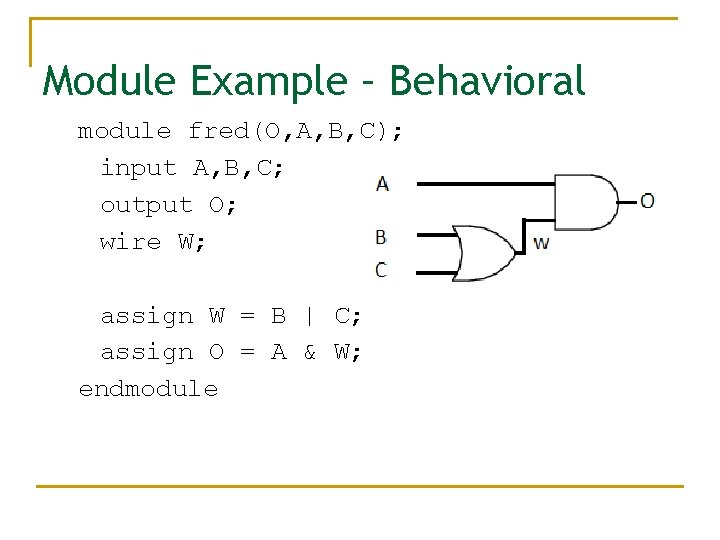
Module Example – Behavioral module fred(O, A, B, C); input A, B, C; output O; wire W; assign W = B | C; assign O = A & W; endmodule
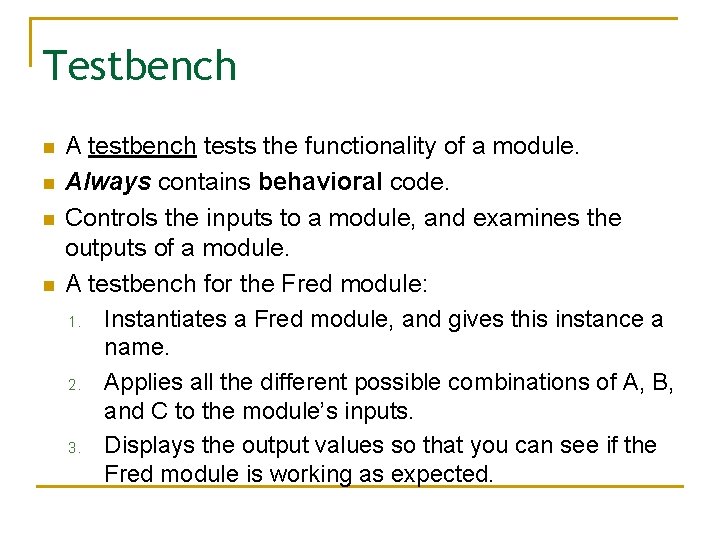
Testbench n n A testbench tests the functionality of a module. Always contains behavioral code. Controls the inputs to a module, and examines the outputs of a module. A testbench for the Fred module: 1. Instantiates a Fred module, and gives this instance a name. 2. Applies all the different possible combinations of A, B, and C to the module’s inputs. 3. Displays the output values so that you can see if the Fred module is working as expected.
![Module Example Test Bench module testbench 4 Fred reg 2 0 switches wire Module Example – Test Bench module testbench 4 Fred(); reg [2: 0] switches; wire](https://slidetodoc.com/presentation_image_h2/3126b5549633d43e64192b54b3de9523/image-15.jpg)
Module Example – Test Bench module testbench 4 Fred(); reg [2: 0] switches; wire y; fred f 1(y, switches[2], switches[1], switches[0]); initial begin switches = 000; $display(“switches=ABCn”); #80 $finish; end always begin y); endmodule switches = switches + 001; #10 $display(“switches=%b, y=%b”, switches,
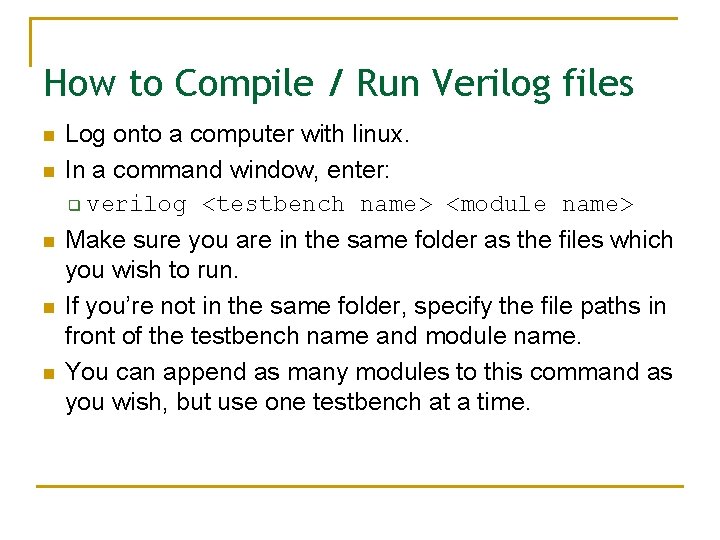
How to Compile / Run Verilog files n n n Log onto a computer with linux. In a command window, enter: q verilog <testbench name> <module name> Make sure you are in the same folder as the files which you wish to run. If you’re not in the same folder, specify the file paths in front of the testbench name and module name. You can append as many modules to this command as you wish, but use one testbench at a time.
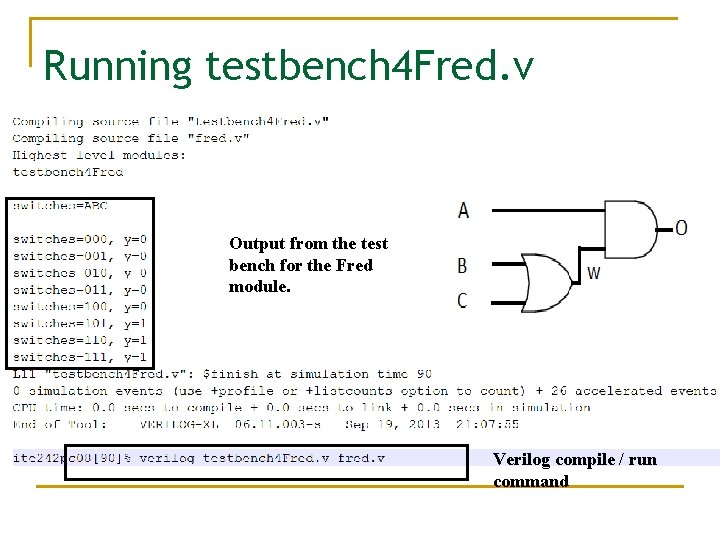
Running testbench 4 Fred. v Output from the test bench for the Fred module. Verilog compile / run command
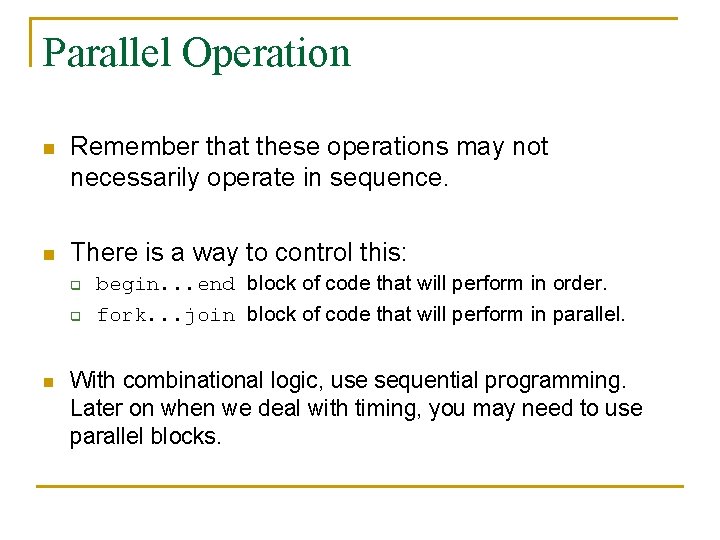
Parallel Operation n Remember that these operations may not necessarily operate in sequence. n There is a way to control this: q q n begin. . . end block of code that will perform in order. fork. . . join block of code that will perform in parallel. With combinational logic, use sequential programming. Later on when we deal with timing, you may need to use parallel blocks.
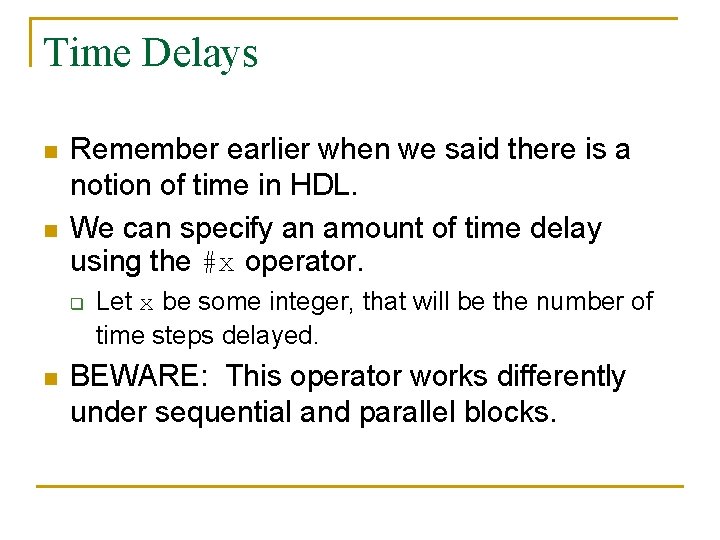
Time Delays n n Remember earlier when we said there is a notion of time in HDL. We can specify an amount of time delay using the #x operator. q n Let x be some integer, that will be the number of time steps delayed. BEWARE: This operator works differently under sequential and parallel blocks.
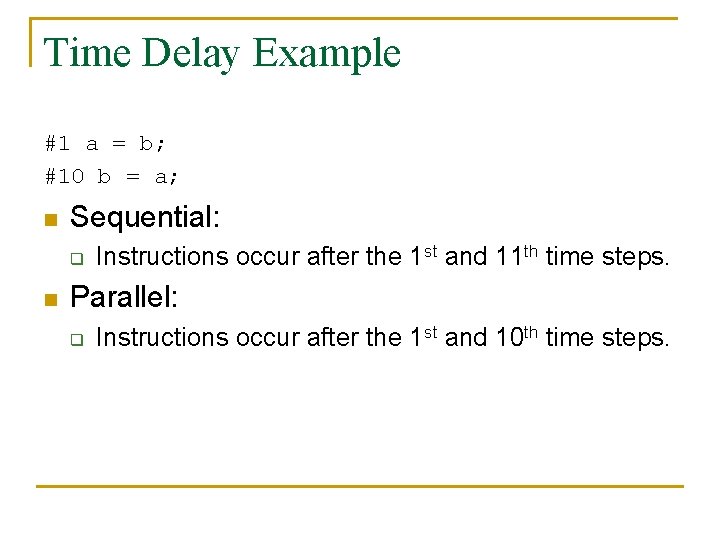
Time Delay Example #1 a = b; #10 b = a; n Sequential: q n Instructions occur after the 1 st and 11 th time steps. Parallel: q Instructions occur after the 1 st and 10 th time steps.
More more more i want more more more more we praise you
More more more i want more more more more we praise you
Cmpe 212
Amateurs talk tactics professionals talk logistics
Simple distillation introduction
Narrative technique in things fall apart
Describe roderick usher weaknesses
Ron mak sjsu
Cantor paradoksu
Cmpe emu
Cmpe 294 sjsu
Cmpe 280
Cmpe 252
Cmpe150
Cmpe 280
Cmpe 280
Duygu çelik ertuğrul
Cmpe 220
Cmpe emu
Cmpe 280
Cmpe 252