Design Patterns CS 123CS 231 1 Outline z
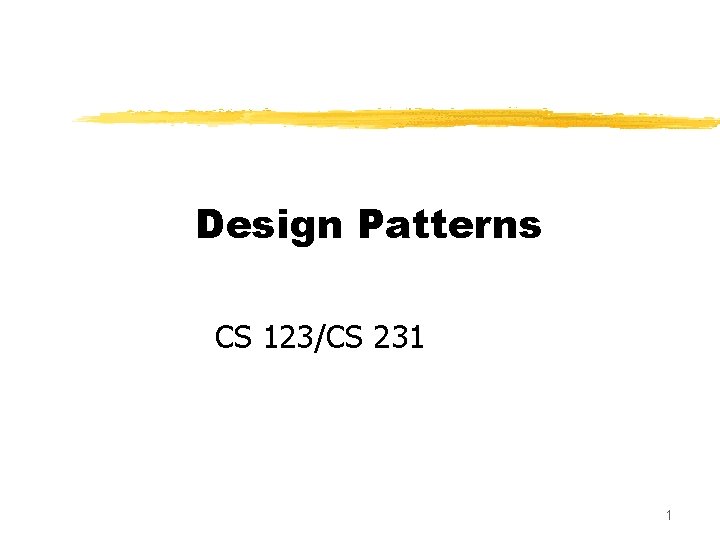
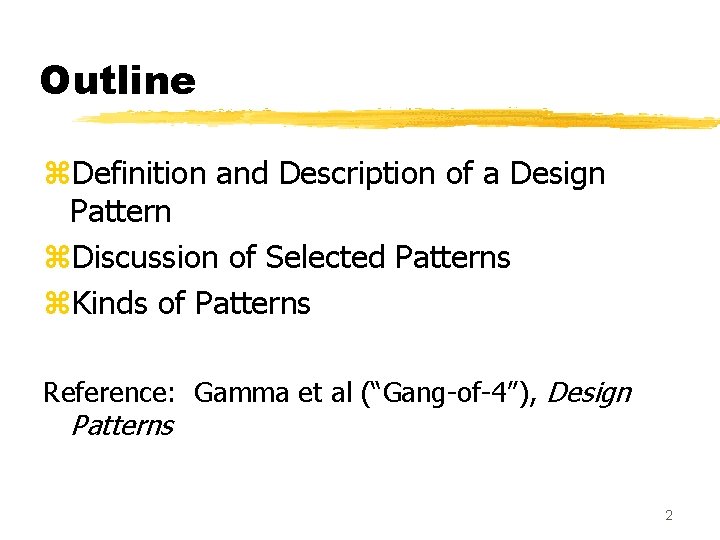
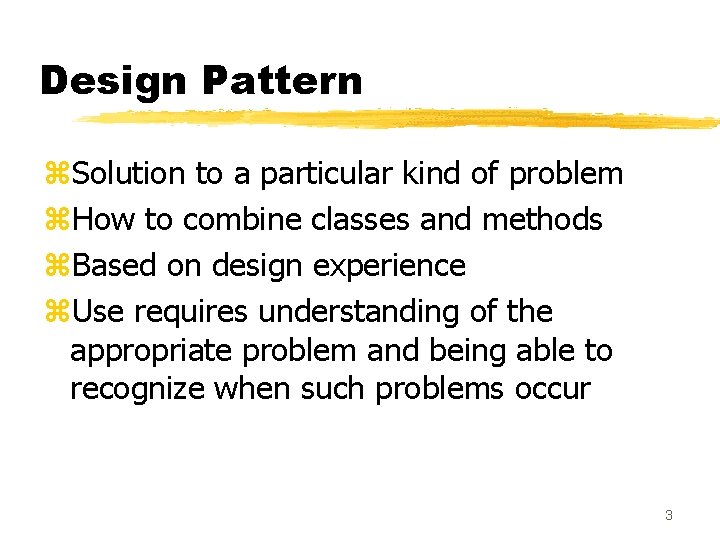
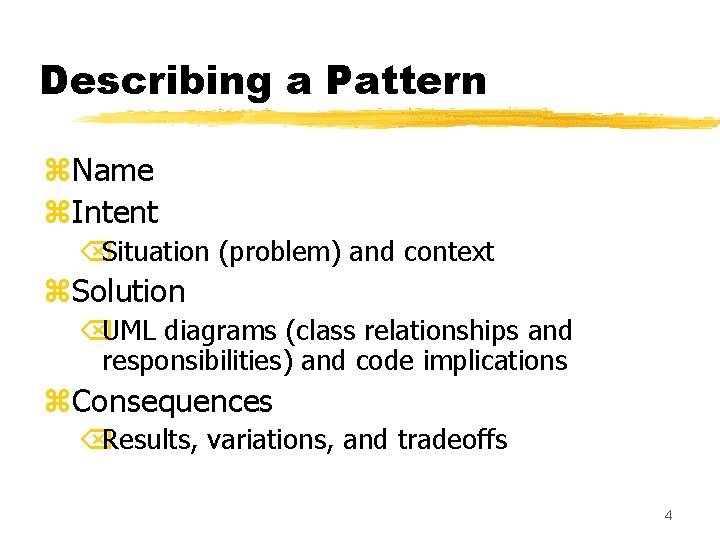
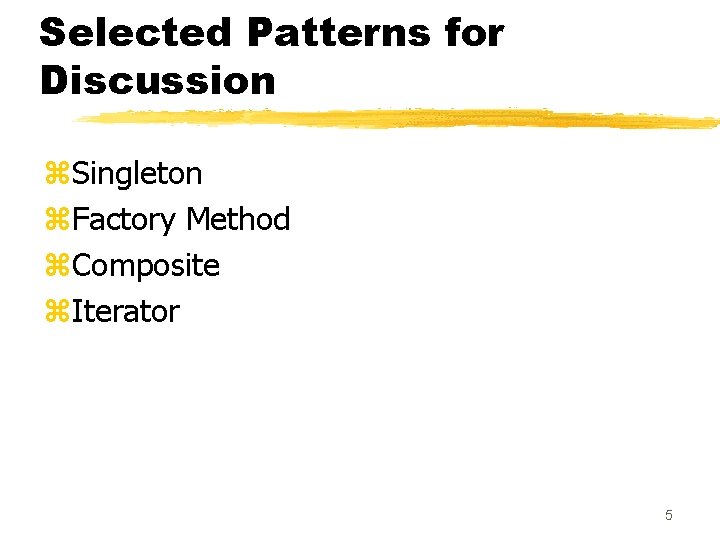
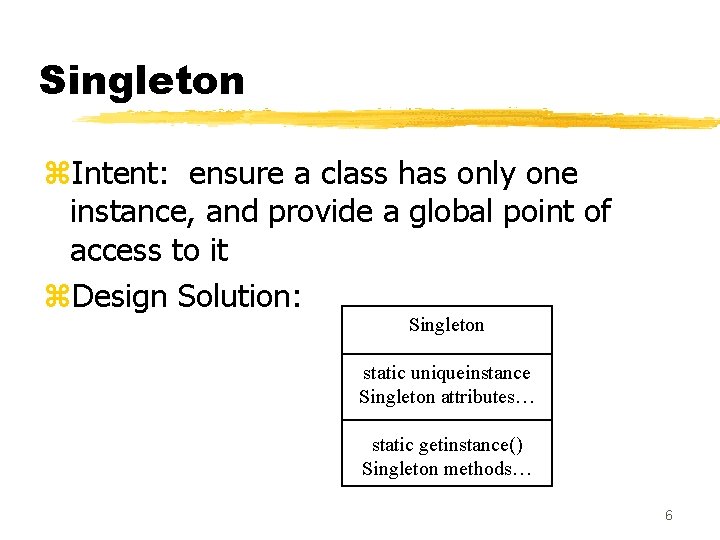
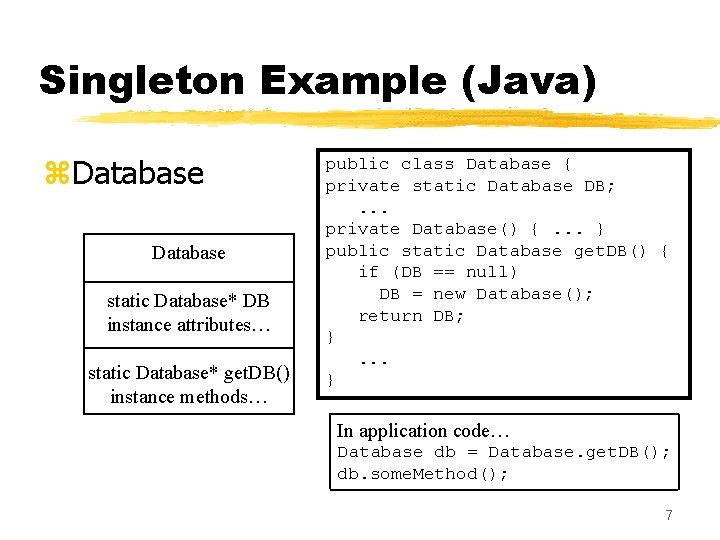
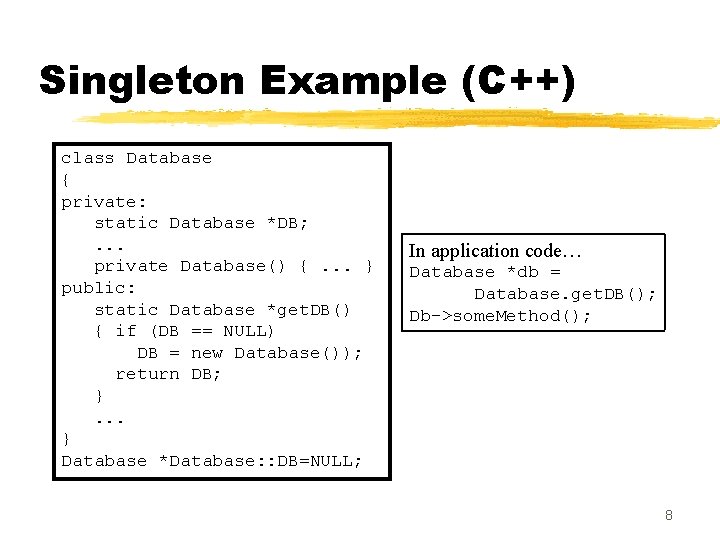
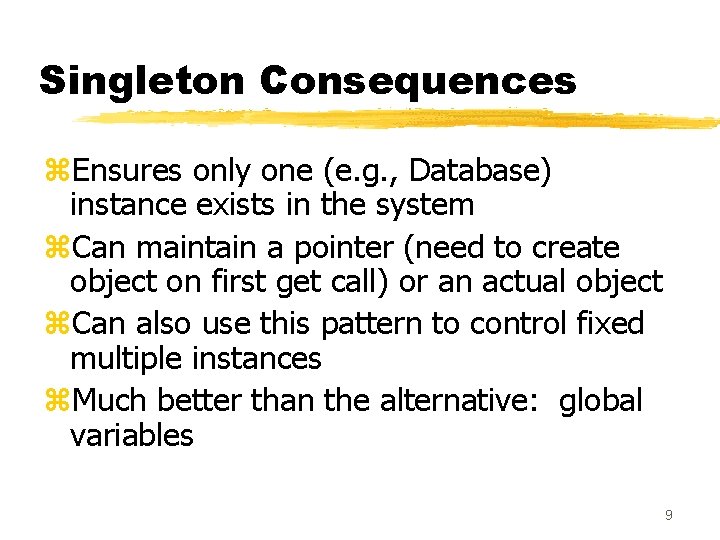
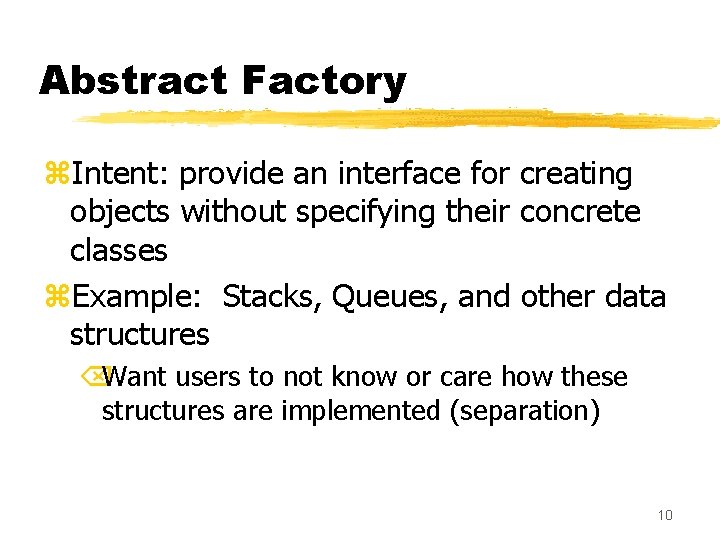
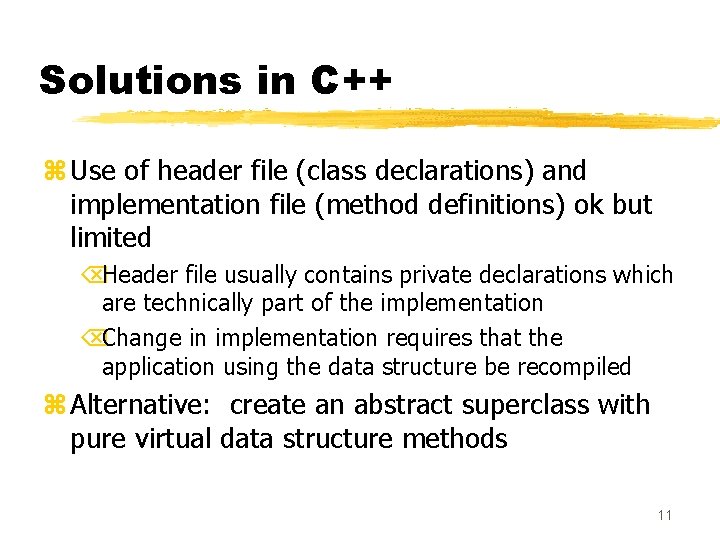
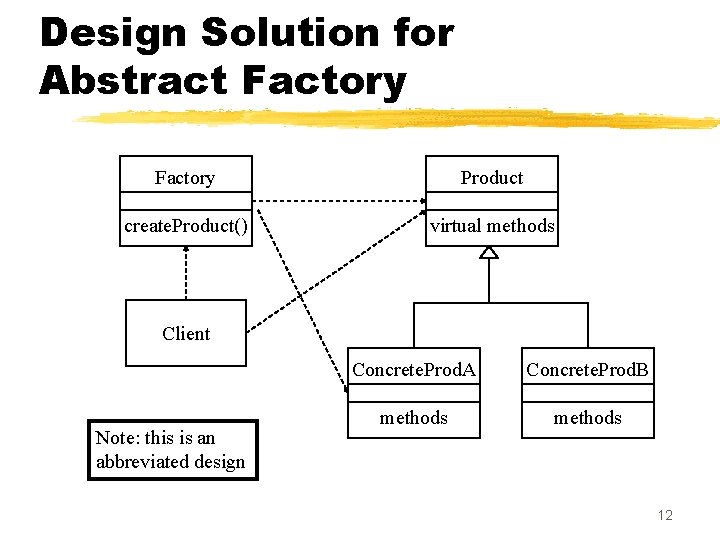
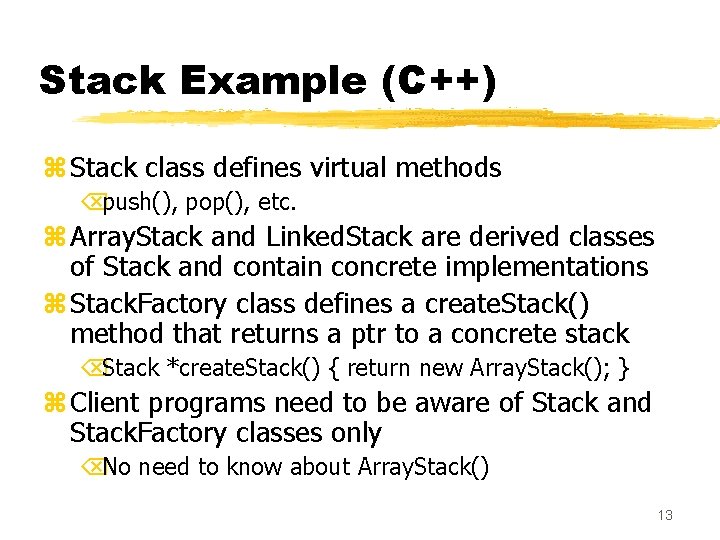
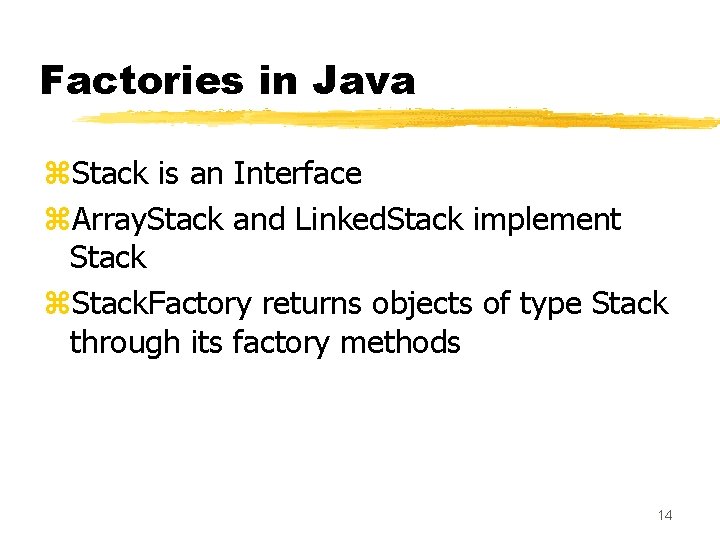
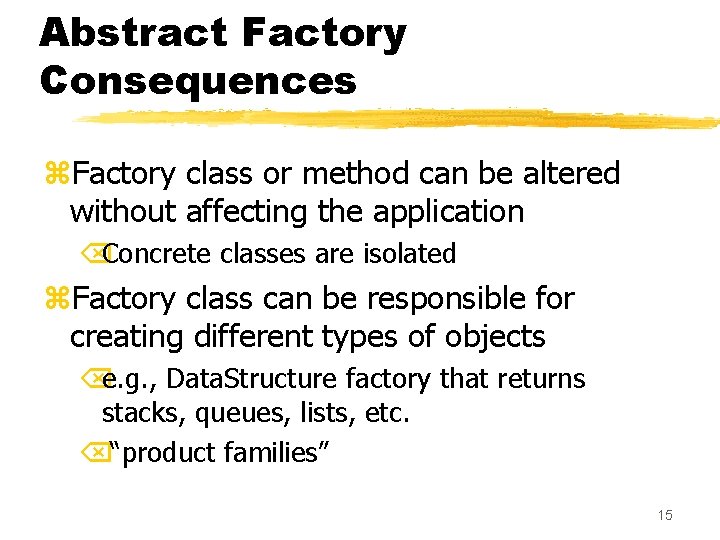
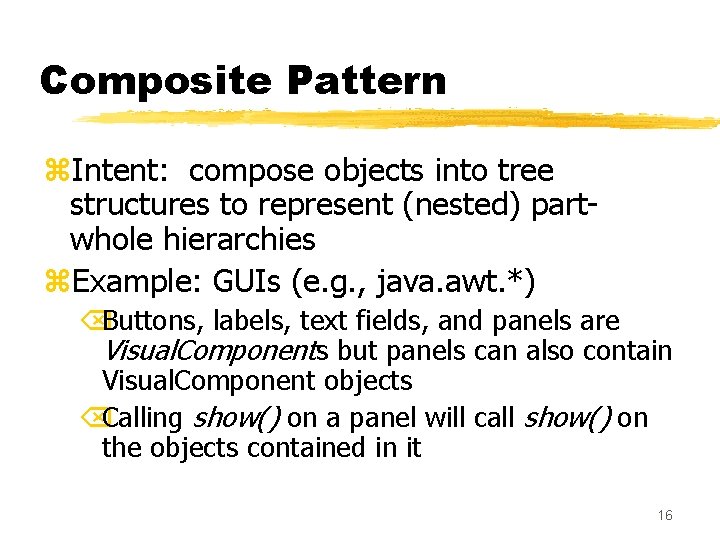
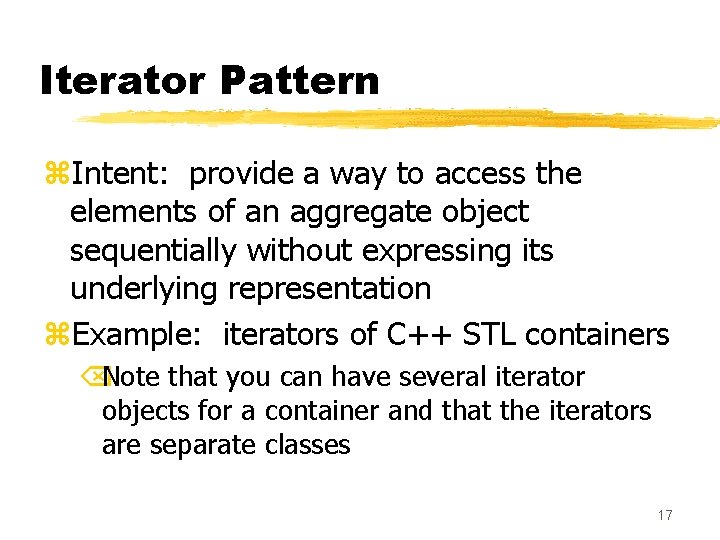
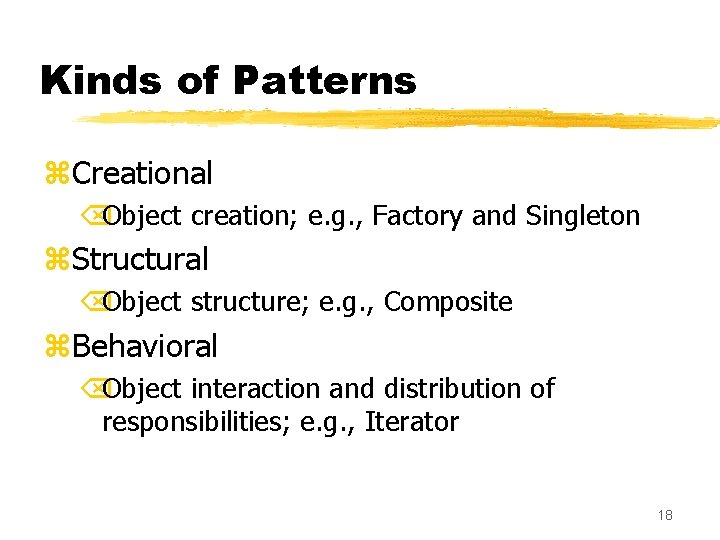
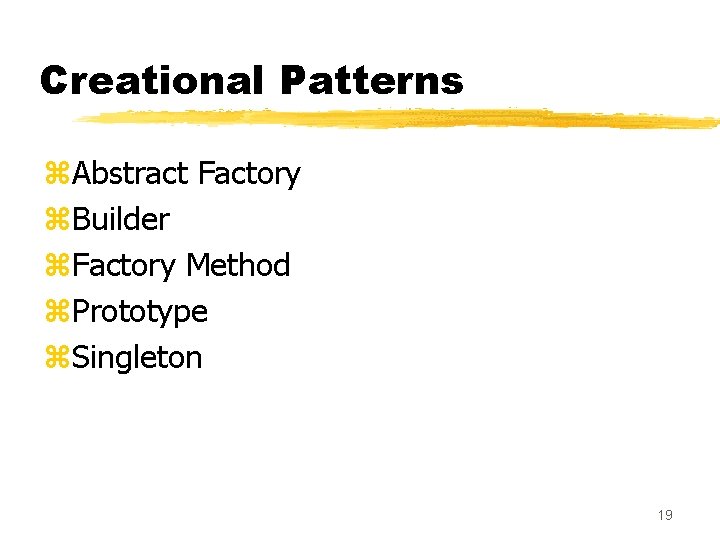
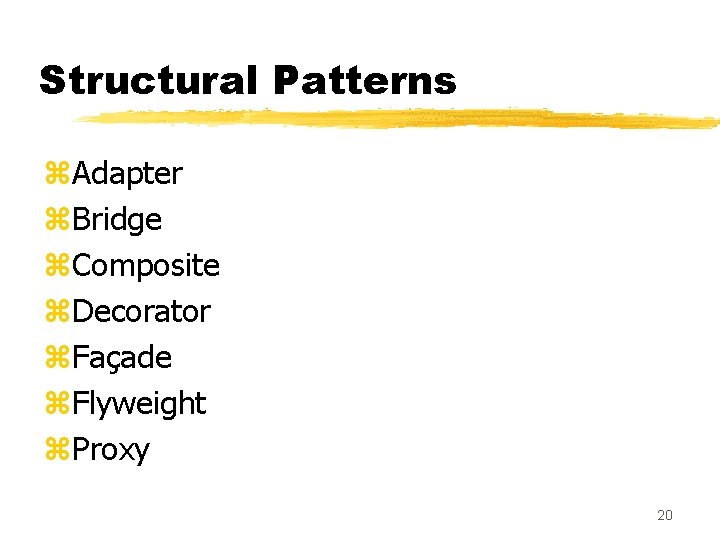
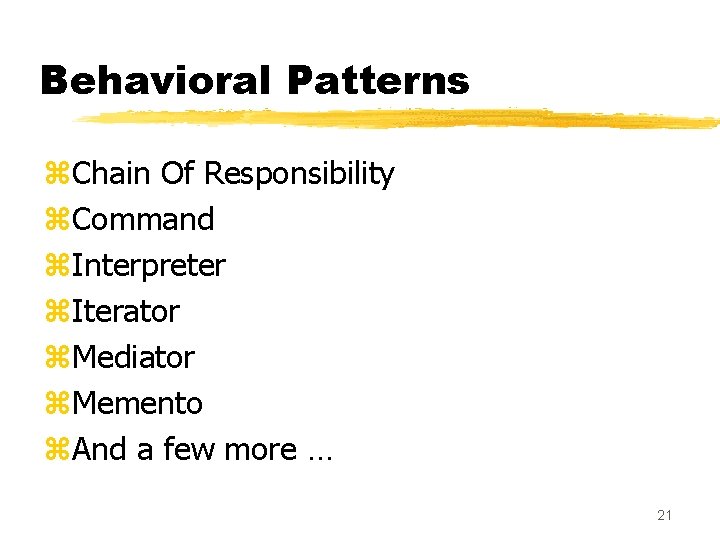
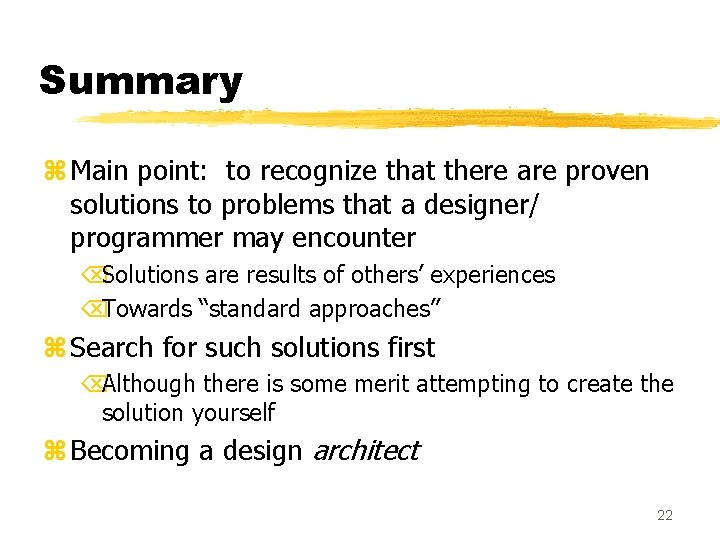
- Slides: 22
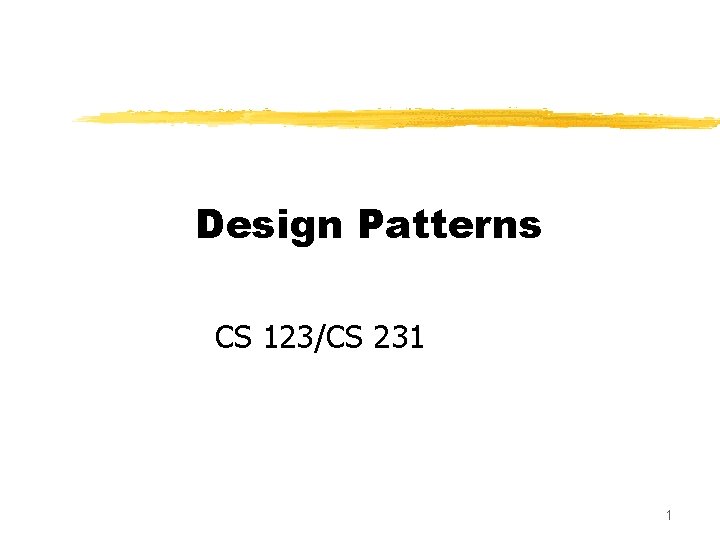
Design Patterns CS 123/CS 231 1
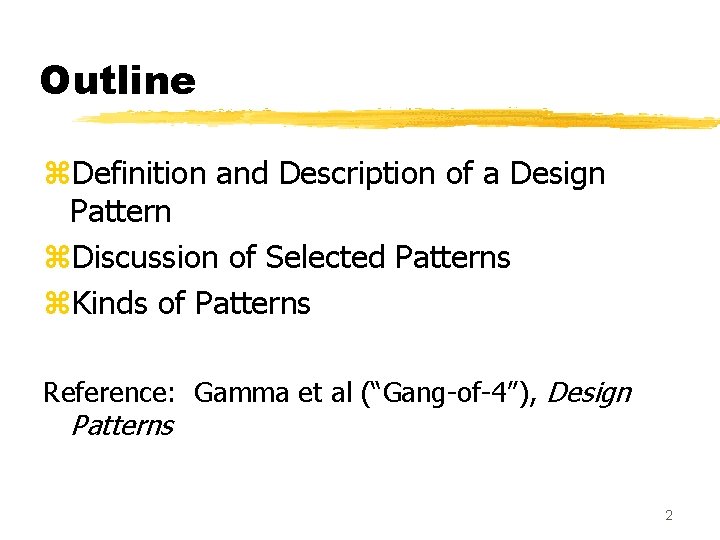
Outline z. Definition and Description of a Design Pattern z. Discussion of Selected Patterns z. Kinds of Patterns Reference: Gamma et al (“Gang-of-4”), Design Patterns 2
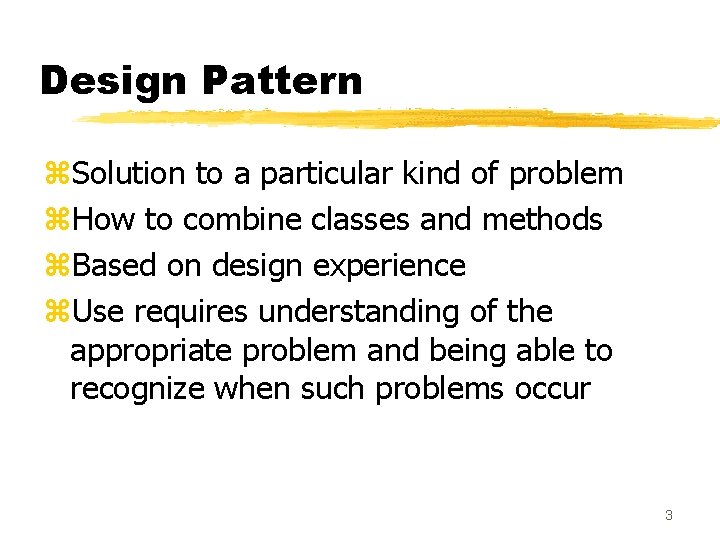
Design Pattern z. Solution to a particular kind of problem z. How to combine classes and methods z. Based on design experience z. Use requires understanding of the appropriate problem and being able to recognize when such problems occur 3
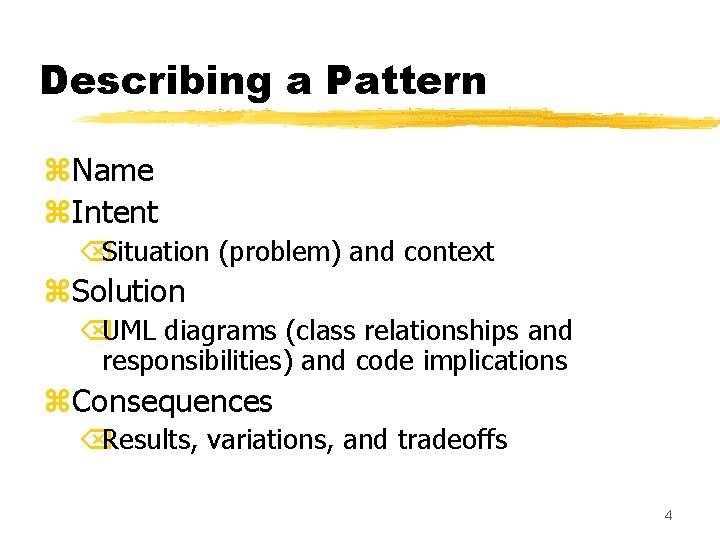
Describing a Pattern z. Name z. Intent ÕSituation (problem) and context z. Solution ÕUML diagrams (class relationships and responsibilities) and code implications z. Consequences ÕResults, variations, and tradeoffs 4
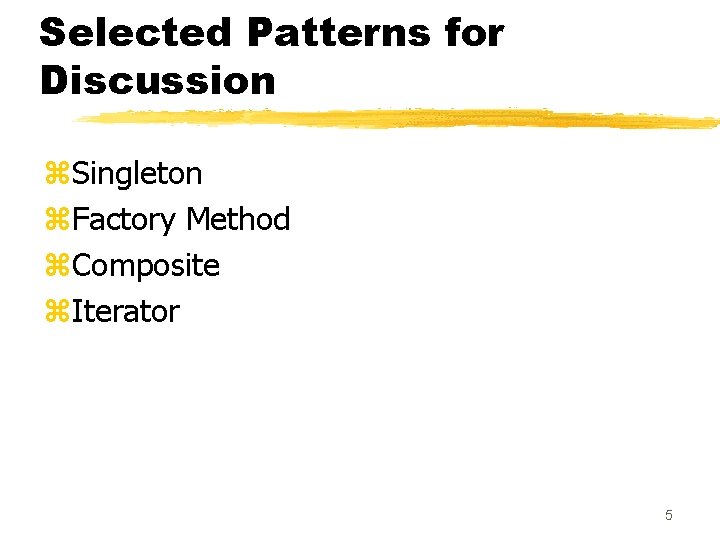
Selected Patterns for Discussion z. Singleton z. Factory Method z. Composite z. Iterator 5
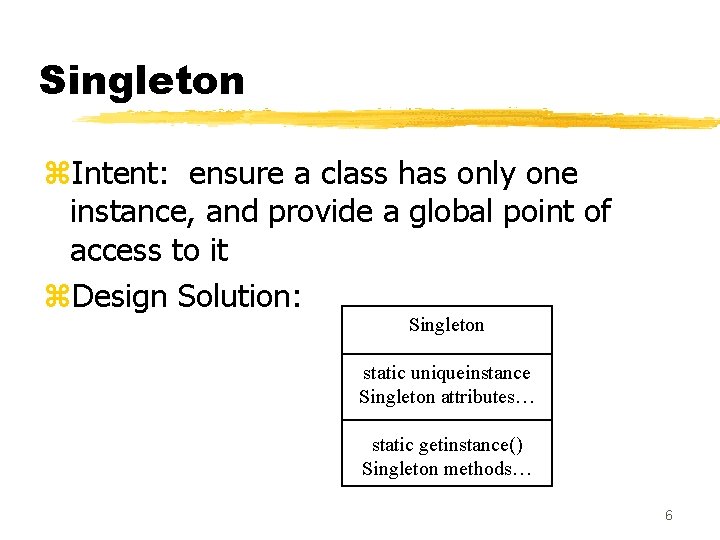
Singleton z. Intent: ensure a class has only one instance, and provide a global point of access to it z. Design Solution: Singleton static uniqueinstance Singleton attributes… static getinstance() Singleton methods… 6
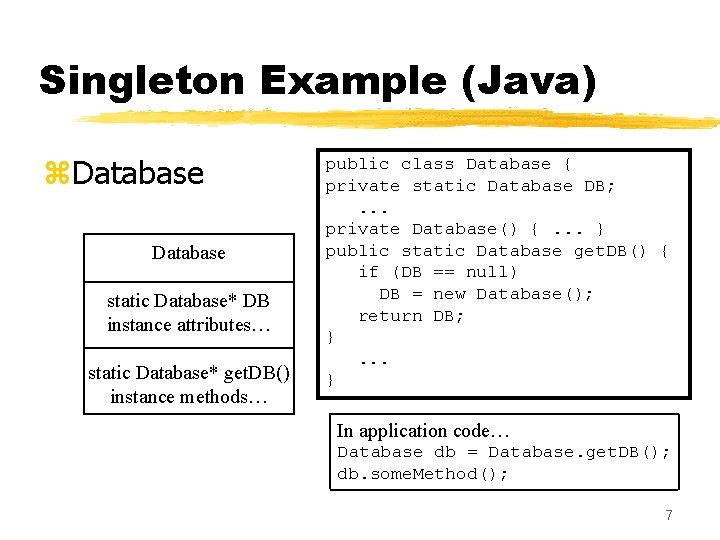
Singleton Example (Java) z. Database static Database* DB instance attributes… static Database* get. DB() instance methods… public class Database { private static Database DB; . . . private Database() {. . . } public static Database get. DB() { if (DB == null) DB = new Database(); return DB; }. . . } In application code… Database db = Database. get. DB(); db. some. Method(); 7
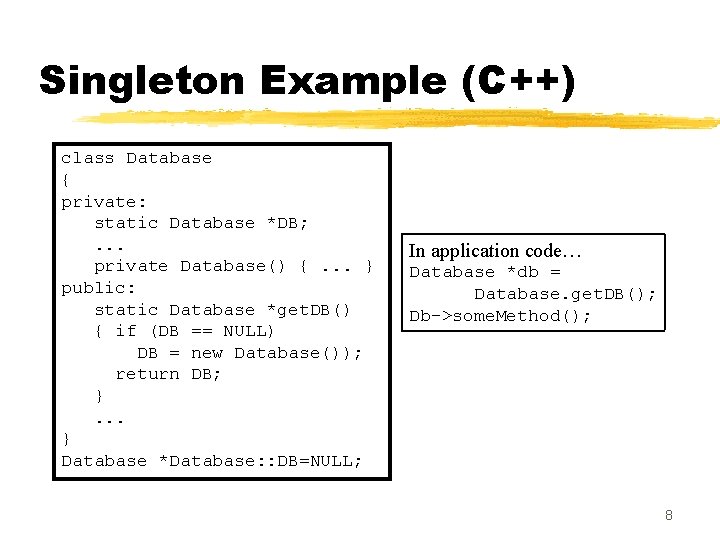
Singleton Example (C++) class Database { private: static Database *DB; . . . private Database() {. . . } public: static Database *get. DB() { if (DB == NULL) DB = new Database()); return DB; }. . . } Database *Database: : DB=NULL; In application code… Database *db = Database. get. DB(); Db->some. Method(); 8
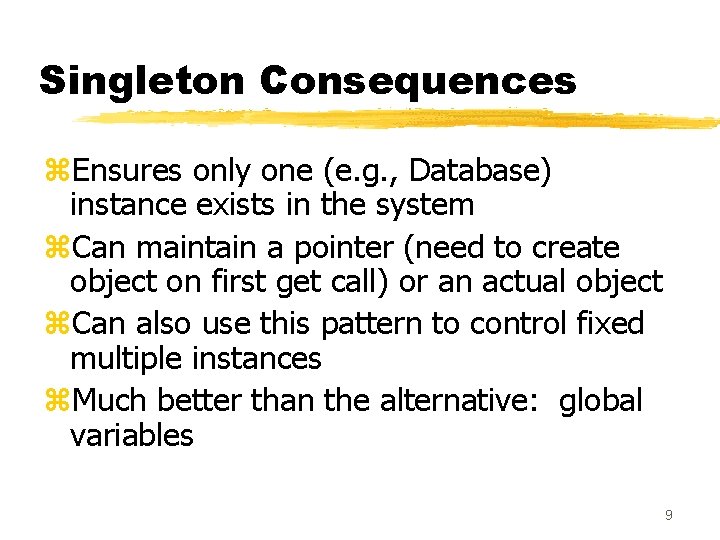
Singleton Consequences z. Ensures only one (e. g. , Database) instance exists in the system z. Can maintain a pointer (need to create object on first get call) or an actual object z. Can also use this pattern to control fixed multiple instances z. Much better than the alternative: global variables 9
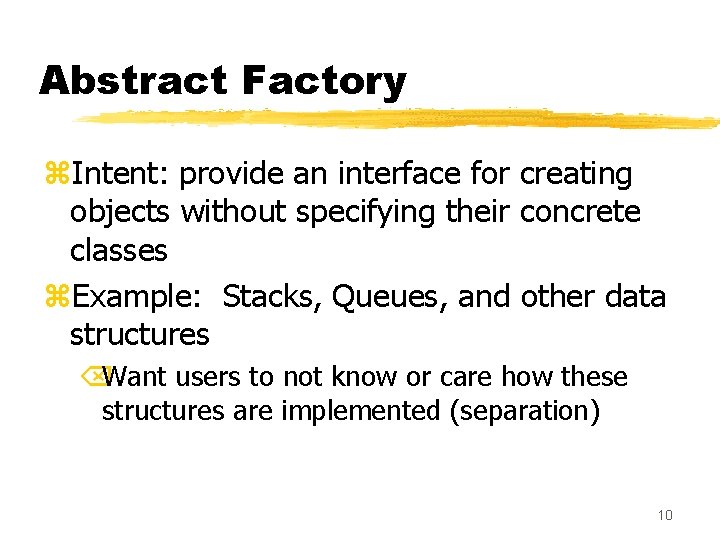
Abstract Factory z. Intent: provide an interface for creating objects without specifying their concrete classes z. Example: Stacks, Queues, and other data structures ÕWant users to not know or care how these structures are implemented (separation) 10
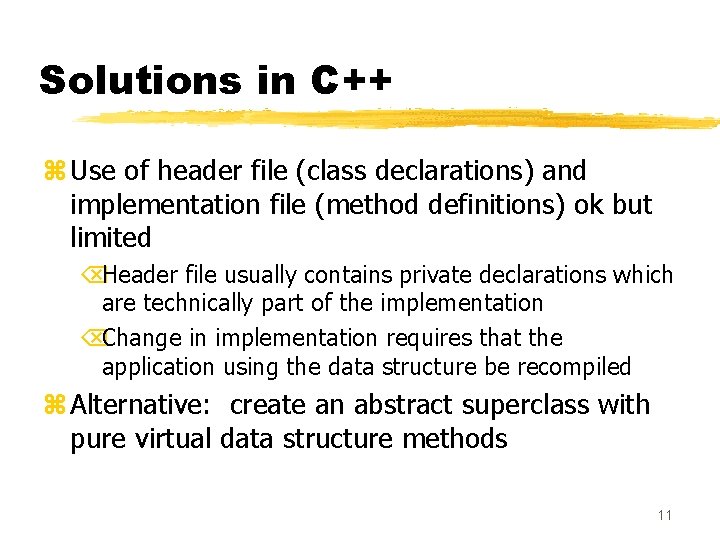
Solutions in C++ z Use of header file (class declarations) and implementation file (method definitions) ok but limited ÕHeader file usually contains private declarations which are technically part of the implementation ÕChange in implementation requires that the application using the data structure be recompiled z Alternative: create an abstract superclass with pure virtual data structure methods 11
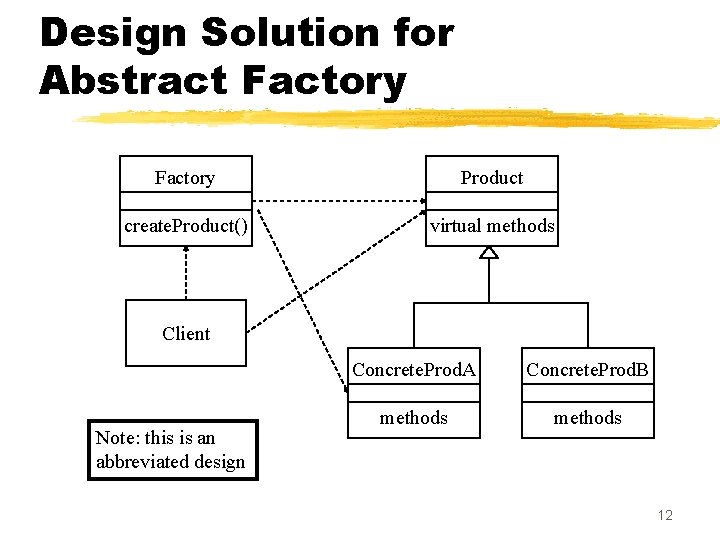
Design Solution for Abstract Factory Product create. Product() virtual methods Client Note: this is an abbreviated design Concrete. Prod. A Concrete. Prod. B methods 12
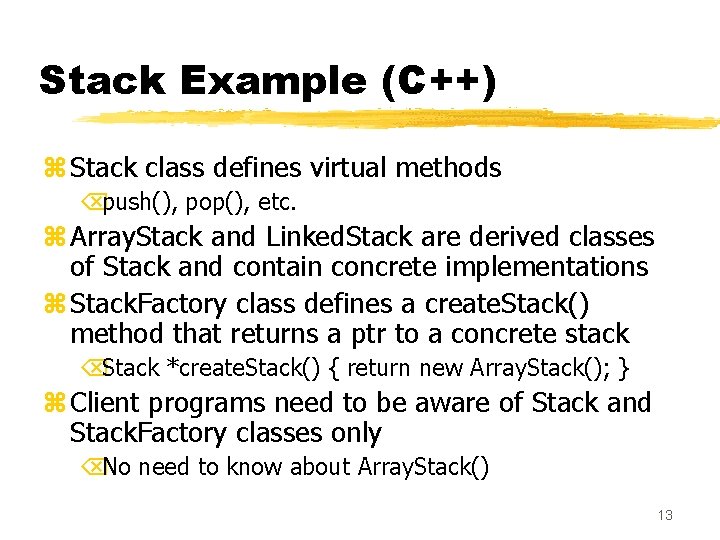
Stack Example (C++) z Stack class defines virtual methods Õpush(), pop(), etc. z Array. Stack and Linked. Stack are derived classes of Stack and contain concrete implementations z Stack. Factory class defines a create. Stack() method that returns a ptr to a concrete stack ÕStack *create. Stack() { return new Array. Stack(); } z Client programs need to be aware of Stack and Stack. Factory classes only ÕNo need to know about Array. Stack() 13
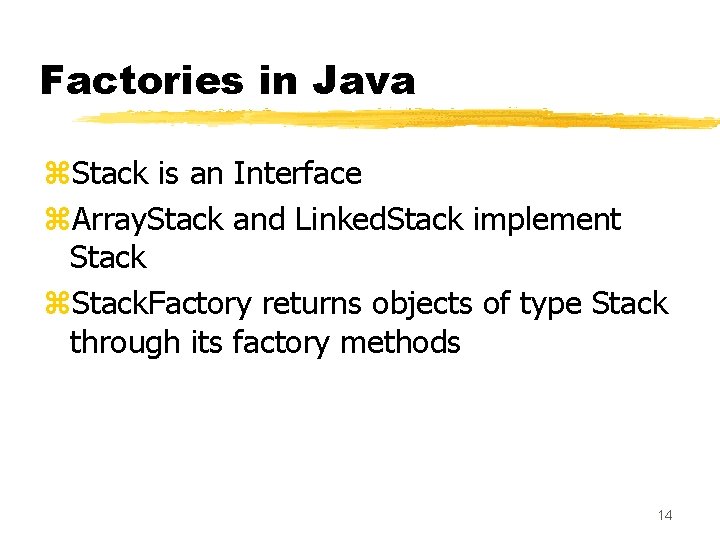
Factories in Java z. Stack is an Interface z. Array. Stack and Linked. Stack implement Stack z. Stack. Factory returns objects of type Stack through its factory methods 14
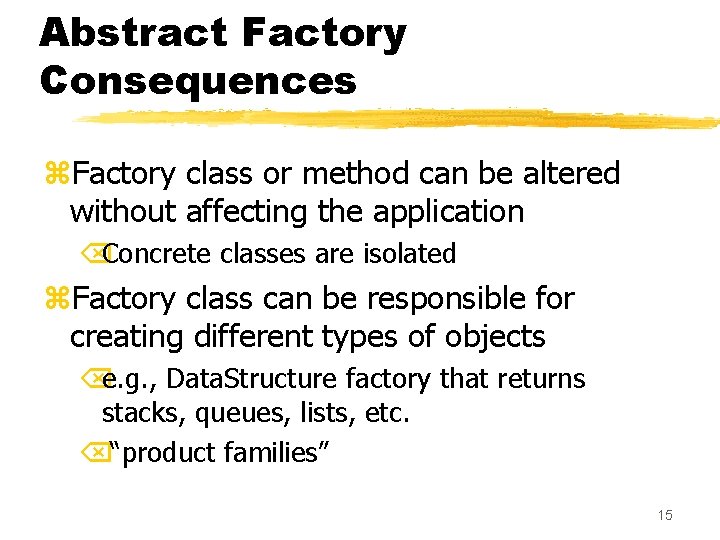
Abstract Factory Consequences z. Factory class or method can be altered without affecting the application ÕConcrete classes are isolated z. Factory class can be responsible for creating different types of objects Õe. g. , Data. Structure factory that returns stacks, queues, lists, etc. Õ“product families” 15
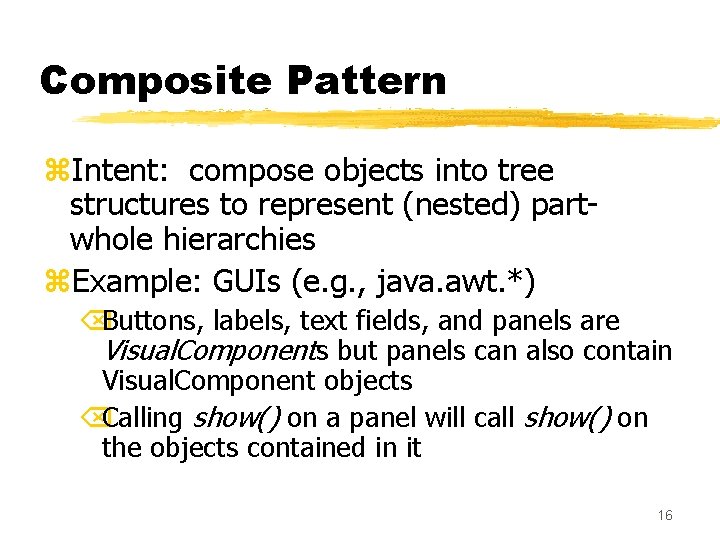
Composite Pattern z. Intent: compose objects into tree structures to represent (nested) partwhole hierarchies z. Example: GUIs (e. g. , java. awt. *) ÕButtons, labels, text fields, and panels are Visual. Components but panels can also contain Visual. Component objects ÕCalling show() on a panel will call show() on the objects contained in it 16
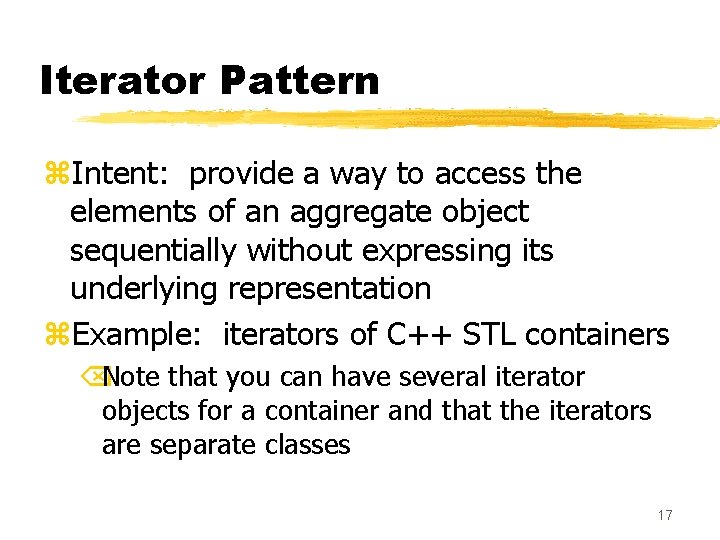
Iterator Pattern z. Intent: provide a way to access the elements of an aggregate object sequentially without expressing its underlying representation z. Example: iterators of C++ STL containers ÕNote that you can have several iterator objects for a container and that the iterators are separate classes 17
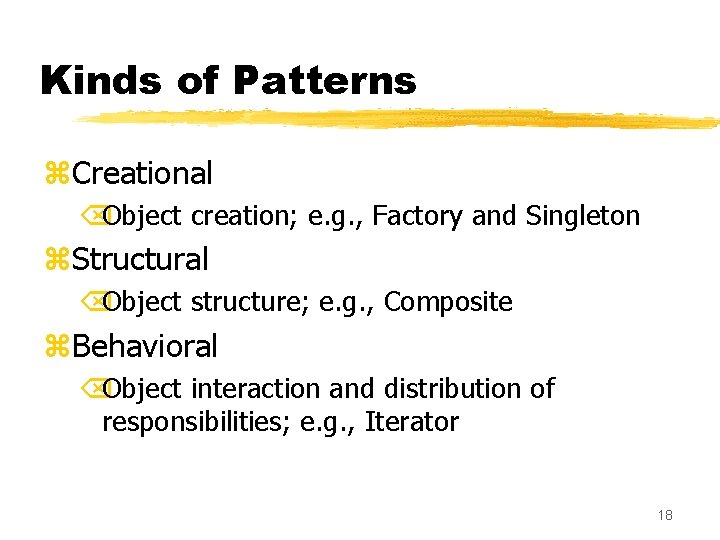
Kinds of Patterns z. Creational ÕObject creation; e. g. , Factory and Singleton z. Structural ÕObject structure; e. g. , Composite z. Behavioral ÕObject interaction and distribution of responsibilities; e. g. , Iterator 18
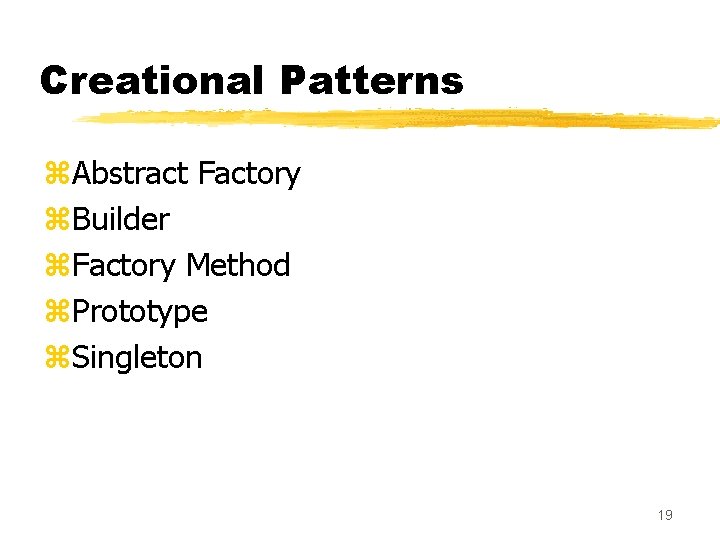
Creational Patterns z. Abstract Factory z. Builder z. Factory Method z. Prototype z. Singleton 19
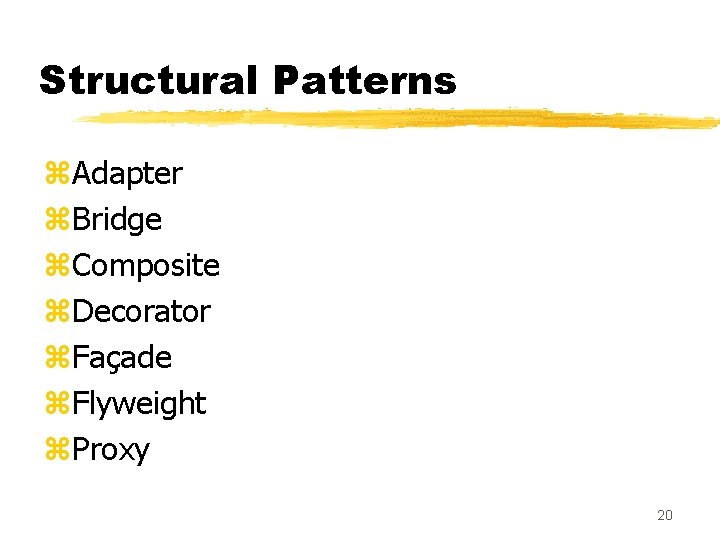
Structural Patterns z. Adapter z. Bridge z. Composite z. Decorator z. Façade z. Flyweight z. Proxy 20
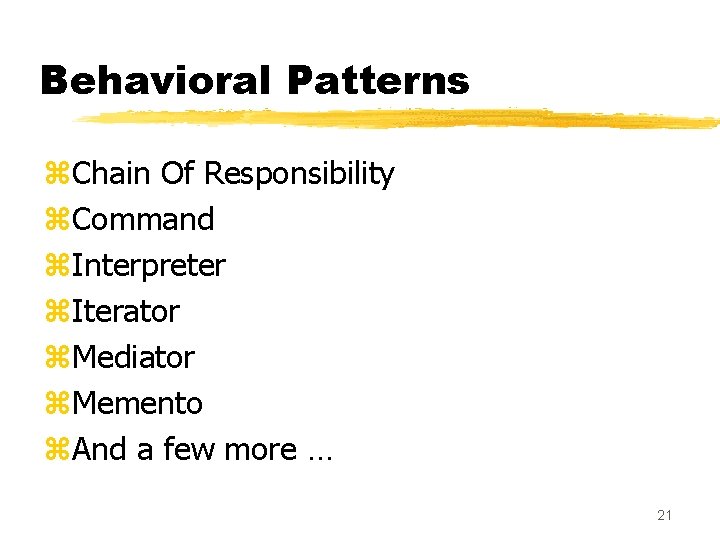
Behavioral Patterns z. Chain Of Responsibility z. Command z. Interpreter z. Iterator z. Mediator z. Memento z. And a few more … 21
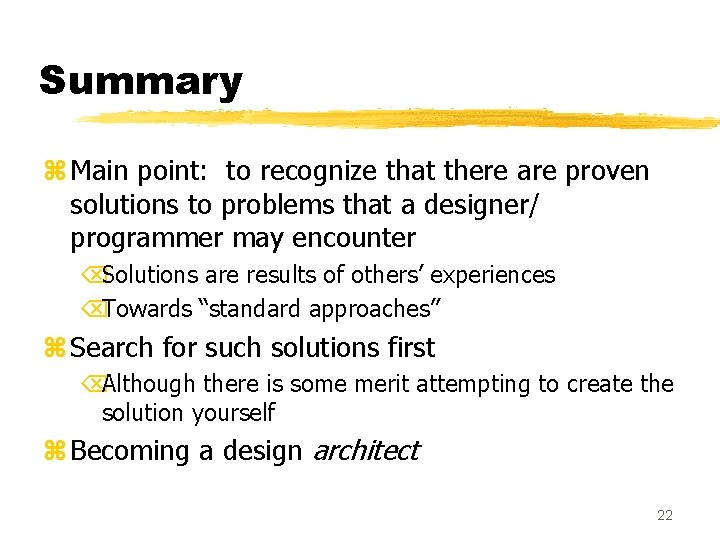
Summary z Main point: to recognize that there are proven solutions to problems that a designer/ programmer may encounter ÕSolutions are results of others’ experiences ÕTowards “standard approaches” z Search for such solutions first ÕAlthough there is some merit attempting to create the solution yourself z Becoming a design architect 22
123cs
Acf 231
Article 231 of the treaty of versailles
123 132 213 231 312 321
Phy 231 msu
231 del 2007
O primeiro natal hino
Gezang 231
Bbm 231
Transitor
Walfisch ikegami model
Draw 231 with base ten blocks
040 231 3666
Cs 231 n
Stanford 231n
Bbm 231
Pengertian berjiran
Germany territorial losses
Toastmasters timer sheet
What is potential gradient
Electric field formula
Cs 231 n
Cs 231 n