Department of Computer and Information Science School of
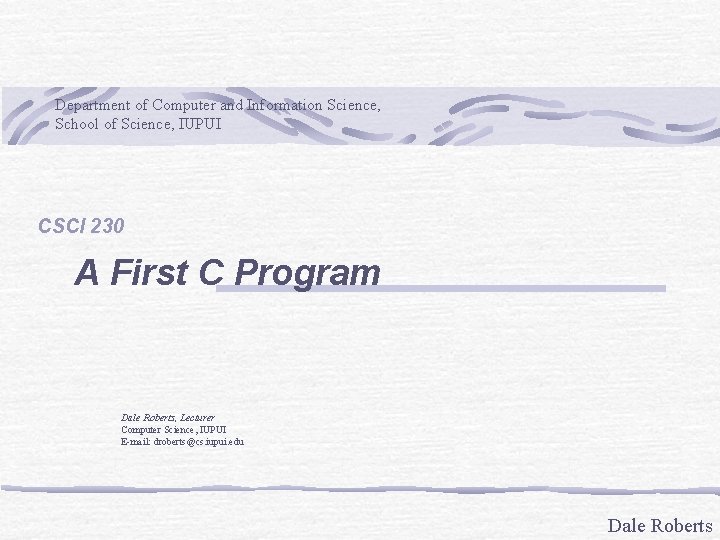
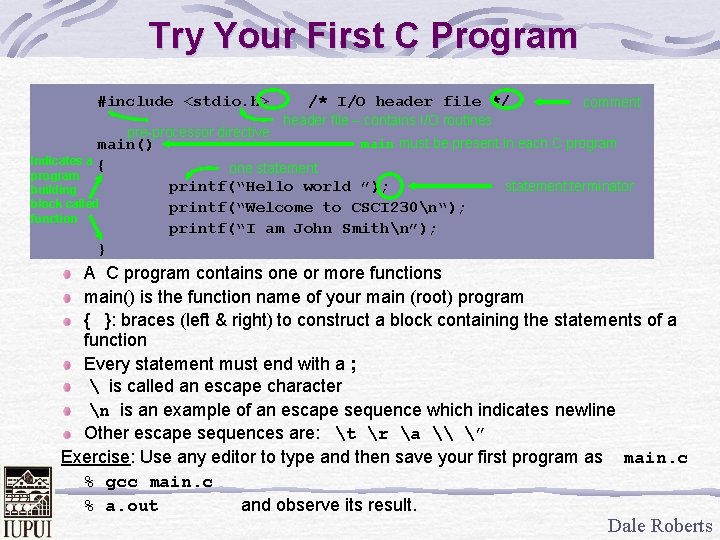
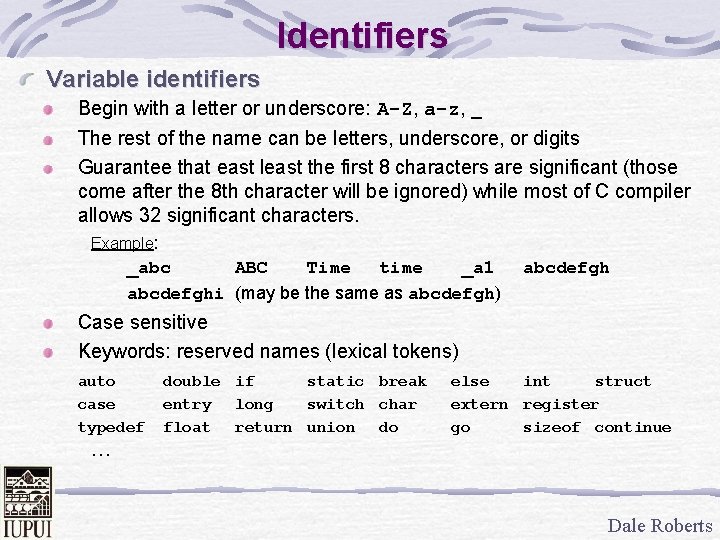
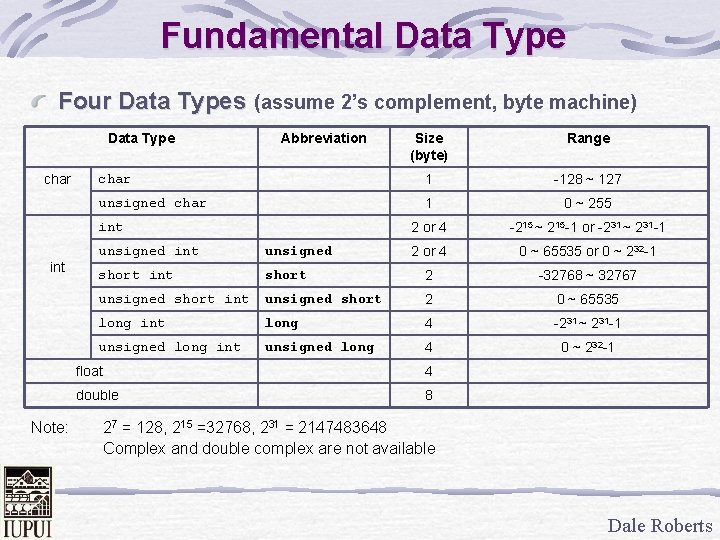
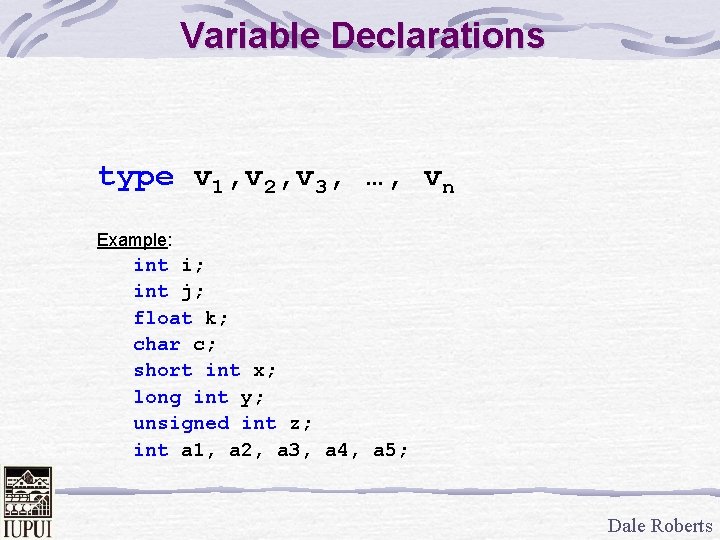
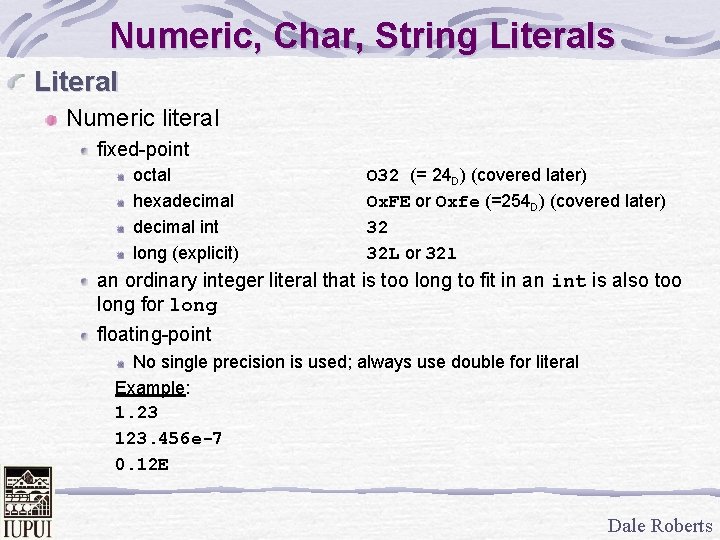
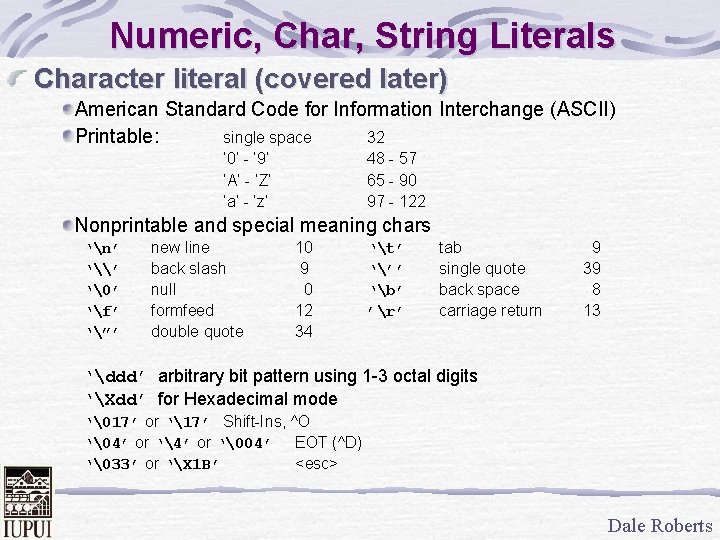
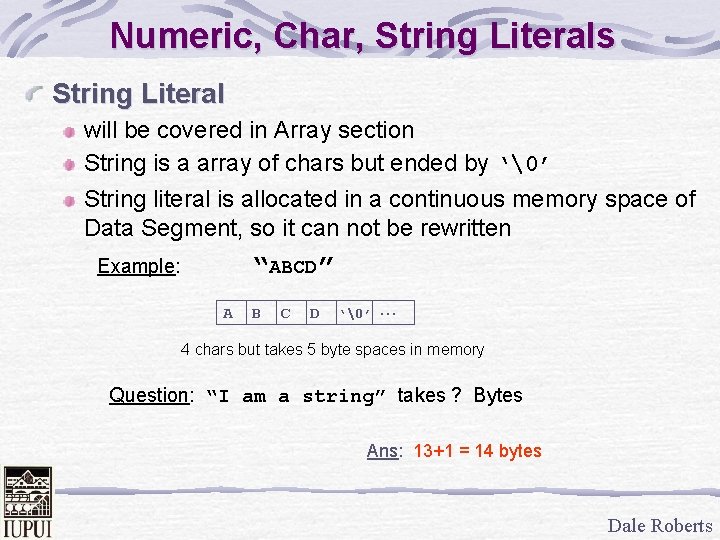
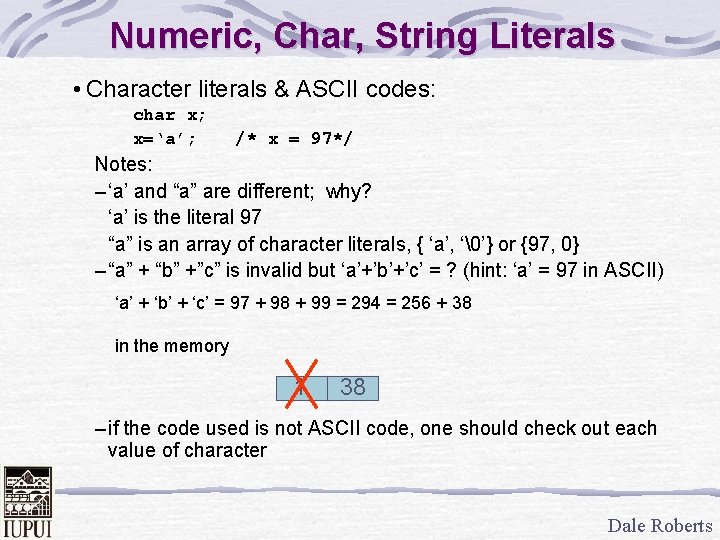
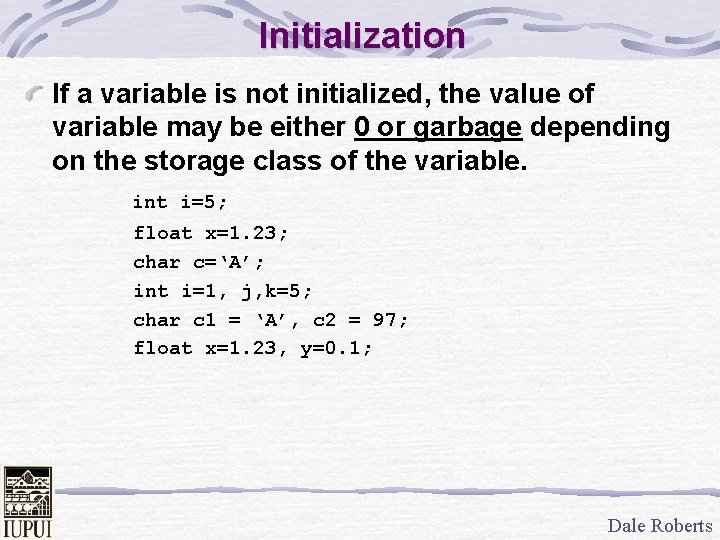
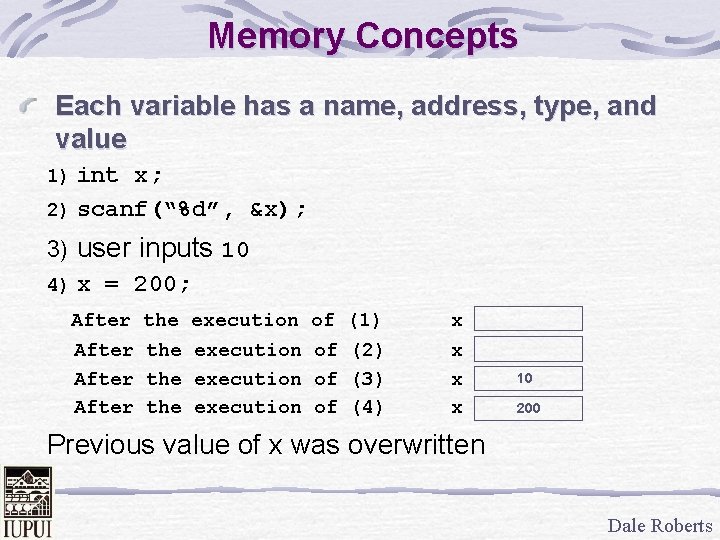
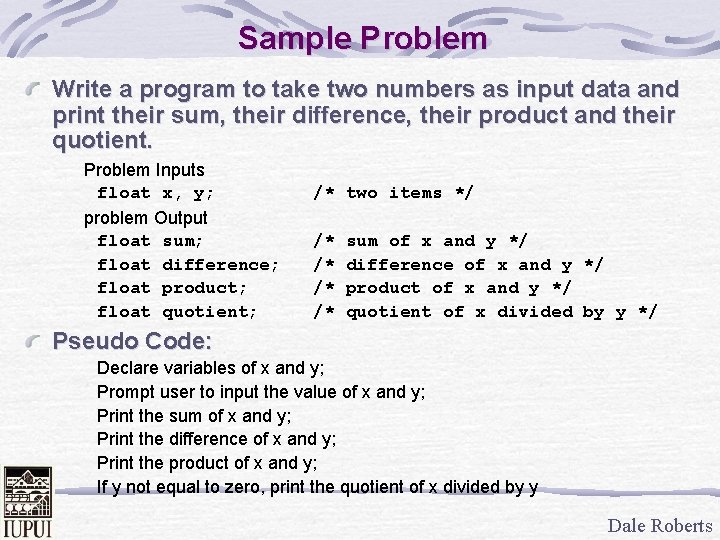
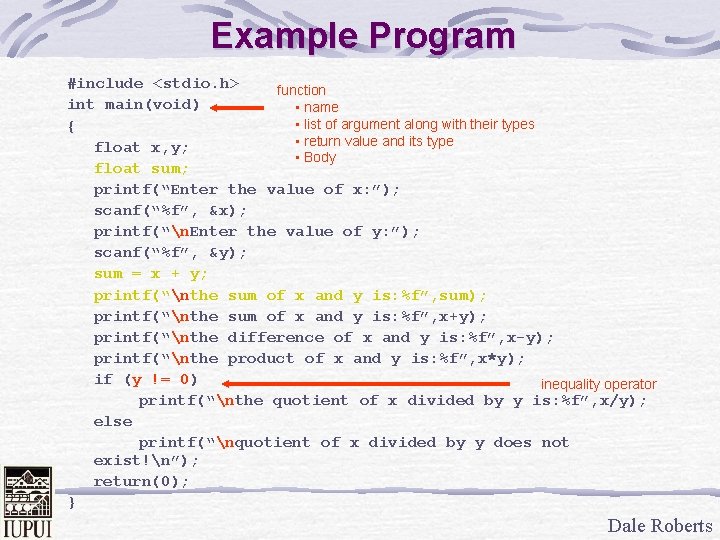
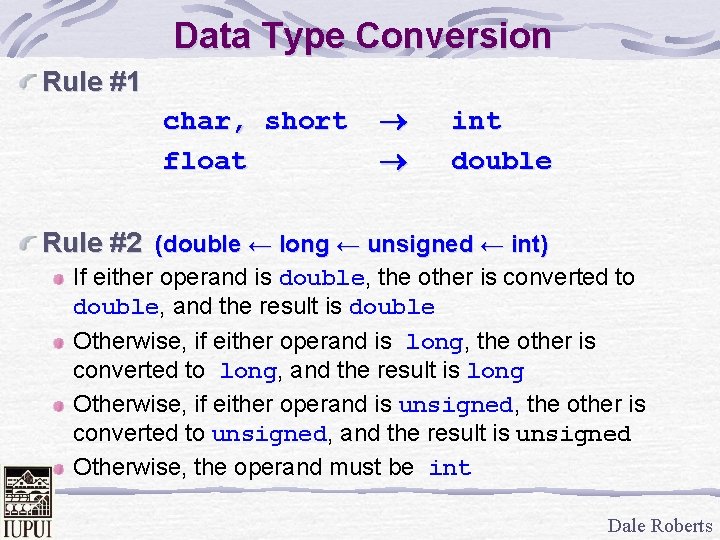
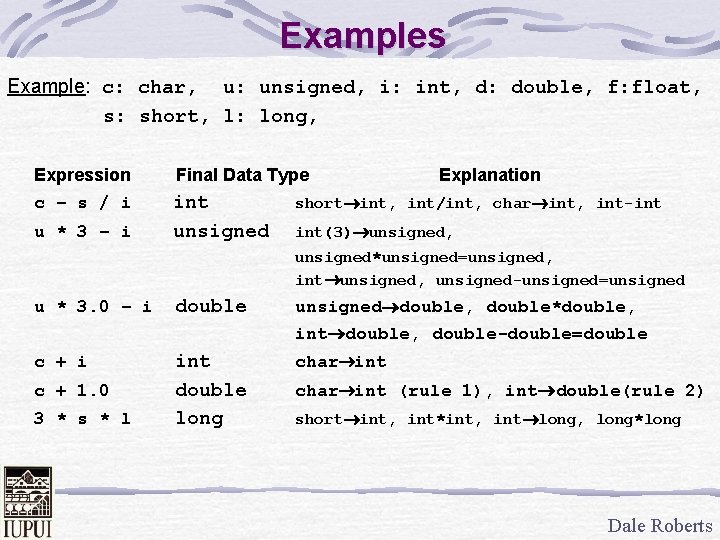
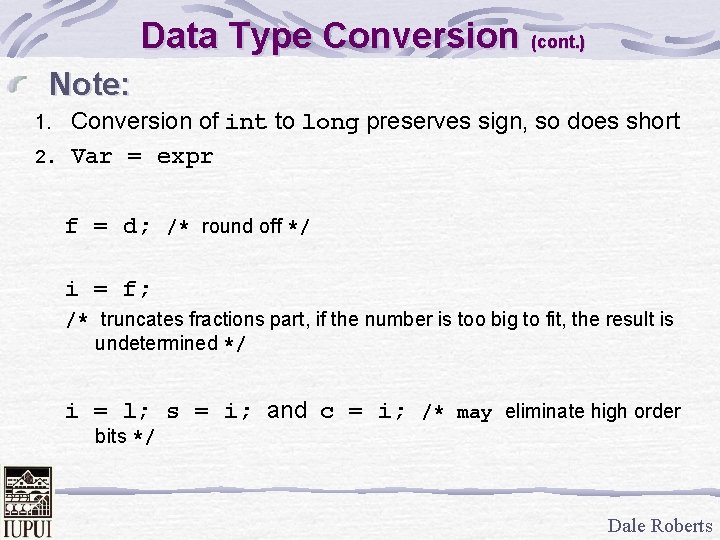
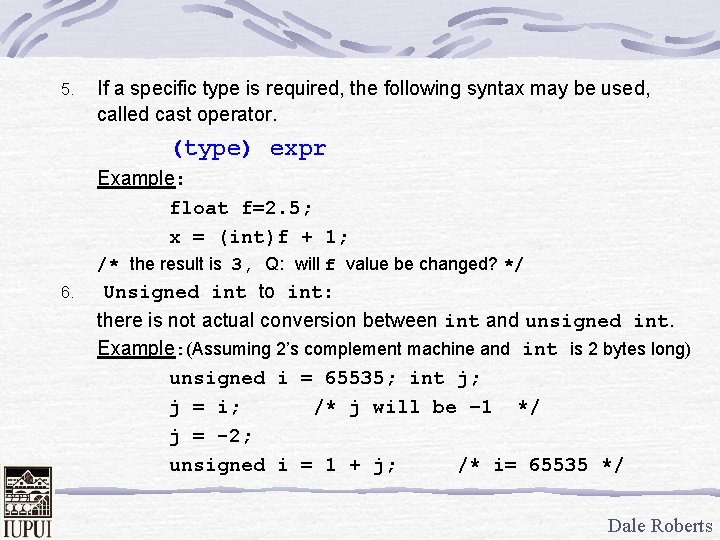
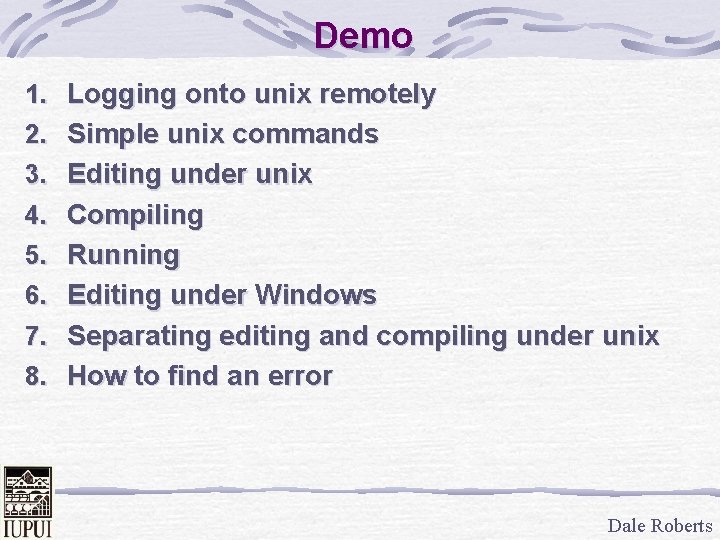
- Slides: 18
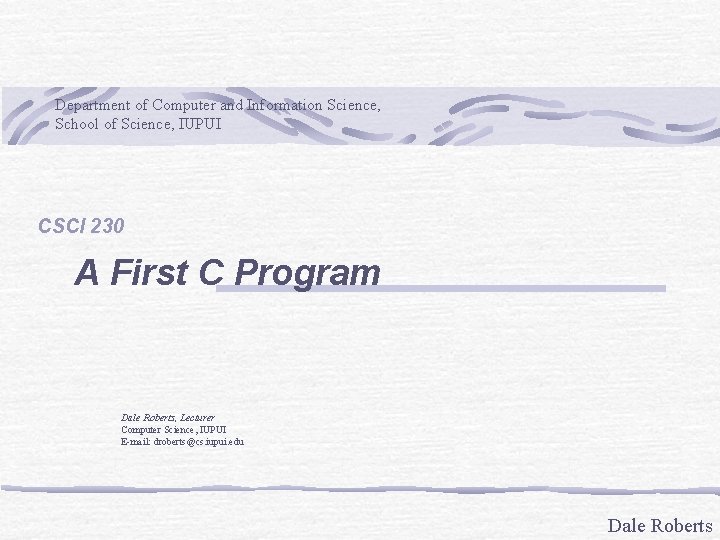
Department of Computer and Information Science, School of Science, IUPUI CSCI 230 A First C Program Dale Roberts, Lecturer Computer Science, IUPUI E-mail: droberts@cs. iupui. edu Dale Roberts
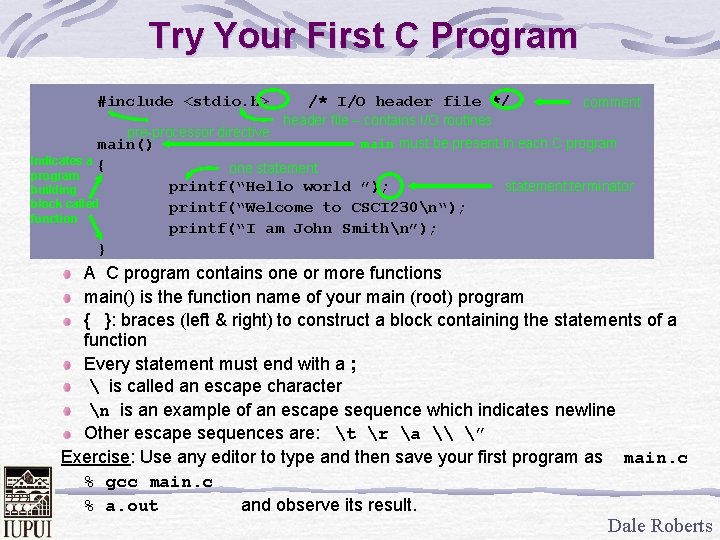
Try Your First C Program #include <stdio. h> pre-processor directive main() Indicates a { program building block called function /* I/O header file */ comment header file – contains I/O routines main must be present in each C program one statement printf(“Hello world ”); printf(“Welcome to CSCI 230n“); printf(“I am John Smithn”); statement terminator } A C program contains one or more functions main() is the function name of your main (root) program { }: braces (left & right) to construct a block containing the statements of a function Every statement must end with a ; is called an escape character n is an example of an escape sequence which indicates newline Other escape sequences are: t r a \ ” Exercise: Use any editor to type and then save your first program as main. c % gcc main. c % a. out and observe its result. Dale Roberts
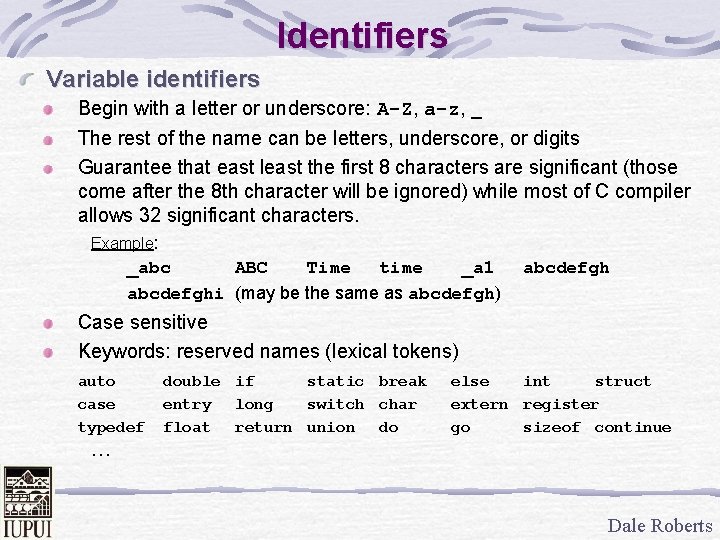
Identifiers Variable identifiers Begin with a letter or underscore: A-Z, a-z, _ The rest of the name can be letters, underscore, or digits Guarantee that east least the first 8 characters are significant (those come after the 8 th character will be ignored) while most of C compiler allows 32 significant characters. Example: _abc ABC Time time _a 1 abcdefghi (may be the same as abcdefgh) abcdefgh Case sensitive Keywords: reserved names (lexical tokens) auto case typedef double if static break entry long switch char float return union do else int struct extern register go sizeof continue … Dale Roberts
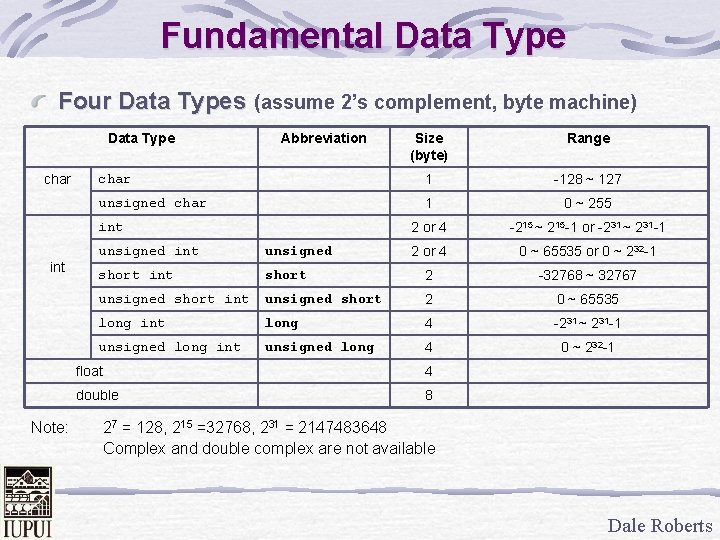
Fundamental Data Type Four Data Types (assume 2’s complement, byte machine) Data Type char Abbreviation Size (byte) Range char 1 -128 ~ 127 unsigned char 1 0 ~ 255 2 or 4 -215 ~ 215 -1 or -231 ~ 231 -1 2 or 4 0 ~ 65535 or 0 ~ 232 -1 int Note: unsigned int unsigned short int short 2 -32768 ~ 32767 unsigned short int unsigned short 2 0 ~ 65535 long int long 4 -231 ~ 231 -1 unsigned long int unsigned long 4 0 ~ 232 -1 float 4 double 8 27 = 128, 215 =32768, 231 = 2147483648 Complex and double complex are not available Dale Roberts
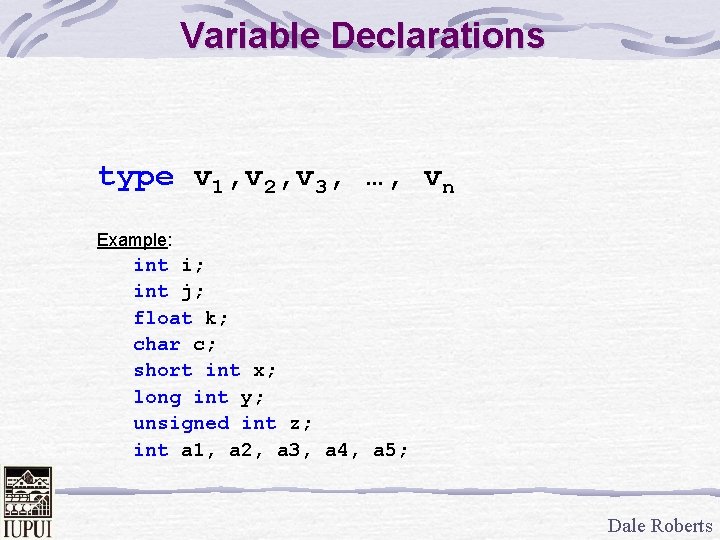
Variable Declarations type v 1, v 2, v 3, …, vn Example: int i; int j; float k; char c; short int x; long int y; unsigned int z; int a 1, a 2, a 3, a 4, a 5; Dale Roberts
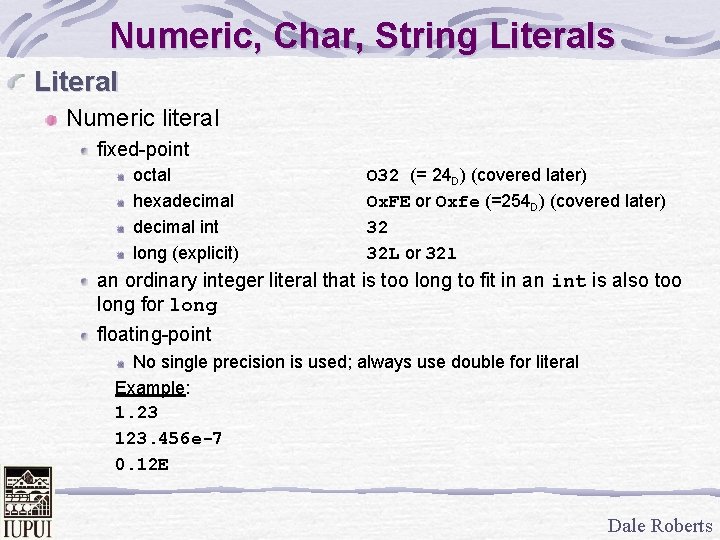
Numeric, Char, String Literals Literal Numeric literal fixed-point octal hexadecimal int long (explicit) O 32 (= 24 D) (covered later) Ox. FE or Oxfe (=254 D) (covered later) 32 32 L or 32 l an ordinary integer literal that is too long to fit in an int is also too long for long floating-point No single precision is used; always use double for literal Example: 1. 23 123. 456 e-7 0. 12 E Dale Roberts
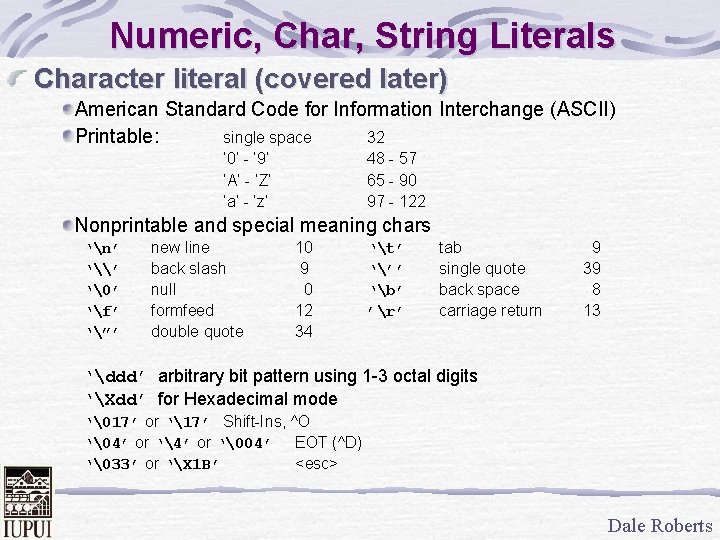
Numeric, Char, String Literals Character literal (covered later) American Standard Code for Information Interchange (ASCII) Printable: single space 32 ‘ 0’ - ‘ 9’ ‘A’ - ‘Z’ ‘a’ - ‘z’ 48 - 57 65 - 90 97 - 122 Nonprintable and special meaning chars ‘n’ ‘\’ ‘ ’ ‘f’ ‘”’ new line back slash null formfeed double quote 10 9 0 12 34 ‘t’ ‘’’ ‘b’ ’r’ tab single quote back space carriage return 9 39 8 13 ‘ddd’ arbitrary bit pattern using 1 -3 octal digits ‘Xdd’ for Hexadecimal mode ‘ 17’ or ‘17’ Shift-Ins, ^O ‘ 4’ or ‘ 04’ EOT (^D) ‘ 33’ or ‘X 1 B’ <esc> Dale Roberts
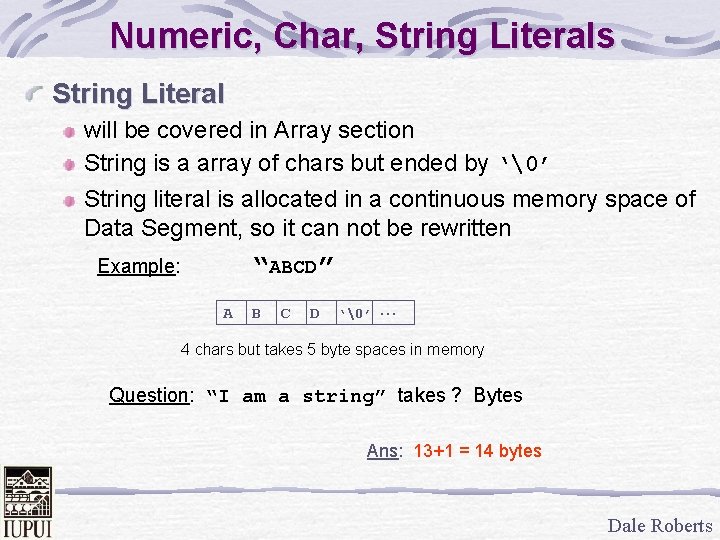
Numeric, Char, String Literals String Literal will be covered in Array section String is a array of chars but ended by ‘ ’ String literal is allocated in a continuous memory space of Data Segment, so it can not be rewritten “ABCD” Example: A B C D ‘ ’ . . . 4 chars but takes 5 byte spaces in memory Question: “I am a string” takes ? Bytes Ans: 13+1 = 14 bytes Dale Roberts
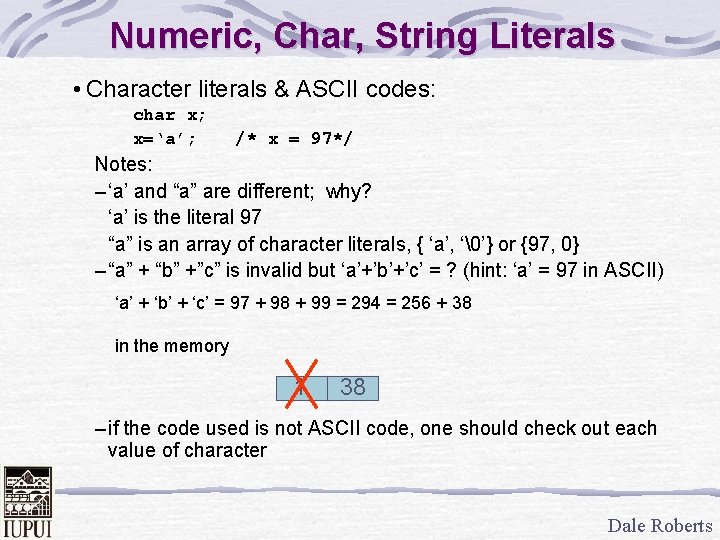
Numeric, Char, String Literals • Character literals & ASCII codes: char x; x=‘a’; /* x = 97*/ Notes: – ‘a’ and “a” are different; why? ‘a’ is the literal 97 “a” is an array of character literals, { ‘a’, ‘ ’} or {97, 0} – “a” + “b” +”c” is invalid but ‘a’+’b’+’c’ = ? (hint: ‘a’ = 97 in ASCII) ‘a’ + ‘b’ + ‘c’ = 97 + 98 + 99 = 294 = 256 + 38 in the memory 1 38 – if the code used is not ASCII code, one should check out each value of character Dale Roberts
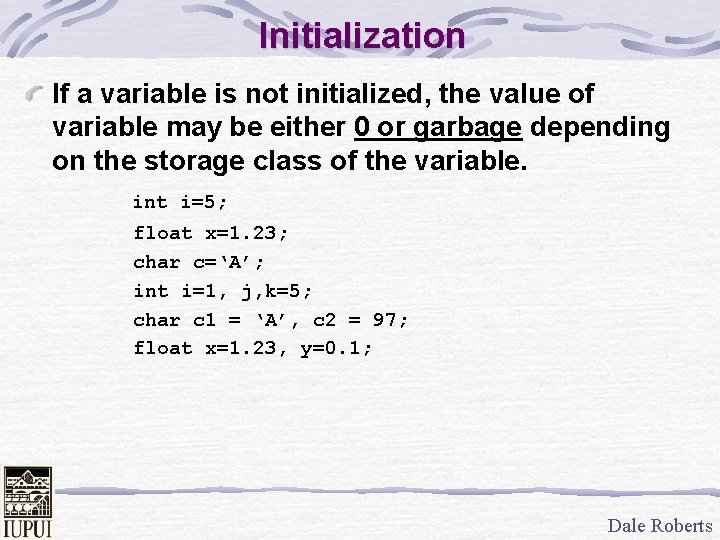
Initialization If a variable is not initialized, the value of variable may be either 0 or garbage depending on the storage class of the variable. int i=5; float x=1. 23; char c=‘A’; int i=1, j, k=5; char c 1 = ‘A’, c 2 = 97; float x=1. 23, y=0. 1; Dale Roberts
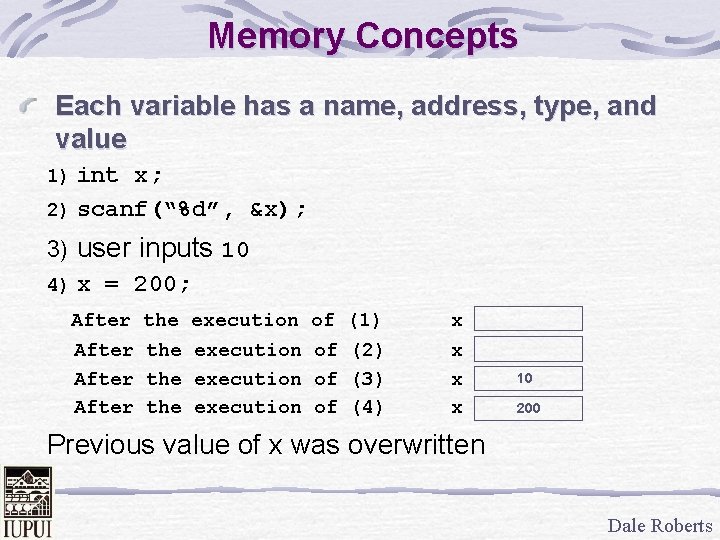
Memory Concepts Each variable has a name, address, type, and value int x; 2) scanf(“%d”, &x); 1) 3) user inputs 10 4) x = 200; After the execution of (1) x After the execution of (2) After the execution of (3) After the execution of (4) x x x 10 200 Previous value of x was overwritten Dale Roberts
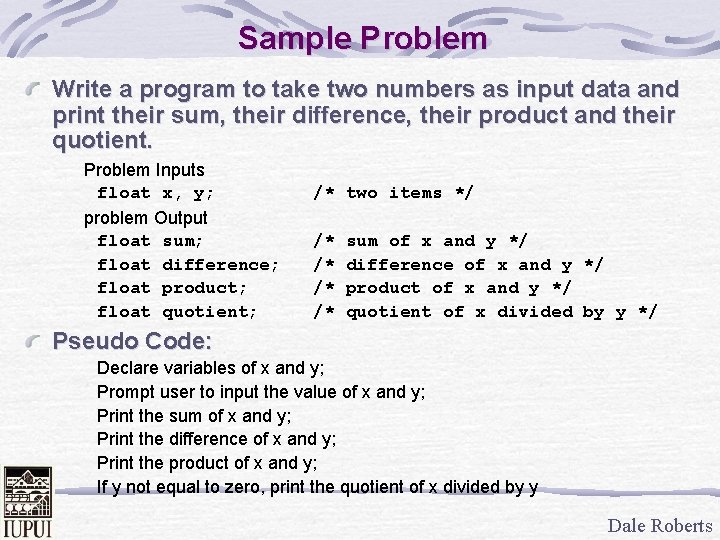
Sample Problem Write a program to take two numbers as input data and print their sum, their difference, their product and their quotient. Problem Inputs float x, y; problem Output float sum; float difference; float product; float quotient; /* two items */ /* /* sum of x and y */ difference of x and y */ product of x and y */ quotient of x divided by y */ Pseudo Code: Declare variables of x and y; Prompt user to input the value of x and y; Print the sum of x and y; Print the difference of x and y; Print the product of x and y; If y not equal to zero, print the quotient of x divided by y Dale Roberts
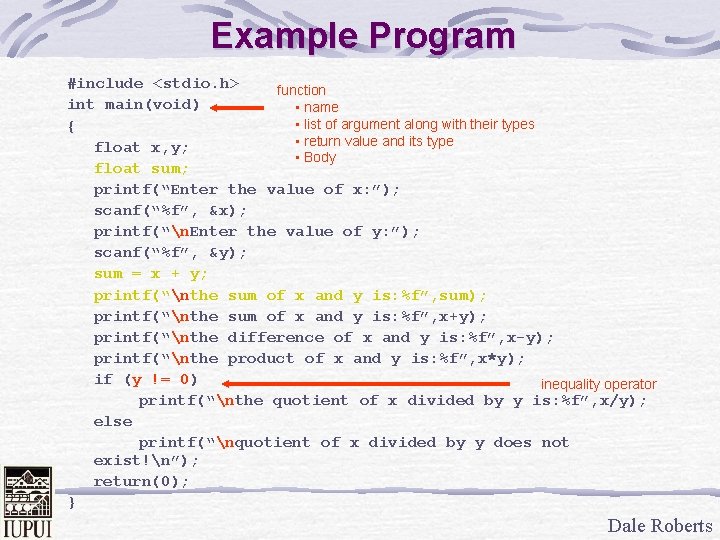
Example Program #include <stdio. h> function int main(void) • name • list of argument along with their types { • return value and its type float x, y; • Body float sum; printf(“Enter the value of x: ”); scanf(“%f”, &x); printf(“n. Enter the value of y: ”); scanf(“%f”, &y); sum = x + y; printf(“nthe sum of x and y is: %f”, sum); printf(“nthe sum of x and y is: %f”, x+y); printf(“nthe difference of x and y is: %f”, x-y); printf(“nthe product of x and y is: %f”, x*y); if (y != 0) inequality operator printf(“nthe quotient of x divided by y is: %f”, x/y); else printf(“nquotient of x divided by y does not exist!n”); return(0); } Dale Roberts
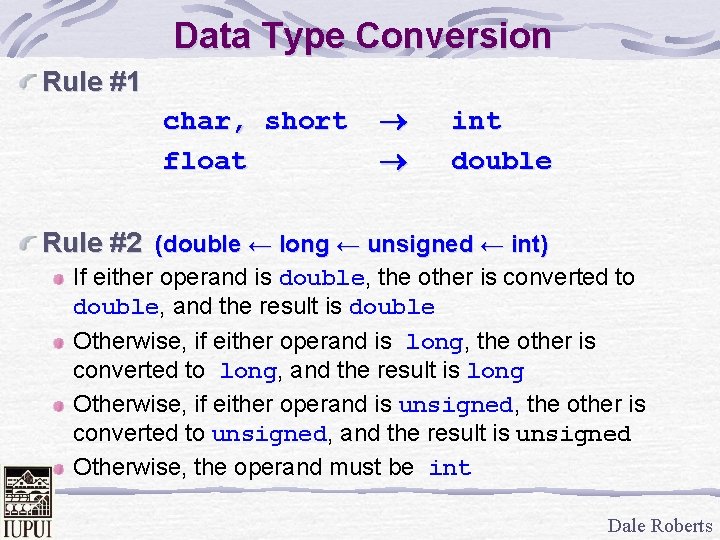
Data Type Conversion Rule #1 char, short float int double Rule #2 (double ← long ← unsigned ← int) If either operand is double, the other is converted to double, and the result is double Otherwise, if either operand is long, the other is converted to long, and the result is long Otherwise, if either operand is unsigned, the other is converted to unsigned, and the result is unsigned Otherwise, the operand must be int Dale Roberts
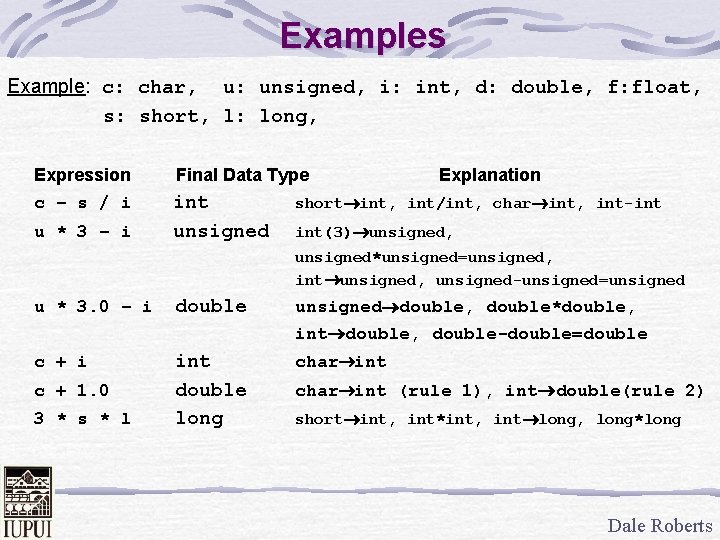
Examples Example: c: char, u: unsigned, i: int, d: double, f: float, s: short, l: long, Expression c – s / i u * 3 – i Final Data Type int unsigned Explanation short int, int/int, char int, int-int int(3) unsigned, unsigned*unsigned=unsigned, int unsigned, unsigned-unsigned=unsigned u * 3. 0 – i double unsigned double, double*double, int double, double-double=double c + i int double long char int c + 1. 0 3 * s * l char int (rule 1), int double(rule 2) short int, int*int, int long, long*long Dale Roberts
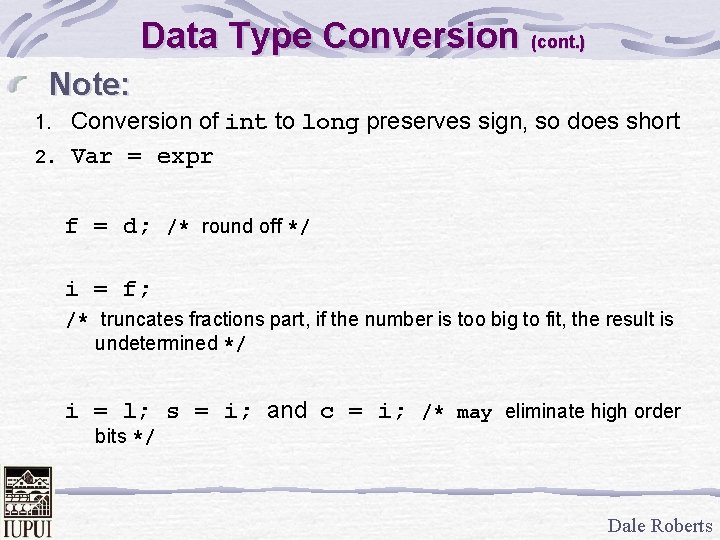
Data Type Conversion (cont. ) Note: Conversion of int to long preserves sign, so does short 2. Var = expr 1. f = d; /* round off */ i = f; /* truncates fractions part, if the number is too big to fit, the result is undetermined */ i = l; s = i; and c = i; /* may eliminate high order bits */ Dale Roberts
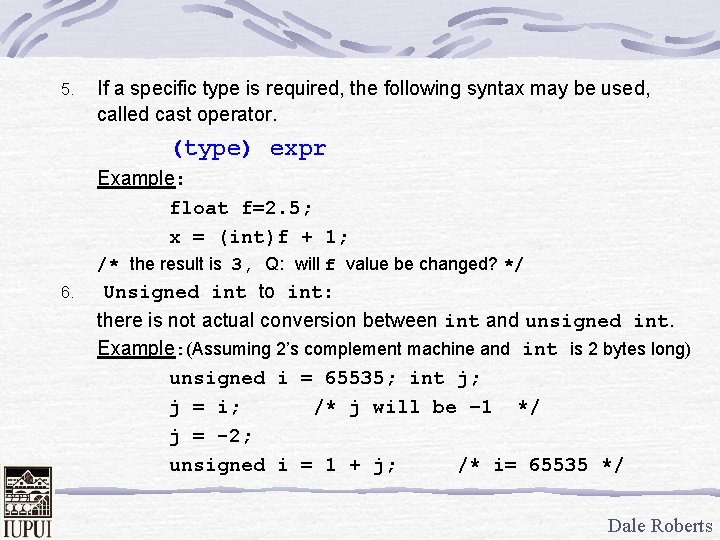
5. If a specific type is required, the following syntax may be used, called cast operator. (type) expr Example: float f=2. 5; x = (int)f + 1; /* the result is 3, Q: will f value be changed? */ 6. Unsigned int to int: there is not actual conversion between int and unsigned int. Example: (Assuming 2’s complement machine and int is 2 bytes long) unsigned i = 65535; int j; j = i; /* j will be – 1 */ j = -2; unsigned i = 1 + j; /* i= 65535 */ Dale Roberts
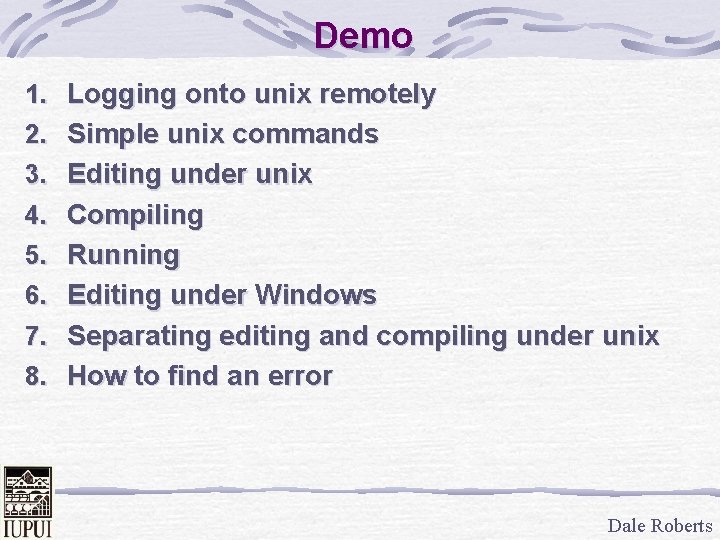
Demo 1. Logging onto unix remotely 2. Simple unix commands 3. Editing under unix 4. Compiling 5. Running 6. Editing under Windows 7. Separating editing and compiling under unix 8. How to find an error Dale Roberts
Ucl meng computer science
Northwestern university computer engineering
Computer science department rutgers
Computer science department stanford
Fsu cybersecurity major
Ubc computer science department
Bhargavi goswami
Computer science department columbia
My favourite subject is
Erik jonsson school of engineering and computer science
Erik jonsson school of engineering and computer science
Erik jonsson school of engineering and computer science
Efi arazi school of computer science
Iit delhi material science
Tum
Latest electronic and information technology in odisha
School education and literacy department
Department of forensic science dc
Ohio department of education science standards