Determinate Loop Pattern Javas for Statement and Scanner
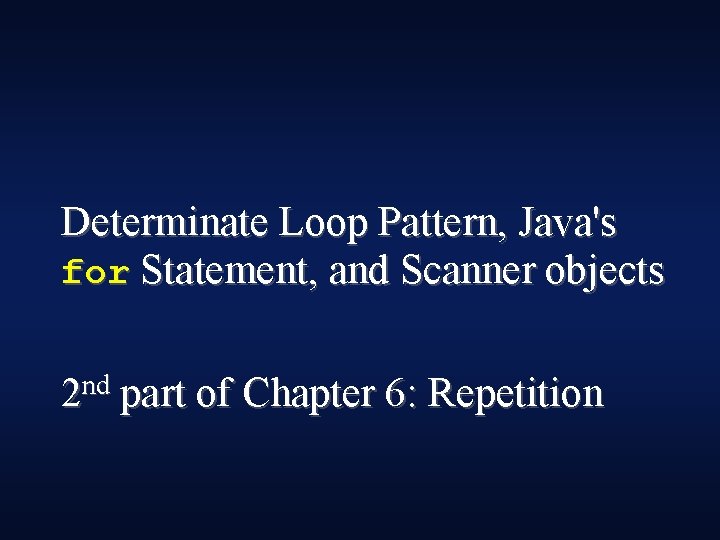
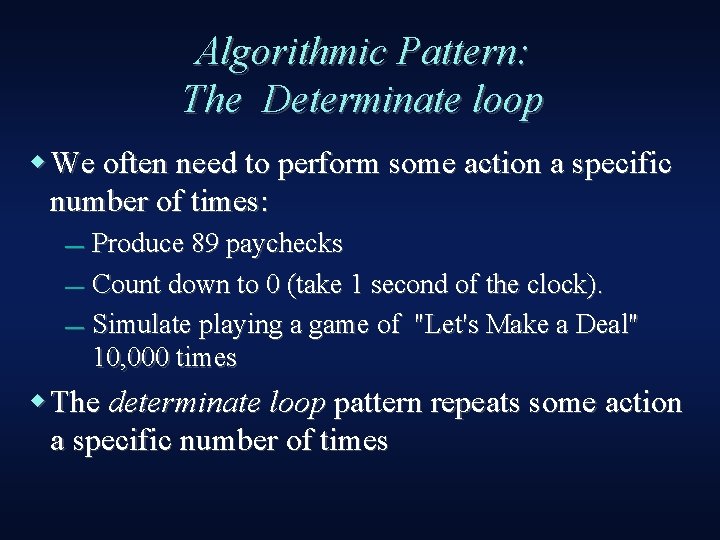
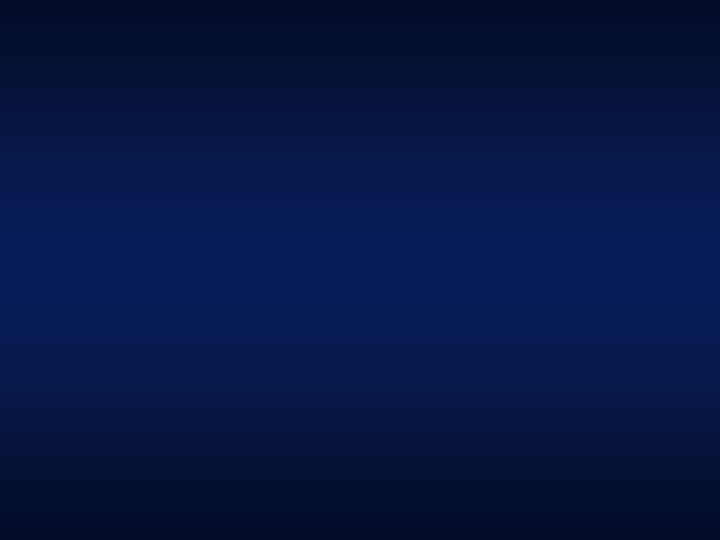
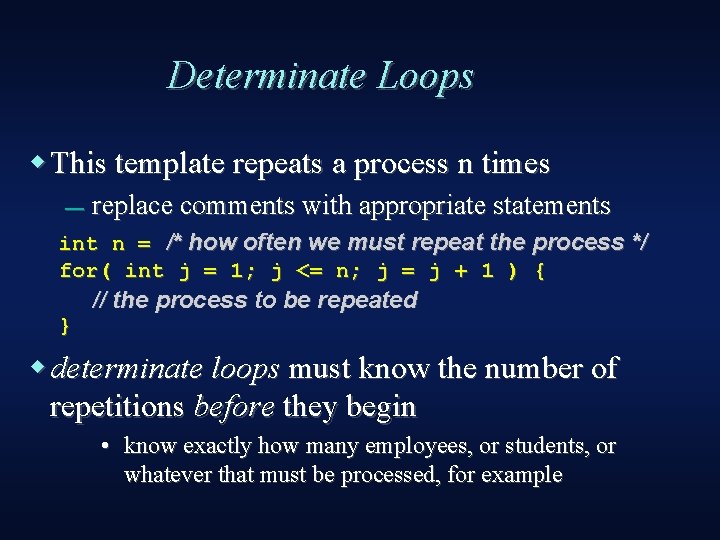
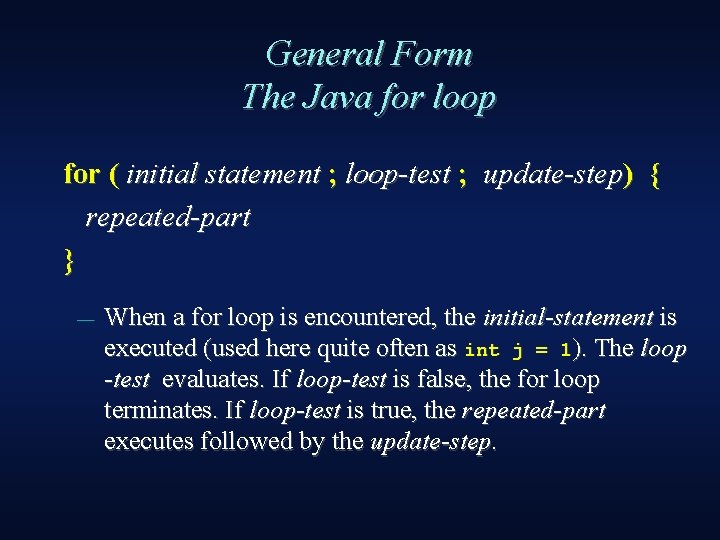
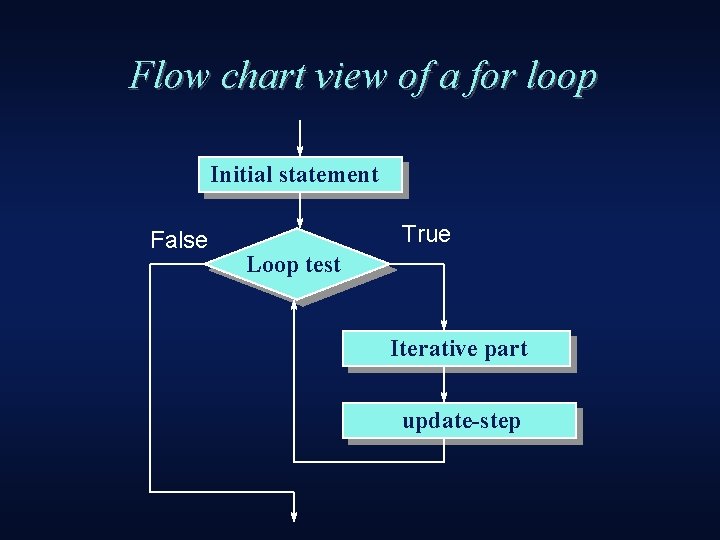
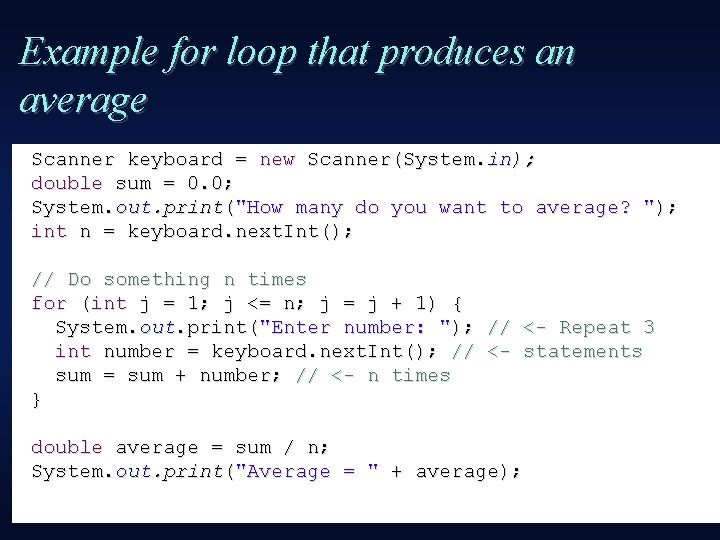
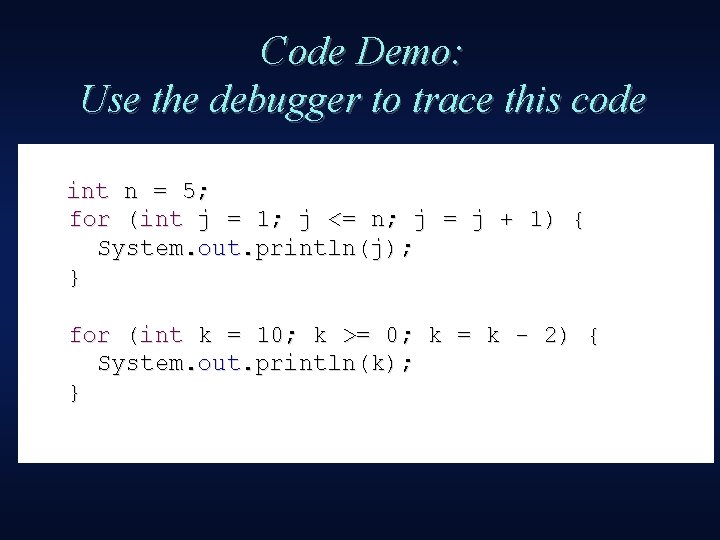
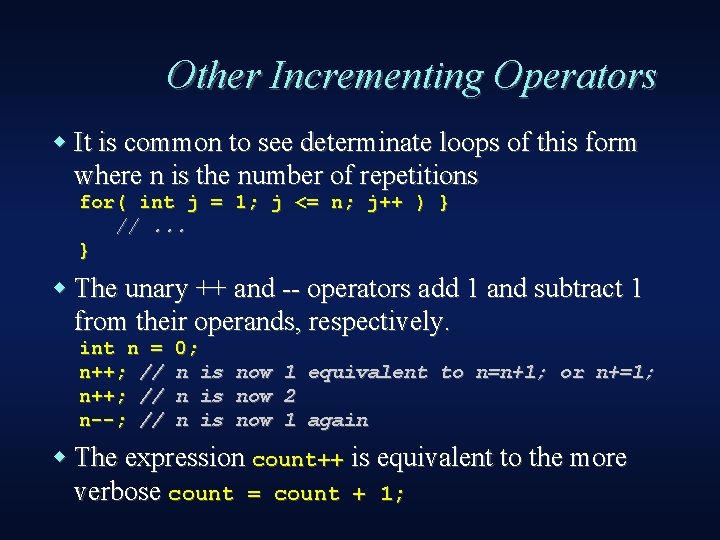
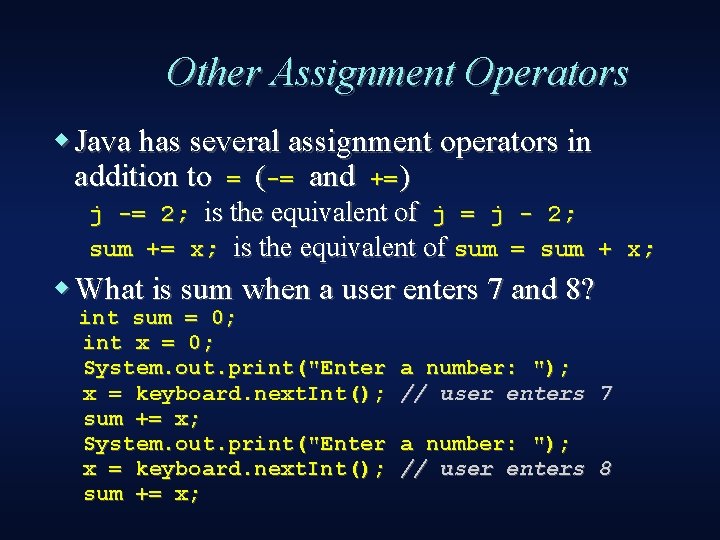
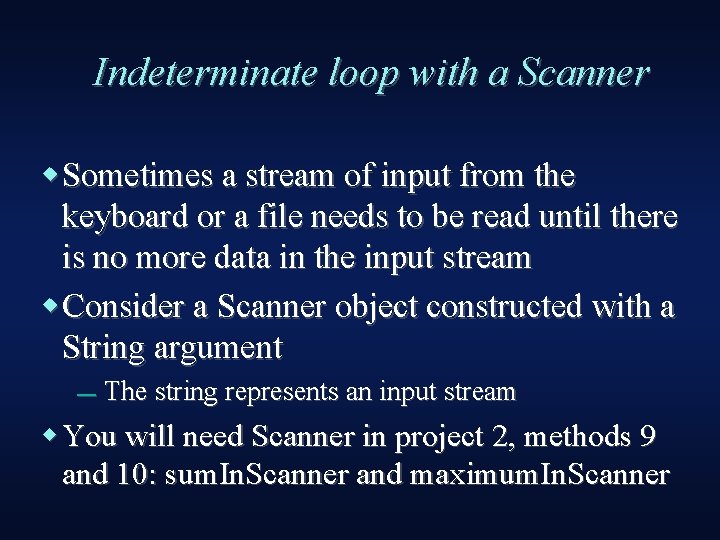
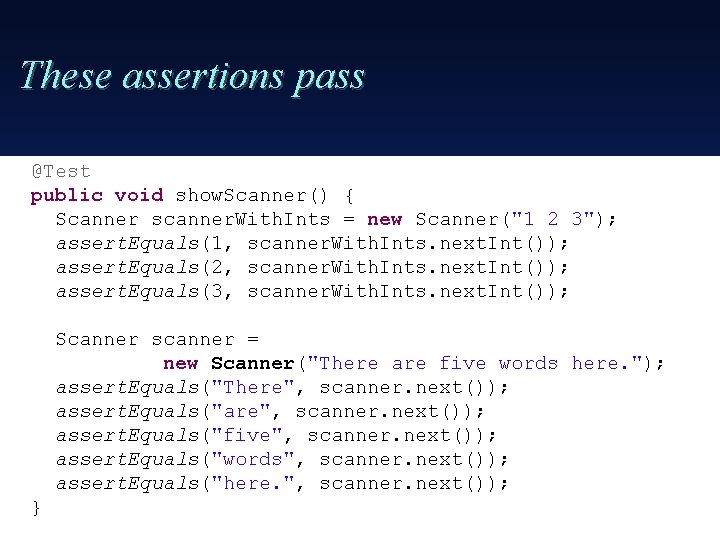
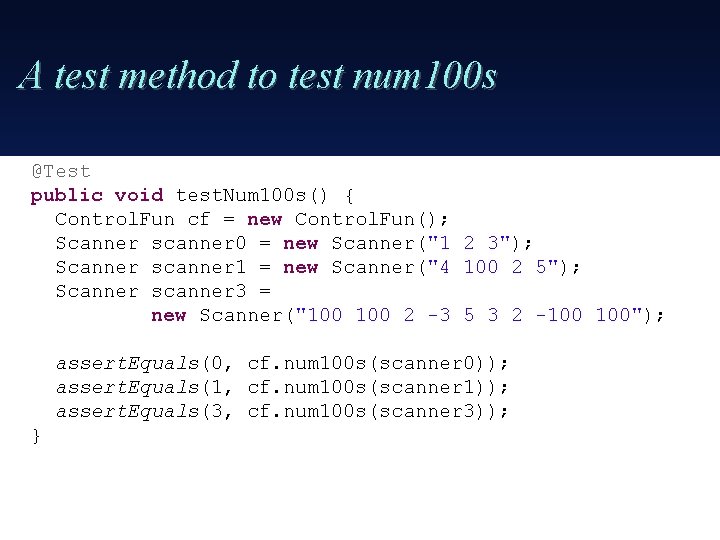
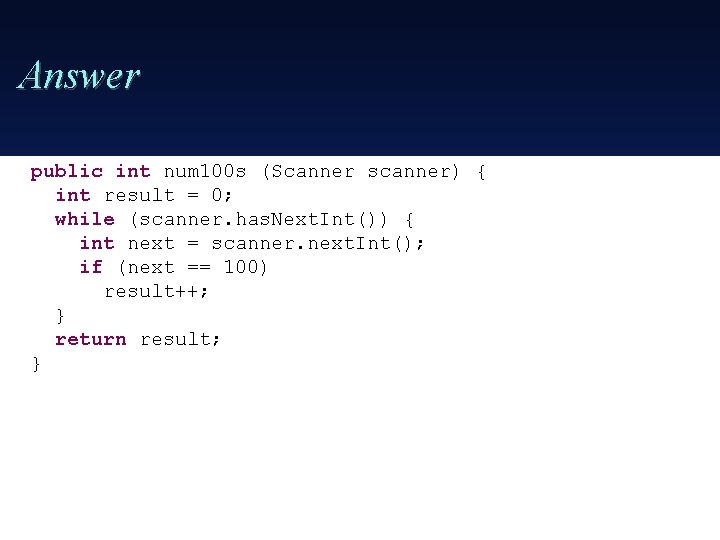
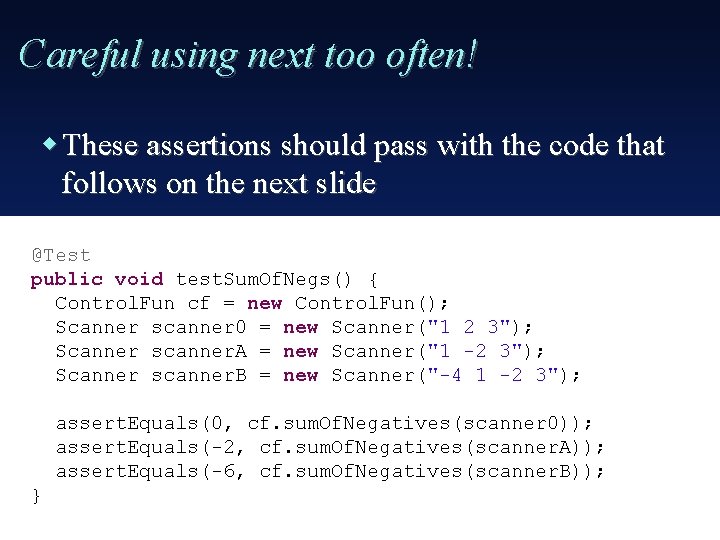
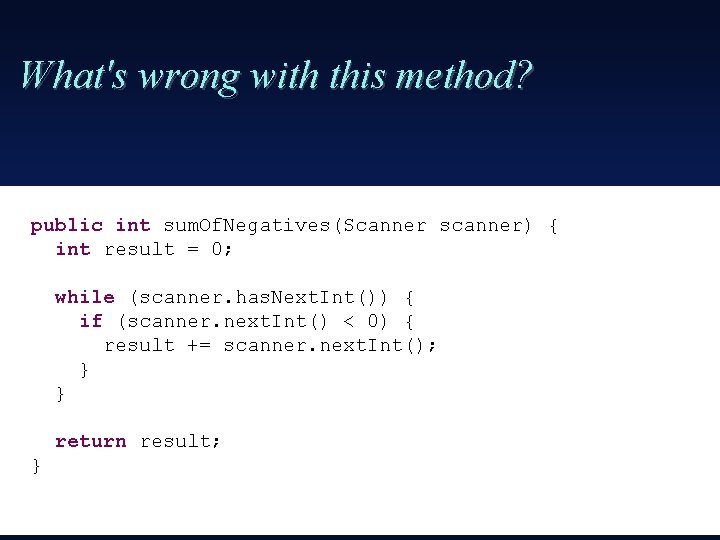
- Slides: 16
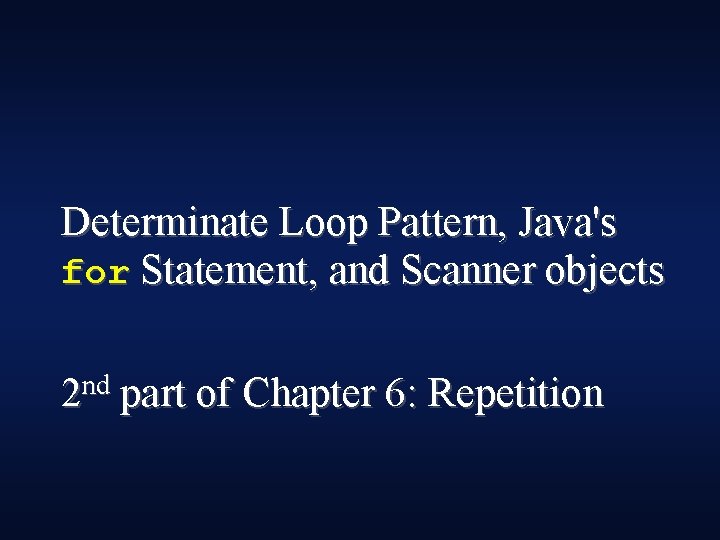
Determinate Loop Pattern, Java's for Statement, and Scanner objects 2 nd part of Chapter 6: Repetition
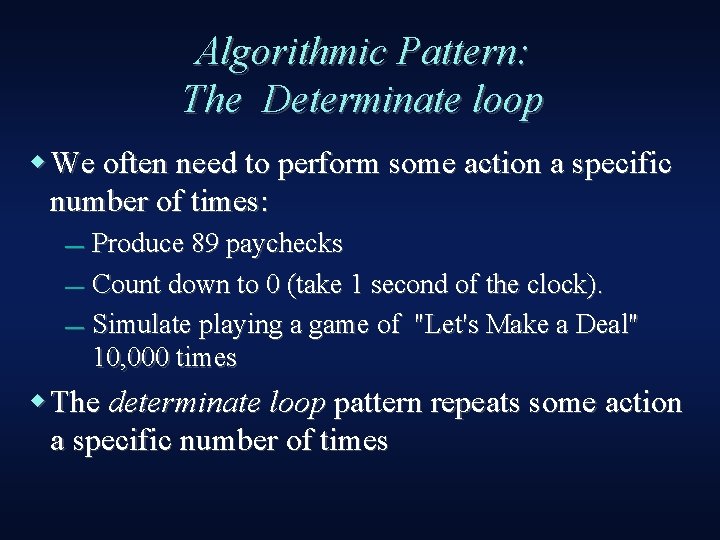
Algorithmic Pattern: The Determinate loop We often need to perform some action a specific number of times: Produce 89 paychecks — Count down to 0 (take 1 second of the clock). — Simulate playing a game of "Let's Make a Deal" 10, 000 times — The determinate loop pattern repeats some action a specific number of times
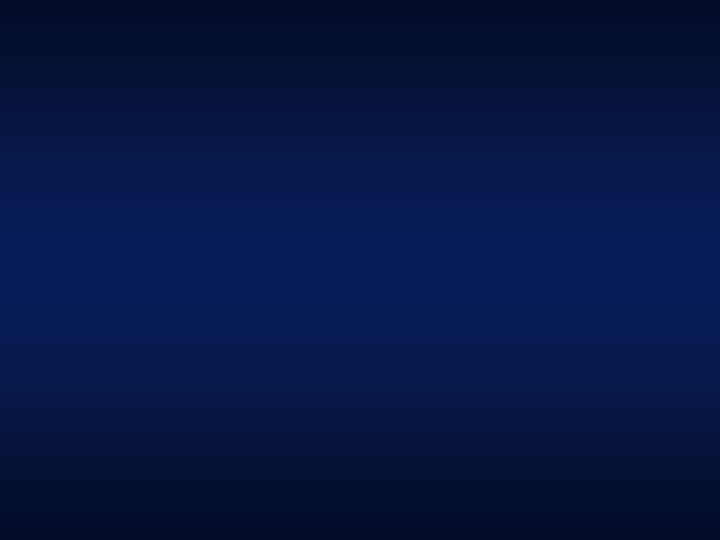
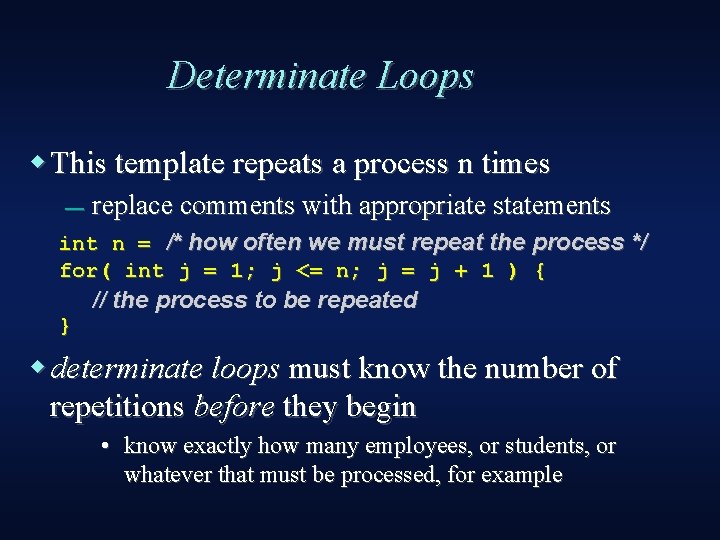
Determinate Loops This template repeats a process n times — replace comments with appropriate statements int n = /* how often we must repeat the process */ for( int j = 1; j <= n; j = j + 1 ) { // the process to be repeated } determinate loops must know the number of repetitions before they begin • know exactly how many employees, or students, or whatever that must be processed, for example
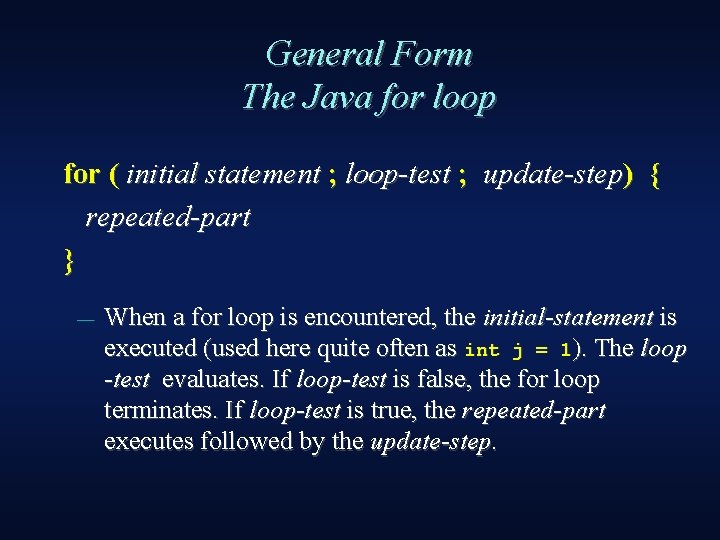
General Form The Java for loop for ( initial statement ; loop-test ; update-step) { repeated-part } — When a for loop is encountered, the initial-statement is executed (used here quite often as int j = 1). The loop -test evaluates. If loop-test is false, the for loop terminates. If loop-test is true, the repeated-part executes followed by the update-step.
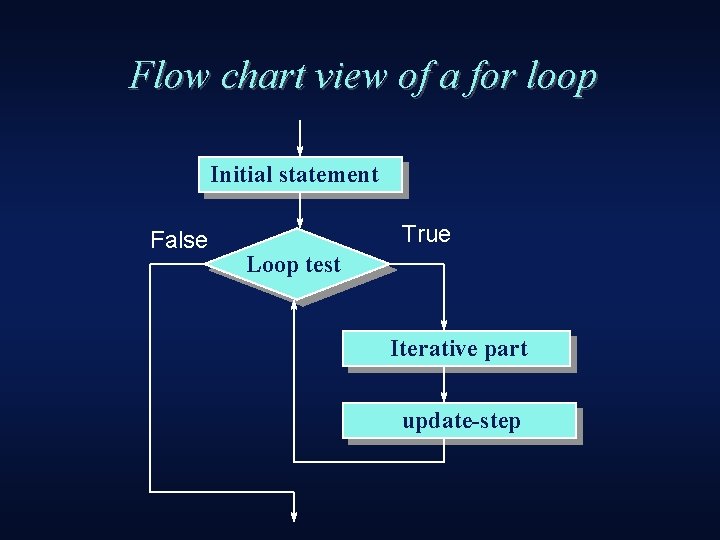
Flow chart view of a for loop Initial statement False True Loop test Iterative part update-step
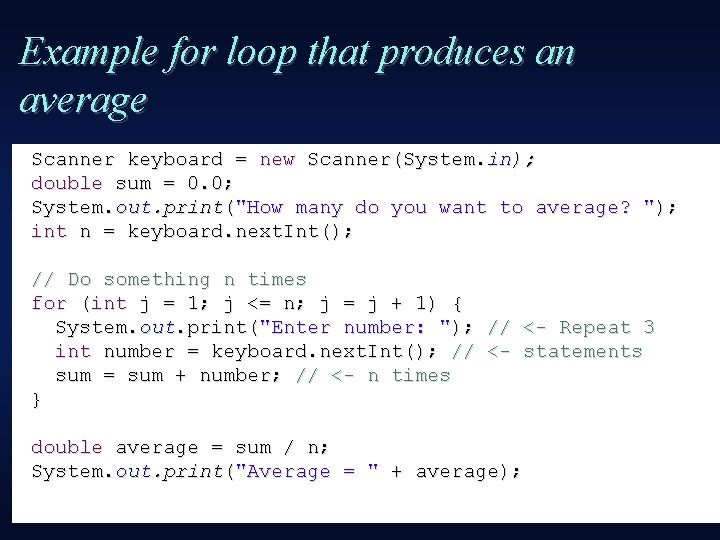
Example for loop that produces an average Scanner keyboard = new Scanner(System. in); double sum = 0. 0; System. out. print("How many do you want to average? "); int n = keyboard. next. Int(); // Do something n times for (int j = 1; j <= n; j = j + 1) { System. out. print("Enter number: "); // <- Repeat 3 int number = keyboard. next. Int(); // <- statements sum = sum + number; // <- n times } double average = sum / n; System. out. print("Average = " + average);
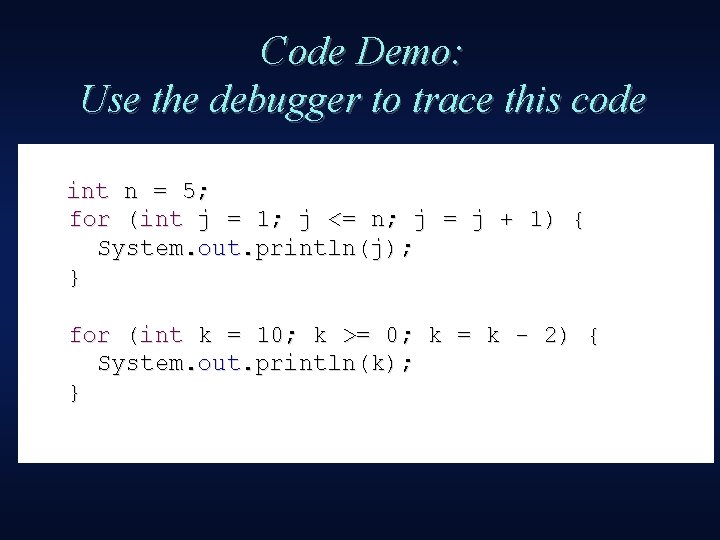
Code Demo: Use the debugger to trace this code int n = 5; for (int j = 1; j <= n; j = j + 1) { System. out. println(j); } for (int k = 10; k >= 0; k = k - 2) { System. out. println(k); }
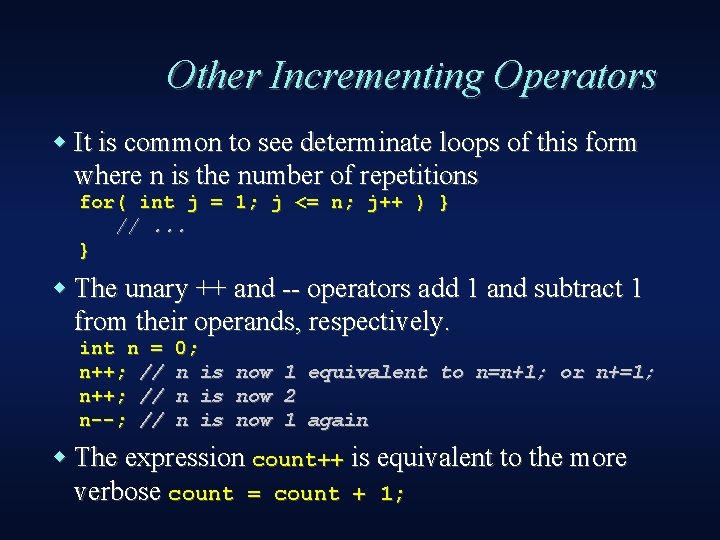
Other Incrementing Operators It is common to see determinate loops of this form where n is the number of repetitions for( int j = 1; j <= n; j++ ) } //. . . } The unary ++ and -- operators add 1 and subtract 1 from their operands, respectively. int n = n++; // n--; // 0; n is now 1 equivalent to n=n+1; or n+=1; n is now 2 n is now 1 again The expression count++ is equivalent to the more verbose count = count + 1;
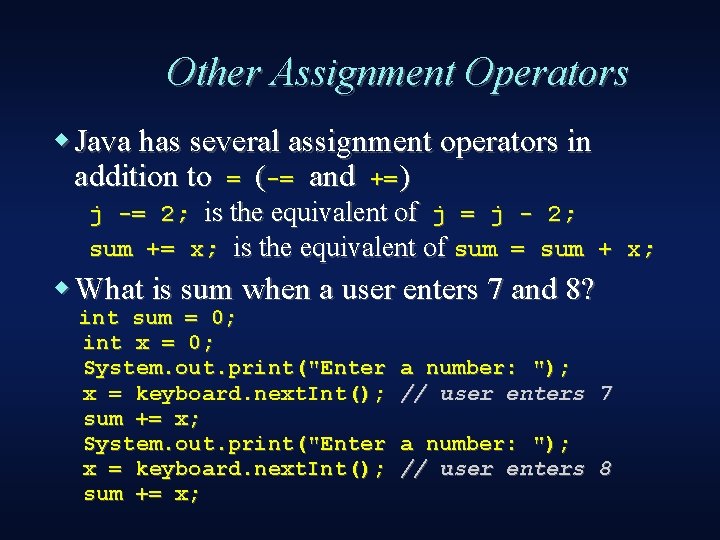
Other Assignment Operators Java has several assignment operators in addition to = (-= and +=) j -= 2; is the equivalent of j = j - 2; sum += x; is the equivalent of sum = sum + x; What is sum when a user enters 7 and 8? int sum = 0; int x = 0; System. out. print("Enter x = keyboard. next. Int(); sum += x; a number: "); // user enters 7 a number: "); // user enters 8
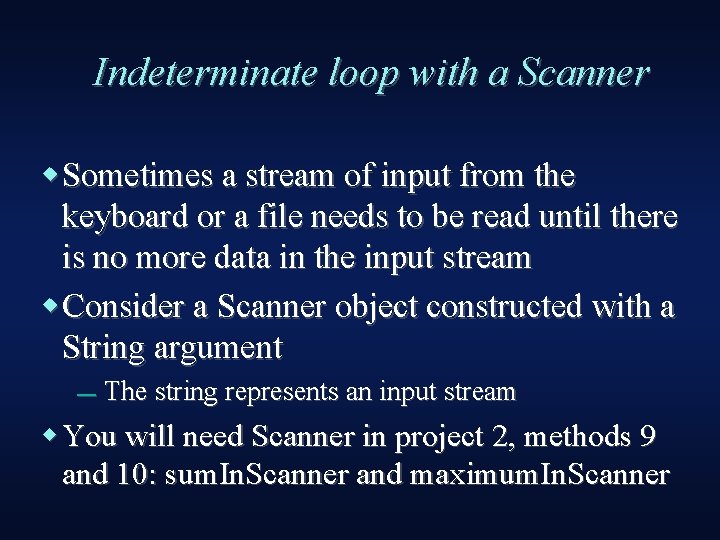
Indeterminate loop with a Scanner Sometimes a stream of input from the keyboard or a file needs to be read until there is no more data in the input stream Consider a Scanner object constructed with a String argument — The string represents an input stream You will need Scanner in project 2, methods 9 and 10: sum. In. Scanner and maximum. In. Scanner
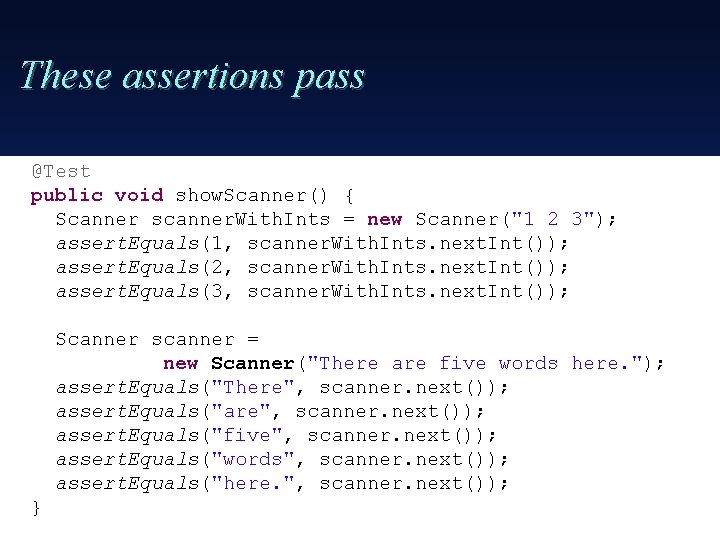
These assertions pass @Test public void show. Scanner() { Scanner scanner. With. Ints = new Scanner("1 2 3"); assert. Equals(1, scanner. With. Ints. next. Int()); assert. Equals(2, scanner. With. Ints. next. Int()); assert. Equals(3, scanner. With. Ints. next. Int()); Scanner scanner = new Scanner("There are five words here. "); assert. Equals("There", scanner. next()); assert. Equals("are", scanner. next()); assert. Equals("five", scanner. next()); assert. Equals("words", scanner. next()); assert. Equals("here. ", scanner. next()); }
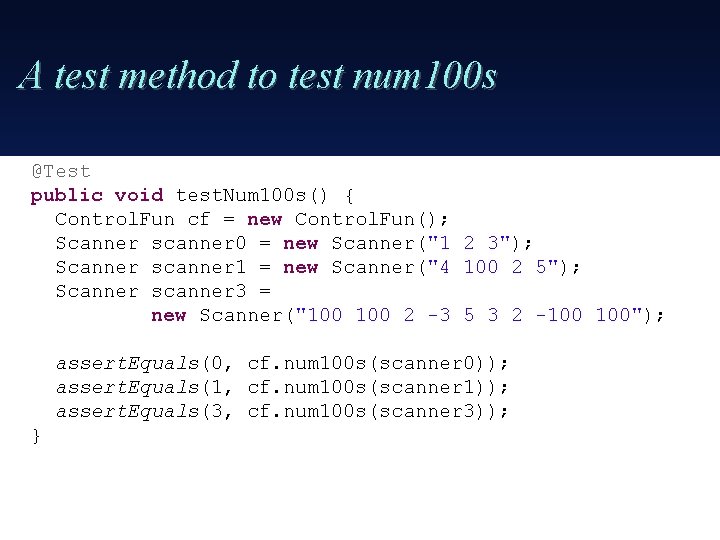
A test method to test num 100 s @Test public void test. Num 100 s() { Control. Fun cf = new Control. Fun(); Scanner scanner 0 = new Scanner("1 2 3"); Scanner scanner 1 = new Scanner("4 100 2 5"); Scanner scanner 3 = new Scanner("100 2 -3 5 3 2 -100 100"); assert. Equals(0, cf. num 100 s(scanner 0)); assert. Equals(1, cf. num 100 s(scanner 1)); assert. Equals(3, cf. num 100 s(scanner 3)); }
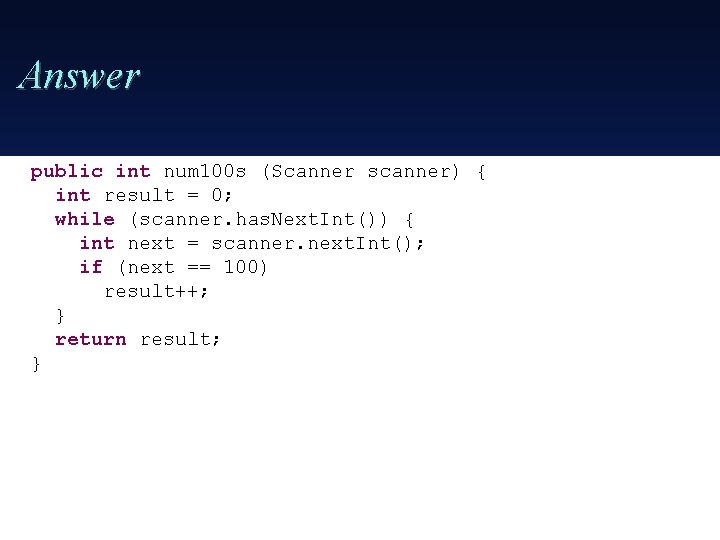
Answer public int num 100 s (Scanner scanner) { int result = 0; while (scanner. has. Next. Int()) { int next = scanner. next. Int(); if (next == 100) result++; } return result; }
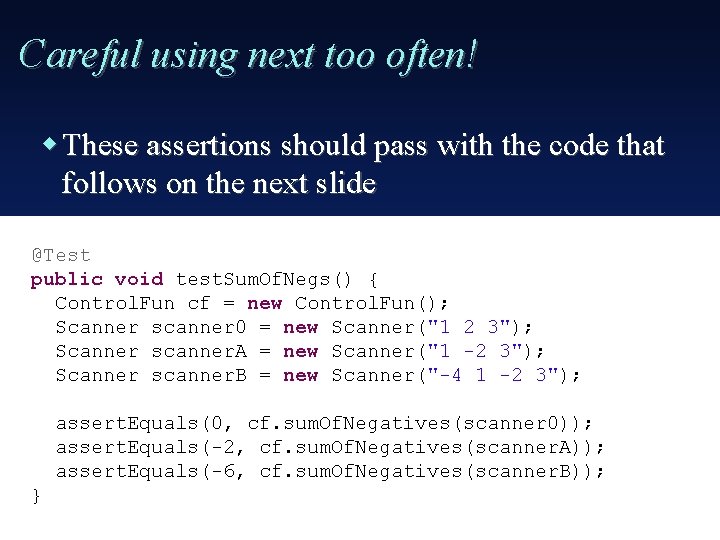
Careful using next too often! These assertions should pass with the code that follows on the next slide @Test public void test. Sum. Of. Negs() { Control. Fun cf = new Control. Fun(); Scanner scanner 0 = new Scanner("1 2 3"); Scanner scanner. A = new Scanner("1 -2 3"); Scanner scanner. B = new Scanner("-4 1 -2 3"); assert. Equals(0, cf. sum. Of. Negatives(scanner 0)); assert. Equals(-2, cf. sum. Of. Negatives(scanner. A)); assert. Equals(-6, cf. sum. Of. Negatives(scanner. B)); }
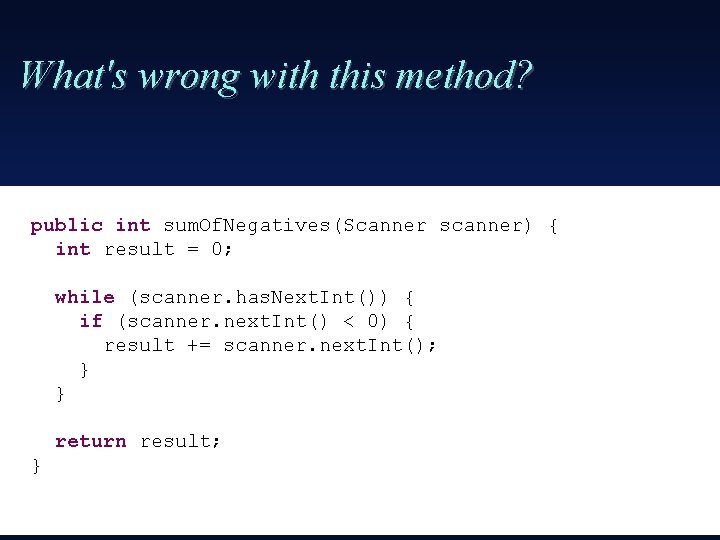
What's wrong with this method? public int sum. Of. Negatives(Scanner scanner) { int result = 0; while (scanner. has. Next. Int()) { if (scanner. next. Int() < 0) { result += scanner. next. Int(); } } return result; }