Class Relationships in C CS 123CS 231 1
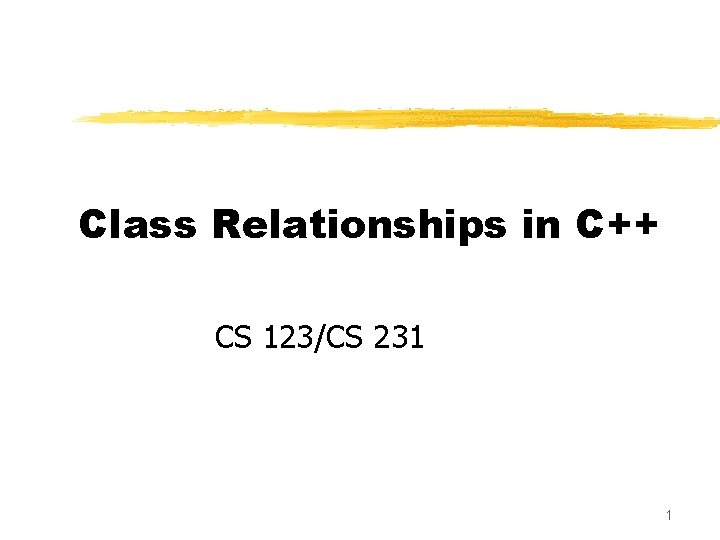
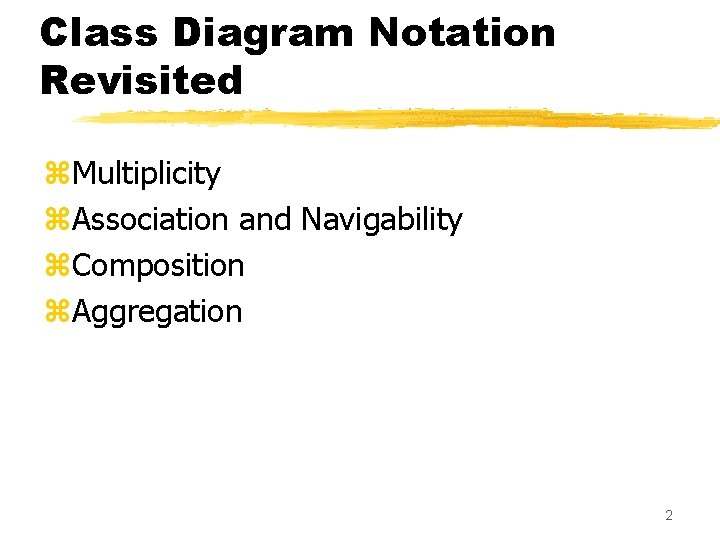
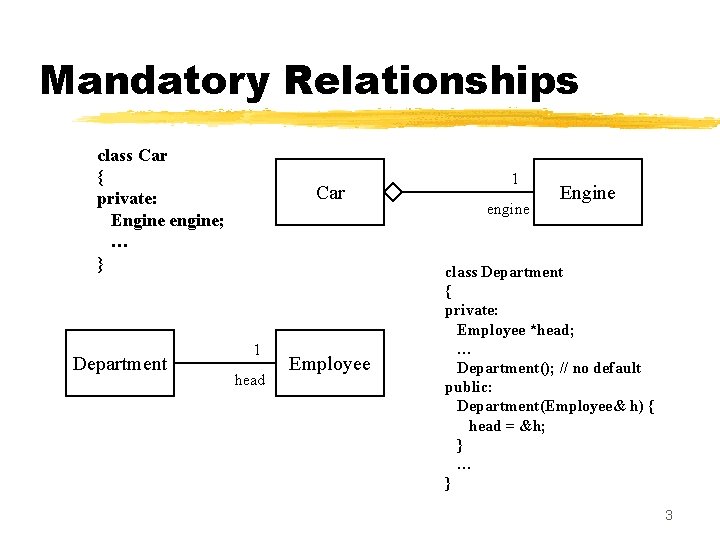
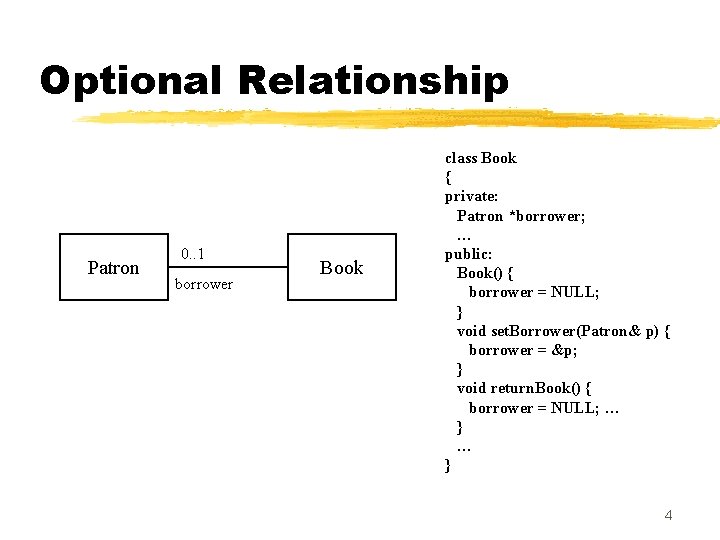
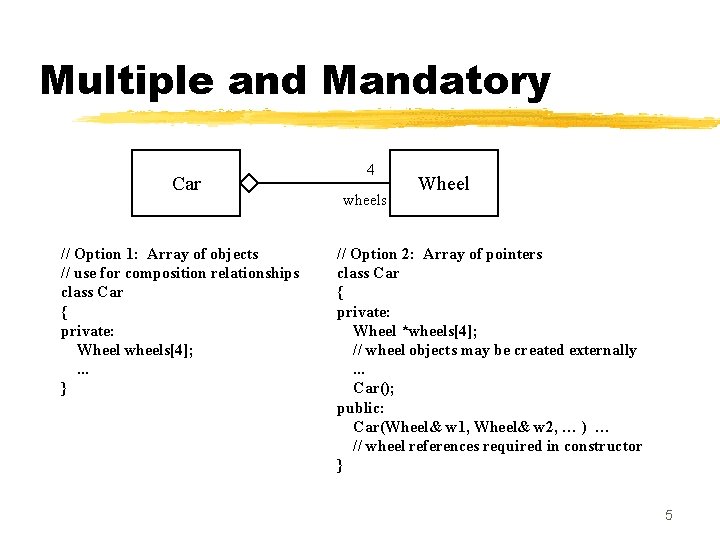
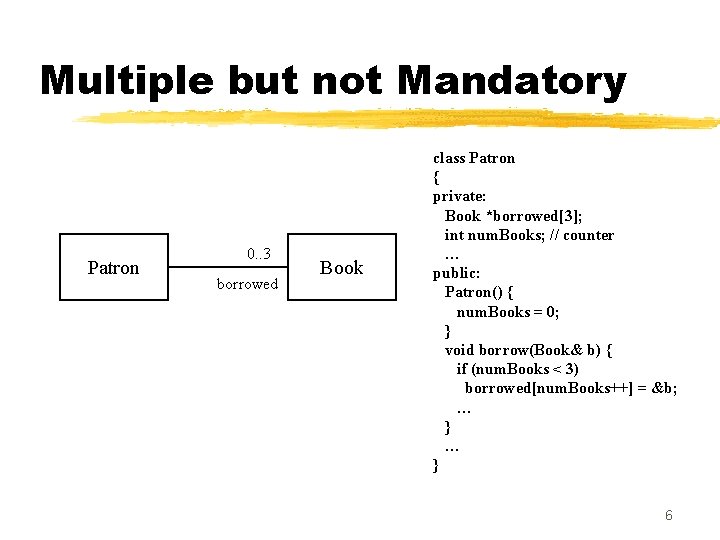
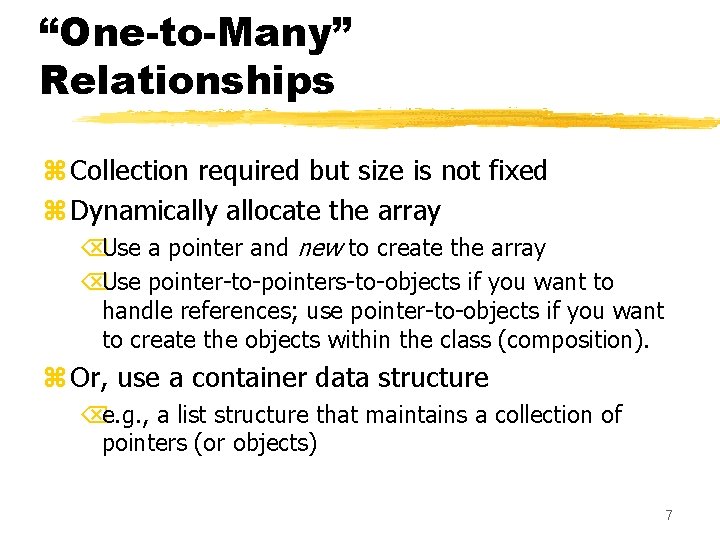
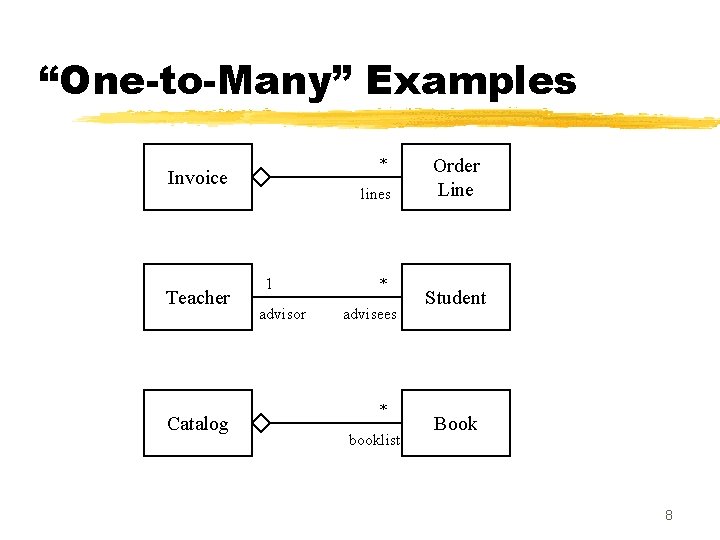
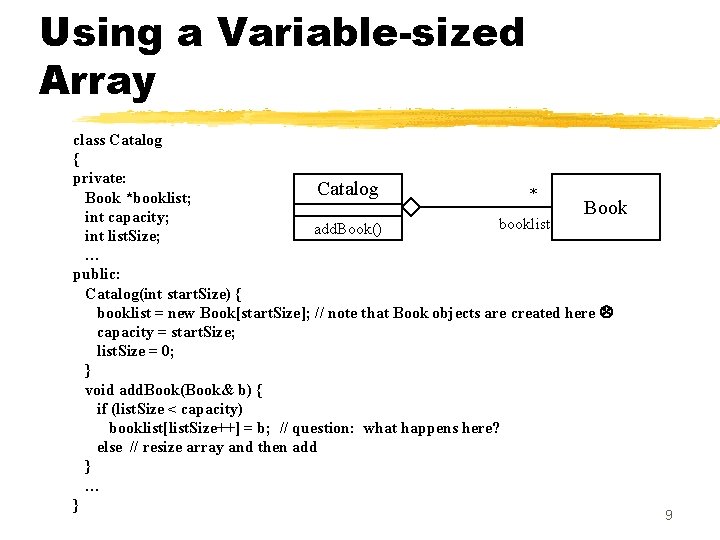
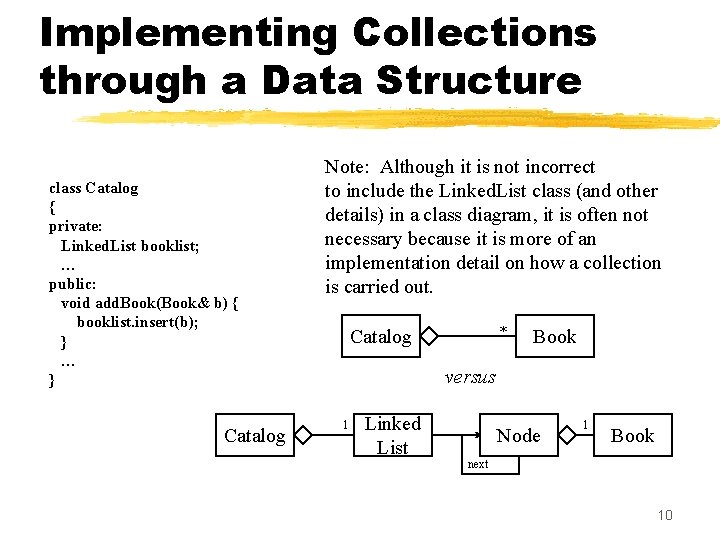
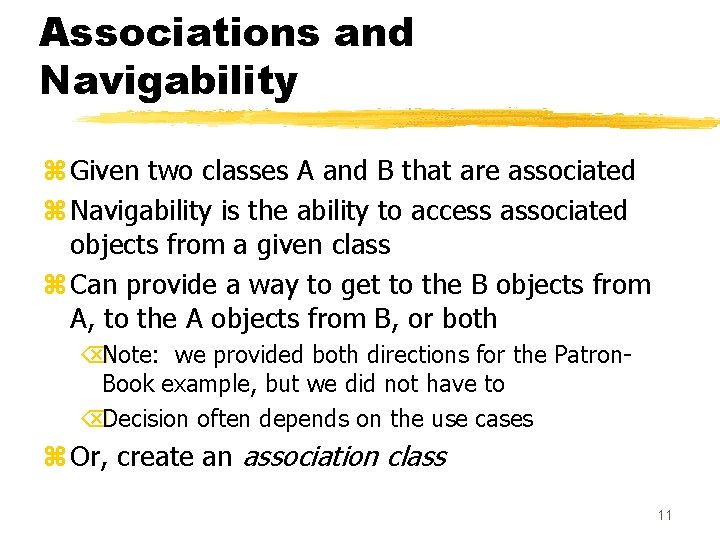
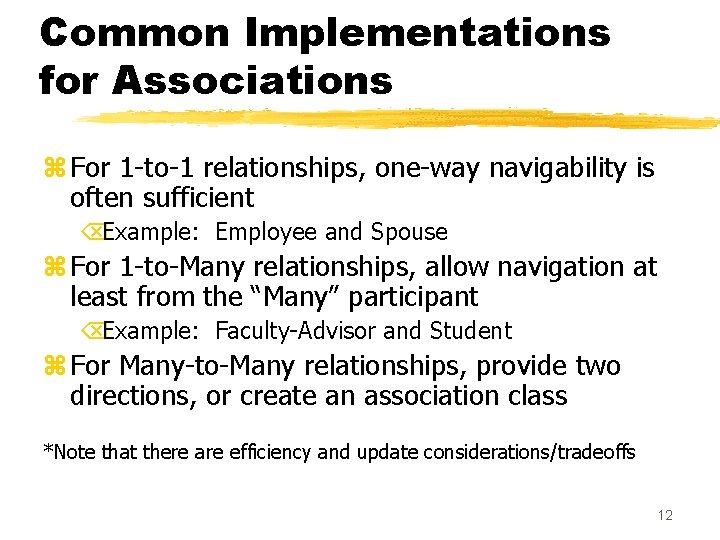
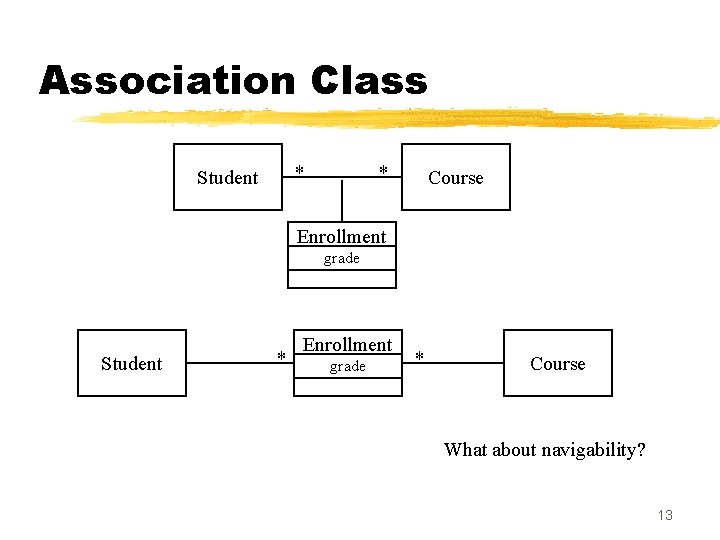
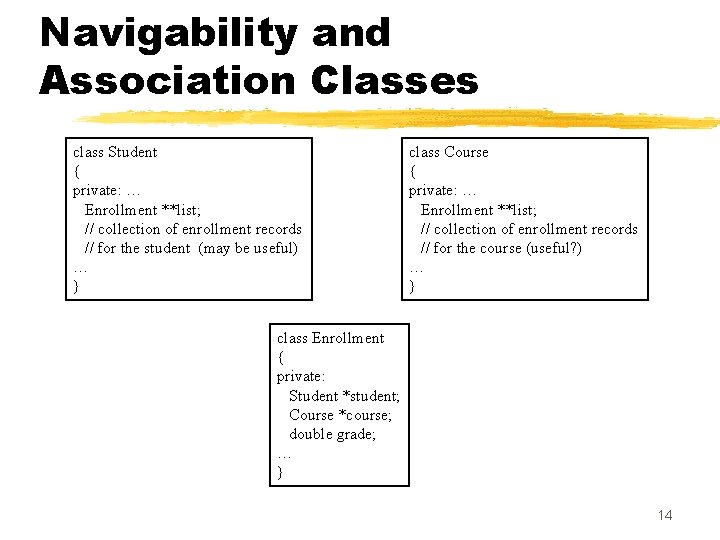
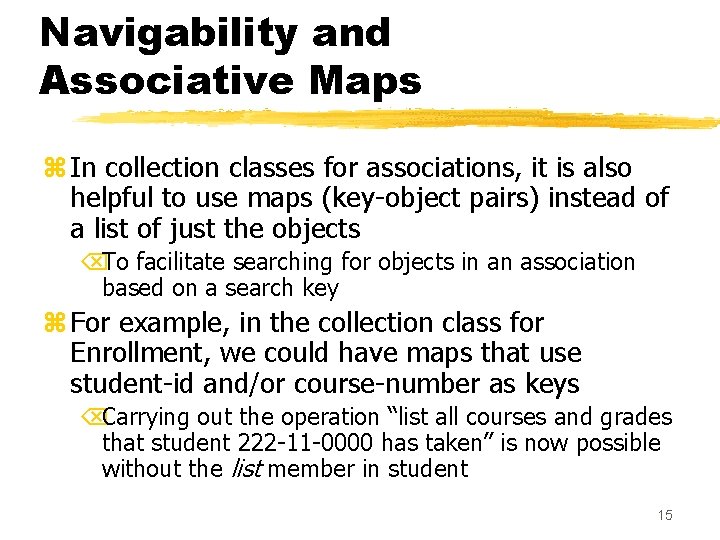
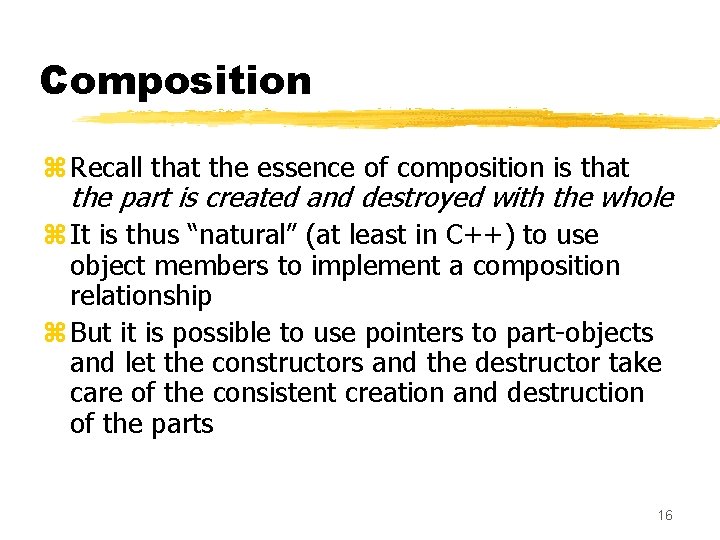
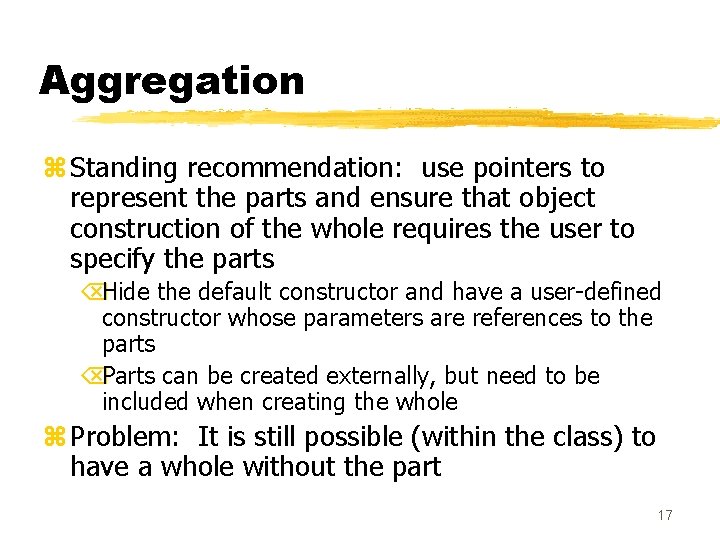
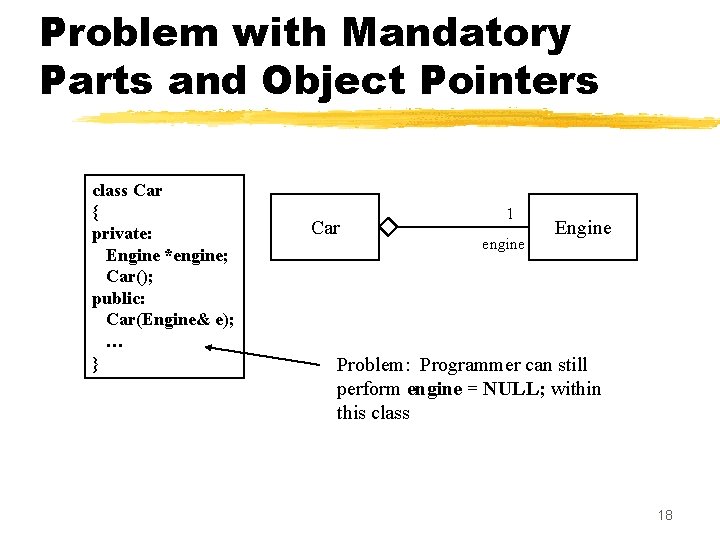
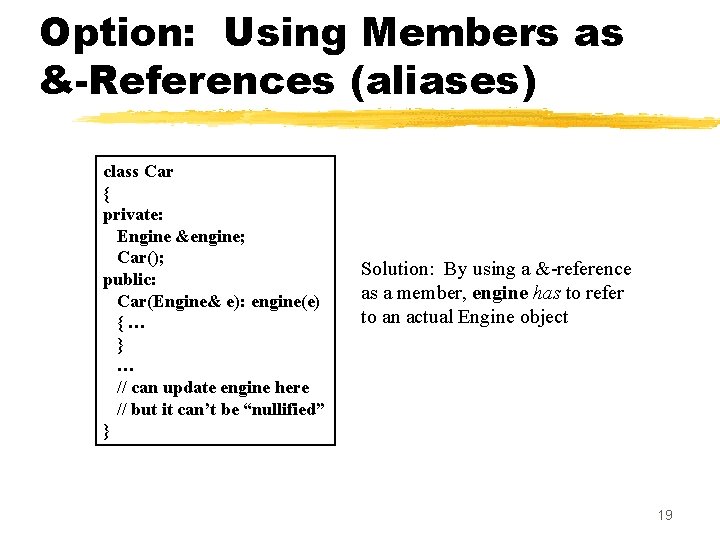
- Slides: 19
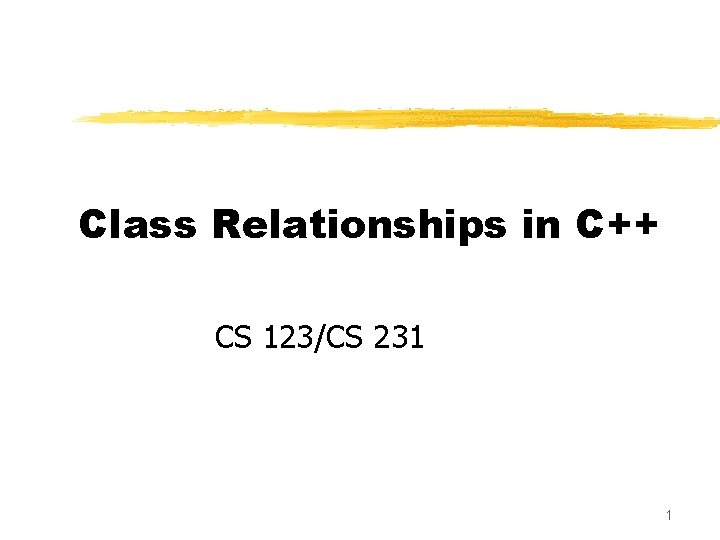
Class Relationships in C++ CS 123/CS 231 1
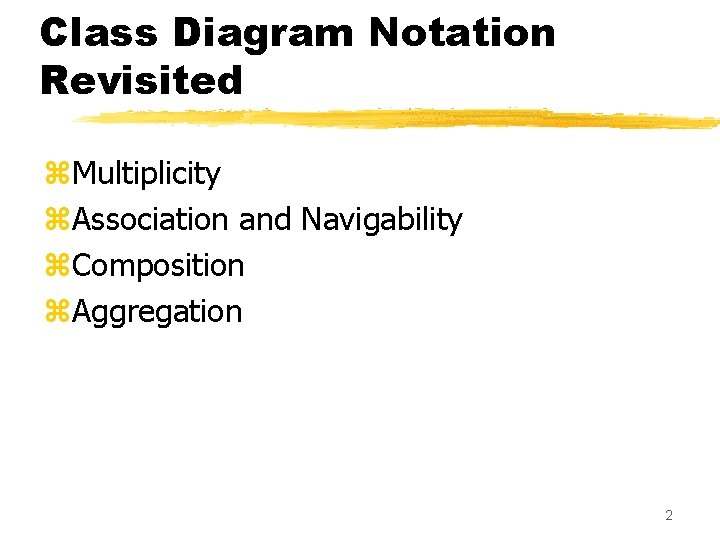
Class Diagram Notation Revisited z. Multiplicity z. Association and Navigability z. Composition z. Aggregation 2
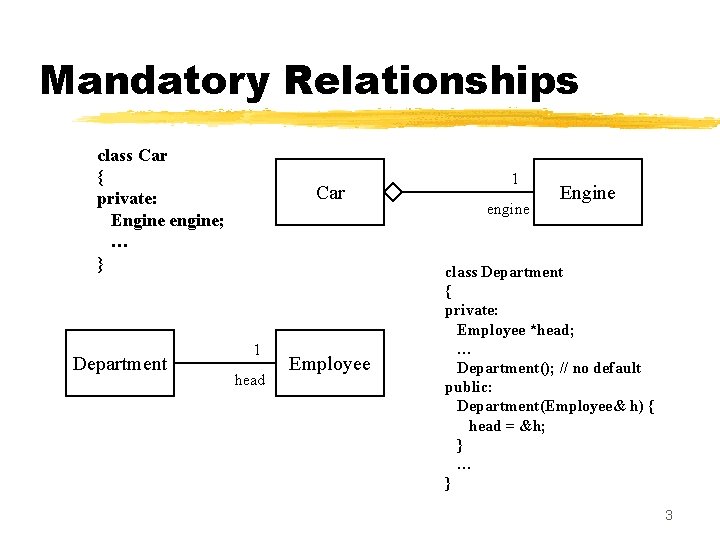
Mandatory Relationships class Car { private: Engine engine; … } Department Car 1 head Employee 1 engine Engine class Department { private: Employee *head; … Department(); // no default public: Department(Employee& h) { head = &h; } … } 3
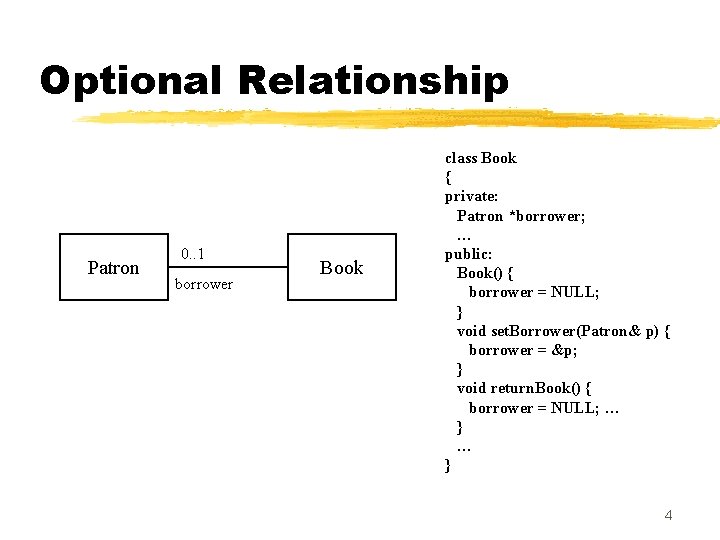
Optional Relationship Patron 0. . 1 borrower Book class Book { private: Patron *borrower; … public: Book() { borrower = NULL; } void set. Borrower(Patron& p) { borrower = &p; } void return. Book() { borrower = NULL; … } 4
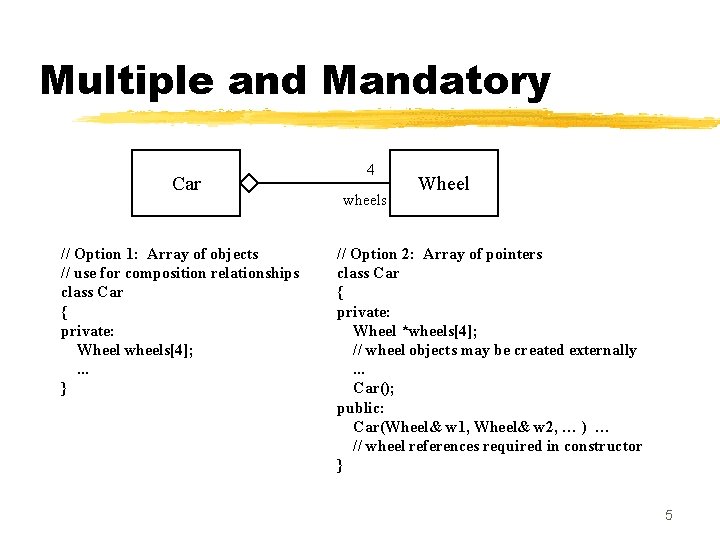
Multiple and Mandatory Car // Option 1: Array of objects // use for composition relationships class Car { private: Wheel wheels[4]; . . . } 4 wheels Wheel // Option 2: Array of pointers class Car { private: Wheel *wheels[4]; // wheel objects may be created externally. . . Car(); public: Car(Wheel& w 1, Wheel& w 2, … ) … // wheel references required in constructor } 5
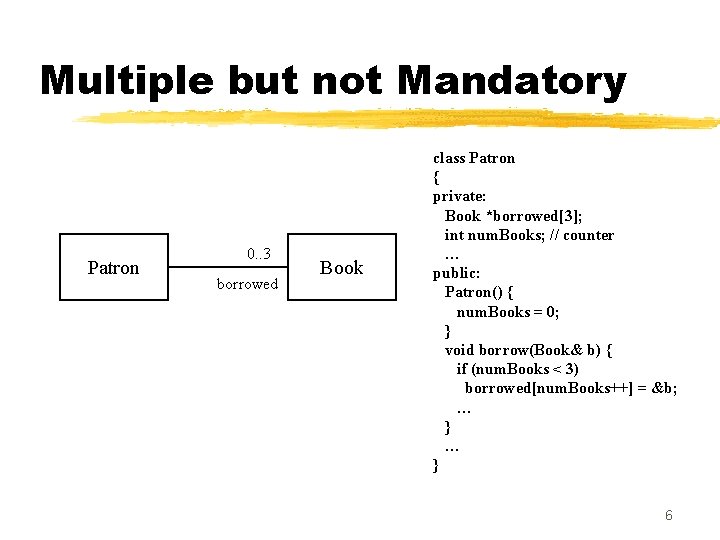
Multiple but not Mandatory Patron 0. . 3 borrowed Book class Patron { private: Book *borrowed[3]; int num. Books; // counter … public: Patron() { num. Books = 0; } void borrow(Book& b) { if (num. Books < 3) borrowed[num. Books++] = &b; … } 6
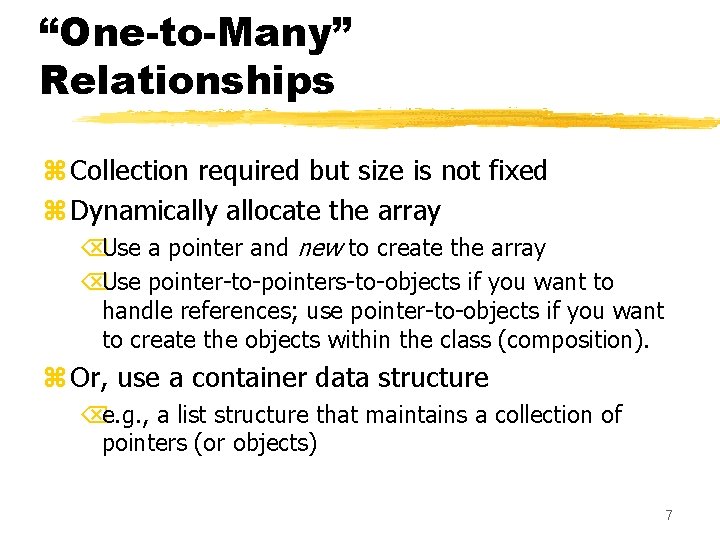
“One-to-Many” Relationships z Collection required but size is not fixed z Dynamically allocate the array ÕUse a pointer and new to create the array ÕUse pointer-to-pointers-to-objects if you want to handle references; use pointer-to-objects if you want to create the objects within the class (composition). z Or, use a container data structure Õe. g. , a list structure that maintains a collection of pointers (or objects) 7
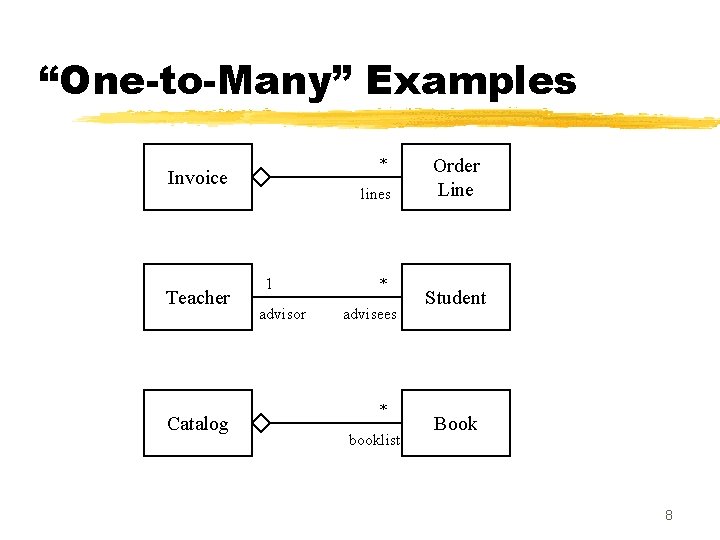
“One-to-Many” Examples * Invoice Teacher Catalog lines 1 advisor * advisees * booklist Order Line Student Book 8
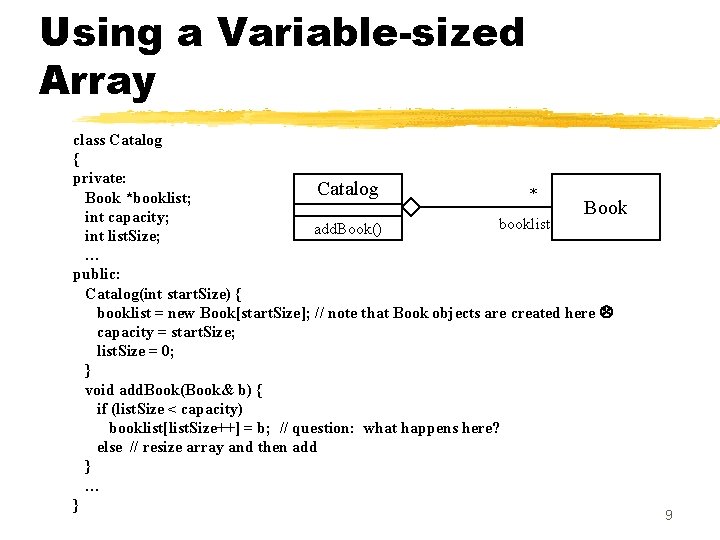
Using a Variable-sized Array class Catalog { private: Catalog * Book *booklist; Book int capacity; booklist add. Book() int list. Size; … public: Catalog(int start. Size) { booklist = new Book[start. Size]; // note that Book objects are created here capacity = start. Size; list. Size = 0; } void add. Book(Book& b) { if (list. Size < capacity) booklist[list. Size++] = b; // question: what happens here? else // resize array and then add } … } 9
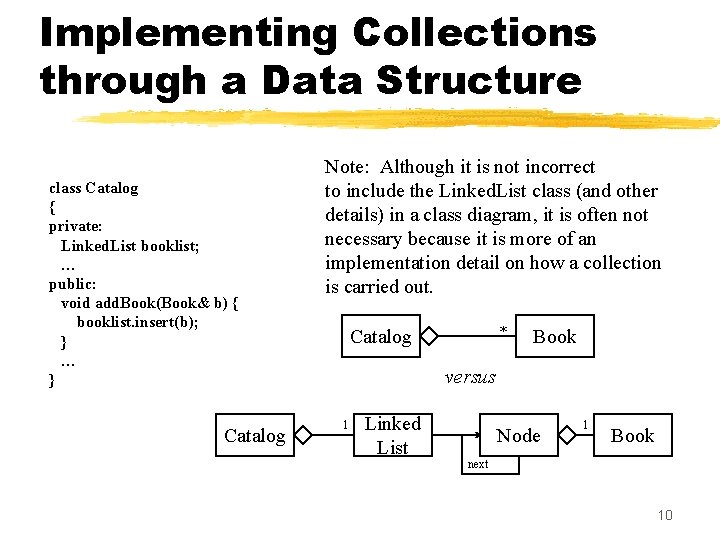
Implementing Collections through a Data Structure class Catalog { private: Linked. List booklist; … public: void add. Book(Book& b) { booklist. insert(b); } … } Catalog Note: Although it is not incorrect to include the Linked. List class (and other details) in a class diagram, it is often not necessary because it is more of an implementation detail on how a collection is carried out. * Catalog Book versus 1 Linked List Node 1 Book next 10
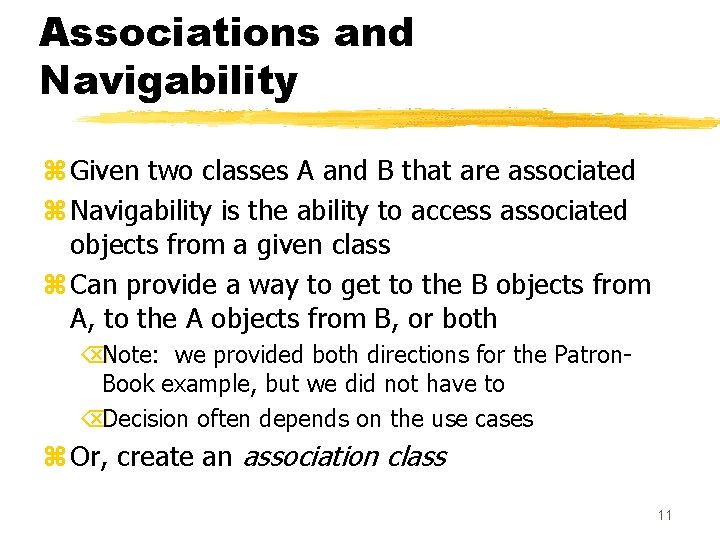
Associations and Navigability z Given two classes A and B that are associated z Navigability is the ability to access associated objects from a given class z Can provide a way to get to the B objects from A, to the A objects from B, or both ÕNote: we provided both directions for the Patron. Book example, but we did not have to ÕDecision often depends on the use cases z Or, create an association class 11
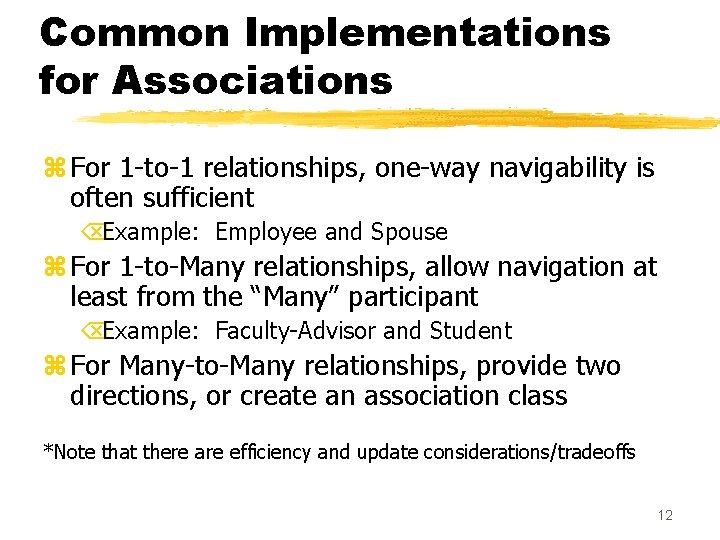
Common Implementations for Associations z For 1 -to-1 relationships, one-way navigability is often sufficient ÕExample: Employee and Spouse z For 1 -to-Many relationships, allow navigation at least from the “Many” participant ÕExample: Faculty-Advisor and Student z For Many-to-Many relationships, provide two directions, or create an association class *Note that there are efficiency and update considerations/tradeoffs 12
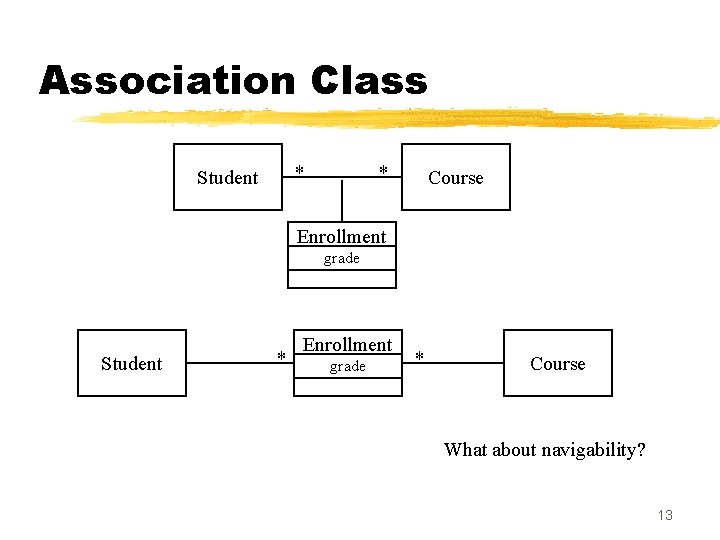
Association Class * Student * Course Enrollment grade Student * Enrollment grade * Course What about navigability? 13
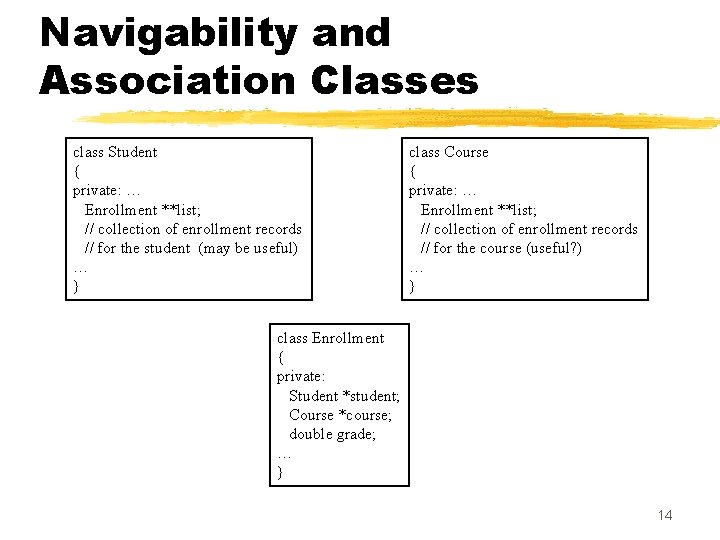
Navigability and Association Classes class Student { private: … Enrollment **list; // collection of enrollment records // for the student (may be useful) … } class Course { private: … Enrollment **list; // collection of enrollment records // for the course (useful? ) … } class Enrollment { private: Student *student; Course *course; double grade; … } 14
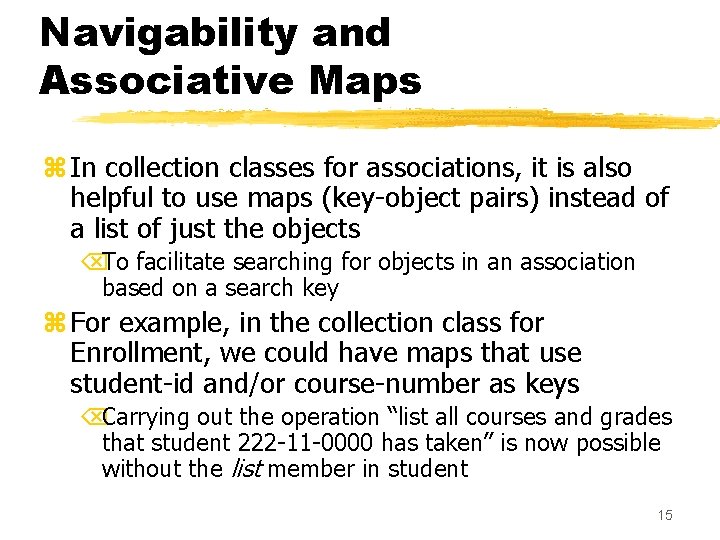
Navigability and Associative Maps z In collection classes for associations, it is also helpful to use maps (key-object pairs) instead of a list of just the objects ÕTo facilitate searching for objects in an association based on a search key z For example, in the collection class for Enrollment, we could have maps that use student-id and/or course-number as keys ÕCarrying out the operation “list all courses and grades that student 222 -11 -0000 has taken” is now possible without the list member in student 15
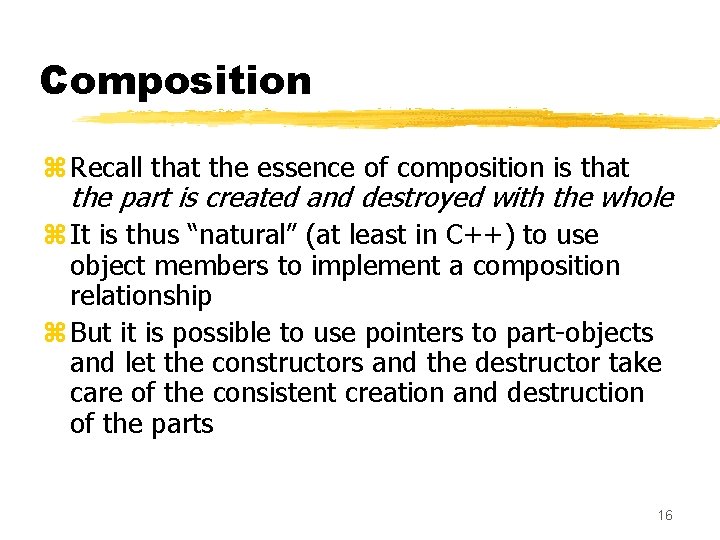
Composition z Recall that the essence of composition is that the part is created and destroyed with the whole z It is thus “natural” (at least in C++) to use object members to implement a composition relationship z But it is possible to use pointers to part-objects and let the constructors and the destructor take care of the consistent creation and destruction of the parts 16
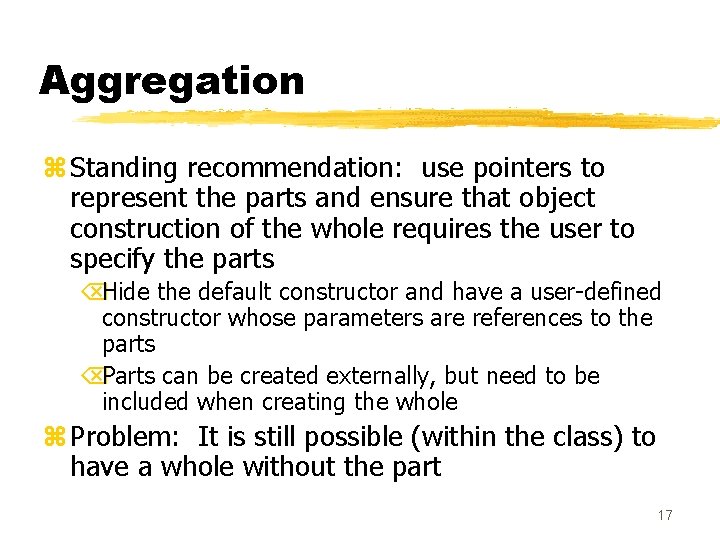
Aggregation z Standing recommendation: use pointers to represent the parts and ensure that object construction of the whole requires the user to specify the parts ÕHide the default constructor and have a user-defined constructor whose parameters are references to the parts ÕParts can be created externally, but need to be included when creating the whole z Problem: It is still possible (within the class) to have a whole without the part 17
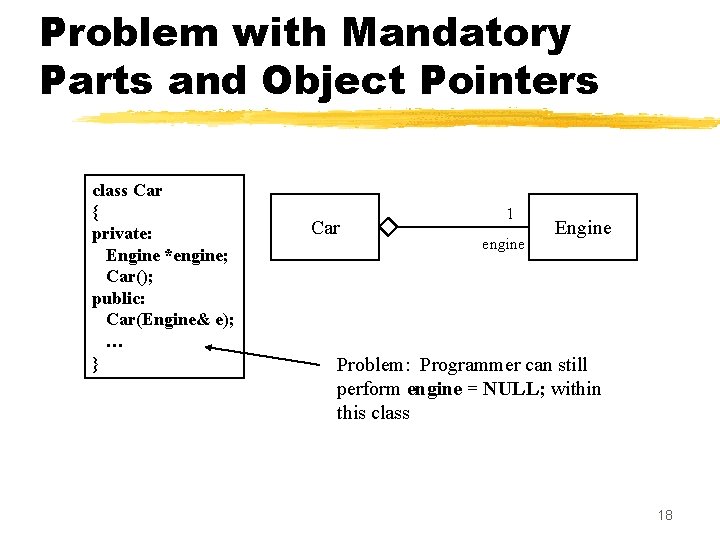
Problem with Mandatory Parts and Object Pointers class Car { private: Engine *engine; Car(); public: Car(Engine& e); … } Car 1 engine Engine Problem: Programmer can still perform engine = NULL; within this class 18
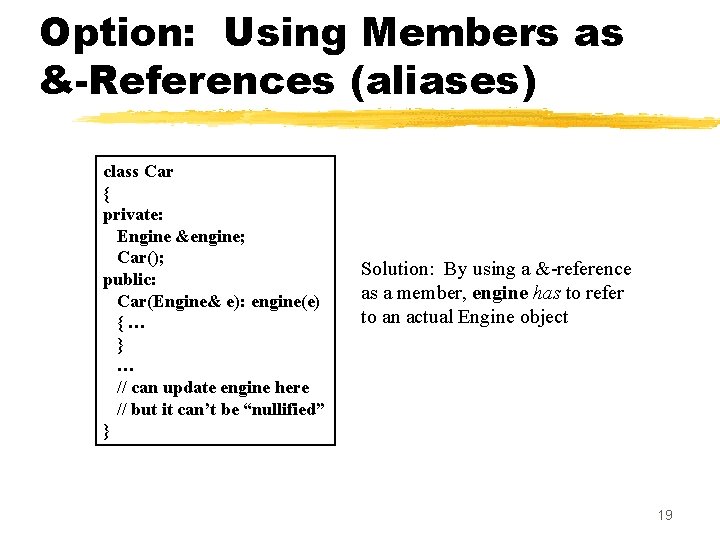
Option: Using Members as &-References (aliases) class Car { private: Engine &engine; Car(); public: Car(Engine& e): engine(e) {… } … // can update engine here // but it can’t be “nullified” } Solution: By using a &-reference as a member, engine has to refer to an actual Engine object 19
Java 231
Acf 231
Article 231 of the treaty of versailles
123+132+321+312
Phy 231 msu
D.lgs. 231/2007
Eis que um anjo proclamou o primeiro natal
Gezang 231
Counters
Cs 231
Walfisch ikegami model
Draw 231 with base ten blocks
040 231 3666
Mvcnn pytorch
Stanford cs231
Bbm 231
Al quran muka surat 226
German territorial losses
Toastmasters pledge
Potential gradient