Computer and Information Sciences College Computer Science Department
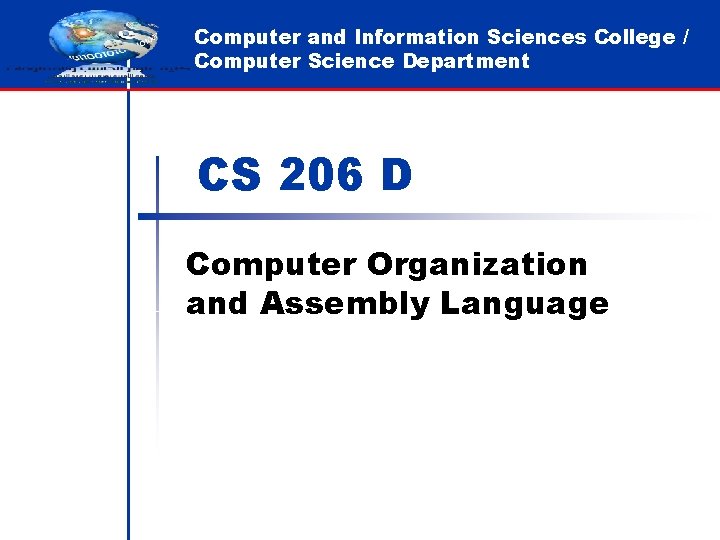
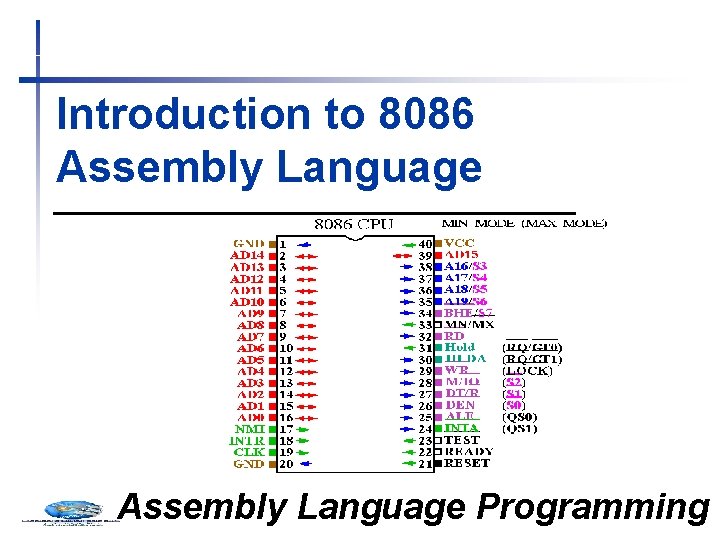
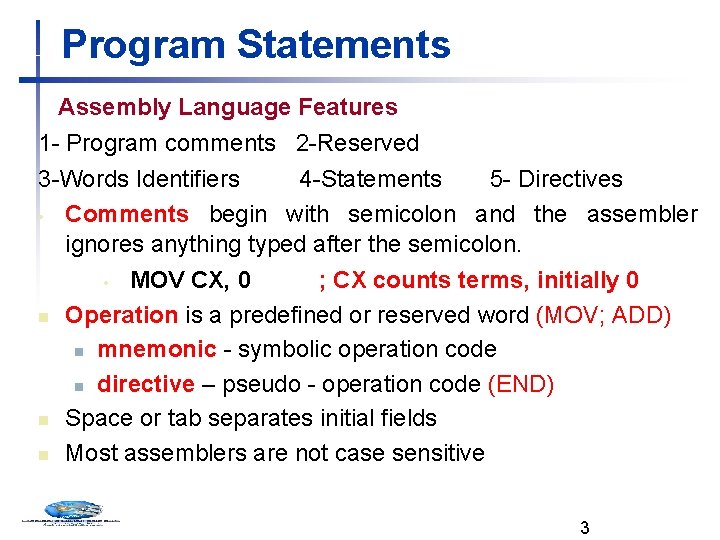
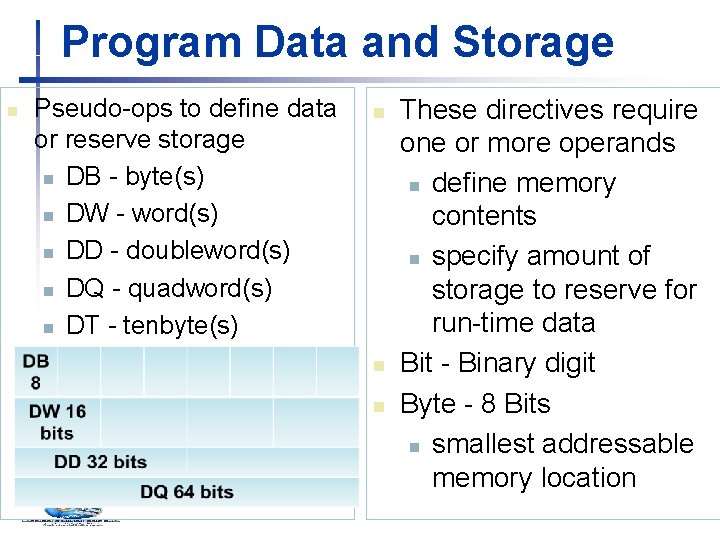
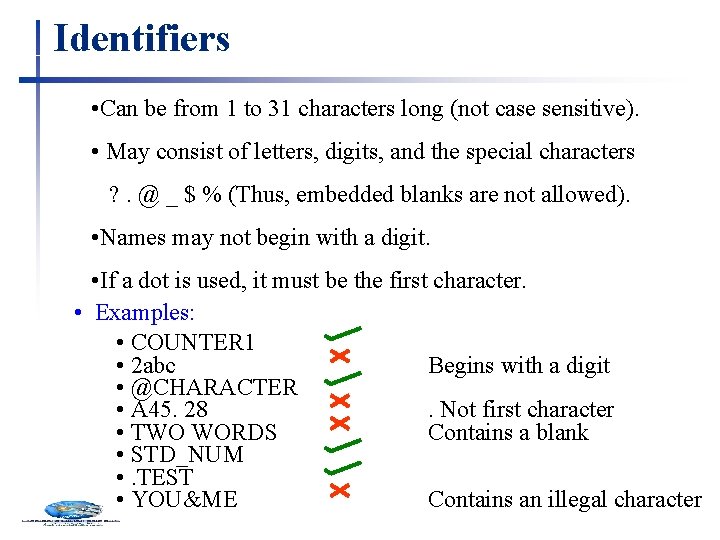
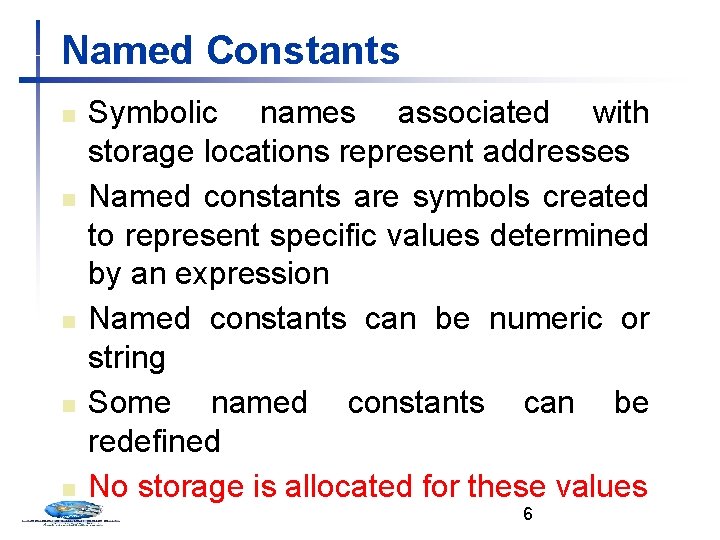
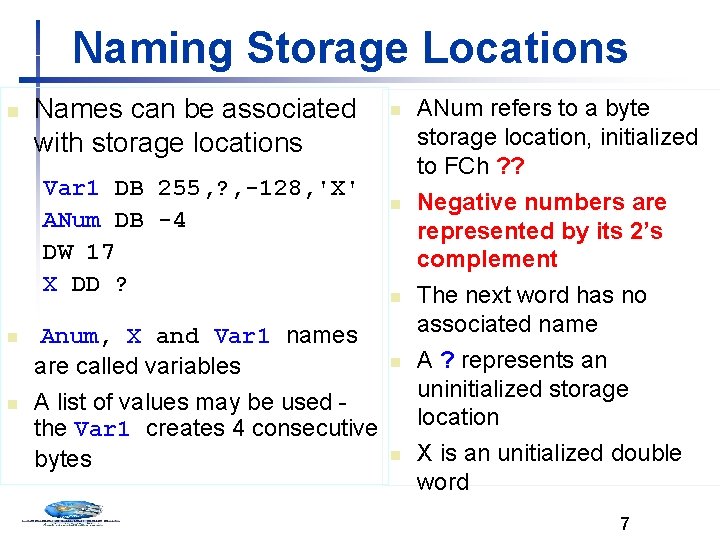
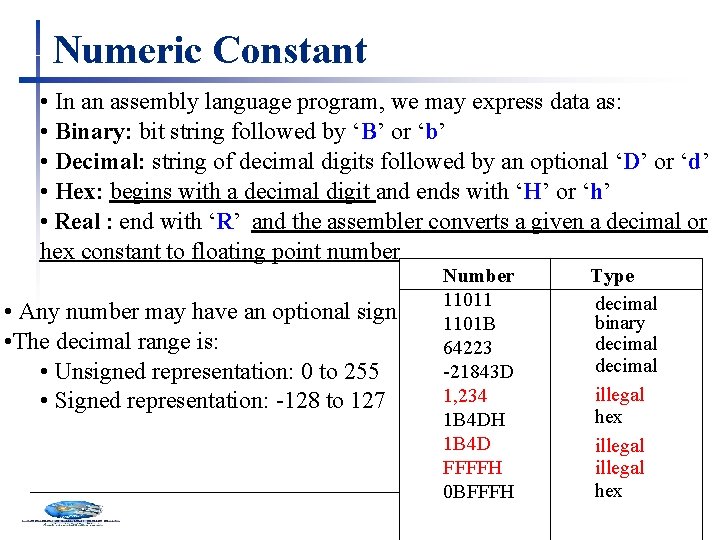
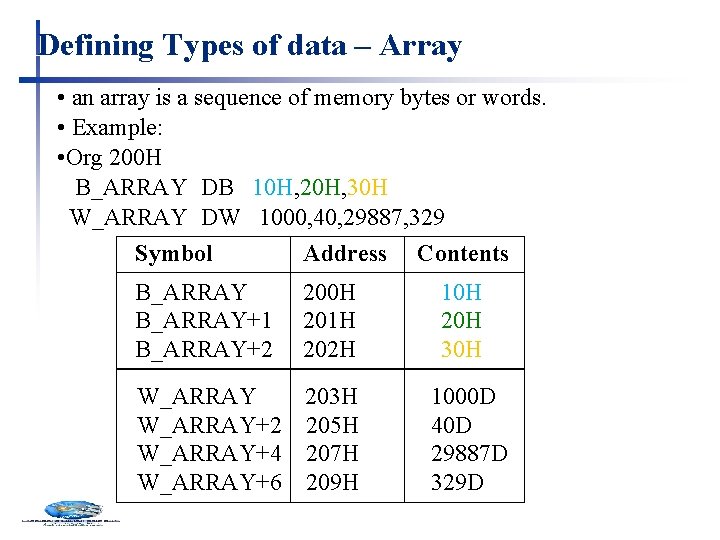
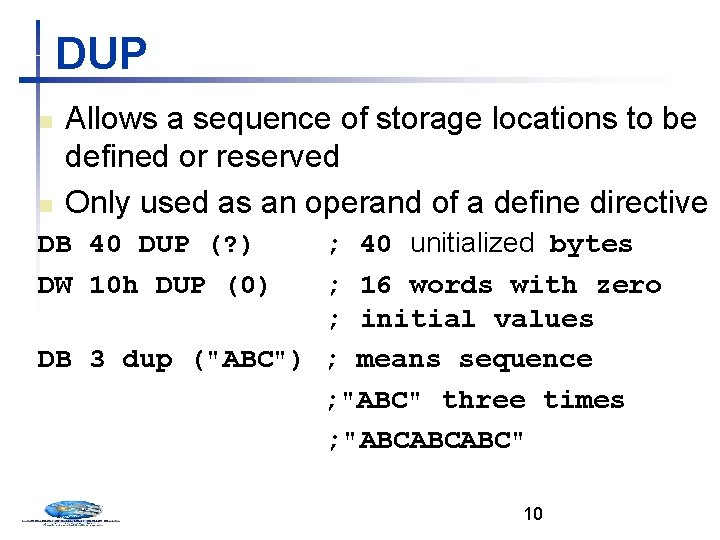
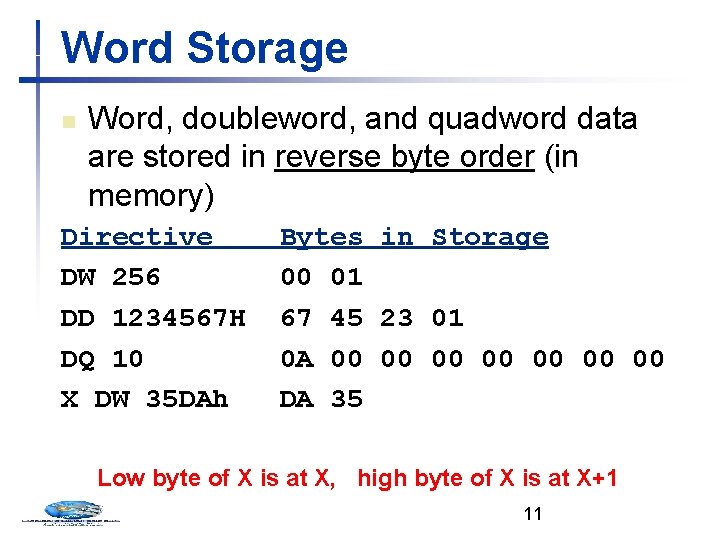
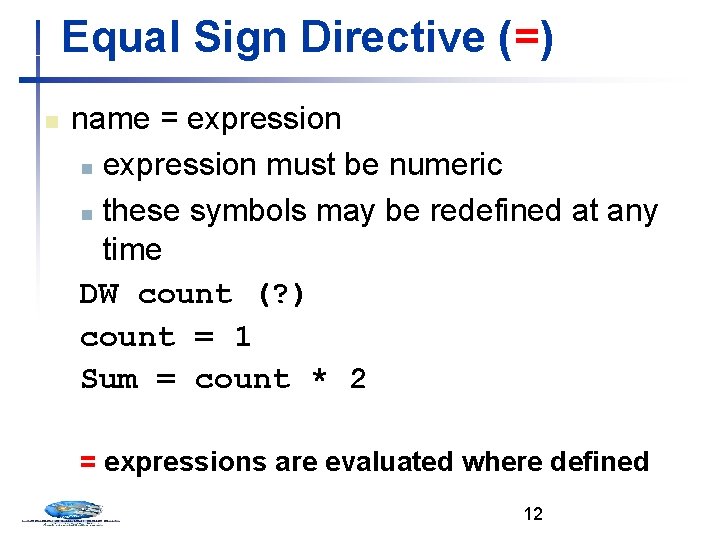
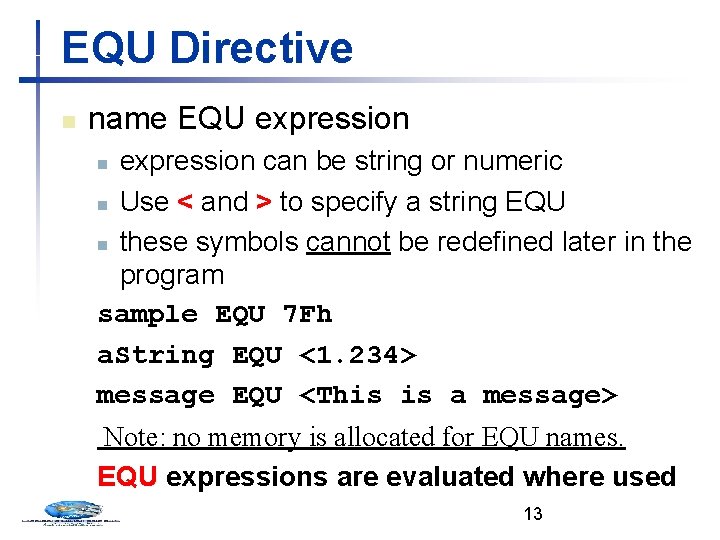
![Statements • Both instructions and directives have up to four fields: [identifier ] operation Statements • Both instructions and directives have up to four fields: [identifier ] operation](https://slidetodoc.com/presentation_image_h/2222071b3790402be4832ac38f7c9857/image-14.jpg)
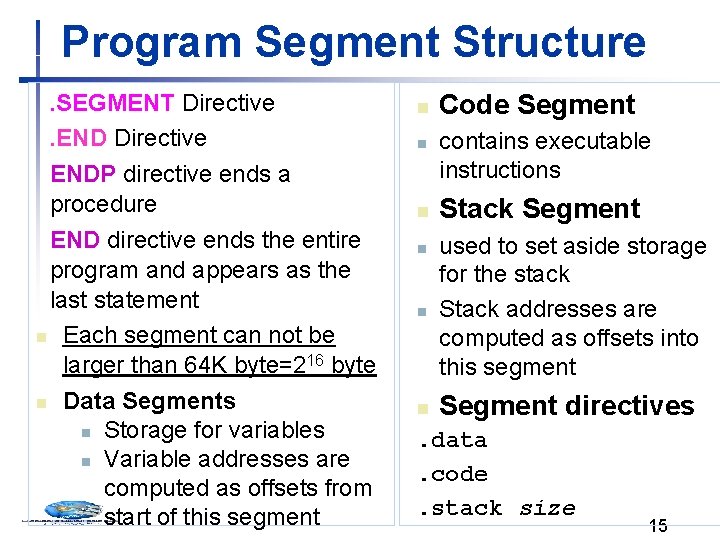
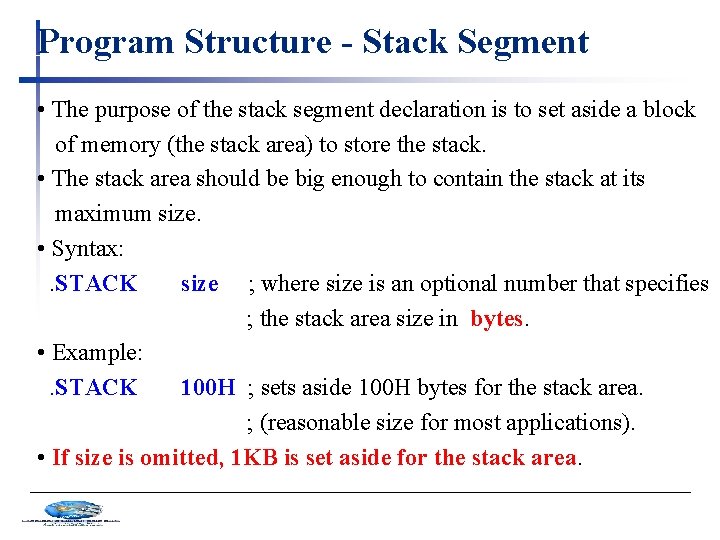
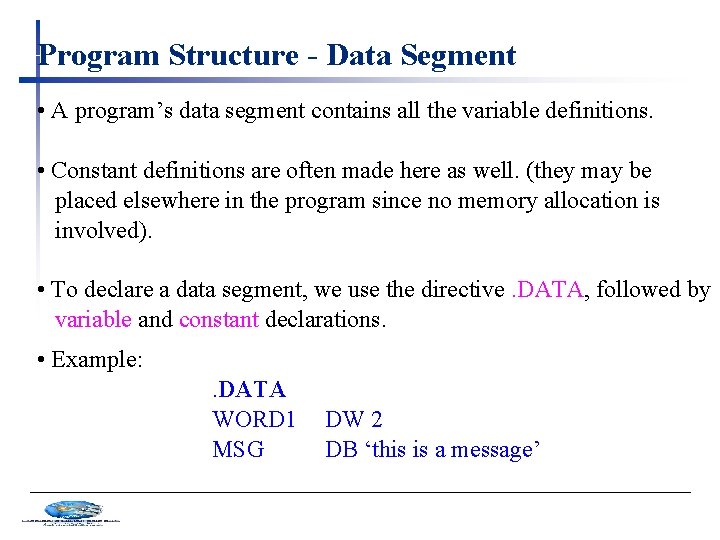
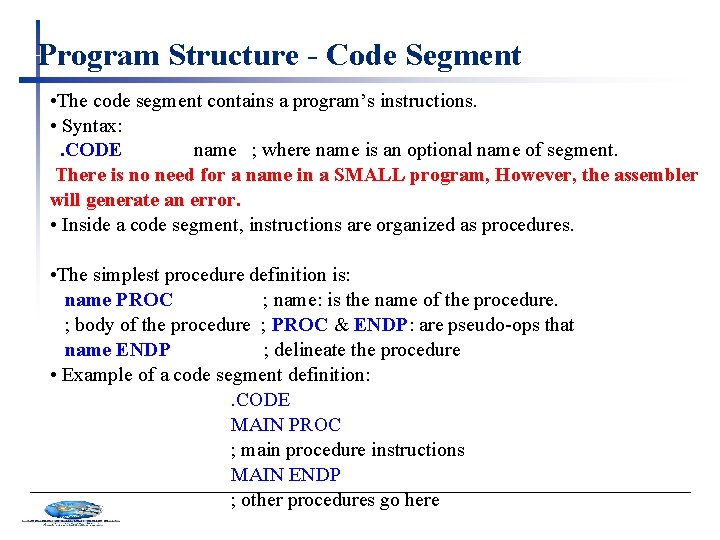
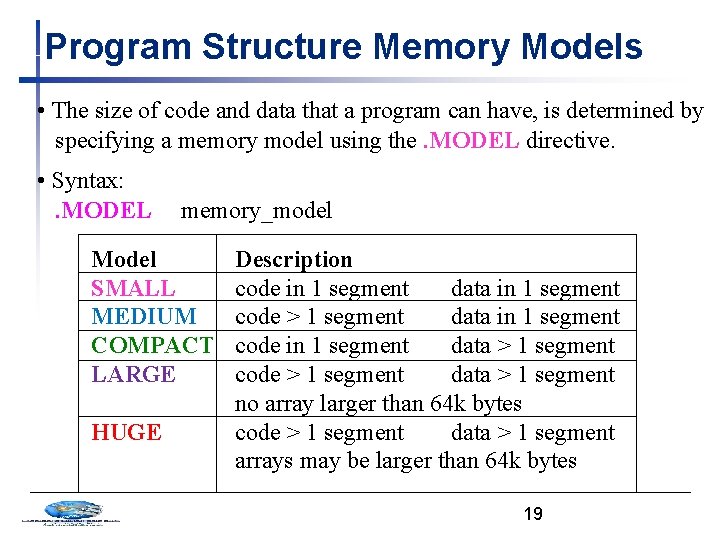
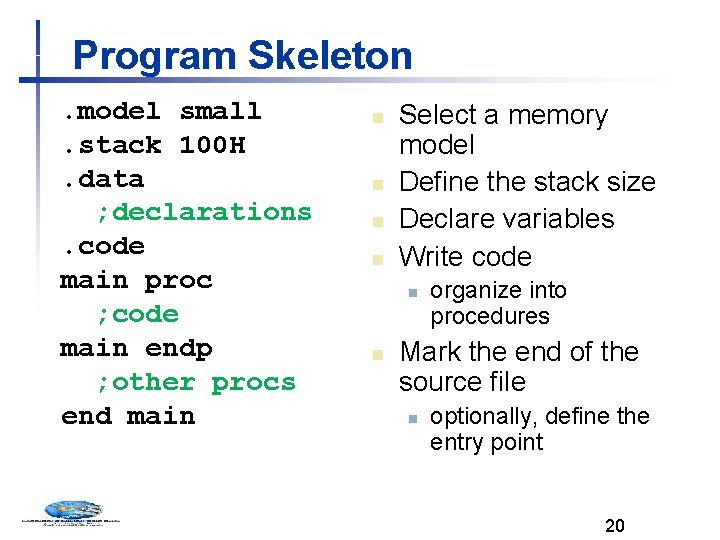
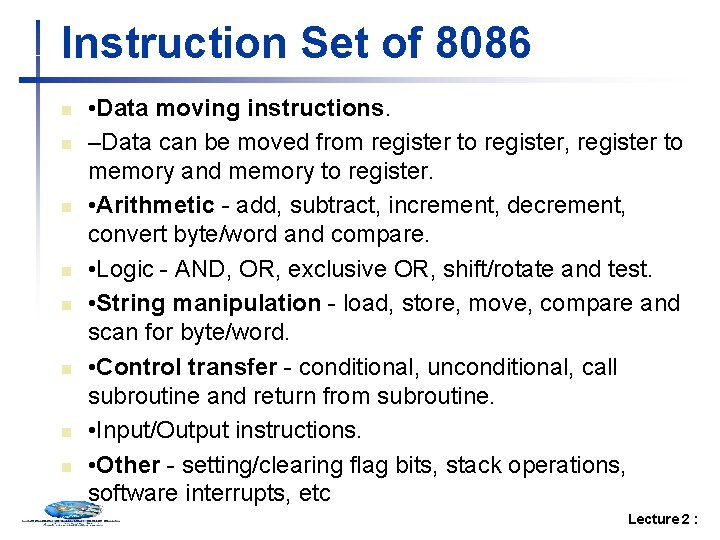
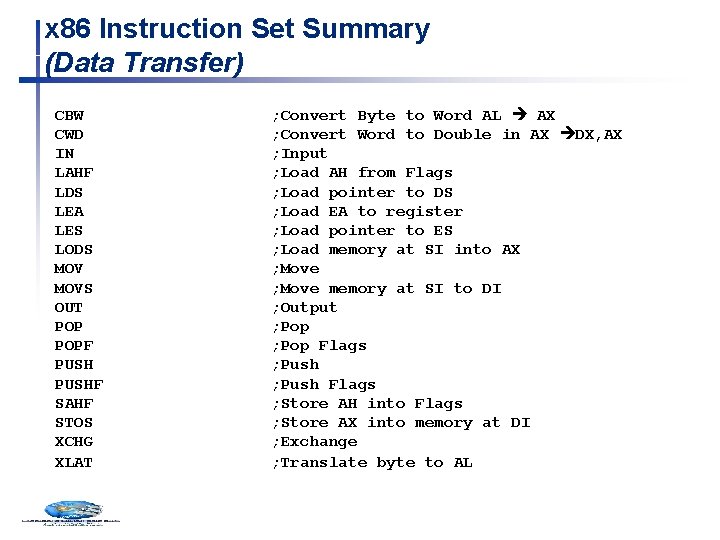
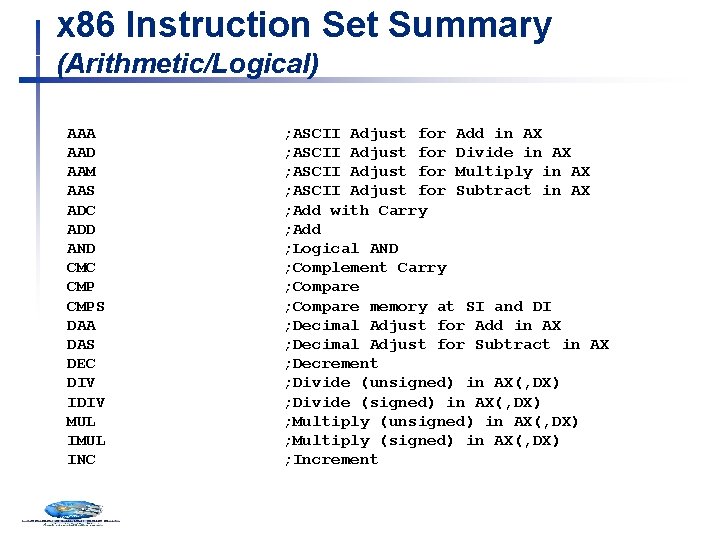
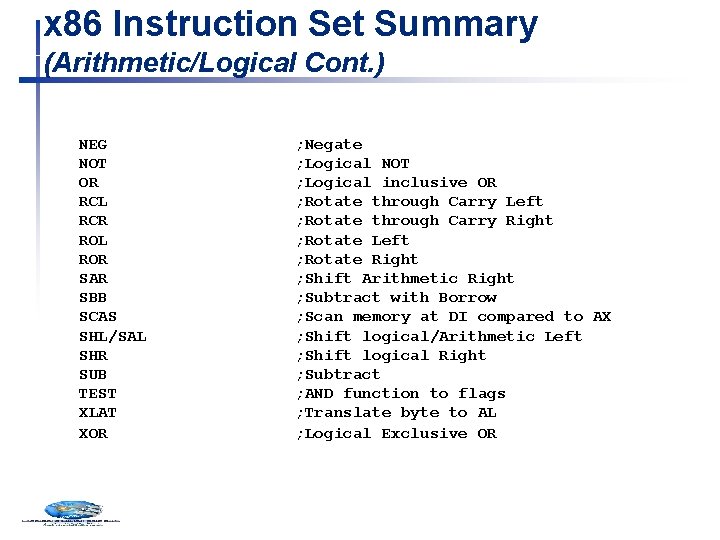
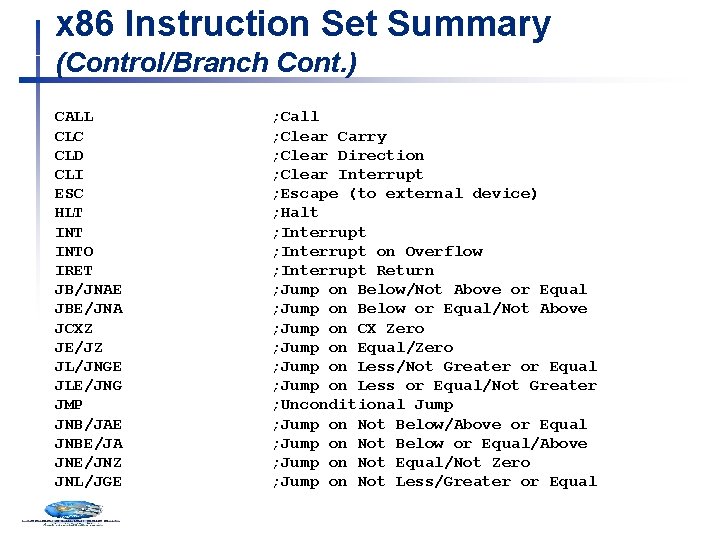
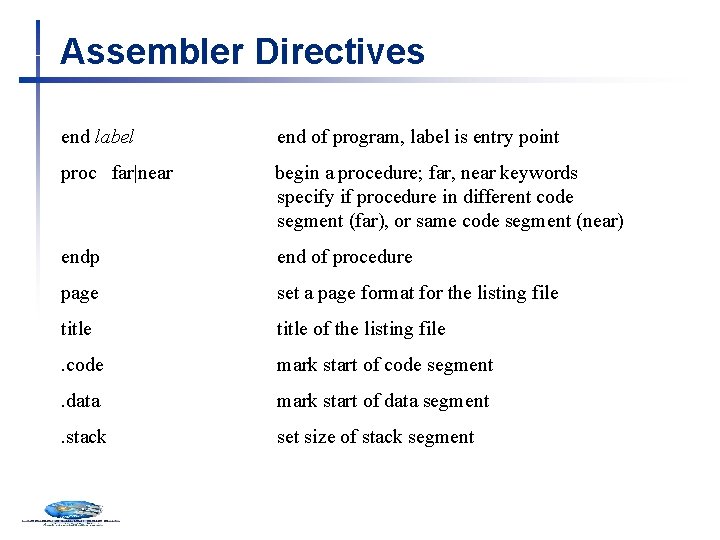
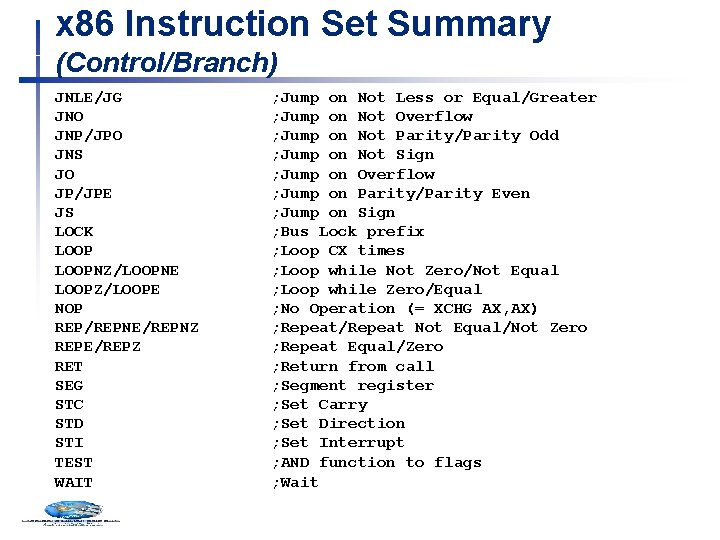
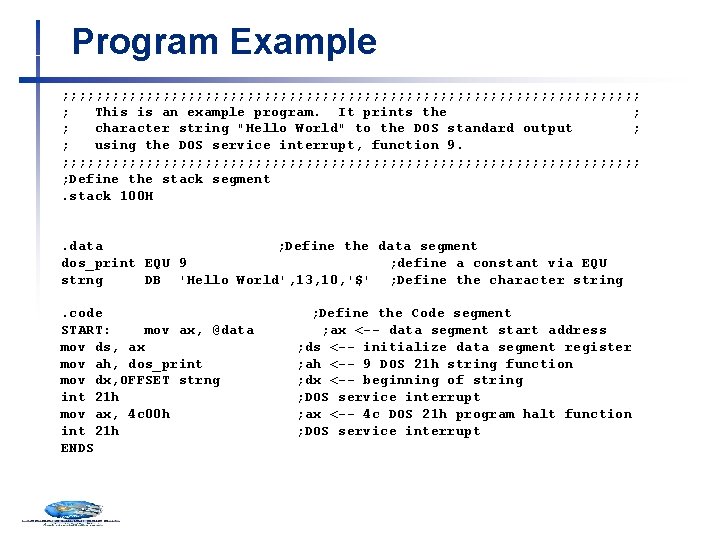
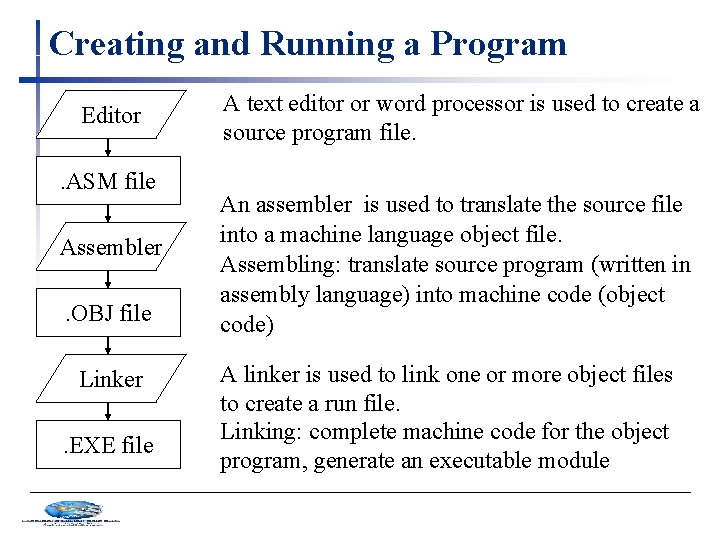
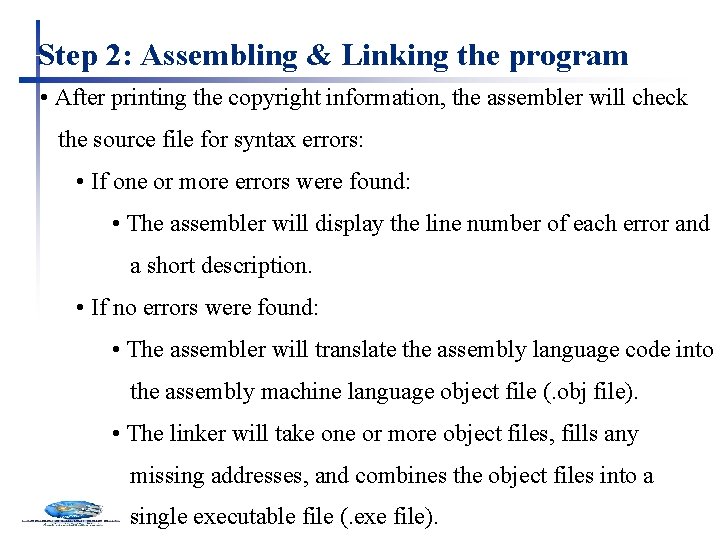
- Slides: 30
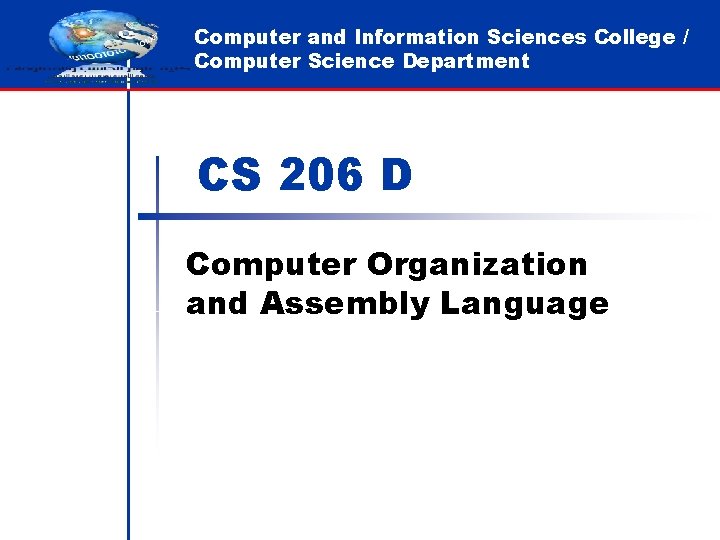
Computer and Information Sciences College / Computer Science Department CS 206 D Computer Organization and Assembly Language
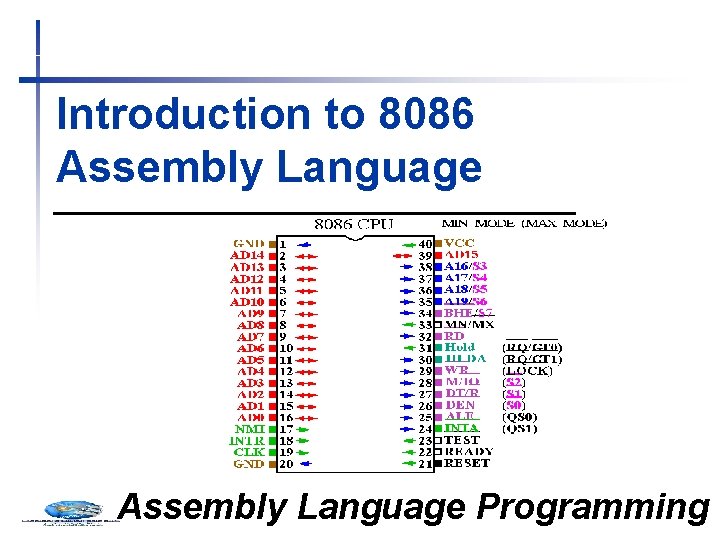
Introduction to 8086 Assembly Language Programming
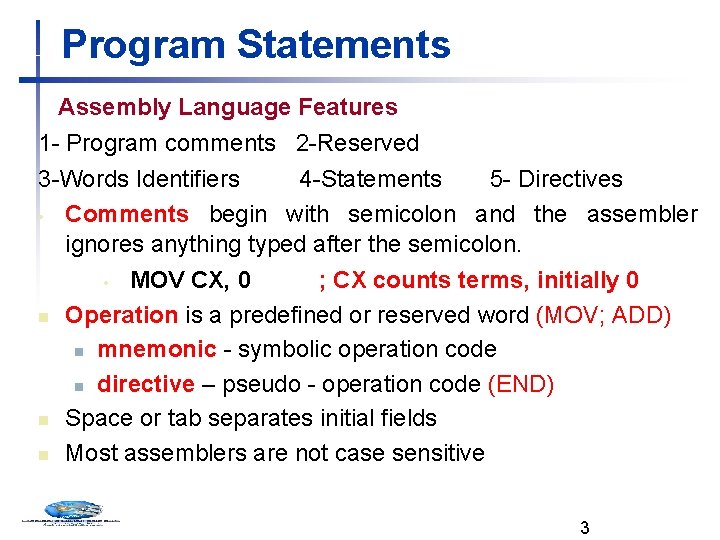
Program Statements Assembly Language Features 1 - Program comments 2 -Reserved 3 -Words Identifiers 4 -Statements 5 - Directives • Comments begin with semicolon and the assembler ignores anything typed after the semicolon. • MOV CX, 0 ; CX counts terms, initially 0 n Operation is a predefined or reserved word (MOV; ADD) n mnemonic - symbolic operation code n directive – pseudo - operation code (END) n Space or tab separates initial fields n Most assemblers are not case sensitive 3
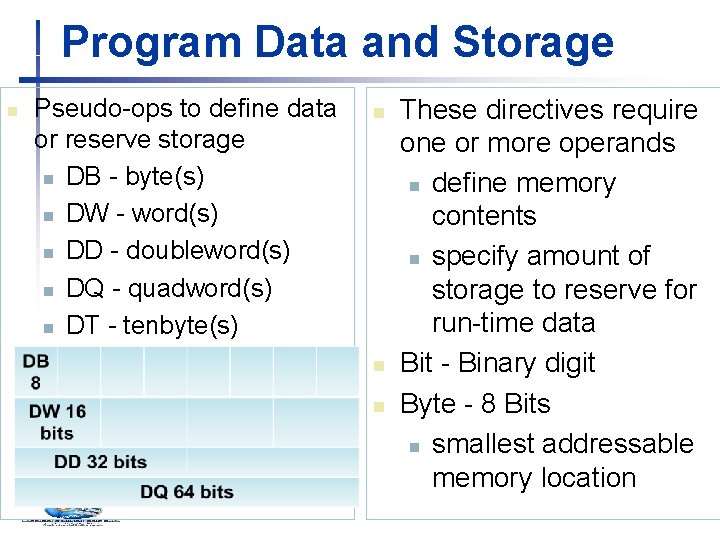
Program Data and Storage n Pseudo-ops to define data or reserve storage n DB - byte(s) n DW - word(s) n DD - doubleword(s) n DQ - quadword(s) n DT - tenbyte(s) n n n These directives require one or more operands n define memory contents n specify amount of storage to reserve for run-time data Bit - Binary digit Byte - 8 Bits n smallest addressable memory location
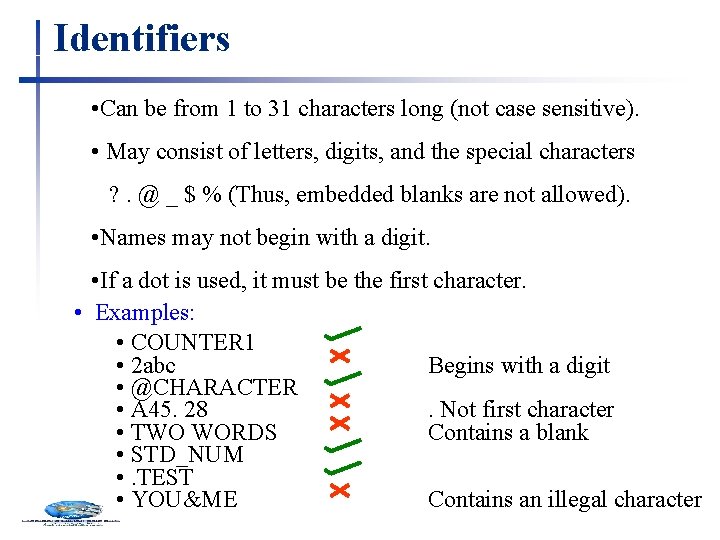
Identifiers • Can be from 1 to 31 characters long (not case sensitive). • May consist of letters, digits, and the special characters ? . @ _ $ % (Thus, embedded blanks are not allowed). • Names may not begin with a digit. • If a dot is used, it must be the first character. • Examples: • COUNTER 1 • 2 abc Begins with a digit • @CHARACTER • A 45. 28. Not first character • TWO WORDS Contains a blank • STD_NUM • . TEST • YOU&ME Contains an illegal character
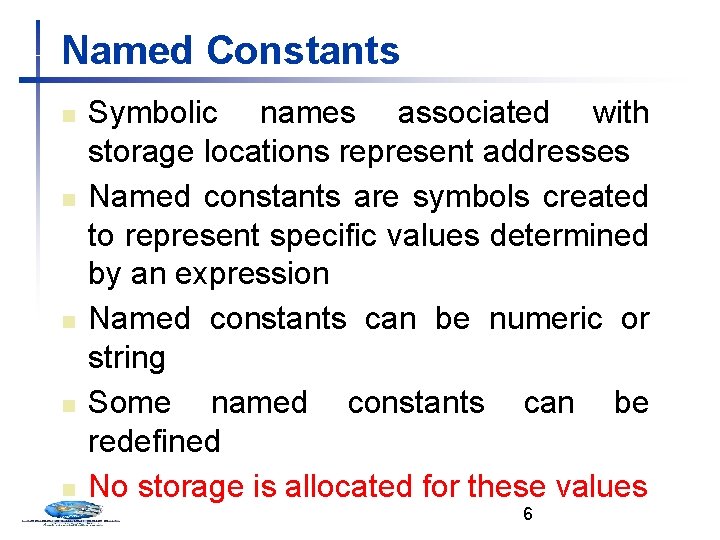
Named Constants n n n Symbolic names associated with storage locations represent addresses Named constants are symbols created to represent specific values determined by an expression Named constants can be numeric or string Some named constants can be redefined No storage is allocated for these values 6
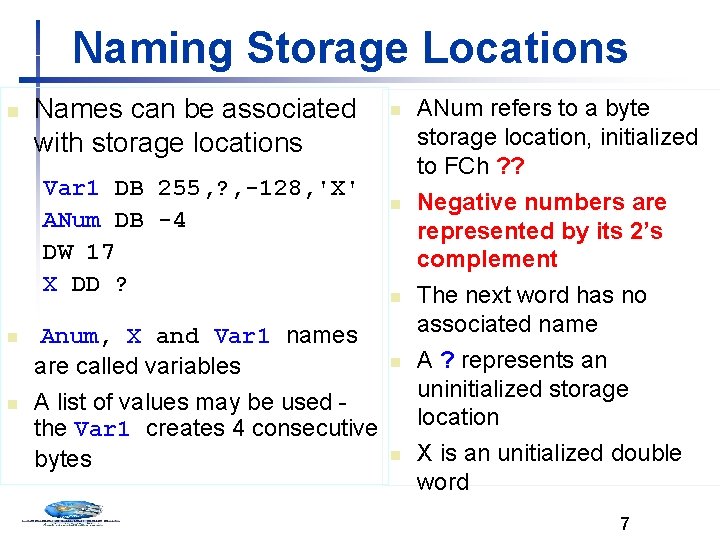
Naming Storage Locations n Names can be associated with storage locations Var 1 DB 255, ? , -128, 'X' ANum DB -4 DW 17 X DD ? n n Anum, X and Var 1 names are called variables A list of values may be used the Var 1 creates 4 consecutive bytes n n n ANum refers to a byte storage location, initialized to FCh ? ? Negative numbers are represented by its 2’s complement The next word has no associated name A ? represents an uninitialized storage location X is an unitialized double word 7
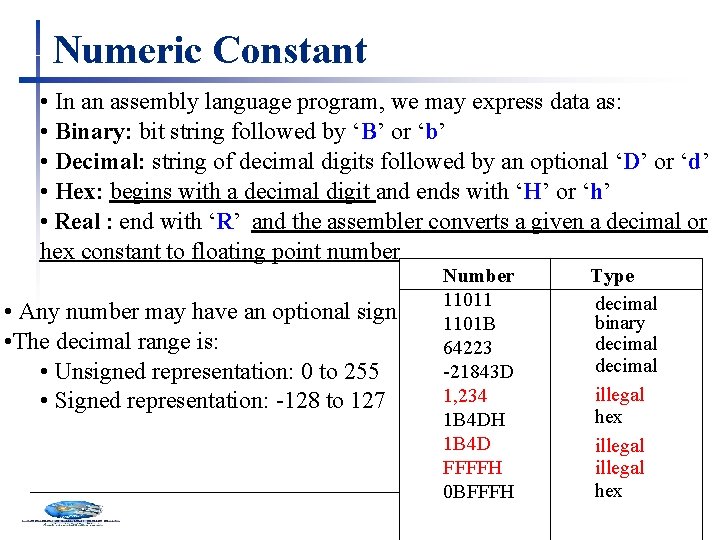
Numeric Constant • In an assembly language program, we may express data as: • Binary: bit string followed by ‘B’ or ‘b’ • Decimal: string of decimal digits followed by an optional ‘D’ or ‘d’ • Hex: begins with a decimal digit and ends with ‘H’ or ‘h’ • Real : end with ‘R’ and the assembler converts a given a decimal or hex constant to floating point number • Any number may have an optional sign. • The decimal range is: • Unsigned representation: 0 to 255 • Signed representation: -128 to 127 Number 11011 1101 B 64223 -21843 D 1, 234 1 B 4 DH 1 B 4 D FFFFH 0 BFFFH Type decimal binary decimal illegal hex
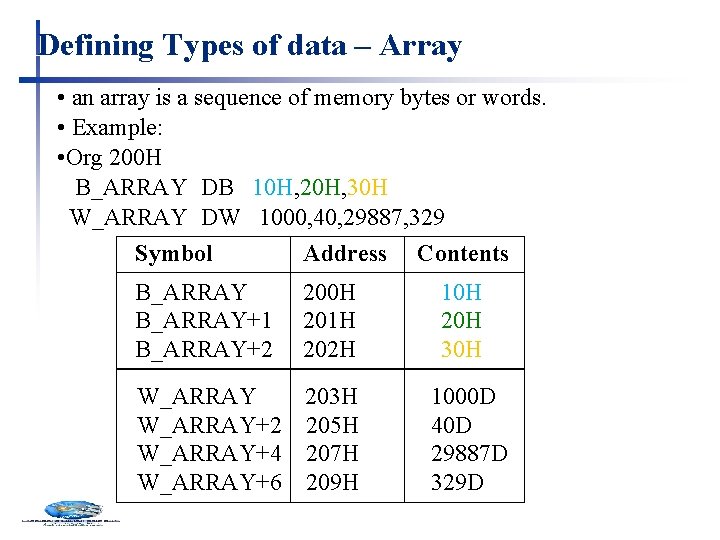
Defining Types of data – Array • an array is a sequence of memory bytes or words. • Example: • Org 200 H B_ARRAY DB 10 H, 20 H, 30 H W_ARRAY DW 1000, 40, 29887, 329 Symbol Address Contents B_ARRAY+1 B_ARRAY+2 200 H 201 H 202 H W_ARRAY 203 H W_ARRAY+2 205 H W_ARRAY+4 207 H W_ARRAY+6 209 H 10 H 20 H 30 H 1000 D 40 D 29887 D 329 D
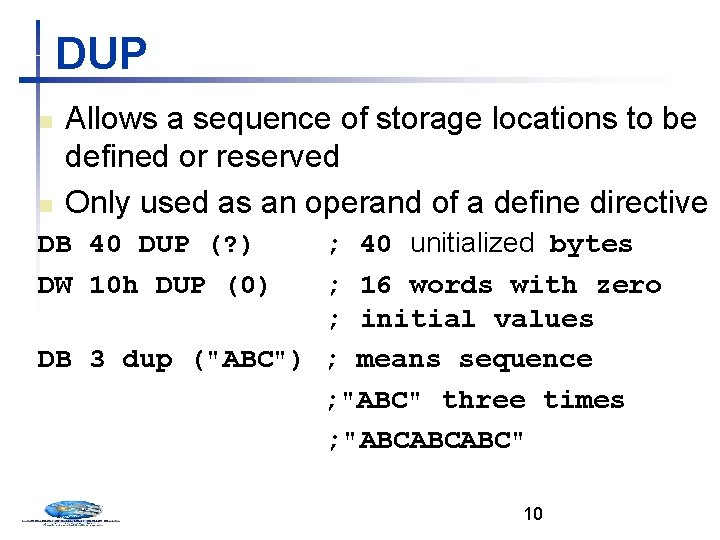
DUP n n Allows a sequence of storage locations to be defined or reserved Only used as an operand of a define directive ; 40 unitialized bytes ; 16 words with zero ; initial values DB 3 dup ("ABC") ; means sequence ; "ABC" three times ; "ABCABCABC" DB 40 DUP (? ) DW 10 h DUP (0) 10
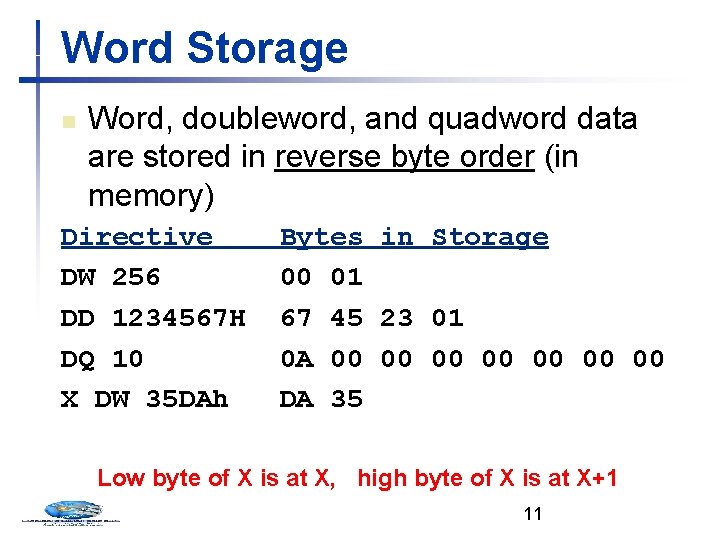
Word Storage n Word, doubleword, and quadword data are stored in reverse byte order (in memory) Directive DW 256 DD 1234567 H DQ 10 X DW 35 DAh Bytes in Storage 00 01 67 45 23 01 0 A 00 00 DA 35 Low byte of X is at X, high byte of X is at X+1 11
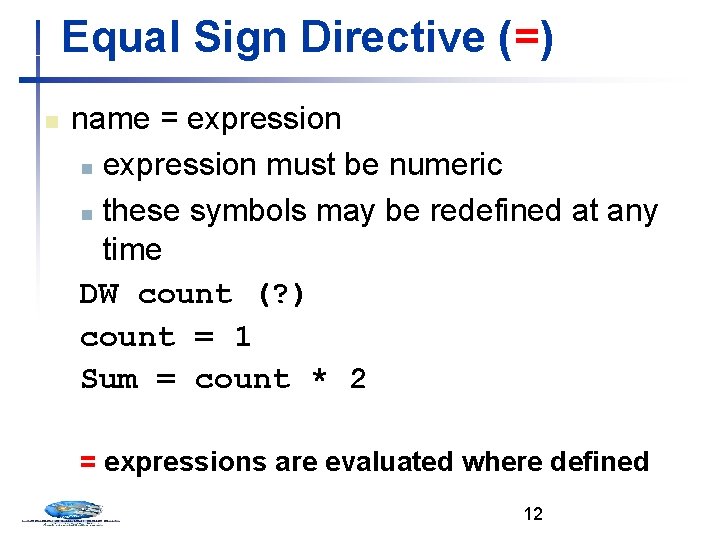
Equal Sign Directive (=) n name = expression n expression must be numeric n these symbols may be redefined at any time DW count (? ) count = 1 Sum = count * 2 = expressions are evaluated where defined 12
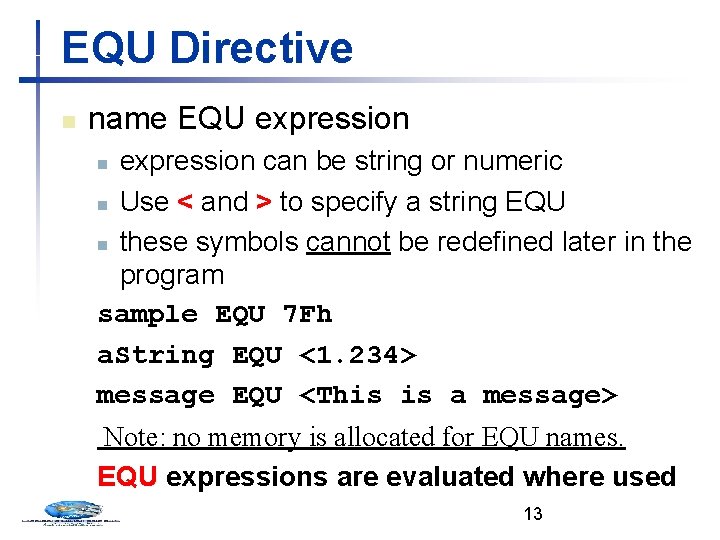
EQU Directive n name EQU expression can be string or numeric n Use < and > to specify a string EQU n these symbols cannot be redefined later in the program sample EQU 7 Fh a. String EQU <1. 234> message EQU <This is a message> n Note: no memory is allocated for EQU names. EQU expressions are evaluated where used 13
![Statements Both instructions and directives have up to four fields identifier operation Statements • Both instructions and directives have up to four fields: [identifier ] operation](https://slidetodoc.com/presentation_image_h/2222071b3790402be4832ac38f7c9857/image-14.jpg)
Statements • Both instructions and directives have up to four fields: [identifier ] operation [operand(s)] [comment] • [Name Fields are optional] • At least one blank or tab character must separate the fields. • The fields do not have to be aligned in a particular column, but they must appear in the above order. • An example of an instruction: START: MOV CX, 5 ; initialize counter • An example of an assembler directive: MAIN PROC
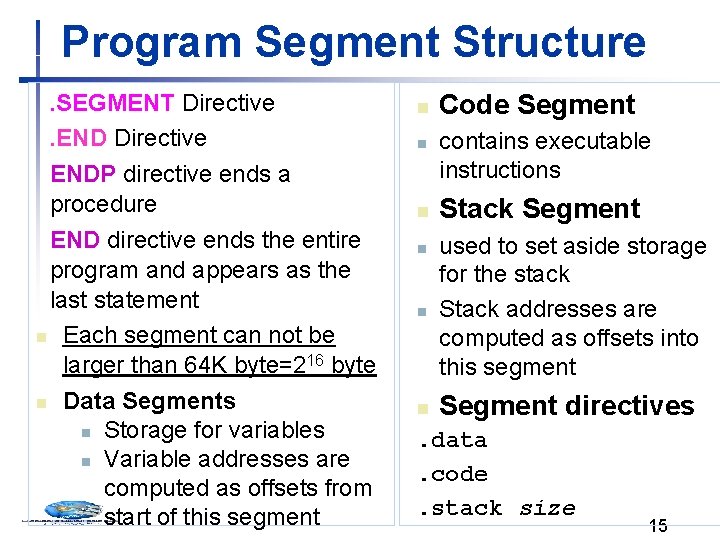
Program Segment Structure. SEGMENT Directive. END Directive ENDP directive ends a procedure END directive ends the entire program and appears as the last statement n Each segment can not be larger than 64 K byte=216 byte n Data Segments n Storage for variables n Variable addresses are computed as offsets from start of this segment n n n Code Segment contains executable instructions Stack Segment n used to set aside storage for the stack Stack addresses are computed as offsets into this segment n Segment directives n . data. code. stack size 15
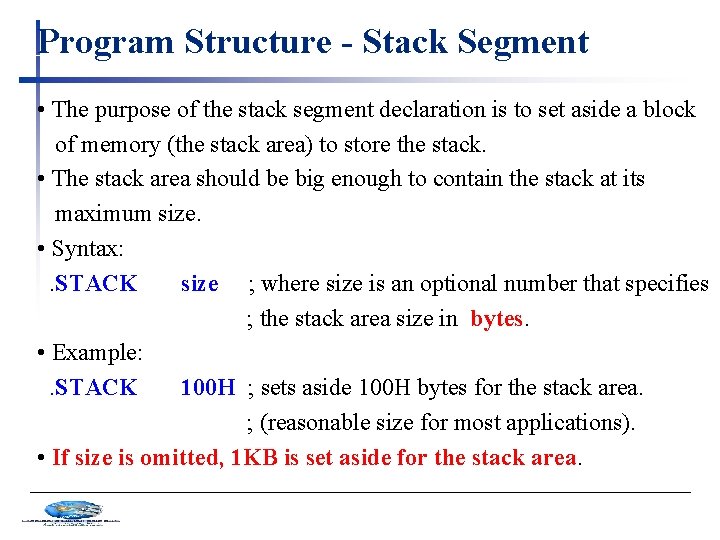
Program Structure - Stack Segment • The purpose of the stack segment declaration is to set aside a block of memory (the stack area) to store the stack. • The stack area should be big enough to contain the stack at its maximum size. • Syntax: . STACK size ; where size is an optional number that specifies ; the stack area size in bytes. • Example: . STACK 100 H ; sets aside 100 H bytes for the stack area. ; (reasonable size for most applications). • If size is omitted, 1 KB is set aside for the stack area.
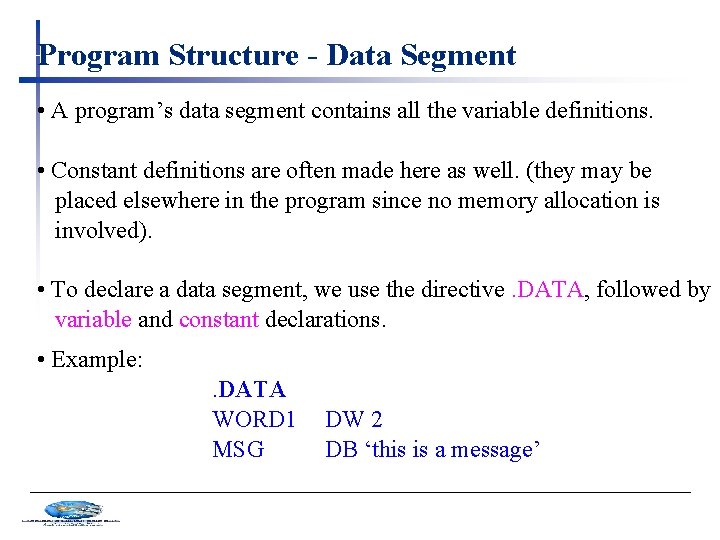
Program Structure - Data Segment • A program’s data segment contains all the variable definitions. • Constant definitions are often made here as well. (they may be placed elsewhere in the program since no memory allocation is involved). • To declare a data segment, we use the directive. DATA, followed by variable and constant declarations. • Example: . DATA WORD 1 MSG DW 2 DB ‘this is a message’
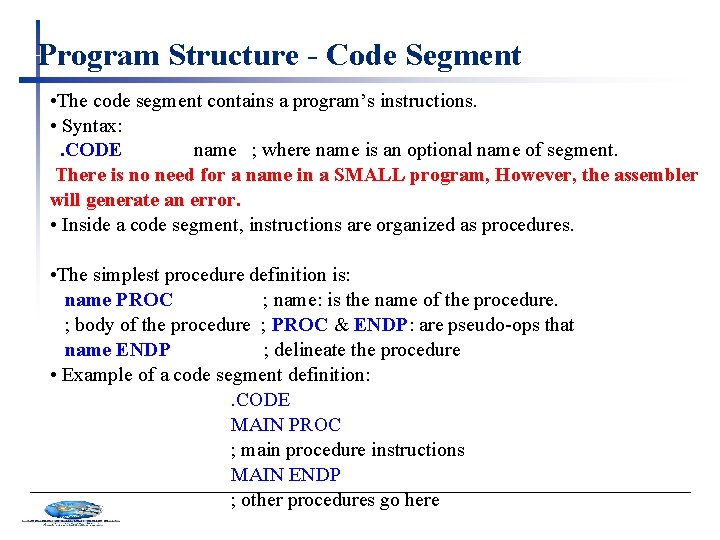
Program Structure - Code Segment • The code segment contains a program’s instructions. • Syntax: . CODE name ; where name is an optional name of segment. There is no need for a name in a SMALL program, However, the assembler will generate an error. • Inside a code segment, instructions are organized as procedures. • The simplest procedure definition is: name PROC ; name: is the name of the procedure. ; body of the procedure ; PROC & ENDP: are pseudo-ops that name ENDP ; delineate the procedure • Example of a code segment definition: . CODE MAIN PROC ; main procedure instructions MAIN ENDP ; other procedures go here
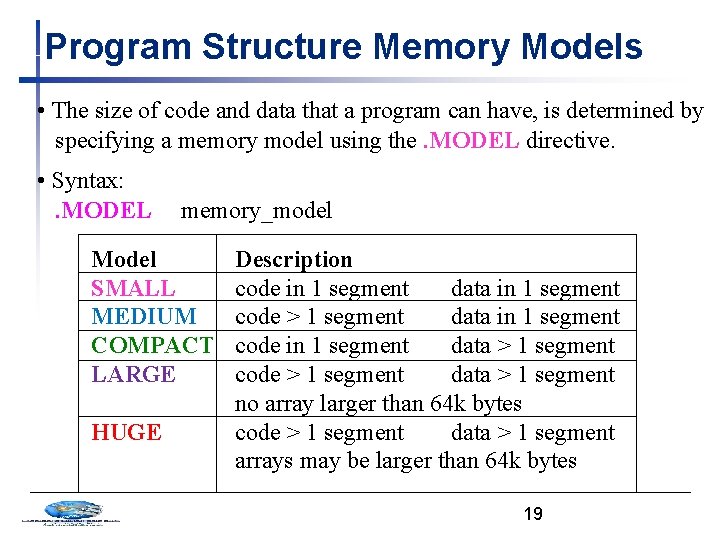
Program Structure Memory Models • The size of code and data that a program can have, is determined by specifying a memory model using the. MODEL directive. • Syntax: . MODEL memory_model Model SMALL MEDIUM COMPACT LARGE HUGE Description code in 1 segment data in 1 segment code > 1 segment data in 1 segment code in 1 segment data > 1 segment code > 1 segment data > 1 segment no array larger than 64 k bytes code > 1 segment data > 1 segment arrays may be larger than 64 k bytes 19
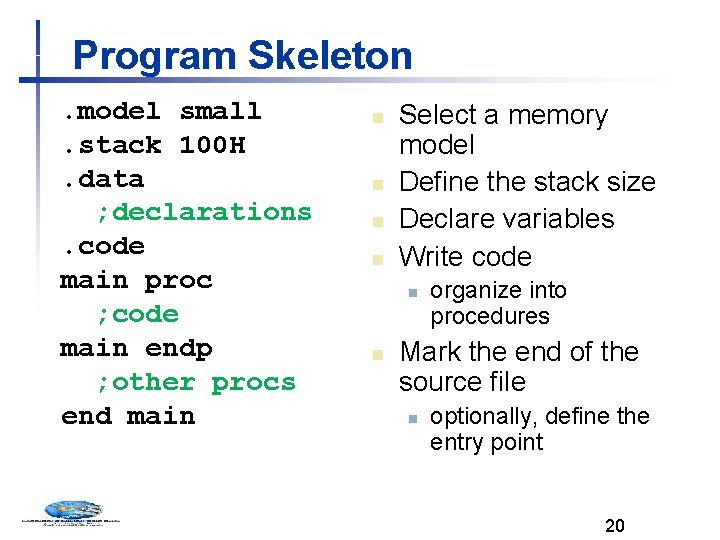
Program Skeleton. model small. stack 100 H. data ; declarations. code main proc ; code main endp ; other procs end main n n Select a memory model Define the stack size Declare variables Write code n n organize into procedures Mark the end of the source file n optionally, define the entry point 20
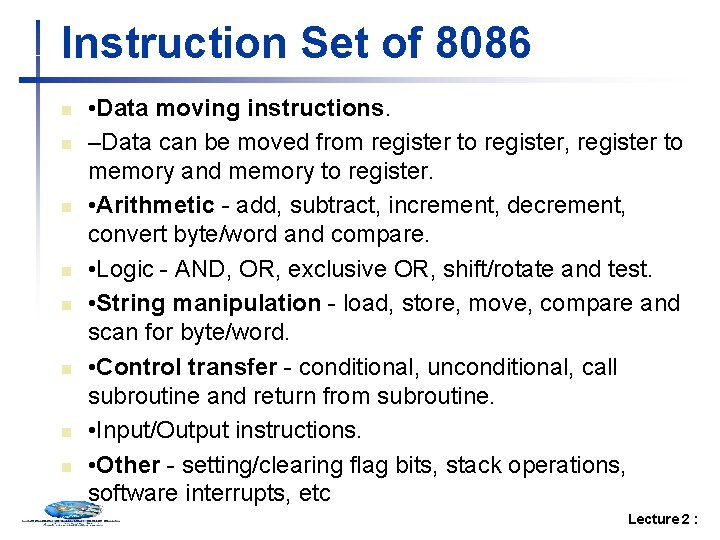
Instruction Set of 8086 n n n n • Data moving instructions. –Data can be moved from register to register, register to memory and memory to register. • Arithmetic - add, subtract, increment, decrement, convert byte/word and compare. • Logic - AND, OR, exclusive OR, shift/rotate and test. • String manipulation - load, store, move, compare and scan for byte/word. • Control transfer - conditional, unconditional, call subroutine and return from subroutine. • Input/Output instructions. • Other - setting/clearing flag bits, stack operations, software interrupts, etc Lecture 2 :
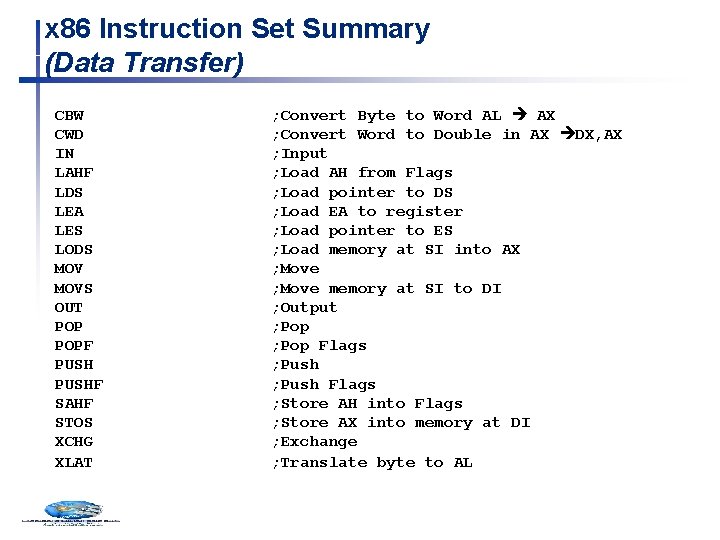
x 86 Instruction Set Summary (Data Transfer) CBW CWD IN LAHF LDS LEA LES LODS MOVS OUT POPF PUSHF SAHF STOS XCHG XLAT ; Convert Byte to Word AL AX ; Convert Word to Double in AX DX, AX ; Input ; Load AH from Flags ; Load pointer to DS ; Load EA to register ; Load pointer to ES ; Load memory at SI into AX ; Move memory at SI to DI ; Output ; Pop Flags ; Push Flags ; Store AH into Flags ; Store AX into memory at DI ; Exchange ; Translate byte to AL
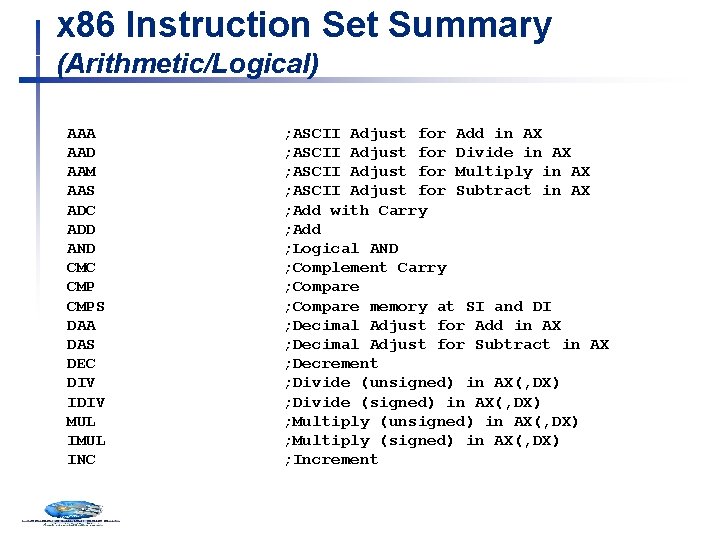
x 86 Instruction Set Summary (Arithmetic/Logical) AAA AAD AAM AAS ADC ADD AND CMC CMPS DAA DAS DEC DIV IDIV MUL INC ; ASCII Adjust for Add in AX ; ASCII Adjust for Divide in AX ; ASCII Adjust for Multiply in AX ; ASCII Adjust for Subtract in AX ; Add with Carry ; Add ; Logical AND ; Complement Carry ; Compare memory at SI and DI ; Decimal Adjust for Add in AX ; Decimal Adjust for Subtract in AX ; Decrement ; Divide (unsigned) in AX(, DX) ; Divide (signed) in AX(, DX) ; Multiply (unsigned) in AX(, DX) ; Multiply (signed) in AX(, DX) ; Increment
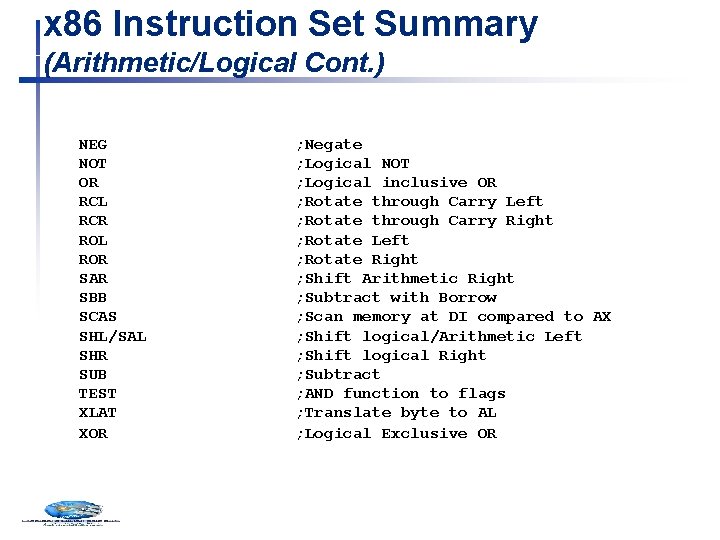
x 86 Instruction Set Summary (Arithmetic/Logical Cont. ) NEG NOT OR RCL RCR ROL ROR SAR SBB SCAS SHL/SAL SHR SUB TEST XLAT XOR ; Negate ; Logical NOT ; Logical inclusive OR ; Rotate through Carry Left ; Rotate through Carry Right ; Rotate Left ; Rotate Right ; Shift Arithmetic Right ; Subtract with Borrow ; Scan memory at DI compared to AX ; Shift logical/Arithmetic Left ; Shift logical Right ; Subtract ; AND function to flags ; Translate byte to AL ; Logical Exclusive OR
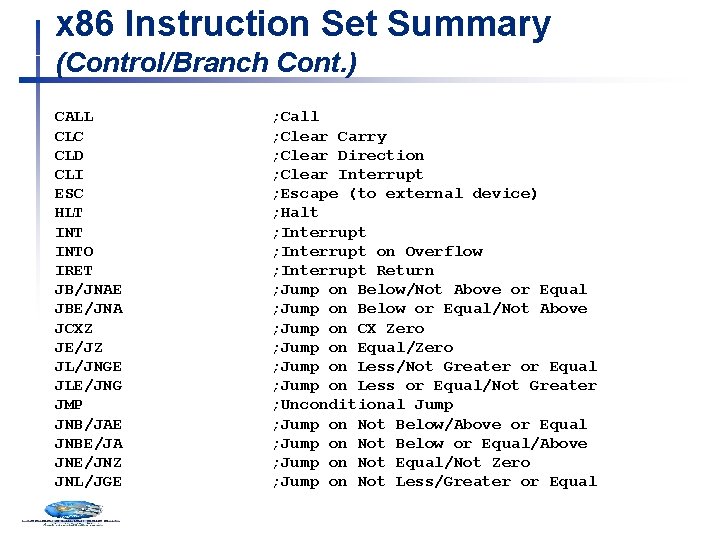
x 86 Instruction Set Summary (Control/Branch Cont. ) CALL CLC CLD CLI ESC HLT INTO IRET JB/JNAE JBE/JNA JCXZ JE/JZ JL/JNGE JLE/JNG JMP JNB/JAE JNBE/JA JNE/JNZ JNL/JGE ; Call ; Clear Carry ; Clear Direction ; Clear Interrupt ; Escape (to external device) ; Halt ; Interrupt on Overflow ; Interrupt Return ; Jump on Below/Not Above or Equal ; Jump on Below or Equal/Not Above ; Jump on CX Zero ; Jump on Equal/Zero ; Jump on Less/Not Greater or Equal ; Jump on Less or Equal/Not Greater ; Unconditional Jump ; Jump on Not Below/Above or Equal ; Jump on Not Below or Equal/Above ; Jump on Not Equal/Not Zero ; Jump on Not Less/Greater or Equal
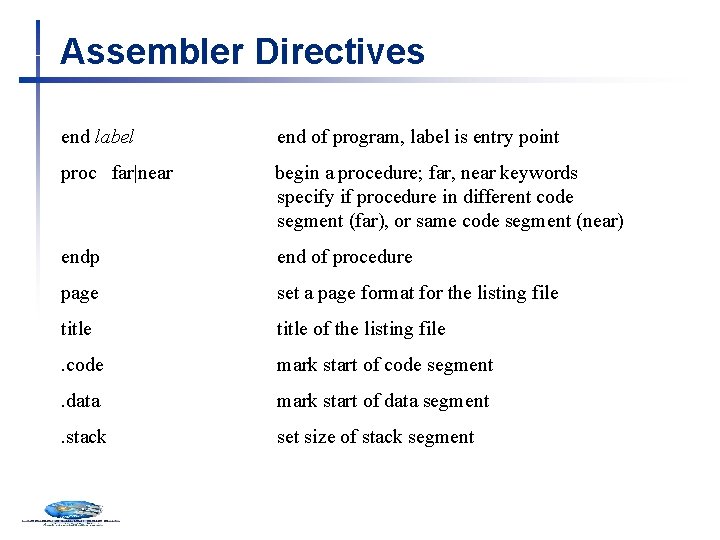
Assembler Directives end label end of program, label is entry point proc far|near begin a procedure; far, near keywords specify if procedure in different code segment (far), or same code segment (near) endp end of procedure page set a page format for the listing file title of the listing file . code mark start of code segment . data mark start of data segment . stack set size of stack segment
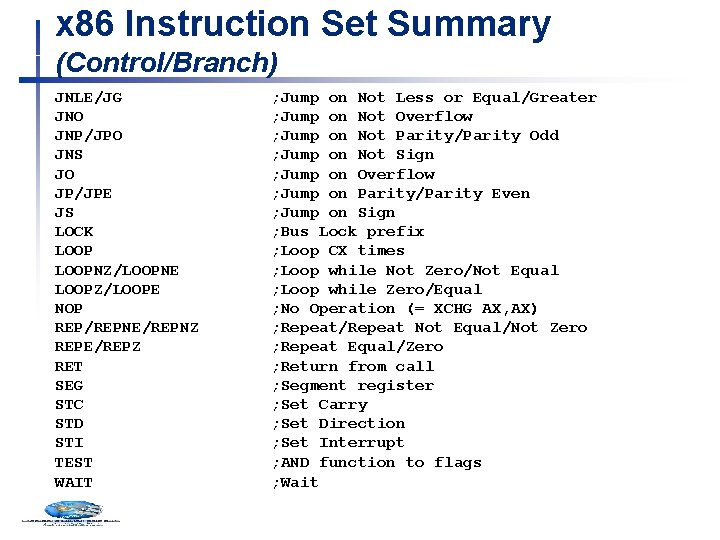
x 86 Instruction Set Summary (Control/Branch) JNLE/JG JNO JNP/JPO JNS JO JP/JPE JS LOCK LOOPNZ/LOOPNE LOOPZ/LOOPE NOP REP/REPNE/REPNZ REPE/REPZ RET SEG STC STD STI TEST WAIT ; Jump on Not Less or Equal/Greater ; Jump on Not Overflow ; Jump on Not Parity/Parity Odd ; Jump on Not Sign ; Jump on Overflow ; Jump on Parity/Parity Even ; Jump on Sign ; Bus Lock prefix ; Loop CX times ; Loop while Not Zero/Not Equal ; Loop while Zero/Equal ; No Operation (= XCHG AX, AX) ; Repeat/Repeat Not Equal/Not Zero ; Repeat Equal/Zero ; Return from call ; Segment register ; Set Carry ; Set Direction ; Set Interrupt ; AND function to flags ; Wait
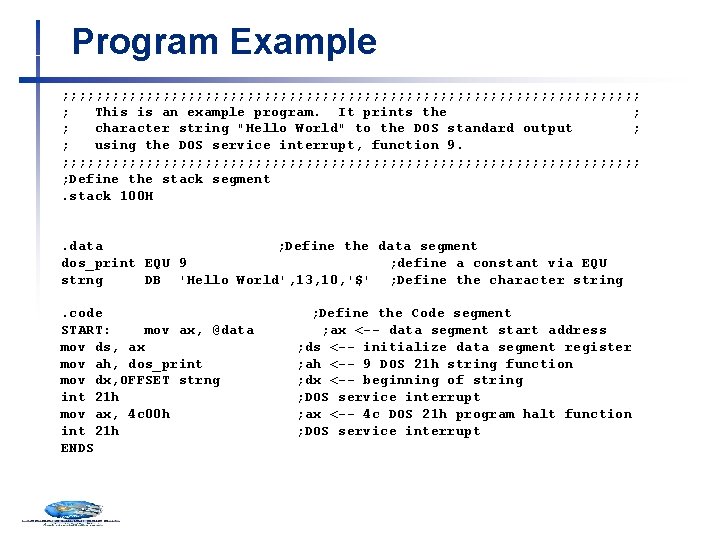
Program Example ; ; ; ; ; ; ; ; ; ; ; ; ; ; ; ; ; ; This is an example program. It prints the ; ; character string "Hello World" to the DOS standard output ; ; using the DOS service interrupt, function 9. ; ; ; ; ; ; ; ; ; ; ; ; ; ; ; ; ; ; Define the stack segment. stack 100 H. data ; Define the data segment dos_print EQU 9 ; define a constant via EQU strng DB 'Hello World', 13, 10, '$' ; Define the character string. code START: mov ax, @data mov ds, ax mov ah, dos_print mov dx, OFFSET strng int 21 h mov ax, 4 c 00 h int 21 h ENDS ; Define the Code segment ; ax <-- data segment start address ; ds <-- initialize data segment register ; ah <-- 9 DOS 21 h string function ; dx <-- beginning of string ; DOS service interrupt ; ax <-- 4 c DOS 21 h program halt function ; DOS service interrupt
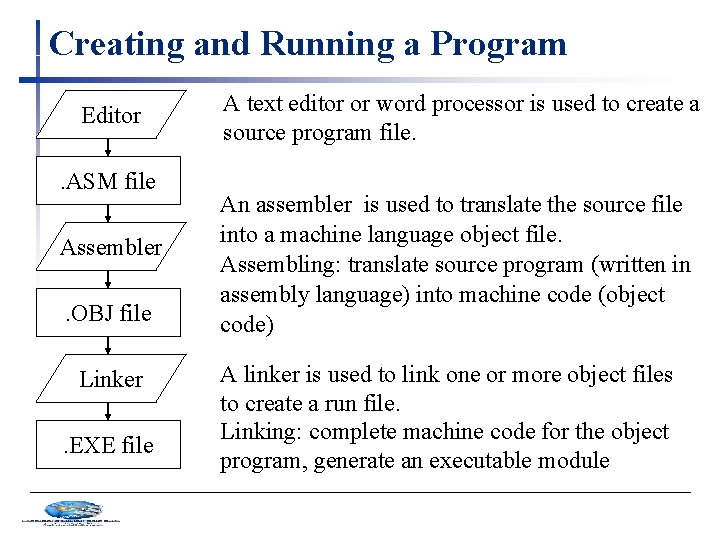
Creating and Running a Program Editor. ASM file Assembler. OBJ file Linker. EXE file A text editor or word processor is used to create a source program file. An assembler is used to translate the source file into a machine language object file. Assembling: translate source program (written in assembly language) into machine code (object code) A linker is used to link one or more object files to create a run file. Linking: complete machine code for the object program, generate an executable module
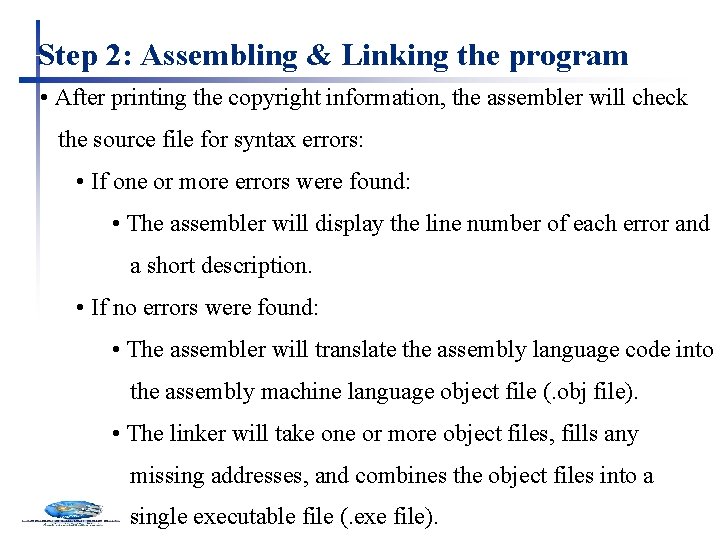
Step 2: Assembling & Linking the program • After printing the copyright information, the assembler will check the source file for syntax errors: • If one or more errors were found: • The assembler will display the line number of each error and a short description. • If no errors were found: • The assembler will translate the assembly language code into the assembly machine language object file (. obj file). • The linker will take one or more object files, fills any missing addresses, and combines the object files into a single executable file (. exe file).