OOP in Java and C CS 123CS 231
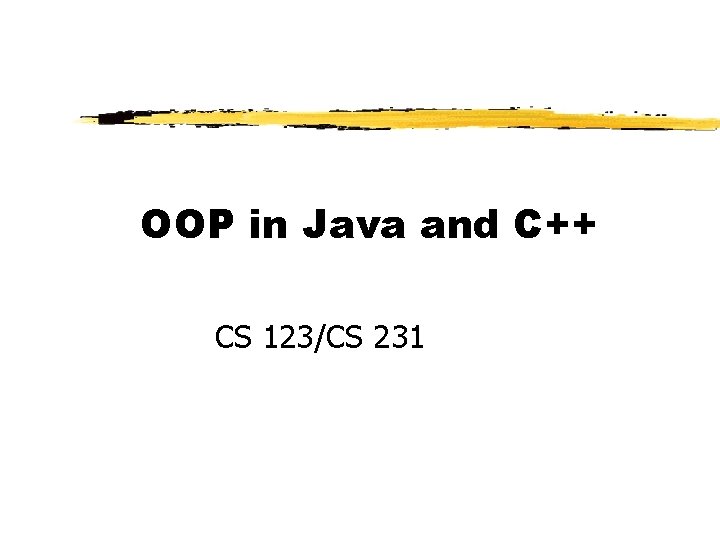
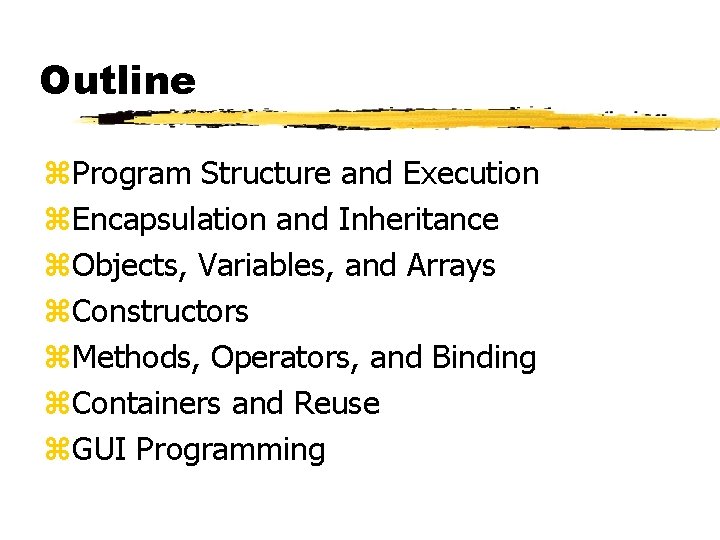
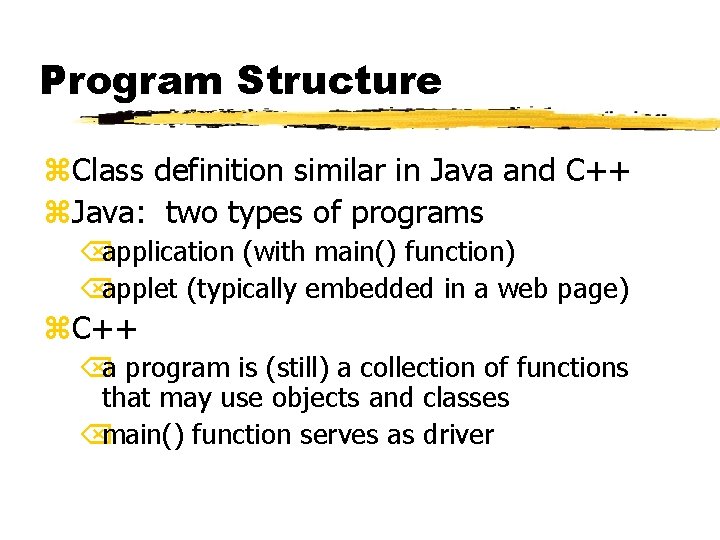
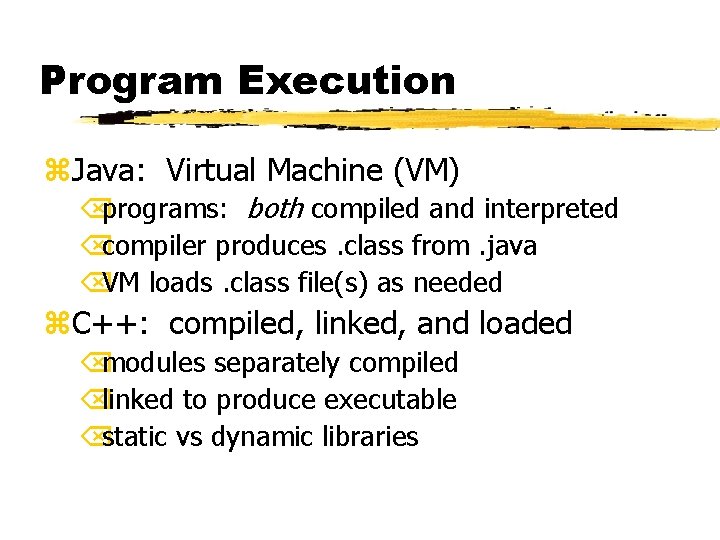
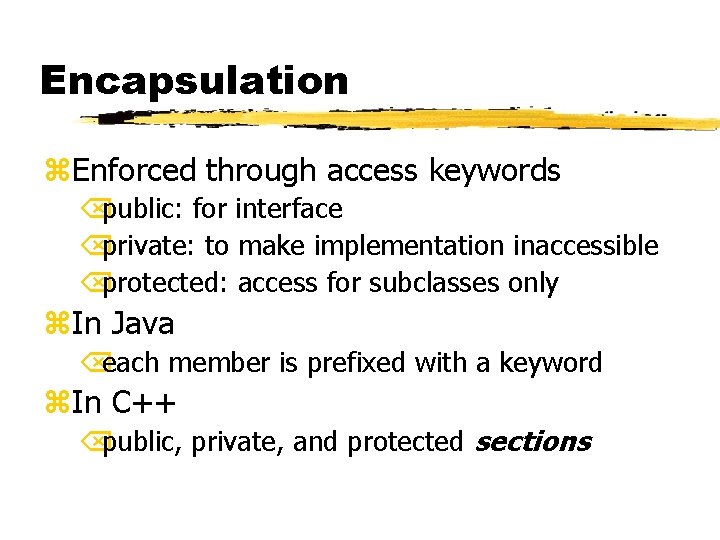
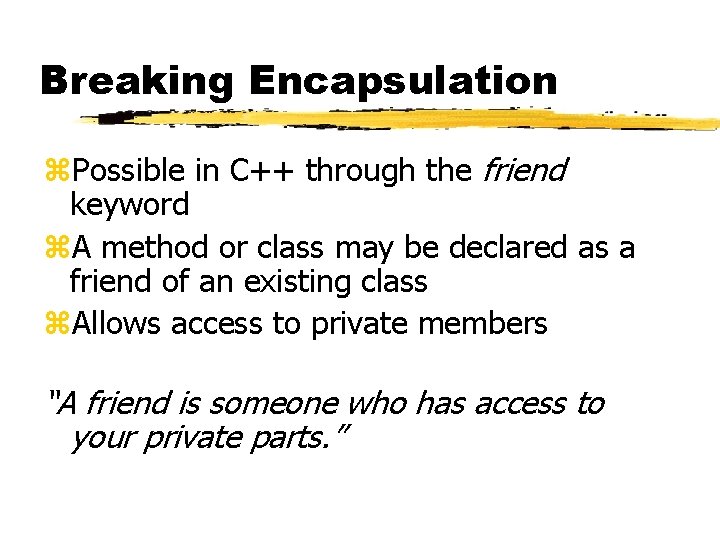
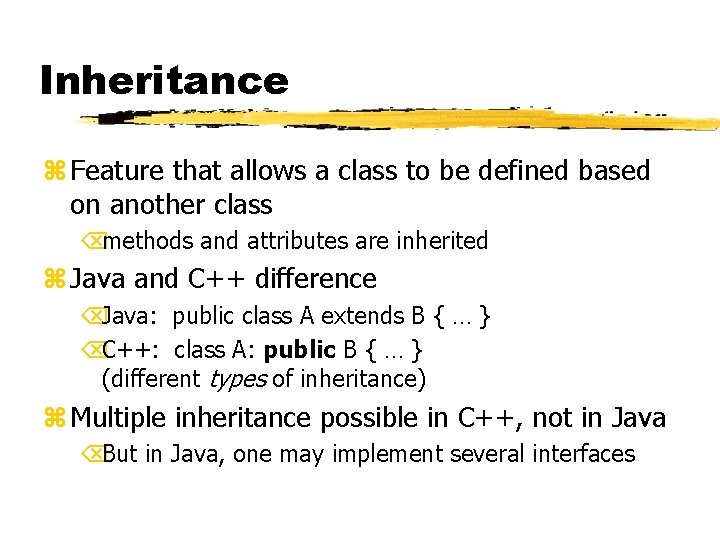
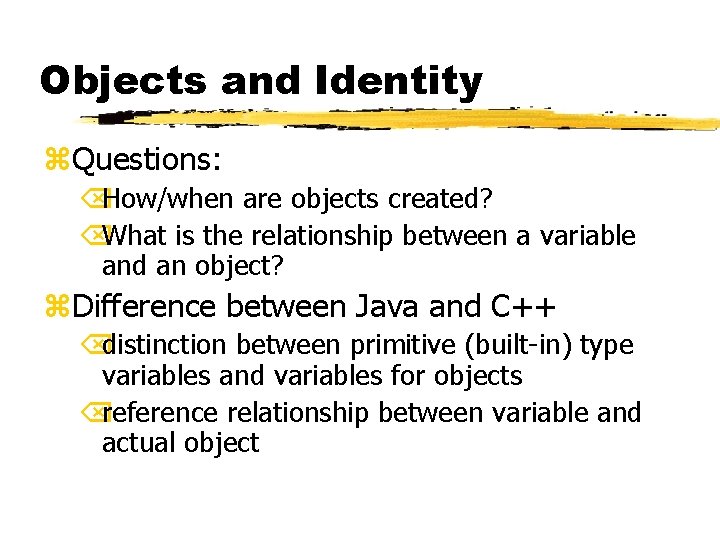
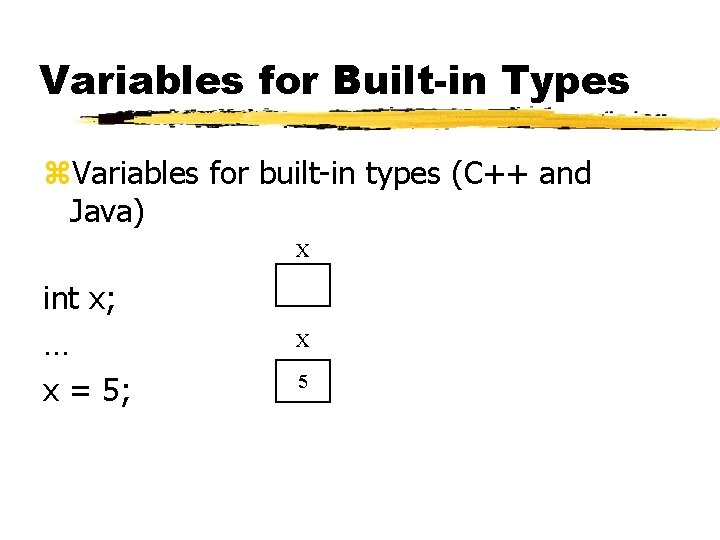
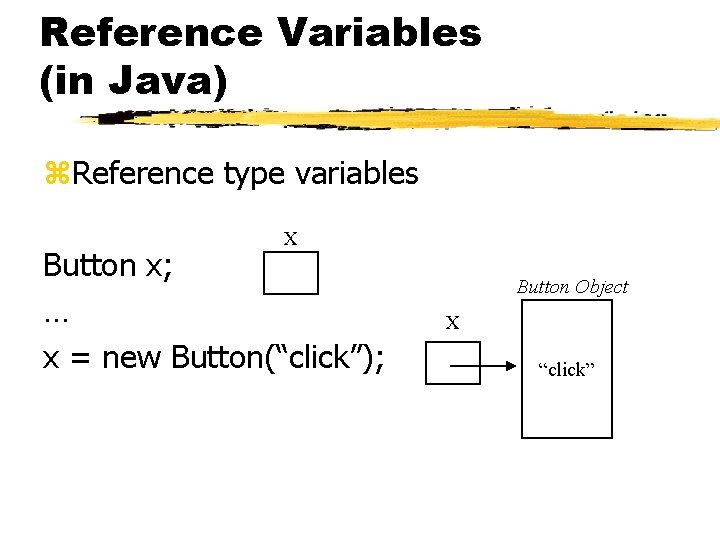
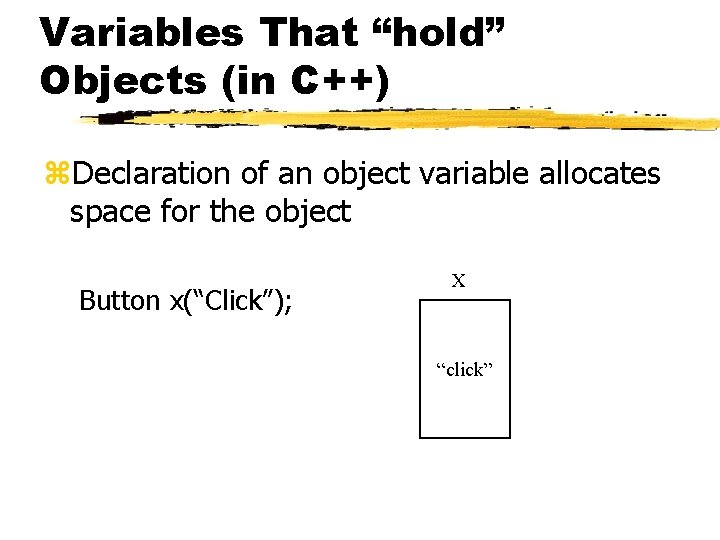
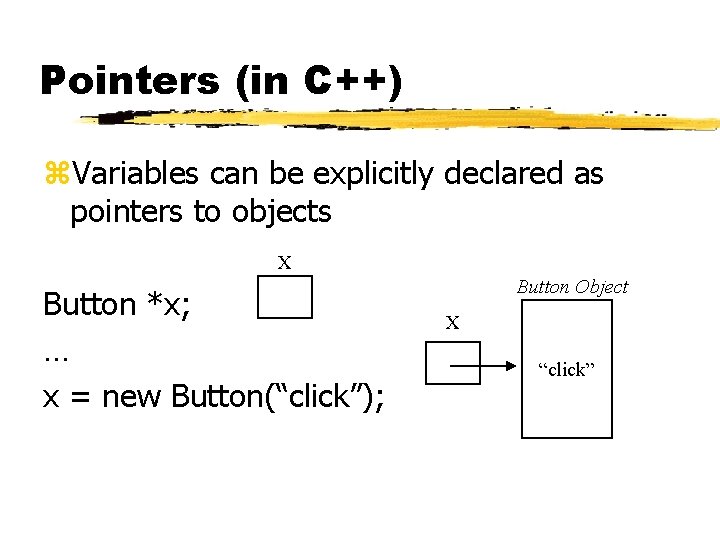
![Arrays zint x[20]; Button b[20]; ÕValid declarations in C++, not in Java ÕCreates 20 Arrays zint x[20]; Button b[20]; ÕValid declarations in C++, not in Java ÕCreates 20](https://slidetodoc.com/presentation_image/30be384f759c94ece1d07ea7d4820e43/image-13.jpg)
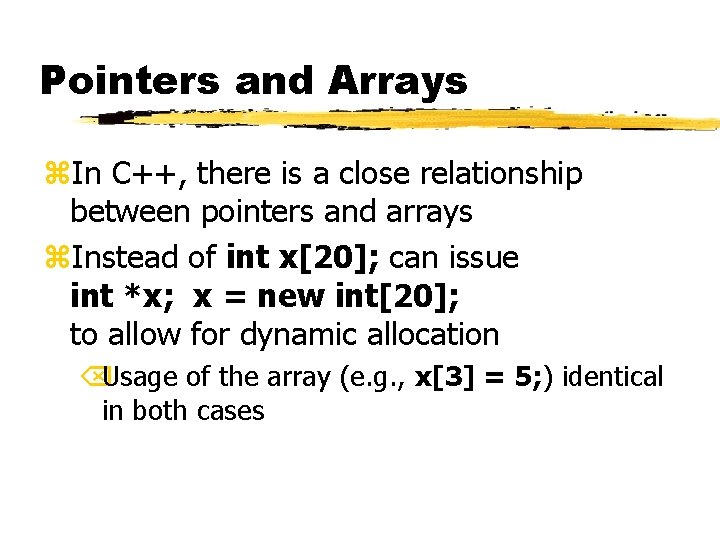
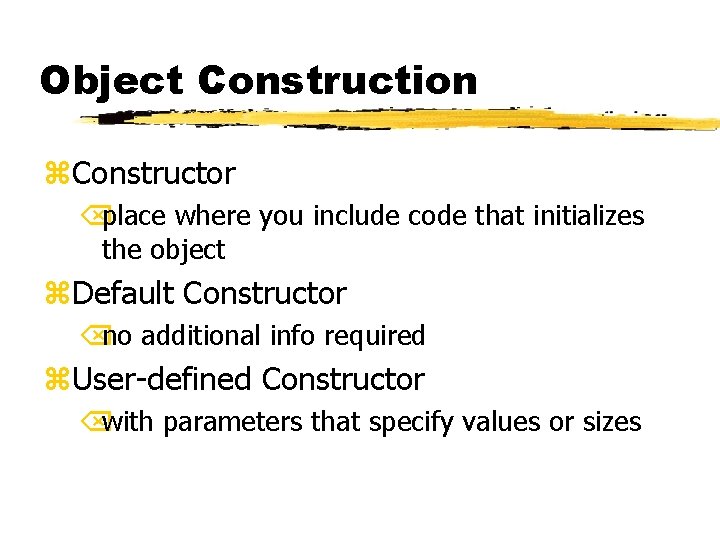
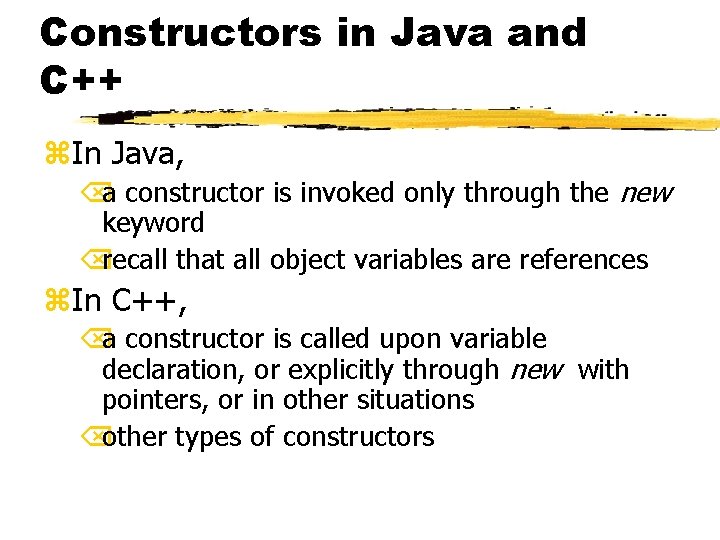
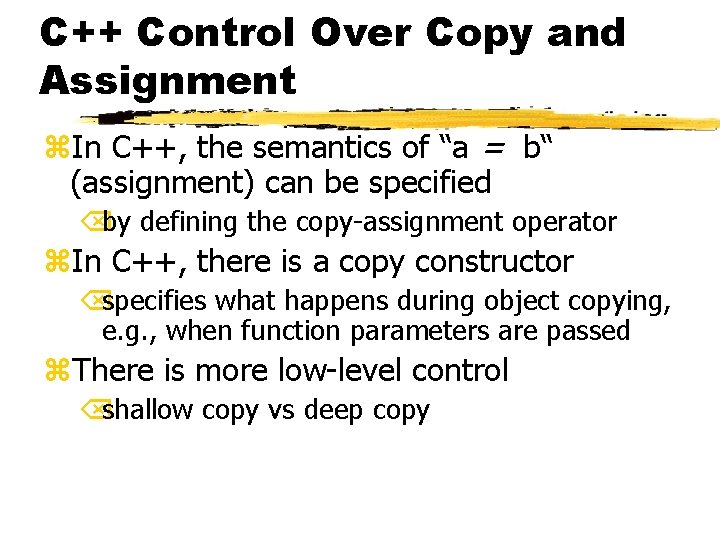
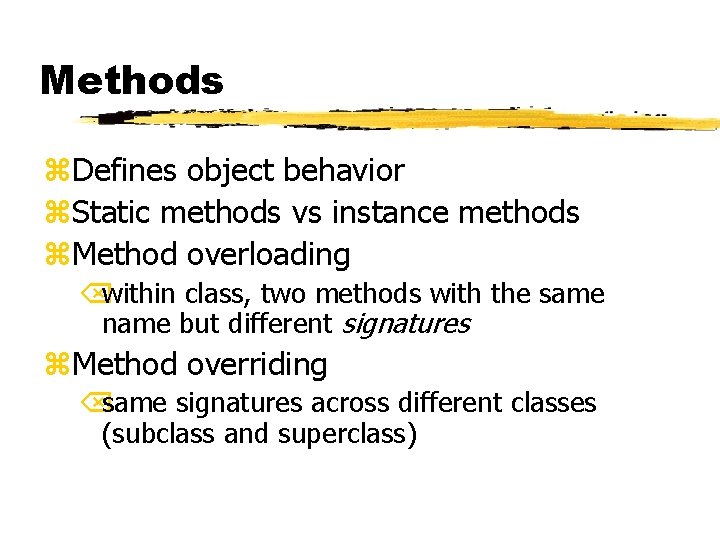
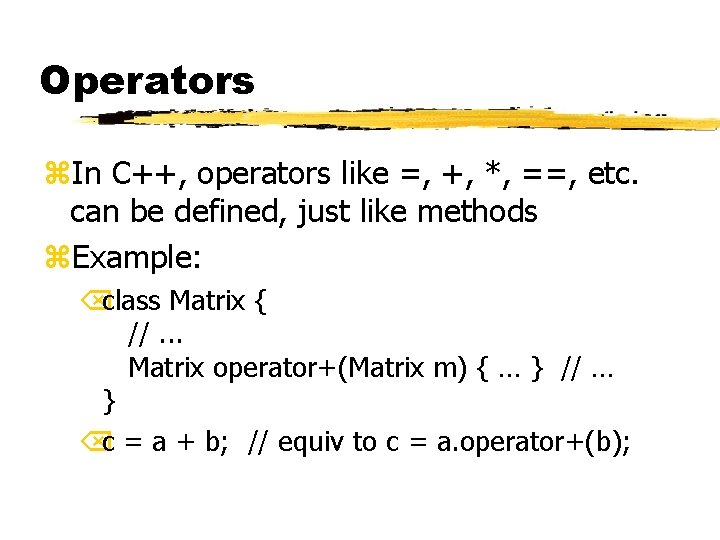
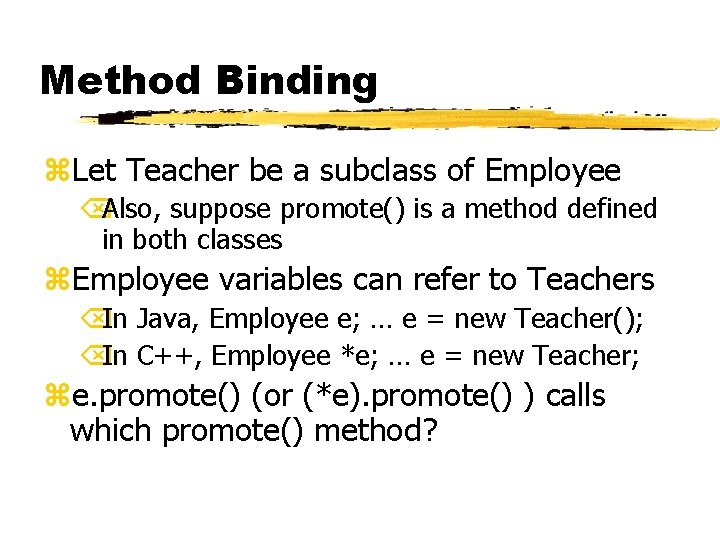
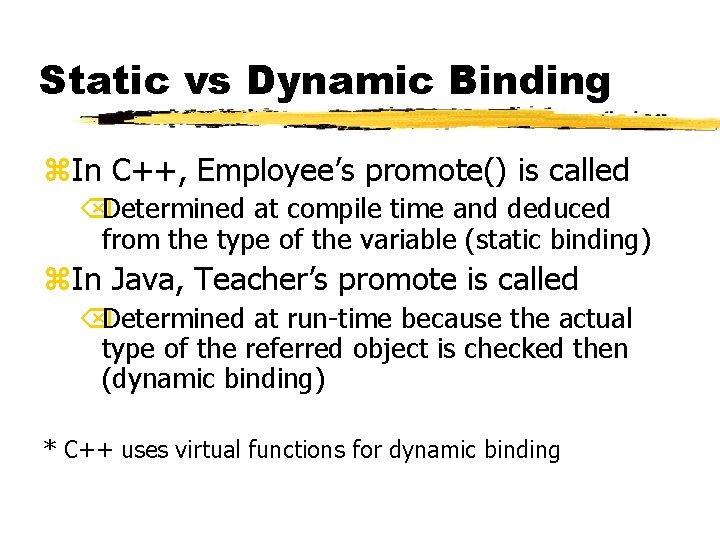
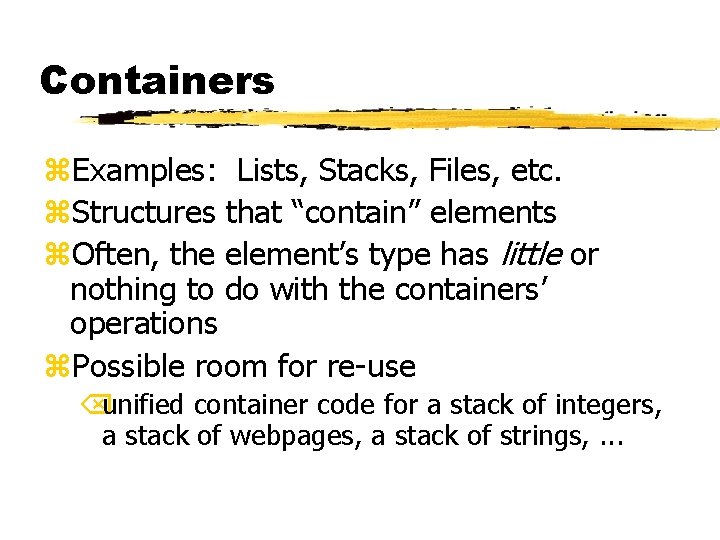
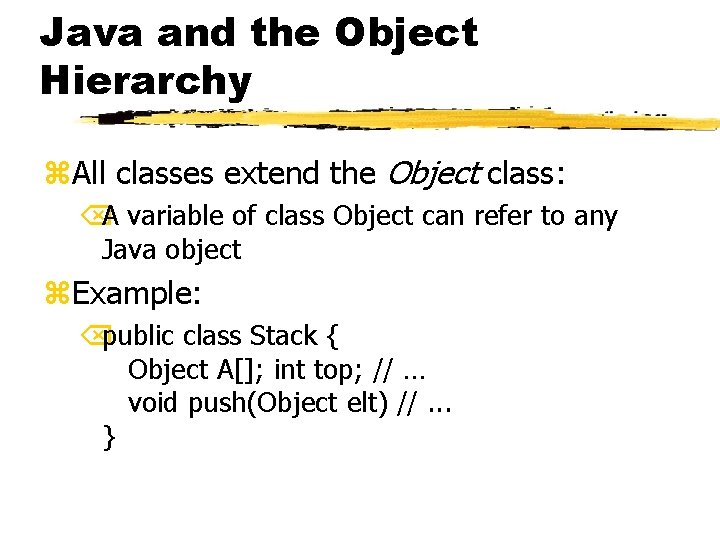
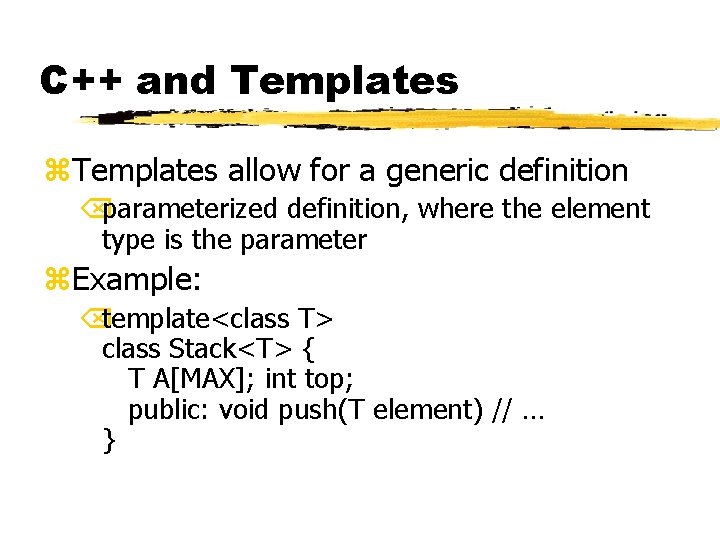
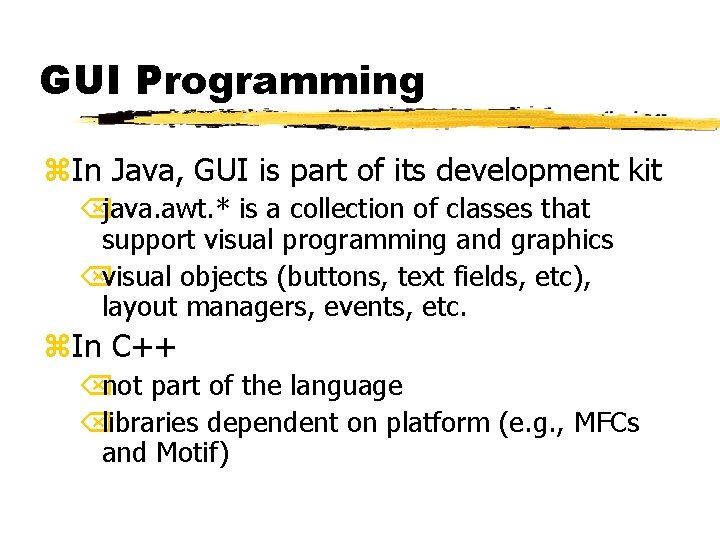
- Slides: 25
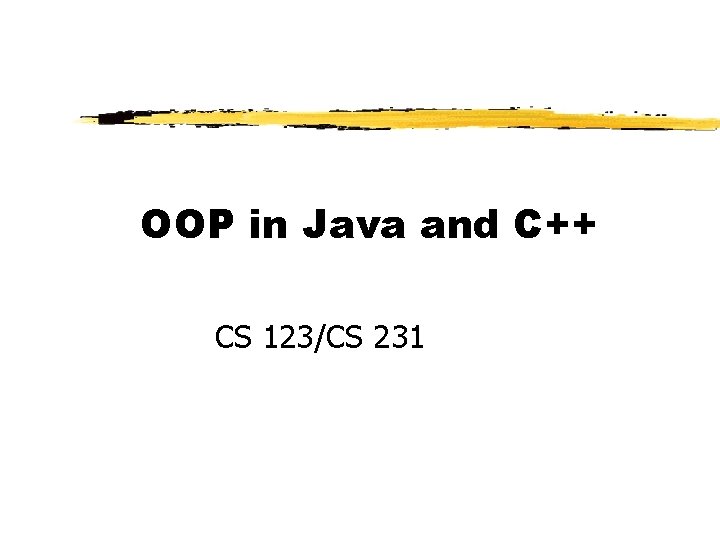
OOP in Java and C++ CS 123/CS 231
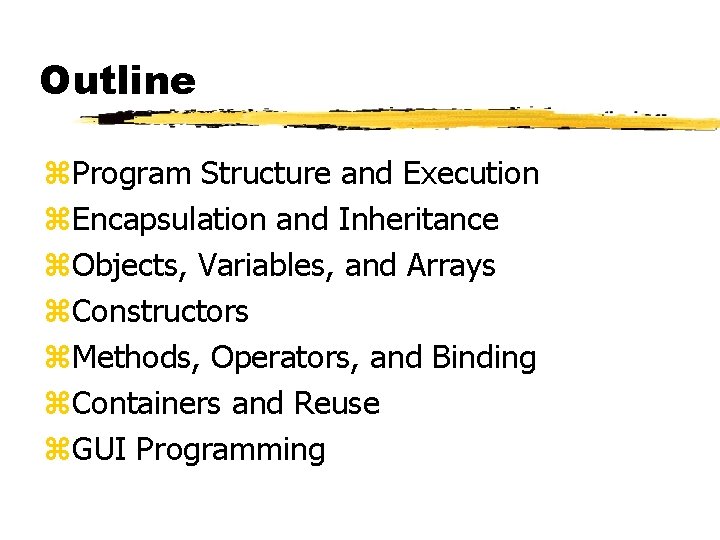
Outline z. Program Structure and Execution z. Encapsulation and Inheritance z. Objects, Variables, and Arrays z. Constructors z. Methods, Operators, and Binding z. Containers and Reuse z. GUI Programming
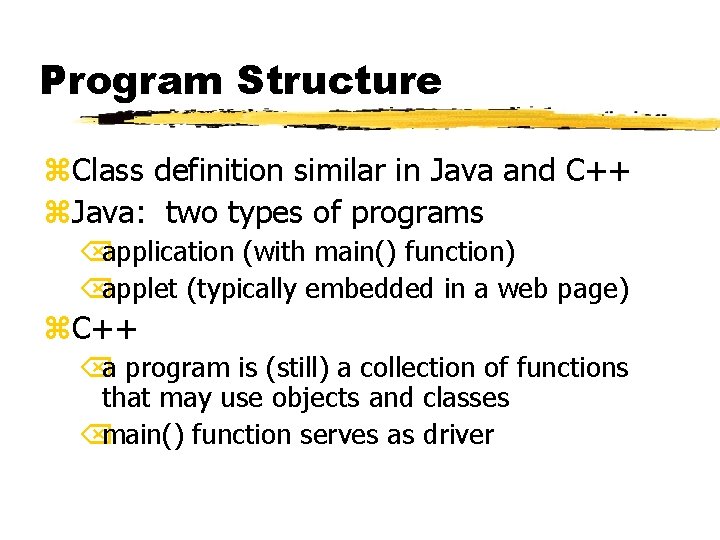
Program Structure z. Class definition similar in Java and C++ z. Java: two types of programs Õapplication (with main() function) Õapplet (typically embedded in a web page) z. C++ Õa program is (still) a collection of functions that may use objects and classes Õmain() function serves as driver
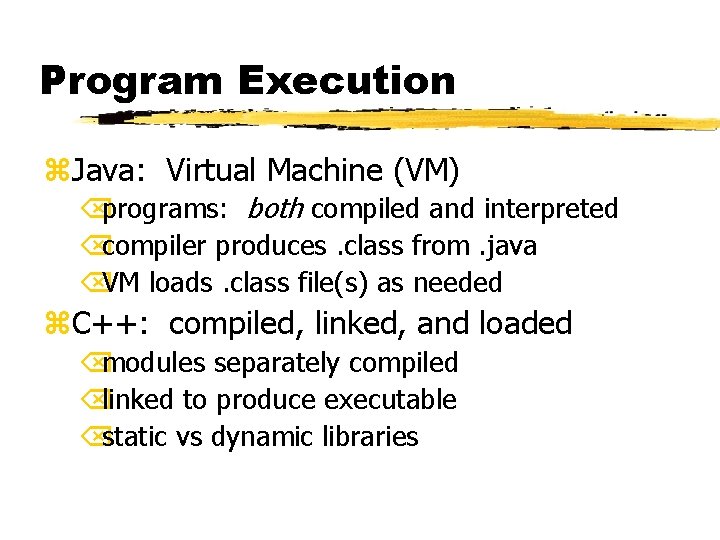
Program Execution z. Java: Virtual Machine (VM) Õprograms: both compiled and interpreted Õcompiler produces. class from. java ÕVM loads. class file(s) as needed z. C++: compiled, linked, and loaded Õmodules separately compiled Õlinked to produce executable Õstatic vs dynamic libraries
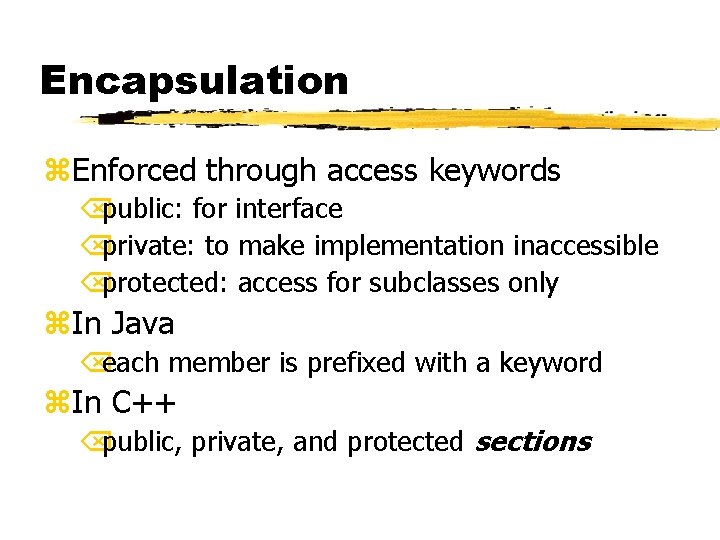
Encapsulation z. Enforced through access keywords Õpublic: for interface Õprivate: to make implementation inaccessible Õprotected: access for subclasses only z. In Java Õeach member is prefixed with a keyword z. In C++ Õpublic, private, and protected sections
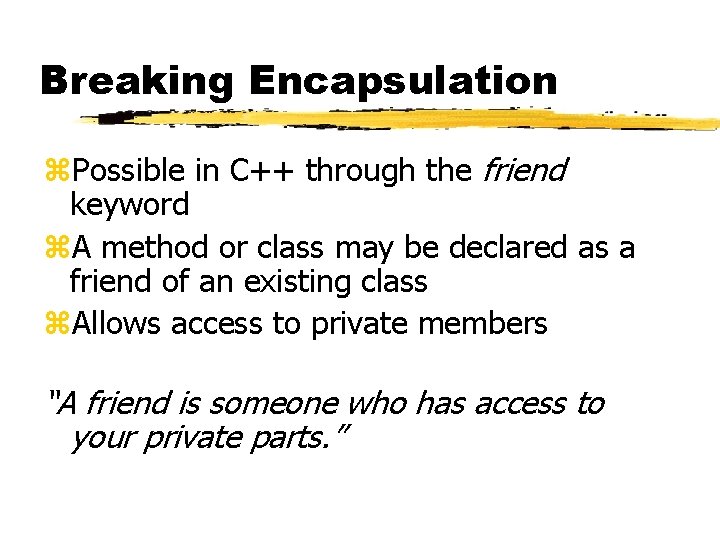
Breaking Encapsulation z. Possible in C++ through the friend keyword z. A method or class may be declared as a friend of an existing class z. Allows access to private members “A friend is someone who has access to your private parts. ”
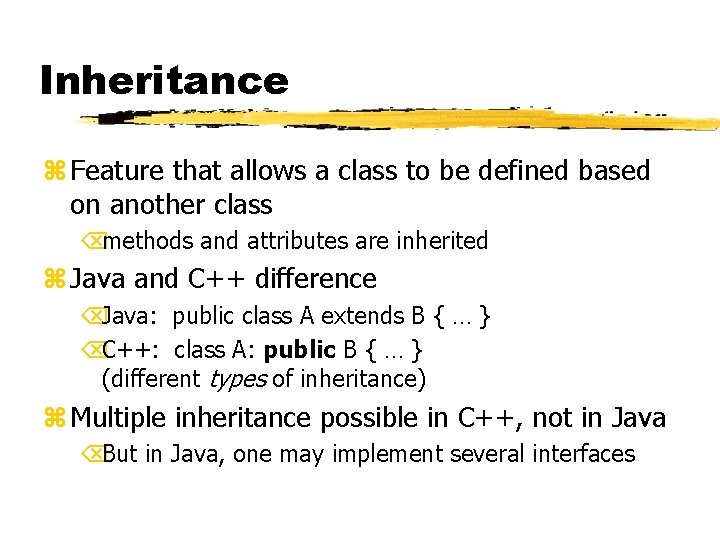
Inheritance z Feature that allows a class to be defined based on another class Õmethods and attributes are inherited z Java and C++ difference ÕJava: public class A extends B { … } ÕC++: class A: public B { … } (different types of inheritance) z Multiple inheritance possible in C++, not in Java ÕBut in Java, one may implement several interfaces
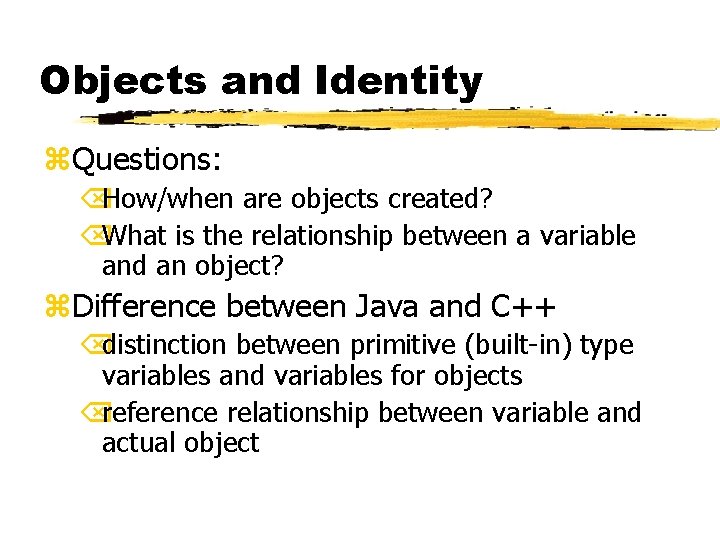
Objects and Identity z. Questions: ÕHow/when are objects created? ÕWhat is the relationship between a variable and an object? z. Difference between Java and C++ Õdistinction between primitive (built-in) type variables and variables for objects Õreference relationship between variable and actual object
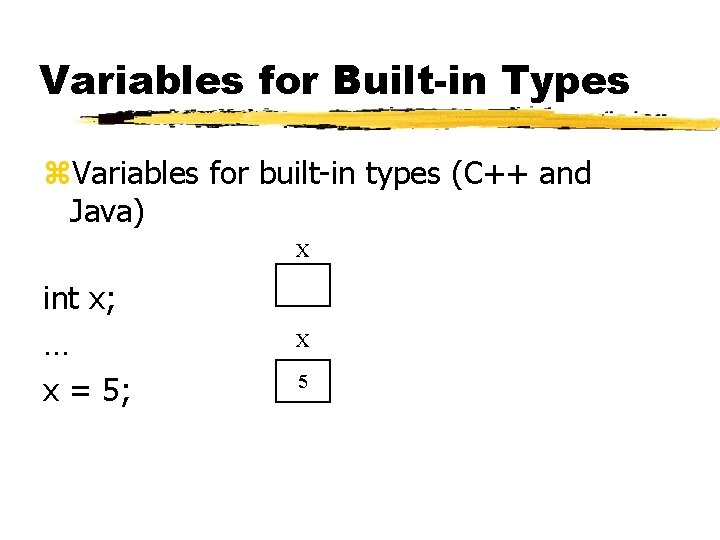
Variables for Built-in Types z. Variables for built-in types (C++ and Java) X int x; … x = 5; X 5
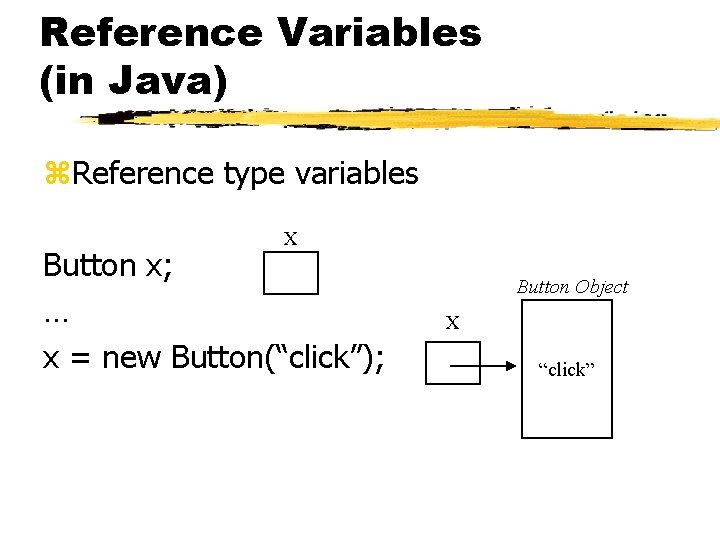
Reference Variables (in Java) z. Reference type variables X Button x; … x = new Button(“click”); Button Object X “click”
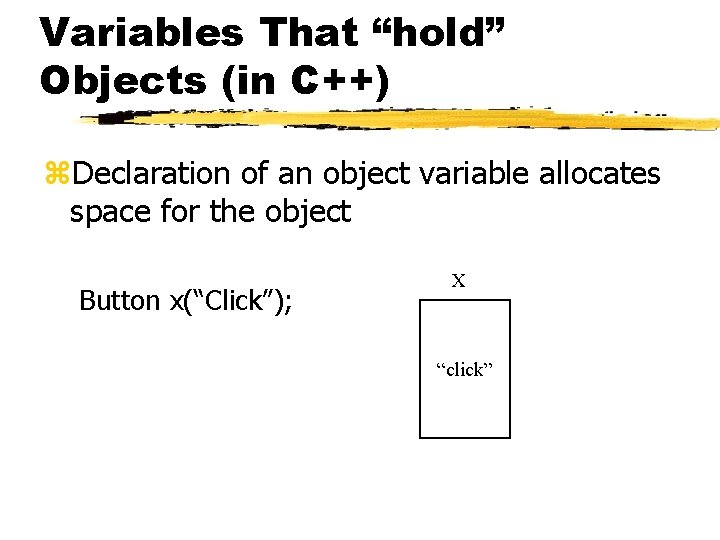
Variables That “hold” Objects (in C++) z. Declaration of an object variable allocates space for the object Button x(“Click”); X “click”
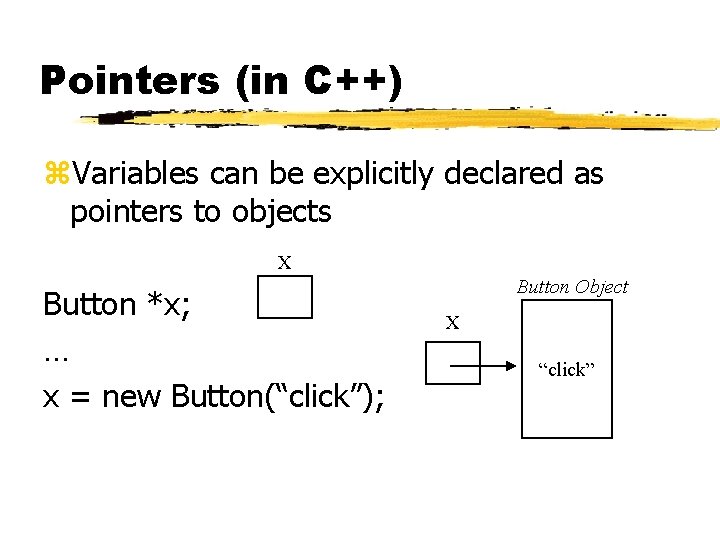
Pointers (in C++) z. Variables can be explicitly declared as pointers to objects X Button *x; … x = new Button(“click”); Button Object X “click”
![Arrays zint x20 Button b20 ÕValid declarations in C not in Java ÕCreates 20 Arrays zint x[20]; Button b[20]; ÕValid declarations in C++, not in Java ÕCreates 20](https://slidetodoc.com/presentation_image/30be384f759c94ece1d07ea7d4820e43/image-13.jpg)
Arrays zint x[20]; Button b[20]; ÕValid declarations in C++, not in Java ÕCreates 20 ints and 20 Button objects z. In Java, ÕDeclaration and array creation separate ÕFor object arrays, individual object creation necessary
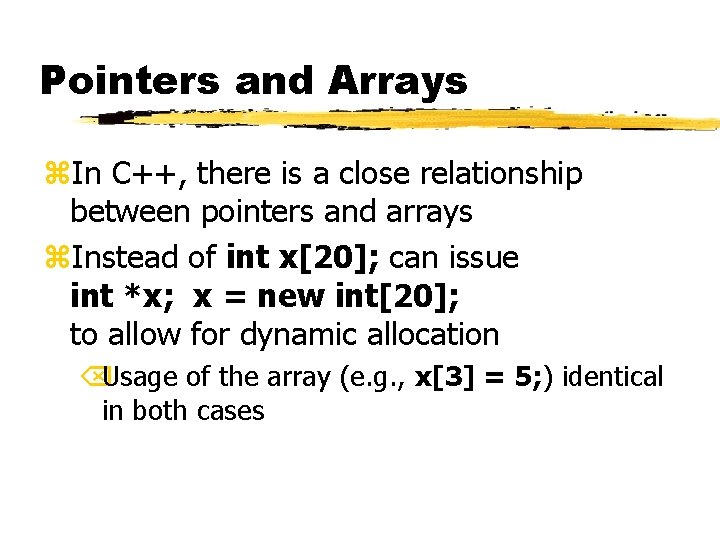
Pointers and Arrays z. In C++, there is a close relationship between pointers and arrays z. Instead of int x[20]; can issue int *x; x = new int[20]; to allow for dynamic allocation ÕUsage of the array (e. g. , x[3] = 5; ) identical in both cases
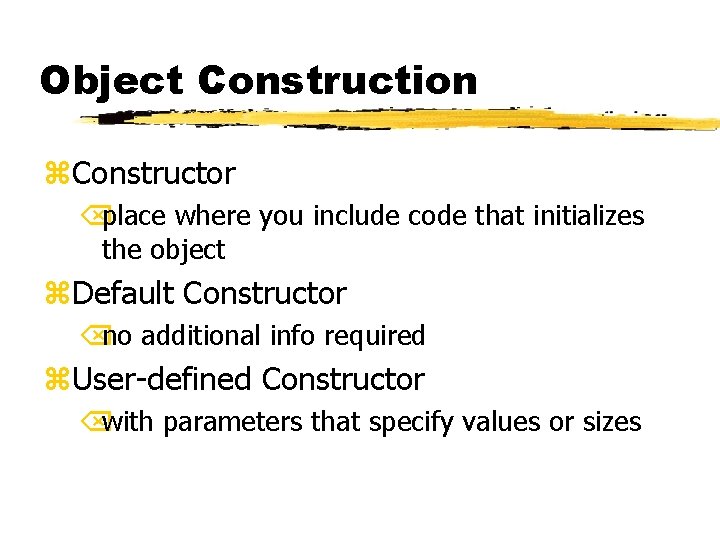
Object Construction z. Constructor Õplace where you include code that initializes the object z. Default Constructor Õno additional info required z. User-defined Constructor Õwith parameters that specify values or sizes
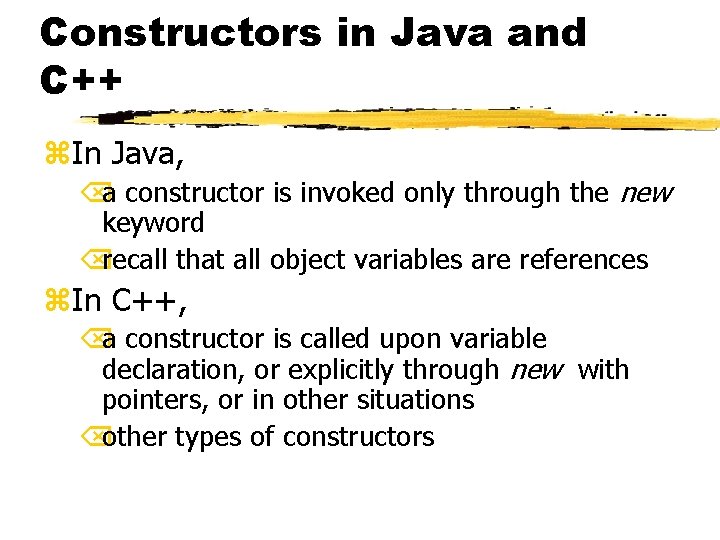
Constructors in Java and C++ z. In Java, Õa constructor is invoked only through the new keyword Õrecall that all object variables are references z. In C++, Õa constructor is called upon variable declaration, or explicitly through new with pointers, or in other situations Õother types of constructors
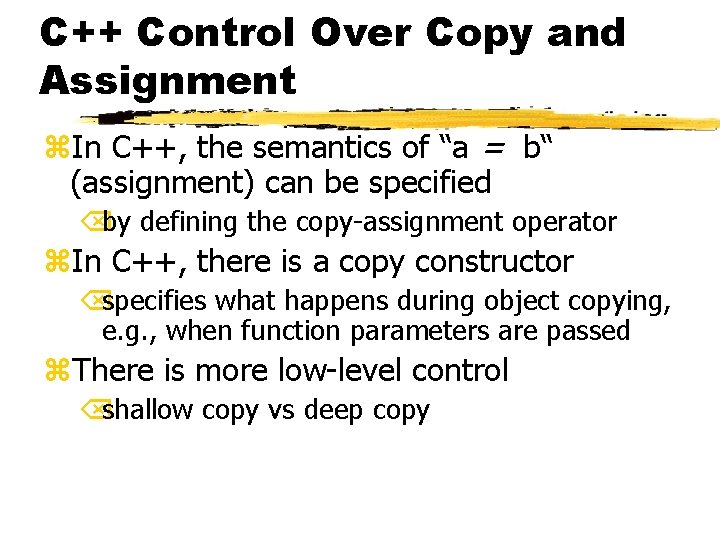
C++ Control Over Copy and Assignment z. In C++, the semantics of “a = b“ (assignment) can be specified Õby defining the copy-assignment operator z. In C++, there is a copy constructor Õspecifies what happens during object copying, e. g. , when function parameters are passed z. There is more low-level control Õshallow copy vs deep copy
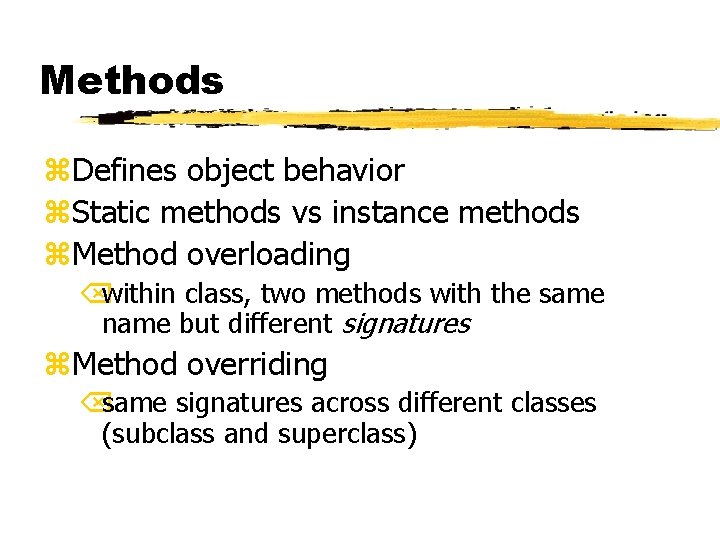
Methods z. Defines object behavior z. Static methods vs instance methods z. Method overloading Õwithin class, two methods with the same name but different signatures z. Method overriding Õsame signatures across different classes (subclass and superclass)
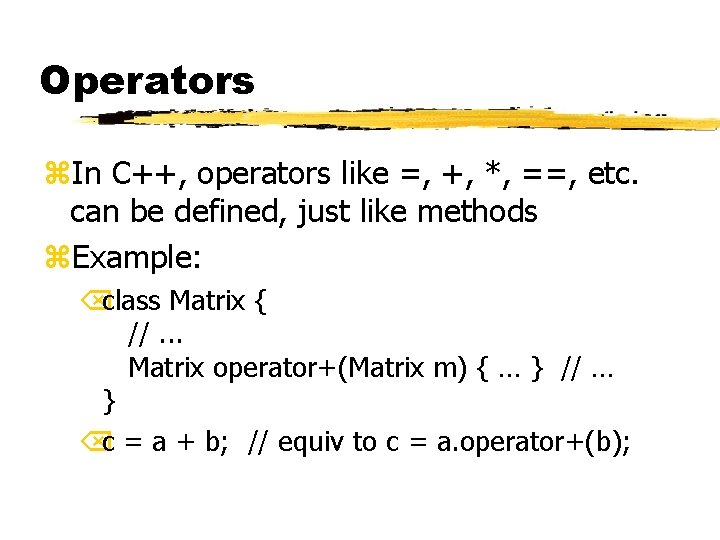
Operators z. In C++, operators like =, +, *, ==, etc. can be defined, just like methods z. Example: Õclass Matrix { //. . . Matrix operator+(Matrix m) { … } // … } Õc = a + b; // equiv to c = a. operator+(b);
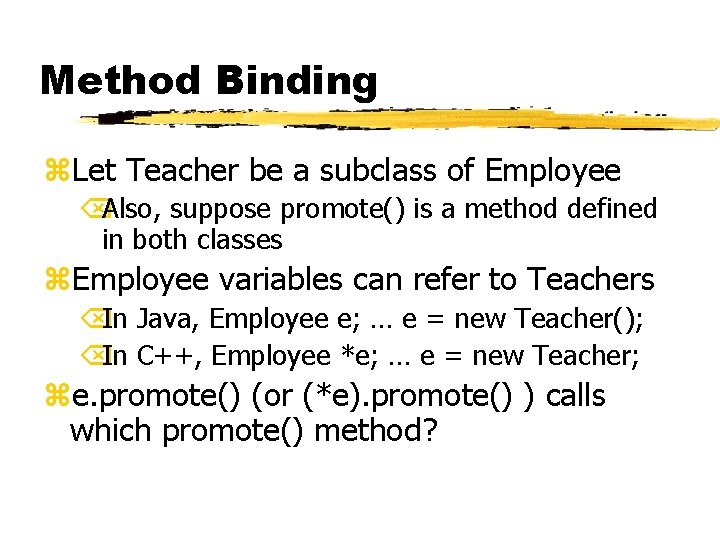
Method Binding z. Let Teacher be a subclass of Employee ÕAlso, suppose promote() is a method defined in both classes z. Employee variables can refer to Teachers ÕIn Java, Employee e; … e = new Teacher(); ÕIn C++, Employee *e; … e = new Teacher; ze. promote() (or (*e). promote() ) calls which promote() method?
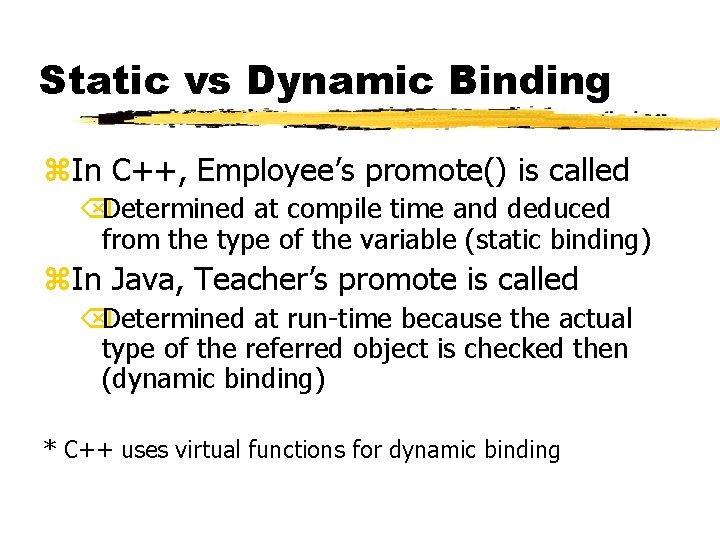
Static vs Dynamic Binding z. In C++, Employee’s promote() is called ÕDetermined at compile time and deduced from the type of the variable (static binding) z. In Java, Teacher’s promote is called ÕDetermined at run-time because the actual type of the referred object is checked then (dynamic binding) * C++ uses virtual functions for dynamic binding
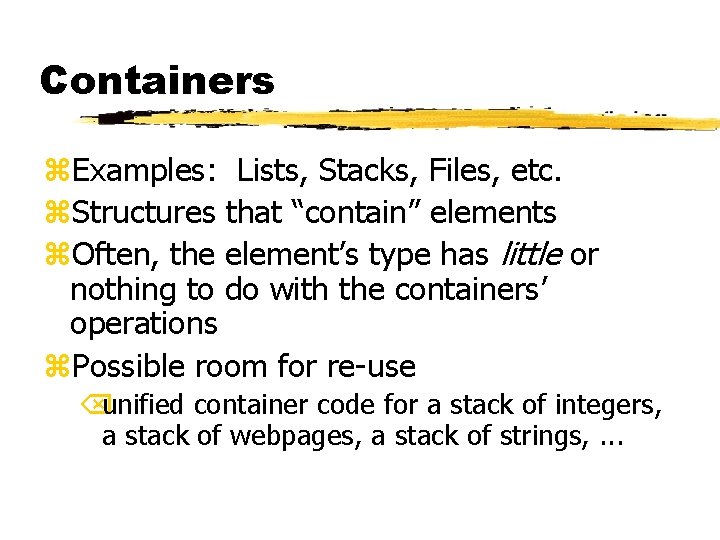
Containers z. Examples: Lists, Stacks, Files, etc. z. Structures that “contain” elements z. Often, the element’s type has little or nothing to do with the containers’ operations z. Possible room for re-use Õunified container code for a stack of integers, a stack of webpages, a stack of strings, . . .
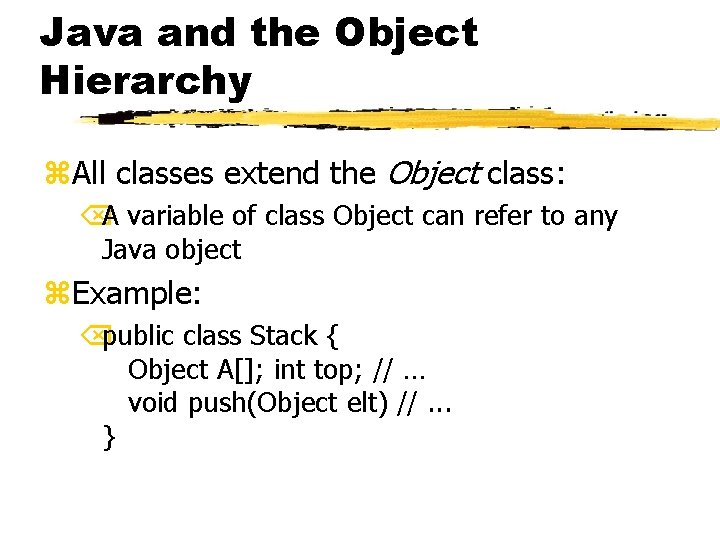
Java and the Object Hierarchy z. All classes extend the Object class: ÕA variable of class Object can refer to any Java object z. Example: Õpublic class Stack { Object A[]; int top; // … void push(Object elt) //. . . }
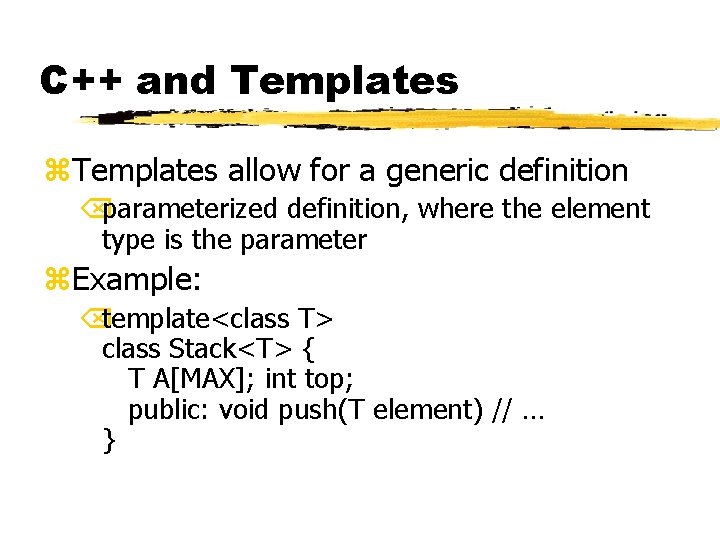
C++ and Templates z. Templates allow for a generic definition Õparameterized definition, where the element type is the parameter z. Example: Õtemplate<class T> class Stack<T> { T A[MAX]; int top; public: void push(T element) // … }
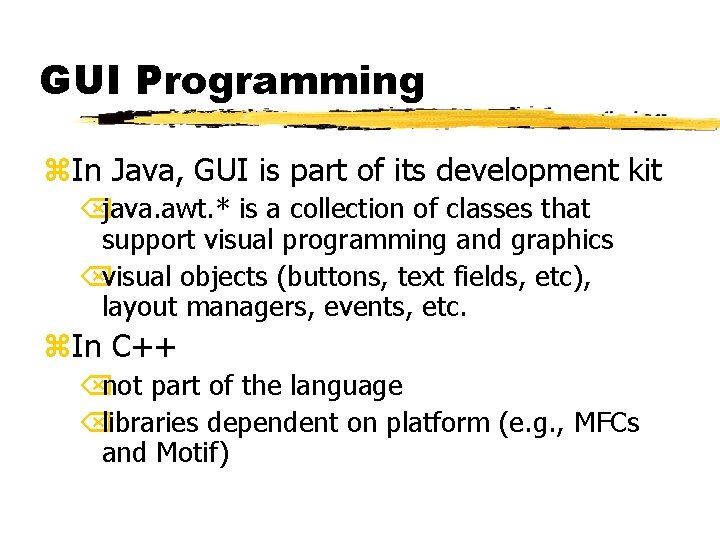
GUI Programming z. In Java, GUI is part of its development kit Õjava. awt. * is a collection of classes that support visual programming and graphics Õvisual objects (buttons, text fields, etc), layout managers, events, etc. z. In C++ Õnot part of the language Õlibraries dependent on platform (e. g. , MFCs and Motif)