CSS 342 DATA S TR U CTURES ALGO
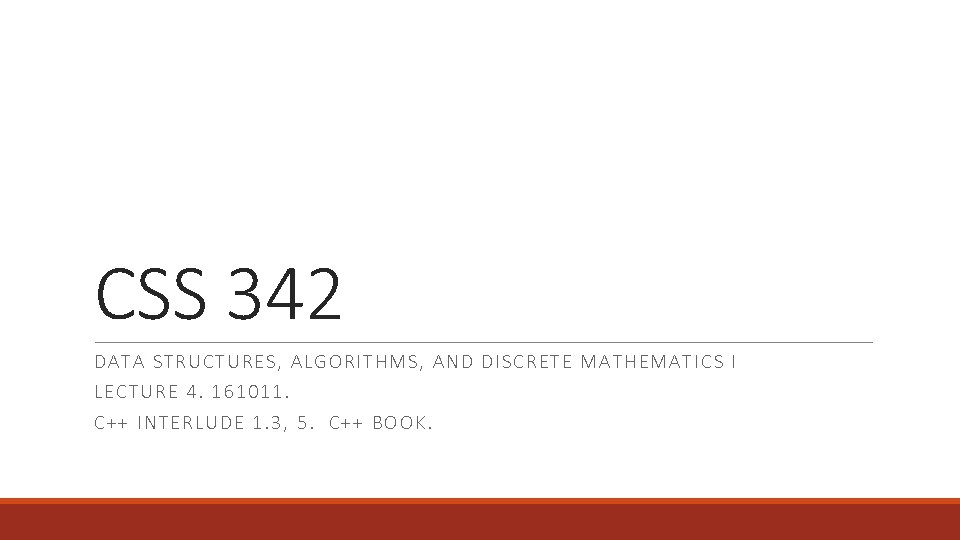
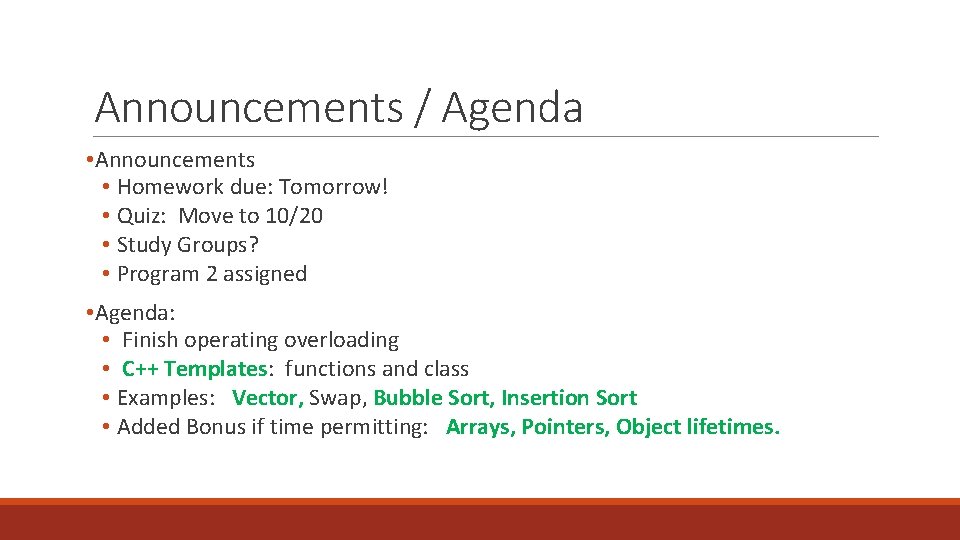
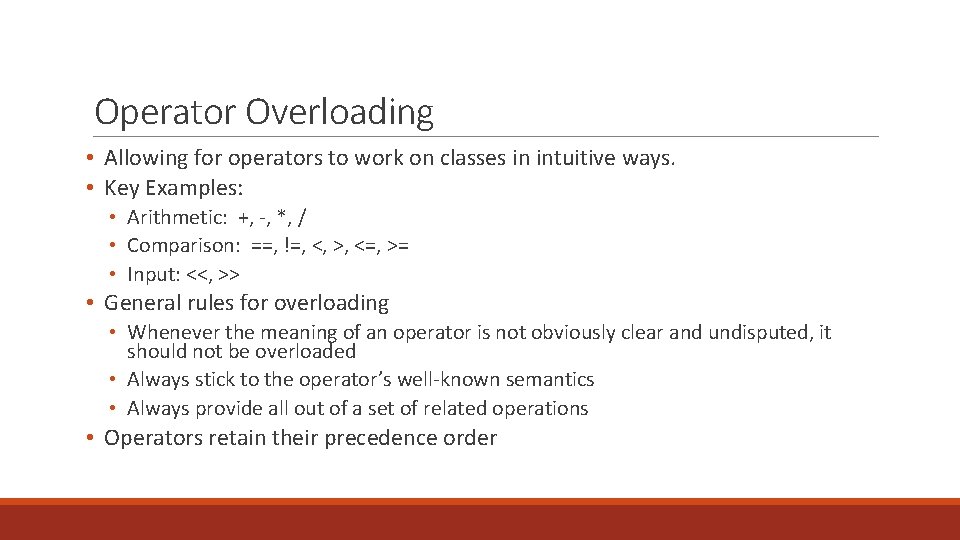
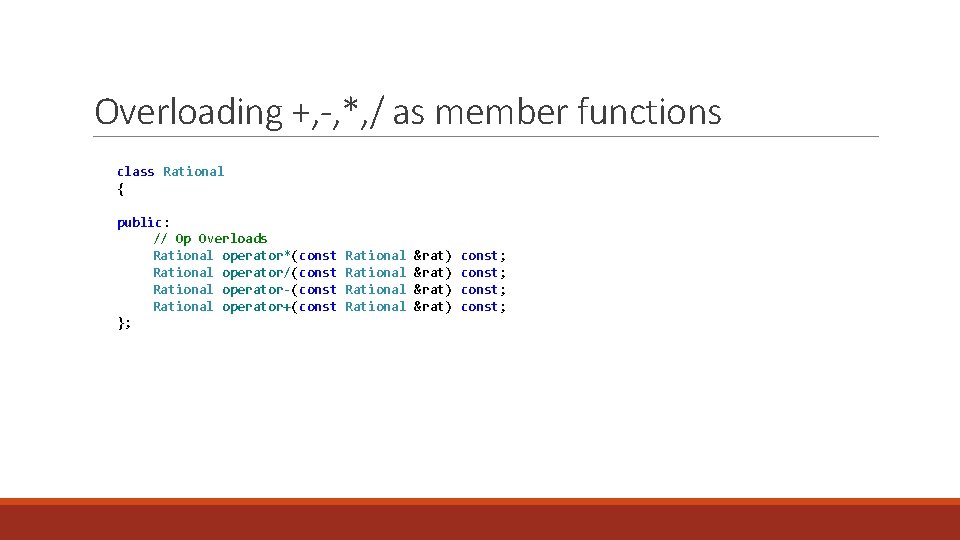
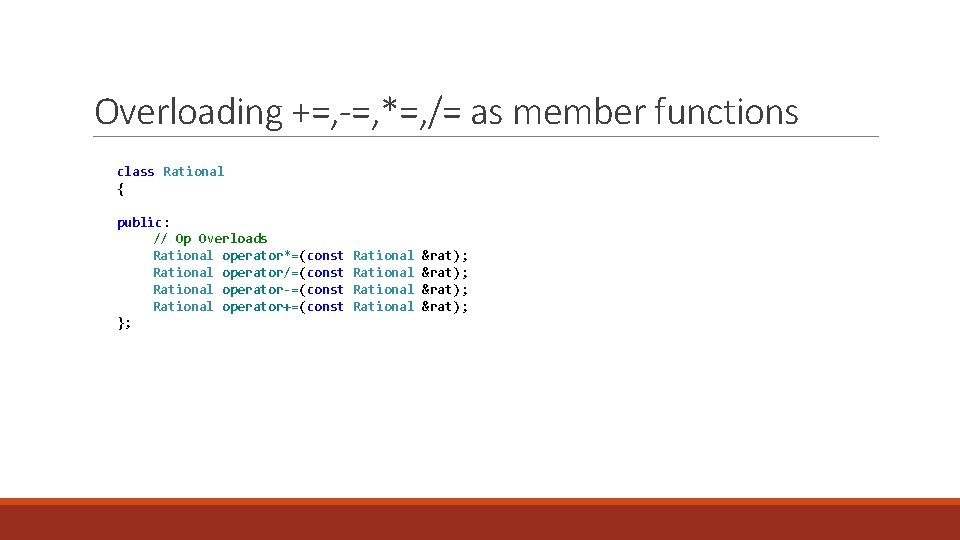
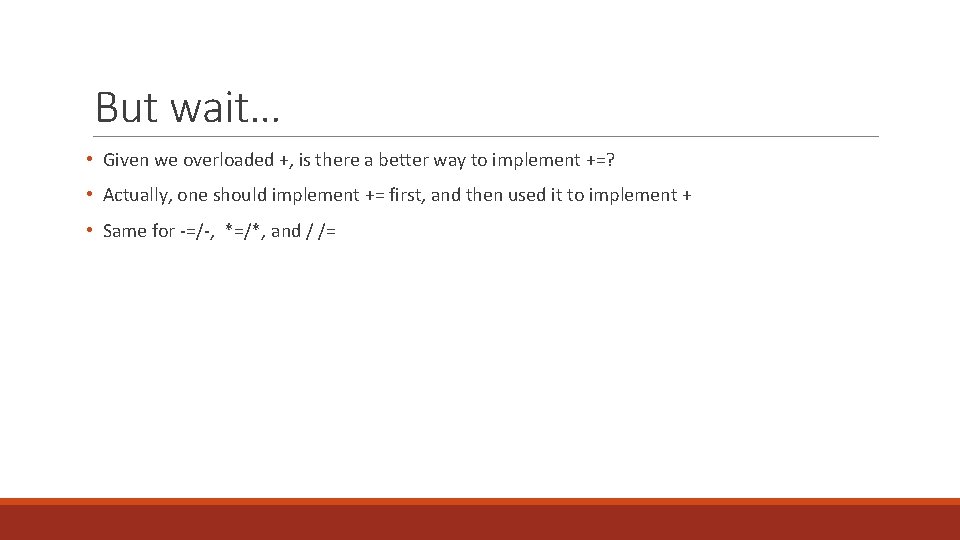
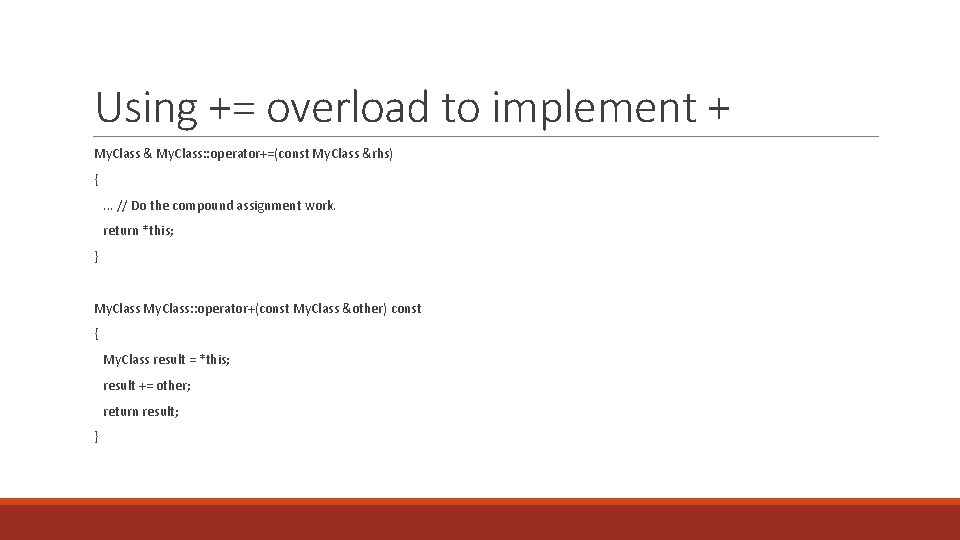
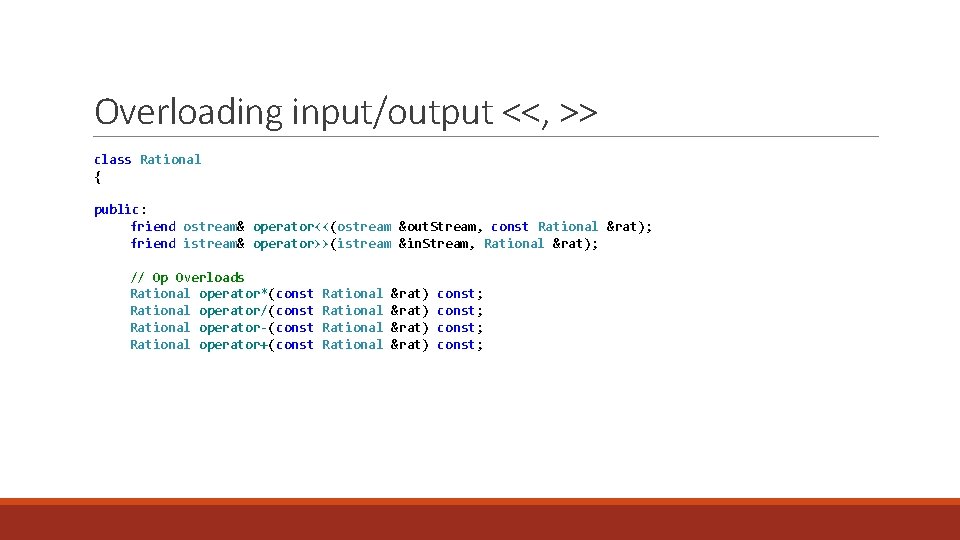
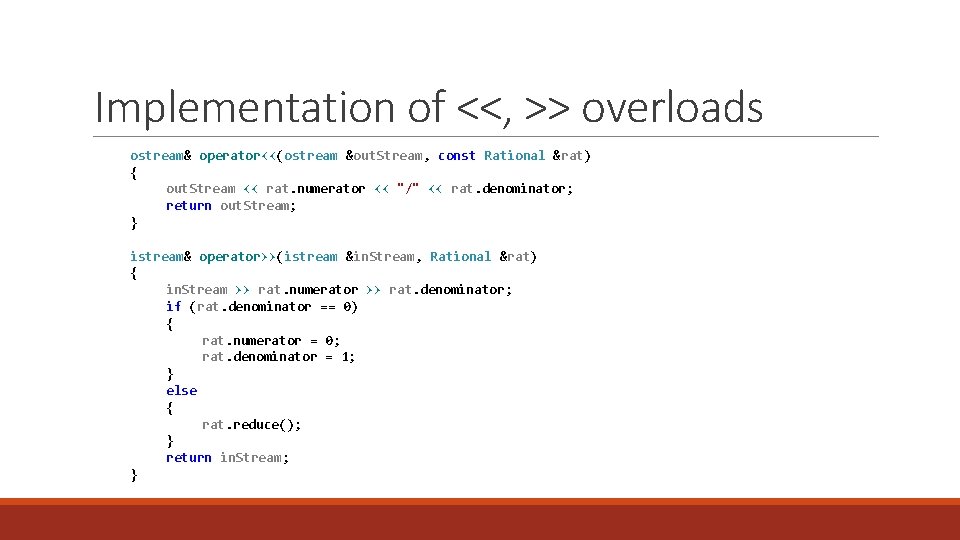
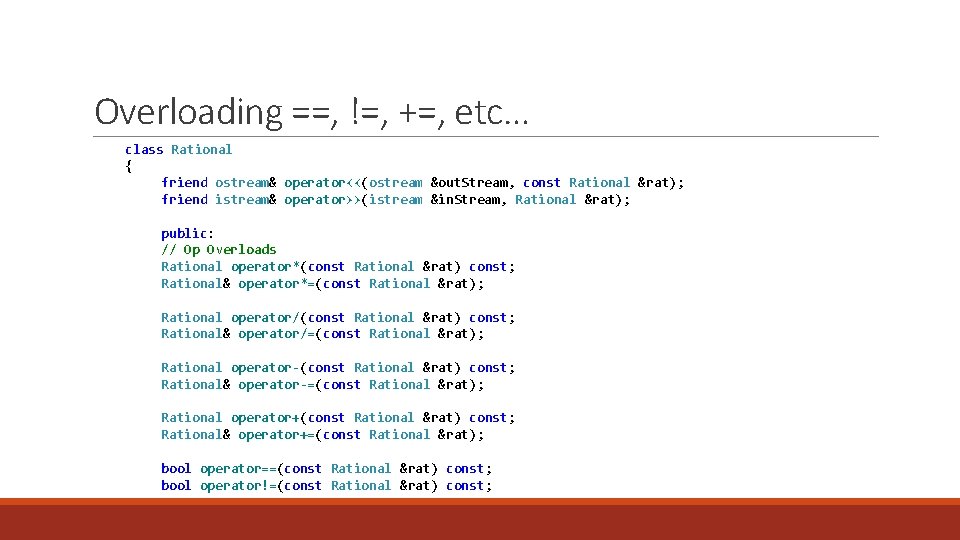
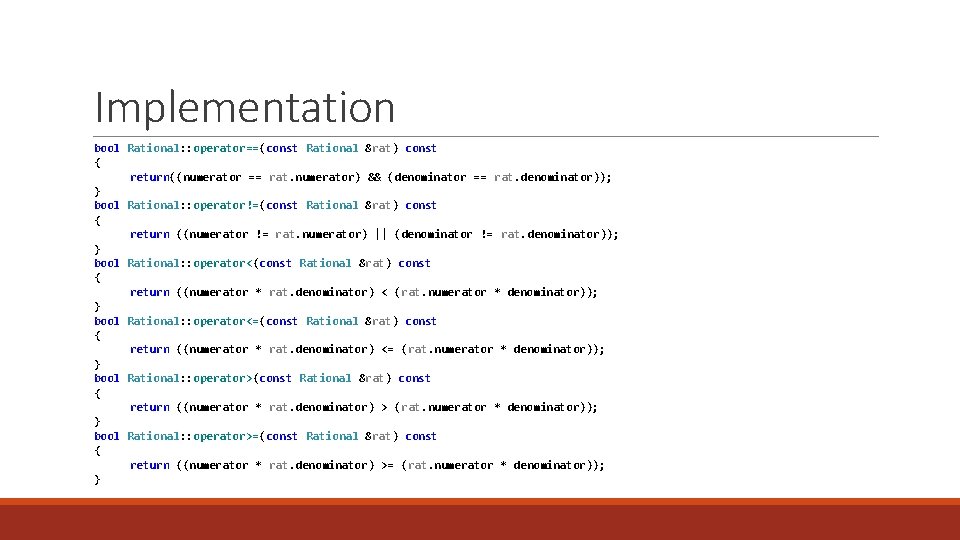
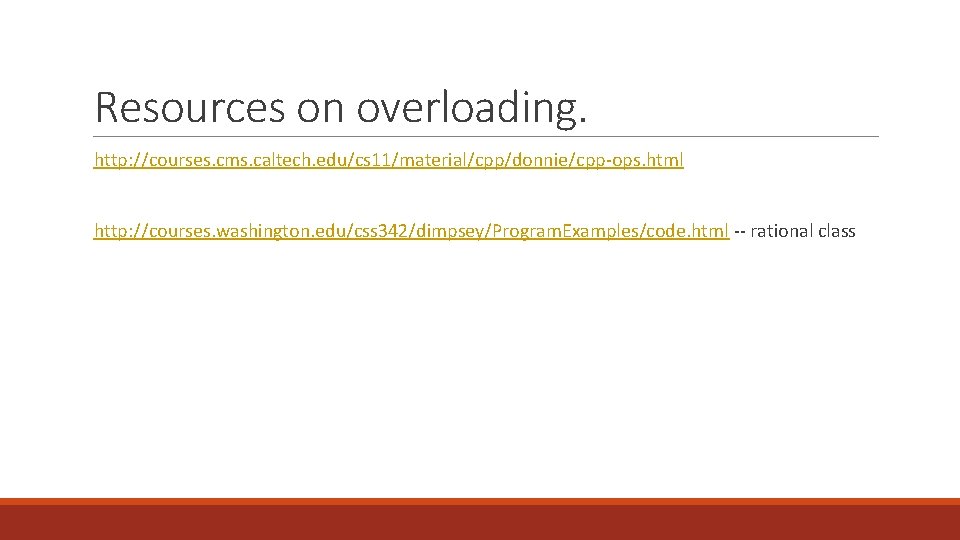
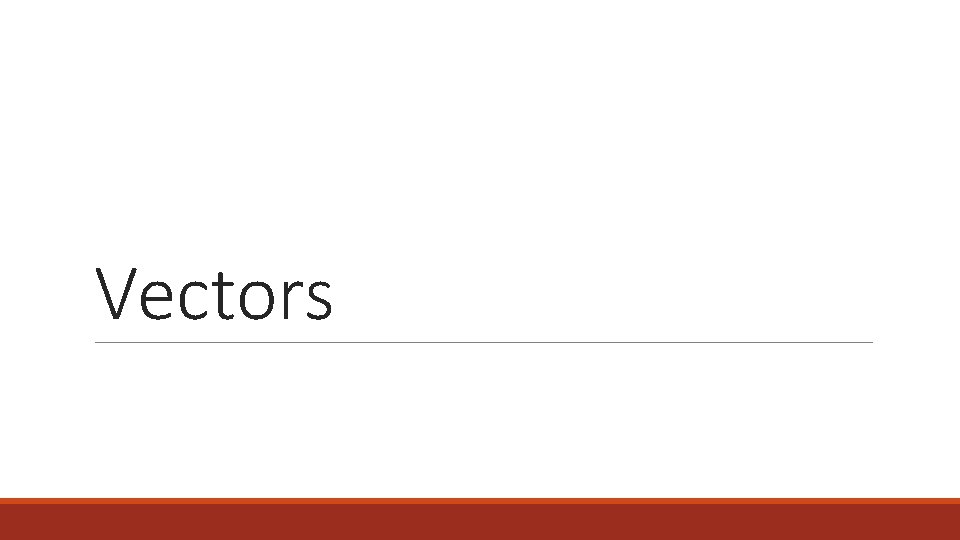
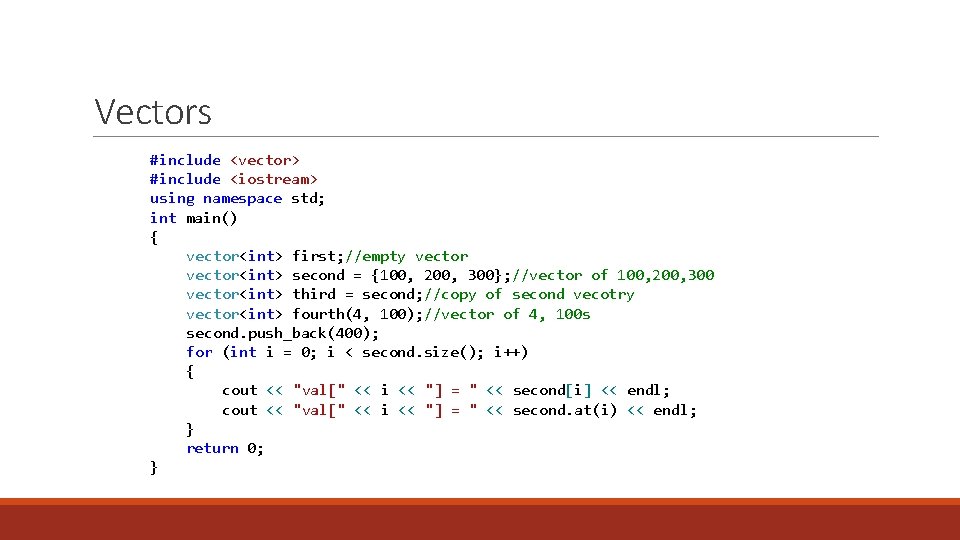
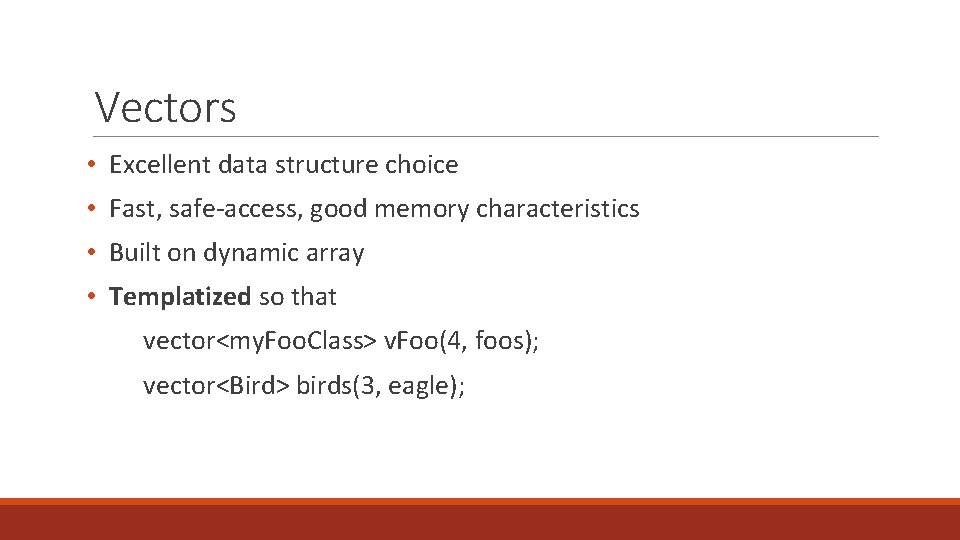
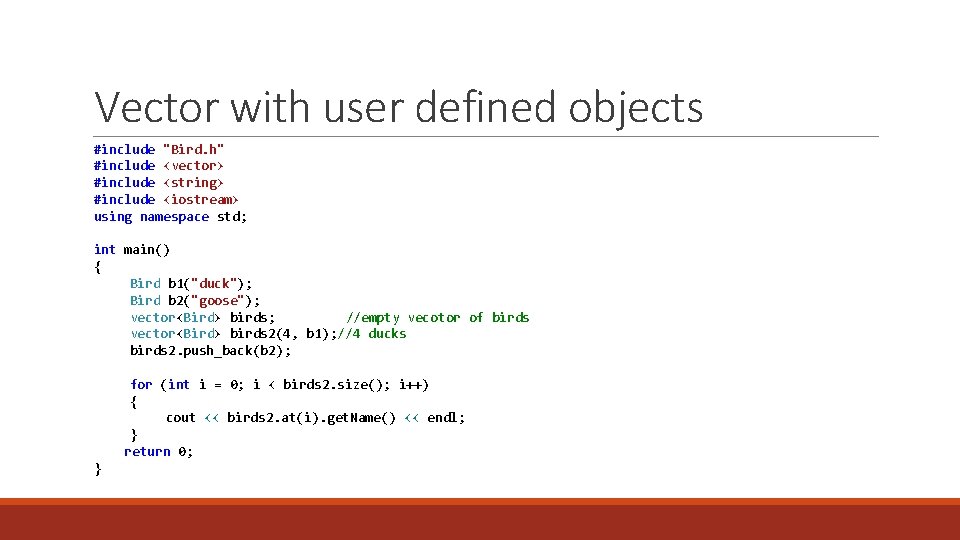
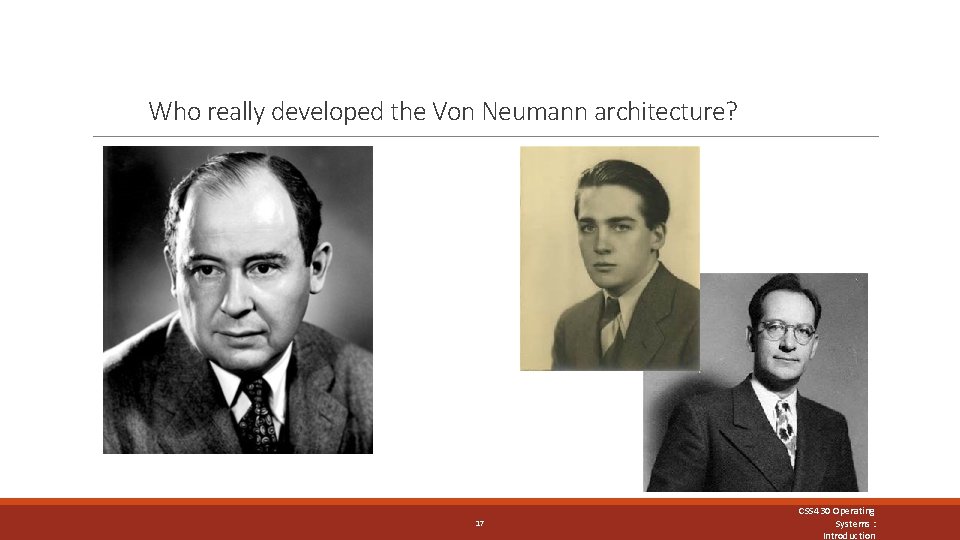
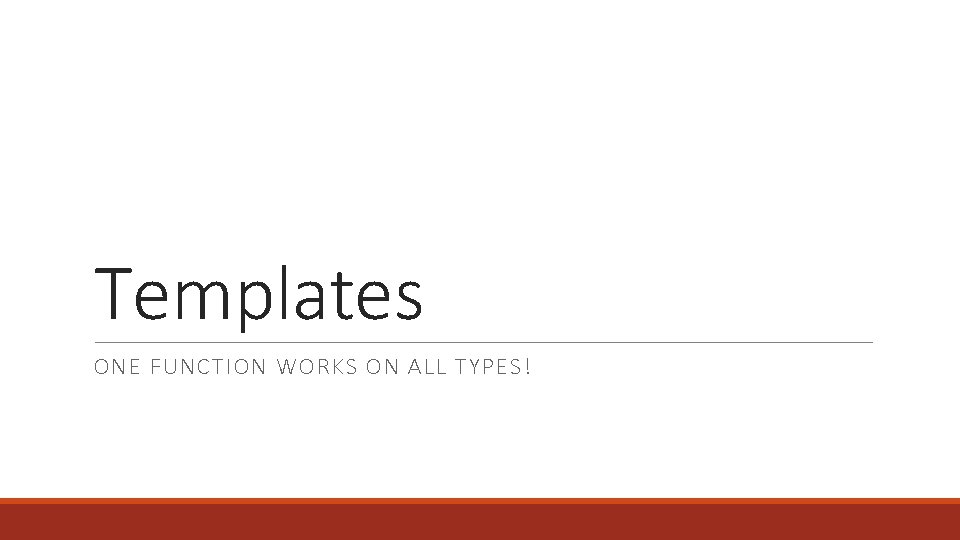
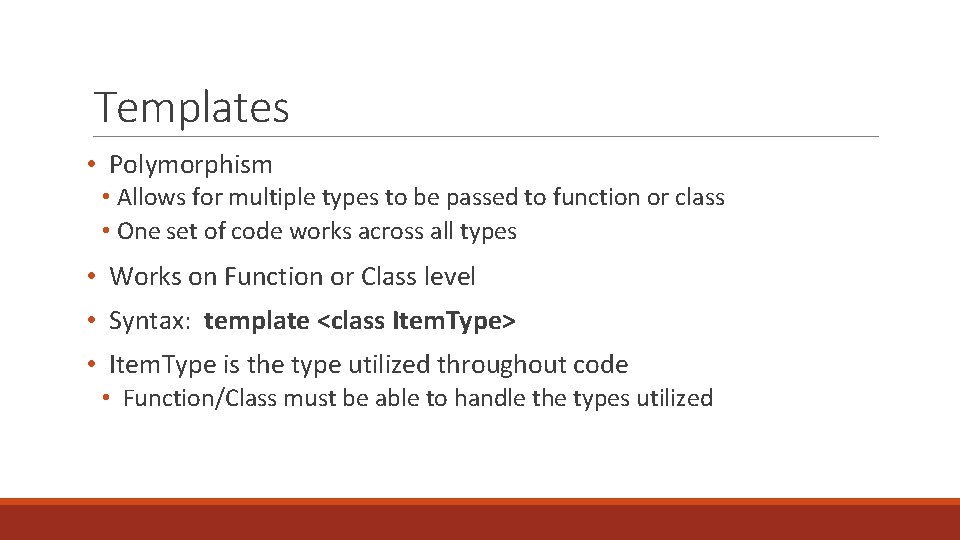
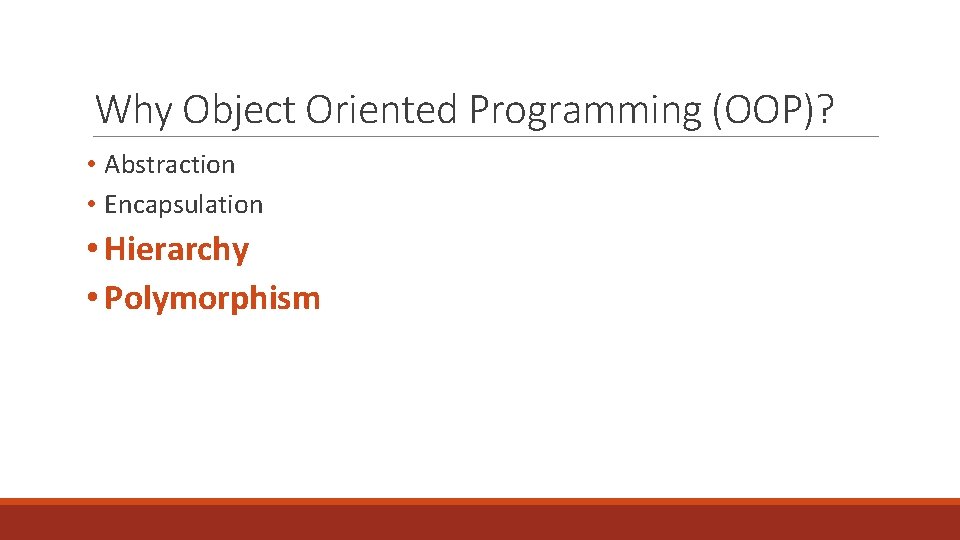
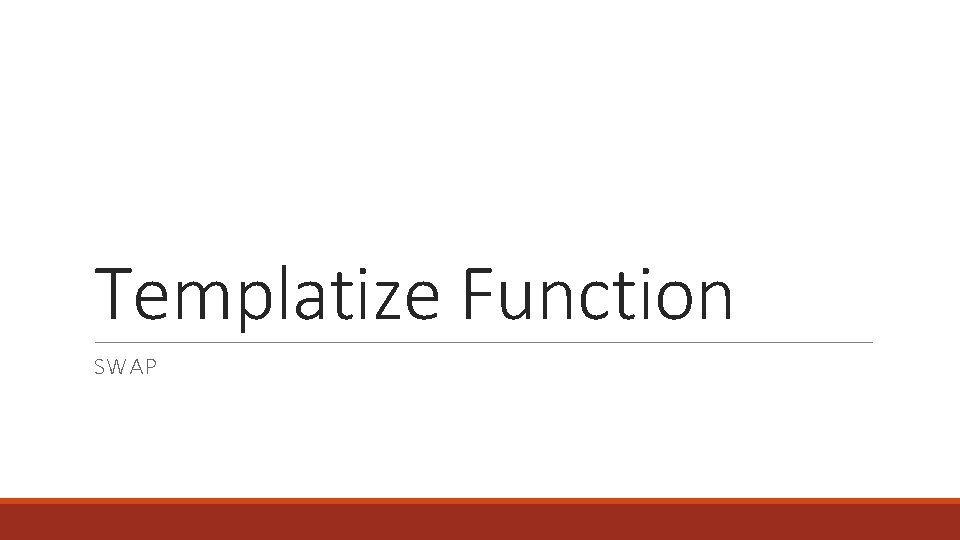
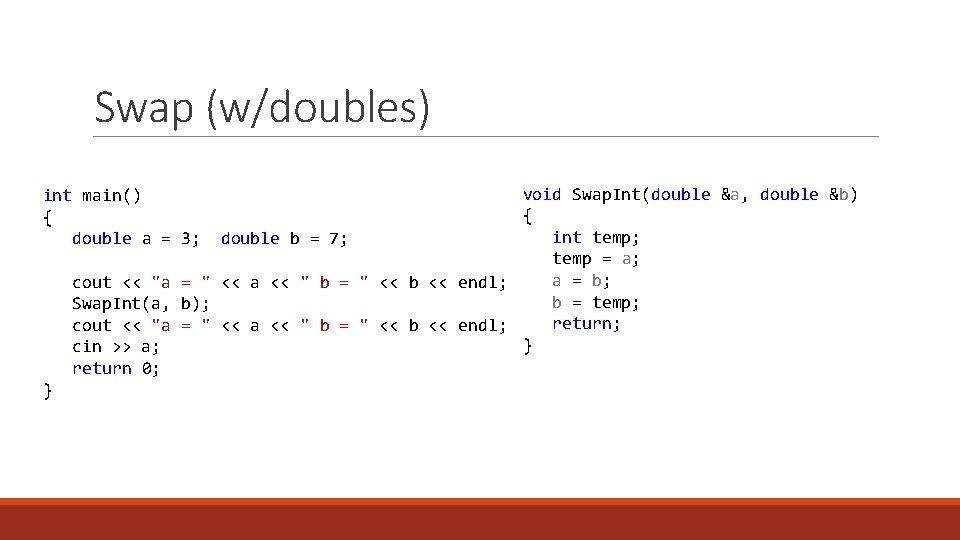
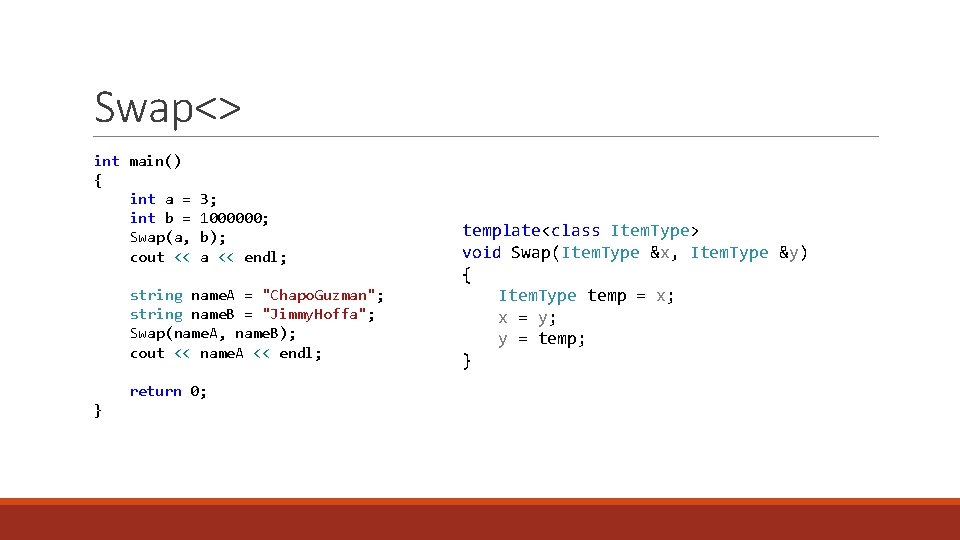
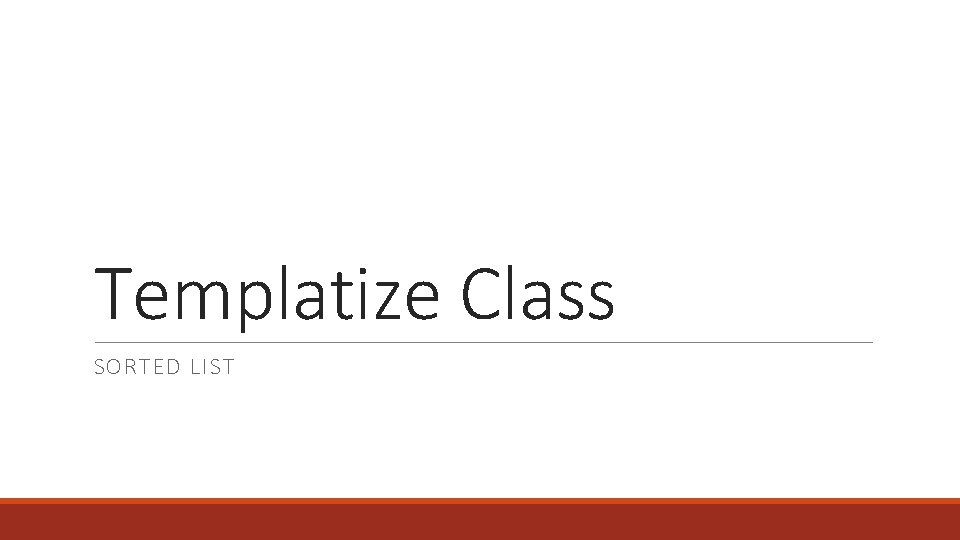
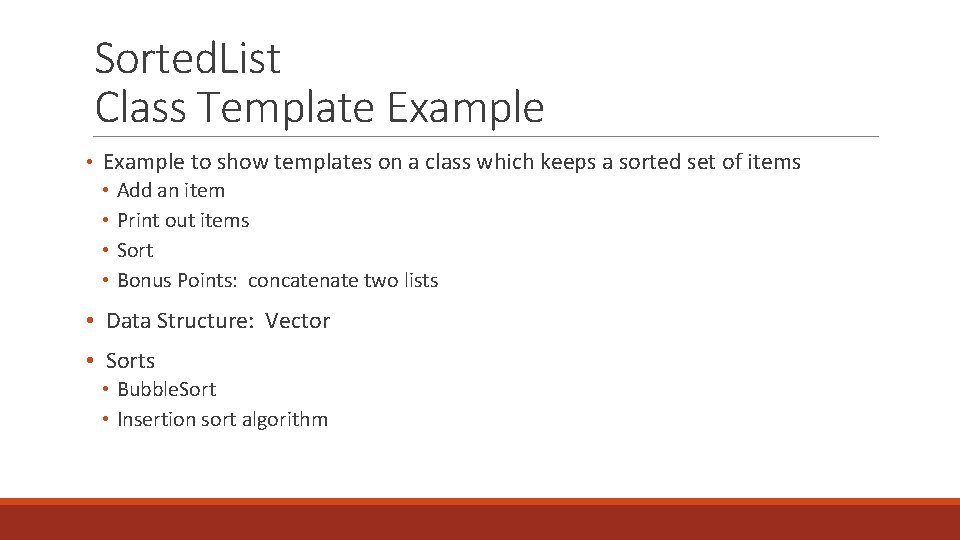
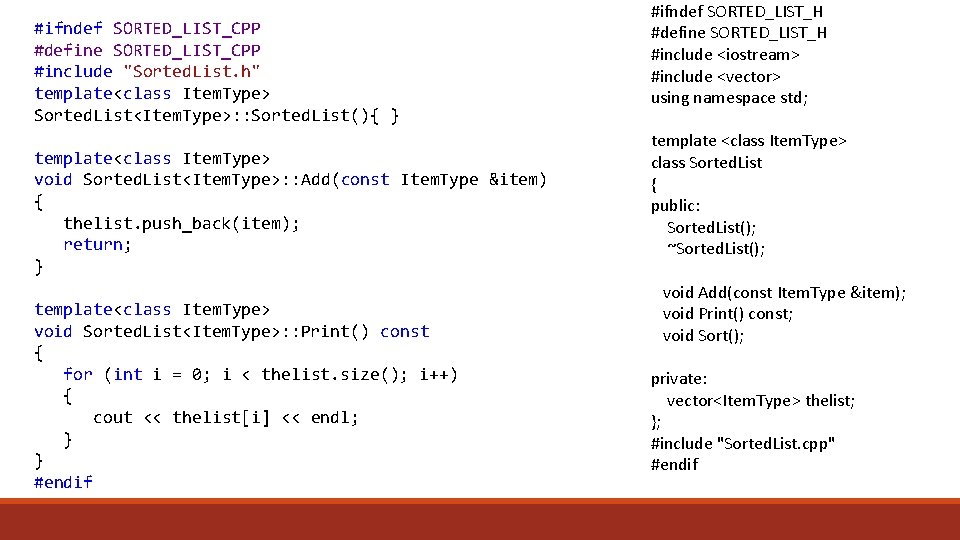
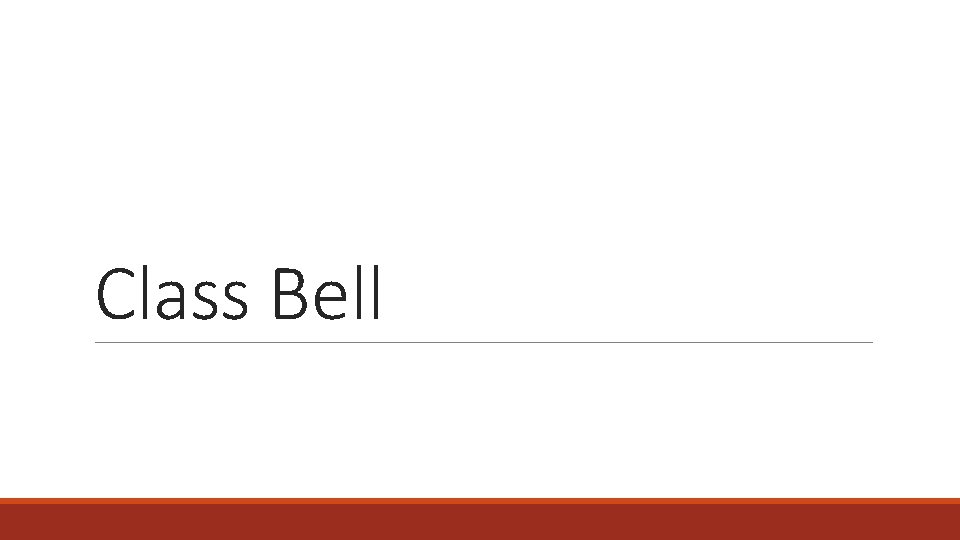
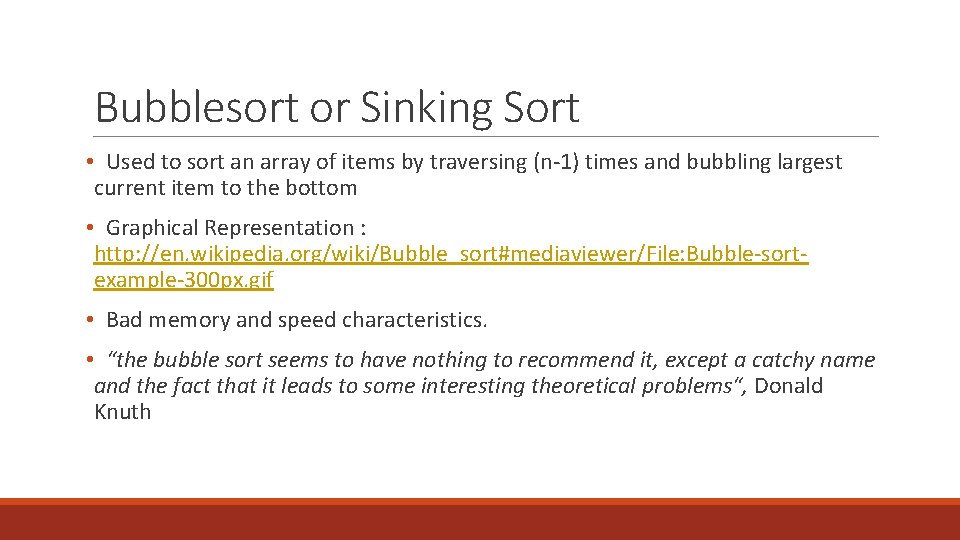
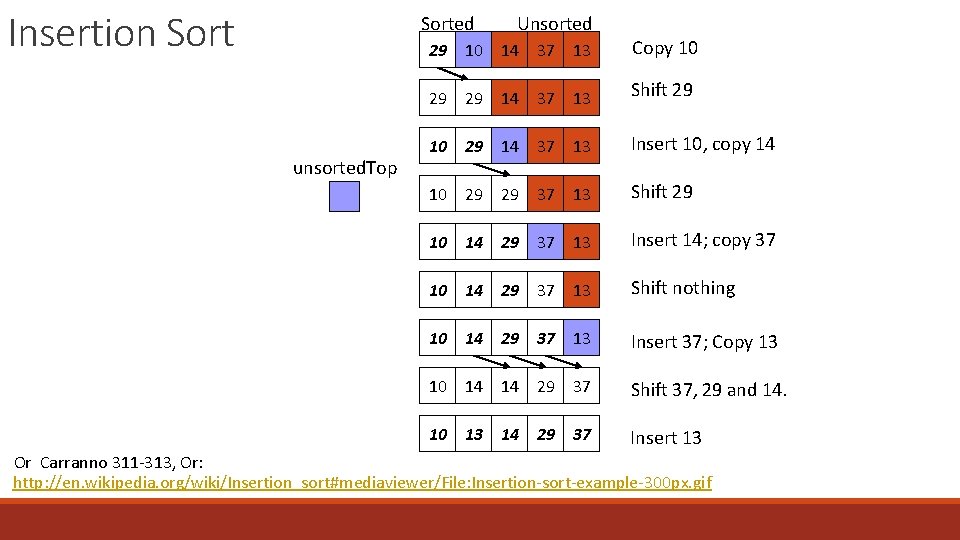
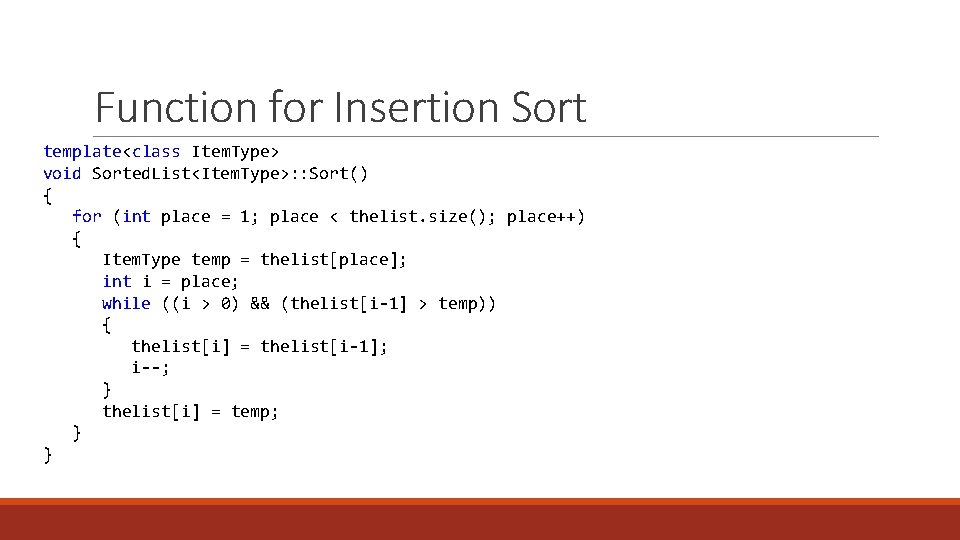
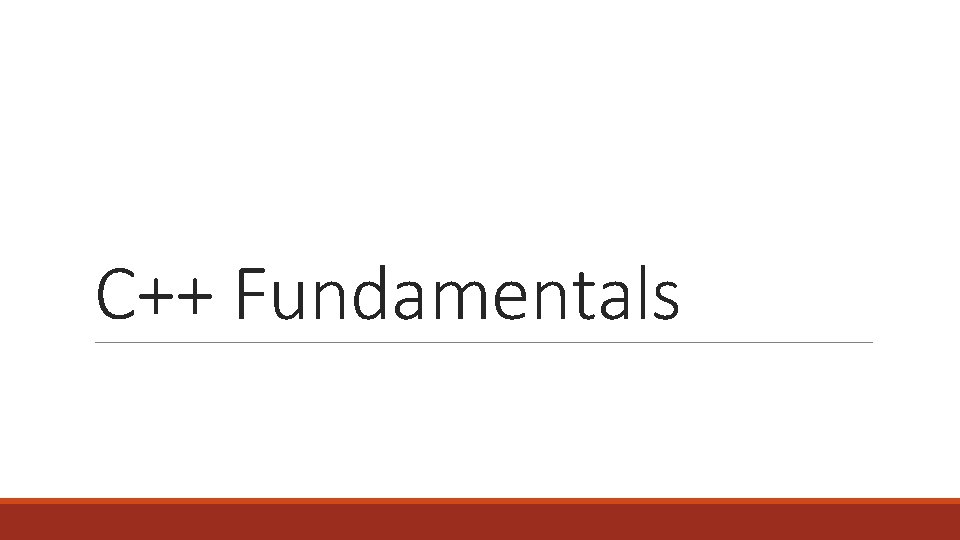
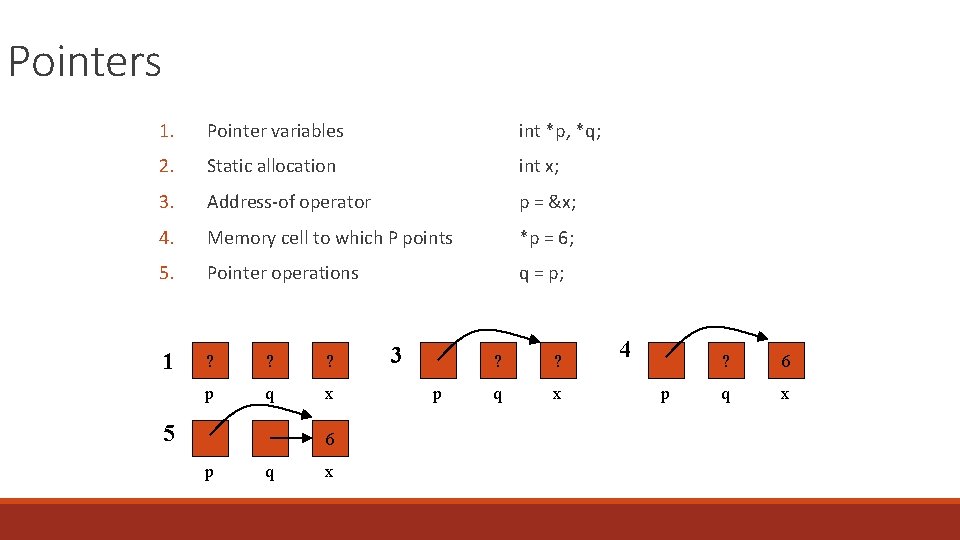
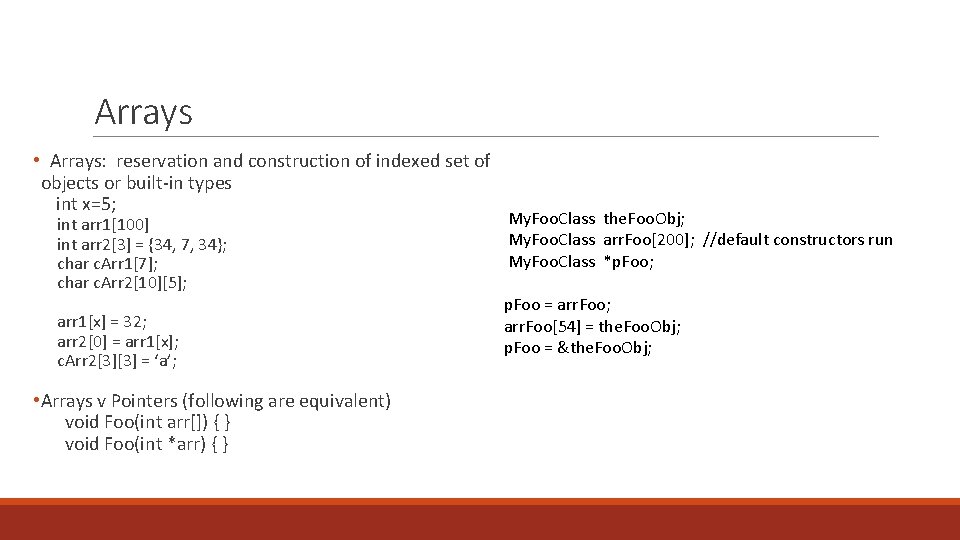
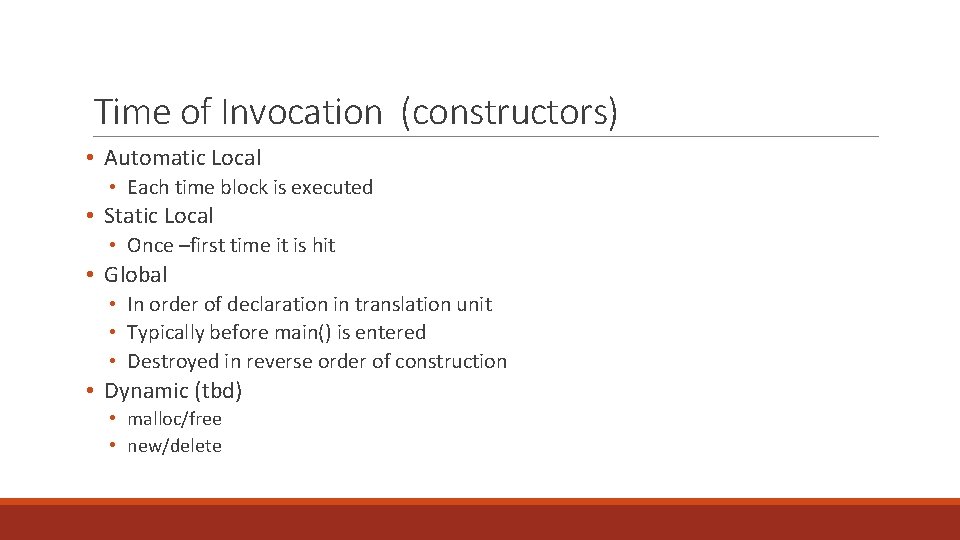
- Slides: 34
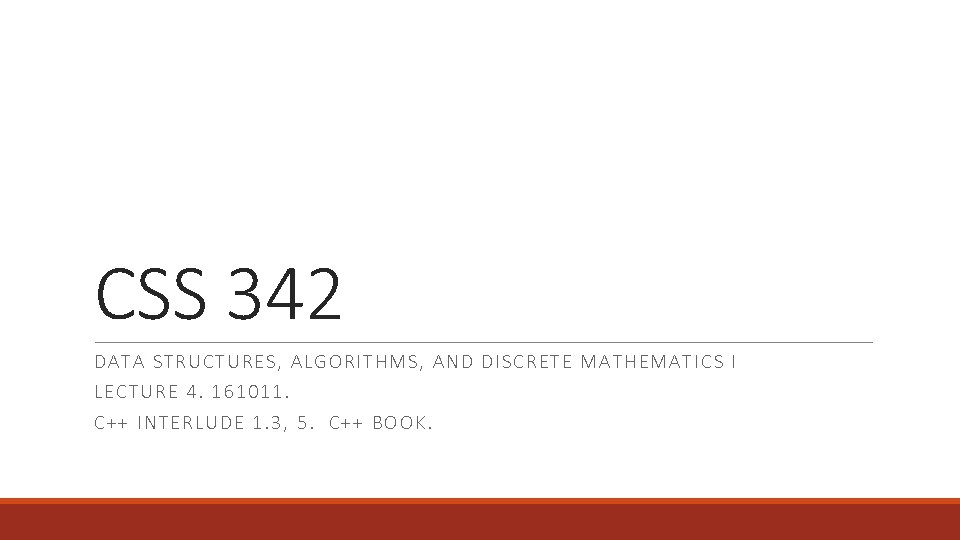
CSS 342 DATA S TR U CTURES, ALGO RITHMS , AND DISCRETE MATHEMATICS I LECTU RE 4. 161011. C++ INT ERLUDE 1. 3, 5. C+ + B OO K.
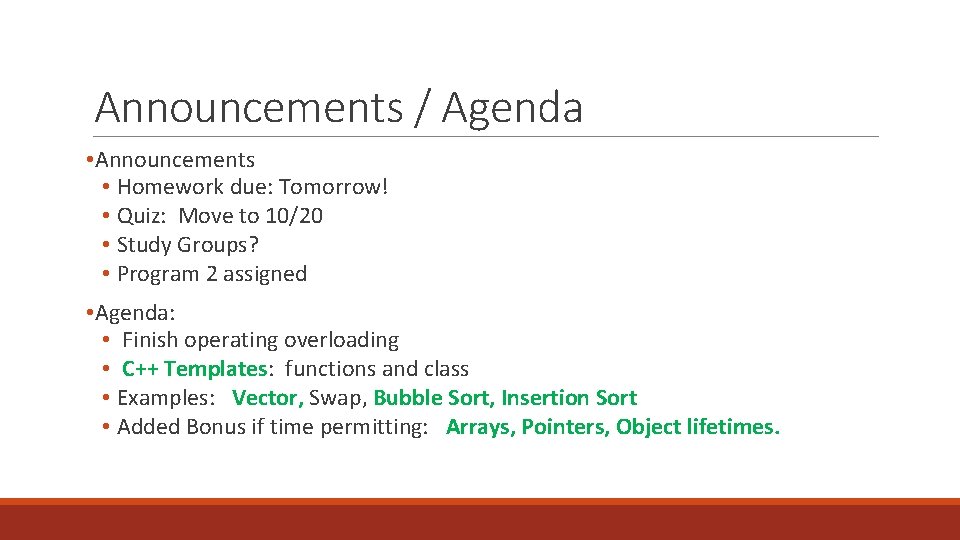
Announcements / Agenda • Announcements • Homework due: Tomorrow! • Quiz: Move to 10/20 • Study Groups? • Program 2 assigned • Agenda: • Finish operating overloading • C++ Templates: functions and class • Examples: Vector, Swap, Bubble Sort, Insertion Sort • Added Bonus if time permitting: Arrays, Pointers, Object lifetimes.
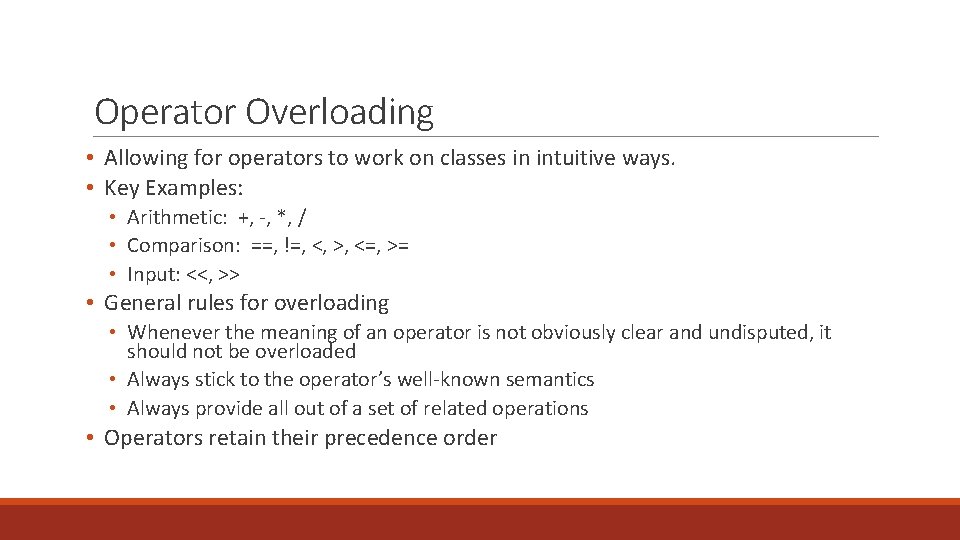
Operator Overloading • Allowing for operators to work on classes in intuitive ways. • Key Examples: • Arithmetic: +, -, *, / • Comparison: ==, !=, <, >, <=, >= • Input: <<, >> • General rules for overloading • Whenever the meaning of an operator is not obviously clear and undisputed, it should not be overloaded • Always stick to the operator’s well-known semantics • Always provide all out of a set of related operations • Operators retain their precedence order
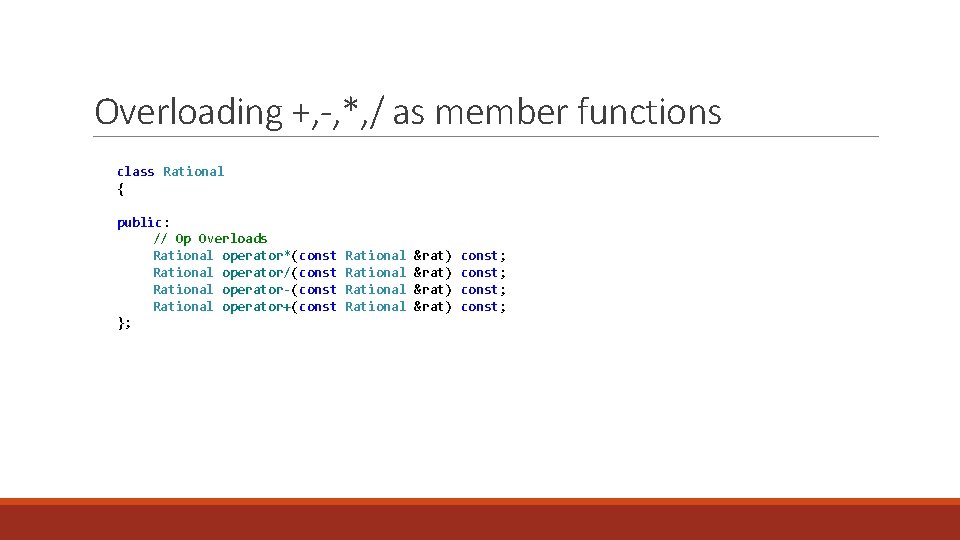
Overloading +, -, *, / as member functions class Rational { public: // Op Overloads Rational operator*(const Rational operator/(const Rational operator-(const Rational operator+(const }; Rational &rat) const;
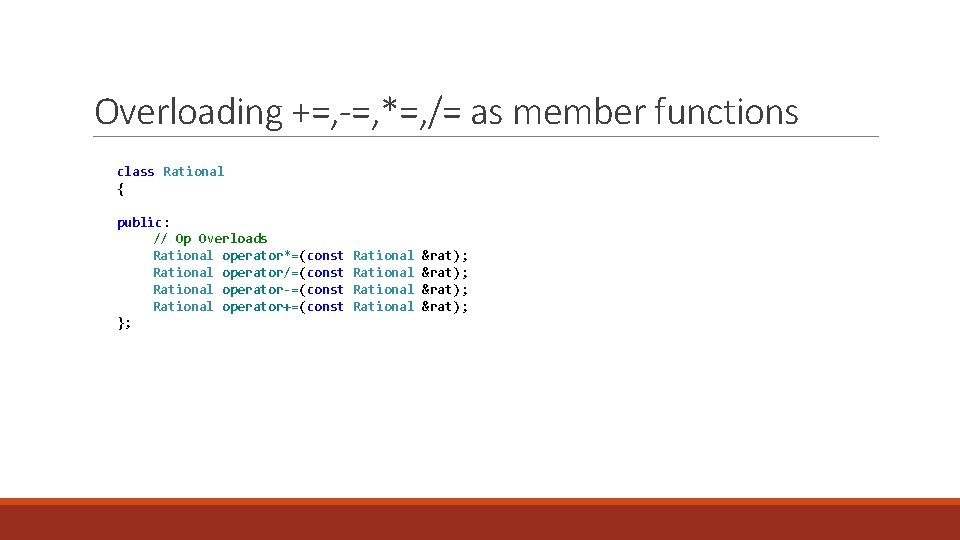
Overloading +=, -=, *=, /= as member functions class Rational { public: // Op Overloads Rational operator*=(const Rational operator/=(const Rational operator-=(const Rational operator+=(const }; Rational &rat);
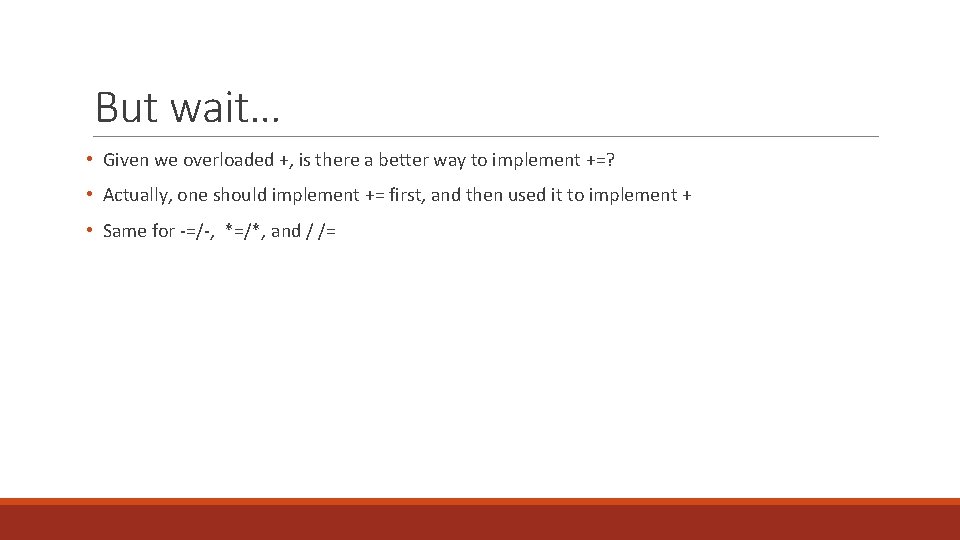
But wait… • Given we overloaded +, is there a better way to implement +=? • Actually, one should implement += first, and then used it to implement + • Same for -=/-, *=/*, and / /=
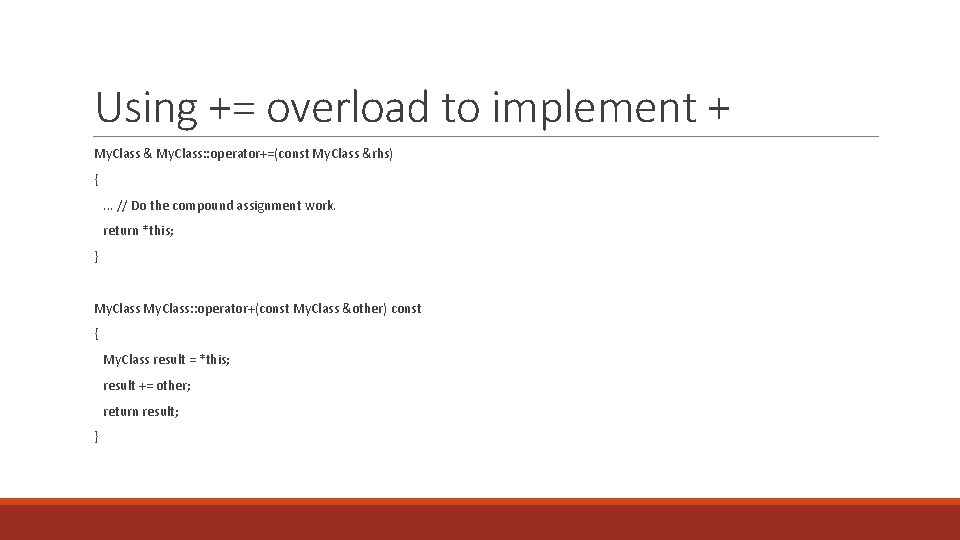
Using += overload to implement + My. Class & My. Class: : operator+=(const My. Class &rhs) {. . . // Do the compound assignment work. return *this; } My. Class: : operator+(const My. Class &other) const { My. Class result = *this; result += other; return result; }
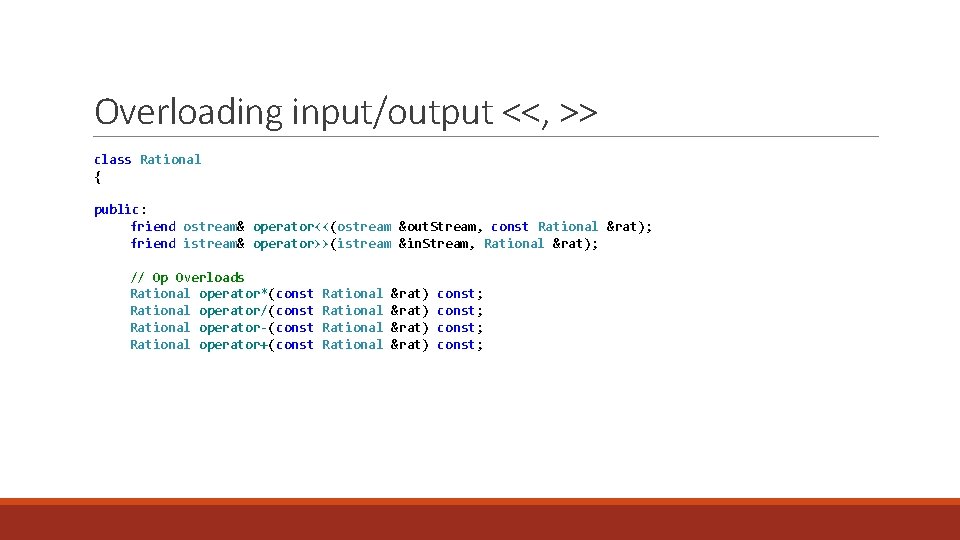
Overloading input/output <<, >> class Rational { public: friend ostream& operator<<(ostream &out. Stream, const Rational &rat); friend istream& operator>>(istream &in. Stream, Rational &rat); // Op Overloads Rational operator*(const Rational operator/(const Rational operator-(const Rational operator+(const Rational &rat) const;
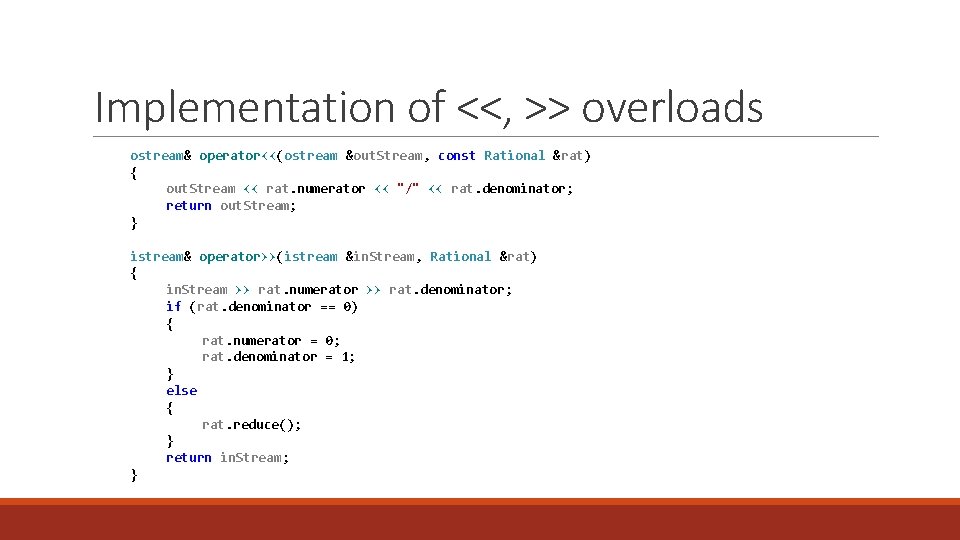
Implementation of <<, >> overloads ostream& operator<<(ostream &out. Stream, const Rational &rat) { out. Stream << rat. numerator << "/" << rat. denominator; return out. Stream; } istream& operator>>(istream &in. Stream, Rational &rat) { in. Stream >> rat. numerator >> rat. denominator; if (rat. denominator == 0) { rat. numerator = 0; rat. denominator = 1; } else { rat. reduce(); } return in. Stream; }
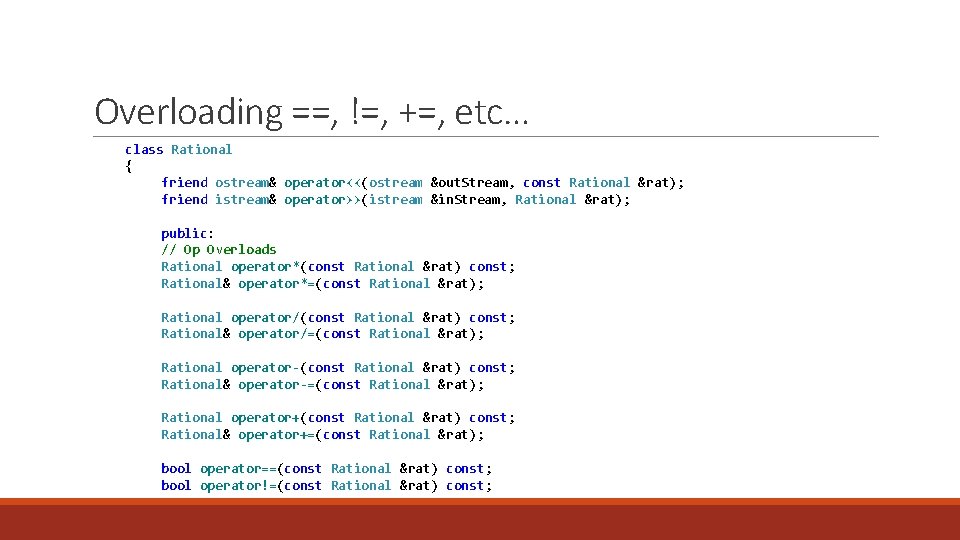
Overloading ==, !=, +=, etc… class Rational { friend ostream& operator<<(ostream &out. Stream, const Rational &rat); friend istream& operator>>(istream &in. Stream, Rational &rat); public: // Op Overloads Rational operator*(const Rational &rat) const; Rational& operator*=(const Rational &rat); Rational operator/(const Rational &rat) const; Rational& operator/=(const Rational &rat); Rational operator-(const Rational &rat) const; Rational& operator-=(const Rational &rat); Rational operator+(const Rational &rat) const; Rational& operator+=(const Rational &rat); bool operator==(const Rational &rat) const; bool operator!=(const Rational &rat) const;
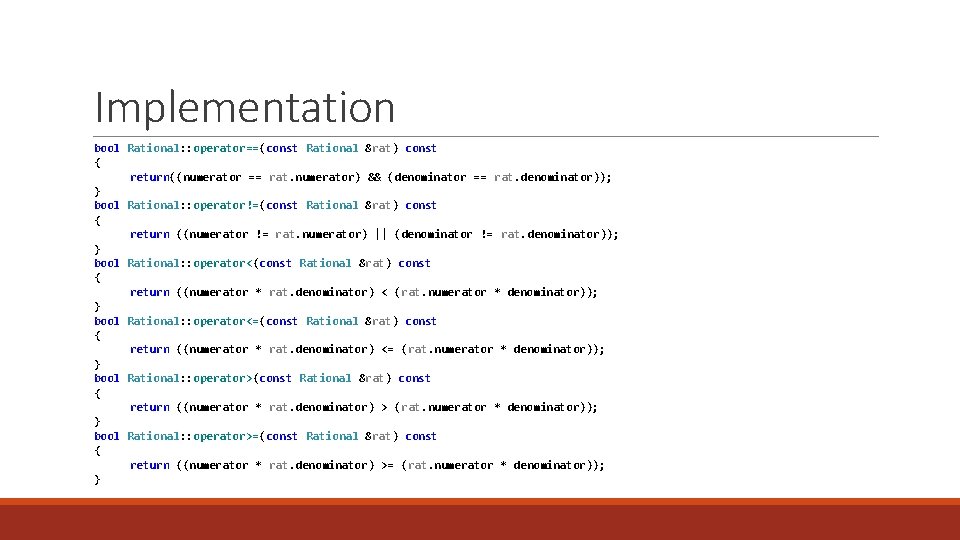
Implementation bool Rational: : operator==(const Rational &rat) const { return((numerator == rat. numerator) && (denominator == rat. denominator)); } bool Rational: : operator!=(const Rational &rat) const { return ((numerator != rat. numerator) || (denominator != rat. denominator)); } bool Rational: : operator<(const Rational &rat) const { return ((numerator * rat. denominator) < (rat. numerator * denominator)); } bool Rational: : operator<=(const Rational &rat) const { return ((numerator * rat. denominator) <= (rat. numerator * denominator)); } bool Rational: : operator>(const Rational &rat) const { return ((numerator * rat. denominator) > (rat. numerator * denominator)); } bool Rational: : operator>=(const Rational &rat) const { return ((numerator * rat. denominator) >= (rat. numerator * denominator)); }
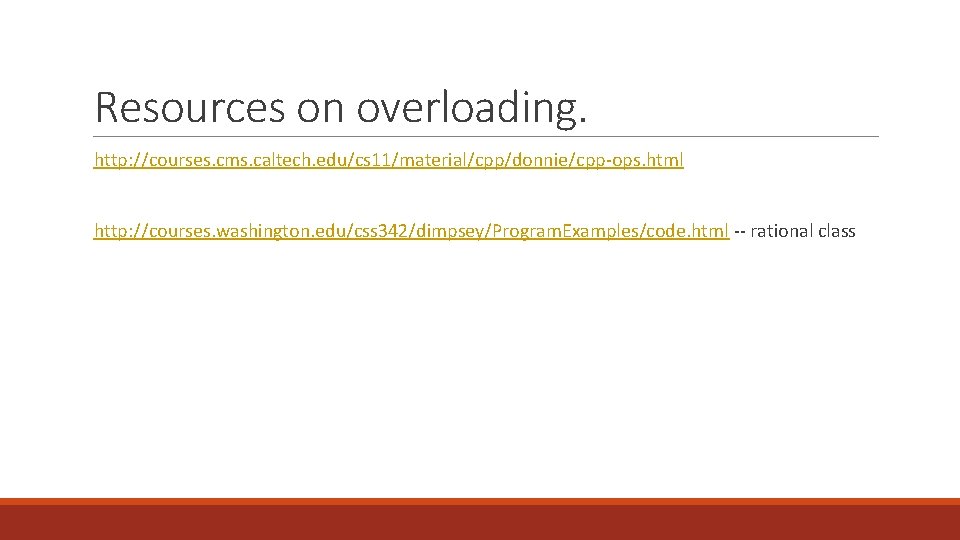
Resources on overloading. http: //courses. cms. caltech. edu/cs 11/material/cpp/donnie/cpp-ops. html http: //courses. washington. edu/css 342/dimpsey/Program. Examples/code. html -- rational class
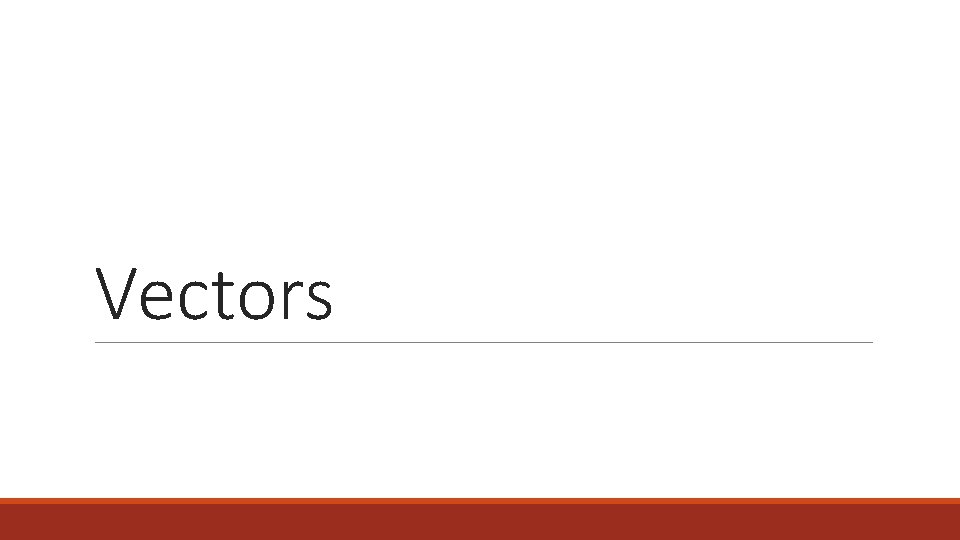
Vectors
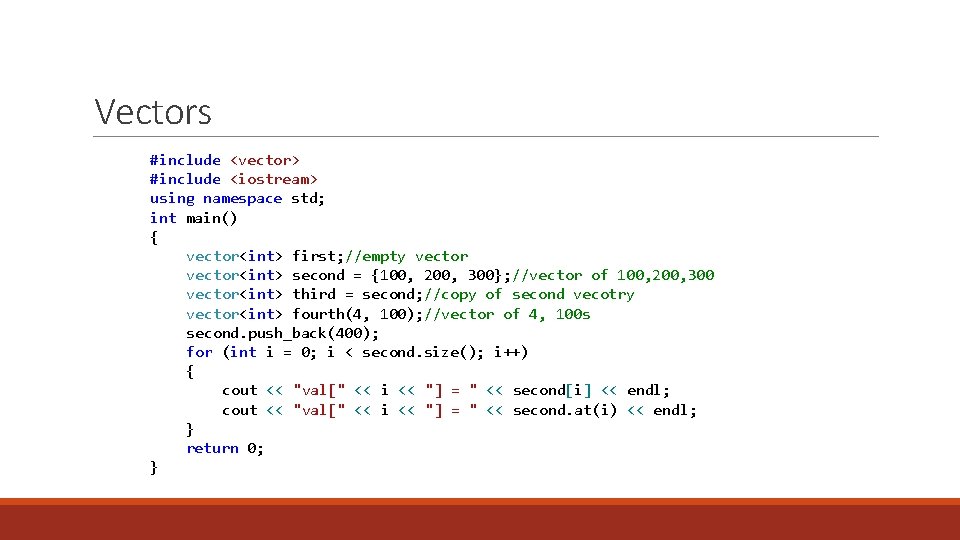
Vectors #include <vector> #include <iostream> using namespace std; int main() { vector<int> first; //empty vector<int> second = {100, 200, 300}; //vector of 100, 200, 300 vector<int> third = second; //copy of second vecotry vector<int> fourth(4, 100); //vector of 4, 100 s second. push_back(400); for (int i = 0; i < second. size(); i++) { cout << "val[" << i << "] = " << second[i] << endl; cout << "val[" << i << "] = " << second. at(i) << endl; } return 0; }
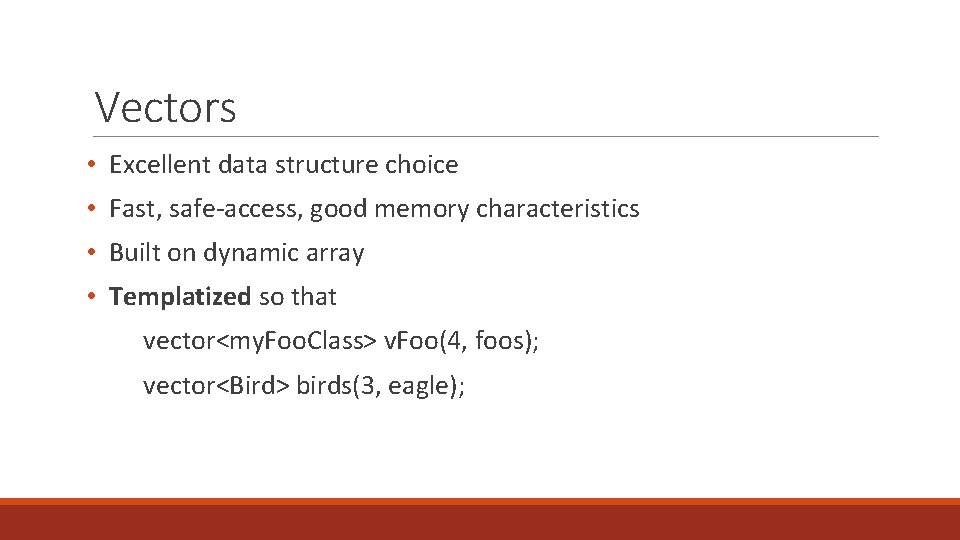
Vectors • Excellent data structure choice • Fast, safe-access, good memory characteristics • Built on dynamic array • Templatized so that vector<my. Foo. Class> v. Foo(4, foos); vector<Bird> birds(3, eagle);
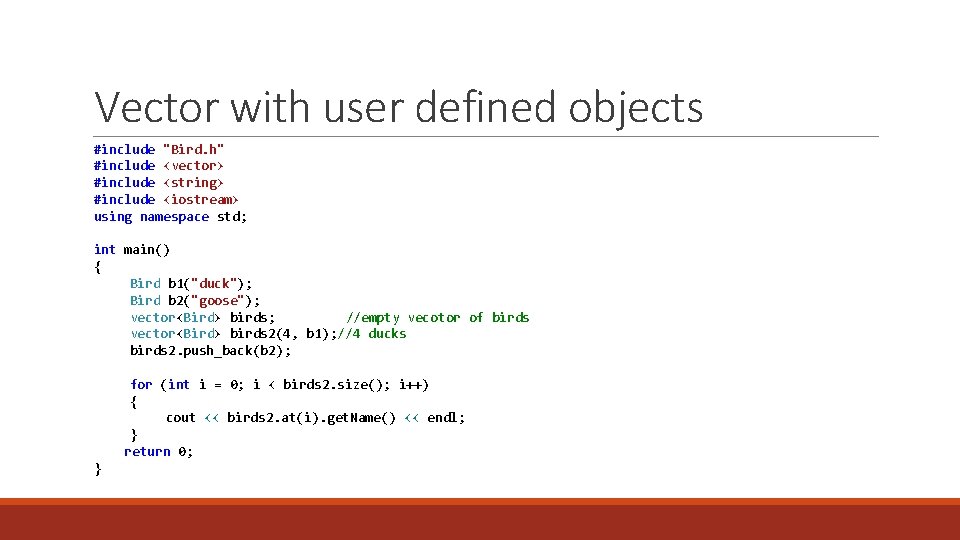
Vector with user defined objects #include "Bird. h" #include <vector> #include <string> #include <iostream> using namespace std; int main() { Bird b 1("duck"); Bird b 2("goose"); vector<Bird> birds; //empty vecotor of birds vector<Bird> birds 2(4, b 1); //4 ducks birds 2. push_back(b 2); for (int i = 0; i < birds 2. size(); i++) { cout << birds 2. at(i). get. Name() << endl; } return 0; }
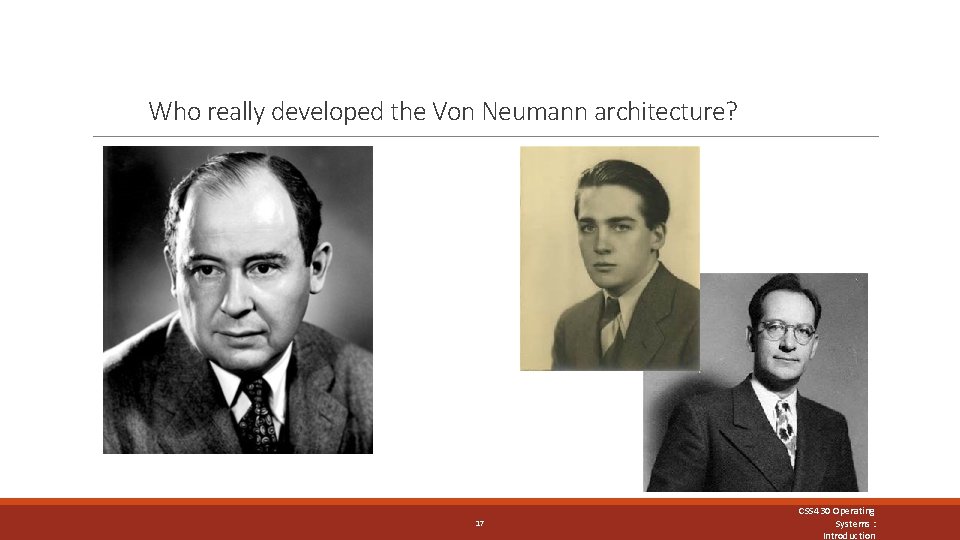
Who really developed the Von Neumann architecture? 17 CSS 430 Operating Systems : Introduction
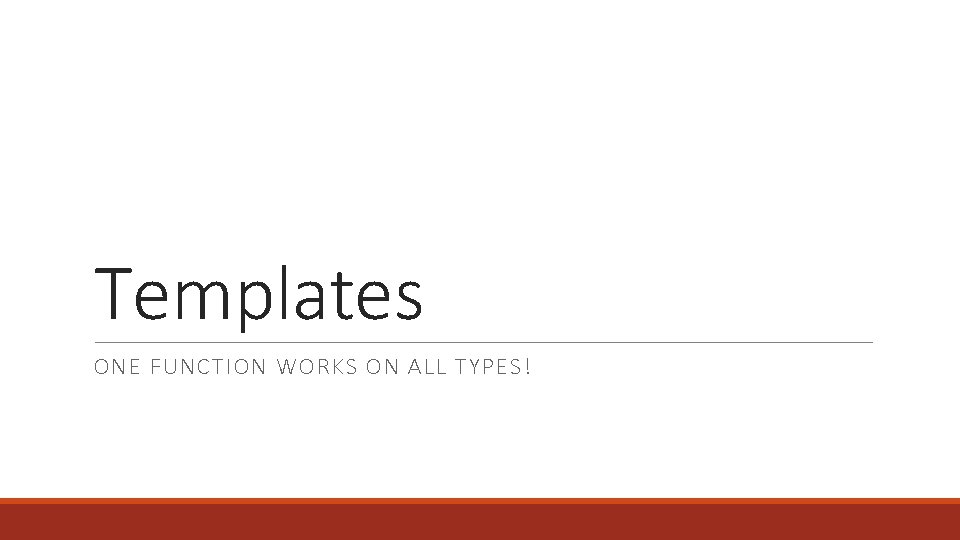
Templates ONE FUNCTION WORKS ON ALL TYPES!
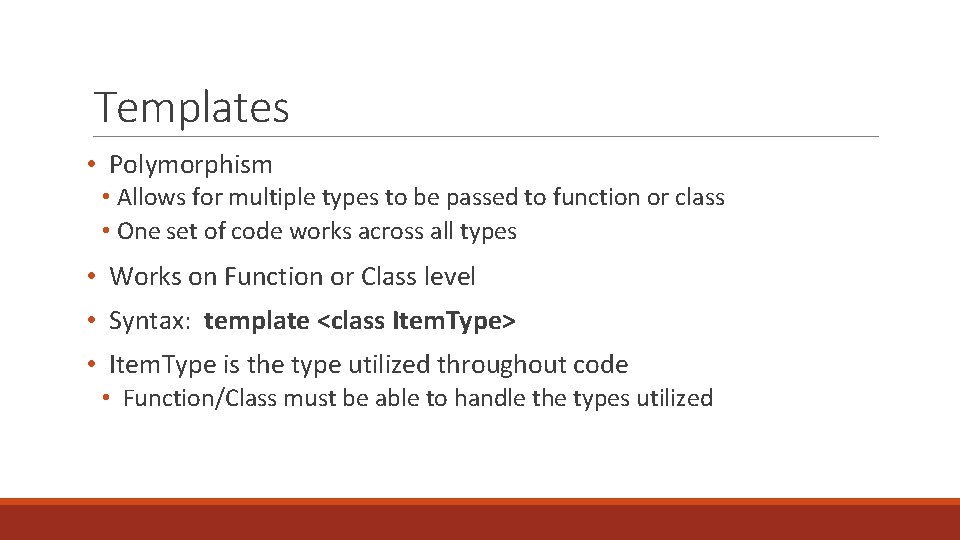
Templates • Polymorphism • Allows for multiple types to be passed to function or class • One set of code works across all types • Works on Function or Class level • Syntax: template <class Item. Type> • Item. Type is the type utilized throughout code • Function/Class must be able to handle the types utilized
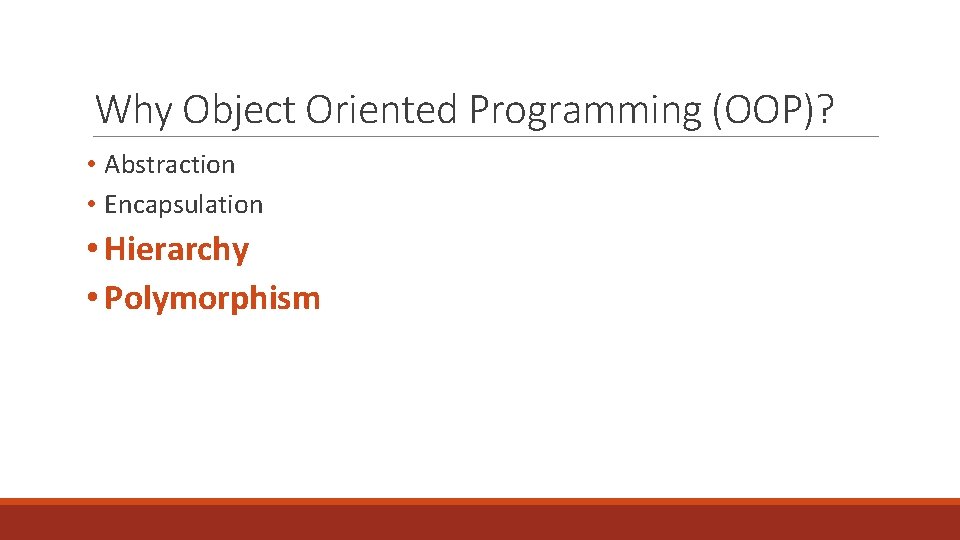
Why Object Oriented Programming (OOP)? • Abstraction • Encapsulation • Hierarchy • Polymorphism
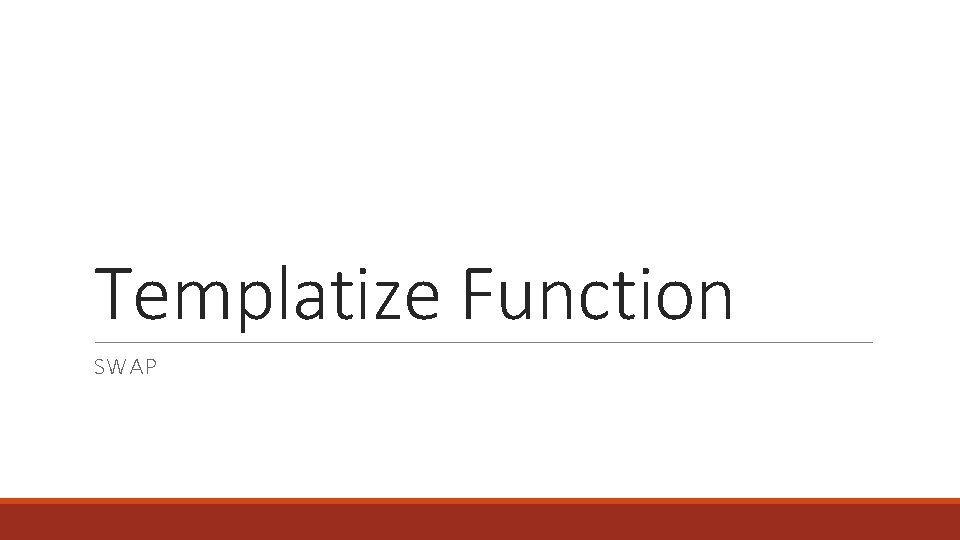
Templatize Function SWAP
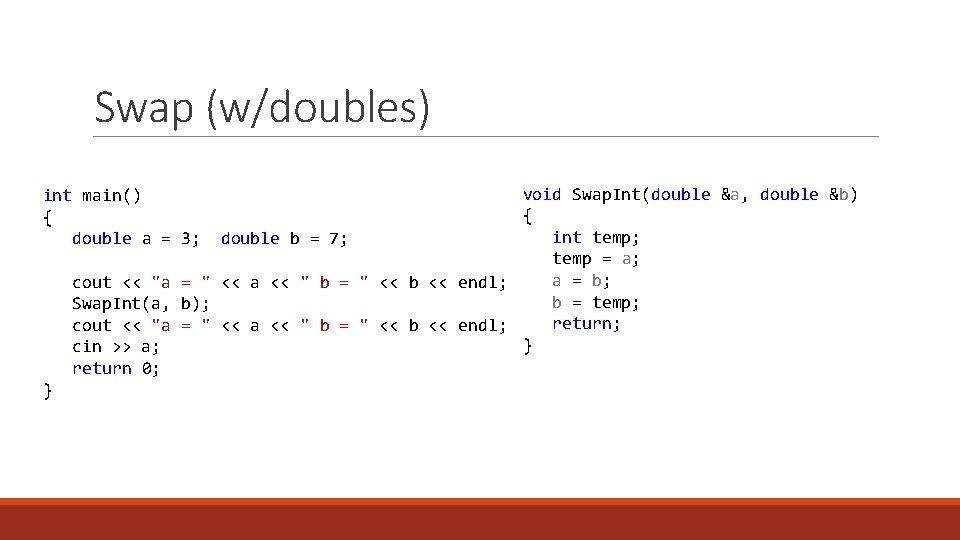
Swap (w/doubles) void Swap. Int(double &a, double &b) { int temp; double b = 7; temp = a; a = b; cout << "a = " << a << " b = " << b << endl; b = temp; Swap. Int(a, b); return; cout << "a = " << a << " b = " << b << endl; } cin >> a; return 0; int main() { double a = 3; }
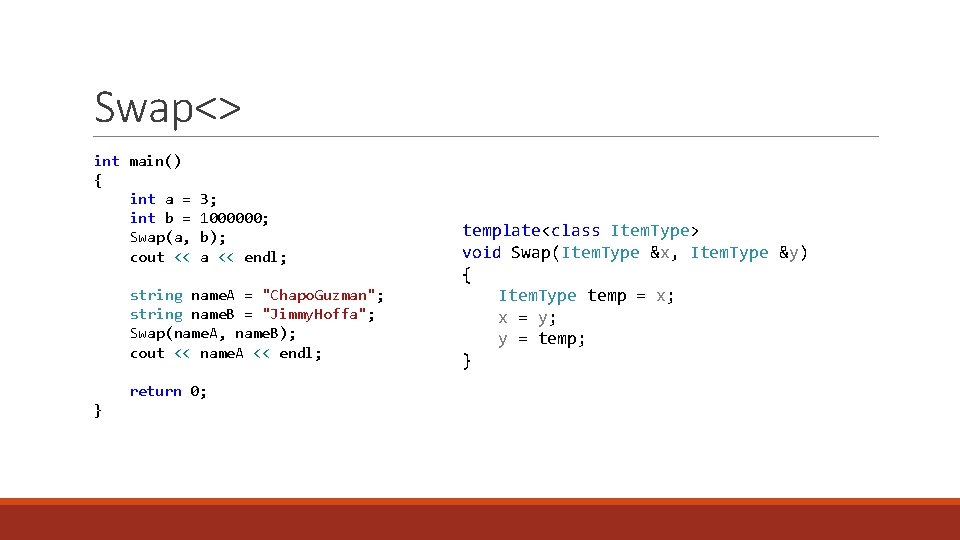
Swap<> int main() { int a = int b = Swap(a, cout << 3; 1000000; b); a << endl; string name. A = "Chapo. Guzman"; string name. B = "Jimmy. Hoffa"; Swap(name. A, name. B); cout << name. A << endl; return 0; } template<class Item. Type> void Swap(Item. Type &x, Item. Type &y) { Item. Type temp = x; x = y; y = temp; }
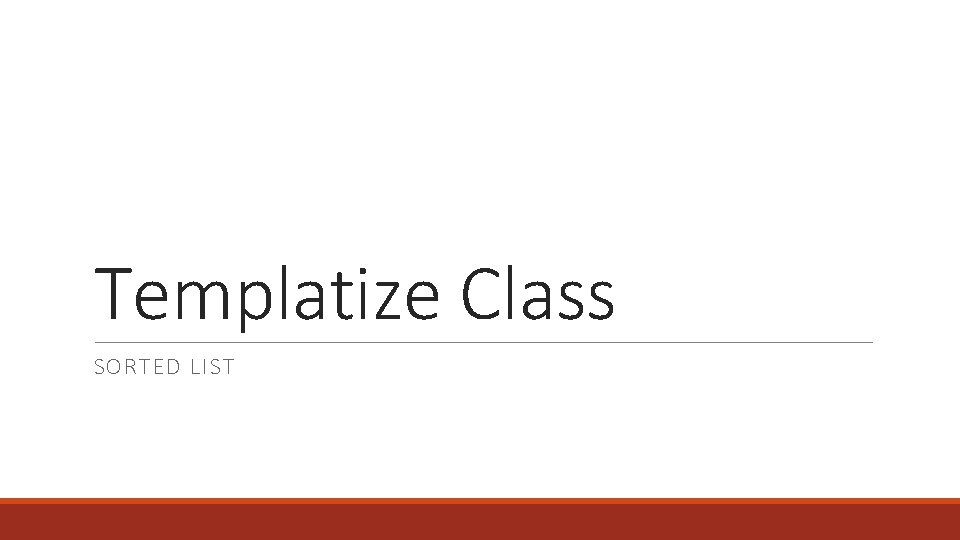
Templatize Class SORTED LIST
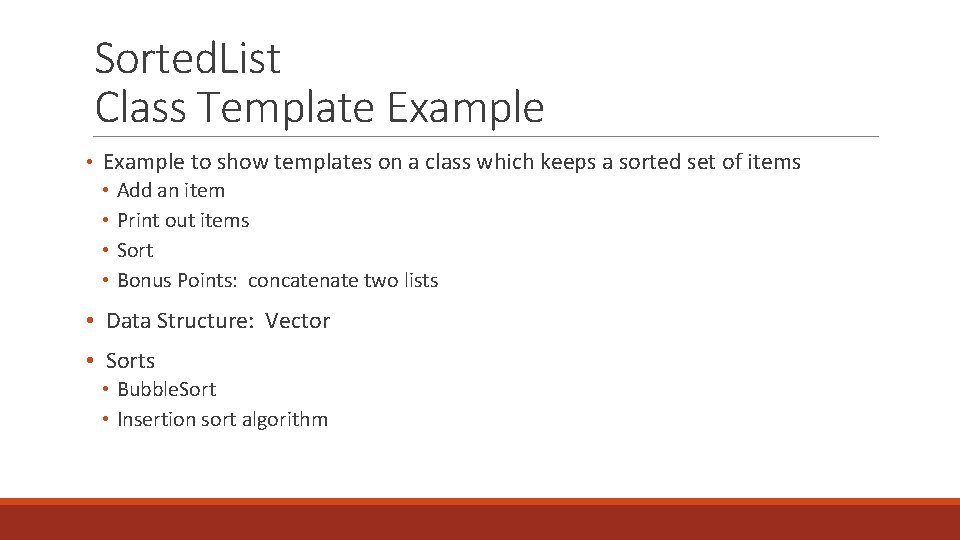
Sorted. List Class Template Example • Example to show templates on a class which keeps a sorted set of items • Add an item • Print out items • Sort • Bonus Points: concatenate two lists • Data Structure: Vector • Sorts • Bubble. Sort • Insertion sort algorithm
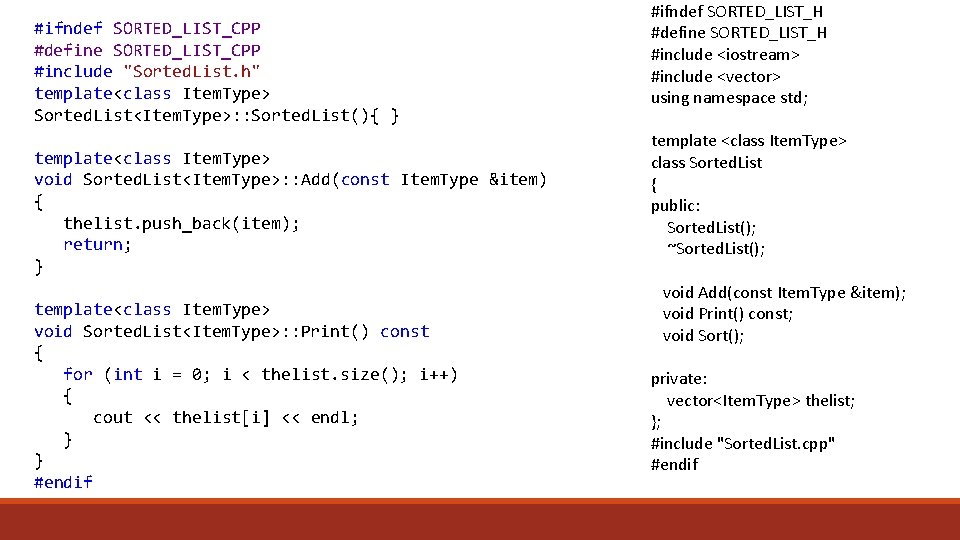
#ifndef SORTED_LIST_CPP #define SORTED_LIST_CPP #include "Sorted. List. h" template<class Item. Type> Sorted. List<Item. Type>: : Sorted. List(){ } template<class Item. Type> void Sorted. List<Item. Type>: : Add(const Item. Type &item) { thelist. push_back(item); return; } template<class Item. Type> void Sorted. List<Item. Type>: : Print() const { for (int i = 0; i < thelist. size(); i++) { cout << thelist[i] << endl; } } #endif #ifndef SORTED_LIST_H #define SORTED_LIST_H #include <iostream> #include <vector> using namespace std; template <class Item. Type> class Sorted. List { public: Sorted. List(); ~Sorted. List(); void Add(const Item. Type &item); void Print() const; void Sort(); private: vector<Item. Type> thelist; }; #include "Sorted. List. cpp" #endif
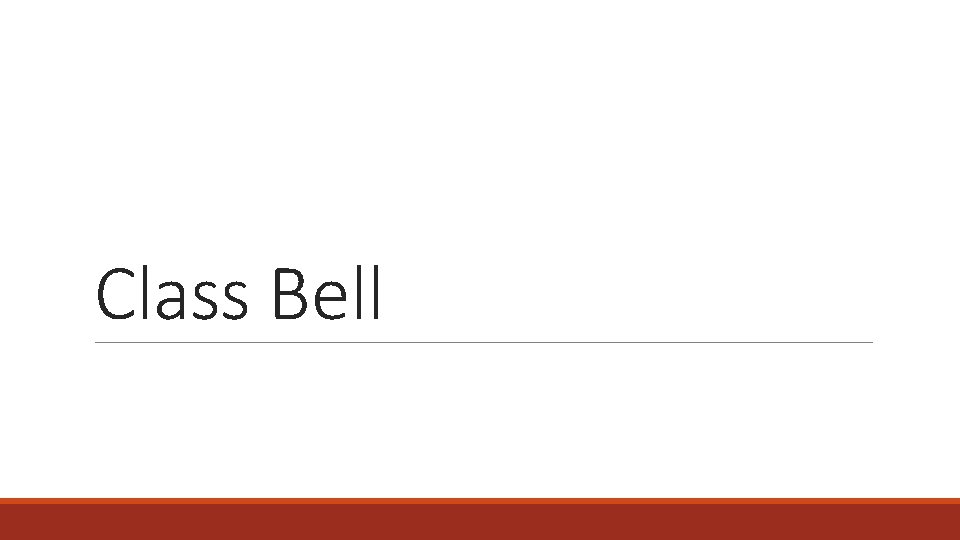
Class Bell
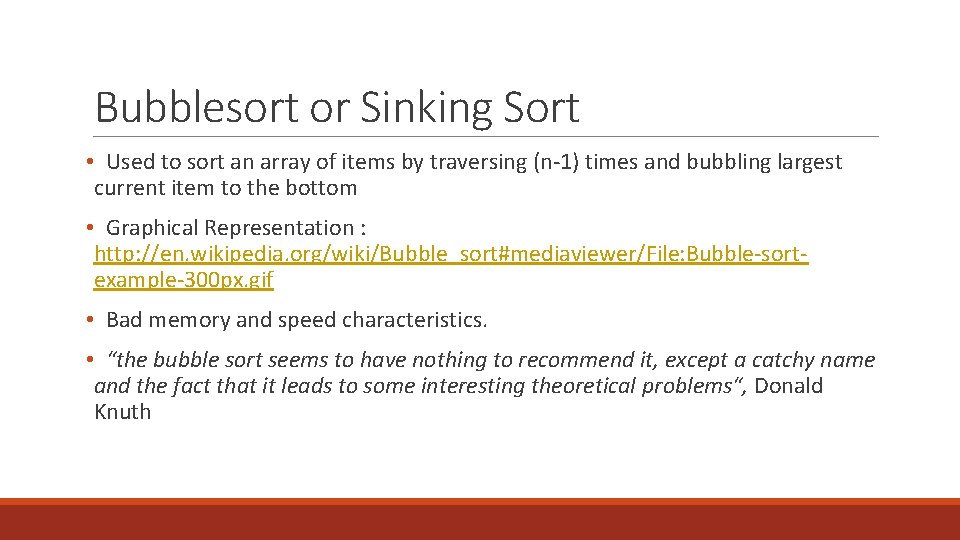
Bubblesort or Sinking Sort • Used to sort an array of items by traversing (n-1) times and bubbling largest current item to the bottom • Graphical Representation : http: //en. wikipedia. org/wiki/Bubble_sort#mediaviewer/File: Bubble-sortexample-300 px. gif • Bad memory and speed characteristics. • “the bubble sort seems to have nothing to recommend it, except a catchy name and the fact that it leads to some interesting theoretical problems“, Donald Knuth
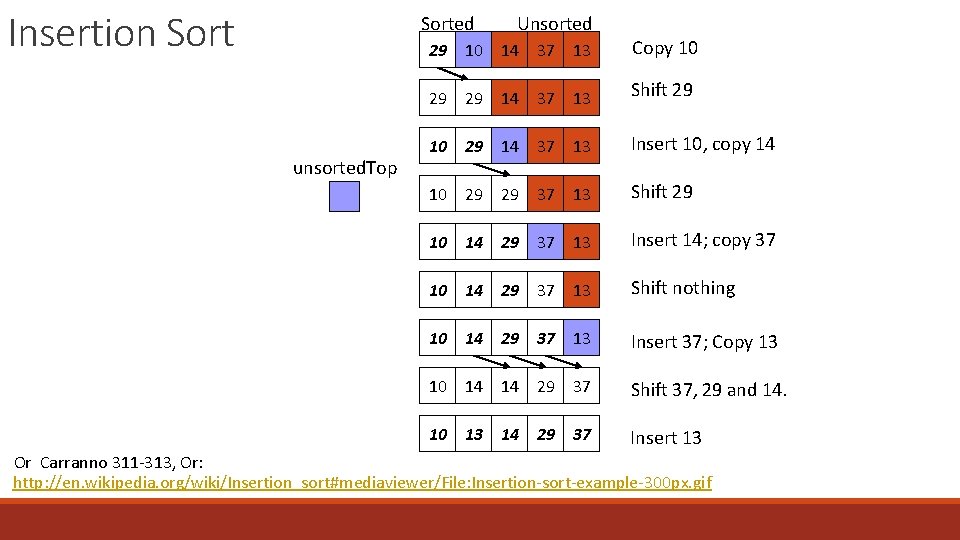
Insertion Sorted unsorted. Top Unsorted 29 10 14 37 13 Copy 10 29 29 14 37 13 Shift 29 10 29 14 37 13 Insert 10, copy 14 10 29 29 37 13 Shift 29 10 14 29 37 13 Insert 14; copy 37 10 14 29 37 13 Shift nothing 10 14 29 37 13 Insert 37; Copy 13 10 14 14 29 37 Shift 37, 29 and 14. 10 13 14 29 37 Insert 13 Or Carranno 311 -313, Or: http: //en. wikipedia. org/wiki/Insertion_sort#mediaviewer/File: Insertion-sort-example-300 px. gif
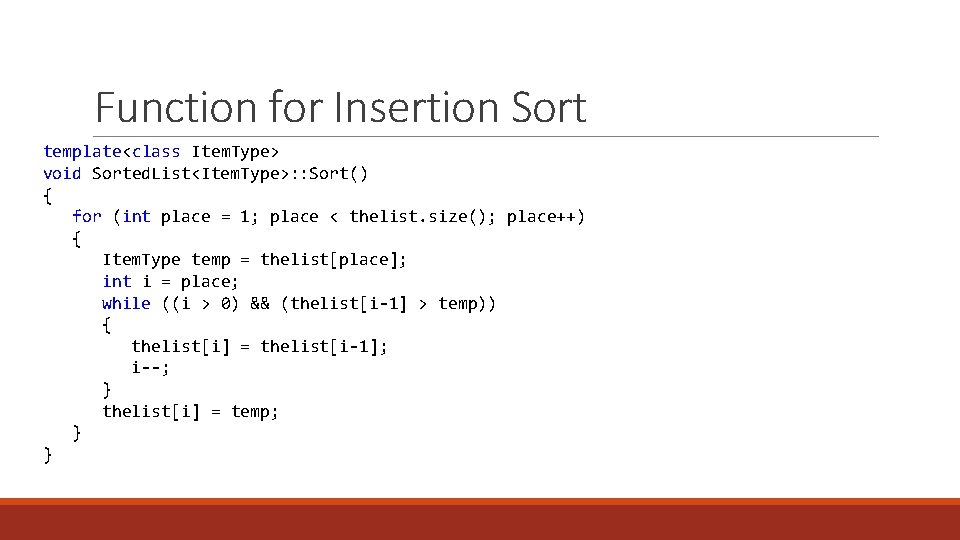
Function for Insertion Sort template<class Item. Type> void Sorted. List<Item. Type>: : Sort() { for (int place = 1; place < thelist. size(); place++) { Item. Type temp = thelist[place]; int i = place; while ((i > 0) && (thelist[i-1] > temp)) { thelist[i] = thelist[i-1]; i--; } thelist[i] = temp; } }
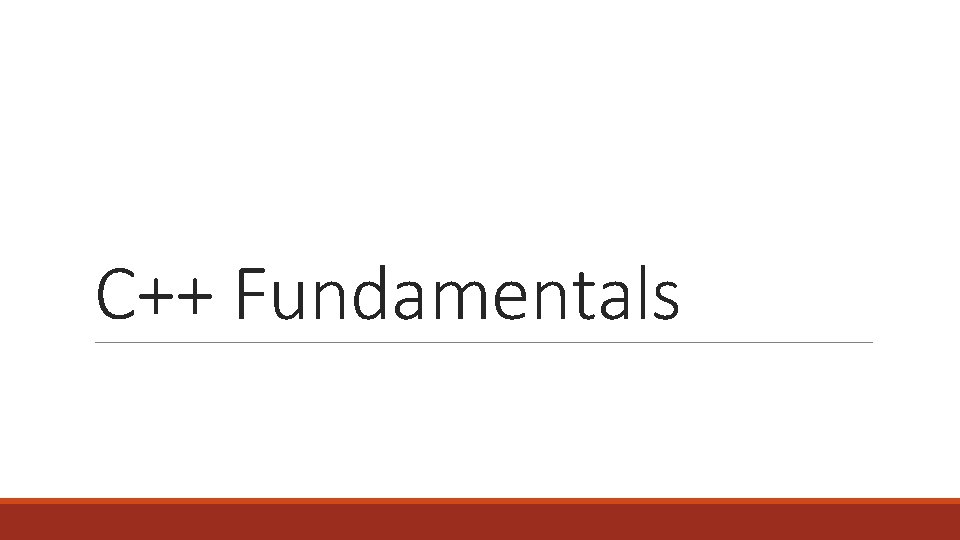
C++ Fundamentals
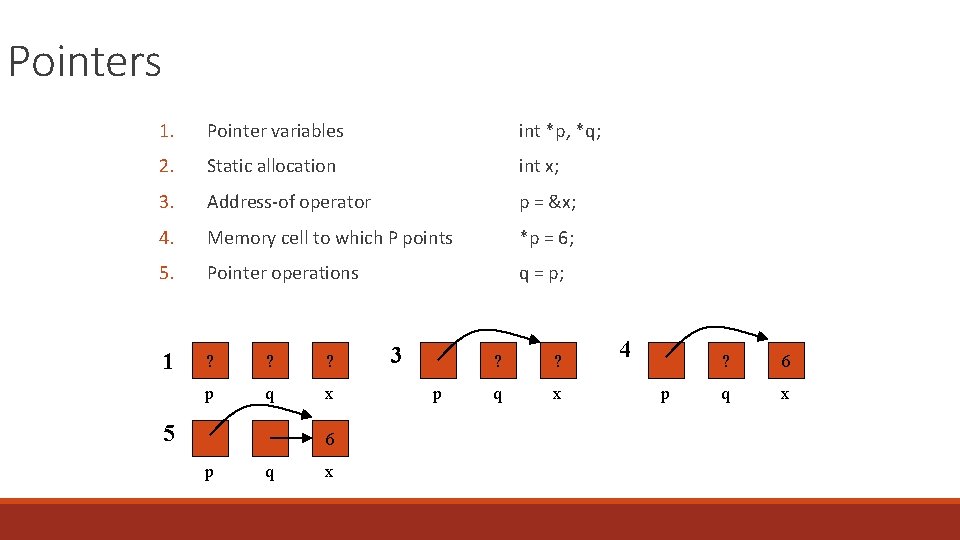
Pointers 1. Pointer variables int *p, *q; 2. Static allocation int x; 3. Address-of operator p = &x; 4. Memory cell to which P points *p = 6; 5. Pointer operations q = p; 1 ? ? ? p q x 5 6 p q x 3 p ? ? q x 4 p ? 6 q x
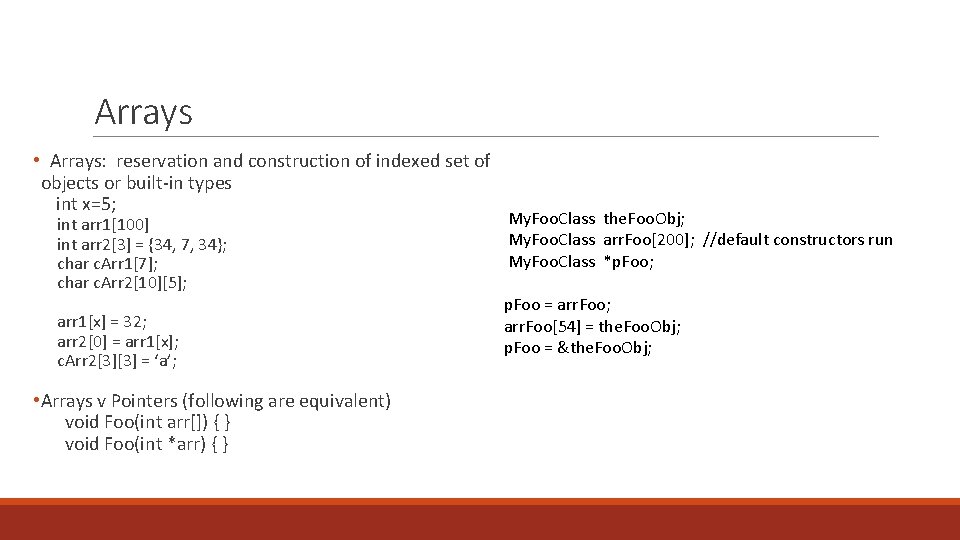
Arrays • Arrays: reservation and construction of indexed set of objects or built-in types int x=5; int arr 1[100] int arr 2[3] = {34, 7, 34}; char c. Arr 1[7]; char c. Arr 2[10][5]; arr 1[x] = 32; arr 2[0] = arr 1[x]; c. Arr 2[3][3] = ‘a’; • Arrays v Pointers (following are equivalent) void Foo(int arr[]) { } void Foo(int *arr) { } My. Foo. Class the. Foo. Obj; My. Foo. Class arr. Foo[200]; //default constructors run My. Foo. Class *p. Foo; p. Foo = arr. Foo; arr. Foo[54] = the. Foo. Obj; p. Foo = &the. Foo. Obj;
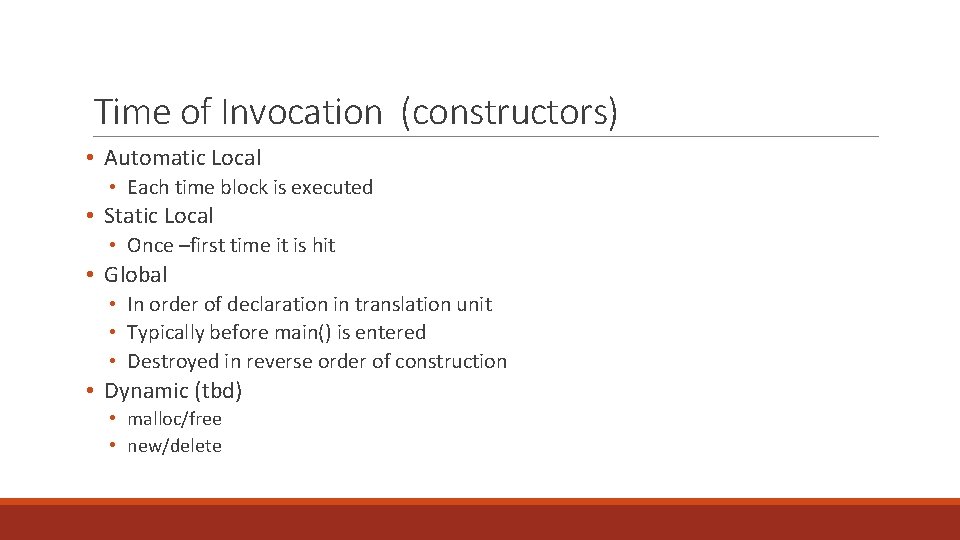
Time of Invocation (constructors) • Automatic Local • Each time block is executed • Static Local • Once –first time it is hit • Global • In order of declaration in translation unit • Typically before main() is entered • Destroyed in reverse order of construction • Dynamic (tbd) • malloc/free • new/delete