Directory File Handling Topics Directory Current Path Change
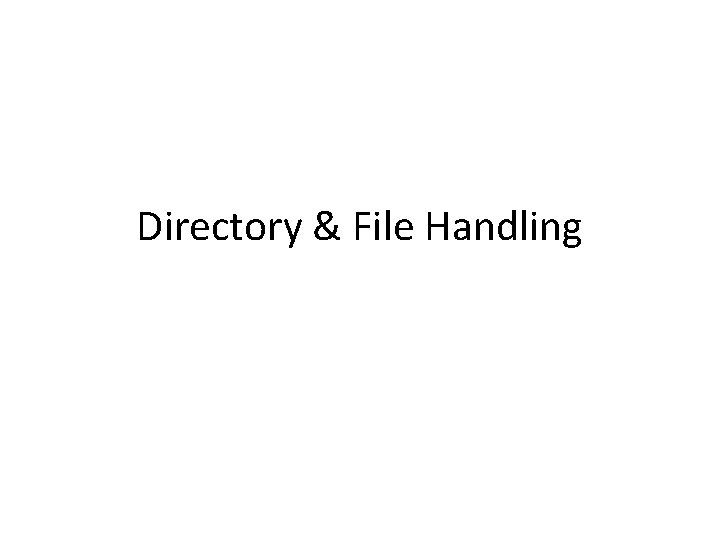
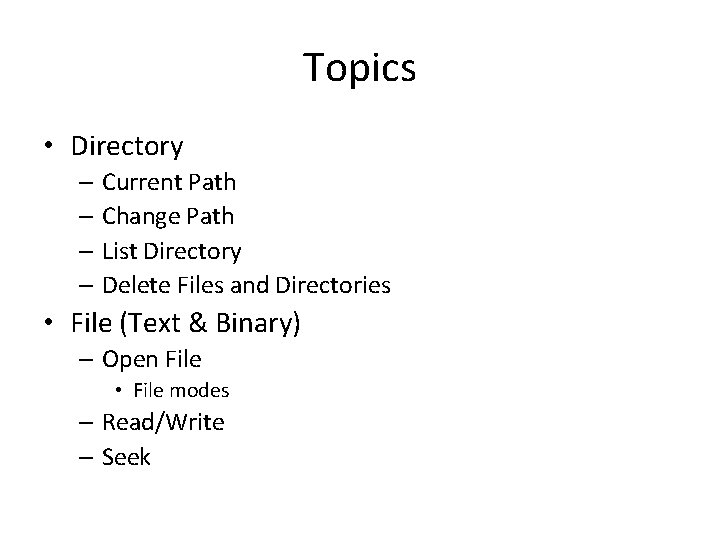
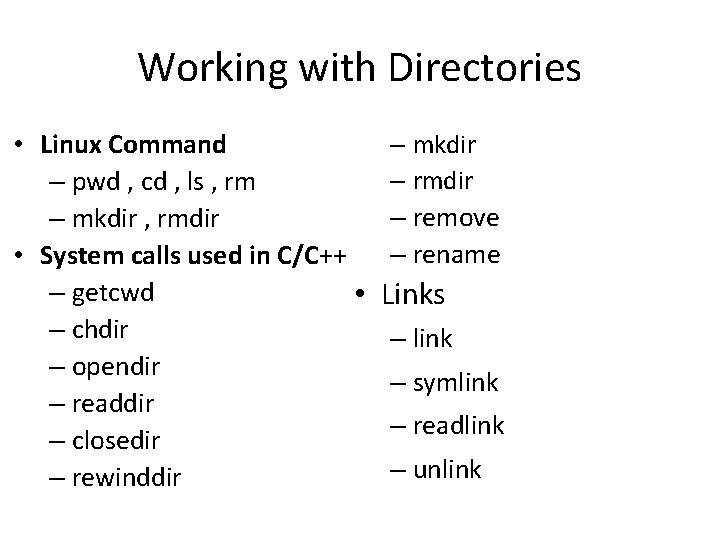
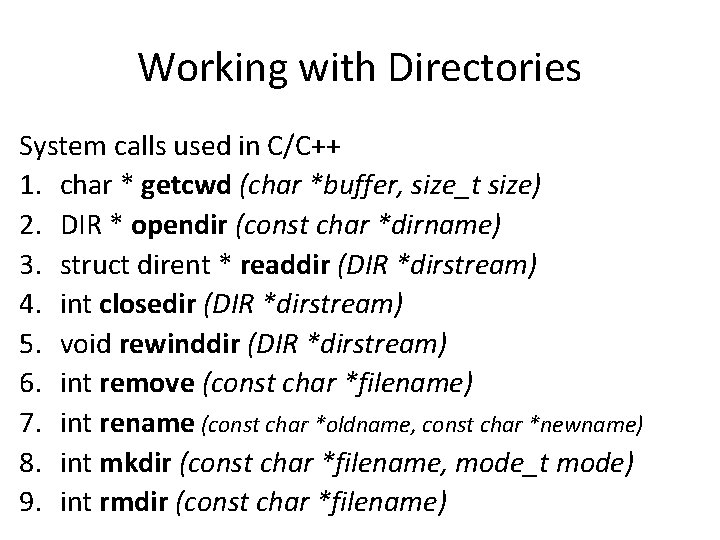
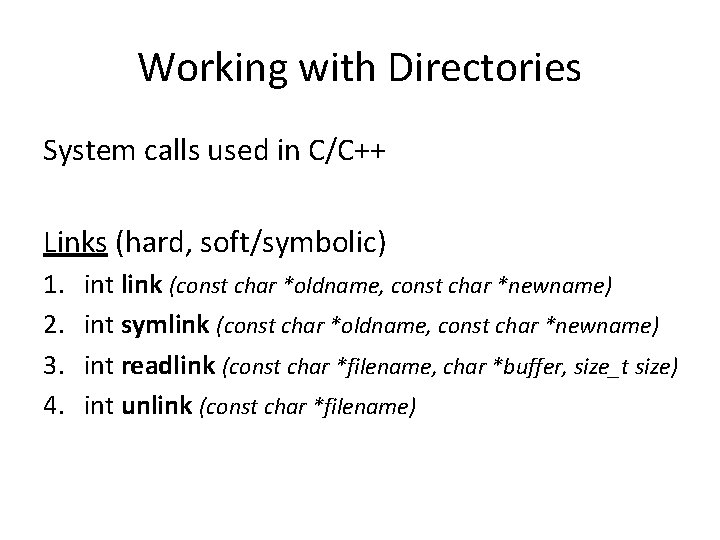
![struct dirent (Directory Entry) • • char d_name[] ino_t d_fileno unsigned char d_namlen unsigned struct dirent (Directory Entry) • • char d_name[] ino_t d_fileno unsigned char d_namlen unsigned](https://slidetodoc.com/presentation_image_h2/4c32f0ccc59aaa68357830c825223337/image-6.jpg)
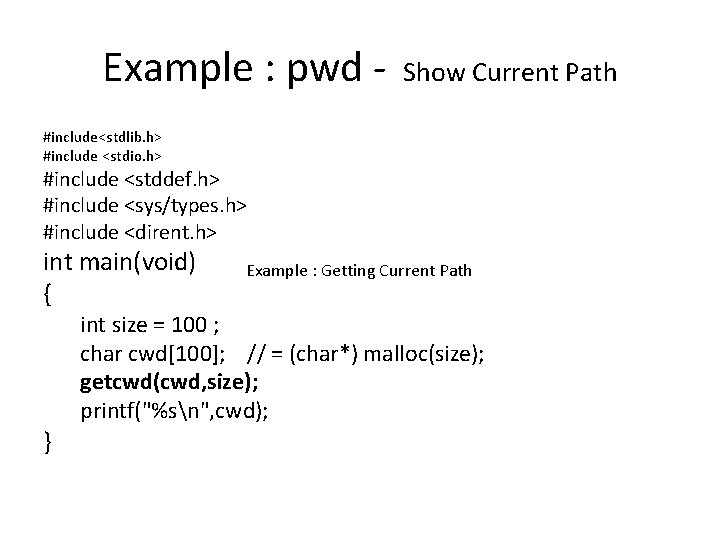
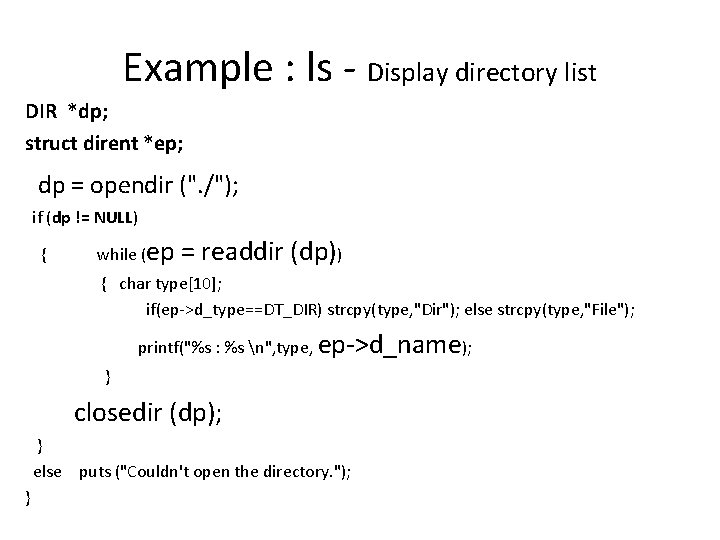
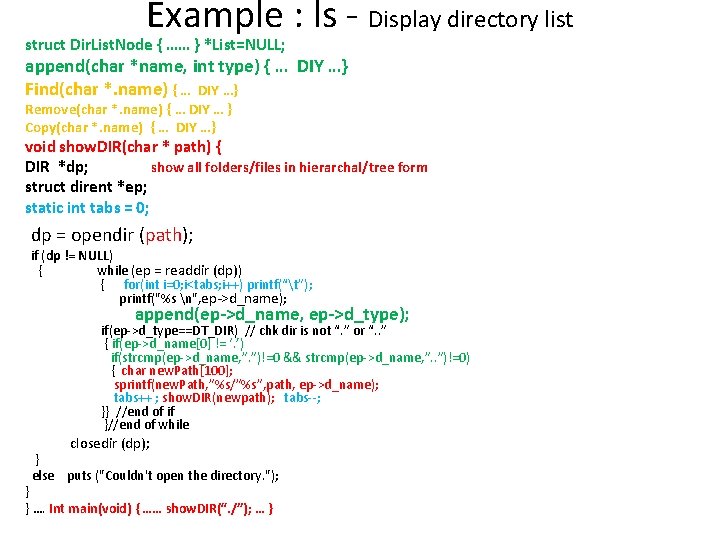
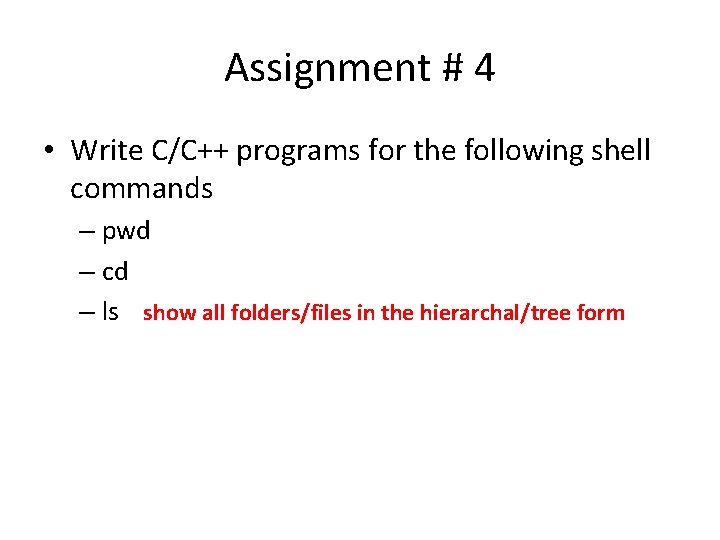
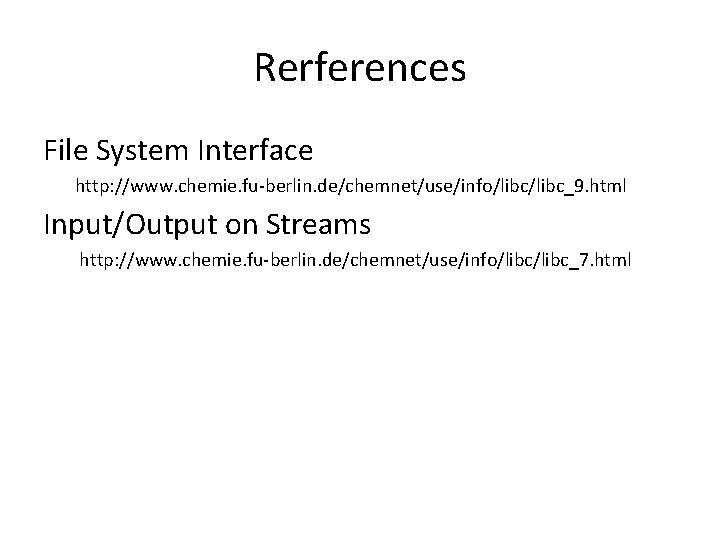
- Slides: 11
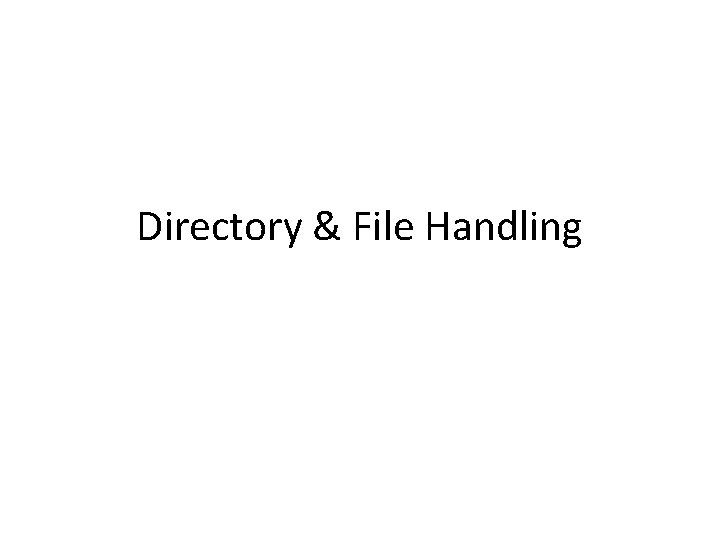
Directory & File Handling
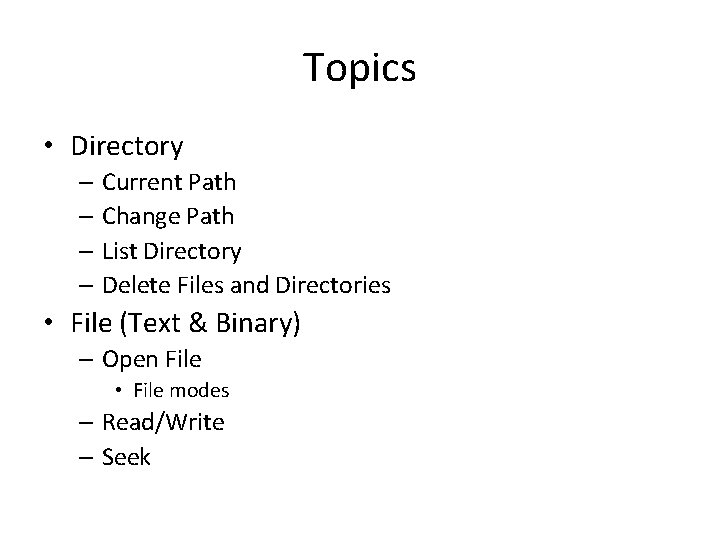
Topics • Directory – Current Path – Change Path – List Directory – Delete Files and Directories • File (Text & Binary) – Open File • File modes – Read/Write – Seek
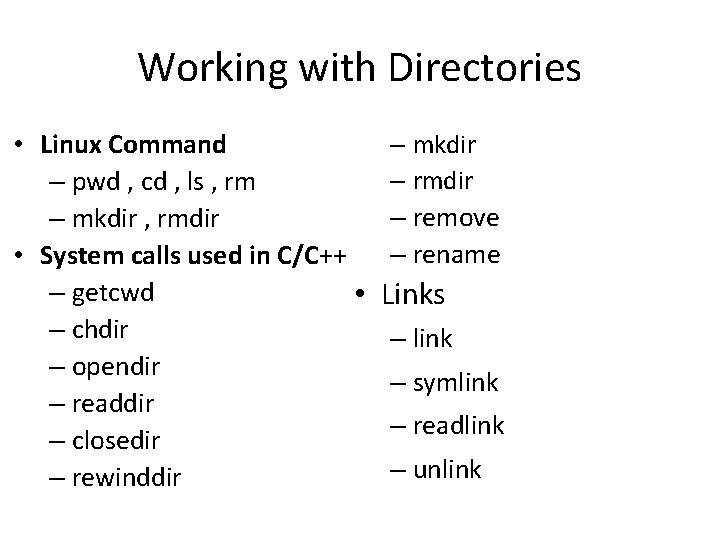
Working with Directories • Linux Command – pwd , cd , ls , rm – mkdir , rmdir • System calls used in C/C++ – getcwd • – chdir – opendir – readdir – closedir – rewinddir – mkdir – rmdir – remove – rename Links – link – symlink – readlink – unlink
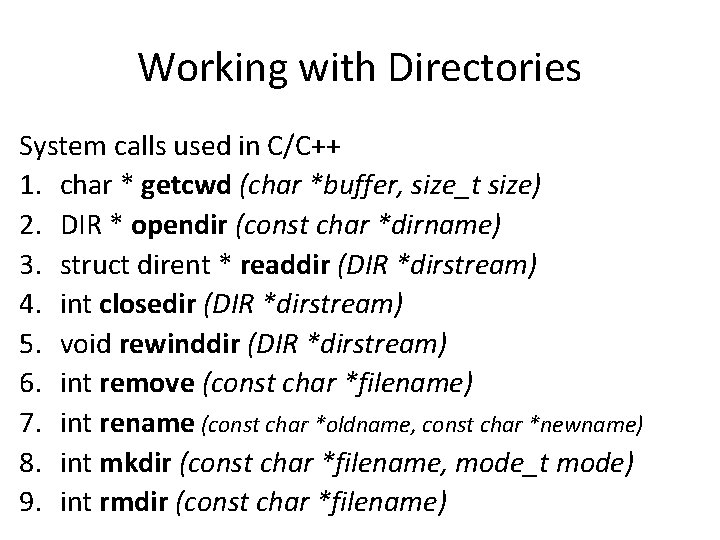
Working with Directories System calls used in C/C++ 1. char * getcwd (char *buffer, size_t size) 2. DIR * opendir (const char *dirname) 3. struct dirent * readdir (DIR *dirstream) 4. int closedir (DIR *dirstream) 5. void rewinddir (DIR *dirstream) 6. int remove (const char *filename) 7. int rename (const char *oldname, const char *newname) 8. int mkdir (const char *filename, mode_t mode) 9. int rmdir (const char *filename)
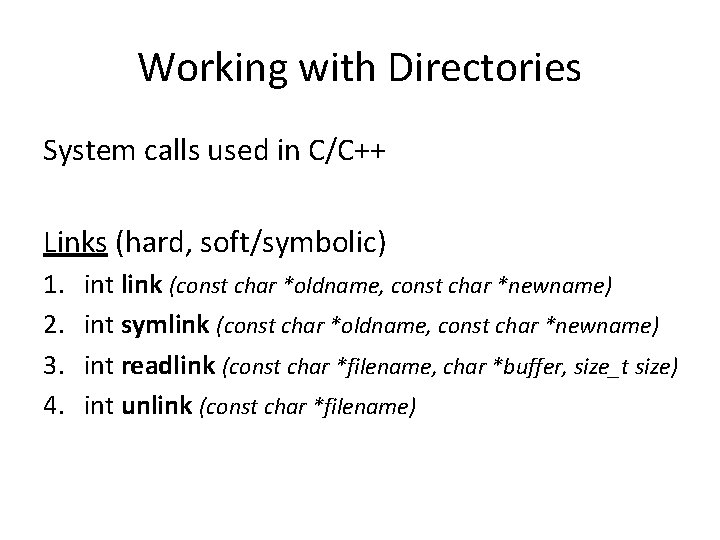
Working with Directories System calls used in C/C++ Links (hard, soft/symbolic) 1. 2. 3. 4. int link (const char *oldname, const char *newname) int symlink (const char *oldname, const char *newname) int readlink (const char *filename, char *buffer, size_t size) int unlink (const char *filename)
![struct dirent Directory Entry char dname inot dfileno unsigned char dnamlen unsigned struct dirent (Directory Entry) • • char d_name[] ino_t d_fileno unsigned char d_namlen unsigned](https://slidetodoc.com/presentation_image_h2/4c32f0ccc59aaa68357830c825223337/image-6.jpg)
struct dirent (Directory Entry) • • char d_name[] ino_t d_fileno unsigned char d_namlen unsigned char d_type – – – – DT_UNKNOWN DT_REG DT_DIR DT_FIFO DT_SOCK DT_CHR DT_BLK regular file directory named pipe local-domain socket character device block device
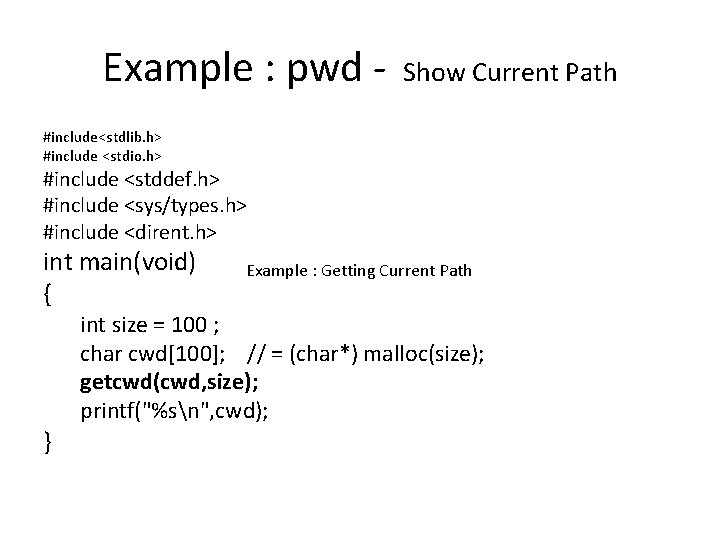
Example : pwd - Show Current Path #include<stdlib. h> #include <stdio. h> #include <stddef. h> #include <sys/types. h> #include <dirent. h> int main(void) { } Example : Getting Current Path int size = 100 ; char cwd[100]; // = (char*) malloc(size); getcwd(cwd, size); printf("%sn", cwd);
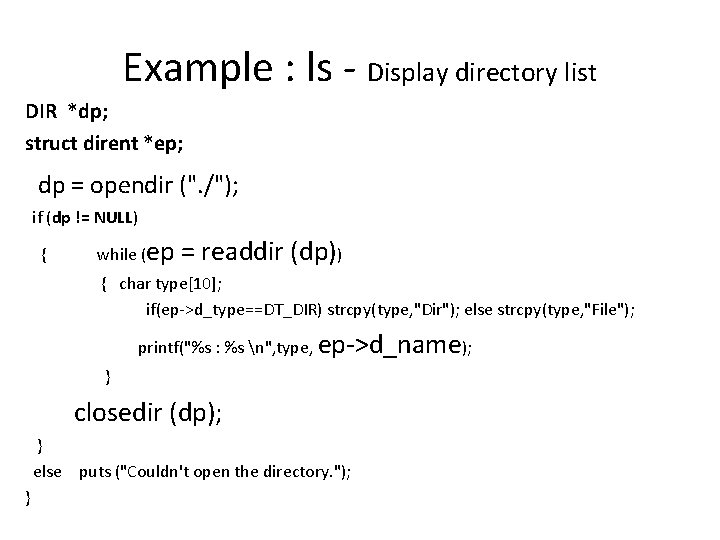
Example : ls - Display directory list DIR *dp; struct dirent *ep; dp = opendir (". /"); if (dp != NULL) { while (ep = readdir (dp)) { char type[10]; if(ep->d_type==DT_DIR) strcpy(type, "Dir"); else strcpy(type, "File"); printf("%s : %s n", type, ep->d_name); } closedir (dp); } else puts ("Couldn't open the directory. "); }
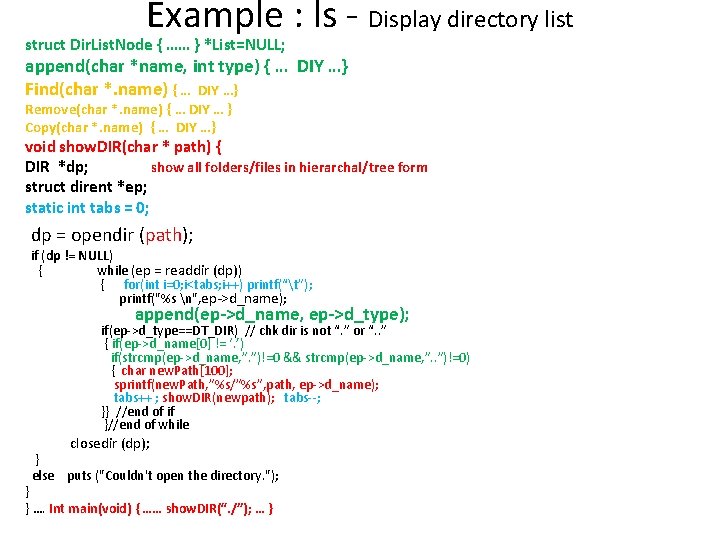
Example : ls - Display directory list struct Dir. List. Node { …… } *List=NULL; append(char *name, int type) { … DIY …} Find(char *. name) { … DIY …} Remove(char *. name) { … DIY … } Copy(char *. name) { … DIY …} void show. DIR(char * path) { DIR *dp; show all folders/files in hierarchal/tree form struct dirent *ep; static int tabs = 0; dp = opendir (path); if (dp != NULL) { while (ep = readdir (dp)) { for(int i=0; i<tabs; i++) printf(“t”); printf("%s n", ep->d_name); append(ep->d_name, ep->d_type); if(ep->d_type==DT_DIR) // chk dir is not “. ” or “. . ” { if(ep->d_name[0] != ‘. ’) if(strcmp(ep->d_name, ”. ”)!=0 && strcmp(ep->d_name, ”. . ”)!=0) { char new. Path[100]; sprintf(new. Path, ”%s/”%s”, path, ep->d_name); tabs++ ; show. DIR(newpath); tabs--; }} //end of if }//end of while closedir (dp); } else puts ("Couldn't open the directory. "); } } …. Int main(void) { …… show. DIR(“. /”); … }
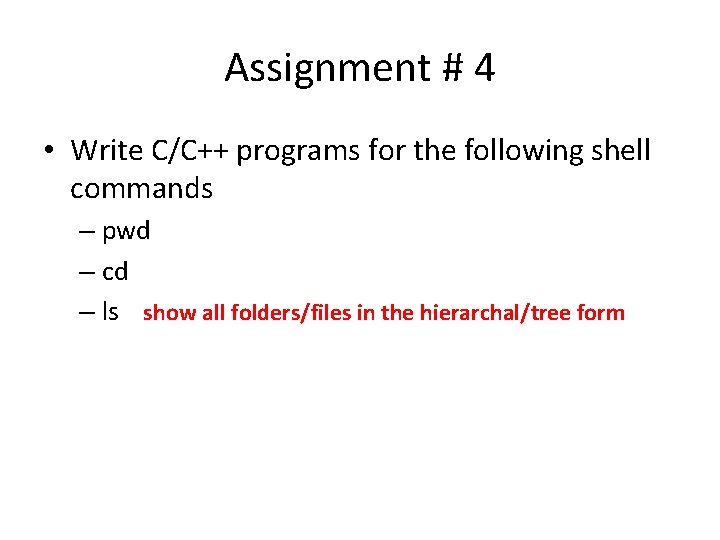
Assignment # 4 • Write C/C++ programs for the following shell commands – pwd – cd – ls show all folders/files in the hierarchal/tree form
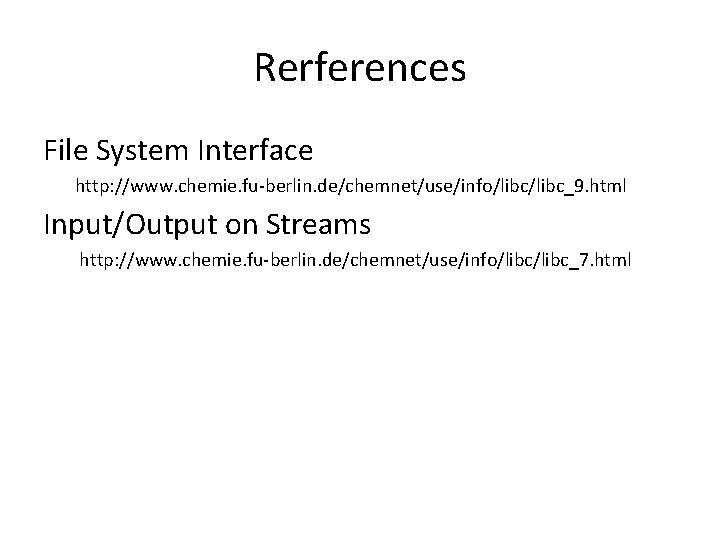
Rerferences File System Interface http: //www. chemie. fu-berlin. de/chemnet/use/info/libc_9. html Input/Output on Streams http: //www. chemie. fu-berlin. de/chemnet/use/info/libc_7. html
File-file yang dibuat oleh user pada jenis file di linux
Manajemen file pada unix
File handling in c
Exec cics startbr
File handling computer science
Procedures in assembly language
Topics in sports nutrition
Unity signalr
Current path linux command
Return current path
A closed path that electric current follows is a(n) ______.
Map a file path